diff --git a/hived/conftest.py b/hived/conftest.py new file mode 100644 index 0000000000000000000000000000000000000000..337443a615131ee7fb7a4989001c778ea5134033 --- /dev/null +++ b/hived/conftest.py @@ -0,0 +1,7 @@ +from pytest import fixture + + +def pytest_addoption(parser): + parser.addoption( "--ref", action="store", type=str, help='specifies address of reference node') + parser.addoption( "--test", action="store", type=str, help='specifies address of tested service') + parser.addoption( "--hashes", action="store", type=str, help='specifies path to file with hashes to check (one per line)') \ No newline at end of file diff --git a/hived/jsonsocket.py b/hived/jsonsocket.py new file mode 100644 index 0000000000000000000000000000000000000000..0ce98f58954b5f8e01ce566b192c48a08f2106f9 --- /dev/null +++ b/hived/jsonsocket.py @@ -0,0 +1,124 @@ +#!/usr/bin/env python3 +import sys +import json +import socket + +class JSONSocket(object): + """ + Class encapsulates socket object with special handling json rpc. + timeout is ignored now - nonblicking socket not supported. + """ + def __init__(self, host, port, path, timeout=None): + """ + host in form [http[s]://]<ip_address>[:port] + if port not in host must be spefified as argument + """ + self.__sock = None + if host.find("http://") == 0 or host.find("https://") == 0: + host = host[host.find("//")+2 : len(host)] + _host = host + if port != None: + host = host + ":" + str(port) + else: + colon_pos = host.rfind(":") + _host = host[0 : colon_pos] + port = int(host[colon_pos+1 : len(host)]) + self.__fullpath = host + path + self.__host = bytes(host, "utf-8") + self.__path = bytes(path, "utf-8") + #print("<host>: {}; <port>: {}".format(_host, port)) + self.__sock = socket.create_connection((_host, port)) + self.__sock.setblocking(True) + #self.__sock.settimeout(timeout) + self.__head = b"POST " + self.__path + b" HTTP/1.0\r\n" + \ + b"HOST: " + self.__host + b"\r\n" + \ + b"Content-type: application/json\r\n" + + def get_fullpath(self): + return self.__fullpath + + def request(self, data=None, json=None): + """ + data - complete binary form of json request (ends with '\r\n' + json - json request as python dict + + return value in form of json response as python dict + """ + if data == None: + data = bytes(json.dumps(json), "utf-8") + b"\r\n" + length = bytes(str(len(data)), "utf-8") + request = self.__head + \ + b"Content-length: " + length + b"\r\n\r\n" + \ + data + #print("request:", request.decode("utf-8")) + self.__sock.sendall(request) + #self.__sock.send(request) + status, response = self.__read() + #if response == {}: + # print("response is empty for request:", request.decode("utf-8")) + return status, response + + def __call__(self, data=None, json=None): + return self.request(data, json) + + def __read(self): + response = '' + binary = b'' + while True: + temp = self.__sock.recv(4096) + if not temp: break + binary += temp + + response = binary.decode("utf-8") + + if response.find("HTTP") == 0: + response = response[response.find("\r\n\r\n")+4 : len(response)] + if response and response != '': + r = json.loads(response) + if 'result' in r: + return True, r + else: + return False, r + + return False, {} + + def __del__(self): + if self.__sock: + self.__sock.close() + + +def hived_call(host, data=None, json=None, max_tries=10, timeout=0.1): + """ + host - [http[s]://<ip_address>:<port> + data - binary form of request body, if missing json object should be provided (as python dict/array) + """ +# try: +# jsocket = JSONSocket(host, None, "/rpc", timeout) +# except: +# print("Cannot open socket for:", host) +# return False, {} + + for i in range(max_tries): + try: + jsocket = JSONSocket(host, None, "/rpc", timeout) + except: + type, value, traceback = sys.exc_info() + print("Error: {}:{} {} {}".format(1, type, value, traceback)) + print("Error: Cannot open JSONSocket for:", host) + continue + try: + status, response = jsocket(data, json) + if status: + return status, response + except: + type, value, traceback = sys.exc_info() + print("Error: {}:{} {} {}".format(1, type, value, traceback)) + print("Error: JSONSocket request failed for: {} ({})".format(host, data.decode("utf-8"))) + continue + else: + return False, {} + +def universal_call(url, data, *args, **kwargs): + from requests import post + result = post(url, json=data) + return [result.status_code, result.text] diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_owner.pat.json b/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_owner.pat.json deleted file mode 100644 index d6dcde293af54735461ad6abad1361451e9eb739..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_owner.pat.json +++ /dev/null @@ -1,7 +0,0 @@ -{ - "accounts": [ - [ - "gtg" - ] - ] -} diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_owner.tavern.yaml b/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_owner.tavern.yaml deleted file mode 100644 index e68f4c989ec0a3342043e09384cfefd4577e6e8e..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_owner.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_key_references - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_key_references - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_by_key_api.get_key_references" - params: {"keys":["STM5RLQ1Jh8Kf56go3xpzoodg4vRsgCeWhANXoEXrYH7bLEwSVyjh"]} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "gtg_owner" - directory: "account_by_key_api_patterns/get_key_references" \ No newline at end of file diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_posting.pat.json b/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_posting.pat.json deleted file mode 100644 index d6dcde293af54735461ad6abad1361451e9eb739..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_posting.pat.json +++ /dev/null @@ -1,7 +0,0 @@ -{ - "accounts": [ - [ - "gtg" - ] - ] -} diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_posting.tavern.yaml b/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_posting.tavern.yaml deleted file mode 100644 index bae236b27fdfe54db7d15101d02d5816dadb2ec6..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/gtg_posting.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_key_references - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_key_references - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_by_key_api.get_key_references" - params: {"keys":["STM5tp5hWbGLL1R3tMVsgYdYxLPyAQFdKoYFbT2hcWUmrU42p1MQC"]} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "gtg_posting" - directory: "account_by_key_api_patterns/get_key_references" diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/multiple.pat.json b/hived/tavern/account_by_key_api_patterns/get_key_references/multiple.pat.json deleted file mode 100644 index 2b18a8397dabd67d25cfed9d60216279bd77037e..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/multiple.pat.json +++ /dev/null @@ -1,32 +0,0 @@ -{ - "accounts": [ - [ - "gtg" - ], - [ - "alibaba", - "amazon", - "citibank", - "dan", - "ebay", - "grumpymutt", - "hsbc", - "jpmorgan", - "ned-scott", - "softbank", - "steemit1", - "troller", - "ukon", - "walmart" - ], - [ - "ben", - "bitdev100", - "buysbd", - "imadev" - ], - [ - "silver" - ] - ] -} diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/multiple.tavern.yaml b/hived/tavern/account_by_key_api_patterns/get_key_references/multiple.tavern.yaml deleted file mode 100644 index 15601763a77c659a18d08349be45decd3a30c70f..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/multiple.tavern.yaml +++ /dev/null @@ -1,33 +0,0 @@ ---- - test_name: Hived account_history_api get_key_references - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_key_references - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_by_key_api.get_key_references" - params: {"keys":[ - "STM5tp5hWbGLL1R3tMVsgYdYxLPyAQFdKoYFbT2hcWUmrU42p1MQC", - "STM7sw22HqsXbz7D2CmJfmMwt9rimtk518dRzsR1f8Cgw52dQR1pR", - "STM5ya7Y8XKBRuWL2uL8NsSnB691cCzca87Ho9MXaPZ1ZYdGaJBBc", - "STM8epVc3pAcL44kvfvntsQGWVAyeQmFKC9nFdbPFYW1fCEg4sM14" - ]} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "multiple" - directory: "account_by_key_api_patterns/get_key_references" diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_active.pat.json b/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_active.pat.json deleted file mode 100644 index 500b4b6ce44cd90dc678c7eb9ced0336e4a006c1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_active.pat.json +++ /dev/null @@ -1,7 +0,0 @@ -{ - "accounts": [ - [ - "tulpa" - ] - ] -} diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_active.tavern.yaml b/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_active.tavern.yaml deleted file mode 100644 index 6893cc8e4f4a325b73c0aa376bb52f9da2fc22dc..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_active.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_key_references tulpa_active - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_key_references tulpa_active - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_by_key_api.get_key_references" - params: {"keys":["STM6dgT1QxaxngdWJ892vb1FfoKYW8DXAMoZHd5LYswDvPMacBen9"]} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "tulpa_active" - directory: "account_by_key_api_patterns/get_key_references" diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_memo.pat.json b/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_memo.pat.json deleted file mode 100644 index 54fc6dce74bea3b433e3c59a6311b3a65fd61588..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_memo.pat.json +++ /dev/null @@ -1,5 +0,0 @@ -{ - "accounts": [ - [] - ] -} diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_memo.tavern.yaml b/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_memo.tavern.yaml deleted file mode 100644 index 6bf8bc5f02e00ae6f7f2aeee02ae5ca4b26c44c6..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_memo.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_key_references tulpa_memo - - marks: - - patterntest # empty - memo keys are not returned - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_key_references tulpa_memo - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_by_key_api.get_key_references" - params: {"keys":["STM5VL4YZ1U74VZfdUrSq2Npy4mv2hc7HiBvgkWaV8nwMLT8gjaV4"]} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "tulpa_memo" - directory: "account_by_key_api_patterns/get_key_references" diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_owner.pat.json b/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_owner.pat.json deleted file mode 100644 index 500b4b6ce44cd90dc678c7eb9ced0336e4a006c1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_owner.pat.json +++ /dev/null @@ -1,7 +0,0 @@ -{ - "accounts": [ - [ - "tulpa" - ] - ] -} diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_owner.tavern.yaml b/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_owner.tavern.yaml deleted file mode 100644 index ea89c0b8e54d9b15ffdfb805612e9dd07bd2c5a6..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_owner.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_key_references tulpa_owner - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_key_references tulpa_owner - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_by_key_api.get_key_references" - params: {"keys":["STM6wxeXR9kg8uu7vX5LS4HBgKw8sdqHBpzAaacqPwPxYfRx9h5bS"]} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "tulpa_owner" - directory: "account_by_key_api_patterns/get_key_references" diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_posting.pat.json b/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_posting.pat.json deleted file mode 100644 index 500b4b6ce44cd90dc678c7eb9ced0336e4a006c1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_posting.pat.json +++ /dev/null @@ -1,7 +0,0 @@ -{ - "accounts": [ - [ - "tulpa" - ] - ] -} diff --git a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_posting.tavern.yaml b/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_posting.tavern.yaml deleted file mode 100644 index b15585308d7799d127f45eedf4a014237d1f4e5c..0000000000000000000000000000000000000000 --- a/hived/tavern/account_by_key_api_patterns/get_key_references/tulpa_posting.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_key_references tulpa_posting - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_key_references tulpa_posting - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_by_key_api.get_key_references" - params: {"keys":["STM6wxeXR9kg8uu7vX5LS4HBgKw8sdqHBpzAaacqPwPxYfRx9h5bS"]} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "tulpa_posting" - directory: "account_by_key_api_patterns/get_key_references" diff --git a/hived/tavern/account_history_api_negative/get_transaction/unknown_transaction.pat.json b/hived/tavern/account_history_api_negative/get_transaction/unknown_transaction.pat.json deleted file mode 100644 index e5c445638bfa7be996d51d89cdd9dad1869e1d68..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_negative/get_transaction/unknown_transaction.pat.json +++ /dev/null @@ -1,25 +0,0 @@ -{ - "code": -32003, - "data": { - "code": 10, - "message": "Assert Exception", - "name": "assert_exception", - "stack": [ - { - "context": { - "file": "account_history_api.cpp", - "hostname": "", - "level": "error", - "line": 203, - "method": "get_transaction", - "timestamp": "2020-10-22T13:44:23" - }, - "data": { - "t": "1000000000000000000000000000000000000000" - }, - "format": "false: Unknown Transaction ${t}" - } - ] - }, - "message": "Assert Exception:false: Unknown Transaction 1000000000000000000000000000000000000000" -} diff --git a/hived/tavern/account_history_api_negative/get_transaction/unknown_transaction.tavern.yaml b/hived/tavern/account_history_api_negative/get_transaction/unknown_transaction.tavern.yaml deleted file mode 100644 index 6ceff99b54d6e061bc8edccf268e1ed75c1acc45..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_negative/get_transaction/unknown_transaction.tavern.yaml +++ /dev/null @@ -1,30 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction unknown - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction unknown - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_transaction" - params: {"id":"1000"} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "unknown_transaction" - directory: "account_history_api_negative/get_transaction" - error_response: true - ignore_tags: ['context'] diff --git a/hived/tavern/account_history_api_patterns/enum_virtual_ops/first100k.pat.json b/hived/tavern/account_history_api_patterns/enum_virtual_ops/first100k.pat.json deleted file mode 100644 index da3ec7d73e9659af0aa7f620586d6bd6d7a1c4cd..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/enum_virtual_ops/first100k.pat.json +++ /dev/null @@ -1,7787 +0,0 @@ -{ - "next_block_range_begin": 100976, - "next_operation_begin": 0, - "ops": [ - { - "block": 1, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "initminer", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775808", - "timestamp": "2016-03-24T16:05:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1093, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dark", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775817", - "timestamp": "2016-03-24T17:00:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1094, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "admin", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775822", - "timestamp": "2016-03-24T17:00:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1095, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775829", - "timestamp": "2016-03-24T17:00:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1114, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "moderator", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775868", - "timestamp": "2016-03-24T17:01:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1115, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775870", - "timestamp": "2016-03-24T17:01:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1116, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775872", - "timestamp": "2016-03-24T17:01:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1117, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thisisnice", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775873", - "timestamp": "2016-03-24T17:01:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1118, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "cloop2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775874", - "timestamp": "2016-03-24T17:01:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1119, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "cloop1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775876", - "timestamp": "2016-03-24T17:01:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1120, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775878", - "timestamp": "2016-03-24T17:01:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1122, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt4", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775881", - "timestamp": "2016-03-24T17:01:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1123, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steem", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775882", - "timestamp": "2016-03-24T17:01:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1124, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thisisnice0", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775883", - "timestamp": "2016-03-24T17:01:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1125, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "any", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775884", - "timestamp": "2016-03-24T17:01:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1127, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "root", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775886", - "timestamp": "2016-03-24T17:01:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1128, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt5", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775887", - "timestamp": "2016-03-24T17:02:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1129, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "administrator", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775888", - "timestamp": "2016-03-24T17:02:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1130, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775889", - "timestamp": "2016-03-24T17:02:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1131, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775890", - "timestamp": "2016-03-24T17:02:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1147, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman6", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775896", - "timestamp": "2016-03-24T17:03:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1150, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman5", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775897", - "timestamp": "2016-03-24T17:03:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1153, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775900", - "timestamp": "2016-03-24T17:03:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1163, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman7", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775904", - "timestamp": "2016-03-24T17:03:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1176, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt6", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775906", - "timestamp": "2016-03-24T17:04:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1191, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775909", - "timestamp": "2016-03-24T17:05:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1206, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thisisnice2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775912", - "timestamp": "2016-03-24T17:06:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1223, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman8", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775914", - "timestamp": "2016-03-24T17:06:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1256, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775917", - "timestamp": "2016-03-24T17:08:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1277, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "cloop3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775920", - "timestamp": "2016-03-24T17:09:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1301, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thisisnice3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775922", - "timestamp": "2016-03-24T17:10:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1308, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt7", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775925", - "timestamp": "2016-03-24T17:11:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1338, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775930", - "timestamp": "2016-03-24T17:12:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1359, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thisisnice4", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775931", - "timestamp": "2016-03-24T17:13:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1375, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt8", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775932", - "timestamp": "2016-03-24T17:14:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1392, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman4", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775934", - "timestamp": "2016-03-24T17:15:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1419, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit10", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775940", - "timestamp": "2016-03-24T17:16:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1441, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt9", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775943", - "timestamp": "2016-03-24T17:17:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1466, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman9", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775944", - "timestamp": "2016-03-24T17:19:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1488, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thisisnice5", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775945", - "timestamp": "2016-03-24T17:20:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1511, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit11", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775947", - "timestamp": "2016-03-24T17:21:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1533, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit12", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775949", - "timestamp": "2016-03-24T17:22:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1537, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thisisnice6", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775950", - "timestamp": "2016-03-24T17:22:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1559, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit13", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775953", - "timestamp": "2016-03-24T17:23:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1588, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit15", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775954", - "timestamp": "2016-03-24T17:25:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1603, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit16", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775955", - "timestamp": "2016-03-24T17:25:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1631, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thisisnice7", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775959", - "timestamp": "2016-03-24T17:27:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1659, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit17", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775962", - "timestamp": "2016-03-24T17:28:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1677, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thisisnice8", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775963", - "timestamp": "2016-03-24T17:29:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1690, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit18", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775965", - "timestamp": "2016-03-24T17:30:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1710, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit19", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775967", - "timestamp": "2016-03-24T17:31:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1727, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thisisnice9", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775968", - "timestamp": "2016-03-24T17:32:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1748, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775971", - "timestamp": "2016-03-24T17:33:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1781, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit20", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775973", - "timestamp": "2016-03-24T17:34:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1792, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit21", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775974", - "timestamp": "2016-03-24T17:35:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1847, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit22", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775980", - "timestamp": "2016-03-24T17:38:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1859, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit23", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775981", - "timestamp": "2016-03-24T17:38:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1878, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit25", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775984", - "timestamp": "2016-03-24T17:39:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1901, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit26", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775986", - "timestamp": "2016-03-24T17:40:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1924, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit27", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775988", - "timestamp": "2016-03-24T17:41:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1948, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit28", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775992", - "timestamp": "2016-03-24T17:43:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1964, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit29", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775993", - "timestamp": "2016-03-24T17:43:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 1995, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775995", - "timestamp": "2016-03-24T17:45:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2015, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit30", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775998", - "timestamp": "2016-03-24T17:46:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2021, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit31", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854775999", - "timestamp": "2016-03-24T17:46:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2045, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit32", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776003", - "timestamp": "2016-03-24T17:48:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2067, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "arsahk", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776004", - "timestamp": "2016-03-24T17:49:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2085, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit33", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776005", - "timestamp": "2016-03-24T17:50:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2135, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit35", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776009", - "timestamp": "2016-03-24T17:52:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2182, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit36", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776010", - "timestamp": "2016-03-24T17:54:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2203, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit37", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776011", - "timestamp": "2016-03-24T17:55:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2215, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit38", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776014", - "timestamp": "2016-03-24T17:56:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2234, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit39", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776016", - "timestamp": "2016-03-24T17:57:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2268, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit4", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776020", - "timestamp": "2016-03-24T17:59:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2280, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit40", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776022", - "timestamp": "2016-03-24T17:59:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2310, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit41", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776024", - "timestamp": "2016-03-24T18:01:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2348, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit42", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776027", - "timestamp": "2016-03-24T18:03:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2353, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit43", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776028", - "timestamp": "2016-03-24T18:03:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2407, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bavak", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776030", - "timestamp": "2016-03-24T18:06:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2448, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit45", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776031", - "timestamp": "2016-03-24T18:08:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2468, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit46", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776033", - "timestamp": "2016-03-24T18:09:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2502, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit47", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776035", - "timestamp": "2016-03-24T18:10:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2535, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit48", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776039", - "timestamp": "2016-03-24T18:12:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2570, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit49", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776045", - "timestamp": "2016-03-24T18:14:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2620, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit5", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776049", - "timestamp": "2016-03-24T18:16:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2685, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit50", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776053", - "timestamp": "2016-03-24T18:20:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2699, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit51", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776054", - "timestamp": "2016-03-24T18:20:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2782, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mn12defgj1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776058", - "timestamp": "2016-03-24T18:24:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2807, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bavihm", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776059", - "timestamp": "2016-03-24T18:26:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2853, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mr11acdee", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776063", - "timestamp": "2016-03-24T18:28:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2878, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit52", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776068", - "timestamp": "2016-03-24T18:29:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2907, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mr11acdee1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776073", - "timestamp": "2016-03-24T18:31:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2925, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "chana", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776075", - "timestamp": "2016-03-24T18:32:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2954, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit53", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776076", - "timestamp": "2016-03-24T18:33:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 2989, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "brayden", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776077", - "timestamp": "2016-03-24T18:35:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 3035, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit55", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776082", - "timestamp": "2016-03-24T18:37:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 3065, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "hunter", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776085", - "timestamp": "2016-03-24T18:39:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 3071, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "danea", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776087", - "timestamp": "2016-03-24T18:39:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 3094, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit56", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776089", - "timestamp": "2016-03-24T18:40:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 3303, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mottler", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776100", - "timestamp": "2016-03-24T18:51:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 3389, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "darah", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776107", - "timestamp": "2016-03-24T18:55:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 3498, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "erath", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776110", - "timestamp": "2016-03-24T19:01:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 3535, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit60", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776113", - "timestamp": "2016-03-24T19:03:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 3587, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit61", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776118", - "timestamp": "2016-03-24T19:05:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 4591, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman21", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776166", - "timestamp": "2016-03-24T19:57:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 4660, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit57", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776170", - "timestamp": "2016-03-24T20:00:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 4685, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit58", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776172", - "timestamp": "2016-03-24T20:01:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 4757, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit59", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776177", - "timestamp": "2016-03-24T20:05:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 4844, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit6", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776183", - "timestamp": "2016-03-24T20:09:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 4899, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mottler-1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776186", - "timestamp": "2016-03-24T20:12:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 4952, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit62", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776189", - "timestamp": "2016-03-24T20:15:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 4978, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "fminerten", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776192", - "timestamp": "2016-03-24T20:16:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 4988, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit63", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776193", - "timestamp": "2016-03-24T20:17:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5006, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit65", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776195", - "timestamp": "2016-03-24T20:17:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5021, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mottler-2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776197", - "timestamp": "2016-03-24T20:18:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5114, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit66", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776204", - "timestamp": "2016-03-24T20:23:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5182, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "faddy", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776208", - "timestamp": "2016-03-24T20:27:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5206, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit67", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776209", - "timestamp": "2016-03-24T20:28:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5223, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit68", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776210", - "timestamp": "2016-03-24T20:29:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5254, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit69", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776214", - "timestamp": "2016-03-24T20:30:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5277, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit7", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776215", - "timestamp": "2016-03-24T20:32:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5298, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit70", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776216", - "timestamp": "2016-03-24T20:33:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5329, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit71", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776219", - "timestamp": "2016-03-24T20:34:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5356, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit72", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776221", - "timestamp": "2016-03-24T20:36:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5513, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit73", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776229", - "timestamp": "2016-03-24T20:44:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5526, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit75", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776231", - "timestamp": "2016-03-24T20:44:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5547, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit76", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776233", - "timestamp": "2016-03-24T20:45:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5585, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit77", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776234", - "timestamp": "2016-03-24T20:47:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5596, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemit78", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776235", - "timestamp": "2016-03-24T20:48:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5702, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "fminerten1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776246", - "timestamp": "2016-03-24T20:53:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5809, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "batel", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776248", - "timestamp": "2016-03-24T20:59:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 5889, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "fminerten2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776255", - "timestamp": "2016-03-24T21:03:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 6030, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "fminerten3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776264", - "timestamp": "2016-03-24T21:10:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 6180, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "fminerten4", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776274", - "timestamp": "2016-03-24T21:17:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 6257, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "batel1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776277", - "timestamp": "2016-03-24T21:21:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 6279, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "faddy3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776282", - "timestamp": "2016-03-24T21:22:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 6368, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "fminerten5", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776285", - "timestamp": "2016-03-24T21:27:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 6733, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "fminerten6", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776303", - "timestamp": "2016-03-24T21:46:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7055, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "fminerten8", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776323", - "timestamp": "2016-03-24T22:03:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7171, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "batel2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776326", - "timestamp": "2016-03-24T22:09:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7371, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "batel3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776333", - "timestamp": "2016-03-24T22:19:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7522, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgen11", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776341", - "timestamp": "2016-03-24T22:27:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7541, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgen10", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776343", - "timestamp": "2016-03-24T22:28:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7578, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgen1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776346", - "timestamp": "2016-03-24T22:29:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7652, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxt10", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776353", - "timestamp": "2016-03-24T22:33:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7739, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgen6", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776356", - "timestamp": "2016-03-24T22:38:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7757, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgen2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776360", - "timestamp": "2016-03-24T22:39:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7760, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgen12", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776361", - "timestamp": "2016-03-24T22:39:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 7899, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steemthebest", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776366", - "timestamp": "2016-03-24T22:46:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 8323, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman10", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776391", - "timestamp": "2016-03-24T23:09:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 8473, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "erihn", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776398", - "timestamp": "2016-03-24T23:17:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 9023, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgen13", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776428", - "timestamp": "2016-03-24T23:44:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 9032, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgen7", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776429", - "timestamp": "2016-03-24T23:45:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 9060, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgen3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776431", - "timestamp": "2016-03-24T23:46:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 9277, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "biggest", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776443", - "timestamp": "2016-03-24T23:57:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 9774, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "brown", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776467", - "timestamp": "2016-03-25T00:23:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 9787, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "france", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776469", - "timestamp": "2016-03-25T00:24:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 9824, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "itsascam", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776471", - "timestamp": "2016-03-25T00:26:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 9833, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "james", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776473", - "timestamp": "2016-03-25T00:26:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 9860, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "diego", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776476", - "timestamp": "2016-03-25T00:27:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 10532, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "analisa", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776508", - "timestamp": "2016-03-25T01:01:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 10977, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "anastacia", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776524", - "timestamp": "2016-03-25T01:24:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 11032, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgen16", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776530", - "timestamp": "2016-03-25T01:27:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 11404, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "panadacoin", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776551", - "timestamp": "2016-03-25T01:47:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 11440, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "hiva", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776554", - "timestamp": "2016-03-25T01:49:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 11830, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "aftergut", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776575", - "timestamp": "2016-03-25T02:08:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 12148, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "fmooo", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776588", - "timestamp": "2016-03-25T02:24:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 12265, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mottler-3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776597", - "timestamp": "2016-03-25T02:30:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 12346, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "drsteem", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776603", - "timestamp": "2016-03-25T02:34:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 13051, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "abdul", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776637", - "timestamp": "2016-03-25T03:10:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 13323, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "teem", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776651", - "timestamp": "2016-03-25T03:24:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 13702, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "ashleigh", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776668", - "timestamp": "2016-03-25T03:43:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 13755, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "donkeyman1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776672", - "timestamp": "2016-03-25T03:46:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 13790, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "hiva1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776675", - "timestamp": "2016-03-25T03:47:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 14760, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "aguilar", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776724", - "timestamp": "2016-03-25T04:36:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 14807, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "arroyo", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776727", - "timestamp": "2016-03-25T04:38:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 14906, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "back", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776732", - "timestamp": "2016-03-25T04:43:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 15500, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "jones", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776759", - "timestamp": "2016-03-25T05:13:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 15808, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "azeroth", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776778", - "timestamp": "2016-03-25T05:29:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 16166, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "augusta", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776795", - "timestamp": "2016-03-25T05:49:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 16668, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "scam", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776823", - "timestamp": "2016-03-25T06:14:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 16766, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mary", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776828", - "timestamp": "2016-03-25T06:20:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 16810, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "franz", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776829", - "timestamp": "2016-03-25T06:22:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 16999, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bitcointalker", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776836", - "timestamp": "2016-03-25T06:32:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 17146, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blackrock", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776845", - "timestamp": "2016-03-25T06:40:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 17487, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "essence", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776865", - "timestamp": "2016-03-25T06:57:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 17725, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "barrie", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776878", - "timestamp": "2016-03-25T07:09:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 17818, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "chen", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776882", - "timestamp": "2016-03-25T07:13:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 17882, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bendixen", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776885", - "timestamp": "2016-03-25T07:17:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 18331, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "thomass", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776909", - "timestamp": "2016-03-25T07:39:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 18538, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "aeon", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776919", - "timestamp": "2016-03-25T07:51:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 18652, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "monero", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776928", - "timestamp": "2016-03-25T07:58:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 18680, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "function", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776929", - "timestamp": "2016-03-25T08:00:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 19129, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "structure", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776951", - "timestamp": "2016-03-25T08:24:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 19170, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "benita", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776954", - "timestamp": "2016-03-25T08:26:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 19378, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "yomamasofat", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776966", - "timestamp": "2016-03-25T08:36:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 19469, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "kkk", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776973", - "timestamp": "2016-03-25T08:41:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 19944, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bess", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854776993", - "timestamp": "2016-03-25T09:05:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 20086, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "lll", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777001", - "timestamp": "2016-03-25T09:12:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 20199, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blanche", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777006", - "timestamp": "2016-03-25T09:17:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 20345, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "style", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777011", - "timestamp": "2016-03-25T09:25:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 20361, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman11", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777014", - "timestamp": "2016-03-25T09:25:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 20540, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "cherly", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777029", - "timestamp": "2016-03-25T09:35:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 20590, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "claudine", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777032", - "timestamp": "2016-03-25T09:37:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 20638, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mmm", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777035", - "timestamp": "2016-03-25T09:40:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 20788, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nnn", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777043", - "timestamp": "2016-03-25T09:47:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 20993, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "lee", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777054", - "timestamp": "2016-03-25T09:57:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 21111, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nextgencrypto9", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777059", - "timestamp": "2016-03-25T10:03:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 21327, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "adrian", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777070", - "timestamp": "2016-03-25T10:15:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 21610, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman12", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777086", - "timestamp": "2016-03-25T10:30:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 21907, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman13", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777100", - "timestamp": "2016-03-25T10:45:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 22583, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "lin", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777132", - "timestamp": "2016-03-25T11:21:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 22677, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "italy", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777140", - "timestamp": "2016-03-25T11:26:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 22959, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "anna", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777154", - "timestamp": "2016-03-25T11:41:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 23069, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "emily", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777158", - "timestamp": "2016-03-25T11:47:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 23230, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "galel", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777165", - "timestamp": "2016-03-25T11:55:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 23332, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dantheman22", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777174", - "timestamp": "2016-03-25T12:00:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 23426, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "norway", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777180", - "timestamp": "2016-03-25T12:04:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 23898, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "scamcoin", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777204", - "timestamp": "2016-03-25T12:28:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 23974, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "gamath", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777209", - "timestamp": "2016-03-25T12:32:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 24261, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steempty2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777221", - "timestamp": "2016-03-25T12:47:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 24307, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steempty3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777226", - "timestamp": "2016-03-25T12:49:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 24812, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "scamconfirmed", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777251", - "timestamp": "2016-03-25T13:14:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 25280, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steempty", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777274", - "timestamp": "2016-03-25T13:38:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 25530, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steempty1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777289", - "timestamp": "2016-03-25T13:50:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 25592, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "hannah", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777296", - "timestamp": "2016-03-25T13:53:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 26723, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "smooth", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777348", - "timestamp": "2016-03-25T14:50:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 27027, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "steempty4", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777365", - "timestamp": "2016-03-25T15:05:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 27382, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "jenn", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777384", - "timestamp": "2016-03-25T15:23:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 27724, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bottai", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777400", - "timestamp": "2016-03-25T15:41:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 27807, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "brownrigg", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777403", - "timestamp": "2016-03-25T15:45:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 28038, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bucci", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777417", - "timestamp": "2016-03-25T15:56:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 28577, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "alice", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777442", - "timestamp": "2016-03-25T16:23:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 29099, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bob", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777470", - "timestamp": "2016-03-25T16:49:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 29144, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "figaro", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777474", - "timestamp": "2016-03-25T16:52:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 29884, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "aaa", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777516", - "timestamp": "2016-03-25T17:31:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30006, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "starky", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777522", - "timestamp": "2016-03-25T17:37:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30097, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "user", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777527", - "timestamp": "2016-03-25T17:42:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30198, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777532", - "timestamp": "2016-03-25T17:47:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30269, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer10", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777538", - "timestamp": "2016-03-25T17:50:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30542, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer11", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777550", - "timestamp": "2016-03-25T18:05:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30590, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer12", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777556", - "timestamp": "2016-03-25T18:07:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30613, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "madison", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777557", - "timestamp": "2016-03-25T18:08:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30635, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer13", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777558", - "timestamp": "2016-03-25T18:09:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30671, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer15", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777561", - "timestamp": "2016-03-25T18:11:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30695, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer16", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777563", - "timestamp": "2016-03-25T18:12:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30704, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer17", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777565", - "timestamp": "2016-03-25T18:13:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30783, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer18", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777569", - "timestamp": "2016-03-25T18:17:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30809, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer19", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777571", - "timestamp": "2016-03-25T18:18:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30850, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777576", - "timestamp": "2016-03-25T18:20:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30878, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer20", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777579", - "timestamp": "2016-03-25T18:21:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30893, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer21", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777582", - "timestamp": "2016-03-25T18:22:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30934, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer22", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777584", - "timestamp": "2016-03-25T18:24:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 30978, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer23", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777587", - "timestamp": "2016-03-25T18:26:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31015, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer25", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777589", - "timestamp": "2016-03-25T18:28:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31033, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer26", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777591", - "timestamp": "2016-03-25T18:29:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31060, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer27", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777594", - "timestamp": "2016-03-25T18:31:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31086, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer28", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777597", - "timestamp": "2016-03-25T18:32:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31110, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer29", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777599", - "timestamp": "2016-03-25T18:33:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31176, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777604", - "timestamp": "2016-03-25T18:36:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31205, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer30", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777606", - "timestamp": "2016-03-25T18:38:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31217, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer31", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777607", - "timestamp": "2016-03-25T18:38:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31235, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer32", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777609", - "timestamp": "2016-03-25T18:39:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31249, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer33", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777611", - "timestamp": "2016-03-25T18:40:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31283, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer35", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777613", - "timestamp": "2016-03-25T18:42:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31293, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer36", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777614", - "timestamp": "2016-03-25T18:42:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31387, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer37", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777619", - "timestamp": "2016-03-25T18:47:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31399, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer38", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777623", - "timestamp": "2016-03-25T18:48:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31469, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer39", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777626", - "timestamp": "2016-03-25T18:51:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31497, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer4", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777627", - "timestamp": "2016-03-25T18:52:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31560, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer40", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777631", - "timestamp": "2016-03-25T18:56:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31612, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer41", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777634", - "timestamp": "2016-03-25T18:58:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31640, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer42", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777635", - "timestamp": "2016-03-25T19:00:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31658, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer43", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777636", - "timestamp": "2016-03-25T19:00:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31680, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer45", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777637", - "timestamp": "2016-03-25T19:02:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31714, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer46", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777642", - "timestamp": "2016-03-25T19:03:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31763, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer47", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777646", - "timestamp": "2016-03-25T19:06:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31787, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer48", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777650", - "timestamp": "2016-03-25T19:07:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31843, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer49", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777653", - "timestamp": "2016-03-25T19:10:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31907, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer5", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777659", - "timestamp": "2016-03-25T19:13:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31946, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer50", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777660", - "timestamp": "2016-03-25T19:15:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 31975, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer51", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777663", - "timestamp": "2016-03-25T19:16:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32004, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer52", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777664", - "timestamp": "2016-03-25T19:18:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32025, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer53", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777665", - "timestamp": "2016-03-25T19:19:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32051, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer55", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777667", - "timestamp": "2016-03-25T19:20:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32124, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer56", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777672", - "timestamp": "2016-03-25T19:24:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32141, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer57", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777674", - "timestamp": "2016-03-25T19:25:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32164, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer58", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777675", - "timestamp": "2016-03-25T19:26:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32343, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer59", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777684", - "timestamp": "2016-03-25T19:35:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32396, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer6", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777688", - "timestamp": "2016-03-25T19:37:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32408, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer60", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777689", - "timestamp": "2016-03-25T19:38:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32443, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer61", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777693", - "timestamp": "2016-03-25T19:40:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32451, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer62", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777694", - "timestamp": "2016-03-25T19:40:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32518, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer63", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777698", - "timestamp": "2016-03-25T19:43:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32534, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer65", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777701", - "timestamp": "2016-03-25T19:44:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32575, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer66", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777704", - "timestamp": "2016-03-25T19:46:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32840, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer67", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777718", - "timestamp": "2016-03-25T20:00:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 32871, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer68", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777721", - "timestamp": "2016-03-25T20:01:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 33080, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer69", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777733", - "timestamp": "2016-03-25T20:12:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 33879, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "gifthana", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777773", - "timestamp": "2016-03-25T20:52:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 34767, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "graavor", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777815", - "timestamp": "2016-03-25T21:36:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 36621, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer7", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777907", - "timestamp": "2016-03-25T23:09:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 36635, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer70", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777909", - "timestamp": "2016-03-25T23:09:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 36658, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer71", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777910", - "timestamp": "2016-03-25T23:11:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 36809, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer72", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777917", - "timestamp": "2016-03-25T23:18:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 36834, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer73", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777919", - "timestamp": "2016-03-25T23:19:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 36888, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer75", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777923", - "timestamp": "2016-03-25T23:22:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 36910, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer76", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777924", - "timestamp": "2016-03-25T23:23:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 36962, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer77", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777928", - "timestamp": "2016-03-25T23:26:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 37139, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer78", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777938", - "timestamp": "2016-03-25T23:35:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 37386, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "fff", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777952", - "timestamp": "2016-03-25T23:47:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 37531, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "anonymous", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777959", - "timestamp": "2016-03-25T23:54:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 37562, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "ggg", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777961", - "timestamp": "2016-03-25T23:56:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 37909, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer79", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777976", - "timestamp": "2016-03-26T00:13:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 37934, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer8", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854777978", - "timestamp": "2016-03-26T00:14:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 41172, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "rainman", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778135", - "timestamp": "2016-03-26T02:56:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 41845, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bbb", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778165", - "timestamp": "2016-03-26T03:30:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 42003, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "jamgotin", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778176", - "timestamp": "2016-03-26T03:38:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 43694, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "ashley", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778259", - "timestamp": "2016-03-26T05:02:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 43795, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "brianna", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778266", - "timestamp": "2016-03-26T05:07:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 45057, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "hailey", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778328", - "timestamp": "2016-03-26T06:11:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 45348, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer80", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778340", - "timestamp": "2016-03-26T06:25:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 45371, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer81", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778341", - "timestamp": "2016-03-26T06:26:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 45431, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer82", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778346", - "timestamp": "2016-03-26T06:29:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 45536, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer83", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778353", - "timestamp": "2016-03-26T06:35:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 46043, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer85", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778379", - "timestamp": "2016-03-26T07:00:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 46313, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "lessys", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778393", - "timestamp": "2016-03-26T07:13:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 46962, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "colorblue", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778424", - "timestamp": "2016-03-26T07:46:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 47128, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "charlesmanson", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778432", - "timestamp": "2016-03-26T07:54:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 47317, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "jacktheripper", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778440", - "timestamp": "2016-03-26T08:04:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 48767, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "rainmac", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778516", - "timestamp": "2016-03-26T09:17:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 48901, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "jeffreydahmer", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778522", - "timestamp": "2016-03-26T09:24:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 49403, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "berniesanders", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778545", - "timestamp": "2016-03-26T09:49:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 50616, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "americanpegasus", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778606", - "timestamp": "2016-03-26T10:51:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 50767, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "jasmine", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778613", - "timestamp": "2016-03-26T10:58:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 50851, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "penambang", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778619", - "timestamp": "2016-03-26T11:02:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 51608, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "binaryfate", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778654", - "timestamp": "2016-03-26T11:40:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 51984, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "lisp", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778673", - "timestamp": "2016-03-26T11:59:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 52024, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "kevphanis", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778676", - "timestamp": "2016-03-26T12:01:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 52360, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "debruyne", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778693", - "timestamp": "2016-03-26T12:18:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 53403, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "canadiancruz", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778740", - "timestamp": "2016-03-26T13:10:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 53560, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "arctic", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778750", - "timestamp": "2016-03-26T13:18:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 55703, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "earth", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778855", - "timestamp": "2016-03-26T15:05:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 56390, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bach", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778891", - "timestamp": "2016-03-26T15:39:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 56573, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "atlantic", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778899", - "timestamp": "2016-03-26T15:48:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 56955, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "dnaleor", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854778920", - "timestamp": "2016-03-26T16:07:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 60813, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "edison", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779105", - "timestamp": "2016-03-26T19:20:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 62849, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer86", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779204", - "timestamp": "2016-03-26T21:02:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 63180, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer87", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779221", - "timestamp": "2016-03-26T21:19:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 63244, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer88", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779223", - "timestamp": "2016-03-26T21:22:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 63319, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer89", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779230", - "timestamp": "2016-03-26T21:26:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 63373, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer9", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779233", - "timestamp": "2016-03-26T21:28:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 63450, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mars", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779239", - "timestamp": "2016-03-26T21:32:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 63695, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "sminer90", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779251", - "timestamp": "2016-03-26T21:44:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 67061, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "bearface", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779417", - "timestamp": "2016-03-27T00:33:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 69343, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "cleta", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779525", - "timestamp": "2016-03-27T02:28:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 69545, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "berkah", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779539", - "timestamp": "2016-03-27T02:38:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 70176, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "rainman-2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779567", - "timestamp": "2016-03-27T03:10:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 71491, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxtgencrpto11", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779632", - "timestamp": "2016-03-27T04:16:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 72289, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "alvaro", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779672", - "timestamp": "2016-03-27T04:55:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 73166, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "hello", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854779712", - "timestamp": "2016-03-27T05:39:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 78235, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "hanyuu", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780015", - "timestamp": "2016-03-27T09:55:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 78351, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "rainman-3", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780023", - "timestamp": "2016-03-27T10:01:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 79469, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mastersmaster", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780079", - "timestamp": "2016-03-27T10:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 79526, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "kevtorin", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780083", - "timestamp": "2016-03-27T11:00:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 82323, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxtgencrpto1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780220", - "timestamp": "2016-03-27T13:20:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 83516, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxtgencrpto12", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780279", - "timestamp": "2016-03-27T14:19:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 84557, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "cucistim", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780328", - "timestamp": "2016-03-27T15:11:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 85138, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxtgencrpto10", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780356", - "timestamp": "2016-03-27T15:41:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 85714, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "tyler", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780390", - "timestamp": "2016-03-27T16:09:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 86201, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "summon", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780417", - "timestamp": "2016-03-27T16:34:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 87251, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "summon1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780468", - "timestamp": "2016-03-27T17:27:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 87810, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "natsu", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780497", - "timestamp": "2016-03-27T17:55:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 89168, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mottler-4", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780560", - "timestamp": "2016-03-27T19:04:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 89190, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mottler-5", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780562", - "timestamp": "2016-03-27T19:05:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 89559, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mottler-6", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780582", - "timestamp": "2016-03-27T19:24:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 90063, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "mottler-7", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780608", - "timestamp": "2016-03-27T19:49:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 90655, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "unix", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780638", - "timestamp": "2016-03-27T20:19:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 91366, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "charleskoch", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780671", - "timestamp": "2016-03-27T20:54:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 92104, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "luigi", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780712", - "timestamp": "2016-03-27T21:31:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 93775, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "lxteem", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780790", - "timestamp": "2016-03-27T22:56:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 94928, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "kalimdor", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780847", - "timestamp": "2016-03-27T23:53:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 95918, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nxtgencrpto2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780899", - "timestamp": "2016-03-28T00:43:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 96457, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "kidrock", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780925", - "timestamp": "2016-03-28T01:10:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 97502, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "nerivyre", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854780983", - "timestamp": "2016-03-28T02:02:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 98506, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "lightfoot", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854781029", - "timestamp": "2016-03-28T02:52:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 99208, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "node1", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854781064", - "timestamp": "2016-03-28T03:27:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 99348, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "node2", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854781072", - "timestamp": "2016-03-28T03:34:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - }, - { - "block": 99463, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "star", - "vesting_shares": { - "amount": "1000000", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": "9223372036854781077", - "timestamp": "2016-03-28T03:40:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - "ops_by_block": [] -} diff --git a/hived/tavern/account_history_api_patterns/enum_virtual_ops/first100k.tavern.yaml b/hived/tavern/account_history_api_patterns/enum_virtual_ops/first100k.tavern.yaml deleted file mode 100644 index 6c9d6fa6e0ba8f98573f18b7a776b24b46b02da5..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/enum_virtual_ops/first100k.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api enum_virtual_ops first 100k blocks - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api enum_virtual_ops first 100k blocks - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.enum_virtual_ops" - params: {"block_range_begin": 1, "block_range_end": 100000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "first100k" - directory: "account_history_api_patterns/enum_virtual_ops" - ignore_tags: ['operation_id'] \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_create_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/account_create_operation.pat.json deleted file mode 100644 index 8bdcedba94645639367a147eb7830ec73b1d554e..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_create_operation.pat.json +++ /dev/null @@ -1,564 +0,0 @@ -{ - "history": [ - [ - 3, - { - "block": 160790, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM8HCf7QLUexogEviN8x1SpKRhFwg2sc8LrWuJqv7QsmWrua6ZyR", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "hello", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "{}", - "memo_key": "STM6Gkj27XMkoGsr4zwEvkjNhh4dykbXmPFzHhT8g86jWsqu3U38X", - "new_account_name": "fabian", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM8MN3FNBa8WbEpxz3wGL3L1mkt6sGnncH8iuto7r8Wa3T9NSSGT", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM8EhGWcEuQ2pqCKkGHnbmcTNpWYZDjGTT7ketVBp4gUStDr2brz", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T07:05:03", - "trx_id": "3c1eae5754cc4f70cf0efb12f9e4f9671a8df2a0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 4, - { - "block": 160790, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM8QZe4wNZbMhYmjNq4UCPcHzdFyNPZh43a67B3SvZhLJ6wRYdMv", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "hello", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "{}", - "memo_key": "STM7rw7CCGfxZAcdW2rkJdPun1tYjkk1ipJncDQMdTv3XxyR6gzkp", - "new_account_name": "schuh", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM679rrUFrdzy2JV5JAL3ZW5Ub44pzt5cuT5oR1bqgDTyqqxkskA", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7gVAkiYKirUycvj5oLBLkeQYco8mMwHurpqWCuzfZqNJDoqgZB", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T07:05:03", - "trx_id": "9a239d5e373d2cd076253231866a3dd3e41f4606", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 5, - { - "block": 160790, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM7ko5nzqaYfjbD4tKWGmiy3xtT9eQFZ3Pcmq5JmygTRptWSiVQy", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "hello", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "{}", - "memo_key": "STM8ZSyzjPm48GmUuMSRufkVYkwYbZzbxeMysAVp7KFQwbTf98TcG", - "new_account_name": "usd", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5b4i9gBqvh4sbgrooXPu2dbGLewNPZkXeuNeBjyiswnu2szgXx", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5xAKxnMT2y9VoVJdF63K8xRQAohsiQy9bA33aHeyMB5vgkzaay", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T07:05:03", - "trx_id": "75906618242c596718f4d657de70c56cae6e7d74", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 6, - { - "block": 160790, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM5p3Q8ZsCpR5ypDgnEq8smKe4XRG5w1UpbFpGLKxZXuDX7gfdPt", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "hello", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "{}", - "memo_key": "STM5xLG25Sr5UcNwAgqH87wzYyUJzeywvzNcAeCY8mBXrLjVx8Ymj", - "new_account_name": "bitshares", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM85NWws3Ux7JNYCwiTRkhYeEuwbhqxcvU1RhsV5uHFzi5deBv3k", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM51RGJoMhzZ54PKqymhjpq6jr8fbhbJdtzT2KNhqde1u6ufRHSK", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T07:05:03", - "trx_id": "1f7ac7c1cf925d4240ab403a8a7384ce639f4033", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 7, - { - "block": 160817, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM74TXbqeNFgo63hsGssbQhtd6c4UeX28XyFsnc9SuXaW1Q35Qok", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "hello", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "{}", - "memo_key": "STM54gLcrBZuZzxbtM1VLAHBy7pSdJncv1rUq2Q6barCrGtg3Wjar", - "new_account_name": "fabi", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM8LxMwKZKk26HXX5xSBHavuJqAmDudYcLpuMtNmJHSt3ybDkG4G", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM8ESkvC3V1ubgThoGA35US4Ar1FkF2CuvcMCdW6wdiso9mFg6Nd", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T07:06:24", - "trx_id": "2ba97f6fd25566d987848fdcae45db6c48996ced", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8, - { - "block": 160839, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM8fn223tX8QVo2mzBGCyVpehGikz7TGq2TsNo3Mak4d288NBTvB", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "hello", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "{}", - "memo_key": "STM5FwfwgQYrd5zWXhAsLrSQewRjcfmz3qYn5CVMiBTqkX2ukBoHf", - "new_account_name": "com", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM711dbukXZ7R9qPhMQwpB65UYR7RGb2tYG2wrGzDehZfdt29AAv", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM6aGwFpcz7AmjVuJX41piL589DxPcLHjqQRF1JjJmuHfjwcz4Ve", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T07:07:30", - "trx_id": "9bee9f3a0aaa15f267a8518e11d8dade70fba1da", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9, - { - "block": 160839, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM67NuZTpMR1zQVvrsZLKnH5uA7vMiEjHpBnSxszxHVU6jLHKX93", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "hello", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "{}", - "memo_key": "STM7sMVcg1SycN1h98uKNKWtFiZNQYZHZ95LUrzMCucZXbotubVcu", - "new_account_name": "org", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5ZkQxHQvCbmQ56YCdZdg5Lqe5kQGJD1m1inK25sCP5msb3NSJ4", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM88NVEbrFP8TTfXNvMWemuLTMFQjtExRs8EZqXxJqLdvqhYKAcH", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T07:07:30", - "trx_id": "f35a7022a2b0d882723ed231a436e4098d316e84", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 10, - { - "block": 160839, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM5fQVDcxr4UUZkLFMie9hdRtEt1BNhq3237xoKHEZ2ftXzoPd5T", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "hello", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "{}", - "memo_key": "STM8LhAeMhumWJdZnKSYBbh5PzjkXcR5r5HCbgqaZ5yYP4KcHktFo", - "new_account_name": "info", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM6z56W5RyXgm2wwg74PuZ8n7jxT7BSUbc1fuiDuumxK2zR2GjdG", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM8Nuf4E19YQTh8rr1XvSyBaQ6FcoAJj6ngHJmw4ZzwFkzNYwLFd", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T07:07:30", - "trx_id": "0a864615d5584e5fc32cd77666b02f611d00f0ba", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 11, - { - "block": 160839, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6jJLdHaKwuBBmsxmrrDmLrgSpBoi3Ak5tWsWU5wi3XKqmHx94M", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "hello", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "{}", - "memo_key": "STM7C5p6xr8wvWPS2wQSKCtzZaXgy4YgTNieUWnw76mKvZa7SoVbm", - "new_account_name": "news", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM52gKmvxJa4hNR2H1yZpYye2fnPxaLM3todZdqZQd7DdeS4EPsK", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM73G8NX181Vh6gpbaZcEJ5PYc6TEeo2Y8WtZQrTprBHJ891Wijb", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T07:07:30", - "trx_id": "e3a2113b04f310953486f716175cf5284347ff0e", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 18, - { - "block": 422612, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6tzLFnJayp64rfuEkPe9VJLErMgjbcpwr7bVr7ngto9jxaWURP", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "hello", - "fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "{}", - "memo_key": "STM5G654zQimHWNQHACCathTZQBhiRTN547zWq4Z4ysUnFv7VrEwm", - "new_account_name": "xeroc", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM7mgtsF5XPU9tokFpEz2zN9sQ89oAcRfcaSkZLsiqfWMtRDNKkc", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM8aJtoKdTsrRrWg3PB9XsbsCgZbVeDhQS3VUM1jkcXfVSjbv4T8", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-08T13:48:54", - "trx_id": "ce087bce41cf248c357da740eb4ae4185c2c973b", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_create_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/account_create_operation.tavern.yaml deleted file mode 100644 index b9c7c3bc87e3d486bb0ed3afa0e642fe86ccd0fa..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_create_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to account_create_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history account_create_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"hello","operation_filter_low": 512,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "account_create_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_create_with_delegation_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/account_create_with_delegation_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_create_with_delegation_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_create_with_delegation_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/account_create_with_delegation_operation.tavern.yaml deleted file mode 100644 index e2ae50e192f54bb6a3fc4b41d5684711e4b4b19f..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_create_with_delegation_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api account_create_with_delegation_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api account_create_with_delegation_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 2199023255552, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "account_create_with_delegation_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_update2_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/account_update2_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_update2_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_update2_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/account_update2_operation.tavern.yaml deleted file mode 100644 index 152f6ed393a7c8228825bfd3a77655f97cef1fbc..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_update2_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api account_update2_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api account_update2_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 8796093022208, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "account_update2_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_update_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/account_update_operation.pat.json deleted file mode 100644 index 294993f81d689d7e37c76a86d1f9bd1e224339e7..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_update_operation.pat.json +++ /dev/null @@ -1,244 +0,0 @@ -{ - "history": [ - [ - 139, - { - "block": 2885318, - "op": { - "type": "account_update_operation", - "value": { - "account": "gtg", - "json_metadata": "", - "memo_key": "STM5F9tCbND6zWPwksy1rEN24WjPiQWSU2vwGgegQVjAcYDe1zTWi", - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM69ZG1hx2rdU2hxQkkmX5MmYkHPCmdNeXg4r6CR7gvKUzYwWPPZ", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T20:54:21", - "trx_id": "28590cbf62ba9f0ec6dd6fe9669852068772ee59", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 140, - { - "block": 2885370, - "op": { - "type": "account_update_operation", - "value": { - "account": "gtg", - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6AzXNwWRzTWCVTgP4oKQEALTW8HmDuRq1avGWjHH23XBNhux6U", - 1 - ] - ], - "weight_threshold": 1 - }, - "json_metadata": "", - "memo_key": "STM5F9tCbND6zWPwksy1rEN24WjPiQWSU2vwGgegQVjAcYDe1zTWi" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T20:56:57", - "trx_id": "60a00c1016cede7d7924f6b32ba4190252b369b7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 141, - { - "block": 2885375, - "op": { - "type": "account_update_operation", - "value": { - "account": "gtg", - "json_metadata": "", - "memo_key": "STM78Vaf41p9UUMMJvafLTjMurnnnuAiTqChiT5GBph7VDWahQRsz" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T20:57:12", - "trx_id": "57a8a136ff02b6b89c31b55314c7e3112939be14", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 142, - { - "block": 2885409, - "op": { - "type": "account_update_operation", - "value": { - "account": "gtg", - "json_metadata": "", - "memo_key": "STM6EY4jgDpLKrJa1RE5y49oUu64BLTrjo4SAso7fxtsaY8Mpzqgx" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T20:58:54", - "trx_id": "3fc900bf7e4ce2f81664359d399ec0c61826f0a0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 143, - { - "block": 2885463, - "op": { - "type": "account_update_operation", - "value": { - "account": "gtg", - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6AzXNwWRzTWCVTgP4oKQEALTW8HmDuRq1avGWjHH23XBNhux6U", - 1 - ] - ], - "weight_threshold": 1 - }, - "json_metadata": "", - "memo_key": "STM78Vaf41p9UUMMJvafLTjMurnnnuAiTqChiT5GBph7VDWahQRsz", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5F9tCbND6zWPwksy1rEN24WjPiQWSU2vwGgegQVjAcYDe1zTWi", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM69ZG1hx2rdU2hxQkkmX5MmYkHPCmdNeXg4r6CR7gvKUzYwWPPZ", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T21:01:36", - "trx_id": "59ccc2dd299ada55308a5f4fd9e2735847e92a58", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 355, - { - "block": 3191323, - "op": { - "type": "account_update_operation", - "value": { - "account": "gtg", - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6AzXNwWRzTWCVTgP4oKQEALTW8HmDuRq1avGWjHH23XBNhux6U", - 1 - ] - ], - "weight_threshold": 1 - }, - "json_metadata": "", - "memo_key": "STM78Vaf41p9UUMMJvafLTjMurnnnuAiTqChiT5GBph7VDWahQRsz", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM6oUcdLthTMc2CDQk5Docc8pB9Zh9s5EQ1wjBZwnMvggbMkRBth", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM69ZG1hx2rdU2hxQkkmX5MmYkHPCmdNeXg4r6CR7gvKUzYwWPPZ", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-14T13:29:03", - "trx_id": "8edfc3159e6b8490e86262dc066336f85b730be7", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 377, - { - "block": 3399203, - "op": { - "type": "account_update_operation", - "value": { - "account": "gtg", - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM4vuEE8F2xyJhwiNCnHxjUVLNXxdFXtVxgghBq5LVLt49zLKLRX", - 1 - ] - ], - "weight_threshold": 1 - }, - "json_metadata": "", - "memo_key": "STM4uD3dfLvbz7Tkd7of4K9VYGnkgrY5BHSQt52vE52CBL5qBfKHN", - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5tp5hWbGLL1R3tMVsgYdYxLPyAQFdKoYFbT2hcWUmrU42p1MQC", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-21T21:48:06", - "trx_id": "5a3a345d3265d40fd4f7ac1906fddec59ac177c3", - "trx_in_block": 1, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_update_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/account_update_operation.tavern.yaml deleted file mode 100644 index 8e491a06c900315a7e63e8be361224a17da41ac3..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_update_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to account_update_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history account_update_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"gtg","operation_filter_low": 1024,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "account_update_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_witness_proxy_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/account_witness_proxy_operation.pat.json deleted file mode 100644 index e5dcd051bb906d24f3bb20c618ce4fa5b73a1e94..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_witness_proxy_operation.pat.json +++ /dev/null @@ -1,23 +0,0 @@ -{ - "history": [ - [ - 759, - { - "block": 3924580, - "op": { - "type": "account_witness_proxy_operation", - "value": { - "account": "geoffrey", - "proxy": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T06:52:00", - "trx_id": "481a52b2a98177c699590240ad845c3b354dd77e", - "trx_in_block": 1, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_witness_proxy_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/account_witness_proxy_operation.tavern.yaml deleted file mode 100644 index 993e2826c3d08b4738ae797c12aca032cb64a820..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_witness_proxy_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to account_witness_proxy_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history account_witness_proxy_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"gtg","operation_filter_low": 8192,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "account_witness_proxy_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_witness_vote_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/account_witness_vote_operation.pat.json deleted file mode 100644 index 19a30778300bba0ec10f1002ca05487ae3fbd512..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_witness_vote_operation.pat.json +++ /dev/null @@ -1,704 +0,0 @@ -{ - "history": [ - [ - 103, - { - "block": 2881334, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:35:00", - "trx_id": "1e49a977ca64c7762042f8ef55d8583d66faad66", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 301, - { - "block": 3100789, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "pfunk" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-11T09:40:45", - "trx_id": "7387eac7188e69e610b412074875352b3002945a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 304, - { - "block": 3104291, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "arhag" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-11T12:36:12", - "trx_id": "8540eb01bab6982a2d477f1949cbfedcb335a2da", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 305, - { - "block": 3105480, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "witness.svk" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-11T13:35:54", - "trx_id": "e888067abbcbe74920013a8a441b9eb683ed9aa6", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 306, - { - "block": 3105636, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "smooth.witness" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-11T13:43:42", - "trx_id": "50e85ae81e53ce8793e03f736eb97ea8162c37da", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 328, - { - "block": 3144657, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "anyx", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-12T22:23:33", - "trx_id": "43f186092b37a9ceab49f4f6369a861709523e11", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 404, - { - "block": 3647042, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "liondani" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T14:17:06", - "trx_id": "9cc490746a6e3449180a22fc3641bd643708bf67", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 405, - { - "block": 3647231, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "anyx" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T14:26:42", - "trx_id": "e1af257db286696d487ee9cf16b3dc29a04a4082", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 406, - { - "block": 3647439, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "roadscape" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T14:37:15", - "trx_id": "a8994fd0ba8aa0f91db19445fb2e07bb73dd28ae", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 423, - { - "block": 3701313, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "riverhead" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-01T11:45:42", - "trx_id": "59d382c45ee0b92e770afa05551a912fc2926285", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 459, - { - "block": 3784027, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "complexring" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-04T08:56:48", - "trx_id": "4a85960e6181f411ff5c5409411b472fa652cb16", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 460, - { - "block": 3784059, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-04T08:58:24", - "trx_id": "d448cf3bbe37ea308d8905ea580b79ff25b3dc43", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 488, - { - "block": 3820266, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "blocktrades", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:28:00", - "trx_id": "58ad1534d3bebbd2597aec774859ec9892457fdb", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 526, - { - "block": 3821908, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "inertia", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T16:50:24", - "trx_id": "a7a65ec3e971eeb4a5bf724513e6fbdbbed08982", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 542, - { - "block": 3823310, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "felixxx", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T18:00:48", - "trx_id": "09609c5bf3b56f0e33081f671dfe9b027e3767b6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 579, - { - "block": 3827447, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "chitty" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T21:29:00", - "trx_id": "e604c679b75357ae92ce86ce3ed5b7bdcf5f3ef5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 580, - { - "block": 3827465, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "chitty", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T21:29:54", - "trx_id": "0dd584b564b868150d6619f0a212ac62fcfb1198", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 664, - { - "block": 3846204, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "dave-mohican", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T13:11:06", - "trx_id": "e2cf917960a80b4c06ccde992c2bd832b2bb0dd7", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 668, - { - "block": 3849814, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "keung0109", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T16:12:03", - "trx_id": "ae32b6cc44f73c0be7c423f2e3c1ddcadfbbb509", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 670, - { - "block": 3850577, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "thebluepanda", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T16:50:15", - "trx_id": "a29d26cabcfdd985c988d0fb4f09f9665d21ea54", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 678, - { - "block": 3853759, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "fydel", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T19:29:45", - "trx_id": "110368b476b60871e9e52df55b642ab0fbb96890", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 679, - { - "block": 3854924, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "heimindanger", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T20:28:09", - "trx_id": "53c39c99441fdd23785e16b512ebec26955ae827", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 682, - { - "block": 3857664, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "geoffrey", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T22:45:45", - "trx_id": "d9f8c3ebbb04d7652dde97027af657429e2c25f7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 689, - { - "block": 3866830, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "steems", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-07T06:25:21", - "trx_id": "7dc8b63a6a828d7712699c5a79ea0b56063ffa45", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 719, - { - "block": 3892188, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "jesus2", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-08T03:38:51", - "trx_id": "9d0c9228771c188fbaa8dfe86551ef9ab53b6021", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 721, - { - "block": 3893448, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "myheadhurts", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-08T04:42:09", - "trx_id": "211f47c061adbac3f7669ac55df68939e350b2ea", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 733, - { - "block": 3902222, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "spaninv", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-08T12:02:06", - "trx_id": "7005646617a7f33cd49c1b1fb04814ce58e9f803", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 756, - { - "block": 3923321, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "geoffrey" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T05:49:03", - "trx_id": "f3103c6232525d7ff40151af618cd8d954c254db", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 774, - { - "block": 3937158, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "dave-hughes", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T17:21:09", - "trx_id": "a088f4aa24e994ac3a99556618d9a02fd4dfd22a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 780, - { - "block": 3940856, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "dan", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T20:29:30", - "trx_id": "9a2b05e8ae3c5494f65e8a9a1be90c5a6f98b9c6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 781, - { - "block": 3940858, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "dantheman", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T20:29:36", - "trx_id": "66e08a863fd1131d66c770bfe39fac1658c55506", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 782, - { - "block": 3941301, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "dashpaymag", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T20:51:48", - "trx_id": "d7e98b40ffc96df685bb8fa4f8a8c79020ef63a0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 783, - { - "block": 3941303, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "steemed", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T20:51:54", - "trx_id": "34e99d1149636597220197a4de7587b461e98eb8", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 899, - { - "block": 3993894, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "pumpkin", - "approve": true, - "witness": "gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T16:48:03", - "trx_id": "d14424769d27d9a1a454c51fcd45baa5850ce7fa", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 984, - { - "block": 4024103, - "op": { - "type": "account_witness_vote_operation", - "value": { - "account": "gtg", - "approve": true, - "witness": "wackou" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-12T18:07:06", - "trx_id": "c705f03051dbae27787c23b8fefe50bb2ccc0164", - "trx_in_block": 2, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/account_witness_vote_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/account_witness_vote_operation.tavern.yaml deleted file mode 100644 index e0b29a0ddec8657af2334761e972070c4d78bfe2..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/account_witness_vote_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to account_witness_vote_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history account_witness_vote_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"gtg","operation_filter_low": 4096,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "account_witness_vote_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/author_reward_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/author_reward_operation.pat.json deleted file mode 100644 index 8ec282b165199b8ea6a18e2d5c36e062f296b586..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/author_reward_operation.pat.json +++ /dev/null @@ -1,199 +0,0 @@ -{ - "history": [ - [ - 18069, - { - "block": 3426457, - "op": { - "type": "author_reward_operation", - "value": { - "author": "xeroc", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "21", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "this-piston-rocks-public-steem-steem-api-for-piston-users-and-developers", - "vesting_payout": { - "amount": "29859881", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-22T20:47:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 18082, - { - "block": 3426576, - "op": { - "type": "author_reward_operation", - "value": { - "author": "xeroc", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "28", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "changelog-python-steem-0-2rc1", - "vesting_payout": { - "amount": "34124528", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-22T20:53:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 18185, - { - "block": 3427233, - "op": { - "type": "author_reward_operation", - "value": { - "author": "xeroc", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "27", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "python-steem-0-1", - "vesting_payout": { - "amount": "34115256", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-22T21:26:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 18603, - { - "block": 3431278, - "op": { - "type": "author_reward_operation", - "value": { - "author": "xeroc", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "17", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "steem-api", - "vesting_payout": { - "amount": "25551869", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-23T00:50:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 18934, - { - "block": 3436603, - "op": { - "type": "author_reward_operation", - "value": { - "author": "xeroc", - "curators_vesting_payout": { - "amount": "833208256", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "1037", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "re-thecryptodrive-steemit-whales-stockpile-sp-government-spending-analogy-and-the-solution-revealed-20160722t051308630z", - "vesting_payout": { - "amount": "1279569822", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-23T05:19:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 278 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/author_reward_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/author_reward_operation.tavern.yaml deleted file mode 100644 index 38c09f5170351d8762dcaf121abcae1788ae2a29..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/author_reward_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api author_reward_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api author_reward_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"xeroc", "operation_filter_low": 562949953421312, "start": 18934, "limit": 5} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "author_reward_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/camilla.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/camilla.pat.json deleted file mode 100644 index 46b47a21074be67d5a6b55bdcaed9827509890eb..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/camilla.pat.json +++ /dev/null @@ -1,60 +0,0 @@ -{ - "history": [ - [ - 0, - { - "block": 1364796, - "op": { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM5weDe4DrNTc1Nrgi3puni6ABCvAiQnAmY2iMZ32PEoFcfLsKJK", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steem", - "fee": { - "amount": "10000", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "", - "memo_key": "STM5G989HCZJMoc6rT5vxEigV8nSMm7t8cGNpFQo8y6neYtXze6fT", - "new_account_name": "camilla", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5YFkx87y8yBz5es3XBDGZ32J9XnExHPTiGSNHWL5mmAoc8adZX", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5nYML8BLcAjEWmNs2e5zvjyDH1WtMGXFH3TAa7WYcGJYhwdKT6", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-11T21:11:09", - "trx_id": "f06f79e658cebfca176eaa7f225376c3ad1174d7", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/camilla.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/camilla.tavern.yaml deleted file mode 100644 index 796e5f67919b1ac77d9e8e162fbe0d500ebf1bfb..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/camilla.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction camilla - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction gtg - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account": "camilla","start": 0,"limit": 1} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "camilla" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/cancel_transfer_from_savings_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/cancel_transfer_from_savings_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/cancel_transfer_from_savings_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/cancel_transfer_from_savings_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/cancel_transfer_from_savings_operation.tavern.yaml deleted file mode 100644 index b9b9aa37fb5e84ba945d415d13ed711f768341b1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/cancel_transfer_from_savings_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api cancel_transfer_from_savings_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api cancel_transfer_from_savings_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 17179869184, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "cancel_transfer_from_savings_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/change_recovery_account_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/change_recovery_account_operation.pat.json deleted file mode 100644 index d52a58465b2bf7d1fe86b6b4aaacd979805127ee..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/change_recovery_account_operation.pat.json +++ /dev/null @@ -1,24 +0,0 @@ -{ - "history": [ - [ - 90, - { - "block": 3291975, - "op": { - "type": "change_recovery_account_operation", - "value": { - "account_to_recover": "barrie", - "extensions": [], - "new_recovery_account": "boombastic" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-18T03:38:33", - "trx_id": "f1a629b702ed5552959f5a629d37314e1da2e80c", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/change_recovery_account_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/change_recovery_account_operation.tavern.yaml deleted file mode 100644 index a9c160864c9d2931d6e21a229fcc126aba96e510..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/change_recovery_account_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api change_recovery_account_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api change_recovery_account_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"barrie", "operation_filter_low": 67108864, "start": 90, "limit": 1} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "change_recovery_account_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/claim_account_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/claim_account_operation.pat.json deleted file mode 100644 index 4002a4b7524d6948f3d27d1941627f5b69c2b7ce..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/claim_account_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/claim_account_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/claim_account_operation.tavern.yaml deleted file mode 100644 index 994b1777aafda2db053f67259ee77bbac29ce410..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/claim_account_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to claim_account_operation - - marks: - - patterntest # no noempty result - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history claim_account_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades","operation_filter_low": 4194304,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "claim_account_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance2_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance2_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance2_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance2_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance2_operation.tavern.yaml deleted file mode 100644 index 198a70046470771e83057cd49dc3875081c6a6a5..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance2_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api claim_reward_balance2_operation - - marks: - - patterntest # no noempty result in 5mln blocks - # only if HIVE_ENABLE_SMT - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api claim_reward_balance2_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 281474976710656, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "claim_reward_balance2_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance_operation.tavern.yaml deleted file mode 100644 index 5bad8f48d42205ae19fc3d958d45b4c829441532..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/claim_reward_balance_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api claim_reward_balance_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api claim_reward_balance_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 549755813888, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "claim_reward_balance_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/clear_null_account_balance_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/clear_null_account_balance_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/clear_null_account_balance_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/clear_null_account_balance_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/clear_null_account_balance_operation.tavern.yaml deleted file mode 100644 index 2757da3f4a8062d3fb94d1f89a3ea1fa3416cb91..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/clear_null_account_balance_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api clear_null_account_balance_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api clear_null_account_balance_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 9223372036854775808, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "clear_null_account_balance_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/comment_benefactor_reward_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/comment_benefactor_reward_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/comment_benefactor_reward_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/comment_benefactor_reward_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/comment_benefactor_reward_operation.tavern.yaml deleted file mode 100644 index 36792c2d69f767d82dfc695742938857a1111881..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/comment_benefactor_reward_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api comment_benefactor_reward_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api comment_benefactor_reward_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 2305843009213693952, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "comment_benefactor_reward_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/comment_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/comment_operation.pat.json deleted file mode 100644 index fcab76e3de0f559b8698d7104381fc6097bb26b0..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/comment_operation.pat.json +++ /dev/null @@ -1,148 +0,0 @@ -{ - "history": [ - [ - 205196, - { - "block": 4982094, - "op": { - "type": "comment_operation", - "value": { - "author": "quigua", - "body": "At first, I couldn't see more than 4 points at the same time. But, then I follow all points with the eyes making a circle pattern during 15 seconds, and surprising!. I can see the 12 points, and now I can not see the blinking points again. That is really crazy...", - "json_metadata": "{\"tags\":[\"optical\"]}", - "parent_author": "blocktrades", - "parent_permlink": "re-ilovesteemit-can-you-see-all-twelve-dots-in-this-image-nope-you-cannot-20160915t034947752z", - "permlink": "re-blocktrades-re-ilovesteemit-can-you-see-all-twelve-dots-in-this-image-nope-you-cannot-20160915t044305573z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T04:43:09", - "trx_id": "40ae1f796b8d0409a799fe62b3b423972b83c9e5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205222, - { - "block": 4982610, - "op": { - "type": "comment_operation", - "value": { - "author": "blocktrades", - "body": "I tried that same pattern, but the most I could see was 4 at a time. So now I wonder, is your brain remembering what it saw and assuming it's still there, or is there enough information that your brain is now better able to interpret what it's seeing in your peripheral vision...", - "json_metadata": "{\"tags\":[\"optical\"]}", - "parent_author": "quigua", - "parent_permlink": "re-blocktrades-re-ilovesteemit-can-you-see-all-twelve-dots-in-this-image-nope-you-cannot-20160915t044305573z", - "permlink": "re-quigua-re-blocktrades-re-ilovesteemit-can-you-see-all-twelve-dots-in-this-image-nope-you-cannot-20160915t050900278z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T05:08:57", - "trx_id": "e363ad2c2c7e8aab96952e772446b177e7ee1624", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205229, - { - "block": 4982731, - "op": { - "type": "comment_operation", - "value": { - "author": "blocktrades", - "body": "Hmm, it may be an actual function of my weak vision. When I get further from the screen, it gets hard for me to see any of the dots. So next I tried to get very close to the screen (normally I keep my monitor pretty far away) and I was able to see about 8 of them at once. I'm guessing having a smaller monitor might help too, based on the article, since I suppose they would be less in our peripheral vision then.", - "json_metadata": "{\"tags\":[\"optical\"]}", - "parent_author": "quigua", - "parent_permlink": "re-blocktrades-re-ilovesteemit-can-you-see-all-twelve-dots-in-this-image-nope-you-cannot-20160915t044305573z", - "permlink": "re-quigua-re-blocktrades-re-ilovesteemit-can-you-see-all-twelve-dots-in-this-image-nope-you-cannot-20160915t051505312z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T05:15:00", - "trx_id": "e1a1c74b9c999a20381d4eafefd3ad0f4467a0cc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205261, - { - "block": 4983394, - "op": { - "type": "comment_operation", - "value": { - "author": "quigua", - "body": "I am not sure, but it is not funny anymore because I see the 12 points every time. Maybe my brain just recorded the pattern and remember it. I can not switch back again.\nAlso is truth that I work with crystal simetry pattern daily, so, maybe my brain is trained to see patterns and keep it. Also, in this occasion, I knew what was looking for...\nThis make me think how different we perceive the universe around us... Even when everybody is seeing the same thing...", - "json_metadata": "{\"tags\":[\"optical\"]}", - "parent_author": "blocktrades", - "parent_permlink": "re-quigua-re-blocktrades-re-ilovesteemit-can-you-see-all-twelve-dots-in-this-image-nope-you-cannot-20160915t050900278z", - "permlink": "re-blocktrades-re-quigua-re-blocktrades-re-ilovesteemit-can-you-see-all-twelve-dots-in-this-image-nope-you-cannot-20160915t054809925z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T05:48:12", - "trx_id": "5824e046a9235be9ba99440e3e8eb99860e8a63a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205902, - { - "block": 4995964, - "op": { - "type": "comment_operation", - "value": { - "author": "piedpiper", - "body": "It just occurred to me that witness updates like this could make for interesting video material. Maybe a segment in our SteemSmart show?", - "json_metadata": "{\"tags\":[\"witness-category\"]}", - "parent_author": "blocktrades", - "parent_permlink": "witness-report-for-blocktrades-for-last-week-of-august", - "permlink": "re-blocktrades-witness-report-for-blocktrades-for-last-week-of-august-20160915t161707257z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T16:18:12", - "trx_id": "3ae419f4d37860c7d2dabec530ccf3ad79e79784", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 206145, - { - "block": 4999840, - "op": { - "type": "comment_operation", - "value": { - "author": "blocktrades", - "body": "When will the t-shirt ship?", - "json_metadata": "{\"tags\":[\"steem\"]}", - "parent_author": "cryptojoy.com", - "parent_permlink": "help-steem-buy-steemit-apparel-day-2", - "permlink": "re-cryptojoycom-help-steem-buy-steemit-apparel-day-2-20160915t193858376z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T19:38:48", - "trx_id": "ef94aba27f9875d6da33b26dae44ee63f06f89a2", - "trx_in_block": 1, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/comment_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/comment_operation.tavern.yaml deleted file mode 100644 index ddb7c367e29713e6224dc4d386fe7acfb2547577..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/comment_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to comment_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history comment_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades","operation_filter_low": 2, "start": 206145, "limit": 6} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "comment_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/comment_options_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/comment_options_operation.pat.json deleted file mode 100644 index de1a6095b693887e5dbf3544abce160113dba49a..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/comment_options_operation.pat.json +++ /dev/null @@ -1,116 +0,0 @@ -{ - "history": [ - [ - 723, - { - "block": 4973993, - "op": { - "type": "comment_options_operation", - "value": { - "allow_curation_rewards": true, - "allow_votes": true, - "author": "djangothegod", - "extensions": [], - "max_accepted_payout": { - "amount": "1000000000", - "nai": "@@000000013", - "precision": 3 - }, - "percent_hbd": 0, - "permlink": "i-did-mean-it-in-no-bad-way" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T21:57:48", - "trx_id": "3ca1ef0fc0d035222245327ef524e724bc5d7de5", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 789, - { - "block": 4987662, - "op": { - "type": "comment_options_operation", - "value": { - "allow_curation_rewards": true, - "allow_votes": true, - "author": "djangothegod", - "extensions": [], - "max_accepted_payout": { - "amount": "1000000000", - "nai": "@@000000013", - "precision": 3 - }, - "percent_hbd": 0, - "permlink": "we-haven-t-been-able-to-grow" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T09:22:24", - "trx_id": "10a97c80d2b757d6215f56740548360b87171b3b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 795, - { - "block": 4988323, - "op": { - "type": "comment_options_operation", - "value": { - "allow_curation_rewards": true, - "allow_votes": true, - "author": "djangothegod", - "extensions": [], - "max_accepted_payout": { - "amount": "1000000000", - "nai": "@@000000013", - "precision": 3 - }, - "percent_hbd": 0, - "permlink": "you-want-godzilla-roll" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T09:55:45", - "trx_id": "ec31328dd62df34b8d3f934a94064dd7a9003da0", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 808, - { - "block": 4989612, - "op": { - "type": "comment_options_operation", - "value": { - "allow_curation_rewards": true, - "allow_votes": true, - "author": "djangothegod", - "extensions": [], - "max_accepted_payout": { - "amount": "1000000000", - "nai": "@@000000013", - "precision": 3 - }, - "percent_hbd": 0, - "permlink": "high-rollers-influencing-california-s-ballot" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T11:00:15", - "trx_id": "8f232a3f417e916bf1dd0890db1f23e1c8b6e505", - "trx_in_block": 3, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/comment_options_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/comment_options_operation.tavern.yaml deleted file mode 100644 index 894aeb5178bcda228be9efec8224eaa07576321b..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/comment_options_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to comment_options_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history comment_options_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"djangothegod","operation_filter_low": 524288,"start": 808,"limit": 4} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "comment_options_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/comment_payout_update_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/comment_payout_update_operation.pat.json deleted file mode 100644 index 9a1dd0e6825940c7ce0d932da0ca04a7cb49905f..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/comment_payout_update_operation.pat.json +++ /dev/null @@ -1,118 +0,0 @@ -{ - "history": [ - [ - 3338, - { - "block": 4636165, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "gtg", - "permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T03:24:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 12 - } - ], - [ - 3339, - { - "block": 4636165, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "gtg", - "permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160805t184622691z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T03:24:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 3510, - { - "block": 4695861, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-05T05:17:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 3841, - { - "block": 4825192, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "gtg", - "permlink": "re-wackou-btstools-development-bounties-20160811t173426180z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T17:18:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 15 - } - ], - [ - 3907, - { - "block": 4854936, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "gtg", - "permlink": "re-heretickitten-re-cheetah-cheetah-bot-explained-20160811t093103978z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T18:15:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 41 - } - ], - [ - 4218, - { - "block": 4984966, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "gtg", - "permlink": "re-liondani-don-t-remove-curation-rewards-just-stop-them-when-the-post-reach-the-trending-page-20160815t123329115z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T07:07:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 22 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/comment_payout_update_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/comment_payout_update_operation.tavern.yaml deleted file mode 100644 index 6b9c436282dbfc00052893587c7e6397a5021707..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/comment_payout_update_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api comment_payout_update_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api comment_payout_update_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"gtg", "operation_filter_low": 576460752303423488, "start": 4218, "limit": 6} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "comment_payout_update_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/comment_reward_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/comment_reward_operation.pat.json deleted file mode 100644 index 35ff3b03c44d821e875878a97ed8cf6cf98ed228..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/comment_reward_operation.pat.json +++ /dev/null @@ -1,204 +0,0 @@ -{ - "history": [ - [ - 18070, - { - "block": 3426457, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "xeroc", - "author_rewards": 13, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "388196", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "45", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "this-piston-rocks-public-steem-steem-api-for-piston-users-and-developers", - "total_payout_value": { - "amount": "967021", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-22T20:47:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 18083, - { - "block": 3426576, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "xeroc", - "author_rewards": 16, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "1595", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "56", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "changelog-python-steem-0-2rc1", - "total_payout_value": { - "amount": "11630", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-22T20:53:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 18186, - { - "block": 3427233, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "xeroc", - "author_rewards": 16, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "63453", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "55", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "python-steem-0-1", - "total_payout_value": { - "amount": "63510", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-22T21:26:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 18604, - { - "block": 3431278, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "xeroc", - "author_rewards": 11, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "493", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "38", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "steem-api", - "total_payout_value": { - "amount": "2135", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-23T00:50:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 18935, - { - "block": 3436603, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "xeroc", - "author_rewards": 601, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "677", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "2755", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-thecryptodrive-steemit-whales-stockpile-sp-government-spending-analogy-and-the-solution-revealed-20160722t051308630z", - "total_payout_value": { - "amount": "2077", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-23T05:19:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 279 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/comment_reward_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/comment_reward_operation.tavern.yaml deleted file mode 100644 index 0faa01656633a20f63f1f9f31b504ef13a02ba1d..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/comment_reward_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api comment_reward_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api comment_reward_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"xeroc", "operation_filter_low": 2251799813685248, "start": 18935, "limit": 5} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "comment_reward_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/consolidate_treasury_balance_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/consolidate_treasury_balance_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/consolidate_treasury_balance_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/consolidate_treasury_balance_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/consolidate_treasury_balance_operation.tavern.yaml deleted file mode 100644 index 189a43eb17a799c22c38c140c1f6176e3a58c57d..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/consolidate_treasury_balance_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api consolidate_treasury_balance_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api consolidate_treasury_balance_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_high": 32, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "consolidate_treasury_balance_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/convert_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/convert_operation.pat.json deleted file mode 100644 index aca29bea7549800d4751de04d7bd799de127971b..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/convert_operation.pat.json +++ /dev/null @@ -1,52 +0,0 @@ -{ - "history": [ - [ - 188, - { - "block": 2912870, - "op": { - "type": "convert_operation", - "value": { - "amount": { - "amount": "127144", - "nai": "@@000000013", - "precision": 3 - }, - "owner": "gtg", - "requestid": 1467663446 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T20:18:00", - "trx_id": "8930ea36f61aef6fa7c5c79922cc9892f2d7373b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 189, - { - "block": 2912956, - "op": { - "type": "convert_operation", - "value": { - "amount": { - "amount": "2", - "nai": "@@000000013", - "precision": 3 - }, - "owner": "gtg", - "requestid": 1467663726 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T20:22:18", - "trx_id": "804339e4617544b47018e165d7a8ed45ac38f002", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/convert_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/convert_operation.tavern.yaml deleted file mode 100644 index dceaa42e6202ba13d06fc338b17e069cd4c5454c..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/convert_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to convert_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history convert_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"gtg","operation_filter_low": 256,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "convert_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/create_claimed_account_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/create_claimed_account_operation.pat.json deleted file mode 100644 index 4002a4b7524d6948f3d27d1941627f5b69c2b7ce..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/create_claimed_account_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/create_claimed_account_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/create_claimed_account_operation.tavern.yaml deleted file mode 100644 index 7d33a9eacb13ad6b3f1857f00ca33e2cfb111b5b..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/create_claimed_account_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to create_claimed_account_operation - - marks: - - patterntest # no noempty result - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history create_claimed_account_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades","operation_filter_low": 8388608,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "create_claimed_account_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/create_proposal_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/create_proposal_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/create_proposal_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/create_proposal_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/create_proposal_operation.tavern.yaml deleted file mode 100644 index a8ddafcf14aeb0ae182fa222c989e93aa209f7c0..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/create_proposal_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api create_proposal_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api create_proposal_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 17592186044416, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "create_proposal_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/curation_reward_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/curation_reward_operation.pat.json deleted file mode 100644 index ec7e3bdabb84a2deee803eac46af2952198a0f9d..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/curation_reward_operation.pat.json +++ /dev/null @@ -1,329 +0,0 @@ -{ - "history": [ - [ - 3220, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "abit", - "comment_permlink": "abit-first-post", - "curator": "adm", - "reward": { - "amount": "3386877857", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 198 - } - ], - [ - 3221, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "abit", - "comment_permlink": "abit-witness-post", - "curator": "adm", - "reward": { - "amount": "36810703386", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 205 - } - ], - [ - 3222, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "abit", - "comment_permlink": "abit-witness-post-re3", - "curator": "adm", - "reward": { - "amount": "3659084423", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 217 - } - ], - [ - 3223, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "abit", - "comment_permlink": "abit-witness-post-re3-re1", - "curator": "adm", - "reward": { - "amount": "335023466", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 222 - } - ], - [ - 3224, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "abit", - "comment_permlink": "come-on-miners", - "curator": "adm", - "reward": { - "amount": "3454929498", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 259 - } - ], - [ - 3225, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "abit", - "comment_permlink": "re-masteryoda-test-post-test-20160612t105535643z", - "curator": "adm", - "reward": { - "amount": "3821361415", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 10155 - } - ], - [ - 3226, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "mummyimperfect", - "comment_permlink": "8-weeks-ive-become-one-with-the-sofa-9th-jan-2016", - "curator": "adm", - "reward": { - "amount": "942253499", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 33569 - } - ], - [ - 3227, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "gavvet", - "comment_permlink": "how-to-defeat-a-bot-add-a-little-chaos", - "curator": "adm", - "reward": { - "amount": "34277088420", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 40076 - } - ], - [ - 3228, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alyssas", - "comment_permlink": "re-liondani-re-alyssas-re-abit-ned-started-voting-randomly-on-new-posts-20160602t152250745z", - "curator": "adm", - "reward": { - "amount": "3821361415", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 40206 - } - ], - [ - 3229, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "oholiab", - "comment_permlink": "vrhackspace-proof-of-concept", - "curator": "adm", - "reward": { - "amount": "1245868516", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 40222 - } - ], - [ - 3230, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "nanzo-scoop", - "comment_permlink": "an-idiots-to-what-the-dollar-figure-against-your-post-means-i-think", - "curator": "adm", - "reward": { - "amount": "5250445889", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 41012 - } - ], - [ - 3231, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "gregory-f", - "comment_permlink": "clueless-users-guide-to-upgrading-to-version-0", - "curator": "adm", - "reward": { - "amount": "25456548715", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 50607 - } - ], - [ - 3232, - { - "block": 2889020, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "liondani", - "comment_permlink": "re-tuck-fheman-re-galina-hello-im-galina-and-you--20160610t154341363z", - "curator": "adm", - "reward": { - "amount": "3774248740", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T00:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1733 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/curation_reward_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/curation_reward_operation.tavern.yaml deleted file mode 100644 index d729c186465d577e777c283857eb7fc10218692c..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/curation_reward_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api curation_reward_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api curation_reward_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"adm", "operation_filter_low": 1125899906842624, "start": 3232, "limit": 13} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "curation_reward_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/custom_binary_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/custom_binary_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/custom_binary_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/custom_binary_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/custom_binary_operation.tavern.yaml deleted file mode 100644 index f658c42c3babd6e79c261b2001230ce078ebb800..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/custom_binary_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api custom_binary_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api custom_binary_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 34359738368, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "custom_binary_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/custom_json_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/custom_json_operation.pat.json deleted file mode 100644 index 87b2b412134c727cfe2732d47ab510939ffb0cb7..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/custom_json_operation.pat.json +++ /dev/null @@ -1,372 +0,0 @@ -{ - "history": [ - [ - 2960, - { - "block": 3537570, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"zajac\",\"what\":[\"blog\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-26T18:22:27", - "trx_id": "50e456c7203377d2d6bd014f23a03fd3351031f6", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 2961, - { - "block": 3537571, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"zajac\",\"what\":[]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-26T18:22:30", - "trx_id": "927585031e241a615610113ebc472e047d3502b7", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 2962, - { - "block": 3545428, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"jeeves\",\"what\":[\"ignore\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-27T00:58:42", - "trx_id": "54b35eac1d53ae1f8d2d62f5aa6ee90d74f93255", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 2977, - { - "block": 3574031, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"fyrstikken\",\"what\":[\"blog\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-28T01:02:36", - "trx_id": "3457be9f1ada8caaa36fe1c9eb3061bb6a2c6d62", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 3027, - { - "block": 3624783, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"wang\",\"what\":[\"ignore\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-29T19:34:42", - "trx_id": "d9943676e099ef450c7a326ec93d68fde09cd53f", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 3028, - { - "block": 3624784, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"weenis\",\"what\":[\"ignore\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-29T19:34:45", - "trx_id": "4b70eeb996a2433a6066ef46c41e287b1047f456", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 3029, - { - "block": 3625087, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"weenis\",\"what\":[]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-29T19:49:54", - "trx_id": "d3d703d18f0f619123ce911c70b4943c675ad83d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 3030, - { - "block": 3625088, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"weenis\",\"what\":[\"ignore\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-29T19:49:57", - "trx_id": "5115597f2612f8ca7d0b2dc30449a58c3100f477", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 3031, - { - "block": 3625281, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"koolaidssss\",\"what\":[\"ignore\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-29T19:59:45", - "trx_id": "8032225983f889c56a40134e06638654c7561018", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 3054, - { - "block": 3645302, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"jeeves\",\"what\":[]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T12:49:09", - "trx_id": "2413cf4372d67b4e4a09e6a29f1441661b736df6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 3055, - { - "block": 3645312, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"jeeves\",\"what\":[\"ignore\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T12:49:39", - "trx_id": "2c0b6580226864da6ccf3b89ca2bc690c9437395", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 3147, - { - "block": 3674919, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"msjennifer\",\"what\":[\"ignore\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-31T13:40:45", - "trx_id": "154cb33ac72c82aba146bd21ffafd3e676be9fa9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 3284, - { - "block": 3764002, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"steemitblog\",\"what\":[\"blog\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-03T16:11:30", - "trx_id": "cdd14fa4a82a6d63330cb43d51bdb884659e1160", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 3318, - { - "block": 3909295, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"falkvinge\",\"what\":[\"blog\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-08T18:01:09", - "trx_id": "1179856faa82f46643a912d9b279b9f61fe4e566", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 3356, - { - "block": 4001223, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "{\"follower\":\"jsc\",\"following\":\"williambanks\",\"what\":[\"blog\"]}", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T23:01:24", - "trx_id": "4756949250f9db61a531f3c2e95abf0eb8ce5b19", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 3590, - { - "block": 4595203, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"jsc\",\"following\":\"jamesc\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "jsc" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-01T17:08:00", - "trx_id": "2fd47d659c7ec04554fa08a13397802277e41596", - "trx_in_block": 4, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/custom_json_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/custom_json_operation.tavern.yaml deleted file mode 100644 index 9c13f077342cd58a8068a844ae483cffd2b07348..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/custom_json_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to custom_json_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history custom_json_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"jsc","operation_filter_low": 262144,"start": 3590,"limit": 16} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "custom_json_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/custom_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/custom_operation.pat.json deleted file mode 100644 index 59766c5fd28a7eb8a249c3b5404f5c91fee05cc1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/custom_operation.pat.json +++ /dev/null @@ -1,92 +0,0 @@ -{ - "history": [ - [ - 21, - { - "block": 1297952, - "op": { - "type": "custom_operation", - "value": { - "data": "0a627974656d617374657207737465656d697402a3d13897d82114466ad87a74b73a53292d8331d1bd1d3082da6bfbcff19ed097029db013797711c88cccca3692407f9ff9b9ce7221aaa2d797f1692be2215d0a5f6d2a8cab6832050078bc5729201e3ea24ea9f7873e6dbdc65a6bd9899053b9acda876dc69f11a13df9ca8b26b6", - "id": 777, - "required_auths": [ - "bytemaster" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T13:20:18", - "trx_id": "539b047b2217bec87fb970dc2b329d1a92c1bfd4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22, - { - "block": 1298523, - "op": { - "type": "custom_operation", - "value": { - "data": "0a627974656d617374657207737465656d697402a3d13897d82114466ad87a74b73a53292d8331d1bd1d3082da6bfbcff19ed097029db013797711c88cccca3692407f9ff9b9ce7221aaa2d797f1692be2215d0a5f3f639c116932050042b5d8d72001a6879ed30b5804308b05b4b92e40c5bd46833d6cf6b17328cedd32c928db8f", - "id": 777, - "required_auths": [ - "bytemaster" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T13:48:51", - "trx_id": "0c7f96affd69e51858e181fe665e8313a99bcc45", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 25, - { - "block": 1305110, - "op": { - "type": "custom_operation", - "value": { - "data": "0a627974656d617374657207737465656d697402a3d13897d82114466ad87a74b73a53292d8331d1bd1d3082da6bfbcff19ed097029db013797711c88cccca3692407f9ff9b9ce7221aaa2d797f1692be2215d0a5f607de8b06d3205000ff825a32029a2df80c7cc67d0179fc54d87e4d795f4209b8aeebc93ada0fce7092f92b6d8", - "id": 777, - "required_auths": [ - "bytemaster" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T19:19:42", - "trx_id": "4b727871d1863ac8246d4a09541ac68ee5fdeda8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 26, - { - "block": 1305332, - "op": { - "type": "custom_operation", - "value": { - "data": "0a627974656d617374657207737465656d697402a3d13897d82114466ad87a74b73a53292d8331d1bd1d3082da6bfbcff19ed097029db013797711c88cccca3692407f9ff9b9ce7221aaa2d797f1692be2215d0a5fdf7185d86d320500155a3d622035b791e5964910159aed5e613401141350bd8a95efa4cd98e2119dddc40c63ee", - "id": 777, - "required_auths": [ - "bytemaster" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T19:30:48", - "trx_id": "2fe833d74beff51bced121434d0ecaef783acb76", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/custom_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/custom_operation.tavern.yaml deleted file mode 100644 index 99c78f3ee5c32c7ef7ba8166d4173678c0970627..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/custom_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to custom_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history custom_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"bytemaster","operation_filter_low": 32768,"start": 8000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "custom_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/decline_voting_rights_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/decline_voting_rights_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/decline_voting_rights_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/decline_voting_rights_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/decline_voting_rights_operation.tavern.yaml deleted file mode 100644 index edfd7223fc6673c3dd9a9f7f819ca6001d52c8c5..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/decline_voting_rights_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api decline_voting_rights_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api decline_voting_rights_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 68719476736, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "decline_voting_rights_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/default_val.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/default_val.pat.json deleted file mode 100644 index 59aab7691513e749fba5588f8ed3088a7c3eb413..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/default_val.pat.json +++ /dev/null @@ -1,24474 +0,0 @@ -{ - "history": [ - [ - 21420, - { - "block": 4636980, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "voter": "ihashfury", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T04:04:51", - "trx_id": "f5b6ee9b862a31f6f634175bebbfa6bffbbee4b5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21421, - { - "block": 4638421, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "553", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "rshares": "32643849525", - "total_vote_weight": "3829413232259789320", - "voter": "ihash", - "weight": "95142689658372277" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T05:16:57", - "trx_id": "a72737d1495f7c844dcb83f7e6f3f8980caaaafa", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21422, - { - "block": 4638421, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "voter": "ihash", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T05:16:57", - "trx_id": "a72737d1495f7c844dcb83f7e6f3f8980caaaafa", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21423, - { - "block": 4641586, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "mammasitta", - "pending_payout": { - "amount": "47513", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "kaffeehaus-culture-the-viennese-coffee-house-an-intangible-cultural-heritage-and-the-secession-an-artist-movement", - "rshares": "164730268571", - "total_vote_weight": "15264579291642129899", - "voter": "camilla", - "weight": "22768549144487203" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T07:55:27", - "trx_id": "815b2329001e75d120386be8367aae62d04ac25c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21424, - { - "block": 4641586, - "op": { - "type": "vote_operation", - "value": { - "author": "mammasitta", - "permlink": "kaffeehaus-culture-the-viennese-coffee-house-an-intangible-cultural-heritage-and-the-secession-an-artist-movement", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T07:55:27", - "trx_id": "815b2329001e75d120386be8367aae62d04ac25c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21425, - { - "block": 4641650, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "juliac", - "pending_payout": { - "amount": "76", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "mythical-creature-original-artwork", - "rshares": "164730268571", - "total_vote_weight": "763455956958478835", - "voter": "camilla", - "weight": "243476636684815717" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T07:58:39", - "trx_id": "edea19cfe8aebffbf02ad36164a9bea2c7414170", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21426, - { - "block": 4641650, - "op": { - "type": "vote_operation", - "value": { - "author": "juliac", - "permlink": "mythical-creature-original-artwork", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T07:58:39", - "trx_id": "edea19cfe8aebffbf02ad36164a9bea2c7414170", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21427, - { - "block": 4641682, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "ndbeledrifts", - "pending_payout": { - "amount": "93", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "i-met-a-girl-from-germany-once", - "rshares": "164730268571", - "total_vote_weight": "919116914030368039", - "voter": "camilla", - "weight": "713798441564533819" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:00:15", - "trx_id": "7d0ec7b9ca709d0c4ef6c320efb39367b86ee22f", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21428, - { - "block": 4641682, - "op": { - "type": "vote_operation", - "value": { - "author": "ndbeledrifts", - "permlink": "i-met-a-girl-from-germany-once", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:00:15", - "trx_id": "7d0ec7b9ca709d0c4ef6c320efb39367b86ee22f", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21429, - { - "block": 4641717, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "alexbeyman", - "pending_payout": { - "amount": "34999", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "psychedelics-the-beautiful-bossy-dryad-my-encounters-with-forest-intelligence", - "rshares": "164730268571", - "total_vote_weight": "14802732646898194280", - "voter": "camilla", - "weight": "29888265851259884" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:02:00", - "trx_id": "6658deb8e94aad374df3c9005bec6c26eee12728", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21430, - { - "block": 4641717, - "op": { - "type": "vote_operation", - "value": { - "author": "alexbeyman", - "permlink": "psychedelics-the-beautiful-bossy-dryad-my-encounters-with-forest-intelligence", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:02:00", - "trx_id": "6658deb8e94aad374df3c9005bec6c26eee12728", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21431, - { - "block": 4641731, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "opheliafu", - "pending_payout": { - "amount": "617618", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "i-was-a-different-person-yesterday", - "rshares": "160506415531", - "total_vote_weight": "17503335622713708578", - "voter": "camilla", - "weight": "1940011779435887" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:02:42", - "trx_id": "0dc66c2630085e6793cbc607a3d15c1cd10e5e96", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21432, - { - "block": 4641731, - "op": { - "type": "vote_operation", - "value": { - "author": "opheliafu", - "permlink": "i-was-a-different-person-yesterday", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:02:42", - "trx_id": "0dc66c2630085e6793cbc607a3d15c1cd10e5e96", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21433, - { - "block": 4642714, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "586", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "rshares": "63316513317", - "total_vote_weight": "4010488821265777010", - "voter": "jason", - "weight": "181075589005987690" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:51:51", - "trx_id": "3314d662a860b169ba84ce9b4c4bcc542598da93", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21434, - { - "block": 4642714, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "voter": "jason", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:51:51", - "trx_id": "3314d662a860b169ba84ce9b4c4bcc542598da93", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21435, - { - "block": 4643114, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "opheliafu", - "pending_payout": { - "amount": "44433", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "say-hello-to-big-bob", - "rshares": "164730268571", - "total_vote_weight": "15228331304238682767", - "voter": "camilla", - "weight": "23292114192878337" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:11:54", - "trx_id": "f1d33cf9b8f051a2350957ed75fa72d431919c4b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21436, - { - "block": 4643114, - "op": { - "type": "vote_operation", - "value": { - "author": "opheliafu", - "permlink": "say-hello-to-big-bob", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:11:54", - "trx_id": "f1d33cf9b8f051a2350957ed75fa72d431919c4b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21437, - { - "block": 4643118, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "127675", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "pura-vida-my-experience-working-on-a-permaculture-farm-in-costa-rica", - "rshares": "206968798974", - "total_vote_weight": "16472049860403995460", - "voter": "camilla", - "weight": "10998591732923581" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:12:06", - "trx_id": "f51caa9d51dc7db146b392848e03863c2e32b209", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21438, - { - "block": 4643118, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "pura-vida-my-experience-working-on-a-permaculture-farm-in-costa-rica", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:12:06", - "trx_id": "f51caa9d51dc7db146b392848e03863c2e32b209", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21439, - { - "block": 4643121, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "89", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-sustainable-food-dialogue-an-overview-of-vertical-farming", - "rshares": "206968798974", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:12:15", - "trx_id": "9ce855db47f09bf5b3d72b12be94aecd9bfc2ad7", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21440, - { - "block": 4643121, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "a-sustainable-food-dialogue-an-overview-of-vertical-farming", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:12:15", - "trx_id": "9ce855db47f09bf5b3d72b12be94aecd9bfc2ad7", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21441, - { - "block": 4643140, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "allasyummyfood", - "pending_payout": { - "amount": "25821", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "alla-goes-to-usa-part-1-san-francisco", - "rshares": "206968798974", - "total_vote_weight": "14329012925793520767", - "voter": "camilla", - "weight": "47797748458381905" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:13:12", - "trx_id": "ced73ce0a0918a9d113b69c9c1b5ddcaaab271be", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21442, - { - "block": 4643140, - "op": { - "type": "vote_operation", - "value": { - "author": "allasyummyfood", - "permlink": "alla-goes-to-usa-part-1-san-francisco", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:13:12", - "trx_id": "ced73ce0a0918a9d113b69c9c1b5ddcaaab271be", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21443, - { - "block": 4643156, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "allasyummyfood", - "pending_payout": { - "amount": "120", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "russian-napoleon-cake-recipe-steemit-food-challenge", - "rshares": "206968798974", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:14:00", - "trx_id": "b262fc00a8a5ac932dbb2c57127480913ca45cc9", - "trx_in_block": 11, - "virtual_op": 1 - } - ], - [ - 21444, - { - "block": 4643156, - "op": { - "type": "vote_operation", - "value": { - "author": "allasyummyfood", - "permlink": "russian-napoleon-cake-recipe-steemit-food-challenge", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:14:00", - "trx_id": "b262fc00a8a5ac932dbb2c57127480913ca45cc9", - "trx_in_block": 11, - "virtual_op": 0 - } - ], - [ - 21445, - { - "block": 4643207, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "162", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "anwen-meditates-a-steemit-health-channel-11-yoga-poses-for-digestion-plus-fresh-squeezed-pear-juice", - "rshares": "202744945934", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:16:33", - "trx_id": "9bb95156c7ca92d056b763a9318934717dabc521", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21446, - { - "block": 4643207, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "anwen-meditates-a-steemit-health-channel-11-yoga-poses-for-digestion-plus-fresh-squeezed-pear-juice", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:16:33", - "trx_id": "9bb95156c7ca92d056b763a9318934717dabc521", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21447, - { - "block": 4647979, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "anca3drandom", - "comment_permlink": "steemart-the-art-of-quilling-paper-steemit-logo", - "curator": "camilla", - "reward": { - "amount": "80336806", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T13:15:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 38 - } - ], - [ - 21448, - { - "block": 4648623, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "7", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-alexft-re-camilla-the-castle-from-my-dreams-20160830t141415563z", - "rshares": "17043021152", - "total_vote_weight": 0, - "voter": "fury", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T13:47:45", - "trx_id": "9cda2a9835a6c967a50be5553e5efb2b1c3ac9a7", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21449, - { - "block": 4648623, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-alexft-re-camilla-the-castle-from-my-dreams-20160830t141415563z", - "voter": "fury", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T13:47:45", - "trx_id": "9cda2a9835a6c967a50be5553e5efb2b1c3ac9a7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21450, - { - "block": 4650309, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "favorit", - "comment_permlink": "enchanted-forest", - "curator": "camilla", - "reward": { - "amount": "77087879", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T15:12:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21451, - { - "block": 4650600, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "dabaisha", - "comment_permlink": "7-major-anti-inflammatory-food-eating-a-healthy-save-beer-belly", - "curator": "camilla", - "reward": { - "amount": "80295266", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T15:26:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 21452, - { - "block": 4650906, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "mrlee", - "comment_permlink": "beautiful-macarons", - "curator": "camilla", - "reward": { - "amount": "80290443", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T15:42:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 21453, - { - "block": 4651096, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "tanyabtc", - "comment_permlink": "dragon-ferocious", - "curator": "camilla", - "reward": { - "amount": "80287412", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T15:51:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 15 - } - ], - [ - 21454, - { - "block": 4651244, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "jpiper20", - "comment_permlink": "hand-drawn-typography-from-this-week", - "curator": "camilla", - "reward": { - "amount": "105976313", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T15:58:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 28 - } - ], - [ - 21455, - { - "block": 4653163, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "storyseeker", - "comment_permlink": "my-process-step-by-step-start-to-finish-intricate-collage-and-acrylic-painting", - "curator": "camilla", - "reward": { - "amount": "4118671564", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T17:35:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 21456, - { - "block": 4653405, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "587", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "rshares": 3820934326, - "total_vote_weight": "4021272685842403703", - "voter": "sompitonov", - "weight": "10783864576626693" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T17:47:09", - "trx_id": "50186445bdd28b5086ce61de163cd827cecbef20", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21457, - { - "block": 4653405, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "voter": "sompitonov", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T17:47:09", - "trx_id": "50186445bdd28b5086ce61de163cd827cecbef20", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21458, - { - "block": 4655414, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "opheliafu", - "comment_permlink": "i-was-a-different-person-yesterday", - "curator": "camilla", - "reward": { - "amount": "60966556", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T19:27:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 54 - } - ], - [ - 21459, - { - "block": 4656578, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "yangyang", - "comment_permlink": "mermaid-drawing", - "curator": "camilla", - "reward": { - "amount": "285514715", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T20:26:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21460, - { - "block": 4657501, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alexbeyman", - "comment_permlink": "psychedelics-the-beautiful-bossy-dryad-my-encounters-with-forest-intelligence", - "curator": "camilla", - "reward": { - "amount": "60941504", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T21:12:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 17 - } - ], - [ - 21461, - { - "block": 4663596, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "allasyummyfood", - "comment_permlink": "alla-goes-to-usa-part-1-san-francisco", - "curator": "camilla", - "reward": { - "amount": "108922513", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T02:17:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 29 - } - ], - [ - 21462, - { - "block": 4669230, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "mammasitta", - "comment_permlink": "kaffeehaus-culture-the-viennese-coffee-house-an-intangible-cultural-heritage-and-the-secession-an-artist-movement", - "curator": "camilla", - "reward": { - "amount": "70401302", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T06:59:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 21463, - { - "block": 4669469, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "ndbeledrifts", - "comment_permlink": "i-met-a-girl-from-germany-once", - "curator": "camilla", - "reward": { - "amount": "63998182", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T07:11:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21464, - { - "block": 4671716, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "juliac", - "comment_permlink": "mythical-creature-original-artwork", - "curator": "camilla", - "reward": { - "amount": "422201508", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T09:04:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21465, - { - "block": 4672446, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "anwenbaumeister", - "comment_permlink": "pura-vida-my-experience-working-on-a-permaculture-farm-in-costa-rica", - "curator": "camilla", - "reward": { - "amount": "313408097", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T09:41:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 42 - } - ], - [ - 21466, - { - "block": 4673465, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "opheliafu", - "comment_permlink": "say-hello-to-big-bob", - "curator": "camilla", - "reward": { - "amount": "687441625", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T10:34:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 18 - } - ], - [ - 21467, - { - "block": 4675025, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "82", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colours-of-a-peacock", - "rshares": "186528421973", - "total_vote_weight": 0, - "voter": "jamielefay", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T11:54:24", - "trx_id": "78e39e4fd8a59c73058aabb3112230750d460460", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21468, - { - "block": 4675025, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colours-of-a-peacock", - "voter": "jamielefay", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T11:54:24", - "trx_id": "78e39e4fd8a59c73058aabb3112230750d460460", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21469, - { - "block": 4675057, - "op": { - "type": "comment_operation", - "value": { - "author": "jamielefay", - "body": "Soooo beautiful. All my favourite colours. Hope you post more art soon. J.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colours-of-a-peacock", - "permlink": "re-camilla-colours-of-a-peacock-20160904t115600052z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T11:56:00", - "trx_id": "9aefb0b8dd9fde1e3ccbbeca7b896b381e5213a5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21470, - { - "block": 4681217, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-pixielolz-can-steemit-take-some-fanart--20160807t105639754z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T17:04:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 15 - } - ], - [ - 21471, - { - "block": 4681217, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "pixielolz", - "comment_permlink": "re-camilla-re-pixielolz-can-steemit-take-some-fanart--20160807t183600081z", - "curator": "camilla", - "reward": { - "amount": "76621057", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T17:04:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 21472, - { - "block": 4694018, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "274", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "the-magic-world-of-books", - "vesting_payout": { - "amount": "1031792689", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-05T03:45:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21473, - { - "block": 4694018, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 647, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "123", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "549", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-magic-world-of-books", - "total_payout_value": { - "amount": "2309", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-05T03:45:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 21474, - { - "block": 4694018, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "the-magic-world-of-books" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-05T03:45:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21475, - { - "block": 4694018, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alexft", - "comment_permlink": "re-camilla-the-magic-world-of-books-20160809t081535352z", - "curator": "camilla", - "reward": { - "amount": "76429088", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-05T03:45:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 11 - } - ], - [ - 21476, - { - "block": 4724362, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-inkha-when-i-see-my-steem-friends-are-active-but-haven-t-upvoted-my-new-post-yet-20160806t170640062z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:05:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 21477, - { - "block": 4725117, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "crazy-flood-on-the-subway-tracks" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21478, - { - "block": 4725117, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-xapo-re-camilla-crazy-flood-on-the-subway-tracks-20160806t171143178z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21479, - { - "block": 4725117, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-gidlark-re-camilla-crazy-flood-on-the-subway-tracks-20160806t212819558z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 21480, - { - "block": 4725117, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-btcbtcbtc20155-re-camilla-crazy-flood-on-the-subway-tracks-20160806t212932042z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 9 - } - ], - [ - 21481, - { - "block": 4725117, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alexft", - "comment_permlink": "re-camilla-crazy-flood-on-the-subway-tracks-20160807t062503313z", - "curator": "camilla", - "reward": { - "amount": "79130255", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 10 - } - ], - [ - 21482, - { - "block": 4725117, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "joelinux", - "comment_permlink": "re-camilla-crazy-flood-on-the-subway-tracks-20160810t164432985z", - "curator": "camilla", - "reward": { - "amount": "82295466", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 21483, - { - "block": 4727278, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-sphenix-malaysia-food-chinese-food-wan-ton-mee-another-hong-kong-style-20160806t170453956z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:31:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 21484, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "my-ladybug-drawing" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21485, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-saharov-re-camilla-my-ladybug-drawing-20160806t130140608z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21486, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-btcbtcbtc20155-re-camilla-my-ladybug-drawing-20160806t131159046z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 9 - } - ], - [ - 21487, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-camilla-re-btcbtcbtc20155-re-camilla-my-ladybug-drawing-20160806t131222091z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 10 - } - ], - [ - 21488, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-mun-re-camilla-my-ladybug-drawing-20160806t131509504z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 11 - } - ], - [ - 21489, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-yonuts-re-camilla-my-ladybug-drawing-20160806t133934665z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 18 - } - ], - [ - 21490, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-picker-re-camilla-my-ladybug-drawing-20160806t134002505z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 19 - } - ], - [ - 21491, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-btcbtcbtc20155-re-camilla-re-camilla-re-btcbtcbtc20155-re-camilla-my-ladybug-drawing-20160806t134043064z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 20 - } - ], - [ - 21492, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-alexft-re-camilla-my-ladybug-drawing-20160806t205601159z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 24 - } - ], - [ - 21493, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-richman-re-camilla-my-ladybug-drawing-20160806t205631480z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 25 - } - ], - [ - 21494, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-fairytalelife-re-camilla-my-ladybug-drawing-20160806t205859483z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 26 - } - ], - [ - 21495, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-alexft-re-camilla-re-alexft-re-camilla-my-ladybug-drawing-20160806t212406972z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 29 - } - ], - [ - 21496, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-cryptoiskey-re-camilla-my-ladybug-drawing-20160806t212537411z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 30 - } - ], - [ - 21497, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-acidyo-re-camilla-my-ladybug-drawing-20160806t232502838z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 34 - } - ], - [ - 21498, - { - "block": 4727781, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "monopoly-man", - "comment_permlink": "re-camilla-my-ladybug-drawing-20160807t081105049z", - "curator": "camilla", - "reward": { - "amount": "75925581", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 49 - } - ], - [ - 21499, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-camilla-re-alexft-re-camilla-re-alexft-re-camilla-my-ladybug-drawing-20160807t105359677z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 54 - } - ], - [ - 21500, - { - "block": 4727781, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "helle", - "comment_permlink": "re-camilla-my-ladybug-drawing-20160807t184458232z", - "curator": "camilla", - "reward": { - "amount": "79089147", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 55 - } - ], - [ - 21501, - { - "block": 4727781, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "juvyjabian", - "comment_permlink": "re-camilla-my-ladybug-drawing-20160808t072735516z", - "curator": "camilla", - "reward": { - "amount": "79089147", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 59 - } - ], - [ - 21502, - { - "block": 4760179, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "acidyo", - "pending_payout": { - "amount": "113950", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "gemo-dna-introduction-and-prologue-sci-fi-action-rpg-futurism", - "rshares": "215458718337", - "total_vote_weight": "2959357778273831534", - "voter": "camilla", - "weight": "5949925842861737" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T10:59:18", - "trx_id": "4f70a5bb027b1c5691b8643394acdc2b07a71a4a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21503, - { - "block": 4760179, - "op": { - "type": "vote_operation", - "value": { - "author": "acidyo", - "permlink": "gemo-dna-introduction-and-prologue-sci-fi-action-rpg-futurism", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T10:59:18", - "trx_id": "4f70a5bb027b1c5691b8643394acdc2b07a71a4a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21504, - { - "block": 4760181, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "250577", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-cross-country-road-trip-greater-than-sf-greater-than-vegas-greater-than-boulder-greater-than-houston-greater-than-new-orleans", - "rshares": "211234037585", - "total_vote_weight": "17059321300395903263", - "voter": "camilla", - "weight": "5532611969834533" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T10:59:24", - "trx_id": "ec31d3d98020404a4f1ac1a2662d6838f85d0c38", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21505, - { - "block": 4760181, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "my-cross-country-road-trip-greater-than-sf-greater-than-vegas-greater-than-boulder-greater-than-houston-greater-than-new-orleans", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T10:59:24", - "trx_id": "ec31d3d98020404a4f1ac1a2662d6838f85d0c38", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21506, - { - "block": 4760207, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "fairytalelife", - "pending_payout": { - "amount": "85", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "how-to-draw-lips", - "rshares": "211234037585", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:00:42", - "trx_id": "b1b8baf2e1839dec8f2e66bb2c16130f9d5ef7bf", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21507, - { - "block": 4760207, - "op": { - "type": "vote_operation", - "value": { - "author": "fairytalelife", - "permlink": "how-to-draw-lips", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:00:42", - "trx_id": "b1b8baf2e1839dec8f2e66bb2c16130f9d5ef7bf", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21508, - { - "block": 4760218, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "97", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "anwen-meditates-a-steemit-health-channel-how-savasana-prepares-me-for-death", - "rshares": "211234037585", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:01:15", - "trx_id": "5b8118f4915757bc21e3e71b3971339ff3102b6b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21509, - { - "block": 4760218, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "anwen-meditates-a-steemit-health-channel-how-savasana-prepares-me-for-death", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:01:15", - "trx_id": "5b8118f4915757bc21e3e71b3971339ff3102b6b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21510, - { - "block": 4760233, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "infovore", - "pending_payout": { - "amount": "596545", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-rise-of-the-human-cyborgs-outsmarting-smartphones-and-the-internables-trend", - "rshares": "211234037585", - "total_vote_weight": "17534666943612674070", - "voter": "camilla", - "weight": "2387716540162740" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:02:00", - "trx_id": "cc1ba235aee2e2e3ee7a11af6f11db3d9b4d81c0", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21511, - { - "block": 4760233, - "op": { - "type": "vote_operation", - "value": { - "author": "infovore", - "permlink": "the-rise-of-the-human-cyborgs-outsmarting-smartphones-and-the-internables-trend", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:02:00", - "trx_id": "cc1ba235aee2e2e3ee7a11af6f11db3d9b4d81c0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21512, - { - "block": 4769868, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "a-drawing-to-express-the-power-of-steem", - "voter": "gavicrane", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T19:04:57", - "trx_id": "201af50904ee29156e60d138ecc9a466edf5eff9", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 21513, - { - "block": 4769874, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-tessaddavis-re-camilla-a-drawing-to-express-the-power-of-steem-20160719t110026969z", - "voter": "gavicrane", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T19:05:15", - "trx_id": "6acf603f41c5eabd2d4e1cdd33ba24df7d7e287b", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21514, - { - "block": 4780729, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-anwenbaumeister-anwen-meditates-a-steemit-health-channel-a-lovingkindness-meditation-and-an-original-recipe-for-slow-cooked-root-veggies-20160808t180327396z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T04:08:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 13 - } - ], - [ - 21515, - { - "block": 4783685, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "acidyo", - "comment_permlink": "gemo-dna-introduction-and-prologue-sci-fi-action-rpg-futurism", - "curator": "camilla", - "reward": { - "amount": "291037297", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T06:36:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 31 - } - ], - [ - 21516, - { - "block": 4784890, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-pixielolz-mew-meets-steemit-speed-drawing-video-photos-20160808t180911948z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:36:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21517, - { - "block": 4784890, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-pixielolz-mew-meets-steemit-speed-drawing-video-photos-20160808t181130683z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:36:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 6 - } - ], - [ - 21518, - { - "block": 4785210, - "op": { - "type": "interest_operation", - "value": { - "interest": { - "amount": "2260", - "nai": "@@000000013", - "precision": 3 - }, - "owner": "camilla" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21519, - { - "block": 4785210, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "15", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "a-steem-whale-in-a-dream-world-art-and-poem", - "vesting_payout": { - "amount": "59441772", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 21520, - { - "block": 4785210, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 38, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "1111", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "30", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-steem-whale-in-a-dream-world-art-and-poem", - "total_payout_value": { - "amount": "4588", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21521, - { - "block": 4785210, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "a-steem-whale-in-a-dream-world-art-and-poem" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21522, - { - "block": 4785210, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-gidlark-re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160808t160539173z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 21523, - { - "block": 4785210, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-anca3drandom-re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160808t162615517z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 9 - } - ], - [ - 21524, - { - "block": 4785210, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-inkha-re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160809t180445870z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 19 - } - ], - [ - 21525, - { - "block": 4785210, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "joelinux", - "comment_permlink": "re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160810t164328721z", - "curator": "camilla", - "reward": { - "amount": "68827315", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 20 - } - ], - [ - 21526, - { - "block": 4785210, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "steemswede", - "comment_permlink": "re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160828t214107644z", - "curator": "camilla", - "reward": { - "amount": "34413657", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 24 - } - ], - [ - 21527, - { - "block": 4785210, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-steemswede-re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160828t215937477z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 28 - } - ], - [ - 21528, - { - "block": 4785909, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "anwenbaumeister", - "comment_permlink": "my-cross-country-road-trip-greater-than-sf-greater-than-vegas-greater-than-boulder-greater-than-houston-greater-than-new-orleans", - "curator": "camilla", - "reward": { - "amount": "203326016", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T08:27:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 63 - } - ], - [ - 21529, - { - "block": 4788433, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "infovore", - "comment_permlink": "the-rise-of-the-human-cyborgs-outsmarting-smartphones-and-the-internables-trend", - "curator": "camilla", - "reward": { - "amount": "168834816", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T10:34:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 89 - } - ], - [ - 21530, - { - "block": 4815612, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "stellabelle", - "pending_payout": { - "amount": "120454", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "new-idea-for-steemit-let-s-open-our-own-bars", - "rshares": "215462930329", - "total_vote_weight": "16410291079042728394", - "voter": "camilla", - "weight": "12182373335164876" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:17:39", - "trx_id": "8eaa66efbb94cc46cd51326af05306b66c7701f2", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21531, - { - "block": 4815612, - "op": { - "type": "vote_operation", - "value": { - "author": "stellabelle", - "permlink": "new-idea-for-steemit-let-s-open-our-own-bars", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:17:39", - "trx_id": "8eaa66efbb94cc46cd51326af05306b66c7701f2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21532, - { - "block": 4815614, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "stellabelle", - "pending_payout": { - "amount": "91", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "some-benefits-of-being-an-atheist", - "rshares": "211238166990", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:17:45", - "trx_id": "3ab5d102803f963155a4423ba5438446a82aafec", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21533, - { - "block": 4815614, - "op": { - "type": "vote_operation", - "value": { - "author": "stellabelle", - "permlink": "some-benefits-of-being-an-atheist", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:17:45", - "trx_id": "3ab5d102803f963155a4423ba5438446a82aafec", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21534, - { - "block": 4815619, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "stellabelle", - "pending_payout": { - "amount": "241", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "secret-writer-my-parents-were-alcoholics-and-i-saved-my-mom-s-life-twice", - "rshares": "211238166990", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:18:00", - "trx_id": "f2cce4a3c6aabb23df5282f2704cde1d8e36043b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21535, - { - "block": 4815619, - "op": { - "type": "vote_operation", - "value": { - "author": "stellabelle", - "permlink": "secret-writer-my-parents-were-alcoholics-and-i-saved-my-mom-s-life-twice", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:18:00", - "trx_id": "f2cce4a3c6aabb23df5282f2704cde1d8e36043b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21536, - { - "block": 4817530, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "22214", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "fennec-fox-step-by-step-of-my-drawing-process-from-sketch-to-finished-artwork", - "rshares": "211238166990", - "total_vote_weight": "14051387273185141180", - "voter": "camilla", - "weight": "56011909777174591" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:54:03", - "trx_id": "91623ad16bedd872f8243c375ecb29b52555ecf3", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21537, - { - "block": 4817530, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "fennec-fox-step-by-step-of-my-drawing-process-from-sketch-to-finished-artwork", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:54:03", - "trx_id": "91623ad16bedd872f8243c375ecb29b52555ecf3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21538, - { - "block": 4817545, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "31174", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "copic-markers-a-beginner-s-guide", - "rshares": "211238166990", - "total_vote_weight": "14656706708420804802", - "voter": "camilla", - "weight": "41573594419093336" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:54:48", - "trx_id": "992f73454dd64e6fa7cd9d65c818e4f88ca289e3", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21539, - { - "block": 4817545, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "copic-markers-a-beginner-s-guide", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:54:48", - "trx_id": "992f73454dd64e6fa7cd9d65c818e4f88ca289e3", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21540, - { - "block": 4817547, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"pixielolz\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:54:54", - "trx_id": "b42a3f4fec38b943f5f71aaac4be130d02d3c650", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21541, - { - "block": 4817575, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "92", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "trading-card-collection-showcasing-the-cards-i-ve-made-the-last-9-months", - "rshares": "211238166990", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:56:18", - "trx_id": "bb884d2af5c220acb4b55892c657a6aa5dec0c6e", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21542, - { - "block": 4817575, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "trading-card-collection-showcasing-the-cards-i-ve-made-the-last-9-months", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:56:18", - "trx_id": "bb884d2af5c220acb4b55892c657a6aa5dec0c6e", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21543, - { - "block": 4817585, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "92", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "through-time-step-by-step-of-my-drawing-process-from-sketch-to-finished-artwork", - "rshares": "211238166990", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:56:48", - "trx_id": "1a1c70c6de09511a6a13e038f58d06d19d712b14", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21544, - { - "block": 4817585, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "through-time-step-by-step-of-my-drawing-process-from-sketch-to-finished-artwork", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:56:48", - "trx_id": "1a1c70c6de09511a6a13e038f58d06d19d712b14", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21545, - { - "block": 4817615, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "119", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "illustrations-for-silence-in-time-a-short-story-by-liberosist", - "rshares": "207013403650", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:58:18", - "trx_id": "5f870b4685657a21c8d0c3d4c84858e2a13a4a2e", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21546, - { - "block": 4817615, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "illustrations-for-silence-in-time-a-short-story-by-liberosist", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:58:18", - "trx_id": "5f870b4685657a21c8d0c3d4c84858e2a13a4a2e", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21547, - { - "block": 4829727, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "pixielolz", - "comment_permlink": "copic-markers-a-beginner-s-guide", - "curator": "camilla", - "reward": { - "amount": "93056250", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T21:06:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 23 - } - ], - [ - 21548, - { - "block": 4831404, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "pixielolz", - "comment_permlink": "fennec-fox-step-by-step-of-my-drawing-process-from-sketch-to-finished-artwork", - "curator": "camilla", - "reward": { - "amount": "71320251", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T22:32:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 21549, - { - "block": 4832860, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "stellabelle", - "comment_permlink": "new-idea-for-steemit-let-s-open-our-own-bars", - "curator": "camilla", - "reward": { - "amount": "80600453", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T23:45:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 43 - } - ], - [ - 21550, - { - "block": 4847456, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "fairytalelife", - "pending_payout": { - "amount": "836882", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "how-to-draw-a-braid", - "rshares": "215464179712", - "total_vote_weight": "17696363500200331189", - "voter": "camilla", - "weight": "1647825388296973" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T11:58:39", - "trx_id": "a38d027833317a53a6db3c56b0b463a8f5ed5b28", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21551, - { - "block": 4847456, - "op": { - "type": "vote_operation", - "value": { - "author": "fairytalelife", - "permlink": "how-to-draw-a-braid", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T11:58:39", - "trx_id": "a38d027833317a53a6db3c56b0b463a8f5ed5b28", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21552, - { - "block": 4847500, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "infovore", - "pending_payout": { - "amount": "518655", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend", - "rshares": "211239391874", - "total_vote_weight": "17497519072959523574", - "voter": "camilla", - "weight": "2586516889892580" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:00:51", - "trx_id": "9ef06b675e6e7c61d019a20f853cf378d8067c5b", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21553, - { - "block": 4847500, - "op": { - "type": "vote_operation", - "value": { - "author": "infovore", - "permlink": "a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:00:51", - "trx_id": "9ef06b675e6e7c61d019a20f853cf378d8067c5b", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21554, - { - "block": 4847541, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "great article infovore :D", - "json_metadata": "{\"tags\":[\"steemmag\"]}", - "parent_author": "infovore", - "parent_permlink": "a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend", - "permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:03:00", - "trx_id": "51274db60900301f3ae4eac5bcd92b43681d5a00", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21555, - { - "block": 4847547, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "80", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "pura-vida-my-experience-working-on-a-permaculture-farm-in-costa-rica-part-two", - "rshares": "211239391874", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:03:18", - "trx_id": "8c81f1f31bf385cc594e462630dc56a23be2c0d6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21556, - { - "block": 4847547, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "pura-vida-my-experience-working-on-a-permaculture-farm-in-costa-rica-part-two", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:03:18", - "trx_id": "8c81f1f31bf385cc594e462630dc56a23be2c0d6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21557, - { - "block": 4847565, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "infovore", - "pending_payout": { - "amount": "1107151", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "steemmag-steemit-s-weekend-digest-9-of-community-curation-and-reputation-chats-with-a-whale-steemit-chat-lead-moderators-a-day", - "rshares": "211239391874", - "total_vote_weight": "17791220144631813368", - "voter": "camilla", - "weight": "1232503267820026" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:04:12", - "trx_id": "e531290d33bc74838270939b7036db7e2872ecdd", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21558, - { - "block": 4847565, - "op": { - "type": "vote_operation", - "value": { - "author": "infovore", - "permlink": "steemmag-steemit-s-weekend-digest-9-of-community-curation-and-reputation-chats-with-a-whale-steemit-chat-lead-moderators-a-day", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:04:12", - "trx_id": "e531290d33bc74838270939b7036db7e2872ecdd", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21559, - { - "block": 4847590, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "opheliafu", - "pending_payout": { - "amount": "80", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "dream", - "rshares": "211239391874", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:05:27", - "trx_id": "f4a38bff870c28d178786a6a6206af15bd0b6499", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21560, - { - "block": 4847590, - "op": { - "type": "vote_operation", - "value": { - "author": "opheliafu", - "permlink": "dream", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:05:27", - "trx_id": "f4a38bff870c28d178786a6a6206af15bd0b6499", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21561, - { - "block": 4847798, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "lyubovbar", - "pending_payout": { - "amount": "84", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "gentle-casserole-of-minced-chicken-and-vegetables-recipe-and-cooking-lyubovbar", - "rshares": "207014604037", - "total_vote_weight": "960116344927767918", - "voter": "camilla", - "weight": "902153156129896529" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:15:51", - "trx_id": "4e6b205f98a3d09b62db0671b10f16a6fec9ae47", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21562, - { - "block": 4847798, - "op": { - "type": "vote_operation", - "value": { - "author": "lyubovbar", - "permlink": "gentle-casserole-of-minced-chicken-and-vegetables-recipe-and-cooking-lyubovbar", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:15:51", - "trx_id": "4e6b205f98a3d09b62db0671b10f16a6fec9ae47", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21563, - { - "block": 4847813, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "ekaterina4ka", - "pending_payout": { - "amount": "20158", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "knitting-needles-my-passion-post-14-vyazanie-spicami-moe-uvlechenie-post-14", - "rshares": "207014604037", - "total_vote_weight": "14117644497751956775", - "voter": "camilla", - "weight": "53225957273500042" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:16:36", - "trx_id": "4fc7301f6f82b3069f7f88d4a3f303ffc8532caf", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21564, - { - "block": 4847813, - "op": { - "type": "vote_operation", - "value": { - "author": "ekaterina4ka", - "permlink": "knitting-needles-my-passion-post-14-vyazanie-spicami-moe-uvlechenie-post-14", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:16:36", - "trx_id": "4fc7301f6f82b3069f7f88d4a3f303ffc8532caf", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21565, - { - "block": 4855539, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "187", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "rshares": "487083675640", - "total_vote_weight": "2002438233053738410", - "voter": "infovore", - "weight": "2002438233053738410" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T18:45:42", - "trx_id": "338e3d07db6a21a59e9fdffa84cd177a03a8ccd5", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21566, - { - "block": 4855539, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "voter": "infovore", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T18:45:42", - "trx_id": "338e3d07db6a21a59e9fdffa84cd177a03a8ccd5", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21567, - { - "block": 4855580, - "op": { - "type": "comment_operation", - "value": { - "author": "infovore", - "body": "Thanks @camilla . Great to see you drop by.. How are yo doing today?", - "json_metadata": "{\"tags\":[\"steemmag\"],\"users\":[\"camilla\"]}", - "parent_author": "camilla", - "parent_permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "permlink": "re-camilla-re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t184739329z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T18:47:45", - "trx_id": "0c869279e01f8f00ce45c2d334c872388fc47d05", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21568, - { - "block": 4861233, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "### I colored this drawing with color pencils and markers. It took me about 6 hours.\nhttps://i.imgsafe.org/496152e4bb.jpg\n\n### The drawing is from Johanna Basfords coloring book: Enchanted Forest.\n### I hope your autumn days are bright, peaceful and full of colors :)", - "json_metadata": "{\"tags\":[\"art\",\"coloring\",\"flowers\",\"\"],\"image\":[\"https://i.imgsafe.org/496152e4bb.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "colors-of-the-falling-leaves", - "title": "Colors of the Falling Leaves" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:03", - "trx_id": "ab378409dc2b9e81a6b30a78dae53ca69a4acfb2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21569, - { - "block": 4861233, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "79", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "215464179712", - "total_vote_weight": "942864750061894927", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:03", - "trx_id": "ab378409dc2b9e81a6b30a78dae53ca69a4acfb2", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21570, - { - "block": 4861233, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:03", - "trx_id": "ab378409dc2b9e81a6b30a78dae53ca69a4acfb2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21571, - { - "block": 4861237, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "83", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "9342417724", - "total_vote_weight": "981571504716947568", - "voter": "goldmatters", - "weight": "258045031033684" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:15", - "trx_id": "41f82960cbf4033b4bbafca616e441bc87240270", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21572, - { - "block": 4861237, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "goldmatters", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:15", - "trx_id": "41f82960cbf4033b4bbafca616e441bc87240270", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21573, - { - "block": 4861248, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -77,16 +77,44 @@\n 6 hours\n+ to color in all the details\n .%0Ahttps:\n", - "json_metadata": "{\"tags\":[\"art\",\"coloring\",\"flowers\",\"\"],\"image\":[\"https://i.imgsafe.org/496152e4bb.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "colors-of-the-falling-leaves", - "title": "Colors of the Falling Leaves" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:48", - "trx_id": "4ec95086082881f1933fc51deb44808586236c47", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21574, - { - "block": 4861257, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "374", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "651645205561", - "total_vote_weight": "3315460247733921376", - "voter": "clains", - "weight": "93355549720678952" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:32:15", - "trx_id": "a83693680d2a21066e9076b45dcb806a44f867ae", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21575, - { - "block": 4861257, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "clains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:32:15", - "trx_id": "a83693680d2a21066e9076b45dcb806a44f867ae", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21576, - { - "block": 4861263, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "441", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "130765248410", - "total_vote_weight": "3710619089052759354", - "voter": "psylains", - "weight": "19757942065941898" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:32:33", - "trx_id": "f1a17268864f8b28c1fe6a23c0bfafc639abcdce", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21577, - { - "block": 4861263, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "psylains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:32:33", - "trx_id": "f1a17268864f8b28c1fe6a23c0bfafc639abcdce", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21578, - { - "block": 4861274, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "442", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 167755251, - "total_vote_weight": "3711112772370702390", - "voter": "myxxo", - "weight": "33735026726107" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:06", - "trx_id": "985e950136848950e6228f7165dbeccec355abbc", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21579, - { - "block": 4861274, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "myxxo", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:06", - "trx_id": "985e950136848950e6228f7165dbeccec355abbc", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21580, - { - "block": 4861278, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "448", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "11146913938", - "total_vote_weight": "3743842826315368099", - "voter": "asim", - "weight": "2454754045849928" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:18", - "trx_id": "bc8fb50c578058a985b35a269670c3ab051b9e1e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21581, - { - "block": 4861278, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "asim", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:18", - "trx_id": "bc8fb50c578058a985b35a269670c3ab051b9e1e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21582, - { - "block": 4861282, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "448", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1413116466, - "total_vote_weight": "3747981698857805172", - "voter": "murh", - "weight": "338007924299027" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:30", - "trx_id": "08d0ed18adab2db76b9899b4d2f785ca61b05d7c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21583, - { - "block": 4861282, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "murh", - "weight": 3301 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:30", - "trx_id": "08d0ed18adab2db76b9899b4d2f785ca61b05d7c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21584, - { - "block": 4861283, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "660", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "376199691813", - "total_vote_weight": "4772726281932303484", - "voter": "anonymous", - "weight": "85395381922874859" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:33", - "trx_id": "8f59b3c9c0ef02e6680fa86f4b31d3973ac3e185", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21585, - { - "block": 4861283, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "anonymous", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:33", - "trx_id": "8f59b3c9c0ef02e6680fa86f4b31d3973ac3e185", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21586, - { - "block": 4861305, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "660", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 446928899, - "total_vote_weight": "4773858721503540961", - "voter": "runridefly", - "weight": "137780147833893" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:34:42", - "trx_id": "064a66dbb472995ece4bdbfac413b29fd002f80e", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21587, - { - "block": 4861305, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "runridefly", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:34:42", - "trx_id": "064a66dbb472995ece4bdbfac413b29fd002f80e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21588, - { - "block": 4861306, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "664", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5888362824", - "total_vote_weight": "4788761305590533862", - "voter": "bitspace", - "weight": "1837985370729124" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:34:45", - "trx_id": "f595e39e07494f9463464fe7604fc77941579211", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21589, - { - "block": 4861306, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "bitspace", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:34:45", - "trx_id": "f595e39e07494f9463464fe7604fc77941579211", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21590, - { - "block": 4861315, - "op": { - "type": "comment_operation", - "value": { - "author": "runridefly", - "body": "Pretty", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t233510136z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:35:12", - "trx_id": "6cffe592a70d339479a69eb7c992b6324933c046", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21591, - { - "block": 4861358, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "664", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 286701566, - "total_vote_weight": "4789486075952524692", - "voter": "riosparada", - "weight": "152201776018074" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:37:21", - "trx_id": "8a3023a21bc2d2ba82b568fc5633d1e6b611221f", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21592, - { - "block": 4861358, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "riosparada", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:37:21", - "trx_id": "8a3023a21bc2d2ba82b568fc5633d1e6b611221f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21593, - { - "block": 4861421, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "711", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "76309016178", - "total_vote_weight": "4979695521725803466", - "voter": "thecryptofiend", - "weight": "59915975418582813" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:40:30", - "trx_id": "948066bebb5c927206c9ec64adbb2af02c0c9858", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21594, - { - "block": 4861421, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "thecryptofiend", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:40:30", - "trx_id": "948066bebb5c927206c9ec64adbb2af02c0c9858", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21595, - { - "block": 4861425, - "op": { - "type": "comment_operation", - "value": { - "author": "thecryptofiend", - "body": "Beautifully done.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234043251z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:40:42", - "trx_id": "52622f3a5be5a814ccfe8f64424e379b39f3e9a5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21596, - { - "block": 4861441, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "runridefly", - "pending_payout": { - "amount": "78", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t233510136z", - "rshares": "211239391874", - "total_vote_weight": "925304557063353830", - "voter": "camilla", - "weight": "194313956983304304" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:41:30", - "trx_id": "bb2f867251b1c4ac9b6f1d3652ebb778407346cf", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21597, - { - "block": 4861441, - "op": { - "type": "vote_operation", - "value": { - "author": "runridefly", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t233510136z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:41:30", - "trx_id": "bb2f867251b1c4ac9b6f1d3652ebb778407346cf", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21598, - { - "block": 4861442, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "711", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 758446790, - "total_vote_weight": "4981559454058925084", - "voter": "dajohns1420", - "weight": "652376316592566" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:41:33", - "trx_id": "c9d21ad41671069dcfdc379bc425bf9da9e4f2eb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21599, - { - "block": 4861442, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "dajohns1420", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:41:33", - "trx_id": "c9d21ad41671069dcfdc379bc425bf9da9e4f2eb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21600, - { - "block": 4861447, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thank you :)", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "thecryptofiend", - "parent_permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234043251z", - "permlink": "re-thecryptofiend-re-camilla-colors-of-the-falling-leaves-20160910t234147377z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:41:48", - "trx_id": "05dc0ba561cd32f233ebd9218c90a08afab50ef8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21601, - { - "block": 4861455, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thanks!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "runridefly", - "parent_permlink": "re-camilla-colors-of-the-falling-leaves-20160910t233510136z", - "permlink": "re-runridefly-re-camilla-colors-of-the-falling-leaves-20160910t234213368z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:42:12", - "trx_id": "e595a76431f6d0fd95ccddb26e0ee8d621f9f6a8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21602, - { - "block": 4861507, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "714", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1609908331, - "total_vote_weight": "4985514199071180117", - "voter": "bullionstackers", - "weight": "1819182705637315" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:44:51", - "trx_id": "107888d01ad020877e204098042e8d3b5a4516f2", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21603, - { - "block": 4861507, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "bullionstackers", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:44:51", - "trx_id": "107888d01ad020877e204098042e8d3b5a4516f2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21604, - { - "block": 4861523, - "op": { - "type": "comment_operation", - "value": { - "author": "bullionstackers", - "body": "Amazing \ud83d\udc4d\u2705\ud83c\udd99\nSweet \u2764\ufe0f", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234539398z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:45:39", - "trx_id": "20e6f5c072523bcfdbffc043c0c614ad6807fda6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21605, - { - "block": 4861594, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2334", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "2043524972373", - "total_vote_weight": "8641125258482638496", - "voter": "badassmother", - "weight": "2211644690943932319" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:49:12", - "trx_id": "d67777f06253fd76026e917b8422c3b3280f3a20", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21606, - { - "block": 4861594, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "badassmother", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:49:12", - "trx_id": "d67777f06253fd76026e917b8422c3b3280f3a20", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21607, - { - "block": 4861611, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2811", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "469477368872", - "total_vote_weight": "9216964533196661783", - "voter": "boatymcboatface", - "weight": "364698207318881415" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:03", - "trx_id": "26a4f80f777e0598f81888acacc5b86f09c9d8e3", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21608, - { - "block": 4861611, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "boatymcboatface", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:03", - "trx_id": "26a4f80f777e0598f81888acacc5b86f09c9d8e3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21609, - { - "block": 4861611, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2815", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 3142023228, - "total_vote_weight": "9220590649066884760", - "voter": "glitterpig", - "weight": "2296540051141218" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:03", - "trx_id": "339bf9c191ca9b854148930b45e2b1ec1a8162fd", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21610, - { - "block": 4861611, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "glitterpig", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:03", - "trx_id": "339bf9c191ca9b854148930b45e2b1ec1a8162fd", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21611, - { - "block": 4861613, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "bullionstackers", - "pending_payout": { - "amount": "78", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234539398z", - "rshares": "211239391874", - "total_vote_weight": "925304557063353830", - "voter": "camilla", - "weight": "138795683559503074" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "2a804b16897696efd4d1f9db5c997b67674b9f23", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21612, - { - "block": 4861613, - "op": { - "type": "vote_operation", - "value": { - "author": "bullionstackers", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234539398z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "2a804b16897696efd4d1f9db5c997b67674b9f23", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21613, - { - "block": 4861613, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4066", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "1086156024356", - "total_vote_weight": "10323774733997980525", - "voter": "rossco99", - "weight": "702360534072797637" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "7e75d48d6f3d4b319e06bfadadf660a13ad2b416", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21614, - { - "block": 4861613, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "rossco99", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "7e75d48d6f3d4b319e06bfadadf660a13ad2b416", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21615, - { - "block": 4861613, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4141", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "59824941705", - "total_vote_weight": "10376922050468705210", - "voter": "theshell", - "weight": "33837124819694716" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "842ae95f2699f21bb822b91a5f4259a34b96733c", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21616, - { - "block": 4861613, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "theshell", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "842ae95f2699f21bb822b91a5f4259a34b96733c", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21617, - { - "block": 4861615, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4141", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 442913138, - "total_vote_weight": "10377312932517177095", - "voter": "karen13", - "weight": "250164511022006" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:15", - "trx_id": "a3c6840b6e79a68858f5245e0aac9687324e5600", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21618, - { - "block": 4861615, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "karen13", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:15", - "trx_id": "a3c6840b6e79a68858f5245e0aac9687324e5600", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21619, - { - "block": 4861616, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4143", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 918895431, - "total_vote_weight": "10378123760218338031", - "voter": "taker", - "weight": "520281108244933" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:18", - "trx_id": "5fa8ce6e3fe39a29c75f7b18a4a95011289cc6a6", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21620, - { - "block": 4861616, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "taker", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:18", - "trx_id": "5fa8ce6e3fe39a29c75f7b18a4a95011289cc6a6", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21621, - { - "block": 4861620, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4144", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1027351719, - "total_vote_weight": "10379030096175788546", - "voter": "coar", - "weight": "587607812413750" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:30", - "trx_id": "7ab0666e464154190d614f1fd33895117ffaabdb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21622, - { - "block": 4861620, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "coar", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:30", - "trx_id": "7ab0666e464154190d614f1fd33895117ffaabdb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21623, - { - "block": 4861749, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4154", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 4188095885, - "total_vote_weight": "10382722753969179236", - "voter": "fubar-bdhr", - "weight": "3187994561627295" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:56:57", - "trx_id": "46ee895d4b883b7542d529eae6dd88e93af6ad36", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21624, - { - "block": 4861749, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "fubar-bdhr", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:56:57", - "trx_id": "46ee895d4b883b7542d529eae6dd88e93af6ad36", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21625, - { - "block": 4861771, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4166", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "7528587638", - "total_vote_weight": "10389352241341337948", - "voter": "furion", - "weight": "5966538634942840" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:03", - "trx_id": "01b7797a422b611a8cebf22c9d96a0076e573c6f", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21626, - { - "block": 4861771, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "furion", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:03", - "trx_id": "01b7797a422b611a8cebf22c9d96a0076e573c6f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21627, - { - "block": 4861772, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4168", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1643275913, - "total_vote_weight": "10390797820305061860", - "voter": "positive", - "weight": "1303430365624393" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "25ccd12e68ae60683792cb86ad162a2551461b10", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21628, - { - "block": 4861772, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "positive", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "25ccd12e68ae60683792cb86ad162a2551461b10", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21629, - { - "block": 4861772, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4290", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "95270090466", - "total_vote_weight": "10473728653351718274", - "voter": "laonie", - "weight": "74775967797068533" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "0850310bdd0e6ab53cf98faed8dc54977ef8c1aa", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21630, - { - "block": 4861772, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "laonie", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "0850310bdd0e6ab53cf98faed8dc54977ef8c1aa", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21631, - { - "block": 4861772, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4303", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "10675325035", - "total_vote_weight": "10482915065453226980", - "voter": "xiaohui", - "weight": "8283081578193683" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "300419a4ecd8dff31f77b53cfd71aa2b24cbaafd", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21632, - { - "block": 4861772, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "xiaohui", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "300419a4ecd8dff31f77b53cfd71aa2b24cbaafd", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21633, - { - "block": 4861772, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4306", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 2027172658, - "total_vote_weight": "10484657112458893762", - "voter": "fkn", - "weight": "1570745716776215" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "72f1bad886b8d35a33a8bf714de59715122beffe", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21634, - { - "block": 4861772, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "fkn", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "72f1bad886b8d35a33a8bf714de59715122beffe", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21635, - { - "block": 4861772, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4309", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 2666300169, - "total_vote_weight": "10486947232389218712", - "voter": "elishagh1", - "weight": "2064924803842996" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "32b1930e1a54ef6eccc101f2cb63b9dfae662b0b", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 21636, - { - "block": 4861772, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "elishagh1", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "32b1930e1a54ef6eccc101f2cb63b9dfae662b0b", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 21637, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4334", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "19377752514", - "total_vote_weight": "10503551539749126072", - "voter": "somebody", - "weight": "14999224315116315" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "7919d9f1d4f6885deeaff8cb391483d35505a741", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21638, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "somebody", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "7919d9f1d4f6885deeaff8cb391483d35505a741", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21639, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4335", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 446441859, - "total_vote_weight": "10503933268175068386", - "voter": "xiaokongcom", - "weight": "344828011434556" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "98f0216f62a78f00dbeb255c535d87fa2d1131fa", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21640, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "xiaokongcom", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "98f0216f62a78f00dbeb255c535d87fa2d1131fa", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21641, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4336", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 899777990, - "total_vote_weight": "10504702508469112725", - "voter": "xianjun", - "weight": "694880398953386" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "fe5755839b5588c54275e838460e5aedcf773cd8", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21642, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "xianjun", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "fe5755839b5588c54275e838460e5aedcf773cd8", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21643, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4364", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "21517750872", - "total_vote_weight": "10523054228899113010", - "voter": "kimziv", - "weight": "16577720788433590" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "d54ed386d18de451d18c462b61130bdab74e87b0", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21644, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "kimziv", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "d54ed386d18de451d18c462b61130bdab74e87b0", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21645, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4369", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 3947218827, - "total_vote_weight": "10526411468808214056", - "voter": "myfirst", - "weight": "3032706717887944" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "a8a43ecc52033121fb72a6a017010c561c52f20f", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 21646, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "myfirst", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "a8a43ecc52033121fb72a6a017010c561c52f20f", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 21647, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4369", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 59017260, - "total_vote_weight": "10526461643350211360", - "voter": "microluck", - "weight": "45324336270897" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "3f5698b07a3f1406b5828c4c64f9a983fea3a899", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 21648, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "microluck", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "3f5698b07a3f1406b5828c4c64f9a983fea3a899", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 21649, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4370", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 966298641, - "total_vote_weight": "10527283068410058847", - "voter": "flysaga", - "weight": "742020637395563" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "ca07f6a029a45f50c7cf69cc0549ee26d63ab08f", - "trx_in_block": 8, - "virtual_op": 1 - } - ], - [ - 21650, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "flysaga", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "ca07f6a029a45f50c7cf69cc0549ee26d63ab08f", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 21651, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4377", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5708182705", - "total_vote_weight": "10532131970458603469", - "voter": "midnightoil", - "weight": "4380174850518641" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "bbfe11f85d10cc32806fc169b2a44cd1ce96c381", - "trx_in_block": 9, - "virtual_op": 1 - } - ], - [ - 21652, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "midnightoil", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "bbfe11f85d10cc32806fc169b2a44cd1ce96c381", - "trx_in_block": 9, - "virtual_op": 0 - } - ], - [ - 21653, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4381", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 2685190882, - "total_vote_weight": "10534410893458449984", - "voter": "sisterholics", - "weight": "2058627109861351" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "41c97d8d492ddb239b65e28bf5c0658cfd4a38f2", - "trx_in_block": 11, - "virtual_op": 1 - } - ], - [ - 21654, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "sisterholics", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "41c97d8d492ddb239b65e28bf5c0658cfd4a38f2", - "trx_in_block": 11, - "virtual_op": 0 - } - ], - [ - 21655, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4381", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 52858601, - "total_vote_weight": "10534455741411155393", - "voter": "ch0c0latechip", - "weight": "40512650610552" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "cb76c0e3e43723b3b3b903391f70f14592e0fd3d", - "trx_in_block": 13, - "virtual_op": 1 - } - ], - [ - 21656, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "ch0c0latechip", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "cb76c0e3e43723b3b3b903391f70f14592e0fd3d", - "trx_in_block": 13, - "virtual_op": 0 - } - ], - [ - 21657, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4381", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 54905658, - "total_vote_weight": "10534502325653869728", - "voter": "fnait", - "weight": "42081099251949" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "eaac0bcfebfb11d446729cf7cd8b0d585f87044d", - "trx_in_block": 16, - "virtual_op": 1 - } - ], - [ - 21658, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "fnait", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "eaac0bcfebfb11d446729cf7cd8b0d585f87044d", - "trx_in_block": 16, - "virtual_op": 0 - } - ], - [ - 21659, - { - "block": 4861785, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4396", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "10966759140", - "total_vote_weight": "10543795996096921811", - "voter": "acidyo", - "weight": "8581155709084756" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:45", - "trx_id": "f0d46b2c2ce35031d8557b025817f342f0f339de", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21660, - { - "block": 4861785, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "acidyo", - "weight": 4200 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:45", - "trx_id": "f0d46b2c2ce35031d8557b025817f342f0f339de", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21661, - { - "block": 4861792, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4425", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "21529337232", - "total_vote_weight": "10561977462931279207", - "voter": "jl777", - "weight": "16999671490124165" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:06", - "trx_id": "2ea8fd4f67fe9ebb398724c437bfece264b6c2ca", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21662, - { - "block": 4861792, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "jl777", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:06", - "trx_id": "2ea8fd4f67fe9ebb398724c437bfece264b6c2ca", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21663, - { - "block": 4861793, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4427", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1816956761, - "total_vote_weight": "10563508050743123362", - "voter": "proto", - "weight": "1433650583760691" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:09", - "trx_id": "39f8ed7c216ddf201ccf9c42bac4a8d4ff9f354c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21664, - { - "block": 4861793, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "proto", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:09", - "trx_id": "39f8ed7c216ddf201ccf9c42bac4a8d4ff9f354c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21665, - { - "block": 4861795, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4431", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 2586674942, - "total_vote_weight": "10565686017257802111", - "voter": "yefet", - "weight": "2047288523798024" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:15", - "trx_id": "a1b7ae5e0b8dcbed8002f761969b6e22f8a9a31d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21666, - { - "block": 4861795, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "yefet", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:15", - "trx_id": "a1b7ae5e0b8dcbed8002f761969b6e22f8a9a31d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21667, - { - "block": 4861797, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4432", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 992068506, - "total_vote_weight": "10566521014395361912", - "voter": "smisi", - "weight": "787680633098078" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:21", - "trx_id": "6ff284737fe558f2aa540ab1aeaffe85bf02a185", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21668, - { - "block": 4861797, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "smisi", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:21", - "trx_id": "6ff284737fe558f2aa540ab1aeaffe85bf02a185", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21669, - { - "block": 4861804, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4434", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 740454861, - "total_vote_weight": "10567144119852226283", - "voter": "steem1653", - "weight": "595065711305474" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:42", - "trx_id": "8b038f96aac44f05d59cd26778b2a69904bdee5d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21670, - { - "block": 4861804, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "steem1653", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:42", - "trx_id": "8b038f96aac44f05d59cd26778b2a69904bdee5d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21671, - { - "block": 4861870, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4448", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "7395923269", - "total_vote_weight": "10573362512636721672", - "voter": "dasha", - "weight": "6218392784495389" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:03:00", - "trx_id": "122ed469619cfd4247bb0c845ef9e5baa5fb50a0", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21672, - { - "block": 4861870, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "dasha", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:03:00", - "trx_id": "122ed469619cfd4247bb0c845ef9e5baa5fb50a0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21673, - { - "block": 4862066, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4426", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1457681508, - "total_vote_weight": "10574586953973812133", - "voter": "benthegameboy", - "weight": "1224441337090461" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:12:48", - "trx_id": "dab654729e339ce2935e85d17053eb787d2f3fc0", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21674, - { - "block": 4862066, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "benthegameboy", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:12:48", - "trx_id": "dab654729e339ce2935e85d17053eb787d2f3fc0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21675, - { - "block": 4862096, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4431", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 3905082034, - "total_vote_weight": "10577865317090407148", - "voter": "sompitonov", - "weight": "3278363116595015" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:14:18", - "trx_id": "3f5e92db2e9c54505807d9a5984fff37c36fb122", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21676, - { - "block": 4862096, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "sompitonov", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:14:18", - "trx_id": "3f5e92db2e9c54505807d9a5984fff37c36fb122", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21677, - { - "block": 4862180, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "For a long time I have not seen you here.\nBeautiful as always Camilla!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t001828852z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:18:30", - "trx_id": "16e3f759b22037a3d774a64ab4e7a908d34e1a9b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21678, - { - "block": 4862440, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4461", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "9150087358", - "total_vote_weight": "10585536240712091932", - "voter": "rpf", - "weight": "7670923621684784" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:31:30", - "trx_id": "6abec28fe0b14f187825264716d0d76889e5aa8a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21679, - { - "block": 4862440, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "rpf", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:31:30", - "trx_id": "6abec28fe0b14f187825264716d0d76889e5aa8a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21680, - { - "block": 4863012, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4464", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 158821861, - "total_vote_weight": "10585669256066109250", - "voter": "tycho", - "weight": "133015354017318" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:00:12", - "trx_id": "0c5bfc37c9a1cc5c5ea8eb31a9d07cf2405da6f0", - "trx_in_block": 9, - "virtual_op": 1 - } - ], - [ - 21681, - { - "block": 4863012, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "tycho", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:00:12", - "trx_id": "0c5bfc37c9a1cc5c5ea8eb31a9d07cf2405da6f0", - "trx_in_block": 9, - "virtual_op": 0 - } - ], - [ - 21682, - { - "block": 4863030, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5376", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "667121759026", - "total_vote_weight": "11107308194521940665", - "voter": "silver", - "weight": "521638938455831415" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:06", - "trx_id": "7df61195407d5b1fa445ad2da0c684125a1846d1", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21683, - { - "block": 4863030, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "silver", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:06", - "trx_id": "7df61195407d5b1fa445ad2da0c684125a1846d1", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21684, - { - "block": 4863031, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "7922", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "1625458470769", - "total_vote_weight": "12128799463619749613", - "voter": "silversteem", - "weight": "1021491269097808948" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:09", - "trx_id": "a60835407a87606515626dc5552df07b4126ae05", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21685, - { - "block": 4863031, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "silversteem", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:09", - "trx_id": "a60835407a87606515626dc5552df07b4126ae05", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21686, - { - "block": 4863031, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "11322", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "1817776274078", - "total_vote_weight": "12979717477225621428", - "voter": "nextgencrypto", - "weight": "850918013605871815" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:09", - "trx_id": "6323e92940e604a8595e1c60f0048f1e946c36e9", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21687, - { - "block": 4863031, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "nextgencrypto", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:09", - "trx_id": "6323e92940e604a8595e1c60f0048f1e946c36e9", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21688, - { - "block": 4863032, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "42415", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "10514445664165", - "total_vote_weight": "15373717560397404335", - "voter": "berniesanders", - "weight": "2394000083171782907" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:12", - "trx_id": "ddb891664d6d0939fa74ac5b4c263671eed427ef", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21689, - { - "block": 4863032, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "berniesanders", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:12", - "trx_id": "ddb891664d6d0939fa74ac5b4c263671eed427ef", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21690, - { - "block": 4863033, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "43308", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "228778017848", - "total_vote_weight": "15402720955096056998", - "voter": "justin", - "weight": "29003394698652663" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:15", - "trx_id": "fd6b85e506840c49dc2c53cf3b8ad94cd060f160", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21691, - { - "block": 4863033, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "justin", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:15", - "trx_id": "fd6b85e506840c49dc2c53cf3b8ad94cd060f160", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21692, - { - "block": 4863034, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "43837", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "134451929559", - "total_vote_weight": "15419512125538623936", - "voter": "steemservices", - "weight": "16791170442566938" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:18", - "trx_id": "50b3cba71af5e773c5fcfc0439c4cfe435d89013", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21693, - { - "block": 4863034, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "steemservices", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:18", - "trx_id": "50b3cba71af5e773c5fcfc0439c4cfe435d89013", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21694, - { - "block": 4863035, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44092", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "64047892423", - "total_vote_weight": "15427445845003439585", - "voter": "nextgenwitness", - "weight": "7933719464815649" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:21", - "trx_id": "7c71e92dcca5b545a697a7ca8bd029c65ad67938", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21695, - { - "block": 4863035, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "nextgenwitness", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:21", - "trx_id": "7c71e92dcca5b545a697a7ca8bd029c65ad67938", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21696, - { - "block": 4863275, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "43902", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5890677916", - "total_vote_weight": "15428173445384453319", - "voter": "the.bot", - "weight": "727600381013734" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:13:21", - "trx_id": "0bf93bd4edc4db6ba18376ed89798b1719bb21ca", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21697, - { - "block": 4863275, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "the.bot", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:13:21", - "trx_id": "0bf93bd4edc4db6ba18376ed89798b1719bb21ca", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21698, - { - "block": 4863304, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "43970", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "10971913263", - "total_vote_weight": "15429527731559523836", - "voter": "thebotkiller", - "weight": "1354286175070517" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:48", - "trx_id": "a9e26027ff1bdf1d39f5e77341145f3bd77e56f1", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21699, - { - "block": 4863304, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "thebotkiller", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:48", - "trx_id": "a9e26027ff1bdf1d39f5e77341145f3bd77e56f1", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21700, - { - "block": 4863305, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44002", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "8035250377", - "total_vote_weight": "15430518768699936261", - "voter": "johnbradshaw", - "weight": "991037140412425" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:51", - "trx_id": "29720c0a0997ee60889bfe8635bc28fd49e77387", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21701, - { - "block": 4863305, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "johnbradshaw", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:51", - "trx_id": "29720c0a0997ee60889bfe8635bc28fd49e77387", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21702, - { - "block": 4863306, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44029", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "6605970020", - "total_vote_weight": "15431333036355277936", - "voter": "iloveporn", - "weight": "814267655341675" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:54", - "trx_id": "36e35e536a91b2a5188ff45b08a83a398875140f", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21703, - { - "block": 4863306, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "iloveporn", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:54", - "trx_id": "36e35e536a91b2a5188ff45b08a83a398875140f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21704, - { - "block": 4863338, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44090", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "6024180188", - "total_vote_weight": "15432075208030422416", - "voter": "kissmybutt", - "weight": "742171675144480" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:16:30", - "trx_id": "5f05367b69c5bc9bc3db5dcb3e4582e142e88233", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21705, - { - "block": 4863338, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "kissmybutt", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:16:30", - "trx_id": "5f05367b69c5bc9bc3db5dcb3e4582e142e88233", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21706, - { - "block": 4863374, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44140", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5718018880", - "total_vote_weight": "15432779323126653888", - "voter": "the.whale", - "weight": "704115096231472" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:18:18", - "trx_id": "8a63eb11e2e1c6c1d2b7728a3f9efd17c1a1d240", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21707, - { - "block": 4863374, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "the.whale", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:18:18", - "trx_id": "8a63eb11e2e1c6c1d2b7728a3f9efd17c1a1d240", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21708, - { - "block": 4863456, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44255", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5716399817", - "total_vote_weight": "15433482910157930839", - "voter": "vote", - "weight": "703587031276951" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:22:24", - "trx_id": "1664229025160a05681bd822aa26bfa4a27b58e4", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21709, - { - "block": 4863456, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "vote", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:22:24", - "trx_id": "1664229025160a05681bd822aa26bfa4a27b58e4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21710, - { - "block": 4863492, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44292", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5415715980", - "total_vote_weight": "15434149185399671893", - "voter": "unicornfarts", - "weight": "666275241741054" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:24:12", - "trx_id": "3bfda7884bca9ead7019a1734d3e744149b9dc7a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21711, - { - "block": 4863492, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "unicornfarts", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:24:12", - "trx_id": "3bfda7884bca9ead7019a1734d3e744149b9dc7a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21712, - { - "block": 4863566, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44374", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "6684459064", - "total_vote_weight": "15434971143187442470", - "voter": "fuck.off", - "weight": "821957787770577" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:27:54", - "trx_id": "0fd0fa69b5db97b47a9900da886d8c4746c28907", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21713, - { - "block": 4863566, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "fuck.off", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:27:54", - "trx_id": "0fd0fa69b5db97b47a9900da886d8c4746c28907", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21714, - { - "block": 4863594, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44562", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "92325410560", - "total_vote_weight": "15446278274614161998", - "voter": "thecyclist", - "weight": "11307131426719528" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:29:18", - "trx_id": "70265f5e4c1b9df306c65e0e325b154e3c01e22c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21715, - { - "block": 4863594, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "thecyclist", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:29:18", - "trx_id": "70265f5e4c1b9df306c65e0e325b154e3c01e22c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21716, - { - "block": 4863681, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44624", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "6459214622", - "total_vote_weight": "15447066160476335869", - "voter": "bentley", - "weight": "787885862173871" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:33:42", - "trx_id": "115ca2ea95998f73771533eedb501f7651fa8f44", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21717, - { - "block": 4863681, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "bentley", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:33:42", - "trx_id": "115ca2ea95998f73771533eedb501f7651fa8f44", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21718, - { - "block": 4863693, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44637", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 284822451, - "total_vote_weight": "15447100893190931307", - "voter": "jimmytwoshoes", - "weight": "34732714595438" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:34:18", - "trx_id": "9321bbf13dde49da4bc3182781bebff196549ca5", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21719, - { - "block": 4863693, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "jimmytwoshoes", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:34:18", - "trx_id": "9321bbf13dde49da4bc3182781bebff196549ca5", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21720, - { - "block": 4863949, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44818", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "13689306732", - "total_vote_weight": "15448769289856751954", - "voter": "thecurator", - "weight": "1668396665820647" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:47:06", - "trx_id": "334d8d5713e130964f5c8779cb93a09fbe5851f5", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21721, - { - "block": 4863949, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "thecurator", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:47:06", - "trx_id": "334d8d5713e130964f5c8779cb93a09fbe5851f5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21722, - { - "block": 4864302, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44463", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 154551739, - "total_vote_weight": "15448788115395185897", - "voter": "reddust", - "weight": "18825538433943" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T02:04:48", - "trx_id": "690e9fe84997d74ffe42d51eb0a53237954ae4ca", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21723, - { - "block": 4864302, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "reddust", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T02:04:48", - "trx_id": "690e9fe84997d74ffe42d51eb0a53237954ae4ca", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21724, - { - "block": 4866343, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "45862", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "27080912220", - "total_vote_weight": "15452083123473503571", - "voter": "tmendieta", - "weight": "3295008078317674" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T03:47:21", - "trx_id": "9f0e3175ce315d68d3dfb09db5a50d7c2e6501f2", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21725, - { - "block": 4866343, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "tmendieta", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T03:47:21", - "trx_id": "9f0e3175ce315d68d3dfb09db5a50d7c2e6501f2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21726, - { - "block": 4866361, - "op": { - "type": "comment_operation", - "value": { - "author": "tmendieta", - "body": "Beautiful! It must have been relaxing to color that, too.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t034905376z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T03:48:15", - "trx_id": "e735986566a7897dc0b1597a27f67b4edd821991", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21727, - { - "block": 4867542, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-runridefly-re-camilla-colors-of-the-falling-leaves-20160910t234213368z", - "rshares": 595937241, - "total_vote_weight": "2747866053251289", - "voter": "runridefly", - "weight": "2747866053251289" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T04:47:33", - "trx_id": "10d42dc7de5e86fc9ea71b9d87faa9d8eb1a149c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21728, - { - "block": 4867542, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-runridefly-re-camilla-colors-of-the-falling-leaves-20160910t234213368z", - "voter": "runridefly", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T04:47:33", - "trx_id": "10d42dc7de5e86fc9ea71b9d87faa9d8eb1a149c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21729, - { - "block": 4867952, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "47072", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1640965017, - "total_vote_weight": "15452282551414441746", - "voter": "gidlark", - "weight": "199427940938175" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T05:08:06", - "trx_id": "5276e6b8889704c01ba028c0afafeef145d05479", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21730, - { - "block": 4867952, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "gidlark", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T05:08:06", - "trx_id": "5276e6b8889704c01ba028c0afafeef145d05479", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21731, - { - "block": 4868633, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "fairytalelife", - "comment_permlink": "how-to-draw-a-braid", - "curator": "camilla", - "reward": { - "amount": "92369054", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T05:42:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 71 - } - ], - [ - 21732, - { - "block": 4869126, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "47890", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 3600677639, - "total_vote_weight": "15452720051905923183", - "voter": "alexft", - "weight": "437500491481437" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:07:09", - "trx_id": "d02a7d2f24f2d8234bd20ecc0062773dd489dc3c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21733, - { - "block": 4869126, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "alexft", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:07:09", - "trx_id": "d02a7d2f24f2d8234bd20ecc0062773dd489dc3c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21734, - { - "block": 4869148, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "47950", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "7760931567", - "total_vote_weight": "15453662609632857945", - "voter": "sykochica", - "weight": "942557726934762" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:08:18", - "trx_id": "f48cab8e18dcb69fd7a687b23f2f66f6fcdfddc0", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21735, - { - "block": 4869148, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "sykochica", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:08:18", - "trx_id": "f48cab8e18dcb69fd7a687b23f2f66f6fcdfddc0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21736, - { - "block": 4869556, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "48399", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "15163479832", - "total_vote_weight": "15455502488331945333", - "voter": "randyclemens", - "weight": "1839878699087388" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:28:45", - "trx_id": "f4a758d30d1cc9817cbdca59fce5873f7f8ee757", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21737, - { - "block": 4869556, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "randyclemens", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:28:45", - "trx_id": "f4a758d30d1cc9817cbdca59fce5873f7f8ee757", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21738, - { - "block": 4870013, - "op": { - "type": "comment_operation", - "value": { - "author": "alexft", - "body": "I like it ! Great job !!!\nhttps://www.steemimg.com/images/2016/09/11/KALIBRI67d19.gif", - "json_metadata": "{\"tags\":[\"art\"],\"image\":[\"https://www.steemimg.com/images/2016/09/11/KALIBRI67d19.gif\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t065104109z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:51:39", - "trx_id": "e44c8b3f6ad20b94b87eb8d12263da3298a69a78", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21739, - { - "block": 4871461, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "46385", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "8925161251", - "total_vote_weight": "15456584376150636889", - "voter": "kyriacos", - "weight": "1081887818691556" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T08:04:21", - "trx_id": "ff32f21ca0f3403919f9513cae85fb581441eb4f", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21740, - { - "block": 4871461, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "kyriacos", - "weight": 2900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T08:04:21", - "trx_id": "ff32f21ca0f3403919f9513cae85fb581441eb4f", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21741, - { - "block": 4871660, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "infovore", - "comment_permlink": "a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend", - "curator": "camilla", - "reward": { - "amount": "95393246", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T08:14:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 68 - } - ], - [ - 21742, - { - "block": 4871660, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "196940896", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "73", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "vesting_payout": { - "amount": "298488546", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T08:14:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 203 - } - ], - [ - 21743, - { - "block": 4871660, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 193, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "48", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "196", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "total_payout_value": { - "amount": "147", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T08:14:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 204 - } - ], - [ - 21744, - { - "block": 4872663, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "wow! alexft your photoshop skills are amazing :D thank you for making my art come alive!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "alexft", - "parent_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t065104109z", - "permlink": "re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t090442054z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:04:42", - "trx_id": "c5f1bbe41acbf760293579a47248681137035786", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21745, - { - "block": 4872665, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "alexft", - "pending_payout": { - "amount": "95", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t065104109z", - "rshares": "215466659591", - "total_vote_weight": "1089265210197592604", - "voter": "camilla", - "weight": "926752391840549520" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:04:48", - "trx_id": "de837658831f4966fd554d33b973b140e2398eea", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21746, - { - "block": 4872665, - "op": { - "type": "vote_operation", - "value": { - "author": "alexft", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t065104109z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:04:48", - "trx_id": "de837658831f4966fd554d33b973b140e2398eea", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21747, - { - "block": 4872675, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thank you! it was very meditative :D", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "tmendieta", - "parent_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t034905376z", - "permlink": "re-tmendieta-re-camilla-colors-of-the-falling-leaves-20160911t090516241z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:05:18", - "trx_id": "dbc973baece2f36d373ff1e1cf708567aef31690", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21748, - { - "block": 4872677, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "sompitonov", - "pending_payout": { - "amount": "79", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t001828852z", - "rshares": "211241823129", - "total_vote_weight": "925314672627650822", - "voter": "camilla", - "weight": "925314672627650822" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:05:24", - "trx_id": "3d0d8373f759d8604f7373d48cfb4c5632a5e6d7", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21749, - { - "block": 4872677, - "op": { - "type": "vote_operation", - "value": { - "author": "sompitonov", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t001828852z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:05:24", - "trx_id": "3d0d8373f759d8604f7373d48cfb4c5632a5e6d7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21750, - { - "block": 4872683, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "I have been sick but Im back now :D", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "sompitonov", - "parent_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t001828852z", - "permlink": "re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t090541444z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:05:42", - "trx_id": "b789ecfe867331a2a49406fc1d1a91c44f23f60e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21751, - { - "block": 4872697, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "tmendieta", - "pending_payout": { - "amount": "79", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t034905376z", - "rshares": "211241823129", - "total_vote_weight": "925314672627650822", - "voter": "camilla", - "weight": "925314672627650822" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:06:24", - "trx_id": "a2049922a326fead433a4a76649c5fc8454ff9bc", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21752, - { - "block": 4872697, - "op": { - "type": "vote_operation", - "value": { - "author": "tmendieta", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t034905376z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:06:24", - "trx_id": "a2049922a326fead433a4a76649c5fc8454ff9bc", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21753, - { - "block": 4873207, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "With recovery! :D", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t090541444z", - "permlink": "re-camilla-re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t093158180z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:31:57", - "trx_id": "687f00253c6a38c18221a64072ff2ca9d5407ad3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21754, - { - "block": 4873216, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "infovore", - "comment_permlink": "steemmag-steemit-s-weekend-digest-9-of-community-curation-and-reputation-chats-with-a-whale-steemit-chat-lead-moderators-a-day", - "curator": "camilla", - "reward": { - "amount": "113822821", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:32:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 116 - } - ], - [ - 21755, - { - "block": 4873251, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "45438", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "69790115190", - "total_vote_weight": "15465017266140717410", - "voter": "bacchist", - "weight": "8432889990080521" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:34:09", - "trx_id": "24a95834bacb223f1e1074023016818fbe7d6eba", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21756, - { - "block": 4873251, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "bacchist", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:34:09", - "trx_id": "24a95834bacb223f1e1074023016818fbe7d6eba", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21757, - { - "block": 4873672, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "### Where the Trees will not grow in the cold weather, where the houses are far apart but the hearts are close together.\n\nhttps://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14202547_700195886812456_2583028795874812758_n.jpg?oh=4bc315321a733f73f19c05ac7789a9db&oe=587989D0\n\n### My Mother grew up here and I lived my first year in worlds most northern city called Hammerfest. The soil here is frozen, the land is flat and full of stones but still the tourists are very impressed.\n\nhttps://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\n\n### On a small island called Ertsvika my great grand parents lived with their 13 children. It was a hard life in poverty and cold winters, but their health where strong and their hearts where warm.\n\nhttps://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\n\n### They lived of fishing and gathering berries. The next neighbors where 8 hours away by foot. My great grand mother gave birth to all the children without any doctor to help her, the oldest daughter was the midwife. The oldest son had to fish with his father on the stormy ocean when he was 8 years old, he died early from hard work. \nhttps://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14264218_700195843479127_5684473362085111018_n.jpg?oh=f2679a94a09ac2bd847852dcf98df9de&oe=5876E718\n\n### Now the ancestors visit this place from time to time, where the old house used to be there is now a small cabin. There is a peaceful land far far north where nobody lives only birds and raindeers. \n\nhttps://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14289943_700195916812453_6155886871633627964_o.jpg\n\n### The pictures are taken by my mother who is there now, visiting her mother and her childhood places. I hope you enjoyed this glimpse from the past.", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14202547_700195886812456_2583028795874812758_n.jpg?oh=4bc315321a733f73f19c05ac7789a9db&oe=587989D0\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14264218_700195843479127_5684473362085111018_n.jpg?oh=f2679a94a09ac2bd847852dcf98df9de&oe=5876E718\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14289943_700195916812453_6155886871633627964_o.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:24", - "trx_id": "b32e4cb901a4a9975ad1f671e6bbc4f1641118ba", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21758, - { - "block": 4873672, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "79", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "211242392243", - "total_vote_weight": "925317040501509267", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:24", - "trx_id": "b32e4cb901a4a9975ad1f671e6bbc4f1641118ba", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21759, - { - "block": 4873672, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:24", - "trx_id": "b32e4cb901a4a9975ad1f671e6bbc4f1641118ba", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21760, - { - "block": 4873682, - "op": { - "type": "comment_operation", - "value": { - "author": "skeptic", - "body": "dickbutt\nhttps://steemit.com/dickbutt/@skeptic/dickbutt-has-taken-over-steemit", - "json_metadata": "{\"tags\":[\"history\"],\"links\":[\"https://steemit.com/dickbutt/@skeptic/dickbutt-has-taken-over-steemit\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t095553071z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:54", - "trx_id": "ff234139309f83dff10452a8ff8b9ad3542b7569", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21761, - { - "block": 4873683, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "79", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 111977218, - "total_vote_weight": "925782924035033837", - "voter": "skeptic", - "weight": "8541198114617" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:57", - "trx_id": "56e2c19aa29e1762753ece8fbb6ffb29e7a1977e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21762, - { - "block": 4873683, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "skeptic", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:57", - "trx_id": "56e2c19aa29e1762753ece8fbb6ffb29e7a1977e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21763, - { - "block": 4873708, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -127,144 +127,36 @@\n s://\n-scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14202547_700195886812456_2583028795874812758_n.jpg?oh=4bc315321a733f73f19c05ac7789a9db&oe=587989D0\n+i.imgsafe.org/52a5ddd3b8.jpg\n %0A%0A##\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14264218_700195843479127_5684473362085111018_n.jpg?oh=f2679a94a09ac2bd847852dcf98df9de&oe=5876E718\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14289943_700195916812453_6155886871633627964_o.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:57:12", - "trx_id": "0769fda0a66ef464397393c726f24f16d73be912", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21764, - { - "block": 4873750, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -1502,91 +1502,32 @@\n s://\n-scontent-arn2-1.xx.fbcdn.net/t31.0-8/14289943_700195916812453_6155886871633627964_o\n+i.imgsafe.org/52ad9349ed\n .jpg\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14264218_700195843479127_5684473362085111018_n.jpg?oh=f2679a94a09ac2bd847852dcf98df9de&oe=5876E718\",\"https://i.imgsafe.org/52ad9349ed.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:59:18", - "trx_id": "e24fb802687e51a260486c1702d98b1f4f9c04ed", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21765, - { - "block": 4873755, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "375", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "651645205561", - "total_vote_weight": "3273603472621919941", - "voter": "clains", - "weight": "324781842554519244" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:59:33", - "trx_id": "816d4634d0529ccd8da80473220dc190964d6800", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21766, - { - "block": 4873755, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "clains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:59:33", - "trx_id": "816d4634d0529ccd8da80473220dc190964d6800", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21767, - { - "block": 4873759, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "444", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "130765248410", - "total_vote_weight": "3670922843880873379", - "voter": "psylains", - "weight": "57611308832548248" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:59:45", - "trx_id": "9b138b8ad5a1ca9e3653e5c98b5ae848af084c92", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21768, - { - "block": 4873759, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "psylains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:59:45", - "trx_id": "9b138b8ad5a1ca9e3653e5c98b5ae848af084c92", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21769, - { - "block": 4873767, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "447", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "5888362824", - "total_vote_weight": "3688325130236049009", - "voter": "bitspace", - "weight": "2755362006236141" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:09", - "trx_id": "063dd139e93c41c4cee5fa1fc15276468dc5a3b0", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21770, - { - "block": 4873767, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "bitspace", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:09", - "trx_id": "063dd139e93c41c4cee5fa1fc15276468dc5a3b0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21771, - { - "block": 4873778, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "662", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "376233439931", - "total_vote_weight": "4721198610118978963", - "voter": "anonymous", - "weight": "182474314779317625" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:42", - "trx_id": "d8fb5d584bc14a3b9977a9b72db0d9b6af2bafc0", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21772, - { - "block": 4873778, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "anonymous", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:42", - "trx_id": "d8fb5d584bc14a3b9977a9b72db0d9b6af2bafc0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21773, - { - "block": 4873778, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "662", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1413239642, - "total_vote_weight": "4724805901034719817", - "voter": "murh", - "weight": "637288061780884" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:42", - "trx_id": "80531b7655b9c27e072eef7ca0e4ce5996017b35", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21774, - { - "block": 4873778, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "murh", - "weight": 3301 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:42", - "trx_id": "80531b7655b9c27e072eef7ca0e4ce5996017b35", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21775, - { - "block": 4873791, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "664", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1913344238, - "total_vote_weight": "4729686687557132567", - "voter": "earnest", - "weight": "968022660278528" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:01:21", - "trx_id": "b7f83027f36160b219a1ace2c0056b5f496c4f1a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21776, - { - "block": 4873791, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "earnest", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:01:21", - "trx_id": "b7f83027f36160b219a1ace2c0056b5f496c4f1a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21777, - { - "block": 4873814, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -1144,152 +1144,45 @@\n k. %0A\n+%0A\n https://\n-scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14264218_700195843479127_5684473362085111018_n.jpg?oh=f2679a94a09ac2bd847852dcf98df9de&oe=5876E718\n+i.imgsafe.org/52b994c30e.jpg\n %0A%0A##\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\",\"https://i.imgsafe.org/52b994c30e.jpg\",\"https://i.imgsafe.org/52ad9349ed.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:02:30", - "trx_id": "db0fd5d71745c5c000afb12dbc751cc2435cab3d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21778, - { - "block": 4873828, - "op": { - "type": "comment_operation", - "value": { - "author": "earnest", - "body": "are you the same person that sucks the cock stellabelle would like to have?\nthink about it, upvoted", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t100311500z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:03:12", - "trx_id": "8153c8b04e5761bd2332fc0621f296cb05883d50", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21779, - { - "block": 4873842, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -668,144 +668,36 @@\n s://\n-scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\n+i.imgsafe.org/52be4729ec.jpg\n %0A%0A##\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://i.imgsafe.org/52be4729ec.jpg\",\"https://i.imgsafe.org/52b994c30e.jpg\",\"https://i.imgsafe.org/52ad9349ed.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:03:54", - "trx_id": "b4bb1969f4274333db37b79a77eaf5887bd1d90b", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 21780, - { - "block": 4873847, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1130", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "698098902296", - "total_vote_weight": "6305360690629014465", - "voter": "pfunk", - "weight": "459571584229298886" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:04:09", - "trx_id": "46c4fa75449125874544669b5a587d8f22cfe28a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21781, - { - "block": 4873847, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "pfunk", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:04:09", - "trx_id": "46c4fa75449125874544669b5a587d8f22cfe28a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21782, - { - "block": 4873862, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1131", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 62244532, - "total_vote_weight": "6305485042805156949", - "voter": "socialbutterfly", - "weight": "39378189111786" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:04:54", - "trx_id": "5db1f637f4c56c8714e8898140d89d7703623445", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21783, - { - "block": 4873862, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "socialbutterfly", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:04:54", - "trx_id": "5db1f637f4c56c8714e8898140d89d7703623445", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21784, - { - "block": 4873865, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -684,16 +684,16 @@\n g/52\n-be4729ec\n+c38d5ff5\n .jpg\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://i.imgsafe.org/52c38d5ff5.jpg\",\"https://i.imgsafe.org/52b994c30e.jpg\",\"https://i.imgsafe.org/52ad9349ed.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:05:03", - "trx_id": "4bdf442c011e572ccb86688a0a39adbcccd88441", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21785, - { - "block": 4873874, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1132", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 626660650, - "total_vote_weight": "6306736844055956688", - "voter": "steem1653", - "weight": "421439754435912" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:05:30", - "trx_id": "359a1dc478b58b6b42003bfa8df871f61f1d5760", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21786, - { - "block": 4873874, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "steem1653", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:05:30", - "trx_id": "359a1dc478b58b6b42003bfa8df871f61f1d5760", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21787, - { - "block": 4873883, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1147", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "20202699413", - "total_vote_weight": "6346955395012250957", - "voter": "samether", - "weight": "14143523752963484" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:05:57", - "trx_id": "ed3a545aee0815df385adbb2c7f3c8a7ea25f6b7", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21788, - { - "block": 4873883, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "samether", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:05:57", - "trx_id": "ed3a545aee0815df385adbb2c7f3c8a7ea25f6b7", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21789, - { - "block": 4873889, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -373,90 +373,32 @@\n s://\n-scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o\n+i.imgsafe.org/52c7a83b71\n .jpg\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://i.imgsafe.org/52c7a83b71.jpg\",\"https://i.imgsafe.org/52c38d5ff5.jpg\",\"https://i.imgsafe.org/52b994c30e.jpg\",\"https://i.imgsafe.org/52ad9349ed.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:06:15", - "trx_id": "971676eac8a88d8c3be317b4ccd1569c4eea1431", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21790, - { - "block": 4873900, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dickbutt", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-re-skeptic-re-camilla-there-is-a-land-far-far-north-20160911t100406200z-20160911t100502", - "rshares": -211242392243, - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:06:48", - "trx_id": "bf83b6b33e4422813a97edbd8772a87bc03fcfcb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21791, - { - "block": 4873900, - "op": { - "type": "vote_operation", - "value": { - "author": "dickbutt", - "permlink": "re-re-skeptic-re-camilla-there-is-a-land-far-far-north-20160911t100406200z-20160911t100502", - "voter": "camilla", - "weight": -10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:06:48", - "trx_id": "bf83b6b33e4422813a97edbd8772a87bc03fcfcb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21792, - { - "block": 4873903, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "skeptic", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t095553071z", - "rshares": -211242392243, - "total_vote_weight": "8819524174382965", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:06:57", - "trx_id": "01afbe2fd76f15a55e1dd10f7f9c8e176847d67f", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21793, - { - "block": 4873903, - "op": { - "type": "vote_operation", - "value": { - "author": "skeptic", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t095553071z", - "voter": "camilla", - "weight": -10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:06:57", - "trx_id": "01afbe2fd76f15a55e1dd10f7f9c8e176847d67f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21794, - { - "block": 4873904, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dickbutt", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-re-camilla-there-is-a-land-far-far-north-20160911t095553071z-20160911t095642", - "rshares": -207017544398, - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:07:00", - "trx_id": "957328c81b3dc0f731fbafc2d6535f4840ed0e55", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21795, - { - "block": 4873904, - "op": { - "type": "vote_operation", - "value": { - "author": "dickbutt", - "permlink": "re-re-camilla-there-is-a-land-far-far-north-20160911t095553071z-20160911t095642", - "voter": "camilla", - "weight": -10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:07:00", - "trx_id": "957328c81b3dc0f731fbafc2d6535f4840ed0e55", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21796, - { - "block": 4873920, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1149", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1333641507, - "total_vote_weight": "6349600969497575589", - "voter": "elishagh1", - "weight": "1093504120600847" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:07:48", - "trx_id": "0df67e4c9953df2e06c8063fa80a654ee4130e3e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21797, - { - "block": 4873920, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "elishagh1", - "weight": 400 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:07:48", - "trx_id": "0df67e4c9953df2e06c8063fa80a654ee4130e3e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21798, - { - "block": 4873951, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1158", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "11521200292", - "total_vote_weight": "6372407756132747621", - "voter": "asim", - "weight": "10605155785354994" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:09:21", - "trx_id": "26b05ed779b77641ff1dbc6ffb0a452ad252f22a", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21799, - { - "block": 4873951, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "asim", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:09:21", - "trx_id": "26b05ed779b77641ff1dbc6ffb0a452ad252f22a", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21800, - { - "block": 4873965, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1190", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "42712960461", - "total_vote_weight": "6456215003138078692", - "voter": "alexgr", - "weight": "40925872287603339" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:10:03", - "trx_id": "d2ceb9ed7c5622a3326d58648c5dafbab81dc447", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 21801, - { - "block": 4873965, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "alexgr", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:10:03", - "trx_id": "d2ceb9ed7c5622a3326d58648c5dafbab81dc447", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 21802, - { - "block": 4873970, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "peskov", - "pending_payout": { - "amount": "30320", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-cup-of-tea-featuring-vasily-peskov-as-author", - "rshares": "207017544398", - "total_vote_weight": "14843396579493870159", - "voter": "camilla", - "weight": "36800406375438646" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:10:18", - "trx_id": "20f77aa8acf04044724eaec08df0d2aa334f848b", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21803, - { - "block": 4873970, - "op": { - "type": "vote_operation", - "value": { - "author": "peskov", - "permlink": "a-cup-of-tea-featuring-vasily-peskov-as-author", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:10:18", - "trx_id": "20f77aa8acf04044724eaec08df0d2aa334f848b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21804, - { - "block": 4873984, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1192", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1640980092, - "total_vote_weight": "6459411575179279942", - "voter": "gidlark", - "weight": "1662217461424650" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:11:00", - "trx_id": "ca6c79970f2b858d8d378cfb8089647bbaaf5607", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21805, - { - "block": 4873984, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "gidlark", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:11:00", - "trx_id": "ca6c79970f2b858d8d378cfb8089647bbaaf5607", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21806, - { - "block": 4874034, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3096", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "2044001489909", - "total_vote_weight": "9447689215416383726", - "voter": "badassmother", - "weight": "1802927509609719283" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:30", - "trx_id": "131a48dc630eff741b957976f75b36255be63310", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21807, - { - "block": 4874034, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "badassmother", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:30", - "trx_id": "131a48dc630eff741b957976f75b36255be63310", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21808, - { - "block": 4874036, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3097", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 664445440, - "total_vote_weight": "9448418401242171671", - "voter": "karen13", - "weight": "442372734311353" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:36", - "trx_id": "a68235ba03aefdd2327d72c4ce1ad2e6e39019ae", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21809, - { - "block": 4874036, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "karen13", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:36", - "trx_id": "a68235ba03aefdd2327d72c4ce1ad2e6e39019ae", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21810, - { - "block": 4874037, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3098", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1378514942, - "total_vote_weight": "9449930855245003686", - "voter": "taker", - "weight": "920076185056142" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:39", - "trx_id": "c9c155ae36f3707944785e9c6e3f6a9f1fda6c31", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21811, - { - "block": 4874037, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "taker", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:39", - "trx_id": "c9c155ae36f3707944785e9c6e3f6a9f1fda6c31", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21812, - { - "block": 4874040, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3100", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1027472226, - "total_vote_weight": "9451057827907077089", - "voter": "coar", - "weight": "691209899405020" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:48", - "trx_id": "ecbcaad710fa40faa03849adce42a988b02c8c33", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21813, - { - "block": 4874040, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "coar", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:48", - "trx_id": "ecbcaad710fa40faa03849adce42a988b02c8c33", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21814, - { - "block": 4874049, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3101", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 728479455, - "total_vote_weight": "9451856682294078194", - "voter": "alexma3x", - "weight": "501946839832360" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:15", - "trx_id": "7fad568fc22c5b1f991dd4b00416141facc90d36", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21815, - { - "block": 4874049, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "alexma3x", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:15", - "trx_id": "7fad568fc22c5b1f991dd4b00416141facc90d36", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21816, - { - "block": 4874049, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3101", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 336979073, - "total_vote_weight": "9452226167316916552", - "voter": "robotev1", - "weight": "232159756016768" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:15", - "trx_id": "3def6b10ce324851a9427e4784b8523eeb139edb", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21817, - { - "block": 4874049, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "robotev1", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:15", - "trx_id": "3def6b10ce324851a9427e4784b8523eeb139edb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21818, - { - "block": 4874052, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3105", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 3258419015, - "total_vote_weight": "9455797337387892571", - "voter": "glitterpig", - "weight": "2261741044951478" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:24", - "trx_id": "4fa2e3c409234651b1fd37d76ed9619789fd45dc", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21819, - { - "block": 4874052, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "glitterpig", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:24", - "trx_id": "4fa2e3c409234651b1fd37d76ed9619789fd45dc", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21820, - { - "block": 4874052, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4467", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "1119181397810", - "total_vote_weight": "10534771329717568694", - "voter": "rossco99", - "weight": "683350195142128211" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:24", - "trx_id": "a5a541e8cec1d504d5e034e27d0c92fd7f07abb8", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21821, - { - "block": 4874052, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "rossco99", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:24", - "trx_id": "a5a541e8cec1d504d5e034e27d0c92fd7f07abb8", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21822, - { - "block": 4874053, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5095", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "461536194020", - "total_vote_weight": "10907864778260602227", - "voter": "boatymcboatface", - "weight": "236914339824826293" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:27", - "trx_id": "00f0b60e6ae471301785e2e51f694f8b4b839d48", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21823, - { - "block": 4874053, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "boatymcboatface", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:27", - "trx_id": "00f0b60e6ae471301785e2e51f694f8b4b839d48", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21824, - { - "block": 4874132, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5109", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 3066392990, - "total_vote_weight": "10910225939460354233", - "voter": "funnyman", - "weight": "1814158855142791" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:18:27", - "trx_id": "96934940eb4f05632438487188653ae662a21e61", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21825, - { - "block": 4874132, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "funnyman", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:18:27", - "trx_id": "96934940eb4f05632438487188653ae662a21e61", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21826, - { - "block": 4874199, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5492", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "261981171388", - "total_vote_weight": "11106635929173180858", - "voter": "liberosist", - "weight": "172840790947287430" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:21:48", - "trx_id": "899c30727b0dca6e9627e7492d16b41bd9b8ddc6", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21827, - { - "block": 4874199, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "liberosist", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:21:48", - "trx_id": "899c30727b0dca6e9627e7492d16b41bd9b8ddc6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21828, - { - "block": 4874220, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5508", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "8953896241", - "total_vote_weight": "11113167995769612250", - "voter": "doitvoluntarily", - "weight": "5976840935734723" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:22:51", - "trx_id": "dd90f380d37188236a062c4dc48e566d1abd0ab2", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21829, - { - "block": 4874220, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "doitvoluntarily", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:22:51", - "trx_id": "dd90f380d37188236a062c4dc48e566d1abd0ab2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21830, - { - "block": 4874232, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5555", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "32296817492", - "total_vote_weight": "11136632954558278245", - "voter": "jl777", - "weight": "21939736467402705" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:27", - "trx_id": "dffeaaa4f3d4e8087d5d9fe49997413416439ed6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21831, - { - "block": 4874232, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "jl777", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:27", - "trx_id": "dffeaaa4f3d4e8087d5d9fe49997413416439ed6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21832, - { - "block": 4874233, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5559", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 2725653124, - "total_vote_weight": "11138606383854336184", - "voter": "proto", - "weight": "1848445440640936" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:30", - "trx_id": "9267caa121a1fafdd6cef0fd1132c17ffd5fa8e8", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21833, - { - "block": 4874233, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "proto", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:30", - "trx_id": "9267caa121a1fafdd6cef0fd1132c17ffd5fa8e8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21834, - { - "block": 4874235, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "649886", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-peek-into-pre-columbian-worldviews-found-in-agricultural-practices-duality-complementarity-cyclicity-and-reciprocity", - "rshares": "207017544398", - "total_vote_weight": "17597014638569603285", - "voter": "camilla", - "weight": "2030315015980568" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:36", - "trx_id": "b204fc4e4bce6248b4d66f856ea415a0e1c07c94", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21835, - { - "block": 4874235, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "a-peek-into-pre-columbian-worldviews-found-in-agricultural-practices-duality-complementarity-cyclicity-and-reciprocity", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:36", - "trx_id": "b204fc4e4bce6248b4d66f856ea415a0e1c07c94", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21836, - { - "block": 4874251, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5561", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 50985709, - "total_vote_weight": "11138643288411490344", - "voter": "natord", - "weight": "35674405249021" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:24:24", - "trx_id": "ca55341b991a2b4a7632455f9251f037d44eb4af", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21837, - { - "block": 4874251, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "natord", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:24:24", - "trx_id": "ca55341b991a2b4a7632455f9251f037d44eb4af", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21838, - { - "block": 4874528, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "14", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "a-quick-steem-elf-drawing", - "vesting_payout": { - "amount": "61510564", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21839, - { - "block": 4874528, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 39, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "835", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "29", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-quick-steem-elf-drawing", - "total_payout_value": { - "amount": "6987", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 21840, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "a-quick-steem-elf-drawing" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21841, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-ryan-singer-re-camilla-a-quick-steem-elf-drawing-20160811t215726138z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 21842, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-hedge-x-re-camilla-a-quick-steem-elf-drawing-20160811t215808014z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 21843, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-opheliafu-re-camilla-a-quick-steem-elf-drawing-20160811t222944221z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 10 - } - ], - [ - 21844, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-bullionstackers-re-camilla-a-quick-steem-elf-drawing-20160812t125816467z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 22 - } - ], - [ - 21845, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-juvyjabian-re-camilla-a-quick-steem-elf-drawing-20160812t125915459z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 23 - } - ], - [ - 21846, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-bullionstackers-re-camilla-re-bullionstackers-re-camilla-a-quick-steem-elf-drawing-20160813t152806440z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 25 - } - ], - [ - 21847, - { - "block": 4874585, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5609", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 3484563685, - "total_vote_weight": "11141164607951402125", - "voter": "alexft", - "weight": "2521319539911781" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:41:09", - "trx_id": "4dd1e57bda23e6be0f4859f3c26b3e859c22da8c", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21848, - { - "block": 4874585, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "alexft", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:41:09", - "trx_id": "4dd1e57bda23e6be0f4859f3c26b3e859c22da8c", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21849, - { - "block": 4874635, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5613", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 348014675, - "total_vote_weight": "11141416324760589706", - "voter": "bullionstackers", - "weight": "251716809187581" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:43:39", - "trx_id": "6e8d94c279015618890f109df0d248d9d35a4309", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21850, - { - "block": 4874635, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "bullionstackers", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:43:39", - "trx_id": "6e8d94c279015618890f109df0d248d9d35a4309", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21851, - { - "block": 4874832, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "lyubovbar", - "comment_permlink": "gentle-casserole-of-minced-chicken-and-vegetables-recipe-and-cooking-lyubovbar", - "curator": "camilla", - "reward": { - "amount": "76883771", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:53:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21852, - { - "block": 4875179, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5865", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "131708490559", - "total_vote_weight": "11235450760796871897", - "voter": "team", - "weight": "94034436036282191" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:10:57", - "trx_id": "0b795c1b9159f5105a8ec197a81b7e0c704acb03", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21853, - { - "block": 4875179, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "team", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:10:57", - "trx_id": "0b795c1b9159f5105a8ec197a81b7e0c704acb03", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21854, - { - "block": 4875324, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6235", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "226192934152", - "total_vote_weight": "11391416660045886007", - "voter": "jesta", - "weight": "155965899249014110" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:18:12", - "trx_id": "1800b8fa05d4e0f9ed5edf5e567d2f0aa34abf0a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21855, - { - "block": 4875324, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "jesta", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:18:12", - "trx_id": "1800b8fa05d4e0f9ed5edf5e567d2f0aa34abf0a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21856, - { - "block": 4875398, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "jamielefay", - "pending_payout": { - "amount": "60657", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "ahe-ey-allegiance-an-original-novel-part-18", - "rshares": "207018222530", - "total_vote_weight": "15781801981480933436", - "voter": "camilla", - "weight": "20075379046871439" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:00", - "trx_id": "822ceea944fc6db4a483737a91c09b8f5f7aa71e", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21857, - { - "block": 4875398, - "op": { - "type": "vote_operation", - "value": { - "author": "jamielefay", - "permlink": "ahe-ey-allegiance-an-original-novel-part-18", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:00", - "trx_id": "822ceea944fc6db4a483737a91c09b8f5f7aa71e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21858, - { - "block": 4875400, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "complexring", - "pending_payout": { - "amount": "1159634", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "bypassing-the-great-firewall-of-china", - "rshares": "207018222530", - "total_vote_weight": "17799465840281566834", - "voter": "camilla", - "weight": "1177606706720511" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:06", - "trx_id": "b96ed906ee75ba295a8682a2b00f4c0fe9578750", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21859, - { - "block": 4875400, - "op": { - "type": "vote_operation", - "value": { - "author": "complexring", - "permlink": "bypassing-the-great-firewall-of-china", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:06", - "trx_id": "b96ed906ee75ba295a8682a2b00f4c0fe9578750", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21860, - { - "block": 4875401, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "smailer", - "pending_payout": { - "amount": "37201", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-daytime-latte-art-tryings-200-followers-milestone", - "rshares": "207018222530", - "total_vote_weight": "15116351817870209333", - "voter": "camilla", - "weight": "31412079103338308" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:09", - "trx_id": "6af7ebc02d31c437c2c33ee97184d7c59d757344", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21861, - { - "block": 4875401, - "op": { - "type": "vote_operation", - "value": { - "author": "smailer", - "permlink": "my-daytime-latte-art-tryings-200-followers-milestone", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:09", - "trx_id": "6af7ebc02d31c437c2c33ee97184d7c59d757344", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21862, - { - "block": 4875405, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "aaronkoenig", - "pending_payout": { - "amount": "147540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "steemit-meetup-berlin-with-ned-scott-and-steemians-from-hamburg", - "rshares": "202793360846", - "total_vote_weight": "5016696368721867925", - "voter": "camilla", - "weight": "14826712462658847" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:21", - "trx_id": "2f4e6939c728fc2c7def8f5ad6ff7901bff38bbb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21863, - { - "block": 4875405, - "op": { - "type": "vote_operation", - "value": { - "author": "aaronkoenig", - "permlink": "steemit-meetup-berlin-with-ned-scott-and-steemians-from-hamburg", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:21", - "trx_id": "2f4e6939c728fc2c7def8f5ad6ff7901bff38bbb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21864, - { - "block": 4875430, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "ekaterina4ka", - "comment_permlink": "knitting-needles-my-passion-post-14-vyazanie-spicami-moe-uvlechenie-post-14", - "curator": "camilla", - "reward": { - "amount": "79950080", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:23:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21865, - { - "block": 4875431, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "royalmacro", - "pending_payout": { - "amount": "75139", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "moonlit-night-an-original-abstract-art-and-a-poem", - "rshares": "202793744606", - "total_vote_weight": "16036119854337407944", - "voter": "camilla", - "weight": "16077583325629225" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:23:39", - "trx_id": "f259c00f8ceda578a82526460f2eb9e100c6a36d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21866, - { - "block": 4875431, - "op": { - "type": "vote_operation", - "value": { - "author": "royalmacro", - "permlink": "moonlit-night-an-original-abstract-art-and-a-poem", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:23:39", - "trx_id": "f259c00f8ceda578a82526460f2eb9e100c6a36d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21867, - { - "block": 4875443, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "labradorsem", - "pending_payout": { - "amount": "171934", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "diet-apple-pie-with-apples-and-pears", - "rshares": "202793744606", - "total_vote_weight": "16817917632697667692", - "voter": "camilla", - "weight": "7324415880569540" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:24:15", - "trx_id": "960fd778a7cf80f2a3aa747a2670e311c701a6ca", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21868, - { - "block": 4875443, - "op": { - "type": "vote_operation", - "value": { - "author": "labradorsem", - "permlink": "diet-apple-pie-with-apples-and-pears", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:24:15", - "trx_id": "960fd778a7cf80f2a3aa747a2670e311c701a6ca", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21869, - { - "block": 4875689, - "op": { - "type": "comment_operation", - "value": { - "author": "alexft", - "body": "=) Thank you for your art !!!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t090442054z", - "permlink": "re-camilla-re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t113557524z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:36:33", - "trx_id": "fdea88359b8d845d2a96c1ac35f852231ba5f8d9", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21870, - { - "block": 4875691, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t090442054z", - "rshares": 3484600591, - "total_vote_weight": "16055896728511211", - "voter": "alexft", - "weight": "16055896728511211" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:36:39", - "trx_id": "fb77e3168e5e01387447a9fc26eda34b51bccf9a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21871, - { - "block": 4875691, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t090442054z", - "voter": "alexft", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:36:39", - "trx_id": "fb77e3168e5e01387447a9fc26eda34b51bccf9a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21872, - { - "block": 4875787, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "xiaofang", - "pending_payout": { - "amount": "167211", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-photography-flower-collections", - "rshares": "202793744606", - "total_vote_weight": "16801622792369674535", - "voter": "camilla", - "weight": "7472032505573390" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:41:30", - "trx_id": "f5b6b4ad06a33ba3d187d8b4eb8c6bdd478a56a3", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21873, - { - "block": 4875787, - "op": { - "type": "vote_operation", - "value": { - "author": "xiaofang", - "permlink": "my-photography-flower-collections", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:41:30", - "trx_id": "f5b6b4ad06a33ba3d187d8b4eb8c6bdd478a56a3", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21874, - { - "block": 4875794, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dumar022", - "pending_payout": { - "amount": "174056", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "i-never-plan-to-get-a-new-cactus-but-it-happens-all-the-time", - "rshares": "202793744606", - "total_vote_weight": "16832681387978976235", - "voter": "camilla", - "weight": "7191946835167933" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:41:51", - "trx_id": "5d36cab49154a00be1775a173967bfd3915b55bc", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21875, - { - "block": 4875794, - "op": { - "type": "vote_operation", - "value": { - "author": "dumar022", - "permlink": "i-never-plan-to-get-a-new-cactus-but-it-happens-all-the-time", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:41:51", - "trx_id": "5d36cab49154a00be1775a173967bfd3915b55bc", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21876, - { - "block": 4875855, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6196", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "17414401735", - "total_vote_weight": "11403145110532426308", - "voter": "r4fken", - "weight": "11728450486540301" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:44:54", - "trx_id": "f62ad22c4d94603c925d24caab40a552f965c76b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21877, - { - "block": 4875855, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "r4fken", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:44:54", - "trx_id": "f62ad22c4d94603c925d24caab40a552f965c76b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21878, - { - "block": 4875953, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "diana.catherine", - "pending_payout": { - "amount": "29058", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-last-days-in-greece-cloudy-but-beautiful-shocked-but-alive-part-3", - "rshares": "198568874927", - "total_vote_weight": "14745668559402477023", - "voter": "camilla", - "weight": "37233564351029599" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:49:48", - "trx_id": "69f54f6f36b4fbdbf8f9df148b519d94ea6935ba", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21879, - { - "block": 4875953, - "op": { - "type": "vote_operation", - "value": { - "author": "diana.catherine", - "permlink": "my-last-days-in-greece-cloudy-but-beautiful-shocked-but-alive-part-3", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:49:48", - "trx_id": "69f54f6f36b4fbdbf8f9df148b519d94ea6935ba", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21880, - { - "block": 4876379, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-clains-where-does-the-money-in-steem-come-from-20160811t223515052z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:11:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 11 - } - ], - [ - 21881, - { - "block": 4876485, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6099", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 3985741522, - "total_vote_weight": "11405823991723011311", - "voter": "sompitonov", - "weight": "2678881190585003" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:16:27", - "trx_id": "563421818674195d2d64ffebcd9c5fa84b36b5f9", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21882, - { - "block": 4876485, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "sompitonov", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:16:27", - "trx_id": "563421818674195d2d64ffebcd9c5fa84b36b5f9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21883, - { - "block": 4876557, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6096", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "22980208089", - "total_vote_weight": "11421229704066695932", - "voter": "jasonstaggers", - "weight": "15405712343684621" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:20:03", - "trx_id": "d1ad0fddbba0cb48b64a575f8706c80b75f53117", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21884, - { - "block": 4876557, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "jasonstaggers", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:20:03", - "trx_id": "d1ad0fddbba0cb48b64a575f8706c80b75f53117", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21885, - { - "block": 4877027, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6164", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "8338989775", - "total_vote_weight": "11426803425944967022", - "voter": "givemeyoursteem", - "weight": "5573721878271090" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:43:42", - "trx_id": "f6126443775983f307d39bbf3ae139e14a72c9bc", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21886, - { - "block": 4877027, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "givemeyoursteem", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:43:42", - "trx_id": "f6126443775983f307d39bbf3ae139e14a72c9bc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21887, - { - "block": 4877054, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "46123", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "7998622846", - "total_vote_weight": "15465980719851167154", - "voter": "givemeyoursteem", - "weight": "963453710449744" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:45:03", - "trx_id": "967eeeaff88d57ca73c16edfece0f646777814d5", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21888, - { - "block": 4877054, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "givemeyoursteem", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:45:03", - "trx_id": "967eeeaff88d57ca73c16edfece0f646777814d5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21889, - { - "block": 4877111, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "cold , but very beautiful place!", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:47:54", - "trx_id": "cdc93bf1db588695aaa46a29dc2cf97f92e5ebb6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21890, - { - "block": 4877118, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "cold, but very beautiful place!", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:48:15", - "trx_id": "cc8b2a5acdd2a8079acbff6191829d2fa04357cb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21891, - { - "block": 4877125, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "cold, but very beautiful places!", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:48:36", - "trx_id": "e5a2bac75119d8127c38a6764f13f114a462bbef", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21892, - { - "block": 4877146, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "sompitonov", - "pending_payout": { - "amount": "76", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "rshares": "202793744606", - "total_vote_weight": "890094668884779200", - "voter": "camilla", - "weight": "51922189018278786" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:49:39", - "trx_id": "cf10740bca5bb858bda2d69878d2bf31f6739c60", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21893, - { - "block": 4877146, - "op": { - "type": "vote_operation", - "value": { - "author": "sompitonov", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:49:39", - "trx_id": "cf10740bca5bb858bda2d69878d2bf31f6739c60", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21894, - { - "block": 4877152, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "yes it is beautiful in the summer :)", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "sompitonov", - "parent_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "permlink": "re-sompitonov-re-camilla-there-is-a-land-far-far-north-20160911t124956506z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:49:57", - "trx_id": "0bac48e1b70f88c205eb2cbe1edbce7206a8bb62", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21895, - { - "block": 4877179, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dailybest", - "pending_payout": { - "amount": "143239", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "sketch-4-birds-in-love", - "rshares": "198568874927", - "total_vote_weight": "16806047982407796105", - "voter": "camilla", - "weight": "7276282158252599" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:51:18", - "trx_id": "0739fba20ea9a40cdd32106088dff1257e30c1d2", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21896, - { - "block": 4877179, - "op": { - "type": "vote_operation", - "value": { - "author": "dailybest", - "permlink": "sketch-4-birds-in-love", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:51:18", - "trx_id": "0739fba20ea9a40cdd32106088dff1257e30c1d2", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21897, - { - "block": 4877185, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dailybest", - "pending_payout": { - "amount": "141859", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "sketch-4-birds-in-love", - "rshares": 4224869679, - "total_vote_weight": "16798771700249543506", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:51:36", - "trx_id": "8af4d88f84027314a556a131b34a6e55d956d279", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21898, - { - "block": 4877185, - "op": { - "type": "vote_operation", - "value": { - "author": "dailybest", - "permlink": "sketch-4-birds-in-love", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:51:36", - "trx_id": "8af4d88f84027314a556a131b34a6e55d956d279", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21899, - { - "block": 4877355, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "alexft", - "pending_payout": { - "amount": "73", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t113557524z", - "rshares": "198568874927", - "total_vote_weight": "872428040577635556", - "voter": "camilla", - "weight": "872428040577635556" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:00:09", - "trx_id": "4ea1437797feb5ff91c3e5f0bebceff16388733c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21900, - { - "block": 4877355, - "op": { - "type": "vote_operation", - "value": { - "author": "alexft", - "permlink": "re-camilla-re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t113557524z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:00:09", - "trx_id": "4ea1437797feb5ff91c3e5f0bebceff16388733c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21901, - { - "block": 4877360, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "sompitonov", - "pending_payout": { - "amount": "73", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t093158180z", - "rshares": "198568874927", - "total_vote_weight": "872428040577635556", - "voter": "camilla", - "weight": "872428040577635556" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:00:24", - "trx_id": "954cd9fa0c07baac4df1c975a3318e0cbb6d2e3a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21902, - { - "block": 4877360, - "op": { - "type": "vote_operation", - "value": { - "author": "sompitonov", - "permlink": "re-camilla-re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t093158180z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:00:24", - "trx_id": "954cd9fa0c07baac4df1c975a3318e0cbb6d2e3a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21903, - { - "block": 4877847, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5984", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 327972405, - "total_vote_weight": "11427022459635292626", - "voter": "valenttina", - "weight": "219033690325604" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:25:00", - "trx_id": "ddc2b116e4a2b46e0ec564d68170d9786af97c68", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21904, - { - "block": 4877847, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "valenttina", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:25:00", - "trx_id": "ddc2b116e4a2b46e0ec564d68170d9786af97c68", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21905, - { - "block": 4878056, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "opheliafu", - "pending_payout": { - "amount": "1468", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-blue-raven", - "rshares": "198568874927", - "total_vote_weight": "7219155244931895403", - "voter": "camilla", - "weight": "86285797782688759" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:35:30", - "trx_id": "f4740723aed0bf84cf0023589d43c3687ff47f92", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21906, - { - "block": 4878056, - "op": { - "type": "vote_operation", - "value": { - "author": "opheliafu", - "permlink": "the-blue-raven", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:35:30", - "trx_id": "f4740723aed0bf84cf0023589d43c3687ff47f92", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21907, - { - "block": 4878079, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "terrycraft", - "pending_payout": { - "amount": "286113", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "traveling-to-peru-to-the-wild-jungle-through-the-city-of-shamans-iquitos-puteshestvie-v-ikitos-featuring-njall-as-author", - "rshares": "198568874927", - "total_vote_weight": "17204723480537767766", - "voter": "camilla", - "weight": "3700147498508231" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:36:39", - "trx_id": "fa03bc1d5f1aa03ba698e8c2326205100bde0a41", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21908, - { - "block": 4878079, - "op": { - "type": "vote_operation", - "value": { - "author": "terrycraft", - "permlink": "traveling-to-peru-to-the-wild-jungle-through-the-city-of-shamans-iquitos-puteshestvie-v-ikitos-featuring-njall-as-author", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:36:39", - "trx_id": "fa03bc1d5f1aa03ba698e8c2326205100bde0a41", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21909, - { - "block": 4878288, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6005", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "27088176517", - "total_vote_weight": "11445066013656557447", - "voter": "tmendieta", - "weight": "18043554021264821" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:47:09", - "trx_id": "156d892d824259fe4ee7bef08a04341910f6abb4", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21910, - { - "block": 4878288, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "tmendieta", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:47:09", - "trx_id": "156d892d824259fe4ee7bef08a04341910f6abb4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21911, - { - "block": 4879035, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5886", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "10310589431", - "total_vote_weight": "11451909593834525458", - "voter": "mun", - "weight": "6843580177968011" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T14:24:39", - "trx_id": "66285ae6cb89f738a317b7aed932531ffcf160e5", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21912, - { - "block": 4879035, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "mun", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T14:24:39", - "trx_id": "66285ae6cb89f738a317b7aed932531ffcf160e5", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21913, - { - "block": 4880294, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5776", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "16193082064", - "total_vote_weight": "11462630673607067285", - "voter": "b4bb4r-5h3r", - "weight": "10721079772541827" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T15:27:57", - "trx_id": "0abbcd564fb2eea5c6030eb23372418bbefd20d6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21914, - { - "block": 4880294, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "b4bb4r-5h3r", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T15:27:57", - "trx_id": "0abbcd564fb2eea5c6030eb23372418bbefd20d6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21915, - { - "block": 4880302, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5798", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "14860602062", - "total_vote_weight": "11472440669147249884", - "voter": "randyclemens", - "weight": "9809995540182599" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T15:28:21", - "trx_id": "e304954a7d34fa662b577685b134d2a2f74dc6ca", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21916, - { - "block": 4880302, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "randyclemens", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T15:28:21", - "trx_id": "e304954a7d34fa662b577685b134d2a2f74dc6ca", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21917, - { - "block": 4880788, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-fairytalelife-for-the-love-of-a-pug-20160812t125401776z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T15:52:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 29 - } - ], - [ - 21918, - { - "block": 4880977, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-sompitonov-re-camilla-there-is-a-land-far-far-north-20160911t124956506z", - "rshares": 3985741522, - "total_vote_weight": "18362691214865208", - "voter": "sompitonov", - "weight": "18362691214865208" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T16:02:18", - "trx_id": "6f301c16cb86c26c3aadb5c2312dd7031424544d", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21919, - { - "block": 4880977, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-sompitonov-re-camilla-there-is-a-land-far-far-north-20160911t124956506z", - "voter": "sompitonov", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T16:02:18", - "trx_id": "6f301c16cb86c26c3aadb5c2312dd7031424544d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21920, - { - "block": 4883321, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "diana.catherine", - "comment_permlink": "my-last-days-in-greece-cloudy-but-beautiful-shocked-but-alive-part-3", - "curator": "camilla", - "reward": { - "amount": "67549036", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T18:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 13 - } - ], - [ - 21921, - { - "block": 4884483, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5727", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "24219759293", - "total_vote_weight": "11488370043363254252", - "voter": "thecryptofiend", - "weight": "15929374216004368" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T18:58:30", - "trx_id": "62070e5269dea8573ef09708be431a75148edb09", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21922, - { - "block": 4884483, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "thecryptofiend", - "weight": 3100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T18:58:30", - "trx_id": "62070e5269dea8573ef09708be431a75148edb09", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21923, - { - "block": 4884669, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5760", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "51727323862", - "total_vote_weight": "11522148707244888613", - "voter": "kus-knee", - "weight": "33778663881634361" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T19:07:48", - "trx_id": "36a382c40cb4c534084e96e7d053bdf18d926b23", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21924, - { - "block": 4884669, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "kus-knee", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T19:07:48", - "trx_id": "36a382c40cb4c534084e96e7d053bdf18d926b23", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21925, - { - "block": 4884684, - "op": { - "type": "comment_operation", - "value": { - "author": "kus-knee", - "body": "Very cool shots! UPVOTED!\n\n@kus-knee (The Old Dog)", - "json_metadata": "{\"tags\":[\"history\"],\"users\":[\"kus-knee\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t190836197z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T19:08:36", - "trx_id": "c5417b6d7028dff543bdd901e10a593416f260c1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21926, - { - "block": 4885364, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "kus-knee", - "pending_payout": { - "amount": "71", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t190836197z", - "rshares": "207018945276", - "total_vote_weight": "907727193908588940", - "voter": "camilla", - "weight": "907727193908588940" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T19:42:42", - "trx_id": "641461be71d572c08e6d7e81f339e06df1416d74", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21927, - { - "block": 4885364, - "op": { - "type": "vote_operation", - "value": { - "author": "kus-knee", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t190836197z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T19:42:42", - "trx_id": "641461be71d572c08e6d7e81f339e06df1416d74", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21928, - { - "block": 4886346, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5770", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "9721776176", - "total_vote_weight": "11528460586161603480", - "voter": "andread", - "weight": "6311878916714867" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T20:32:09", - "trx_id": "590fa0c69e9f4198e023a82577043dec8ad4f4c6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21929, - { - "block": 4886346, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "andread", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T20:32:09", - "trx_id": "590fa0c69e9f4198e023a82577043dec8ad4f4c6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21930, - { - "block": 4886355, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "41749", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "9531153114", - "total_vote_weight": "15467127957908421649", - "voter": "andread", - "weight": "1147238057254495" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T20:32:36", - "trx_id": "dce6d93b86f52c8816f3fa50c09b6d2ae2c31525", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21931, - { - "block": 4886355, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "andread", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T20:32:36", - "trx_id": "dce6d93b86f52c8816f3fa50c09b6d2ae2c31525", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21932, - { - "block": 4887018, - "op": { - "type": "comment_operation", - "value": { - "author": "shadowspub", - "body": "Hi @camilla, just stopping back to let you know that I included you as one of my favourite reads on my ramble today. [You can see what I had to say here.](https://steemit.com/life/@shadowspub/sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th)", - "json_metadata": "{\"tags\":[\"history\"],\"users\":[\"camilla\"],\"links\":[\"https://steemit.com/life/@shadowspub/sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t210552014z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:05:57", - "trx_id": "97a5f595a25c454e3d2f91453f7cd314d5d73e3d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21933, - { - "block": 4887445, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5703", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 88662747, - "total_vote_weight": "11528518097644600231", - "voter": "mlialen", - "weight": "57511482996751" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:27:21", - "trx_id": "c77e8042a5e39cb8c0a75d894bea08ba65a4016d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21934, - { - "block": 4887445, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "mlialen", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:27:21", - "trx_id": "c77e8042a5e39cb8c0a75d894bea08ba65a4016d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21935, - { - "block": 4887456, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5706", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1515433531, - "total_vote_weight": "11529500942486959039", - "voter": "robotev", - "weight": "982844842358808" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:27:54", - "trx_id": "fc0ff3f55492df2111d5c31149ab31fd32363c08", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21936, - { - "block": 4887456, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "robotev", - "weight": 4500 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:27:54", - "trx_id": "fc0ff3f55492df2111d5c31149ab31fd32363c08", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21937, - { - "block": 4887469, - "op": { - "type": "comment_operation", - "value": { - "author": "mlialen", - "body": "Really nice pictures. I admit I had to look up where it was :)", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t212832160z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:28:33", - "trx_id": "c2c15e64958c70be96bb73be16702d082e2d9ccd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21938, - { - "block": 4887978, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "anwenbaumeister", - "comment_permlink": "a-peek-into-pre-columbian-worldviews-found-in-agricultural-practices-duality-complementarity-cyclicity-and-reciprocity", - "curator": "camilla", - "reward": { - "amount": "70557284", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:54:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 77 - } - ], - [ - 21939, - { - "block": 4889041, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "mlialen", - "pending_payout": { - "amount": "68", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t212832160z", - "rshares": "211244174496", - "total_vote_weight": "925324455796039880", - "voter": "camilla", - "weight": "925324455796039880" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:47:30", - "trx_id": "b1888d99f9d5919cc21f6a8075f14a2f5d2757b9", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21940, - { - "block": 4889041, - "op": { - "type": "vote_operation", - "value": { - "author": "mlialen", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t212832160z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:47:30", - "trx_id": "b1888d99f9d5919cc21f6a8075f14a2f5d2757b9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21941, - { - "block": 4889045, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shadowspub", - "pending_payout": { - "amount": "68", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t210552014z", - "rshares": "211244174496", - "total_vote_weight": "925324455796039880", - "voter": "camilla", - "weight": "925324455796039880" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:47:42", - "trx_id": "ccc6d704e4f1c97b4d9cb5bf5745d7a9b46b1b58", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21942, - { - "block": 4889045, - "op": { - "type": "vote_operation", - "value": { - "author": "shadowspub", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t210552014z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:47:42", - "trx_id": "ccc6d704e4f1c97b4d9cb5bf5745d7a9b46b1b58", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21943, - { - "block": 4889061, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shadowspub", - "pending_payout": { - "amount": "149", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th", - "rshares": "211244174496", - "total_vote_weight": "1816970872818667988", - "voter": "camilla", - "weight": "831309056670076610" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:48:30", - "trx_id": "38d160844669cade401ef475c7434212b041df05", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21944, - { - "block": 4889061, - "op": { - "type": "vote_operation", - "value": { - "author": "shadowspub", - "permlink": "sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:48:30", - "trx_id": "38d160844669cade401ef475c7434212b041df05", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21945, - { - "block": 4889077, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thank you! :)", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "shadowspub", - "parent_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t210552014z", - "permlink": "re-shadowspub-re-camilla-there-is-a-land-far-far-north-20160911t224915410z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:49:18", - "trx_id": "132fbba08c250a60a87a63014cb090ab88513ee6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21946, - { - "block": 4889093, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "so cool that you actually looked it up :D", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "mlialen", - "parent_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t212832160z", - "permlink": "re-mlialen-re-camilla-there-is-a-land-far-far-north-20160911t225003383z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:50:06", - "trx_id": "8862c70edfed8ec80a52a72ab4c1f09e9e0dbfd7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21947, - { - "block": 4889132, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"anca3drandom\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:52:09", - "trx_id": "5404b0f28338eaa69e26248cc8fe86a550bb9ee0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21948, - { - "block": 4889160, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"gretepe\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:53:33", - "trx_id": "641ca9fbef69cc7e52fe2e146885aafe2e3077cd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21949, - { - "block": 4889162, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"stellabelle\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:53:39", - "trx_id": "3c26b8176e0426a7df165793c57f60063471c8c8", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21950, - { - "block": 4889166, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"soulsistashakti\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:53:51", - "trx_id": "a9d775c6b9f2a3742ef68963fb2ba09dc22e2c36", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21951, - { - "block": 4889197, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "good! how about you?", - "json_metadata": "{\"tags\":[\"steemmag\"]}", - "parent_author": "infovore", - "parent_permlink": "re-camilla-re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t184739329z", - "permlink": "re-infovore-re-camilla-re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160911t225522735z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:55:24", - "trx_id": "40293d46e503463069293936ce6a0fb1dd0ec363", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21952, - { - "block": 4889199, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "infovore", - "pending_payout": { - "amount": "66", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t184739329z", - "rshares": "207019291006", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:55:30", - "trx_id": "e1f7e70ca2babb397f7e6f498a23a49c747d5bb6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21953, - { - "block": 4889199, - "op": { - "type": "vote_operation", - "value": { - "author": "infovore", - "permlink": "re-camilla-re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t184739329z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:55:30", - "trx_id": "e1f7e70ca2babb397f7e6f498a23a49c747d5bb6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21954, - { - "block": 4889381, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "kus-knee", - "pending_payout": { - "amount": "36773", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-old-dog-investigates-the-curious-case-of-the-swiss-bomb-shelters", - "rshares": "207019291006", - "total_vote_weight": "15367557175106939833", - "voter": "camilla", - "weight": "26833134392282322" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T23:04:42", - "trx_id": "7033e8aca84300efc9827c50677e045e81df22e1", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21955, - { - "block": 4889381, - "op": { - "type": "vote_operation", - "value": { - "author": "kus-knee", - "permlink": "the-old-dog-investigates-the-curious-case-of-the-swiss-bomb-shelters", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T23:04:42", - "trx_id": "7033e8aca84300efc9827c50677e045e81df22e1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21956, - { - "block": 4889541, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "sirwinchester", - "pending_payout": { - "amount": "325749", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "bitcoin-exchange-berlin-meetup-with-ned-on-skype", - "rshares": "207019291006", - "total_vote_weight": "17352183617643928010", - "voter": "camilla", - "weight": "3236812831396389" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T23:12:42", - "trx_id": "3360bf4fd1c4e0dc8f57c4b7ac617cc1a480d56b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21957, - { - "block": 4889541, - "op": { - "type": "vote_operation", - "value": { - "author": "sirwinchester", - "permlink": "bitcoin-exchange-berlin-meetup-with-ned-on-skype", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T23:12:42", - "trx_id": "3360bf4fd1c4e0dc8f57c4b7ac617cc1a480d56b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21958, - { - "block": 4891107, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-innuendo-should-we-establish-a-long-run-cap-for-steem-supply-20160812t120413402z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T00:31:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 11 - } - ], - [ - 21959, - { - "block": 4891910, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "23456651896", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "17038", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "vesting_payout": { - "amount": "72543343847", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 48 - } - ], - [ - 21960, - { - "block": 4891910, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 47330, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "5509", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "39587", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "total_payout_value": { - "amount": "34076", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 49 - } - ], - [ - 21961, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "runridefly", - "comment_permlink": "re-camilla-colors-of-the-falling-leaves-20160910t233510136z", - "curator": "camilla", - "reward": { - "amount": "12261710", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 50 - } - ], - [ - 21962, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "bullionstackers", - "comment_permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234539398z", - "curator": "camilla", - "reward": { - "amount": "9196282", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 53 - } - ], - [ - 21963, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "sompitonov", - "comment_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t001828852z", - "curator": "camilla", - "reward": { - "amount": "70504834", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 56 - } - ], - [ - 21964, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "tmendieta", - "comment_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t034905376z", - "curator": "camilla", - "reward": { - "amount": "70504834", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 59 - } - ], - [ - 21965, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alexft", - "comment_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t065104109z", - "curator": "camilla", - "reward": { - "amount": "70504834", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 62 - } - ], - [ - 21966, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "sompitonov", - "comment_permlink": "re-camilla-re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t093158180z", - "curator": "camilla", - "reward": { - "amount": "67439406", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 65 - } - ], - [ - 21967, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alexft", - "comment_permlink": "re-camilla-re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t113557524z", - "curator": "camilla", - "reward": { - "amount": "67439406", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 68 - } - ], - [ - 21968, - { - "block": 4898733, - "op": { - "type": "comment_operation", - "value": { - "author": "natord", - "body": "It reminded me of my homeland, behind Arctic-circle. North nature is special. harsh and beautiful.\n\nhttps://steemimg.com/images/2016/09/12/0f97bbb545b2a54.jpg", - "json_metadata": "{\"tags\":[\"history\"],\"image\":[\"https://steemimg.com/images/2016/09/12/0f97bbb545b2a54.jpg\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160912t065441930z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T06:54:39", - "trx_id": "8fb3d72454efa5d6b6170da94c17c6b59770bea5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21969, - { - "block": 4901590, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "royalmacro", - "comment_permlink": "moonlit-night-an-original-abstract-art-and-a-poem", - "curator": "camilla", - "reward": { - "amount": "70375405", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T09:19:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 15 - } - ], - [ - 21970, - { - "block": 4902469, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "peskov", - "comment_permlink": "a-cup-of-tea-featuring-vasily-peskov-as-author", - "curator": "camilla", - "reward": { - "amount": "70363715", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T10:03:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 9 - } - ], - [ - 21971, - { - "block": 4903433, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "smailer", - "comment_permlink": "my-daytime-latte-art-tryings-200-followers-milestone", - "curator": "camilla", - "reward": { - "amount": "76468318", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T10:52:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 21972, - { - "block": 4903881, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "complexring", - "comment_permlink": "bypassing-the-great-firewall-of-china", - "curator": "camilla", - "reward": { - "amount": "91754176", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:14:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 62 - } - ], - [ - 21973, - { - "block": 4903978, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "2284636695", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "2597", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "vesting_payout": { - "amount": "11062290396", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 26 - } - ], - [ - 21974, - { - "block": 4903978, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 7234, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "536", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "5730", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "total_payout_value": { - "amount": "5194", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 27 - } - ], - [ - 21975, - { - "block": 4903978, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "sompitonov", - "comment_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "curator": "camilla", - "reward": { - "amount": "3058415", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 28 - } - ], - [ - 21976, - { - "block": 4903978, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "kus-knee", - "comment_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t190836197z", - "curator": "camilla", - "reward": { - "amount": "73401982", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 31 - } - ], - [ - 21977, - { - "block": 4903978, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "shadowspub", - "comment_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t210552014z", - "curator": "camilla", - "reward": { - "amount": "73401982", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 34 - } - ], - [ - 21978, - { - "block": 4903978, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "mlialen", - "comment_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t212832160z", - "curator": "camilla", - "reward": { - "amount": "73401982", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 37 - } - ], - [ - 21979, - { - "block": 4904290, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "dumar022", - "comment_permlink": "i-never-plan-to-get-a-new-cactus-but-it-happens-all-the-time", - "curator": "camilla", - "reward": { - "amount": "70339420", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:34:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 21980, - { - "block": 4904405, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "xiaofang", - "comment_permlink": "my-photography-flower-collections", - "curator": "camilla", - "reward": { - "amount": "73396053", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:40:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21981, - { - "block": 4904867, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "aaronkoenig", - "comment_permlink": "steemit-meetup-berlin-with-ned-scott-and-steemians-from-hamburg", - "curator": "camilla", - "reward": { - "amount": "1128365664", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T12:04:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 21 - } - ], - [ - 21982, - { - "block": 4905213, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "labradorsem", - "comment_permlink": "diet-apple-pie-with-apples-and-pears", - "curator": "camilla", - "reward": { - "amount": "61154024", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T12:21:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 21983, - { - "block": 4906606, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "terrycraft", - "comment_permlink": "traveling-to-peru-to-the-wild-jungle-through-the-city-of-shamans-iquitos-puteshestvie-v-ikitos-featuring-njall-as-author", - "curator": "camilla", - "reward": { - "amount": "61137899", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T13:31:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 31 - } - ], - [ - 21984, - { - "block": 4906955, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "opheliafu", - "comment_permlink": "the-blue-raven", - "curator": "camilla", - "reward": { - "amount": "314839300", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T13:48:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 21985, - { - "block": 4910096, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "kus-knee", - "comment_permlink": "the-old-dog-investigates-the-curious-case-of-the-swiss-bomb-shelters", - "curator": "camilla", - "reward": { - "amount": "64152384", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T16:26:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 17 - } - ], - [ - 21986, - { - "block": 4911002, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "### I made this drawing with my new Prismacolor pencils. I got them from the talented artist @Pixielols. I met her in real life today at the University and she is the sweetest and loveliest person you can imagine.\n\nhttps://i.imgsafe.org/6dc35d7e3c.jpg\n\n ### I had to test them right away so I just spent an hour on this autumn inspired apple drawing. These color pencils are very soft and it is a joy to work with them.\n\nhttps://i.imgsafe.org/6dd4fe4912.jpg\n\n### The apple have felt the warm sun on its red skin, drawn energy from the soil, nourished birds and children and heard the cold autumn wind in the leaves. It is a sweet gift from nature for you to enjoy.\n\nhttps://i.imgsafe.org/6ddd3dee6b.jpg\n\n### Thank you for visiting my post! I hope you liked it.", - "json_metadata": "{\"tags\":[\"art\",\"apple\",\"autumn\",\"colours\"],\"image\":[\"https://i.imgsafe.org/6dc35d7e3c.jpg\",\"https://i.imgsafe.org/6dd4fe4912.jpg\",\"https://i.imgsafe.org/6ddd3dee6b.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "apple-and-leaves", - "title": "Apple and Leaves" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:11:45", - "trx_id": "e9de33a2769e3c56a2eb04cbca3068802148eb2d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21987, - { - "block": 4911002, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "66", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "215909081377", - "total_vote_weight": "944711921076433591", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:11:45", - "trx_id": "e9de33a2769e3c56a2eb04cbca3068802148eb2d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21988, - { - "block": 4911002, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:11:45", - "trx_id": "e9de33a2769e3c56a2eb04cbca3068802148eb2d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21989, - { - "block": 4911009, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "66", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 51423955, - "total_vote_weight": "944925401186763676", - "voter": "sergey44", - "weight": "2490601287184" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:06", - "trx_id": "6f06ca0ecd432cec40db13747a60b7b707228119", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21990, - { - "block": 4911009, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "sergey44", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:06", - "trx_id": "6f06ca0ecd432cec40db13747a60b7b707228119", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21991, - { - "block": 4911009, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "306", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "651650151993", - "total_vote_weight": "3287976972277509707", - "voter": "clains", - "weight": "27335601662725370" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:06", - "trx_id": "14f3af6075b9fa0dc31befd2d38aacfff43af33c", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21992, - { - "block": 4911009, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "clains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:06", - "trx_id": "14f3af6075b9fa0dc31befd2d38aacfff43af33c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21993, - { - "block": 4911014, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "361", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "130765654564", - "total_vote_weight": "3684554976556033924", - "voter": "psylains", - "weight": "7931560085570484" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:21", - "trx_id": "37856cc1fe9064955103b31796156066b00633b6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21994, - { - "block": 4911014, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "psylains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:21", - "trx_id": "37856cc1fe9064955103b31796156066b00633b6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21995, - { - "block": 4911015, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "362", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 551289713, - "total_vote_weight": "3686182974326598443", - "voter": "jessicanicklos", - "weight": "35273285028897" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:24", - "trx_id": "a17a63e263c32e389106d40292d212fec02efab6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21996, - { - "block": 4911015, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "jessicanicklos", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:24", - "trx_id": "a17a63e263c32e389106d40292d212fec02efab6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21997, - { - "block": 4911017, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "364", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "5888378458", - "total_vote_weight": "3703549401054751072", - "voter": "bitspace", - "weight": "434160668203815" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:30", - "trx_id": "cafd3e437c1355d5543fe3ae64aea271461caea8", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21998, - { - "block": 4911017, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "bitspace", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:30", - "trx_id": "cafd3e437c1355d5543fe3ae64aea271461caea8", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21999, - { - "block": 4911022, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "13670562023", - "total_vote_weight": 0, - "voter": "goldmatters", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:45", - "trx_id": "4a256e361159a952b0598bb19ddc2b2410f71f83", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22000, - { - "block": 4911022, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "goldmatters", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:45", - "trx_id": "4a256e361159a952b0598bb19ddc2b2410f71f83", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22001, - { - "block": 4911023, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "370", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "13670562023", - "total_vote_weight": "3743710464654223262", - "voter": "goldmatters", - "weight": "1405637225981526" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:48", - "trx_id": "c89823b7ea1b94808b19778975da55d5336f6777", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22002, - { - "block": 4911023, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "goldmatters", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:48", - "trx_id": "c89823b7ea1b94808b19778975da55d5336f6777", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22003, - { - "block": 4911030, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "289482062341", - "total_vote_weight": "4545573627196467795", - "voter": "anonymous", - "weight": "37420280918638078" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:13:09", - "trx_id": "47da48cdcd8d98927598df0d7fdb0b3fbe470c79", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22004, - { - "block": 4911030, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "anonymous", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:13:09", - "trx_id": "47da48cdcd8d98927598df0d7fdb0b3fbe470c79", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22005, - { - "block": 4911031, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 706760886, - "total_vote_weight": "4547424334362593573", - "voter": "murh", - "weight": "89450846362745" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:13:12", - "trx_id": "1b0c8d4f24f9d1e3e88a213ed1c181318ad5388d", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22006, - { - "block": 4911031, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "murh", - "weight": 1509 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:13:12", - "trx_id": "1b0c8d4f24f9d1e3e88a213ed1c181318ad5388d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22007, - { - "block": 4911034, - "op": { - "type": "comment_operation", - "value": { - "author": "goldmatters", - "body": "Lovely@", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t171320575z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:13:21", - "trx_id": "e7b4df539b542e4bac5d18940131cd123e799e0e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22008, - { - "block": 4911070, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "504", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 321100442, - "total_vote_weight": "4548264997516278957", - "voter": "luisucv34", - "weight": "95275157417676" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:09", - "trx_id": "2ea9c341cb484092e5bc37faf3760ee0cf25b2de", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22009, - { - "block": 4911070, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "luisucv34", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:09", - "trx_id": "2ea9c341cb484092e5bc37faf3760ee0cf25b2de", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22010, - { - "block": 4911072, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "504", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 229890255, - "total_vote_weight": "4548866803633534287", - "voter": "sjamayee", - "weight": "70210713679788" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:15", - "trx_id": "4cff3a69c210b194b88a1c0067c75cd34ce4bd1b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22011, - { - "block": 4911072, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "sjamayee", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:15", - "trx_id": "4cff3a69c210b194b88a1c0067c75cd34ce4bd1b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22012, - { - "block": 4911077, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "504", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 1027866325, - "total_vote_weight": "4551556912136215489", - "voter": "ola1", - "weight": "336263562835150" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:30", - "trx_id": "c1792db11b1ea2eaaaff0c393b4bbba923dcfd94", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22013, - { - "block": 4911077, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ola1", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:30", - "trx_id": "c1792db11b1ea2eaaaff0c393b4bbba923dcfd94", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22014, - { - "block": 4911102, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 155364835, - "total_vote_weight": "4551963438869269926", - "voter": "insight2incite", - "weight": "67754455509072" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:16:45", - "trx_id": "9654c16e81d58b07cd1865e5789d6ff60a021bc0", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22015, - { - "block": 4911102, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "insight2incite", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:16:45", - "trx_id": "9654c16e81d58b07cd1865e5789d6ff60a021bc0", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22016, - { - "block": 4911115, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "502", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 143358574, - "total_vote_weight": "4552338528988548564", - "voter": "anomaly", - "weight": "70641972464143" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:17:24", - "trx_id": "bc36e5c2780f5c5a333444e9dbf74e58a9ad9cee", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22017, - { - "block": 4911115, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "anomaly", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:17:24", - "trx_id": "bc36e5c2780f5c5a333444e9dbf74e58a9ad9cee", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22018, - { - "block": 4911130, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 597059749, - "total_vote_weight": "4553900486432503298", - "voter": "ashwim", - "weight": "333217588043676" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:18:09", - "trx_id": "ebba9f5ce4ccb5c3df2ef7de56c7ebe154eb65b3", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22019, - { - "block": 4911130, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ashwim", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:18:09", - "trx_id": "ebba9f5ce4ccb5c3df2ef7de56c7ebe154eb65b3", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22020, - { - "block": 4911136, - "op": { - "type": "comment_operation", - "value": { - "author": "ashwim", - "body": "very nice artwork ... thanks for sharing... keep it up...", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t171828553z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:18:27", - "trx_id": "b87dc9ccfe43ab91670eed2fc43edd735af9c7e9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22021, - { - "block": 4911165, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 59551730, - "total_vote_weight": "4554056259399344170", - "voter": "techguru", - "weight": "42318322658436" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:19:54", - "trx_id": "8b0e8accd4f91ab07c05bbe43a8f55302857e67f", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22022, - { - "block": 4911165, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "techguru", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:19:54", - "trx_id": "8b0e8accd4f91ab07c05bbe43a8f55302857e67f", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22023, - { - "block": 4911201, - "op": { - "type": "comment_operation", - "value": { - "author": "acidyo", - "body": ">I got them from the talented artist @Pixielols. I met her in real life today at the University\n\nOh wow, the Steemit world really is still small! Hehe\n\nGreat drawing and really nice colors!\n\nHer post about the Copic markers made me want to order them, hehe! Currently sketching to fund them. :D", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t172139964z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:21:42", - "trx_id": "f2c7d9f355c307a93f0bab76ac957b1e93bf03cb", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 22024, - { - "block": 4911203, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 63850166, - "total_vote_weight": "4554223272157884585", - "voter": "aavkc", - "weight": "55949274111039" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:21:48", - "trx_id": "8374918c977f445d1f8fd7535f0022aad9c25649", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22025, - { - "block": 4911203, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "aavkc", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:21:48", - "trx_id": "8374918c977f445d1f8fd7535f0022aad9c25649", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22026, - { - "block": 4911205, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "29251115097", - "total_vote_weight": "4630315371958732953", - "voter": "acidyo", - "weight": "25744493765953697" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:21:54", - "trx_id": "fa56c82f2877c591dde364cbf83c16bab3d16b09", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22027, - { - "block": 4911205, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "acidyo", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:21:54", - "trx_id": "fa56c82f2877c591dde364cbf83c16bab3d16b09", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22028, - { - "block": 4911208, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 849659123, - "total_vote_weight": "4632513168685677847", - "voter": "coar", - "weight": "754576876251080" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:03", - "trx_id": "551be3eaff76b21d0c34165e38f802a67d2b8e79", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22029, - { - "block": 4911208, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "coar", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:03", - "trx_id": "551be3eaff76b21d0c34165e38f802a67d2b8e79", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22030, - { - "block": 4911209, - "op": { - "type": "comment_operation", - "value": { - "author": "aavkc", - "body": "good job", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t172202293z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:06", - "trx_id": "b64cb1a44ca79d22fef07442cca8cc3f35bf5748", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22031, - { - "block": 4911212, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 58273844, - "total_vote_weight": "4632663878882512400", - "voter": "hotgirlktm", - "weight": "52748568892093" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:15", - "trx_id": "9600fef571caa241ed298e925b0da75201ef3843", - "trx_in_block": 9, - "virtual_op": 1 - } - ], - [ - 22032, - { - "block": 4911212, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "hotgirlktm", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:15", - "trx_id": "9600fef571caa241ed298e925b0da75201ef3843", - "trx_in_block": 9, - "virtual_op": 0 - } - ], - [ - 22033, - { - "block": 4911213, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 117630527, - "total_vote_weight": "4632968089740256431", - "voter": "pokemon", - "weight": "106980818306650" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:18", - "trx_id": "6241f92f3bcd7b554bdaa4b7c768d43bf7e227af", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 22034, - { - "block": 4911213, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "pokemon", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:18", - "trx_id": "6241f92f3bcd7b554bdaa4b7c768d43bf7e227af", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 22035, - { - "block": 4911213, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 244245816, - "total_vote_weight": "4633599704657956435", - "voter": "cryptochannel", - "weight": "222117912724501" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:18", - "trx_id": "53f88c0a2f43c9f53d14197a91ce6d2b42fba05a", - "trx_in_block": 9, - "virtual_op": 1 - } - ], - [ - 22036, - { - "block": 4911213, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "cryptochannel", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:18", - "trx_id": "53f88c0a2f43c9f53d14197a91ce6d2b42fba05a", - "trx_in_block": 9, - "virtual_op": 0 - } - ], - [ - 22037, - { - "block": 4911214, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 246459444, - "total_vote_weight": "4634236985430254670", - "voter": "strawhat", - "weight": "225172539545376" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:21", - "trx_id": "34202ef335284894eee6a433a48d2dfd4cd66299", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22038, - { - "block": 4911214, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "strawhat", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:21", - "trx_id": "34202ef335284894eee6a433a48d2dfd4cd66299", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22039, - { - "block": 4911275, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 1541722449, - "total_vote_weight": "4638222149353602136", - "voter": "gidlark", - "weight": "1813249585123097" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:24", - "trx_id": "e4db024ecb0b303a45a7a47214f675039c2fd515", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22040, - { - "block": 4911275, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "gidlark", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:24", - "trx_id": "e4db024ecb0b303a45a7a47214f675039c2fd515", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22041, - { - "block": 4911281, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 56509947, - "total_vote_weight": "4638368176964869032", - "voter": "alex.gaud", - "weight": "67902839239106" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:42", - "trx_id": "adb97a82965257019bf7841e6269331437ba9167", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22042, - { - "block": 4911281, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "alex.gaud", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:42", - "trx_id": "adb97a82965257019bf7841e6269331437ba9167", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22043, - { - "block": 4911283, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 1322723922, - "total_vote_weight": "4641785351864380218", - "voter": "elishagh1", - "weight": "1600376911271072" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:48", - "trx_id": "2fb8c4197a9606ec94ba93d7433d19c655d57142", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22044, - { - "block": 4911283, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "elishagh1", - "weight": 400 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:48", - "trx_id": "2fb8c4197a9606ec94ba93d7433d19c655d57142", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22045, - { - "block": 4911304, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "Yes there are not so many Steemers here in Norway yet, I think I know them all :D\n\nThank you for the compliment on my art.\n\nI agree I also want to buy the Copic markers, they look so awsome! Haha yes they are pretty expensive but I think it might be worth it.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "acidyo", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t172139964z", - "permlink": "re-acidyo-re-camilla-apple-and-leaves-20160912t172655245z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:26:54", - "trx_id": "6a1e5517b38b9e6bb16719bc644b57d4000d15aa", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 22046, - { - "block": 4911312, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thank you :D I will", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "ashwim", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t171828553z", - "permlink": "re-ashwim-re-camilla-apple-and-leaves-20160912t172717002z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:18", - "trx_id": "917f500de52ba30caef4e80c4ab444d58edff69c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22047, - { - "block": 4911316, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "goldmatters", - "pending_payout": { - "amount": "63", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t171320575z", - "rshares": "211675569978", - "total_vote_weight": "927617181348547604", - "voter": "camilla", - "weight": "437265678791358974" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:30", - "trx_id": "bceb1643a37cd96bff304630e31f5e3c2912f60b", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22048, - { - "block": 4911316, - "op": { - "type": "vote_operation", - "value": { - "author": "goldmatters", - "permlink": "re-camilla-apple-and-leaves-20160912t171320575z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:30", - "trx_id": "bceb1643a37cd96bff304630e31f5e3c2912f60b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22049, - { - "block": 4911319, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thanks!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "goldmatters", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t171320575z", - "permlink": "re-goldmatters-re-camilla-apple-and-leaves-20160912t172738146z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:39", - "trx_id": "45529c0456c9bb93d6c7e0ee8f022c7fe5581b86", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22050, - { - "block": 4911322, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "aavkc", - "pending_payout": { - "amount": "63", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t172202293z", - "rshares": "211675569978", - "total_vote_weight": "927119147988210213", - "voter": "camilla", - "weight": "176152638117759940" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:48", - "trx_id": "d9e47525fa85ae9b91170cc4cb3accbec9063d1a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22051, - { - "block": 4911322, - "op": { - "type": "vote_operation", - "value": { - "author": "aavkc", - "permlink": "re-camilla-apple-and-leaves-20160912t172202293z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:48", - "trx_id": "d9e47525fa85ae9b91170cc4cb3accbec9063d1a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22052, - { - "block": 4911324, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "ashwim", - "pending_payout": { - "amount": "64", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t171828553z", - "rshares": "211675569978", - "total_vote_weight": "930394407468503154", - "voter": "camilla", - "weight": "291930460175032994" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:54", - "trx_id": "a4c1fb0430ec2b321c84cae41a1d6fb2f76fdfec", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22053, - { - "block": 4911324, - "op": { - "type": "vote_operation", - "value": { - "author": "ashwim", - "permlink": "re-camilla-apple-and-leaves-20160912t171828553z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:54", - "trx_id": "a4c1fb0430ec2b321c84cae41a1d6fb2f76fdfec", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22054, - { - "block": 4911343, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "kalipo", - "pending_payout": { - "amount": "121552", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "raptor-art-drawing-all-day", - "rshares": "211675569978", - "total_vote_weight": "16739375280083409273", - "voter": "camilla", - "weight": "8403846313740628" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:28:51", - "trx_id": "447d5d37f569ab88e343458f61fe580fd418a16d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22055, - { - "block": 4911343, - "op": { - "type": "vote_operation", - "value": { - "author": "kalipo", - "permlink": "raptor-art-drawing-all-day", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:28:51", - "trx_id": "447d5d37f569ab88e343458f61fe580fd418a16d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22056, - { - "block": 4911347, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "alex2016", - "pending_payout": { - "amount": "20722", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-new-works-from-genuine-leather", - "rshares": "207442058578", - "total_vote_weight": "14586536023317668623", - "voter": "camilla", - "weight": "42352343323010257" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:29:03", - "trx_id": "60d684ff1b8695f0a27d5131e9c964ea6f5c0251", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 22057, - { - "block": 4911347, - "op": { - "type": "vote_operation", - "value": { - "author": "alex2016", - "permlink": "my-new-works-from-genuine-leather", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:29:03", - "trx_id": "60d684ff1b8695f0a27d5131e9c964ea6f5c0251", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 22058, - { - "block": 4911373, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "### I made this drawing with my new Prismacolor pencils. I got them from the talented artist @Pixielols. I met her in real life today at the University and she is the sweetest and loveliest person you can imagine.\n\nhttps://i.imgsafe.org/6dc35d7e3c.jpg\n\n ### I had to test them right away so I just spent an hour on this autumn inspired apple drawing. These color pencils are very soft and it is a joy to work with them.\n\nhttps://i.imgsafe.org/6dd4fe4912.jpg\n\n### The apple have felt the warm sun on its red skin, drawn energy from the soil, nourished birds and children and heard the cold autumn wind in the leaves. It is a sweet gift from nature for you to enjoy.\n\nhttps://i.imgsafe.org/6ddd3dee6b.jpg\n\n### Thank you for visiting my post! I hope you liked it.", - "json_metadata": "{\"tags\":[\"art\",\"apple\",\"autumn\",\"colours\"],\"image\":[\"https://i.imgsafe.org/6dc35d7e3c.jpg\",\"https://i.imgsafe.org/6dd4fe4912.jpg\",\"https://i.imgsafe.org/6ddd3dee6b.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "apple-and-leaves", - "title": "Apple Art" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:21", - "trx_id": "178037dd18b61c4c3dc005c1aa1466e964f0374c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22059, - { - "block": 4911383, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "739", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "436172432967", - "total_vote_weight": "5683335719784144663", - "voter": "boatymcboatface", - "weight": "663120400908916696" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:51", - "trx_id": "69c2b13c13f94e2002b6c06202b8a130daf7377c", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 22060, - { - "block": 4911383, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "boatymcboatface", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:51", - "trx_id": "69c2b13c13f94e2002b6c06202b8a130daf7377c", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 22061, - { - "block": 4911383, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "773", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "63365513478", - "total_vote_weight": "5821715387235604396", - "voter": "theshell", - "weight": "88101721610762696" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:51", - "trx_id": "6bd6c348115631a86f4acc661c256741b165f470", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 22062, - { - "block": 4911383, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "theshell", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:51", - "trx_id": "6bd6c348115631a86f4acc661c256741b165f470", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 22063, - { - "block": 4911384, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1505", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "1152457041840", - "total_vote_weight": "7901162747347395947", - "voter": "rossco99", - "weight": "1327380564871360273" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:54", - "trx_id": "bc737880167153d8be286de2eafaeccc37377d01", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22064, - { - "block": 4911384, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "rossco99", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:54", - "trx_id": "bc737880167153d8be286de2eafaeccc37377d01", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22065, - { - "block": 4911384, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1507", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 3505969711, - "total_vote_weight": "7906444182342513581", - "voter": "glitterpig", - "weight": "3371316005216756" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:54", - "trx_id": "049b66a72a1520486541f37735fe7b27dbf76753", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22066, - { - "block": 4911384, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "glitterpig", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:54", - "trx_id": "049b66a72a1520486541f37735fe7b27dbf76753", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22067, - { - "block": 4911402, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "acidyo", - "pending_payout": { - "amount": "5710", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "sketch-kitty-cat-oc", - "rshares": "207442058578", - "total_vote_weight": "11822623121092878973", - "voter": "camilla", - "weight": "125700686784290108" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:31:51", - "trx_id": "82fd894d61ced60cffa90d17f1dff0bd223d9383", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22068, - { - "block": 4911402, - "op": { - "type": "vote_operation", - "value": { - "author": "acidyo", - "permlink": "sketch-kitty-cat-oc", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:31:51", - "trx_id": "82fd894d61ced60cffa90d17f1dff0bd223d9383", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22069, - { - "block": 4911408, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "awww it is sooo cute! I love it <3", - "json_metadata": "{\"tags\":[\"drawing\"]}", - "parent_author": "acidyo", - "parent_permlink": "sketch-kitty-cat-oc", - "permlink": "re-acidyo-sketch-kitty-cat-oc-20160912t173207452z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:32:09", - "trx_id": "22ece8e946f60946e713ce988b0781477ce0b849", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22070, - { - "block": 4911427, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-acidyo-re-camilla-apple-and-leaves-20160912t172655245z", - "rshares": 609398231, - "total_vote_weight": "2809925210693923", - "voter": "acidyo", - "weight": "580717876876744" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:06", - "trx_id": "d1a36bafc96db1a8af411c942ff71cdeeffbce74", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22071, - { - "block": 4911427, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-acidyo-re-camilla-apple-and-leaves-20160912t172655245z", - "voter": "acidyo", - "weight": 100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:06", - "trx_id": "d1a36bafc96db1a8af411c942ff71cdeeffbce74", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22072, - { - "block": 4911434, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1511", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "6349496599", - "total_vote_weight": "7915995686847249607", - "voter": "orcish", - "weight": "6908921591759058" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:27", - "trx_id": "2a6317cee4fb6fa90f4040c2ed0d2670169f8647", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22073, - { - "block": 4911434, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "orcish", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:27", - "trx_id": "2a6317cee4fb6fa90f4040c2ed0d2670169f8647", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22074, - { - "block": 4911444, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-acidyo-sketch-kitty-cat-oc-20160912t173207452z", - "rshares": 609398231, - "total_vote_weight": "2809925210693923", - "voter": "acidyo", - "weight": "168595512641635" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:57", - "trx_id": "7c5f243ade91f1d0b448d0e7072764dcecab07b5", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22075, - { - "block": 4911444, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-acidyo-sketch-kitty-cat-oc-20160912t173207452z", - "voter": "acidyo", - "weight": 100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:57", - "trx_id": "7c5f243ade91f1d0b448d0e7072764dcecab07b5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22076, - { - "block": 4911449, - "op": { - "type": "comment_operation", - "value": { - "author": "acidyo", - "body": "Thanks :3", - "json_metadata": "{\"tags\":[\"drawing\"]}", - "parent_author": "camilla", - "parent_permlink": "re-acidyo-sketch-kitty-cat-oc-20160912t173207452z", - "permlink": "re-camilla-re-acidyo-sketch-kitty-cat-oc-20160912t173408484z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:34:12", - "trx_id": "5c0383ed515eb551803d219e47635b19a46fa6ef", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22077, - { - "block": 4911465, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "33217", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "what-is-culture-a-look-into-various-explanations-of-culture-and-forming-my-own-definition", - "rshares": "207442058578", - "total_vote_weight": "15320294595211071057", - "voter": "camilla", - "weight": "27723884101111043" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:35:00", - "trx_id": "202fecdca5542351d9f72ce4917a3e7351f79a39", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22078, - { - "block": 4911465, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "what-is-culture-a-look-into-various-explanations-of-culture-and-forming-my-own-definition", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:35:00", - "trx_id": "202fecdca5542351d9f72ce4917a3e7351f79a39", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22079, - { - "block": 4911512, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -236,16 +236,16 @@\n g/6d\n-c35d7e3c\n+d4fe4912\n .jpg\n@@ -435,32 +435,32 @@\n gsafe.org/6d\n-d4fe4912\n+c35d7e3c\n .jpg%0A%0A### Th\n", - "json_metadata": "{\"tags\":[\"art\",\"apple\",\"autumn\",\"colours\"],\"image\":[\"https://i.imgsafe.org/6dd4fe4912.jpg\",\"https://i.imgsafe.org/6dc35d7e3c.jpg\",\"https://i.imgsafe.org/6ddd3dee6b.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "apple-and-leaves", - "title": "Apple Art" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:37:21", - "trx_id": "67762af9744c9763dd9858225ae9ae31ff935075", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22080, - { - "block": 4911521, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1529", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "16221469512", - "total_vote_weight": "7940319109157654746", - "voter": "jademont", - "weight": "21120838372868462" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:37:48", - "trx_id": "163f7f6ce3c69130b85a114b1db6d3d10edd45ca", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22081, - { - "block": 4911521, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "jademont", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:37:48", - "trx_id": "163f7f6ce3c69130b85a114b1db6d3d10edd45ca", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22082, - { - "block": 4911525, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1536", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "9220777116", - "total_vote_weight": "7954095260687687295", - "voter": "r4fken", - "weight": "12054132588778480" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:38:00", - "trx_id": "f76dc0148ab3b1a35fded252b6f9991c827d1939", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22083, - { - "block": 4911525, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "r4fken", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:38:00", - "trx_id": "f76dc0148ab3b1a35fded252b6f9991c827d1939", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22084, - { - "block": 4911534, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -387,11 +387,8 @@\n and \n-it \n is a\n@@ -404,21 +404,16 @@\n ork with\n- them\n .%0A%0Ahttps\n", - "json_metadata": "{\"tags\":[\"art\",\"apple\",\"autumn\",\"colours\"],\"image\":[\"https://i.imgsafe.org/6dd4fe4912.jpg\",\"https://i.imgsafe.org/6dc35d7e3c.jpg\",\"https://i.imgsafe.org/6ddd3dee6b.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "apple-and-leaves", - "title": "Apple Art" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:38:27", - "trx_id": "64b921869a1707f6137d54ddc8908282833a123d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22085, - { - "block": 4911579, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "fajrilgooner", - "pending_payout": { - "amount": "124071", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "efficacy-of-turmeric-and-honey", - "rshares": "207442058578", - "total_vote_weight": "16751744895383774296", - "voter": "camilla", - "weight": "8115785431724820" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:42", - "trx_id": "9f57266b2cf5ddd5350dc050e1ddb20065ab766c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22086, - { - "block": 4911579, - "op": { - "type": "vote_operation", - "value": { - "author": "fajrilgooner", - "permlink": "efficacy-of-turmeric-and-honey", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:42", - "trx_id": "9f57266b2cf5ddd5350dc050e1ddb20065ab766c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22087, - { - "block": 4911582, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 56157882, - "total_vote_weight": "7954179051772419968", - "voter": "jarvis", - "weight": "81277352190692" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:51", - "trx_id": "674d2d9e9f5321980fe04ae0686205e1446ffa08", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22088, - { - "block": 4911582, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "jarvis", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:51", - "trx_id": "674d2d9e9f5321980fe04ae0686205e1446ffa08", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22089, - { - "block": 4911583, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 53039566, - "total_vote_weight": "7954258188905109201", - "voter": "thomasaustin", - "weight": "76894913929704" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:54", - "trx_id": "7a6e7d6b947b38163120e5df9ab8bdc297586ce3", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22090, - { - "block": 4911583, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "thomasaustin", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:54", - "trx_id": "7a6e7d6b947b38163120e5df9ab8bdc297586ce3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22091, - { - "block": 4911584, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 57196572, - "total_vote_weight": "7954343527117623005", - "voter": "ciao", - "weight": "83062526846769" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:57", - "trx_id": "d227a86a4fc0f3839440f1df179e97f35be9edb9", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22092, - { - "block": 4911584, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ciao", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:57", - "trx_id": "d227a86a4fc0f3839440f1df179e97f35be9edb9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22093, - { - "block": 4911587, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50441122, - "total_vote_weight": "7954418784937339049", - "voter": "eavy", - "weight": "73627233622196" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:06", - "trx_id": "157e45a06fbcd158a39c2282ac969fc4e01c4f65", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22094, - { - "block": 4911587, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "eavy", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:06", - "trx_id": "157e45a06fbcd158a39c2282ac969fc4e01c4f65", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22095, - { - "block": 4911589, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 53056158, - "total_vote_weight": "7954497943208450318", - "voter": "johnbyrd", - "weight": "77707036140895" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:12", - "trx_id": "f364eccb18ae414bb353e5c3d98e14155024e78c", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22096, - { - "block": 4911589, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "johnbyrd", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:12", - "trx_id": "f364eccb18ae414bb353e5c3d98e14155024e78c", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22097, - { - "block": 4911600, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1538", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 54313084, - "total_vote_weight": "7954578975540051524", - "voter": "fortuner", - "weight": "81032331601206" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:45", - "trx_id": "a746a03aa3ba97b18b537e3f082b54efa209280a", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22098, - { - "block": 4911600, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "fortuner", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:45", - "trx_id": "a746a03aa3ba97b18b537e3f082b54efa209280a", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22099, - { - "block": 4911614, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1536", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 148091845, - "total_vote_weight": "7954799914607325206", - "voter": "buffett", - "weight": "220939067273682" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:27", - "trx_id": "f53f8fd5f8e4f8abfbaf5e8e3959bc4f9d678a2f", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22100, - { - "block": 4911614, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "buffett", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:27", - "trx_id": "f53f8fd5f8e4f8abfbaf5e8e3959bc4f9d678a2f", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22101, - { - "block": 4911616, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1539", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 4263594327, - "total_vote_weight": "7961156807460496684", - "voter": "fubar-bdhr", - "weight": "6356892853171478" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:33", - "trx_id": "85f0d8a90f3b37cc618d6e8d5768646a0d51a214", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22102, - { - "block": 4911616, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "fubar-bdhr", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:33", - "trx_id": "85f0d8a90f3b37cc618d6e8d5768646a0d51a214", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22103, - { - "block": 4911617, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1539", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50271139, - "total_vote_weight": "7961231714291952756", - "voter": "hitherise", - "weight": "74906831456072" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:36", - "trx_id": "dbf7a9e9f785a327a2d7c9b5f14565885123c355", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22104, - { - "block": 4911617, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "hitherise", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:36", - "trx_id": "dbf7a9e9f785a327a2d7c9b5f14565885123c355", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22105, - { - "block": 4911619, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 53030738, - "total_vote_weight": "7961310731921542285", - "voter": "widell", - "weight": "79017629589529" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:42", - "trx_id": "42870856dd77f9b73ebd60e968682db311bc7b30", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22106, - { - "block": 4911619, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "widell", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:42", - "trx_id": "42870856dd77f9b73ebd60e968682db311bc7b30", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22107, - { - "block": 4911622, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 60342922, - "total_vote_weight": "7961400643509054273", - "voter": "lillianjones", - "weight": "89911587511988" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:51", - "trx_id": "78fdd04f6b0cee4ccb3254b2c9741f6c93292eb1", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22108, - { - "block": 4911622, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "lillianjones", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:51", - "trx_id": "78fdd04f6b0cee4ccb3254b2c9741f6c93292eb1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22109, - { - "block": 4911623, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 55306941, - "total_vote_weight": "7961483050077691417", - "voter": "steema", - "weight": "82406568637144" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:54", - "trx_id": "d9f704e5dea33a4e232d69c6115fc015de978fc4", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22110, - { - "block": 4911623, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "steema", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:54", - "trx_id": "d9f704e5dea33a4e232d69c6115fc015de978fc4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22111, - { - "block": 4911623, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 72812589, - "total_vote_weight": "7961591537841891468", - "voter": "igtes", - "weight": "108487764200051" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:54", - "trx_id": "30c9df603c5d62c5153e8a1461517a95cf239713", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22112, - { - "block": 4911623, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "igtes", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:54", - "trx_id": "30c9df603c5d62c5153e8a1461517a95cf239713", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22113, - { - "block": 4911631, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50370757, - "total_vote_weight": "7961666586885319766", - "voter": "yorsens", - "weight": "75049043428298" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:18", - "trx_id": "1414e564a4a0cd382666fd2fe5ba971e21b5a714", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22114, - { - "block": 4911631, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "yorsens", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:18", - "trx_id": "1414e564a4a0cd382666fd2fe5ba971e21b5a714", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22115, - { - "block": 4911633, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50588612, - "total_vote_weight": "7961741959436738384", - "voter": "troich", - "weight": "75372551418618" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:24", - "trx_id": "2f90f3f307b8518dfc538c01d2790c8261d76b35", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 22116, - { - "block": 4911633, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "troich", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:24", - "trx_id": "2f90f3f307b8518dfc538c01d2790c8261d76b35", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 22117, - { - "block": 4911634, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 51562255, - "total_vote_weight": "7961818781514878772", - "voter": "curpose", - "weight": "76822078140388" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:27", - "trx_id": "a0d14f813e5f4de4a52d7bb855c6d4eab296bada", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 22118, - { - "block": 4911634, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "curpose", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:27", - "trx_id": "a0d14f813e5f4de4a52d7bb855c6d4eab296bada", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 22119, - { - "block": 4911635, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 53048942, - "total_vote_weight": "7961897817417952081", - "voter": "ficholl", - "weight": "79035903073309" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:30", - "trx_id": "09b44c1a2e8f3b5837565fffd8a602d4e5a78754", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22120, - { - "block": 4911635, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ficholl", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:30", - "trx_id": "09b44c1a2e8f3b5837565fffd8a602d4e5a78754", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22121, - { - "block": 4911641, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50059754, - "total_vote_weight": "7961972398733769868", - "voter": "vive", - "weight": "74581315817787" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:48", - "trx_id": "051120398c87df25af71eff1e3057ca7619bde83", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22122, - { - "block": 4911641, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "vive", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:48", - "trx_id": "051120398c87df25af71eff1e3057ca7619bde83", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22123, - { - "block": 4911642, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50710305, - "total_vote_weight": "7962047948188487545", - "voter": "prof", - "weight": "75549454717677" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:51", - "trx_id": "15162fc3381cca34e7493cb22954ba208b727f6b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22124, - { - "block": 4911642, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "prof", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:51", - "trx_id": "15162fc3381cca34e7493cb22954ba208b727f6b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22125, - { - "block": 4911643, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 71268878, - "total_vote_weight": "7962154124468599372", - "voter": "confucius", - "weight": "106176280111827" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:54", - "trx_id": "fc806b1287f4c0723a2c598f1188f581c14a0585", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22126, - { - "block": 4911643, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "confucius", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:54", - "trx_id": "fc806b1287f4c0723a2c598f1188f581c14a0585", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22127, - { - "block": 4911646, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 149597919, - "total_vote_weight": "7962376988255986211", - "voter": "steemster1", - "weight": "222863787386839" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:03", - "trx_id": "4db90a5179b242f330942a9b3b7bad06daef72ea", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22128, - { - "block": 4911646, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "steemster1", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:03", - "trx_id": "4db90a5179b242f330942a9b3b7bad06daef72ea", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22129, - { - "block": 4911654, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50054445, - "total_vote_weight": "7962451554846249289", - "voter": "coad", - "weight": "74566590263078" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:27", - "trx_id": "ad175c2578195003cd0a27d52a521f5e685a50ff", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22130, - { - "block": 4911654, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "coad", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:27", - "trx_id": "ad175c2578195003cd0a27d52a521f5e685a50ff", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22131, - { - "block": 4911656, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 59219358, - "total_vote_weight": "7962539773126208430", - "voter": "sharon", - "weight": "88218279959141" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:33", - "trx_id": "dd16a3de7b55bc1b29fd83417e36271e4b9f4e90", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22132, - { - "block": 4911656, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "sharon", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:33", - "trx_id": "dd16a3de7b55bc1b29fd83417e36271e4b9f4e90", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22133, - { - "block": 4911666, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1542", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50454901, - "total_vote_weight": "7962614933941361087", - "voter": "roto", - "weight": "75160815152657" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:03", - "trx_id": "f6e3d95673f04c95d72af93ee08340592e120dde", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22134, - { - "block": 4911666, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "roto", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:03", - "trx_id": "f6e3d95673f04c95d72af93ee08340592e120dde", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22135, - { - "block": 4911670, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thank you :) it was very fun to draw", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "aavkc", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t172202293z", - "permlink": "re-aavkc-re-camilla-apple-and-leaves-20160912t174516163z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:15", - "trx_id": "5d285c8a57d57fc920b7683b2e901f9ef1c77a6d", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22136, - { - "block": 4911671, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1542", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 60806273, - "total_vote_weight": "7962705513383702711", - "voter": "msjennifer", - "weight": "90579442341624" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:18", - "trx_id": "c4d32c0eb6cbc91a6a1475d355ee9391d54b63a2", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 22137, - { - "block": 4911671, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "msjennifer", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:18", - "trx_id": "c4d32c0eb6cbc91a6a1475d355ee9391d54b63a2", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 22138, - { - "block": 4911675, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1542", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50065875, - "total_vote_weight": "7962780092327196405", - "voter": "bane", - "weight": "74578943493694" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:30", - "trx_id": "1593a38695fb9e10dbc931743a20e0e227fd67be", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22139, - { - "block": 4911675, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "bane", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:30", - "trx_id": "1593a38695fb9e10dbc931743a20e0e227fd67be", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22140, - { - "block": 4911677, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1538", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50262756, - "total_vote_weight": "7962854963480532525", - "voter": "wiss", - "weight": "74871153336120" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:36", - "trx_id": "c577ccf193e1c5dc8277d79bdfd23438000f25e8", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22141, - { - "block": 4911677, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "wiss", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:36", - "trx_id": "c577ccf193e1c5dc8277d79bdfd23438000f25e8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22142, - { - "block": 4911678, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50712038, - "total_vote_weight": "7962930502798362229", - "voter": "thadm", - "weight": "75539317829704" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:39", - "trx_id": "859e7d25e4d311c6e9a98bfd3df95ffb10f5df4b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22143, - { - "block": 4911678, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "thadm", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:39", - "trx_id": "859e7d25e4d311c6e9a98bfd3df95ffb10f5df4b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22144, - { - "block": 4911688, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1538", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 53037689, - "total_vote_weight": "7963009505180164108", - "voter": "thermor", - "weight": "79002381801879" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:09", - "trx_id": "8122a81202cb7157b6b58f2e4d0b143c05cea072", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22145, - { - "block": 4911688, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "thermor", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:09", - "trx_id": "8122a81202cb7157b6b58f2e4d0b143c05cea072", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22146, - { - "block": 4911693, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1538", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 51033798, - "total_vote_weight": "7963085521538613938", - "voter": "stroully", - "weight": "76016358449830" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:24", - "trx_id": "65d8174d8c442b3aacb1ad72bfd5aac6100d3cd8", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22147, - { - "block": 4911693, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "stroully", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:24", - "trx_id": "65d8174d8c442b3aacb1ad72bfd5aac6100d3cd8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22148, - { - "block": 4911694, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "acidyo", - "pending_payout": { - "amount": "61", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-acidyo-sketch-kitty-cat-oc-20160912t173408484z", - "rshares": "203208547179", - "total_vote_weight": "891827284162997537", - "voter": "camilla", - "weight": "364162807699890660" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:27", - "trx_id": "7ea2e1fdb2e9d3f951c34425b12606185392f20d", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22149, - { - "block": 4911694, - "op": { - "type": "vote_operation", - "value": { - "author": "acidyo", - "permlink": "re-camilla-re-acidyo-sketch-kitty-cat-oc-20160912t173408484z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:27", - "trx_id": "7ea2e1fdb2e9d3f951c34425b12606185392f20d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22150, - { - "block": 4911696, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "acidyo", - "pending_payout": { - "amount": "63", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t172139964z", - "rshares": "203208547179", - "total_vote_weight": "915905112022831395", - "voter": "camilla", - "weight": "736653884490449334" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:33", - "trx_id": "7c215d409dc2b333298ff13ce1dad06c53fd4b2b", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22151, - { - "block": 4911696, - "op": { - "type": "vote_operation", - "value": { - "author": "acidyo", - "permlink": "re-camilla-apple-and-leaves-20160912t172139964z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:33", - "trx_id": "7c215d409dc2b333298ff13ce1dad06c53fd4b2b", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22152, - { - "block": 4911701, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1538", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50593879, - "total_vote_weight": "7963160881536491251", - "voter": "crion", - "weight": "75359997877313" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:48", - "trx_id": "ac6dbba5351c6f87f2262183a2f91a624970f8be", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22153, - { - "block": 4911701, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "crion", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:48", - "trx_id": "ac6dbba5351c6f87f2262183a2f91a624970f8be", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22154, - { - "block": 4911715, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1539", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 52651308, - "total_vote_weight": "7963239304941345177", - "voter": "revelbrooks", - "weight": "78423404853926" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:47:30", - "trx_id": "c31aae44e8233ebcbd28a67cb293b7c3d532f416", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22155, - { - "block": 4911715, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "revelbrooks", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:47:30", - "trx_id": "c31aae44e8233ebcbd28a67cb293b7c3d532f416", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22156, - { - "block": 4911717, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1539", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 55449983, - "total_vote_weight": "7963321895665919466", - "voter": "steemo", - "weight": "82590724574289" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:47:36", - "trx_id": "36c3a544d88b42686c062e5263e827661013dc93", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22157, - { - "block": 4911717, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "steemo", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:47:36", - "trx_id": "36c3a544d88b42686c062e5263e827661013dc93", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22158, - { - "block": 4911761, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 1165238399, - "total_vote_weight": "7965057174750676078", - "voter": "bullionstackers", - "weight": "1735279084756612" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:49:48", - "trx_id": "b565c0f8117c2912ea26f544828aff905b070aae", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22159, - { - "block": 4911761, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "bullionstackers", - "weight": 3400 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:49:48", - "trx_id": "b565c0f8117c2912ea26f544828aff905b070aae", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22160, - { - "block": 4911819, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1543", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 2308410567, - "total_vote_weight": "7968493176021161572", - "voter": "the-future", - "weight": "3436001270485494" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:52:48", - "trx_id": "0d9a12310ee72c6cf893354391bd05fda1ba73a9", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22161, - { - "block": 4911819, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "the-future", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:52:48", - "trx_id": "0d9a12310ee72c6cf893354391bd05fda1ba73a9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22162, - { - "block": 4911840, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1546", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "5091917697", - "total_vote_weight": "7976064386660099763", - "voter": "ben99", - "weight": "7571210638938191" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:53:51", - "trx_id": "8b6b819e7e536306eac0e296cd5bd614b0ecd120", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22163, - { - "block": 4911840, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ben99", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:53:51", - "trx_id": "8b6b819e7e536306eac0e296cd5bd614b0ecd120", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22164, - { - "block": 4912206, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1565", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "26148166391", - "total_vote_weight": "8014772570251297440", - "voter": "pixielolz", - "weight": "38708183591197677" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:12:09", - "trx_id": "d596699aea8cd68ba033c1d7a12513dd9e61de09", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22165, - { - "block": 4912206, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "pixielolz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:12:09", - "trx_id": "d596699aea8cd68ba033c1d7a12513dd9e61de09", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22166, - { - "block": 4912541, - "op": { - "type": "comment_operation", - "value": { - "author": "pixielolz", - "body": "That turned out pretty! Glad you like them ^_^ \n\nAnd thank you, and same to you :) I normally get super uncomfortable around new people, but not this time. ^_^", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t182902111z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:29:00", - "trx_id": "439e2674d1c8ac0b8e3d3c9e8f3d9ac96d8388f9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22167, - { - "block": 4912631, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "61", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t182902111z", - "rshares": "203208547179", - "total_vote_weight": "891827284162997537", - "voter": "camilla", - "weight": "133774092624449630" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:33:30", - "trx_id": "34e36fccfaef2aec6a9d60daacc4c4e2c720f9c9", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22168, - { - "block": 4912631, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "re-camilla-apple-and-leaves-20160912t182902111z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:33:30", - "trx_id": "34e36fccfaef2aec6a9d60daacc4c4e2c720f9c9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22169, - { - "block": 4912642, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "Thank you! I agree, it was so nice talking to you ^^", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "pixielolz", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t182902111z", - "permlink": "re-pixielolz-re-camilla-apple-and-leaves-20160912t183403150z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:34:03", - "trx_id": "4f27121b1b82683f0af6388d861618c38fa74fc9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22170, - { - "block": 4912716, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "wow! beautiful. Where is this?", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "natord", - "parent_permlink": "re-camilla-there-is-a-land-far-far-north-20160912t065441930z", - "permlink": "re-natord-re-camilla-there-is-a-land-far-far-north-20160912t183743684z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:37:45", - "trx_id": "6be4d6509f58bc3566d9451175468d92f8691096", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22171, - { - "block": 4912719, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "natord", - "pending_payout": { - "amount": "61", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160912t065441930z", - "rshares": "203208547179", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:37:54", - "trx_id": "1b58eeb64fae78266053d560c8d82fae1fe08f86", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22172, - { - "block": 4912719, - "op": { - "type": "vote_operation", - "value": { - "author": "natord", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160912t065441930z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:37:54", - "trx_id": "1b58eeb64fae78266053d560c8d82fae1fe08f86", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22173, - { - "block": 4912746, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1566", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "4487075430", - "total_vote_weight": "8021386217386554492", - "voter": "alexft", - "weight": "6613647135257052" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:39:18", - "trx_id": "7deff2e5e96a0f015627f3b4513e320ce6a7f5a9", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22174, - { - "block": 4912746, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "alexft", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:39:18", - "trx_id": "7deff2e5e96a0f015627f3b4513e320ce6a7f5a9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22175, - { - "block": 4912961, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "zivile", - "pending_payout": { - "amount": "125340", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "pumpkin-pie-with-meat", - "rshares": "203208547179", - "total_vote_weight": "16757885479050732772", - "voter": "camilla", - "weight": "7891753150417841" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:50:12", - "trx_id": "383099ec5dbeb22202801d5f4cf4747ab912a820", - "trx_in_block": 8, - "virtual_op": 1 - } - ], - [ - 22176, - { - "block": 4912961, - "op": { - "type": "vote_operation", - "value": { - "author": "zivile", - "permlink": "pumpkin-pie-with-meat", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:50:12", - "trx_id": "383099ec5dbeb22202801d5f4cf4747ab912a820", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 22177, - { - "block": 4913092, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1571", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "5731769098", - "total_vote_weight": "8029822271331451431", - "voter": "shortcut", - "weight": "8436053944896939" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:56:45", - "trx_id": "863a0a77eb6137ee0f109bab6a0083c851536414", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22178, - { - "block": 4913092, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "shortcut", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:56:45", - "trx_id": "863a0a77eb6137ee0f109bab6a0083c851536414", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22179, - { - "block": 4913106, - "op": { - "type": "comment_operation", - "value": { - "author": "shortcut", - "body": "Very nice. Seems to be an awesome tool.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t185728030z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:57:27", - "trx_id": "17f5277f62a6b5d32202198fec5f8eba5188978f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22180, - { - "block": 4913113, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shortcut", - "pending_payout": { - "amount": "61", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t185728030z", - "rshares": "203208547179", - "total_vote_weight": "891827284162997537", - "voter": "camilla", - "weight": "10404651648568304" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:57:48", - "trx_id": "bd17e905b5d092365e514261c28c21d3770bf567", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22181, - { - "block": 4913113, - "op": { - "type": "vote_operation", - "value": { - "author": "shortcut", - "permlink": "re-camilla-apple-and-leaves-20160912t185728030z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:57:48", - "trx_id": "bd17e905b5d092365e514261c28c21d3770bf567", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22182, - { - "block": 4913127, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "yes it is :) I would recommend them if you're an artist", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "shortcut", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t185728030z", - "permlink": "re-shortcut-re-camilla-apple-and-leaves-20160912t185830677z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:58:30", - "trx_id": "ec945b1d34bec580107a0e215ccb210976fd2a97", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22183, - { - "block": 4913184, - "op": { - "type": "comment_operation", - "value": { - "author": "shortcut", - "body": "I will see if I can get my hands on some here in germany.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "re-shortcut-re-camilla-apple-and-leaves-20160912t185830677z", - "permlink": "re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190120534z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:01:21", - "trx_id": "c8380e7afd771047bd0e7e515d74b6476b951c84", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22184, - { - "block": 4913269, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "curie", - "pending_payout": { - "amount": "340548", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "project-curie-s-daily-curation-list-11-sept-12-sept-2016", - "rshares": "203208547179", - "total_vote_weight": "17405155559370604577", - "voter": "camilla", - "weight": "2996416609912119" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:05:39", - "trx_id": "338a8fc1fdd1c1bcf829a9ad28b44f21b6d35fbb", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22185, - { - "block": 4913269, - "op": { - "type": "vote_operation", - "value": { - "author": "curie", - "permlink": "project-curie-s-daily-curation-list-11-sept-12-sept-2016", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:05:39", - "trx_id": "338a8fc1fdd1c1bcf829a9ad28b44f21b6d35fbb", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22186, - { - "block": 4913334, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shortcut", - "pending_payout": { - "amount": "60", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190120534z", - "rshares": "198975035779", - "total_vote_weight": "874127979041787408", - "voter": "camilla", - "weight": "219988874725516497" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:08:54", - "trx_id": "0d99fdf69a62ddd1417af8034d432bd28c6e8f4f", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 22187, - { - "block": 4913334, - "op": { - "type": "vote_operation", - "value": { - "author": "shortcut", - "permlink": "re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190120534z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:08:54", - "trx_id": "0d99fdf69a62ddd1417af8034d432bd28c6e8f4f", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 22188, - { - "block": 4913349, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "They are impossible to find in Norway, but you can order them online.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "shortcut", - "parent_permlink": "re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190120534z", - "permlink": "re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190939876z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:09:39", - "trx_id": "662aa1b35ba6b161c6af447438e187eeed37f112", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22189, - { - "block": 4913588, - "op": { - "type": "comment_operation", - "value": { - "author": "shortcut", - "body": "Sure, thanks again for your recommendation. I will most likely give them a try.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190939876z", - "permlink": "re-camilla-re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t192137286z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:21:36", - "trx_id": "21867d35699d4d1e052514949eae68ba1fb27ae7", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22190, - { - "block": 4913806, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shortcut", - "pending_payout": { - "amount": "60", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t192137286z", - "rshares": "198975035779", - "total_vote_weight": "874127979041787408", - "voter": "camilla", - "weight": "317599832385182758" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:32:30", - "trx_id": "980e478eaf85743c9cb0344aa0d513afe000fb98", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22191, - { - "block": 4913806, - "op": { - "type": "vote_operation", - "value": { - "author": "shortcut", - "permlink": "re-camilla-re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t192137286z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:32:30", - "trx_id": "980e478eaf85743c9cb0344aa0d513afe000fb98", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22192, - { - "block": 4914122, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"alexft\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:48:24", - "trx_id": "d2850ffda9af92ab4b605c9797c95267834f800b", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22193, - { - "block": 4914123, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"rarepepe\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:48:27", - "trx_id": "0659c06168130b94ae530500c5d1a8f55b65f3ba", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22194, - { - "block": 4914131, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"spectral\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:48:51", - "trx_id": "06b1aef2bf81b355f3b294dd0b19017af8d5fe78", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22195, - { - "block": 4914133, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"mun\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:48:57", - "trx_id": "c962338bd14ffd438776a22935c8dfd627943258", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22196, - { - "block": 4914134, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"kus-knee\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:49:00", - "trx_id": "faf88fccb59ef477073b27e64dae6767c5b9d720", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22197, - { - "block": 4915014, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "jamielefay", - "comment_permlink": "ahe-ey-allegiance-an-original-novel-part-18", - "curator": "camilla", - "reward": { - "amount": "1147565547", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T20:33:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 30 - } - ], - [ - 22198, - { - "block": 4916296, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "https://i.imgsafe.org/71f423f2a9.jpg\n\n### Drawing made with Prismacolor pencils on gray toned paper.", - "json_metadata": "{\"tags\":[\"art\",\"portrait\",\"\"],\"image\":[\"https://i.imgsafe.org/71f423f2a9.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "girl-with-a-pink-bow", - "title": "Girl with a Pink Bow" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:37:27", - "trx_id": "80c965965523ce8acfc51f73b884e7a054a35cd2", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22199, - { - "block": 4916296, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "63", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "203214055493", - "total_vote_weight": "891850289889720953", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:37:27", - "trx_id": "80c965965523ce8acfc51f73b884e7a054a35cd2", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22200, - { - "block": 4916296, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:37:27", - "trx_id": "80c965965523ce8acfc51f73b884e7a054a35cd2", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22201, - { - "block": 4916302, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "67", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "13292298415", - "total_vote_weight": "947191102219314573", - "voter": "goldmatters", - "weight": "553408123295936" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:37:45", - "trx_id": "dd597d493c3db0ce10accb154fef71299918e7bb", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22202, - { - "block": 4916302, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "goldmatters", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:37:45", - "trx_id": "dd597d493c3db0ce10accb154fef71299918e7bb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22203, - { - "block": 4916318, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "313", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "651650151993", - "total_vote_weight": "3289676669365350333", - "voter": "clains", - "weight": "85891137462021311" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:38:33", - "trx_id": "ff53785405c90524eb14733a62089b958f10c070", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 22204, - { - "block": 4916318, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "clains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:38:33", - "trx_id": "ff53785405c90524eb14733a62089b958f10c070", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 22205, - { - "block": 4916323, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "370", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "130765654564", - "total_vote_weight": "3686166908016189812", - "voter": "psylains", - "weight": "17842060739287776" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:38:48", - "trx_id": "e28e29607feec76332c15c9a999045cd22a0ea55", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 22206, - { - "block": 4916323, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "psylains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:38:48", - "trx_id": "e28e29607feec76332c15c9a999045cd22a0ea55", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 22207, - { - "block": 4916338, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "506", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "289496406729", - "total_vote_weight": "4494184275858851371", - "voter": "anonymous", - "weight": "56561215748986309" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:39:33", - "trx_id": "bef8d8547aeda34ea8ea5eac653dc1d8a751c32f", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22208, - { - "block": 4916338, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "anonymous", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:39:33", - "trx_id": "bef8d8547aeda34ea8ea5eac653dc1d8a751c32f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22209, - { - "block": 4916338, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "506", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 706772487, - "total_vote_weight": "4496048721254833974", - "voter": "murh", - "weight": "130511177718782" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:39:33", - "trx_id": "fd6ceb6f771ec2253c192556c371833962d168d8", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22210, - { - "block": 4916338, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "murh", - "weight": 1509 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:39:33", - "trx_id": "fd6ceb6f771ec2253c192556c371833962d168d8", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22211, - { - "block": 4916360, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -20,17 +20,17 @@\n rg/7\n-1f423f2a9\n+20a7b6b2e\n .jpg\n", - "json_metadata": "{\"tags\":[\"art\",\"portrait\",\"\"],\"image\":[\"https://i.imgsafe.org/720a7b6b2e.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "girl-with-a-pink-bow", - "title": "Girl with a Pink Bow" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:40:39", - "trx_id": "a2071624a1a449c2999d18d9e83cf0d2067deeef", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22212, - { - "block": 4916366, - "op": { - "type": "comment_operation", - "value": { - "author": "kalipo", - "body": "very nice post :) would be nice if you check my channel, perhaps u like my art. i followed you! keep it on <3 kalipo", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "girl-with-a-pink-bow", - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t214058303z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:40:57", - "trx_id": "24e934a2692eecc3de16a50f7ac7601c1c72b247", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22213, - { - "block": 4916369, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "515", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "17018803209", - "total_vote_weight": "4540793856788001810", - "voter": "samether", - "weight": "5518566715757366" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:41:09", - "trx_id": "e6de8a33e981a8690d70c57d277d06d271118290", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22214, - { - "block": 4916369, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "samether", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:41:09", - "trx_id": "e6de8a33e981a8690d70c57d277d06d271118290", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22215, - { - "block": 4916400, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"kalipo\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:42:42", - "trx_id": "0b7a86690f3a9f5d35a5be3ed10a8c1fb12b6705", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22216, - { - "block": 4916410, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "515", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 143406202, - "total_vote_weight": "4541169675001274297", - "voter": "anomaly", - "weight": "72031824210560" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:43:12", - "trx_id": "15d9a75cd6dc97335c7d7ed062827acf6e84e3b5", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22217, - { - "block": 4916410, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "anomaly", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:43:12", - "trx_id": "15d9a75cd6dc97335c7d7ed062827acf6e84e3b5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22218, - { - "block": 4916412, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "I love your art :) I follow you too ^^", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "kalipo", - "parent_permlink": "re-camilla-girl-with-a-pink-bow-20160912t214058303z", - "permlink": "re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t214319415z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:43:18", - "trx_id": "7e96c2fd0123e831143cbc360541744c7064e5c7", - "trx_in_block": 15, - "virtual_op": 0 - } - ], - [ - 22219, - { - "block": 4916444, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "516", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 2340059283, - "total_vote_weight": "4547299294718017826", - "voter": "steemswede", - "weight": "1522188896324643" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:44:54", - "trx_id": "df8a1f4d39cd63d72c8f4b896e0dfaadeb1648c5", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22220, - { - "block": 4916444, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "steemswede", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:44:54", - "trx_id": "df8a1f4d39cd63d72c8f4b896e0dfaadeb1648c5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22221, - { - "block": 4916475, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "516", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 661462066, - "total_vote_weight": "4549030968269369775", - "voter": "elishagh1", - "weight": "519502065405584" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:46:27", - "trx_id": "b90ef08565b157be3936add6f220d13a2ee0785e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22222, - { - "block": 4916475, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "elishagh1", - "weight": 200 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:46:27", - "trx_id": "b90ef08565b157be3936add6f220d13a2ee0785e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22223, - { - "block": 4916492, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "9721807408", - "total_vote_weight": "4574432474926443145", - "voter": "andread", - "weight": "8340161352405756" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:47:18", - "trx_id": "104c5e979574ce38dbbe4be2788e79d112a66cb7", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22224, - { - "block": 4916492, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "andread", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:47:18", - "trx_id": "104c5e979574ce38dbbe4be2788e79d112a66cb7", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22225, - { - "block": 4916571, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "517", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 472813942, - "total_vote_weight": "4575665493525935200", - "voter": "wuyueling", - "weight": "567188555766345" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:51:15", - "trx_id": "177d9df39d6ebde2134b872df92d0637797eef63", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22226, - { - "block": 4916571, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "wuyueling", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:51:15", - "trx_id": "177d9df39d6ebde2134b872df92d0637797eef63", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22227, - { - "block": 4916587, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "517", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 174554557, - "total_vote_weight": "4576120646820479706", - "voter": "chadcrypto", - "weight": "221507936678326" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:52:03", - "trx_id": "676c86f57df989194825764317b4be0ff7843f30", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22228, - { - "block": 4916587, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "chadcrypto", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:52:03", - "trx_id": "676c86f57df989194825764317b4be0ff7843f30", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22229, - { - "block": 4916675, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "517", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 3321707002, - "total_vote_weight": "4584776352339379143", - "voter": "glitterpig", - "weight": "5481946828636310" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:56:27", - "trx_id": "1d7249cc7441e461430860771500e4dfae7527b4", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22230, - { - "block": 4916675, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "glitterpig", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:56:27", - "trx_id": "1d7249cc7441e461430860771500e4dfae7527b4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22231, - { - "block": 4916677, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "546", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "59861247996", - "total_vote_weight": "4738931872898669206", - "voter": "theshell", - "weight": "98145681422748006" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:56:33", - "trx_id": "48aed6599d43339b41438dc0f3b67e051661be10", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22232, - { - "block": 4916677, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "theshell", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:56:33", - "trx_id": "48aed6599d43339b41438dc0f3b67e051661be10", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22233, - { - "block": 4916692, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "549", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "5606477483", - "total_vote_weight": "4753194336789149113", - "voter": "shortcut", - "weight": "9436996940867538" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:57:18", - "trx_id": "83e60e2795eeddbd13ee88f12f34c818d3650cf7", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22234, - { - "block": 4916692, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "shortcut", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:57:18", - "trx_id": "83e60e2795eeddbd13ee88f12f34c818d3650cf7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22235, - { - "block": 4916736, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -21,16 +21,16 @@\n g/72\n-0a7b6b2e\n+52ed86f2\n .jpg\n", - "json_metadata": "{\"tags\":[\"art\",\"portrait\",\"\"],\"image\":[\"https://i.imgsafe.org/7252ed86f2.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "girl-with-a-pink-bow", - "title": "Girl with a Pink Bow" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:59:33", - "trx_id": "584db637e10b487887e595df3dd7b30b167e25b5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22236, - { - "block": 4916748, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "560", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "26148166391", - "total_vote_weight": "4819323227520767625", - "voter": "pixielolz", - "weight": "50037527320258007" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:09", - "trx_id": "5cd6808ea328edd01a08c38503bc537aef69404a", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22237, - { - "block": 4916748, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "pixielolz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:09", - "trx_id": "5cd6808ea328edd01a08c38503bc537aef69404a", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22238, - { - "block": 4916749, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "566", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "12188532385", - "total_vote_weight": "4849930351141385874", - "voter": "acidyo", - "weight": "23210402078968838" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:12", - "trx_id": "b43483f8c49ff4038c8a1b37e6a4eba41fea9d9e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22239, - { - "block": 4916749, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "acidyo", - "weight": 4200 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:12", - "trx_id": "b43483f8c49ff4038c8a1b37e6a4eba41fea9d9e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22240, - { - "block": 4916756, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "kalipo", - "pending_payout": { - "amount": "62", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t214058303z", - "rshares": "203214055493", - "total_vote_weight": "891850289889720953", - "voter": "camilla", - "weight": "582675522727951022" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:33", - "trx_id": "f5bff67af518a6694d53487017269cd4569e2a2c", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22241, - { - "block": 4916756, - "op": { - "type": "vote_operation", - "value": { - "author": "kalipo", - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t214058303z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:33", - "trx_id": "f5bff67af518a6694d53487017269cd4569e2a2c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22242, - { - "block": 4916757, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "565", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 760600032, - "total_vote_weight": "4851835768230529166", - "voter": "coar", - "weight": "1470346853788906" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:36", - "trx_id": "7b057dccf6e308177c64a1424b6cb2e334b7d91b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22243, - { - "block": 4916757, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "coar", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:36", - "trx_id": "7b057dccf6e308177c64a1424b6cb2e334b7d91b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22244, - { - "block": 4916758, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "565", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 102583242, - "total_vote_weight": "4852092713758591955", - "voter": "cryptochannel", - "weight": "198704541701890" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:39", - "trx_id": "7196d8de9ff44afcbb96a7628072d83f99d3e976", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22245, - { - "block": 4916758, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "cryptochannel", - "weight": 4200 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:39", - "trx_id": "7196d8de9ff44afcbb96a7628072d83f99d3e976", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22246, - { - "block": 4916758, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "565", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 103512966, - "total_vote_weight": "4852351978169368919", - "voter": "strawhat", - "weight": "200497811000852" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:39", - "trx_id": "ed35f7d315d328863fe444f3c3c461b5525f1229", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22247, - { - "block": 4916758, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "strawhat", - "weight": 4200 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:39", - "trx_id": "ed35f7d315d328863fe444f3c3c461b5525f1229", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22248, - { - "block": 4916780, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "567", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 2337983869, - "total_vote_weight": "4858205191457479243", - "voter": "cryptoeasy", - "weight": "4741102763369362" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:01:45", - "trx_id": "1a78053e811a41f83f7b60f8172e77dc40f430f3", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22249, - { - "block": 4916780, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "cryptoeasy", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:01:45", - "trx_id": "1a78053e811a41f83f7b60f8172e77dc40f430f3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22250, - { - "block": 4916808, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dollarvigilante", - "pending_payout": { - "amount": "309284", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "boom-end-game-nears-as-central-banks-buying-up-gold-mining-companies", - "rshares": "198980429337", - "total_vote_weight": "17347502297607352140", - "voter": "camilla", - "weight": "3262736250790415" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:03:09", - "trx_id": "128a754b49f592ce7ed2d36cc331d07d0d5bd576", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22251, - { - "block": 4916808, - "op": { - "type": "vote_operation", - "value": { - "author": "dollarvigilante", - "permlink": "boom-end-game-nears-as-central-banks-buying-up-gold-mining-companies", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:03:09", - "trx_id": "128a754b49f592ce7ed2d36cc331d07d0d5bd576", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22252, - { - "block": 4916810, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "timsaid", - "pending_payout": { - "amount": "274975", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "life-explorers-the-human-senses-part-iii-touch", - "rshares": "198980429337", - "total_vote_weight": "17283096775208858980", - "voter": "camilla", - "weight": "3663011961919186" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:03:15", - "trx_id": "5681959624007665e9daf2ebaa79af7325b24739", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22253, - { - "block": 4916810, - "op": { - "type": "vote_operation", - "value": { - "author": "timsaid", - "permlink": "life-explorers-the-human-senses-part-iii-touch", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:03:15", - "trx_id": "5681959624007665e9daf2ebaa79af7325b24739", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22254, - { - "block": 4916984, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "572", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "11707843677", - "total_vote_weight": "4887440477506390273", - "voter": "primus", - "weight": "29235286048911030" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:12:00", - "trx_id": "3e9a27d17780f835cc1e5b29a0294fb9110b6660", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22255, - { - "block": 4916984, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "primus", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:12:00", - "trx_id": "3e9a27d17780f835cc1e5b29a0294fb9110b6660", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22256, - { - "block": 4917019, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "so strange! I have no idea how this happened?", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "shenanigator", - "parent_permlink": "re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t220903089z", - "permlink": "re-shenanigator-re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t221343480z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:13:45", - "trx_id": "7db07dbca76911fe76023de2a15ecb7bbffbcaa9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22257, - { - "block": 4917068, - "op": { - "type": "delete_comment_operation", - "value": { - "author": "camilla", - "permlink": "re-shenanigator-re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t221343480z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:16:12", - "trx_id": "0ee71502d76304c9db7f67d03bf30e05f1b1ec37", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22258, - { - "block": 4917086, - "op": { - "type": "delete_comment_operation", - "value": { - "author": "camilla", - "permlink": "re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t214319415z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:17:06", - "trx_id": "653622fd30607eebe79634ea272aa9a28a1dc085", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22259, - { - "block": 4917112, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shenanigator", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t220903089z", - "rshares": -198980429337, - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:18:24", - "trx_id": "46e2666911f2d83905eb98617ce1b194d013dffe", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22260, - { - "block": 4917112, - "op": { - "type": "vote_operation", - "value": { - "author": "shenanigator", - "permlink": "re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t220903089z", - "voter": "camilla", - "weight": -10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:18:24", - "trx_id": "46e2666911f2d83905eb98617ce1b194d013dffe", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22261, - { - "block": 4917164, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "573", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "8529222483", - "total_vote_weight": "4908659449446431993", - "voter": "mione", - "weight": "21218971940041720" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:21:00", - "trx_id": "98d18c96ce0f5d80843e040f46744a8d48986f83", - "trx_in_block": 8, - "virtual_op": 1 - } - ], - [ - 22262, - { - "block": 4917164, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "mione", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:21:00", - "trx_id": "98d18c96ce0f5d80843e040f46744a8d48986f83", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 22263, - { - "block": 4917165, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "659", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "166818556981", - "total_vote_weight": "5310714918838842190", - "voter": "highasfuck", - "weight": "402055469392410197" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:21:03", - "trx_id": "930d3ad18443bb7b97ae4af3defdf1bf8aff3da3", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22264, - { - "block": 4917165, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "highasfuck", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:21:03", - "trx_id": "930d3ad18443bb7b97ae4af3defdf1bf8aff3da3", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22265, - { - "block": 4917752, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "877", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "464346063318", - "total_vote_weight": "6313703114050534435", - "voter": "glitterfart", - "weight": "1002988195211692245" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:50:27", - "trx_id": "b600c85b0b4cb2a154bf388b22a92df4f0376aee", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22266, - { - "block": 4917752, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "glitterfart", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:50:27", - "trx_id": "b600c85b0b4cb2a154bf388b22a92df4f0376aee", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22267, - { - "block": 4917799, - "op": { - "type": "comment_operation", - "value": { - "author": "karenb54", - "body": "Fab drawing, Well done\n I can't draw wish I could", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "girl-with-a-pink-bow", - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t225246008z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:52:48", - "trx_id": "c16690c695adf35fdc21ef351effe74cd374c907", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 22268, - { - "block": 4917804, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "875", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 508520907, - "total_vote_weight": "6314717567476877096", - "voter": "karenb54", - "weight": "1014453426342661" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:53:03", - "trx_id": "b7dad8ffe592b9195b507de4164f04f962766178", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22269, - { - "block": 4917804, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "karenb54", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:53:03", - "trx_id": "b7dad8ffe592b9195b507de4164f04f962766178", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22270, - { - "block": 4918292, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1497", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 1192255729, - "total_vote_weight": "8031575325835864463", - "voter": "ndbeledrifts", - "weight": "1753054504413032" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T23:17:36", - "trx_id": "48a05bad4d1d2ab9db971e836030d8d28172983b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22271, - { - "block": 4918292, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ndbeledrifts", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T23:17:36", - "trx_id": "48a05bad4d1d2ab9db971e836030d8d28172983b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22272, - { - "block": 4919558, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 916860564, - "total_vote_weight": 0, - "voter": "gretepe", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:21:03", - "trx_id": "e6fc3478ca7807d76b6c60d397861af6b95df2ce", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22273, - { - "block": 4919558, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "gretepe", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:21:03", - "trx_id": "e6fc3478ca7807d76b6c60d397861af6b95df2ce", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22274, - { - "block": 4919579, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 898882906, - "total_vote_weight": 0, - "voter": "gretepe", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:22:06", - "trx_id": "cd4bb7587bfa68de9a8e252471f64a8f765a61a2", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22275, - { - "block": 4919579, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "gretepe", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:22:06", - "trx_id": "cd4bb7587bfa68de9a8e252471f64a8f765a61a2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22276, - { - "block": 4919592, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1490", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 898882906, - "total_vote_weight": "8032896624299636937", - "voter": "gretepe", - "weight": "1321298463772474" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:22:45", - "trx_id": "2289ce7db23ba1807eb571ed3a8e735fe9f1950d", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22277, - { - "block": 4919592, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "gretepe", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:22:45", - "trx_id": "2289ce7db23ba1807eb571ed3a8e735fe9f1950d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22278, - { - "block": 4920186, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "868", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 116538182, - "total_vote_weight": "6314950026765510483", - "voter": "bullionstackers", - "weight": "232459288633387" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:52:33", - "trx_id": "62a1e247a332ff9b1ecec53b6107eb9791f7dfc9", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22279, - { - "block": 4920186, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "bullionstackers", - "weight": 100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:52:33", - "trx_id": "62a1e247a332ff9b1ecec53b6107eb9791f7dfc9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22280, - { - "block": 4920201, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "876", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "12345609219", - "total_vote_weight": "6339525518887297481", - "voter": "asim", - "weight": "24575492121786998" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:53:18", - "trx_id": "fae458bf640f2448856675cd48a113fc80f4e1f2", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22281, - { - "block": 4920201, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "asim", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:53:18", - "trx_id": "fae458bf640f2448856675cd48a113fc80f4e1f2", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22282, - { - "block": 4920233, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "shadowspub", - "comment_permlink": "sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th", - "curator": "camilla", - "reward": { - "amount": "97569025", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:54:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 22283, - { - "block": 4921186, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "sirwinchester", - "comment_permlink": "bitcoin-exchange-berlin-meetup-with-ned-on-skype", - "curator": "camilla", - "reward": { - "amount": "164618133", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T01:42:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 64 - } - ], - [ - 22284, - { - "block": 4922034, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1516", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 3892676217, - "total_vote_weight": "8038614733696221237", - "voter": "alchemage", - "weight": "5718109396584300" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:25:12", - "trx_id": "c2a1254b37f0429e30bda8adea60ae1099df59af", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22285, - { - "block": 4922034, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "alchemage", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:25:12", - "trx_id": "c2a1254b37f0429e30bda8adea60ae1099df59af", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22286, - { - "block": 4922045, - "op": { - "type": "comment_operation", - "value": { - "author": "alchemage", - "body": "Prisma makes the best colouring tools! They're just sooooo expensive!\n\nBeautiful work! :D", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160913t022535929z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:25:45", - "trx_id": "3fe18bc624bc25492a8c9ebf1424d927ba5d3129", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22287, - { - "block": 4922381, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1519", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 2911202361, - "total_vote_weight": "8042887013992325664", - "voter": "ace108", - "weight": "4272280296104427" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:42:39", - "trx_id": "3e85ccfa7ee00166bf7bcc91f70613a2833a3625", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22288, - { - "block": 4922381, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ace108", - "weight": 8600 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:42:39", - "trx_id": "3e85ccfa7ee00166bf7bcc91f70613a2833a3625", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22289, - { - "block": 4922572, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1977901532, - "total_vote_weight": 0, - "voter": "haphazard-hstead", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:52:12", - "trx_id": "0ae91d565a959caacb9acacd6876eddf23a763bd", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22290, - { - "block": 4922572, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "haphazard-hstead", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:52:12", - "trx_id": "0ae91d565a959caacb9acacd6876eddf23a763bd", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22291, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "how-high-can-steem-fly-butterfly-art-and-thoughts" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 22292, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-trendwizard-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t135705568z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 22293, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-mun-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t141310004z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 22294, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-alexft-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t141337301z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 22295, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-acidyo-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t143931038z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 12 - } - ], - [ - 22296, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-mun-re-camilla-re-mun-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t152547164z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 22297, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-tmendieta-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t195024022z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 20 - } - ], - [ - 22298, - { - "block": 4925961, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "837", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "4362079757", - "total_vote_weight": "6348185001005246927", - "voter": "alexft", - "weight": "8659482117949446" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T05:42:33", - "trx_id": "e43d6fb2e402e345d3b37e2403476968263fb22a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22299, - { - "block": 4925961, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "alexft", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T05:42:33", - "trx_id": "e43d6fb2e402e345d3b37e2403476968263fb22a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22300, - { - "block": 4926223, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "838", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 3912738831, - "total_vote_weight": "6355941931682000971", - "voter": "sompitonov", - "weight": "7756930676754044" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T05:55:39", - "trx_id": "4cc65bc0edcbbcea32e83819b0eada407e9197fe", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22301, - { - "block": 4926223, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "sompitonov", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T05:55:39", - "trx_id": "4cc65bc0edcbbcea32e83819b0eada407e9197fe", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22302, - { - "block": 4926263, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "Wow! Her eyes freezes me!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "girl-with-a-pink-bow", - "permlink": "re-camilla-girl-with-a-pink-bow-20160913t055737074z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T05:57:39", - "trx_id": "4f21554c97bf1c48f3725e8340107787b5ceb4f3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22303, - { - "block": 4928546, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "854", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 154310336, - "total_vote_weight": "6356247645135058621", - "voter": "sergey44", - "weight": "305713453057650" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T07:52:09", - "trx_id": "36ebf81223e4fae438d8c258f10e8cc6030fdc5c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22304, - { - "block": 4928546, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "sergey44", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T07:52:09", - "trx_id": "36ebf81223e4fae438d8c258f10e8cc6030fdc5c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22305, - { - "block": 4929416, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "sompitonov", - "pending_payout": { - "amount": "61", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-girl-with-a-pink-bow-20160913t055737074z", - "rshares": "215916271116", - "total_vote_weight": "944741768690820328", - "voter": "camilla", - "weight": "944741768690820328" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:35:45", - "trx_id": "e7ff13a260287b16cde8f21e07179b9c060f26c5", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22306, - { - "block": 4929416, - "op": { - "type": "vote_operation", - "value": { - "author": "sompitonov", - "permlink": "re-camilla-girl-with-a-pink-bow-20160913t055737074z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:35:45", - "trx_id": "e7ff13a260287b16cde8f21e07179b9c060f26c5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22307, - { - "block": 4929419, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "karenb54", - "pending_payout": { - "amount": "60", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t225246008z", - "rshares": "211682618741", - "total_vote_weight": "929686492364995100", - "voter": "camilla", - "weight": "926872755111837607" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:35:54", - "trx_id": "7ecd982e14f18bc256f8af041d27ae52c9eeb0ba", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22308, - { - "block": 4929419, - "op": { - "type": "vote_operation", - "value": { - "author": "karenb54", - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t225246008z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:35:54", - "trx_id": "7ecd982e14f18bc256f8af041d27ae52c9eeb0ba", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22309, - { - "block": 4929479, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "aldentan", - "pending_payout": { - "amount": "60", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "from-weak-ass-bitch-to-ripped-my-fight-with-hyperthyroidism", - "rshares": "211682618741", - "total_vote_weight": "928876702511532069", - "voter": "camilla", - "weight": "597889664822415824" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:38:54", - "trx_id": "b5b7a8a56ceb5ff72e526ecd54ef37857cda8b95", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22310, - { - "block": 4929479, - "op": { - "type": "vote_operation", - "value": { - "author": "aldentan", - "permlink": "from-weak-ass-bitch-to-ripped-my-fight-with-hyperthyroidism", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:38:54", - "trx_id": "b5b7a8a56ceb5ff72e526ecd54ef37857cda8b95", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22311, - { - "block": 4929501, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "hisnameisolllie", - "pending_payout": { - "amount": "40089", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-last-12-weeks-at-the-university-of-steemit-where-has-the-time-gone", - "rshares": "207448966366", - "total_vote_weight": "15660660608835024624", - "voter": "camilla", - "weight": "19649366308309222" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:40:00", - "trx_id": "0a992b0c3949f50611be07a5c4eb9d608e8222fb", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22312, - { - "block": 4929501, - "op": { - "type": "vote_operation", - "value": { - "author": "hisnameisolllie", - "permlink": "the-last-12-weeks-at-the-university-of-steemit-where-has-the-time-gone", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:40:00", - "trx_id": "0a992b0c3949f50611be07a5c4eb9d608e8222fb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22313, - { - "block": 4929505, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "elfkitchen", - "pending_payout": { - "amount": "5847", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "i-want-to-take-you-into-the-chinese-vegetable-market", - "rshares": "207448966366", - "total_vote_weight": "12047910933874615266", - "voter": "camilla", - "weight": "117224190815448576" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:40:12", - "trx_id": "dcf5095a210294298ff3be5916d22abfc4933748", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22314, - { - "block": 4929505, - "op": { - "type": "vote_operation", - "value": { - "author": "elfkitchen", - "permlink": "i-want-to-take-you-into-the-chinese-vegetable-market", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:40:12", - "trx_id": "dcf5095a210294298ff3be5916d22abfc4933748", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22315, - { - "block": 4929545, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-helle-hello-steemit-world-20160813t200219117z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:42:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 22316, - { - "block": 4929545, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-faraz-re-helle-hello-steemit-world-20160813t200531944z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:42:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 12 - } - ], - [ - 22317, - { - "block": 4930158, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1544", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "75110722747", - "total_vote_weight": "8151914442177577478", - "voter": "easteagle13", - "weight": "109027428185251814" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:13:00", - "trx_id": "1af044527f5c98948ea8141c4a61d0bc1e653615", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22318, - { - "block": 4930158, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "easteagle13", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:13:00", - "trx_id": "1af044527f5c98948ea8141c4a61d0bc1e653615", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22319, - { - "block": 4930528, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "smailer", - "pending_payout": { - "amount": "4061", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-daytime-latte-art-tryings-crown", - "rshares": "211682618741", - "total_vote_weight": "11027982296404596121", - "voter": "camilla", - "weight": "93032810721398963" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:31:33", - "trx_id": "edd2d9a16c95c44ed1a7f43068378380465951c9", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22320, - { - "block": 4930528, - "op": { - "type": "vote_operation", - "value": { - "author": "smailer", - "permlink": "my-daytime-latte-art-tryings-crown", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:31:33", - "trx_id": "edd2d9a16c95c44ed1a7f43068378380465951c9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22321, - { - "block": 4930534, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "yangyang", - "pending_payout": { - "amount": "8042", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-happy-whale", - "rshares": "207448966366", - "total_vote_weight": "12773029243061429974", - "voter": "camilla", - "weight": "91970812938821268" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:31:51", - "trx_id": "6037f736be2e45af53db6fd07670796d4d4dde85", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22322, - { - "block": 4930534, - "op": { - "type": "vote_operation", - "value": { - "author": "yangyang", - "permlink": "my-happy-whale", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:31:51", - "trx_id": "6037f736be2e45af53db6fd07670796d4d4dde85", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22323, - { - "block": 4932009, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-clains-free-will-and-conscious-freedom-20160813t203846578z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 22324, - { - "block": 4932009, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-spetey-re-clains-free-will-and-conscious-freedom-20160814t035556706z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 46 - } - ], - [ - 22325, - { - "block": 4932009, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-camilla-re-spetey-re-clains-free-will-and-conscious-freedom-20160814t035643710z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 47 - } - ], - [ - 22326, - { - "block": 4932009, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-freeradical-re-clains-free-will-and-conscious-freedom-20160814t040458759z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 48 - } - ], - [ - 22327, - { - "block": 4932009, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "395495911", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "138", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "re-spetey-re-camilla-re-spetey-re-clains-free-will-and-conscious-freedom-20160814t111905211z", - "vesting_payout": { - "amount": "611497524", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 64 - } - ], - [ - 22328, - { - "block": 4932009, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 401, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "89", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "366", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-spetey-re-camilla-re-spetey-re-clains-free-will-and-conscious-freedom-20160814t111905211z", - "total_payout_value": { - "amount": "276", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 65 - } - ], - [ - 22329, - { - "block": 4932009, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-spetey-re-camilla-re-spetey-re-clains-free-will-and-conscious-freedom-20160814t111905211z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 66 - } - ], - [ - 22330, - { - "block": 4932204, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "905", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 1608900298, - "total_vote_weight": "6359434213254634812", - "voter": "gidlark", - "weight": "3186568119576191" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:55:36", - "trx_id": "a959195a587828ef88d3f51198076afd57074f3e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22331, - { - "block": 4932204, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "gidlark", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:55:36", - "trx_id": "a959195a587828ef88d3f51198076afd57074f3e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22332, - { - "block": 4932837, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "940", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "46148758185", - "total_vote_weight": "6450126173147164114", - "voter": "isteemit", - "weight": "90691959892529302" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T11:27:21", - "trx_id": "4e9dcfa51dc773e72e20cf86fe83fc89361756ac", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22333, - { - "block": 4932837, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "isteemit", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T11:27:21", - "trx_id": "4e9dcfa51dc773e72e20cf86fe83fc89361756ac", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22334, - { - "block": 4933254, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "945", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 290559594, - "total_vote_weight": "6450692872404442054", - "voter": "sonyanka", - "weight": "566699257277940" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T11:48:21", - "trx_id": "acf3bd90598e4c71bfe91eb9c11cbd58f7c6bbab", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22335, - { - "block": 4933254, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "sonyanka", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T11:48:21", - "trx_id": "acf3bd90598e4c71bfe91eb9c11cbd58f7c6bbab", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22336, - { - "block": 4939802, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alex2016", - "comment_permlink": "my-new-works-from-genuine-leather", - "curator": "camilla", - "reward": { - "amount": "57718574", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:17:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 22337, - { - "block": 4939875, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "kalipo", - "comment_permlink": "raptor-art-drawing-all-day", - "curator": "camilla", - "reward": { - "amount": "60755575", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:21:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 22338, - { - "block": 4939951, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "805", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 330853272, - "total_vote_weight": "6451338094129531484", - "voter": "helle", - "weight": "645221725089430" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:45", - "trx_id": "70d923ecbbbbc1c8567f88491e141ec38791287b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22339, - { - "block": 4939951, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:45", - "trx_id": "70d923ecbbbbc1c8567f88491e141ec38791287b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22340, - { - "block": 4939952, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1381", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 324365953, - "total_vote_weight": "8152380322344461750", - "voter": "helle", - "weight": "465880166884272" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:48", - "trx_id": "e53e84b7c168554f6bdf4bf12a338d07d52998fb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22341, - { - "block": 4939952, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:48", - "trx_id": "e53e84b7c168554f6bdf4bf12a338d07d52998fb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22342, - { - "block": 4939955, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 324365953, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:57", - "trx_id": "3d41bd7704c57aeb1049339ef6485c5dd6cc1033", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22343, - { - "block": 4939955, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:57", - "trx_id": "3d41bd7704c57aeb1049339ef6485c5dd6cc1033", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22344, - { - "block": 4940027, - "op": { - "type": "comment_operation", - "value": { - "author": "helle", - "body": "Love the pictures and you write with your soul <3 proud of you :)", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160913t172833838z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:28:33", - "trx_id": "aa33f94b355d32c5ae0f46ba86d8a54b74b0f3fc", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22345, - { - "block": 4940035, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 324365953, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:28:57", - "trx_id": "a66c5640ce6432756dd2a1fbfa6c4c5f4dad6c98", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22346, - { - "block": 4940035, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:28:57", - "trx_id": "a66c5640ce6432756dd2a1fbfa6c4c5f4dad6c98", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22347, - { - "block": 4940038, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-castle-from-my-dreams", - "rshares": 317878634, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:06", - "trx_id": "bdaa3513584e7dd83c1739b08c498a633cfb17f2", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22348, - { - "block": 4940038, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "the-castle-from-my-dreams", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:06", - "trx_id": "bdaa3513584e7dd83c1739b08c498a633cfb17f2", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22349, - { - "block": 4940040, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "48", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colours-of-a-peacock", - "rshares": 317878634, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:12", - "trx_id": "a1ab35bd31b6031b4f1131a97a368b4a3f18d72e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22350, - { - "block": 4940040, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colours-of-a-peacock", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:12", - "trx_id": "a1ab35bd31b6031b4f1131a97a368b4a3f18d72e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22351, - { - "block": 4940041, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "do-you-like-to-colour", - "rshares": 317878634, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:15", - "trx_id": "89e62eb2882d6ff5c4f4141fbd13df2ee4411df4", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22352, - { - "block": 4940041, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "do-you-like-to-colour", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:15", - "trx_id": "89e62eb2882d6ff5c4f4141fbd13df2ee4411df4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22353, - { - "block": 4940043, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "zendoodle-drawing", - "rshares": 317878634, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:21", - "trx_id": "d3b5cda99c5a76f057848434b15d6c38d50c0e35", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22354, - { - "block": 4940043, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "zendoodle-drawing", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:21", - "trx_id": "d3b5cda99c5a76f057848434b15d6c38d50c0e35", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22355, - { - "block": 4940047, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "broccoli-and-avocado-soup", - "rshares": 311391315, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:33", - "trx_id": "252a7b954eaaf3f654d493f7aafcd82886267c50", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22356, - { - "block": 4940047, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "broccoli-and-avocado-soup", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:33", - "trx_id": "252a7b954eaaf3f654d493f7aafcd82886267c50", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22357, - { - "block": 4940048, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "bluebird-on-golden-cup", - "rshares": 311391315, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:36", - "trx_id": "df480401e8a6e086b6e5f7c6a3a3b3b084da84dd", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22358, - { - "block": 4940048, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "bluebird-on-golden-cup", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:36", - "trx_id": "df480401e8a6e086b6e5f7c6a3a3b3b084da84dd", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22359, - { - "block": 4940050, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "portrait-of-a-lady-on-blue-background", - "rshares": 311391315, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:42", - "trx_id": "ce95168d83eda8afff590cad387fdfb52fe2877d", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22360, - { - "block": 4940050, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "portrait-of-a-lady-on-blue-background", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:42", - "trx_id": "ce95168d83eda8afff590cad387fdfb52fe2877d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22361, - { - "block": 4940051, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-steemycyborg-elf-with-magic-powers", - "rshares": 311391315, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:45", - "trx_id": "ff0106b65c173c97f65947a35f1cdbf2280fe7ec", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22362, - { - "block": 4940051, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "a-steemycyborg-elf-with-magic-powers", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:45", - "trx_id": "ff0106b65c173c97f65947a35f1cdbf2280fe7ec", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22363, - { - "block": 4940052, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "how-high-can-steem-fly-butterfly-art-and-thoughts", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:48", - "trx_id": "7561321f00112a38711f0a500a71d4de2a7b6693", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22364, - { - "block": 4940056, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "a-steem-whale-in-a-dream-world-art-and-poem", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:00", - "trx_id": "d9d0de2b48c6fc1878f79d144aea96b40a87d0ec", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22365, - { - "block": 4940058, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "crazy-flood-on-the-subway-tracks", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:06", - "trx_id": "41655908ee032c9570105e699a3cc08affe8b867", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22366, - { - "block": 4940060, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "my-ladybug-drawing", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:12", - "trx_id": "9dbc9f51eee41973a1b64256c23f6305fe83f39d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22367, - { - "block": 4940064, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "how-to-survive-as-a-student", - "rshares": 304903995, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:24", - "trx_id": "0be7622f8865a3e2ad0418d971538f2bc5c0f7eb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22368, - { - "block": 4940064, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "how-to-survive-as-a-student", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:24", - "trx_id": "0be7622f8865a3e2ad0418d971538f2bc5c0f7eb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22369, - { - "block": 4940066, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-lsd-spirits", - "rshares": 304903995, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:30", - "trx_id": "4fa3108991be26a460f47632e63448ad80befcf2", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 22370, - { - "block": 4940066, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "my-lsd-spirits", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:30", - "trx_id": "4fa3108991be26a460f47632e63448ad80befcf2", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 22371, - { - "block": 4940599, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "431305890", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "641", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "vesting_payout": { - "amount": "2906758714", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 11 - } - ], - [ - 22372, - { - "block": 4940599, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 1914, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "95", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "1377", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "total_payout_value": { - "amount": "1282", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 12 - } - ], - [ - 22373, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "goldmatters", - "comment_permlink": "re-camilla-apple-and-leaves-20160912t171320575z", - "curator": "camilla", - "reward": { - "amount": "27336288", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 13 - } - ], - [ - 22374, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "ashwim", - "comment_permlink": "re-camilla-apple-and-leaves-20160912t171828553z", - "curator": "camilla", - "reward": { - "amount": "18224192", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 22375, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "acidyo", - "comment_permlink": "re-camilla-apple-and-leaves-20160912t172139964z", - "curator": "camilla", - "reward": { - "amount": "45560481", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 19 - } - ], - [ - 22376, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "aavkc", - "comment_permlink": "re-camilla-apple-and-leaves-20160912t172202293z", - "curator": "camilla", - "reward": { - "amount": "9112096", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 22 - } - ], - [ - 22377, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "pixielolz", - "comment_permlink": "re-camilla-apple-and-leaves-20160912t182902111z", - "curator": "camilla", - "reward": { - "amount": "6074730", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 25 - } - ], - [ - 22378, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "shortcut", - "comment_permlink": "re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190120534z", - "curator": "camilla", - "reward": { - "amount": "12149461", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 30 - } - ], - [ - 22379, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "shortcut", - "comment_permlink": "re-camilla-re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t192137286z", - "curator": "camilla", - "reward": { - "amount": "18224192", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 33 - } - ], - [ - 22380, - { - "block": 4941317, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "zivile", - "comment_permlink": "pumpkin-pie-with-meat", - "curator": "camilla", - "reward": { - "amount": "54665193", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T18:33:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 6 - } - ], - [ - 22381, - { - "block": 4942131, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "helle", - "pending_payout": { - "amount": "53", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160913t172833838z", - "rshares": "215935794309", - "total_vote_weight": "944822817118870055", - "voter": "camilla", - "weight": "944822817118870055" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T19:14:00", - "trx_id": "8b9abebba5b4d9813bf6237b158d12a06a17aa9f", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 22382, - { - "block": 4942131, - "op": { - "type": "vote_operation", - "value": { - "author": "helle", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160913t172833838z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T19:14:00", - "trx_id": "8b9abebba5b4d9813bf6237b158d12a06a17aa9f", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 22383, - { - "block": 4942425, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "curie", - "comment_permlink": "project-curie-s-daily-curation-list-11-sept-12-sept-2016", - "curator": "camilla", - "reward": { - "amount": "60726492", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T19:28:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 46 - } - ], - [ - 22384, - { - "block": 4945441, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "acidyo", - "comment_permlink": "sketch-kitty-cat-oc", - "curator": "camilla", - "reward": { - "amount": "324702661", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T22:00:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 19 - } - ], - [ - 22385, - { - "block": 4945441, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "acidyo", - "comment_permlink": "re-camilla-re-acidyo-sketch-kitty-cat-oc-20160912t173408484z", - "curator": "camilla", - "reward": { - "amount": "21242230", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T22:00:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 98 - } - ], - [ - 22386, - { - "block": 4947246, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "248753346", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "370", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "vesting_payout": { - "amount": "1689702606", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T23:30:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 12 - } - ], - [ - 22387, - { - "block": 4947246, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 1113, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "54", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "795", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "total_payout_value": { - "amount": "740", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T23:30:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 13 - } - ], - [ - 22388, - { - "block": 4947246, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "karenb54", - "comment_permlink": "re-camilla-girl-with-a-pink-bow-20160912t225246008z", - "curator": "camilla", - "reward": { - "amount": "57637970", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T23:30:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 19 - } - ], - [ - 22389, - { - "block": 4947246, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "sompitonov", - "comment_permlink": "re-camilla-girl-with-a-pink-bow-20160913t055737074z", - "curator": "camilla", - "reward": { - "amount": "60671547", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T23:30:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 22 - } - ], - [ - 22390, - { - "block": 4947620, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "anwenbaumeister", - "comment_permlink": "what-is-culture-a-look-into-various-explanations-of-culture-and-forming-my-own-definition", - "curator": "camilla", - "reward": { - "amount": "500505034", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T23:49:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 37 - } - ], - [ - 22391, - { - "block": 4949022, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "timsaid", - "comment_permlink": "life-explorers-the-human-senses-part-iii-touch", - "curator": "camilla", - "reward": { - "amount": "227442475", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T00:59:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 42 - } - ], - [ - 22392, - { - "block": 4949759, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "dollarvigilante", - "comment_permlink": "boom-end-game-nears-as-central-banks-buying-up-gold-mining-companies", - "curator": "camilla", - "reward": { - "amount": "200121755", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T01:36:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 53 - } - ], - [ - 22393, - { - "block": 4952412, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "291", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-first-psychology-lecture", - "rshares": "6533369417", - "total_vote_weight": 0, - "voter": "acidyo", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:30", - "trx_id": "b295e5472bb8113674835dd14d9dffdaecabfd06", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22394, - { - "block": 4952412, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "my-first-psychology-lecture", - "voter": "acidyo", - "weight": 2100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:30", - "trx_id": "b295e5472bb8113674835dd14d9dffdaecabfd06", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22395, - { - "block": 4952416, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "292", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-first-psychology-lecture", - "rshares": 1911037334, - "total_vote_weight": 0, - "voter": "getssidetracked", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:42", - "trx_id": "cd98d8040dea7d3f3eecfa12858c2d2b1fb72e81", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22396, - { - "block": 4952416, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "my-first-psychology-lecture", - "voter": "getssidetracked", - "weight": 2100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:42", - "trx_id": "cd98d8040dea7d3f3eecfa12858c2d2b1fb72e81", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22397, - { - "block": 4952416, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "297", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-first-psychology-lecture", - "rshares": "15621109400", - "total_vote_weight": 0, - "voter": "venuspcs", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:42", - "trx_id": "e2dabc4a3875787c4da0616cb245b193d8a6701b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22398, - { - "block": 4952416, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "my-first-psychology-lecture", - "voter": "venuspcs", - "weight": 2100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:42", - "trx_id": "e2dabc4a3875787c4da0616cb245b193d8a6701b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22399, - { - "block": 4957270, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "yangyang", - "comment_permlink": "my-happy-whale", - "curator": "camilla", - "reward": { - "amount": "57529742", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T07:53:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 22400, - { - "block": 4957832, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "159", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "re-laivi-this-is-how-coloured-hats-make-norwegians-flirt-in-the-mountains-20160814t224155942z", - "vesting_payout": { - "amount": "735697523", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T08:21:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 22401, - { - "block": 4957832, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 485, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "319", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-laivi-this-is-how-coloured-hats-make-norwegians-flirt-in-the-mountains-20160814t224155942z", - "total_payout_value": { - "amount": "318", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T08:21:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 15 - } - ], - [ - 22402, - { - "block": 4957832, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-laivi-this-is-how-coloured-hats-make-norwegians-flirt-in-the-mountains-20160814t224155942z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T08:21:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 22403, - { - "block": 4958047, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "elfkitchen", - "comment_permlink": "i-want-to-take-you-into-the-chinese-vegetable-market", - "curator": "camilla", - "reward": { - "amount": "63576246", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T08:32:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 22404, - { - "block": 4958215, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "aldentan", - "comment_permlink": "from-weak-ass-bitch-to-ripped-my-fight-with-hyperthyroidism", - "curator": "camilla", - "reward": { - "amount": "39355477", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T08:40:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 22405, - { - "block": 4959301, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "hisnameisolllie", - "comment_permlink": "the-last-12-weeks-at-the-university-of-steemit-where-has-the-time-gone", - "curator": "camilla", - "reward": { - "amount": "108962269", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T09:35:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 31 - } - ], - [ - 22406, - { - "block": 4959683, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "smailer", - "comment_permlink": "my-daytime-latte-art-tryings-crown", - "curator": "camilla", - "reward": { - "amount": "93821903", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T09:54:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 13 - } - ], - [ - 22407, - { - "block": 4968236, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "7508323572", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:02:51", - "trx_id": "e6c65bbd66154b95b32bd25b4554d5f27a8b65cb", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22408, - { - "block": 4968236, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:02:51", - "trx_id": "e6c65bbd66154b95b32bd25b4554d5f27a8b65cb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22409, - { - "block": 4968238, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "7508323572", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:02:57", - "trx_id": "958963c3f1e30d05cb3ef7001226df54910ac989", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22410, - { - "block": 4968238, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:02:57", - "trx_id": "958963c3f1e30d05cb3ef7001226df54910ac989", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22411, - { - "block": 4968239, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "do-you-like-to-colour", - "rshares": "7333711396", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:00", - "trx_id": "38d2503bac59c7b7d72ba51b2b65b9b79c55d9a9", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22412, - { - "block": 4968239, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "do-you-like-to-colour", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:00", - "trx_id": "38d2503bac59c7b7d72ba51b2b65b9b79c55d9a9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22413, - { - "block": 4968242, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "zendoodle-drawing", - "rshares": "7333711396", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:09", - "trx_id": "319e23d39d4f0e0103b3fa7de096dbab69e8cc9a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22414, - { - "block": 4968242, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "zendoodle-drawing", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:09", - "trx_id": "319e23d39d4f0e0103b3fa7de096dbab69e8cc9a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22415, - { - "block": 4968253, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "how-to-survive-as-a-student", - "rshares": "7333711396", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:42", - "trx_id": "07001e21fa3ee51d19c706b48867de377ece8cb8", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22416, - { - "block": 4968253, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "how-to-survive-as-a-student", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:42", - "trx_id": "07001e21fa3ee51d19c706b48867de377ece8cb8", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22417, - { - "block": 4991895, - "op": { - "type": "comment_operation", - "value": { - "author": "beanz", - "body": "Hi! You've been featured in my post.\n[Petition to take down EARNEST](https://steemit.com/steemit-abuse/@beanz/petition-to-take-down-earnest-fao-dan-and-ned)", - "json_metadata": "{\"tags\":[\"art\"],\"links\":[\"https://steemit.com/steemit-abuse/@beanz/petition-to-take-down-earnest-fao-dan-and-ned\"]}", - "parent_author": "camilla", - "parent_permlink": "girl-with-a-pink-bow", - "permlink": "re-camilla-girl-with-a-pink-bow-20160915t125435394z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:54:36", - "trx_id": "dd0ebcb2019cff741fdd544c1aa7ac784946b8dd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22418, - { - "block": 4991898, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "5942642822", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:54:45", - "trx_id": "62b8c07b00e8410bae8c324d4c5309737bf3f22b", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 22419, - { - "block": 4991898, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:54:45", - "trx_id": "62b8c07b00e8410bae8c324d4c5309737bf3f22b", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 22420, - { - "block": 4997949, - "op": { - "type": "comment_operation", - "value": { - "author": "linkback-bot-v0", - "body": "<div> <p> This post has been linked to from another place on Steem. </p> <ul> <li> <a href=\"https://steemit.com/steemit-abuse/@beanz/petition-to-take-down-earnest-fao-dan-and-ned\"> Petition to End steemit harassment FAO @dan & @ned </a> by <a href=\"https://steemit.com/@beanz\"> @beanz </a> </li> <li> <a href=\"https://steemit.com/life/@shadowspub/sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th\"> Sunday Ramble Through Steemit -- Notes on My Favourite Reads Sept 11th </a> by <a href=\"https://steemit.com/@shadowspub\"> @shadowspub </a> </li> </ul> <p> Learn more about <a href=\"https://steemit.com/steem/@ontofractal/steem-linkback-bot-v0-3-released\"> linkback bot v0.3</a> </p> <p>Upvote if you want the bot to continue posting linkbacks for your posts. Flag if otherwise. Built by @ontofractal</p></div>", - "json_metadata": "{}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-linkbacks", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:58:45", - "trx_id": "64e216922cbc97d029f3e6db16f64fb0a0199039", - "trx_in_block": 1, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/default_val.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/default_val.tavern.yaml deleted file mode 100644 index ed13baa012fa64eb05567b4a4372d6c02f66aa2e..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/default_val.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history default values - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history default values - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"camilla","start":-1,"limit":1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "default_val" - directory: "account_history_api_patterns/get_account_history" - ignore_tags: ['history'] \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/delayed_voting_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/delayed_voting_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/delayed_voting_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/delayed_voting_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/delayed_voting_operation.tavern.yaml deleted file mode 100644 index ef78e59e7b96e0e5c636e051009d476c7302945a..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/delayed_voting_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api delayed_voting_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api delayed_voting_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_high": 16, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "delayed_voting_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/delegate_vesting_shares_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/delegate_vesting_shares_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/delegate_vesting_shares_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/delegate_vesting_shares_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/delegate_vesting_shares_operation.tavern.yaml deleted file mode 100644 index d55a071d8ec1d19199fc4822e43e68387334c090..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/delegate_vesting_shares_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api delegate_vesting_shares_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api delegate_vesting_shares_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 1099511627776, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "delegate_vesting_shares_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/delete_comment_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/delete_comment_operation.pat.json deleted file mode 100644 index b8ae44791861c1e155ef0d7b8586f04eaa00e856..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/delete_comment_operation.pat.json +++ /dev/null @@ -1,118 +0,0 @@ -{ - "history": [ - [ - 2722, - { - "block": 3374957, - "op": { - "type": "delete_comment_operation", - "value": { - "author": "jsc", - "permlink": "test" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-21T01:22:39", - "trx_id": "b67f5a7096a2932524f1d29e59711f7d39245661", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 2947, - { - "block": 3510528, - "op": { - "type": "delete_comment_operation", - "value": { - "author": "jsc", - "permlink": "title" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-25T19:36:45", - "trx_id": "5d184d3cabb12c23bcc662224a5e8a3388ddea2c", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 2994, - { - "block": 3592106, - "op": { - "type": "delete_comment_operation", - "value": { - "author": "jsc", - "permlink": "test" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-28T16:12:36", - "trx_id": "1806681f3c6e06c132f91fa1c95cef09033ec215", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 3164, - { - "block": 3683400, - "op": { - "type": "delete_comment_operation", - "value": { - "author": "jsc", - "permlink": "re-jsc-re-lauralemons-we-need-a-mute-feature-to-protect-women-from-stalkers-i-know-because-i-have-one-20160731t195809034z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-31T20:46:21", - "trx_id": "a816a9686f0a546704a8b4e5008c6ef39105dc4f", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 3203, - { - "block": 3743169, - "op": { - "type": "delete_comment_operation", - "value": { - "author": "jsc", - "permlink": "re-stephenking989-thank-you-steemit-my-skepticism-is-now-gone-20160802t223818289z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-02T22:46:06", - "trx_id": "edd46e6e1f0ce026e994ee8c81e487aa7f0c5fdc", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 3556, - { - "block": 4428887, - "op": { - "type": "delete_comment_operation", - "value": { - "author": "jsc", - "permlink": "test-utf-8-chars-in-a-link" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-26T22:04:21", - "trx_id": "e00573f09fbb522e1f0e14f26bced5acc9a201eb", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/delete_comment_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/delete_comment_operation.tavern.yaml deleted file mode 100644 index fc08298e6040599a48d7d63caa68d009ac66de51..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/delete_comment_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to delete_comment_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history delete_comment_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"jsc","operation_filter_low": 131072,"start": 3556,"limit": 6} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "delete_comment_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/effective_comment_vote_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/effective_comment_vote_operation.pat.json deleted file mode 100644 index 5dc970356370c6d7330bf5101987c5f100e3b86c..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/effective_comment_vote_operation.pat.json +++ /dev/null @@ -1,984 +0,0 @@ -{ - "history": [ - [ - 2041, - { - "block": 1265961, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "5934063", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-part-1-capital-gainslosses-for-crypto", - "rshares": "101251038008", - "total_vote_weight": "35765176822296", - "voter": "jamie", - "weight": 231110 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T10:36:45", - "trx_id": "2558930eb829e336a218334d2051ebd030e16821", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2049, - { - "block": 1266083, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "5960135", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": 82385000, - "total_vote_weight": "36191989068740", - "voter": "officialinfotech", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T10:42:51", - "trx_id": "e1681450d6219fa2aebb583de37d33b6a56b6dd1", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2054, - { - "block": 1266137, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "5960404", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": 85935000, - "total_vote_weight": "36191989068740", - "voter": "officialfinance", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T10:45:36", - "trx_id": "40e3a599b3c1be72c5830d10ccb041ad00565edf", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2057, - { - "block": 1266149, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "5960472", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": 66163433, - "total_vote_weight": "36191989068740", - "voter": "infowars.com", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T10:46:12", - "trx_id": "092bb0d84866bf54ece397ff4dc36b12717cc02b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2062, - { - "block": 1266212, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "5960780", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": 65582386, - "total_vote_weight": "36191989068740", - "voter": "alexjones", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T10:49:21", - "trx_id": "ae2476009f43ac2b3be638fbfc3cf99447730d53", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2065, - { - "block": 1266221, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "5960836", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": 81042100, - "total_vote_weight": "36191989068740", - "voter": "officialfuzzy", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T10:49:48", - "trx_id": "19d0fa04d702d4fd5cd5cbb9782e9fee82a5218c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2067, - { - "block": 1266232, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "6997209", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": "5802465695828", - "total_vote_weight": "36228680528856", - "voter": "fuzzyvest", - "weight": "36691460116" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T10:50:21", - "trx_id": "2a7a8cd61b956611f1e385ea31b3b53515ba8089", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2176, - { - "block": 1268489, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "16855591", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "openledger-pre-sale-of-dao-tokens-is-now-live", - "rshares": "19583245200", - "total_vote_weight": "39531087086040", - "voter": "cass", - "weight": 575 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T12:43:21", - "trx_id": "c947f9e6a5faa8b38fbf5526e55bf8d8cc2f6732", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2263, - { - "block": 1270270, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "7005375", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": 523009760, - "total_vote_weight": "36228680528856", - "voter": "bobsunday", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T14:12:36", - "trx_id": "72052d5d69d91d073d2644237c9f8c7ceeed20ae", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2265, - { - "block": 1270271, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "5936597", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-part-1-capital-gainslosses-for-crypto", - "rshares": 511123174, - "total_vote_weight": "35765176822296", - "voter": "bobsunday", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T14:12:39", - "trx_id": "a958555bf28f0c9c2a4bb2179b1f87fd5ace8a8d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2379, - { - "block": 1272592, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "24353743", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "-blocktrades-adds-support-for-directly-buyingselling-steem", - "rshares": 674378022, - "total_vote_weight": "37008940034691", - "voter": "jazzycrypt", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T16:09:00", - "trx_id": "c084bca2026aab71c0c2c2ed304bf14cc846e79a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 2382, - { - "block": 1272628, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "6769917", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "is-vote-changing-an-important-feature-for-steem", - "rshares": 608861538, - "total_vote_weight": "39484775670330", - "voter": "anonimau5", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T16:10:48", - "trx_id": "e5556e52bb9a0ecebbcdb08743767a6d84c4f9ed", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2396, - { - "block": 1272856, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "24362756", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "-blocktrades-adds-support-for-directly-buyingselling-steem", - "rshares": "11752974784", - "total_vote_weight": "37008940034721", - "voter": "jpb3", - "weight": 30 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T16:22:15", - "trx_id": "f9248f71a416abb99af675cf7bd3181fedcb0a42", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2475, - { - "block": 1274459, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "13419166", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": "29885796722307", - "total_vote_weight": "38751835134587", - "voter": "berniesanders", - "weight": "2523154605731" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T17:42:33", - "trx_id": "2b7cb091c3dce8f73b61af83a582684d2d4ba1b8", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2477, - { - "block": 1274460, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "11872749", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-part-1-capital-gainslosses-for-crypto", - "rshares": "29885796722307", - "total_vote_weight": "38607747369088", - "voter": "berniesanders", - "weight": "2842570546792" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T17:42:36", - "trx_id": "4271a3284b005f4f93721da73dca5b32c2778550", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2530, - { - "block": 1275555, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "23403510", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "-blocktrades-adds-support-for-directly-buyingselling-steem", - "rshares": 108985000, - "total_vote_weight": "37008940034721", - "voter": "chocolate", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T18:37:30", - "trx_id": "615718c1c45b9701708caf7c306f4ef2e002f2f4", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2533, - { - "block": 1275560, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "16125879", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "openledger-pre-sale-of-dao-tokens-is-now-live", - "rshares": 104625600, - "total_vote_weight": "39531087086040", - "voter": "chocolate", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T18:37:45", - "trx_id": "f5d9bc6c0860971082a3117d9364fc615f166708", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 2535, - { - "block": 1275565, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "13117371", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": 100266200, - "total_vote_weight": "38751835134587", - "voter": "chocolate", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T18:38:00", - "trx_id": "2323c79ccb3a6528e2b454a0606c41e208cc2cae", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 2537, - { - "block": 1275568, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "11670182", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-part-1-capital-gainslosses-for-crypto", - "rshares": 98086500, - "total_vote_weight": "38607747369088", - "voter": "chocolate", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T18:38:09", - "trx_id": "325116386afee87e71931b54eba78aa1bef34403", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 2641, - { - "block": 1277711, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "13128142", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": 672908871, - "total_vote_weight": "38751835134587", - "voter": "jbradford", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T20:25:36", - "trx_id": "e0875ec0350c2a7ef1438c928bcf53078dbc2973", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2643, - { - "block": 1277712, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "11679756", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-part-1-capital-gainslosses-for-crypto", - "rshares": 672908871, - "total_vote_weight": "38607747369088", - "voter": "jbradford", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-08T20:25:39", - "trx_id": "d79bd7c3e419d05733dcb93ed268a60cd9091a24", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2858, - { - "block": 1282083, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "149", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "confirmation-of-accounts-i-control-on-bitcointalkbitsharestalk", - "rshares": "31560555001", - "total_vote_weight": "31560555001", - "voter": "pfunk", - "weight": "31560555001" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T00:04:39", - "trx_id": "48da7244c34b03ae70815ee05a5500e310ee1646", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 2922, - { - "block": 1283361, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "151", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "confirmation-of-accounts-i-control-on-bitcointalkbitsharestalk", - "rshares": 564236662, - "total_vote_weight": "31560729062", - "voter": "predator", - "weight": 174061 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T01:08:39", - "trx_id": "91ffb2551bcde991681d7d594f16f062658c977b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3098, - { - "block": 1286939, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "13109255", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": "2408772048948", - "total_vote_weight": "38753096448056", - "voter": "nextgencrypto", - "weight": 1261313469 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T04:08:12", - "trx_id": "bc329d9e8bba39bef3c84030cf42f9e78431730c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3389, - { - "block": 1292969, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "25456142", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "-blocktrades-adds-support-for-directly-buyingselling-steem", - "rshares": "9901240448969", - "total_vote_weight": "37025571774787", - "voter": "blackjack", - "weight": "16631740066" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T09:10:21", - "trx_id": "20323bd68eec46d388c51e197bfe97aeb8193383", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3503, - { - "block": 1295318, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "15385322", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "openledger-pre-sale-of-dao-tokens-is-now-live", - "rshares": "282881642383", - "total_vote_weight": "39531088811759", - "voter": "liondani", - "weight": 1725719 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T11:08:12", - "trx_id": "50feaa113762f41edb11bb398cdb8470ab4e850b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3516, - { - "block": 1295551, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "159", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "confirmation-of-accounts-i-control-on-bitcointalkbitsharestalk", - "rshares": 2712531910, - "total_vote_weight": "31577174104", - "voter": "aaaaa", - "weight": 16445042 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T11:19:51", - "trx_id": "086e466f9d8f834da2c8a0c1e2c1f3fef80e4f0a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3546, - { - "block": 1296139, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "tuck-fheman", - "pending_payout": { - "amount": "1868989", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "bitshares-dev-hangout-transcript--may-6th-2016", - "rshares": "35082265948601", - "total_vote_weight": "30529437689766", - "voter": "blocktrades", - "weight": "28847679693161" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T11:49:24", - "trx_id": "f6b40bc59cf82cedda94f9249b37b008987924d3", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3679, - { - "block": 1298876, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "bavak", - "pending_payout": { - "amount": "1667673", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "campaign-myths-regarding-the-economy-by-greg-mankiw", - "rshares": "35090183111946", - "total_vote_weight": "33848255033113", - "voter": "blocktrades", - "weight": "32446962004213" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T14:06:45", - "trx_id": "d2254506426386de9a034e78a669bd51f2861e1d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3718, - { - "block": 1299641, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "12989014", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": 641550645, - "total_vote_weight": "38753096448056", - "voter": "mldorton", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T14:45:12", - "trx_id": "ebe50cef0d8e17ddf5e516f2773370aeb8ccdab3", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3760, - { - "block": 1300461, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "15669107", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "openledger-pre-sale-of-dao-tokens-is-now-live", - "rshares": "1418163165834", - "total_vote_weight": "39531300962875", - "voter": "au1nethyb1", - "weight": 212151116 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T15:26:18", - "trx_id": "e3831ef2961db4311989ca521cbfde6d4153d743", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3770, - { - "block": 1300627, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "blocktrades", - "pending_payout": { - "amount": "12960273", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "rshares": "9563415137", - "total_vote_weight": "38753096448134", - "voter": "proctologic", - "weight": 78 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T15:34:42", - "trx_id": "147fc624d5d8671a22d3d8ce8f5ca4bdda59c06c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3899, - { - "block": 1303283, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "tuck-fheman", - "pending_payout": { - "amount": "1576764", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "steem-now-on-coingecko", - "rshares": "35102886402201", - "total_vote_weight": "33883771029211", - "voter": "blocktrades", - "weight": "33197653354719" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T17:48:06", - "trx_id": "917ea199d933a88622de2fc2b06331de781f8004", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3907, - { - "block": 1303382, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "jupiter00000", - "pending_payout": { - "amount": "1440800", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "new-social-media-platform-which-pays-you-to-post", - "rshares": "34414874547441", - "total_vote_weight": "34374221471221", - "voter": "blocktrades", - "weight": "34355032051051" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T17:53:09", - "trx_id": "d70a20eb4d7e6d4d8c8ec60436833f30e2b83680", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3930, - { - "block": 1303768, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "lafona", - "pending_payout": { - "amount": "1441613", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "coindesk-article-is-blockchain-the-key-to-user-controlled-social-media", - "rshares": "34415994487184", - "total_vote_weight": "34312874909990", - "voter": "blocktrades", - "weight": "34244154701321" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-09T18:12:30", - "trx_id": "55cd5b1ca9cbe29c550c0c7c1186136a71d29413", - "trx_in_block": 0, - "virtual_op": 1 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/effective_comment_vote_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/effective_comment_vote_operation.tavern.yaml deleted file mode 100644 index 3a8e019fc84560cd9696697463e47b325f41566f..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/effective_comment_vote_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api effective_comment_vote_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api effective_comment_vote_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_high": 64, "start": 3976, "limit": 50} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "effective_comment_vote_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/escrow_approve_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/escrow_approve_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/escrow_approve_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/escrow_approve_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/escrow_approve_operation.tavern.yaml deleted file mode 100644 index 7a045c834b01f84334952d75e95ac2fd845ef72e..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/escrow_approve_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api escrow_approve_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api escrow_approve_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 2147483648, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "escrow_approve_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/escrow_dispute_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/escrow_dispute_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/escrow_dispute_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/escrow_dispute_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/escrow_dispute_operation.tavern.yaml deleted file mode 100644 index 33e307fdf22e44b77f4d14ce72c51ba4f41daad2..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/escrow_dispute_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api escrow_dispute_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api escrow_dispute_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"barrie", "operation_filter_low": 268435456, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "escrow_transfer_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/escrow_release_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/escrow_release_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/escrow_release_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/escrow_release_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/escrow_release_operation.tavern.yaml deleted file mode 100644 index b5580c3d3c41075972e7e1cf304b2c284b0c65e3..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/escrow_release_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api escrow_release_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api escrow_release_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"barrie", "operation_filter_low": 536870912, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "escrow_release_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/escrow_transfer_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/escrow_transfer_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/escrow_transfer_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/escrow_transfer_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/escrow_transfer_operation.tavern.yaml deleted file mode 100644 index 3d7ffcd69e7e25fe3a3f4418c9e6ece79e29a7c4..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/escrow_transfer_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api escrow_transfer_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api escrow_transfer_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"barrie", "operation_filter_low": 134217728, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "escrow_transfer_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/feed_publish_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/feed_publish_operation.pat.json deleted file mode 100644 index 89521271118891f2e715fc3e81240351db3e4198..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/feed_publish_operation.pat.json +++ /dev/null @@ -1,94 +0,0 @@ -{ - "history": [ - [ - 5579, - { - "block": 1337175, - "op": { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "237", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-10T22:06:36", - "trx_id": "2cccd6b21a642bba0d56c6c133874c808df26e17", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 5639, - { - "block": 1338414, - "op": { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "246", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-10T23:08:39", - "trx_id": "9414f7c21a3ce6e3c069b59465396ebf7f615363", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 5898, - { - "block": 1343510, - "op": { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "238", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-11T03:23:48", - "trx_id": "e89a2efb797e7ec35892096ba61f9b4aa4f5ff9e", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/feed_publish_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/feed_publish_operation.tavern.yaml deleted file mode 100644 index d0747dc4b6b52656393af53e3a4eedf1e77f4c0d..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/feed_publish_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to feed_publish_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history feed_publish_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades","operation_filter_low": 128,"start": 6000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "feed_publish_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/fill_convert_request_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/fill_convert_request_operation.pat.json deleted file mode 100644 index 9f48d6340ff4b5e7cb964de8d9c68b74613da014..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/fill_convert_request_operation.pat.json +++ /dev/null @@ -1,33 +0,0 @@ -{ - "history": [ - [ - 15169, - { - "block": 3327426, - "op": { - "type": "fill_convert_request_operation", - "value": { - "amount_in": { - "amount": "2000000", - "nai": "@@000000013", - "precision": 3 - }, - "amount_out": { - "amount": "605143", - "nai": "@@000000021", - "precision": 3 - }, - "owner": "xeroc", - "requestid": 1468315395 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-19T09:23:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/fill_convert_request_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/fill_convert_request_operation.tavern.yaml deleted file mode 100644 index 3ffe3844f3507bc0d62e9aa8865cef752a59804a..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/fill_convert_request_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api fill_convert_request_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api fill_convert_request_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"xeroc", "operation_filter_low": 281474976710656, "start": 15169, "limit": 1} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "fill_convert_request_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/fill_order_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/fill_order_operation.pat.json deleted file mode 100644 index 9b915bd71e3daa70e69c6f74bbe0131e488c751d..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/fill_order_operation.pat.json +++ /dev/null @@ -1,3104 +0,0 @@ -{ - "history": [ - [ - 3660, - { - "block": 2890526, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 10001, - "current_owner": "adm", - "current_pays": { - "amount": "1241", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 1467595909, - "open_owner": "nextgencrypto", - "open_pays": { - "amount": "297", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:32:06", - "trx_id": "377b7f29708e614281309626b2295ced3160e949", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3663, - { - "block": 2890527, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 10001, - "current_owner": "adm", - "current_pays": { - "amount": "1126", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 1467595909, - "open_owner": "nextgencrypto", - "open_pays": { - "amount": "270", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:32:09", - "trx_id": "2ce893d4c768155bd21080dd6319ffc87175e3f3", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3674, - { - "block": 2890536, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 1467595954, - "current_owner": "nextgencrypto", - "current_pays": { - "amount": "250", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "1022", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:32:36", - "trx_id": "a5096ec6e5663ca49d0aaffd058837275b68f57f", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3694, - { - "block": 2890822, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 10001, - "current_owner": "adm", - "current_pays": { - "amount": "49770", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 1467596727, - "open_owner": "steemychicken1", - "open_pays": { - "amount": "13438", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:47:54", - "trx_id": "521593e00054683c0813ddb3f3765fd40991196f", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3697, - { - "block": 2890822, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 1467596869, - "current_owner": "dedriss", - "current_pays": { - "amount": "121557", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "450230", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:47:54", - "trx_id": "3c43466c565077ee3f86dd7ca5fed229c244b68d", - "trx_in_block": 3, - "virtual_op": 2 - } - ], - [ - 3698, - { - "block": 2890823, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 10001, - "current_owner": "adm", - "current_pays": { - "amount": "69140", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 1467596869, - "open_owner": "dedriss", - "open_pays": { - "amount": "18668", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:47:57", - "trx_id": "4dde6882a987ee3f87e0ba837fb1ffcbfaed706c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3703, - { - "block": 2890842, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50310, - "current_owner": "abit", - "current_pays": { - "amount": "366685", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "84341", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:48:54", - "trx_id": "76e65f73f0dc958b24d8ace2da66abad2c019dca", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3705, - { - "block": 2890844, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50313, - "current_owner": "abit", - "current_pays": { - "amount": "68079", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "15659", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:49:00", - "trx_id": "2e23976be4c51d0d80efc5e536f45403f0c1b05a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3710, - { - "block": 2890852, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 49316, - "current_owner": "abit", - "current_pays": { - "amount": "60022", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "230863", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:49:24", - "trx_id": "7b950a4e23c1c84811d0f49acfca83c7e37b4fe7", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3715, - { - "block": 2890864, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50376, - "current_owner": "abit", - "current_pays": { - "amount": "425650", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "97903", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:50:00", - "trx_id": "27a2ec441f42fe7ce85ebf3e508dea8ca928aa98", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3717, - { - "block": 2890865, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50379, - "current_owner": "abit", - "current_pays": { - "amount": "9116", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "2097", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:50:03", - "trx_id": "97aa6f882ea2503cc6d9b76f7404f0f4599981dd", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3722, - { - "block": 2890873, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 49379, - "current_owner": "abit", - "current_pays": { - "amount": "12352", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "47511", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:50:27", - "trx_id": "5fb981d125303971effe47f47554625783b0790f", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3724, - { - "block": 2890885, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50442, - "current_owner": "abit", - "current_pays": { - "amount": "434763", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "100000", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:51:06", - "trx_id": "5c82ac346ae6993e2cdeda581420ff4df0b30182", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3729, - { - "block": 2890893, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 49442, - "current_owner": "abit", - "current_pays": { - "amount": "44692", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "171900", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:51:30", - "trx_id": "1a443e79118dfdb2686220d57ffac8dea1da0fa1", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3732, - { - "block": 2890905, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50505, - "current_owner": "abit", - "current_pays": { - "amount": "434763", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "100000", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:52:09", - "trx_id": "0e1e779bf78de5b8688c0b43f29e4e1b6228f335", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3737, - { - "block": 2890913, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 49505, - "current_owner": "abit", - "current_pays": { - "amount": "31022", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "119322", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:52:33", - "trx_id": "8918bbd5381084121f9d1e3da7e1c6e79ed92e44", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3739, - { - "block": 2890926, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50568, - "current_owner": "abit", - "current_pays": { - "amount": "434763", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "100000", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:53:12", - "trx_id": "83b1f43c916f7a6e840b27247e22a28637d2faa9", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3744, - { - "block": 2890934, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 49568, - "current_owner": "abit", - "current_pays": { - "amount": "44691", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "171896", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:53:36", - "trx_id": "c3f52aaf072f1a081fbca7f9158a6094a4859ce8", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3747, - { - "block": 2890937, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 1467597205, - "current_owner": "tadakaluri", - "current_pays": { - "amount": "19845", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "76331", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:53:45", - "trx_id": "0f3e5430b3c0761cc4bce50a310d18b8740b739f", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3749, - { - "block": 2890947, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50631, - "current_owner": "abit", - "current_pays": { - "amount": "412774", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "94942", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:54:15", - "trx_id": "0bf11d999add4d69f178ef7103fd974f1558c63e", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3751, - { - "block": 2890948, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50634, - "current_owner": "abit", - "current_pays": { - "amount": "21990", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "5058", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:54:18", - "trx_id": "383fba746df6c2ae07775eec28c0463a24d25867", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3756, - { - "block": 2890952, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 1467596919, - "current_owner": "piedpiper", - "current_pays": { - "amount": "260", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:54:30", - "trx_id": "166171d8e1bffb009deb7433b6ec73c56f4372a0", - "trx_in_block": 0, - "virtual_op": 2 - } - ], - [ - 3757, - { - "block": 2890954, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 1467597276, - "current_owner": "vasco-el-jim", - "current_pays": { - "amount": "10917", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "41991", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:54:36", - "trx_id": "0a443423deef6e1feb98e4a2f5230122ac499fe6", - "trx_in_block": 1, - "virtual_op": 2 - } - ], - [ - 3759, - { - "block": 2890969, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50697, - "current_owner": "abit", - "current_pays": { - "amount": "347710", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "79976", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:55:21", - "trx_id": "a9f7d37560142bdbd2bdf9b9d376388abbb27173", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3761, - { - "block": 2890970, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50700, - "current_owner": "abit", - "current_pays": { - "amount": "85260", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19610", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:55:24", - "trx_id": "5578000025183a8e51227136f2466e6a1d933cfe", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3763, - { - "block": 2890971, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50703, - "current_owner": "abit", - "current_pays": { - "amount": "1799", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "414", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:55:27", - "trx_id": "1b95321ba769cc5db4c4fa469c60f67582cebd19", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3768, - { - "block": 2890975, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 49691, - "current_owner": "abit", - "current_pays": { - "amount": "44238", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "163851", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:55:39", - "trx_id": "d112e39f204ac6d59db57b5ed777844fab7e1bdf", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3773, - { - "block": 2890992, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50766, - "current_owner": "abit", - "current_pays": { - "amount": "375202", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "86300", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:56:30", - "trx_id": "aeff4d443cc94881f993b77742d8b908571a42ea", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3776, - { - "block": 2890993, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50769, - "current_owner": "abit", - "current_pays": { - "amount": "59562", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "13700", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:56:33", - "trx_id": "fa65c8a1be0ea1c223e17c4e43bd909845cf3eb2", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 3781, - { - "block": 2890996, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 49754, - "current_owner": "abit", - "current_pays": { - "amount": "2286", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "8469", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:56:42", - "trx_id": "0449949066b9d165ce6bbbb358c2f162c9d1524e", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 3783, - { - "block": 2891014, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50832, - "current_owner": "abit", - "current_pays": { - "amount": "289950", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "66691", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:57:36", - "trx_id": "3dd172476c40c4c392968336971ac4717bd9b39b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3786, - { - "block": 2891016, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50838, - "current_owner": "abit", - "current_pays": { - "amount": "59559", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "13699", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:57:42", - "trx_id": "fb83e1096df24550bdf2334fa458a34bba07ff64", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3788, - { - "block": 2891017, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 49817, - "current_owner": "abit", - "current_pays": { - "amount": "59833", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "221614", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:57:45", - "trx_id": "145d22c3b65bbd1e9059bd0ccb2dbc19f60761d5", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3790, - { - "block": 2891019, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50847, - "current_owner": "abit", - "current_pays": { - "amount": "85257", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19610", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:57:51", - "trx_id": "353e8595f45d65574172478f92675dea59bef574", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3793, - { - "block": 2891039, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 49883, - "current_owner": "abit", - "current_pays": { - "amount": "25305", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "93726", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:58:51", - "trx_id": "05094c397c1c9177117a3a6f1767f7abe006d7e0", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3796, - { - "block": 2891040, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50910, - "current_owner": "abit", - "current_pays": { - "amount": "341047", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "78444", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:58:54", - "trx_id": "76f4ac6bf62799d8cb9bf63ef89cf69fd9989f3c", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 3798, - { - "block": 2891041, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50913, - "current_owner": "abit", - "current_pays": { - "amount": "85261", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19610", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:58:57", - "trx_id": "8a26d137d0de670a3d925f1409835e6fe06bef63", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3800, - { - "block": 2891042, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50916, - "current_owner": "abit", - "current_pays": { - "amount": "8460", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "1946", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:59:00", - "trx_id": "dbe663b4d2cbb5cbe2a80e48dcc64daf01d92b74", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3805, - { - "block": 2891060, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 49946, - "current_owner": "abit", - "current_pays": { - "amount": "34528", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "127887", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:59:54", - "trx_id": "a2fa65fa6dd8bfaef15a794913c495fae5f83295", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3809, - { - "block": 2891063, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50979, - "current_owner": "abit", - "current_pays": { - "amount": "371365", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "85417", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:00:03", - "trx_id": "6a3e34d37bfd95a5fffa2fcc467609394cf1e53a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3811, - { - "block": 2891065, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50982, - "current_owner": "abit", - "current_pays": { - "amount": "63401", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "14583", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:00:09", - "trx_id": "12d3af36e3c280e61980bfbc7746d40fb7a7ae4b", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3816, - { - "block": 2891080, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50009, - "current_owner": "abit", - "current_pays": { - "amount": "13794", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "51093", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:00:57", - "trx_id": "3f9715ed93a98a2aa4fc6354c638c8e1ef4b01f4", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3818, - { - "block": 2891084, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51048, - "current_owner": "abit", - "current_pays": { - "amount": "326172", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "75022", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:01:12", - "trx_id": "bde21844fac374770b50c38886302298c7e248d2", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3821, - { - "block": 2891085, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51051, - "current_owner": "abit", - "current_pays": { - "amount": "84620", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19463", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:01:15", - "trx_id": "e9b084d3c80c3e9855cd5bbf9c8d44581cdf7790", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 3823, - { - "block": 2891086, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51054, - "current_owner": "abit", - "current_pays": { - "amount": "23977", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "5515", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:01:18", - "trx_id": "115b3d8c5ecc51f9327338c781c2f4084c1ed53d", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3828, - { - "block": 2891100, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50072, - "current_owner": "abit", - "current_pays": { - "amount": "49015", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "181546", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:02:00", - "trx_id": "633f272f5b7f1cecda3598eef0f23df8207ad946", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3833, - { - "block": 2891107, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51117, - "current_owner": "abit", - "current_pays": { - "amount": "369119", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "84901", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:02:21", - "trx_id": "5c379d633c67d77fa89c8350916b327e6eef7592", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3835, - { - "block": 2891108, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51120, - "current_owner": "abit", - "current_pays": { - "amount": "65644", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "15099", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:02:24", - "trx_id": "2539722facb63b905bed786a9a65c8343dc99b74", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3840, - { - "block": 2891121, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50135, - "current_owner": "abit", - "current_pays": { - "amount": "3150", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "11669", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:03:03", - "trx_id": "6eb90c1e29ef425944473bb5f1749b1eabe5f45e", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3842, - { - "block": 2891128, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51183, - "current_owner": "abit", - "current_pays": { - "amount": "284505", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "65439", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:03:27", - "trx_id": "1b894831005a16102245e420f0f4b3a06ed1a873", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3844, - { - "block": 2891129, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51186, - "current_owner": "abit", - "current_pays": { - "amount": "84620", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19463", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:03:30", - "trx_id": "e1a52a9138dfbb24b49767379e88e36775a00867", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3846, - { - "block": 2891131, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51189, - "current_owner": "abit", - "current_pays": { - "amount": "65640", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "15098", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:03:36", - "trx_id": "c9204e25d751bce63f7c6ada5d9b5c9238d881ad", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3851, - { - "block": 2891141, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50198, - "current_owner": "abit", - "current_pays": { - "amount": "60265", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "223213", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:04:06", - "trx_id": "86d18ba71557ef57160292f77e9fe190af89e89c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3856, - { - "block": 2891150, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51252, - "current_owner": "abit", - "current_pays": { - "amount": "369123", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "84902", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:04:36", - "trx_id": "3d10823d3606dee7d866f1822139d0f76bd78a7f", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3859, - { - "block": 2891152, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51255, - "current_owner": "abit", - "current_pays": { - "amount": "65640", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "15098", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:04:42", - "trx_id": "25c89459290795f56ce70293207a40c428009140", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3864, - { - "block": 2891162, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50264, - "current_owner": "abit", - "current_pays": { - "amount": "3149", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "11665", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:05:12", - "trx_id": "1786b5216f06f41d9de02c2a4ebe041f624927e5", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3866, - { - "block": 2891172, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51318, - "current_owner": "abit", - "current_pays": { - "amount": "284505", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "65439", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:05:42", - "trx_id": "a478cf0b9aa09fafd8025e8fe1ed42299b40b2b6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3868, - { - "block": 2891173, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51321, - "current_owner": "abit", - "current_pays": { - "amount": "84620", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19463", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:05:45", - "trx_id": "28e872b3088c3b9cec48985a4e420a15e553968d", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3870, - { - "block": 2891174, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51327, - "current_owner": "abit", - "current_pays": { - "amount": "65640", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "15098", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:05:51", - "trx_id": "0c9a1bc5e2a52d03f5cc750bc4c32e04fe415cb6", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3875, - { - "block": 2891182, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50327, - "current_owner": "abit", - "current_pays": { - "amount": "60265", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "223213", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:06:15", - "trx_id": "ca437a41dfdd233a3dc8b64a9c398896ce1f1b51", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3880, - { - "block": 2891195, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51390, - "current_owner": "abit", - "current_pays": { - "amount": "369123", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "84902", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:06:54", - "trx_id": "330d76bdfdf24efa52aeaa76e1679d02ff2a3ea1", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3882, - { - "block": 2891196, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51393, - "current_owner": "abit", - "current_pays": { - "amount": "65640", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "15098", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:06:57", - "trx_id": "df6b468d2f7b4afb0bb12118adf180189a50b799", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3890, - { - "block": 2891203, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50390, - "current_owner": "abit", - "current_pays": { - "amount": "3149", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "11665", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:07:18", - "trx_id": "9f6c3c3446e1faf08bceb9547ad5b4fb00763dc2", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 3892, - { - "block": 2891223, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51474, - "current_owner": "abit", - "current_pays": { - "amount": "284505", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "67146", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:08:18", - "trx_id": "b3dcb1e4e9b05422762ec530b34590348ee99a10", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3894, - { - "block": 2891224, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50453, - "current_owner": "abit", - "current_pays": { - "amount": "60265", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "223213", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:08:21", - "trx_id": "3cb49b0a8dd66fdd5403bd32c8a3826901d01e04", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3895, - { - "block": 2891224, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51477, - "current_owner": "abit", - "current_pays": { - "amount": "84620", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19971", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:08:21", - "trx_id": "0e9de4cbb6b49c52786d5c0b937fcc8a432fa442", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 3897, - { - "block": 2891225, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51480, - "current_owner": "abit", - "current_pays": { - "amount": "54586", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "12883", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:08:24", - "trx_id": "4a4832caad2e2f06e07dbe15b0a5a55a31962b41", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3899, - { - "block": 2891244, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50516, - "current_owner": "abit", - "current_pays": { - "amount": "76813", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "284505", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:09:24", - "trx_id": "883cfe3dc124bbb5634b0a1c87224ed99c39a07c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3903, - { - "block": 2891246, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51546, - "current_owner": "abit", - "current_pays": { - "amount": "368512", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "86972", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:09:30", - "trx_id": "645f5499f1ed22ce621fd59fb522b3a0ecf6e4ec", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3905, - { - "block": 2891247, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51549, - "current_owner": "abit", - "current_pays": { - "amount": "55200", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "13028", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:09:33", - "trx_id": "4f0eb316d0b1c48adb36217c463148c9a83d5a8a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3910, - { - "block": 2891265, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50579, - "current_owner": "abit", - "current_pays": { - "amount": "14737", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "54586", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:10:27", - "trx_id": "6859653ac32ab0dc1e9b34e1c818cabd5f8473b5", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3912, - { - "block": 2891268, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51612, - "current_owner": "abit", - "current_pays": { - "amount": "337866", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "79739", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:10:36", - "trx_id": "9cdc4f67478507bd172c7d318637912c8a732375", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3914, - { - "block": 2891269, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51615, - "current_owner": "abit", - "current_pays": { - "amount": "84620", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19971", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:10:39", - "trx_id": "4539d12d4c2c2ef8161b8356d05da1c26cf1361b", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3916, - { - "block": 2891271, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51618, - "current_owner": "abit", - "current_pays": { - "amount": "1228", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "290", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:10:45", - "trx_id": "58a24f5735ed78a7d6acbbccd3b92fc0b31616d2", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3921, - { - "block": 2891285, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50642, - "current_owner": "abit", - "current_pays": { - "amount": "45858", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "169852", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:11:30", - "trx_id": "8947ef1dfd8749d3cea8eb0225b8068df4cb134c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3926, - { - "block": 2891290, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51681, - "current_owner": "abit", - "current_pays": { - "amount": "380174", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "89725", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:11:45", - "trx_id": "7952c228bef5a296e230f333dd3b57cf0be6b9c7", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3928, - { - "block": 2891291, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51684, - "current_owner": "abit", - "current_pays": { - "amount": "43536", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "10275", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:11:48", - "trx_id": "5e311b635eb8051653fbe62efdb2b041aed85b00", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3933, - { - "block": 2891305, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50708, - "current_owner": "abit", - "current_pays": { - "amount": "11589", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "42924", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:12:36", - "trx_id": "7932e1957285c78ea882417bd7bb0193e2e7d5ae", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3935, - { - "block": 2891310, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51747, - "current_owner": "abit", - "current_pays": { - "amount": "337868", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "79740", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:12:51", - "trx_id": "6e42cb85940a47c97e3457121e0e67d07bdcd5e5", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3937, - { - "block": 2891311, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51750, - "current_owner": "abit", - "current_pays": { - "amount": "84620", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19971", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:12:54", - "trx_id": "4d5bd57c3e952d93221d7e446ddcabafdac515b8", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3940, - { - "block": 2891312, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51753, - "current_owner": "abit", - "current_pays": { - "amount": "1224", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "289", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:12:57", - "trx_id": "daefb24b7b08088d933dd6d04913c410abcf7818", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3945, - { - "block": 2891326, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50771, - "current_owner": "abit", - "current_pays": { - "amount": "45857", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "169850", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:13:39", - "trx_id": "87bb9a14dab47951d703041b6fefcf54e23e36ce", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3950, - { - "block": 2891333, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51816, - "current_owner": "abit", - "current_pays": { - "amount": "380176", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "89725", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:14:00", - "trx_id": "7930e0811f299daa913d45df1b0eb36a8b75ce2f", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3952, - { - "block": 2891334, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51819, - "current_owner": "abit", - "current_pays": { - "amount": "43536", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "10275", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:14:03", - "trx_id": "8a2cea211902a3f2d892ab8961a47e88c00a5629", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3957, - { - "block": 2891348, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50837, - "current_owner": "abit", - "current_pays": { - "amount": "11588", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "42922", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:14:45", - "trx_id": "bbd00334fdf435e3079321b21bf4a50e0fbbca87", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3959, - { - "block": 2891355, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51882, - "current_owner": "abit", - "current_pays": { - "amount": "337866", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "79739", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:15:06", - "trx_id": "93dfbf52ffcf22e81e0db7fc09d9727f71d0f271", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3961, - { - "block": 2891356, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51885, - "current_owner": "abit", - "current_pays": { - "amount": "84620", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19971", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:15:09", - "trx_id": "46f5a2dddb144a87b0423b4a4fa45c7d0cbbd9d4", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3963, - { - "block": 2891357, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51888, - "current_owner": "abit", - "current_pays": { - "amount": "1228", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "290", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:15:12", - "trx_id": "5458590262cc16a4d2224f7731f03c2f983be833", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3971, - { - "block": 2891368, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50900, - "current_owner": "abit", - "current_pays": { - "amount": "45858", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "169852", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:15:48", - "trx_id": "00c0ba3328f8ddbcfb11d8eb32d0524fae3b81c7", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3976, - { - "block": 2891385, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51978, - "current_owner": "abit", - "current_pays": { - "amount": "380174", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "89724", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:16:42", - "trx_id": "fbc5adca47f948e23ca0484d898cd5e2db65b16b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3978, - { - "block": 2891386, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51981, - "current_owner": "abit", - "current_pays": { - "amount": "43540", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "10276", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:16:45", - "trx_id": "ffb07641b007f8d32dce771b566623957d11cbd9", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3983, - { - "block": 2891390, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 50969, - "current_owner": "abit", - "current_pays": { - "amount": "11589", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "42924", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:16:57", - "trx_id": "be42220fe1437fb9e05f324f954cfda83dea586f", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3985, - { - "block": 2891406, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 52044, - "current_owner": "abit", - "current_pays": { - "amount": "337864", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "79739", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:17:48", - "trx_id": "084ac73000f8de2e2ef33fbca5c707a0f9507656", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3987, - { - "block": 2891407, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 52047, - "current_owner": "abit", - "current_pays": { - "amount": "84620", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "19971", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:17:51", - "trx_id": "c7d3988b35ac7a838f65ea644a65b1800016b252", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3989, - { - "block": 2891408, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 52050, - "current_owner": "abit", - "current_pays": { - "amount": "1228", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "290", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:17:54", - "trx_id": "3e0a18ff32765abd8427db3c209aa5f229a00813", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3992, - { - "block": 2891409, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51032, - "current_owner": "abit", - "current_pays": { - "amount": "45858", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "169854", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:18:00", - "trx_id": "5c089b5eb4a1f73961141753fff1fdc926b9897a", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 3994, - { - "block": 2891428, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 52119, - "current_owner": "abit", - "current_pays": { - "amount": "337866", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "79739", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:19:03", - "trx_id": "fc4fbf6308e8cd9f191a97f020d7db2ea142468f", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3995, - { - "block": 2891428, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51095, - "current_owner": "abit", - "current_pays": { - "amount": "45858", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "169852", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:19:03", - "trx_id": "c7df5118cff1f505eb312009d8090ab441ed83b4", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 3997, - { - "block": 2891429, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 52122, - "current_owner": "abit", - "current_pays": { - "amount": "85848", - "nai": "@@000000021", - "precision": 3 - }, - "open_orderid": 20001, - "open_owner": "adm", - "open_pays": { - "amount": "20261", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:19:06", - "trx_id": "74ddcaeef82278b04b7b25702155c1baf86bccbe", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 4000, - { - "block": 2891449, - "op": { - "type": "fill_order_operation", - "value": { - "current_orderid": 51158, - "current_owner": "abit", - "current_pays": { - "amount": "91220", - "nai": "@@000000013", - "precision": 3 - }, - "open_orderid": 10001, - "open_owner": "adm", - "open_pays": { - "amount": "337866", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:20:06", - "trx_id": "64a7bb5fd9ad2fe0081fcda4370edee83cdc03b7", - "trx_in_block": 0, - "virtual_op": 1 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/fill_order_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/fill_order_operation.tavern.yaml deleted file mode 100644 index 704b5fc19c2694c590aae8375500c35d15c85595..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/fill_order_operation.tavern.yaml +++ /dev/null @@ -1,27 +0,0 @@ ---- - test_name: Hived account_history_api fill_order_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api fill_order_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"adm", "operation_filter_low": 36028797018963968, "start": 4000, "limit": 100} - response: - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "fill_order_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/fill_transfer_from_savings_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/fill_transfer_from_savings_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/fill_transfer_from_savings_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/fill_transfer_from_savings_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/fill_transfer_from_savings_operation.tavern.yaml deleted file mode 100644 index f640ee2cd731f63a6498cb6f8fd5be998f0b8f24..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/fill_transfer_from_savings_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api fill_transfer_from_savings_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api fill_transfer_from_savings_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 144115188075855872, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "fill_transfer_from_savings_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/fill_vesting_withdraw_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/fill_vesting_withdraw_operation.pat.json deleted file mode 100644 index b69de8a2483e456de98699ebc91ef1f720e6391f..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/fill_vesting_withdraw_operation.pat.json +++ /dev/null @@ -1,33 +0,0 @@ -{ - "history": [ - [ - 3210, - { - "block": 2888724, - "op": { - "type": "fill_vesting_withdraw_operation", - "value": { - "deposited": { - "amount": "299792", - "nai": "@@000000021", - "precision": 3 - }, - "from_account": "adm", - "to_account": "adm", - "withdrawn": { - "amount": "1569493022171", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T23:45:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/fill_vesting_withdraw_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/fill_vesting_withdraw_operation.tavern.yaml deleted file mode 100644 index c00dfc06f0f48ef19fcc2cd0a6ea2f7776619fb1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/fill_vesting_withdraw_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api fill_vesting_withdraw_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api fill_vesting_withdraw_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"adm", "operation_filter_low": 18014398509481984, "start": 3210, "limit": 1} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "fill_vesting_withdraw_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/gtg.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/gtg.pat.json deleted file mode 100644 index 78a68334b01f55f3a97f0f78c0af9a131aa59281..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/gtg.pat.json +++ /dev/null @@ -1,251 +0,0 @@ -{ - "history": [ - [ - 1, - { - "block": 2796791, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "gtg", - "vesting_shares": { - "amount": "5401442811", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T18:59:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 2, - { - "block": 2797050, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pfunk", - "pending_payout": { - "amount": "812683", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "guide-maximize-your-mining-hashrate-in-windows-by-mining-steem-in-a-vm", - "rshares": 27547358, - "total_vote_weight": "20780999058208", - "voter": "gtg", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:12:21", - "trx_id": "e0cd24f9aba3b668b1443786bee3891f644ff23f", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 3, - { - "block": 2797050, - "op": { - "type": "vote_operation", - "value": { - "author": "pfunk", - "permlink": "guide-maximize-your-mining-hashrate-in-windows-by-mining-steem-in-a-vm", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:12:21", - "trx_id": "e0cd24f9aba3b668b1443786bee3891f644ff23f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 4, - { - "block": 2797188, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pfunk", - "pending_payout": { - "amount": "506396", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "security-b-sides-msp-2016-information-security-conference-day-1-opening-keynote-notes-and-recap", - "rshares": 27007214, - "total_vote_weight": "35589961187203", - "voter": "gtg", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:19:15", - "trx_id": "be93d0b153a5b9a12547180a1d88f2aad1669754", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 5, - { - "block": 2797188, - "op": { - "type": "vote_operation", - "value": { - "author": "pfunk", - "permlink": "security-b-sides-msp-2016-information-security-conference-day-1-opening-keynote-notes-and-recap", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:19:15", - "trx_id": "be93d0b153a5b9a12547180a1d88f2aad1669754", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 6, - { - "block": 2797362, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pfunk", - "pending_payout": { - "amount": "387792", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "lets-discuss-verification-of-user-accounts-posting-previous-work-to-prevent-impersonation", - "rshares": 27007214, - "total_vote_weight": "30456732837113", - "voter": "gtg", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:27:57", - "trx_id": "c7a8b59428f96adc3245345bd4fd7c587aad3383", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 7, - { - "block": 2797362, - "op": { - "type": "vote_operation", - "value": { - "author": "pfunk", - "permlink": "lets-discuss-verification-of-user-accounts-posting-previous-work-to-prevent-impersonation", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:27:57", - "trx_id": "c7a8b59428f96adc3245345bd4fd7c587aad3383", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8, - { - "block": 2797645, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "zajac", - "pending_payout": { - "amount": "3541186", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "hi-steemit-people-lets-waste-some-time-together-", - "rshares": 27007214, - "total_vote_weight": "29308749708982", - "voter": "gtg", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:42:09", - "trx_id": "f98b305720148c4b37b19c1c7616ad9057e46c32", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 9, - { - "block": 2797645, - "op": { - "type": "vote_operation", - "value": { - "author": "zajac", - "permlink": "hi-steemit-people-lets-waste-some-time-together-", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:42:09", - "trx_id": "f98b305720148c4b37b19c1c7616ad9057e46c32", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 10, - { - "block": 2812987, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "cylonmaker2053", - "pending_payout": { - "amount": "84920", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "steemit-infrastructure-security-scalability-and-points-of-failure", - "rshares": 27547358, - "total_vote_weight": "15377444600345", - "voter": "gtg", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-01T08:30:48", - "trx_id": "0b42bdd9a3961b740dc5f32857b645b1220e95d7", - "trx_in_block": 0, - "virtual_op": 1 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/gtg.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/gtg.tavern.yaml deleted file mode 100644 index cb6c4fb2befceb19247eb6af1f262e25c15f848c..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/gtg.tavern.yaml +++ /dev/null @@ -1,32 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction gtg - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction gtg - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: { - "account": "gtg", - "start": 10, - "limit": 10 - } - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "gtg" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_operation.tavern.yaml deleted file mode 100644 index c81778c3558920b1f7896d0e7132ccc3b848178d..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api hardfork_hive_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api hardfork_hive_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_high": 4, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "hardfork_hive_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_restore_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_restore_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_restore_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_restore_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_restore_operation.tavern.yaml deleted file mode 100644 index 0934f2a883bf912eab0b1e495a7b5b915ebbe5cd..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_hive_restore_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api hardfork_hive_restore_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api hardfork_hive_restore_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_high": 8, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "hardfork_hive_restore_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/hardfork_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/hardfork_operation.tavern.yaml deleted file mode 100644 index 03d379e809f10160f080c879171d45ab177b8775..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/hardfork_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api hardfork_operation - - marks: - - patterntest # virtual - # not assigned to the account - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api hardfork_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 288230376151711744, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "hardfork_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/ineffective_delete_comment_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/ineffective_delete_comment_operation.pat.json deleted file mode 100644 index 6cbc6d9567d233498010f8e08f3f5979f97960a9..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/ineffective_delete_comment_operation.pat.json +++ /dev/null @@ -1,23 +0,0 @@ -{ - "history": [ - [ - 595, - { - "block": 2111808, - "op": { - "type": "ineffective_delete_comment_operation", - "value": { - "author": "jsc", - "permlink": "re-vadimberkut8-just-test-20160603t163718014z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-06T21:38:54", - "trx_id": "3461cfaabbf36ed0571d71008ee20ba4ae74400d", - "trx_in_block": 0, - "virtual_op": 1 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/ineffective_delete_comment_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/ineffective_delete_comment_operation.tavern.yaml deleted file mode 100644 index 42e755c9a28818dda8136a65eb0ddca6b125d495..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/ineffective_delete_comment_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api ineffective_delete_comment_operation - - marks: - - patterntest # virtual - # next and last one is 791 of the same account - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api ineffective_delete_comment_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"jsc", "operation_filter_high": 128, "start": 595, "limit": 2} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "ineffective_delete_comment_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/interest_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/interest_operation.pat.json deleted file mode 100644 index 243f2302b0e25e9221ee442a0f2f8ce34c7c4e5f..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/interest_operation.pat.json +++ /dev/null @@ -1,27 +0,0 @@ -{ - "history": [ - [ - 8115, - { - "block": 2893044, - "op": { - "type": "interest_operation", - "value": { - "interest": { - "amount": "247", - "nai": "@@000000013", - "precision": 3 - }, - "owner": "xeroc" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T03:42:21", - "trx_id": "f9e46925b19db80ab57f2e5824b02eae3392133a", - "trx_in_block": 2, - "virtual_op": 1 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/interest_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/interest_operation.tavern.yaml deleted file mode 100644 index 390e61d777b252253b37d8dc78f179006d947f34..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/interest_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api interest_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api interest_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"xeroc", "operation_filter_low": 9007199254740992, "start": 8115, "limit": 1} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "interest_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_cancel_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/limit_order_cancel_operation.pat.json deleted file mode 100644 index cb25287a2767a9101603db0791364fcde01419d1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_cancel_operation.pat.json +++ /dev/null @@ -1,99 +0,0 @@ -{ - "history": [ - [ - 1503, - { - "block": 2832389, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 1, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T00:42:45", - "trx_id": "20711c439525ddba796086438fba5d48b971cb80", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 1506, - { - "block": 2832439, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 1, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T00:45:15", - "trx_id": "7ef08c9b439f4b572dc8362b69ca0011590fc67e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 1542, - { - "block": 2833513, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 1, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T01:39:15", - "trx_id": "4c01c8681e265580568fa328fc697ebdd7b81827", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 1550, - { - "block": 2833711, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 1, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T01:49:12", - "trx_id": "44b2ea943ac95e30b1bac5b8f96f19242c735eaa", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 1819, - { - "block": 2842098, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 1, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T08:49:24", - "trx_id": "f8fcf933d669a0f7d5a5bd482341bfe3f3e1d139", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_cancel_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/limit_order_cancel_operation.tavern.yaml deleted file mode 100644 index d9e62ebb70421a14d581a98eab1d37988dfdcbbf..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_cancel_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to limit_order_cancel_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history limit_order_cancel_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"adm","operation_filter_low": 64,"start": 2000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "limit_order_cancel_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create2_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create2_operation.pat.json deleted file mode 100644 index 4002a4b7524d6948f3d27d1941627f5b69c2b7ce..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create2_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create2_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create2_operation.tavern.yaml deleted file mode 100644 index 3f93b46e99696aedf3460980b538f690041a9497..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create2_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to limit_order_create2_operation - - marks: - - patterntest # no noempty result - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history limit_order_create2_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades","operation_filter_low": 2097152,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "limit_order_create2_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_and_cancel.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_and_cancel.pat.json deleted file mode 100644 index 7142c2ca46068106e6633fa812c0592fbdfb87fd..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_and_cancel.pat.json +++ /dev/null @@ -1,25532 +0,0 @@ -{ - "history": [ - [ - 8851, - { - "block": 2905471, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:07:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:18", - "trx_id": "a9d32e4d22bb76cca9a969cf91df0233d6c87d89", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8852, - { - "block": 2905472, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:21", - "trx_id": "a417282d469de3c1bd29b7b733d85d9601fcb750", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8853, - { - "block": 2905472, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:07:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:21", - "trx_id": "474c6ccae7cc25522523bb4e210cfbce204a0c3b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8854, - { - "block": 2905473, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:24", - "trx_id": "b380e4b56a839f41489e021cafd1cd86e9f2c67f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8855, - { - "block": 2905473, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:07:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:24", - "trx_id": "838dbdbc36fe733e609e62c82f344d9ba3e2f4b6", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8856, - { - "block": 2905474, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:27", - "trx_id": "e74afb4ed797fc554e1efda4047337054e7238d6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8857, - { - "block": 2905475, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:30", - "trx_id": "04fcb48f21304e174ecdd8d6a5cbd7724a668287", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8858, - { - "block": 2905477, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:36", - "trx_id": "323536387e4b3ffdd53aa1003b957a3663c9e73d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8859, - { - "block": 2905477, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:36", - "trx_id": "2bc02a2566c85be8af174aa28b932c4faa6cbefc", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8860, - { - "block": 2905477, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:36", - "trx_id": "8dfac3a9c1ebb446801fcc20f08a1212b2342ce1", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8861, - { - "block": 2905477, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:36", - "trx_id": "6b1f1e16963c2306368560744d3dbb5672550628", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 8862, - { - "block": 2905478, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:39", - "trx_id": "3a66911bfd6e8d03d25d0a1300b893b59f18ba90", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8863, - { - "block": 2905479, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:42", - "trx_id": "089d0d8af75357deecbeea1663e483d3dbf86bc0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8864, - { - "block": 2905479, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:42", - "trx_id": "865f7426d6fde18faf22e15cd5a5ffef4b100235", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8865, - { - "block": 2905479, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:42", - "trx_id": "4c77dafe9dff216ab180c3d4035ab747688df84e", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8867, - { - "block": 2905480, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:45", - "trx_id": "67b2c7d6ede0fae7bb819ec38ab3968779b5ed22", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8869, - { - "block": 2905480, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:45", - "trx_id": "b8d4628abb81aacc4afd53983187fb2c73a93988", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8870, - { - "block": 2905480, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:45", - "trx_id": "527770b483aae26030f6fda3b46fa8f0e14d9fc2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 8872, - { - "block": 2905481, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:48", - "trx_id": "636350f2013f534ac33c114f0cd8e73c4693b873", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8873, - { - "block": 2905482, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:51", - "trx_id": "cfcb1e8b96b1a0eeea64eade0a96d33411ff2372", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8874, - { - "block": 2905482, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:51", - "trx_id": "7814ff331581a1fd77338047fed7fa3c094ccb56", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8875, - { - "block": 2905482, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:51", - "trx_id": "6478bd9fc5c910063655fa3db97b6f0272437ca1", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8876, - { - "block": 2905483, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:54", - "trx_id": "0c807bde1a05cb3ea13e60a8c9b19469f026682c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8877, - { - "block": 2905484, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:57", - "trx_id": "66090c42ab8d243dc95875b3d87cd4162e127aab", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8878, - { - "block": 2905484, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:57", - "trx_id": "dd8be2237a7aea5475d23ccb504b9b9aac3294ee", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8879, - { - "block": 2905484, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:06:57", - "trx_id": "40b4108c01a4bbce1ad944a22116ca25c078b85d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8882, - { - "block": 2905485, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:00", - "trx_id": "b4e4ac124cba092c308a37f3bb3974651ce3b80d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8883, - { - "block": 2905485, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:00", - "trx_id": "07e40d0c24a8e81fb5094056a6b7917c5e83a3c3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8884, - { - "block": 2905485, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "417567", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:00", - "trx_id": "13447b9c7fe1f8e27fd1287f53a1ac404111b69a", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8886, - { - "block": 2905486, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:03", - "trx_id": "659251c50c10a6327abaef3039420bc98bb6146d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8888, - { - "block": 2905487, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:06", - "trx_id": "c80b578e44c395b54db1ae4a5fabc6a6e5357237", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8889, - { - "block": 2905488, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:09", - "trx_id": "09e12450145cf8c239442ef76142cfdf3d5f1b53", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8890, - { - "block": 2905488, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:09", - "trx_id": "c8d3ed14cf2b5552991dbd403f143a49fd2e25f1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8892, - { - "block": 2905489, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:12", - "trx_id": "e38a15ef67e4751c1f3c702a6aef9ac72854abc9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8893, - { - "block": 2905489, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:12", - "trx_id": "dca482baeee51142d0de0795cfea3d2df9ddb446", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8894, - { - "block": 2905490, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:15", - "trx_id": "98aea847efe5c14166a35abb64f166c228a14625", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8895, - { - "block": 2905491, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:18", - "trx_id": "83c995d15bdc67f7d73ab0d08846bcb4f3be07a0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8896, - { - "block": 2905491, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:18", - "trx_id": "c325ef41b3d167e4473f7f930930a709551e74d8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8897, - { - "block": 2905491, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:18", - "trx_id": "27eab303494240466501218ba94f4e91f32126af", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8898, - { - "block": 2905492, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:21", - "trx_id": "95e97e8c465857b5287cdf879690551c212c1111", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8899, - { - "block": 2905492, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:21", - "trx_id": "41dd7622cce31bcea37ae8a629d0106943fc44a4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8900, - { - "block": 2905493, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:24", - "trx_id": "b0ac9b60fc618685ed98a7166ecbabe6d3d0f516", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8901, - { - "block": 2905493, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:24", - "trx_id": "f2869c774993a58602571194ed717e0f133a3d94", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8902, - { - "block": 2905494, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:27", - "trx_id": "d34cd49e5bbf352e42dc4bc5ed3aebaf4fd93cf2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8903, - { - "block": 2905494, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:08:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:27", - "trx_id": "16ed57bc36f893cf457eeb0a686dfddb6ff47115", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8904, - { - "block": 2905495, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:30", - "trx_id": "d7d192a4289c0747f4e4119623993f1b861843bc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8905, - { - "block": 2905495, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:30", - "trx_id": "c3a24585742367115191bb9f6aac8dbfe62a767a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8906, - { - "block": 2905496, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:33", - "trx_id": "04e525062b60467aa3abf66c00c48f730392c7f4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8907, - { - "block": 2905497, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:36", - "trx_id": "f42667faca46cf486a0dc6c42d3bd4c82a71545c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8908, - { - "block": 2905498, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:39", - "trx_id": "f68b0b35e1dd17264d74d4049776c7dba3bf25dd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8909, - { - "block": 2905498, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:39", - "trx_id": "a7ba01679df4e0b0c3e27efd6cb77adb3a96d3e8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8910, - { - "block": 2905499, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:42", - "trx_id": "3fc34a351552c95847cc22801f14712800270429", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8911, - { - "block": 2905500, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:45", - "trx_id": "ae35a820292c247e372f2465f1fcebeb7808a33f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8912, - { - "block": 2905500, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:45", - "trx_id": "177101cdd71c555c2fb481cda340c325dce4030e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8913, - { - "block": 2905500, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:45", - "trx_id": "2c26755941694f72eb4df84d08d61bfe5ac770a0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8914, - { - "block": 2905501, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:48", - "trx_id": "50c4b6ce6af6e47ebe5b8f7ee6aed68bf46b3dd1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8915, - { - "block": 2905502, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:51", - "trx_id": "b6206a1a40698ff7137016a2a7907bba93b25a8d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8916, - { - "block": 2905502, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:51", - "trx_id": "a692733ac72c0d6e247be2981d38855672f94e5f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8917, - { - "block": 2905503, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:54", - "trx_id": "04692f2e93dd1e80483a1ef48c87ee2961ea2fbe", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8918, - { - "block": 2905503, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:54", - "trx_id": "d28239a4d450e54fc4356b47d198020a305b2131", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8919, - { - "block": 2905503, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:54", - "trx_id": "e61ff392a7ff8ee7d30608c38df43f4b8c3fdaa0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8920, - { - "block": 2905504, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:57", - "trx_id": "f2c5361130b339fc80c4df6cf4b95545a896b19d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8921, - { - "block": 2905504, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:07:57", - "trx_id": "1c0f4ff2b24c95cffd11bb78d9b1a4c35d88a2a4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8922, - { - "block": 2905505, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:00", - "trx_id": "f7d5a72452505ad64392a65fe66482152ca172e1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8924, - { - "block": 2905505, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:00", - "trx_id": "5fa91dc1f232df1bedfe0b8fe82baeaed13ce4f6", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8925, - { - "block": 2905506, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:03", - "trx_id": "9193c52218c2c2530618ad943b13b50e244efc2f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8926, - { - "block": 2905506, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:03", - "trx_id": "521a8cabc26ed885572a561a69816abff37adb41", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8928, - { - "block": 2905506, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:03", - "trx_id": "855c1a742b0c8710a8d4077bc071298a624e333a", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 8929, - { - "block": 2905507, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:06", - "trx_id": "58ea9aca879097e21176196c593b59235db69101", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8930, - { - "block": 2905507, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:06", - "trx_id": "33f0f265aba58b7e06c927a7ecd98f7a8afd1563", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8931, - { - "block": 2905508, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:09", - "trx_id": "114e81384e6c0318bac3db1598aa0832c7949b19", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8932, - { - "block": 2905508, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:09", - "trx_id": "60c0747c3eab6641fcfb24eb6332cb8e6497124b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8933, - { - "block": 2905509, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:12", - "trx_id": "1433cbb94b67a9b9967ef8a41fb4ab73d8f405db", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8934, - { - "block": 2905509, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:12", - "trx_id": "9ba08c1c047200a79207105945096b753d407a3e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8935, - { - "block": 2905510, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:15", - "trx_id": "c76508b6fe1f67d794da8f89726210c238ceb905", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8936, - { - "block": 2905510, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:15", - "trx_id": "e79a6f772aa5e31e6d07d474bddb944fb5ffeece", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8937, - { - "block": 2905511, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:18", - "trx_id": "9bb73042367134aa3a6d2de48186b97a028330d7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8938, - { - "block": 2905511, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:18", - "trx_id": "ef65489582c12918f45cae5a35ccbd9fbfe07aca", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8939, - { - "block": 2905512, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:21", - "trx_id": "909d32c61665dea36bbcf186b2b3358d82a8f3a5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8940, - { - "block": 2905512, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:21", - "trx_id": "b86a7209c9fa878bf590898a096d395f094679b3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8941, - { - "block": 2905513, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:24", - "trx_id": "8a6ee41dd5e663530dd67565569beaf4ac2bdc93", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8942, - { - "block": 2905514, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:09:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:27", - "trx_id": "b406cf99ed71fe2a4e3462d2cfd70c3d4d717d97", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8943, - { - "block": 2905515, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:30", - "trx_id": "e5e29a2bea3aa51be6acd771b1b0b601fef5e9c8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8944, - { - "block": 2905515, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:30", - "trx_id": "faa18433b1b37c15421614dec52f1b124dc62883", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8946, - { - "block": 2905516, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:33", - "trx_id": "1c08e988e4e37b66e83111d6c5bc9c1735cd91a7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8947, - { - "block": 2905516, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:33", - "trx_id": "c6a16e433dc42e74d7915ebc4341467ccfd63c9d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8948, - { - "block": 2905517, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:36", - "trx_id": "f3679884e3bf72d5310cdc840039cf172f3df9cc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8949, - { - "block": 2905517, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:36", - "trx_id": "fd50f8c4d568ee60a13df671bf5986c415b87bc9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8950, - { - "block": 2905518, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:39", - "trx_id": "9c85898e8789668b660145e5690cf629ff9ef82e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8951, - { - "block": 2905519, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:42", - "trx_id": "3c68b02febca23fb018527379fb6d9559f1281d6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8952, - { - "block": 2905519, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:42", - "trx_id": "5b34ae65c1000572595aecd54bf5f9faa5ac2503", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8953, - { - "block": 2905519, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:42", - "trx_id": "49c774280d765cc05d932cdd10f4b8dbf4a20b61", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8954, - { - "block": 2905521, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:48", - "trx_id": "854e1750fb5662a08458bdc89ff95cebf06d4c9d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8955, - { - "block": 2905521, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:48", - "trx_id": "2bdb699902e91b2767629c100e2c882850731005", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8956, - { - "block": 2905521, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:48", - "trx_id": "6d8a7d034d655bcb396046b9df4b5e6cfacc4d7d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8957, - { - "block": 2905521, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:48", - "trx_id": "84b1e8270973307e4a08cb24772b382a7d96cd8b", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 8958, - { - "block": 2905522, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:51", - "trx_id": "d54aebfb6bff65b36411320df0690e9c163ee2b8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8959, - { - "block": 2905523, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:54", - "trx_id": "05496c0e6a2ee5a027b0639fc7a9226249f84a5a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8960, - { - "block": 2905523, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:54", - "trx_id": "a88474da43a10c94e6af6e60cd0eedd21aedb834", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8961, - { - "block": 2905524, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:57", - "trx_id": "cdac0c488c994e06121961455a6108145ca17283", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8962, - { - "block": 2905524, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:57", - "trx_id": "9347ee65b82fd4ea6331c9416db18846e7d1d6ba", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8963, - { - "block": 2905524, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:08:57", - "trx_id": "a92e93c6a281a78aa73f1996238c06c56c954bb3", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8964, - { - "block": 2905525, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:00", - "trx_id": "65abccab7f6f7b186b979c5cbce62749a517bd2f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8965, - { - "block": 2905525, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:00", - "trx_id": "1759787cde1ddc7864d6ba04f481db4daba2cd1e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8966, - { - "block": 2905526, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:03", - "trx_id": "a6341fafbf6b2c734745f353926fd218f0df3a51", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8967, - { - "block": 2905526, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:03", - "trx_id": "dc69c207164e4dc6b75733f029e98dbddf58a69b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8969, - { - "block": 2905527, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:06", - "trx_id": "6851c849b64a55e4e3ad27cc5a14db21330c549f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8970, - { - "block": 2905527, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:06", - "trx_id": "c6e13885639ea84ba78e227a9099d207a111b2e4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8971, - { - "block": 2905527, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:06", - "trx_id": "818f1cde531249a3d190db8dfb542ffb47c59f77", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8974, - { - "block": 2905528, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:09", - "trx_id": "a7757c324e56f8fa9140acd8af39f43132533ea7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8976, - { - "block": 2905529, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:12", - "trx_id": "8fe90428d10c4a5c67a923bc6ef79269720f7cd1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8977, - { - "block": 2905529, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:12", - "trx_id": "8d61e50c4909599fdb283227bf4aab5526ce5e54", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8978, - { - "block": 2905530, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:15", - "trx_id": "f11ca219a76141345f3907acae22335a1b226ee9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8979, - { - "block": 2905530, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:15", - "trx_id": "a97eefd2674c11542df9782070c5705b1623f6f4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 8980, - { - "block": 2905531, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:18", - "trx_id": "39480e5caae2c519870c0963153add9a43481555", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8981, - { - "block": 2905531, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:18", - "trx_id": "0139f023c6ed3a71831e9fa17e04bfd398b033d2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8982, - { - "block": 2905532, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:21", - "trx_id": "be34f8dbac3c6bf449b6ccfb2cfb2b8f942432c3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8983, - { - "block": 2905532, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:21", - "trx_id": "7aaad8970ac6750a68a10d89d7df0270ada352fe", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8984, - { - "block": 2905532, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:21", - "trx_id": "6eb79ab504bbccbb4b5e60863affeb401c4d9bfb", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 8985, - { - "block": 2905533, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:24", - "trx_id": "5ffa7dfc60c6e759cddebdfa179acb61ff4bff8c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8986, - { - "block": 2905533, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:24", - "trx_id": "3ffdff650b413e8dae4f114417cce92c05343c2c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8987, - { - "block": 2905534, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:27", - "trx_id": "09ac28b0b74cf59fb13292a5106f50839c9b3fa8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8988, - { - "block": 2905534, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:10:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:27", - "trx_id": "c52f37b824984a5fcfbb183a6ba1c88d76043547", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8989, - { - "block": 2905535, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:30", - "trx_id": "5cf63d807226f750d0bdde2df6c3e68ae05e80fc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8990, - { - "block": 2905535, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:30", - "trx_id": "22d0717b16a478e965acc75d2ebcbd5ba079d5f5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8991, - { - "block": 2905536, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:33", - "trx_id": "d7e3681cb26c64bcc6bd6a7ab44b43f859d841af", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8992, - { - "block": 2905537, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:36", - "trx_id": "1ad83fbd6407a563eb88b61f5cc484096df7a6c5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8993, - { - "block": 2905538, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:42", - "trx_id": "4d58f63efee935b9f166dd6f1321f8b29021f2d4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8994, - { - "block": 2905538, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:42", - "trx_id": "51c6ae39ed33e44393f19c7dff32222feb9e3704", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8996, - { - "block": 2905539, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:45", - "trx_id": "43be172996297cae6636b003055716347ca4907d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8997, - { - "block": 2905539, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:45", - "trx_id": "8f6fe67e98c3665ffef9281b236b9896c6dd9d34", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 8998, - { - "block": 2905540, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:48", - "trx_id": "d6e329d7fd2fbc5d4d997bf960a9b55a41964aca", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8999, - { - "block": 2905540, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:48", - "trx_id": "e05fc4c1d63a5019d2372b678e950b12d7789a6c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9000, - { - "block": 2905541, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:51", - "trx_id": "6b3b5f30504aa7b19b2eeb4f120f8d747f97e344", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9001, - { - "block": 2905541, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:51", - "trx_id": "d2e9e6d89941f506eeffb37c21b199a8d5355226", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9002, - { - "block": 2905542, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:54", - "trx_id": "dbee5f86b0f2751855d526f3261db83a5b432085", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9003, - { - "block": 2905543, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:57", - "trx_id": "14c69f96951404ac82d6e7a565838be77dac50d8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9004, - { - "block": 2905543, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:57", - "trx_id": "b3a231a47282e3708e4ed12de353181fe1d70329", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9005, - { - "block": 2905543, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:09:57", - "trx_id": "42c70f45ec4dd458c574f28a36e86a814a7485c0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9006, - { - "block": 2905544, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:00", - "trx_id": "51b328bb4a38d257efe40a191301ab29626f42e2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9007, - { - "block": 2905544, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:00", - "trx_id": "9d45d0640c9a46c40bd317bb86b4e931eae6dae4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9008, - { - "block": 2905545, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:03", - "trx_id": "94f1093e4401733e4320490e9a00c5a06eeb0357", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9009, - { - "block": 2905545, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:03", - "trx_id": "df3b86a6a9745cda207251fb47eda957d3439190", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9010, - { - "block": 2905546, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:06", - "trx_id": "d0e72755ef5f897f83d807c7e8cae2521abe1a42", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9011, - { - "block": 2905546, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:06", - "trx_id": "ea2c08eb18d90d91654def63e04eb00bb0ed7b35", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9013, - { - "block": 2905547, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:09", - "trx_id": "0942440f299a39081d6770e9d07b36b68b4683da", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9014, - { - "block": 2905547, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:09", - "trx_id": "7dc810f22bdadf00b6fbfc8651c6aff71baf84bf", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9016, - { - "block": 2905547, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:09", - "trx_id": "13711fc541ca21e97d779142648036350bc2b9b2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9017, - { - "block": 2905548, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:12", - "trx_id": "f91c7cad67759f0795742d7e9d3aaa3b3d0f2b1f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9018, - { - "block": 2905548, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:12", - "trx_id": "1dccf0c0fe2a5a47d17ea1c2b4607461f51a0384", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9020, - { - "block": 2905549, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:15", - "trx_id": "980bcd072eab06c4a2df3e392749378a73ee424d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9021, - { - "block": 2905549, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:15", - "trx_id": "9640efdbf968899a5cc25d312efbf7974668488c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9022, - { - "block": 2905550, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:18", - "trx_id": "6512bd8f6d7cf0da86e1f78550147d09b572389d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9023, - { - "block": 2905551, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:21", - "trx_id": "9e26acc3e066c5fe365a9748c4dcaeb516fe4e2b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9024, - { - "block": 2905551, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:21", - "trx_id": "967d183cc7d685ff885e00525b5a73303e60d6da", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9025, - { - "block": 2905551, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:21", - "trx_id": "30fd4c83cc5ed799bcf4a7ac28ac5d2dafde90ab", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9026, - { - "block": 2905552, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:24", - "trx_id": "aa559636bd921811b4e8e3977f61f2b6b2fde167", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9027, - { - "block": 2905552, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:24", - "trx_id": "ebae2148d0bba1f3144c3c4685c4b4a69dde3876", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9028, - { - "block": 2905553, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:27", - "trx_id": "9aac1e91e119b8f81d6d6e42d3bbd26b517f76f5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9029, - { - "block": 2905553, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:11:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:27", - "trx_id": "abb34923ae329e6c1922ac2b624cbe18a0d12709", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9030, - { - "block": 2905554, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:30", - "trx_id": "f2d4c9872b201c531541d8018eaf5371be51aebe", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9031, - { - "block": 2905554, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:30", - "trx_id": "4f26b1d939924dd1f287dba6075ca1f121757297", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9032, - { - "block": 2905555, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:33", - "trx_id": "ce148e004b6735427620443ad788a631e1766583", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9033, - { - "block": 2905555, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:33", - "trx_id": "92b8cf92fdf95cb1f24482935b0981273cca838d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9034, - { - "block": 2905556, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:36", - "trx_id": "491ca6532e01c22d5f0c22851ad8cb599ecd52f0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9035, - { - "block": 2905556, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:36", - "trx_id": "f1e41854fddfecccce89b24e8b934d877c9c239c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9036, - { - "block": 2905557, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:39", - "trx_id": "c06f7f06b6bb9179602ed7afef96563fe74bc406", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9037, - { - "block": 2905557, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:39", - "trx_id": "dab3406449e7d6334f45c1264fcf7ecda1dedaba", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9038, - { - "block": 2905558, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:42", - "trx_id": "e221e96ea65e4cc86941cbbb8bed5d7c7203f5c2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9039, - { - "block": 2905559, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:45", - "trx_id": "e8fab007f2675e3f287753c898c164e145507d33", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9040, - { - "block": 2905559, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:45", - "trx_id": "4b6961789f1cf5a0a5b8d1babd77015c47fcda94", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9041, - { - "block": 2905560, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:48", - "trx_id": "cb5f1f0d07decfd2b7f25eafe089129d0bb2e27e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9042, - { - "block": 2905560, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:48", - "trx_id": "b40b7c9d44043dfa0d23c1e99b7a5bbeca28757a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9043, - { - "block": 2905560, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:48", - "trx_id": "02fb8139efaa90b4007c2b39943412cda385321c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9044, - { - "block": 2905561, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:51", - "trx_id": "751271f214c413e84fb698616bd11572c57e52ba", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9045, - { - "block": 2905561, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:51", - "trx_id": "1704678e515a84b477d02356d30dde9b8c4c8e2a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9046, - { - "block": 2905562, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:54", - "trx_id": "582402afbc6f11b5fe5df11dc670db43bf236820", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9047, - { - "block": 2905562, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:54", - "trx_id": "7a609df3ac6ae2bbf19125d38d285d88e1dbf1eb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9048, - { - "block": 2905563, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:57", - "trx_id": "56eb3f6ecd7648f7aee9f78668066672930eeb3f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9049, - { - "block": 2905563, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:10:57", - "trx_id": "d5b9ecf6cffb94e5a18fc39ea5e52a394e7457de", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9050, - { - "block": 2905564, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:00", - "trx_id": "c5365977cfb65c3c1d1256e440b1ce6bba360e4a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9051, - { - "block": 2905565, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:03", - "trx_id": "71cb7eb1068951c39f65d63897644788bc552d0d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9052, - { - "block": 2905565, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:03", - "trx_id": "9bfaf8c0d98ca47003b1d6874d7702fe9bb3db92", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9053, - { - "block": 2905565, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:03", - "trx_id": "89608155d75779ecccedfaa9421a50c342da9ebf", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9054, - { - "block": 2905566, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:06", - "trx_id": "21af28806a5c8727241460bd36bf2c3c37ea69d6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9055, - { - "block": 2905567, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:09", - "trx_id": "80aaa8f56943234784617f8758950696b0860efa", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9056, - { - "block": 2905567, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:09", - "trx_id": "a81f70b0a351f788d6cbea17895f18e43c8b66dd", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9057, - { - "block": 2905567, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:09", - "trx_id": "8db7308e134bb83fcad5e64095382fb4f92d3f20", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9059, - { - "block": 2905568, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:12", - "trx_id": "b30dc01c4a7b09feb3f3e79636c378d13eafadb1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9061, - { - "block": 2905568, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:12", - "trx_id": "a2593583436d218a0423aa73f7f0c296aa2d776d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9062, - { - "block": 2905568, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:12", - "trx_id": "852e9d4509fe2f4faeb34ace706a4cfe3030f4ac", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9063, - { - "block": 2905569, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:15", - "trx_id": "00d5ff06b2d39346863e7162dca512b25d1c2e15", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9064, - { - "block": 2905569, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:15", - "trx_id": "a2c352993901c468067f6ebb08f913aaa8c4eaea", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9066, - { - "block": 2905570, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:18", - "trx_id": "d9fc946dc33e764cfd0dbd9989f8861debf18dfc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9067, - { - "block": 2905570, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:18", - "trx_id": "4cb76b230c1b832258677ba13d8ba95412e7dcb3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9069, - { - "block": 2905571, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:21", - "trx_id": "cb55ee0258b6f39663eda81a0910696ad438aa4d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9070, - { - "block": 2905571, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:21", - "trx_id": "24695afc5acf0f931a5f8119102e934c2f5fd934", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9071, - { - "block": 2905572, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:24", - "trx_id": "8ec8d87ab0e7b38c2bd822c2b5c7a53aba91cd7f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9072, - { - "block": 2905572, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:24", - "trx_id": "d36f96605e1e9b76bb4d6a4d1124dd535321dff6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9073, - { - "block": 2905573, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:27", - "trx_id": "7a4c019f00f734af5ac352970c5695f157a1c66f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9074, - { - "block": 2905574, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:12:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:30", - "trx_id": "2cd41aab56e2b81019459637d0c9992f01f5c3eb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9075, - { - "block": 2905574, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:30", - "trx_id": "d7e3bb7a914a50d334514e1e2ea390be20257f21", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9076, - { - "block": 2905575, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:33", - "trx_id": "18a6f515975f252ed76c488472bd51ec34e1241e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9077, - { - "block": 2905575, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:33", - "trx_id": "c34abcc6f2a17f81d29829ca131b54c9fca2a670", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9078, - { - "block": 2905575, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:33", - "trx_id": "275535dbb3a14b4e1397a1802d8050223e7b1ee0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9079, - { - "block": 2905576, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:36", - "trx_id": "506fdacc2d7a6f56f14581958d342d5c9188ac2d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9080, - { - "block": 2905576, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:36", - "trx_id": "95efaea0440f5155cdad7508330809b28920ccc2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9081, - { - "block": 2905577, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:39", - "trx_id": "560beeededf0ddda208d0ecf1d5953f27896715a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9082, - { - "block": 2905577, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:39", - "trx_id": "cbe5c640db29bc7ba61815e984e5b0b71fb3693e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9083, - { - "block": 2905578, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:42", - "trx_id": "2035a822de84f15e14c0256b938560c7b6cbc6bf", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9084, - { - "block": 2905579, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:45", - "trx_id": "48dcaf63ada328f2fa4bc4bcd2141e6480b0021d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9085, - { - "block": 2905579, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:45", - "trx_id": "f448d9566f2acdecec3a26f5c46260befd5cac95", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9086, - { - "block": 2905580, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:48", - "trx_id": "d87688d76dcf29178d5ca88042579a0ff612cc36", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9087, - { - "block": 2905580, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:48", - "trx_id": "18a359b43c5fbbe6b06a706c0d9da5e52532a303", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9088, - { - "block": 2905580, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:48", - "trx_id": "293a696e85805bea591807c22d8da379fdda0fe0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9089, - { - "block": 2905581, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:51", - "trx_id": "e17b6d490dcde54f022aada5860ddcc07a416f53", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9090, - { - "block": 2905581, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:51", - "trx_id": "ed3dbdc55edec6ad89dae7d564df4aa9fd898065", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9091, - { - "block": 2905582, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:54", - "trx_id": "a3379f00e0bbf7082bd517823edb09b5da299db8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9092, - { - "block": 2905583, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:11:57", - "trx_id": "ae27615d3dfd6a653b7b92fc4100247917f82d89", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9093, - { - "block": 2905584, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:00", - "trx_id": "7ed969d47abfb48d6879a4a3a511a9f3a5355f59", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9095, - { - "block": 2905584, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:00", - "trx_id": "f3d92e947d8c7aea6cde3bdce371201799137cc7", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9096, - { - "block": 2905585, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:03", - "trx_id": "38fbd62bbd26ff6d468211675bc8a886736b0288", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9097, - { - "block": 2905585, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:03", - "trx_id": "66ac521acfa260657c06df7b382c689ab149819a", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9098, - { - "block": 2905586, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:06", - "trx_id": "e25bfaef8101bdbe7ae9e913a4d9e21522c9766a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9099, - { - "block": 2905586, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:06", - "trx_id": "bee606c7de7e5151ee292aeec5ff2a025896d99d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9100, - { - "block": 2905587, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:09", - "trx_id": "3e2c93ad75974c9adeb77a412da30d5f5b5dcb2f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9101, - { - "block": 2905587, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:09", - "trx_id": "b44abda2367da0f284896bfcaf55319dcd8a5e52", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9102, - { - "block": 2905588, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:12", - "trx_id": "d2884b818446f346db4e11862de39a9a5261852d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9104, - { - "block": 2905588, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:12", - "trx_id": "721c76efc70dca7293a27b5bc9142807f82f8fb4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9105, - { - "block": 2905589, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:15", - "trx_id": "04cf5b6b8ca7e472bd05454b0eea8870570d0594", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9106, - { - "block": 2905589, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "80396", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:15", - "trx_id": "629c3bad2451a69facb52b67894e3c78004c317d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9110, - { - "block": 2905590, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:13:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:12:18", - "trx_id": "c340699845957d0d7d89dee9507cabffb0fae55e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9114, - { - "block": 2905610, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:14:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:18", - "trx_id": "68f102aaad49dc4ea8bfd109234b95e92280e556", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9116, - { - "block": 2905611, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:21", - "trx_id": "49e4c5db1bf145dcc6748a9b277ce546d8029d42", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9118, - { - "block": 2905611, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:14:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:21", - "trx_id": "90a00e0814598121e308f41c3bba58ab22715912", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9120, - { - "block": 2905612, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:24", - "trx_id": "6f6337a8593072e78538f594a98e02ae0668fe50", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9121, - { - "block": 2905612, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:14:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:24", - "trx_id": "3bc5e8171adbf93e8bd5f43a675d54d6d5162bc7", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9122, - { - "block": 2905612, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:14:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:24", - "trx_id": "f28ee5401abd428f05bfe32f8d537e78bab1bab7", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9123, - { - "block": 2905613, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:27", - "trx_id": "d15e3a3ebfbbeb725ac1bfe79dfdda687bbeeec9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9124, - { - "block": 2905613, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:14:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:27", - "trx_id": "dc9a97bb7313406bbaa7911f9d10e5ecc527b77a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9125, - { - "block": 2905614, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:30", - "trx_id": "a561d6dd8663280fd3ecdb0f761db5e107244094", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9126, - { - "block": 2905615, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:33", - "trx_id": "0d2ab015a8b1c51ec751b1bea4de5c7aa95f9f58", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9127, - { - "block": 2905616, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:36", - "trx_id": "0d832871a1f48da03d2b46ba78c52770682e781b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9128, - { - "block": 2905616, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:36", - "trx_id": "b40d7485a748f67c657df1fb695028503dd048d9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9130, - { - "block": 2905617, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:39", - "trx_id": "7bf5083e4203d0f45c3c64ca78ff5a816d387b98", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9131, - { - "block": 2905617, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:39", - "trx_id": "05125cecfe12dca75f130f087b8692cce7ad70d1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9132, - { - "block": 2905618, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:42", - "trx_id": "55c4a0b4e371f0507fb31e1c540efb307dc6b268", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9133, - { - "block": 2905619, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:45", - "trx_id": "a3e37876123bd09053ad0d253e27ff94a3580681", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9134, - { - "block": 2905619, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:45", - "trx_id": "88dac0182bf67c69f6f9f122b1e338dea5cf232a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9135, - { - "block": 2905619, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:45", - "trx_id": "bd7fdde7d1b9ce83c9565807eda0d95cc36ef9f2", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9136, - { - "block": 2905620, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:48", - "trx_id": "9dd649f74facf35cf865b04a1065f468043840ae", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9137, - { - "block": 2905620, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:48", - "trx_id": "1e7a1bf76089cbb6d0531228578d021215cb5cd6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9138, - { - "block": 2905621, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:51", - "trx_id": "f0579821dff5ece166883aa13bac01c74949ab0d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9139, - { - "block": 2905622, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:54", - "trx_id": "533b983af6bab8ad10d0689063f68bca59603f6d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9140, - { - "block": 2905622, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:54", - "trx_id": "876ea6a2afd15eac680667f29bd85ed0718d9d19", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9141, - { - "block": 2905623, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:57", - "trx_id": "e86f68c489c7ce4b6e89f377df6246afe81a9c4e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9142, - { - "block": 2905623, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:57", - "trx_id": "d76367736d5ce43d91dd6cc4421d57b070b3c217", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9143, - { - "block": 2905623, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:13:57", - "trx_id": "654b5004159200485b2cab07cbedfae8dd39bc6d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9144, - { - "block": 2905624, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:00", - "trx_id": "b8a6e3005c6be40844029c50758b1d7118a7e3cf", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9145, - { - "block": 2905625, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:03", - "trx_id": "73a959feec5ca753328b9cd14b6c167182e9f799", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9146, - { - "block": 2905625, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:03", - "trx_id": "4706d2d0f072daa3bea71c1fa2da43ab87d6afa7", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9147, - { - "block": 2905625, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:03", - "trx_id": "faab2ae99c68642b9db36dcc201cfab6c78feac3", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9148, - { - "block": 2905626, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:06", - "trx_id": "8b539a1548f8c31e046851038ff54497410c01da", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9149, - { - "block": 2905626, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:06", - "trx_id": "28b4d050b785feb16ff24b3011f5acb6c00ec63b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9150, - { - "block": 2905627, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:09", - "trx_id": "46dcae2924d97fb6ccd1c9a481ec925e1c66a241", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9151, - { - "block": 2905627, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:09", - "trx_id": "af06edb29e1511aeecaace18eee8bbf0b3a28038", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9152, - { - "block": 2905628, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:12", - "trx_id": "92cb127f558a3c6bd82e53f61a0a48bed4e381ff", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9153, - { - "block": 2905628, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:12", - "trx_id": "f67569a18af3ce41428d6b51c22bd2fcd0e47fe6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9154, - { - "block": 2905629, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:15", - "trx_id": "a8e14fec6586c1b55feacc46524cf45e8188283d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9155, - { - "block": 2905629, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:15", - "trx_id": "e43cdd6f96ab121f5394252ed10326a78b416c87", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9156, - { - "block": 2905630, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:18", - "trx_id": "39b377dbce913b6018fed7bf01f9f88de4094bf2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9157, - { - "block": 2905630, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:18", - "trx_id": "6a1c1cf8abc5c46f3ef395db6f968c00d0f60de8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9158, - { - "block": 2905631, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:21", - "trx_id": "997e1f5d6b2f8c1ed200615ab0fc10562b592e15", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9159, - { - "block": 2905631, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:21", - "trx_id": "ce0aada0c21915a6da2cd3b0efc4466d4d33f412", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9160, - { - "block": 2905632, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:24", - "trx_id": "2a2e7b9bc0278c873c6fb3f62893a043872264c7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9161, - { - "block": 2905632, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:24", - "trx_id": "c3cbec377dcf427cec4f8f6942c2acee28da1426", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9163, - { - "block": 2905633, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:27", - "trx_id": "2172a4afda9d61fce9c2e1bd424eb0c55448f9f8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9164, - { - "block": 2905633, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:27", - "trx_id": "e1a00b3a2ead41c40aff1953ad0c5b954f049919", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9165, - { - "block": 2905633, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:15:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:27", - "trx_id": "98d37f15952ea3ded242f487bac265896173e993", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9167, - { - "block": 2905634, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:30", - "trx_id": "55b270f59e4d29d032698f87ab321feed7ac21dd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9169, - { - "block": 2905635, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:33", - "trx_id": "c9bc624c55d467e1c2a49e59fa44faf02e5435a1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9170, - { - "block": 2905635, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:33", - "trx_id": "5bb9feef5ffb9af083ce2c22367ab4b7bcd50278", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9171, - { - "block": 2905635, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:33", - "trx_id": "f5355e1917f04a6d17efd9e61e69e3e45b4fbd43", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9172, - { - "block": 2905636, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:36", - "trx_id": "50ffe8bfa32eef28ebda8ea515e94f799dbdc5ef", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9173, - { - "block": 2905636, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:36", - "trx_id": "b0cbc00855a923ca3fb2866540b0a4e11bde2076", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9174, - { - "block": 2905637, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:39", - "trx_id": "16304d9751c0e8b3bf25bbabb171738fc81d0486", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9175, - { - "block": 2905637, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:39", - "trx_id": "2e9cdb1b853143c484b546e0bebba64a2b430be6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9176, - { - "block": 2905638, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:42", - "trx_id": "60fa2ec146ff17cfa99d0656f238feb93d7a9ccf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9177, - { - "block": 2905638, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:42", - "trx_id": "3683f6e666d7e24ebf58e05e85129332dbfaf95a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9178, - { - "block": 2905639, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:45", - "trx_id": "b7235046fb4b02dffd4f358e2f64db1c37161d54", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9179, - { - "block": 2905639, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:45", - "trx_id": "4326f8b728469f5dceda44fd75cb00121ccfe621", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9180, - { - "block": 2905640, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:48", - "trx_id": "bb053db9bc36c208cba44cafab76fd9b1cacf89b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9181, - { - "block": 2905640, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:48", - "trx_id": "0b033161f6d58ec25e9296418c9bffe6b2cfe741", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9182, - { - "block": 2905641, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:51", - "trx_id": "7ab9e731b351c020ec3f16257d914d5d2e02d3a6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9183, - { - "block": 2905641, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:51", - "trx_id": "ced06ee8893c526ea1e9ae21c0f7e4301087f14c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9184, - { - "block": 2905642, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:54", - "trx_id": "cd431feea46fc5e96e0168da66d29d4ad97d3a54", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9185, - { - "block": 2905642, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:54", - "trx_id": "17f5959d42ab0a07dbfe0bda13876c782562765b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9186, - { - "block": 2905643, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:57", - "trx_id": "431910e4a0321c509aa88a533b1977a60435d115", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9187, - { - "block": 2905643, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:14:57", - "trx_id": "420289b7345246d07b849db0b8f2b06f797a72d5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9188, - { - "block": 2905644, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:00", - "trx_id": "e8f808e37921caed1aa2bb5004b644071b6a5a18", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9189, - { - "block": 2905645, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:03", - "trx_id": "3804a2c2b9c8c896b5be07ef847f88736d7e5231", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9190, - { - "block": 2905645, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:03", - "trx_id": "d96750b1b05288d93739a09f502bf527562498ea", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9191, - { - "block": 2905645, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:03", - "trx_id": "f20fc5003c4a1578099b79a86ca9fe5f22710c7f", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9192, - { - "block": 2905646, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:06", - "trx_id": "78759e0eb12601dc77c44d45514bdb4a6f483e13", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9193, - { - "block": 2905646, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:06", - "trx_id": "dc65f88f0f109fe904295518a000fefc06977f95", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9194, - { - "block": 2905647, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:09", - "trx_id": "a6e13272438f22c9d84171912097941287735089", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9195, - { - "block": 2905648, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:12", - "trx_id": "2c00cde5624d97d330735e80beaecb54cdb7144c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9196, - { - "block": 2905648, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:12", - "trx_id": "ba61cbf09ebd4ac87786cbd5e0f2c90c7013094c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9197, - { - "block": 2905648, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:12", - "trx_id": "5dddb865fdb57e7dde19c7cf146b5143e54fa604", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9198, - { - "block": 2905649, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:15", - "trx_id": "e0982117e4fee9b86bc47528c475049cbefddabf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9199, - { - "block": 2905649, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:15", - "trx_id": "4fc016ff322f90e1c5998dc7248a0bb03e1eb548", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9200, - { - "block": 2905650, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:18", - "trx_id": "db895382061df7785f0ad6d2d4161532512bcf40", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9201, - { - "block": 2905651, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:21", - "trx_id": "c9be7175dd613bfc9d1a52b0993289541f7616ae", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9202, - { - "block": 2905651, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:21", - "trx_id": "89f37f62070ea976d3b7e44fd04330e33d558b3a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9203, - { - "block": 2905651, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:21", - "trx_id": "432fe4deed9e142fdcafa3b496bd54728091e4f4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9204, - { - "block": 2905652, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:24", - "trx_id": "c5b864e38c07761c1eecadf002340913a6782415", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9205, - { - "block": 2905652, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:24", - "trx_id": "b68fca441b08641f7876cea942da675ed43ab269", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9206, - { - "block": 2905653, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:27", - "trx_id": "da88e9be3638b270277c87180c972604b683d64e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9207, - { - "block": 2905653, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:16:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:27", - "trx_id": "847c89bbf243e329aac7c43301e810e41537835f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9209, - { - "block": 2905654, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:30", - "trx_id": "b3882904e1c9db2366e6fa5dc2b4ea0aa4530ea2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9212, - { - "block": 2905655, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:33", - "trx_id": "27cb8283217645df413b027da72c1f26ce52f87c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9213, - { - "block": 2905655, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:33", - "trx_id": "25b22cb3f3a9b62b240366137bd852b38437a150", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9214, - { - "block": 2905655, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:33", - "trx_id": "9f194d85cd468dc98e3c8f4ba342b39bedb1c41f", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9216, - { - "block": 2905656, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:36", - "trx_id": "58b22c032236405eddb7c2718d47879300c1770f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9218, - { - "block": 2905656, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:36", - "trx_id": "09ba30e6dbeaaffc9e8a97d8d1461ade836286fe", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9219, - { - "block": 2905657, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:39", - "trx_id": "f61219924ca0213da0ff774fa580bfebdfd07a41", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9220, - { - "block": 2905657, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:39", - "trx_id": "6f2b516556e70a66b03b9852673848d6f0769cc2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9221, - { - "block": 2905657, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:39", - "trx_id": "5e93f7933956e9b11b98f8ac88874fbbb59f4603", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9222, - { - "block": 2905658, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:42", - "trx_id": "44bd3d27c59b95512f4191606f1a499ece003879", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9223, - { - "block": 2905659, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:45", - "trx_id": "5e2f9aea3d97d76ea9d467e8f7b253ab5b8770d2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9224, - { - "block": 2905659, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:45", - "trx_id": "4317ee9fbf7d704cc3b19431b1e36219cdadc2c4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9225, - { - "block": 2905660, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:48", - "trx_id": "ad8ae3a50fecf7b9ce26196bc9864fdefff823c5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9226, - { - "block": 2905660, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:48", - "trx_id": "6d6bf2bcdbf599a2c4636fa114570ce2412fe690", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9227, - { - "block": 2905660, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:48", - "trx_id": "597c587a35e39cae962841ec35805bdd3c231adb", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9228, - { - "block": 2905661, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:51", - "trx_id": "c08dd73578afe42f938c2dbb5f2b3acdcaa061e3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9229, - { - "block": 2905662, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:54", - "trx_id": "cf935dc29036a48636470e37e29716ff02ce27d2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9230, - { - "block": 2905662, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:54", - "trx_id": "ba79ecc901e5887028f0e10f3c1c494bfe11db6e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9231, - { - "block": 2905663, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:57", - "trx_id": "9b9c9e99519b156cb3864d15bb4e3fabf823bfe6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9232, - { - "block": 2905663, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:15:57", - "trx_id": "234b188dcfd3f60e90d88ec7d895052d63dc9ce1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9233, - { - "block": 2905664, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:00", - "trx_id": "37a86429cd6be4781dfa64b3cb0b8d647dbf6ce2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9234, - { - "block": 2905664, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:00", - "trx_id": "810ae2760fb23286017272afadeebb106d14a241", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9235, - { - "block": 2905665, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:03", - "trx_id": "09f24808a92071e468adee65b877c5e79b42a31f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9236, - { - "block": 2905665, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:03", - "trx_id": "f9166438a30116d705ddb2551173513c5d71e85a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9237, - { - "block": 2905665, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:03", - "trx_id": "8e075bfd9a34bd0d6f16970e37522169f773aeb8", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9238, - { - "block": 2905666, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:06", - "trx_id": "94fb73239caa7741415ea2905982839a45137cfb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9239, - { - "block": 2905666, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:06", - "trx_id": "ff6d35adbd271f16899a6d0cb3b96274e8405d35", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9240, - { - "block": 2905667, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:09", - "trx_id": "ee7005f5f428d414a6ad7c6873f9cd028868f102", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9241, - { - "block": 2905668, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:12", - "trx_id": "6b9ef54ee270c6b3da4e9f045329437a1f28f265", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9242, - { - "block": 2905668, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:12", - "trx_id": "79c0ac1ef8b6a0884d42f8f7cf70ba0c94e42498", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9243, - { - "block": 2905668, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:12", - "trx_id": "cc9474b25886c2459ac0f23febc0d9843c3ed035", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9244, - { - "block": 2905669, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:15", - "trx_id": "73c275a9d2eef5429f768d3b2b223cb994eeb032", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9245, - { - "block": 2905669, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:15", - "trx_id": "cbe927a05f5773aa50ea2660b9982ffd0c19395c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9246, - { - "block": 2905670, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:18", - "trx_id": "b0cd84fd52bbd95d57658e42c494d10be51b00bb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9247, - { - "block": 2905670, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:18", - "trx_id": "14432fc4eb7d293ca4bfcfa94b3d3430a58f2393", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9248, - { - "block": 2905671, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:21", - "trx_id": "c3f5de72ac0b4db5f1528bb85c9bf6844f452835", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9249, - { - "block": 2905671, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:21", - "trx_id": "11703dc64be5a9050f610ea6d4aa314c571480e5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9250, - { - "block": 2905672, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:24", - "trx_id": "5ba345cfd9842a515a7caf0240ec41081fe6a7fd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9251, - { - "block": 2905672, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:24", - "trx_id": "ac4ff263480acea9c81d536845105231164c670b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9252, - { - "block": 2905673, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:27", - "trx_id": "3f67df4e7a0da78e9b05fee033e0429c7e9d9c6f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9253, - { - "block": 2905673, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:17:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:27", - "trx_id": "1b9c6c83f93f10cc4f16139494658b0626447b90", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9254, - { - "block": 2905674, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:30", - "trx_id": "ce63195f5e815874cdcb7e2aa6a0d69aa3e6cf69", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9256, - { - "block": 2905674, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:30", - "trx_id": "6ba3627050322026c2ffbef33763ee7650e7beeb", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9257, - { - "block": 2905675, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:33", - "trx_id": "17e29aa5a1d428bb85dbaa6d0339a192faaa5978", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9258, - { - "block": 2905675, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:33", - "trx_id": "00c615f853c50efc3c771293ed3067d88761771f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9260, - { - "block": 2905675, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:33", - "trx_id": "61aa1fc365f00fc9a553d30e623b2638d3e9c609", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9261, - { - "block": 2905676, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:36", - "trx_id": "07204e4415588604a3e85e77adce1a158229daec", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9262, - { - "block": 2905676, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:36", - "trx_id": "cb7911cab76bf811a2558e8309a51dddd9707e86", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9264, - { - "block": 2905677, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:39", - "trx_id": "d0372469092a01e6aeb8f97f06d17e2f18f0ef4c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9266, - { - "block": 2905677, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:39", - "trx_id": "c65ce02ed58533ff734ff005cd6b03e3877b93eb", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9267, - { - "block": 2905678, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:42", - "trx_id": "704f7189e0e131af303d19d63627fcf74381cd68", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9268, - { - "block": 2905678, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:42", - "trx_id": "d5200b5dab0673be4a5664480d7c687637fca98e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9270, - { - "block": 2905679, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:45", - "trx_id": "4da8c4f8944cf084cac0c6f483a0374fff79f9cc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9271, - { - "block": 2905679, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:45", - "trx_id": "e93d1b095942d31fcc560fce35cc6a4872b25ec1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9272, - { - "block": 2905680, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:48", - "trx_id": "5c40e6377e26be4a67eca4dbae4908f2682f4e4b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9273, - { - "block": 2905681, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:51", - "trx_id": "56e5561b390aa5e3823288e4b4f7ca41c06b929e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9274, - { - "block": 2905681, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:51", - "trx_id": "0f2bdd215c6afbbfcc925e446a2382098ab02f50", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9275, - { - "block": 2905681, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:51", - "trx_id": "6ed3e632392fbe20555a5527afe5e726302fc592", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9276, - { - "block": 2905682, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:54", - "trx_id": "4fd6e7287f6d29094b8b7cb06794bd97626bfccf", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9277, - { - "block": 2905683, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:16:57", - "trx_id": "6e783010eb01a9678ff723a5e54de2b1a252b1d4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9278, - { - "block": 2905684, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:00", - "trx_id": "035bfd30247a1e5e9f83726b29cd773545edf94b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9279, - { - "block": 2905684, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:00", - "trx_id": "df6eb3b599becaae8cec0db0b3a511d670d1b9e6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9280, - { - "block": 2905685, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:03", - "trx_id": "fdd6fb80bfa0eb7f7d784a3b651d2a3b2a8b5ac9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9281, - { - "block": 2905686, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:06", - "trx_id": "47ea5cafb166f7ef10fc384315a2dc11029285ba", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9282, - { - "block": 2905686, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:06", - "trx_id": "26dfbf0b1015c9d93376d8842e7d381841cb664a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9283, - { - "block": 2905687, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:09", - "trx_id": "b5160724c2ad87c993f80fe934d0a78c565de3d8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9284, - { - "block": 2905687, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:09", - "trx_id": "2952d2cd1284d7a5810d53196c7bfdf1c6722e69", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9285, - { - "block": 2905687, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:09", - "trx_id": "a6dc6ed049779607f8991b6aa28909dfccc3d700", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9286, - { - "block": 2905688, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:12", - "trx_id": "1ccfd243df1740c481902b576749075f62d3fd08", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9287, - { - "block": 2905689, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:15", - "trx_id": "ff11f5a587bcd5e2dbe2aaa3749ea073126a7104", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9288, - { - "block": 2905689, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:15", - "trx_id": "1c9463003d9143881cae5296b5157e96e9653f18", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9289, - { - "block": 2905689, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:15", - "trx_id": "88840d87e74b32ec5a1cba5e843ec32f750e17d4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9290, - { - "block": 2905690, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:18", - "trx_id": "a4a2a5e0918f862964cc4afb4b12aa98fd7b56e8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9291, - { - "block": 2905690, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:18", - "trx_id": "d92b7f9e36ef6d4be759054460fafb5c7d8d2e26", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9292, - { - "block": 2905691, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:21", - "trx_id": "c9b99dc2dc2f4821c628f095ee226233a7774fd4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9293, - { - "block": 2905691, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:21", - "trx_id": "29d26106702250aec56ea784d3abc26ab1cd3fac", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9294, - { - "block": 2905692, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:24", - "trx_id": "922ef87a99ff17dd8b50520d30895635be6610ac", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9295, - { - "block": 2905693, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:27", - "trx_id": "580d19f2b072df7fd75f0286713234d4dde08f30", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9296, - { - "block": 2905693, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:27", - "trx_id": "4c65638903c51b9f4732bbe0ca2e0dd0ee4ce4ae", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9297, - { - "block": 2905693, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:18:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:27", - "trx_id": "33dcd9ccdea6c1b59ed10ddc80915e52fd6e36f2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9298, - { - "block": 2905694, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:30", - "trx_id": "59bd1434527d73c5954891ed90027666fb106585", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9300, - { - "block": 2905695, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:33", - "trx_id": "a8bd24236d291ca823249b4476a7c135e85e5608", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9301, - { - "block": 2905696, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:36", - "trx_id": "eb321bbe761765bc0c15aacc46c72d2756ff701c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9303, - { - "block": 2905697, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:39", - "trx_id": "9a21f4bbfbbcfdea75c817909ba8b2411a2e0dac", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9305, - { - "block": 2905697, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:39", - "trx_id": "c1383be2e94b705b2764fd2c492d0ca4801dc901", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9306, - { - "block": 2905698, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:42", - "trx_id": "49dd9a95ff74e76eba16bb7183cdc0be5894a9fb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9308, - { - "block": 2905698, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:42", - "trx_id": "bb86550fbad332edfdaa689f8d55d4b923666290", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9309, - { - "block": 2905699, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:45", - "trx_id": "68520987ce91a01d589dd9cd964ab621ea3bb024", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9310, - { - "block": 2905699, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:45", - "trx_id": "425316750a76943cc1a5ca03917191a57aee4dc3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9311, - { - "block": 2905700, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:48", - "trx_id": "a8cfbc566e90bb68ff540160c2b2d8fde58d7f3f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9312, - { - "block": 2905700, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:48", - "trx_id": "3be05cabf6574b0efe739b38a53e6fcfe34fc554", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9313, - { - "block": 2905700, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:48", - "trx_id": "dcadc0ee4e6e6b293475be564da97f03bbf9985f", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9314, - { - "block": 2905700, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:48", - "trx_id": "3d82dfae68f2168e2e7b2c7d202ca84eb60f7b9c", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9315, - { - "block": 2905701, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:51", - "trx_id": "aa16016b3eb0d367d0553fa2f25cd4aa6e83dcfb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9316, - { - "block": 2905702, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:54", - "trx_id": "9993120b9aad04356d3edf103e92b120a21079cf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9317, - { - "block": 2905702, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:54", - "trx_id": "b5b219c42f61989a140af9542a03f5a9f1150eda", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9318, - { - "block": 2905702, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:54", - "trx_id": "b60f733f263fbb4ba7c81b48d5bcdeaf673348e2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9319, - { - "block": 2905703, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:57", - "trx_id": "df9e9f37b4b1d4dd389895fea2968c9af9d6e11c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9320, - { - "block": 2905703, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:17:57", - "trx_id": "b6de1208f4c93b8a7ea25208cb2ec3847efa3b6e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9321, - { - "block": 2905704, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:00", - "trx_id": "07d0e9b7e197461d558c7ef8320a16022f2483b9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9322, - { - "block": 2905705, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:03", - "trx_id": "16aea48df727ccea3e430665b01016d1c509b39a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9323, - { - "block": 2905705, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:03", - "trx_id": "c38efd497268e1aece820478803a5ce99e6cb490", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9324, - { - "block": 2905705, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:03", - "trx_id": "2595db38159e92bb4bd12be2269c9488684afa39", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9325, - { - "block": 2905706, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:06", - "trx_id": "3997040cc3db87ef87796231a11535af8e0373ed", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9326, - { - "block": 2905706, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:06", - "trx_id": "6d1cc167bcb360f4d002cb27318eed7925bb0b61", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9327, - { - "block": 2905707, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:09", - "trx_id": "4167b9cc834be81e772c8aad3b31212da24c803f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9328, - { - "block": 2905707, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:09", - "trx_id": "d650200af7624f1f5d6bc3f9deeaa6f95f9293b0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9329, - { - "block": 2905708, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:12", - "trx_id": "66b55691bd708ce2d02c19c95f828477695eab74", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9330, - { - "block": 2905709, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:15", - "trx_id": "759973dc90045b0e3c9a08935789f29cfd77f702", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9331, - { - "block": 2905709, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:15", - "trx_id": "9e3caf771f691cfe5c4e0eb4cd80c882f2494aff", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9332, - { - "block": 2905710, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:18", - "trx_id": "42b5a8767f83740f764145fee20ffcc94b106b86", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9333, - { - "block": 2905710, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:18", - "trx_id": "6221d72c767008f7f35acc9c896728e6ab3cc766", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9334, - { - "block": 2905710, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:18", - "trx_id": "d13430b64c72d812833828ee7e2d763b936426d8", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9335, - { - "block": 2905711, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:21", - "trx_id": "e93d8391f78345eca75973e3e7eaad7c13e22999", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9336, - { - "block": 2905711, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:19:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:18:21", - "trx_id": "56bf034b7b30caa282f4c69b914fc76dcd1e66fa", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9337, - { - "block": 2905753, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:21:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:20:27", - "trx_id": "f723f41aa2ba54aa40a57a10a23fa1355df47978", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9338, - { - "block": 2905753, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:21:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:20:27", - "trx_id": "c98066e862cb1fa5b463179c39c026b2a913770f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9339, - { - "block": 2905754, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:20:30", - "trx_id": "055a2907330fa4f73677c6441ac258c4373957b9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9340, - { - "block": 2905754, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:22:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:20:30", - "trx_id": "ba3deeead92b4e966af27c9c436b5374a4aafd0a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9341, - { - "block": 2905755, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:20:33", - "trx_id": "116e4b612206ce6b0bd7cad02e137251a18793ff", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9342, - { - "block": 2905755, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:22:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91997", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:20:33", - "trx_id": "13f9f7a6286a7d72fa6a81f975f6e268b42cee3c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9344, - { - "block": 2905774, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:23:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:21:30", - "trx_id": "894adfb205ee761b9687f80b6ad7521bacf6ddeb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9349, - { - "block": 2905786, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:23:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91997", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:22:06", - "trx_id": "866e2d02ab982a44f9a21a5f49bfe7755bf15002", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9350, - { - "block": 2905805, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:24:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:23:03", - "trx_id": "3ffa7045354e18565ac481090227ddfafafa79a2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9351, - { - "block": 2905810, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:23:18", - "trx_id": "86ee104285620ec12249d903610ba4c4a03451e4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9352, - { - "block": 2905810, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:24:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91797", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:23:18", - "trx_id": "29c26cd0ea62d362d5e86c7ef03ccbfa14b45a08", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9354, - { - "block": 2905836, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:26:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "498740", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:24:36", - "trx_id": "8df48e6ebb9f32e8d75c0db04f438f72d4c6deec", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9355, - { - "block": 2905841, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:26:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91797", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:24:51", - "trx_id": "0c1475848664254edc967590898aa8825692d894", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9356, - { - "block": 2905848, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:25:15", - "trx_id": "5991ad06e6faf10f905a31ea78ce2c1e606e5d97", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9357, - { - "block": 2905848, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:26:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "490184", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:25:15", - "trx_id": "0d09cd186287e3ddc3932156d75238122076220e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9358, - { - "block": 2905871, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:27:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91797", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:26:24", - "trx_id": "0f555be3f8a3eb6df088dcf4d69d7201ecf8b352", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9360, - { - "block": 2905874, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:28:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91997", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:26:33", - "trx_id": "bbfe9a99c9df29c641af3e4ba23de6344b50f946", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9361, - { - "block": 2905879, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:28:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "490184", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:26:48", - "trx_id": "77cd042ffb6c370a55ec3aca2daf4b7bdeaffc65", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9362, - { - "block": 2905905, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:29:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91997", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:28:06", - "trx_id": "8f47ca0dbf9e410c5255c3c2c912826f03b598a0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9363, - { - "block": 2905910, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:29:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "490184", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:28:21", - "trx_id": "538e4e70cbf604452dc18558b01d57aec7c849d1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9364, - { - "block": 2905936, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:31:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91997", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:29:39", - "trx_id": "3ae8e5e9a174ccfb163e1320b81ded4709c59c5f", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9365, - { - "block": 2905941, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:31:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "490184", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:29:54", - "trx_id": "5182c10d3b5749b95bc6c5f15fd082bf219f54b1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9366, - { - "block": 2905967, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:32:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91997", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:31:12", - "trx_id": "d6e48ad9bdd4229d7633c268d27d4648c2d1b8f6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9367, - { - "block": 2905972, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:32:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "490184", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:31:27", - "trx_id": "4a8b53ace004ab92c733716a53efaedaa1dd4312", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9368, - { - "block": 2905982, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:31:57", - "trx_id": "0c505dd5a4a80d94593126c95fbf5ea9aba82312", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9369, - { - "block": 2905982, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:33:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "435957", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:31:57", - "trx_id": "931e1ff1b8f00a562113095bddd0ddc527ee937c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9370, - { - "block": 2905997, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:34:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91997", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:32:45", - "trx_id": "c506d65614e0daf5ca4c0bc629c23fb55c691b9e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9372, - { - "block": 2906000, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:34:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91997", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:32:54", - "trx_id": "6ec6515792983570c49e9cf22a54f13efaddc909", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9373, - { - "block": 2906012, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:35:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "490184", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:33:30", - "trx_id": "a650e586c70fb2bd6377ba8b55219284cf4ee143", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9374, - { - "block": 2906031, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:35:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91997", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:34:27", - "trx_id": "5e306ff77cafb54ec4cb11f00ceb84f6238e8741", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9375, - { - "block": 2906043, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:36:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "490184", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:35:03", - "trx_id": "4705445d1b9470682093d2abac4d037a14cba63f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9376, - { - "block": 2906062, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:37:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91997", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:36:00", - "trx_id": "4ff11651a43cb9c777fcfcd6e1e01ad55369474a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9377, - { - "block": 2906074, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:38:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "490184", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:36:36", - "trx_id": "403a7d6daf1ad8d8a72d0682188ceddd604714d1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9379, - { - "block": 2906080, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:36:54", - "trx_id": "1114798151fc1007579c18d32fdcb37b6e99eca9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9380, - { - "block": 2906080, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:38:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91797", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:36:54", - "trx_id": "50461064d32e088e540e6b7f2f3d510c67c7c7ed", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9381, - { - "block": 2906105, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:39:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "490184", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:38:09", - "trx_id": "f8c3dbab2285ebab93f0ac5d68eb926c7cf75fdf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9382, - { - "block": 2906111, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:39:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91797", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:38:27", - "trx_id": "a4a6b88973145e43d42d8fdaa56d5b862b07c4a3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9383, - { - "block": 2906136, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:41:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "490184", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:39:42", - "trx_id": "5ec7a692d2491ddddec3eed30acaa6f5dae01ae6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9384, - { - "block": 2906142, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:41:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "91797", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:40:00", - "trx_id": "4650f2c56394e4880719d478f0f048912f8658c2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9385, - { - "block": 2906148, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:40:18", - "trx_id": "a838077a9f521910d24ac0cf3b05df418a733d65", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9386, - { - "block": 2906148, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:41:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "89998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:40:18", - "trx_id": "39bbb2250e71a913629a0ce603549ade90dd24cb", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9387, - { - "block": 2906156, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:40:42", - "trx_id": "8c46d88b9249874ecdc046fb896bd259afdaf6c8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9388, - { - "block": 2906156, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:42:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:40:42", - "trx_id": "cec64da0bf5129caccb37dff4896df63c44eb7e6", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9390, - { - "block": 2906178, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:43:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "89998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:41:51", - "trx_id": "dedb0b159d1329684d7db7b5349defdb6bdaf7a8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9391, - { - "block": 2906186, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:43:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:42:15", - "trx_id": "5b237a3e4ef2db9628c29505dd1b6e61d9f9c132", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9393, - { - "block": 2906209, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:44:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "89998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:43:24", - "trx_id": "1ec227793e19100d63138a155eb5cfa4e899e1f6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9394, - { - "block": 2906217, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:45:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:43:48", - "trx_id": "9e9bc367044015fe0ab13556c77f685b248d68f5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9396, - { - "block": 2906228, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:44:21", - "trx_id": "647dc7a702031c067313755e147f1e382ede1cef", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9398, - { - "block": 2906228, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:45:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "89998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:44:21", - "trx_id": "c4441c1fae17aec96ac4ccded364ff9852705fcb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9400, - { - "block": 2906228, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:45:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "444508", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:44:21", - "trx_id": "3dc1d64d938a513138128f4c6eb77ccb6588b274", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9402, - { - "block": 2906229, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:45:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "89998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:44:24", - "trx_id": "ccedd1a435c4aa198845dcba9f221c26491c091a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9406, - { - "block": 2906230, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "111539", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:45:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "50191", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:44:27", - "trx_id": "f3cd3501d24971a0596c5e88045ff866e1e61f69", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9409, - { - "block": 2906231, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "74872", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:46:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "33691", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:44:30", - "trx_id": "498f07c3392d7a9d1767fee286c670e326f41732", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9413, - { - "block": 2906259, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:47:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:45:54", - "trx_id": "5ba9365da7d3e98ec221e69b243c4abc72cca24b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9414, - { - "block": 2906262, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:47:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "89998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:46:03", - "trx_id": "03c0384144abbc9e0c0059628d37301b61693059", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9415, - { - "block": 2906290, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:48:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:27", - "trx_id": "34972791735dee2510b44eccf9674b8708f3a3b1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9416, - { - "block": 2906293, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:36", - "trx_id": "5042716c6c5911b400d5eebef309924c3380cda6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9417, - { - "block": 2906294, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:39", - "trx_id": "34be97b3afd34357d9b1a436703c3665f41db9cd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9418, - { - "block": 2906294, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:39", - "trx_id": "e054f1d8c1651c2af248349a5d214fea35fcb929", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9419, - { - "block": 2906295, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:42", - "trx_id": "bd8aa74543bcba16dd6ce98506ae76d1df82c898", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9420, - { - "block": 2906295, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:42", - "trx_id": "6c06271154f03dcc67c9119d0fe79313d226e8a4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9422, - { - "block": 2906296, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:45", - "trx_id": "8ba0d813d2daf9e3ea30db182ddf8beb09de93a0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9423, - { - "block": 2906296, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:45", - "trx_id": "8f998948f5adf6eddbf080b30b209285b27fbeba", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9424, - { - "block": 2906296, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:45", - "trx_id": "2422fa28264da4824a53c5706abc3056ea973d3d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9426, - { - "block": 2906297, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:48", - "trx_id": "7e6fd5520ab12ea923e1c36083a283419c7b94c9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9427, - { - "block": 2906297, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:48", - "trx_id": "56d909b03cf114608cad593740996549a5f68edf", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9428, - { - "block": 2906298, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:51", - "trx_id": "66575113e1c08ed0d9c14ad40ea9a5b858f7b51a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9429, - { - "block": 2906299, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:54", - "trx_id": "a6ad2a1a5ce447f213956ec494ade3084374739c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9430, - { - "block": 2906299, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:54", - "trx_id": "2603dfc16b15ce8e0017a98eaced84f2bfb365dc", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9431, - { - "block": 2906300, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:47:57", - "trx_id": "7fa71e9e667f0c37c42f066ba5bfe22554286e88", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9432, - { - "block": 2906301, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:00", - "trx_id": "c75be41afec01bf015ff4c21ab1b30e9f7e55347", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9434, - { - "block": 2906301, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:00", - "trx_id": "c926b43eecf3761828231912962ad2c0263d6876", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9435, - { - "block": 2906302, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:03", - "trx_id": "94d61fbba187958e0d793663a18eb367bd3fb9b1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9436, - { - "block": 2906302, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:03", - "trx_id": "e522db763d5bdf67b1d27598d04a5fc5397b57f6", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9437, - { - "block": 2906303, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:06", - "trx_id": "7b63a2a6b0ba710e4e92deea3b235074c9c741b1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9438, - { - "block": 2906303, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:06", - "trx_id": "5bdc18050440aae3bac8b87e93d3a657ba9202e8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9439, - { - "block": 2906304, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:09", - "trx_id": "d2e278d3345d3a69867e415300596fbde4fd0163", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9440, - { - "block": 2906304, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:09", - "trx_id": "1916676a55a857dc6f5a400b0f0cfc4ff94d49ca", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9441, - { - "block": 2906305, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:12", - "trx_id": "21594f122765cf6026ae16445d6f9f7f38b2158a", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9442, - { - "block": 2906306, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:15", - "trx_id": "5039b2bfd8214301acbefafab3d5915cffa004f4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9443, - { - "block": 2906308, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:21", - "trx_id": "512c59a467598f2a48e5b80062dba3e8bd0f78ec", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9444, - { - "block": 2906308, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:21", - "trx_id": "ad9051ec8d301b32d221ac99e594faa882295ab8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9445, - { - "block": 2906308, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:21", - "trx_id": "3d4e1fcb324b93055ec9b9f1cbf1a97d68611e12", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9446, - { - "block": 2906308, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:21", - "trx_id": "b7e144e7ce0b6fc0ffaf023418e4e604d9efefe3", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9447, - { - "block": 2906309, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:24", - "trx_id": "49e71a4c6c9a11dbcd51210829486745aff8e2bf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9448, - { - "block": 2906309, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:24", - "trx_id": "13c40eafc8be4c071c94c3eb52398a77805c042b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9449, - { - "block": 2906310, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:27", - "trx_id": "88ac4be32494eea53d83b666e59370e1c231a01f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9450, - { - "block": 2906311, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:50:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:30", - "trx_id": "25450599921c3641df045624bd208629102b9b09", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9451, - { - "block": 2906311, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:30", - "trx_id": "e9e3ee423dfc29d3f311648d2f7f3d6aae1fa116", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9452, - { - "block": 2906312, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:49:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:33", - "trx_id": "55cc7400ee70e00f1adc13482a71d8001db383fc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9453, - { - "block": 2906312, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:33", - "trx_id": "38b7ccbe6b74e1dac9d4ed2c33f794e3e91bf0d0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9454, - { - "block": 2906312, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:50:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "95798", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:33", - "trx_id": "dd12c926b920523091226db3b03a18eaa92f7f5d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9455, - { - "block": 2906313, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:36", - "trx_id": "33d1bb565897863322164b55c4edd8e724e6de43", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9456, - { - "block": 2906313, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:50:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:48:36", - "trx_id": "cab31b69388f147f4e61f3715d8392c559a7bc51", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9457, - { - "block": 2906323, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:49:06", - "trx_id": "9cdfbb7c32de07f440a334832491fa62c0807359", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9458, - { - "block": 2906323, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:50:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "90002", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:49:06", - "trx_id": "fff19677d446e149d535d31ebd71083cb8f10f9e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9459, - { - "block": 2906327, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:50:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:49:18", - "trx_id": "8cf5cf661379a4ec46234e694f70bd890050d0ce", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9460, - { - "block": 2906354, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:52:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "90002", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:50:39", - "trx_id": "3cc20315aeaeb4eb9280f62f2061e5aa6304b3b3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9461, - { - "block": 2906358, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:52:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:50:51", - "trx_id": "6ac3a1556c5175560ecd381198c827ca25b62894", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9462, - { - "block": 2906385, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:53:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "90002", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:52:12", - "trx_id": "55647cac060d219f442dcfa48a49e2d587ee32fc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9463, - { - "block": 2906389, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:53:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:52:24", - "trx_id": "7e1ade2cd294b1c886fb66f830f6e7a23a76bdec", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9469, - { - "block": 2906406, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:54:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "90002", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:53:15", - "trx_id": "a51e500ae0be7c86dfa8ecb98d841b6a9ff2b499", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9471, - { - "block": 2906410, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:54:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:53:27", - "trx_id": "79f17555d284e3fae848f0d0ecc01e9fc7178b91", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9475, - { - "block": 2906427, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:55:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "90002", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:54:18", - "trx_id": "96ba667f4456c15b1d4d0fcf970bfc6c25c6e5d4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9477, - { - "block": 2906431, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:56:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:54:30", - "trx_id": "ceb564f63e60f8bfcdadccc91cb552e52b06da81", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9480, - { - "block": 2906448, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:56:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "90002", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:55:21", - "trx_id": "b3fb590164068cb15636b7db0928e1b151713241", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9482, - { - "block": 2906452, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:57:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:55:33", - "trx_id": "8c7c044a09d6da6f4afff2c434e10b90c44e8c23", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9487, - { - "block": 2906465, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:12", - "trx_id": "704eb202262d43775b4b72be2d1f8eaeda206a64", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9488, - { - "block": 2906465, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:57:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:12", - "trx_id": "c3a09acf3a421f226e558eea08b51f6e2210921b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9489, - { - "block": 2906466, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:15", - "trx_id": "53e307b8b7d199e0721610d9f31f4fdc61eea2eb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9490, - { - "block": 2906467, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:57:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:18", - "trx_id": "9a596e92694f366c8741575fc4ed199286377b94", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9491, - { - "block": 2906467, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:18", - "trx_id": "3a5af0a419ebadb29e7c0efb2797879a578dfe7d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9492, - { - "block": 2906467, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:57:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:18", - "trx_id": "8163f91c8478caa38c8ff00796d63b2e4351f55e", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9493, - { - "block": 2906468, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:21", - "trx_id": "87ccfe669465249c6464187c14ca8f87cd479063", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9494, - { - "block": 2906469, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:57:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:24", - "trx_id": "a06b8accfd9b681110080ff13f8f7cd514903822", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9495, - { - "block": 2906469, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:24", - "trx_id": "4ff6ed16621f28ebc231f7ca352f929c89d23ad5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9496, - { - "block": 2906469, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:57:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:24", - "trx_id": "1831577128a02e4da6c8f507ed4b71c29f8389cb", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9497, - { - "block": 2906470, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:27", - "trx_id": "8f45ce065d5830078f4a9aa52e2620f64b82226b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9498, - { - "block": 2906471, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:57:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:30", - "trx_id": "f9e35fc94633d70d29a3c31ba15a81b939894f0b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9499, - { - "block": 2906471, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:30", - "trx_id": "f4e901698a5d6b49d58a4b8718bb715980bea001", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9500, - { - "block": 2906471, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:30", - "trx_id": "61bd7108bcc36ffc308201d57c9a8eac3b37bf09", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9501, - { - "block": 2906472, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:33", - "trx_id": "c0c541c97a8cadcee45e69c5ca223b1642458905", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9502, - { - "block": 2906472, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:33", - "trx_id": "570ff262c0ac2bfc40c0f11143accd614bbeb7a0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9504, - { - "block": 2906473, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:36", - "trx_id": "202b121bf29119ee42963c0bef2f4e5658444589", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9505, - { - "block": 2906473, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:36", - "trx_id": "c4febad271a95846f8f3c7c8d5894256f8d53ac5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9506, - { - "block": 2906473, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:36", - "trx_id": "efee7ecaee9a6c3cb03ec8e4d0cc97a3b945f074", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9508, - { - "block": 2906474, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:39", - "trx_id": "678b29bea43f5a74badc3d7eb9aeed419a203eb4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9509, - { - "block": 2906474, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:39", - "trx_id": "b3b5c81d7379d67064717d5fbd9ed7073d6eeccd", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9510, - { - "block": 2906475, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:42", - "trx_id": "c81e27a20742cdd0426cddfb71ca07f7cb9ed9b6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9511, - { - "block": 2906475, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:42", - "trx_id": "f380de9c797601e231829ec97bc1442c9e06fc0a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9512, - { - "block": 2906476, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:45", - "trx_id": "e25af52eb6820dfef2901f4ef1bfa0ea3d55e38f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9513, - { - "block": 2906476, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:45", - "trx_id": "0a87fdae1ac6ee272bd8373a5cc5cd17e86ee264", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9514, - { - "block": 2906477, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:48", - "trx_id": "7cfb5c139615ffe86d08f1e7c235a79403ecc805", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9515, - { - "block": 2906477, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:48", - "trx_id": "e716258ce9c6cb1942b3814b4f0371a69ec4e4da", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9516, - { - "block": 2906478, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:51", - "trx_id": "56dc8dd37b26c35cf9fb3672fbf216bff24c553d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9517, - { - "block": 2906479, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:54", - "trx_id": "d582d8411e5bfb7331d31d3c8f22075afb1bf930", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9518, - { - "block": 2906479, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:54", - "trx_id": "2a10b87390a6e877f6f9f97ba785aa7042ab5e1b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9519, - { - "block": 2906480, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:57", - "trx_id": "db4877875b397c519e3a8bc82303f7cbcfc63d6f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9520, - { - "block": 2906480, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:57", - "trx_id": "5656ea18a0d10c8448454f0a5d8c01154e3eec08", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9521, - { - "block": 2906480, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:56:57", - "trx_id": "712890a3726dfa54f828f1620aab9df44fb5c480", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 9522, - { - "block": 2906481, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:00", - "trx_id": "fa8a1965db21690c8691d4d6f1a31fbf199628a3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9523, - { - "block": 2906481, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:00", - "trx_id": "44693a1e3186415f82542f03ebda5ecf6a3703d3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9524, - { - "block": 2906482, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:03", - "trx_id": "256e0467d9e67456150276f8e4406f81360863f5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9525, - { - "block": 2906482, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:03", - "trx_id": "87538659f22e07221920614287e5187292d96fa4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9526, - { - "block": 2906483, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:06", - "trx_id": "943b1c260ee78a264155b4ef00d7f5fe59d48fe3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9527, - { - "block": 2906483, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:06", - "trx_id": "fc5f906fc0c9969b63bad57dfd64e6c0edf7eb87", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9528, - { - "block": 2906484, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:09", - "trx_id": "afa749fca9738499987005f3e82deb8c3bc65cc7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9529, - { - "block": 2906484, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:09", - "trx_id": "d61f98d621c35e57754a2943e0a0cd3fb4b279a7", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9530, - { - "block": 2906485, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:12", - "trx_id": "9bbced21f7a5be64afbaaa48688a16443d3ae724", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9531, - { - "block": 2906485, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:12", - "trx_id": "9b87df940672f7be7c61ae0e82239c8518e0b51a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9532, - { - "block": 2906486, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:15", - "trx_id": "5697cc19e5604b2f9f703f0a189ab28b4856f6ff", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9533, - { - "block": 2906486, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:15", - "trx_id": "245dc724d04d6f0192481bd163459113ea37bc0c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9534, - { - "block": 2906487, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:18", - "trx_id": "9b9b10687906aa6f1457141d69af8cd92a98443c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9535, - { - "block": 2906487, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:18", - "trx_id": "3c45b5600b1550022082d72deab7399ff4161ef5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9536, - { - "block": 2906488, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:21", - "trx_id": "729c3d69d99f3e27f2e5c3cea1d0d0f1ecd38bc9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9537, - { - "block": 2906488, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:21", - "trx_id": "1b6fb2e0290f2989927f8a52a9d4c39e77735793", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9538, - { - "block": 2906489, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:24", - "trx_id": "6cf9c3bf66a1d369f653a5937a4062e786e2c841", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9539, - { - "block": 2906490, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:27", - "trx_id": "540110d055ff3f6472635e663c8a161f827c94ba", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9540, - { - "block": 2906490, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:27", - "trx_id": "556afbbc21dcce7e15e62e1075e6282b14f13108", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9541, - { - "block": 2906490, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:58:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:27", - "trx_id": "55183b13e5915d7d1ad77f234a933ee9f60350a0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9542, - { - "block": 2906491, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:30", - "trx_id": "d001d1b44be2aad8085c6fef157aa220e6eb862f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9543, - { - "block": 2906491, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:30", - "trx_id": "63345210527b6700cbe59149f67dc4864e980117", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9544, - { - "block": 2906492, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:33", - "trx_id": "f75842e6c0896644d85b955a1d74dcbce37bb17f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9545, - { - "block": 2906492, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:33", - "trx_id": "26d71ea155788ece75e71a55f7bb4f80d6be3b31", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9546, - { - "block": 2906493, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:39", - "trx_id": "fe2b160d57f0d86af9ae1c5c5c2f399d6ee9be17", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9547, - { - "block": 2906493, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:39", - "trx_id": "aba0a2f74d1c996b6b675f5558eee3ce3d17dfd1", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9549, - { - "block": 2906494, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:42", - "trx_id": "4c2fcfe402f3735f8dfb90744b19afe74f239f42", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9550, - { - "block": 2906494, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:42", - "trx_id": "d9911f4ff98076a0184b655052d4ed6473b6fe9a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9551, - { - "block": 2906494, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:42", - "trx_id": "b1285575af505e4f3b49254a6cf9791c5d5c6183", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9554, - { - "block": 2906495, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:45", - "trx_id": "4388580eadf30712543d06bc32ea1a2051cde510", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9555, - { - "block": 2906495, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:45", - "trx_id": "0d6d53affae550e562a3deb224eb711f2e525004", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9557, - { - "block": 2906496, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:48", - "trx_id": "ef5516d046590a09d75509af45e114f5e5a3207d", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 9558, - { - "block": 2906497, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:19", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:51", - "trx_id": "31a9c88d93b59bee766035b13e5cbd636570f2a1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9559, - { - "block": 2906497, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:51", - "trx_id": "7d50ecb4565f2bae8476877954a65ccb62aaa141", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9560, - { - "block": 2906497, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:51", - "trx_id": "f08e0946d641d2b53aea240f08a4ef7c9e2d84ad", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9561, - { - "block": 2906498, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:54", - "trx_id": "978c90f6ccabec8698fd0627a3c576446c52640d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9562, - { - "block": 2906498, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:54", - "trx_id": "0a72c03d417348dba35f4f8bfdb5fc7d457f8a92", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9563, - { - "block": 2906499, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:57", - "trx_id": "e5825e0c5bda71b70c875ee9fddd4ede5534d6a2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9564, - { - "block": 2906499, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:57:57", - "trx_id": "2e9ced12973013f40c22fc754a9dbd8e9afe21ef", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9565, - { - "block": 2906500, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:00", - "trx_id": "11848d5ef77b4a90c44216f2c54d886e89e80688", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9566, - { - "block": 2906501, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:03", - "trx_id": "205a41d2d4af6eec1c6099858d9fefa86f61a4be", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9567, - { - "block": 2906502, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:06", - "trx_id": "96a42de02ecb6551e1e49ce66ed71d410a15d566", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9568, - { - "block": 2906502, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:06", - "trx_id": "d05aec051bd9fdabaa68e891d208bf9770be8f2a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9569, - { - "block": 2906503, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:09", - "trx_id": "e5fd3f787fd01e1bd6a7a6197c5fcc913bb15d11", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9570, - { - "block": 2906503, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:09", - "trx_id": "0bf51f25a7a9c339ee1c8d70cad0293372c65feb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9571, - { - "block": 2906504, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:12", - "trx_id": "e09247bba681c2faa2e6f1b0aa0eb8294baafc02", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9572, - { - "block": 2906504, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:12", - "trx_id": "aebebfa2615f0069ffc42bd82f1fecf8b9276416", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9573, - { - "block": 2906505, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:15", - "trx_id": "047ae9297d79cdf700a7b12d6d507d0884441076", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9574, - { - "block": 2906506, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:18", - "trx_id": "0bb4b188c54e5dcfbdf71a315bcccada5436ec20", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9575, - { - "block": 2906506, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:18", - "trx_id": "072e5406f65bff9d97f4aa262e72ba24a3778cca", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9576, - { - "block": 2906507, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:21", - "trx_id": "7c8cb052dde72aa8676f714b9ebc2da5c184a002", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9577, - { - "block": 2906508, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:24", - "trx_id": "dac05e222e3fbeabf8a76a34ea8d6e23ef332c50", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9578, - { - "block": 2906508, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T14:59:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:24", - "trx_id": "b2aa9cb274c9f7810f296c1e7f655a1c0a6a1ea5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9579, - { - "block": 2906509, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:27", - "trx_id": "e82a599672a3328b1d5de8607868d283c5f16c42", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9580, - { - "block": 2906510, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:30", - "trx_id": "ff82fd5be64e0a043b51898593be99e14518e39d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9581, - { - "block": 2906511, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:33", - "trx_id": "5eaacc2a2446ebf076d187f2a86d51577afea231", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9582, - { - "block": 2906511, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:33", - "trx_id": "ec6ffd4d8223d49767b223093bef70fcabc6e913", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9583, - { - "block": 2906512, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:36", - "trx_id": "6f3eaa5ee05a940a856a80b27ddf6784c1d879c2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9584, - { - "block": 2906513, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:39", - "trx_id": "69708a68a3247b564648f28e0c4c137acd12727f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9585, - { - "block": 2906513, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:39", - "trx_id": "2b3d7b46fefd679804619b0284d7ee290ee8e88b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9586, - { - "block": 2906513, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:39", - "trx_id": "c02a4cc3ac1c0382ce32481e7eb416966d23734d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9587, - { - "block": 2906514, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:42", - "trx_id": "e38c2d1d01a276a131bbd1c378a4e95ab9b16158", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9589, - { - "block": 2906514, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:42", - "trx_id": "c0566265b50d42b906edc9d382cc7211496c73bd", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9590, - { - "block": 2906515, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:45", - "trx_id": "ad69d0dec0b6bc5828b87a8781dde0958ff2461a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9591, - { - "block": 2906515, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:45", - "trx_id": "a8c495b4fe1ec38e038553ab8503dd0e576e993a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9592, - { - "block": 2906515, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:45", - "trx_id": "f823b6e5b190e9008652f2da0e21b2d7ffc7f981", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9595, - { - "block": 2906516, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:48", - "trx_id": "f9d0431f16e86bcc4dd0e8be2ef543f08cf24161", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9597, - { - "block": 2906517, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:51", - "trx_id": "ba68a9901b298f3c6ad99d35a6c572a7ed2f5d7f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9598, - { - "block": 2906517, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:51", - "trx_id": "857fe3833701fccaff64e21c9997f7b93264d5ea", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9599, - { - "block": 2906517, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:51", - "trx_id": "a5b12c739314b34d3efe96d490318a0486bef945", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9600, - { - "block": 2906518, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:54", - "trx_id": "b13d7db59cf558f1613858296b5f31796b6515b3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9601, - { - "block": 2906518, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:54", - "trx_id": "2889377b22476ca72c3e066acd19bdebf60affc8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9602, - { - "block": 2906519, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:57", - "trx_id": "78d01fb076ce1b2715785f6596ee5080acabd2fa", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9603, - { - "block": 2906519, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:58:57", - "trx_id": "d592200170c0e60f325a6d739caf537c8b5cd90c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9604, - { - "block": 2906520, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:00", - "trx_id": "87f22d8e2a603b0252402d25412de7b06b98f809", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9605, - { - "block": 2906520, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:00", - "trx_id": "8a71150bc585ee152ac2fe2991970395571aa836", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9606, - { - "block": 2906521, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:03", - "trx_id": "bdce272e7f126e081df3a42a3f251a79bc3db9c0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9607, - { - "block": 2906521, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:03", - "trx_id": "fa744c244ecde389ca1ef4713c444233374ec0b0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9608, - { - "block": 2906522, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:06", - "trx_id": "43edee7b944888fd9a0c043e00796660011fc724", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9609, - { - "block": 2906523, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:09", - "trx_id": "fc1b9a64c8bf18b62ce43da37cd92d2a60350737", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9610, - { - "block": 2906523, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:09", - "trx_id": "d1c5808ab7dca5462b8ae63b1568661aabf3d1d0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9611, - { - "block": 2906524, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:12", - "trx_id": "00b74e899cd6eeb057af65907bef7c943431ef1f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9612, - { - "block": 2906524, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:12", - "trx_id": "0238e09c1a85f4b29c5b444c961e7a92f96188b8", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9613, - { - "block": 2906524, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:12", - "trx_id": "3ab70e618db3eee3e9d694aa4faf106fa8ba9127", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9614, - { - "block": 2906525, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:15", - "trx_id": "7178eae670efb07dd309995583642a6866251d1b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9615, - { - "block": 2906526, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:18", - "trx_id": "06c2a26eacf8bc8c334dac81f92d572916e2775b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9616, - { - "block": 2906526, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:18", - "trx_id": "c10e9122741c51566d42bbc7f8fdb5324d9d0e49", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9617, - { - "block": 2906526, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:18", - "trx_id": "6d11add403e838747d1904977515b2afffbff649", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9618, - { - "block": 2906527, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:21", - "trx_id": "788b1dd7900effd75791080ae24f5ab43747ef59", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9619, - { - "block": 2906527, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:21", - "trx_id": "8513b7ba9d9c0b42aa6704fe1b8a3b508194b114", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9620, - { - "block": 2906528, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:24", - "trx_id": "71a157cdc7802e4fedb497a774e3d3f0dd2f21c1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9621, - { - "block": 2906528, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:00:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:24", - "trx_id": "beda03bcad2b90b88e02e5f9c648378e373d1774", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9622, - { - "block": 2906529, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:27", - "trx_id": "1946f2aa8e3b527b949e14508a95502f0afc581e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9623, - { - "block": 2906530, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:30", - "trx_id": "b9464403d0c06fb058938e3c895ef9248ada0311", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9624, - { - "block": 2906530, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:30", - "trx_id": "29646ce5cf3092f0f64e352caf20cfb0d12e2c30", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9625, - { - "block": 2906531, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:33", - "trx_id": "be2b0a337a252fbb89c80e6beaa9ca6073d91f29", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9626, - { - "block": 2906532, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:36", - "trx_id": "c9431f1eef04e392e4667d9097d71d7f1c5e78ea", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9628, - { - "block": 2906532, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:36", - "trx_id": "8426b8b8b80a6e6598399e32a87e490861c002f5", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9629, - { - "block": 2906533, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:39", - "trx_id": "7f0fdf8fb139cabcbcab1dc8b1a880e1b2659d06", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9630, - { - "block": 2906533, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:39", - "trx_id": "22a85bcad8c2e55258783c808a0d761792f239a1", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9631, - { - "block": 2906534, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:42", - "trx_id": "cf55d26aa7703edd2d779f3bd9cab5dd8598edf5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9632, - { - "block": 2906535, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:45", - "trx_id": "12f574dde9c6ce5e3f84fce9b089db43183acdf8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9633, - { - "block": 2906535, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:45", - "trx_id": "9dd5f3dc70156068a7ab4a2270f0213578147619", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9634, - { - "block": 2906535, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:45", - "trx_id": "0f5bb0e92d4a7cffe032dc0fe3422dc779b140c2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9636, - { - "block": 2906536, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:48", - "trx_id": "ef51597f40013b5d16f17aa0ff7291813392f984", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9637, - { - "block": 2906536, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:48", - "trx_id": "9ad20096c2342bf395b12f5ab6593975b5791b69", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9638, - { - "block": 2906536, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:48", - "trx_id": "5bf14d7ac66cbb0c7ae01ef6f7caf2cd9fa6869a", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9640, - { - "block": 2906537, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:51", - "trx_id": "7d44e4442842372c5009a2eda262615159fd32c5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9641, - { - "block": 2906537, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:51", - "trx_id": "d1e4f6d21cd336ef54da7da1fa24ddc25b5e4ad6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9642, - { - "block": 2906538, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:54", - "trx_id": "d621309008ad0f55d445ca41e35fa417084be1cf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9643, - { - "block": 2906539, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:57", - "trx_id": "91106dce727d4a047b1ebbaed85b749ac6c637e2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9644, - { - "block": 2906539, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T14:59:57", - "trx_id": "b25288ef5232a1ff5452c14e9e891e47e7dcab7f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9646, - { - "block": 2906549, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:01:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:27", - "trx_id": "157098cc0a3c0339061de3fc1eaa2b2988e870c2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9647, - { - "block": 2906550, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:30", - "trx_id": "b0274fdd33c51b56ad89141e5ae07db5b30ee9e6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9648, - { - "block": 2906550, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:30", - "trx_id": "8c00eddcda1379a45c925b34945154a464d3722d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9649, - { - "block": 2906551, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:33", - "trx_id": "715e4f15136236f0e1d31f6503375649b9b22bd7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9650, - { - "block": 2906551, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:33", - "trx_id": "b82dc342c1a098b1c08128a441b16ee04985a0ad", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9651, - { - "block": 2906552, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:36", - "trx_id": "33edf37470a3aa9b24c47d331fd8ade43ca531a8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9652, - { - "block": 2906553, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:39", - "trx_id": "6ced002fec9bbad167e25ba17174c6cc85fa88f4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9653, - { - "block": 2906553, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:39", - "trx_id": "2c722ced93b153a33ff0fdfc6b93685d227df674", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9654, - { - "block": 2906554, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:42", - "trx_id": "032ad048a60ee0689eea8f908e639efa35711980", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9655, - { - "block": 2906554, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:42", - "trx_id": "58a963421a70652cd5559b84c83e4aaace1569e2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9656, - { - "block": 2906554, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:42", - "trx_id": "7e7f72d0d0dc3e9aef775d6cf05fcb88be3e79e8", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9658, - { - "block": 2906555, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:45", - "trx_id": "07977888fe13d2dec29a9e068a7502ccf855283b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9659, - { - "block": 2906555, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:45", - "trx_id": "81d7fa1dd8386acdb07c51cb397e051a084f0640", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9661, - { - "block": 2906556, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:48", - "trx_id": "74b0357e33d3f78b7b266ec2cf40811119d710b3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9662, - { - "block": 2906557, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:51", - "trx_id": "c306e83019857a2f46794702ee1d783ace4b9f0d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9663, - { - "block": 2906557, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:51", - "trx_id": "38abf36e0933547cc0e901ed6cef0f9d5504fe90", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9664, - { - "block": 2906557, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:51", - "trx_id": "d48803ae13579e076d702b5f47e2a8eb44c29613", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9666, - { - "block": 2906557, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:51", - "trx_id": "9843580665d3f7d5a72a4325188909ed87725511", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 9667, - { - "block": 2906558, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:54", - "trx_id": "b627b07429d7bbbc660c2daf832480100c0af1f3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9668, - { - "block": 2906558, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:54", - "trx_id": "069882f2d8fd66d8950be62c1f07112b82025d1c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9670, - { - "block": 2906559, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:57", - "trx_id": "a6a0c52a0873fcfb6fd7a9d7dda3fbb89a0da5c6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9671, - { - "block": 2906559, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:00:57", - "trx_id": "2b60a5ecda1d4988618137775f1dbfa2f0137542", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9672, - { - "block": 2906560, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:00", - "trx_id": "1893968399f610a385f7db9c20fbc35e68399fd6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9673, - { - "block": 2906560, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:00", - "trx_id": "984960817a8486e0a8d236098b83594905c45988", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9674, - { - "block": 2906561, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:03", - "trx_id": "a5fe773d94653d621ab1a7df35153769d82df1ea", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9675, - { - "block": 2906562, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:06", - "trx_id": "a6430c9ceaef859a3783be5441ddb7074dc0d215", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9676, - { - "block": 2906563, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:09", - "trx_id": "6c59cdf0f27d9acc0fcb2bcca2ccdc39fb7c3162", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9677, - { - "block": 2906563, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:09", - "trx_id": "94fc3ccfe1bbb0a0578dee28532029fbc8260357", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9678, - { - "block": 2906564, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:12", - "trx_id": "3b36b277741dd3339803a790af6aee0113436486", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9679, - { - "block": 2906564, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:12", - "trx_id": "ec0f4922d2d4582231d6d19961f37485d6f27509", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9680, - { - "block": 2906566, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:18", - "trx_id": "ad83ed5bc4ebfb6dfd835c9f34c37c632e40acf5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9681, - { - "block": 2906566, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:18", - "trx_id": "1d47b608ca829e239ee318ced5670ae11af76432", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9682, - { - "block": 2906567, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:21", - "trx_id": "7e50bcc3c1396a4bcb56b0418f7360d521be0cf8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9683, - { - "block": 2906567, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:21", - "trx_id": "9c94cdaefb924722c29f1563ab330563301163b5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9684, - { - "block": 2906568, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:24", - "trx_id": "0dd6a7f0cb6f3b0744fabbd1fe4bad18f4c0ea53", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9685, - { - "block": 2906568, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:24", - "trx_id": "aadd752bc9ee1a526db1815ee285e34a204c2a80", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9686, - { - "block": 2906569, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:27", - "trx_id": "c346d361536296103836ef771aa8d716c409fd1d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9687, - { - "block": 2906569, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:02:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:27", - "trx_id": "38e2f969577addcf479660dcfba32f681cfd6ed4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9688, - { - "block": 2906570, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:30", - "trx_id": "f20a2462a306c14825624787ef8fa88825b34c34", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9689, - { - "block": 2906570, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:30", - "trx_id": "59704e8630d4b1b34453e3e029fdec592ff53304", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9690, - { - "block": 2906571, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:33", - "trx_id": "63f37e8c7fcd2c955ecf350dafc0f9b8d63d33d6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9691, - { - "block": 2906571, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:33", - "trx_id": "a9a06c4b02126bcd1dc358f485e95e590ac2b277", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9692, - { - "block": 2906572, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:36", - "trx_id": "129dd4323f3b31301af2aa8df58123a1e14cabf9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9693, - { - "block": 2906572, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:36", - "trx_id": "6aaa72cb316cad3203b1d84d30152c5fd5d60059", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9694, - { - "block": 2906573, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:39", - "trx_id": "153555e03175e7558138c02eee9197f919d6cfe9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9695, - { - "block": 2906574, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:42", - "trx_id": "ceb91b1ee6fadb637df7a9dd47ccb49bfcda3dda", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9696, - { - "block": 2906574, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:42", - "trx_id": "9ca0d8f3665b64ea1f8e67903b95853675302c5c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9697, - { - "block": 2906574, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:42", - "trx_id": "208370320f7da64c1b6602a777df8f7890d105db", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9698, - { - "block": 2906575, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:45", - "trx_id": "5341e1c87e9485412f1d203d147a2746cfedec17", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9699, - { - "block": 2906576, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:48", - "trx_id": "d5377b1ae551cd1a0ffcf9b503d6a6e83842eafd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9700, - { - "block": 2906577, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:51", - "trx_id": "8b80b7704d18f71e9558d425a76c636151b5b366", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9701, - { - "block": 2906577, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:51", - "trx_id": "b78a905dd6e7a675d8051c2b206908ba9f9910d0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9703, - { - "block": 2906578, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:54", - "trx_id": "3c761064d50632145678d18fb26654fbd3c5a86e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9704, - { - "block": 2906578, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:54", - "trx_id": "765b0a438da67eeb955c59e5b1aaf768d7796ec7", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9705, - { - "block": 2906578, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:54", - "trx_id": "b8fc9e0b20203ce4193438f05fba71582a54fb27", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9708, - { - "block": 2906579, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:57", - "trx_id": "ea8236ca631d87ed0a92b229c0290c41fd06b3bf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9710, - { - "block": 2906579, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:01:57", - "trx_id": "f478c6fcff079bae67c34e50649c05d920b10f20", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9711, - { - "block": 2906580, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:00", - "trx_id": "5a03e3e78fa0d997cef8ea9ded8dcea68bde5e0f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9712, - { - "block": 2906580, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:00", - "trx_id": "00ffb919533be0fc35751c7a6f0b16f3092cfaad", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9713, - { - "block": 2906581, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:03", - "trx_id": "6f2c0dea4fed123a1f4b22d8a69a7f2d55cb26ed", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9714, - { - "block": 2906582, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:06", - "trx_id": "fbe03a5d14e2908c663e625b0d13d6080168fec2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9715, - { - "block": 2906582, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:06", - "trx_id": "c30f95e6786bee51d6156472fb1d6110cfb5ae0f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9716, - { - "block": 2906583, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:09", - "trx_id": "4a1540ce9ef647577ec2e3228a18e826c2ea6819", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9717, - { - "block": 2906583, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:09", - "trx_id": "eba484f71d1144001effdd3296d5bd3507f3bc6b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9718, - { - "block": 2906583, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:09", - "trx_id": "eb5e9ddec1becee4f1c6f76ec20d4dc00b13fab2", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9719, - { - "block": 2906584, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:12", - "trx_id": "ca9efc9fb7ef66f566d1b6e9c16434bf17b5dad1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9720, - { - "block": 2906584, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:12", - "trx_id": "f3b3c2998db6127f5f614670a92199d68a9c99a3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9721, - { - "block": 2906585, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:15", - "trx_id": "f368ca7795fc83cd059fa3c10dcd646c79dd1e15", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9722, - { - "block": 2906585, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:15", - "trx_id": "72f49f1f715096202863055a98a35af3b479f19e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9723, - { - "block": 2906586, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:18", - "trx_id": "dd66264022abdd9f9bc785e8817aa1dd12ed66cb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9724, - { - "block": 2906586, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:18", - "trx_id": "e83baa51a869836c1321ecb6e0292db510fc33c8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9725, - { - "block": 2906587, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:21", - "trx_id": "bffb3de20b6c5759d8913fe505eb2398755bd77a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9726, - { - "block": 2906587, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:21", - "trx_id": "3204431b7a8686544e2e5376a45a335cd27bcc50", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9727, - { - "block": 2906588, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:24", - "trx_id": "e1684b3022d8f1378ad0a71451366bc49a69e774", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9728, - { - "block": 2906588, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:24", - "trx_id": "4f909b6f8222cbca1f978dbddb9615d451e66bfc", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9729, - { - "block": 2906589, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:27", - "trx_id": "3a4e87b4ad4ba73c59cd18841adf22c003ecda0a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9730, - { - "block": 2906589, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:03:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:27", - "trx_id": "91f29f35cf15fc1ac9c750e6c036cc2059548e22", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9731, - { - "block": 2906590, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:30", - "trx_id": "6c1eb622a1643841130901f19d9d2f4087e45774", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9732, - { - "block": 2906590, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:30", - "trx_id": "166dc75f1ff47432dde53469b9f2e58296319a2e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9733, - { - "block": 2906591, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:33", - "trx_id": "06d45a8c6fb3a4309195804237a11e1c2fd2f69b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9734, - { - "block": 2906592, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:36", - "trx_id": "472449fa09d43878f84d9cb28ec05df76ee1a033", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9735, - { - "block": 2906592, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:36", - "trx_id": "df20e6b19558d8d99f0b28e303db5a56398ef752", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9736, - { - "block": 2906593, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:39", - "trx_id": "615aac0b7ed65187c8bd933f583c4faba005c9e4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9737, - { - "block": 2906593, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:39", - "trx_id": "44400fa5452d8c30492ebb7a80c94384df5c7a04", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9738, - { - "block": 2906593, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:10", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:39", - "trx_id": "b52121dc60aafc96d5f33bf701c47b747b2bc5b2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9739, - { - "block": 2906594, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:42", - "trx_id": "6595e2a577ba7b2972a89f5023dcf86c1d954e66", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9740, - { - "block": 2906594, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:42", - "trx_id": "5e1308ee03009a64012c39cf426917b7a23a68b1", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9741, - { - "block": 2906595, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:45", - "trx_id": "ed4f8dcc61f77265c6333501ac8d035c1caaa5ee", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9742, - { - "block": 2906596, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:48", - "trx_id": "97ba28abcbd802bfa59603e2d663166d573be89e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9743, - { - "block": 2906596, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:48", - "trx_id": "172c33f9971eb28d85cf0764f9a087dc6ad54936", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9744, - { - "block": 2906597, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:51", - "trx_id": "a32e691b00a5002684c281c750effac27f26bc8e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9745, - { - "block": 2906597, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:51", - "trx_id": "7d1c6a57523a65be9168862fb995ccd05069ed25", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9746, - { - "block": 2906597, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:51", - "trx_id": "42fc74946098f7250214f29a9b45aba0061a0203", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9747, - { - "block": 2906598, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:54", - "trx_id": "85249529da3848c8a72d47782b46cfe05f352836", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9749, - { - "block": 2906599, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:57", - "trx_id": "1e280ae31888910b103a33a786c4a66d8554af59", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9750, - { - "block": 2906599, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:57", - "trx_id": "06c27f6dddc061c3cd40e0d2422ccba07b9db307", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9751, - { - "block": 2906599, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:57", - "trx_id": "b20d53345f532b89632b9839866cae1e6717d356", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9753, - { - "block": 2906599, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:02:57", - "trx_id": "57657f5540a79ffcff0f1917b5fcbbb9003027ba", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 9755, - { - "block": 2906600, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:00", - "trx_id": "80a25cf9f8ffcbba86b28539c07dff56bdb8bdd7", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9756, - { - "block": 2906600, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:00", - "trx_id": "270f28f86f413c1cf6ddc7426bf6c187ac07d406", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9757, - { - "block": 2906601, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:03", - "trx_id": "f748c6db07c2a7ee65f8c860be2e6b60585cc86e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9759, - { - "block": 2906601, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:03", - "trx_id": "9205b3c3231e8ea21a761cb13df2d36ca07b5bb8", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9760, - { - "block": 2906602, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:06", - "trx_id": "f971300b7e14170d864489b7c498b36a51876a00", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9761, - { - "block": 2906603, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:09", - "trx_id": "0f2ba39d07ed8525a34dd74057f82e005f76e614", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9762, - { - "block": 2906603, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:09", - "trx_id": "d06cb230c6b8e13e5a9a2691d023fcab556d0412", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9763, - { - "block": 2906604, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:12", - "trx_id": "38c37acfdb136ddf2cda8e40c460b0a319c8c6e7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9764, - { - "block": 2906604, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:12", - "trx_id": "8824509af6bca4d3da8d0dc9296d645b1c8d95e8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9765, - { - "block": 2906604, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:12", - "trx_id": "1cffcc5baa5a8cbd2753b6c89f1cfa7e47fa44c9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9766, - { - "block": 2906606, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:18", - "trx_id": "bcf57ef43be98347c95793c7fd6dad54492b38f1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9767, - { - "block": 2906606, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:04:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:03:18", - "trx_id": "6c85c2c2faa72d6ae872377b6421dd72945e67a1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9768, - { - "block": 2906635, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:04:48", - "trx_id": "f792e4de61dc41021eb09e231e4d2241f57b3611", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9769, - { - "block": 2906635, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:04:48", - "trx_id": "f56adf2114e780eadad38ad46906de99307ea5b9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9770, - { - "block": 2906635, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:19", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:04:48", - "trx_id": "0af4a65a2c7eb5e36ae28212f8d7f24971e25899", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9771, - { - "block": 2906636, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:04:51", - "trx_id": "52b5f70f177639808fd947ae1bfa9ecae6c29714", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9772, - { - "block": 2906636, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:04:51", - "trx_id": "411bc620ff397d909a591bb39719569d6ad554fb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9773, - { - "block": 2906637, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:04:54", - "trx_id": "4075a2123361628be5e3710570212e2c10bb0e8c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9774, - { - "block": 2906637, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:04:54", - "trx_id": "d144daba10655253787458e1b1201e44a57507b3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9775, - { - "block": 2906638, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:04:57", - "trx_id": "dff7cb4769a0a213f3a230703e072c6157361ab1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9776, - { - "block": 2906638, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:04:57", - "trx_id": "deeeb99a2eadd25cc247089f6443d51bd04ba110", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9777, - { - "block": 2906639, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:00", - "trx_id": "b5b6bc739022d973766054270229be4f96aae129", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9778, - { - "block": 2906640, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:03", - "trx_id": "3fd8e3b2081fe6cec1a9f1c98b4b42430b963ad9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9779, - { - "block": 2906640, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:03", - "trx_id": "61e36c1d5ae059fd42b4808d4c5e094344078906", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9780, - { - "block": 2906640, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:03", - "trx_id": "4dc5539845a31e3ce09baa373b3730dee72c9263", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9781, - { - "block": 2906641, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:06", - "trx_id": "72bf41eededfaf977000c5e526af367cb77111a8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9782, - { - "block": 2906642, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:09", - "trx_id": "c2cdf6389471357ca1d8eda69a79bb9b4b9b358c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9783, - { - "block": 2906642, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:09", - "trx_id": "4df26a479235700b32fdf5e48569679483023ec8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9784, - { - "block": 2906642, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:09", - "trx_id": "c7824528496e0530413b787b9cfdd872a3a91b8f", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9785, - { - "block": 2906643, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:12", - "trx_id": "f5030e9d7388ac549fefb25e23bd05f8eb126ee0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9786, - { - "block": 2906643, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:12", - "trx_id": "3cbe491a4687030104a5b804af92588f2a91c45f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9787, - { - "block": 2906644, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:15", - "trx_id": "9cec2cf082ecbf22c57dd72f59efb2e24e3712e0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9788, - { - "block": 2906645, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:18", - "trx_id": "c546f01ab627510d029f8bc166fc6bb6f77e6d1e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9789, - { - "block": 2906645, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:18", - "trx_id": "95ba5d53adc24de7e19c2b46c8a802e22a722a80", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9790, - { - "block": 2906645, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:18", - "trx_id": "2bbd1523ae2898924f15c93eb959685fc76b40a4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9791, - { - "block": 2906646, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:21", - "trx_id": "5ebafaa25de4fa1d5d746a1b856d64f6ad876d23", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9792, - { - "block": 2906646, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:21", - "trx_id": "da45098f0bc99867824e05a300f48d6e1c2804f8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9793, - { - "block": 2906647, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:24", - "trx_id": "4dc92b51cad23e9e61acf03592691a4e420158fc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9794, - { - "block": 2906648, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:27", - "trx_id": "c5ddd11c938016cb9ed9cb35380e0337a54285b6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9795, - { - "block": 2906648, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:27", - "trx_id": "71ebf57883d6f1fb12c8ebb45932db3ce1450903", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9796, - { - "block": 2906648, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:06:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:27", - "trx_id": "7c1cdd229cc605bd91aad2784ecea4453f14c67b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9797, - { - "block": 2906649, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:30", - "trx_id": "209d48b7da3b06219a1ecc7b4b185d9ab4faad70", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9798, - { - "block": 2906649, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:07:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:30", - "trx_id": "522d2b5bfc9e299e6f8d7e6cfeca4f3ea571ed11", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9799, - { - "block": 2906650, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:33", - "trx_id": "254a5305735c7f4efe64690eaa9ac1b8d1f3c693", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9800, - { - "block": 2906650, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:07:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:33", - "trx_id": "24cccf6c33727834cbf418925eee58d7543c2695", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9801, - { - "block": 2906651, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:36", - "trx_id": "0e5fe6f50994a95e6e56cefe28389000682c4082", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9802, - { - "block": 2906651, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:07:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:36", - "trx_id": "c27d0204b7b3c91bbce3def8731a4116f22da489", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9803, - { - "block": 2906652, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:39", - "trx_id": "315c247458854a6e5954ee69d6e08d80e7b4d4f0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9804, - { - "block": 2906652, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:07:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:39", - "trx_id": "ebf3c69c057bd12638ee94d0f414e1289dbe868a", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9805, - { - "block": 2906653, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:42", - "trx_id": "da9f7c856f0f3f5636ce295907858d94dccb7b4f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9806, - { - "block": 2906653, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:07:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:42", - "trx_id": "18572a4ea562dbe72c9180392ecfa97c56620e31", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9807, - { - "block": 2906654, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:45", - "trx_id": "305b81ce6ea07d561be9f42af9c3d01adedd36bb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9808, - { - "block": 2906654, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:07:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:45", - "trx_id": "137c41e857c544ee0513b63af06bc69da92a5f88", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9809, - { - "block": 2906655, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:48", - "trx_id": "e4010bce6418771a044f234640e8ed2ae500c963", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9810, - { - "block": 2906655, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:07:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:48", - "trx_id": "4a193ed767d296b3c61334b42e3cebf953aac73e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9812, - { - "block": 2906656, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:51", - "trx_id": "457302eada8b3fd60f5d684176343a0db2913a1c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9813, - { - "block": 2906656, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:07:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:51", - "trx_id": "4a6fabf9ee2e93fa22ed305aacda0b461265e83c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9816, - { - "block": 2906657, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:07:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:54", - "trx_id": "3b657e0777d79df34992051b222052f4f4dda47c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9817, - { - "block": 2906657, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:54", - "trx_id": "643aaed75e70564f76dfac18fe678cd3968b05d7", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9819, - { - "block": 2906658, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:07:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "85998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:05:57", - "trx_id": "7313134bf138432624a7418a456f0765211453e3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9822, - { - "block": 2906678, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:08:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:06:57", - "trx_id": "a6bb750f3bf86d8831449dedcbeb04581ae29387", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9826, - { - "block": 2906679, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:08:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:07:00", - "trx_id": "4257447ac718e22e9358ce2d5d554242e40d75a9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9831, - { - "block": 2906700, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:09:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:08:03", - "trx_id": "0a49678be94417839fccb68fa06eaa6d9fbc6edf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9832, - { - "block": 2906700, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:09:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:08:03", - "trx_id": "746e3cc72a52e4fb9698c387407d718228028f82", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9835, - { - "block": 2906721, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:10:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:09:06", - "trx_id": "8dd1555542434428be30b30b654d922990a6b4c6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9836, - { - "block": 2906721, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:10:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:09:06", - "trx_id": "65d9541ca32194975072fec775a9488613dd2442", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9841, - { - "block": 2906742, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:11:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92998", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:10:09", - "trx_id": "8b27470c353464ed23e8219e70caf8261a2c4027", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9844, - { - "block": 2906743, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:11:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:10:12", - "trx_id": "b4253f157d0abd152a9c3e36e60794a26d6ceef6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9845, - { - "block": 2906749, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:10:30", - "trx_id": "f45c338932642be367a364b0750e13984e7193fe", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9846, - { - "block": 2906751, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:12:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92797", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:10:36", - "trx_id": "115f359d8b9b3a7217d1883088fb1e822a0b7324", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9848, - { - "block": 2906764, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:12:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:11:15", - "trx_id": "525700b63d0ab011417cb992c7860fdf3e33b518", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9852, - { - "block": 2906772, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92797", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:11:39", - "trx_id": "6bcea5b0d4b3120e137168d99d49da1c6fc76d1e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9853, - { - "block": 2906780, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:03", - "trx_id": "ef8fb2e6a1b8bc254ddff72c11d41b4dccb91697", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9854, - { - "block": 2906780, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:03", - "trx_id": "52cad80e84a112f5bb11428baa0565c32a360c11", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9855, - { - "block": 2906781, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:06", - "trx_id": "014f9bdd0a49abb35e5cf3294dd7879e5503b7b2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9856, - { - "block": 2906782, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:09", - "trx_id": "46218a963c10e55731c3e52a50332c5f097a7dc9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9857, - { - "block": 2906782, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:09", - "trx_id": "3f542b6d7234cc0b263243391760d49f31f65fb8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9858, - { - "block": 2906782, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:09", - "trx_id": "011d8d7269d31cc306a0530bf49b5760b264ca64", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9859, - { - "block": 2906783, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:12", - "trx_id": "872cf564b56d5dc99b33d1fe289dfd5d3177117d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9860, - { - "block": 2906783, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:12", - "trx_id": "7a12b5894a905a22c8da0a0bc0bfe0e3cc38894d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9861, - { - "block": 2906784, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:15", - "trx_id": "f672195a742c5ac7856168448e1e9a83f1681cca", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9862, - { - "block": 2906784, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:15", - "trx_id": "92577ea771409131f7d5f2f016ea14e5451b6d25", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9864, - { - "block": 2906785, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:18", - "trx_id": "ba185975e61a26e9b69137df767c35311b3cade7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9865, - { - "block": 2906785, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:18", - "trx_id": "6a6683226dfdabd48c275d7a689cb1db1388b573", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9867, - { - "block": 2906785, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:18", - "trx_id": "d90e121b970d83968d1d20d22bde71fd7ccd375c", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 9869, - { - "block": 2906786, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:21", - "trx_id": "ebb93c9f1d8f9885773298ce2a93d4da77eec860", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9870, - { - "block": 2906787, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:24", - "trx_id": "74ffedd90ee3ccf834e033d1d216c149ca75b372", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9871, - { - "block": 2906787, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:24", - "trx_id": "7a4fef3a0a050bf8215cdcc9c1a434872f5a203d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9872, - { - "block": 2906787, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:24", - "trx_id": "d8eb05a24ba3e2d603f09b24bce43e04a037a34d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9873, - { - "block": 2906788, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:27", - "trx_id": "4b89d8a6810fa446403b9286d9ef0dbafe36ef0e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9874, - { - "block": 2906789, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:13:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:30", - "trx_id": "f7e3c7bc43951581620b4505a56e57ab99988ce3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9875, - { - "block": 2906789, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:30", - "trx_id": "6ae2782e0f91d6a91fb287dda325aec0892308e9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9876, - { - "block": 2906789, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:30", - "trx_id": "aea657de082fbb7451f9ffd82d3cbf5430d632b3", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9877, - { - "block": 2906790, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:33", - "trx_id": "12acc779dc54917a6c7984cd04a967db98e7f5e8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9878, - { - "block": 2906791, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:36", - "trx_id": "67b4730d53999373cdd1f5fd9340338e96b911a7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9880, - { - "block": 2906793, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:42", - "trx_id": "47a7f8680939ebcce1611a64fcaa3f80892924b6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9881, - { - "block": 2906793, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:42", - "trx_id": "c6ec2cd1e2d18759e655e8accd7648036611909b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9882, - { - "block": 2906793, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:42", - "trx_id": "e5fa038f5e6d6e1830cdbda97f6d3dc692fc1c29", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9883, - { - "block": 2906794, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:45", - "trx_id": "ae227d7261ebfcdcc1b170bb15601c048311b6f7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9884, - { - "block": 2906794, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:45", - "trx_id": "e7090afe2235c70b90f71803d8aeb6b4123e353a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9885, - { - "block": 2906794, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:45", - "trx_id": "e02b02032b00113fceef297ebec9375e0ed12db8", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9886, - { - "block": 2906795, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:48", - "trx_id": "9a173e4d88d950de0735a6ffa867e71d14ad423b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9887, - { - "block": 2906796, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:51", - "trx_id": "85c8d7bc4c1b73d01c8640af5b365e85e11bc673", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9888, - { - "block": 2906796, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:51", - "trx_id": "f131d0afc748a4be3da8246d08c9a6a4eec27773", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9889, - { - "block": 2906796, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:51", - "trx_id": "f36aa3d32af76c61916472e063a51f6831ad1c0d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9890, - { - "block": 2906797, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:54", - "trx_id": "f982f74e6f0fb93ce3dd066a1fe1deba2cc781a3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9891, - { - "block": 2906797, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:54", - "trx_id": "ab4c4d61e01420f29a5d5d910f148cc1a1ae9280", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9892, - { - "block": 2906798, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:57", - "trx_id": "4afc9862af94c14554f48385dfaaf7c98bd04e38", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9893, - { - "block": 2906798, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:12:57", - "trx_id": "e69de3cf3b0a7f8cab1cb192b207b224501093e7", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9894, - { - "block": 2906799, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:00", - "trx_id": "fdc984b19edb76100d09330aa3b67bc6b7e0b08e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9895, - { - "block": 2906799, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:00", - "trx_id": "21bf7257d27f88c608538640a97d9259f76f0216", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9896, - { - "block": 2906800, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:03", - "trx_id": "2fd0180410434d96b585944513c180c25d3e8a17", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9897, - { - "block": 2906800, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:03", - "trx_id": "61fa550bd228206e51546a5048a1016e1585fbb8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9898, - { - "block": 2906801, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:06", - "trx_id": "023aa272aa9a5ad8a38ea3519801de2abe41a8d4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9899, - { - "block": 2906801, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:06", - "trx_id": "3f5497619b1e6d2019c1024803f37b2dd0819125", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9900, - { - "block": 2906802, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:09", - "trx_id": "24317b20a28bd34dde03225d7cf663488253fa34", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9901, - { - "block": 2906802, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:09", - "trx_id": "900bb0d96a28add8c999de61190463666f0bf7a3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9902, - { - "block": 2906803, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:12", - "trx_id": "6ea192f2a403eba1b358c213dc0e11ac7a6572ff", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9903, - { - "block": 2906803, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:12", - "trx_id": "d2127423c8495cba9f236bab78209e902f711d41", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9904, - { - "block": 2906804, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:15", - "trx_id": "ff11c9f06506072a9a869960f0b2233c95751af9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9905, - { - "block": 2906805, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:18", - "trx_id": "56a64db6e3b3929808d1ff5a6c9d037fec334945", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9906, - { - "block": 2906805, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:18", - "trx_id": "eba488f686d46a8a72dced189bf96f081dc2d94d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9907, - { - "block": 2906806, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:21", - "trx_id": "5cefd6a3347b130cbde42d0f9615c921b44b38c2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9908, - { - "block": 2906806, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:21", - "trx_id": "695213f887f0437be8aa9af10d6316f68fba3d09", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9909, - { - "block": 2906806, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:21", - "trx_id": "5b1ff447efcb4e010505c84b7757914da916246d", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9910, - { - "block": 2906807, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:24", - "trx_id": "0a08d364fa5b9bc9c08af8f011243f57257e6650", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9911, - { - "block": 2906807, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:24", - "trx_id": "a86f9f1b5010816411a008c8d1d71c76ac4052fe", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9912, - { - "block": 2906808, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:27", - "trx_id": "a5565a38ad8d3e2199147ea8f9188572b395a69a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9913, - { - "block": 2906808, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:14:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:27", - "trx_id": "08e7af4c13b89021e5678e58903aa1728b38db50", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9914, - { - "block": 2906809, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:30", - "trx_id": "4d103266c70047b6641d410381843a854c4df775", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9915, - { - "block": 2906809, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:30", - "trx_id": "c556fa0996c1582744a4b871c1d14e82cdfa9b33", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9916, - { - "block": 2906810, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:33", - "trx_id": "0f4e4f03bb4a271378785d7d94539910f2adfb86", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9917, - { - "block": 2906811, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:36", - "trx_id": "91f6ec1c0b89dd7688554bdb8734253e1f5db19d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9918, - { - "block": 2906811, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:36", - "trx_id": "963db9bba96e7248e1935f49aaf24b3e81d89d2d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9919, - { - "block": 2906811, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:36", - "trx_id": "e5da1ced1f98cbaa0efe3148ef9102d001a0a014", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9920, - { - "block": 2906812, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:39", - "trx_id": "a9980350c1836b4439b2ec1dcabeea94946b506f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9921, - { - "block": 2906813, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:42", - "trx_id": "17433430a091db693dff4c1cf90f7b845c6ba8fc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9922, - { - "block": 2906813, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:42", - "trx_id": "870a3920a66e482ab9f09d4f52831e9cf6016103", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9923, - { - "block": 2906814, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:45", - "trx_id": "08ccf2b644b0f4f04c2aa4c10aab23584674a5e1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9924, - { - "block": 2906815, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:48", - "trx_id": "9c1a19e6e6be10de46079794790500a6a6b4db74", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9925, - { - "block": 2906815, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:48", - "trx_id": "618af9c2c18d001675566fbbc99d6fedc4bc9b5c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9926, - { - "block": 2906816, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:51", - "trx_id": "8d91c1ee77f0f34dcc36a6ce287bb9bb1e76c69a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9927, - { - "block": 2906816, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:51", - "trx_id": "c17de81a6e4f767d28c0e4786990840aa836591f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9928, - { - "block": 2906816, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:51", - "trx_id": "80e96ad8e1ef1017e76be239eb602ec3bcaecc9c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9929, - { - "block": 2906817, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:54", - "trx_id": "1c99cbab2e3f39db90703ee10c6d960084934984", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9930, - { - "block": 2906817, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:54", - "trx_id": "0b66c9d8dc85afde14fab97ceab4d2d7db2a5b0c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9931, - { - "block": 2906818, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:13:57", - "trx_id": "368b12b076a94e2a030b50065e72b72d48773d62", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9932, - { - "block": 2906819, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:00", - "trx_id": "8284a17632701cedac4f9d94356ba5602479e7b8", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9933, - { - "block": 2906820, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:03", - "trx_id": "3c1558128482390348cc795b282459d5a62f6533", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9934, - { - "block": 2906820, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:03", - "trx_id": "f06dfc54950427f430315b8ce8dd3bf98146641e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9935, - { - "block": 2906821, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:06", - "trx_id": "753fef8335920b4fcb2e0cfc16ed512797bd47ac", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9936, - { - "block": 2906821, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:06", - "trx_id": "0af2dc68048ad499f910309d5f6969a0039bcbff", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9937, - { - "block": 2906822, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:09", - "trx_id": "d23cee3fd265dd5b10a1223da647d66290058894", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9938, - { - "block": 2906822, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:09", - "trx_id": "95f565ea562e11aeff4f763fb87d02af8ec7ed6a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9939, - { - "block": 2906823, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:12", - "trx_id": "31b96f483d1ba26bab4d5c801ca829839abd65e5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9940, - { - "block": 2906823, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:12", - "trx_id": "4f3f750f33d379491b625104ca9bf0c512bada59", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9941, - { - "block": 2906824, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:15", - "trx_id": "31b22c4156f079e4961dc9ec5d36564ff685ff98", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9942, - { - "block": 2906824, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:15", - "trx_id": "f5cc56389f79fb7eda456101f39f618df077f7ba", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9943, - { - "block": 2906825, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:18", - "trx_id": "f02c1b4d283b85b51e46c41aab901ba859065227", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9944, - { - "block": 2906825, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:18", - "trx_id": "1bf8142b30fc47e43a685bfb0ab64f6b8080f8e3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9945, - { - "block": 2906826, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:21", - "trx_id": "d40a9b8bcbe62e5b49e0b23bb0085ac0f9b028b1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9946, - { - "block": 2906826, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:21", - "trx_id": "a00e8744e5c102d494a55639931bc4d3f9142d3c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9947, - { - "block": 2906827, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:24", - "trx_id": "8b0ec7835f644fdb24c3accde338acd4b2144906", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9948, - { - "block": 2906828, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:15:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:27", - "trx_id": "ed4add2eea5a005ad7cc14e7340dbed43a923a60", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9949, - { - "block": 2906828, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:27", - "trx_id": "eebcb93b91a88665339f6b5af44a86ca8ad48c4f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9950, - { - "block": 2906829, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:30", - "trx_id": "c5c66a0785e16511e7c0056d24128fe31e7a1d1d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9951, - { - "block": 2906829, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:30", - "trx_id": "dad11d354918623c6ce811335983b96f7fa848b1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9952, - { - "block": 2906830, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:33", - "trx_id": "66ed478e8d4a0bdd6adc5d069b209bf55e4a9a37", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9953, - { - "block": 2906831, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:36", - "trx_id": "00e07df627e1d9de76d2ad3788658a15f6740985", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9954, - { - "block": 2906831, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:36", - "trx_id": "6b09c0cdb3a329c03e68d5c68adef3ee1eaec165", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9955, - { - "block": 2906832, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:39", - "trx_id": "a43feaf915f62776f825ae062c76cd8c3a0d2c6d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9956, - { - "block": 2906832, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:39", - "trx_id": "56cbb12bb4d365c13965b4baceb4dfaa21e7af86", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9957, - { - "block": 2906833, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:42", - "trx_id": "33b09b5a891388b95a65c8480557137409debd4d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9958, - { - "block": 2906833, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:42", - "trx_id": "39f50c5303c30cac3294af6d5a7b80274206f89b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9959, - { - "block": 2906834, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:45", - "trx_id": "2d110ccaa0a802b74b907085b30f3be834840a93", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9960, - { - "block": 2906834, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:15", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:45", - "trx_id": "e8c05441de473a98f557a31fe973de8e56dee570", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9961, - { - "block": 2906835, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:48", - "trx_id": "1500543ef3b093dbd3fb4b4d64effecf711199eb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9962, - { - "block": 2906835, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:18", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:48", - "trx_id": "c37ac65e3570c3e4a13dd30ccc291701773a7705", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9963, - { - "block": 2906836, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:51", - "trx_id": "b652370846d12f7cb639902ebebe7d624c0505c9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9964, - { - "block": 2906836, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:21", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:51", - "trx_id": "1848c0e6447a0d4ebe9d032fddb98fe364b6266d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9965, - { - "block": 2906837, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:54", - "trx_id": "a7d3b5347db4b60a89536cd3ae6f3d4b5629e65b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9966, - { - "block": 2906837, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:24", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:54", - "trx_id": "2dd77fde6e80857c3f0719fcf2c7a41d5564c6f9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9967, - { - "block": 2906838, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:57", - "trx_id": "043e34edc4eb1b1a3818be16be08f19fa5c34858", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9968, - { - "block": 2906838, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:27", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:14:57", - "trx_id": "cd3a152ef4902decfc8ece2af00ff3cc56668744", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9969, - { - "block": 2906839, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:00", - "trx_id": "b0d3568f1f62e0858f2126a2acf66979eab19a50", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9970, - { - "block": 2906839, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:30", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:00", - "trx_id": "87a3ef0a516ecc71c3f5bc77dc45c5c83f3de835", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9971, - { - "block": 2906840, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:03", - "trx_id": "2f4ae3fa3c26b0687292b3846303d8e9c1955944", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9972, - { - "block": 2906840, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:33", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:03", - "trx_id": "93618cc98cf4a4e855c157e9bb435f8f8046303c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9973, - { - "block": 2906841, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:06", - "trx_id": "28387b1ea9360be438f9c50703500e36080ef5af", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9974, - { - "block": 2906841, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:36", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:06", - "trx_id": "d77974703d598a0999b2afeaf9aa259ec94da1ae", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9975, - { - "block": 2906842, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:09", - "trx_id": "ff530ac10735e9242aa1cd0a81cd39549ef5bd48", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9976, - { - "block": 2906842, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:09", - "trx_id": "d38d4bbe7442249c5d652505f04f4177e5613d32", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9977, - { - "block": 2906843, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:12", - "trx_id": "2785c49bd2e8f2029daba09f8122a072caf9998b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9978, - { - "block": 2906843, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:42", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:12", - "trx_id": "0c50984ac85bd012f3c4a93b46b81ad3c5f49a0f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9979, - { - "block": 2906844, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:15", - "trx_id": "2ee66edc066935f4be46efacd7c2774a56b04b59", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9980, - { - "block": 2906845, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:18", - "trx_id": "ad0f4e1a039c58343e43de57e05c7313ad8e7039", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9981, - { - "block": 2906845, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:18", - "trx_id": "0ea0ca9f9af16893eef3a0e0e0d1b1152775b8d5", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9982, - { - "block": 2906845, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:18", - "trx_id": "f3b172384040c398762c7cfa22a20f87bc7e9272", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 9983, - { - "block": 2906846, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:21", - "trx_id": "d3ab7ff6d2a8720933273c4d83c01f23ce53cd36", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9984, - { - "block": 2906846, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:51", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:21", - "trx_id": "34123347c3d375a927dbe7630b208c0cd88b1cd4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9985, - { - "block": 2906847, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:24", - "trx_id": "f36ac8be9757c6d29f6c90d01da456eb61feee06", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9986, - { - "block": 2906847, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000013", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "487792", - "nai": "@@000000021", - "precision": 3 - }, - "orderid": 20001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:24", - "trx_id": "68df070243673adcd24f237b69b1b51e9540f303", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9987, - { - "block": 2906847, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:54", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:24", - "trx_id": "2a8d8dc2aa1e1eab14d66ed0f1db2eb541d21bf1", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9988, - { - "block": 2906848, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:27", - "trx_id": "c301bdcea80a29c2f6db551d27e8ba2cfca3f31b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9989, - { - "block": 2906849, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:16:57", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:30", - "trx_id": "6a49148b67af96fc60039045bf92b5c33e8b49ee", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9990, - { - "block": 2906849, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:30", - "trx_id": "2f23648cd6776e366e79da3f6cdcbfcdfe425fd7", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 9991, - { - "block": 2906850, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:17:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:33", - "trx_id": "456e97b356e06ebfec154382d6fc563d0d73fde4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9992, - { - "block": 2906850, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:33", - "trx_id": "e41d46c9455aaad94953ae57a23e6e97032921ec", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9993, - { - "block": 2906850, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:17:03", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:33", - "trx_id": "cbddb7659473cefd99c15bf53b74e17c23149cfa", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 9994, - { - "block": 2906851, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:36", - "trx_id": "4a3f15247f74e79ef48f80893a199f45594f59a6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9995, - { - "block": 2906851, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:17:06", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:36", - "trx_id": "dc28ce1695fa24c4956ea96d5521a29f5b791f2a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9996, - { - "block": 2906852, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:39", - "trx_id": "4935f01cb7f4a7c3a707d3aaaeb85309e6843cde", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9997, - { - "block": 2906852, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:17:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:39", - "trx_id": "49d1884fb3516f1bf21d43a5f9c118256b9fb46a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 9998, - { - "block": 2906853, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:42", - "trx_id": "68f67b5882fb12522fca57e9008889249e5a767e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9999, - { - "block": 2906853, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "200000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-07-04T15:17:12", - "fill_or_kill": false, - "min_to_receive": { - "amount": "92598", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:42", - "trx_id": "003e61248c81f1df26541dc17048fa0efda71684", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 10000, - { - "block": 2906854, - "op": { - "type": "limit_order_cancel_operation", - "value": { - "orderid": 10001, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T15:15:45", - "trx_id": "19786de74ec1f26c4001c94a38b209cf7b4aaa06", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_and_cancel.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_and_cancel.tavern.yaml deleted file mode 100644 index 616d0379886fa5db3c2e248fb1c4b273f61dab21..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_and_cancel.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api limit_order create and cancel operations - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api limit_order create and cancel - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"adm", "operation_filter_low": 96, "start": 10000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "limit_order_create_and_cancel" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_operation.pat.json deleted file mode 100644 index 9015a667fe11ea51177257ac3a6d75791dc2d078..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_operation.pat.json +++ /dev/null @@ -1,283 +0,0 @@ -{ - "history": [ - [ - 992, - { - "block": 1939739, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-05-31T21:44:00", - "fill_or_kill": false, - "min_to_receive": { - "amount": "1000", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 1, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-31T21:39:00", - "trx_id": "af06cce9f6f5f81752aeb09d257b4b915c286e89", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 993, - { - "block": 1939852, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "2000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-05-31T21:49:39", - "fill_or_kill": false, - "min_to_receive": { - "amount": "3000", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 1, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-31T21:44:39", - "trx_id": "c0284c619aea02bb1a734b1e697dd5445703161b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 994, - { - "block": 1939862, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "4000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-05-31T21:50:09", - "fill_or_kill": false, - "min_to_receive": { - "amount": "5000", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 2, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-31T21:45:09", - "trx_id": "512f274e1f15b672d55a15cdeca603fa0e906dac", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 995, - { - "block": 1939872, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "2000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-05-31T21:50:38", - "fill_or_kill": false, - "min_to_receive": { - "amount": "6000", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 3, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-31T21:45:39", - "trx_id": "174518a2978b7206b0a926fec68aa53c8e90ea7d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 996, - { - "block": 1940062, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "2000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-05-31T22:00:11", - "fill_or_kill": false, - "min_to_receive": { - "amount": "6000", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 1, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-31T21:55:09", - "trx_id": "6ae436bd822b6e4f62eca1e9d9695d6477aa0a24", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 997, - { - "block": 1972388, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "2000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-06-02T01:00:48", - "fill_or_kill": false, - "min_to_receive": { - "amount": "6000", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 1, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-02T00:55:48", - "trx_id": "e7778bd0e76a4e26872f58e21e506a3a01099865", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 998, - { - "block": 1972548, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-06-02T01:08:56", - "fill_or_kill": false, - "min_to_receive": { - "amount": "6000", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 1, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-02T01:03:54", - "trx_id": "0f401ada3d10ae029a45598c8444496f887cf180", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 999, - { - "block": 1972585, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "2000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-06-02T01:10:45", - "fill_or_kill": false, - "min_to_receive": { - "amount": "7000", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 2, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-02T01:05:45", - "trx_id": "d61a9d787ea2870d6b82d939220b2fd8c1b203a3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 1000, - { - "block": 1972848, - "op": { - "type": "limit_order_create_operation", - "value": { - "amount_to_sell": { - "amount": "2000", - "nai": "@@000000021", - "precision": 3 - }, - "expiration": "2016-06-02T01:23:58", - "fill_or_kill": false, - "min_to_receive": { - "amount": "7000", - "nai": "@@000000013", - "precision": 3 - }, - "orderid": 2, - "owner": "adm" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-02T01:18:57", - "trx_id": "7a0073e773f7c605bc957a3014fb4720442a797d", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_operation.tavern.yaml deleted file mode 100644 index 7bfc02f388cfd7947e7a69d1553cf44ad49a25df..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/limit_order_create_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to limit_order_create_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history limit_order_create_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"adm","operation_filter_low": 32,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "limit_order_create_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/liquidity_reward_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/liquidity_reward_operation.pat.json deleted file mode 100644 index 74aa122cd7a3d29a3a9b04a7676b971529d4b77a..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/liquidity_reward_operation.pat.json +++ /dev/null @@ -1,165 +0,0 @@ -{ - "history": [ - [ - 15232, - { - "block": 2937600, - "op": { - "type": "liquidity_reward_operation", - "value": { - "owner": "adm", - "payout": { - "amount": "1200000", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T17:10:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 15342, - { - "block": 2938800, - "op": { - "type": "liquidity_reward_operation", - "value": { - "owner": "adm", - "payout": { - "amount": "1200000", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T18:10:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 15457, - { - "block": 2940000, - "op": { - "type": "liquidity_reward_operation", - "value": { - "owner": "adm", - "payout": { - "amount": "1200000", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T19:10:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 15577, - { - "block": 2941200, - "op": { - "type": "liquidity_reward_operation", - "value": { - "owner": "adm", - "payout": { - "amount": "1200000", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T20:11:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 15701, - { - "block": 2942400, - "op": { - "type": "liquidity_reward_operation", - "value": { - "owner": "adm", - "payout": { - "amount": "1200000", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T21:11:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 15812, - { - "block": 2943600, - "op": { - "type": "liquidity_reward_operation", - "value": { - "owner": "adm", - "payout": { - "amount": "1200000", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T22:14:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 15915, - { - "block": 2944800, - "op": { - "type": "liquidity_reward_operation", - "value": { - "owner": "adm", - "payout": { - "amount": "1200000", - "nai": "@@000000021", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T23:14:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/liquidity_reward_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/liquidity_reward_operation.tavern.yaml deleted file mode 100644 index 7632b0e2e0a72e3b04cbe28f3eeea47ea1dd733a..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/liquidity_reward_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api liquidity_reward_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api liquidity_reward_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"adm", "operation_filter_low": 4503599627370496, "start": 15915, "limit": 7} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "liquidity_reward_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/only_account.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/only_account.pat.json deleted file mode 100644 index 59aab7691513e749fba5588f8ed3088a7c3eb413..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/only_account.pat.json +++ /dev/null @@ -1,24474 +0,0 @@ -{ - "history": [ - [ - 21420, - { - "block": 4636980, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "voter": "ihashfury", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T04:04:51", - "trx_id": "f5b6ee9b862a31f6f634175bebbfa6bffbbee4b5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21421, - { - "block": 4638421, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "553", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "rshares": "32643849525", - "total_vote_weight": "3829413232259789320", - "voter": "ihash", - "weight": "95142689658372277" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T05:16:57", - "trx_id": "a72737d1495f7c844dcb83f7e6f3f8980caaaafa", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21422, - { - "block": 4638421, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "voter": "ihash", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T05:16:57", - "trx_id": "a72737d1495f7c844dcb83f7e6f3f8980caaaafa", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21423, - { - "block": 4641586, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "mammasitta", - "pending_payout": { - "amount": "47513", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "kaffeehaus-culture-the-viennese-coffee-house-an-intangible-cultural-heritage-and-the-secession-an-artist-movement", - "rshares": "164730268571", - "total_vote_weight": "15264579291642129899", - "voter": "camilla", - "weight": "22768549144487203" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T07:55:27", - "trx_id": "815b2329001e75d120386be8367aae62d04ac25c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21424, - { - "block": 4641586, - "op": { - "type": "vote_operation", - "value": { - "author": "mammasitta", - "permlink": "kaffeehaus-culture-the-viennese-coffee-house-an-intangible-cultural-heritage-and-the-secession-an-artist-movement", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T07:55:27", - "trx_id": "815b2329001e75d120386be8367aae62d04ac25c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21425, - { - "block": 4641650, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "juliac", - "pending_payout": { - "amount": "76", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "mythical-creature-original-artwork", - "rshares": "164730268571", - "total_vote_weight": "763455956958478835", - "voter": "camilla", - "weight": "243476636684815717" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T07:58:39", - "trx_id": "edea19cfe8aebffbf02ad36164a9bea2c7414170", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21426, - { - "block": 4641650, - "op": { - "type": "vote_operation", - "value": { - "author": "juliac", - "permlink": "mythical-creature-original-artwork", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T07:58:39", - "trx_id": "edea19cfe8aebffbf02ad36164a9bea2c7414170", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21427, - { - "block": 4641682, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "ndbeledrifts", - "pending_payout": { - "amount": "93", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "i-met-a-girl-from-germany-once", - "rshares": "164730268571", - "total_vote_weight": "919116914030368039", - "voter": "camilla", - "weight": "713798441564533819" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:00:15", - "trx_id": "7d0ec7b9ca709d0c4ef6c320efb39367b86ee22f", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21428, - { - "block": 4641682, - "op": { - "type": "vote_operation", - "value": { - "author": "ndbeledrifts", - "permlink": "i-met-a-girl-from-germany-once", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:00:15", - "trx_id": "7d0ec7b9ca709d0c4ef6c320efb39367b86ee22f", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21429, - { - "block": 4641717, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "alexbeyman", - "pending_payout": { - "amount": "34999", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "psychedelics-the-beautiful-bossy-dryad-my-encounters-with-forest-intelligence", - "rshares": "164730268571", - "total_vote_weight": "14802732646898194280", - "voter": "camilla", - "weight": "29888265851259884" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:02:00", - "trx_id": "6658deb8e94aad374df3c9005bec6c26eee12728", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21430, - { - "block": 4641717, - "op": { - "type": "vote_operation", - "value": { - "author": "alexbeyman", - "permlink": "psychedelics-the-beautiful-bossy-dryad-my-encounters-with-forest-intelligence", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:02:00", - "trx_id": "6658deb8e94aad374df3c9005bec6c26eee12728", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21431, - { - "block": 4641731, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "opheliafu", - "pending_payout": { - "amount": "617618", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "i-was-a-different-person-yesterday", - "rshares": "160506415531", - "total_vote_weight": "17503335622713708578", - "voter": "camilla", - "weight": "1940011779435887" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:02:42", - "trx_id": "0dc66c2630085e6793cbc607a3d15c1cd10e5e96", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21432, - { - "block": 4641731, - "op": { - "type": "vote_operation", - "value": { - "author": "opheliafu", - "permlink": "i-was-a-different-person-yesterday", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:02:42", - "trx_id": "0dc66c2630085e6793cbc607a3d15c1cd10e5e96", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21433, - { - "block": 4642714, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "586", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "rshares": "63316513317", - "total_vote_weight": "4010488821265777010", - "voter": "jason", - "weight": "181075589005987690" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:51:51", - "trx_id": "3314d662a860b169ba84ce9b4c4bcc542598da93", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21434, - { - "block": 4642714, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "voter": "jason", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T08:51:51", - "trx_id": "3314d662a860b169ba84ce9b4c4bcc542598da93", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21435, - { - "block": 4643114, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "opheliafu", - "pending_payout": { - "amount": "44433", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "say-hello-to-big-bob", - "rshares": "164730268571", - "total_vote_weight": "15228331304238682767", - "voter": "camilla", - "weight": "23292114192878337" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:11:54", - "trx_id": "f1d33cf9b8f051a2350957ed75fa72d431919c4b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21436, - { - "block": 4643114, - "op": { - "type": "vote_operation", - "value": { - "author": "opheliafu", - "permlink": "say-hello-to-big-bob", - "voter": "camilla", - "weight": 7700 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:11:54", - "trx_id": "f1d33cf9b8f051a2350957ed75fa72d431919c4b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21437, - { - "block": 4643118, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "127675", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "pura-vida-my-experience-working-on-a-permaculture-farm-in-costa-rica", - "rshares": "206968798974", - "total_vote_weight": "16472049860403995460", - "voter": "camilla", - "weight": "10998591732923581" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:12:06", - "trx_id": "f51caa9d51dc7db146b392848e03863c2e32b209", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21438, - { - "block": 4643118, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "pura-vida-my-experience-working-on-a-permaculture-farm-in-costa-rica", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:12:06", - "trx_id": "f51caa9d51dc7db146b392848e03863c2e32b209", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21439, - { - "block": 4643121, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "89", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-sustainable-food-dialogue-an-overview-of-vertical-farming", - "rshares": "206968798974", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:12:15", - "trx_id": "9ce855db47f09bf5b3d72b12be94aecd9bfc2ad7", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21440, - { - "block": 4643121, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "a-sustainable-food-dialogue-an-overview-of-vertical-farming", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:12:15", - "trx_id": "9ce855db47f09bf5b3d72b12be94aecd9bfc2ad7", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21441, - { - "block": 4643140, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "allasyummyfood", - "pending_payout": { - "amount": "25821", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "alla-goes-to-usa-part-1-san-francisco", - "rshares": "206968798974", - "total_vote_weight": "14329012925793520767", - "voter": "camilla", - "weight": "47797748458381905" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:13:12", - "trx_id": "ced73ce0a0918a9d113b69c9c1b5ddcaaab271be", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21442, - { - "block": 4643140, - "op": { - "type": "vote_operation", - "value": { - "author": "allasyummyfood", - "permlink": "alla-goes-to-usa-part-1-san-francisco", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:13:12", - "trx_id": "ced73ce0a0918a9d113b69c9c1b5ddcaaab271be", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21443, - { - "block": 4643156, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "allasyummyfood", - "pending_payout": { - "amount": "120", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "russian-napoleon-cake-recipe-steemit-food-challenge", - "rshares": "206968798974", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:14:00", - "trx_id": "b262fc00a8a5ac932dbb2c57127480913ca45cc9", - "trx_in_block": 11, - "virtual_op": 1 - } - ], - [ - 21444, - { - "block": 4643156, - "op": { - "type": "vote_operation", - "value": { - "author": "allasyummyfood", - "permlink": "russian-napoleon-cake-recipe-steemit-food-challenge", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:14:00", - "trx_id": "b262fc00a8a5ac932dbb2c57127480913ca45cc9", - "trx_in_block": 11, - "virtual_op": 0 - } - ], - [ - 21445, - { - "block": 4643207, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "162", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "anwen-meditates-a-steemit-health-channel-11-yoga-poses-for-digestion-plus-fresh-squeezed-pear-juice", - "rshares": "202744945934", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:16:33", - "trx_id": "9bb95156c7ca92d056b763a9318934717dabc521", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21446, - { - "block": 4643207, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "anwen-meditates-a-steemit-health-channel-11-yoga-poses-for-digestion-plus-fresh-squeezed-pear-juice", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T09:16:33", - "trx_id": "9bb95156c7ca92d056b763a9318934717dabc521", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21447, - { - "block": 4647979, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "anca3drandom", - "comment_permlink": "steemart-the-art-of-quilling-paper-steemit-logo", - "curator": "camilla", - "reward": { - "amount": "80336806", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T13:15:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 38 - } - ], - [ - 21448, - { - "block": 4648623, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "7", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-alexft-re-camilla-the-castle-from-my-dreams-20160830t141415563z", - "rshares": "17043021152", - "total_vote_weight": 0, - "voter": "fury", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T13:47:45", - "trx_id": "9cda2a9835a6c967a50be5553e5efb2b1c3ac9a7", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21449, - { - "block": 4648623, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-alexft-re-camilla-the-castle-from-my-dreams-20160830t141415563z", - "voter": "fury", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T13:47:45", - "trx_id": "9cda2a9835a6c967a50be5553e5efb2b1c3ac9a7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21450, - { - "block": 4650309, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "favorit", - "comment_permlink": "enchanted-forest", - "curator": "camilla", - "reward": { - "amount": "77087879", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T15:12:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21451, - { - "block": 4650600, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "dabaisha", - "comment_permlink": "7-major-anti-inflammatory-food-eating-a-healthy-save-beer-belly", - "curator": "camilla", - "reward": { - "amount": "80295266", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T15:26:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 21452, - { - "block": 4650906, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "mrlee", - "comment_permlink": "beautiful-macarons", - "curator": "camilla", - "reward": { - "amount": "80290443", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T15:42:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 21453, - { - "block": 4651096, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "tanyabtc", - "comment_permlink": "dragon-ferocious", - "curator": "camilla", - "reward": { - "amount": "80287412", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T15:51:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 15 - } - ], - [ - 21454, - { - "block": 4651244, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "jpiper20", - "comment_permlink": "hand-drawn-typography-from-this-week", - "curator": "camilla", - "reward": { - "amount": "105976313", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T15:58:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 28 - } - ], - [ - 21455, - { - "block": 4653163, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "storyseeker", - "comment_permlink": "my-process-step-by-step-start-to-finish-intricate-collage-and-acrylic-painting", - "curator": "camilla", - "reward": { - "amount": "4118671564", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T17:35:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 21456, - { - "block": 4653405, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "587", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "rshares": 3820934326, - "total_vote_weight": "4021272685842403703", - "voter": "sompitonov", - "weight": "10783864576626693" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T17:47:09", - "trx_id": "50186445bdd28b5086ce61de163cd827cecbef20", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21457, - { - "block": 4653405, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-sompitonov-re-camilla-re-sompitonov-re-camilla-do-you-like-to-colour-20160902t173457804z", - "voter": "sompitonov", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T17:47:09", - "trx_id": "50186445bdd28b5086ce61de163cd827cecbef20", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21458, - { - "block": 4655414, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "opheliafu", - "comment_permlink": "i-was-a-different-person-yesterday", - "curator": "camilla", - "reward": { - "amount": "60966556", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T19:27:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 54 - } - ], - [ - 21459, - { - "block": 4656578, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "yangyang", - "comment_permlink": "mermaid-drawing", - "curator": "camilla", - "reward": { - "amount": "285514715", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T20:26:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21460, - { - "block": 4657501, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alexbeyman", - "comment_permlink": "psychedelics-the-beautiful-bossy-dryad-my-encounters-with-forest-intelligence", - "curator": "camilla", - "reward": { - "amount": "60941504", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-03T21:12:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 17 - } - ], - [ - 21461, - { - "block": 4663596, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "allasyummyfood", - "comment_permlink": "alla-goes-to-usa-part-1-san-francisco", - "curator": "camilla", - "reward": { - "amount": "108922513", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T02:17:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 29 - } - ], - [ - 21462, - { - "block": 4669230, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "mammasitta", - "comment_permlink": "kaffeehaus-culture-the-viennese-coffee-house-an-intangible-cultural-heritage-and-the-secession-an-artist-movement", - "curator": "camilla", - "reward": { - "amount": "70401302", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T06:59:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 21463, - { - "block": 4669469, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "ndbeledrifts", - "comment_permlink": "i-met-a-girl-from-germany-once", - "curator": "camilla", - "reward": { - "amount": "63998182", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T07:11:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21464, - { - "block": 4671716, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "juliac", - "comment_permlink": "mythical-creature-original-artwork", - "curator": "camilla", - "reward": { - "amount": "422201508", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T09:04:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21465, - { - "block": 4672446, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "anwenbaumeister", - "comment_permlink": "pura-vida-my-experience-working-on-a-permaculture-farm-in-costa-rica", - "curator": "camilla", - "reward": { - "amount": "313408097", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T09:41:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 42 - } - ], - [ - 21466, - { - "block": 4673465, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "opheliafu", - "comment_permlink": "say-hello-to-big-bob", - "curator": "camilla", - "reward": { - "amount": "687441625", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T10:34:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 18 - } - ], - [ - 21467, - { - "block": 4675025, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "82", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colours-of-a-peacock", - "rshares": "186528421973", - "total_vote_weight": 0, - "voter": "jamielefay", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T11:54:24", - "trx_id": "78e39e4fd8a59c73058aabb3112230750d460460", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21468, - { - "block": 4675025, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colours-of-a-peacock", - "voter": "jamielefay", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T11:54:24", - "trx_id": "78e39e4fd8a59c73058aabb3112230750d460460", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21469, - { - "block": 4675057, - "op": { - "type": "comment_operation", - "value": { - "author": "jamielefay", - "body": "Soooo beautiful. All my favourite colours. Hope you post more art soon. J.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colours-of-a-peacock", - "permlink": "re-camilla-colours-of-a-peacock-20160904t115600052z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T11:56:00", - "trx_id": "9aefb0b8dd9fde1e3ccbbeca7b896b381e5213a5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21470, - { - "block": 4681217, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-pixielolz-can-steemit-take-some-fanart--20160807t105639754z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T17:04:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 15 - } - ], - [ - 21471, - { - "block": 4681217, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "pixielolz", - "comment_permlink": "re-camilla-re-pixielolz-can-steemit-take-some-fanart--20160807t183600081z", - "curator": "camilla", - "reward": { - "amount": "76621057", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-04T17:04:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 21472, - { - "block": 4694018, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "274", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "the-magic-world-of-books", - "vesting_payout": { - "amount": "1031792689", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-05T03:45:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21473, - { - "block": 4694018, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 647, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "123", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "549", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-magic-world-of-books", - "total_payout_value": { - "amount": "2309", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-05T03:45:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 21474, - { - "block": 4694018, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "the-magic-world-of-books" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-05T03:45:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21475, - { - "block": 4694018, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alexft", - "comment_permlink": "re-camilla-the-magic-world-of-books-20160809t081535352z", - "curator": "camilla", - "reward": { - "amount": "76429088", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-05T03:45:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 11 - } - ], - [ - 21476, - { - "block": 4724362, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-inkha-when-i-see-my-steem-friends-are-active-but-haven-t-upvoted-my-new-post-yet-20160806t170640062z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:05:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 21477, - { - "block": 4725117, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "crazy-flood-on-the-subway-tracks" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21478, - { - "block": 4725117, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-xapo-re-camilla-crazy-flood-on-the-subway-tracks-20160806t171143178z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21479, - { - "block": 4725117, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-gidlark-re-camilla-crazy-flood-on-the-subway-tracks-20160806t212819558z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 21480, - { - "block": 4725117, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-btcbtcbtc20155-re-camilla-crazy-flood-on-the-subway-tracks-20160806t212932042z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 9 - } - ], - [ - 21481, - { - "block": 4725117, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alexft", - "comment_permlink": "re-camilla-crazy-flood-on-the-subway-tracks-20160807t062503313z", - "curator": "camilla", - "reward": { - "amount": "79130255", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 10 - } - ], - [ - 21482, - { - "block": 4725117, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "joelinux", - "comment_permlink": "re-camilla-crazy-flood-on-the-subway-tracks-20160810t164432985z", - "curator": "camilla", - "reward": { - "amount": "82295466", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T05:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 21483, - { - "block": 4727278, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-sphenix-malaysia-food-chinese-food-wan-ton-mee-another-hong-kong-style-20160806t170453956z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:31:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 21484, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "my-ladybug-drawing" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21485, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-saharov-re-camilla-my-ladybug-drawing-20160806t130140608z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21486, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-btcbtcbtc20155-re-camilla-my-ladybug-drawing-20160806t131159046z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 9 - } - ], - [ - 21487, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-camilla-re-btcbtcbtc20155-re-camilla-my-ladybug-drawing-20160806t131222091z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 10 - } - ], - [ - 21488, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-mun-re-camilla-my-ladybug-drawing-20160806t131509504z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 11 - } - ], - [ - 21489, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-yonuts-re-camilla-my-ladybug-drawing-20160806t133934665z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 18 - } - ], - [ - 21490, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-picker-re-camilla-my-ladybug-drawing-20160806t134002505z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 19 - } - ], - [ - 21491, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-btcbtcbtc20155-re-camilla-re-camilla-re-btcbtcbtc20155-re-camilla-my-ladybug-drawing-20160806t134043064z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 20 - } - ], - [ - 21492, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-alexft-re-camilla-my-ladybug-drawing-20160806t205601159z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 24 - } - ], - [ - 21493, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-richman-re-camilla-my-ladybug-drawing-20160806t205631480z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 25 - } - ], - [ - 21494, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-fairytalelife-re-camilla-my-ladybug-drawing-20160806t205859483z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 26 - } - ], - [ - 21495, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-alexft-re-camilla-re-alexft-re-camilla-my-ladybug-drawing-20160806t212406972z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 29 - } - ], - [ - 21496, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-cryptoiskey-re-camilla-my-ladybug-drawing-20160806t212537411z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 30 - } - ], - [ - 21497, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-acidyo-re-camilla-my-ladybug-drawing-20160806t232502838z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 34 - } - ], - [ - 21498, - { - "block": 4727781, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "monopoly-man", - "comment_permlink": "re-camilla-my-ladybug-drawing-20160807t081105049z", - "curator": "camilla", - "reward": { - "amount": "75925581", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 49 - } - ], - [ - 21499, - { - "block": 4727781, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-camilla-re-alexft-re-camilla-re-alexft-re-camilla-my-ladybug-drawing-20160807t105359677z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 54 - } - ], - [ - 21500, - { - "block": 4727781, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "helle", - "comment_permlink": "re-camilla-my-ladybug-drawing-20160807t184458232z", - "curator": "camilla", - "reward": { - "amount": "79089147", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 55 - } - ], - [ - 21501, - { - "block": 4727781, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "juvyjabian", - "comment_permlink": "re-camilla-my-ladybug-drawing-20160808t072735516z", - "curator": "camilla", - "reward": { - "amount": "79089147", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-06T07:56:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 59 - } - ], - [ - 21502, - { - "block": 4760179, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "acidyo", - "pending_payout": { - "amount": "113950", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "gemo-dna-introduction-and-prologue-sci-fi-action-rpg-futurism", - "rshares": "215458718337", - "total_vote_weight": "2959357778273831534", - "voter": "camilla", - "weight": "5949925842861737" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T10:59:18", - "trx_id": "4f70a5bb027b1c5691b8643394acdc2b07a71a4a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21503, - { - "block": 4760179, - "op": { - "type": "vote_operation", - "value": { - "author": "acidyo", - "permlink": "gemo-dna-introduction-and-prologue-sci-fi-action-rpg-futurism", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T10:59:18", - "trx_id": "4f70a5bb027b1c5691b8643394acdc2b07a71a4a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21504, - { - "block": 4760181, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "250577", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-cross-country-road-trip-greater-than-sf-greater-than-vegas-greater-than-boulder-greater-than-houston-greater-than-new-orleans", - "rshares": "211234037585", - "total_vote_weight": "17059321300395903263", - "voter": "camilla", - "weight": "5532611969834533" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T10:59:24", - "trx_id": "ec31d3d98020404a4f1ac1a2662d6838f85d0c38", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21505, - { - "block": 4760181, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "my-cross-country-road-trip-greater-than-sf-greater-than-vegas-greater-than-boulder-greater-than-houston-greater-than-new-orleans", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T10:59:24", - "trx_id": "ec31d3d98020404a4f1ac1a2662d6838f85d0c38", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21506, - { - "block": 4760207, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "fairytalelife", - "pending_payout": { - "amount": "85", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "how-to-draw-lips", - "rshares": "211234037585", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:00:42", - "trx_id": "b1b8baf2e1839dec8f2e66bb2c16130f9d5ef7bf", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21507, - { - "block": 4760207, - "op": { - "type": "vote_operation", - "value": { - "author": "fairytalelife", - "permlink": "how-to-draw-lips", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:00:42", - "trx_id": "b1b8baf2e1839dec8f2e66bb2c16130f9d5ef7bf", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21508, - { - "block": 4760218, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "97", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "anwen-meditates-a-steemit-health-channel-how-savasana-prepares-me-for-death", - "rshares": "211234037585", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:01:15", - "trx_id": "5b8118f4915757bc21e3e71b3971339ff3102b6b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21509, - { - "block": 4760218, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "anwen-meditates-a-steemit-health-channel-how-savasana-prepares-me-for-death", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:01:15", - "trx_id": "5b8118f4915757bc21e3e71b3971339ff3102b6b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21510, - { - "block": 4760233, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "infovore", - "pending_payout": { - "amount": "596545", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-rise-of-the-human-cyborgs-outsmarting-smartphones-and-the-internables-trend", - "rshares": "211234037585", - "total_vote_weight": "17534666943612674070", - "voter": "camilla", - "weight": "2387716540162740" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:02:00", - "trx_id": "cc1ba235aee2e2e3ee7a11af6f11db3d9b4d81c0", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21511, - { - "block": 4760233, - "op": { - "type": "vote_operation", - "value": { - "author": "infovore", - "permlink": "the-rise-of-the-human-cyborgs-outsmarting-smartphones-and-the-internables-trend", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T11:02:00", - "trx_id": "cc1ba235aee2e2e3ee7a11af6f11db3d9b4d81c0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21512, - { - "block": 4769868, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "a-drawing-to-express-the-power-of-steem", - "voter": "gavicrane", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T19:04:57", - "trx_id": "201af50904ee29156e60d138ecc9a466edf5eff9", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 21513, - { - "block": 4769874, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-tessaddavis-re-camilla-a-drawing-to-express-the-power-of-steem-20160719t110026969z", - "voter": "gavicrane", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T19:05:15", - "trx_id": "6acf603f41c5eabd2d4e1cdd33ba24df7d7e287b", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21514, - { - "block": 4780729, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-anwenbaumeister-anwen-meditates-a-steemit-health-channel-a-lovingkindness-meditation-and-an-original-recipe-for-slow-cooked-root-veggies-20160808t180327396z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T04:08:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 13 - } - ], - [ - 21515, - { - "block": 4783685, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "acidyo", - "comment_permlink": "gemo-dna-introduction-and-prologue-sci-fi-action-rpg-futurism", - "curator": "camilla", - "reward": { - "amount": "291037297", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T06:36:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 31 - } - ], - [ - 21516, - { - "block": 4784890, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-pixielolz-mew-meets-steemit-speed-drawing-video-photos-20160808t180911948z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:36:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21517, - { - "block": 4784890, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-pixielolz-mew-meets-steemit-speed-drawing-video-photos-20160808t181130683z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:36:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 6 - } - ], - [ - 21518, - { - "block": 4785210, - "op": { - "type": "interest_operation", - "value": { - "interest": { - "amount": "2260", - "nai": "@@000000013", - "precision": 3 - }, - "owner": "camilla" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21519, - { - "block": 4785210, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "15", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "a-steem-whale-in-a-dream-world-art-and-poem", - "vesting_payout": { - "amount": "59441772", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 21520, - { - "block": 4785210, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 38, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "1111", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "30", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-steem-whale-in-a-dream-world-art-and-poem", - "total_payout_value": { - "amount": "4588", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21521, - { - "block": 4785210, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "a-steem-whale-in-a-dream-world-art-and-poem" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21522, - { - "block": 4785210, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-gidlark-re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160808t160539173z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 21523, - { - "block": 4785210, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-anca3drandom-re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160808t162615517z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 9 - } - ], - [ - 21524, - { - "block": 4785210, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-inkha-re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160809t180445870z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 19 - } - ], - [ - 21525, - { - "block": 4785210, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "joelinux", - "comment_permlink": "re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160810t164328721z", - "curator": "camilla", - "reward": { - "amount": "68827315", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 20 - } - ], - [ - 21526, - { - "block": 4785210, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "steemswede", - "comment_permlink": "re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160828t214107644z", - "curator": "camilla", - "reward": { - "amount": "34413657", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 24 - } - ], - [ - 21527, - { - "block": 4785210, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-steemswede-re-camilla-a-steem-whale-in-a-dream-world-art-and-poem-20160828t215937477z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T07:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 28 - } - ], - [ - 21528, - { - "block": 4785909, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "anwenbaumeister", - "comment_permlink": "my-cross-country-road-trip-greater-than-sf-greater-than-vegas-greater-than-boulder-greater-than-houston-greater-than-new-orleans", - "curator": "camilla", - "reward": { - "amount": "203326016", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T08:27:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 63 - } - ], - [ - 21529, - { - "block": 4788433, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "infovore", - "comment_permlink": "the-rise-of-the-human-cyborgs-outsmarting-smartphones-and-the-internables-trend", - "curator": "camilla", - "reward": { - "amount": "168834816", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T10:34:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 89 - } - ], - [ - 21530, - { - "block": 4815612, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "stellabelle", - "pending_payout": { - "amount": "120454", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "new-idea-for-steemit-let-s-open-our-own-bars", - "rshares": "215462930329", - "total_vote_weight": "16410291079042728394", - "voter": "camilla", - "weight": "12182373335164876" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:17:39", - "trx_id": "8eaa66efbb94cc46cd51326af05306b66c7701f2", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21531, - { - "block": 4815612, - "op": { - "type": "vote_operation", - "value": { - "author": "stellabelle", - "permlink": "new-idea-for-steemit-let-s-open-our-own-bars", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:17:39", - "trx_id": "8eaa66efbb94cc46cd51326af05306b66c7701f2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21532, - { - "block": 4815614, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "stellabelle", - "pending_payout": { - "amount": "91", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "some-benefits-of-being-an-atheist", - "rshares": "211238166990", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:17:45", - "trx_id": "3ab5d102803f963155a4423ba5438446a82aafec", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21533, - { - "block": 4815614, - "op": { - "type": "vote_operation", - "value": { - "author": "stellabelle", - "permlink": "some-benefits-of-being-an-atheist", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:17:45", - "trx_id": "3ab5d102803f963155a4423ba5438446a82aafec", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21534, - { - "block": 4815619, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "stellabelle", - "pending_payout": { - "amount": "241", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "secret-writer-my-parents-were-alcoholics-and-i-saved-my-mom-s-life-twice", - "rshares": "211238166990", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:18:00", - "trx_id": "f2cce4a3c6aabb23df5282f2704cde1d8e36043b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21535, - { - "block": 4815619, - "op": { - "type": "vote_operation", - "value": { - "author": "stellabelle", - "permlink": "secret-writer-my-parents-were-alcoholics-and-i-saved-my-mom-s-life-twice", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T09:18:00", - "trx_id": "f2cce4a3c6aabb23df5282f2704cde1d8e36043b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21536, - { - "block": 4817530, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "22214", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "fennec-fox-step-by-step-of-my-drawing-process-from-sketch-to-finished-artwork", - "rshares": "211238166990", - "total_vote_weight": "14051387273185141180", - "voter": "camilla", - "weight": "56011909777174591" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:54:03", - "trx_id": "91623ad16bedd872f8243c375ecb29b52555ecf3", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21537, - { - "block": 4817530, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "fennec-fox-step-by-step-of-my-drawing-process-from-sketch-to-finished-artwork", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:54:03", - "trx_id": "91623ad16bedd872f8243c375ecb29b52555ecf3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21538, - { - "block": 4817545, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "31174", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "copic-markers-a-beginner-s-guide", - "rshares": "211238166990", - "total_vote_weight": "14656706708420804802", - "voter": "camilla", - "weight": "41573594419093336" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:54:48", - "trx_id": "992f73454dd64e6fa7cd9d65c818e4f88ca289e3", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21539, - { - "block": 4817545, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "copic-markers-a-beginner-s-guide", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:54:48", - "trx_id": "992f73454dd64e6fa7cd9d65c818e4f88ca289e3", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21540, - { - "block": 4817547, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"pixielolz\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:54:54", - "trx_id": "b42a3f4fec38b943f5f71aaac4be130d02d3c650", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21541, - { - "block": 4817575, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "92", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "trading-card-collection-showcasing-the-cards-i-ve-made-the-last-9-months", - "rshares": "211238166990", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:56:18", - "trx_id": "bb884d2af5c220acb4b55892c657a6aa5dec0c6e", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21542, - { - "block": 4817575, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "trading-card-collection-showcasing-the-cards-i-ve-made-the-last-9-months", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:56:18", - "trx_id": "bb884d2af5c220acb4b55892c657a6aa5dec0c6e", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21543, - { - "block": 4817585, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "92", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "through-time-step-by-step-of-my-drawing-process-from-sketch-to-finished-artwork", - "rshares": "211238166990", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:56:48", - "trx_id": "1a1c70c6de09511a6a13e038f58d06d19d712b14", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21544, - { - "block": 4817585, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "through-time-step-by-step-of-my-drawing-process-from-sketch-to-finished-artwork", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:56:48", - "trx_id": "1a1c70c6de09511a6a13e038f58d06d19d712b14", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21545, - { - "block": 4817615, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "119", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "illustrations-for-silence-in-time-a-short-story-by-liberosist", - "rshares": "207013403650", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:58:18", - "trx_id": "5f870b4685657a21c8d0c3d4c84858e2a13a4a2e", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21546, - { - "block": 4817615, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "illustrations-for-silence-in-time-a-short-story-by-liberosist", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T10:58:18", - "trx_id": "5f870b4685657a21c8d0c3d4c84858e2a13a4a2e", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21547, - { - "block": 4829727, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "pixielolz", - "comment_permlink": "copic-markers-a-beginner-s-guide", - "curator": "camilla", - "reward": { - "amount": "93056250", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T21:06:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 23 - } - ], - [ - 21548, - { - "block": 4831404, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "pixielolz", - "comment_permlink": "fennec-fox-step-by-step-of-my-drawing-process-from-sketch-to-finished-artwork", - "curator": "camilla", - "reward": { - "amount": "71320251", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T22:32:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 21549, - { - "block": 4832860, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "stellabelle", - "comment_permlink": "new-idea-for-steemit-let-s-open-our-own-bars", - "curator": "camilla", - "reward": { - "amount": "80600453", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-09T23:45:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 43 - } - ], - [ - 21550, - { - "block": 4847456, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "fairytalelife", - "pending_payout": { - "amount": "836882", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "how-to-draw-a-braid", - "rshares": "215464179712", - "total_vote_weight": "17696363500200331189", - "voter": "camilla", - "weight": "1647825388296973" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T11:58:39", - "trx_id": "a38d027833317a53a6db3c56b0b463a8f5ed5b28", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21551, - { - "block": 4847456, - "op": { - "type": "vote_operation", - "value": { - "author": "fairytalelife", - "permlink": "how-to-draw-a-braid", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T11:58:39", - "trx_id": "a38d027833317a53a6db3c56b0b463a8f5ed5b28", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21552, - { - "block": 4847500, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "infovore", - "pending_payout": { - "amount": "518655", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend", - "rshares": "211239391874", - "total_vote_weight": "17497519072959523574", - "voter": "camilla", - "weight": "2586516889892580" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:00:51", - "trx_id": "9ef06b675e6e7c61d019a20f853cf378d8067c5b", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21553, - { - "block": 4847500, - "op": { - "type": "vote_operation", - "value": { - "author": "infovore", - "permlink": "a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:00:51", - "trx_id": "9ef06b675e6e7c61d019a20f853cf378d8067c5b", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21554, - { - "block": 4847541, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "great article infovore :D", - "json_metadata": "{\"tags\":[\"steemmag\"]}", - "parent_author": "infovore", - "parent_permlink": "a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend", - "permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:03:00", - "trx_id": "51274db60900301f3ae4eac5bcd92b43681d5a00", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21555, - { - "block": 4847547, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "80", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "pura-vida-my-experience-working-on-a-permaculture-farm-in-costa-rica-part-two", - "rshares": "211239391874", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:03:18", - "trx_id": "8c81f1f31bf385cc594e462630dc56a23be2c0d6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21556, - { - "block": 4847547, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "pura-vida-my-experience-working-on-a-permaculture-farm-in-costa-rica-part-two", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:03:18", - "trx_id": "8c81f1f31bf385cc594e462630dc56a23be2c0d6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21557, - { - "block": 4847565, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "infovore", - "pending_payout": { - "amount": "1107151", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "steemmag-steemit-s-weekend-digest-9-of-community-curation-and-reputation-chats-with-a-whale-steemit-chat-lead-moderators-a-day", - "rshares": "211239391874", - "total_vote_weight": "17791220144631813368", - "voter": "camilla", - "weight": "1232503267820026" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:04:12", - "trx_id": "e531290d33bc74838270939b7036db7e2872ecdd", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21558, - { - "block": 4847565, - "op": { - "type": "vote_operation", - "value": { - "author": "infovore", - "permlink": "steemmag-steemit-s-weekend-digest-9-of-community-curation-and-reputation-chats-with-a-whale-steemit-chat-lead-moderators-a-day", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:04:12", - "trx_id": "e531290d33bc74838270939b7036db7e2872ecdd", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21559, - { - "block": 4847590, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "opheliafu", - "pending_payout": { - "amount": "80", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "dream", - "rshares": "211239391874", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:05:27", - "trx_id": "f4a38bff870c28d178786a6a6206af15bd0b6499", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21560, - { - "block": 4847590, - "op": { - "type": "vote_operation", - "value": { - "author": "opheliafu", - "permlink": "dream", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:05:27", - "trx_id": "f4a38bff870c28d178786a6a6206af15bd0b6499", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21561, - { - "block": 4847798, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "lyubovbar", - "pending_payout": { - "amount": "84", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "gentle-casserole-of-minced-chicken-and-vegetables-recipe-and-cooking-lyubovbar", - "rshares": "207014604037", - "total_vote_weight": "960116344927767918", - "voter": "camilla", - "weight": "902153156129896529" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:15:51", - "trx_id": "4e6b205f98a3d09b62db0671b10f16a6fec9ae47", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21562, - { - "block": 4847798, - "op": { - "type": "vote_operation", - "value": { - "author": "lyubovbar", - "permlink": "gentle-casserole-of-minced-chicken-and-vegetables-recipe-and-cooking-lyubovbar", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:15:51", - "trx_id": "4e6b205f98a3d09b62db0671b10f16a6fec9ae47", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21563, - { - "block": 4847813, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "ekaterina4ka", - "pending_payout": { - "amount": "20158", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "knitting-needles-my-passion-post-14-vyazanie-spicami-moe-uvlechenie-post-14", - "rshares": "207014604037", - "total_vote_weight": "14117644497751956775", - "voter": "camilla", - "weight": "53225957273500042" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:16:36", - "trx_id": "4fc7301f6f82b3069f7f88d4a3f303ffc8532caf", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21564, - { - "block": 4847813, - "op": { - "type": "vote_operation", - "value": { - "author": "ekaterina4ka", - "permlink": "knitting-needles-my-passion-post-14-vyazanie-spicami-moe-uvlechenie-post-14", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T12:16:36", - "trx_id": "4fc7301f6f82b3069f7f88d4a3f303ffc8532caf", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21565, - { - "block": 4855539, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "187", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "rshares": "487083675640", - "total_vote_weight": "2002438233053738410", - "voter": "infovore", - "weight": "2002438233053738410" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T18:45:42", - "trx_id": "338e3d07db6a21a59e9fdffa84cd177a03a8ccd5", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21566, - { - "block": 4855539, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "voter": "infovore", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T18:45:42", - "trx_id": "338e3d07db6a21a59e9fdffa84cd177a03a8ccd5", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21567, - { - "block": 4855580, - "op": { - "type": "comment_operation", - "value": { - "author": "infovore", - "body": "Thanks @camilla . Great to see you drop by.. How are yo doing today?", - "json_metadata": "{\"tags\":[\"steemmag\"],\"users\":[\"camilla\"]}", - "parent_author": "camilla", - "parent_permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "permlink": "re-camilla-re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t184739329z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T18:47:45", - "trx_id": "0c869279e01f8f00ce45c2d334c872388fc47d05", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21568, - { - "block": 4861233, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "### I colored this drawing with color pencils and markers. It took me about 6 hours.\nhttps://i.imgsafe.org/496152e4bb.jpg\n\n### The drawing is from Johanna Basfords coloring book: Enchanted Forest.\n### I hope your autumn days are bright, peaceful and full of colors :)", - "json_metadata": "{\"tags\":[\"art\",\"coloring\",\"flowers\",\"\"],\"image\":[\"https://i.imgsafe.org/496152e4bb.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "colors-of-the-falling-leaves", - "title": "Colors of the Falling Leaves" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:03", - "trx_id": "ab378409dc2b9e81a6b30a78dae53ca69a4acfb2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21569, - { - "block": 4861233, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "79", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "215464179712", - "total_vote_weight": "942864750061894927", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:03", - "trx_id": "ab378409dc2b9e81a6b30a78dae53ca69a4acfb2", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21570, - { - "block": 4861233, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:03", - "trx_id": "ab378409dc2b9e81a6b30a78dae53ca69a4acfb2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21571, - { - "block": 4861237, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "83", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "9342417724", - "total_vote_weight": "981571504716947568", - "voter": "goldmatters", - "weight": "258045031033684" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:15", - "trx_id": "41f82960cbf4033b4bbafca616e441bc87240270", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21572, - { - "block": 4861237, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "goldmatters", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:15", - "trx_id": "41f82960cbf4033b4bbafca616e441bc87240270", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21573, - { - "block": 4861248, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -77,16 +77,44 @@\n 6 hours\n+ to color in all the details\n .%0Ahttps:\n", - "json_metadata": "{\"tags\":[\"art\",\"coloring\",\"flowers\",\"\"],\"image\":[\"https://i.imgsafe.org/496152e4bb.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "colors-of-the-falling-leaves", - "title": "Colors of the Falling Leaves" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:31:48", - "trx_id": "4ec95086082881f1933fc51deb44808586236c47", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21574, - { - "block": 4861257, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "374", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "651645205561", - "total_vote_weight": "3315460247733921376", - "voter": "clains", - "weight": "93355549720678952" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:32:15", - "trx_id": "a83693680d2a21066e9076b45dcb806a44f867ae", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21575, - { - "block": 4861257, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "clains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:32:15", - "trx_id": "a83693680d2a21066e9076b45dcb806a44f867ae", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21576, - { - "block": 4861263, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "441", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "130765248410", - "total_vote_weight": "3710619089052759354", - "voter": "psylains", - "weight": "19757942065941898" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:32:33", - "trx_id": "f1a17268864f8b28c1fe6a23c0bfafc639abcdce", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21577, - { - "block": 4861263, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "psylains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:32:33", - "trx_id": "f1a17268864f8b28c1fe6a23c0bfafc639abcdce", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21578, - { - "block": 4861274, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "442", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 167755251, - "total_vote_weight": "3711112772370702390", - "voter": "myxxo", - "weight": "33735026726107" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:06", - "trx_id": "985e950136848950e6228f7165dbeccec355abbc", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21579, - { - "block": 4861274, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "myxxo", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:06", - "trx_id": "985e950136848950e6228f7165dbeccec355abbc", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21580, - { - "block": 4861278, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "448", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "11146913938", - "total_vote_weight": "3743842826315368099", - "voter": "asim", - "weight": "2454754045849928" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:18", - "trx_id": "bc8fb50c578058a985b35a269670c3ab051b9e1e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21581, - { - "block": 4861278, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "asim", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:18", - "trx_id": "bc8fb50c578058a985b35a269670c3ab051b9e1e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21582, - { - "block": 4861282, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "448", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1413116466, - "total_vote_weight": "3747981698857805172", - "voter": "murh", - "weight": "338007924299027" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:30", - "trx_id": "08d0ed18adab2db76b9899b4d2f785ca61b05d7c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21583, - { - "block": 4861282, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "murh", - "weight": 3301 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:30", - "trx_id": "08d0ed18adab2db76b9899b4d2f785ca61b05d7c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21584, - { - "block": 4861283, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "660", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "376199691813", - "total_vote_weight": "4772726281932303484", - "voter": "anonymous", - "weight": "85395381922874859" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:33", - "trx_id": "8f59b3c9c0ef02e6680fa86f4b31d3973ac3e185", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21585, - { - "block": 4861283, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "anonymous", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:33:33", - "trx_id": "8f59b3c9c0ef02e6680fa86f4b31d3973ac3e185", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21586, - { - "block": 4861305, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "660", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 446928899, - "total_vote_weight": "4773858721503540961", - "voter": "runridefly", - "weight": "137780147833893" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:34:42", - "trx_id": "064a66dbb472995ece4bdbfac413b29fd002f80e", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21587, - { - "block": 4861305, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "runridefly", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:34:42", - "trx_id": "064a66dbb472995ece4bdbfac413b29fd002f80e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21588, - { - "block": 4861306, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "664", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5888362824", - "total_vote_weight": "4788761305590533862", - "voter": "bitspace", - "weight": "1837985370729124" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:34:45", - "trx_id": "f595e39e07494f9463464fe7604fc77941579211", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21589, - { - "block": 4861306, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "bitspace", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:34:45", - "trx_id": "f595e39e07494f9463464fe7604fc77941579211", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21590, - { - "block": 4861315, - "op": { - "type": "comment_operation", - "value": { - "author": "runridefly", - "body": "Pretty", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t233510136z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:35:12", - "trx_id": "6cffe592a70d339479a69eb7c992b6324933c046", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21591, - { - "block": 4861358, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "664", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 286701566, - "total_vote_weight": "4789486075952524692", - "voter": "riosparada", - "weight": "152201776018074" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:37:21", - "trx_id": "8a3023a21bc2d2ba82b568fc5633d1e6b611221f", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21592, - { - "block": 4861358, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "riosparada", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:37:21", - "trx_id": "8a3023a21bc2d2ba82b568fc5633d1e6b611221f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21593, - { - "block": 4861421, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "711", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "76309016178", - "total_vote_weight": "4979695521725803466", - "voter": "thecryptofiend", - "weight": "59915975418582813" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:40:30", - "trx_id": "948066bebb5c927206c9ec64adbb2af02c0c9858", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21594, - { - "block": 4861421, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "thecryptofiend", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:40:30", - "trx_id": "948066bebb5c927206c9ec64adbb2af02c0c9858", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21595, - { - "block": 4861425, - "op": { - "type": "comment_operation", - "value": { - "author": "thecryptofiend", - "body": "Beautifully done.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234043251z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:40:42", - "trx_id": "52622f3a5be5a814ccfe8f64424e379b39f3e9a5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21596, - { - "block": 4861441, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "runridefly", - "pending_payout": { - "amount": "78", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t233510136z", - "rshares": "211239391874", - "total_vote_weight": "925304557063353830", - "voter": "camilla", - "weight": "194313956983304304" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:41:30", - "trx_id": "bb2f867251b1c4ac9b6f1d3652ebb778407346cf", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21597, - { - "block": 4861441, - "op": { - "type": "vote_operation", - "value": { - "author": "runridefly", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t233510136z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:41:30", - "trx_id": "bb2f867251b1c4ac9b6f1d3652ebb778407346cf", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21598, - { - "block": 4861442, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "711", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 758446790, - "total_vote_weight": "4981559454058925084", - "voter": "dajohns1420", - "weight": "652376316592566" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:41:33", - "trx_id": "c9d21ad41671069dcfdc379bc425bf9da9e4f2eb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21599, - { - "block": 4861442, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "dajohns1420", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:41:33", - "trx_id": "c9d21ad41671069dcfdc379bc425bf9da9e4f2eb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21600, - { - "block": 4861447, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thank you :)", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "thecryptofiend", - "parent_permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234043251z", - "permlink": "re-thecryptofiend-re-camilla-colors-of-the-falling-leaves-20160910t234147377z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:41:48", - "trx_id": "05dc0ba561cd32f233ebd9218c90a08afab50ef8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21601, - { - "block": 4861455, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thanks!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "runridefly", - "parent_permlink": "re-camilla-colors-of-the-falling-leaves-20160910t233510136z", - "permlink": "re-runridefly-re-camilla-colors-of-the-falling-leaves-20160910t234213368z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:42:12", - "trx_id": "e595a76431f6d0fd95ccddb26e0ee8d621f9f6a8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21602, - { - "block": 4861507, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "714", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1609908331, - "total_vote_weight": "4985514199071180117", - "voter": "bullionstackers", - "weight": "1819182705637315" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:44:51", - "trx_id": "107888d01ad020877e204098042e8d3b5a4516f2", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21603, - { - "block": 4861507, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "bullionstackers", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:44:51", - "trx_id": "107888d01ad020877e204098042e8d3b5a4516f2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21604, - { - "block": 4861523, - "op": { - "type": "comment_operation", - "value": { - "author": "bullionstackers", - "body": "Amazing \ud83d\udc4d\u2705\ud83c\udd99\nSweet \u2764\ufe0f", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234539398z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:45:39", - "trx_id": "20e6f5c072523bcfdbffc043c0c614ad6807fda6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21605, - { - "block": 4861594, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2334", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "2043524972373", - "total_vote_weight": "8641125258482638496", - "voter": "badassmother", - "weight": "2211644690943932319" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:49:12", - "trx_id": "d67777f06253fd76026e917b8422c3b3280f3a20", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21606, - { - "block": 4861594, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "badassmother", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:49:12", - "trx_id": "d67777f06253fd76026e917b8422c3b3280f3a20", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21607, - { - "block": 4861611, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2811", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "469477368872", - "total_vote_weight": "9216964533196661783", - "voter": "boatymcboatface", - "weight": "364698207318881415" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:03", - "trx_id": "26a4f80f777e0598f81888acacc5b86f09c9d8e3", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21608, - { - "block": 4861611, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "boatymcboatface", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:03", - "trx_id": "26a4f80f777e0598f81888acacc5b86f09c9d8e3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21609, - { - "block": 4861611, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2815", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 3142023228, - "total_vote_weight": "9220590649066884760", - "voter": "glitterpig", - "weight": "2296540051141218" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:03", - "trx_id": "339bf9c191ca9b854148930b45e2b1ec1a8162fd", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21610, - { - "block": 4861611, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "glitterpig", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:03", - "trx_id": "339bf9c191ca9b854148930b45e2b1ec1a8162fd", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21611, - { - "block": 4861613, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "bullionstackers", - "pending_payout": { - "amount": "78", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234539398z", - "rshares": "211239391874", - "total_vote_weight": "925304557063353830", - "voter": "camilla", - "weight": "138795683559503074" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "2a804b16897696efd4d1f9db5c997b67674b9f23", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21612, - { - "block": 4861613, - "op": { - "type": "vote_operation", - "value": { - "author": "bullionstackers", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234539398z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "2a804b16897696efd4d1f9db5c997b67674b9f23", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21613, - { - "block": 4861613, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4066", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "1086156024356", - "total_vote_weight": "10323774733997980525", - "voter": "rossco99", - "weight": "702360534072797637" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "7e75d48d6f3d4b319e06bfadadf660a13ad2b416", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21614, - { - "block": 4861613, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "rossco99", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "7e75d48d6f3d4b319e06bfadadf660a13ad2b416", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21615, - { - "block": 4861613, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4141", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "59824941705", - "total_vote_weight": "10376922050468705210", - "voter": "theshell", - "weight": "33837124819694716" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "842ae95f2699f21bb822b91a5f4259a34b96733c", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21616, - { - "block": 4861613, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "theshell", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:09", - "trx_id": "842ae95f2699f21bb822b91a5f4259a34b96733c", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21617, - { - "block": 4861615, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4141", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 442913138, - "total_vote_weight": "10377312932517177095", - "voter": "karen13", - "weight": "250164511022006" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:15", - "trx_id": "a3c6840b6e79a68858f5245e0aac9687324e5600", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21618, - { - "block": 4861615, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "karen13", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:15", - "trx_id": "a3c6840b6e79a68858f5245e0aac9687324e5600", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21619, - { - "block": 4861616, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4143", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 918895431, - "total_vote_weight": "10378123760218338031", - "voter": "taker", - "weight": "520281108244933" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:18", - "trx_id": "5fa8ce6e3fe39a29c75f7b18a4a95011289cc6a6", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21620, - { - "block": 4861616, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "taker", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:18", - "trx_id": "5fa8ce6e3fe39a29c75f7b18a4a95011289cc6a6", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21621, - { - "block": 4861620, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4144", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1027351719, - "total_vote_weight": "10379030096175788546", - "voter": "coar", - "weight": "587607812413750" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:30", - "trx_id": "7ab0666e464154190d614f1fd33895117ffaabdb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21622, - { - "block": 4861620, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "coar", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:50:30", - "trx_id": "7ab0666e464154190d614f1fd33895117ffaabdb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21623, - { - "block": 4861749, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4154", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 4188095885, - "total_vote_weight": "10382722753969179236", - "voter": "fubar-bdhr", - "weight": "3187994561627295" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:56:57", - "trx_id": "46ee895d4b883b7542d529eae6dd88e93af6ad36", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21624, - { - "block": 4861749, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "fubar-bdhr", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:56:57", - "trx_id": "46ee895d4b883b7542d529eae6dd88e93af6ad36", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21625, - { - "block": 4861771, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4166", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "7528587638", - "total_vote_weight": "10389352241341337948", - "voter": "furion", - "weight": "5966538634942840" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:03", - "trx_id": "01b7797a422b611a8cebf22c9d96a0076e573c6f", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21626, - { - "block": 4861771, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "furion", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:03", - "trx_id": "01b7797a422b611a8cebf22c9d96a0076e573c6f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21627, - { - "block": 4861772, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4168", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1643275913, - "total_vote_weight": "10390797820305061860", - "voter": "positive", - "weight": "1303430365624393" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "25ccd12e68ae60683792cb86ad162a2551461b10", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21628, - { - "block": 4861772, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "positive", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "25ccd12e68ae60683792cb86ad162a2551461b10", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21629, - { - "block": 4861772, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4290", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "95270090466", - "total_vote_weight": "10473728653351718274", - "voter": "laonie", - "weight": "74775967797068533" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "0850310bdd0e6ab53cf98faed8dc54977ef8c1aa", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21630, - { - "block": 4861772, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "laonie", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "0850310bdd0e6ab53cf98faed8dc54977ef8c1aa", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21631, - { - "block": 4861772, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4303", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "10675325035", - "total_vote_weight": "10482915065453226980", - "voter": "xiaohui", - "weight": "8283081578193683" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "300419a4ecd8dff31f77b53cfd71aa2b24cbaafd", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21632, - { - "block": 4861772, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "xiaohui", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "300419a4ecd8dff31f77b53cfd71aa2b24cbaafd", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21633, - { - "block": 4861772, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4306", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 2027172658, - "total_vote_weight": "10484657112458893762", - "voter": "fkn", - "weight": "1570745716776215" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "72f1bad886b8d35a33a8bf714de59715122beffe", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21634, - { - "block": 4861772, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "fkn", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "72f1bad886b8d35a33a8bf714de59715122beffe", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21635, - { - "block": 4861772, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4309", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 2666300169, - "total_vote_weight": "10486947232389218712", - "voter": "elishagh1", - "weight": "2064924803842996" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "32b1930e1a54ef6eccc101f2cb63b9dfae662b0b", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 21636, - { - "block": 4861772, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "elishagh1", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:06", - "trx_id": "32b1930e1a54ef6eccc101f2cb63b9dfae662b0b", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 21637, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4334", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "19377752514", - "total_vote_weight": "10503551539749126072", - "voter": "somebody", - "weight": "14999224315116315" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "7919d9f1d4f6885deeaff8cb391483d35505a741", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21638, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "somebody", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "7919d9f1d4f6885deeaff8cb391483d35505a741", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21639, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4335", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 446441859, - "total_vote_weight": "10503933268175068386", - "voter": "xiaokongcom", - "weight": "344828011434556" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "98f0216f62a78f00dbeb255c535d87fa2d1131fa", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21640, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "xiaokongcom", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "98f0216f62a78f00dbeb255c535d87fa2d1131fa", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21641, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4336", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 899777990, - "total_vote_weight": "10504702508469112725", - "voter": "xianjun", - "weight": "694880398953386" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "fe5755839b5588c54275e838460e5aedcf773cd8", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21642, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "xianjun", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "fe5755839b5588c54275e838460e5aedcf773cd8", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21643, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4364", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "21517750872", - "total_vote_weight": "10523054228899113010", - "voter": "kimziv", - "weight": "16577720788433590" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "d54ed386d18de451d18c462b61130bdab74e87b0", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21644, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "kimziv", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "d54ed386d18de451d18c462b61130bdab74e87b0", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21645, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4369", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 3947218827, - "total_vote_weight": "10526411468808214056", - "voter": "myfirst", - "weight": "3032706717887944" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "a8a43ecc52033121fb72a6a017010c561c52f20f", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 21646, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "myfirst", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "a8a43ecc52033121fb72a6a017010c561c52f20f", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 21647, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4369", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 59017260, - "total_vote_weight": "10526461643350211360", - "voter": "microluck", - "weight": "45324336270897" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "3f5698b07a3f1406b5828c4c64f9a983fea3a899", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 21648, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "microluck", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "3f5698b07a3f1406b5828c4c64f9a983fea3a899", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 21649, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4370", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 966298641, - "total_vote_weight": "10527283068410058847", - "voter": "flysaga", - "weight": "742020637395563" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "ca07f6a029a45f50c7cf69cc0549ee26d63ab08f", - "trx_in_block": 8, - "virtual_op": 1 - } - ], - [ - 21650, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "flysaga", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "ca07f6a029a45f50c7cf69cc0549ee26d63ab08f", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 21651, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4377", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5708182705", - "total_vote_weight": "10532131970458603469", - "voter": "midnightoil", - "weight": "4380174850518641" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "bbfe11f85d10cc32806fc169b2a44cd1ce96c381", - "trx_in_block": 9, - "virtual_op": 1 - } - ], - [ - 21652, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "midnightoil", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "bbfe11f85d10cc32806fc169b2a44cd1ce96c381", - "trx_in_block": 9, - "virtual_op": 0 - } - ], - [ - 21653, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4381", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 2685190882, - "total_vote_weight": "10534410893458449984", - "voter": "sisterholics", - "weight": "2058627109861351" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "41c97d8d492ddb239b65e28bf5c0658cfd4a38f2", - "trx_in_block": 11, - "virtual_op": 1 - } - ], - [ - 21654, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "sisterholics", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "41c97d8d492ddb239b65e28bf5c0658cfd4a38f2", - "trx_in_block": 11, - "virtual_op": 0 - } - ], - [ - 21655, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4381", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 52858601, - "total_vote_weight": "10534455741411155393", - "voter": "ch0c0latechip", - "weight": "40512650610552" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "cb76c0e3e43723b3b3b903391f70f14592e0fd3d", - "trx_in_block": 13, - "virtual_op": 1 - } - ], - [ - 21656, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "ch0c0latechip", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "cb76c0e3e43723b3b3b903391f70f14592e0fd3d", - "trx_in_block": 13, - "virtual_op": 0 - } - ], - [ - 21657, - { - "block": 4861773, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4381", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 54905658, - "total_vote_weight": "10534502325653869728", - "voter": "fnait", - "weight": "42081099251949" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "eaac0bcfebfb11d446729cf7cd8b0d585f87044d", - "trx_in_block": 16, - "virtual_op": 1 - } - ], - [ - 21658, - { - "block": 4861773, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "fnait", - "weight": 900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:09", - "trx_id": "eaac0bcfebfb11d446729cf7cd8b0d585f87044d", - "trx_in_block": 16, - "virtual_op": 0 - } - ], - [ - 21659, - { - "block": 4861785, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4396", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "10966759140", - "total_vote_weight": "10543795996096921811", - "voter": "acidyo", - "weight": "8581155709084756" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:45", - "trx_id": "f0d46b2c2ce35031d8557b025817f342f0f339de", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21660, - { - "block": 4861785, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "acidyo", - "weight": 4200 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:58:45", - "trx_id": "f0d46b2c2ce35031d8557b025817f342f0f339de", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21661, - { - "block": 4861792, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4425", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "21529337232", - "total_vote_weight": "10561977462931279207", - "voter": "jl777", - "weight": "16999671490124165" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:06", - "trx_id": "2ea8fd4f67fe9ebb398724c437bfece264b6c2ca", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21662, - { - "block": 4861792, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "jl777", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:06", - "trx_id": "2ea8fd4f67fe9ebb398724c437bfece264b6c2ca", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21663, - { - "block": 4861793, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4427", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1816956761, - "total_vote_weight": "10563508050743123362", - "voter": "proto", - "weight": "1433650583760691" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:09", - "trx_id": "39f8ed7c216ddf201ccf9c42bac4a8d4ff9f354c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21664, - { - "block": 4861793, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "proto", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:09", - "trx_id": "39f8ed7c216ddf201ccf9c42bac4a8d4ff9f354c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21665, - { - "block": 4861795, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4431", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 2586674942, - "total_vote_weight": "10565686017257802111", - "voter": "yefet", - "weight": "2047288523798024" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:15", - "trx_id": "a1b7ae5e0b8dcbed8002f761969b6e22f8a9a31d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21666, - { - "block": 4861795, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "yefet", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:15", - "trx_id": "a1b7ae5e0b8dcbed8002f761969b6e22f8a9a31d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21667, - { - "block": 4861797, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4432", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 992068506, - "total_vote_weight": "10566521014395361912", - "voter": "smisi", - "weight": "787680633098078" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:21", - "trx_id": "6ff284737fe558f2aa540ab1aeaffe85bf02a185", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21668, - { - "block": 4861797, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "smisi", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:21", - "trx_id": "6ff284737fe558f2aa540ab1aeaffe85bf02a185", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21669, - { - "block": 4861804, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4434", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 740454861, - "total_vote_weight": "10567144119852226283", - "voter": "steem1653", - "weight": "595065711305474" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:42", - "trx_id": "8b038f96aac44f05d59cd26778b2a69904bdee5d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21670, - { - "block": 4861804, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "steem1653", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-10T23:59:42", - "trx_id": "8b038f96aac44f05d59cd26778b2a69904bdee5d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21671, - { - "block": 4861870, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4448", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "7395923269", - "total_vote_weight": "10573362512636721672", - "voter": "dasha", - "weight": "6218392784495389" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:03:00", - "trx_id": "122ed469619cfd4247bb0c845ef9e5baa5fb50a0", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21672, - { - "block": 4861870, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "dasha", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:03:00", - "trx_id": "122ed469619cfd4247bb0c845ef9e5baa5fb50a0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21673, - { - "block": 4862066, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4426", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1457681508, - "total_vote_weight": "10574586953973812133", - "voter": "benthegameboy", - "weight": "1224441337090461" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:12:48", - "trx_id": "dab654729e339ce2935e85d17053eb787d2f3fc0", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21674, - { - "block": 4862066, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "benthegameboy", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:12:48", - "trx_id": "dab654729e339ce2935e85d17053eb787d2f3fc0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21675, - { - "block": 4862096, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4431", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 3905082034, - "total_vote_weight": "10577865317090407148", - "voter": "sompitonov", - "weight": "3278363116595015" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:14:18", - "trx_id": "3f5e92db2e9c54505807d9a5984fff37c36fb122", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21676, - { - "block": 4862096, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "sompitonov", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:14:18", - "trx_id": "3f5e92db2e9c54505807d9a5984fff37c36fb122", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21677, - { - "block": 4862180, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "For a long time I have not seen you here.\nBeautiful as always Camilla!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t001828852z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:18:30", - "trx_id": "16e3f759b22037a3d774a64ab4e7a908d34e1a9b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21678, - { - "block": 4862440, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4461", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "9150087358", - "total_vote_weight": "10585536240712091932", - "voter": "rpf", - "weight": "7670923621684784" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:31:30", - "trx_id": "6abec28fe0b14f187825264716d0d76889e5aa8a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21679, - { - "block": 4862440, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "rpf", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T00:31:30", - "trx_id": "6abec28fe0b14f187825264716d0d76889e5aa8a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21680, - { - "block": 4863012, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4464", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 158821861, - "total_vote_weight": "10585669256066109250", - "voter": "tycho", - "weight": "133015354017318" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:00:12", - "trx_id": "0c5bfc37c9a1cc5c5ea8eb31a9d07cf2405da6f0", - "trx_in_block": 9, - "virtual_op": 1 - } - ], - [ - 21681, - { - "block": 4863012, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "tycho", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:00:12", - "trx_id": "0c5bfc37c9a1cc5c5ea8eb31a9d07cf2405da6f0", - "trx_in_block": 9, - "virtual_op": 0 - } - ], - [ - 21682, - { - "block": 4863030, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5376", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "667121759026", - "total_vote_weight": "11107308194521940665", - "voter": "silver", - "weight": "521638938455831415" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:06", - "trx_id": "7df61195407d5b1fa445ad2da0c684125a1846d1", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21683, - { - "block": 4863030, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "silver", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:06", - "trx_id": "7df61195407d5b1fa445ad2da0c684125a1846d1", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21684, - { - "block": 4863031, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "7922", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "1625458470769", - "total_vote_weight": "12128799463619749613", - "voter": "silversteem", - "weight": "1021491269097808948" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:09", - "trx_id": "a60835407a87606515626dc5552df07b4126ae05", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21685, - { - "block": 4863031, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "silversteem", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:09", - "trx_id": "a60835407a87606515626dc5552df07b4126ae05", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21686, - { - "block": 4863031, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "11322", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "1817776274078", - "total_vote_weight": "12979717477225621428", - "voter": "nextgencrypto", - "weight": "850918013605871815" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:09", - "trx_id": "6323e92940e604a8595e1c60f0048f1e946c36e9", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21687, - { - "block": 4863031, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "nextgencrypto", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:09", - "trx_id": "6323e92940e604a8595e1c60f0048f1e946c36e9", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21688, - { - "block": 4863032, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "42415", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "10514445664165", - "total_vote_weight": "15373717560397404335", - "voter": "berniesanders", - "weight": "2394000083171782907" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:12", - "trx_id": "ddb891664d6d0939fa74ac5b4c263671eed427ef", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21689, - { - "block": 4863032, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "berniesanders", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:12", - "trx_id": "ddb891664d6d0939fa74ac5b4c263671eed427ef", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21690, - { - "block": 4863033, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "43308", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "228778017848", - "total_vote_weight": "15402720955096056998", - "voter": "justin", - "weight": "29003394698652663" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:15", - "trx_id": "fd6b85e506840c49dc2c53cf3b8ad94cd060f160", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21691, - { - "block": 4863033, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "justin", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:15", - "trx_id": "fd6b85e506840c49dc2c53cf3b8ad94cd060f160", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21692, - { - "block": 4863034, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "43837", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "134451929559", - "total_vote_weight": "15419512125538623936", - "voter": "steemservices", - "weight": "16791170442566938" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:18", - "trx_id": "50b3cba71af5e773c5fcfc0439c4cfe435d89013", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21693, - { - "block": 4863034, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "steemservices", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:18", - "trx_id": "50b3cba71af5e773c5fcfc0439c4cfe435d89013", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21694, - { - "block": 4863035, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44092", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "64047892423", - "total_vote_weight": "15427445845003439585", - "voter": "nextgenwitness", - "weight": "7933719464815649" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:21", - "trx_id": "7c71e92dcca5b545a697a7ca8bd029c65ad67938", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21695, - { - "block": 4863035, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "nextgenwitness", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:01:21", - "trx_id": "7c71e92dcca5b545a697a7ca8bd029c65ad67938", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21696, - { - "block": 4863275, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "43902", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5890677916", - "total_vote_weight": "15428173445384453319", - "voter": "the.bot", - "weight": "727600381013734" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:13:21", - "trx_id": "0bf93bd4edc4db6ba18376ed89798b1719bb21ca", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21697, - { - "block": 4863275, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "the.bot", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:13:21", - "trx_id": "0bf93bd4edc4db6ba18376ed89798b1719bb21ca", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21698, - { - "block": 4863304, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "43970", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "10971913263", - "total_vote_weight": "15429527731559523836", - "voter": "thebotkiller", - "weight": "1354286175070517" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:48", - "trx_id": "a9e26027ff1bdf1d39f5e77341145f3bd77e56f1", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21699, - { - "block": 4863304, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "thebotkiller", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:48", - "trx_id": "a9e26027ff1bdf1d39f5e77341145f3bd77e56f1", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21700, - { - "block": 4863305, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44002", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "8035250377", - "total_vote_weight": "15430518768699936261", - "voter": "johnbradshaw", - "weight": "991037140412425" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:51", - "trx_id": "29720c0a0997ee60889bfe8635bc28fd49e77387", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21701, - { - "block": 4863305, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "johnbradshaw", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:51", - "trx_id": "29720c0a0997ee60889bfe8635bc28fd49e77387", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21702, - { - "block": 4863306, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44029", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "6605970020", - "total_vote_weight": "15431333036355277936", - "voter": "iloveporn", - "weight": "814267655341675" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:54", - "trx_id": "36e35e536a91b2a5188ff45b08a83a398875140f", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21703, - { - "block": 4863306, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "iloveporn", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:14:54", - "trx_id": "36e35e536a91b2a5188ff45b08a83a398875140f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21704, - { - "block": 4863338, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44090", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "6024180188", - "total_vote_weight": "15432075208030422416", - "voter": "kissmybutt", - "weight": "742171675144480" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:16:30", - "trx_id": "5f05367b69c5bc9bc3db5dcb3e4582e142e88233", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21705, - { - "block": 4863338, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "kissmybutt", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:16:30", - "trx_id": "5f05367b69c5bc9bc3db5dcb3e4582e142e88233", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21706, - { - "block": 4863374, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44140", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5718018880", - "total_vote_weight": "15432779323126653888", - "voter": "the.whale", - "weight": "704115096231472" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:18:18", - "trx_id": "8a63eb11e2e1c6c1d2b7728a3f9efd17c1a1d240", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21707, - { - "block": 4863374, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "the.whale", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:18:18", - "trx_id": "8a63eb11e2e1c6c1d2b7728a3f9efd17c1a1d240", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21708, - { - "block": 4863456, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44255", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5716399817", - "total_vote_weight": "15433482910157930839", - "voter": "vote", - "weight": "703587031276951" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:22:24", - "trx_id": "1664229025160a05681bd822aa26bfa4a27b58e4", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21709, - { - "block": 4863456, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "vote", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:22:24", - "trx_id": "1664229025160a05681bd822aa26bfa4a27b58e4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21710, - { - "block": 4863492, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44292", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "5415715980", - "total_vote_weight": "15434149185399671893", - "voter": "unicornfarts", - "weight": "666275241741054" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:24:12", - "trx_id": "3bfda7884bca9ead7019a1734d3e744149b9dc7a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21711, - { - "block": 4863492, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "unicornfarts", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:24:12", - "trx_id": "3bfda7884bca9ead7019a1734d3e744149b9dc7a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21712, - { - "block": 4863566, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44374", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "6684459064", - "total_vote_weight": "15434971143187442470", - "voter": "fuck.off", - "weight": "821957787770577" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:27:54", - "trx_id": "0fd0fa69b5db97b47a9900da886d8c4746c28907", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21713, - { - "block": 4863566, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "fuck.off", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:27:54", - "trx_id": "0fd0fa69b5db97b47a9900da886d8c4746c28907", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21714, - { - "block": 4863594, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44562", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "92325410560", - "total_vote_weight": "15446278274614161998", - "voter": "thecyclist", - "weight": "11307131426719528" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:29:18", - "trx_id": "70265f5e4c1b9df306c65e0e325b154e3c01e22c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21715, - { - "block": 4863594, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "thecyclist", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:29:18", - "trx_id": "70265f5e4c1b9df306c65e0e325b154e3c01e22c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21716, - { - "block": 4863681, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44624", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "6459214622", - "total_vote_weight": "15447066160476335869", - "voter": "bentley", - "weight": "787885862173871" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:33:42", - "trx_id": "115ca2ea95998f73771533eedb501f7651fa8f44", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21717, - { - "block": 4863681, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "bentley", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:33:42", - "trx_id": "115ca2ea95998f73771533eedb501f7651fa8f44", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21718, - { - "block": 4863693, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44637", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 284822451, - "total_vote_weight": "15447100893190931307", - "voter": "jimmytwoshoes", - "weight": "34732714595438" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:34:18", - "trx_id": "9321bbf13dde49da4bc3182781bebff196549ca5", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21719, - { - "block": 4863693, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "jimmytwoshoes", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:34:18", - "trx_id": "9321bbf13dde49da4bc3182781bebff196549ca5", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21720, - { - "block": 4863949, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44818", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "13689306732", - "total_vote_weight": "15448769289856751954", - "voter": "thecurator", - "weight": "1668396665820647" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:47:06", - "trx_id": "334d8d5713e130964f5c8779cb93a09fbe5851f5", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21721, - { - "block": 4863949, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "thecurator", - "weight": 4000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T01:47:06", - "trx_id": "334d8d5713e130964f5c8779cb93a09fbe5851f5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21722, - { - "block": 4864302, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "44463", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 154551739, - "total_vote_weight": "15448788115395185897", - "voter": "reddust", - "weight": "18825538433943" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T02:04:48", - "trx_id": "690e9fe84997d74ffe42d51eb0a53237954ae4ca", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21723, - { - "block": 4864302, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "reddust", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T02:04:48", - "trx_id": "690e9fe84997d74ffe42d51eb0a53237954ae4ca", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21724, - { - "block": 4866343, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "45862", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "27080912220", - "total_vote_weight": "15452083123473503571", - "voter": "tmendieta", - "weight": "3295008078317674" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T03:47:21", - "trx_id": "9f0e3175ce315d68d3dfb09db5a50d7c2e6501f2", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21725, - { - "block": 4866343, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "tmendieta", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T03:47:21", - "trx_id": "9f0e3175ce315d68d3dfb09db5a50d7c2e6501f2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21726, - { - "block": 4866361, - "op": { - "type": "comment_operation", - "value": { - "author": "tmendieta", - "body": "Beautiful! It must have been relaxing to color that, too.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t034905376z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T03:48:15", - "trx_id": "e735986566a7897dc0b1597a27f67b4edd821991", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21727, - { - "block": 4867542, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-runridefly-re-camilla-colors-of-the-falling-leaves-20160910t234213368z", - "rshares": 595937241, - "total_vote_weight": "2747866053251289", - "voter": "runridefly", - "weight": "2747866053251289" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T04:47:33", - "trx_id": "10d42dc7de5e86fc9ea71b9d87faa9d8eb1a149c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21728, - { - "block": 4867542, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-runridefly-re-camilla-colors-of-the-falling-leaves-20160910t234213368z", - "voter": "runridefly", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T04:47:33", - "trx_id": "10d42dc7de5e86fc9ea71b9d87faa9d8eb1a149c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21729, - { - "block": 4867952, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "47072", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 1640965017, - "total_vote_weight": "15452282551414441746", - "voter": "gidlark", - "weight": "199427940938175" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T05:08:06", - "trx_id": "5276e6b8889704c01ba028c0afafeef145d05479", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21730, - { - "block": 4867952, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "gidlark", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T05:08:06", - "trx_id": "5276e6b8889704c01ba028c0afafeef145d05479", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21731, - { - "block": 4868633, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "fairytalelife", - "comment_permlink": "how-to-draw-a-braid", - "curator": "camilla", - "reward": { - "amount": "92369054", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T05:42:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 71 - } - ], - [ - 21732, - { - "block": 4869126, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "47890", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 3600677639, - "total_vote_weight": "15452720051905923183", - "voter": "alexft", - "weight": "437500491481437" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:07:09", - "trx_id": "d02a7d2f24f2d8234bd20ecc0062773dd489dc3c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21733, - { - "block": 4869126, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "alexft", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:07:09", - "trx_id": "d02a7d2f24f2d8234bd20ecc0062773dd489dc3c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21734, - { - "block": 4869148, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "47950", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "7760931567", - "total_vote_weight": "15453662609632857945", - "voter": "sykochica", - "weight": "942557726934762" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:08:18", - "trx_id": "f48cab8e18dcb69fd7a687b23f2f66f6fcdfddc0", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21735, - { - "block": 4869148, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "sykochica", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:08:18", - "trx_id": "f48cab8e18dcb69fd7a687b23f2f66f6fcdfddc0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21736, - { - "block": 4869556, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "48399", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "15163479832", - "total_vote_weight": "15455502488331945333", - "voter": "randyclemens", - "weight": "1839878699087388" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:28:45", - "trx_id": "f4a758d30d1cc9817cbdca59fce5873f7f8ee757", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21737, - { - "block": 4869556, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "randyclemens", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:28:45", - "trx_id": "f4a758d30d1cc9817cbdca59fce5873f7f8ee757", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21738, - { - "block": 4870013, - "op": { - "type": "comment_operation", - "value": { - "author": "alexft", - "body": "I like it ! Great job !!!\nhttps://www.steemimg.com/images/2016/09/11/KALIBRI67d19.gif", - "json_metadata": "{\"tags\":[\"art\"],\"image\":[\"https://www.steemimg.com/images/2016/09/11/KALIBRI67d19.gif\"]}", - "parent_author": "camilla", - "parent_permlink": "colors-of-the-falling-leaves", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t065104109z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T06:51:39", - "trx_id": "e44c8b3f6ad20b94b87eb8d12263da3298a69a78", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21739, - { - "block": 4871461, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "46385", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "8925161251", - "total_vote_weight": "15456584376150636889", - "voter": "kyriacos", - "weight": "1081887818691556" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T08:04:21", - "trx_id": "ff32f21ca0f3403919f9513cae85fb581441eb4f", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21740, - { - "block": 4871461, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "kyriacos", - "weight": 2900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T08:04:21", - "trx_id": "ff32f21ca0f3403919f9513cae85fb581441eb4f", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21741, - { - "block": 4871660, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "infovore", - "comment_permlink": "a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend", - "curator": "camilla", - "reward": { - "amount": "95393246", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T08:14:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 68 - } - ], - [ - 21742, - { - "block": 4871660, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "196940896", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "73", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "vesting_payout": { - "amount": "298488546", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T08:14:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 203 - } - ], - [ - 21743, - { - "block": 4871660, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 193, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "48", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "196", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t120251791z", - "total_payout_value": { - "amount": "147", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T08:14:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 204 - } - ], - [ - 21744, - { - "block": 4872663, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "wow! alexft your photoshop skills are amazing :D thank you for making my art come alive!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "alexft", - "parent_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t065104109z", - "permlink": "re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t090442054z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:04:42", - "trx_id": "c5f1bbe41acbf760293579a47248681137035786", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21745, - { - "block": 4872665, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "alexft", - "pending_payout": { - "amount": "95", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t065104109z", - "rshares": "215466659591", - "total_vote_weight": "1089265210197592604", - "voter": "camilla", - "weight": "926752391840549520" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:04:48", - "trx_id": "de837658831f4966fd554d33b973b140e2398eea", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21746, - { - "block": 4872665, - "op": { - "type": "vote_operation", - "value": { - "author": "alexft", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t065104109z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:04:48", - "trx_id": "de837658831f4966fd554d33b973b140e2398eea", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21747, - { - "block": 4872675, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thank you! it was very meditative :D", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "tmendieta", - "parent_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t034905376z", - "permlink": "re-tmendieta-re-camilla-colors-of-the-falling-leaves-20160911t090516241z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:05:18", - "trx_id": "dbc973baece2f36d373ff1e1cf708567aef31690", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21748, - { - "block": 4872677, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "sompitonov", - "pending_payout": { - "amount": "79", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t001828852z", - "rshares": "211241823129", - "total_vote_weight": "925314672627650822", - "voter": "camilla", - "weight": "925314672627650822" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:05:24", - "trx_id": "3d0d8373f759d8604f7373d48cfb4c5632a5e6d7", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21749, - { - "block": 4872677, - "op": { - "type": "vote_operation", - "value": { - "author": "sompitonov", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t001828852z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:05:24", - "trx_id": "3d0d8373f759d8604f7373d48cfb4c5632a5e6d7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21750, - { - "block": 4872683, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "I have been sick but Im back now :D", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "sompitonov", - "parent_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t001828852z", - "permlink": "re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t090541444z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:05:42", - "trx_id": "b789ecfe867331a2a49406fc1d1a91c44f23f60e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21751, - { - "block": 4872697, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "tmendieta", - "pending_payout": { - "amount": "79", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t034905376z", - "rshares": "211241823129", - "total_vote_weight": "925314672627650822", - "voter": "camilla", - "weight": "925314672627650822" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:06:24", - "trx_id": "a2049922a326fead433a4a76649c5fc8454ff9bc", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21752, - { - "block": 4872697, - "op": { - "type": "vote_operation", - "value": { - "author": "tmendieta", - "permlink": "re-camilla-colors-of-the-falling-leaves-20160911t034905376z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:06:24", - "trx_id": "a2049922a326fead433a4a76649c5fc8454ff9bc", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21753, - { - "block": 4873207, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "With recovery! :D", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t090541444z", - "permlink": "re-camilla-re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t093158180z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:31:57", - "trx_id": "687f00253c6a38c18221a64072ff2ca9d5407ad3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21754, - { - "block": 4873216, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "infovore", - "comment_permlink": "steemmag-steemit-s-weekend-digest-9-of-community-curation-and-reputation-chats-with-a-whale-steemit-chat-lead-moderators-a-day", - "curator": "camilla", - "reward": { - "amount": "113822821", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:32:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 116 - } - ], - [ - 21755, - { - "block": 4873251, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "45438", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "69790115190", - "total_vote_weight": "15465017266140717410", - "voter": "bacchist", - "weight": "8432889990080521" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:34:09", - "trx_id": "24a95834bacb223f1e1074023016818fbe7d6eba", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21756, - { - "block": 4873251, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "bacchist", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:34:09", - "trx_id": "24a95834bacb223f1e1074023016818fbe7d6eba", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21757, - { - "block": 4873672, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "### Where the Trees will not grow in the cold weather, where the houses are far apart but the hearts are close together.\n\nhttps://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14202547_700195886812456_2583028795874812758_n.jpg?oh=4bc315321a733f73f19c05ac7789a9db&oe=587989D0\n\n### My Mother grew up here and I lived my first year in worlds most northern city called Hammerfest. The soil here is frozen, the land is flat and full of stones but still the tourists are very impressed.\n\nhttps://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\n\n### On a small island called Ertsvika my great grand parents lived with their 13 children. It was a hard life in poverty and cold winters, but their health where strong and their hearts where warm.\n\nhttps://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\n\n### They lived of fishing and gathering berries. The next neighbors where 8 hours away by foot. My great grand mother gave birth to all the children without any doctor to help her, the oldest daughter was the midwife. The oldest son had to fish with his father on the stormy ocean when he was 8 years old, he died early from hard work. \nhttps://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14264218_700195843479127_5684473362085111018_n.jpg?oh=f2679a94a09ac2bd847852dcf98df9de&oe=5876E718\n\n### Now the ancestors visit this place from time to time, where the old house used to be there is now a small cabin. There is a peaceful land far far north where nobody lives only birds and raindeers. \n\nhttps://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14289943_700195916812453_6155886871633627964_o.jpg\n\n### The pictures are taken by my mother who is there now, visiting her mother and her childhood places. I hope you enjoyed this glimpse from the past.", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14202547_700195886812456_2583028795874812758_n.jpg?oh=4bc315321a733f73f19c05ac7789a9db&oe=587989D0\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14264218_700195843479127_5684473362085111018_n.jpg?oh=f2679a94a09ac2bd847852dcf98df9de&oe=5876E718\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14289943_700195916812453_6155886871633627964_o.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:24", - "trx_id": "b32e4cb901a4a9975ad1f671e6bbc4f1641118ba", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21758, - { - "block": 4873672, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "79", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "211242392243", - "total_vote_weight": "925317040501509267", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:24", - "trx_id": "b32e4cb901a4a9975ad1f671e6bbc4f1641118ba", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21759, - { - "block": 4873672, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:24", - "trx_id": "b32e4cb901a4a9975ad1f671e6bbc4f1641118ba", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21760, - { - "block": 4873682, - "op": { - "type": "comment_operation", - "value": { - "author": "skeptic", - "body": "dickbutt\nhttps://steemit.com/dickbutt/@skeptic/dickbutt-has-taken-over-steemit", - "json_metadata": "{\"tags\":[\"history\"],\"links\":[\"https://steemit.com/dickbutt/@skeptic/dickbutt-has-taken-over-steemit\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t095553071z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:54", - "trx_id": "ff234139309f83dff10452a8ff8b9ad3542b7569", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21761, - { - "block": 4873683, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "79", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 111977218, - "total_vote_weight": "925782924035033837", - "voter": "skeptic", - "weight": "8541198114617" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:57", - "trx_id": "56e2c19aa29e1762753ece8fbb6ffb29e7a1977e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21762, - { - "block": 4873683, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "skeptic", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:55:57", - "trx_id": "56e2c19aa29e1762753ece8fbb6ffb29e7a1977e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21763, - { - "block": 4873708, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -127,144 +127,36 @@\n s://\n-scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14202547_700195886812456_2583028795874812758_n.jpg?oh=4bc315321a733f73f19c05ac7789a9db&oe=587989D0\n+i.imgsafe.org/52a5ddd3b8.jpg\n %0A%0A##\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14264218_700195843479127_5684473362085111018_n.jpg?oh=f2679a94a09ac2bd847852dcf98df9de&oe=5876E718\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14289943_700195916812453_6155886871633627964_o.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:57:12", - "trx_id": "0769fda0a66ef464397393c726f24f16d73be912", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21764, - { - "block": 4873750, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -1502,91 +1502,32 @@\n s://\n-scontent-arn2-1.xx.fbcdn.net/t31.0-8/14289943_700195916812453_6155886871633627964_o\n+i.imgsafe.org/52ad9349ed\n .jpg\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14264218_700195843479127_5684473362085111018_n.jpg?oh=f2679a94a09ac2bd847852dcf98df9de&oe=5876E718\",\"https://i.imgsafe.org/52ad9349ed.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:59:18", - "trx_id": "e24fb802687e51a260486c1702d98b1f4f9c04ed", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21765, - { - "block": 4873755, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "375", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "651645205561", - "total_vote_weight": "3273603472621919941", - "voter": "clains", - "weight": "324781842554519244" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:59:33", - "trx_id": "816d4634d0529ccd8da80473220dc190964d6800", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21766, - { - "block": 4873755, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "clains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:59:33", - "trx_id": "816d4634d0529ccd8da80473220dc190964d6800", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21767, - { - "block": 4873759, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "444", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "130765248410", - "total_vote_weight": "3670922843880873379", - "voter": "psylains", - "weight": "57611308832548248" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:59:45", - "trx_id": "9b138b8ad5a1ca9e3653e5c98b5ae848af084c92", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21768, - { - "block": 4873759, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "psylains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T09:59:45", - "trx_id": "9b138b8ad5a1ca9e3653e5c98b5ae848af084c92", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21769, - { - "block": 4873767, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "447", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "5888362824", - "total_vote_weight": "3688325130236049009", - "voter": "bitspace", - "weight": "2755362006236141" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:09", - "trx_id": "063dd139e93c41c4cee5fa1fc15276468dc5a3b0", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21770, - { - "block": 4873767, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "bitspace", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:09", - "trx_id": "063dd139e93c41c4cee5fa1fc15276468dc5a3b0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21771, - { - "block": 4873778, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "662", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "376233439931", - "total_vote_weight": "4721198610118978963", - "voter": "anonymous", - "weight": "182474314779317625" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:42", - "trx_id": "d8fb5d584bc14a3b9977a9b72db0d9b6af2bafc0", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21772, - { - "block": 4873778, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "anonymous", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:42", - "trx_id": "d8fb5d584bc14a3b9977a9b72db0d9b6af2bafc0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21773, - { - "block": 4873778, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "662", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1413239642, - "total_vote_weight": "4724805901034719817", - "voter": "murh", - "weight": "637288061780884" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:42", - "trx_id": "80531b7655b9c27e072eef7ca0e4ce5996017b35", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21774, - { - "block": 4873778, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "murh", - "weight": 3301 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:00:42", - "trx_id": "80531b7655b9c27e072eef7ca0e4ce5996017b35", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21775, - { - "block": 4873791, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "664", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1913344238, - "total_vote_weight": "4729686687557132567", - "voter": "earnest", - "weight": "968022660278528" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:01:21", - "trx_id": "b7f83027f36160b219a1ace2c0056b5f496c4f1a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21776, - { - "block": 4873791, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "earnest", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:01:21", - "trx_id": "b7f83027f36160b219a1ace2c0056b5f496c4f1a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21777, - { - "block": 4873814, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -1144,152 +1144,45 @@\n k. %0A\n+%0A\n https://\n-scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14264218_700195843479127_5684473362085111018_n.jpg?oh=f2679a94a09ac2bd847852dcf98df9de&oe=5876E718\n+i.imgsafe.org/52b994c30e.jpg\n %0A%0A##\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\",\"https://i.imgsafe.org/52b994c30e.jpg\",\"https://i.imgsafe.org/52ad9349ed.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:02:30", - "trx_id": "db0fd5d71745c5c000afb12dbc751cc2435cab3d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21778, - { - "block": 4873828, - "op": { - "type": "comment_operation", - "value": { - "author": "earnest", - "body": "are you the same person that sucks the cock stellabelle would like to have?\nthink about it, upvoted", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t100311500z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:03:12", - "trx_id": "8153c8b04e5761bd2332fc0621f296cb05883d50", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21779, - { - "block": 4873842, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -668,144 +668,36 @@\n s://\n-scontent-arn2-1.xx.fbcdn.net/v/t1.0-9/14225586_700245476807497_6573676145389719452_n.jpg?oh=8f4b3a87ec2231ca7384130bb5420d57&oe=58420C19\n+i.imgsafe.org/52be4729ec.jpg\n %0A%0A##\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://i.imgsafe.org/52be4729ec.jpg\",\"https://i.imgsafe.org/52b994c30e.jpg\",\"https://i.imgsafe.org/52ad9349ed.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:03:54", - "trx_id": "b4bb1969f4274333db37b79a77eaf5887bd1d90b", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 21780, - { - "block": 4873847, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1130", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "698098902296", - "total_vote_weight": "6305360690629014465", - "voter": "pfunk", - "weight": "459571584229298886" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:04:09", - "trx_id": "46c4fa75449125874544669b5a587d8f22cfe28a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21781, - { - "block": 4873847, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "pfunk", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:04:09", - "trx_id": "46c4fa75449125874544669b5a587d8f22cfe28a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21782, - { - "block": 4873862, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1131", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 62244532, - "total_vote_weight": "6305485042805156949", - "voter": "socialbutterfly", - "weight": "39378189111786" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:04:54", - "trx_id": "5db1f637f4c56c8714e8898140d89d7703623445", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21783, - { - "block": 4873862, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "socialbutterfly", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:04:54", - "trx_id": "5db1f637f4c56c8714e8898140d89d7703623445", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21784, - { - "block": 4873865, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -684,16 +684,16 @@\n g/52\n-be4729ec\n+c38d5ff5\n .jpg\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o.jpg\",\"https://i.imgsafe.org/52c38d5ff5.jpg\",\"https://i.imgsafe.org/52b994c30e.jpg\",\"https://i.imgsafe.org/52ad9349ed.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:05:03", - "trx_id": "4bdf442c011e572ccb86688a0a39adbcccd88441", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21785, - { - "block": 4873874, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1132", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 626660650, - "total_vote_weight": "6306736844055956688", - "voter": "steem1653", - "weight": "421439754435912" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:05:30", - "trx_id": "359a1dc478b58b6b42003bfa8df871f61f1d5760", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21786, - { - "block": 4873874, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "steem1653", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:05:30", - "trx_id": "359a1dc478b58b6b42003bfa8df871f61f1d5760", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21787, - { - "block": 4873883, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1147", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "20202699413", - "total_vote_weight": "6346955395012250957", - "voter": "samether", - "weight": "14143523752963484" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:05:57", - "trx_id": "ed3a545aee0815df385adbb2c7f3c8a7ea25f6b7", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21788, - { - "block": 4873883, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "samether", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:05:57", - "trx_id": "ed3a545aee0815df385adbb2c7f3c8a7ea25f6b7", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21789, - { - "block": 4873889, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -373,90 +373,32 @@\n s://\n-scontent-arn2-1.xx.fbcdn.net/t31.0-8/14257710_700195950145783_743288503190092860_o\n+i.imgsafe.org/52c7a83b71\n .jpg\n", - "json_metadata": "{\"tags\":[\"history\",\"memories\",\"family\",\"photography\"],\"image\":[\"https://i.imgsafe.org/52a5ddd3b8.jpg\",\"https://i.imgsafe.org/52c7a83b71.jpg\",\"https://i.imgsafe.org/52c38d5ff5.jpg\",\"https://i.imgsafe.org/52b994c30e.jpg\",\"https://i.imgsafe.org/52ad9349ed.jpg\"]}", - "parent_author": "", - "parent_permlink": "history", - "permlink": "there-is-a-land-far-far-north", - "title": "There is a Land far, far North..." - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:06:15", - "trx_id": "971676eac8a88d8c3be317b4ccd1569c4eea1431", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21790, - { - "block": 4873900, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dickbutt", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-re-skeptic-re-camilla-there-is-a-land-far-far-north-20160911t100406200z-20160911t100502", - "rshares": -211242392243, - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:06:48", - "trx_id": "bf83b6b33e4422813a97edbd8772a87bc03fcfcb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21791, - { - "block": 4873900, - "op": { - "type": "vote_operation", - "value": { - "author": "dickbutt", - "permlink": "re-re-skeptic-re-camilla-there-is-a-land-far-far-north-20160911t100406200z-20160911t100502", - "voter": "camilla", - "weight": -10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:06:48", - "trx_id": "bf83b6b33e4422813a97edbd8772a87bc03fcfcb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21792, - { - "block": 4873903, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "skeptic", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t095553071z", - "rshares": -211242392243, - "total_vote_weight": "8819524174382965", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:06:57", - "trx_id": "01afbe2fd76f15a55e1dd10f7f9c8e176847d67f", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21793, - { - "block": 4873903, - "op": { - "type": "vote_operation", - "value": { - "author": "skeptic", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t095553071z", - "voter": "camilla", - "weight": -10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:06:57", - "trx_id": "01afbe2fd76f15a55e1dd10f7f9c8e176847d67f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21794, - { - "block": 4873904, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dickbutt", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-re-camilla-there-is-a-land-far-far-north-20160911t095553071z-20160911t095642", - "rshares": -207017544398, - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:07:00", - "trx_id": "957328c81b3dc0f731fbafc2d6535f4840ed0e55", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21795, - { - "block": 4873904, - "op": { - "type": "vote_operation", - "value": { - "author": "dickbutt", - "permlink": "re-re-camilla-there-is-a-land-far-far-north-20160911t095553071z-20160911t095642", - "voter": "camilla", - "weight": -10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:07:00", - "trx_id": "957328c81b3dc0f731fbafc2d6535f4840ed0e55", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21796, - { - "block": 4873920, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1149", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1333641507, - "total_vote_weight": "6349600969497575589", - "voter": "elishagh1", - "weight": "1093504120600847" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:07:48", - "trx_id": "0df67e4c9953df2e06c8063fa80a654ee4130e3e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21797, - { - "block": 4873920, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "elishagh1", - "weight": 400 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:07:48", - "trx_id": "0df67e4c9953df2e06c8063fa80a654ee4130e3e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21798, - { - "block": 4873951, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1158", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "11521200292", - "total_vote_weight": "6372407756132747621", - "voter": "asim", - "weight": "10605155785354994" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:09:21", - "trx_id": "26b05ed779b77641ff1dbc6ffb0a452ad252f22a", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21799, - { - "block": 4873951, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "asim", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:09:21", - "trx_id": "26b05ed779b77641ff1dbc6ffb0a452ad252f22a", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21800, - { - "block": 4873965, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1190", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "42712960461", - "total_vote_weight": "6456215003138078692", - "voter": "alexgr", - "weight": "40925872287603339" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:10:03", - "trx_id": "d2ceb9ed7c5622a3326d58648c5dafbab81dc447", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 21801, - { - "block": 4873965, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "alexgr", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:10:03", - "trx_id": "d2ceb9ed7c5622a3326d58648c5dafbab81dc447", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 21802, - { - "block": 4873970, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "peskov", - "pending_payout": { - "amount": "30320", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-cup-of-tea-featuring-vasily-peskov-as-author", - "rshares": "207017544398", - "total_vote_weight": "14843396579493870159", - "voter": "camilla", - "weight": "36800406375438646" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:10:18", - "trx_id": "20f77aa8acf04044724eaec08df0d2aa334f848b", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21803, - { - "block": 4873970, - "op": { - "type": "vote_operation", - "value": { - "author": "peskov", - "permlink": "a-cup-of-tea-featuring-vasily-peskov-as-author", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:10:18", - "trx_id": "20f77aa8acf04044724eaec08df0d2aa334f848b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21804, - { - "block": 4873984, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1192", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1640980092, - "total_vote_weight": "6459411575179279942", - "voter": "gidlark", - "weight": "1662217461424650" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:11:00", - "trx_id": "ca6c79970f2b858d8d378cfb8089647bbaaf5607", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21805, - { - "block": 4873984, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "gidlark", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:11:00", - "trx_id": "ca6c79970f2b858d8d378cfb8089647bbaaf5607", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21806, - { - "block": 4874034, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3096", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "2044001489909", - "total_vote_weight": "9447689215416383726", - "voter": "badassmother", - "weight": "1802927509609719283" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:30", - "trx_id": "131a48dc630eff741b957976f75b36255be63310", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21807, - { - "block": 4874034, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "badassmother", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:30", - "trx_id": "131a48dc630eff741b957976f75b36255be63310", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21808, - { - "block": 4874036, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3097", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 664445440, - "total_vote_weight": "9448418401242171671", - "voter": "karen13", - "weight": "442372734311353" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:36", - "trx_id": "a68235ba03aefdd2327d72c4ce1ad2e6e39019ae", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21809, - { - "block": 4874036, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "karen13", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:36", - "trx_id": "a68235ba03aefdd2327d72c4ce1ad2e6e39019ae", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21810, - { - "block": 4874037, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3098", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1378514942, - "total_vote_weight": "9449930855245003686", - "voter": "taker", - "weight": "920076185056142" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:39", - "trx_id": "c9c155ae36f3707944785e9c6e3f6a9f1fda6c31", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21811, - { - "block": 4874037, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "taker", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:39", - "trx_id": "c9c155ae36f3707944785e9c6e3f6a9f1fda6c31", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21812, - { - "block": 4874040, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3100", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1027472226, - "total_vote_weight": "9451057827907077089", - "voter": "coar", - "weight": "691209899405020" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:48", - "trx_id": "ecbcaad710fa40faa03849adce42a988b02c8c33", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21813, - { - "block": 4874040, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "coar", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:13:48", - "trx_id": "ecbcaad710fa40faa03849adce42a988b02c8c33", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21814, - { - "block": 4874049, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3101", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 728479455, - "total_vote_weight": "9451856682294078194", - "voter": "alexma3x", - "weight": "501946839832360" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:15", - "trx_id": "7fad568fc22c5b1f991dd4b00416141facc90d36", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21815, - { - "block": 4874049, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "alexma3x", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:15", - "trx_id": "7fad568fc22c5b1f991dd4b00416141facc90d36", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21816, - { - "block": 4874049, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3101", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 336979073, - "total_vote_weight": "9452226167316916552", - "voter": "robotev1", - "weight": "232159756016768" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:15", - "trx_id": "3def6b10ce324851a9427e4784b8523eeb139edb", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21817, - { - "block": 4874049, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "robotev1", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:15", - "trx_id": "3def6b10ce324851a9427e4784b8523eeb139edb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21818, - { - "block": 4874052, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3105", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 3258419015, - "total_vote_weight": "9455797337387892571", - "voter": "glitterpig", - "weight": "2261741044951478" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:24", - "trx_id": "4fa2e3c409234651b1fd37d76ed9619789fd45dc", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21819, - { - "block": 4874052, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "glitterpig", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:24", - "trx_id": "4fa2e3c409234651b1fd37d76ed9619789fd45dc", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21820, - { - "block": 4874052, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4467", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "1119181397810", - "total_vote_weight": "10534771329717568694", - "voter": "rossco99", - "weight": "683350195142128211" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:24", - "trx_id": "a5a541e8cec1d504d5e034e27d0c92fd7f07abb8", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21821, - { - "block": 4874052, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "rossco99", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:24", - "trx_id": "a5a541e8cec1d504d5e034e27d0c92fd7f07abb8", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21822, - { - "block": 4874053, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5095", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "461536194020", - "total_vote_weight": "10907864778260602227", - "voter": "boatymcboatface", - "weight": "236914339824826293" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:27", - "trx_id": "00f0b60e6ae471301785e2e51f694f8b4b839d48", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21823, - { - "block": 4874053, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "boatymcboatface", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:14:27", - "trx_id": "00f0b60e6ae471301785e2e51f694f8b4b839d48", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21824, - { - "block": 4874132, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5109", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 3066392990, - "total_vote_weight": "10910225939460354233", - "voter": "funnyman", - "weight": "1814158855142791" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:18:27", - "trx_id": "96934940eb4f05632438487188653ae662a21e61", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21825, - { - "block": 4874132, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "funnyman", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:18:27", - "trx_id": "96934940eb4f05632438487188653ae662a21e61", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21826, - { - "block": 4874199, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5492", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "261981171388", - "total_vote_weight": "11106635929173180858", - "voter": "liberosist", - "weight": "172840790947287430" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:21:48", - "trx_id": "899c30727b0dca6e9627e7492d16b41bd9b8ddc6", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21827, - { - "block": 4874199, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "liberosist", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:21:48", - "trx_id": "899c30727b0dca6e9627e7492d16b41bd9b8ddc6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21828, - { - "block": 4874220, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5508", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "8953896241", - "total_vote_weight": "11113167995769612250", - "voter": "doitvoluntarily", - "weight": "5976840935734723" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:22:51", - "trx_id": "dd90f380d37188236a062c4dc48e566d1abd0ab2", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21829, - { - "block": 4874220, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "doitvoluntarily", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:22:51", - "trx_id": "dd90f380d37188236a062c4dc48e566d1abd0ab2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21830, - { - "block": 4874232, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5555", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "32296817492", - "total_vote_weight": "11136632954558278245", - "voter": "jl777", - "weight": "21939736467402705" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:27", - "trx_id": "dffeaaa4f3d4e8087d5d9fe49997413416439ed6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21831, - { - "block": 4874232, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "jl777", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:27", - "trx_id": "dffeaaa4f3d4e8087d5d9fe49997413416439ed6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21832, - { - "block": 4874233, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5559", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 2725653124, - "total_vote_weight": "11138606383854336184", - "voter": "proto", - "weight": "1848445440640936" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:30", - "trx_id": "9267caa121a1fafdd6cef0fd1132c17ffd5fa8e8", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21833, - { - "block": 4874233, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "proto", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:30", - "trx_id": "9267caa121a1fafdd6cef0fd1132c17ffd5fa8e8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21834, - { - "block": 4874235, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "649886", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-peek-into-pre-columbian-worldviews-found-in-agricultural-practices-duality-complementarity-cyclicity-and-reciprocity", - "rshares": "207017544398", - "total_vote_weight": "17597014638569603285", - "voter": "camilla", - "weight": "2030315015980568" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:36", - "trx_id": "b204fc4e4bce6248b4d66f856ea415a0e1c07c94", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21835, - { - "block": 4874235, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "a-peek-into-pre-columbian-worldviews-found-in-agricultural-practices-duality-complementarity-cyclicity-and-reciprocity", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:23:36", - "trx_id": "b204fc4e4bce6248b4d66f856ea415a0e1c07c94", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21836, - { - "block": 4874251, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5561", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 50985709, - "total_vote_weight": "11138643288411490344", - "voter": "natord", - "weight": "35674405249021" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:24:24", - "trx_id": "ca55341b991a2b4a7632455f9251f037d44eb4af", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21837, - { - "block": 4874251, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "natord", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:24:24", - "trx_id": "ca55341b991a2b4a7632455f9251f037d44eb4af", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21838, - { - "block": 4874528, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "14", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "a-quick-steem-elf-drawing", - "vesting_payout": { - "amount": "61510564", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21839, - { - "block": 4874528, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 39, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "835", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "29", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-quick-steem-elf-drawing", - "total_payout_value": { - "amount": "6987", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 21840, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "a-quick-steem-elf-drawing" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 21841, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-ryan-singer-re-camilla-a-quick-steem-elf-drawing-20160811t215726138z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 21842, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-hedge-x-re-camilla-a-quick-steem-elf-drawing-20160811t215808014z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 21843, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-opheliafu-re-camilla-a-quick-steem-elf-drawing-20160811t222944221z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 10 - } - ], - [ - 21844, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-bullionstackers-re-camilla-a-quick-steem-elf-drawing-20160812t125816467z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 22 - } - ], - [ - 21845, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-juvyjabian-re-camilla-a-quick-steem-elf-drawing-20160812t125915459z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 23 - } - ], - [ - 21846, - { - "block": 4874528, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-bullionstackers-re-camilla-re-bullionstackers-re-camilla-a-quick-steem-elf-drawing-20160813t152806440z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:38:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 25 - } - ], - [ - 21847, - { - "block": 4874585, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5609", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 3484563685, - "total_vote_weight": "11141164607951402125", - "voter": "alexft", - "weight": "2521319539911781" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:41:09", - "trx_id": "4dd1e57bda23e6be0f4859f3c26b3e859c22da8c", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21848, - { - "block": 4874585, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "alexft", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:41:09", - "trx_id": "4dd1e57bda23e6be0f4859f3c26b3e859c22da8c", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21849, - { - "block": 4874635, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5613", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 348014675, - "total_vote_weight": "11141416324760589706", - "voter": "bullionstackers", - "weight": "251716809187581" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:43:39", - "trx_id": "6e8d94c279015618890f109df0d248d9d35a4309", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21850, - { - "block": 4874635, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "bullionstackers", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:43:39", - "trx_id": "6e8d94c279015618890f109df0d248d9d35a4309", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21851, - { - "block": 4874832, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "lyubovbar", - "comment_permlink": "gentle-casserole-of-minced-chicken-and-vegetables-recipe-and-cooking-lyubovbar", - "curator": "camilla", - "reward": { - "amount": "76883771", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T10:53:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 21852, - { - "block": 4875179, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5865", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "131708490559", - "total_vote_weight": "11235450760796871897", - "voter": "team", - "weight": "94034436036282191" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:10:57", - "trx_id": "0b795c1b9159f5105a8ec197a81b7e0c704acb03", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21853, - { - "block": 4875179, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "team", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:10:57", - "trx_id": "0b795c1b9159f5105a8ec197a81b7e0c704acb03", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21854, - { - "block": 4875324, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6235", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "226192934152", - "total_vote_weight": "11391416660045886007", - "voter": "jesta", - "weight": "155965899249014110" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:18:12", - "trx_id": "1800b8fa05d4e0f9ed5edf5e567d2f0aa34abf0a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21855, - { - "block": 4875324, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "jesta", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:18:12", - "trx_id": "1800b8fa05d4e0f9ed5edf5e567d2f0aa34abf0a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21856, - { - "block": 4875398, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "jamielefay", - "pending_payout": { - "amount": "60657", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "ahe-ey-allegiance-an-original-novel-part-18", - "rshares": "207018222530", - "total_vote_weight": "15781801981480933436", - "voter": "camilla", - "weight": "20075379046871439" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:00", - "trx_id": "822ceea944fc6db4a483737a91c09b8f5f7aa71e", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21857, - { - "block": 4875398, - "op": { - "type": "vote_operation", - "value": { - "author": "jamielefay", - "permlink": "ahe-ey-allegiance-an-original-novel-part-18", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:00", - "trx_id": "822ceea944fc6db4a483737a91c09b8f5f7aa71e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21858, - { - "block": 4875400, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "complexring", - "pending_payout": { - "amount": "1159634", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "bypassing-the-great-firewall-of-china", - "rshares": "207018222530", - "total_vote_weight": "17799465840281566834", - "voter": "camilla", - "weight": "1177606706720511" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:06", - "trx_id": "b96ed906ee75ba295a8682a2b00f4c0fe9578750", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21859, - { - "block": 4875400, - "op": { - "type": "vote_operation", - "value": { - "author": "complexring", - "permlink": "bypassing-the-great-firewall-of-china", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:06", - "trx_id": "b96ed906ee75ba295a8682a2b00f4c0fe9578750", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21860, - { - "block": 4875401, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "smailer", - "pending_payout": { - "amount": "37201", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-daytime-latte-art-tryings-200-followers-milestone", - "rshares": "207018222530", - "total_vote_weight": "15116351817870209333", - "voter": "camilla", - "weight": "31412079103338308" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:09", - "trx_id": "6af7ebc02d31c437c2c33ee97184d7c59d757344", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21861, - { - "block": 4875401, - "op": { - "type": "vote_operation", - "value": { - "author": "smailer", - "permlink": "my-daytime-latte-art-tryings-200-followers-milestone", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:09", - "trx_id": "6af7ebc02d31c437c2c33ee97184d7c59d757344", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21862, - { - "block": 4875405, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "aaronkoenig", - "pending_payout": { - "amount": "147540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "steemit-meetup-berlin-with-ned-scott-and-steemians-from-hamburg", - "rshares": "202793360846", - "total_vote_weight": "5016696368721867925", - "voter": "camilla", - "weight": "14826712462658847" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:21", - "trx_id": "2f4e6939c728fc2c7def8f5ad6ff7901bff38bbb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21863, - { - "block": 4875405, - "op": { - "type": "vote_operation", - "value": { - "author": "aaronkoenig", - "permlink": "steemit-meetup-berlin-with-ned-scott-and-steemians-from-hamburg", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:22:21", - "trx_id": "2f4e6939c728fc2c7def8f5ad6ff7901bff38bbb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21864, - { - "block": 4875430, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "ekaterina4ka", - "comment_permlink": "knitting-needles-my-passion-post-14-vyazanie-spicami-moe-uvlechenie-post-14", - "curator": "camilla", - "reward": { - "amount": "79950080", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:23:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21865, - { - "block": 4875431, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "royalmacro", - "pending_payout": { - "amount": "75139", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "moonlit-night-an-original-abstract-art-and-a-poem", - "rshares": "202793744606", - "total_vote_weight": "16036119854337407944", - "voter": "camilla", - "weight": "16077583325629225" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:23:39", - "trx_id": "f259c00f8ceda578a82526460f2eb9e100c6a36d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21866, - { - "block": 4875431, - "op": { - "type": "vote_operation", - "value": { - "author": "royalmacro", - "permlink": "moonlit-night-an-original-abstract-art-and-a-poem", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:23:39", - "trx_id": "f259c00f8ceda578a82526460f2eb9e100c6a36d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21867, - { - "block": 4875443, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "labradorsem", - "pending_payout": { - "amount": "171934", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "diet-apple-pie-with-apples-and-pears", - "rshares": "202793744606", - "total_vote_weight": "16817917632697667692", - "voter": "camilla", - "weight": "7324415880569540" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:24:15", - "trx_id": "960fd778a7cf80f2a3aa747a2670e311c701a6ca", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21868, - { - "block": 4875443, - "op": { - "type": "vote_operation", - "value": { - "author": "labradorsem", - "permlink": "diet-apple-pie-with-apples-and-pears", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:24:15", - "trx_id": "960fd778a7cf80f2a3aa747a2670e311c701a6ca", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21869, - { - "block": 4875689, - "op": { - "type": "comment_operation", - "value": { - "author": "alexft", - "body": "=) Thank you for your art !!!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t090442054z", - "permlink": "re-camilla-re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t113557524z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:36:33", - "trx_id": "fdea88359b8d845d2a96c1ac35f852231ba5f8d9", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21870, - { - "block": 4875691, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t090442054z", - "rshares": 3484600591, - "total_vote_weight": "16055896728511211", - "voter": "alexft", - "weight": "16055896728511211" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:36:39", - "trx_id": "fb77e3168e5e01387447a9fc26eda34b51bccf9a", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21871, - { - "block": 4875691, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t090442054z", - "voter": "alexft", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:36:39", - "trx_id": "fb77e3168e5e01387447a9fc26eda34b51bccf9a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21872, - { - "block": 4875787, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "xiaofang", - "pending_payout": { - "amount": "167211", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-photography-flower-collections", - "rshares": "202793744606", - "total_vote_weight": "16801622792369674535", - "voter": "camilla", - "weight": "7472032505573390" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:41:30", - "trx_id": "f5b6b4ad06a33ba3d187d8b4eb8c6bdd478a56a3", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21873, - { - "block": 4875787, - "op": { - "type": "vote_operation", - "value": { - "author": "xiaofang", - "permlink": "my-photography-flower-collections", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:41:30", - "trx_id": "f5b6b4ad06a33ba3d187d8b4eb8c6bdd478a56a3", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21874, - { - "block": 4875794, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dumar022", - "pending_payout": { - "amount": "174056", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "i-never-plan-to-get-a-new-cactus-but-it-happens-all-the-time", - "rshares": "202793744606", - "total_vote_weight": "16832681387978976235", - "voter": "camilla", - "weight": "7191946835167933" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:41:51", - "trx_id": "5d36cab49154a00be1775a173967bfd3915b55bc", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21875, - { - "block": 4875794, - "op": { - "type": "vote_operation", - "value": { - "author": "dumar022", - "permlink": "i-never-plan-to-get-a-new-cactus-but-it-happens-all-the-time", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:41:51", - "trx_id": "5d36cab49154a00be1775a173967bfd3915b55bc", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21876, - { - "block": 4875855, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6196", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "17414401735", - "total_vote_weight": "11403145110532426308", - "voter": "r4fken", - "weight": "11728450486540301" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:44:54", - "trx_id": "f62ad22c4d94603c925d24caab40a552f965c76b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21877, - { - "block": 4875855, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "r4fken", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:44:54", - "trx_id": "f62ad22c4d94603c925d24caab40a552f965c76b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21878, - { - "block": 4875953, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "diana.catherine", - "pending_payout": { - "amount": "29058", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-last-days-in-greece-cloudy-but-beautiful-shocked-but-alive-part-3", - "rshares": "198568874927", - "total_vote_weight": "14745668559402477023", - "voter": "camilla", - "weight": "37233564351029599" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:49:48", - "trx_id": "69f54f6f36b4fbdbf8f9df148b519d94ea6935ba", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21879, - { - "block": 4875953, - "op": { - "type": "vote_operation", - "value": { - "author": "diana.catherine", - "permlink": "my-last-days-in-greece-cloudy-but-beautiful-shocked-but-alive-part-3", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T11:49:48", - "trx_id": "69f54f6f36b4fbdbf8f9df148b519d94ea6935ba", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21880, - { - "block": 4876379, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-clains-where-does-the-money-in-steem-come-from-20160811t223515052z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:11:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 11 - } - ], - [ - 21881, - { - "block": 4876485, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6099", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 3985741522, - "total_vote_weight": "11405823991723011311", - "voter": "sompitonov", - "weight": "2678881190585003" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:16:27", - "trx_id": "563421818674195d2d64ffebcd9c5fa84b36b5f9", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21882, - { - "block": 4876485, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "sompitonov", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:16:27", - "trx_id": "563421818674195d2d64ffebcd9c5fa84b36b5f9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21883, - { - "block": 4876557, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6096", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "22980208089", - "total_vote_weight": "11421229704066695932", - "voter": "jasonstaggers", - "weight": "15405712343684621" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:20:03", - "trx_id": "d1ad0fddbba0cb48b64a575f8706c80b75f53117", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21884, - { - "block": 4876557, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "jasonstaggers", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:20:03", - "trx_id": "d1ad0fddbba0cb48b64a575f8706c80b75f53117", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21885, - { - "block": 4877027, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6164", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "8338989775", - "total_vote_weight": "11426803425944967022", - "voter": "givemeyoursteem", - "weight": "5573721878271090" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:43:42", - "trx_id": "f6126443775983f307d39bbf3ae139e14a72c9bc", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21886, - { - "block": 4877027, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "givemeyoursteem", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:43:42", - "trx_id": "f6126443775983f307d39bbf3ae139e14a72c9bc", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21887, - { - "block": 4877054, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "46123", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "7998622846", - "total_vote_weight": "15465980719851167154", - "voter": "givemeyoursteem", - "weight": "963453710449744" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:45:03", - "trx_id": "967eeeaff88d57ca73c16edfece0f646777814d5", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21888, - { - "block": 4877054, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "givemeyoursteem", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:45:03", - "trx_id": "967eeeaff88d57ca73c16edfece0f646777814d5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21889, - { - "block": 4877111, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "cold , but very beautiful place!", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:47:54", - "trx_id": "cdc93bf1db588695aaa46a29dc2cf97f92e5ebb6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21890, - { - "block": 4877118, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "cold, but very beautiful place!", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:48:15", - "trx_id": "cc8b2a5acdd2a8079acbff6191829d2fa04357cb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21891, - { - "block": 4877125, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "cold, but very beautiful places!", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:48:36", - "trx_id": "e5a2bac75119d8127c38a6764f13f114a462bbef", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21892, - { - "block": 4877146, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "sompitonov", - "pending_payout": { - "amount": "76", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "rshares": "202793744606", - "total_vote_weight": "890094668884779200", - "voter": "camilla", - "weight": "51922189018278786" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:49:39", - "trx_id": "cf10740bca5bb858bda2d69878d2bf31f6739c60", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21893, - { - "block": 4877146, - "op": { - "type": "vote_operation", - "value": { - "author": "sompitonov", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:49:39", - "trx_id": "cf10740bca5bb858bda2d69878d2bf31f6739c60", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21894, - { - "block": 4877152, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "yes it is beautiful in the summer :)", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "sompitonov", - "parent_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "permlink": "re-sompitonov-re-camilla-there-is-a-land-far-far-north-20160911t124956506z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:49:57", - "trx_id": "0bac48e1b70f88c205eb2cbe1edbce7206a8bb62", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21895, - { - "block": 4877179, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dailybest", - "pending_payout": { - "amount": "143239", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "sketch-4-birds-in-love", - "rshares": "198568874927", - "total_vote_weight": "16806047982407796105", - "voter": "camilla", - "weight": "7276282158252599" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:51:18", - "trx_id": "0739fba20ea9a40cdd32106088dff1257e30c1d2", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 21896, - { - "block": 4877179, - "op": { - "type": "vote_operation", - "value": { - "author": "dailybest", - "permlink": "sketch-4-birds-in-love", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:51:18", - "trx_id": "0739fba20ea9a40cdd32106088dff1257e30c1d2", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 21897, - { - "block": 4877185, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dailybest", - "pending_payout": { - "amount": "141859", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "sketch-4-birds-in-love", - "rshares": 4224869679, - "total_vote_weight": "16798771700249543506", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:51:36", - "trx_id": "8af4d88f84027314a556a131b34a6e55d956d279", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21898, - { - "block": 4877185, - "op": { - "type": "vote_operation", - "value": { - "author": "dailybest", - "permlink": "sketch-4-birds-in-love", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T12:51:36", - "trx_id": "8af4d88f84027314a556a131b34a6e55d956d279", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21899, - { - "block": 4877355, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "alexft", - "pending_payout": { - "amount": "73", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t113557524z", - "rshares": "198568874927", - "total_vote_weight": "872428040577635556", - "voter": "camilla", - "weight": "872428040577635556" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:00:09", - "trx_id": "4ea1437797feb5ff91c3e5f0bebceff16388733c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21900, - { - "block": 4877355, - "op": { - "type": "vote_operation", - "value": { - "author": "alexft", - "permlink": "re-camilla-re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t113557524z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:00:09", - "trx_id": "4ea1437797feb5ff91c3e5f0bebceff16388733c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21901, - { - "block": 4877360, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "sompitonov", - "pending_payout": { - "amount": "73", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t093158180z", - "rshares": "198568874927", - "total_vote_weight": "872428040577635556", - "voter": "camilla", - "weight": "872428040577635556" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:00:24", - "trx_id": "954cd9fa0c07baac4df1c975a3318e0cbb6d2e3a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21902, - { - "block": 4877360, - "op": { - "type": "vote_operation", - "value": { - "author": "sompitonov", - "permlink": "re-camilla-re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t093158180z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:00:24", - "trx_id": "954cd9fa0c07baac4df1c975a3318e0cbb6d2e3a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21903, - { - "block": 4877847, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5984", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 327972405, - "total_vote_weight": "11427022459635292626", - "voter": "valenttina", - "weight": "219033690325604" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:25:00", - "trx_id": "ddc2b116e4a2b46e0ec564d68170d9786af97c68", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21904, - { - "block": 4877847, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "valenttina", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:25:00", - "trx_id": "ddc2b116e4a2b46e0ec564d68170d9786af97c68", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21905, - { - "block": 4878056, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "opheliafu", - "pending_payout": { - "amount": "1468", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-blue-raven", - "rshares": "198568874927", - "total_vote_weight": "7219155244931895403", - "voter": "camilla", - "weight": "86285797782688759" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:35:30", - "trx_id": "f4740723aed0bf84cf0023589d43c3687ff47f92", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21906, - { - "block": 4878056, - "op": { - "type": "vote_operation", - "value": { - "author": "opheliafu", - "permlink": "the-blue-raven", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:35:30", - "trx_id": "f4740723aed0bf84cf0023589d43c3687ff47f92", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21907, - { - "block": 4878079, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "terrycraft", - "pending_payout": { - "amount": "286113", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "traveling-to-peru-to-the-wild-jungle-through-the-city-of-shamans-iquitos-puteshestvie-v-ikitos-featuring-njall-as-author", - "rshares": "198568874927", - "total_vote_weight": "17204723480537767766", - "voter": "camilla", - "weight": "3700147498508231" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:36:39", - "trx_id": "fa03bc1d5f1aa03ba698e8c2326205100bde0a41", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 21908, - { - "block": 4878079, - "op": { - "type": "vote_operation", - "value": { - "author": "terrycraft", - "permlink": "traveling-to-peru-to-the-wild-jungle-through-the-city-of-shamans-iquitos-puteshestvie-v-ikitos-featuring-njall-as-author", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:36:39", - "trx_id": "fa03bc1d5f1aa03ba698e8c2326205100bde0a41", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 21909, - { - "block": 4878288, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "6005", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "27088176517", - "total_vote_weight": "11445066013656557447", - "voter": "tmendieta", - "weight": "18043554021264821" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:47:09", - "trx_id": "156d892d824259fe4ee7bef08a04341910f6abb4", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21910, - { - "block": 4878288, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "tmendieta", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T13:47:09", - "trx_id": "156d892d824259fe4ee7bef08a04341910f6abb4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21911, - { - "block": 4879035, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5886", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "10310589431", - "total_vote_weight": "11451909593834525458", - "voter": "mun", - "weight": "6843580177968011" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T14:24:39", - "trx_id": "66285ae6cb89f738a317b7aed932531ffcf160e5", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21912, - { - "block": 4879035, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "mun", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T14:24:39", - "trx_id": "66285ae6cb89f738a317b7aed932531ffcf160e5", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21913, - { - "block": 4880294, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5776", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "16193082064", - "total_vote_weight": "11462630673607067285", - "voter": "b4bb4r-5h3r", - "weight": "10721079772541827" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T15:27:57", - "trx_id": "0abbcd564fb2eea5c6030eb23372418bbefd20d6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21914, - { - "block": 4880294, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "b4bb4r-5h3r", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T15:27:57", - "trx_id": "0abbcd564fb2eea5c6030eb23372418bbefd20d6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21915, - { - "block": 4880302, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5798", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "14860602062", - "total_vote_weight": "11472440669147249884", - "voter": "randyclemens", - "weight": "9809995540182599" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T15:28:21", - "trx_id": "e304954a7d34fa662b577685b134d2a2f74dc6ca", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21916, - { - "block": 4880302, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "randyclemens", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T15:28:21", - "trx_id": "e304954a7d34fa662b577685b134d2a2f74dc6ca", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21917, - { - "block": 4880788, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-fairytalelife-for-the-love-of-a-pug-20160812t125401776z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T15:52:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 29 - } - ], - [ - 21918, - { - "block": 4880977, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-sompitonov-re-camilla-there-is-a-land-far-far-north-20160911t124956506z", - "rshares": 3985741522, - "total_vote_weight": "18362691214865208", - "voter": "sompitonov", - "weight": "18362691214865208" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T16:02:18", - "trx_id": "6f301c16cb86c26c3aadb5c2312dd7031424544d", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21919, - { - "block": 4880977, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-sompitonov-re-camilla-there-is-a-land-far-far-north-20160911t124956506z", - "voter": "sompitonov", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T16:02:18", - "trx_id": "6f301c16cb86c26c3aadb5c2312dd7031424544d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21920, - { - "block": 4883321, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "diana.catherine", - "comment_permlink": "my-last-days-in-greece-cloudy-but-beautiful-shocked-but-alive-part-3", - "curator": "camilla", - "reward": { - "amount": "67549036", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T18:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 13 - } - ], - [ - 21921, - { - "block": 4884483, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5727", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "24219759293", - "total_vote_weight": "11488370043363254252", - "voter": "thecryptofiend", - "weight": "15929374216004368" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T18:58:30", - "trx_id": "62070e5269dea8573ef09708be431a75148edb09", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21922, - { - "block": 4884483, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "thecryptofiend", - "weight": 3100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T18:58:30", - "trx_id": "62070e5269dea8573ef09708be431a75148edb09", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21923, - { - "block": 4884669, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5760", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "51727323862", - "total_vote_weight": "11522148707244888613", - "voter": "kus-knee", - "weight": "33778663881634361" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T19:07:48", - "trx_id": "36a382c40cb4c534084e96e7d053bdf18d926b23", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21924, - { - "block": 4884669, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "kus-knee", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T19:07:48", - "trx_id": "36a382c40cb4c534084e96e7d053bdf18d926b23", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21925, - { - "block": 4884684, - "op": { - "type": "comment_operation", - "value": { - "author": "kus-knee", - "body": "Very cool shots! UPVOTED!\n\n@kus-knee (The Old Dog)", - "json_metadata": "{\"tags\":[\"history\"],\"users\":[\"kus-knee\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t190836197z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T19:08:36", - "trx_id": "c5417b6d7028dff543bdd901e10a593416f260c1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21926, - { - "block": 4885364, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "kus-knee", - "pending_payout": { - "amount": "71", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t190836197z", - "rshares": "207018945276", - "total_vote_weight": "907727193908588940", - "voter": "camilla", - "weight": "907727193908588940" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T19:42:42", - "trx_id": "641461be71d572c08e6d7e81f339e06df1416d74", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21927, - { - "block": 4885364, - "op": { - "type": "vote_operation", - "value": { - "author": "kus-knee", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t190836197z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T19:42:42", - "trx_id": "641461be71d572c08e6d7e81f339e06df1416d74", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21928, - { - "block": 4886346, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5770", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "9721776176", - "total_vote_weight": "11528460586161603480", - "voter": "andread", - "weight": "6311878916714867" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T20:32:09", - "trx_id": "590fa0c69e9f4198e023a82577043dec8ad4f4c6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21929, - { - "block": 4886346, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "andread", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T20:32:09", - "trx_id": "590fa0c69e9f4198e023a82577043dec8ad4f4c6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21930, - { - "block": 4886355, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "41749", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "9531153114", - "total_vote_weight": "15467127957908421649", - "voter": "andread", - "weight": "1147238057254495" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T20:32:36", - "trx_id": "dce6d93b86f52c8816f3fa50c09b6d2ae2c31525", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21931, - { - "block": 4886355, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "andread", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T20:32:36", - "trx_id": "dce6d93b86f52c8816f3fa50c09b6d2ae2c31525", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21932, - { - "block": 4887018, - "op": { - "type": "comment_operation", - "value": { - "author": "shadowspub", - "body": "Hi @camilla, just stopping back to let you know that I included you as one of my favourite reads on my ramble today. [You can see what I had to say here.](https://steemit.com/life/@shadowspub/sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th)", - "json_metadata": "{\"tags\":[\"history\"],\"users\":[\"camilla\"],\"links\":[\"https://steemit.com/life/@shadowspub/sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t210552014z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:05:57", - "trx_id": "97a5f595a25c454e3d2f91453f7cd314d5d73e3d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21933, - { - "block": 4887445, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5703", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 88662747, - "total_vote_weight": "11528518097644600231", - "voter": "mlialen", - "weight": "57511482996751" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:27:21", - "trx_id": "c77e8042a5e39cb8c0a75d894bea08ba65a4016d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21934, - { - "block": 4887445, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "mlialen", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:27:21", - "trx_id": "c77e8042a5e39cb8c0a75d894bea08ba65a4016d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21935, - { - "block": 4887456, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5706", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1515433531, - "total_vote_weight": "11529500942486959039", - "voter": "robotev", - "weight": "982844842358808" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:27:54", - "trx_id": "fc0ff3f55492df2111d5c31149ab31fd32363c08", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21936, - { - "block": 4887456, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "robotev", - "weight": 4500 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:27:54", - "trx_id": "fc0ff3f55492df2111d5c31149ab31fd32363c08", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21937, - { - "block": 4887469, - "op": { - "type": "comment_operation", - "value": { - "author": "mlialen", - "body": "Really nice pictures. I admit I had to look up where it was :)", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t212832160z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:28:33", - "trx_id": "c2c15e64958c70be96bb73be16702d082e2d9ccd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21938, - { - "block": 4887978, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "anwenbaumeister", - "comment_permlink": "a-peek-into-pre-columbian-worldviews-found-in-agricultural-practices-duality-complementarity-cyclicity-and-reciprocity", - "curator": "camilla", - "reward": { - "amount": "70557284", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:54:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 77 - } - ], - [ - 21939, - { - "block": 4889041, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "mlialen", - "pending_payout": { - "amount": "68", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t212832160z", - "rshares": "211244174496", - "total_vote_weight": "925324455796039880", - "voter": "camilla", - "weight": "925324455796039880" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:47:30", - "trx_id": "b1888d99f9d5919cc21f6a8075f14a2f5d2757b9", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21940, - { - "block": 4889041, - "op": { - "type": "vote_operation", - "value": { - "author": "mlialen", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t212832160z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:47:30", - "trx_id": "b1888d99f9d5919cc21f6a8075f14a2f5d2757b9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21941, - { - "block": 4889045, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shadowspub", - "pending_payout": { - "amount": "68", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t210552014z", - "rshares": "211244174496", - "total_vote_weight": "925324455796039880", - "voter": "camilla", - "weight": "925324455796039880" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:47:42", - "trx_id": "ccc6d704e4f1c97b4d9cb5bf5745d7a9b46b1b58", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21942, - { - "block": 4889045, - "op": { - "type": "vote_operation", - "value": { - "author": "shadowspub", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160911t210552014z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:47:42", - "trx_id": "ccc6d704e4f1c97b4d9cb5bf5745d7a9b46b1b58", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21943, - { - "block": 4889061, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shadowspub", - "pending_payout": { - "amount": "149", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th", - "rshares": "211244174496", - "total_vote_weight": "1816970872818667988", - "voter": "camilla", - "weight": "831309056670076610" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:48:30", - "trx_id": "38d160844669cade401ef475c7434212b041df05", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21944, - { - "block": 4889061, - "op": { - "type": "vote_operation", - "value": { - "author": "shadowspub", - "permlink": "sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:48:30", - "trx_id": "38d160844669cade401ef475c7434212b041df05", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21945, - { - "block": 4889077, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thank you! :)", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "shadowspub", - "parent_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t210552014z", - "permlink": "re-shadowspub-re-camilla-there-is-a-land-far-far-north-20160911t224915410z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:49:18", - "trx_id": "132fbba08c250a60a87a63014cb090ab88513ee6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21946, - { - "block": 4889093, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "so cool that you actually looked it up :D", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "mlialen", - "parent_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t212832160z", - "permlink": "re-mlialen-re-camilla-there-is-a-land-far-far-north-20160911t225003383z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:50:06", - "trx_id": "8862c70edfed8ec80a52a72ab4c1f09e9e0dbfd7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21947, - { - "block": 4889132, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"anca3drandom\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:52:09", - "trx_id": "5404b0f28338eaa69e26248cc8fe86a550bb9ee0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21948, - { - "block": 4889160, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"gretepe\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:53:33", - "trx_id": "641ca9fbef69cc7e52fe2e146885aafe2e3077cd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21949, - { - "block": 4889162, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"stellabelle\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:53:39", - "trx_id": "3c26b8176e0426a7df165793c57f60063471c8c8", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21950, - { - "block": 4889166, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"soulsistashakti\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:53:51", - "trx_id": "a9d775c6b9f2a3742ef68963fb2ba09dc22e2c36", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21951, - { - "block": 4889197, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "good! how about you?", - "json_metadata": "{\"tags\":[\"steemmag\"]}", - "parent_author": "infovore", - "parent_permlink": "re-camilla-re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t184739329z", - "permlink": "re-infovore-re-camilla-re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160911t225522735z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:55:24", - "trx_id": "40293d46e503463069293936ce6a0fb1dd0ec363", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21952, - { - "block": 4889199, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "infovore", - "pending_payout": { - "amount": "66", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t184739329z", - "rshares": "207019291006", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:55:30", - "trx_id": "e1f7e70ca2babb397f7e6f498a23a49c747d5bb6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21953, - { - "block": 4889199, - "op": { - "type": "vote_operation", - "value": { - "author": "infovore", - "permlink": "re-camilla-re-infovore-a-day-in-the-life-of-a-steemcleaner-steemians-speak-fondest-moment-on-steemit-this-week-on-steemit-steemmag-steemit-s-weekend-20160910t184739329z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T22:55:30", - "trx_id": "e1f7e70ca2babb397f7e6f498a23a49c747d5bb6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21954, - { - "block": 4889381, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "kus-knee", - "pending_payout": { - "amount": "36773", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-old-dog-investigates-the-curious-case-of-the-swiss-bomb-shelters", - "rshares": "207019291006", - "total_vote_weight": "15367557175106939833", - "voter": "camilla", - "weight": "26833134392282322" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T23:04:42", - "trx_id": "7033e8aca84300efc9827c50677e045e81df22e1", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21955, - { - "block": 4889381, - "op": { - "type": "vote_operation", - "value": { - "author": "kus-knee", - "permlink": "the-old-dog-investigates-the-curious-case-of-the-swiss-bomb-shelters", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T23:04:42", - "trx_id": "7033e8aca84300efc9827c50677e045e81df22e1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21956, - { - "block": 4889541, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "sirwinchester", - "pending_payout": { - "amount": "325749", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "bitcoin-exchange-berlin-meetup-with-ned-on-skype", - "rshares": "207019291006", - "total_vote_weight": "17352183617643928010", - "voter": "camilla", - "weight": "3236812831396389" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T23:12:42", - "trx_id": "3360bf4fd1c4e0dc8f57c4b7ac617cc1a480d56b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21957, - { - "block": 4889541, - "op": { - "type": "vote_operation", - "value": { - "author": "sirwinchester", - "permlink": "bitcoin-exchange-berlin-meetup-with-ned-on-skype", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T23:12:42", - "trx_id": "3360bf4fd1c4e0dc8f57c4b7ac617cc1a480d56b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21958, - { - "block": 4891107, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-innuendo-should-we-establish-a-long-run-cap-for-steem-supply-20160812t120413402z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T00:31:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 11 - } - ], - [ - 21959, - { - "block": 4891910, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "23456651896", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "17038", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "vesting_payout": { - "amount": "72543343847", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 48 - } - ], - [ - 21960, - { - "block": 4891910, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 47330, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "5509", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "39587", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "total_payout_value": { - "amount": "34076", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 49 - } - ], - [ - 21961, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "runridefly", - "comment_permlink": "re-camilla-colors-of-the-falling-leaves-20160910t233510136z", - "curator": "camilla", - "reward": { - "amount": "12261710", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 50 - } - ], - [ - 21962, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "bullionstackers", - "comment_permlink": "re-camilla-colors-of-the-falling-leaves-20160910t234539398z", - "curator": "camilla", - "reward": { - "amount": "9196282", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 53 - } - ], - [ - 21963, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "sompitonov", - "comment_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t001828852z", - "curator": "camilla", - "reward": { - "amount": "70504834", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 56 - } - ], - [ - 21964, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "tmendieta", - "comment_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t034905376z", - "curator": "camilla", - "reward": { - "amount": "70504834", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 59 - } - ], - [ - 21965, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alexft", - "comment_permlink": "re-camilla-colors-of-the-falling-leaves-20160911t065104109z", - "curator": "camilla", - "reward": { - "amount": "70504834", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 62 - } - ], - [ - 21966, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "sompitonov", - "comment_permlink": "re-camilla-re-sompitonov-re-camilla-colors-of-the-falling-leaves-20160911t093158180z", - "curator": "camilla", - "reward": { - "amount": "67439406", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 65 - } - ], - [ - 21967, - { - "block": 4891910, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alexft", - "comment_permlink": "re-camilla-re-alexft-re-camilla-colors-of-the-falling-leaves-20160911t113557524z", - "curator": "camilla", - "reward": { - "amount": "67439406", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T01:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 68 - } - ], - [ - 21968, - { - "block": 4898733, - "op": { - "type": "comment_operation", - "value": { - "author": "natord", - "body": "It reminded me of my homeland, behind Arctic-circle. North nature is special. harsh and beautiful.\n\nhttps://steemimg.com/images/2016/09/12/0f97bbb545b2a54.jpg", - "json_metadata": "{\"tags\":[\"history\"],\"image\":[\"https://steemimg.com/images/2016/09/12/0f97bbb545b2a54.jpg\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160912t065441930z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T06:54:39", - "trx_id": "8fb3d72454efa5d6b6170da94c17c6b59770bea5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21969, - { - "block": 4901590, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "royalmacro", - "comment_permlink": "moonlit-night-an-original-abstract-art-and-a-poem", - "curator": "camilla", - "reward": { - "amount": "70375405", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T09:19:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 15 - } - ], - [ - 21970, - { - "block": 4902469, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "peskov", - "comment_permlink": "a-cup-of-tea-featuring-vasily-peskov-as-author", - "curator": "camilla", - "reward": { - "amount": "70363715", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T10:03:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 9 - } - ], - [ - 21971, - { - "block": 4903433, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "smailer", - "comment_permlink": "my-daytime-latte-art-tryings-200-followers-milestone", - "curator": "camilla", - "reward": { - "amount": "76468318", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T10:52:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 21972, - { - "block": 4903881, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "complexring", - "comment_permlink": "bypassing-the-great-firewall-of-china", - "curator": "camilla", - "reward": { - "amount": "91754176", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:14:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 62 - } - ], - [ - 21973, - { - "block": 4903978, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "2284636695", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "2597", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "vesting_payout": { - "amount": "11062290396", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 26 - } - ], - [ - 21974, - { - "block": 4903978, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 7234, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "536", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "5730", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "total_payout_value": { - "amount": "5194", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 27 - } - ], - [ - 21975, - { - "block": 4903978, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "sompitonov", - "comment_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t124743311z", - "curator": "camilla", - "reward": { - "amount": "3058415", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 28 - } - ], - [ - 21976, - { - "block": 4903978, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "kus-knee", - "comment_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t190836197z", - "curator": "camilla", - "reward": { - "amount": "73401982", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 31 - } - ], - [ - 21977, - { - "block": 4903978, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "shadowspub", - "comment_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t210552014z", - "curator": "camilla", - "reward": { - "amount": "73401982", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 34 - } - ], - [ - 21978, - { - "block": 4903978, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "mlialen", - "comment_permlink": "re-camilla-there-is-a-land-far-far-north-20160911t212832160z", - "curator": "camilla", - "reward": { - "amount": "73401982", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:19:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 37 - } - ], - [ - 21979, - { - "block": 4904290, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "dumar022", - "comment_permlink": "i-never-plan-to-get-a-new-cactus-but-it-happens-all-the-time", - "curator": "camilla", - "reward": { - "amount": "70339420", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:34:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 21980, - { - "block": 4904405, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "xiaofang", - "comment_permlink": "my-photography-flower-collections", - "curator": "camilla", - "reward": { - "amount": "73396053", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T11:40:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 21981, - { - "block": 4904867, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "aaronkoenig", - "comment_permlink": "steemit-meetup-berlin-with-ned-scott-and-steemians-from-hamburg", - "curator": "camilla", - "reward": { - "amount": "1128365664", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T12:04:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 21 - } - ], - [ - 21982, - { - "block": 4905213, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "labradorsem", - "comment_permlink": "diet-apple-pie-with-apples-and-pears", - "curator": "camilla", - "reward": { - "amount": "61154024", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T12:21:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 21983, - { - "block": 4906606, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "terrycraft", - "comment_permlink": "traveling-to-peru-to-the-wild-jungle-through-the-city-of-shamans-iquitos-puteshestvie-v-ikitos-featuring-njall-as-author", - "curator": "camilla", - "reward": { - "amount": "61137899", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T13:31:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 31 - } - ], - [ - 21984, - { - "block": 4906955, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "opheliafu", - "comment_permlink": "the-blue-raven", - "curator": "camilla", - "reward": { - "amount": "314839300", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T13:48:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 21985, - { - "block": 4910096, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "kus-knee", - "comment_permlink": "the-old-dog-investigates-the-curious-case-of-the-swiss-bomb-shelters", - "curator": "camilla", - "reward": { - "amount": "64152384", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T16:26:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 17 - } - ], - [ - 21986, - { - "block": 4911002, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "### I made this drawing with my new Prismacolor pencils. I got them from the talented artist @Pixielols. I met her in real life today at the University and she is the sweetest and loveliest person you can imagine.\n\nhttps://i.imgsafe.org/6dc35d7e3c.jpg\n\n ### I had to test them right away so I just spent an hour on this autumn inspired apple drawing. These color pencils are very soft and it is a joy to work with them.\n\nhttps://i.imgsafe.org/6dd4fe4912.jpg\n\n### The apple have felt the warm sun on its red skin, drawn energy from the soil, nourished birds and children and heard the cold autumn wind in the leaves. It is a sweet gift from nature for you to enjoy.\n\nhttps://i.imgsafe.org/6ddd3dee6b.jpg\n\n### Thank you for visiting my post! I hope you liked it.", - "json_metadata": "{\"tags\":[\"art\",\"apple\",\"autumn\",\"colours\"],\"image\":[\"https://i.imgsafe.org/6dc35d7e3c.jpg\",\"https://i.imgsafe.org/6dd4fe4912.jpg\",\"https://i.imgsafe.org/6ddd3dee6b.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "apple-and-leaves", - "title": "Apple and Leaves" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:11:45", - "trx_id": "e9de33a2769e3c56a2eb04cbca3068802148eb2d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21987, - { - "block": 4911002, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "66", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "215909081377", - "total_vote_weight": "944711921076433591", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:11:45", - "trx_id": "e9de33a2769e3c56a2eb04cbca3068802148eb2d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21988, - { - "block": 4911002, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:11:45", - "trx_id": "e9de33a2769e3c56a2eb04cbca3068802148eb2d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21989, - { - "block": 4911009, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "66", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 51423955, - "total_vote_weight": "944925401186763676", - "voter": "sergey44", - "weight": "2490601287184" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:06", - "trx_id": "6f06ca0ecd432cec40db13747a60b7b707228119", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 21990, - { - "block": 4911009, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "sergey44", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:06", - "trx_id": "6f06ca0ecd432cec40db13747a60b7b707228119", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 21991, - { - "block": 4911009, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "306", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "651650151993", - "total_vote_weight": "3287976972277509707", - "voter": "clains", - "weight": "27335601662725370" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:06", - "trx_id": "14f3af6075b9fa0dc31befd2d38aacfff43af33c", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 21992, - { - "block": 4911009, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "clains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:06", - "trx_id": "14f3af6075b9fa0dc31befd2d38aacfff43af33c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 21993, - { - "block": 4911014, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "361", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "130765654564", - "total_vote_weight": "3684554976556033924", - "voter": "psylains", - "weight": "7931560085570484" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:21", - "trx_id": "37856cc1fe9064955103b31796156066b00633b6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21994, - { - "block": 4911014, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "psylains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:21", - "trx_id": "37856cc1fe9064955103b31796156066b00633b6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21995, - { - "block": 4911015, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "362", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 551289713, - "total_vote_weight": "3686182974326598443", - "voter": "jessicanicklos", - "weight": "35273285028897" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:24", - "trx_id": "a17a63e263c32e389106d40292d212fec02efab6", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 21996, - { - "block": 4911015, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "jessicanicklos", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:24", - "trx_id": "a17a63e263c32e389106d40292d212fec02efab6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21997, - { - "block": 4911017, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "364", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "5888378458", - "total_vote_weight": "3703549401054751072", - "voter": "bitspace", - "weight": "434160668203815" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:30", - "trx_id": "cafd3e437c1355d5543fe3ae64aea271461caea8", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 21998, - { - "block": 4911017, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "bitspace", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:30", - "trx_id": "cafd3e437c1355d5543fe3ae64aea271461caea8", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 21999, - { - "block": 4911022, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "13670562023", - "total_vote_weight": 0, - "voter": "goldmatters", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:45", - "trx_id": "4a256e361159a952b0598bb19ddc2b2410f71f83", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22000, - { - "block": 4911022, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "goldmatters", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:45", - "trx_id": "4a256e361159a952b0598bb19ddc2b2410f71f83", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22001, - { - "block": 4911023, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "370", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "13670562023", - "total_vote_weight": "3743710464654223262", - "voter": "goldmatters", - "weight": "1405637225981526" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:48", - "trx_id": "c89823b7ea1b94808b19778975da55d5336f6777", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22002, - { - "block": 4911023, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "goldmatters", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:12:48", - "trx_id": "c89823b7ea1b94808b19778975da55d5336f6777", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22003, - { - "block": 4911030, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "289482062341", - "total_vote_weight": "4545573627196467795", - "voter": "anonymous", - "weight": "37420280918638078" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:13:09", - "trx_id": "47da48cdcd8d98927598df0d7fdb0b3fbe470c79", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22004, - { - "block": 4911030, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "anonymous", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:13:09", - "trx_id": "47da48cdcd8d98927598df0d7fdb0b3fbe470c79", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22005, - { - "block": 4911031, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 706760886, - "total_vote_weight": "4547424334362593573", - "voter": "murh", - "weight": "89450846362745" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:13:12", - "trx_id": "1b0c8d4f24f9d1e3e88a213ed1c181318ad5388d", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22006, - { - "block": 4911031, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "murh", - "weight": 1509 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:13:12", - "trx_id": "1b0c8d4f24f9d1e3e88a213ed1c181318ad5388d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22007, - { - "block": 4911034, - "op": { - "type": "comment_operation", - "value": { - "author": "goldmatters", - "body": "Lovely@", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t171320575z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:13:21", - "trx_id": "e7b4df539b542e4bac5d18940131cd123e799e0e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22008, - { - "block": 4911070, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "504", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 321100442, - "total_vote_weight": "4548264997516278957", - "voter": "luisucv34", - "weight": "95275157417676" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:09", - "trx_id": "2ea9c341cb484092e5bc37faf3760ee0cf25b2de", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22009, - { - "block": 4911070, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "luisucv34", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:09", - "trx_id": "2ea9c341cb484092e5bc37faf3760ee0cf25b2de", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22010, - { - "block": 4911072, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "504", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 229890255, - "total_vote_weight": "4548866803633534287", - "voter": "sjamayee", - "weight": "70210713679788" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:15", - "trx_id": "4cff3a69c210b194b88a1c0067c75cd34ce4bd1b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22011, - { - "block": 4911072, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "sjamayee", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:15", - "trx_id": "4cff3a69c210b194b88a1c0067c75cd34ce4bd1b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22012, - { - "block": 4911077, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "504", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 1027866325, - "total_vote_weight": "4551556912136215489", - "voter": "ola1", - "weight": "336263562835150" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:30", - "trx_id": "c1792db11b1ea2eaaaff0c393b4bbba923dcfd94", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22013, - { - "block": 4911077, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ola1", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:15:30", - "trx_id": "c1792db11b1ea2eaaaff0c393b4bbba923dcfd94", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22014, - { - "block": 4911102, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 155364835, - "total_vote_weight": "4551963438869269926", - "voter": "insight2incite", - "weight": "67754455509072" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:16:45", - "trx_id": "9654c16e81d58b07cd1865e5789d6ff60a021bc0", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22015, - { - "block": 4911102, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "insight2incite", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:16:45", - "trx_id": "9654c16e81d58b07cd1865e5789d6ff60a021bc0", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22016, - { - "block": 4911115, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "502", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 143358574, - "total_vote_weight": "4552338528988548564", - "voter": "anomaly", - "weight": "70641972464143" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:17:24", - "trx_id": "bc36e5c2780f5c5a333444e9dbf74e58a9ad9cee", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22017, - { - "block": 4911115, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "anomaly", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:17:24", - "trx_id": "bc36e5c2780f5c5a333444e9dbf74e58a9ad9cee", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22018, - { - "block": 4911130, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 597059749, - "total_vote_weight": "4553900486432503298", - "voter": "ashwim", - "weight": "333217588043676" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:18:09", - "trx_id": "ebba9f5ce4ccb5c3df2ef7de56c7ebe154eb65b3", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22019, - { - "block": 4911130, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ashwim", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:18:09", - "trx_id": "ebba9f5ce4ccb5c3df2ef7de56c7ebe154eb65b3", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22020, - { - "block": 4911136, - "op": { - "type": "comment_operation", - "value": { - "author": "ashwim", - "body": "very nice artwork ... thanks for sharing... keep it up...", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t171828553z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:18:27", - "trx_id": "b87dc9ccfe43ab91670eed2fc43edd735af9c7e9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22021, - { - "block": 4911165, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 59551730, - "total_vote_weight": "4554056259399344170", - "voter": "techguru", - "weight": "42318322658436" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:19:54", - "trx_id": "8b0e8accd4f91ab07c05bbe43a8f55302857e67f", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22022, - { - "block": 4911165, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "techguru", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:19:54", - "trx_id": "8b0e8accd4f91ab07c05bbe43a8f55302857e67f", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22023, - { - "block": 4911201, - "op": { - "type": "comment_operation", - "value": { - "author": "acidyo", - "body": ">I got them from the talented artist @Pixielols. I met her in real life today at the University\n\nOh wow, the Steemit world really is still small! Hehe\n\nGreat drawing and really nice colors!\n\nHer post about the Copic markers made me want to order them, hehe! Currently sketching to fund them. :D", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t172139964z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:21:42", - "trx_id": "f2c7d9f355c307a93f0bab76ac957b1e93bf03cb", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 22024, - { - "block": 4911203, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "503", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 63850166, - "total_vote_weight": "4554223272157884585", - "voter": "aavkc", - "weight": "55949274111039" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:21:48", - "trx_id": "8374918c977f445d1f8fd7535f0022aad9c25649", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22025, - { - "block": 4911203, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "aavkc", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:21:48", - "trx_id": "8374918c977f445d1f8fd7535f0022aad9c25649", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22026, - { - "block": 4911205, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "29251115097", - "total_vote_weight": "4630315371958732953", - "voter": "acidyo", - "weight": "25744493765953697" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:21:54", - "trx_id": "fa56c82f2877c591dde364cbf83c16bab3d16b09", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22027, - { - "block": 4911205, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "acidyo", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:21:54", - "trx_id": "fa56c82f2877c591dde364cbf83c16bab3d16b09", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22028, - { - "block": 4911208, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 849659123, - "total_vote_weight": "4632513168685677847", - "voter": "coar", - "weight": "754576876251080" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:03", - "trx_id": "551be3eaff76b21d0c34165e38f802a67d2b8e79", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22029, - { - "block": 4911208, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "coar", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:03", - "trx_id": "551be3eaff76b21d0c34165e38f802a67d2b8e79", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22030, - { - "block": 4911209, - "op": { - "type": "comment_operation", - "value": { - "author": "aavkc", - "body": "good job", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t172202293z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:06", - "trx_id": "b64cb1a44ca79d22fef07442cca8cc3f35bf5748", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22031, - { - "block": 4911212, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 58273844, - "total_vote_weight": "4632663878882512400", - "voter": "hotgirlktm", - "weight": "52748568892093" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:15", - "trx_id": "9600fef571caa241ed298e925b0da75201ef3843", - "trx_in_block": 9, - "virtual_op": 1 - } - ], - [ - 22032, - { - "block": 4911212, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "hotgirlktm", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:15", - "trx_id": "9600fef571caa241ed298e925b0da75201ef3843", - "trx_in_block": 9, - "virtual_op": 0 - } - ], - [ - 22033, - { - "block": 4911213, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 117630527, - "total_vote_weight": "4632968089740256431", - "voter": "pokemon", - "weight": "106980818306650" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:18", - "trx_id": "6241f92f3bcd7b554bdaa4b7c768d43bf7e227af", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 22034, - { - "block": 4911213, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "pokemon", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:18", - "trx_id": "6241f92f3bcd7b554bdaa4b7c768d43bf7e227af", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 22035, - { - "block": 4911213, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 244245816, - "total_vote_weight": "4633599704657956435", - "voter": "cryptochannel", - "weight": "222117912724501" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:18", - "trx_id": "53f88c0a2f43c9f53d14197a91ce6d2b42fba05a", - "trx_in_block": 9, - "virtual_op": 1 - } - ], - [ - 22036, - { - "block": 4911213, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "cryptochannel", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:18", - "trx_id": "53f88c0a2f43c9f53d14197a91ce6d2b42fba05a", - "trx_in_block": 9, - "virtual_op": 0 - } - ], - [ - 22037, - { - "block": 4911214, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 246459444, - "total_vote_weight": "4634236985430254670", - "voter": "strawhat", - "weight": "225172539545376" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:21", - "trx_id": "34202ef335284894eee6a433a48d2dfd4cd66299", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22038, - { - "block": 4911214, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "strawhat", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:22:21", - "trx_id": "34202ef335284894eee6a433a48d2dfd4cd66299", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22039, - { - "block": 4911275, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 1541722449, - "total_vote_weight": "4638222149353602136", - "voter": "gidlark", - "weight": "1813249585123097" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:24", - "trx_id": "e4db024ecb0b303a45a7a47214f675039c2fd515", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22040, - { - "block": 4911275, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "gidlark", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:24", - "trx_id": "e4db024ecb0b303a45a7a47214f675039c2fd515", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22041, - { - "block": 4911281, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 56509947, - "total_vote_weight": "4638368176964869032", - "voter": "alex.gaud", - "weight": "67902839239106" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:42", - "trx_id": "adb97a82965257019bf7841e6269331437ba9167", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22042, - { - "block": 4911281, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "alex.gaud", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:42", - "trx_id": "adb97a82965257019bf7841e6269331437ba9167", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22043, - { - "block": 4911283, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 1322723922, - "total_vote_weight": "4641785351864380218", - "voter": "elishagh1", - "weight": "1600376911271072" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:48", - "trx_id": "2fb8c4197a9606ec94ba93d7433d19c655d57142", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22044, - { - "block": 4911283, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "elishagh1", - "weight": 400 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:25:48", - "trx_id": "2fb8c4197a9606ec94ba93d7433d19c655d57142", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22045, - { - "block": 4911304, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "Yes there are not so many Steemers here in Norway yet, I think I know them all :D\n\nThank you for the compliment on my art.\n\nI agree I also want to buy the Copic markers, they look so awsome! Haha yes they are pretty expensive but I think it might be worth it.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "acidyo", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t172139964z", - "permlink": "re-acidyo-re-camilla-apple-and-leaves-20160912t172655245z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:26:54", - "trx_id": "6a1e5517b38b9e6bb16719bc644b57d4000d15aa", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 22046, - { - "block": 4911312, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thank you :D I will", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "ashwim", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t171828553z", - "permlink": "re-ashwim-re-camilla-apple-and-leaves-20160912t172717002z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:18", - "trx_id": "917f500de52ba30caef4e80c4ab444d58edff69c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22047, - { - "block": 4911316, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "goldmatters", - "pending_payout": { - "amount": "63", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t171320575z", - "rshares": "211675569978", - "total_vote_weight": "927617181348547604", - "voter": "camilla", - "weight": "437265678791358974" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:30", - "trx_id": "bceb1643a37cd96bff304630e31f5e3c2912f60b", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22048, - { - "block": 4911316, - "op": { - "type": "vote_operation", - "value": { - "author": "goldmatters", - "permlink": "re-camilla-apple-and-leaves-20160912t171320575z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:30", - "trx_id": "bceb1643a37cd96bff304630e31f5e3c2912f60b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22049, - { - "block": 4911319, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thanks!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "goldmatters", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t171320575z", - "permlink": "re-goldmatters-re-camilla-apple-and-leaves-20160912t172738146z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:39", - "trx_id": "45529c0456c9bb93d6c7e0ee8f022c7fe5581b86", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22050, - { - "block": 4911322, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "aavkc", - "pending_payout": { - "amount": "63", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t172202293z", - "rshares": "211675569978", - "total_vote_weight": "927119147988210213", - "voter": "camilla", - "weight": "176152638117759940" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:48", - "trx_id": "d9e47525fa85ae9b91170cc4cb3accbec9063d1a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22051, - { - "block": 4911322, - "op": { - "type": "vote_operation", - "value": { - "author": "aavkc", - "permlink": "re-camilla-apple-and-leaves-20160912t172202293z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:48", - "trx_id": "d9e47525fa85ae9b91170cc4cb3accbec9063d1a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22052, - { - "block": 4911324, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "ashwim", - "pending_payout": { - "amount": "64", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t171828553z", - "rshares": "211675569978", - "total_vote_weight": "930394407468503154", - "voter": "camilla", - "weight": "291930460175032994" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:54", - "trx_id": "a4c1fb0430ec2b321c84cae41a1d6fb2f76fdfec", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22053, - { - "block": 4911324, - "op": { - "type": "vote_operation", - "value": { - "author": "ashwim", - "permlink": "re-camilla-apple-and-leaves-20160912t171828553z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:27:54", - "trx_id": "a4c1fb0430ec2b321c84cae41a1d6fb2f76fdfec", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22054, - { - "block": 4911343, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "kalipo", - "pending_payout": { - "amount": "121552", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "raptor-art-drawing-all-day", - "rshares": "211675569978", - "total_vote_weight": "16739375280083409273", - "voter": "camilla", - "weight": "8403846313740628" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:28:51", - "trx_id": "447d5d37f569ab88e343458f61fe580fd418a16d", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22055, - { - "block": 4911343, - "op": { - "type": "vote_operation", - "value": { - "author": "kalipo", - "permlink": "raptor-art-drawing-all-day", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:28:51", - "trx_id": "447d5d37f569ab88e343458f61fe580fd418a16d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22056, - { - "block": 4911347, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "alex2016", - "pending_payout": { - "amount": "20722", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-new-works-from-genuine-leather", - "rshares": "207442058578", - "total_vote_weight": "14586536023317668623", - "voter": "camilla", - "weight": "42352343323010257" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:29:03", - "trx_id": "60d684ff1b8695f0a27d5131e9c964ea6f5c0251", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 22057, - { - "block": 4911347, - "op": { - "type": "vote_operation", - "value": { - "author": "alex2016", - "permlink": "my-new-works-from-genuine-leather", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:29:03", - "trx_id": "60d684ff1b8695f0a27d5131e9c964ea6f5c0251", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 22058, - { - "block": 4911373, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "### I made this drawing with my new Prismacolor pencils. I got them from the talented artist @Pixielols. I met her in real life today at the University and she is the sweetest and loveliest person you can imagine.\n\nhttps://i.imgsafe.org/6dc35d7e3c.jpg\n\n ### I had to test them right away so I just spent an hour on this autumn inspired apple drawing. These color pencils are very soft and it is a joy to work with them.\n\nhttps://i.imgsafe.org/6dd4fe4912.jpg\n\n### The apple have felt the warm sun on its red skin, drawn energy from the soil, nourished birds and children and heard the cold autumn wind in the leaves. It is a sweet gift from nature for you to enjoy.\n\nhttps://i.imgsafe.org/6ddd3dee6b.jpg\n\n### Thank you for visiting my post! I hope you liked it.", - "json_metadata": "{\"tags\":[\"art\",\"apple\",\"autumn\",\"colours\"],\"image\":[\"https://i.imgsafe.org/6dc35d7e3c.jpg\",\"https://i.imgsafe.org/6dd4fe4912.jpg\",\"https://i.imgsafe.org/6ddd3dee6b.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "apple-and-leaves", - "title": "Apple Art" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:21", - "trx_id": "178037dd18b61c4c3dc005c1aa1466e964f0374c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22059, - { - "block": 4911383, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "739", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "436172432967", - "total_vote_weight": "5683335719784144663", - "voter": "boatymcboatface", - "weight": "663120400908916696" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:51", - "trx_id": "69c2b13c13f94e2002b6c06202b8a130daf7377c", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 22060, - { - "block": 4911383, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "boatymcboatface", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:51", - "trx_id": "69c2b13c13f94e2002b6c06202b8a130daf7377c", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 22061, - { - "block": 4911383, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "773", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "63365513478", - "total_vote_weight": "5821715387235604396", - "voter": "theshell", - "weight": "88101721610762696" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:51", - "trx_id": "6bd6c348115631a86f4acc661c256741b165f470", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 22062, - { - "block": 4911383, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "theshell", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:51", - "trx_id": "6bd6c348115631a86f4acc661c256741b165f470", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 22063, - { - "block": 4911384, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1505", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "1152457041840", - "total_vote_weight": "7901162747347395947", - "voter": "rossco99", - "weight": "1327380564871360273" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:54", - "trx_id": "bc737880167153d8be286de2eafaeccc37377d01", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22064, - { - "block": 4911384, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "rossco99", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:54", - "trx_id": "bc737880167153d8be286de2eafaeccc37377d01", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22065, - { - "block": 4911384, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1507", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 3505969711, - "total_vote_weight": "7906444182342513581", - "voter": "glitterpig", - "weight": "3371316005216756" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:54", - "trx_id": "049b66a72a1520486541f37735fe7b27dbf76753", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22066, - { - "block": 4911384, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "glitterpig", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:30:54", - "trx_id": "049b66a72a1520486541f37735fe7b27dbf76753", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22067, - { - "block": 4911402, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "acidyo", - "pending_payout": { - "amount": "5710", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "sketch-kitty-cat-oc", - "rshares": "207442058578", - "total_vote_weight": "11822623121092878973", - "voter": "camilla", - "weight": "125700686784290108" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:31:51", - "trx_id": "82fd894d61ced60cffa90d17f1dff0bd223d9383", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22068, - { - "block": 4911402, - "op": { - "type": "vote_operation", - "value": { - "author": "acidyo", - "permlink": "sketch-kitty-cat-oc", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:31:51", - "trx_id": "82fd894d61ced60cffa90d17f1dff0bd223d9383", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22069, - { - "block": 4911408, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "awww it is sooo cute! I love it <3", - "json_metadata": "{\"tags\":[\"drawing\"]}", - "parent_author": "acidyo", - "parent_permlink": "sketch-kitty-cat-oc", - "permlink": "re-acidyo-sketch-kitty-cat-oc-20160912t173207452z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:32:09", - "trx_id": "22ece8e946f60946e713ce988b0781477ce0b849", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22070, - { - "block": 4911427, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-acidyo-re-camilla-apple-and-leaves-20160912t172655245z", - "rshares": 609398231, - "total_vote_weight": "2809925210693923", - "voter": "acidyo", - "weight": "580717876876744" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:06", - "trx_id": "d1a36bafc96db1a8af411c942ff71cdeeffbce74", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22071, - { - "block": 4911427, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-acidyo-re-camilla-apple-and-leaves-20160912t172655245z", - "voter": "acidyo", - "weight": 100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:06", - "trx_id": "d1a36bafc96db1a8af411c942ff71cdeeffbce74", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22072, - { - "block": 4911434, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1511", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "6349496599", - "total_vote_weight": "7915995686847249607", - "voter": "orcish", - "weight": "6908921591759058" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:27", - "trx_id": "2a6317cee4fb6fa90f4040c2ed0d2670169f8647", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22073, - { - "block": 4911434, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "orcish", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:27", - "trx_id": "2a6317cee4fb6fa90f4040c2ed0d2670169f8647", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22074, - { - "block": 4911444, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-acidyo-sketch-kitty-cat-oc-20160912t173207452z", - "rshares": 609398231, - "total_vote_weight": "2809925210693923", - "voter": "acidyo", - "weight": "168595512641635" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:57", - "trx_id": "7c5f243ade91f1d0b448d0e7072764dcecab07b5", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22075, - { - "block": 4911444, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "re-acidyo-sketch-kitty-cat-oc-20160912t173207452z", - "voter": "acidyo", - "weight": 100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:33:57", - "trx_id": "7c5f243ade91f1d0b448d0e7072764dcecab07b5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22076, - { - "block": 4911449, - "op": { - "type": "comment_operation", - "value": { - "author": "acidyo", - "body": "Thanks :3", - "json_metadata": "{\"tags\":[\"drawing\"]}", - "parent_author": "camilla", - "parent_permlink": "re-acidyo-sketch-kitty-cat-oc-20160912t173207452z", - "permlink": "re-camilla-re-acidyo-sketch-kitty-cat-oc-20160912t173408484z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:34:12", - "trx_id": "5c0383ed515eb551803d219e47635b19a46fa6ef", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22077, - { - "block": 4911465, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "anwenbaumeister", - "pending_payout": { - "amount": "33217", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "what-is-culture-a-look-into-various-explanations-of-culture-and-forming-my-own-definition", - "rshares": "207442058578", - "total_vote_weight": "15320294595211071057", - "voter": "camilla", - "weight": "27723884101111043" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:35:00", - "trx_id": "202fecdca5542351d9f72ce4917a3e7351f79a39", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22078, - { - "block": 4911465, - "op": { - "type": "vote_operation", - "value": { - "author": "anwenbaumeister", - "permlink": "what-is-culture-a-look-into-various-explanations-of-culture-and-forming-my-own-definition", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:35:00", - "trx_id": "202fecdca5542351d9f72ce4917a3e7351f79a39", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22079, - { - "block": 4911512, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -236,16 +236,16 @@\n g/6d\n-c35d7e3c\n+d4fe4912\n .jpg\n@@ -435,32 +435,32 @@\n gsafe.org/6d\n-d4fe4912\n+c35d7e3c\n .jpg%0A%0A### Th\n", - "json_metadata": "{\"tags\":[\"art\",\"apple\",\"autumn\",\"colours\"],\"image\":[\"https://i.imgsafe.org/6dd4fe4912.jpg\",\"https://i.imgsafe.org/6dc35d7e3c.jpg\",\"https://i.imgsafe.org/6ddd3dee6b.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "apple-and-leaves", - "title": "Apple Art" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:37:21", - "trx_id": "67762af9744c9763dd9858225ae9ae31ff935075", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22080, - { - "block": 4911521, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1529", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "16221469512", - "total_vote_weight": "7940319109157654746", - "voter": "jademont", - "weight": "21120838372868462" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:37:48", - "trx_id": "163f7f6ce3c69130b85a114b1db6d3d10edd45ca", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22081, - { - "block": 4911521, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "jademont", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:37:48", - "trx_id": "163f7f6ce3c69130b85a114b1db6d3d10edd45ca", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22082, - { - "block": 4911525, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1536", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "9220777116", - "total_vote_weight": "7954095260687687295", - "voter": "r4fken", - "weight": "12054132588778480" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:38:00", - "trx_id": "f76dc0148ab3b1a35fded252b6f9991c827d1939", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22083, - { - "block": 4911525, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "r4fken", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:38:00", - "trx_id": "f76dc0148ab3b1a35fded252b6f9991c827d1939", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22084, - { - "block": 4911534, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -387,11 +387,8 @@\n and \n-it \n is a\n@@ -404,21 +404,16 @@\n ork with\n- them\n .%0A%0Ahttps\n", - "json_metadata": "{\"tags\":[\"art\",\"apple\",\"autumn\",\"colours\"],\"image\":[\"https://i.imgsafe.org/6dd4fe4912.jpg\",\"https://i.imgsafe.org/6dc35d7e3c.jpg\",\"https://i.imgsafe.org/6ddd3dee6b.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "apple-and-leaves", - "title": "Apple Art" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:38:27", - "trx_id": "64b921869a1707f6137d54ddc8908282833a123d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22085, - { - "block": 4911579, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "fajrilgooner", - "pending_payout": { - "amount": "124071", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "efficacy-of-turmeric-and-honey", - "rshares": "207442058578", - "total_vote_weight": "16751744895383774296", - "voter": "camilla", - "weight": "8115785431724820" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:42", - "trx_id": "9f57266b2cf5ddd5350dc050e1ddb20065ab766c", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22086, - { - "block": 4911579, - "op": { - "type": "vote_operation", - "value": { - "author": "fajrilgooner", - "permlink": "efficacy-of-turmeric-and-honey", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:42", - "trx_id": "9f57266b2cf5ddd5350dc050e1ddb20065ab766c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22087, - { - "block": 4911582, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 56157882, - "total_vote_weight": "7954179051772419968", - "voter": "jarvis", - "weight": "81277352190692" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:51", - "trx_id": "674d2d9e9f5321980fe04ae0686205e1446ffa08", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22088, - { - "block": 4911582, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "jarvis", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:51", - "trx_id": "674d2d9e9f5321980fe04ae0686205e1446ffa08", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22089, - { - "block": 4911583, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 53039566, - "total_vote_weight": "7954258188905109201", - "voter": "thomasaustin", - "weight": "76894913929704" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:54", - "trx_id": "7a6e7d6b947b38163120e5df9ab8bdc297586ce3", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22090, - { - "block": 4911583, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "thomasaustin", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:54", - "trx_id": "7a6e7d6b947b38163120e5df9ab8bdc297586ce3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22091, - { - "block": 4911584, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 57196572, - "total_vote_weight": "7954343527117623005", - "voter": "ciao", - "weight": "83062526846769" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:57", - "trx_id": "d227a86a4fc0f3839440f1df179e97f35be9edb9", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22092, - { - "block": 4911584, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ciao", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:40:57", - "trx_id": "d227a86a4fc0f3839440f1df179e97f35be9edb9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22093, - { - "block": 4911587, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50441122, - "total_vote_weight": "7954418784937339049", - "voter": "eavy", - "weight": "73627233622196" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:06", - "trx_id": "157e45a06fbcd158a39c2282ac969fc4e01c4f65", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22094, - { - "block": 4911587, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "eavy", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:06", - "trx_id": "157e45a06fbcd158a39c2282ac969fc4e01c4f65", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22095, - { - "block": 4911589, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 53056158, - "total_vote_weight": "7954497943208450318", - "voter": "johnbyrd", - "weight": "77707036140895" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:12", - "trx_id": "f364eccb18ae414bb353e5c3d98e14155024e78c", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22096, - { - "block": 4911589, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "johnbyrd", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:12", - "trx_id": "f364eccb18ae414bb353e5c3d98e14155024e78c", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22097, - { - "block": 4911600, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1538", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 54313084, - "total_vote_weight": "7954578975540051524", - "voter": "fortuner", - "weight": "81032331601206" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:45", - "trx_id": "a746a03aa3ba97b18b537e3f082b54efa209280a", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22098, - { - "block": 4911600, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "fortuner", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:41:45", - "trx_id": "a746a03aa3ba97b18b537e3f082b54efa209280a", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22099, - { - "block": 4911614, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1536", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 148091845, - "total_vote_weight": "7954799914607325206", - "voter": "buffett", - "weight": "220939067273682" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:27", - "trx_id": "f53f8fd5f8e4f8abfbaf5e8e3959bc4f9d678a2f", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22100, - { - "block": 4911614, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "buffett", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:27", - "trx_id": "f53f8fd5f8e4f8abfbaf5e8e3959bc4f9d678a2f", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22101, - { - "block": 4911616, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1539", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 4263594327, - "total_vote_weight": "7961156807460496684", - "voter": "fubar-bdhr", - "weight": "6356892853171478" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:33", - "trx_id": "85f0d8a90f3b37cc618d6e8d5768646a0d51a214", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22102, - { - "block": 4911616, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "fubar-bdhr", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:33", - "trx_id": "85f0d8a90f3b37cc618d6e8d5768646a0d51a214", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22103, - { - "block": 4911617, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1539", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50271139, - "total_vote_weight": "7961231714291952756", - "voter": "hitherise", - "weight": "74906831456072" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:36", - "trx_id": "dbf7a9e9f785a327a2d7c9b5f14565885123c355", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22104, - { - "block": 4911617, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "hitherise", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:36", - "trx_id": "dbf7a9e9f785a327a2d7c9b5f14565885123c355", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22105, - { - "block": 4911619, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 53030738, - "total_vote_weight": "7961310731921542285", - "voter": "widell", - "weight": "79017629589529" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:42", - "trx_id": "42870856dd77f9b73ebd60e968682db311bc7b30", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22106, - { - "block": 4911619, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "widell", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:42", - "trx_id": "42870856dd77f9b73ebd60e968682db311bc7b30", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22107, - { - "block": 4911622, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 60342922, - "total_vote_weight": "7961400643509054273", - "voter": "lillianjones", - "weight": "89911587511988" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:51", - "trx_id": "78fdd04f6b0cee4ccb3254b2c9741f6c93292eb1", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22108, - { - "block": 4911622, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "lillianjones", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:51", - "trx_id": "78fdd04f6b0cee4ccb3254b2c9741f6c93292eb1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22109, - { - "block": 4911623, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 55306941, - "total_vote_weight": "7961483050077691417", - "voter": "steema", - "weight": "82406568637144" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:54", - "trx_id": "d9f704e5dea33a4e232d69c6115fc015de978fc4", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22110, - { - "block": 4911623, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "steema", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:54", - "trx_id": "d9f704e5dea33a4e232d69c6115fc015de978fc4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22111, - { - "block": 4911623, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 72812589, - "total_vote_weight": "7961591537841891468", - "voter": "igtes", - "weight": "108487764200051" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:54", - "trx_id": "30c9df603c5d62c5153e8a1461517a95cf239713", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22112, - { - "block": 4911623, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "igtes", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:42:54", - "trx_id": "30c9df603c5d62c5153e8a1461517a95cf239713", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22113, - { - "block": 4911631, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50370757, - "total_vote_weight": "7961666586885319766", - "voter": "yorsens", - "weight": "75049043428298" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:18", - "trx_id": "1414e564a4a0cd382666fd2fe5ba971e21b5a714", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22114, - { - "block": 4911631, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "yorsens", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:18", - "trx_id": "1414e564a4a0cd382666fd2fe5ba971e21b5a714", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22115, - { - "block": 4911633, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50588612, - "total_vote_weight": "7961741959436738384", - "voter": "troich", - "weight": "75372551418618" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:24", - "trx_id": "2f90f3f307b8518dfc538c01d2790c8261d76b35", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 22116, - { - "block": 4911633, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "troich", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:24", - "trx_id": "2f90f3f307b8518dfc538c01d2790c8261d76b35", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 22117, - { - "block": 4911634, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 51562255, - "total_vote_weight": "7961818781514878772", - "voter": "curpose", - "weight": "76822078140388" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:27", - "trx_id": "a0d14f813e5f4de4a52d7bb855c6d4eab296bada", - "trx_in_block": 7, - "virtual_op": 1 - } - ], - [ - 22118, - { - "block": 4911634, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "curpose", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:27", - "trx_id": "a0d14f813e5f4de4a52d7bb855c6d4eab296bada", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 22119, - { - "block": 4911635, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 53048942, - "total_vote_weight": "7961897817417952081", - "voter": "ficholl", - "weight": "79035903073309" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:30", - "trx_id": "09b44c1a2e8f3b5837565fffd8a602d4e5a78754", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22120, - { - "block": 4911635, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ficholl", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:30", - "trx_id": "09b44c1a2e8f3b5837565fffd8a602d4e5a78754", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22121, - { - "block": 4911641, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1540", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50059754, - "total_vote_weight": "7961972398733769868", - "voter": "vive", - "weight": "74581315817787" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:48", - "trx_id": "051120398c87df25af71eff1e3057ca7619bde83", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22122, - { - "block": 4911641, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "vive", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:48", - "trx_id": "051120398c87df25af71eff1e3057ca7619bde83", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22123, - { - "block": 4911642, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50710305, - "total_vote_weight": "7962047948188487545", - "voter": "prof", - "weight": "75549454717677" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:51", - "trx_id": "15162fc3381cca34e7493cb22954ba208b727f6b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22124, - { - "block": 4911642, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "prof", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:51", - "trx_id": "15162fc3381cca34e7493cb22954ba208b727f6b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22125, - { - "block": 4911643, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 71268878, - "total_vote_weight": "7962154124468599372", - "voter": "confucius", - "weight": "106176280111827" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:54", - "trx_id": "fc806b1287f4c0723a2c598f1188f581c14a0585", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22126, - { - "block": 4911643, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "confucius", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:43:54", - "trx_id": "fc806b1287f4c0723a2c598f1188f581c14a0585", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22127, - { - "block": 4911646, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 149597919, - "total_vote_weight": "7962376988255986211", - "voter": "steemster1", - "weight": "222863787386839" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:03", - "trx_id": "4db90a5179b242f330942a9b3b7bad06daef72ea", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22128, - { - "block": 4911646, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "steemster1", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:03", - "trx_id": "4db90a5179b242f330942a9b3b7bad06daef72ea", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22129, - { - "block": 4911654, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50054445, - "total_vote_weight": "7962451554846249289", - "voter": "coad", - "weight": "74566590263078" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:27", - "trx_id": "ad175c2578195003cd0a27d52a521f5e685a50ff", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22130, - { - "block": 4911654, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "coad", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:27", - "trx_id": "ad175c2578195003cd0a27d52a521f5e685a50ff", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22131, - { - "block": 4911656, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 59219358, - "total_vote_weight": "7962539773126208430", - "voter": "sharon", - "weight": "88218279959141" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:33", - "trx_id": "dd16a3de7b55bc1b29fd83417e36271e4b9f4e90", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22132, - { - "block": 4911656, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "sharon", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:44:33", - "trx_id": "dd16a3de7b55bc1b29fd83417e36271e4b9f4e90", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22133, - { - "block": 4911666, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1542", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50454901, - "total_vote_weight": "7962614933941361087", - "voter": "roto", - "weight": "75160815152657" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:03", - "trx_id": "f6e3d95673f04c95d72af93ee08340592e120dde", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22134, - { - "block": 4911666, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "roto", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:03", - "trx_id": "f6e3d95673f04c95d72af93ee08340592e120dde", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22135, - { - "block": 4911670, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "thank you :) it was very fun to draw", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "aavkc", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t172202293z", - "permlink": "re-aavkc-re-camilla-apple-and-leaves-20160912t174516163z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:15", - "trx_id": "5d285c8a57d57fc920b7683b2e901f9ef1c77a6d", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22136, - { - "block": 4911671, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1542", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 60806273, - "total_vote_weight": "7962705513383702711", - "voter": "msjennifer", - "weight": "90579442341624" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:18", - "trx_id": "c4d32c0eb6cbc91a6a1475d355ee9391d54b63a2", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 22137, - { - "block": 4911671, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "msjennifer", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:18", - "trx_id": "c4d32c0eb6cbc91a6a1475d355ee9391d54b63a2", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 22138, - { - "block": 4911675, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1542", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50065875, - "total_vote_weight": "7962780092327196405", - "voter": "bane", - "weight": "74578943493694" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:30", - "trx_id": "1593a38695fb9e10dbc931743a20e0e227fd67be", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22139, - { - "block": 4911675, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "bane", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:30", - "trx_id": "1593a38695fb9e10dbc931743a20e0e227fd67be", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22140, - { - "block": 4911677, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1538", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50262756, - "total_vote_weight": "7962854963480532525", - "voter": "wiss", - "weight": "74871153336120" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:36", - "trx_id": "c577ccf193e1c5dc8277d79bdfd23438000f25e8", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22141, - { - "block": 4911677, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "wiss", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:36", - "trx_id": "c577ccf193e1c5dc8277d79bdfd23438000f25e8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22142, - { - "block": 4911678, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1537", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50712038, - "total_vote_weight": "7962930502798362229", - "voter": "thadm", - "weight": "75539317829704" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:39", - "trx_id": "859e7d25e4d311c6e9a98bfd3df95ffb10f5df4b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22143, - { - "block": 4911678, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "thadm", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:45:39", - "trx_id": "859e7d25e4d311c6e9a98bfd3df95ffb10f5df4b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22144, - { - "block": 4911688, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1538", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 53037689, - "total_vote_weight": "7963009505180164108", - "voter": "thermor", - "weight": "79002381801879" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:09", - "trx_id": "8122a81202cb7157b6b58f2e4d0b143c05cea072", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22145, - { - "block": 4911688, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "thermor", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:09", - "trx_id": "8122a81202cb7157b6b58f2e4d0b143c05cea072", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22146, - { - "block": 4911693, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1538", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 51033798, - "total_vote_weight": "7963085521538613938", - "voter": "stroully", - "weight": "76016358449830" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:24", - "trx_id": "65d8174d8c442b3aacb1ad72bfd5aac6100d3cd8", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22147, - { - "block": 4911693, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "stroully", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:24", - "trx_id": "65d8174d8c442b3aacb1ad72bfd5aac6100d3cd8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22148, - { - "block": 4911694, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "acidyo", - "pending_payout": { - "amount": "61", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-acidyo-sketch-kitty-cat-oc-20160912t173408484z", - "rshares": "203208547179", - "total_vote_weight": "891827284162997537", - "voter": "camilla", - "weight": "364162807699890660" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:27", - "trx_id": "7ea2e1fdb2e9d3f951c34425b12606185392f20d", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22149, - { - "block": 4911694, - "op": { - "type": "vote_operation", - "value": { - "author": "acidyo", - "permlink": "re-camilla-re-acidyo-sketch-kitty-cat-oc-20160912t173408484z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:27", - "trx_id": "7ea2e1fdb2e9d3f951c34425b12606185392f20d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22150, - { - "block": 4911696, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "acidyo", - "pending_payout": { - "amount": "63", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t172139964z", - "rshares": "203208547179", - "total_vote_weight": "915905112022831395", - "voter": "camilla", - "weight": "736653884490449334" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:33", - "trx_id": "7c215d409dc2b333298ff13ce1dad06c53fd4b2b", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22151, - { - "block": 4911696, - "op": { - "type": "vote_operation", - "value": { - "author": "acidyo", - "permlink": "re-camilla-apple-and-leaves-20160912t172139964z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:33", - "trx_id": "7c215d409dc2b333298ff13ce1dad06c53fd4b2b", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22152, - { - "block": 4911701, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1538", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 50593879, - "total_vote_weight": "7963160881536491251", - "voter": "crion", - "weight": "75359997877313" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:48", - "trx_id": "ac6dbba5351c6f87f2262183a2f91a624970f8be", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22153, - { - "block": 4911701, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "crion", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:46:48", - "trx_id": "ac6dbba5351c6f87f2262183a2f91a624970f8be", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22154, - { - "block": 4911715, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1539", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 52651308, - "total_vote_weight": "7963239304941345177", - "voter": "revelbrooks", - "weight": "78423404853926" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:47:30", - "trx_id": "c31aae44e8233ebcbd28a67cb293b7c3d532f416", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22155, - { - "block": 4911715, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "revelbrooks", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:47:30", - "trx_id": "c31aae44e8233ebcbd28a67cb293b7c3d532f416", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22156, - { - "block": 4911717, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1539", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 55449983, - "total_vote_weight": "7963321895665919466", - "voter": "steemo", - "weight": "82590724574289" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:47:36", - "trx_id": "36c3a544d88b42686c062e5263e827661013dc93", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22157, - { - "block": 4911717, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "steemo", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:47:36", - "trx_id": "36c3a544d88b42686c062e5263e827661013dc93", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22158, - { - "block": 4911761, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1541", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 1165238399, - "total_vote_weight": "7965057174750676078", - "voter": "bullionstackers", - "weight": "1735279084756612" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:49:48", - "trx_id": "b565c0f8117c2912ea26f544828aff905b070aae", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22159, - { - "block": 4911761, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "bullionstackers", - "weight": 3400 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:49:48", - "trx_id": "b565c0f8117c2912ea26f544828aff905b070aae", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22160, - { - "block": 4911819, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1543", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 2308410567, - "total_vote_weight": "7968493176021161572", - "voter": "the-future", - "weight": "3436001270485494" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:52:48", - "trx_id": "0d9a12310ee72c6cf893354391bd05fda1ba73a9", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22161, - { - "block": 4911819, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "the-future", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:52:48", - "trx_id": "0d9a12310ee72c6cf893354391bd05fda1ba73a9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22162, - { - "block": 4911840, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1546", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "5091917697", - "total_vote_weight": "7976064386660099763", - "voter": "ben99", - "weight": "7571210638938191" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:53:51", - "trx_id": "8b6b819e7e536306eac0e296cd5bd614b0ecd120", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22163, - { - "block": 4911840, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ben99", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T17:53:51", - "trx_id": "8b6b819e7e536306eac0e296cd5bd614b0ecd120", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22164, - { - "block": 4912206, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1565", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "26148166391", - "total_vote_weight": "8014772570251297440", - "voter": "pixielolz", - "weight": "38708183591197677" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:12:09", - "trx_id": "d596699aea8cd68ba033c1d7a12513dd9e61de09", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22165, - { - "block": 4912206, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "pixielolz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:12:09", - "trx_id": "d596699aea8cd68ba033c1d7a12513dd9e61de09", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22166, - { - "block": 4912541, - "op": { - "type": "comment_operation", - "value": { - "author": "pixielolz", - "body": "That turned out pretty! Glad you like them ^_^ \n\nAnd thank you, and same to you :) I normally get super uncomfortable around new people, but not this time. ^_^", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t182902111z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:29:00", - "trx_id": "439e2674d1c8ac0b8e3d3c9e8f3d9ac96d8388f9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22167, - { - "block": 4912631, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pixielolz", - "pending_payout": { - "amount": "61", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t182902111z", - "rshares": "203208547179", - "total_vote_weight": "891827284162997537", - "voter": "camilla", - "weight": "133774092624449630" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:33:30", - "trx_id": "34e36fccfaef2aec6a9d60daacc4c4e2c720f9c9", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22168, - { - "block": 4912631, - "op": { - "type": "vote_operation", - "value": { - "author": "pixielolz", - "permlink": "re-camilla-apple-and-leaves-20160912t182902111z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:33:30", - "trx_id": "34e36fccfaef2aec6a9d60daacc4c4e2c720f9c9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22169, - { - "block": 4912642, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "Thank you! I agree, it was so nice talking to you ^^", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "pixielolz", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t182902111z", - "permlink": "re-pixielolz-re-camilla-apple-and-leaves-20160912t183403150z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:34:03", - "trx_id": "4f27121b1b82683f0af6388d861618c38fa74fc9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22170, - { - "block": 4912716, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "wow! beautiful. Where is this?", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "natord", - "parent_permlink": "re-camilla-there-is-a-land-far-far-north-20160912t065441930z", - "permlink": "re-natord-re-camilla-there-is-a-land-far-far-north-20160912t183743684z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:37:45", - "trx_id": "6be4d6509f58bc3566d9451175468d92f8691096", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22171, - { - "block": 4912719, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "natord", - "pending_payout": { - "amount": "61", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160912t065441930z", - "rshares": "203208547179", - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:37:54", - "trx_id": "1b58eeb64fae78266053d560c8d82fae1fe08f86", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22172, - { - "block": 4912719, - "op": { - "type": "vote_operation", - "value": { - "author": "natord", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160912t065441930z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:37:54", - "trx_id": "1b58eeb64fae78266053d560c8d82fae1fe08f86", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22173, - { - "block": 4912746, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1566", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "4487075430", - "total_vote_weight": "8021386217386554492", - "voter": "alexft", - "weight": "6613647135257052" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:39:18", - "trx_id": "7deff2e5e96a0f015627f3b4513e320ce6a7f5a9", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22174, - { - "block": 4912746, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "alexft", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:39:18", - "trx_id": "7deff2e5e96a0f015627f3b4513e320ce6a7f5a9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22175, - { - "block": 4912961, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "zivile", - "pending_payout": { - "amount": "125340", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "pumpkin-pie-with-meat", - "rshares": "203208547179", - "total_vote_weight": "16757885479050732772", - "voter": "camilla", - "weight": "7891753150417841" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:50:12", - "trx_id": "383099ec5dbeb22202801d5f4cf4747ab912a820", - "trx_in_block": 8, - "virtual_op": 1 - } - ], - [ - 22176, - { - "block": 4912961, - "op": { - "type": "vote_operation", - "value": { - "author": "zivile", - "permlink": "pumpkin-pie-with-meat", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:50:12", - "trx_id": "383099ec5dbeb22202801d5f4cf4747ab912a820", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 22177, - { - "block": 4913092, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1571", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "5731769098", - "total_vote_weight": "8029822271331451431", - "voter": "shortcut", - "weight": "8436053944896939" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:56:45", - "trx_id": "863a0a77eb6137ee0f109bab6a0083c851536414", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22178, - { - "block": 4913092, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "shortcut", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:56:45", - "trx_id": "863a0a77eb6137ee0f109bab6a0083c851536414", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22179, - { - "block": 4913106, - "op": { - "type": "comment_operation", - "value": { - "author": "shortcut", - "body": "Very nice. Seems to be an awesome tool.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160912t185728030z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:57:27", - "trx_id": "17f5277f62a6b5d32202198fec5f8eba5188978f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22180, - { - "block": 4913113, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shortcut", - "pending_payout": { - "amount": "61", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-apple-and-leaves-20160912t185728030z", - "rshares": "203208547179", - "total_vote_weight": "891827284162997537", - "voter": "camilla", - "weight": "10404651648568304" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:57:48", - "trx_id": "bd17e905b5d092365e514261c28c21d3770bf567", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22181, - { - "block": 4913113, - "op": { - "type": "vote_operation", - "value": { - "author": "shortcut", - "permlink": "re-camilla-apple-and-leaves-20160912t185728030z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:57:48", - "trx_id": "bd17e905b5d092365e514261c28c21d3770bf567", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22182, - { - "block": 4913127, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "yes it is :) I would recommend them if you're an artist", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "shortcut", - "parent_permlink": "re-camilla-apple-and-leaves-20160912t185728030z", - "permlink": "re-shortcut-re-camilla-apple-and-leaves-20160912t185830677z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T18:58:30", - "trx_id": "ec945b1d34bec580107a0e215ccb210976fd2a97", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22183, - { - "block": 4913184, - "op": { - "type": "comment_operation", - "value": { - "author": "shortcut", - "body": "I will see if I can get my hands on some here in germany.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "re-shortcut-re-camilla-apple-and-leaves-20160912t185830677z", - "permlink": "re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190120534z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:01:21", - "trx_id": "c8380e7afd771047bd0e7e515d74b6476b951c84", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22184, - { - "block": 4913269, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "curie", - "pending_payout": { - "amount": "340548", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "project-curie-s-daily-curation-list-11-sept-12-sept-2016", - "rshares": "203208547179", - "total_vote_weight": "17405155559370604577", - "voter": "camilla", - "weight": "2996416609912119" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:05:39", - "trx_id": "338a8fc1fdd1c1bcf829a9ad28b44f21b6d35fbb", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22185, - { - "block": 4913269, - "op": { - "type": "vote_operation", - "value": { - "author": "curie", - "permlink": "project-curie-s-daily-curation-list-11-sept-12-sept-2016", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:05:39", - "trx_id": "338a8fc1fdd1c1bcf829a9ad28b44f21b6d35fbb", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22186, - { - "block": 4913334, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shortcut", - "pending_payout": { - "amount": "60", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190120534z", - "rshares": "198975035779", - "total_vote_weight": "874127979041787408", - "voter": "camilla", - "weight": "219988874725516497" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:08:54", - "trx_id": "0d99fdf69a62ddd1417af8034d432bd28c6e8f4f", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 22187, - { - "block": 4913334, - "op": { - "type": "vote_operation", - "value": { - "author": "shortcut", - "permlink": "re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190120534z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:08:54", - "trx_id": "0d99fdf69a62ddd1417af8034d432bd28c6e8f4f", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 22188, - { - "block": 4913349, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "They are impossible to find in Norway, but you can order them online.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "shortcut", - "parent_permlink": "re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190120534z", - "permlink": "re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190939876z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:09:39", - "trx_id": "662aa1b35ba6b161c6af447438e187eeed37f112", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22189, - { - "block": 4913588, - "op": { - "type": "comment_operation", - "value": { - "author": "shortcut", - "body": "Sure, thanks again for your recommendation. I will most likely give them a try.", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190939876z", - "permlink": "re-camilla-re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t192137286z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:21:36", - "trx_id": "21867d35699d4d1e052514949eae68ba1fb27ae7", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22190, - { - "block": 4913806, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shortcut", - "pending_payout": { - "amount": "60", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t192137286z", - "rshares": "198975035779", - "total_vote_weight": "874127979041787408", - "voter": "camilla", - "weight": "317599832385182758" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:32:30", - "trx_id": "980e478eaf85743c9cb0344aa0d513afe000fb98", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22191, - { - "block": 4913806, - "op": { - "type": "vote_operation", - "value": { - "author": "shortcut", - "permlink": "re-camilla-re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t192137286z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:32:30", - "trx_id": "980e478eaf85743c9cb0344aa0d513afe000fb98", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22192, - { - "block": 4914122, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"alexft\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:48:24", - "trx_id": "d2850ffda9af92ab4b605c9797c95267834f800b", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22193, - { - "block": 4914123, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"rarepepe\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:48:27", - "trx_id": "0659c06168130b94ae530500c5d1a8f55b65f3ba", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22194, - { - "block": 4914131, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"spectral\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:48:51", - "trx_id": "06b1aef2bf81b355f3b294dd0b19017af8d5fe78", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22195, - { - "block": 4914133, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"mun\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:48:57", - "trx_id": "c962338bd14ffd438776a22935c8dfd627943258", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22196, - { - "block": 4914134, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"kus-knee\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T19:49:00", - "trx_id": "faf88fccb59ef477073b27e64dae6767c5b9d720", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22197, - { - "block": 4915014, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "jamielefay", - "comment_permlink": "ahe-ey-allegiance-an-original-novel-part-18", - "curator": "camilla", - "reward": { - "amount": "1147565547", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T20:33:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 30 - } - ], - [ - 22198, - { - "block": 4916296, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "https://i.imgsafe.org/71f423f2a9.jpg\n\n### Drawing made with Prismacolor pencils on gray toned paper.", - "json_metadata": "{\"tags\":[\"art\",\"portrait\",\"\"],\"image\":[\"https://i.imgsafe.org/71f423f2a9.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "girl-with-a-pink-bow", - "title": "Girl with a Pink Bow" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:37:27", - "trx_id": "80c965965523ce8acfc51f73b884e7a054a35cd2", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22199, - { - "block": 4916296, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "63", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "203214055493", - "total_vote_weight": "891850289889720953", - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:37:27", - "trx_id": "80c965965523ce8acfc51f73b884e7a054a35cd2", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22200, - { - "block": 4916296, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:37:27", - "trx_id": "80c965965523ce8acfc51f73b884e7a054a35cd2", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22201, - { - "block": 4916302, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "67", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "13292298415", - "total_vote_weight": "947191102219314573", - "voter": "goldmatters", - "weight": "553408123295936" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:37:45", - "trx_id": "dd597d493c3db0ce10accb154fef71299918e7bb", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22202, - { - "block": 4916302, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "goldmatters", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:37:45", - "trx_id": "dd597d493c3db0ce10accb154fef71299918e7bb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22203, - { - "block": 4916318, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "313", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "651650151993", - "total_vote_weight": "3289676669365350333", - "voter": "clains", - "weight": "85891137462021311" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:38:33", - "trx_id": "ff53785405c90524eb14733a62089b958f10c070", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 22204, - { - "block": 4916318, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "clains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:38:33", - "trx_id": "ff53785405c90524eb14733a62089b958f10c070", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 22205, - { - "block": 4916323, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "370", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "130765654564", - "total_vote_weight": "3686166908016189812", - "voter": "psylains", - "weight": "17842060739287776" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:38:48", - "trx_id": "e28e29607feec76332c15c9a999045cd22a0ea55", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 22206, - { - "block": 4916323, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "psylains", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:38:48", - "trx_id": "e28e29607feec76332c15c9a999045cd22a0ea55", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 22207, - { - "block": 4916338, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "506", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "289496406729", - "total_vote_weight": "4494184275858851371", - "voter": "anonymous", - "weight": "56561215748986309" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:39:33", - "trx_id": "bef8d8547aeda34ea8ea5eac653dc1d8a751c32f", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22208, - { - "block": 4916338, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "anonymous", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:39:33", - "trx_id": "bef8d8547aeda34ea8ea5eac653dc1d8a751c32f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22209, - { - "block": 4916338, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "506", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 706772487, - "total_vote_weight": "4496048721254833974", - "voter": "murh", - "weight": "130511177718782" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:39:33", - "trx_id": "fd6ceb6f771ec2253c192556c371833962d168d8", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22210, - { - "block": 4916338, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "murh", - "weight": 1509 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:39:33", - "trx_id": "fd6ceb6f771ec2253c192556c371833962d168d8", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22211, - { - "block": 4916360, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -20,17 +20,17 @@\n rg/7\n-1f423f2a9\n+20a7b6b2e\n .jpg\n", - "json_metadata": "{\"tags\":[\"art\",\"portrait\",\"\"],\"image\":[\"https://i.imgsafe.org/720a7b6b2e.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "girl-with-a-pink-bow", - "title": "Girl with a Pink Bow" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:40:39", - "trx_id": "a2071624a1a449c2999d18d9e83cf0d2067deeef", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22212, - { - "block": 4916366, - "op": { - "type": "comment_operation", - "value": { - "author": "kalipo", - "body": "very nice post :) would be nice if you check my channel, perhaps u like my art. i followed you! keep it on <3 kalipo", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "girl-with-a-pink-bow", - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t214058303z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:40:57", - "trx_id": "24e934a2692eecc3de16a50f7ac7601c1c72b247", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22213, - { - "block": 4916369, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "515", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "17018803209", - "total_vote_weight": "4540793856788001810", - "voter": "samether", - "weight": "5518566715757366" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:41:09", - "trx_id": "e6de8a33e981a8690d70c57d277d06d271118290", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22214, - { - "block": 4916369, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "samether", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:41:09", - "trx_id": "e6de8a33e981a8690d70c57d277d06d271118290", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22215, - { - "block": 4916400, - "op": { - "type": "custom_json_operation", - "value": { - "id": "follow", - "json": "[\"follow\",{\"follower\":\"camilla\",\"following\":\"kalipo\",\"what\":[\"blog\"]}]", - "required_auths": [], - "required_posting_auths": [ - "camilla" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:42:42", - "trx_id": "0b7a86690f3a9f5d35a5be3ed10a8c1fb12b6705", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22216, - { - "block": 4916410, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "515", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 143406202, - "total_vote_weight": "4541169675001274297", - "voter": "anomaly", - "weight": "72031824210560" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:43:12", - "trx_id": "15d9a75cd6dc97335c7d7ed062827acf6e84e3b5", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22217, - { - "block": 4916410, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "anomaly", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:43:12", - "trx_id": "15d9a75cd6dc97335c7d7ed062827acf6e84e3b5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22218, - { - "block": 4916412, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "I love your art :) I follow you too ^^", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "kalipo", - "parent_permlink": "re-camilla-girl-with-a-pink-bow-20160912t214058303z", - "permlink": "re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t214319415z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:43:18", - "trx_id": "7e96c2fd0123e831143cbc360541744c7064e5c7", - "trx_in_block": 15, - "virtual_op": 0 - } - ], - [ - 22219, - { - "block": 4916444, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "516", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 2340059283, - "total_vote_weight": "4547299294718017826", - "voter": "steemswede", - "weight": "1522188896324643" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:44:54", - "trx_id": "df8a1f4d39cd63d72c8f4b896e0dfaadeb1648c5", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22220, - { - "block": 4916444, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "steemswede", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:44:54", - "trx_id": "df8a1f4d39cd63d72c8f4b896e0dfaadeb1648c5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22221, - { - "block": 4916475, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "516", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 661462066, - "total_vote_weight": "4549030968269369775", - "voter": "elishagh1", - "weight": "519502065405584" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:46:27", - "trx_id": "b90ef08565b157be3936add6f220d13a2ee0785e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22222, - { - "block": 4916475, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "elishagh1", - "weight": 200 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:46:27", - "trx_id": "b90ef08565b157be3936add6f220d13a2ee0785e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22223, - { - "block": 4916492, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "518", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "9721807408", - "total_vote_weight": "4574432474926443145", - "voter": "andread", - "weight": "8340161352405756" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:47:18", - "trx_id": "104c5e979574ce38dbbe4be2788e79d112a66cb7", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22224, - { - "block": 4916492, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "andread", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:47:18", - "trx_id": "104c5e979574ce38dbbe4be2788e79d112a66cb7", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22225, - { - "block": 4916571, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "517", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 472813942, - "total_vote_weight": "4575665493525935200", - "voter": "wuyueling", - "weight": "567188555766345" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:51:15", - "trx_id": "177d9df39d6ebde2134b872df92d0637797eef63", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22226, - { - "block": 4916571, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "wuyueling", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:51:15", - "trx_id": "177d9df39d6ebde2134b872df92d0637797eef63", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22227, - { - "block": 4916587, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "517", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 174554557, - "total_vote_weight": "4576120646820479706", - "voter": "chadcrypto", - "weight": "221507936678326" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:52:03", - "trx_id": "676c86f57df989194825764317b4be0ff7843f30", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22228, - { - "block": 4916587, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "chadcrypto", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:52:03", - "trx_id": "676c86f57df989194825764317b4be0ff7843f30", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22229, - { - "block": 4916675, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "517", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 3321707002, - "total_vote_weight": "4584776352339379143", - "voter": "glitterpig", - "weight": "5481946828636310" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:56:27", - "trx_id": "1d7249cc7441e461430860771500e4dfae7527b4", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22230, - { - "block": 4916675, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "glitterpig", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:56:27", - "trx_id": "1d7249cc7441e461430860771500e4dfae7527b4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22231, - { - "block": 4916677, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "546", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "59861247996", - "total_vote_weight": "4738931872898669206", - "voter": "theshell", - "weight": "98145681422748006" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:56:33", - "trx_id": "48aed6599d43339b41438dc0f3b67e051661be10", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22232, - { - "block": 4916677, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "theshell", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:56:33", - "trx_id": "48aed6599d43339b41438dc0f3b67e051661be10", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22233, - { - "block": 4916692, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "549", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "5606477483", - "total_vote_weight": "4753194336789149113", - "voter": "shortcut", - "weight": "9436996940867538" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:57:18", - "trx_id": "83e60e2795eeddbd13ee88f12f34c818d3650cf7", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22234, - { - "block": 4916692, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "shortcut", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:57:18", - "trx_id": "83e60e2795eeddbd13ee88f12f34c818d3650cf7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22235, - { - "block": 4916736, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "@@ -21,16 +21,16 @@\n g/72\n-0a7b6b2e\n+52ed86f2\n .jpg\n", - "json_metadata": "{\"tags\":[\"art\",\"portrait\",\"\"],\"image\":[\"https://i.imgsafe.org/7252ed86f2.jpg\"]}", - "parent_author": "", - "parent_permlink": "art", - "permlink": "girl-with-a-pink-bow", - "title": "Girl with a Pink Bow" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T21:59:33", - "trx_id": "584db637e10b487887e595df3dd7b30b167e25b5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22236, - { - "block": 4916748, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "560", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "26148166391", - "total_vote_weight": "4819323227520767625", - "voter": "pixielolz", - "weight": "50037527320258007" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:09", - "trx_id": "5cd6808ea328edd01a08c38503bc537aef69404a", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22237, - { - "block": 4916748, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "pixielolz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:09", - "trx_id": "5cd6808ea328edd01a08c38503bc537aef69404a", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22238, - { - "block": 4916749, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "566", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "12188532385", - "total_vote_weight": "4849930351141385874", - "voter": "acidyo", - "weight": "23210402078968838" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:12", - "trx_id": "b43483f8c49ff4038c8a1b37e6a4eba41fea9d9e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22239, - { - "block": 4916749, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "acidyo", - "weight": 4200 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:12", - "trx_id": "b43483f8c49ff4038c8a1b37e6a4eba41fea9d9e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22240, - { - "block": 4916756, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "kalipo", - "pending_payout": { - "amount": "62", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t214058303z", - "rshares": "203214055493", - "total_vote_weight": "891850289889720953", - "voter": "camilla", - "weight": "582675522727951022" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:33", - "trx_id": "f5bff67af518a6694d53487017269cd4569e2a2c", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22241, - { - "block": 4916756, - "op": { - "type": "vote_operation", - "value": { - "author": "kalipo", - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t214058303z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:33", - "trx_id": "f5bff67af518a6694d53487017269cd4569e2a2c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22242, - { - "block": 4916757, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "565", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 760600032, - "total_vote_weight": "4851835768230529166", - "voter": "coar", - "weight": "1470346853788906" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:36", - "trx_id": "7b057dccf6e308177c64a1424b6cb2e334b7d91b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22243, - { - "block": 4916757, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "coar", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:36", - "trx_id": "7b057dccf6e308177c64a1424b6cb2e334b7d91b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22244, - { - "block": 4916758, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "565", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 102583242, - "total_vote_weight": "4852092713758591955", - "voter": "cryptochannel", - "weight": "198704541701890" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:39", - "trx_id": "7196d8de9ff44afcbb96a7628072d83f99d3e976", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22245, - { - "block": 4916758, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "cryptochannel", - "weight": 4200 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:39", - "trx_id": "7196d8de9ff44afcbb96a7628072d83f99d3e976", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22246, - { - "block": 4916758, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "565", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 103512966, - "total_vote_weight": "4852351978169368919", - "voter": "strawhat", - "weight": "200497811000852" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:39", - "trx_id": "ed35f7d315d328863fe444f3c3c461b5525f1229", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22247, - { - "block": 4916758, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "strawhat", - "weight": 4200 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:00:39", - "trx_id": "ed35f7d315d328863fe444f3c3c461b5525f1229", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22248, - { - "block": 4916780, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "567", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 2337983869, - "total_vote_weight": "4858205191457479243", - "voter": "cryptoeasy", - "weight": "4741102763369362" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:01:45", - "trx_id": "1a78053e811a41f83f7b60f8172e77dc40f430f3", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22249, - { - "block": 4916780, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "cryptoeasy", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:01:45", - "trx_id": "1a78053e811a41f83f7b60f8172e77dc40f430f3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22250, - { - "block": 4916808, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "dollarvigilante", - "pending_payout": { - "amount": "309284", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "boom-end-game-nears-as-central-banks-buying-up-gold-mining-companies", - "rshares": "198980429337", - "total_vote_weight": "17347502297607352140", - "voter": "camilla", - "weight": "3262736250790415" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:03:09", - "trx_id": "128a754b49f592ce7ed2d36cc331d07d0d5bd576", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22251, - { - "block": 4916808, - "op": { - "type": "vote_operation", - "value": { - "author": "dollarvigilante", - "permlink": "boom-end-game-nears-as-central-banks-buying-up-gold-mining-companies", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:03:09", - "trx_id": "128a754b49f592ce7ed2d36cc331d07d0d5bd576", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22252, - { - "block": 4916810, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "timsaid", - "pending_payout": { - "amount": "274975", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "life-explorers-the-human-senses-part-iii-touch", - "rshares": "198980429337", - "total_vote_weight": "17283096775208858980", - "voter": "camilla", - "weight": "3663011961919186" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:03:15", - "trx_id": "5681959624007665e9daf2ebaa79af7325b24739", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22253, - { - "block": 4916810, - "op": { - "type": "vote_operation", - "value": { - "author": "timsaid", - "permlink": "life-explorers-the-human-senses-part-iii-touch", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:03:15", - "trx_id": "5681959624007665e9daf2ebaa79af7325b24739", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22254, - { - "block": 4916984, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "572", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "11707843677", - "total_vote_weight": "4887440477506390273", - "voter": "primus", - "weight": "29235286048911030" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:12:00", - "trx_id": "3e9a27d17780f835cc1e5b29a0294fb9110b6660", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22255, - { - "block": 4916984, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "primus", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:12:00", - "trx_id": "3e9a27d17780f835cc1e5b29a0294fb9110b6660", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22256, - { - "block": 4917019, - "op": { - "type": "comment_operation", - "value": { - "author": "camilla", - "body": "so strange! I have no idea how this happened?", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "shenanigator", - "parent_permlink": "re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t220903089z", - "permlink": "re-shenanigator-re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t221343480z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:13:45", - "trx_id": "7db07dbca76911fe76023de2a15ecb7bbffbcaa9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22257, - { - "block": 4917068, - "op": { - "type": "delete_comment_operation", - "value": { - "author": "camilla", - "permlink": "re-shenanigator-re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t221343480z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:16:12", - "trx_id": "0ee71502d76304c9db7f67d03bf30e05f1b1ec37", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22258, - { - "block": 4917086, - "op": { - "type": "delete_comment_operation", - "value": { - "author": "camilla", - "permlink": "re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t214319415z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:17:06", - "trx_id": "653622fd30607eebe79634ea272aa9a28a1dc085", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22259, - { - "block": 4917112, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "shenanigator", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t220903089z", - "rshares": -198980429337, - "total_vote_weight": 0, - "voter": "camilla", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:18:24", - "trx_id": "46e2666911f2d83905eb98617ce1b194d013dffe", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22260, - { - "block": 4917112, - "op": { - "type": "vote_operation", - "value": { - "author": "shenanigator", - "permlink": "re-kalipo-re-camilla-girl-with-a-pink-bow-20160912t220903089z", - "voter": "camilla", - "weight": -10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:18:24", - "trx_id": "46e2666911f2d83905eb98617ce1b194d013dffe", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22261, - { - "block": 4917164, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "573", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "8529222483", - "total_vote_weight": "4908659449446431993", - "voter": "mione", - "weight": "21218971940041720" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:21:00", - "trx_id": "98d18c96ce0f5d80843e040f46744a8d48986f83", - "trx_in_block": 8, - "virtual_op": 1 - } - ], - [ - 22262, - { - "block": 4917164, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "mione", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:21:00", - "trx_id": "98d18c96ce0f5d80843e040f46744a8d48986f83", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 22263, - { - "block": 4917165, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "659", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "166818556981", - "total_vote_weight": "5310714918838842190", - "voter": "highasfuck", - "weight": "402055469392410197" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:21:03", - "trx_id": "930d3ad18443bb7b97ae4af3defdf1bf8aff3da3", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22264, - { - "block": 4917165, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "highasfuck", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:21:03", - "trx_id": "930d3ad18443bb7b97ae4af3defdf1bf8aff3da3", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22265, - { - "block": 4917752, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "877", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "464346063318", - "total_vote_weight": "6313703114050534435", - "voter": "glitterfart", - "weight": "1002988195211692245" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:50:27", - "trx_id": "b600c85b0b4cb2a154bf388b22a92df4f0376aee", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22266, - { - "block": 4917752, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "glitterfart", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:50:27", - "trx_id": "b600c85b0b4cb2a154bf388b22a92df4f0376aee", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22267, - { - "block": 4917799, - "op": { - "type": "comment_operation", - "value": { - "author": "karenb54", - "body": "Fab drawing, Well done\n I can't draw wish I could", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "girl-with-a-pink-bow", - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t225246008z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:52:48", - "trx_id": "c16690c695adf35fdc21ef351effe74cd374c907", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 22268, - { - "block": 4917804, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "875", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 508520907, - "total_vote_weight": "6314717567476877096", - "voter": "karenb54", - "weight": "1014453426342661" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:53:03", - "trx_id": "b7dad8ffe592b9195b507de4164f04f962766178", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22269, - { - "block": 4917804, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "karenb54", - "weight": 1000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T22:53:03", - "trx_id": "b7dad8ffe592b9195b507de4164f04f962766178", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22270, - { - "block": 4918292, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1497", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 1192255729, - "total_vote_weight": "8031575325835864463", - "voter": "ndbeledrifts", - "weight": "1753054504413032" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T23:17:36", - "trx_id": "48a05bad4d1d2ab9db971e836030d8d28172983b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22271, - { - "block": 4918292, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ndbeledrifts", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-12T23:17:36", - "trx_id": "48a05bad4d1d2ab9db971e836030d8d28172983b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22272, - { - "block": 4919558, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 916860564, - "total_vote_weight": 0, - "voter": "gretepe", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:21:03", - "trx_id": "e6fc3478ca7807d76b6c60d397861af6b95df2ce", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22273, - { - "block": 4919558, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "gretepe", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:21:03", - "trx_id": "e6fc3478ca7807d76b6c60d397861af6b95df2ce", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22274, - { - "block": 4919579, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "3", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 898882906, - "total_vote_weight": 0, - "voter": "gretepe", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:22:06", - "trx_id": "cd4bb7587bfa68de9a8e252471f64a8f765a61a2", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22275, - { - "block": 4919579, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "gretepe", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:22:06", - "trx_id": "cd4bb7587bfa68de9a8e252471f64a8f765a61a2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22276, - { - "block": 4919592, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1490", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 898882906, - "total_vote_weight": "8032896624299636937", - "voter": "gretepe", - "weight": "1321298463772474" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:22:45", - "trx_id": "2289ce7db23ba1807eb571ed3a8e735fe9f1950d", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22277, - { - "block": 4919592, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "gretepe", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:22:45", - "trx_id": "2289ce7db23ba1807eb571ed3a8e735fe9f1950d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22278, - { - "block": 4920186, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "868", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 116538182, - "total_vote_weight": "6314950026765510483", - "voter": "bullionstackers", - "weight": "232459288633387" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:52:33", - "trx_id": "62a1e247a332ff9b1ecec53b6107eb9791f7dfc9", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22279, - { - "block": 4920186, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "bullionstackers", - "weight": 100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:52:33", - "trx_id": "62a1e247a332ff9b1ecec53b6107eb9791f7dfc9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22280, - { - "block": 4920201, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "876", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "12345609219", - "total_vote_weight": "6339525518887297481", - "voter": "asim", - "weight": "24575492121786998" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:53:18", - "trx_id": "fae458bf640f2448856675cd48a113fc80f4e1f2", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22281, - { - "block": 4920201, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "asim", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:53:18", - "trx_id": "fae458bf640f2448856675cd48a113fc80f4e1f2", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22282, - { - "block": 4920233, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "shadowspub", - "comment_permlink": "sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th", - "curator": "camilla", - "reward": { - "amount": "97569025", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T00:54:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 22283, - { - "block": 4921186, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "sirwinchester", - "comment_permlink": "bitcoin-exchange-berlin-meetup-with-ned-on-skype", - "curator": "camilla", - "reward": { - "amount": "164618133", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T01:42:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 64 - } - ], - [ - 22284, - { - "block": 4922034, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1516", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 3892676217, - "total_vote_weight": "8038614733696221237", - "voter": "alchemage", - "weight": "5718109396584300" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:25:12", - "trx_id": "c2a1254b37f0429e30bda8adea60ae1099df59af", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22285, - { - "block": 4922034, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "alchemage", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:25:12", - "trx_id": "c2a1254b37f0429e30bda8adea60ae1099df59af", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22286, - { - "block": 4922045, - "op": { - "type": "comment_operation", - "value": { - "author": "alchemage", - "body": "Prisma makes the best colouring tools! They're just sooooo expensive!\n\nBeautiful work! :D", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "apple-and-leaves", - "permlink": "re-camilla-apple-and-leaves-20160913t022535929z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:25:45", - "trx_id": "3fe18bc624bc25492a8c9ebf1424d927ba5d3129", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22287, - { - "block": 4922381, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1519", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 2911202361, - "total_vote_weight": "8042887013992325664", - "voter": "ace108", - "weight": "4272280296104427" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:42:39", - "trx_id": "3e85ccfa7ee00166bf7bcc91f70613a2833a3625", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22288, - { - "block": 4922381, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "ace108", - "weight": 8600 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:42:39", - "trx_id": "3e85ccfa7ee00166bf7bcc91f70613a2833a3625", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22289, - { - "block": 4922572, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 1977901532, - "total_vote_weight": 0, - "voter": "haphazard-hstead", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:52:12", - "trx_id": "0ae91d565a959caacb9acacd6876eddf23a763bd", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22290, - { - "block": 4922572, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "haphazard-hstead", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T02:52:12", - "trx_id": "0ae91d565a959caacb9acacd6876eddf23a763bd", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22291, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "how-high-can-steem-fly-butterfly-art-and-thoughts" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 22292, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-trendwizard-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t135705568z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 4 - } - ], - [ - 22293, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-mun-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t141310004z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 22294, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-alexft-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t141337301z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 22295, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-acidyo-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t143931038z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 12 - } - ], - [ - 22296, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-mun-re-camilla-re-mun-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t152547164z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 22297, - { - "block": 4924088, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-tmendieta-re-camilla-how-high-can-steem-fly-butterfly-art-and-thoughts-20160813t195024022z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T04:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 20 - } - ], - [ - 22298, - { - "block": 4925961, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "837", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "4362079757", - "total_vote_weight": "6348185001005246927", - "voter": "alexft", - "weight": "8659482117949446" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T05:42:33", - "trx_id": "e43d6fb2e402e345d3b37e2403476968263fb22a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22299, - { - "block": 4925961, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "alexft", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T05:42:33", - "trx_id": "e43d6fb2e402e345d3b37e2403476968263fb22a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22300, - { - "block": 4926223, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "838", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 3912738831, - "total_vote_weight": "6355941931682000971", - "voter": "sompitonov", - "weight": "7756930676754044" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T05:55:39", - "trx_id": "4cc65bc0edcbbcea32e83819b0eada407e9197fe", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22301, - { - "block": 4926223, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "sompitonov", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T05:55:39", - "trx_id": "4cc65bc0edcbbcea32e83819b0eada407e9197fe", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22302, - { - "block": 4926263, - "op": { - "type": "comment_operation", - "value": { - "author": "sompitonov", - "body": "Wow! Her eyes freezes me!", - "json_metadata": "{\"tags\":[\"art\"]}", - "parent_author": "camilla", - "parent_permlink": "girl-with-a-pink-bow", - "permlink": "re-camilla-girl-with-a-pink-bow-20160913t055737074z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T05:57:39", - "trx_id": "4f21554c97bf1c48f3725e8340107787b5ceb4f3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22303, - { - "block": 4928546, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "854", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 154310336, - "total_vote_weight": "6356247645135058621", - "voter": "sergey44", - "weight": "305713453057650" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T07:52:09", - "trx_id": "36ebf81223e4fae438d8c258f10e8cc6030fdc5c", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22304, - { - "block": 4928546, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "sergey44", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T07:52:09", - "trx_id": "36ebf81223e4fae438d8c258f10e8cc6030fdc5c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22305, - { - "block": 4929416, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "sompitonov", - "pending_payout": { - "amount": "61", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-girl-with-a-pink-bow-20160913t055737074z", - "rshares": "215916271116", - "total_vote_weight": "944741768690820328", - "voter": "camilla", - "weight": "944741768690820328" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:35:45", - "trx_id": "e7ff13a260287b16cde8f21e07179b9c060f26c5", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22306, - { - "block": 4929416, - "op": { - "type": "vote_operation", - "value": { - "author": "sompitonov", - "permlink": "re-camilla-girl-with-a-pink-bow-20160913t055737074z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:35:45", - "trx_id": "e7ff13a260287b16cde8f21e07179b9c060f26c5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22307, - { - "block": 4929419, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "karenb54", - "pending_payout": { - "amount": "60", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t225246008z", - "rshares": "211682618741", - "total_vote_weight": "929686492364995100", - "voter": "camilla", - "weight": "926872755111837607" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:35:54", - "trx_id": "7ecd982e14f18bc256f8af041d27ae52c9eeb0ba", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22308, - { - "block": 4929419, - "op": { - "type": "vote_operation", - "value": { - "author": "karenb54", - "permlink": "re-camilla-girl-with-a-pink-bow-20160912t225246008z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:35:54", - "trx_id": "7ecd982e14f18bc256f8af041d27ae52c9eeb0ba", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22309, - { - "block": 4929479, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "aldentan", - "pending_payout": { - "amount": "60", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "from-weak-ass-bitch-to-ripped-my-fight-with-hyperthyroidism", - "rshares": "211682618741", - "total_vote_weight": "928876702511532069", - "voter": "camilla", - "weight": "597889664822415824" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:38:54", - "trx_id": "b5b7a8a56ceb5ff72e526ecd54ef37857cda8b95", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22310, - { - "block": 4929479, - "op": { - "type": "vote_operation", - "value": { - "author": "aldentan", - "permlink": "from-weak-ass-bitch-to-ripped-my-fight-with-hyperthyroidism", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:38:54", - "trx_id": "b5b7a8a56ceb5ff72e526ecd54ef37857cda8b95", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22311, - { - "block": 4929501, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "hisnameisolllie", - "pending_payout": { - "amount": "40089", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-last-12-weeks-at-the-university-of-steemit-where-has-the-time-gone", - "rshares": "207448966366", - "total_vote_weight": "15660660608835024624", - "voter": "camilla", - "weight": "19649366308309222" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:40:00", - "trx_id": "0a992b0c3949f50611be07a5c4eb9d608e8222fb", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22312, - { - "block": 4929501, - "op": { - "type": "vote_operation", - "value": { - "author": "hisnameisolllie", - "permlink": "the-last-12-weeks-at-the-university-of-steemit-where-has-the-time-gone", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:40:00", - "trx_id": "0a992b0c3949f50611be07a5c4eb9d608e8222fb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22313, - { - "block": 4929505, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "elfkitchen", - "pending_payout": { - "amount": "5847", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "i-want-to-take-you-into-the-chinese-vegetable-market", - "rshares": "207448966366", - "total_vote_weight": "12047910933874615266", - "voter": "camilla", - "weight": "117224190815448576" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:40:12", - "trx_id": "dcf5095a210294298ff3be5916d22abfc4933748", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22314, - { - "block": 4929505, - "op": { - "type": "vote_operation", - "value": { - "author": "elfkitchen", - "permlink": "i-want-to-take-you-into-the-chinese-vegetable-market", - "voter": "camilla", - "weight": 9900 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:40:12", - "trx_id": "dcf5095a210294298ff3be5916d22abfc4933748", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22315, - { - "block": 4929545, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-helle-hello-steemit-world-20160813t200219117z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:42:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 22316, - { - "block": 4929545, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-faraz-re-helle-hello-steemit-world-20160813t200531944z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T08:42:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 12 - } - ], - [ - 22317, - { - "block": 4930158, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1544", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": "75110722747", - "total_vote_weight": "8151914442177577478", - "voter": "easteagle13", - "weight": "109027428185251814" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:13:00", - "trx_id": "1af044527f5c98948ea8141c4a61d0bc1e653615", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22318, - { - "block": 4930158, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "easteagle13", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:13:00", - "trx_id": "1af044527f5c98948ea8141c4a61d0bc1e653615", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22319, - { - "block": 4930528, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "smailer", - "pending_payout": { - "amount": "4061", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-daytime-latte-art-tryings-crown", - "rshares": "211682618741", - "total_vote_weight": "11027982296404596121", - "voter": "camilla", - "weight": "93032810721398963" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:31:33", - "trx_id": "edd2d9a16c95c44ed1a7f43068378380465951c9", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22320, - { - "block": 4930528, - "op": { - "type": "vote_operation", - "value": { - "author": "smailer", - "permlink": "my-daytime-latte-art-tryings-crown", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:31:33", - "trx_id": "edd2d9a16c95c44ed1a7f43068378380465951c9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22321, - { - "block": 4930534, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "yangyang", - "pending_payout": { - "amount": "8042", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-happy-whale", - "rshares": "207448966366", - "total_vote_weight": "12773029243061429974", - "voter": "camilla", - "weight": "91970812938821268" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:31:51", - "trx_id": "6037f736be2e45af53db6fd07670796d4d4dde85", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22322, - { - "block": 4930534, - "op": { - "type": "vote_operation", - "value": { - "author": "yangyang", - "permlink": "my-happy-whale", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T09:31:51", - "trx_id": "6037f736be2e45af53db6fd07670796d4d4dde85", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22323, - { - "block": 4932009, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-clains-free-will-and-conscious-freedom-20160813t203846578z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 22324, - { - "block": 4932009, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-spetey-re-clains-free-will-and-conscious-freedom-20160814t035556706z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 46 - } - ], - [ - 22325, - { - "block": 4932009, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-camilla-re-spetey-re-clains-free-will-and-conscious-freedom-20160814t035643710z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 47 - } - ], - [ - 22326, - { - "block": 4932009, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-freeradical-re-clains-free-will-and-conscious-freedom-20160814t040458759z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 48 - } - ], - [ - 22327, - { - "block": 4932009, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "395495911", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "138", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "re-spetey-re-camilla-re-spetey-re-clains-free-will-and-conscious-freedom-20160814t111905211z", - "vesting_payout": { - "amount": "611497524", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 64 - } - ], - [ - 22328, - { - "block": 4932009, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 401, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "89", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "366", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-spetey-re-camilla-re-spetey-re-clains-free-will-and-conscious-freedom-20160814t111905211z", - "total_payout_value": { - "amount": "276", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 65 - } - ], - [ - 22329, - { - "block": 4932009, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-spetey-re-camilla-re-spetey-re-clains-free-will-and-conscious-freedom-20160814t111905211z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:45:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 66 - } - ], - [ - 22330, - { - "block": 4932204, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "905", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 1608900298, - "total_vote_weight": "6359434213254634812", - "voter": "gidlark", - "weight": "3186568119576191" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:55:36", - "trx_id": "a959195a587828ef88d3f51198076afd57074f3e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22331, - { - "block": 4932204, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "gidlark", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T10:55:36", - "trx_id": "a959195a587828ef88d3f51198076afd57074f3e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22332, - { - "block": 4932837, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "940", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "46148758185", - "total_vote_weight": "6450126173147164114", - "voter": "isteemit", - "weight": "90691959892529302" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T11:27:21", - "trx_id": "4e9dcfa51dc773e72e20cf86fe83fc89361756ac", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22333, - { - "block": 4932837, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "isteemit", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T11:27:21", - "trx_id": "4e9dcfa51dc773e72e20cf86fe83fc89361756ac", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22334, - { - "block": 4933254, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "945", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 290559594, - "total_vote_weight": "6450692872404442054", - "voter": "sonyanka", - "weight": "566699257277940" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T11:48:21", - "trx_id": "acf3bd90598e4c71bfe91eb9c11cbd58f7c6bbab", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22335, - { - "block": 4933254, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "sonyanka", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T11:48:21", - "trx_id": "acf3bd90598e4c71bfe91eb9c11cbd58f7c6bbab", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22336, - { - "block": 4939802, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "alex2016", - "comment_permlink": "my-new-works-from-genuine-leather", - "curator": "camilla", - "reward": { - "amount": "57718574", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:17:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 7 - } - ], - [ - 22337, - { - "block": 4939875, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "kalipo", - "comment_permlink": "raptor-art-drawing-all-day", - "curator": "camilla", - "reward": { - "amount": "60755575", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:21:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 8 - } - ], - [ - 22338, - { - "block": 4939951, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "805", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": 330853272, - "total_vote_weight": "6451338094129531484", - "voter": "helle", - "weight": "645221725089430" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:45", - "trx_id": "70d923ecbbbbc1c8567f88491e141ec38791287b", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22339, - { - "block": 4939951, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:45", - "trx_id": "70d923ecbbbbc1c8567f88491e141ec38791287b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22340, - { - "block": 4939952, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1381", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "rshares": 324365953, - "total_vote_weight": "8152380322344461750", - "voter": "helle", - "weight": "465880166884272" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:48", - "trx_id": "e53e84b7c168554f6bdf4bf12a338d07d52998fb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22341, - { - "block": 4939952, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "apple-and-leaves", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:48", - "trx_id": "e53e84b7c168554f6bdf4bf12a338d07d52998fb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22342, - { - "block": 4939955, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": 324365953, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:57", - "trx_id": "3d41bd7704c57aeb1049339ef6485c5dd6cc1033", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22343, - { - "block": 4939955, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:24:57", - "trx_id": "3d41bd7704c57aeb1049339ef6485c5dd6cc1033", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22344, - { - "block": 4940027, - "op": { - "type": "comment_operation", - "value": { - "author": "helle", - "body": "Love the pictures and you write with your soul <3 proud of you :)", - "json_metadata": "{\"tags\":[\"history\"]}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160913t172833838z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:28:33", - "trx_id": "aa33f94b355d32c5ae0f46ba86d8a54b74b0f3fc", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22345, - { - "block": 4940035, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": 324365953, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:28:57", - "trx_id": "a66c5640ce6432756dd2a1fbfa6c4c5f4dad6c98", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22346, - { - "block": 4940035, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:28:57", - "trx_id": "a66c5640ce6432756dd2a1fbfa6c4c5f4dad6c98", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22347, - { - "block": 4940038, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "the-castle-from-my-dreams", - "rshares": 317878634, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:06", - "trx_id": "bdaa3513584e7dd83c1739b08c498a633cfb17f2", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22348, - { - "block": 4940038, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "the-castle-from-my-dreams", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:06", - "trx_id": "bdaa3513584e7dd83c1739b08c498a633cfb17f2", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22349, - { - "block": 4940040, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "48", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colours-of-a-peacock", - "rshares": 317878634, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:12", - "trx_id": "a1ab35bd31b6031b4f1131a97a368b4a3f18d72e", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22350, - { - "block": 4940040, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colours-of-a-peacock", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:12", - "trx_id": "a1ab35bd31b6031b4f1131a97a368b4a3f18d72e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22351, - { - "block": 4940041, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "do-you-like-to-colour", - "rshares": 317878634, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:15", - "trx_id": "89e62eb2882d6ff5c4f4141fbd13df2ee4411df4", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22352, - { - "block": 4940041, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "do-you-like-to-colour", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:15", - "trx_id": "89e62eb2882d6ff5c4f4141fbd13df2ee4411df4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22353, - { - "block": 4940043, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "zendoodle-drawing", - "rshares": 317878634, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:21", - "trx_id": "d3b5cda99c5a76f057848434b15d6c38d50c0e35", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22354, - { - "block": 4940043, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "zendoodle-drawing", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:21", - "trx_id": "d3b5cda99c5a76f057848434b15d6c38d50c0e35", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22355, - { - "block": 4940047, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "broccoli-and-avocado-soup", - "rshares": 311391315, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:33", - "trx_id": "252a7b954eaaf3f654d493f7aafcd82886267c50", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22356, - { - "block": 4940047, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "broccoli-and-avocado-soup", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:33", - "trx_id": "252a7b954eaaf3f654d493f7aafcd82886267c50", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22357, - { - "block": 4940048, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "bluebird-on-golden-cup", - "rshares": 311391315, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:36", - "trx_id": "df480401e8a6e086b6e5f7c6a3a3b3b084da84dd", - "trx_in_block": 3, - "virtual_op": 1 - } - ], - [ - 22358, - { - "block": 4940048, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "bluebird-on-golden-cup", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:36", - "trx_id": "df480401e8a6e086b6e5f7c6a3a3b3b084da84dd", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 22359, - { - "block": 4940050, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "portrait-of-a-lady-on-blue-background", - "rshares": 311391315, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:42", - "trx_id": "ce95168d83eda8afff590cad387fdfb52fe2877d", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22360, - { - "block": 4940050, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "portrait-of-a-lady-on-blue-background", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:42", - "trx_id": "ce95168d83eda8afff590cad387fdfb52fe2877d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22361, - { - "block": 4940051, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "4", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "a-steemycyborg-elf-with-magic-powers", - "rshares": 311391315, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:45", - "trx_id": "ff0106b65c173c97f65947a35f1cdbf2280fe7ec", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22362, - { - "block": 4940051, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "a-steemycyborg-elf-with-magic-powers", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:45", - "trx_id": "ff0106b65c173c97f65947a35f1cdbf2280fe7ec", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22363, - { - "block": 4940052, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "how-high-can-steem-fly-butterfly-art-and-thoughts", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:29:48", - "trx_id": "7561321f00112a38711f0a500a71d4de2a7b6693", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22364, - { - "block": 4940056, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "a-steem-whale-in-a-dream-world-art-and-poem", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:00", - "trx_id": "d9d0de2b48c6fc1878f79d144aea96b40a87d0ec", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22365, - { - "block": 4940058, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "crazy-flood-on-the-subway-tracks", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:06", - "trx_id": "41655908ee032c9570105e699a3cc08affe8b867", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22366, - { - "block": 4940060, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "my-ladybug-drawing", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:12", - "trx_id": "9dbc9f51eee41973a1b64256c23f6305fe83f39d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22367, - { - "block": 4940064, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "how-to-survive-as-a-student", - "rshares": 304903995, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:24", - "trx_id": "0be7622f8865a3e2ad0418d971538f2bc5c0f7eb", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22368, - { - "block": 4940064, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "how-to-survive-as-a-student", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:24", - "trx_id": "0be7622f8865a3e2ad0418d971538f2bc5c0f7eb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22369, - { - "block": 4940066, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-lsd-spirits", - "rshares": 304903995, - "total_vote_weight": 0, - "voter": "helle", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:30", - "trx_id": "4fa3108991be26a460f47632e63448ad80befcf2", - "trx_in_block": 6, - "virtual_op": 1 - } - ], - [ - 22370, - { - "block": 4940066, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "my-lsd-spirits", - "voter": "helle", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:30:30", - "trx_id": "4fa3108991be26a460f47632e63448ad80befcf2", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 22371, - { - "block": 4940599, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "431305890", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "641", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "vesting_payout": { - "amount": "2906758714", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 11 - } - ], - [ - 22372, - { - "block": 4940599, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 1914, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "95", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "1377", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "apple-and-leaves", - "total_payout_value": { - "amount": "1282", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 12 - } - ], - [ - 22373, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "goldmatters", - "comment_permlink": "re-camilla-apple-and-leaves-20160912t171320575z", - "curator": "camilla", - "reward": { - "amount": "27336288", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 13 - } - ], - [ - 22374, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "ashwim", - "comment_permlink": "re-camilla-apple-and-leaves-20160912t171828553z", - "curator": "camilla", - "reward": { - "amount": "18224192", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 22375, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "acidyo", - "comment_permlink": "re-camilla-apple-and-leaves-20160912t172139964z", - "curator": "camilla", - "reward": { - "amount": "45560481", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 19 - } - ], - [ - 22376, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "aavkc", - "comment_permlink": "re-camilla-apple-and-leaves-20160912t172202293z", - "curator": "camilla", - "reward": { - "amount": "9112096", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 22 - } - ], - [ - 22377, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "pixielolz", - "comment_permlink": "re-camilla-apple-and-leaves-20160912t182902111z", - "curator": "camilla", - "reward": { - "amount": "6074730", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 25 - } - ], - [ - 22378, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "shortcut", - "comment_permlink": "re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t190120534z", - "curator": "camilla", - "reward": { - "amount": "12149461", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 30 - } - ], - [ - 22379, - { - "block": 4940599, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "shortcut", - "comment_permlink": "re-camilla-re-shortcut-re-camilla-re-shortcut-re-camilla-apple-and-leaves-20160912t192137286z", - "curator": "camilla", - "reward": { - "amount": "18224192", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T17:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 33 - } - ], - [ - 22380, - { - "block": 4941317, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "zivile", - "comment_permlink": "pumpkin-pie-with-meat", - "curator": "camilla", - "reward": { - "amount": "54665193", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T18:33:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 6 - } - ], - [ - 22381, - { - "block": 4942131, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "helle", - "pending_payout": { - "amount": "53", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-camilla-there-is-a-land-far-far-north-20160913t172833838z", - "rshares": "215935794309", - "total_vote_weight": "944822817118870055", - "voter": "camilla", - "weight": "944822817118870055" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T19:14:00", - "trx_id": "8b9abebba5b4d9813bf6237b158d12a06a17aa9f", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 22382, - { - "block": 4942131, - "op": { - "type": "vote_operation", - "value": { - "author": "helle", - "permlink": "re-camilla-there-is-a-land-far-far-north-20160913t172833838z", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T19:14:00", - "trx_id": "8b9abebba5b4d9813bf6237b158d12a06a17aa9f", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 22383, - { - "block": 4942425, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "curie", - "comment_permlink": "project-curie-s-daily-curation-list-11-sept-12-sept-2016", - "curator": "camilla", - "reward": { - "amount": "60726492", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T19:28:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 46 - } - ], - [ - 22384, - { - "block": 4945441, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "acidyo", - "comment_permlink": "sketch-kitty-cat-oc", - "curator": "camilla", - "reward": { - "amount": "324702661", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T22:00:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 19 - } - ], - [ - 22385, - { - "block": 4945441, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "acidyo", - "comment_permlink": "re-camilla-re-acidyo-sketch-kitty-cat-oc-20160912t173408484z", - "curator": "camilla", - "reward": { - "amount": "21242230", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T22:00:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 98 - } - ], - [ - 22386, - { - "block": 4947246, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "248753346", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "370", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "vesting_payout": { - "amount": "1689702606", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T23:30:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 12 - } - ], - [ - 22387, - { - "block": 4947246, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 1113, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "54", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "795", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "total_payout_value": { - "amount": "740", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T23:30:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 13 - } - ], - [ - 22388, - { - "block": 4947246, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "karenb54", - "comment_permlink": "re-camilla-girl-with-a-pink-bow-20160912t225246008z", - "curator": "camilla", - "reward": { - "amount": "57637970", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T23:30:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 19 - } - ], - [ - 22389, - { - "block": 4947246, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "sompitonov", - "comment_permlink": "re-camilla-girl-with-a-pink-bow-20160913t055737074z", - "curator": "camilla", - "reward": { - "amount": "60671547", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T23:30:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 22 - } - ], - [ - 22390, - { - "block": 4947620, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "anwenbaumeister", - "comment_permlink": "what-is-culture-a-look-into-various-explanations-of-culture-and-forming-my-own-definition", - "curator": "camilla", - "reward": { - "amount": "500505034", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-13T23:49:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 37 - } - ], - [ - 22391, - { - "block": 4949022, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "timsaid", - "comment_permlink": "life-explorers-the-human-senses-part-iii-touch", - "curator": "camilla", - "reward": { - "amount": "227442475", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T00:59:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 42 - } - ], - [ - 22392, - { - "block": 4949759, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "dollarvigilante", - "comment_permlink": "boom-end-game-nears-as-central-banks-buying-up-gold-mining-companies", - "curator": "camilla", - "reward": { - "amount": "200121755", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T01:36:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 53 - } - ], - [ - 22393, - { - "block": 4952412, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "291", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-first-psychology-lecture", - "rshares": "6533369417", - "total_vote_weight": 0, - "voter": "acidyo", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:30", - "trx_id": "b295e5472bb8113674835dd14d9dffdaecabfd06", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22394, - { - "block": 4952412, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "my-first-psychology-lecture", - "voter": "acidyo", - "weight": 2100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:30", - "trx_id": "b295e5472bb8113674835dd14d9dffdaecabfd06", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22395, - { - "block": 4952416, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "292", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-first-psychology-lecture", - "rshares": 1911037334, - "total_vote_weight": 0, - "voter": "getssidetracked", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:42", - "trx_id": "cd98d8040dea7d3f3eecfa12858c2d2b1fb72e81", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22396, - { - "block": 4952416, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "my-first-psychology-lecture", - "voter": "getssidetracked", - "weight": 2100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:42", - "trx_id": "cd98d8040dea7d3f3eecfa12858c2d2b1fb72e81", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22397, - { - "block": 4952416, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "297", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "my-first-psychology-lecture", - "rshares": "15621109400", - "total_vote_weight": 0, - "voter": "venuspcs", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:42", - "trx_id": "e2dabc4a3875787c4da0616cb245b193d8a6701b", - "trx_in_block": 2, - "virtual_op": 1 - } - ], - [ - 22398, - { - "block": 4952416, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "my-first-psychology-lecture", - "voter": "venuspcs", - "weight": 2100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T03:49:42", - "trx_id": "e2dabc4a3875787c4da0616cb245b193d8a6701b", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 22399, - { - "block": 4957270, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "yangyang", - "comment_permlink": "my-happy-whale", - "curator": "camilla", - "reward": { - "amount": "57529742", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T07:53:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 5 - } - ], - [ - 22400, - { - "block": 4957832, - "op": { - "type": "author_reward_operation", - "value": { - "author": "camilla", - "curators_vesting_payout": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - }, - "hbd_payout": { - "amount": "159", - "nai": "@@000000013", - "precision": 3 - }, - "hive_payout": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "permlink": "re-laivi-this-is-how-coloured-hats-make-norwegians-flirt-in-the-mountains-20160814t224155942z", - "vesting_payout": { - "amount": "735697523", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T08:21:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 14 - } - ], - [ - 22401, - { - "block": 4957832, - "op": { - "type": "comment_reward_operation", - "value": { - "author": "camilla", - "author_rewards": 485, - "beneficiary_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "curator_payout_value": { - "amount": "0", - "nai": "@@000000013", - "precision": 3 - }, - "payout": { - "amount": "319", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "re-laivi-this-is-how-coloured-hats-make-norwegians-flirt-in-the-mountains-20160814t224155942z", - "total_payout_value": { - "amount": "318", - "nai": "@@000000013", - "precision": 3 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T08:21:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 15 - } - ], - [ - 22402, - { - "block": 4957832, - "op": { - "type": "comment_payout_update_operation", - "value": { - "author": "camilla", - "permlink": "re-laivi-this-is-how-coloured-hats-make-norwegians-flirt-in-the-mountains-20160814t224155942z" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T08:21:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 16 - } - ], - [ - 22403, - { - "block": 4958047, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "elfkitchen", - "comment_permlink": "i-want-to-take-you-into-the-chinese-vegetable-market", - "curator": "camilla", - "reward": { - "amount": "63576246", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T08:32:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 3 - } - ], - [ - 22404, - { - "block": 4958215, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "aldentan", - "comment_permlink": "from-weak-ass-bitch-to-ripped-my-fight-with-hyperthyroidism", - "curator": "camilla", - "reward": { - "amount": "39355477", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T08:40:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 2 - } - ], - [ - 22405, - { - "block": 4959301, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "hisnameisolllie", - "comment_permlink": "the-last-12-weeks-at-the-university-of-steemit-where-has-the-time-gone", - "curator": "camilla", - "reward": { - "amount": "108962269", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T09:35:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 31 - } - ], - [ - 22406, - { - "block": 4959683, - "op": { - "type": "curation_reward_operation", - "value": { - "comment_author": "smailer", - "comment_permlink": "my-daytime-latte-art-tryings-crown", - "curator": "camilla", - "reward": { - "amount": "93821903", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T09:54:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 13 - } - ], - [ - 22407, - { - "block": 4968236, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "5", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "there-is-a-land-far-far-north", - "rshares": "7508323572", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:02:51", - "trx_id": "e6c65bbd66154b95b32bd25b4554d5f27a8b65cb", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22408, - { - "block": 4968236, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "there-is-a-land-far-far-north", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:02:51", - "trx_id": "e6c65bbd66154b95b32bd25b4554d5f27a8b65cb", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22409, - { - "block": 4968238, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "colors-of-the-falling-leaves", - "rshares": "7508323572", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:02:57", - "trx_id": "958963c3f1e30d05cb3ef7001226df54910ac989", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22410, - { - "block": 4968238, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "colors-of-the-falling-leaves", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:02:57", - "trx_id": "958963c3f1e30d05cb3ef7001226df54910ac989", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22411, - { - "block": 4968239, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "do-you-like-to-colour", - "rshares": "7333711396", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:00", - "trx_id": "38d2503bac59c7b7d72ba51b2b65b9b79c55d9a9", - "trx_in_block": 0, - "virtual_op": 1 - } - ], - [ - 22412, - { - "block": 4968239, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "do-you-like-to-colour", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:00", - "trx_id": "38d2503bac59c7b7d72ba51b2b65b9b79c55d9a9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22413, - { - "block": 4968242, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "zendoodle-drawing", - "rshares": "7333711396", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:09", - "trx_id": "319e23d39d4f0e0103b3fa7de096dbab69e8cc9a", - "trx_in_block": 1, - "virtual_op": 1 - } - ], - [ - 22414, - { - "block": 4968242, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "zendoodle-drawing", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:09", - "trx_id": "319e23d39d4f0e0103b3fa7de096dbab69e8cc9a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 22415, - { - "block": 4968253, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "2", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "how-to-survive-as-a-student", - "rshares": "7333711396", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:42", - "trx_id": "07001e21fa3ee51d19c706b48867de377ece8cb8", - "trx_in_block": 4, - "virtual_op": 1 - } - ], - [ - 22416, - { - "block": 4968253, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "how-to-survive-as-a-student", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:03:42", - "trx_id": "07001e21fa3ee51d19c706b48867de377ece8cb8", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 22417, - { - "block": 4991895, - "op": { - "type": "comment_operation", - "value": { - "author": "beanz", - "body": "Hi! You've been featured in my post.\n[Petition to take down EARNEST](https://steemit.com/steemit-abuse/@beanz/petition-to-take-down-earnest-fao-dan-and-ned)", - "json_metadata": "{\"tags\":[\"art\"],\"links\":[\"https://steemit.com/steemit-abuse/@beanz/petition-to-take-down-earnest-fao-dan-and-ned\"]}", - "parent_author": "camilla", - "parent_permlink": "girl-with-a-pink-bow", - "permlink": "re-camilla-girl-with-a-pink-bow-20160915t125435394z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:54:36", - "trx_id": "dd0ebcb2019cff741fdd544c1aa7ac784946b8dd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 22418, - { - "block": 4991898, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "camilla", - "pending_payout": { - "amount": "1", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "girl-with-a-pink-bow", - "rshares": "5942642822", - "total_vote_weight": 0, - "voter": "beanz", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:54:45", - "trx_id": "62b8c07b00e8410bae8c324d4c5309737bf3f22b", - "trx_in_block": 5, - "virtual_op": 1 - } - ], - [ - 22419, - { - "block": 4991898, - "op": { - "type": "vote_operation", - "value": { - "author": "camilla", - "permlink": "girl-with-a-pink-bow", - "voter": "beanz", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:54:45", - "trx_id": "62b8c07b00e8410bae8c324d4c5309737bf3f22b", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 22420, - { - "block": 4997949, - "op": { - "type": "comment_operation", - "value": { - "author": "linkback-bot-v0", - "body": "<div> <p> This post has been linked to from another place on Steem. </p> <ul> <li> <a href=\"https://steemit.com/steemit-abuse/@beanz/petition-to-take-down-earnest-fao-dan-and-ned\"> Petition to End steemit harassment FAO @dan & @ned </a> by <a href=\"https://steemit.com/@beanz\"> @beanz </a> </li> <li> <a href=\"https://steemit.com/life/@shadowspub/sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th\"> Sunday Ramble Through Steemit -- Notes on My Favourite Reads Sept 11th </a> by <a href=\"https://steemit.com/@shadowspub\"> @shadowspub </a> </li> </ul> <p> Learn more about <a href=\"https://steemit.com/steem/@ontofractal/steem-linkback-bot-v0-3-released\"> linkback bot v0.3</a> </p> <p>Upvote if you want the bot to continue posting linkbacks for your posts. Flag if otherwise. Built by @ontofractal</p></div>", - "json_metadata": "{}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-linkbacks", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:58:45", - "trx_id": "64e216922cbc97d029f3e6db16f64fb0a0199039", - "trx_in_block": 1, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/only_account.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/only_account.tavern.yaml deleted file mode 100644 index f5b304cc3a90a7e0a682c2146013f560cf08d910..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/only_account.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history only account - - marks: - - patterntest # 5mln block - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history only account - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account": "camilla"} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "only_account" - directory: "account_history_api_patterns/get_account_history" - ignore_tags: ['history'] \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/only_limit.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/only_limit.pat.json deleted file mode 100644 index 574997930745002312ef1abe720f1e4e9739cd20..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/only_limit.pat.json +++ /dev/null @@ -1,28 +0,0 @@ -{ - "history": [ - [ - 22420, - { - "block": 4997949, - "op": { - "type": "comment_operation", - "value": { - "author": "linkback-bot-v0", - "body": "<div> <p> This post has been linked to from another place on Steem. </p> <ul> <li> <a href=\"https://steemit.com/steemit-abuse/@beanz/petition-to-take-down-earnest-fao-dan-and-ned\"> Petition to End steemit harassment FAO @dan & @ned </a> by <a href=\"https://steemit.com/@beanz\"> @beanz </a> </li> <li> <a href=\"https://steemit.com/life/@shadowspub/sunday-ramble-through-steemit-notes-on-my-favourite-reads-sept-11th\"> Sunday Ramble Through Steemit -- Notes on My Favourite Reads Sept 11th </a> by <a href=\"https://steemit.com/@shadowspub\"> @shadowspub </a> </li> </ul> <p> Learn more about <a href=\"https://steemit.com/steem/@ontofractal/steem-linkback-bot-v0-3-released\"> linkback bot v0.3</a> </p> <p>Upvote if you want the bot to continue posting linkbacks for your posts. Flag if otherwise. Built by @ontofractal</p></div>", - "json_metadata": "{}", - "parent_author": "camilla", - "parent_permlink": "there-is-a-land-far-far-north", - "permlink": "re-camilla-there-is-a-land-far-far-north-linkbacks", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:58:45", - "trx_id": "64e216922cbc97d029f3e6db16f64fb0a0199039", - "trx_in_block": 1, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/only_limit.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/only_limit.tavern.yaml deleted file mode 100644 index 454741c423f31ebcbbf8d75ff61803999d27fabc..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/only_limit.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history only limit - - marks: - - patterntest # 5mln block - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history only limit - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account": "camilla","limit": 1} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "only_limit" - directory: "account_history_api_patterns/get_account_history" - ignore_tags: ['history'] \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/pow2_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/pow2_operation.pat.json deleted file mode 100644 index 5e600a7e1b9980f5484865a9e0b93ea24c6cae83..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/pow2_operation.pat.json +++ /dev/null @@ -1,1632 +0,0 @@ -{ - "history": [ - [ - 149, - { - "block": 4107533, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862599931", - "prev_block": "003ead0c90b0cd80e9145805d303957015c50ef1", - "worker_account": "thedao" - }, - "pow_summary": 3878270667 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-15T16:01:57", - "trx_id": "ea4f84871e4a07f923d692792cf06ddc13c31b86", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 151, - { - "block": 4111389, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862600875", - "prev_block": "003ebc1cb58199c927540a25ee69e3b04e900423", - "worker_account": "thedao" - }, - "pow_summary": 3829683784 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-15T19:15:54", - "trx_id": "166d3e15dad506377a3668ab99647b4e73005557", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 153, - { - "block": 4114769, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862615779", - "prev_block": "003ec95089dcba0637752d96cb3f1b2d4d956545", - "worker_account": "thedao" - }, - "pow_summary": 3820773691 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-15T22:06:18", - "trx_id": "e4e2eaa73bb26725e69cd646ede87fb2350b649d", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 155, - { - "block": 4117921, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862581179", - "prev_block": "003ed5a081f9530f16b087759a5f280c6e6c542c", - "worker_account": "thedao" - }, - "pow_summary": 3872323657 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-16T00:44:36", - "trx_id": "1b6e6d9adb447a875352dbe6c83e64eedf2d753c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 157, - { - "block": 4127540, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862605867", - "prev_block": "003efb33c11a4e36627f9e2683b7a8f248f23f6b", - "worker_account": "thedao" - }, - "pow_summary": 3869522173 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-16T08:47:30", - "trx_id": "37cfdd291126b7846bfc101802153c5b8abd5ad5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 159, - { - "block": 4139320, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862607595", - "prev_block": "003f293729d2c77db8a100bdfd4bd64db721f5fa", - "worker_account": "thedao" - }, - "pow_summary": 3842934122 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-16T18:38:54", - "trx_id": "8c5227e46f75fe1c5fde055891c7927df5d69f7b", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 161, - { - "block": 4149881, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862612563", - "prev_block": "003f5278daedc87e453e5e4157b2971e275ac5eb", - "worker_account": "thedao" - }, - "pow_summary": 3833255380 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-17T03:28:57", - "trx_id": "043e77240d50f132aa81a9a672a17fd131efe7d5", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 163, - { - "block": 4152783, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862597363", - "prev_block": "003f5dce989ee66635d4a10003a607cf0943e086", - "worker_account": "thedao" - }, - "pow_summary": 3868085905 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-17T05:54:27", - "trx_id": "54ffcce808361a065b8a14d55ab9397be4443088", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 165, - { - "block": 4155045, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862576763", - "prev_block": "003f66a49f64c7f426efe009b1945311cde8706b", - "worker_account": "thedao" - }, - "pow_summary": 3828461005 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-17T07:48:45", - "trx_id": "efdf335525e3181cf7a23fb8fc9b6a6287fc8354", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 167, - { - "block": 4163566, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862577411", - "prev_block": "003f87ed5a866b3091d77bc6fa55f4b8906261ab", - "worker_account": "thedao" - }, - "pow_summary": 3857286930 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-17T14:59:21", - "trx_id": "0f673b42da50f9aceafb5c80c56bf162396a6df9", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 169, - { - "block": 4174546, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862609827", - "prev_block": "003fb2d12c0d0fead6dafbb3d0af43208f25c13e", - "worker_account": "thedao" - }, - "pow_summary": 3842470927 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-18T00:10:12", - "trx_id": "b29d06499f30f8a84e6b60f246aea0886f4e3817", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 171, - { - "block": 4176607, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213621174", - "prev_block": "003fbadefb7fbed4f4ed5697a49a27910f1630d7", - "worker_account": "thedao" - }, - "pow_summary": 3845861386 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-18T01:53:42", - "trx_id": "4d6721d363b7751d9c9f4e074e57c33e70c49754", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 173, - { - "block": 4188850, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213600234", - "prev_block": "003feab195c995003c89846fd95bfaa429a57fee", - "worker_account": "thedao" - }, - "pow_summary": 3843425931 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-18T12:07:57", - "trx_id": "1287ffffd956f9a1594174dad176e6672ffe4d58", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 175, - { - "block": 4193112, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213561330", - "prev_block": "003ffb573942c0e73034646d0df43e38a0b68bb0", - "worker_account": "thedao" - }, - "pow_summary": 3855448947 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-18T15:41:57", - "trx_id": "ea80fa75cf478fd22af95b963647fa0619027e1c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 177, - { - "block": 4198541, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213588558", - "prev_block": "0040108c2249f558abd37728092ac899dfff4e00", - "worker_account": "thedao" - }, - "pow_summary": 3825934993 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-18T20:15:51", - "trx_id": "09a993bd7a5aa2597154fd7cb2ab4c45b517a5d4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 179, - { - "block": 4201214, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862618355", - "prev_block": "00401afde0eb75827cd0503f8126ff72193acaf0", - "worker_account": "thedao" - }, - "pow_summary": 3858072637 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-18T22:31:12", - "trx_id": "7fb0a631dcde539ed780f93010ebd4158b68a9e2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 181, - { - "block": 4203175, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213566166", - "prev_block": "004022a6cc0c8b2afb008a2c3d46955b27b086ae", - "worker_account": "thedao" - }, - "pow_summary": 3869503479 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-19T00:10:27", - "trx_id": "7c8b4b3e5e0bbdf7d6cb1d57c24ee655f0ad5f28", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 183, - { - "block": 4215162, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213610434", - "prev_block": "00405179641e3d2ba958c5f3105f2e37a92f8db6", - "worker_account": "thedao" - }, - "pow_summary": 3859269654 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-19T10:18:06", - "trx_id": "9f040023930e4387cac86f5a6a6cc755636bb5ca", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 185, - { - "block": 4225121, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213596958", - "prev_block": "00407860fddc0d1381b9f262e9189ff30789471b", - "worker_account": "thedao" - }, - "pow_summary": 3860593420 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-19T18:43:36", - "trx_id": "20646ff043eb220d75526bbe33a28d90bbeb5795", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 187, - { - "block": 4237125, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213578322", - "prev_block": "0040a744b113f9d54dcf3c417eb8ab09a2e884d1", - "worker_account": "thedao" - }, - "pow_summary": 3848509802 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-20T04:49:33", - "trx_id": "feab8167c2a9e55220f793ca314600063d7c8796", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 189, - { - "block": 4243528, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862601379", - "prev_block": "0040c0476876ad1fb4404d8b846d8bc70c5dd0e1", - "worker_account": "thedao" - }, - "pow_summary": 3849101281 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-20T10:11:09", - "trx_id": "03282dbd8a41dc9f63e027e5bd5ebeaa47c6defb", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 191, - { - "block": 4251099, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862597499", - "prev_block": "0040ddda4604ac9581f3d20efac665bc3ad73be5", - "worker_account": "thedao" - }, - "pow_summary": 3876333888 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-20T16:31:27", - "trx_id": "98320a140fb7b862894bfebd54d6c81ab60f5025", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 193, - { - "block": 4268264, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213586038", - "prev_block": "004120e756476b5197118d62534718b4452d3645", - "worker_account": "thedao" - }, - "pow_summary": 3861527237 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-21T06:52:48", - "trx_id": "2c2d6be0a5f41ff396cfaa3a48ccc4ae50225664", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 195, - { - "block": 4280503, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862579019", - "prev_block": "004150b6f4cf582a0509c5e7107deb1ddf264c4b", - "worker_account": "thedao" - }, - "pow_summary": 3857279385 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-21T17:11:39", - "trx_id": "29a2afb717f4299c6cd11f4693d2e0f9475f1f34", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 197, - { - "block": 4282453, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213594450", - "prev_block": "00415854e9f3699b33743131ddb43916cf85a358", - "worker_account": "thedao" - }, - "pow_summary": 3834479031 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-21T18:49:54", - "trx_id": "85c57c3be92898a9cb92cc478c82e0eee96602c0", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 199, - { - "block": 4284883, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213622398", - "prev_block": "004161d24c4c86db4bb9a22444bc0ba9ab75f32a", - "worker_account": "thedao" - }, - "pow_summary": 3870591646 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-21T20:52:39", - "trx_id": "a44b486550629c999a3f7d26c0d79ce7b9cd2c99", - "trx_in_block": 10, - "virtual_op": 0 - } - ], - [ - 201, - { - "block": 4290430, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213567150", - "prev_block": "0041777db3cc75621645c13d0202d9e0af2e90aa", - "worker_account": "thedao" - }, - "pow_summary": 3795337896 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-22T01:32:54", - "trx_id": "33be640207167d97451b4e67a8a9968404a45a78", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 203, - { - "block": 4295304, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862576595", - "prev_block": "00418a87fc700508c1bfa6a9c227b22ebc42cd6f", - "worker_account": "thedao" - }, - "pow_summary": 3848235912 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-22T05:40:00", - "trx_id": "212d689c99b9dd01739f4e42ac4146e7b0e1e171", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205, - { - "block": 4301083, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213593358", - "prev_block": "0041a11afea5dae78aa47899c14441ea2c50a8e8", - "worker_account": "thedao" - }, - "pow_summary": 3829832113 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-22T10:32:18", - "trx_id": "580a90fca17afd84a1408093281bcc2efd2c42c3", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 207, - { - "block": 4314030, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213606882", - "prev_block": "0041d3adcd200ac3a4b6e85244375bc8d70f0cd0", - "worker_account": "thedao" - }, - "pow_summary": 3833651137 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-22T21:27:39", - "trx_id": "a77bba0c13fef97bf2800fccd828e3ce59c8abb9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 209, - { - "block": 4322954, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213604674", - "prev_block": "0041f68906a7037c4f78f2c45f43d817fe5905b7", - "worker_account": "thedao" - }, - "pow_summary": 3805885089 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-23T04:57:42", - "trx_id": "313ac5d3a235b95ca1fcd122c25cd795f127b5f4", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 211, - { - "block": 4334265, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213596790", - "prev_block": "004222b88f200dec46fc14c7d3d7ca0b44140dd2", - "worker_account": "thedao" - }, - "pow_summary": 3848277892 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-23T14:28:09", - "trx_id": "9e12798ac9cc4a6d962f11ad0f37d40ce6042c9c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 213, - { - "block": 4348120, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213570810", - "prev_block": "004258d709a775da79fc0d3e682f30d4c2c9a0e1", - "worker_account": "thedao" - }, - "pow_summary": 3842920592 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-24T02:06:54", - "trx_id": "f533887f036025db135325c6a541d05023b48d49", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 215, - { - "block": 4353228, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213568734", - "prev_block": "00426ccb8fcec80c7a3390a173f47e3e97485538", - "worker_account": "thedao" - }, - "pow_summary": 3852443490 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-24T06:23:24", - "trx_id": "b91b569d814f1d6db6f16e3325cb219741dbab64", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 217, - { - "block": 4364095, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213587490", - "prev_block": "0042973e2b91208c94ead3a7b2b290c2ec10769f", - "worker_account": "thedao" - }, - "pow_summary": 3826553292 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-24T15:28:42", - "trx_id": "f163b83e3f358b06ab7f8bf645d04444961369c1", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 219, - { - "block": 4387348, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862587899", - "prev_block": "0042f213a5d450bcedb80b0fe26000cf46f0048c", - "worker_account": "thedao" - }, - "pow_summary": 3871753598 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-25T11:07:12", - "trx_id": "2f306e130a43363614f9adb54d948b6f66ae61a2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 221, - { - "block": 4396529, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "4886069312213584718", - "prev_block": "004315f0c807004b66d28b8e7379841bd8d17e26", - "worker_account": "thedao" - }, - "pow_summary": 3833633367 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-25T18:50:18", - "trx_id": "66b70e92d22ac1f2d861e5e0643857a89b42579a", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 223, - { - "block": 4407729, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862577411", - "prev_block": "004341b0cdbc33ed20433a4ec66ec37432d6b642", - "worker_account": "thedao" - }, - "pow_summary": 3802563114 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-26T04:18:03", - "trx_id": "a7e908ccf48fa94e3d755df6750b37ca58cba213", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 225, - { - "block": 4426401, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862591587", - "prev_block": "00438aa07f2df6630b517f12939d0f25e9311fed", - "worker_account": "thedao" - }, - "pow_summary": 3817367755 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-26T19:53:48", - "trx_id": "a9c7372e7df6a2a06fd69b2b2c0fb06487b1575e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 227, - { - "block": 4460856, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862601579", - "prev_block": "00441137a81a4062bbc75c43703376f265c031e3", - "worker_account": "thedao" - }, - "pow_summary": 3854501877 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-28T00:54:12", - "trx_id": "8b07b2fc6dc744a4b7aa6bf195cbc70da771b072", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 229, - { - "block": 4471925, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862590963", - "prev_block": "00443c74b25c2fd52ebc8ad15c9ca6bf1de523ac", - "worker_account": "thedao" - }, - "pow_summary": 3790463174 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-28T10:09:51", - "trx_id": "d816861dd61be228cf608948c2536f7891c6510b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 231, - { - "block": 4476985, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862624427", - "prev_block": "0044503888da4ee62b736faa51a420a8718614b6", - "worker_account": "thedao" - }, - "pow_summary": 3821739046 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-28T14:23:15", - "trx_id": "2ce28e4c005e98ade8309f3326b209b735bab1b4", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 233, - { - "block": 4750691, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862615011", - "prev_block": "00487d62f51ec7d03b983bc0719dccb69ec7211b", - "worker_account": "thedao" - }, - "pow_summary": 3810424935 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-07T03:04:15", - "trx_id": "b3731e19c88abe85cf4a0c659b959cd6550941fb", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 235, - { - "block": 4969137, - "op": { - "type": "pow2_operation", - "value": { - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "type": "pow2", - "value": { - "input": { - "nonce": "2363830237862597227", - "prev_block": "004bd2b0ee0b95743da86e6bc04ae0fcc07beb9a", - "worker_account": "thedao" - }, - "pow_summary": 3789314109 - } - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:50:18", - "trx_id": "6cf67a78101e13d38d464d7d4573d1932e3c4a32", - "trx_in_block": 6, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/pow2_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/pow2_operation.tavern.yaml deleted file mode 100644 index 078f4172e00bd17e4915d8de4c7298bf97359fe1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/pow2_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api pow2_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api pow2_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"thedao", "operation_filter_low": 1073741824, "start": 235,"limit": 50} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "pow2_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/pow_and_custom_ops.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/pow_and_custom_ops.pat.json deleted file mode 100644 index d249b4155b78ad09ba5003f4342d9117a0a19606..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/pow_and_custom_ops.pat.json +++ /dev/null @@ -1,656 +0,0 @@ -{ - "history": [ - [ - 0, - { - "block": 268044, - "op": { - "type": "pow_operation", - "value": { - "block_id": "0004170b88f080c1dbb72858d15c1e81df399827", - "nonce": "15917283189219591012", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "e822507714b1c67fdacc5cfd20bc5c498eba195ad1ed6e85d72a2d84f6411c2f", - "signature": "20585b276e535901381a51d8a5459c118fa8dcab2e38703a256447c74b680de8186f2c8510d14602c2a5035037f48b6d85c219bb7fcbf56181c7b6eaba5d0e14c5", - "work": "0000000b4a83b36cb224d32393f83481fc9725d843cf830ef17dd9a424aebee8", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-03T01:07:18", - "trx_id": "fb891ec96ebe83285ae10116f4b518ded7ac8f49", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 2, - { - "block": 270225, - "op": { - "type": "pow_operation", - "value": { - "block_id": "00041f905822f0e88f31b38a8ef3a294a8fc5b00", - "nonce": "15917283189219559054", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "f3a664599174fc0d64ca2bb4d90b550f2d9c0c071879d29bbc255e9857f885bf", - "signature": "20e7f61e93d14c0efcb05376a591f49db57725638925209c54ff0b292f66c3497a575e4a7bacd6b018bff37920afe316e29a43bf26c23bbca5bc4d78bb6af0ea8b", - "work": "0000001a483498b376ce881c94b4e966e3f9da0ddfce2a2cee62be2a29611f7c", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-03T02:56:21", - "trx_id": "2cbb8405923c160e0482bbdcbf9f366a5be19bd4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 3, - { - "block": 278356, - "op": { - "type": "pow_operation", - "value": { - "block_id": "00043f537d920d2cc160e9a34f48c58b41f4a4df", - "nonce": "15917283189219545624", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "b1017354f6786cf379f4341b6225f16526a606e60d1bd39d425f4c834479690f", - "signature": "2043c5d1b41567199c10ba5f76ca83d6770e85967ad08def7f30086d155b7486313beeb5a0d006b397905511b99ffd7f67d3be486e6431848560c3e2a773627135", - "work": "000000041f7ce6d8c15374991e5277c6a7d65d49361a1e720e44d49f2251ded8", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-03T09:54:03", - "trx_id": "51f8bc0db495e2d0f0f910b208119cf9f2359dbd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 5, - { - "block": 281779, - "op": { - "type": "pow_operation", - "value": { - "block_id": "00044cb299fc33c812fb662f104c57a8afe15458", - "nonce": "15917283189219554441", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "62f1becb457479deed205200d262d686666a59a4afc155579582aab6ec900aa2", - "signature": "1fc3eaf058187e11e91de60d5981cd9d8cecdb91acb8a621c2ef1f12131c84a2196403784b72b9dd4c4ec43490acd3cb005685c2c3067681d4a66bf7c03f6e7962", - "work": "0000000a82e02b5c45536d32b4a4701e8ffd10dc220242f7c94361a82604a597", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-03T12:49:48", - "trx_id": "bde455fbdb2eb54fccc4a03956cedc5fa07af67d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 8, - { - "block": 287104, - "op": { - "type": "pow_operation", - "value": { - "block_id": "0004617f87b394d94588eca634c7ad076c7bfc5e", - "nonce": "15917283189219572220", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "783164103923e578aae17663f5c359ee44e0c40832dcc7929d348a96e4bbcff5", - "signature": "1f8d7df31025c4fa9a91c5dfdd8fe6437a4cd27c8eedf7bfec1ece69e2f7c97621751448733221ac4a81c176ecfcbc5f16bd47540ce0b8e67f2610ba9ce5020101", - "work": "00000009732f5adfbfdef8927c607d974ab3cfc7c6f8868784bf8d45f258ba7e", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-03T17:19:09", - "trx_id": "c960805122b80e669b4d070d6a044edba9d33726", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9, - { - "block": 289483, - "op": { - "type": "pow_operation", - "value": { - "block_id": "00046acafe81f298a24e59753ff5809d20aaf856", - "nonce": "15917283189219538825", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "874158c67943c12fcbf2d83b2dae4100546f09e100c1cf03a100be844d62a804", - "signature": "1f7a788489b23e3c39d6c1289555d05a7fb2e46cd2ff21bfa98a4f6685eae7541e2ebe2819e60d9281258b6b47cc6070f10de37502b54456b4c4f2ae2a3275c027", - "work": "0000000a9b664e137808dbb55f5072026d1d04af6d34f8a474bcc1c3549ecbe4", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-03T19:22:57", - "trx_id": "74176c71d484c751bac5597cc6f70ff041c0cff5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 10, - { - "block": 291947, - "op": { - "type": "pow_operation", - "value": { - "block_id": "0004746a8e7e2186fba41bbc75386aa84251d5a7", - "nonce": "15917283189219619259", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "4da105ec2690574bc4dc791c4ffe9a53cbf36a3504b56302d936f8ea5ed1902c", - "signature": "1f7d19a89162b0099d1525c1a8c6407a22617eedc73239901e01c4272dbf634611051708bdf87954eda7941822177f11ffe20328c039929655b66ddbd428fc910f", - "work": "00000005ac559a5388b63bf23acbc44006cdf86945a991216a698b476b0b4589", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-03T21:27:57", - "trx_id": "ca9b8cc4858afda6e5ef654e92ee8123d4f35c41", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 12, - { - "block": 295815, - "op": { - "type": "pow_operation", - "value": { - "block_id": "00048386e119c955bfaf0e18b9ef3922180040a0", - "nonce": "15917283189219546117", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "832fd46949bcb17c86dd345345077e9977c53b2040e11bc00d37268a3d5d7c74", - "signature": "1f6819e94348697371e74f7d58a3f3390bd42193eb987e557def7ed46647dc813d6a47ed3594ccd1aa7e445912821894637ec3fa66132f8b5421e45ce6cca12ddc", - "work": "0000000d37699ccb6122fabde88c9fe19c2ed541b8815d6f4e17d8a191457e3f", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-04T00:41:42", - "trx_id": "d2521e3c04082b0615eca4fb715ace3f79a23c7f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 13, - { - "block": 300568, - "op": { - "type": "pow_operation", - "value": { - "block_id": "00049617656369cb1abcd2ec1e8b73b6e78f61f7", - "nonce": "15917283189219612401", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "bd097cff74f0b53d1392dfcc287cc79765f7a7215f3bd64809271b0bcb8b6e9d", - "signature": "2013d754e5904739c084428ffec48cef695cf8e9c3deb89e045af9f41e632a06d93fc9c8a7deb4c62a8ab0b603264e014947ecb4c766968f77ae16898c38026119", - "work": "0000000cf106ab5ac7de9f0f8d199ae5516cbb6cb036cef2212766f084edab92", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-04T04:45:42", - "trx_id": "3d670ce53024575350da55f6c459893d785d9963", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 14, - { - "block": 308096, - "op": { - "type": "pow_operation", - "value": { - "block_id": "0004b37ffee6d6f9edbf75d364dce80caa580d4d", - "nonce": "15917283189219571334", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "429c00688bfb5baad4cf2edd802bb5a1f3d34c2ff91fd7e546d8c3ac07e954ed", - "signature": "1f3b225bfcd98f95e92e986608678496162bf5069f68c6c0f14461a188de71724f6da378b7b0e2edb30305fe763a09c2b35be310878b91c5a36a9020f13fd3e77b", - "work": "0000001c42f940aa11479f4d02efd7e1dd6fa1470d2499de897c0d6439fed1c8", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-04T11:13:45", - "trx_id": "5566f51b425f668fc68a9f39b2739268e967e3f4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 17, - { - "block": 313467, - "op": { - "type": "pow_operation", - "value": { - "block_id": "0004c87a8b5e7be6cbdbdf65cf61d1f3d6d51fad", - "nonce": "15917283189219536218", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "47955ab7c9fe4f2b7c87cb092dbfe7f6a4067b606875b3581c75d15dc7dc688b", - "signature": "1f76b8b071cd45b501fba81a80ef8fb1039fa979b8131ef7e06b85e4e712d5894e49b5e02e21b04e4e734f34b0cada717b80fc4b3bf6527eb48783405fb973b487", - "work": "00000002746548f94ff5271f567109553fec646d64375eee3d409adc3331848e", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-04T15:47:09", - "trx_id": "ee4bececc6d68a83fd4022d6443f0e152634e469", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 18, - { - "block": 319454, - "op": { - "type": "pow_operation", - "value": { - "block_id": "0004dfdd3f276d3ba551d698b9f2bfbe61f001fa", - "nonce": "15917283189219591482", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "e99e2da2a7c46a1b0e56549225a1a89ba8c8c23d4baee60e44fc8332d70fbbe5", - "signature": "2035737124c5a2367bf56112bbc1e496ce12b40aff77048bd858283239ae0ec42b28663e50ffb11e5f5bb7d0f0435465dd770319f1214aa2c20a98e886f36f2d79", - "work": "00000005314602ae28f922f1d5bad00b02061e15769e8cb4c4d8380a5355f4ba", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-04T20:53:15", - "trx_id": "6d097b62b8de57e0d5ff28fcd7c5c49ba8c53bee", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 19, - { - "block": 322820, - "op": { - "type": "pow_operation", - "value": { - "block_id": "0004ed03546d3915356d4b0d7fe556aafb8f2d16", - "nonce": "15917283189219603070", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "84a56728e80277e20d62b946bdf8e78a07af60ae1445be0fde0824a68d7f86f6", - "signature": "1f9f9573ebb270a02899cff87d6905c65e20f17c76b29966aff2814038c03050f93531d39e39d53a73b456332f93a2512cc81d36a5d736def7a9b341cb6e19fb0d", - "work": "0000000203bcbf98eed9b147810744425008406128c769f132dccc690ee841ca", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-04T23:47:12", - "trx_id": "5103f3419561eca07e05b9a18c4a7f117c0b0185", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21, - { - "block": 326642, - "op": { - "type": "pow_operation", - "value": { - "block_id": "0004fbf168451a3ce7d9e3288f802213948399df", - "nonce": "15917283189219598847", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "e432d257aa45f495a77021fa7a01ddbee9eeda6bc8193864069a0896ada8ba5f", - "signature": "1fb681569ccf2cc1409993bec405f6cc8fa19f54366812aca134b8e8d176d998306ca33cca29e359bcf68fa5614eb5b0016d1336ccfe4b64cfdafb953a9914065b", - "work": "00000009193ae6cfab7fd8a0a69a388f430fb2df43849ea93fbcb4377da3f803", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-05T03:05:12", - "trx_id": "ac6d3a9a986ede9c0e2fa040b0a426b8a67fc816", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 23, - { - "block": 332008, - "op": { - "type": "pow_operation", - "value": { - "block_id": "000510e75c24a0d80507f35eac52280748877ed9", - "nonce": "15917283189219536073", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "9e5fb9f1e553352b3294c221965119f55b13f598ff9482de260a957ddcfc6689", - "signature": "204929709b26d484fd73aaaa64f1462f4488ec41266522d86687090bb02f8a30950faa68c094956dcde40fea1e29e97236dd97478bf96186d6ab79045533ba5875", - "work": "00000003ca83624c47556efa045ad9a825d44441486e305416c117d68cc215e5", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-05T07:38:21", - "trx_id": "7d6ca4a33a0b099e5ba380bcbe069db531675449", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 24, - { - "block": 335122, - "op": { - "type": "pow_operation", - "value": { - "block_id": "00051d110a2b9716f3dd1293ed4e51c54293b5f9", - "nonce": "15917283189219547547", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "e289f0611c1101aac805f9040772c31f4b0b315fe357af4c3ca7bce37960f0a5", - "signature": "206333098b89170113f7d41276e9a3ae691b2156ac8046535a307bf2cbfb40821036b06aad211136270b0b2ec33618349f2ac0e9ffe252e7ef16297cdbbefcf5df", - "work": "0000000093d2944677098980d2d03d9ff694de6edc67e954990a6b741c21d5b8", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-05T10:20:57", - "trx_id": "419771c0e86542f26bf9958a14c93e45eaa58b80", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 26, - { - "block": 354159, - "op": { - "type": "pow_operation", - "value": { - "block_id": "0005676e691a3218ce4cbd996fb92c0d07cf96b0", - "nonce": "15917283189219566589", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "850af5875b93efc9a245132a32b4449d6e259880020382eadf4bf8b6c3fa19e1", - "signature": "20c2da3f757cbb13367650048ca8aea71c60c41cb175152e3c152c8ffc2e8380175c129762e7cc6c580b97cd48958ff844cac1dbe7b2f3a005da7d6ba594d74ce9", - "work": "00000005d6bdc20da71e6ee24b4b0179f3dca0f12fbde1b29766756bf2ad6051", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-06T02:25:09", - "trx_id": "558cced6d2be41677a2ff8b629bbc2446a22972e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 27, - { - "block": 362446, - "op": { - "type": "pow_operation", - "value": { - "block_id": "000587cd23e583b96f50e88d4c88473b054e822f", - "nonce": "15917283189219582179", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "5780bb31c385bd1b39b30f8b7d4b7fd2b2c16ecffee20bf860557dbe235e5786", - "signature": "1f5a4a4dfce253bb6431974eaece0a67e5b30d5db1ebe0a874ccc5a185f33f695b4a83e17c4f11e5a18932c89a59606e09615ddb7ba51000b83734d44b66866d52", - "work": "000000070f151a2666f12285cc2573e1961919106b19277ef9e76e11e9d2917f", - "worker": "STM5btrah64BwLcwWbdrH2KGziHcd4MgqQwazQStYRwTZDrPg8UGd" - }, - "worker_account": "alex" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-04-06T09:46:30", - "trx_id": "5c80d6fcffd535166d336aa75f200c23392c1486", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 37, - { - "block": 1771032, - "op": { - "type": "custom_operation", - "value": { - "data": "04616c6578036a7363025e03fd975da390210c7d61c840dc7aac269f0a27c4b825e1e73116fc935f03030337ed23badb55b11da91ab0333258261a3c74a63d9a9e28d656249d88669ce2d6ae2ff9f6b33305004fe77cdb202451f3f8d5c58b37534287eb0881dfd1495f1eecb03cd9f6e427633912330bb5", - "id": 777, - "required_auths": [ - "alex" - ] - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-26T00:35:18", - "trx_id": "d2b39ecade9620349229ff87a08ee40b4bef0f16", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/pow_and_custom_ops.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/pow_and_custom_ops.tavern.yaml deleted file mode 100644 index 63361532ae65a2366d2dc137557f9eacdd98b994..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/pow_and_custom_ops.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api pow and custom operations - - marks: - - patterntest # account with only 102 operations - # parameter start is skipped - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api pow with custom ops - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"alex", "operation_filter_low": 49152, "start": 14000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "pow_and_custom_ops" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/pow_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/pow_operation.pat.json deleted file mode 100644 index 2fef3d98537d360eb35a9166bb9de0a973c8c5a7..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/pow_operation.pat.json +++ /dev/null @@ -1,144 +0,0 @@ -{ - "history": [ - [ - 0, - { - "block": 152447, - "op": { - "type": "pow_operation", - "value": { - "block_id": "0002537ef28b6258e68882944162060d236e3f36", - "nonce": "7375180734000095590", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "38c4bdc32a2ffc585f817be42f2c84a6cc29876128791e7b083cc006f1da9cfa", - "signature": "2071b73b17987ab392ee8138a6ddf88cd52459e75b596508b349659573db8006ad443e1dbfab563a8cf15074c2b11699b8e6ac0de315d98623306641dbfe016ab7", - "work": "0000000635993a69081f52f6401293e2b73b283d37eacc07e0d786fb0107aaef", - "worker": "STM6sK2WuCasEYXK5gbVjGQZMdNcTNuFghr9Uj2CAKKdDH6ucVUqu" - }, - "worker_account": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T00:04:33", - "trx_id": "bdb74f0af41fb6ac1d4745d9f30b50768bd41bfe", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 2, - { - "block": 162117, - "op": { - "type": "pow_operation", - "value": { - "block_id": "000279440012743f7a07fc21dd28ee99c6fbdfbd", - "nonce": "7375180734000054237", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "58e92f85e1b192de519acdbc0a7e2db4b00c251337065ba34804df83b45e2fc2", - "signature": "1f1359b6d99176fdf15d34c51bed54a82a41219125877780dbb665846ac9b0198f3cb77ee24a6e8a13dd27b29985cf63b17b4ee0df3cd5728e670bd8fa0565cb4d", - "work": "0000000557dbe6f08635b61eeda17040bcd0675c9c20795617b08f83dc17861d", - "worker": "STM6sK2WuCasEYXK5gbVjGQZMdNcTNuFghr9Uj2CAKKdDH6ucVUqu" - }, - "worker_account": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T08:12:27", - "trx_id": "94adb9e44624372d76ad30d02cd75803a6047bbb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 3, - { - "block": 166681, - "op": { - "type": "pow_operation", - "value": { - "block_id": "00028b186103b0056ae870f63ade0fd5da6d91f2", - "nonce": "7375180734000056486", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "221326c2fbeac8cff4a1156f379dbc8e45b0f95fef8e5e0dc077fc5e522e3df7", - "signature": "1f77f6112f632b2d92febbab5d8e6c67aed2e77308b482f5554ea44ebfe37d9baf4104499df8850da093e054290712275d5266c55ba7864d0aa7944a875f33d253", - "work": "00000003eaf1950c4d98c18a06b2b75743077848091028c366b9d0a40e278ea7", - "worker": "STM6sK2WuCasEYXK5gbVjGQZMdNcTNuFghr9Uj2CAKKdDH6ucVUqu" - }, - "worker_account": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T12:00:39", - "trx_id": "3da25bc97437b4cde990096929c4c6527d1216c6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 4, - { - "block": 168925, - "op": { - "type": "pow_operation", - "value": { - "block_id": "000293dcd49fd6527b34cedaa7b7e8efdcf2ee44", - "nonce": "7375180734000037558", - "props": { - "account_creation_fee": { - "amount": "100000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "7ff7894e5bbedd3c9f732a217317213a7446f67f52d4fd4ac38c88e21eb3d1d5", - "signature": "1fb7c9a96cd7ac7a82b8ca992be69cebee2cd4e702ff0cc6b9e1fea20035760b097abee0453b305903ca5e79fdc0ec23cb65700c8667a59ce806757c8599bc765a", - "work": "0000000df71896f1756d6a3456905da575407b4917d922042f72e076f586a2b4", - "worker": "STM6sK2WuCasEYXK5gbVjGQZMdNcTNuFghr9Uj2CAKKdDH6ucVUqu" - }, - "worker_account": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-03-30T13:52:51", - "trx_id": "bb9771712a55e7f230e7164ffe7d753ff9c10c3a", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/pow_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/pow_operation.tavern.yaml deleted file mode 100644 index 5dffc4eecae7e5e645017c6f9d904e1db5388ee1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/pow_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to pow_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history pow_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades","operation_filter_low": 16384,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "pow_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/producer_reward_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/producer_reward_operation.pat.json deleted file mode 100644 index e8846e7921733a7e948acd7f32baececf02185f8..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/producer_reward_operation.pat.json +++ /dev/null @@ -1,23004 +0,0 @@ -{ - "history": [ - [ - 18946, - { - "block": 1605657, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9184049340", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:33:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18947, - { - "block": 1605668, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9183990296", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:34:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18948, - { - "block": 1605687, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9183886525", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:35:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18949, - { - "block": 1605718, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9183720137", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:36:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18950, - { - "block": 1605741, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9183593114", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:37:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18951, - { - "block": 1605758, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9183501874", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:38:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18952, - { - "block": 1605774, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9183414215", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:39:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18953, - { - "block": 1605801, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9183267522", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:40:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18954, - { - "block": 1605822, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9183154823", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:41:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18955, - { - "block": 1605834, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9183090424", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:42:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18956, - { - "block": 1605869, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9182900812", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:44:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18957, - { - "block": 1605884, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9182816741", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:44:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18958, - { - "block": 1605899, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9182734461", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:45:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18959, - { - "block": 1605914, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9182653971", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:46:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18960, - { - "block": 1605939, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9182518035", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:47:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18961, - { - "block": 1605970, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9182346332", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:49:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18962, - { - "block": 1605983, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9182276580", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:49:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18963, - { - "block": 1606010, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9182128136", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:51:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18964, - { - "block": 1606038, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9181977909", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:52:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18965, - { - "block": 1606050, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9181911739", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:53:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18966, - { - "block": 1606078, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9181754365", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:54:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18967, - { - "block": 1606099, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9181641704", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:55:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18968, - { - "block": 1606105, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9181609515", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:56:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18969, - { - "block": 1606129, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9181480764", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:57:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18970, - { - "block": 1606156, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9181332346", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:58:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18971, - { - "block": 1606180, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9181203602", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T06:59:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18972, - { - "block": 1606191, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9181142808", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:00:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18973, - { - "block": 1606219, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9180990825", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:01:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18974, - { - "block": 1606244, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9180853152", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:02:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18975, - { - "block": 1606252, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9180810241", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:03:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18976, - { - "block": 1606276, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9180674361", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:04:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18977, - { - "block": 1606298, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9180554575", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:05:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18978, - { - "block": 1606328, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9180393674", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:07:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18979, - { - "block": 1606348, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9180282834", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:08:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18980, - { - "block": 1606357, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9180234566", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:08:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18981, - { - "block": 1606379, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9180116580", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:09:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18982, - { - "block": 1606396, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9180025410", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:10:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18983, - { - "block": 1606421, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9179891341", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18984, - { - "block": 1606452, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9179721526", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:13:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18985, - { - "block": 1606471, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9179617853", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:14:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18986, - { - "block": 1606481, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9179562442", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:14:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18987, - { - "block": 1606501, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9179451623", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:15:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18988, - { - "block": 1606542, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9179229992", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:17:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18989, - { - "block": 1606562, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9179122756", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:18:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18990, - { - "block": 1606574, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9179054841", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:19:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18991, - { - "block": 1606603, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9178897567", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:20:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18992, - { - "block": 1606624, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9178783190", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:21:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18993, - { - "block": 1606628, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9178758170", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:22:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18994, - { - "block": 1606662, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9178574102", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:23:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18995, - { - "block": 1606680, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9178475816", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:24:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18996, - { - "block": 1606703, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9178350728", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:25:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18997, - { - "block": 1606726, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9178225643", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:27:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18998, - { - "block": 1606741, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9178145233", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:27:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 18999, - { - "block": 1606770, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9177984418", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:29:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19000, - { - "block": 1606789, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9177882572", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:30:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19001, - { - "block": 1606814, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9177744993", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:31:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19002, - { - "block": 1606823, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9177696752", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:31:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19003, - { - "block": 1606843, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9177587765", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:32:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19004, - { - "block": 1606873, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9177426970", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:34:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19005, - { - "block": 1606888, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9177343002", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:35:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19006, - { - "block": 1606910, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9177223305", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:36:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19007, - { - "block": 1606926, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9177137553", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:37:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19008, - { - "block": 1606958, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9176966056", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:38:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19009, - { - "block": 1606983, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9176826719", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:39:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19010, - { - "block": 1606999, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9176739189", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:40:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19011, - { - "block": 1607019, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9176630226", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:41:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19012, - { - "block": 1607032, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9176560562", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:42:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19013, - { - "block": 1607052, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9176453389", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:43:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19014, - { - "block": 1607086, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9176267650", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:45:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19015, - { - "block": 1607094, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9176223003", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:45:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19016, - { - "block": 1607125, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9176051563", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:47:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19017, - { - "block": 1607147, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9175933702", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:48:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19018, - { - "block": 1607165, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9175835487", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:49:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19019, - { - "block": 1607184, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9175730131", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:50:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19020, - { - "block": 1607207, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9175606921", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:51:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19021, - { - "block": 1607226, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9175503357", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:52:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19022, - { - "block": 1607241, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9175419435", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:52:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19023, - { - "block": 1607266, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9175285521", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:54:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19024, - { - "block": 1607282, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9175199818", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:54:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19025, - { - "block": 1607306, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9175069482", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:56:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19026, - { - "block": 1607325, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9174967715", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:57:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19027, - { - "block": 1607361, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9174769543", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:58:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19028, - { - "block": 1607382, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9174657072", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T07:59:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19029, - { - "block": 1607391, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9174607085", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:00:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19030, - { - "block": 1607413, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9174489262", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:01:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19031, - { - "block": 1607425, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9174423211", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:02:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19032, - { - "block": 1607462, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9174221493", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:03:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19033, - { - "block": 1607485, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9174096540", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:05:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19034, - { - "block": 1607498, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9174025140", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:05:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19035, - { - "block": 1607522, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9173894837", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:06:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19036, - { - "block": 1607539, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9173803806", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:07:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19037, - { - "block": 1607565, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9173661016", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:09:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19038, - { - "block": 1607581, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9173575344", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:09:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19039, - { - "block": 1607610, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9173418283", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:11:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19040, - { - "block": 1607615, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9173391512", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:11:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19041, - { - "block": 1607651, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9173196979", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:13:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19042, - { - "block": 1607661, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9173139871", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:13:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19043, - { - "block": 1607689, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9172982825", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:15:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19044, - { - "block": 1607705, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9172895381", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:16:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19045, - { - "block": 1607722, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9172802586", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:16:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19046, - { - "block": 1607742, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9172695517", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:17:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19047, - { - "block": 1607770, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9172543840", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:19:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19048, - { - "block": 1607799, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9172388599", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:20:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19049, - { - "block": 1607813, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9172311873", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:21:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19050, - { - "block": 1607826, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9172242286", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:22:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19051, - { - "block": 1607856, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9172078134", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:23:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19052, - { - "block": 1607877, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9171963946", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:24:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19053, - { - "block": 1607906, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9171806941", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:26:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19054, - { - "block": 1607915, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9171755202", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:26:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19055, - { - "block": 1607941, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9171608909", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:27:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19056, - { - "block": 1607967, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9171469756", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:29:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19057, - { - "block": 1607988, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9171357367", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:30:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19058, - { - "block": 1608002, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9171282443", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:30:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19059, - { - "block": 1608014, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9171216439", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:31:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19060, - { - "block": 1608039, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9171077298", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:32:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19061, - { - "block": 1608074, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9170886433", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:34:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19062, - { - "block": 1608078, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9170865028", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:34:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19063, - { - "block": 1608104, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9170724115", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:36:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19064, - { - "block": 1608128, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9170593908", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:37:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19065, - { - "block": 1608141, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9170522563", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:37:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19066, - { - "block": 1608164, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9170397713", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:39:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19067, - { - "block": 1608189, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9170263948", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:40:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19068, - { - "block": 1608209, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9170155156", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:41:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19069, - { - "block": 1608227, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9170058851", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:42:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19070, - { - "block": 1608264, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9169855545", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:44:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19071, - { - "block": 1608268, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9169832362", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:44:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19072, - { - "block": 1608287, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9169728930", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:45:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19073, - { - "block": 1608323, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9169536339", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:47:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19074, - { - "block": 1608334, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9169475710", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:47:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19075, - { - "block": 1608354, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9169368720", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:48:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19076, - { - "block": 1608376, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9169247468", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:49:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19077, - { - "block": 1608393, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9169154748", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:50:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19078, - { - "block": 1608430, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9168955050", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:52:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19079, - { - "block": 1608435, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9168928305", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:52:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19080, - { - "block": 1608474, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9168716136", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:54:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19081, - { - "block": 1608492, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9168619862", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:55:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19082, - { - "block": 1608505, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9168546765", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:56:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19083, - { - "block": 1608527, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9168429101", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:57:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19084, - { - "block": 1608546, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9168327484", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:58:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19085, - { - "block": 1608578, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9168154562", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T08:59:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19086, - { - "block": 1608585, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9168117126", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:00:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19087, - { - "block": 1608612, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9167967386", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:01:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19088, - { - "block": 1608631, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9167865780", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:02:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19089, - { - "block": 1608644, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9167796261", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:03:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19090, - { - "block": 1608681, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9167593058", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:04:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19091, - { - "block": 1608698, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9167496807", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:05:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19092, - { - "block": 1608709, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9167436206", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:06:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19093, - { - "block": 1608737, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9167281141", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:07:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19094, - { - "block": 1608754, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9167190244", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:08:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19095, - { - "block": 1608779, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9167056575", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:09:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19096, - { - "block": 1608797, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9166958553", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:10:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19097, - { - "block": 1608827, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9166796377", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:12:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19098, - { - "block": 1608850, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9166671630", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:13:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19099, - { - "block": 1608871, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9166557578", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:14:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19100, - { - "block": 1608884, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9166488080", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:15:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19101, - { - "block": 1608908, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9166359777", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:16:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19102, - { - "block": 1608924, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9166272462", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:17:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19103, - { - "block": 1608957, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9166092492", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:18:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19104, - { - "block": 1608974, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9166001619", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:19:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19105, - { - "block": 1608989, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9165917874", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:20:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19106, - { - "block": 1609012, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9165794933", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:21:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19107, - { - "block": 1609040, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9165643489", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:22:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19108, - { - "block": 1609055, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9165563315", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:23:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19109, - { - "block": 1609066, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9165502739", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:24:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19110, - { - "block": 1609097, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9165337052", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:25:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19111, - { - "block": 1609118, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9165223035", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:26:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19112, - { - "block": 1609129, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9165164246", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:27:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19113, - { - "block": 1609150, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9165052014", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:28:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19114, - { - "block": 1609168, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9164950473", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:29:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19115, - { - "block": 1609192, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9164820433", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:30:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19116, - { - "block": 1609217, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9164686834", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:31:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19117, - { - "block": 1609241, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9164556802", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:32:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19118, - { - "block": 1609269, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9164403618", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:34:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19119, - { - "block": 1609275, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9164371557", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:34:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19120, - { - "block": 1609299, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9164239753", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:35:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19121, - { - "block": 1609319, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9164127544", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:36:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19122, - { - "block": 1609345, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9163983280", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:38:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19123, - { - "block": 1609364, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9163878201", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:39:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19124, - { - "block": 1609378, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9163803401", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:39:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19125, - { - "block": 1609412, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9163621749", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:41:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19126, - { - "block": 1609421, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9163573666", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:41:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19127, - { - "block": 1609442, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9163459693", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19128, - { - "block": 1609481, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9163251344", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:44:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19129, - { - "block": 1609485, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9163229975", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:45:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19130, - { - "block": 1609519, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9163046566", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:46:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19131, - { - "block": 1609535, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9162961096", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:47:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19132, - { - "block": 1609564, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9162806186", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:49:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19133, - { - "block": 1609572, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9162758111", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:49:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19134, - { - "block": 1609602, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9162596086", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:51:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19135, - { - "block": 1609619, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9162505283", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:51:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19136, - { - "block": 1609646, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9162355730", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:53:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19137, - { - "block": 1609666, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9162247129", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:54:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19138, - { - "block": 1609675, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9162199061", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:54:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19139, - { - "block": 1609710, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9162008572", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:56:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19140, - { - "block": 1609714, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9161987209", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:56:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19141, - { - "block": 1609751, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9161786049", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:58:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19142, - { - "block": 1609776, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9161648980", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T09:59:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19143, - { - "block": 1609796, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9161540396", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:00:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19144, - { - "block": 1609798, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9161529716", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:00:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19145, - { - "block": 1609834, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9161333916", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:02:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19146, - { - "block": 1609855, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9161219999", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:03:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19147, - { - "block": 1609878, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9161095407", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:04:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19148, - { - "block": 1609901, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9160970818", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:05:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19149, - { - "block": 1609905, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9160949460", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:06:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19150, - { - "block": 1609944, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9160739447", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:08:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19151, - { - "block": 1609963, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9160634445", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:09:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19152, - { - "block": 1609977, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9160557919", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:09:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19153, - { - "block": 1609989, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9160493852", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:10:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19154, - { - "block": 1610018, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9160333688", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:11:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19155, - { - "block": 1610046, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9160184207", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:13:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19156, - { - "block": 1610067, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9160072099", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:14:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19157, - { - "block": 1610077, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9160016936", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:14:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19158, - { - "block": 1610106, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9159860348", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:16:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19159, - { - "block": 1610114, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9159817643", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:16:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19160, - { - "block": 1610153, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9159600566", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:18:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19161, - { - "block": 1610163, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9159547188", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:19:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19162, - { - "block": 1610176, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9159477798", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:19:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19163, - { - "block": 1610215, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9159266075", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:21:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19164, - { - "block": 1610230, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9159182456", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:22:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19165, - { - "block": 1610242, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9159118408", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:23:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19166, - { - "block": 1610270, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9158967189", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:24:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19167, - { - "block": 1610292, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9158849774", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:25:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19168, - { - "block": 1610310, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9158753711", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:26:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19169, - { - "block": 1610325, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9158671880", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:27:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19170, - { - "block": 1610352, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9158526012", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:28:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19171, - { - "block": 1610377, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9158392600", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:29:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19172, - { - "block": 1610388, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9158332121", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:30:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19173, - { - "block": 1610416, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9158179149", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:31:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19174, - { - "block": 1610439, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9158056420", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:32:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19175, - { - "block": 1610469, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9157892786", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:34:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19176, - { - "block": 1610471, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9157882114", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:34:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19177, - { - "block": 1610509, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9157675802", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:36:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19178, - { - "block": 1610514, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9157647346", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:36:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19179, - { - "block": 1610534, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9157538858", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:37:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19180, - { - "block": 1610562, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9157385913", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:39:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19181, - { - "block": 1610587, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9157250757", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:40:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19182, - { - "block": 1610598, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9157190294", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:40:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19183, - { - "block": 1610621, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9157067591", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:42:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19184, - { - "block": 1610645, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9156936001", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:43:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19185, - { - "block": 1610678, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9156758182", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:44:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19186, - { - "block": 1610694, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9156671054", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:45:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19187, - { - "block": 1610704, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9156615933", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:46:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19188, - { - "block": 1610737, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9156438127", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:47:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19189, - { - "block": 1610746, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9156388342", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:48:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19190, - { - "block": 1610766, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9156281663", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:49:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19191, - { - "block": 1610791, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9156144762", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:50:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19192, - { - "block": 1610821, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9155984754", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:52:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19193, - { - "block": 1610847, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9155844306", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:53:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19194, - { - "block": 1610861, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9155767862", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:54:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19195, - { - "block": 1610882, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9155655864", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:55:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19196, - { - "block": 1610895, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9155584756", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:55:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19197, - { - "block": 1610913, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9155488762", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:56:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19198, - { - "block": 1610950, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9155291448", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:58:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19199, - { - "block": 1610968, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9155193682", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:59:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19200, - { - "block": 1610978, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9155140357", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T10:59:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19201, - { - "block": 1611007, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9154982161", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:01:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19202, - { - "block": 1611020, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9154911064", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:02:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19203, - { - "block": 1611051, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9154740435", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:03:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19204, - { - "block": 1611069, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9154642681", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:04:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19205, - { - "block": 1611079, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9154587585", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:04:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19206, - { - "block": 1611112, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9154406305", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:06:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19207, - { - "block": 1611136, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9154278347", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:07:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19208, - { - "block": 1611153, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9154184158", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:08:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19209, - { - "block": 1611169, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9154098856", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:09:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19210, - { - "block": 1611189, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9153992232", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:10:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19211, - { - "block": 1611219, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9153832300", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:11:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19212, - { - "block": 1611246, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9153686589", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:13:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19213, - { - "block": 1611265, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9153585304", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:14:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19214, - { - "block": 1611284, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9153484023", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:15:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19215, - { - "block": 1611295, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9153423610", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:15:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19216, - { - "block": 1611321, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9153285019", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:17:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19217, - { - "block": 1611340, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9153180191", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:18:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19218, - { - "block": 1611360, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9153070034", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:19:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19219, - { - "block": 1611377, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9152979424", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:19:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19220, - { - "block": 1611409, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9152799985", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:21:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19221, - { - "block": 1611434, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9152663191", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:22:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19222, - { - "block": 1611440, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9152631213", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:23:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19223, - { - "block": 1611473, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9152450012", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:24:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19224, - { - "block": 1611495, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9152332768", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:25:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19225, - { - "block": 1611508, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9152263489", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:26:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19226, - { - "block": 1611532, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9152135592", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:27:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19227, - { - "block": 1611541, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9152085855", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:28:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19228, - { - "block": 1611578, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9151885138", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:29:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19229, - { - "block": 1611589, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9151826523", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:30:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19230, - { - "block": 1611605, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9151739490", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:31:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19231, - { - "block": 1611632, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9151592071", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:32:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19232, - { - "block": 1611650, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9151494386", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:33:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19233, - { - "block": 1611677, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9151350527", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:34:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19234, - { - "block": 1611701, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9151220880", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:36:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19235, - { - "block": 1611722, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9151107220", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:37:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19236, - { - "block": 1611735, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9151036184", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:37:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19237, - { - "block": 1611763, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9150885236", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:39:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19238, - { - "block": 1611782, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9150780463", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:40:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19239, - { - "block": 1611812, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9150618870", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:41:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19240, - { - "block": 1611820, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9150576253", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:42:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19241, - { - "block": 1611841, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9150464385", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:43:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19242, - { - "block": 1611870, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9150308129", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:44:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19243, - { - "block": 1611879, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9150256637", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:44:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19244, - { - "block": 1611913, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9150068430", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:46:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19245, - { - "block": 1611929, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9149983206", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:47:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19246, - { - "block": 1611957, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9149834069", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:48:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19247, - { - "block": 1611963, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9149802112", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:49:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19248, - { - "block": 1611990, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9149654756", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:50:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19249, - { - "block": 1612017, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9149509181", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:51:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19250, - { - "block": 1612035, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9149411541", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:52:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19251, - { - "block": 1612058, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9149289051", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:53:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19252, - { - "block": 1612084, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9149147038", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:55:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19253, - { - "block": 1612107, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9149022780", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:56:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19254, - { - "block": 1612116, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9148974853", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:56:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19255, - { - "block": 1612146, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9148813324", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:58:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19256, - { - "block": 1612164, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9148715700", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T11:59:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19257, - { - "block": 1612181, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9148625177", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:00:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19258, - { - "block": 1612199, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9148529332", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:00:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19259, - { - "block": 1612214, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9148442363", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:01:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19260, - { - "block": 1612240, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9148300376", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:03:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19261, - { - "block": 1612266, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9148158394", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:04:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19262, - { - "block": 1612276, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9148105152", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:04:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19263, - { - "block": 1612297, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9147993346", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:05:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19264, - { - "block": 1612318, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9147879767", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:06:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19265, - { - "block": 1612358, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9147665041", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:08:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19266, - { - "block": 1612365, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9147627776", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:09:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19267, - { - "block": 1612386, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9147515981", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:10:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19268, - { - "block": 1612413, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9147370475", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:11:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19269, - { - "block": 1612432, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9147265785", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:12:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19270, - { - "block": 1612464, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9147093672", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:14:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19271, - { - "block": 1612482, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9146990762", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:15:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19272, - { - "block": 1612502, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9146884306", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:16:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19273, - { - "block": 1612522, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9146777853", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:17:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19274, - { - "block": 1612538, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9146689143", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:17:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19275, - { - "block": 1612562, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9146557857", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:19:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19276, - { - "block": 1612586, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9146430123", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:20:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19277, - { - "block": 1612606, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9146323680", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:21:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19278, - { - "block": 1612628, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9146204822", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:22:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19279, - { - "block": 1612633, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9146178212", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:22:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19280, - { - "block": 1612665, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9146007915", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:24:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19281, - { - "block": 1612691, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9145866006", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:25:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19282, - { - "block": 1612715, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9145734743", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:26:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19283, - { - "block": 1612733, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9145637186", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:27:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19284, - { - "block": 1612749, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9145552047", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:28:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19285, - { - "block": 1612764, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9145472230", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:29:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19286, - { - "block": 1612781, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9145379999", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:30:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19287, - { - "block": 1612801, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9145273581", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:31:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19288, - { - "block": 1612824, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9145144110", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:32:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19289, - { - "block": 1612855, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9144977399", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:33:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19290, - { - "block": 1612880, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9144842616", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:35:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19291, - { - "block": 1612891, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9144782319", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:35:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19292, - { - "block": 1612918, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9144635128", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:36:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19293, - { - "block": 1612927, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9144585475", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:37:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19294, - { - "block": 1612968, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9144367360", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:39:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19295, - { - "block": 1612989, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9144250327", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:40:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19296, - { - "block": 1612991, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9144239688", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:40:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19297, - { - "block": 1613031, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9144026909", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:42:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19298, - { - "block": 1613038, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9143989674", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:42:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19299, - { - "block": 1613066, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9143838963", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:44:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19302, - { - "block": 1613092, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9143695349", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:45:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19303, - { - "block": 1613105, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9143626203", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:46:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19304, - { - "block": 1613119, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9143551740", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:46:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19305, - { - "block": 1613154, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9143363813", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:48:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19306, - { - "block": 1613178, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9143232624", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:49:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19307, - { - "block": 1613186, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9143190077", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:50:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19308, - { - "block": 1613219, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9143007484", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:51:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19309, - { - "block": 1613234, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9142924168", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:52:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19310, - { - "block": 1613262, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9142773493", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:54:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19311, - { - "block": 1613281, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9142670682", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:55:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19312, - { - "block": 1613301, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9142562556", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:56:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19313, - { - "block": 1613324, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9142440253", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:57:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19314, - { - "block": 1613330, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9142406576", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:57:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19315, - { - "block": 1613365, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9142218697", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T12:59:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19316, - { - "block": 1613385, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9142112355", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:00:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19317, - { - "block": 1613393, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9142069818", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:00:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19318, - { - "block": 1613421, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9141919172", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:02:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19319, - { - "block": 1613431, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9141864231", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:02:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19320, - { - "block": 1613467, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9141669287", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:04:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19321, - { - "block": 1613493, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9141531059", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:05:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19322, - { - "block": 1613501, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9141486756", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:06:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19323, - { - "block": 1613535, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9141304232", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:07:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19324, - { - "block": 1613545, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9141251071", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:08:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19325, - { - "block": 1613577, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9141073873", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:09:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19326, - { - "block": 1613594, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9140983505", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:10:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19327, - { - "block": 1613604, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9140930348", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:11:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19328, - { - "block": 1613635, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9140763793", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:12:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19329, - { - "block": 1613657, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9140641539", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:13:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19330, - { - "block": 1613671, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9140567125", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:14:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19331, - { - "block": 1613684, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9140498027", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:15:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19332, - { - "block": 1613708, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9140368694", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:16:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19333, - { - "block": 1613726, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9140273025", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:17:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19334, - { - "block": 1613760, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9140088779", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:19:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19335, - { - "block": 1613781, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9139971858", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:20:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19336, - { - "block": 1613803, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9139853168", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:21:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19337, - { - "block": 1613814, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9139794710", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:21:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19338, - { - "block": 1613830, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9139709681", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:22:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19339, - { - "block": 1613871, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9139490032", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:24:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19340, - { - "block": 1613876, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9139463463", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:24:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19341, - { - "block": 1613894, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9139360728", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:25:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19342, - { - "block": 1613926, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9139190689", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:27:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19343, - { - "block": 1613948, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9139073791", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:28:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19344, - { - "block": 1613971, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9138949812", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:29:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19345, - { - "block": 1613984, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9138880739", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:30:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19346, - { - "block": 1614006, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9138762078", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:31:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19347, - { - "block": 1614019, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9138691237", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:31:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19348, - { - "block": 1614042, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9138567269", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:33:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19349, - { - "block": 1614078, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9138372468", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:34:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19350, - { - "block": 1614094, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9138287466", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:35:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19351, - { - "block": 1614113, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9138182988", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:36:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19352, - { - "block": 1614140, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9138037784", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:38:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19353, - { - "block": 1614162, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9137919146", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:39:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19354, - { - "block": 1614184, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9137800510", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:40:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19355, - { - "block": 1614187, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9137784574", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:40:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19356, - { - "block": 1614216, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9137626990", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:41:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19357, - { - "block": 1614240, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9137499509", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:43:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19358, - { - "block": 1614260, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9137393279", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:44:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19359, - { - "block": 1614274, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9137318918", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:44:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19360, - { - "block": 1614303, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9137157809", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:46:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19361, - { - "block": 1614329, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9137017950", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:47:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19362, - { - "block": 1614344, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9136938285", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:48:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19363, - { - "block": 1614371, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9136794892", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:49:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19364, - { - "block": 1614379, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9136752406", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:50:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19365, - { - "block": 1614403, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9136624951", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:51:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19366, - { - "block": 1614428, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9136490419", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:52:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19367, - { - "block": 1614444, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9136401913", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:53:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19368, - { - "block": 1614469, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9136263847", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:54:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19369, - { - "block": 1614489, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9136154105", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:55:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19370, - { - "block": 1614517, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9136003658", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:56:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19371, - { - "block": 1614543, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9135862065", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:58:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19372, - { - "block": 1614563, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9135754103", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:59:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19373, - { - "block": 1614566, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9135738174", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T13:59:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19374, - { - "block": 1614606, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9135524029", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:01:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19375, - { - "block": 1614608, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9135513410", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:01:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19376, - { - "block": 1614642, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9135329360", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:03:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19377, - { - "block": 1614667, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9135196637", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:04:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19378, - { - "block": 1614687, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9135085152", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:05:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19379, - { - "block": 1614692, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9135058608", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:05:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19380, - { - "block": 1614727, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9134869268", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:07:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19381, - { - "block": 1614735, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9134826800", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:07:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19382, - { - "block": 1614757, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9134710016", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:08:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19383, - { - "block": 1614777, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9134603852", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:09:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19384, - { - "block": 1614799, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9134485304", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:11:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19385, - { - "block": 1614834, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9134292450", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:12:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19386, - { - "block": 1614857, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9134166834", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:13:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19387, - { - "block": 1614864, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9134127911", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:14:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19388, - { - "block": 1614882, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9134032375", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:15:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19389, - { - "block": 1614911, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9133876691", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:16:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19390, - { - "block": 1614928, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9133786468", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:17:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19391, - { - "block": 1614954, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9133648483", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:18:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19392, - { - "block": 1614975, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9133533499", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:19:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19393, - { - "block": 1614991, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9133448589", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:20:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19394, - { - "block": 1615023, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9133275237", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:22:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19395, - { - "block": 1615037, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9133199176", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:22:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19396, - { - "block": 1615068, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9133032909", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:24:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19397, - { - "block": 1615080, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9132967465", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:25:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19398, - { - "block": 1615107, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9132824199", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:26:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19399, - { - "block": 1615112, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9132795900", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:26:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19400, - { - "block": 1615145, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9132619036", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:28:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19401, - { - "block": 1615167, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9132502309", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:29:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19402, - { - "block": 1615193, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9132357290", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:30:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19403, - { - "block": 1615196, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9132341373", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:30:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19404, - { - "block": 1615234, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9132136232", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:32:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19405, - { - "block": 1615256, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9132017750", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:33:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19406, - { - "block": 1615269, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9131948784", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:34:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19407, - { - "block": 1615280, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9131888661", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:35:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19408, - { - "block": 1615319, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9131678236", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:37:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19409, - { - "block": 1615332, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9131607507", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:37:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19410, - { - "block": 1615347, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9131527939", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:38:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19411, - { - "block": 1615363, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9131443067", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:39:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19412, - { - "block": 1615401, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9131239736", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:41:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19413, - { - "block": 1615420, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9131137190", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:42:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19414, - { - "block": 1615429, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9131087685", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:42:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19415, - { - "block": 1615456, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9130942712", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:43:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19416, - { - "block": 1615475, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9130838405", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:44:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19417, - { - "block": 1615491, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9130750011", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:45:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19418, - { - "block": 1615520, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9130590906", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:47:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19419, - { - "block": 1615536, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9130506052", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:47:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19420, - { - "block": 1615558, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9130389381", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:49:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19421, - { - "block": 1615585, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9130244430", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:50:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19422, - { - "block": 1615600, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9130164886", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:51:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19423, - { - "block": 1615623, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9130035850", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:52:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19424, - { - "block": 1615646, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9129913889", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:53:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19425, - { - "block": 1615672, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9129776023", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:54:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19426, - { - "block": 1615681, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9129728302", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:55:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19427, - { - "block": 1615716, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9129535652", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:56:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19428, - { - "block": 1615724, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9129493235", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:57:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19429, - { - "block": 1615744, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9129383660", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T14:58:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19430, - { - "block": 1615782, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9129178655", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:00:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19431, - { - "block": 1615793, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9129120336", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:00:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19432, - { - "block": 1615807, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9129044346", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:01:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19433, - { - "block": 1615827, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9128936549", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:02:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19434, - { - "block": 1615849, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9128816384", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:03:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19435, - { - "block": 1615869, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9128708593", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:04:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19436, - { - "block": 1615897, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9128560162", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:05:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19437, - { - "block": 1615913, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9128475347", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:06:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19438, - { - "block": 1615940, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9128330458", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:08:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19439, - { - "block": 1615969, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9128176739", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:09:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19440, - { - "block": 1615983, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9128102532", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:10:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19441, - { - "block": 1615996, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9128031860", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:10:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19442, - { - "block": 1616018, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9127913487", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:12:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19443, - { - "block": 1616039, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9127800417", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:13:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19444, - { - "block": 1616072, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9127625517", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:14:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19445, - { - "block": 1616090, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9127530120", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:15:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19446, - { - "block": 1616106, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9127441791", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:16:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19447, - { - "block": 1616138, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9127268671", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:18:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19448, - { - "block": 1616140, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9127258072", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:18:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19449, - { - "block": 1616177, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9127058464", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:19:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19450, - { - "block": 1616195, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9126961313", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:20:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19451, - { - "block": 1616219, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9126828837", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:22:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19452, - { - "block": 1616230, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9126767016", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:22:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19453, - { - "block": 1616249, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9126664573", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:23:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19454, - { - "block": 1616285, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9126472055", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:25:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19455, - { - "block": 1616307, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9126351957", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:26:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19456, - { - "block": 1616313, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9126320167", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:26:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19457, - { - "block": 1616333, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9126214201", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:27:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19458, - { - "block": 1616358, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9126078215", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:29:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19459, - { - "block": 1616391, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9125903383", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:30:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19460, - { - "block": 1616401, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9125848639", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:31:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19461, - { - "block": 1616417, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9125763875", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:31:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19462, - { - "block": 1616442, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9125631435", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:33:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19463, - { - "block": 1616472, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9125470746", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:34:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19464, - { - "block": 1616493, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9125359503", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:35:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19465, - { - "block": 1616509, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9125272982", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:36:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19466, - { - "block": 1616518, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9125225309", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:37:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19467, - { - "block": 1616544, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9125084056", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:38:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19468, - { - "block": 1616578, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9124902200", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:40:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19469, - { - "block": 1616597, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9124801565", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:41:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19470, - { - "block": 1616602, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9124773316", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:41:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19471, - { - "block": 1616636, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9124589708", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:42:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19472, - { - "block": 1616654, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9124494375", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:43:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19473, - { - "block": 1616675, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9124381391", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:44:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19474, - { - "block": 1616686, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9124319604", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:45:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19475, - { - "block": 1616715, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9124164258", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:46:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19476, - { - "block": 1616747, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9123994796", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:48:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19477, - { - "block": 1616763, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9123906537", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:49:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19478, - { - "block": 1616770, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9123869468", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:49:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19479, - { - "block": 1616801, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9123698248", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:51:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19480, - { - "block": 1616832, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9123530565", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:52:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19481, - { - "block": 1616847, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9123451138", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:53:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19482, - { - "block": 1616860, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9123380538", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:54:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19483, - { - "block": 1616882, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9123264049", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:55:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19484, - { - "block": 1616901, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9123163448", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:56:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19485, - { - "block": 1616919, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9123068144", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:57:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19486, - { - "block": 1616946, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9122923427", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:58:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19487, - { - "block": 1616963, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9122833422", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T15:59:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19488, - { - "block": 1616992, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9122671065", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:00:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19489, - { - "block": 1617006, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9122595183", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:01:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19490, - { - "block": 1617026, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9122489303", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:02:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19491, - { - "block": 1617053, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9122346369", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:03:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19492, - { - "block": 1617068, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9122266963", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:04:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19493, - { - "block": 1617097, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9122113449", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:06:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19494, - { - "block": 1617107, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9122060514", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:06:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19495, - { - "block": 1617146, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9121847018", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:08:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19496, - { - "block": 1617150, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9121824081", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:08:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19497, - { - "block": 1617178, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9121674111", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:10:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19498, - { - "block": 1617191, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9121601774", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:10:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19499, - { - "block": 1617215, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9121472982", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:11:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19500, - { - "block": 1617238, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9121349487", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:13:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19501, - { - "block": 1617272, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9121166014", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:14:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19502, - { - "block": 1617293, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9121054876", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:15:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19503, - { - "block": 1617303, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9121000189", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:16:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19504, - { - "block": 1617328, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9120866123", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:17:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19505, - { - "block": 1617357, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9120705601", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:19:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19506, - { - "block": 1617359, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9120695018", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:19:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19507, - { - "block": 1617383, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9120566252", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:20:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19508, - { - "block": 1617406, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9120442782", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:21:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19509, - { - "block": 1617422, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9120358118", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:22:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19510, - { - "block": 1617444, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9120241709", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:23:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19511, - { - "block": 1617465, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9120130593", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:24:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19512, - { - "block": 1617493, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9119980680", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:25:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19513, - { - "block": 1617514, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9119866044", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:26:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19514, - { - "block": 1617533, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9119763755", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:27:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19515, - { - "block": 1617557, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9119635016", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:29:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19516, - { - "block": 1617573, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9119546841", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:29:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19517, - { - "block": 1617592, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9119442797", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:30:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19518, - { - "block": 1617618, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9119305250", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:32:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19519, - { - "block": 1617651, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9119128915", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:33:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19520, - { - "block": 1617667, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9119044276", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:34:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19521, - { - "block": 1617679, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9118979035", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:35:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19522, - { - "block": 1617699, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9118871477", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:36:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19523, - { - "block": 1617715, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9118783317", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:36:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19524, - { - "block": 1617739, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9118654607", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:38:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19525, - { - "block": 1617760, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9118540004", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:39:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19526, - { - "block": 1617780, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9118430694", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:40:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19527, - { - "block": 1617800, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9118324913", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:41:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19528, - { - "block": 1617830, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9118162719", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:42:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19529, - { - "block": 1617850, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9118056944", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:43:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19530, - { - "block": 1617865, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9117974089", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:44:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19533, - { - "block": 1617891, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9117836587", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:45:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19536, - { - "block": 1617912, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9117723769", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:46:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19537, - { - "block": 1617925, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9117655021", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:47:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19538, - { - "block": 1617963, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9117450548", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:49:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19539, - { - "block": 1617967, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9117427634", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:49:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19540, - { - "block": 1617998, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9117263710", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:51:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19542, - { - "block": 1618021, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9117140333", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:52:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19543, - { - "block": 1618038, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9117048684", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:53:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19548, - { - "block": 1618053, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9116967611", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:53:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19551, - { - "block": 1618077, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9116838956", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:55:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19552, - { - "block": 1618108, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9116673296", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:56:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19553, - { - "block": 1618118, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9116620428", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:57:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19554, - { - "block": 1618144, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9116481210", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:58:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19555, - { - "block": 1618165, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9116368429", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T16:59:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19556, - { - "block": 1618186, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9116253889", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:00:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19557, - { - "block": 1618207, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9116142876", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:01:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19558, - { - "block": 1618232, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9116005435", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:02:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19559, - { - "block": 1618258, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9115866237", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:04:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19560, - { - "block": 1618268, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9115813378", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:04:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19561, - { - "block": 1618288, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9115707661", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:05:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19562, - { - "block": 1618314, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9115568472", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:06:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19563, - { - "block": 1618342, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9115416954", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:08:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19564, - { - "block": 1618358, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9115330627", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:09:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19565, - { - "block": 1618370, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9115267204", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:09:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19566, - { - "block": 1618402, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9115098080", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:11:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19567, - { - "block": 1618413, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9115039945", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:11:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19568, - { - "block": 1618438, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9114906061", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:13:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19569, - { - "block": 1618466, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9114752804", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:14:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19570, - { - "block": 1618483, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9114661205", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:15:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19571, - { - "block": 1618506, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9114537902", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:16:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19572, - { - "block": 1618533, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9114395226", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:17:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19573, - { - "block": 1618542, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9114345907", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:18:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19574, - { - "block": 1618574, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9114176819", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:19:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19575, - { - "block": 1618589, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9114094038", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:20:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19577, - { - "block": 1618611, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9113974273", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:21:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19578, - { - "block": 1618633, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9113854511", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:22:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19579, - { - "block": 1618649, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9113768214", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:23:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19580, - { - "block": 1618675, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9113630847", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:25:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19581, - { - "block": 1618687, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9113567448", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:25:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19582, - { - "block": 1618710, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9113442414", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:26:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19583, - { - "block": 1618729, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9113336754", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:27:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19584, - { - "block": 1618758, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9113183551", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:29:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19585, - { - "block": 1618769, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9113125441", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:29:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19586, - { - "block": 1618795, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9112986333", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:31:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19587, - { - "block": 1618827, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9112813774", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:32:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19588, - { - "block": 1618833, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9112782080", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:32:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19589, - { - "block": 1618868, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9112593683", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:34:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19590, - { - "block": 1618881, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9112523256", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:35:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19591, - { - "block": 1618895, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9112447549", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:36:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19592, - { - "block": 1618916, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9112336631", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:37:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19593, - { - "block": 1618945, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9112181702", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:38:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19594, - { - "block": 1618970, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9112046144", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:39:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19595, - { - "block": 1618979, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9111998612", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:40:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19596, - { - "block": 1619008, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9111843695", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:41:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19597, - { - "block": 1619024, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9111757437", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:42:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19598, - { - "block": 1619038, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9111681742", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:43:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19599, - { - "block": 1619075, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9111484590", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:45:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19600, - { - "block": 1619085, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9111430022", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:45:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19601, - { - "block": 1619118, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9111254003", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:47:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19602, - { - "block": 1619124, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9111220560", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:47:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19603, - { - "block": 1619157, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9111046314", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:49:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19604, - { - "block": 1619180, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9110921354", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:50:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19605, - { - "block": 1619185, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9110894954", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:50:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19606, - { - "block": 1619220, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9110706641", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:52:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19607, - { - "block": 1619235, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9110625686", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:53:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19608, - { - "block": 1619265, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9110465541", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:54:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19609, - { - "block": 1619272, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9110428586", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:54:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19610, - { - "block": 1619296, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9110301883", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:56:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19611, - { - "block": 1619311, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9110219176", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:56:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19612, - { - "block": 1619350, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9110011536", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:58:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19613, - { - "block": 1619367, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9109921796", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T17:59:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19614, - { - "block": 1619381, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9109847894", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:00:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19615, - { - "block": 1619403, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9109730006", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:01:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19616, - { - "block": 1619433, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9109562855", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:02:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19617, - { - "block": 1619446, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9109494237", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:03:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19618, - { - "block": 1619475, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9109339411", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:05:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19619, - { - "block": 1619483, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9109295428", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:05:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19620, - { - "block": 1619507, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9109166998", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:06:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19621, - { - "block": 1619530, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9109043849", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:07:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19622, - { - "block": 1619550, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9108938296", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:08:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19623, - { - "block": 1619564, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9108862651", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:09:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19624, - { - "block": 1619598, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9108679702", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:11:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19625, - { - "block": 1619615, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9108588230", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:12:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19626, - { - "block": 1619630, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9108503795", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:12:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19631, - { - "block": 1619663, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9108329655", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:14:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19632, - { - "block": 1619676, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9108257538", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:15:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19633, - { - "block": 1619706, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9108099238", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:16:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19634, - { - "block": 1619724, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9108004260", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:17:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19635, - { - "block": 1619746, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9107886420", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:18:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19636, - { - "block": 1619770, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9107759789", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:19:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19637, - { - "block": 1619788, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9107664818", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:20:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19638, - { - "block": 1619814, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9107524125", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:22:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19639, - { - "block": 1619832, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9107425642", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:22:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19640, - { - "block": 1619836, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9107402780", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:23:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19641, - { - "block": 1619856, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9107295507", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:24:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19642, - { - "block": 1619875, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9107191754", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:25:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19643, - { - "block": 1619889, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9107116139", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:25:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19644, - { - "block": 1619909, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9107008873", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:26:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19645, - { - "block": 1619923, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9106935019", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:27:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19646, - { - "block": 1619961, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9106732806", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:29:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19647, - { - "block": 1619974, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9106664232", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:30:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19648, - { - "block": 1619985, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9106604450", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:30:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19649, - { - "block": 1620008, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9106483130", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:31:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19650, - { - "block": 1620041, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9106303794", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:33:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19651, - { - "block": 1620046, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9106275664", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:33:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19652, - { - "block": 1620068, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9106156111", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:34:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19653, - { - "block": 1620099, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9105992611", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:36:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19654, - { - "block": 1620112, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9105922290", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:36:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19655, - { - "block": 1620133, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9105811536", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:38:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19656, - { - "block": 1620151, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9105716607", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:38:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19657, - { - "block": 1620190, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9105509176", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:40:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19658, - { - "block": 1620208, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9105410738", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:41:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19659, - { - "block": 1620219, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9105350973", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:42:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19660, - { - "block": 1620236, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9105259569", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:43:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19661, - { - "block": 1620263, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9105115435", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:44:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19662, - { - "block": 1620296, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9104937912", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:46:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19663, - { - "block": 1620306, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9104883426", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:46:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19664, - { - "block": 1620330, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9104756880", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:47:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19665, - { - "block": 1620353, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9104635611", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:49:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19666, - { - "block": 1620375, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9104519617", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:50:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19667, - { - "block": 1620386, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9104461621", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:50:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19668, - { - "block": 1620418, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9104291153", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:52:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19669, - { - "block": 1620430, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9104226131", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:52:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19670, - { - "block": 1620454, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9104096089", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:54:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19671, - { - "block": 1620475, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9103983624", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:55:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19672, - { - "block": 1620505, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9103820203", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:56:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19673, - { - "block": 1620517, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9103756945", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:57:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19674, - { - "block": 1620535, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9103662059", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:58:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19675, - { - "block": 1620552, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9103570690", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T18:59:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19676, - { - "block": 1620582, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9103407284", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:00:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19677, - { - "block": 1620594, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9103344032", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:01:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19678, - { - "block": 1620629, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9103157794", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:02:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19679, - { - "block": 1620636, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9103120899", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:03:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19680, - { - "block": 1620668, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9102950482", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:04:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19681, - { - "block": 1620691, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9102827505", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:06:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19682, - { - "block": 1620708, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9102736152", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:06:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19683, - { - "block": 1620728, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9102625478", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:07:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19684, - { - "block": 1620756, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9102476160", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:09:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19685, - { - "block": 1620776, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9102370762", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:10:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19686, - { - "block": 1620783, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9102332117", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:10:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19687, - { - "block": 1620810, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9102186322", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:12:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19688, - { - "block": 1620834, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9102059854", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:13:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19689, - { - "block": 1620864, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9101898261", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:14:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19690, - { - "block": 1620878, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9101824492", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:15:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19691, - { - "block": 1620897, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9101722623", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:16:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19692, - { - "block": 1620926, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9101568067", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:17:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19693, - { - "block": 1620935, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9101520647", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:18:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19694, - { - "block": 1620955, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9101413516", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:19:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19695, - { - "block": 1620985, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9101253703", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:20:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19696, - { - "block": 1621011, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9101114968", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:22:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19697, - { - "block": 1621016, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9101088626", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:22:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19698, - { - "block": 1621047, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9100918288", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:23:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19699, - { - "block": 1621071, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9100790100", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:25:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19700, - { - "block": 1621095, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9100661915", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:26:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19701, - { - "block": 1621104, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9100612749", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:26:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19702, - { - "block": 1621127, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9100488081", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:27:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19703, - { - "block": 1621140, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9100417848", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:28:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19704, - { - "block": 1621177, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9100222954", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:30:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19705, - { - "block": 1621197, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9100115854", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:31:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19706, - { - "block": 1621201, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9100094786", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:31:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19707, - { - "block": 1621228, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9099950820", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:32:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19708, - { - "block": 1621261, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9099771748", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:34:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19709, - { - "block": 1621276, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9099692747", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:35:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19710, - { - "block": 1621290, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9099617259", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:36:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19711, - { - "block": 1621326, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9099422401", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:37:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19712, - { - "block": 1621328, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9099411869", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:37:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19713, - { - "block": 1621364, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9099222285", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:39:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19714, - { - "block": 1621374, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9099169625", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:40:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19715, - { - "block": 1621391, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9099080103", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:41:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19716, - { - "block": 1621426, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9098892289", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:42:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19717, - { - "block": 1621435, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9098841388", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:43:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19718, - { - "block": 1621453, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9098746607", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:44:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19719, - { - "block": 1621485, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9098572848", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:45:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19720, - { - "block": 1621515, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9098413136", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:47:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19721, - { - "block": 1621530, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9098332405", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:48:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19722, - { - "block": 1621557, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9098188496", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:49:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19723, - { - "block": 1621559, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9098177966", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:49:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19724, - { - "block": 1621598, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9097969132", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:51:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19725, - { - "block": 1621610, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9097905957", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:52:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19726, - { - "block": 1621627, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9097812951", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:52:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19727, - { - "block": 1621647, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9097705909", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:53:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19728, - { - "block": 1621678, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9097539210", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:55:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19729, - { - "block": 1621698, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9097432174", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:56:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19730, - { - "block": 1621713, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9097353215", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:57:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19731, - { - "block": 1621728, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9097272503", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:57:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19732, - { - "block": 1621760, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9097102310", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T19:59:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19733, - { - "block": 1621771, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9097044411", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:00:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19734, - { - "block": 1621809, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9096840893", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:01:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19735, - { - "block": 1621810, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9096835630", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:02:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19736, - { - "block": 1621831, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9096725103", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:03:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19737, - { - "block": 1621860, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9096568965", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:04:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19738, - { - "block": 1621874, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9096493530", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:05:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19739, - { - "block": 1621902, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9096344418", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:06:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19740, - { - "block": 1621919, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9096251445", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:07:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19741, - { - "block": 1621939, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9096146194", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:08:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19742, - { - "block": 1621972, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9095970781", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:10:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19743, - { - "block": 1621980, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9095928683", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:10:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19744, - { - "block": 1622005, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9095795375", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:11:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19745, - { - "block": 1622022, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9095704166", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:12:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19746, - { - "block": 1622047, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9095570864", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:13:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19747, - { - "block": 1622064, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9095477906", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:14:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19748, - { - "block": 1622101, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9095281473", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:16:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19749, - { - "block": 1622110, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9095234119", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:17:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19750, - { - "block": 1622136, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9095097324", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:18:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19751, - { - "block": 1622164, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9094948257", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:19:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19752, - { - "block": 1622168, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9094925459", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:19:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19753, - { - "block": 1622190, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9094806210", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:21:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19754, - { - "block": 1622214, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9094676442", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:22:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19755, - { - "block": 1622244, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9094516868", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:23:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19756, - { - "block": 1622267, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9094394122", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:24:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19757, - { - "block": 1622274, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9094357299", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:25:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19758, - { - "block": 1622313, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9094148642", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:27:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19759, - { - "block": 1622320, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9094110067", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:27:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19760, - { - "block": 1622341, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9093999606", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:28:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19761, - { - "block": 1622368, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9093852329", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:29:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19762, - { - "block": 1622390, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9093733109", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:31:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19763, - { - "block": 1622400, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9093680512", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:31:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19764, - { - "block": 1622431, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9093515715", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:33:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19765, - { - "block": 1622455, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9093389490", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:34:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19766, - { - "block": 1622462, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9093350923", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:34:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19767, - { - "block": 1622487, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9093219444", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:35:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19768, - { - "block": 1622507, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9093114264", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:36:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19769, - { - "block": 1622526, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9093014346", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:37:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19770, - { - "block": 1622550, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9092886383", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:39:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19771, - { - "block": 1622571, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9092774199", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:40:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19772, - { - "block": 1622603, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9092604177", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:41:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19773, - { - "block": 1622612, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9092555099", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:42:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19774, - { - "block": 1622632, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9092444677", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:43:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19775, - { - "block": 1622650, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9092350031", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:44:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19776, - { - "block": 1622680, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9092190540", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:45:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19777, - { - "block": 1622711, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9092024045", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:47:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19778, - { - "block": 1622719, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9091981984", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:47:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19779, - { - "block": 1622738, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9091882091", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:48:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19780, - { - "block": 1622775, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9091682311", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:50:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19781, - { - "block": 1622779, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9091661282", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:50:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19782, - { - "block": 1622813, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9091479035", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:52:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19783, - { - "block": 1622825, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9091412447", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:52:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19784, - { - "block": 1622851, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9091274017", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:54:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19785, - { - "block": 1622874, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9091149609", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:55:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19786, - { - "block": 1622892, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9091053239", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:56:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19787, - { - "block": 1622903, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9090995418", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:56:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19788, - { - "block": 1622927, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9090867514", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:57:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19789, - { - "block": 1622951, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9090737861", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T20:59:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19790, - { - "block": 1622972, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9090623980", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:00:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19791, - { - "block": 1622999, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9090478567", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:01:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19792, - { - "block": 1623024, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9090347173", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:02:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19793, - { - "block": 1623042, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9090249069", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:03:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19794, - { - "block": 1623052, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9090196514", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:04:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19795, - { - "block": 1623070, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9090101916", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:05:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19796, - { - "block": 1623097, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9089956520", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:06:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19797, - { - "block": 1623121, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9089830398", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:07:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19798, - { - "block": 1623148, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9089681507", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:08:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19799, - { - "block": 1623158, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9089628959", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:09:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19800, - { - "block": 1623183, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9089497591", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:10:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19801, - { - "block": 1623197, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9089424026", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:11:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19802, - { - "block": 1623227, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9089266392", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:12:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19803, - { - "block": 1623247, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9089157803", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:13:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19804, - { - "block": 1623260, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9089089498", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:14:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19805, - { - "block": 1623280, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9088982664", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:15:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19806, - { - "block": 1623309, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9088830300", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:17:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19807, - { - "block": 1623332, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9088705960", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:18:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19808, - { - "block": 1623351, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9088602638", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:19:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19809, - { - "block": 1623384, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9088429273", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:20:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19810, - { - "block": 1623386, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9088418766", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:20:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19811, - { - "block": 1623410, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9088289184", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:22:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19812, - { - "block": 1623435, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9088156105", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:23:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19813, - { - "block": 1623449, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9088082562", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:24:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19814, - { - "block": 1623477, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9087931979", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:25:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19815, - { - "block": 1623492, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9087851437", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:26:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19816, - { - "block": 1623514, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9087735879", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:27:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19817, - { - "block": 1623548, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9087555544", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:28:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19818, - { - "block": 1623563, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9087476759", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:29:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19819, - { - "block": 1623588, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9087341953", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:30:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19820, - { - "block": 1623602, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9087268424", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:31:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19821, - { - "block": 1623624, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9087152881", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:32:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19822, - { - "block": 1623643, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9087049595", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:33:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19823, - { - "block": 1623658, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9086970819", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:34:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19824, - { - "block": 1623692, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9086792266", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:36:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19825, - { - "block": 1623718, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9086652229", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:37:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19826, - { - "block": 1623731, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9086582212", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:38:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19827, - { - "block": 1623745, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9086503445", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:38:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19828, - { - "block": 1623769, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9086377420", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:40:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19829, - { - "block": 1623797, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9086226894", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:41:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19830, - { - "block": 1623806, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9086176137", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:41:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19831, - { - "block": 1623832, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9086039620", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:43:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19832, - { - "block": 1623847, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9085960862", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:43:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19833, - { - "block": 1623887, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9085745597", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:45:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19834, - { - "block": 1623894, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9085708846", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:46:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19835, - { - "block": 1623927, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9085532093", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:47:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19836, - { - "block": 1623938, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9085472594", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:48:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19837, - { - "block": 1623952, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9085399096", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:49:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19838, - { - "block": 1623975, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9085276602", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:50:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19840, - { - "block": 1623995, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9085168110", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:51:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19841, - { - "block": 1624035, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9084951134", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:53:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19842, - { - "block": 1624044, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9084902141", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:53:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19843, - { - "block": 1624066, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9084786659", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:54:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19844, - { - "block": 1624078, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9084721921", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:55:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19845, - { - "block": 1624104, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9084583698", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:56:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19846, - { - "block": 1624133, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9084429735", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:58:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19847, - { - "block": 1624142, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9084382497", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T21:58:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19848, - { - "block": 1624177, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9084198799", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:00:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19849, - { - "block": 1624185, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9084156812", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:00:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19850, - { - "block": 1624223, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9083952130", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:02:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19851, - { - "block": 1624235, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9083889153", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:03:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19852, - { - "block": 1624253, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9083794690", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:04:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19853, - { - "block": 1624275, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9083675738", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:05:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19854, - { - "block": 1624296, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9083562038", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:06:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19855, - { - "block": 1624325, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9083408109", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:07:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19856, - { - "block": 1624340, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9083327648", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:08:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19857, - { - "block": 1624359, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9083226200", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:09:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19858, - { - "block": 1624377, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9083131750", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:10:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19859, - { - "block": 1624398, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9083018064", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:11:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19860, - { - "block": 1624430, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9082850162", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:13:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19861, - { - "block": 1624435, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9082823928", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:13:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19862, - { - "block": 1624473, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9082621057", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:15:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19863, - { - "block": 1624494, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9082509133", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:16:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19864, - { - "block": 1624512, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9082411201", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:17:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19865, - { - "block": 1624537, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9082280047", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:18:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19866, - { - "block": 1624556, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9082178623", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:19:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19867, - { - "block": 1624579, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9082057967", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:20:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19868, - { - "block": 1624592, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9081989771", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:21:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19869, - { - "block": 1624614, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9081872617", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:22:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19870, - { - "block": 1624637, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9081748473", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:23:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19871, - { - "block": 1624647, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9081694270", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:23:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19872, - { - "block": 1624678, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9081529916", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:25:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19873, - { - "block": 1624695, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9081437251", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:26:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19874, - { - "block": 1624720, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9081302629", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:27:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19875, - { - "block": 1624734, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9081225703", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:28:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19876, - { - "block": 1624760, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9081089339", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:29:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19877, - { - "block": 1624783, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9080968712", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:30:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19878, - { - "block": 1624797, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9080895289", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:31:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19879, - { - "block": 1624821, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9080765927", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:32:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19880, - { - "block": 1624835, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9080692507", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:33:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19881, - { - "block": 1624862, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9080550915", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:34:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19882, - { - "block": 1624880, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9080456522", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:35:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19883, - { - "block": 1624904, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9080325425", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:36:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19884, - { - "block": 1624924, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9080217054", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:37:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19886, - { - "block": 1624945, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9080106938", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:38:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19887, - { - "block": 1624977, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9079935652", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:40:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19888, - { - "block": 1624983, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9079904192", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:40:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19889, - { - "block": 1625019, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9079713689", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:42:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19890, - { - "block": 1625031, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9079649025", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:43:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19893, - { - "block": 1625053, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9079530185", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:44:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19894, - { - "block": 1625079, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9079393873", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:45:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19895, - { - "block": 1625102, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9079268049", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:46:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19896, - { - "block": 1625120, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9079173684", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:47:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19897, - { - "block": 1625148, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9079026898", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:49:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19898, - { - "block": 1625166, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9078930791", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:49:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19899, - { - "block": 1625180, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9078855653", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:50:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19900, - { - "block": 1625207, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9078712372", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:51:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19901, - { - "block": 1625221, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9078637239", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:52:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19902, - { - "block": 1625241, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9078528909", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:53:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19903, - { - "block": 1625258, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9078439802", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:54:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19904, - { - "block": 1625290, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9078272074", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:56:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19905, - { - "block": 1625315, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9078141041", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:57:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19906, - { - "block": 1625327, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9078076400", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:57:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19907, - { - "block": 1625356, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9077919168", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:59:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19908, - { - "block": 1625360, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9077898204", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T22:59:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19909, - { - "block": 1625399, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9077688571", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:01:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19910, - { - "block": 1625413, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9077613455", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:02:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19911, - { - "block": 1625439, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9077477201", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:03:33", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19912, - { - "block": 1625446, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9077438771", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:03:54", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19913, - { - "block": 1625467, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9077328724", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:04:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19914, - { - "block": 1625491, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9077199465", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:06:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19915, - { - "block": 1625523, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9077028291", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:07:45", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19916, - { - "block": 1625540, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9076935720", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:08:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19917, - { - "block": 1625560, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9076827432", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:09:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19918, - { - "block": 1625581, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9076717400", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:10:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19919, - { - "block": 1625607, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9076579427", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:12:00", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19920, - { - "block": 1625620, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9076511315", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:12:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19921, - { - "block": 1625634, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9076436219", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:13:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19922, - { - "block": 1625655, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9076326197", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:14:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19923, - { - "block": 1625681, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9076189982", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:15:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19924, - { - "block": 1625700, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9076086951", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:16:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19925, - { - "block": 1625730, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9075926297", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:18:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19926, - { - "block": 1625749, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9075825018", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:19:06", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19927, - { - "block": 1625777, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9075676596", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:20:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19928, - { - "block": 1625783, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9075643420", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:20:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19930, - { - "block": 1625819, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9075454845", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:22:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19931, - { - "block": 1625834, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9075374529", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:23:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19932, - { - "block": 1625846, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9075309927", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:23:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19933, - { - "block": 1625883, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9075112637", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:25:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19934, - { - "block": 1625886, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9075096924", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:25:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19935, - { - "block": 1625919, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9074920594", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:27:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19936, - { - "block": 1625930, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9074862982", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:28:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19937, - { - "block": 1625956, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9074723321", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:29:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19938, - { - "block": 1625986, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9074562716", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:30:57", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19939, - { - "block": 1625996, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9074508600", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:31:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19940, - { - "block": 1626017, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9074398626", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:32:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19944, - { - "block": 1626034, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9074309601", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:33:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19959, - { - "block": 1626070, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9074119337", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:35:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19966, - { - "block": 1626091, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9074009372", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:36:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19970, - { - "block": 1626110, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9073904646", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:37:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19975, - { - "block": 1626131, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9073794686", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:38:12", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19976, - { - "block": 1626139, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9073752797", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:38:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19977, - { - "block": 1626173, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9073569537", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:40:18", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19978, - { - "block": 1626196, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9073443877", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:41:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19979, - { - "block": 1626208, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9073377558", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:42:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19980, - { - "block": 1626228, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9073272845", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:43:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19981, - { - "block": 1626253, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9073138468", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:44:21", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19984, - { - "block": 1626279, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9073002349", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:45:39", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19985, - { - "block": 1626300, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9072892411", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:46:42", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19986, - { - "block": 1626323, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9072770260", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:47:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19987, - { - "block": 1626342, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9072670796", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:48:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19988, - { - "block": 1626358, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9072587040", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:49:36", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19989, - { - "block": 1626383, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9072452683", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:50:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19990, - { - "block": 1626407, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9072325309", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:52:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19991, - { - "block": 1626411, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9072302627", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:52:15", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19992, - { - "block": 1626436, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9072168279", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:53:30", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19993, - { - "block": 1626469, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9071993807", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:55:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19994, - { - "block": 1626483, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9071920531", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:55:51", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19995, - { - "block": 1626502, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9071817597", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:56:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19996, - { - "block": 1626527, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9071683264", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:58:03", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19997, - { - "block": 1626555, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9071533234", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-20T23:59:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19998, - { - "block": 1626575, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9071425076", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-21T00:00:27", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 19999, - { - "block": 1626589, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9071351809", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-21T00:01:09", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ], - [ - 20000, - { - "block": 1626602, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "blocktrades", - "vesting_shares": { - "amount": "9071283776", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-05-21T00:01:48", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/producer_reward_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/producer_reward_operation.tavern.yaml deleted file mode 100644 index 58a441a18d1c660e60dbda6381e672871b935e11..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/producer_reward_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api producer_reward_operation - - marks: - - patterntest # virtual - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api producer_reward_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 4611686018427387904, "start": 20000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "producer_reward_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/proposal_pay_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/proposal_pay_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/proposal_pay_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/proposal_pay_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/proposal_pay_operation.tavern.yaml deleted file mode 100644 index a6ae19a5166955e2bbcbf9830b0e1d6785b08660..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/proposal_pay_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api proposal_pay_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api proposal_pay_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_high": 1, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "proposal_pay_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/recover_account_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/recover_account_operation.pat.json deleted file mode 100644 index bb4a44ff08e6086eec2b33fbc81df1ea0fcc8526..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/recover_account_operation.pat.json +++ /dev/null @@ -1,43 +0,0 @@ -{ - "history": [ - [ - 376, - { - "block": 3399202, - "op": { - "type": "recover_account_operation", - "value": { - "account_to_recover": "gtg", - "extensions": [], - "new_owner_authority": { - "account_auths": [], - "key_auths": [ - [ - "STM5RLQ1Jh8Kf56go3xpzoodg4vRsgCeWhANXoEXrYH7bLEwSVyjh", - 1 - ] - ], - "weight_threshold": 1 - }, - "recent_owner_authority": { - "account_auths": [], - "key_auths": [ - [ - "STM5F9tCbND6zWPwksy1rEN24WjPiQWSU2vwGgegQVjAcYDe1zTWi", - 1 - ] - ], - "weight_threshold": 1 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-21T21:48:03", - "trx_id": "80b4c722e31afc093ef0aeeb3e447eae224908a8", - "trx_in_block": 6, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/recover_account_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/recover_account_operation.tavern.yaml deleted file mode 100644 index cae80fc41b75ca06d14f0512b2300515496773e9..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/recover_account_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api recover_account_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api recover_account_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"gtg", "operation_filter_low": 33554432, "start": 376, "limit": 1} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "recover_account_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/remove_proposal_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/remove_proposal_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/remove_proposal_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/remove_proposal_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/remove_proposal_operation.tavern.yaml deleted file mode 100644 index 85c581a1020cfafc3972a1d4ea7e2b98580922f6..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/remove_proposal_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api remove_proposal_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api remove_proposal_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 70368744177664, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "remove_proposal_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/report_over_production_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/report_over_production_operation.pat.json deleted file mode 100644 index 4002a4b7524d6948f3d27d1941627f5b69c2b7ce..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/report_over_production_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/report_over_production_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/report_over_production_operation.tavern.yaml deleted file mode 100644 index c37333498650a654b95128eac92edf1e45a5beab..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/report_over_production_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to report_over_production_operation - - marks: - - patterntest # DEPRECATED since HF4 - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history report_over_production_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"bytemaster","operation_filter_low": 65536,"start": 8000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "report_over_production_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/request_account_recovery_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/request_account_recovery_operation.pat.json deleted file mode 100644 index 67a47fab57f9fafc1d3e3dc559c93256cc668928..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/request_account_recovery_operation.pat.json +++ /dev/null @@ -1,64 +0,0 @@ -{ - "history": [ - [ - 374, - { - "block": 3399063, - "op": { - "type": "request_account_recovery_operation", - "value": { - "account_to_recover": "gtg", - "extensions": [], - "new_owner_authority": { - "account_auths": [], - "key_auths": [ - [ - "STM8bmDfubWHsCjs9LzPNCQEGevk3iJGRDHH1Mvsc2kboTg6ZT6YM", - 1 - ] - ], - "weight_threshold": 1 - }, - "recovery_account": "steem" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-21T21:41:00", - "trx_id": "f0ffadc77bb4e089e0d12ae754f9690144c71edc", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 375, - { - "block": 3399201, - "op": { - "type": "request_account_recovery_operation", - "value": { - "account_to_recover": "gtg", - "extensions": [], - "new_owner_authority": { - "account_auths": [], - "key_auths": [ - [ - "STM5RLQ1Jh8Kf56go3xpzoodg4vRsgCeWhANXoEXrYH7bLEwSVyjh", - 1 - ] - ], - "weight_threshold": 1 - }, - "recovery_account": "steem" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-21T21:48:00", - "trx_id": "4292eb5939be2918552c08b8be0adc23ac939002", - "trx_in_block": 2, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/request_account_recovery_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/request_account_recovery_operation.tavern.yaml deleted file mode 100644 index db5ed56b1a5f30743d829a8423d5a46fdffa2bd2..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/request_account_recovery_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to request_account_recovery_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history request_account_recovery_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"gtg","operation_filter_low": 16777216,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "request_account_recovery_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/reset_account_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/reset_account_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/reset_account_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/reset_account_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/reset_account_operation.tavern.yaml deleted file mode 100644 index 41ccb9453db36c355784b2a64a8d9db6ba89228b..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/reset_account_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api reset_account_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api reset_account_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 137438953472, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "reset_account_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/return_vesting_delegation_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/return_vesting_delegation_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/return_vesting_delegation_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/return_vesting_delegation_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/return_vesting_delegation_operation.tavern.yaml deleted file mode 100644 index a164b04685dc14ea58c9f37dc8130e22873ca82d..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/return_vesting_delegation_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api return_vesting_delegation_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api return_vesting_delegation_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 1152921504606846976, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "return_vesting_delegation_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/set_reset_account_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/set_reset_account_operation.pat.json deleted file mode 100644 index b1faf6a1b4e9cad9ebc2f8c8bfe3e34ea6c768ed..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/set_reset_account_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/set_reset_account_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/set_reset_account_operation.tavern.yaml deleted file mode 100644 index 73d48c192dc21d1d7e01a96d472f249a478a3f82..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/set_reset_account_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api set_reset_account_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api set_reset_account_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 274877906944, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "set_reset_account_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/set_withdraw_vesting_route_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/set_withdraw_vesting_route_operation.pat.json deleted file mode 100644 index 8b864f8b5de046ac75951f7b0f17b8975aed0c24..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/set_withdraw_vesting_route_operation.pat.json +++ /dev/null @@ -1,172 +0,0 @@ -{ - "history": [ - [ - 229, - { - "block": 3155225, - "op": { - "type": "set_withdraw_vesting_route_operation", - "value": { - "auto_vest": false, - "from_account": "aizen24", - "percent": 100, - "to_account": "aizensou" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-13T07:13:27", - "trx_id": "8e8b13ea0622b5255aea2b011f0605c38ad9977a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 233, - { - "block": 3339107, - "op": { - "type": "set_withdraw_vesting_route_operation", - "value": { - "auto_vest": false, - "from_account": "aizen24", - "percent": 100, - "to_account": "bento" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-19T19:11:06", - "trx_id": "a5a23d669949b10f446414e326f4f54554dcf950", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 237, - { - "block": 3354142, - "op": { - "type": "set_withdraw_vesting_route_operation", - "value": { - "auto_vest": false, - "from_account": "aizen24", - "percent": 1, - "to_account": "aizensou" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-20T07:50:21", - "trx_id": "cfdfecd2a67ed091a8fad56b2e13c34f2dcd8fc8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 240, - { - "block": 3354503, - "op": { - "type": "set_withdraw_vesting_route_operation", - "value": { - "auto_vest": false, - "from_account": "aizen24", - "percent": 0, - "to_account": "aizensou" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-20T08:08:33", - "trx_id": "e11af21721952cc0d1b7dfcb03cfad7292f86586", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 241, - { - "block": 3354601, - "op": { - "type": "set_withdraw_vesting_route_operation", - "value": { - "auto_vest": false, - "from_account": "aizen24", - "percent": 10000, - "to_account": "bento" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-20T08:13:33", - "trx_id": "430f31ea1cf468feb9398ee4ffc502a62eb3fc03", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 242, - { - "block": 3389889, - "op": { - "type": "set_withdraw_vesting_route_operation", - "value": { - "auto_vest": false, - "from_account": "aizen24", - "percent": 0, - "to_account": "bento" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-21T13:55:54", - "trx_id": "0d23491cfcc3d0e79d3e3a474c15dd18e050ad84", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 243, - { - "block": 3389890, - "op": { - "type": "set_withdraw_vesting_route_operation", - "value": { - "auto_vest": false, - "from_account": "aizen24", - "percent": 10000, - "to_account": "aizensou" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-21T13:55:57", - "trx_id": "e45e2c2ec28ad63dca4a30e07a51a67e5e5a0daf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 251, - { - "block": 3758898, - "op": { - "type": "set_withdraw_vesting_route_operation", - "value": { - "auto_vest": false, - "from_account": "aizen24", - "percent": 10000, - "to_account": "aizensou" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-03T11:54:33", - "trx_id": "e16da854a387b2284cf645426d841d567ea9aaa2", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/set_withdraw_vesting_route_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/set_withdraw_vesting_route_operation.tavern.yaml deleted file mode 100644 index e30226573e179f1ae93ee6927049819199172217..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/set_withdraw_vesting_route_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to set_withdraw_vesting_route_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history set_withdraw_vesting_route_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"aizen24","operation_filter_low": 1048576,"start": 251,"limit": 8} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "set_withdraw_vesting_route_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/shutdown_witness_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/shutdown_witness_operation.pat.json deleted file mode 100644 index e78acb4373cbaceac8d71cb4cca22f2ad6d003b1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/shutdown_witness_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/shutdown_witness_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/shutdown_witness_operation.tavern.yaml deleted file mode 100644 index a9d265fd2a2e5d1ab6b6fb37f66a5c4413e9280e..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/shutdown_witness_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api shutdown_witness_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api shutdown_witness_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 72057594037927936, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "shutdown_witness_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_contribute_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/smt_contribute_operation.pat.json deleted file mode 100644 index e78acb4373cbaceac8d71cb4cca22f2ad6d003b1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_contribute_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_contribute_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/smt_contribute_operation.tavern.yaml deleted file mode 100644 index 38779aa9c51bc88591d789b1e94f4004d5c43639..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_contribute_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api smt_contribute_operation - - marks: - - patterntest # no noempty result in 5mln blocks - # only if HIVE_ENABLE_SMT - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api smt_contribute_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 18014398509481984, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "smt_contribute_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_create_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/smt_create_operation.pat.json deleted file mode 100644 index e78acb4373cbaceac8d71cb4cca22f2ad6d003b1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_create_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_create_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/smt_create_operation.tavern.yaml deleted file mode 100644 index c95a33120a0877a69de4ed8923d348016e515b63..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_create_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api smt_create_operation - - marks: - - patterntest # no noempty result in 5mln blocks - # only if HIVE_ENABLE_SMT - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api smt_create_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 9007199254740992, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "smt_create_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_set_runtime_parameters_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/smt_set_runtime_parameters_operation.pat.json deleted file mode 100644 index e78acb4373cbaceac8d71cb4cca22f2ad6d003b1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_set_runtime_parameters_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_set_runtime_parameters_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/smt_set_runtime_parameters_operation.tavern.yaml deleted file mode 100644 index d6d24b904135e4f1c60d2860cedae9fed63c1683..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_set_runtime_parameters_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api smt_set_runtime_parameters_operation - - marks: - - patterntest # virtual - # only if HIVE_ENABLE_SMT - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api smt_set_runtime_parameters_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 4503599627370496, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "smt_set_runtime_parameters_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_set_setup_parameters_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/smt_set_setup_parameters_operation.pat.json deleted file mode 100644 index e78acb4373cbaceac8d71cb4cca22f2ad6d003b1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_set_setup_parameters_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_set_setup_parameters_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/smt_set_setup_parameters_operation.tavern.yaml deleted file mode 100644 index cd8cf413f6b86b8dcf98bae99b87400ac5fba8f8..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_set_setup_parameters_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api smt_set_setup_parameters_operation - - marks: - - patterntest # no noempty result in 5mln blocks - # only if HIVE_ENABLE_SMT - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api smt_set_setup_parameters_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 2251799813685248, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "smt_set_setup_parameters_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_emissions_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_emissions_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_emissions_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_emissions_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_emissions_operation.tavern.yaml deleted file mode 100644 index 4798ea04b8f441effe6cdf65529128bfb3e5a8ae..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_emissions_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api smt_setup_emissions_operation - - marks: - - patterntest # no noempty result in 5mln blocks - # only if HIVE_ENABLE_SMT - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api smt_setup_emissions_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 1125899906842624, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "smt_setup_emissions_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_operation.tavern.yaml deleted file mode 100644 index ebb8a10cd365493ccb6e55a0c85eb646a6c15ee0..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/smt_setup_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api smt_setup_operation - - marks: - - patterntest # no noempty result in 5mln blocks - # only if HIVE_ENABLE_SMT - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api smt_setup_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 562949953421312, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "smt_setup_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/sps_convert_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/sps_convert_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/sps_convert_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/sps_convert_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/sps_convert_operation.tavern.yaml deleted file mode 100644 index c40be67e543ede004bb5918880adb53dbb83908a..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/sps_convert_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api sps_convert_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api sps_convert_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_high": 256, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "sps_convert_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/sps_fund_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/sps_fund_operation.pat.json deleted file mode 100644 index 2dd829cfb142b6af3ea7348b18a1b6453a5bb896..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/sps_fund_operation.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "history": [] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/sps_fund_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/sps_fund_operation.tavern.yaml deleted file mode 100644 index 67ce99d35d267132be7a016d761d4ca1b4ee591f..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/sps_fund_operation.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api sps_fund_operation - - marks: - - patterntest # virtual - # no noempty results - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api sps_fund_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_high": 2, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "sps_fund_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/transfer_from_savings_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/transfer_from_savings_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/transfer_from_savings_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/transfer_from_savings_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/transfer_from_savings_operation.tavern.yaml deleted file mode 100644 index bade5efc07d5dd7d89bb07d0c7dc1c76313cf876..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/transfer_from_savings_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api transfer_from_savings_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api transfer_from_savings_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 8589934592, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "transfer_from_savings_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/transfer_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/transfer_operation.pat.json deleted file mode 100644 index 83ac41521540fb0c4d6b179ac26dbb4c3ea08e66..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/transfer_operation.pat.json +++ /dev/null @@ -1,404 +0,0 @@ -{ - "history": [ - [ - 205366, - { - "block": 4985503, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "48000", - "nai": "@@000000013", - "precision": 3 - }, - "from": "aaronkoenig", - "memo": "b4c499fb-5deb-44dc-9646-e7feb3927e12", - "to": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T07:33:57", - "trx_id": "2c918ba666c06946a792657b27ed18af196dfab5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205422, - { - "block": 4986680, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "126225", - "nai": "@@000000021", - "precision": 3 - }, - "from": "schro", - "memo": "d70dfb8e-277a-4070-9812-b38cf01579cf", - "to": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T08:32:51", - "trx_id": "68656136a5cff365a0c5ab873a60a3cf7528e47c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205435, - { - "block": 4986959, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "1059", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "memo": "", - "to": "royalmacro" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T08:46:57", - "trx_id": "7ec30a784f3c0f2eea3305a9530369811d5d9f2d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205539, - { - "block": 4989287, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "52804", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "memo": "", - "to": "bapparabi" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T10:44:00", - "trx_id": "a47552c9b50cfdf8d77dde55aba32b4a2a64d78a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205729, - { - "block": 4992808, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "203445", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "memo": "", - "to": "franciscusb" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T13:40:18", - "trx_id": "2b46ad6609fe91f034b662179f903eb9ae4a930d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 205741, - { - "block": 4993049, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "812190", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "memo": "", - "to": "franciscusb" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T13:52:21", - "trx_id": "3bc4523687ac895e97e185f65f3e5cfaa3c37539", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 205746, - { - "block": 4993122, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "24838", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blackjincrypto", - "memo": "7639138f-62f0-492a-b8e7-bad1add506dd", - "to": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T13:56:00", - "trx_id": "a6e9f45a9b8fb6cd565936f64e13ae5b12ac94f2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205754, - { - "block": 4993287, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "64", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "memo": "", - "to": "deviedev" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T14:04:15", - "trx_id": "85759c4bf28f20bdb7a43f6b506b8f97a8f23cdd", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 205827, - { - "block": 4994642, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "1500000", - "nai": "@@000000013", - "precision": 3 - }, - "from": "good-karma", - "memo": "0c9ca5aa-53c2-445e-912f-d2cf6b111229", - "to": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T15:12:03", - "trx_id": "15a50977270c3b7e4e97715989f03099375b59bb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205834, - { - "block": 4994778, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "50000", - "nai": "@@000000013", - "precision": 3 - }, - "from": "macksby", - "memo": "92bff29e-2ee9-4ea4-ace1-6bb0c1331f67", - "to": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T15:18:51", - "trx_id": "f2c59d933ba1fc08bd6f4c79ec52a0aeed6bb9e9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205909, - { - "block": 4996091, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "148056", - "nai": "@@000000021", - "precision": 3 - }, - "from": "roelandp", - "memo": "298eafac-95bb-4f27-b256-72df9d0ef222", - "to": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T16:24:33", - "trx_id": "b1aa3d5f9308acd9cde664936c1b08eea77c3e49", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 205920, - { - "block": 4996294, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "431587", - "nai": "@@000000013", - "precision": 3 - }, - "from": "thisisbenbrick", - "memo": "0722aa82-10d0-4001-a286-130b633d58df", - "to": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T16:34:42", - "trx_id": "01de85e061adde226a7ce59071973c9daaeca8ba", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205956, - { - "block": 4996880, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "900000", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "memo": "#5JqZrt878ZgW7qKE3Ss3yL8vbxa7kxjVixJjv3oUM9qFQQGxJ9qed6iW8PdqDszU339rZnLudpuZjfBDYWsiYBY1dYoVhKPSic9QH4tjMCdfwzGhQ1RFi755VLqc4voVoBFvhSH3vKKk6xPsaHdoXTABVb1HCncLj348yGh4UVUSV", - "to": "neptun" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:04:00", - "trx_id": "872da4c5c93290bc17384752ce14a231ee50d42c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 206068, - { - "block": 4998632, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "11028", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "memo": "", - "to": "nmeofthestate" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:35:06", - "trx_id": "4edcd2b5b51e7753c07852b8e1cf8824b424ae5e", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 206129, - { - "block": 4999607, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "400000", - "nai": "@@000000013", - "precision": 3 - }, - "from": "condra", - "memo": "f2c3419c-9522-4bb3-aa41-472c8388f215", - "to": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T19:26:39", - "trx_id": "2577322e21a18f9085cc5dba366b5292824f906b", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 206155, - { - "block": 4999997, - "op": { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "1633", - "nai": "@@000000013", - "precision": 3 - }, - "from": "mrwang", - "memo": "a79c09cd-0084-4cd4-ae63-bf6d2514fef9", - "to": "blocktrades" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T19:47:09", - "trx_id": "e75f833ceb62570c25504b55d0f23d86d9d76423", - "trx_in_block": 3, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/transfer_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/transfer_operation.tavern.yaml deleted file mode 100644 index db5dc48fcb73046fc154833b390e58f82a4b8bdf..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/transfer_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to transfer_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history transfer_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades","operation_filter_low": 4, "start": 206155, "limit": 16} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "transfer_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_savings_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_savings_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_savings_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_savings_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_savings_operation.tavern.yaml deleted file mode 100644 index a05a8a44a9eb2d19ede2b5f7e3391c54b62fc9b0..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_savings_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api transfer_to_savings_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api transfer_to_savings_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 4294967296, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "transfer_to_savings_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_vesting_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_vesting_operation.pat.json deleted file mode 100644 index 9dcb80666fd82d041d803e615fced5eb7b4e578d..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_vesting_operation.pat.json +++ /dev/null @@ -1,436 +0,0 @@ -{ - "history": [ - [ - 205617, - { - "block": 4990714, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "107608", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "bapparabi" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T11:55:24", - "trx_id": "cd829483d565b8e2dc8b2590f9ff204ddb20d268", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205639, - { - "block": 4991161, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "634", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "steevc" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:17:45", - "trx_id": "a5c40f340f700d3efd44f4d7a99ed85f47d4e34d", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 205755, - { - "block": 4993288, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "1807", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "triverse" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T14:04:18", - "trx_id": "532af24e4a6db1ecf4c0322f9517e488bce7b311", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 205789, - { - "block": 4993870, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "105767", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "thylbom" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T14:33:24", - "trx_id": "0c2f1569805f4b7d366359531dec53851fd539b2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 205842, - { - "block": 4994915, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "9709", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "steevc" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T15:25:42", - "trx_id": "6503c5fde49adb05120ab09a64809a5f90179210", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205864, - { - "block": 4995353, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "12643", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "tonypeacock" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T15:47:36", - "trx_id": "5c085a7e41ae47e46ef3fdf5770d782b7481e7b0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205874, - { - "block": 4995543, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "3842", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "fernando-sanz" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T15:57:06", - "trx_id": "1364248a43f31f5db0a48bf0cd4b3280c2a7dca6", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 205880, - { - "block": 4995617, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "2108", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "triverse" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T16:00:48", - "trx_id": "cfaae7888f2529f0fb8aadb046d53a0b9bf241eb", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 205946, - { - "block": 4996719, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "530671", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "exploretraveler" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T16:55:57", - "trx_id": "af49f5572949aa9d30efbdc253cd7f7a07ff48ee", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 205977, - { - "block": 4997220, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "2618", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "derekareith" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:21:00", - "trx_id": "b5f4e96b917ddb334eb474bb99f29205482e962c", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 205978, - { - "block": 4997220, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "1173", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "inti" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:21:00", - "trx_id": "85cc0cb17ac0964426e7a684471a27ecc4c65141", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 205987, - { - "block": 4997368, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "946", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "craig-grant" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:28:24", - "trx_id": "f4e33dffa04b7eaf0e1513dc3f6851b0824566db", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205995, - { - "block": 4997495, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "221", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "inti" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:35:00", - "trx_id": "a356c3ab779bc00d40c9317dc0f6b18f053a7279", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 206004, - { - "block": 4997665, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "386", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "kendewitt" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:43:54", - "trx_id": "0449e0b305d190e1b421c72c5ace7dcf6fd9dd0c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 206011, - { - "block": 4997788, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "292", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "rockymtnbarkeep" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:50:21", - "trx_id": "6b07599f4c96e2359dd49aa03adbd8f06713ac2e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 206013, - { - "block": 4997818, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "2073", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "craig-grant" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:51:54", - "trx_id": "13b37832b28fab7577ebd0e1d585d5f6f31fd721", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 206043, - { - "block": 4998371, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "5509", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "peacekeeper" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:21:00", - "trx_id": "0385ecdbe550dda032130f7bbb78a691287785e3", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 206067, - { - "block": 4998632, - "op": { - "type": "transfer_to_vesting_operation", - "value": { - "amount": { - "amount": "172434", - "nai": "@@000000021", - "precision": 3 - }, - "from": "blocktrades", - "to": "peacekeeper" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:35:06", - "trx_id": "c634ffa4f29fbd66078d0969b19bc96f70663e9e", - "trx_in_block": 1, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_vesting_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_vesting_operation.tavern.yaml deleted file mode 100644 index 248349d29eebf3910032e8b15d251f2210c0d08b..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/transfer_to_vesting_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to transfer_to_vesting_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history transfer_to_vesting_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades","operation_filter_low": 8, "start": 206067, "limit": 18} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "transfer_to_vesting_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_operation.tavern.yaml deleted file mode 100644 index e2956f76fe08676f4c0db5a9afcfe2af5387fff4..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api update_proposal_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api update_proposal_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 140737488355328, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "update_proposal_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_votes_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_votes_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_votes_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_votes_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_votes_operation.tavern.yaml deleted file mode 100644 index 4c279f76c46a4de23f7d85ab23a4c39880e2d8af..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/update_proposal_votes_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api update_proposal_votes_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api update_proposal_votes_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 35184372090000, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "update_proposal_votes_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/vote_and_comment_ops.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/vote_and_comment_ops.pat.json deleted file mode 100644 index 7bbfd1d21142afc8cacddad77129fd38c6e56199..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/vote_and_comment_ops.pat.json +++ /dev/null @@ -1,6124 +0,0 @@ -{ - "history": [ - [ - 3, - { - "block": 2797050, - "op": { - "type": "vote_operation", - "value": { - "author": "pfunk", - "permlink": "guide-maximize-your-mining-hashrate-in-windows-by-mining-steem-in-a-vm", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:12:21", - "trx_id": "e0cd24f9aba3b668b1443786bee3891f644ff23f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 5, - { - "block": 2797188, - "op": { - "type": "vote_operation", - "value": { - "author": "pfunk", - "permlink": "security-b-sides-msp-2016-information-security-conference-day-1-opening-keynote-notes-and-recap", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:19:15", - "trx_id": "be93d0b153a5b9a12547180a1d88f2aad1669754", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 7, - { - "block": 2797362, - "op": { - "type": "vote_operation", - "value": { - "author": "pfunk", - "permlink": "lets-discuss-verification-of-user-accounts-posting-previous-work-to-prevent-impersonation", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:27:57", - "trx_id": "c7a8b59428f96adc3245345bd4fd7c587aad3383", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9, - { - "block": 2797645, - "op": { - "type": "vote_operation", - "value": { - "author": "zajac", - "permlink": "hi-steemit-people-lets-waste-some-time-together-", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:42:09", - "trx_id": "f98b305720148c4b37b19c1c7616ad9057e46c32", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 11, - { - "block": 2812987, - "op": { - "type": "vote_operation", - "value": { - "author": "cylonmaker2053", - "permlink": "steemit-infrastructure-security-scalability-and-points-of-failure", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-01T08:30:48", - "trx_id": "0b42bdd9a3961b740dc5f32857b645b1220e95d7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 13, - { - "block": 2813087, - "op": { - "type": "vote_operation", - "value": { - "author": "arhag", - "permlink": "analysis-of-curation-reward-difference-between-v0-8-2-and-v0-8-3", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-01T08:35:54", - "trx_id": "6d7e3ecaaab997f1a0624136a8b7eed6e78ee34f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 15, - { - "block": 2813732, - "op": { - "type": "vote_operation", - "value": { - "author": "inboundinken", - "permlink": "how-seo-is-changing-and-why-communities-like-steem-will-have-an-impact-on-online-search", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-01T09:08:15", - "trx_id": "948748044cb59768e787667faa646cc11ed5bac3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 17, - { - "block": 2813782, - "op": { - "type": "vote_operation", - "value": { - "author": "steemitblog", - "permlink": "overhaul-of-curation-rewards", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-01T09:10:45", - "trx_id": "e0d90e39c0380bf71d4dde45c3a44aad286de5b1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 19, - { - "block": 2819817, - "op": { - "type": "vote_operation", - "value": { - "author": "kingscrown", - "permlink": "superb-interview-with-dan-from-blocktrades", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-01T14:12:30", - "trx_id": "c35193ed63aa3b33e29e4f9a7e289eef1c9760ba", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 21, - { - "block": 2820033, - "op": { - "type": "vote_operation", - "value": { - "author": "dan", - "permlink": "draft-steem-constitution", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-01T14:23:21", - "trx_id": "6af66a49af72cde348209ca136dda5171b9b7364", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 23, - { - "block": 2827089, - "op": { - "type": "vote_operation", - "value": { - "author": "cryptogee", - "permlink": "how-to-increase-the-value-of-steem-the-ultimate-july-4th-strategy", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-01T20:16:36", - "trx_id": "ceb86a90d5e73e4e45e7711347ecc8bf674a2cbd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 25, - { - "block": 2839669, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "blocktrades-supports-buyingselling-dao-coins-without-exchange-risk", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T06:47:42", - "trx_id": "2b79214e07cffd28c8ee026055d92767229d8b45", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 27, - { - "block": 2839669, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "dao-gateway-on-openledger-is-fully-operational", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T06:47:42", - "trx_id": "c02b46ca3eced3c6bd5cc43df2114143ce6c2105", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 29, - { - "block": 2839884, - "op": { - "type": "vote_operation", - "value": { - "author": "complexring", - "permlink": "cryptography-101-an-interactive-class-an-introduction-to-group-theory--week-1", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T06:58:27", - "trx_id": "c6c79616137a833f7d352d4bd8c6e132a9b779a7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 31, - { - "block": 2840585, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "blocktrades-now-offering-steem-power-for-cryptocurrency", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T07:33:33", - "trx_id": "8726774b3c8d53e9dcb870db6b3561a217871d27", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 33, - { - "block": 2840590, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "i-find-steemit-posts-with-summariesanalysis-of-3rd-party-content-more-compelling", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T07:33:48", - "trx_id": "e909cd27baf117473ec99ec9b875a6916927c55e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 35, - { - "block": 2840591, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-part-1-capital-gainslosses-for-crypto", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T07:33:51", - "trx_id": "9174e0f692023ecf91d632f9949a94c808302119", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 37, - { - "block": 2840592, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "tax-issues-facing-us-based-cryptocurrency-holders-and-miners-intro-and-irs-guidelines", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T07:33:54", - "trx_id": "88d2f81c37e1288448b334042c3eee02df6395d9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 39, - { - "block": 2840592, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "is-vote-changing-an-important-feature-for-steem", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T07:33:54", - "trx_id": "4fdfc9bcbc84c0b7d798cf3f0c606269043f14ff", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 41, - { - "block": 2840592, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "openledger-pre-sale-of-dao-tokens-is-now-live", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T07:33:54", - "trx_id": "5179c7745a8a8e729cf8faab0461e97bc1219ba3", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 43, - { - "block": 2840599, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "-blocktrades-adds-support-for-directly-buyingselling-steem", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T07:34:15", - "trx_id": "2bf9b0850282ed3867d9a2470dc8d6af55aa4ea4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 45, - { - "block": 2840637, - "op": { - "type": "vote_operation", - "value": { - "author": "complexring", - "permlink": "cryptography-101-an-interactive-class-more-introduction-to-groups-week-2", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T07:36:09", - "trx_id": "85bb532b9fc5dc4b1d2661a54818958d1e99e08e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 47, - { - "block": 2852954, - "op": { - "type": "vote_operation", - "value": { - "author": "garycovington", - "permlink": "journey-to-black-belt", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T17:52:30", - "trx_id": "3d5e58dbb4517ca64aba4db4d8cc510d9711fd4e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 49, - { - "block": 2856501, - "op": { - "type": "vote_operation", - "value": { - "author": "darya-zaytseva", - "permlink": "greetings-from-between-poland-and-russia-or-the-story-of-scaner", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T20:50:12", - "trx_id": "d88e3a5ab2bd89a069cb625299cc00ab4fd4a708", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 52, - { - "block": 2858870, - "op": { - "type": "vote_operation", - "value": { - "author": "complexring", - "permlink": "what-is-chaos-theory", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-02T22:49:06", - "trx_id": "b2d83887e899dec9cb05073de25bf7e5d1b8bb69", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 57, - { - "block": 2873202, - "op": { - "type": "vote_operation", - "value": { - "author": "elishagh1", - "permlink": "hello-from-ghana-africa", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T10:47:18", - "trx_id": "52640e305b31c83b2c9209655edfa62ac4048caf", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 59, - { - "block": 2873934, - "op": { - "type": "vote_operation", - "value": { - "author": "pfunk", - "permlink": "a-full-steemit-user-s-guide-to-steem-witnesses", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T11:24:27", - "trx_id": "e08fdf2924089fa7c57e188d942b04c4fb0b2566", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 61, - { - "block": 2875453, - "op": { - "type": "vote_operation", - "value": { - "author": "lucasb", - "permlink": "hi-all-i-m-lucas", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T12:40:30", - "trx_id": "b734c3d11989c4a7f9d8213471c50cec27df4596", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 63, - { - "block": 2876936, - "op": { - "type": "vote_operation", - "value": { - "author": "liondani", - "permlink": "hi-liondani-here-aka-daniel-schwarz-happy-husband-father-steem-witness-steemit-enthusiast", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T13:54:45", - "trx_id": "75d2a0172b09f084f546043bee27fc36d39e3945", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 65, - { - "block": 2879567, - "op": { - "type": "vote_operation", - "value": { - "author": "svk", - "permlink": "bitshares-gui-release-2-0-160702", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:06:30", - "trx_id": "aeb1a590dc43a8683a66245bbfdd145221a552c5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 66, - { - "block": 2880136, - "op": { - "type": "comment_operation", - "value": { - "author": "gtg", - "body": "I'm Gandalf.\nThat's not my real name, but even my mom saved my number as ***Gandalf*** in her phone contact list.\nThat counts, right?\nI'm an IT Wizard, system admin, happily married, <strike>owner of a cat</strike>.\nOwned by a cat.\n\n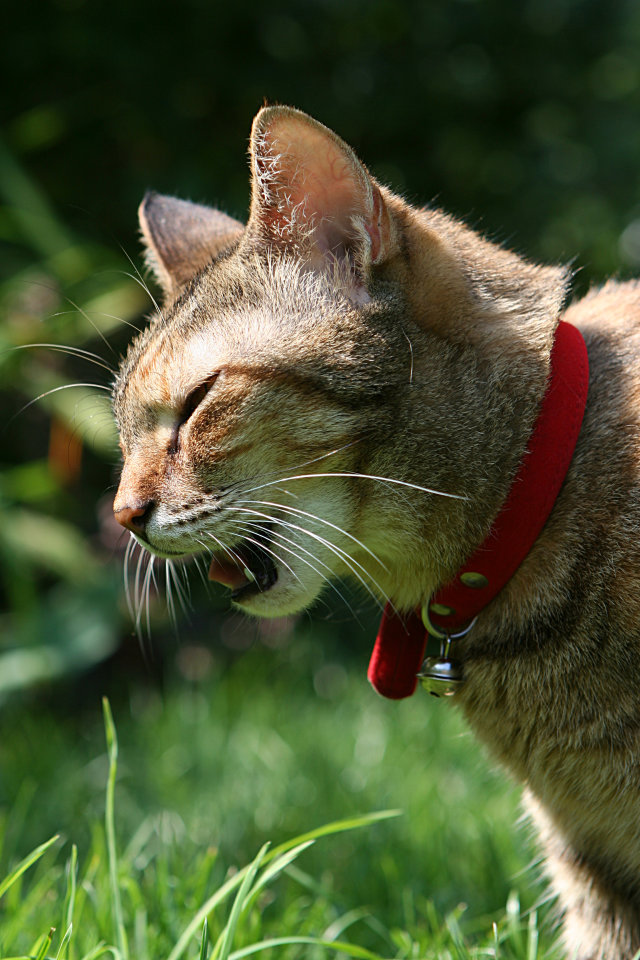\n\n**Nyunya** *(The Cat)* wants to watch everything I do, that's just her way of lending a helping paw. I\u2019m not sure if she is so fascinated by what I do or she wants to make sure I do it right.\n\nWhy am I here? What is my motivation?\nNot much different from that guiding my cat:\nCuriosity and to be a witness (node?) of what is emerging here.\n\nMay the STEEM be with you!", - "json_metadata": "{\"tags\":[\"introduceyourself\",\"cats\"],\"image\":[\"https://grey.house/img/niunia.jpg\"]}", - "parent_author": "", - "parent_permlink": "introduceyourself", - "permlink": "hello-world", - "title": "Hello, World!" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:35:03", - "trx_id": "3f99bf13f5e78fcd4120075bea6933339516f188", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 68, - { - "block": 2880139, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:35:12", - "trx_id": "e65e9b9320dbc02e8dadb7de86e62859dd2512cf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 70, - { - "block": 2880161, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "linouxis9", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:36:18", - "trx_id": "baaa7509860273f10315cf34230feb21919ba592", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 72, - { - "block": 2880192, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "bogdanberkut", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:37:51", - "trx_id": "dc66ababb2c85e013490c89d3e55df1ff1976768", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 74, - { - "block": 2880193, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "vadimberkut8", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:37:54", - "trx_id": "d6e33dfd68b324d9a7d1a2f6ac9dc8b180069d2e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 76, - { - "block": 2880223, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "pheonike", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:39:24", - "trx_id": "9d92a57ebea7570ac49560f1527308a65483df6e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 78, - { - "block": 2880255, - "op": { - "type": "vote_operation", - "value": { - "author": "spectral", - "permlink": "dear-internet-i-am-manuel-lains-spectral", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:41:00", - "trx_id": "56360ae64c012fe3a9ff337d7404a61b3ee929e0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 80, - { - "block": 2880258, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:41:09", - "trx_id": "316de125cbc2dfa8aeebc49b8d3156adcd9fc04f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 82, - { - "block": 2880267, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "complexring", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:41:36", - "trx_id": "f3658f4dc0122cb54939653e9334478f8007e554", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 84, - { - "block": 2880332, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "val", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:44:51", - "trx_id": "c45f530fb6ab4dfcb91d2d0efec5caaec998fd39", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 86, - { - "block": 2880350, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "joseph", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:45:45", - "trx_id": "e070ba28a5b976fb100b6e510272d7f268adbc97", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 88, - { - "block": 2880420, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "camilla", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T16:49:15", - "trx_id": "ad94e3ee521a1e946a36d72b0585a3177ce70a91", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 90, - { - "block": 2880646, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "pal", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:00:33", - "trx_id": "a50ccb84f79e370d2d03c13d174b0418dce11d8a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 92, - { - "block": 2880672, - "op": { - "type": "vote_operation", - "value": { - "author": "luke-williams", - "permlink": "buying-bitcoin-in-taiwan", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:01:51", - "trx_id": "3c6f95391683a90e6e9e070ed02f378e6318f14f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 94, - { - "block": 2880705, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "acidsun", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:03:30", - "trx_id": "38dc989362a9d3b8446446506ea25b904c0fa14e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 96, - { - "block": 2880706, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "pfunk", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:03:33", - "trx_id": "b321af35f9c133b27b1e8c958338274fcd0dd2b6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 98, - { - "block": 2880760, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "omarb", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:06:15", - "trx_id": "e9d83cf5f498d31b85aefbae0ba2b10c265c2a94", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 100, - { - "block": 2880772, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "graystone", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:06:51", - "trx_id": "3925026749e19bb49e61ffe09cd26ba877d88a1a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 102, - { - "block": 2881300, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "konelectric", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:33:18", - "trx_id": "66f090646caa849063054661efd2c043669caa1b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 105, - { - "block": 2881386, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "benthegameboy", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:37:39", - "trx_id": "504892fc0a48741a8ce50fe8d53d6a1d85900e28", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 107, - { - "block": 2881393, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "herethengone", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:38:00", - "trx_id": "64670a21cd06dbb6aaf2107843e40d6ab44d92f0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 109, - { - "block": 2881462, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "steemship", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:41:27", - "trx_id": "5344e174e22da9f9d3ceb5a99f6df5d8c1bc4141", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 112, - { - "block": 2881657, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "trogdor", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:51:12", - "trx_id": "b2954912f61503e17640f4df0c3655c6ef43ec56", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 113, - { - "block": 2881669, - "op": { - "type": "comment_operation", - "value": { - "author": "trogdor", - "body": "Nice, I understand the \"owned by a cat\" sentiment. haha", - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "parent_author": "gtg", - "parent_permlink": "hello-world", - "permlink": "re-gtg-hello-world-20160703t175141501z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T17:51:48", - "trx_id": "86a7bbf1c37601fb1ab5a4b7ff32dc909dd142ec", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 116, - { - "block": 2881985, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "paloma-guerrero", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T18:07:36", - "trx_id": "91c2ee65305712f022bd5dd1bb5948b6f7d8307f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 118, - { - "block": 2882049, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "tuck-fheman", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T18:10:48", - "trx_id": "fd78fafba5925b2ed310d6773b0cfabffb8dabbd", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 120, - { - "block": 2882072, - "op": { - "type": "vote_operation", - "value": { - "author": "lizik", - "permlink": "one-more-cryptogeek-s-girl", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T18:11:57", - "trx_id": "60574ebc4e0474c2a9992cff34cbcf0df5f2cfb2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 123, - { - "block": 2882104, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "gregory-f", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T18:13:33", - "trx_id": "9e2334c98137c0d37e8286a3040a275af254f891", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 125, - { - "block": 2882847, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "hannixx42", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T18:50:45", - "trx_id": "a77943d7e2cb90373a05faf84b3ec9a47c8bc2be", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 127, - { - "block": 2882872, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "benjojo", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T18:52:00", - "trx_id": "55c9d39e63b2ac42097deb66c475ea1c33c69ec0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 129, - { - "block": 2883482, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "kushed", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T19:22:30", - "trx_id": "b773d5b85943af4004c1980c2fc152dad27f195e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 132, - { - "block": 2883838, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "ned", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T19:40:18", - "trx_id": "dfecfadee13f0fda427f45c08c1c123ab5ccb807", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 134, - { - "block": 2884007, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "creator", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T19:48:45", - "trx_id": "4fc57d59164a07e17b064e51254487ea63a87ac3", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 136, - { - "block": 2884019, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "ajvest", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T19:49:21", - "trx_id": "a03862fec5fbd78e9fc3fc8734173a8eb6f628b1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 138, - { - "block": 2884483, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "kenny-crane", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T20:12:33", - "trx_id": "bca81ef01879014d23a973593e21af407bc10696", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 145, - { - "block": 2885659, - "op": { - "type": "vote_operation", - "value": { - "author": "missberg", - "permlink": "why-my-boyfriend-likes-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T21:11:24", - "trx_id": "14a35ac824e3f41e20f26475785aee77e2902e15", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 147, - { - "block": 2885887, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "tinfoilfedora", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T21:22:48", - "trx_id": "454c642d706690955ec540bed537211b3ddfe12e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 149, - { - "block": 2885937, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "lightninggears", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T21:25:18", - "trx_id": "5607f47584ec73a0cefc5b7a613cdabdc0447929", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 151, - { - "block": 2886356, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "pstrident", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T21:46:15", - "trx_id": "b230b032efeb21bc82c018a9618b7a803072067e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 153, - { - "block": 2886896, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "bbqbear", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T22:13:15", - "trx_id": "bd60ff041ecb9552b5701b0a685ccf4e93e58980", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 155, - { - "block": 2887489, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "amartinezque", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T22:43:06", - "trx_id": "5da149fb3a2a611f4fcd99e213df304e340ad56f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 157, - { - "block": 2887502, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "bitcoin-payments-accepted-in-20s-soon-to-be-6s", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T22:43:45", - "trx_id": "9729bd39ffede1ad4d461474012f2868b7d4d8a2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 158, - { - "block": 2887536, - "op": { - "type": "comment_operation", - "value": { - "author": "amartinezque", - "body": "Cats, humans , all we move for instinct and curiosity. Welcome! And your cat also!:P", - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "parent_author": "gtg", - "parent_permlink": "hello-world", - "permlink": "re-gtg-hello-world-20160703t224527020z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T22:45:27", - "trx_id": "bee17bf2245d2b56ccaa71e2e74db943df9ae161", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 160, - { - "block": 2889996, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "murh", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:02:00", - "trx_id": "c20653f1d0cfbba893a8e29f898bd802bb0be4e5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 162, - { - "block": 2890483, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "treeshaface", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T01:29:45", - "trx_id": "9bbf77153e713f4fb6dc6359711d296415395a35", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 164, - { - "block": 2892079, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "merciesmay", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T02:52:54", - "trx_id": "875284d77c647803d7c163f90796d9389e4e4052", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 166, - { - "block": 2893140, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "justtryme90", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T03:47:12", - "trx_id": "c01329f94ed64040f81762f9d8fae84bb03dd890", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 168, - { - "block": 2893633, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "zach-beckett", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T04:12:06", - "trx_id": "cad1777dd63bd4372ea274beeeb538f2c43b6c45", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 170, - { - "block": 2894724, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "joelinux", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T05:06:54", - "trx_id": "6e405dc0426d6930312f787022c930623b9c68ce", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 172, - { - "block": 2898100, - "op": { - "type": "vote_operation", - "value": { - "author": "aboutagirl", - "permlink": "theresa-jones-queen-of-the-bad-selfie", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T07:56:21", - "trx_id": "6866f8e655cb9fca11d6516df2af92629db8e68f", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 174, - { - "block": 2901222, - "op": { - "type": "vote_operation", - "value": { - "author": "suika", - "permlink": "hey-there-my-name-is-suika-and-i-m-a-singer", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T10:32:57", - "trx_id": "74fa5624b3e9e0e9cbf66ee74bee110ab2615aef", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 176, - { - "block": 2904214, - "op": { - "type": "vote_operation", - "value": { - "author": "cryptoctopus", - "permlink": "on-the-goodness-of-greed-on-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T13:03:15", - "trx_id": "7e87957e87933cad394d4513ed8917d2400496ff", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 178, - { - "block": 2905038, - "op": { - "type": "vote_operation", - "value": { - "author": "liondani", - "permlink": "wow-my-post-diluted-50-in-just-22-hours-and-i-know-why", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T13:44:36", - "trx_id": "f04a2e2b4792480942d3ef4ff275ad7e1add2230", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 182, - { - "block": 2910577, - "op": { - "type": "comment_operation", - "value": { - "author": "edu-lopov", - "body": "Welcome to Steemit Gandalf!", - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "parent_author": "gtg", - "parent_permlink": "hello-world", - "permlink": "re-gtg-hello-world-20160704t182251522z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T18:22:51", - "trx_id": "cac4a9c7f617d140d09053600b36bca7c90524a7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 184, - { - "block": 2910863, - "op": { - "type": "vote_operation", - "value": { - "author": "elishagh1", - "permlink": "idea-verified-websites-and-link-sharing-on-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T18:37:09", - "trx_id": "1c187c0d1578de8820704c8c5be5569c5d0a6e03", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 186, - { - "block": 2910892, - "op": { - "type": "vote_operation", - "value": { - "author": "tuck-fheman", - "permlink": "verified-accounts--reputation-system", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T18:38:39", - "trx_id": "f649ef248002da8e5b58e7143d6191837382f7b8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 193, - { - "block": 2914883, - "op": { - "type": "vote_operation", - "value": { - "author": "nomoreheroes7", - "permlink": "how-does-10-interest-on-sd-work", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-04T21:59:06", - "trx_id": "6b1d5ec2e63d9fd8a43a790564f4683252af729d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 195, - { - "block": 2923723, - "op": { - "type": "vote_operation", - "value": { - "author": "cotough", - "permlink": "my-habits-define-me", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T05:23:36", - "trx_id": "e47409d084db99592d9b8fc6257acf86e916745a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 197, - { - "block": 2923910, - "op": { - "type": "vote_operation", - "value": { - "author": "donkeypong", - "permlink": "can-you-guess-amazon-s-1-hot-new-release-in-the-blogging-and-blogs-category", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T05:32:57", - "trx_id": "5d4929825990327904183ec2794c007edf0058cf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 200, - { - "block": 2934342, - "op": { - "type": "vote_operation", - "value": { - "author": "jamtaylor", - "permlink": "steem-of-the-day-close-encounters-with-dying", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T14:26:33", - "trx_id": "8a0f6d5151c9d27e643858d8e67f96b64dc18eec", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 203, - { - "block": 2939142, - "op": { - "type": "vote_operation", - "value": { - "author": "pfunk", - "permlink": "a-user-s-guide-to-the-different-steem-keys-or-passwords", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T18:27:45", - "trx_id": "3b5771d558fa4d6e261b67ccf3aba3b8089bb845", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205, - { - "block": 2942161, - "op": { - "type": "vote_operation", - "value": { - "author": "proctologic", - "permlink": "easy-install-steemd-in-ubuntu", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-05T20:59:09", - "trx_id": "ee16220a3e0e39e690e1e9e9b32c86ca29f85a0d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 208, - { - "block": 2951947, - "op": { - "type": "vote_operation", - "value": { - "author": "bitcube", - "permlink": "steem-mining-in-microsoft-windows-a-builder-s-guide-part-1", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-06T05:13:06", - "trx_id": "fca96579520e1d3a1a2922a8906577cb4744ca59", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 210, - { - "block": 2952982, - "op": { - "type": "vote_operation", - "value": { - "author": "tuck-fheman", - "permlink": "are-chemtrails-real-cia-director-john-o-brennan-seems-to-think-so", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-06T06:04:57", - "trx_id": "c43ee4e4dd607c215f412070431b8ed2173df481", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 213, - { - "block": 2958981, - "op": { - "type": "vote_operation", - "value": { - "author": "press-release", - "permlink": "lbry-beta-goes-live", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-06T11:05:48", - "trx_id": "ae9f5e938314d7fa471920b8ca1f57178bf4498a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 215, - { - "block": 2959714, - "op": { - "type": "vote_operation", - "value": { - "author": "samupaha", - "permlink": "we-need-an-easy-way-to-buy-new-accounts", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-06T11:42:36", - "trx_id": "a8467b26a826e2fd6297f3205c3a76a8dcf22ff5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 217, - { - "block": 2961051, - "op": { - "type": "vote_operation", - "value": { - "author": "sean-king", - "permlink": "i-just-want-to-be-inspired", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-06T12:49:33", - "trx_id": "44ea00bcca04a5343f7a3df72a47193e5391926f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 220, - { - "block": 2981910, - "op": { - "type": "vote_operation", - "value": { - "author": "theoretical", - "permlink": "the-steem-reward-system-part-6-curation-rewards-calculus-with-s-greater-than-0", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-07T06:17:57", - "trx_id": "ecb06c77c87ea7966bab9b2a45b8b73f74fc2c12", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 222, - { - "block": 2982861, - "op": { - "type": "vote_operation", - "value": { - "author": "lauralemons", - "permlink": "my-dad-is-dead-and-i-am-glad-he-died", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-07T07:05:39", - "trx_id": "e1ab7cbe34c1f8b4a291a0f329503000564c1f91", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 224, - { - "block": 2986466, - "op": { - "type": "vote_operation", - "value": { - "author": "pfunk", - "permlink": "re-dailyfeed-cern-detects-exotic-particles-amid-rumors-of-new-physics-20160707t092732596z", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-07T10:06:18", - "trx_id": "f938bb7f0dec67ed6b713fe4c17a0f5236d8245c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 226, - { - "block": 2988124, - "op": { - "type": "vote_operation", - "value": { - "author": "steempower", - "permlink": "preview-your-post-and-how-your-title-image-will-be-displayed-before-you-pull-the-trigger", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-07T11:29:30", - "trx_id": "69fd29bd01cd3d457a82a9c38102c1dfaa1f6a47", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 230, - { - "block": 2994663, - "op": { - "type": "vote_operation", - "value": { - "author": "hisnameisolllie", - "permlink": "steem-dollar-stable-currency-possible", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-07T16:57:30", - "trx_id": "f31692e5275424ff128e93b593418bc9930eca04", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 232, - { - "block": 2995752, - "op": { - "type": "vote_operation", - "value": { - "author": "ash", - "permlink": "til-on-average-people-preferred-to-look-someone-in-the-eyes-for-3-3-seconds", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-07T17:52:06", - "trx_id": "8c73d1a65699fa29c9723613a741de6525d78f65", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 234, - { - "block": 2997036, - "op": { - "type": "vote_operation", - "value": { - "author": "ringin-ringin", - "permlink": "i-hope-here-i-get-a-good-husband", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-07T18:56:21", - "trx_id": "505586196a8fd77ce16b12d33d4531e044c63ad1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 236, - { - "block": 2998656, - "op": { - "type": "vote_operation", - "value": { - "author": "void", - "permlink": "acceptance-is-the-opposite-of-arrogance", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-07T20:17:30", - "trx_id": "05271990b5aa065bb652beff1b9479f5cfb971be", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 238, - { - "block": 3009758, - "op": { - "type": "vote_operation", - "value": { - "author": "steemitindo", - "permlink": "the-ultimate-guide-to-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-08T05:34:06", - "trx_id": "6330886a8d5ccc6a5c6974c5889cae09270fe53f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 240, - { - "block": 3009957, - "op": { - "type": "vote_operation", - "value": { - "author": "steemship", - "permlink": "how-to-vote-smart-and-profit-from-steemit-s-new-curation-rewards", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-08T05:44:12", - "trx_id": "d79f29edf7c72b6194ec4a72a6118badd235e704", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 244, - { - "block": 3020052, - "op": { - "type": "vote_operation", - "value": { - "author": "lauralemons", - "permlink": "living-with-a-rare-and-debilitating-invisible-illness", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-08T14:10:24", - "trx_id": "97ac3f8760adafdd02369988aa5cea3d4d4d6ebd", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 246, - { - "block": 3025887, - "op": { - "type": "vote_operation", - "value": { - "author": "federicopistono", - "permlink": "on-the-importance-of-pattern-recognition", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-08T19:02:57", - "trx_id": "78ececa2530a8e809cedcffd3f5db7a30feb41f7", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 248, - { - "block": 3027614, - "op": { - "type": "vote_operation", - "value": { - "author": "krypto", - "permlink": "making-the-case-for-cryptocurrencies-to-friends-family-small-businesses-and-outright-strangers-steemit-is-the-glue-that-ties", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-08T20:29:36", - "trx_id": "a2dd51ce179a9c44da2ae1db47220f0a61319dab", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 250, - { - "block": 3028691, - "op": { - "type": "vote_operation", - "value": { - "author": "bravenewcoin", - "permlink": "bravenewcoin-bitcoin-s-biggest-venture-capital-deals-of-2016-so-far", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-08T21:23:39", - "trx_id": "43840c34dc32892ad1bb8012d86ac6bb7ebb952b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 256, - { - "block": 3040482, - "op": { - "type": "vote_operation", - "value": { - "author": "guerrint", - "permlink": "hello-it-s-tara-i-m-bringing-girlpower-to-steem", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-09T07:15:15", - "trx_id": "2920c3e210acabef40b41ac622e32fcd770fdeaf", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 258, - { - "block": 3045918, - "op": { - "type": "vote_operation", - "value": { - "author": "bacchist", - "permlink": "save-draft-posts", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-09T11:48:03", - "trx_id": "1cbe212a80ae88d2c5b9229a174125ccb0c26ac2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 262, - { - "block": 3056907, - "op": { - "type": "vote_operation", - "value": { - "author": "piercing", - "permlink": "hey-everyone-i-am-a-body-piercer-and-visual-artist-out-of-seattle-wa-my-names-naomi", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-09T20:59:06", - "trx_id": "06729a34dd7e601a881e518fafe42391067754ad", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 265, - { - "block": 3057124, - "op": { - "type": "vote_operation", - "value": { - "author": "kaylinart", - "permlink": "how-to-apply-deodorant-a-detailed-guide", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-09T21:10:03", - "trx_id": "a799cbc7b6de462ff347b1860ebd0d54a1892641", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 267, - { - "block": 3057137, - "op": { - "type": "vote_operation", - "value": { - "author": "guerrint", - "permlink": "re-berniesanders-re-guerrint-hello-it-s-tara-i-m-bringing-girlpower-to-steem-20160709t042752792z", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-09T21:10:42", - "trx_id": "7dcd352ff453665387c86c7c8b75cc5e879e4976", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 269, - { - "block": 3059524, - "op": { - "type": "vote_operation", - "value": { - "author": "krypto", - "permlink": "wow-explaning-steemit-to-people-is-1000x-easier-than-explaining-bitcoin-or-any-blockchain-project-thus-far-dare-i-say-500", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-09T23:10:27", - "trx_id": "303a8d4104b8517e4583f8e9d9c2a450ecef22df", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 273, - { - "block": 3071156, - "op": { - "type": "vote_operation", - "value": { - "author": "trevonjb", - "permlink": "my-biggest-fear-on-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-10T08:53:57", - "trx_id": "8d438aa265a5a8d2d6f0f92410292d92559c15ac", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 275, - { - "block": 3071180, - "op": { - "type": "vote_operation", - "value": { - "author": "jupiter00000", - "permlink": "re-trevonjb-my-biggest-fear-on-steemit-20160709t191943808z", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-10T08:55:09", - "trx_id": "48e3c552c2e960680727846f3222f6679eb272fe", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 278, - { - "block": 3071243, - "op": { - "type": "vote_operation", - "value": { - "author": "stellabelle", - "permlink": "get-revenue-anonymously-how-to-submit-a-secret-to-the-secret-writer-project", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-10T08:58:18", - "trx_id": "4caa69334d93f058e0c35c1dfaca4c03563937f1", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 279, - { - "block": 3072023, - "op": { - "type": "comment_operation", - "value": { - "author": "gtg", - "body": "Happened to me, my 3rd Jul post https://steemit.com/introduceyourself/@gtg/hello-world \nHit $723.07 at 23:19 UTC+02 ended up ~$256", - "json_metadata": "{\"tags\":[\"catharsis\"],\"links\":[\"https://steemit.com/introduceyourself/@gtg/hello-world\"]}", - "parent_author": "pfunk", - "parent_permlink": "bear-with-me-while-i-bitch-about-losing-usd1000-on-a-post-due-to-the-july-4th-payout-and-fork", - "permlink": "re-pfunk-bear-with-me-while-i-bitch-about-losing-usd1000-on-a-post-due-to-the-july-4th-payout-and-fork-20160710t093728262z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-10T09:37:30", - "trx_id": "722393fcc1264595dd131aa5f12a8e69054ef957", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 281, - { - "block": 3072051, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "re-pfunk-bear-with-me-while-i-bitch-about-losing-usd1000-on-a-post-due-to-the-july-4th-payout-and-fork-20160710t093728262z", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-10T09:38:54", - "trx_id": "38ae097d6971e3452132c9b7a1be0f3ac1f9e5e7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 283, - { - "block": 3072227, - "op": { - "type": "vote_operation", - "value": { - "author": "dantheman", - "permlink": "the-myth-that-proof-of-work-creates-irreversibility", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-10T09:47:42", - "trx_id": "66dac4608379d0b022fd4b949a450b98f42c22b8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 285, - { - "block": 3073997, - "op": { - "type": "vote_operation", - "value": { - "author": "rbur93", - "permlink": "hi-more-girl-power-for-steemit-my-name-is-samantha", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-10T11:16:30", - "trx_id": "cacead98a163d7990c5ccbffdd5402aabc895696", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 286, - { - "block": 3075654, - "op": { - "type": "comment_operation", - "value": { - "author": "gtg", - "body": "I'm Gandalf.\nThat's not my real name, but even my mom saved my number as ***Gandalf*** in her phone contact list.\nThat counts, right?\nI'm an IT Wizard, system admin, happily married, <strike>owner of a cat</strike>.\nOwned by a cat.\n\n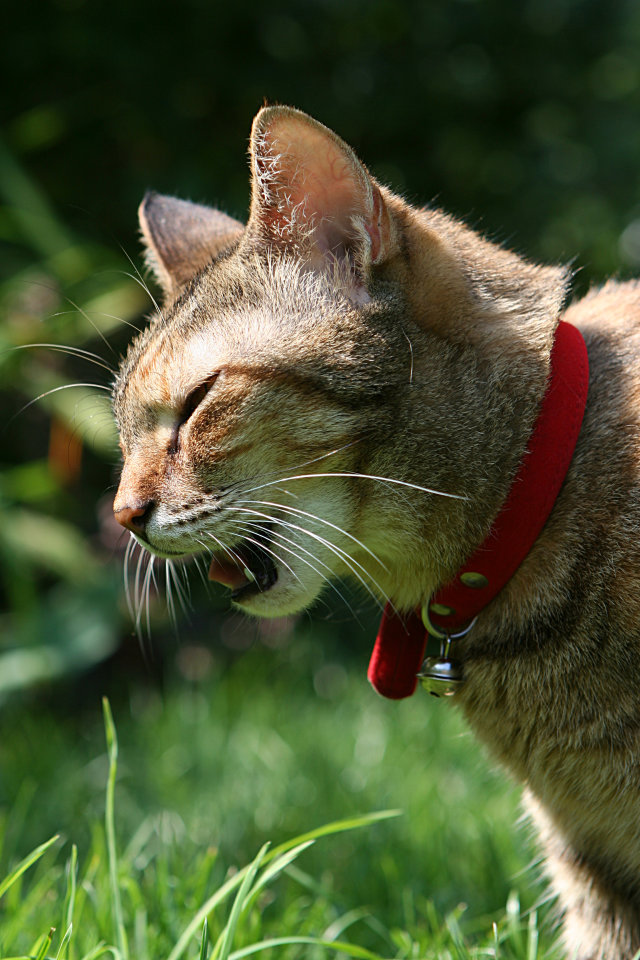\n\n**Nyunya** *(The Cat)* wants to watch everything I do, that's just her way of lending a helping paw. I\u2019m not sure if she is so fascinated by what I do or she wants to make sure I do it right.\n\nWhy am I here? What is my motivation?\nNot much different from that guiding my cat:\nCuriosity and to be a witness (node?) of what is emerging here.\n\nMay the STEEM be with you!", - "json_metadata": "{\"tags\":[\"introduceyourself\",\"cats\"],\"image\":[\"https://grey.house/img/niunia.jpg\"]}", - "parent_author": "", - "parent_permlink": "introduceyourself", - "permlink": "hello-world", - "title": "Hello, World!" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-10T12:39:42", - "trx_id": "a04aa61579b65412036b6e1f79967006b54c508e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 288, - { - "block": 3079906, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "kirill7", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-10T16:12:51", - "trx_id": "7aaf353983b75689639be61d6c211d6ba32f1044", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 291, - { - "block": 3082893, - "op": { - "type": "vote_operation", - "value": { - "author": "magnebit", - "permlink": "addiction-to-steem-syndrome", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-10T18:42:42", - "trx_id": "8d8fc4cc2898161ff6b46315c7f992c258e53212", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 298, - { - "block": 3099275, - "op": { - "type": "vote_operation", - "value": { - "author": "stellabelle", - "permlink": "the-modeling-industry-destroyed-my-soul-part-1", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-11T08:24:48", - "trx_id": "df1661d1f7424db65229e5d9af8c7ffe35aa3fd1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 300, - { - "block": 3100318, - "op": { - "type": "vote_operation", - "value": { - "author": "psychonaut", - "permlink": "greetings-from-an-explorer-of-inner-space", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-11T09:17:06", - "trx_id": "3a6b0d704667a630ac10bb0fb58c412d40ac600e", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 303, - { - "block": 3103497, - "op": { - "type": "vote_operation", - "value": { - "author": "tinfoilfedora", - "permlink": "a-solution-to-copied-content-that-would-make-steemit-explode-with-cool-posts", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-11T11:56:27", - "trx_id": "80832fb4a5f6d075c33e97a09beceb211e1f7ae7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 310, - { - "block": 3111202, - "op": { - "type": "vote_operation", - "value": { - "author": "jlwkolb", - "permlink": "so-you-want-to-illustrate-children-s-books", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-11T18:23:03", - "trx_id": "6d243439e8aeb0ce159d5b03735d9f99919aa618", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 315, - { - "block": 3114328, - "op": { - "type": "vote_operation", - "value": { - "author": "svk", - "permlink": "ann-steemjs-steemjs-lib", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-11T20:59:42", - "trx_id": "fcf987815bd2ea8b3c74917d4f3981c6e6f19a48", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 319, - { - "block": 3124883, - "op": { - "type": "vote_operation", - "value": { - "author": "discombobulated", - "permlink": "are-people-even-reading-posts-before-upvoting", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-12T05:48:30", - "trx_id": "7eaa911c4abee7bc5a3e5fef825ef32bae6fd7b4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 321, - { - "block": 3124998, - "op": { - "type": "vote_operation", - "value": { - "author": "ajvest", - "permlink": "hi-finally-posting-my-intro-w-o-big-boobs-but-i-d-like-to", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-12T05:54:24", - "trx_id": "68be6166bce6fd512e58a5984a08ba77577738eb", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 323, - { - "block": 3132052, - "op": { - "type": "vote_operation", - "value": { - "author": "jlwkolb", - "permlink": "art-on-amphetamines", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-12T11:50:33", - "trx_id": "47b3a24098a3f894b97fe6bd45d100274eb7e73f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 327, - { - "block": 3141796, - "op": { - "type": "vote_operation", - "value": { - "author": "recursive", - "permlink": "important-abuse-reporting-framework", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-12T19:59:51", - "trx_id": "c126280d341816f4bd88726c340e2e131b0f9d57", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 336, - { - "block": 3162790, - "op": { - "type": "vote_operation", - "value": { - "author": "abit", - "permlink": "steemd-resync-and-replay-speed-boost-made-simple", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-13T13:33:12", - "trx_id": "c217a62dd5807392bfb2f372a188602c729bd73b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 338, - { - "block": 3166551, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "mrhankeh", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-13T16:43:57", - "trx_id": "18606480ba8a87de015977f37e119e55667e572c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 342, - { - "block": 3179313, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "hello-world", - "voter": "rom1379", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-14T03:25:00", - "trx_id": "db3b4b67b94065e8ef47e5c4be58d02ef396569c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 344, - { - "block": 3182677, - "op": { - "type": "vote_operation", - "value": { - "author": "blakemiles84", - "permlink": "two-users-are-gaming-the-liquidity-reward-system-and-earning-1200-steem-per-hour", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-14T06:14:09", - "trx_id": "f02626574b3fb5c934be8446e5ebe95b0391c4e2", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 346, - { - "block": 3182941, - "op": { - "type": "vote_operation", - "value": { - "author": "picokernel", - "permlink": "tutorial-mining-get-rich-the-old-fashioned-way", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-14T06:27:30", - "trx_id": "dc9677a1188404a6bbe1abcd08975af8e955ef9b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 348, - { - "block": 3184633, - "op": { - "type": "vote_operation", - "value": { - "author": "cbergmann", - "permlink": "about-the-upcoming-virtual-currency-regulation-in-the-eu-and-what-it-maybe-means-for-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-14T07:52:36", - "trx_id": "e940ce8f81418014a27676efbd53c64321cf7b06", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 350, - { - "block": 3184819, - "op": { - "type": "vote_operation", - "value": { - "author": "corinnestokes", - "permlink": "photographer-homeschooling-mom-dancer-budget-conscious-proponent-of-real-food-hello-world", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-14T08:01:57", - "trx_id": "32d5323015a2c5c27eba23f126d4bf3440e73099", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 352, - { - "block": 3189345, - "op": { - "type": "vote_operation", - "value": { - "author": "roelandp", - "permlink": "steemstream-com-a-realtime-visualisation-of-all-activity-on-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-14T11:49:36", - "trx_id": "2af5dae94264f734141143b5ca69b2148f574f67", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 354, - { - "block": 3190548, - "op": { - "type": "vote_operation", - "value": { - "author": "void", - "permlink": "steemwatch-the-notification-center-for-steemit-version-0-3-0-is-deployed", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-14T12:50:09", - "trx_id": "8ed0d284a7e66b1bd1e8d20911f6e9d3b0299f83", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 359, - { - "block": 3200741, - "op": { - "type": "vote_operation", - "value": { - "author": "steemitblog", - "permlink": "important-security-announcement-steemit-ceo-ned-scott", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-14T21:22:36", - "trx_id": "da008dceab99bcec85704022fc97dae91ba43210", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 362, - { - "block": 3201365, - "op": { - "type": "vote_operation", - "value": { - "author": "anyx", - "permlink": "okay-i-am-mining-steem-how-do-i-set-up-cli-wallet", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-14T21:54:12", - "trx_id": "fcd96cd53df5436c46d9a8936a4934a158841558", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 380, - { - "block": 3560270, - "op": { - "type": "vote_operation", - "value": { - "author": "cass", - "permlink": "steemit-chat-foss-alternative-to-slack-deployed-with-rocketchat", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-27T13:23:42", - "trx_id": "cf7e559ff3afeaabfb2aaa629712591e31e915d4", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 383, - { - "block": 3593223, - "op": { - "type": "vote_operation", - "value": { - "author": "heiditravels", - "permlink": "heidi-travels-to-peru-only-w-ith-steem-dollars", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-28T17:08:36", - "trx_id": "525e3c496df308c43eee1623d71820a0b2c0aee5", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 385, - { - "block": 3594473, - "op": { - "type": "vote_operation", - "value": { - "author": "blockchainblonde", - "permlink": "tokenization-of-commercial-real-estate", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-28T18:11:09", - "trx_id": "1315f301d488bd0e6b8dc3cad3afad4ab4d84bb2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 389, - { - "block": 3619122, - "op": { - "type": "vote_operation", - "value": { - "author": "phoenixmaid", - "permlink": "5-things-every-learner-should-know-before-they-pick-up-a-crochet-hook", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-29T14:50:54", - "trx_id": "e6b24257759c6dfe301d0ac512ad9b9beb6c5022", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 392, - { - "block": 3623467, - "op": { - "type": "vote_operation", - "value": { - "author": "joseph", - "permlink": "proposal-to-hire-and-send-steemit-representatives-into-content-marketing-world-conference-and-expo", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-29T18:28:39", - "trx_id": "abd7f1355caba850a9a2b0d729bab56bb6aba833", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 394, - { - "block": 3624209, - "op": { - "type": "vote_operation", - "value": { - "author": "klye", - "permlink": "flag-taggin-and-uptucks-welcome-to-the-wild-west-of-social-media", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-29T19:05:51", - "trx_id": "50e7c3e5afd89264c7e411f0c3548ef04e5f2f51", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 396, - { - "block": 3625791, - "op": { - "type": "vote_operation", - "value": { - "author": "dantheman", - "permlink": "notice-to-tag-spammers", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-29T20:25:18", - "trx_id": "5296672cf7f27e3034f74f586769ad912b009abd", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 399, - { - "block": 3638719, - "op": { - "type": "vote_operation", - "value": { - "author": "kingscrown", - "permlink": "my-monthly-report-on-cryptos-to-buy-is-out", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T07:16:57", - "trx_id": "5dd178c6140602efc6d0b617738718fa1a98e3c5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 403, - { - "block": 3646900, - "op": { - "type": "vote_operation", - "value": { - "author": "noisy", - "permlink": "mirror-o-tym-jak-zarobilem-11-020-zl-publikujac-posta-na-steemit-a-nie-na-wykop-pl", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T14:09:51", - "trx_id": "0b3aa5aa045d6f98f714f499ab2b9ee48f7db956", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 408, - { - "block": 3649196, - "op": { - "type": "vote_operation", - "value": { - "author": "gord0b", - "permlink": "abusing-steemit-using-facebook-sign-up", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T16:05:57", - "trx_id": "52d8d636da95b1c0a2830a77a4c861787735234d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 410, - { - "block": 3654052, - "op": { - "type": "vote_operation", - "value": { - "author": "lyubovnam", - "permlink": "my-amazing-journey-to-dominican-republic", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T20:10:15", - "trx_id": "f27bb149386e947cd07c91853c206217814a8da2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 412, - { - "block": 3654737, - "op": { - "type": "vote_operation", - "value": { - "author": "dercoco", - "permlink": "the-real-stellabelle", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T20:44:42", - "trx_id": "8fd00814df02570ad3bdb291ed95d5cad6f169c2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 414, - { - "block": 3655891, - "op": { - "type": "vote_operation", - "value": { - "author": "agent", - "permlink": "how-society-is-making-life-hard-for-girls-of-generation-y", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-30T21:42:33", - "trx_id": "bc3c42a3bbef500ce79a188eaf3c71a537cee572", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 421, - { - "block": 3681975, - "op": { - "type": "vote_operation", - "value": { - "author": "razvanelulmarin", - "permlink": "can-you-plagiarize-yourselff-should-we-repost-steemit-articles-after-payout-let-s-discuss", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-31T19:34:51", - "trx_id": "4d9f348f13b1cc5f140260f0e2b5c4fd909c9b21", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 425, - { - "block": 3703621, - "op": { - "type": "vote_operation", - "value": { - "author": "nonlinearone", - "permlink": "hello-world-physics-ph-d-with-a-love-for-particle-physics-code-hiking-and-blockchains-here", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-01T13:41:21", - "trx_id": "aae363deee2a8481c0b243b4ac3c9f28279a7e65", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 427, - { - "block": 3703690, - "op": { - "type": "vote_operation", - "value": { - "author": "jennamarbles", - "permlink": "i-think-that-bots-can-affect-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-01T13:44:48", - "trx_id": "35efd0e340a00cfd785d03e9791462d7ef3d2cda", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 429, - { - "block": 3703953, - "op": { - "type": "vote_operation", - "value": { - "author": "allasyummyfood", - "permlink": "how-to-make-steemit-cake-my-first-youtube-video-tutorial-on-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-01T13:58:00", - "trx_id": "611e8251b15ad66f5347fc1ca071df64f2cc4db1", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 431, - { - "block": 3704651, - "op": { - "type": "vote_operation", - "value": { - "author": "celebr1ty", - "permlink": "patties-bomb", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-01T14:32:57", - "trx_id": "3d0c68db4c8d685b38df2fb6f3ea4e7f2cf1b211", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 436, - { - "block": 3709019, - "op": { - "type": "vote_operation", - "value": { - "author": "johnerfx", - "permlink": "my-first-ever-published-open-source-code-my-story-2min-read", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-01T18:12:36", - "trx_id": "e4ff4db158ed659fb7b4480113fa823a6dd0530f", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 439, - { - "block": 3712766, - "op": { - "type": "vote_operation", - "value": { - "author": "steem-girls", - "permlink": "helping-children-in-mali-introducing-the-steemgirls-network", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-01T21:20:48", - "trx_id": "0a45857b7d10f7c85b0d9f79dca8552c77356d19", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 446, - { - "block": 3759466, - "op": { - "type": "vote_operation", - "value": { - "author": "mefisto", - "permlink": "pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-03T12:23:00", - "trx_id": "a746f3c43900af4b9204a3105c723468666eff82", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 447, - { - "block": 3759799, - "op": { - "type": "comment_operation", - "value": { - "author": "gtg", - "body": "Zaufanie do delegata si\u0119 liczy. Jego narodowo\u015b\u0107 nie.\nG\u0142osowa\u0107 warto, \u015bwiadomie.\nRozumiem, \u017ce nie ka\u017cdy ma czas i ch\u0119ci, by by\u0107 na bie\u017c\u0105co z tym, co tu si\u0119 dzieje 'od kuchni'. Ja staram si\u0119 przygl\u0105da\u0107 temu uwa\u017cnie i g\u0142osuj\u0119 na tych, kt\u00f3rzy sobie zas\u0142u\u017cyli na to wg mojej skromnej, subiektywnej oceny.\nSam r\u00f3wnie\u017c b\u0119d\u0119 si\u0119 stara\u0142 o wy\u017csz\u0105 pozycj\u0119 na li\u015bcie, ale na razie zbyt wiele innych zaj\u0119\u0107 (zwi\u0105zanych ze steemit)\nAby g\u0142osowa\u0107 tak jak ja mo\u017cna w **cli_wallet** wykona\u0107 polecenie\n`set_voting_proxy \"youraccountname\" \"gtg\" true`\nZr\u00f3b to tylko wtedy je\u015bli jeste\u015b w stanie zaufa\u0107 mi co do moich wybor\u00f3w w sprawie delegat\u00f3w.", - "json_metadata": "{\"tags\":[\"polish\"]}", - "parent_author": "mefisto", - "parent_permlink": "pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness", - "permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-03T12:39:42", - "trx_id": "8325110ddfa8b352975e6fd3bff448c3468efc87", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 449, - { - "block": 3759820, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-03T12:40:45", - "trx_id": "beb311ead9ee3c8a69845b1b53e0da5182e7c97d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 450, - { - "block": 3760135, - "op": { - "type": "comment_operation", - "value": { - "author": "mefisto", - "body": "Kryterium wyboru delegata to osobna sprawa. Co delegat powinien potrafi\u0107?Co robi\u0107? Czy powinien poniek\u0105d s\u0142u\u017cy\u0107 swoim wyborcom?\nJedno zdaje si\u0119 by\u0107 pewne - **powinien by\u0107 aktywnym cz\u0142onkiem spo\u0142eczno\u015bci steemit.** \nJestem niemal pewny, \u017ce jestesmy w stanie wybra\u0107 wskaza\u0107 kilka os\u00f3b, kt\u00f3re mog\u0142yby zbiera\u0107 glosy polskiej spo\u0142eczno\u015bci. Je\u015bli si\u0119 nie myl\u0119, g\u0142os\u00f3w b\u0119dzie wi\u0119cej - je\u015bli system g\u0142osowania na dodatkowych kandydat\u00f3w b\u0119dzie prostszy dla przeci\u0119tnego po\u017ceracza blog\u00f3w... np. pojawi si\u0119 \u0142adny graficzny klient pod windoz\u0119.", - "json_metadata": "{\"tags\":[\"polish\"]}", - "parent_author": "gtg", - "parent_permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "permlink": "re-gtg-re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t125639335z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-03T12:56:33", - "trx_id": "66fb777f2f36b7a3666f236228bddd1eeeeed1ed", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 451, - { - "block": 3760145, - "op": { - "type": "comment_operation", - "value": { - "author": "mefisto", - "body": "@@ -66,16 +66,17 @@\n otrafi%C4%87?\n+ \n Co robi%C4%87\n", - "json_metadata": "{\"tags\":[\"polish\"]}", - "parent_author": "gtg", - "parent_permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "permlink": "re-gtg-re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t125639335z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-03T12:57:03", - "trx_id": "176074c6fb5f33db4fdf0291c49b7082e9393b33", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 452, - { - "block": 3760160, - "op": { - "type": "comment_operation", - "value": { - "author": "mefisto", - "body": "@@ -238,17 +238,17 @@\n %C5%BCe jeste\n-s\n+%C5%9B\n my w sta\n@@ -257,16 +257,18 @@\n e wybra%C4%87\n+ /\n wskaza%C4%87\n@@ -331,16 +331,18 @@\n zno%C5%9Bci. \n+%0A%0A\n Je%C5%9Bli si\n", - "json_metadata": "{\"tags\":[\"polish\"]}", - "parent_author": "gtg", - "parent_permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "permlink": "re-gtg-re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t125639335z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-03T12:57:48", - "trx_id": "e6cca453b183babf4de47b155e5fc2fd09adc273", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 453, - { - "block": 3760183, - "op": { - "type": "comment_operation", - "value": { - "author": "mefisto", - "body": "@@ -220,18 +220,16 @@\n tem \n-niemal pew\n+przekona\n ny, \n", - "json_metadata": "{\"tags\":[\"polish\"]}", - "parent_author": "gtg", - "parent_permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "permlink": "re-gtg-re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t125639335z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-03T12:58:57", - "trx_id": "e1e36b533b9ca71b26e28d81f4191f6f79dc874c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 457, - { - "block": 3781402, - "op": { - "type": "vote_operation", - "value": { - "author": "lukmarcus", - "permlink": "mega-statistics-and-analysis-for-top-50-most-popular-steemit-introductions-introduceyourself", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-04T06:45:12", - "trx_id": "b914425c512a8d0235e4e423ebc29663f22f7643", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 462, - { - "block": 3787998, - "op": { - "type": "vote_operation", - "value": { - "author": "blueorgy", - "permlink": "steem-cool-live-reputation-rep-viewer-w-estimated-upvote-rep-value-rep-needed-for-next-level-level-information", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-04T12:15:57", - "trx_id": "47947871577194bb5aa9a458263a0978d66e76c5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 464, - { - "block": 3788329, - "op": { - "type": "vote_operation", - "value": { - "author": "jl777", - "permlink": "specialization-jump-starting-new-steemit-sub-communities-and-creating-insurmountable-obstacles-for-others", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-04T12:32:33", - "trx_id": "28a6ce6fa40a121389f6dec52d0226458019ff42", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 469, - { - "block": 3809194, - "op": { - "type": "vote_operation", - "value": { - "author": "lukmarcus", - "permlink": "my-introduction-i-m-just-a-simple-guy-with-ordinary-life-sometimes-weird-or-funny-maybe-both-play-and-run", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T06:12:12", - "trx_id": "7edb8368e2b83d8ce74126300518ff41d4356779", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 471, - { - "block": 3813997, - "op": { - "type": "vote_operation", - "value": { - "author": "dollarvigilante", - "permlink": "the-dollar-vigilante-is-now-on-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T10:13:18", - "trx_id": "ab3a45080cd6e136dbd4658dc7a0e06a1b060479", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 474, - { - "block": 3818561, - "op": { - "type": "comment_operation", - "value": { - "author": "gtg", - "body": "Hello, World!\n\nMy intention is not to make it into top 19. I could name many other candidates (clearly more than 19) with greater commitment to steem(it) who are a lot more appropriate as witnesses, but I'm sure I can be useful as a backup witness.\n\nI introduced myself here:\nhttps://steemit.com/introduceyourself/@gtg/hello-world\nThose of you who use https://steemit.chat know me as **Gandalf**.\nFor some time, I\u2019ve been using my magic powers (mostly those related to security and infrastructure) to improve steemit. For example, I\u2019ve improved our \"Perfect\" Forward Secrecy and made our communication with site more secure.\n\nMy **seed node** (EU based):\n`seed-node = gtg.steem.house:2001`\nYou can also use it to rsync data dir\n`rsync -Pa rsync://gtg.steem.house/witness_node_data_dir .`\n\nMy **witness node** is on a separate data center (an EU-based one as well).\nIf you believe I can be of value to steemit, please vote for me:\n`vote_for_witness YOURACCOUNT gtg true true`\n\n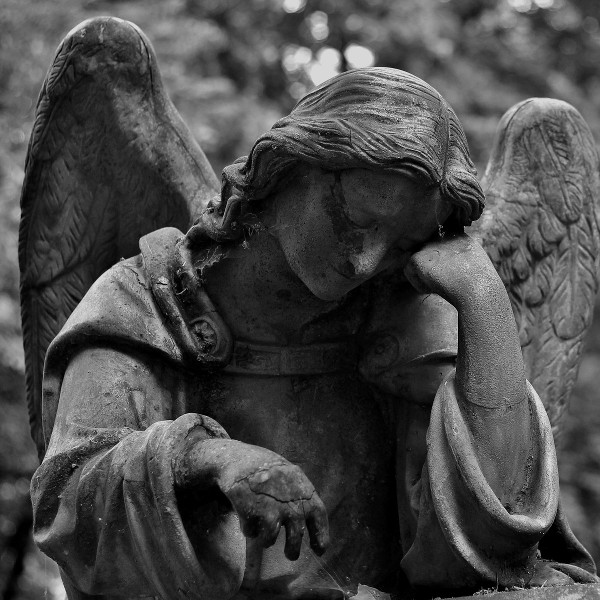", - "json_metadata": "{\"tags\":[\"witness-category\"],\"links\":[\"https://steemit.com/introduceyourself/@gtg/hello-world\"]}", - "parent_author": "", - "parent_permlink": "witness-category", - "permlink": "witness-gtg", - "title": "Witness \"gtg\"" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T14:02:24", - "trx_id": "bf5e83b7ddc4efcaf101f5260653a3588e411f36", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 476, - { - "block": 3818561, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T14:02:24", - "trx_id": "bf5e83b7ddc4efcaf101f5260653a3588e411f36", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 478, - { - "block": 3818587, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "softpunk", - "weight": 10 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T14:03:45", - "trx_id": "46e1ce2efedfaf1d3d8a486936b3930060999c93", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 480, - { - "block": 3818953, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "modeprator", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T14:22:09", - "trx_id": "2eee4ca159a4b689c75fab87344002c021d49cf4", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 483, - { - "block": 3820220, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "steempty", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:25:42", - "trx_id": "eb508042119253bf3292154e00e6bc1e1b311f0a", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 485, - { - "block": 3820231, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "murh", - "weight": 1133 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:26:15", - "trx_id": "802c5cff16c3001d61e9910c8e070270f83e8ebd", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 487, - { - "block": 3820249, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:27:09", - "trx_id": "e2ee9d8e91c6d1be22665df3c7d3ba9ca1e07916", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 490, - { - "block": 3820364, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "naturalista", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:32:54", - "trx_id": "9fa49ab335693aaf82bad8eebf0def3376c6488a", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 492, - { - "block": 3820414, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "hcf27", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:35:24", - "trx_id": "ad5334f14510ba6a9c9ec0e615ff43eb66ba5601", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 494, - { - "block": 3820472, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "jarvis", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:38:18", - "trx_id": "9eec5df047fc46fe9be6936813c7b261d6bab0e6", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 496, - { - "block": 3820474, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "steema", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:38:24", - "trx_id": "d6e8d828dc9ed6dded0ce74f6d863a970f07d1c2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 498, - { - "block": 3820512, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "ciao", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:40:18", - "trx_id": "7341990b654f315dc95b5db7ea6d3d5ba9dfa16e", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 500, - { - "block": 3820565, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "steemo", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:42:57", - "trx_id": "3e2ae879e21429aa87b019124c1fc136847dd9dc", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 502, - { - "block": 3820569, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "confucius", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:43:09", - "trx_id": "511e01cf6b02265ffded1710be12f8b0d8905910", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 504, - { - "block": 3820592, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "cire81", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:44:18", - "trx_id": "a551ad1ccb532b121f209bf833af8a6f5978555c", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 505, - { - "block": 3820615, - "op": { - "type": "comment_operation", - "value": { - "author": "cire81", - "body": "Thank you for spending your time to make steemit secure. Thanks to people like you we have a much safer environment. Were you paid for your effort on steem?", - "json_metadata": "{\"tags\":[\"witness-category\"]}", - "parent_author": "gtg", - "parent_permlink": "witness-gtg", - "permlink": "re-gtg-witness-gtg-20160805t154528701z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:45:27", - "trx_id": "47696dcd765db02c2de5827adf4afe65841158eb", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 507, - { - "block": 3820708, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "piezolit", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:50:06", - "trx_id": "41f58934110bc665d9b7744d8f4d2c20137b2003", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 509, - { - "block": 3820711, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "kibela", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:50:15", - "trx_id": "f8559c7dd2f9011a8be2a29dd2d437206e8af223", - "trx_in_block": 9, - "virtual_op": 0 - } - ], - [ - 511, - { - "block": 3820815, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "lauralemons", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T15:55:27", - "trx_id": "741ef0022e7f781e1b3dfbbcd60a3ffb16717d09", - "trx_in_block": 10, - "virtual_op": 0 - } - ], - [ - 514, - { - "block": 3820935, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "paco", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T16:01:27", - "trx_id": "ff3e84841a91f37fc6421d9400c4c458255cc299", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 516, - { - "block": 3820966, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "nixonnox", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T16:03:03", - "trx_id": "f00aaf8b78bab33cc595020e293e9fdeb8a4acba", - "trx_in_block": 11, - "virtual_op": 0 - } - ], - [ - 518, - { - "block": 3821111, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "iaco", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T16:10:24", - "trx_id": "c2aae138916eee45272219f61673a557889aa4fd", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 519, - { - "block": 3821150, - "op": { - "type": "comment_operation", - "value": { - "author": "iaco", - "body": "As the computer world beyond user interfaces is a magical realm to me, the only way I can get my brain to completely understand it is to just let go and tell my self it's all made of unicorns and runes being cast to make the magic happen :) I thank you for your work:)", - "json_metadata": "{\"tags\":[\"witness-category\"]}", - "parent_author": "gtg", - "parent_permlink": "witness-gtg", - "permlink": "re-gtg-witness-gtg-20160805t161219379z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T16:12:21", - "trx_id": "95e2dd24c9aa395d36da098fc8c9589cd7b95c2a", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 521, - { - "block": 3821225, - "op": { - "type": "vote_operation", - "value": { - "author": "riverhead", - "permlink": "re-sisterholics-chilling-by-the-pool-in-phuket-with-my-sister-a-post-about-home-20160805t152604280z", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T16:16:09", - "trx_id": "7a6e4012dd98a23b571880f3a4939c4235203b63", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 523, - { - "block": 3821347, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "kaylinart", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T16:22:18", - "trx_id": "6c21e790abaf26967150efb2dd217a296ccec767", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 525, - { - "block": 3821410, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "juanmiguelsalas", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T16:25:27", - "trx_id": "1509021cab8f4764aa079073c90e81fbb187c143", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 528, - { - "block": 3822129, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "hanshotfirst", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T17:01:30", - "trx_id": "01f6f696d760f1a98cd2aac49d5221ba0050f10c", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 530, - { - "block": 3822331, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "alrx6918", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T17:11:42", - "trx_id": "637429bc676f02076419a2a1831a3170342f93cf", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 533, - { - "block": 3822445, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "endaksi1", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T17:17:27", - "trx_id": "5d6e4721cba018091f238542f74b37f2557209c2", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 535, - { - "block": 3822506, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "celebr1ty", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T17:20:30", - "trx_id": "6a0a48ee2ff03ab4769707d795854fce1893b06a", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 537, - { - "block": 3822678, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "egjoshslim", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T17:29:06", - "trx_id": "1f76cf11e5eae34377bd7922e8cabccdb3315af9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 539, - { - "block": 3822723, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "brucy", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T17:31:21", - "trx_id": "e0a18424d39839576d85443cffdbcbd645d5e208", - "trx_in_block": 10, - "virtual_op": 0 - } - ], - [ - 541, - { - "block": 3823276, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "sulev", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T17:59:06", - "trx_id": "8a4102d764fe6e2c7155f98fbc418d736c21f9c1", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 544, - { - "block": 3823478, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "furion", - "weight": 100 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T18:09:15", - "trx_id": "aff9f12286ac5caee194a79a4287ca45880fc4bd", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 546, - { - "block": 3824218, - "op": { - "type": "comment_operation", - "value": { - "author": "gtg", - "body": "Dzi\u015b oficjalnie zg\u0142osi\u0142em kandydatur\u0119.\nhttps://steemit.com/witness-category/@gtg/witness-gtg\n> Koncentracja g\u0142os\u00f3w - mo\u017ce z czasem pom\u00f3c wybra\u0107 kogo\u015b z naszych do pierwszej 50-tki.\n\nOd pocz\u0105tku by\u0142em w pierwszej setce i aby g\u0142osowa\u0107 konieczne by\u0142o u\u017cycie `cli_wallet`\nObecnie jestem w pierwszej 50-tce, wi\u0119c mo\u017cna g\u0142osowa\u0107 ju\u017c bezpo\u015brednio ze strony:\nhttps://steemit.com/~witnesses", - "json_metadata": "{\"tags\":[\"polish\"],\"links\":[\"https://steemit.com/witness-category/@gtg/witness-gtg\"]}", - "parent_author": "mefisto", - "parent_permlink": "pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness", - "permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160805t184622691z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T18:46:27", - "trx_id": "9c5197bcd87f4207c122ed47e1be9fb2102c0226", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 548, - { - "block": 3824225, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160805t184622691z", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T18:46:48", - "trx_id": "afa34536bd8441a5170e3c0b2ec7290121f2d191", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 550, - { - "block": 3824290, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "edgeland", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T18:50:09", - "trx_id": "5b1f898791b20e16b9a83fbaf2d17a9dba73927a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 552, - { - "block": 3824407, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "glassice", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T18:56:00", - "trx_id": "c957f80fdb8eed50b745b28f71f987581873dd04", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 554, - { - "block": 3824679, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "vitz81", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T19:09:48", - "trx_id": "5758c6dae95074811717558d3d715b85bd00ea7d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 556, - { - "block": 3824792, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "geoffrey", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T19:15:30", - "trx_id": "1baca92bfe6b0d30931af42eba64d00fbbd88b42", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 558, - { - "block": 3824820, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "glitterfart", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T19:16:57", - "trx_id": "ea764dc459b56d3ff7d19fea008f127c0f7a8b28", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 560, - { - "block": 3824985, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "caitlinm", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T19:25:12", - "trx_id": "29fc850b860acc0ab7404dc294a7497f9e6ca440", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 562, - { - "block": 3825115, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "inertia", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T19:31:42", - "trx_id": "f4edc59b820700bdc0d7d8c54c1c44c2d227ee0c", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 564, - { - "block": 3825171, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "jesse5th", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T19:34:30", - "trx_id": "f6a599788591614f771acbf6cd16e711ef4a1ec4", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 566, - { - "block": 3825243, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "lenar79", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T19:38:12", - "trx_id": "012ca1e636717cfad7c8c660e18592c2420306a5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 569, - { - "block": 3825585, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "jparty", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T19:55:21", - "trx_id": "554be4d104df265d737e36908a94518d95bf79af", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 571, - { - "block": 3826016, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "senseiteekay", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T20:17:06", - "trx_id": "8b10f304f9c0104189e1b841faed088610ec17db", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 573, - { - "block": 3826308, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "madwallace", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T20:31:48", - "trx_id": "3e06c43fff9fe5ac13ab83f0a3da324849e6b4f7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 575, - { - "block": 3826530, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "persianqueen", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T20:42:57", - "trx_id": "cdf18685933030ec9c0c76ca9ec9c42d308cff88", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 577, - { - "block": 3826926, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "r4fken", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T21:02:48", - "trx_id": "506b7eb8eca5ca80d425ce7f52a705c678845f48", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 582, - { - "block": 3827939, - "op": { - "type": "vote_operation", - "value": { - "author": "clains", - "permlink": "witnesses-exposed-what-witnesses-have-done-for-us-this-week-third-edition", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T21:53:42", - "trx_id": "967beacd23ce64b10abf60d380f9a2c95067c2ba", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 584, - { - "block": 3828163, - "op": { - "type": "vote_operation", - "value": { - "author": "xcynosure", - "permlink": "re-verification-and-why-my-and-possibly-your-verification-sucked", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T22:05:06", - "trx_id": "64f301ea9becc3f8194b01f2d9347f0a4bed3b9a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 586, - { - "block": 3828507, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "steeminion", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T22:22:27", - "trx_id": "26dba22a2bdfd84c88d56fe00916651f758e16be", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 588, - { - "block": 3828524, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "steeminer", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T22:23:18", - "trx_id": "86c4bf3b369dd497e6714cdf8000cb03834c66a3", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 591, - { - "block": 3829380, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "bledarus", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T23:06:06", - "trx_id": "008974405225cb7e11b55a1edcad0d5ba9f0115a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 593, - { - "block": 3829666, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "jellenmark", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T23:20:27", - "trx_id": "f5615eb9665ddfabfffef9138bc817be9d6eddc9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 595, - { - "block": 3830126, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "anyx", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-05T23:43:36", - "trx_id": "9f74d9727b527d0568d688dd346a35749dbe57d8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 598, - { - "block": 3830458, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "steemship", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:00:39", - "trx_id": "3f98d3bad855b92aaf4715adbd38edeae0352691", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 600, - { - "block": 3830733, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "complexring", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:14:27", - "trx_id": "cb14fb722477338a5b4a05566cbb7c85556295aa", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 602, - { - "block": 3830746, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "silver", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:15:06", - "trx_id": "dca308ab12c77cf776340ac55b940995e8c81a81", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 604, - { - "block": 3830759, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "justin", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:15:45", - "trx_id": "c77efd6590adae6bfe58e97b4cbd5574e300f072", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 606, - { - "block": 3830760, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "thecurator", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:15:48", - "trx_id": "ca0747ecc8054c64e769ba6313988c30ff199ac5", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 608, - { - "block": 3830761, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "chloe", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:15:51", - "trx_id": "ff79bfe66ce4e693a034af6cfb8299e6cb674287", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 610, - { - "block": 3830762, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "bentley", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:15:54", - "trx_id": "0e576340bbea7550fcdfb71d3b658413d032d632", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 612, - { - "block": 3830763, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "danknugs", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:15:57", - "trx_id": "ee99c3c0d8c1709ec151d5b27d00d9517dec6efc", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 614, - { - "block": 3830764, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "jen", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:16:00", - "trx_id": "a6676c61a27beda814738e88daf819a517163b84", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 616, - { - "block": 3830765, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "steemservices", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:16:03", - "trx_id": "1e5698d807c785918a8fdf26fb9e24d693a30819", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 618, - { - "block": 3830766, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "kelly", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:16:06", - "trx_id": "0417c0336bb91036e4313f333a57a9e5341c7cd1", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 620, - { - "block": 3830767, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "sophia", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T00:16:09", - "trx_id": "921f3e22a95e6073da3c568cd859385f3d3f2cf4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 622, - { - "block": 3831675, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "shukerona", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T01:01:45", - "trx_id": "8f02a0808d73dbad21452dd2e64c85b4c540a0d0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 625, - { - "block": 3833075, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "taoteh1221", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T02:12:18", - "trx_id": "3b8238fef32f8a6ccffe9d5e7f4cc3e22d9c2dae", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 627, - { - "block": 3833221, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "roadscape", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T02:19:36", - "trx_id": "4dca753ea3e3330354760357270e64111da4759b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 629, - { - "block": 3833232, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "murh", - "weight": 3301 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T02:20:09", - "trx_id": "d14b6f7c353a9cb1c0c94bb63cb9da9342fa247e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 632, - { - "block": 3833795, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "cinderphoenix", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T02:48:21", - "trx_id": "b404f1ee112ea4e8e856a007bf0d1847fcd691c3", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 634, - { - "block": 3834566, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "somedude", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T03:27:06", - "trx_id": "c00bf149e3b5e0954b6401b65d1eb41dfc7fc12c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 636, - { - "block": 3834759, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "pal", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T03:36:45", - "trx_id": "6df6753dc0e3595900d939df08d980e10c9b6c84", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 643, - { - "block": 3836815, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "glitterpig", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T05:19:51", - "trx_id": "85493d637bb084edc9f44a04737bbb22172bbe81", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 645, - { - "block": 3836914, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "boatymcboatface", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T05:24:54", - "trx_id": "3fd973ef13648d0d4f57e5128f941f61aafcb784", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 647, - { - "block": 3837637, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "johnsmith", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T06:01:09", - "trx_id": "0350653f9abe63f18063c75fcc6e6f032245bc85", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 654, - { - "block": 3844033, - "op": { - "type": "vote_operation", - "value": { - "author": "thebluepanda", - "permlink": "it-s-okay-to-be-different-my-journey-to-hear-the-world", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T11:22:12", - "trx_id": "6b72b1e104e74fdf51a8b6017b09c018faaaff44", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 658, - { - "block": 3844195, - "op": { - "type": "vote_operation", - "value": { - "author": "thebluepanda", - "permlink": "too-much-awesomeness-that-i-can-barely-handle", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T11:30:24", - "trx_id": "86aac2a42310056dcb08fe359216fa3b55309ada", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 662, - { - "block": 3845686, - "op": { - "type": "vote_operation", - "value": { - "author": "arhag", - "permlink": "proposal-for-new-steem-feature-deadman-switch-will-recovering-accounts-from-lost-passwords", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-06T12:45:06", - "trx_id": "98ac25b9c369c5099a73ff463fd94348013dfd3a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 701, - { - "block": 3879387, - "op": { - "type": "vote_operation", - "value": { - "author": "bondor", - "permlink": "proposal-timely-vs-timeless-content-longform-content-incentives-and-scalability-for-steemit", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-07T16:56:33", - "trx_id": "208023f038d33c3bcdf25ca58045f602f80f08e6", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 704, - { - "block": 3881834, - "op": { - "type": "vote_operation", - "value": { - "author": "arcurus", - "permlink": "tagging-and-flagging-hidden-by-a-whale-how-to-evolve-further", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-07T18:59:30", - "trx_id": "a2e469c417b74ef6e078dea4b131bc4c22bc30ca", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 706, - { - "block": 3882252, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "arcurus", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-07T19:20:30", - "trx_id": "05b967d791974def5a68809519a5c6ae23ae9e35", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 710, - { - "block": 3883243, - "op": { - "type": "vote_operation", - "value": { - "author": "deviedev", - "permlink": "environmental-attorney-girl-with-a-guy-s-name-here-s-my-story", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-07T20:10:18", - "trx_id": "d96e50b98d599f045030f9714fa6fab8de0f7c97", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 714, - { - "block": 3886533, - "op": { - "type": "vote_operation", - "value": { - "author": "thebluepanda", - "permlink": "make-art-not-plagiarism", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-07T22:55:21", - "trx_id": "e17d9fc1c6ddaee6340237d3cef39452befb49db", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 728, - { - "block": 3898184, - "op": { - "type": "vote_operation", - "value": { - "author": "nastik", - "permlink": "friendship-at-large-distances-in-steemit-new-acquaintances-and-new-communication", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-08T08:39:42", - "trx_id": "b874373d1c51757e9b97f6cdc2d6d61e3f751598", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 736, - { - "block": 3902274, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "spaninv", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-08T12:04:45", - "trx_id": "78056f5d25e9733cfe15b5265bfe66573b72a8f7", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 744, - { - "block": 3909046, - "op": { - "type": "vote_operation", - "value": { - "author": "falkvinge", - "permlink": "the-founder-of-the-pirate-party-brings-liberty-ideas-to-steem", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-08T17:48:42", - "trx_id": "46f28efb50868b8dacb32d55a5f3fcdd4575207d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 755, - { - "block": 3923218, - "op": { - "type": "vote_operation", - "value": { - "author": "geoffrey", - "permlink": "witness-geoffrey", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T05:43:54", - "trx_id": "2045a6750e52ecd66fb8c799da0b3489747ce392", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 762, - { - "block": 3925915, - "op": { - "type": "vote_operation", - "value": { - "author": "heiditravels", - "permlink": "upvote-to-improve-the-lives-of-those-who-need-it-most", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T07:58:48", - "trx_id": "6ed36c23e97b127f40e9f2e992b1904870f66ead", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 770, - { - "block": 3933091, - "op": { - "type": "vote_operation", - "value": { - "author": "liondani", - "permlink": "steemit-idea-agjust-properly-the-reputation-numbers-because-they-are-missleading", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T13:57:42", - "trx_id": "1611a0fe91d15a9425d862f867cbef3d26167b5e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 778, - { - "block": 3939464, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "leticiapink", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-09T19:18:51", - "trx_id": "94a16e58927a3542ae9754346139426f5d2015ff", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 805, - { - "block": 3952159, - "op": { - "type": "vote_operation", - "value": { - "author": "xeroc", - "permlink": "steem-transaction-signing-in-a-nutshell", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-10T05:56:30", - "trx_id": "3e1f1925609902839a57e1cc78a5d9bc05849590", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 814, - { - "block": 3955603, - "op": { - "type": "vote_operation", - "value": { - "author": "wackou", - "permlink": "btstools-development-bounties", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-10T08:49:06", - "trx_id": "27eab6da06303345bf21cca9b0009033f366f408", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 819, - { - "block": 3956998, - "op": { - "type": "vote_operation", - "value": { - "author": "arhag", - "permlink": "witness-arhag-update-aug-1-2016-to-aug-8-2016", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-10T09:59:06", - "trx_id": "70fbbe86e2c8024c9968767d84ddad94ba0551d9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 850, - { - "block": 3972802, - "op": { - "type": "vote_operation", - "value": { - "author": "thebluepanda", - "permlink": "albania-hidden-in-the-mediterranean", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-10T23:11:30", - "trx_id": "050cfe1f8b2a4b187de5d77999c17c5ead3da9e8", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 872, - { - "block": 3984692, - "op": { - "type": "vote_operation", - "value": { - "author": "cheetah", - "permlink": "cheetah-bot-explained", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T09:06:45", - "trx_id": "8208e4fda3bd42096dbd970391ff59f0b3d20bba", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 874, - { - "block": 3985180, - "op": { - "type": "comment_operation", - "value": { - "author": "gtg", - "body": "Of course It is truly right. It doesn't manipulate the free market. It says: _\"Hey, better watch out, fake products over there!\"_, so other buyers (upvoters) have choice to follow that advice or not. That's because of that freedom, not against it. Besides, I like cats. :-)", - "json_metadata": "{\"tags\":[\"steemitabuse\"]}", - "parent_author": "heretickitten", - "parent_permlink": "re-cheetah-cheetah-bot-explained-20160811t033141148z", - "permlink": "re-heretickitten-re-cheetah-cheetah-bot-explained-20160811t093103978z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T09:31:09", - "trx_id": "f8d0ac4efa8b392935b82eed25678170853e98ff", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 876, - { - "block": 3985184, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "re-heretickitten-re-cheetah-cheetah-bot-explained-20160811t093103978z", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T09:31:21", - "trx_id": "b32dc2a49e44e1ac4b436f19e9afd222008f3ca6", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 881, - { - "block": 3986354, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "re-heretickitten-re-cheetah-cheetah-bot-explained-20160811t093103978z", - "voter": "arcurus", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T10:30:00", - "trx_id": "14e60be0d776f4aeaf8017e101d85eb1425a999a", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 883, - { - "block": 3986644, - "op": { - "type": "vote_operation", - "value": { - "author": "steemwriters", - "permlink": "weekly-contests-to-reward-fiction-writers", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T10:44:30", - "trx_id": "b8ea65a043f1b5757fed5a55d0ce3b7dca6afb9c", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 892, - { - "block": 3990315, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "re-heretickitten-re-cheetah-cheetah-bot-explained-20160811t093103978z", - "voter": "brendio", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T13:48:33", - "trx_id": "c1ae65599f33d9894e1a3df5d1e5f6bba2ca02ce", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 902, - { - "block": 3994822, - "op": { - "type": "comment_operation", - "value": { - "author": "gtg", - "body": "`overmind`sounds good, but maybe morph it to `overmint`\nWhere `mint`is like in _\u201che made a mint on the stock market\u201d_ and/or like in _\"The United States Mint\"_ + of course like misspelled _\"mind\"_.", - "json_metadata": "{\"tags\":[\"steem\"]}", - "parent_author": "wackou", - "parent_permlink": "btstools-development-bounties", - "permlink": "re-wackou-btstools-development-bounties-20160811t173426180z", - "title": "" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T17:34:33", - "trx_id": "97be0ae220643dc8aff2633140c65ea750f77c41", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 904, - { - "block": 3994825, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "re-wackou-btstools-development-bounties-20160811t173426180z", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T17:34:42", - "trx_id": "d8e45363b2a250709348f9ecf806795c7c3adb3c", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 944, - { - "block": 4009418, - "op": { - "type": "vote_operation", - "value": { - "author": "nastik", - "permlink": "how-to-make-a-brighter-summer-with-their-hands-art-on-steemit-art-and-inspiration-weave-ribbons", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-12T05:52:03", - "trx_id": "f0372dd4a9dddafb644fb6658c812befc8d15035", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 953, - { - "block": 4012164, - "op": { - "type": "vote_operation", - "value": { - "author": "chris.roy", - "permlink": "english-lift-the-german-community-to-the-next-level-hello-i-m-chris", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-12T08:09:27", - "trx_id": "aed87bf0e72ad597a82ecde84630aa9a670a3985", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 979, - { - "block": 4022851, - "op": { - "type": "vote_operation", - "value": { - "author": "peerplays", - "permlink": "announcement-bts-snapshot-date", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-12T17:04:30", - "trx_id": "576a52a04b53da26c550d15c479e392e52d4b20d", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 990, - { - "block": 4025464, - "op": { - "type": "vote_operation", - "value": { - "author": "gtg", - "permlink": "witness-gtg", - "voter": "proctologic", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-12T19:15:24", - "trx_id": "aff8fe63811c4d34f766651c4872f9d1ba75ac4e", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/vote_and_comment_ops.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/vote_and_comment_ops.tavern.yaml deleted file mode 100644 index 4c1db7644540514eea5e7dca0b3d34687255666e..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/vote_and_comment_ops.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api votes and comments operations - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api votes with comments ops - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"gtg", "operation_filter_low": 3, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "vote_and_comment_ops" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/vote_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/vote_operation.pat.json deleted file mode 100644 index d988d7a5cc5154c0ee3e0188e0dabd3b8731ee1f..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/vote_operation.pat.json +++ /dev/null @@ -1,1243 +0,0 @@ -{ - "history": [ - [ - 204351, - { - "block": 4966967, - "op": { - "type": "vote_operation", - "value": { - "author": "lenatramper", - "permlink": "if-the-whole-earth-would-speak-like-adolf-hitler", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T15:59:03", - "trx_id": "ae0f2dce53fb096f46254c20e777e9f5a1e41fa5", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 204400, - { - "block": 4967866, - "op": { - "type": "vote_operation", - "value": { - "author": "themanualbot", - "permlink": "steemit-error-your-facebook-account-has-no-associated-email-address-please-add-email-to-your-facebook-account-and-try-again", - "voter": "blocktrades", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T16:44:00", - "trx_id": "e7ead21286b2d43ed1ef04e679520b1c3b46eca2", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 204405, - { - "block": 4967934, - "op": { - "type": "vote_operation", - "value": { - "author": "iontom", - "permlink": "breaking-news-biotech-giant-bayer-to-acquire-monsanto-in-usd57-billion-deal-largest-takeover-ever-in-german-history", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T16:47:24", - "trx_id": "daf98ec72a76db4e4ca6ebfe4332fd17f56c0506", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 204408, - { - "block": 4967948, - "op": { - "type": "vote_operation", - "value": { - "author": "ketzer85", - "permlink": "space-art", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T16:48:06", - "trx_id": "2f6231bfc375e5672533a587371f23e46065da1f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 204417, - { - "block": 4968079, - "op": { - "type": "vote_operation", - "value": { - "author": "transhuman", - "permlink": "selfies-are-good-for-you-say-scientists", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T16:54:42", - "trx_id": "a2464f66cce446a14a0a7fb19d17edd23fbc0eff", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 204437, - { - "block": 4968404, - "op": { - "type": "vote_operation", - "value": { - "author": "funny", - "permlink": "why-are-bitcoin-steem-and-other-cryptocoins-worth-real-money", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:11:42", - "trx_id": "606b78845d70904930c81d58cb5e8db6181d4b2b", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 204456, - { - "block": 4968700, - "op": { - "type": "vote_operation", - "value": { - "author": "varda", - "permlink": "le-pagnon-borain-belgian-recipe", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:27:18", - "trx_id": "b04ffcb1663c132e41691d66b40915731afbdbae", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 204458, - { - "block": 4968704, - "op": { - "type": "vote_operation", - "value": { - "author": "alex2016", - "permlink": "look-at-my-new-adventures-with-the-camera", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:27:30", - "trx_id": "0db4c7f56ef4a96728b8fa69a157f4d64178a1a0", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 204489, - { - "block": 4969155, - "op": { - "type": "vote_operation", - "value": { - "author": "ketzer85", - "permlink": "space-art", - "voter": "blocktrades", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T17:51:15", - "trx_id": "4cd4c915b526f37108bafb5a1168be3158ea8877", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 204558, - { - "block": 4970385, - "op": { - "type": "vote_operation", - "value": { - "author": "patimaker", - "permlink": "the-next-sketch", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T18:55:54", - "trx_id": "023a76ea90753e78359a750c1dd6b3fe1fa9a705", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 204562, - { - "block": 4970430, - "op": { - "type": "vote_operation", - "value": { - "author": "zaitsevalesyaa", - "permlink": "the-art-of-origami-flowers", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T18:58:15", - "trx_id": "e28ed63aaf8cf287b10f66cf6078cd1f777c84df", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 204568, - { - "block": 4970521, - "op": { - "type": "vote_operation", - "value": { - "author": "mbroek1983", - "permlink": "time-to-sleep", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T19:03:00", - "trx_id": "c9bf5fb9743f473cb6fa43d2e92283d5a6491303", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 204573, - { - "block": 4970565, - "op": { - "type": "vote_operation", - "value": { - "author": "markrmorrisjr", - "permlink": "original-fiction-jacked-book-one-of-the-origin-dime-chronicles-episode-9", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T19:05:21", - "trx_id": "cbcb00d7d71f39ca17c91a92ceed37ad3d2bae12", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 204588, - { - "block": 4970829, - "op": { - "type": "vote_operation", - "value": { - "author": "despina", - "permlink": "greek-lasagne-makaronia-tou-fournou-pastitsio", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T19:19:18", - "trx_id": "a0948beceea0a23091b89b0d6ebcc6e4d8348767", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 204591, - { - "block": 4970850, - "op": { - "type": "vote_operation", - "value": { - "author": "nicoledphoto", - "permlink": "how-to-create-image-overlay-on-shapes-and-text-photoshop", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T19:20:21", - "trx_id": "760d222bc682dc32494dda64d858265d08a6e823", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 204632, - { - "block": 4971572, - "op": { - "type": "vote_operation", - "value": { - "author": "karisa", - "permlink": "vegetable-soup-puree-recipe-specially-for-steemit", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T19:56:36", - "trx_id": "1edda6935bbc26e2d2100b7bf8eefdf53cc7cf13", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 204641, - { - "block": 4971679, - "op": { - "type": "vote_operation", - "value": { - "author": "fairz", - "permlink": "scooby-doo-and-the-stoner-van-animation", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T20:01:57", - "trx_id": "e593e526d475ab0dc230fb1d00ae004a825a632e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 204661, - { - "block": 4972063, - "op": { - "type": "vote_operation", - "value": { - "author": "whatsup", - "permlink": "hot-chick-pic-of-the-day", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T20:21:09", - "trx_id": "4f59e5983ea0803342a7f842d3817d1479e76c17", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 204664, - { - "block": 4972077, - "op": { - "type": "vote_operation", - "value": { - "author": "rebelmeow", - "permlink": "learning-happiness-from-an-old-photograph", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T20:21:51", - "trx_id": "9cdb71a992910e86e8834745d65af1f5226074b1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 204853, - { - "block": 4975801, - "op": { - "type": "vote_operation", - "value": { - "author": "faddat", - "permlink": "go-code-review-request-multithreading-ingestron-the-steem-blockchain-eater", - "voter": "blocktrades", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T23:28:15", - "trx_id": "d6e0bb434788c8abc09dc1404d5d86da7009cc1b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 204856, - { - "block": 4975818, - "op": { - "type": "vote_operation", - "value": { - "author": "sauravrungta", - "permlink": "craziest-laws-from-around-the-world", - "voter": "blocktrades", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T23:29:06", - "trx_id": "5fea8e7b6c14df4acc8ae273e144ddb3709b7b5c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 204860, - { - "block": 4975870, - "op": { - "type": "vote_operation", - "value": { - "author": "alexbeyman", - "permlink": "don-t-get-tricked-bro-how-to-recognize-a-publisher-s-clearing-house-scam", - "voter": "blocktrades", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T23:31:42", - "trx_id": "904268e10a4bc534ae79c8c5d03f6e1aaf272491", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 204864, - { - "block": 4975908, - "op": { - "type": "vote_operation", - "value": { - "author": "carlidos", - "permlink": "psycho-the-cat-is-a-tv-watching-card-playing-weather-predictor", - "voter": "blocktrades", - "weight": 5000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-14T23:33:36", - "trx_id": "e5b07cc880cc8c5b018f4b9f270afe1f85adf224", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 205025, - { - "block": 4979080, - "op": { - "type": "vote_operation", - "value": { - "author": "dgiors", - "permlink": "spider-macro-looks-larger-than-life", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T02:12:18", - "trx_id": "a81096d7ecfbf68e37361976921ce79ada90cb5f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205053, - { - "block": 4979605, - "op": { - "type": "vote_operation", - "value": { - "author": "mcsvi", - "permlink": "black-story-challenge-2-can-you-solve-the-riddle", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T02:38:33", - "trx_id": "7054a1dc368cf538d9e85d9d073c79ae8ad8f1f9", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 205064, - { - "block": 4979763, - "op": { - "type": "vote_operation", - "value": { - "author": "anarchobanker", - "permlink": "truth-is-truth-no-matter-who-says-it", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T02:46:27", - "trx_id": "fe17816ed651f4305abc4567d5b3b5afc3246a94", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 205104, - { - "block": 4980517, - "op": { - "type": "vote_operation", - "value": { - "author": "thedevil", - "permlink": "re-eveningstar92-shit-happens-move-on-nsfw-evening-star-art-20160915t013547486z", - "voter": "blocktrades", - "weight": -10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T03:24:12", - "trx_id": "f4529a0400da6540970b9b55b4ebe0f07013d60f", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 205110, - { - "block": 4980590, - "op": { - "type": "vote_operation", - "value": { - "author": "gardenofeden", - "permlink": "exciting-steemit-announcement-to-build-this-economy-and-support-this-revolutionary-platform-our-online-store-is-now-accepting", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T03:27:51", - "trx_id": "566956258628a97ecf968aedc8a60b10392414f9", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205121, - { - "block": 4980776, - "op": { - "type": "vote_operation", - "value": { - "author": "bola", - "permlink": "sentence-unscrambler-7", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T03:37:09", - "trx_id": "6921cfcddb78cfab56e1f14b810f5deca52460c6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205131, - { - "block": 4980920, - "op": { - "type": "vote_operation", - "value": { - "author": "ilovesteemit", - "permlink": "can-you-see-all-twelve-dots-in-this-image-nope-you-cannot", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T03:44:24", - "trx_id": "b73e47b09280cfe2251ebbf9959c6524e90bb8e4", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205146, - { - "block": 4981164, - "op": { - "type": "vote_operation", - "value": { - "author": "kingscrown", - "permlink": "zerocash-to-be-added-to-bitmex-trading-in-less-than-24h", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T03:56:36", - "trx_id": "347f18f16b74365c7bb78133ffb38dfd641ded80", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205154, - { - "block": 4981267, - "op": { - "type": "vote_operation", - "value": { - "author": "markrmorrisjr", - "permlink": "original-fiction-the-anarchist-s-almanac-episode-14", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T04:01:45", - "trx_id": "e533b8d6d998fd1cea387fed8a8317ae3813e0f5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205450, - { - "block": 4987311, - "op": { - "type": "vote_operation", - "value": { - "author": "blocktrades", - "permlink": "witness-report-for-blocktrades-for-last-week-of-august", - "voter": "pakganern", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T09:04:42", - "trx_id": "b89762657465f62895ff3c8acab554a69a5bab1e", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 205575, - { - "block": 4989982, - "op": { - "type": "vote_operation", - "value": { - "author": "ahmetova", - "permlink": "with-cryptocurrency-the-world-has-gone-mad", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T11:18:48", - "trx_id": "97d78acca445d63b3695fc2b359550d8ccf48a33", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205580, - { - "block": 4990038, - "op": { - "type": "vote_operation", - "value": { - "author": "safar01", - "permlink": "a-variety-of-unique-creations-made-from-eggs", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T11:21:36", - "trx_id": "317f380d3a3a4539c1a117f04bac9e02380ab5dd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205582, - { - "block": 4990049, - "op": { - "type": "vote_operation", - "value": { - "author": "patelincho", - "permlink": "scary-little-things-here-come-a-big-one", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T11:22:09", - "trx_id": "ecbb51326517014a8aec3f5ed41b19d7edcfb578", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205586, - { - "block": 4990080, - "op": { - "type": "vote_operation", - "value": { - "author": "byandreq-art", - "permlink": "this-is-my-style-to-draw-buildings", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T11:23:42", - "trx_id": "364983906c26f5ed0323ebcf91e13fc98b6fd062", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 205661, - { - "block": 4991574, - "op": { - "type": "vote_operation", - "value": { - "author": "rampant", - "permlink": "steemit-daily-drawing-tutorial-gesture-drawings-plus-tool", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:38:33", - "trx_id": "2f0e2ca0e29dcd208c6ffc147791c0c3553b7d7b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 205667, - { - "block": 4991643, - "op": { - "type": "vote_operation", - "value": { - "author": "bruno1122", - "permlink": "it-s-time-to-kill-food-tradition-it-s-time-for-a-drastic-change-to-shopping-centres", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:42:00", - "trx_id": "f69dcb98ab06dc7af3a28095c1722a38c1401dfe", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 205676, - { - "block": 4991803, - "op": { - "type": "vote_operation", - "value": { - "author": "vongohren", - "permlink": "how-american-credit-bankins-is-f-ed-up", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:50:00", - "trx_id": "e31c3b354e718325565a6322260e596b18c680d6", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 205680, - { - "block": 4991846, - "op": { - "type": "vote_operation", - "value": { - "author": "penguinpablo", - "permlink": "how-to-carve-an-apple-leaf", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:52:09", - "trx_id": "f4c7c33a0079cb31096fd57ed93440fcc131f9dc", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205683, - { - "block": 4991867, - "op": { - "type": "vote_operation", - "value": { - "author": "nathanjtaylor", - "permlink": "fire-and-grace-or-art-painting-and-poetry", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T12:53:12", - "trx_id": "02d64522985b730ad8d213e58390528d4628f1a9", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 205782, - { - "block": 4993742, - "op": { - "type": "vote_operation", - "value": { - "author": "yetaras", - "permlink": "in-search-of-mushrooms-part-3", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T14:27:00", - "trx_id": "778ce1f6ec3c8f3d775932e2ac5cca97c5f5b0b8", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 205896, - { - "block": 4995885, - "op": { - "type": "vote_operation", - "value": { - "author": "funnyman", - "permlink": "first-steemit-meetup-in-india-the-worlds-second-biggest-market", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T16:14:15", - "trx_id": "872d4c6c72ddcea2259bc95a46fad289e9e39a30", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 205899, - { - "block": 4995909, - "op": { - "type": "vote_operation", - "value": { - "author": "jonnybitcoin", - "permlink": "results-from-bitshares-facebook-ad-campaign", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T16:15:27", - "trx_id": "8ce5a1594b3cd301dd3331fb237cb44e89da3022", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 205939, - { - "block": 4996648, - "op": { - "type": "vote_operation", - "value": { - "author": "woman-onthe-wing", - "permlink": "my-forest-cats", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T16:52:24", - "trx_id": "7545af4640130419e626c52193fdbd39408d195f", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 205944, - { - "block": 4996694, - "op": { - "type": "vote_operation", - "value": { - "author": "larrytom", - "permlink": "my-brother-s-heroin-addiction-destroyed-our-family", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T16:54:42", - "trx_id": "1c3c95e085ec3060d98def82c8ec5dee5ec8f573", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 205974, - { - "block": 4997168, - "op": { - "type": "vote_operation", - "value": { - "author": "powerup", - "permlink": "17-steem-tools-every-steemian-needs-to-know", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T17:18:24", - "trx_id": "706c6f48cd8e032d1436216e36f14fba88f42066", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 206049, - { - "block": 4998464, - "op": { - "type": "vote_operation", - "value": { - "author": "markrmorrisjr", - "permlink": "original-fiction-anarchist-s-almanac-episode-15", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:26:00", - "trx_id": "1015c32170fb69eace16df1316946141eebe048f", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 206053, - { - "block": 4998501, - "op": { - "type": "vote_operation", - "value": { - "author": "groovedigital", - "permlink": "when-your-dad-works-in-hollywood", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:27:57", - "trx_id": "c796ef246f261c62c76f6a387b844562d8beb1e4", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 206059, - { - "block": 4998571, - "op": { - "type": "vote_operation", - "value": { - "author": "alienbutt", - "permlink": "alien-or-plant", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:31:45", - "trx_id": "a2cf92f1b7027ff69e2dd7722b0d185ed73ba68f", - "trx_in_block": 12, - "virtual_op": 0 - } - ], - [ - 206064, - { - "block": 4998619, - "op": { - "type": "vote_operation", - "value": { - "author": "tanyabtc", - "permlink": "jellyfish", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:34:27", - "trx_id": "a5e9d1ee89b061cf1f7f642e17e814f62cba3cfd", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 206066, - { - "block": 4998627, - "op": { - "type": "vote_operation", - "value": { - "author": "dresden", - "permlink": "re-tanyabtc-jellyfish-20160915t173950477z", - "voter": "blocktrades", - "weight": 2000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:34:51", - "trx_id": "91e131e9a83e3ba2bfc9ac5757e4f2dfb76836f0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 206075, - { - "block": 4998749, - "op": { - "type": "vote_operation", - "value": { - "author": "titusfrost", - "permlink": "new-mr-robot-episode-introduces-e-corp-s-new-ecoin", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:41:18", - "trx_id": "ba5ea077814fce3c9360d815635301a14167c8b7", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 206082, - { - "block": 4998814, - "op": { - "type": "vote_operation", - "value": { - "author": "mihaiart", - "permlink": "the-queen-of-amazon-drawing-a-visual-progression", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:44:45", - "trx_id": "754e4acdbeb088480544639901e38352e844eedb", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 206088, - { - "block": 4998907, - "op": { - "type": "vote_operation", - "value": { - "author": "abudar", - "permlink": "artwork-from-cigarette-wrap", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T18:49:45", - "trx_id": "749c2f29cdfd5dd4395f728ff4aff73c3791fcba", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 206127, - { - "block": 4999574, - "op": { - "type": "vote_operation", - "value": { - "author": "juanmiguelsalas", - "permlink": "how-the-number-pi-sounds-on-a-piano", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T19:24:54", - "trx_id": "e80b9af41ff4d110e4087cf8d6874a35d7734c51", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 206138, - { - "block": 4999714, - "op": { - "type": "vote_operation", - "value": { - "author": "jackgallenhall", - "permlink": "how-to-make-paper-flowers", - "voter": "blocktrades", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T19:32:12", - "trx_id": "48ce10a00e845ffe9a33728d71c6506f1e6f5f95", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 206150, - { - "block": 4999915, - "op": { - "type": "vote_operation", - "value": { - "author": "larrytom", - "permlink": "my-brother-s-heroin-addiction-destroyed-our-family", - "voter": "blocktrades", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-15T19:42:48", - "trx_id": "db81c06d8d156efbb8e4627feabe8093abdcaf3b", - "trx_in_block": 1, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/vote_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/vote_operation.tavern.yaml deleted file mode 100644 index d4f3834b4c78369920a0a60c19a21008ff6d4ebc..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/vote_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to vote_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history vote_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades","operation_filter_low": 1,"start": 206151, "limit": 100} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "vote_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/withdraw_vesting_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/withdraw_vesting_operation.pat.json deleted file mode 100644 index a25ff23d3c6d7a9c5884c6b31e51fe11a21a668d..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/withdraw_vesting_operation.pat.json +++ /dev/null @@ -1,50 +0,0 @@ -{ - "history": [ - [ - 15002, - { - "block": 4795273, - "op": { - "type": "withdraw_vesting_operation", - "value": { - "account": "ned", - "vesting_shares": { - "amount": "0", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-08T16:18:51", - "trx_id": "65860eedd33d04ec629589587d8580a413f0ed60", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 15452, - { - "block": 4887035, - "op": { - "type": "withdraw_vesting_operation", - "value": { - "account": "ned", - "vesting_shares": { - "amount": "12650170467499916", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-09-11T21:06:48", - "trx_id": "d7c7253bdfd793cb535398c9195a181a3915c7aa", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/withdraw_vesting_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/withdraw_vesting_operation.tavern.yaml deleted file mode 100644 index 3899520dc4638d969a398b01ed179c0b622b71b2..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/withdraw_vesting_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to withdraw_vesting_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history withdraw_vesting_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"ned","operation_filter_low": 16, "start": 15452, "limit": 2} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "withdraw_vesting_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/witness_set_properties_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/witness_set_properties_operation.pat.json deleted file mode 100644 index 27b1cc3d5837f3ff5afad45e0470cbd768f18343..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/witness_set_properties_operation.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "history": [] - } - \ No newline at end of file diff --git a/hived/tavern/account_history_api_patterns/get_account_history/witness_set_properties_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/witness_set_properties_operation.tavern.yaml deleted file mode 100644 index ba708649f497f301e75c7de4a81b0f37a15b97b6..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/witness_set_properties_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api witness_set_properties_operation - - marks: - - patterntest # no noempty result in 5mln blocks - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api witness_set_properties_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"blocktrades", "operation_filter_low": 4398046511104, "start": 1000, "limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "witness_set_properties_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_account_history/witness_update_operation.pat.json b/hived/tavern/account_history_api_patterns/get_account_history/witness_update_operation.pat.json deleted file mode 100644 index 8e69c654bc9dbd58928c4de8afc46827b5b40971..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/witness_update_operation.pat.json +++ /dev/null @@ -1,174 +0,0 @@ -{ - "history": [ - [ - 114, - { - "block": 2881920, - "op": { - "type": "witness_update_operation", - "value": { - "block_signing_key": "STM5F9tCbND6zWPwksy1rEN24WjPiQWSU2vwGgegQVjAcYDe1zTWi", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "owner": "gtg", - "props": { - "account_creation_fee": { - "amount": "9999", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "url": "https://steemit.com/introduceyourself/@gtg/hello-world" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-03T18:04:21", - "trx_id": "d1f352e5d1834cbf166166c4810b752d021f0fc0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 324, - { - "block": 3134487, - "op": { - "type": "witness_update_operation", - "value": { - "block_signing_key": "STM776t8h7dXbvM8BYGoLjCr3nYRnmqmvVg9hTrGTn5FQvLkMZKM2", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "owner": "gtg", - "props": { - "account_creation_fee": { - "amount": "9999", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "url": "https://steemit.com/introduceyourself/@gtg/hello-world" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-12T13:52:36", - "trx_id": "4cee4cbe4e1637e6745bde8ad8eb5c2559ae818b", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 332, - { - "block": 3153076, - "op": { - "type": "witness_update_operation", - "value": { - "block_signing_key": "STM776t8h7dXbvM8BYGoLjCr3nYRnmqmvVg9hTrGTn5FQvLkMZKM2", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "owner": "gtg", - "props": { - "account_creation_fee": { - "amount": "5000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "url": "https://steemit.com/introduceyourself/@gtg/hello-world" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-13T05:25:36", - "trx_id": "5dda11f0e7df6c556e7d4d6efdf32c3129fce98b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 422, - { - "block": 3682342, - "op": { - "type": "witness_update_operation", - "value": { - "block_signing_key": "STM776t8h7dXbvM8BYGoLjCr3nYRnmqmvVg9hTrGTn5FQvLkMZKM2", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "owner": "gtg", - "props": { - "account_creation_fee": { - "amount": "3000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 65536 - }, - "url": "https://steemit.com/introduceyourself/@gtg/hello-world" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-07-31T19:53:18", - "trx_id": "6d409ff427aac442de4475b844cd7033ef79a9ca", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 918, - { - "block": 3998630, - "op": { - "type": "witness_update_operation", - "value": { - "block_signing_key": "STM776t8h7dXbvM8BYGoLjCr3nYRnmqmvVg9hTrGTn5FQvLkMZKM2", - "fee": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "owner": "gtg", - "props": { - "account_creation_fee": { - "amount": "3000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 65536 - }, - "url": "https://steemit.com/witness-category/@gtg/witness-gtg" - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-08-11T20:50:36", - "trx_id": "90b1c097689f6a556b13f1223f7e4c3a979a402c", - "trx_in_block": 0, - "virtual_op": 0 - } - ] - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_account_history/witness_update_operation.tavern.yaml b/hived/tavern/account_history_api_patterns/get_account_history/witness_update_operation.tavern.yaml deleted file mode 100644 index 60d7e7a8e17bdf5fe4fb76fee9601250a8bfd983..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_account_history/witness_update_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_account_history filter to witness_update_operation - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_account_history witness_update_operation - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_account_history" - params: {"account":"gtg","operation_filter_low": 2048,"start": 1000,"limit": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "witness_update_operation" - directory: "account_history_api_patterns/get_account_history" diff --git a/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_false.pat.json b/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_false.pat.json deleted file mode 100644 index 4fef1b0c9a4ff540daf67dfc94facaec58342e75..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_false.pat.json +++ /dev/null @@ -1,67 +0,0 @@ -{ - "ops": [ - { - "block": 2797050, - "op": { - "type": "vote_operation", - "value": { - "author": "pfunk", - "permlink": "guide-maximize-your-mining-hashrate-in-windows-by-mining-steem-in-a-vm", - "voter": "gtg", - "weight": 10000 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:12:21", - "trx_id": "e0cd24f9aba3b668b1443786bee3891f644ff23f", - "trx_in_block": 0, - "virtual_op": 0 - }, - { - "block": 2797050, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pfunk", - "pending_payout": { - "amount": "812683", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "guide-maximize-your-mining-hashrate-in-windows-by-mining-steem-in-a-vm", - "rshares": 27547358, - "total_vote_weight": "20780999058208", - "voter": "gtg", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:12:21", - "trx_id": "e0cd24f9aba3b668b1443786bee3891f644ff23f", - "trx_in_block": 0, - "virtual_op": 1 - }, - { - "block": 2797050, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "roadscape", - "vesting_shares": { - "amount": "5400959205", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:12:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_false.tavern.yaml b/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_false.tavern.yaml deleted file mode 100644 index 29a6deb6b5d16e19bde13743fe8599909478b89f..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_false.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_ops_in_block only virtual false - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_ops_in_block only virtual false - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_ops_in_block" - params: {"block_num": 2797050, "only_virtual": false} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "only_virtual_false" - directory: "account_history_api_patterns/get_ops_in_block" diff --git a/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_true.pat.json b/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_true.pat.json deleted file mode 100644 index 684b92c1d2b2047da2dc340bccbed432b557cae1..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_true.pat.json +++ /dev/null @@ -1,49 +0,0 @@ -{ - "ops": [ - { - "block": 2797050, - "op": { - "type": "effective_comment_vote_operation", - "value": { - "author": "pfunk", - "pending_payout": { - "amount": "812683", - "nai": "@@000000013", - "precision": 3 - }, - "permlink": "guide-maximize-your-mining-hashrate-in-windows-by-mining-steem-in-a-vm", - "rshares": 27547358, - "total_vote_weight": "20780999058208", - "voter": "gtg", - "weight": 0 - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:12:21", - "trx_id": "e0cd24f9aba3b668b1443786bee3891f644ff23f", - "trx_in_block": 0, - "virtual_op": 1 - }, - { - "block": 2797050, - "op": { - "type": "producer_reward_operation", - "value": { - "producer": "roadscape", - "vesting_shares": { - "amount": "5400959205", - "nai": "@@000000037", - "precision": 6 - } - } - }, - "op_in_trx": 0, - "operation_id": 0, - "timestamp": "2016-06-30T19:12:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } - ] -} diff --git a/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_true.tavern.yaml b/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_true.tavern.yaml deleted file mode 100644 index 4ef556b43ae880e0bd0c1899ed33fcdb3ca84e5d..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_ops_in_block/only_virtual_true.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_ops_in_block only virtual true - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_ops_in_block only virtual true - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_ops_in_block" - params: {"block_num": 2797050, "only_virtual": true} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "only_virtual_true" - directory: "account_history_api_patterns/get_ops_in_block" diff --git a/hived/tavern/account_history_api_patterns/get_transaction/get_transaction.pat.json b/hived/tavern/account_history_api_patterns/get_transaction/get_transaction.pat.json deleted file mode 100644 index c35445b21bbb7f875948c0681c5d8b1fa193f980..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_transaction/get_transaction.pat.json +++ /dev/null @@ -1,23 +0,0 @@ -{ - "block_num": 2000000, - "expiration": "2016-06-02T23:58:54", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "pal", - "permlink": "re-dantheman-re-pal-httpssteemit-comsteempalsniper-whale-vote-bot-strategy-20160602t162811551z", - "voter": "proctologic", - "weight": 10000 - } - } - ], - "ref_block_num": 33918, - "ref_block_prefix": 2329120500, - "signatures": [ - "1f0ad8680045212314210892e338f14bc4fd34b2573e6217591b036be6222c5d980dbc2d3547429924389330e876ab650a1dd9548284d8a855c96b58c542d0a499" - ], - "transaction_id": "747e19a0a1511d162dfcb5258f62de520294982b", - "transaction_num": 0 -} diff --git a/hived/tavern/account_history_api_patterns/get_transaction/get_transaction.tavern.yaml b/hived/tavern/account_history_api_patterns/get_transaction/get_transaction.tavern.yaml deleted file mode 100644 index 3902f2e89b773120c289bf3e8f85b7a3d5c3611a..0000000000000000000000000000000000000000 --- a/hived/tavern/account_history_api_patterns/get_transaction/get_transaction.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "account_history_api.get_transaction" - params: {"id":"747e19a0a1511d162dfcb5258f62de520294982b"} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "get_transaction" - directory: "account_history_api_patterns/get_transaction" diff --git a/hived/tavern/block_api_patterns/get_block/milion.pat.json b/hived/tavern/block_api_patterns/get_block/milion.pat.json deleted file mode 100644 index eed7a341c868905aba9f5e931a2af994353b6876..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block/milion.pat.json +++ /dev/null @@ -1,14 +0,0 @@ -{ - "block": { - "block_id": "000f4240e8f91385f7bff8f5aeebddc9b14e4281", - "extensions": [], - "previous": "000f423f974857674873d93d1909e0eeb7e4916e", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:12:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f72bd3f4b06e7dc6b156729f0fd7873163814972eecea9d77cb29bae11d0fea3865c814d11a58e818c2494ce19f4c3d4c3e17eab3b1465ebccb102c52c56472c0" - } -} diff --git a/hived/tavern/block_api_patterns/get_block/milion.tavern.yaml b/hived/tavern/block_api_patterns/get_block/milion.tavern.yaml deleted file mode 100644 index 38728728d3e2e667acc0efdaac385552865a2b91..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block/milion.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived block_api.get_block milion - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block milion - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "block_api.get_block" - params: {"block_num": 1000000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "milion" - directory: "block_api_patterns/get_block" diff --git a/hived/tavern/block_api_patterns/get_block/non_existing.pat.json b/hived/tavern/block_api_patterns/get_block/non_existing.pat.json deleted file mode 100644 index 0967ef424bce6791893e9a57bb952f80fd536e93..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block/non_existing.pat.json +++ /dev/null @@ -1 +0,0 @@ -{} diff --git a/hived/tavern/block_api_patterns/get_block/non_existing.tavern.yaml b/hived/tavern/block_api_patterns/get_block/non_existing.tavern.yaml deleted file mode 100644 index 0702a967be43b0e476235b8b529d4dbf5e70fb23..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block/non_existing.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived block_api.get_block non existing - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block non existing - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "block_api.get_block" - params: {"block_num": 1000000000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "non_existing" - directory: "block_api_patterns/get_block" diff --git a/hived/tavern/block_api_patterns/get_block/one.pat.json b/hived/tavern/block_api_patterns/get_block/one.pat.json deleted file mode 100644 index e13ed74906fd04a0352289cec6bc66669abad7af..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block/one.pat.json +++ /dev/null @@ -1,14 +0,0 @@ -{ - "block": { - "block_id": "0000000109833ce528d5bbfb3f6225b39ee10086", - "extensions": [], - "previous": "0000000000000000000000000000000000000000", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204f8ad56a8f5cf722a02b035a61b500aa59b9519b2c33c77a80c0a714680a5a5a7a340d909d19996613c5e4ae92146b9add8a7a663eef37d837ef881477313043" - } -} diff --git a/hived/tavern/block_api_patterns/get_block/one.tavern.yaml b/hived/tavern/block_api_patterns/get_block/one.tavern.yaml deleted file mode 100644 index d374d43fd12ee1f38ce07f072b73602dc52a135c..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block/one.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived block_api.get_block - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "block_api.get_block" - params: {"block_num": 1} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "one" - directory: "block_api_patterns/get_block" diff --git a/hived/tavern/block_api_patterns/get_block_header/milion.pat.json b/hived/tavern/block_api_patterns/get_block_header/milion.pat.json deleted file mode 100644 index fb31fb129119f8405370df2d05385af0291cf9a0..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_header/milion.pat.json +++ /dev/null @@ -1,9 +0,0 @@ -{ - "header": { - "extensions": [], - "previous": "000f423f974857674873d93d1909e0eeb7e4916e", - "timestamp": "2016-04-29T04:12:00", - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "witness": "abit" - } -} diff --git a/hived/tavern/block_api_patterns/get_block_header/milion.tavern.yaml b/hived/tavern/block_api_patterns/get_block_header/milion.tavern.yaml deleted file mode 100644 index 0cfb981c9641c332b5654d624ae8611a8a32c325..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_header/milion.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived block_api.get_block_header milion - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block milion - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "block_api.get_block_header" - params: {"block_num": 1000000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "milion" - directory: "block_api_patterns/get_block_header" diff --git a/hived/tavern/block_api_patterns/get_block_header/non_existing.pat.json b/hived/tavern/block_api_patterns/get_block_header/non_existing.pat.json deleted file mode 100644 index 0967ef424bce6791893e9a57bb952f80fd536e93..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_header/non_existing.pat.json +++ /dev/null @@ -1 +0,0 @@ -{} diff --git a/hived/tavern/block_api_patterns/get_block_header/non_existing.tavern.yaml b/hived/tavern/block_api_patterns/get_block_header/non_existing.tavern.yaml deleted file mode 100644 index 494699b717e0f6ef4c200cb19b5f9328412d89e6..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_header/non_existing.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived block_api.get_block_header non existing - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block_header non existing - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "block_api.get_block" - params: {"block_num": 1000000000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "non_existing" - directory: "block_api_patterns/get_block_header" diff --git a/hived/tavern/block_api_patterns/get_block_header/one.pat.json b/hived/tavern/block_api_patterns/get_block_header/one.pat.json deleted file mode 100644 index a432b4b341a9a0955885a239c39afee1a5d2ff46..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_header/one.pat.json +++ /dev/null @@ -1,9 +0,0 @@ -{ - "header": { - "extensions": [], - "previous": "0000000000000000000000000000000000000000", - "timestamp": "2016-03-24T16:05:00", - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "witness": "initminer" - } -} diff --git a/hived/tavern/block_api_patterns/get_block_header/one.tavern.yaml b/hived/tavern/block_api_patterns/get_block_header/one.tavern.yaml deleted file mode 100644 index 9515559f532bde3a3eafb2562ec3b4ad94939ffe..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_header/one.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived block_api.get_block_header - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block_header - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "block_api.get_block_header" - params: {"block_num": 1} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "one" - directory: "block_api_patterns/get_block_header" diff --git a/hived/tavern/block_api_patterns/get_block_range/milion.pat.json b/hived/tavern/block_api_patterns/get_block_range/milion.pat.json deleted file mode 100644 index 732975944b1d79a06eeb7b520d6455e6f2565907..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_range/milion.pat.json +++ /dev/null @@ -1,14632 +0,0 @@ -{ - "blocks": [ - { - "block_id": "000f4240e8f91385f7bff8f5aeebddc9b14e4281", - "extensions": [], - "previous": "000f423f974857674873d93d1909e0eeb7e4916e", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:12:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f72bd3f4b06e7dc6b156729f0fd7873163814972eecea9d77cb29bae11d0fea3865c814d11a58e818c2494ce19f4c3d4c3e17eab3b1465ebccb102c52c56472c0" - }, - { - "block_id": "000f42415d6176113f39235f61e791149b871432", - "extensions": [], - "previous": "000f4240e8f91385f7bff8f5aeebddc9b14e4281", - "signing_key": "STM73XgqmDfLgXVF5VArK9rvHbUDbbx3HtfCU81Fy9MqVNxLYMpay", - "timestamp": "2016-04-29T04:12:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "jabbasteem", - "witness_signature": "2017d58cce890c814fca755af3fb5ee9be0922b68eaabb151b1b78d10f217dd86068e96068b6a071c06d4345e28c3994b94bbfa831c5f5473ed0737b62b2a9f3f0" - }, - { - "block_id": "000f4242fc4d3dd4b9d2fe91b8d399073ee2289c", - "extensions": [], - "previous": "000f42415d6176113f39235f61e791149b871432", - "signing_key": "STM8RVh9ZnJZhULNSQwRUueSJSKtJUjNMPbnyRdWmEJAVCTJZ5AYT", - "timestamp": "2016-04-29T04:12:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr402", - "witness_signature": "200d5393201561fda1ac271a8edfc801ff7106ba0a6dca4da4e2f0f061fef97d381c0f6c8659b460eda26b16dcb9a13acb78ce7ffa261ffea5dc35b2b28ebb9efd" - }, - { - "block_id": "000f42436e5fce7be846e014d3b04c4bd71a6012", - "extensions": [], - "previous": "000f4242fc4d3dd4b9d2fe91b8d399073ee2289c", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:12:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f543675b9d7dbe9b078ff3be9b1d1fe894bb22a0779c74fb31039283126fc7df4180371b6403bb99e69f2e0695ca9992421ffe9e7264dd88a1d7beb8106d9c0ef" - }, - { - "block_id": "000f4244c10540b8649aada2acdfae08e61417fe", - "extensions": [], - "previous": "000f42436e5fce7be846e014d3b04c4bd71a6012", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:12:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "2021518fd25e8653927013cb57877d026c846a2342041c3e3db090e98f0f03d26475d34b9e2e0201bb351178c6aa2ed6c0acfd113b1458402e5023b781b64c029a" - }, - { - "block_id": "000f42456072eba54976d53f6fbd8e852c7ce5cb", - "extensions": [], - "previous": "000f4244c10540b8649aada2acdfae08e61417fe", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:12:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f6c1a4c4169824c5eeb30167121f8e534a93c8b35e16f7018cdbe49aff795ca564c95c5efb88b8054894c42a0cef69bdf27794053b4bb347b99e59e14b6fe1ff3" - }, - { - "block_id": "000f4246d04e29f229126c31565099e2f87f269d", - "extensions": [], - "previous": "000f42456072eba54976d53f6fbd8e852c7ce5cb", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:12:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f755a092732e224da40323dcac34e46362eca4b8cd42cb5859991555c9752750d46bd185518f81fcb0d920128ecd2ccabd2ebabdee0451b164971dabc0ed160d2" - }, - { - "block_id": "000f42479d7b7b7fc334681456d0f234fa05167c", - "extensions": [], - "previous": "000f4246d04e29f229126c31565099e2f87f269d", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:12:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "201406717ce8bbeac920da1859f030d836065cd6642ce1a483bc38b6fc2c91d91c255c403bb638ae883a77a9a6220e1f01d9e9983c713edb4db5462c38838c60aa" - }, - { - "block_id": "000f424832d74914f983f4f0bc531bb5961978a3", - "extensions": [], - "previous": "000f42479d7b7b7fc334681456d0f234fa05167c", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:12:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "200ac079090967977d529f02eb8484327b740a2388f030b13ee1ede62f3fc1d0493b696e6fe7d2340459d9619b7d008a8b2ec90d8eee46235b12b2774c08c762d9" - }, - { - "block_id": "000f424917e434cb5dc0ecb1179742513e539a8d", - "extensions": [], - "previous": "000f424832d74914f983f4f0bc531bb5961978a3", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:12:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f1f40bedf9f222a01a940d87af35123142ef204a5dd5a10044e937e93ad6a599627cef7b9f26de3f84f37c840d69fbb9ff16433490fb354eccb24d6e9de4ed31d" - }, - { - "block_id": "000f424a1e41bda5d1918c5d6d57cbef06693534", - "extensions": [], - "previous": "000f424917e434cb5dc0ecb1179742513e539a8d", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:12:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f709ec577951a8b75e15fc39eca5c649df7ceb8a4e19b2d29bc61d93676ac7d70563f16e17ad67e6db1b2b61ee65b351a994523b088691f175a3ac03c093afb70" - }, - { - "block_id": "000f424b4e3f3a6069ef7ff86e39ca43628208ad", - "extensions": [], - "previous": "000f424a1e41bda5d1918c5d6d57cbef06693534", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:12:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f7d9d25fef08750dbede3badd39ba39413dbefbbadeff8c10725d1110d0dc65dd2ed39b49664dd95b24d2ba62b2455bc2774d109117ace70c442132f9a3903095" - }, - { - "block_id": "000f424ceba45f761f7af77e3769f29309064606", - "extensions": [], - "previous": "000f424b4e3f3a6069ef7ff86e39ca43628208ad", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:12:36", - "transaction_ids": [ - "5ab7fb8138ef8f701661d68f7d654df5f8e2fcfc" - ], - "transaction_merkle_root": "b373963ebfd9e8016fb1bd40278e0f6b3db9d1dd", - "transactions": [ - { - "expiration": "2016-04-29T05:12:33", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f424b4e3f3a6069ef7ff86e39ca43628208ad", - "nonce": "7402656884829255743", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "62a5ccd974a8f3fb08c75aa8969a9fe518a3b25dbd1c45ffe519dd1b13da1564", - "signature": "2017904d537351ea950ec133f1b2c06c114d5250f798ab977e8e6357d1b759c8865db3827a0bf9dc5b05f108745575b28431f9ec97d4b69172cc1d21a886e35bd7", - "work": "00000009b8dae9e6e043afd09c3d7fade06e7a93ea059d3cc42aa651a336ee31", - "worker": "STM7D7oC3o7YYLoVVd47qXr3tMaABtGGsvWiraQQt2jtnX8gZAjdB" - }, - "worker_account": "hr803" - } - } - ], - "ref_block_num": 16971, - "ref_block_prefix": 1614430030, - "signatures": [] - } - ], - "witness": "bhuz", - "witness_signature": "2032c88716d797ec86bd110d310bb4788665883f3b06dc47ac5eb56b8a0e7eb7b51bf00db0bd2a810d49fae23191bf3afff79a46784143e39d4d45028e58d16f98" - }, - { - "block_id": "000f424dd6f43ea8bbc8c4618fc1945ee18907f2", - "extensions": [], - "previous": "000f424ceba45f761f7af77e3769f29309064606", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:12:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "203cd17ce91bf1f562786fb02c60e5e5959022880e6d7a7c26a612db323da1513138993dc8e29547824486c305cd716893ad190a7a162afc909cf4df0ffeb5057d" - }, - { - "block_id": "000f424e989bc81c6f265b16c7a865675b31cd89", - "extensions": [], - "previous": "000f424dd6f43ea8bbc8c4618fc1945ee18907f2", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:12:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f223aeeb3243a2fa80b97d53e04ff4d3f46b4fa0a5258517581406ff467a7aea24c5d2fc4d6ebd83eea289b0e777b9ae99db0cf151d581b155bd33634dcfdd205" - }, - { - "block_id": "000f424f7a8c0841fcbb30a54bb33ed9807071fe", - "extensions": [], - "previous": "000f424e989bc81c6f265b16c7a865675b31cd89", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:12:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "202654c83026849fa04499502abbc918519fba24c4a9ec9c7543bbff1fafaada43165b6b186a7acb7f2f528ddc9ef90a9107d45381cf5ffb0f5ed7ea0fd523a7b5" - }, - { - "block_id": "000f4250546777fd91682b4ecc899d9c95851520", - "extensions": [], - "previous": "000f424f7a8c0841fcbb30a54bb33ed9807071fe", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:12:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f41d18a22019a0fb41993d6687af27dfae42d23858e894ef9126317a3fca8cf7f64fd2623050fa9325cb73400a6e66488b5c17c6ce7a97ae049f9962cfce1d906" - }, - { - "block_id": "000f4251a4d137ee4b95ce307bcd8bef9c6a4a57", - "extensions": [], - "previous": "000f4250546777fd91682b4ecc899d9c95851520", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:12:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f71130d96260d08cd27e50b32e79c8dc981d63da64fa84b6917b996281d624d3d207127b864d439e57528dac5489889e595443abf6040de7b4da4c0bf72bfa645" - }, - { - "block_id": "000f4252e4e5df00f505810556114ef096e4fe97", - "extensions": [], - "previous": "000f4251a4d137ee4b95ce307bcd8bef9c6a4a57", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:12:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f624b1747abf054725c267bb5facc4daa8b6d4795e9f771569d7e301110e5830634c74744134ff81618bd8a0ca36e94a68b94f9f3b6eb1f811c62108f00e557af" - }, - { - "block_id": "000f42538e1635a6e63872a3b03bfa51d86dcbbf", - "extensions": [], - "previous": "000f4252e4e5df00f505810556114ef096e4fe97", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:12:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "2006b56b80734973cecce398b6600c4c6a8a85a4156a2d43570065a00a1161e68e54c471230037986ac5dea0fec6d4616887899aea7bf693e68848793be77ebac3" - }, - { - "block_id": "000f42545910e5e0d38dc3e310d4ab8fdfca5e52", - "extensions": [], - "previous": "000f42538e1635a6e63872a3b03bfa51d86dcbbf", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:13:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f2dcd1543f33a31614cef7895dfcb86b8f6f7cf1aaf346047862b370d2614e42f2853b0ba2a68352683eeb9bbd01997286fc8ad657ae2eab91301d1876bb5f5a6" - }, - { - "block_id": "000f4255bf9b4b7494e694024f2836edd28e18c0", - "extensions": [], - "previous": "000f42545910e5e0d38dc3e310d4ab8fdfca5e52", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:13:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f0aac7a834010defce1fd843a2166039ef146c20b2a38b382b0a11a98c74269cf2153a52cddb01e3d9873d7ecbfb1895e2b0a2d5494c5ed9ae7b65bac69cb6d5a" - }, - { - "block_id": "000f4256501fa7379762456787ce4dedd7563e83", - "extensions": [], - "previous": "000f4255bf9b4b7494e694024f2836edd28e18c0", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:13:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "205d4a3fb10a1db600a44165b6e22c31443b63701f29d2b12cc2f2c179f37ce3aa192a8fc5e9bee008e9166690ee1d144de00a673bc3637a1341ad8eac6459bdfb" - }, - { - "block_id": "000f425784702437d0aa01d5ee5161ce19d4919f", - "extensions": [], - "previous": "000f4256501fa7379762456787ce4dedd7563e83", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:13:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "2057589170e2f30e8b6a6e9a23753744b3406a25b27544d135f4f4aa6f25002c7b244b5e15fa0b12c058ddd9456480478c0edd7b2c3faa253276b4ab1d954a8b72" - }, - { - "block_id": "000f42581fd705a502b8b949357fec037b6efdac", - "extensions": [], - "previous": "000f425784702437d0aa01d5ee5161ce19d4919f", - "signing_key": "STM8kQi4yD7Ho8gkwPruaGU4oKuu24Lv1qXxxVi51ByaNHnw8Eydy", - "timestamp": "2016-04-29T04:13:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pumpkin", - "witness_signature": "203e6db65300daaa9474cb0d95b8c5a8438962632dd8964468241b1076bd16022f66edde3cde3e709397e2cb9d1e752afacf5a0bb4911ab2f3631bf31fa4b77e80" - }, - { - "block_id": "000f425928a414c55d907e2313380aedcce8a1e0", - "extensions": [], - "previous": "000f42581fd705a502b8b949357fec037b6efdac", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:13:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f1c58d07bf38c871a95a1aaa21a98e63a9561eb2cdc234b5d583365daece6babf6ee672dd4bf933780791a3b3123535fce88e57e621f1848034b5904d097bab12" - }, - { - "block_id": "000f425aadfb169e212e33dcbe95f79ee41e7771", - "extensions": [], - "previous": "000f425928a414c55d907e2313380aedcce8a1e0", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:13:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "201d32722347ffc8593cd885641ed4f2021610bef78d5097c776444a007b16e4e039d5a4afdd2ba46989a46851564cf9dd76803c6ac52abee17439e88f40f8fb2c" - }, - { - "block_id": "000f425bba8a664401b19d29d5490ccc002016c3", - "extensions": [], - "previous": "000f425aadfb169e212e33dcbe95f79ee41e7771", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:13:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f68bbf3d4cfc92e8f7e045edd1ebc5cb97327431d7063dd7e2611e64c093fba593510df4fcab8c4a07666a519fbf882d2b3557056cc8afc90a22d4a9a3fb3acf0" - }, - { - "block_id": "000f425c47564ff6cbc4c9c6068316aad7230053", - "extensions": [], - "previous": "000f425bba8a664401b19d29d5490ccc002016c3", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:13:24", - "transaction_ids": [ - "9c94a31d1a5f60081a9889673eb675e3b4e3684e" - ], - "transaction_merkle_root": "d2bcd03546c99d130988e6f82decc001e570fae4", - "transactions": [ - { - "expiration": "2016-04-29T05:13:21", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f425bba8a664401b19d29d5490ccc002016c3", - "nonce": "911740918991125639", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "3f51a8b8b4136563c5f6a8dbb2da5de3102d8cc64b4ffbd560471b41a65f6de1", - "signature": "1f9971a5ac2fb8946ba4bc4da7040d209e71eded3c2a13dae01e334d4a2f8a1587323102677c91582fe11ff84a6f2a12dbe6b7404e5359c5e15adae4cecbc3714a", - "work": "0000000899a6bc00da904f46d281b924448a1515a9f88b20b1d25bf8ce1442aa", - "worker": "STM79MBtZRHzawavWSBSVYNRAy7WkwM2PMPDEnoWH547WLzXP5CkP" - }, - "worker_account": "hiram" - } - } - ], - "ref_block_num": 16987, - "ref_block_prefix": 1147570874, - "signatures": [] - } - ], - "witness": "nextgencrypto", - "witness_signature": "1f6dd4755a6779b252357cf8aac2ddff0b24af3d2da6a26114e020dcecf08fc71b2b1792f7f7e3d6991a64f3fce0b0bf06ddb8490ee326d480deb447d8ac0f5aeb" - }, - { - "block_id": "000f425d44dd8e266dad8fc6e206d6385cc99782", - "extensions": [], - "previous": "000f425c47564ff6cbc4c9c6068316aad7230053", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:13:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f447266e1a3e778bc671a75652828805c174d34916bae4d4187fee789fa28a57465eb9949d444eb56513bf2f3fcecdc5b930401bf0cdd178dbc1d7e356ecc73b7" - }, - { - "block_id": "000f425eec26d0ce48410f7368fd96ab0a6af52a", - "extensions": [], - "previous": "000f425d44dd8e266dad8fc6e206d6385cc99782", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:13:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f360c08a523428882373e1f88f24783a7968957435a932b772cefe289d856cae9228a3e199bbb2126250f708b4fa587e2b2f32f4cbeb5d2bc0b8b7e8523c715d6" - }, - { - "block_id": "000f425f2dc9c2bb7f0cc85b02ffbb4f549fa872", - "extensions": [], - "previous": "000f425eec26d0ce48410f7368fd96ab0a6af52a", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:13:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f743a52e90002d0597699d9e038aab6a531efd09f5dc1359b2e84e2cb990d24c75a4bde596761aa569d7ed1bf1302019f0e19a39995eccb4383ffa96aeb95e075" - }, - { - "block_id": "000f42602b67174a1ef90740913856ede93d9039", - "extensions": [], - "previous": "000f425f2dc9c2bb7f0cc85b02ffbb4f549fa872", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:13:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "201a0da81ccd8f4657764486d052d386d90e51254310679555c329a8233282cf980dbe6c4373461f14b594cce310edc6c1365c831eb4f19df7465373223e4766e0" - }, - { - "block_id": "000f4261636a480897104d68bbddfadc4fb730e8", - "extensions": [], - "previous": "000f42602b67174a1ef90740913856ede93d9039", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:13:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f184e1d1c704ec9b6d17ba6c609ba002f84830f7da201426999cbdc89a3c0dc137d5c9ce7baa4ce35084f81d957be76865d77b554f1a20a548141052813e5c321" - }, - { - "block_id": "000f42623fb2b79d805753fd5c9ed8d1bfffcfee", - "extensions": [], - "previous": "000f4261636a480897104d68bbddfadc4fb730e8", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:13:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "20659e5b1073236c60d5b1897dd6c6a751514dc0c09c2527bf6ccc13abfcf6912d2d3171aae862628628a09f9b5fea77656271315c5290769c6da71e568787c066" - }, - { - "block_id": "000f4263788e1bf1e32d4a33bc365fedcbb1915d", - "extensions": [], - "previous": "000f42623fb2b79d805753fd5c9ed8d1bfffcfee", - "signing_key": "STM7dLqQkmKLYWNo6TmG8yP47ErGxP4WxSmhRojrUFK3gBRXGDsNS", - "timestamp": "2016-04-29T04:13:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silkroad", - "witness_signature": "205617a7173d9f0ecac5e5b5d02595a7946122c34141884970ffd931f37c66bdd31030451a139204b92db216d8510427256b4e10be05d7ed85d58146ce396150a9" - }, - { - "block_id": "000f4264bdcb60f36d91adc47240206c28f2d6d9", - "extensions": [], - "previous": "000f4263788e1bf1e32d4a33bc365fedcbb1915d", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:13:48", - "transaction_ids": [ - "c5df3e4785fc5d7b200249725e5f70d6b435fffa" - ], - "transaction_merkle_root": "634d05c734afa88fbc7c687f12501fb687468e4b", - "transactions": [ - { - "expiration": "2016-04-29T05:13:45", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f4263788e1bf1e32d4a33bc365fedcbb1915d", - "nonce": "6506893831524263801", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "49c4c3476aec91ddc9b87befd080fdfd8da0c4c7097b044642463f5fc6fe26cd", - "signature": "206185a349bef2bf63e49439c42b799ae9ad5628c60ad8461ae4760c0d5e39f9d4671f3d51e224be0e18ee2151aec67e695902bc3b018c8df6a06d180d428a7674", - "work": "0000000179123ea04d3f0b47e26f5b2c69952add6c50d662997a5fd4c5d5e9da", - "worker": "STM5nJA2HwMMygDH433SH9vVrZUrw5sAEArj7mXLbTavWtsud2MYG" - }, - "worker_account": "turbobit4" - } - } - ], - "ref_block_num": 16995, - "ref_block_prefix": 4045115000, - "signatures": [] - } - ], - "witness": "abit", - "witness_signature": "1f483bf36e7a4144654d4187f0b3bcc6ccfab35d20f178b3c1056cda1c06d87bc7629a0f3f0c1e6c6ea951d577fa34671ce308146f882d979b6528036082b75730" - }, - { - "block_id": "000f4265a8431c4ebd2caf59a0a8201f6f9d5e7d", - "extensions": [], - "previous": "000f4264bdcb60f36d91adc47240206c28f2d6d9", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:13:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "204db610c0425ac5d8b24a596ef728e7cd26d8837ab83f0a3b479797d1488222bb3652d230a7ad278287e13042a252dbee23c317da3e4dbd9c432684c649410d98" - }, - { - "block_id": "000f426653ce59d6f0018a695aae6e6c6f95f0b1", - "extensions": [], - "previous": "000f4265a8431c4ebd2caf59a0a8201f6f9d5e7d", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:13:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f0f86ff14a953b14a0b0b84dc8af7efa6d40dca9b72a23348d2fc0ea0afbc7e553f6e74988322130ab840afeee61ae69e8b3c567d912d89f89ac09aabe00f342a" - }, - { - "block_id": "000f42670b621e67f924002f9bcc3c738347bf7d", - "extensions": [], - "previous": "000f426653ce59d6f0018a695aae6e6c6f95f0b1", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:13:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "201d443161c2dc7db1e04fbb8a974096c73c3567982418902b284a9d3c7fa9fce1415267b0318630d6e6cac2022df978085fef4857ea101c0cbcc25ab2563f80bd" - }, - { - "block_id": "000f42687258b774b3196b8bc1d072d912003bb2", - "extensions": [], - "previous": "000f42670b621e67f924002f9bcc3c738347bf7d", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:14:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f50661d6e53bd7d736d33ed05e81a14f598fec0b50ff18ac48963845e9f404e7f36d82519963b2720201e393c343e81bc0cd59bee7132b5f477ca59313198db30" - }, - { - "block_id": "000f4269efd2830266f005461d6d252fd03aaa25", - "extensions": [], - "previous": "000f42687258b774b3196b8bc1d072d912003bb2", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:14:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f3bc1152e0f88052f52243f95e786190b6226677cbb57f21e664b05aa5d57d3282035574696730987194fad1978ead2a3f35f0a7c3ac165e5c319d9cd4aff4ee0" - }, - { - "block_id": "000f426ad061c3654c3f8187386205fc16b3e27d", - "extensions": [], - "previous": "000f4269efd2830266f005461d6d252fd03aaa25", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:14:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f582bed108b1cadcf9594375a1c72186fc61f4fa0367ee33a980f8c11e4f2449e6a3b15bf21059412abbeb52229928068969a25b06cd6457ad82fa0a30f0b0b45" - }, - { - "block_id": "000f426b4e51990ce9062c327a3d6c68d7ec625e", - "extensions": [], - "previous": "000f426ad061c3654c3f8187386205fc16b3e27d", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:14:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "202c262b8de3bd70972b2504eecb722608b47857b0cff08bc46aebb92eed3ef104408eb97eba076a7201127e0868ccda24bc71d16346aef9759747af42145f8353" - }, - { - "block_id": "000f426cb3875e00dcc170df8db388b2b179557d", - "extensions": [], - "previous": "000f426b4e51990ce9062c327a3d6c68d7ec625e", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:14:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "206a72a631a85c0595b318ff2fdf045ef3661399dcfdbb8260c4162c42ea9eee597a9056da51a8590c4092245046bbc6f58f0eee43f4c1490eaf3944ac942bb0da" - }, - { - "block_id": "000f426d4dca949202bf3e922e3a016dfdf4e8af", - "extensions": [], - "previous": "000f426cb3875e00dcc170df8db388b2b179557d", - "signing_key": "STM4vhtognZwHz1sNX2efiySFap35k5M3g186nyjBAnbwPkTHCBT8", - "timestamp": "2016-04-29T04:14:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "joseph", - "witness_signature": "206c8932f8ef5ad4cccba10dc2478faca2466cf3fc1b7a2b6f802c731e78b4e6860da367d7d4a19cedc82e834f601729dfb920b2b83c3b8614ac74fb9cbdb85a10" - }, - { - "block_id": "000f426ee9850fc6939554fce205a5926b2a0315", - "extensions": [], - "previous": "000f426d4dca949202bf3e922e3a016dfdf4e8af", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:14:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f323af4dbb09cabb4bf37ada947980bebe1877817cef924a9e81b3d2f5c3ae3cb4b91591c93e4dfe974910edd7c7f4f012db7409e5b8c5d3cd6ba502f59667805" - }, - { - "block_id": "000f426fd116b5fcdef927a8bbfdd48504923e38", - "extensions": [], - "previous": "000f426ee9850fc6939554fce205a5926b2a0315", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:14:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "2026fea1f686b09959de5b40eb134a703ceecdc86c8d9888df8dc2a9cae14b5f5e583c4d8218dbc2df3975b60662d01c85a86bf48870285ef4d2f144a46fca4308" - }, - { - "block_id": "000f4270c157ccd0653718086d2ac136ff5bfcd7", - "extensions": [], - "previous": "000f426fd116b5fcdef927a8bbfdd48504923e38", - "signing_key": "STM8KTqm5g3i7wRTpwPsDdxUTNBa7CTJUtjuX7pAWUELBZjBQfwK2", - "timestamp": "2016-04-29T04:14:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "darin", - "witness_signature": "204837d10d33754c4f3afa929940bde9b81c989c34ea8b5b58dea4c01fdd87da6a071d366249c2a718f08297c02e85961b906a88a37811a20f0dc1be05ccd461eb" - }, - { - "block_id": "000f42718fa4267c04a1eb5bb533de7dc0be6b65", - "extensions": [], - "previous": "000f4270c157ccd0653718086d2ac136ff5bfcd7", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:14:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f5fe48662bbbb7787292b0e809b29b806066165d7078cf20c84a0f27975cca43a5acff821743f712d5613ed72ea7a700c1099b2e4bb6b07022ad127cb260c094d" - }, - { - "block_id": "000f42723e7757c41834f8c4a270fae08a55f8f7", - "extensions": [], - "previous": "000f42718fa4267c04a1eb5bb533de7dc0be6b65", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:14:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "204f0ad0d4cad46ece28d473aa5315c753d13749bb5b9340c76615026aaf1fa4bc0459d215940c8e137d3d37b914e27383432e87a8769ba2756f87906af1a847ae" - }, - { - "block_id": "000f42738a8a87b68154d0c2398c0b586ec6dd81", - "extensions": [], - "previous": "000f42723e7757c41834f8c4a270fae08a55f8f7", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:14:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "2049a4e08825b3afc09373186d9ef5904a272628761e02f45f19ea13f9bdc3ade11b8d8888c28b1de8dabfad7e05bb54fec007d4e2e204b21afa19f5815708f46a" - }, - { - "block_id": "000f4274285cea8d132129c82e166e76f79c04f4", - "extensions": [], - "previous": "000f42738a8a87b68154d0c2398c0b586ec6dd81", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:14:36", - "transaction_ids": [ - "f74119c8d8336de55df5f7ac9f326d60a9a432ac" - ], - "transaction_merkle_root": "1a457969a0191d1372d35bd7413bfe27fe8fcbd9", - "transactions": [ - { - "expiration": "2016-04-29T05:14:33", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f42738a8a87b68154d0c2398c0b586ec6dd81", - "nonce": "10291951073203782372", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "1ca63089e3a46a43579442594e380ab054f0def5ae322c36cf379c8609ea9f82", - "signature": "1fbc4f1a5ac428d24bbd5e66f819041e6ff355c8ed5b1394203972df2478ad7720609f9d225895a73351809abe2dee0289e3107aceb8b2289754b46a076c58ccbc", - "work": "0000001fb420bd088d0faf1f4cd768b27c24bc31cf3570c3ec9abbcb5ce60061", - "worker": "STM73wNsVLLhSiyMYPCpXn5arAXQ7Q7mNadH1SgxxgmDMRZasbZ5J" - }, - "worker_account": "turbobit5" - } - } - ], - "ref_block_num": 17011, - "ref_block_prefix": 3062336138, - "signatures": [] - } - ], - "witness": "nextgencrypto", - "witness_signature": "1f45aac723e33f094f70c837bd65e0b698eab0979fac66cbe21fa90ef9c44457bb2189629e76866c9583c0b0794acdaac4f8387793690591656ee8c5657dbb297e" - }, - { - "block_id": "000f42752a12254dcec25e26b6787acd1105e8a7", - "extensions": [], - "previous": "000f4274285cea8d132129c82e166e76f79c04f4", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:14:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "200a0db6128f5a267a4910d25e68c49e3283e1d1bcb65f6146d7cf552c5c7259dd487ea675a17c0d7c57a6f5a959915f58150d4d30b6044acc9935721f0d3ec64e" - }, - { - "block_id": "000f42766052512245e8ad68d9e6ed900bb24bdc", - "extensions": [], - "previous": "000f42752a12254dcec25e26b6787acd1105e8a7", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:14:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f7c969c633f27efad9b435bec5e9cda7015440c6a65f1e1ef0a820a6384898ed878832e9e968ab213437840fecc11a98c8fe3dfa59c6c6eb861d85f39c8eb79c1" - }, - { - "block_id": "000f4277b6e7bf850e394c7a79f6e67bb0d928aa", - "extensions": [], - "previous": "000f42766052512245e8ad68d9e6ed900bb24bdc", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:14:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f4728a4992f0dee166875e345507a7d0af088f70b56b60c57992c7225435012b3133dbb5288d695ceb9a41dd20508ea052f70fae5253fec2f69ce1167c5f2bb3e" - }, - { - "block_id": "000f42789ddd4e3d88a1977b81de6f2ec07016d9", - "extensions": [], - "previous": "000f4277b6e7bf850e394c7a79f6e67bb0d928aa", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:14:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f33baa8487515ab778f07af5cd7f8c0449ba2f698900ac2938467e05ee4b910634a7042bf3bdc6efb9a10aa687db84b7c88fb59065574f00f0d6ea31a90c34324" - }, - { - "block_id": "000f4279cfd8c6487c6e0d875e97a737a50d3a9b", - "extensions": [], - "previous": "000f42789ddd4e3d88a1977b81de6f2ec07016d9", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:14:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f56049bfc08dc57fe07f5b5bb8e4dc76beb50cbbb0bb3f8295b19dc4df78f93a056b5b5aff558ee820b937023a5aeb30e97dd7e0ae3efd9440ddac0f8da29c945" - }, - { - "block_id": "000f427a94aa1d6cd6043e53d869f77b6c0a1507", - "extensions": [], - "previous": "000f4279cfd8c6487c6e0d875e97a737a50d3a9b", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:14:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f1a7e0733a875dafed066d0d7e15a483c46bbf8fccab1a4b6c2108799f678671f7695a2a5d5a833374df65e17d76dfa8b7d9fbc5aaae1721fe2af4848454b97e2" - }, - { - "block_id": "000f427bd23423dec62a93d84f2fccb4d15df237", - "extensions": [], - "previous": "000f427a94aa1d6cd6043e53d869f77b6c0a1507", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:14:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "201dded3bc00aae23ce80e5f2f0bad18d3a8bd63d84b6d4827388292f563db44b92fca0624fb859738b7923db30ee9b09a720a17cdccaa1783f3744d35e9007ded" - }, - { - "block_id": "000f427c6b714f10ede7972efd0fd42debbb848c", - "extensions": [], - "previous": "000f427bd23423dec62a93d84f2fccb4d15df237", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:15:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "204431e331d348c768e509998774e1d976dd64a930a5abdb00a6a6d2647f41fffa741edd06eb8521bce93f47d2dae5ff4a592d9faa3d7b2331e34c24457694189e" - }, - { - "block_id": "000f427d97d2b90bc5250b0a6105cf15bc58803d", - "extensions": [], - "previous": "000f427c6b714f10ede7972efd0fd42debbb848c", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:15:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f0337cd396949cccb67109b2d749fc098b07b3eacc87c765e014f5d4aa7643d945190c78d3c393ee8055136c86d7a99583ca8a19c7420fef0c965e1df2a5d4893" - }, - { - "block_id": "000f427ec36de5d948caab974a8272890262b411", - "extensions": [], - "previous": "000f427d97d2b90bc5250b0a6105cf15bc58803d", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:15:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f17b675e3d93b997401c33ba2e9a2bdcabfa8d989ed31e641659d9a892ba7f4e832a782e0c2c4199711353e12cf3add6ae57af9377702646cdb1c192a6b47e03d" - }, - { - "block_id": "000f427fe639131992b2f0d4a3397b849635af41", - "extensions": [], - "previous": "000f427ec36de5d948caab974a8272890262b411", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:15:09", - "transaction_ids": [ - "017aceb58763182dd19837f307c1602ae1d1ea2c" - ], - "transaction_merkle_root": "5e0f2d34c99bfbc0c12d048aa54e6aca909bc5d1", - "transactions": [ - { - "expiration": "2016-04-29T04:15:36", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "440", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "silversteem" - } - } - ], - "ref_block_num": 17022, - "ref_block_prefix": 3655691715, - "signatures": [ - "1f3cd92d0b6b37e2b1941dbfa73f4bff1f6369dac9ddb980b2adb43314dfc3ccaf50d1bb171bb71b999deaaf8212e1ef1caae6d09c7a81b0666448b542788ddaeb" - ] - } - ], - "witness": "au1nethyb1", - "witness_signature": "1f13259c1b889509ce551b3bdb7378df7e0c17a9049154031f6d6f55262947cd46064cefacfba6893807a3a3a82a6b020556dd5a2d18d50a2470dabd71c07ac827" - }, - { - "block_id": "000f42809e2d4f43c58092c3cd546df6feed6128", - "extensions": [], - "previous": "000f427fe639131992b2f0d4a3397b849635af41", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:15:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "20213a7c1fcb5d6c952669d5f2d497fda3713b332f9fe043adb4f875906ce300966c7376d98ea51ed572b2ce12eba71f8be4dacc47a9e0679c3406434276879df1" - }, - { - "block_id": "000f42818b102324c9c5d647e5f3bb768ade925e", - "extensions": [], - "previous": "000f42809e2d4f43c58092c3cd546df6feed6128", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:15:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f0a9c9a29b7d252507822ed2412c34e0c5aaf227767b53dc0c3c8c3478a8b8c2d4ddc985f8045037566398ba8e32c29f575a01095c6224c4d3c8915e62c883e47" - }, - { - "block_id": "000f4282271278d2722cc7f2bbdc89a585f5ba44", - "extensions": [], - "previous": "000f42818b102324c9c5d647e5f3bb768ade925e", - "signing_key": "STM4y2uqBfLmtcDcJXuCdKwXPyytim1kiC4kTt6pjpuBmQmqPuLzH", - "timestamp": "2016-04-29T04:15:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "velia", - "witness_signature": "1f765f2b987ec1ad45d50c29569268d4cc0d4b861e158b36f53916497be392b9546eea090487569c6ff206b224753afd0ce36fa35ef88c9ecad40c873731a669f4" - }, - { - "block_id": "000f428365b3efa28f1f91e916444192c04e4ff3", - "extensions": [], - "previous": "000f4282271278d2722cc7f2bbdc89a585f5ba44", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:15:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "201d385a057b971d5be567bd43f18e206962113e0bd7495f8abbcab727a262069a5c6a7b132acc6486b0238710f48627496d7de22152f67c6e660fbec332f14079" - }, - { - "block_id": "000f4284d89e01a461b432b129280f1fdca85276", - "extensions": [], - "previous": "000f428365b3efa28f1f91e916444192c04e4ff3", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:15:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f3c648ae003d95c264d7228df2ddb369ddb0c44dbbbe42320308341c23745c68f2bf795314074b93648c5049317569c2b1600ec56936ab14237e62213ab319ba6" - }, - { - "block_id": "000f4285d8c146d514208884aec3901eb471e1e0", - "extensions": [], - "previous": "000f4284d89e01a461b432b129280f1fdca85276", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:15:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f34b80e581c5d05592c1decc9e7816827ef7c1d81584408f5351af0f7997542cf49cc2ae843e3418c77047a619a2e19d31761dc07d16a257ed339301c85182459" - }, - { - "block_id": "000f4286996dc4bb46bee4acfcdf9f84a63f1869", - "extensions": [], - "previous": "000f4285d8c146d514208884aec3901eb471e1e0", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:15:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "205df16785570443a1b42600f6c916ff55cbebcda78238071030c9ea4c49489b57309374b066bd6173e087bec7c4b58122a4c9fd5c9516f3e3c4cc4bd345dee106" - }, - { - "block_id": "000f4287dd25ea02e819f4ea89d9bf0fc054965a", - "extensions": [], - "previous": "000f4286996dc4bb46bee4acfcdf9f84a63f1869", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:15:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "202f925451604469ab15c7051a8d4121589845fe48242b6adf8791e482b211295f214a6acb2fb27e82c53ff3f0579cdd98b859a7316d3d11649e1d2e6ccec6ae9a" - }, - { - "block_id": "000f4288e7880c4c8779e82a3f4c860f1495c86e", - "extensions": [], - "previous": "000f4287dd25ea02e819f4ea89d9bf0fc054965a", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:15:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "2043b2e40e3001a923254342161da1345f754bb909965840b2f2baee7f18c09a7e281ee1cbd28ce43f7947b531345b45d1ce912abef14fa5aa12cc13ef28bbaa68" - }, - { - "block_id": "000f4289be722f4cc6d08d7db693e15edfc27ea3", - "extensions": [], - "previous": "000f4288e7880c4c8779e82a3f4c860f1495c86e", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:15:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "2014a1e703db2765bade88dfebf13e88b2f316dedd31a816cdc20aec1066657b62585ba594035031421f632593e59cd09422fbd7d58664304e2fe170ade311c19f" - }, - { - "block_id": "000f428aa91267eed0d6ed08699c743a54ce632a", - "extensions": [], - "previous": "000f4289be722f4cc6d08d7db693e15edfc27ea3", - "signing_key": "STM7zCGUYKYNsJEDnrwCEU2BwPdNQmFj8NQ1TEhPdVSUgneNMRUEU", - "timestamp": "2016-04-29T04:15:42", - "transaction_ids": [ - "5a23851c0872bec4c32e619674a631e125c7f257" - ], - "transaction_merkle_root": "9e3cddf6b3daf919f08b9693000ab31b39eb4cfb", - "transactions": [ - { - "expiration": "2016-04-29T04:16:09", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "437", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "steemychicken1" - } - } - ], - "ref_block_num": 17033, - "ref_block_prefix": 1278177982, - "signatures": [ - "1f5f372b83d7e8b2d022cc58b4082efe3c500d0cc43ff4a152b7d739fa00ba47116d009e3a437b744cb398e135eb397d6b8c8ba60d28ad4db721b04ef6bff004cf" - ] - } - ], - "witness": "bue", - "witness_signature": "200f765657fc0daffb5e9fda2de2029dcd5e4726c6e1fa31a86cdddcd224a786713d51d668d54c5545f7e57eab35af62cafedf553a9e5894a765d1cbddd3438643" - }, - { - "block_id": "000f428bd8510c0aa0e5cf2a5fcede4f4f83dda2", - "extensions": [], - "previous": "000f428aa91267eed0d6ed08699c743a54ce632a", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:15:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "203eef684778b9cbf341c3d958188ac91e1eaa10fec971471bd3f7015035f43c463346cc5fdb3e235ef28f94762670305d2dd8e39979ecc1af76e92cb463d40784" - }, - { - "block_id": "000f428ccc8e91e15172e5d7585f89878d42fc17", - "extensions": [], - "previous": "000f428bd8510c0aa0e5cf2a5fcede4f4f83dda2", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:15:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f44e958c1c8a5c99f2f0b346e68981590f83627645e75056898ed67b4f0ecfe316908843b3aee362baab5c0abf3060bcf4659c09bc95b6573319e900f97388661" - }, - { - "block_id": "000f428d11e5c2020b2b6cc478261f6195e37f0a", - "extensions": [], - "previous": "000f428ccc8e91e15172e5d7585f89878d42fc17", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:15:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f3a0d9732fc62f65220429ef7c5dc3046d1c8a6fca894a2dd73c6986513903e760d8392e3ebc338114eb6aba7dca385a0b2db86218cac6d49965c0ecdffd9453f" - }, - { - "block_id": "000f428eb6fcd0fca77a2b908f86d64f6e5531ae", - "extensions": [], - "previous": "000f428d11e5c2020b2b6cc478261f6195e37f0a", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:15:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "200563e3bb40e48724499fe9961df2fa125843646a274fb23806056617328f8ee741f8f3891c6fa80e3b63097a166d0fed5ee0a0ab64b204ac75edb79a75af0424" - }, - { - "block_id": "000f428fda271c3ff26b10ad1283060c3e37063a", - "extensions": [], - "previous": "000f428eb6fcd0fca77a2b908f86d64f6e5531ae", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:15:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f7d1e516df55abd89d34e66d1720f1dd929a41d6a5a81552e42517ee9ef2fb65e7b9b51b739de498b2bdefc9d1cc92d680345db578fea0db140deafb4e181a549" - }, - { - "block_id": "000f4290b64f1cd702985e078f36ab627804a3eb", - "extensions": [], - "previous": "000f428fda271c3ff26b10ad1283060c3e37063a", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:16:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f03bfaee7522390566fe6cdbd943a4d79cc6278a1f37b9674077f424e79e413065de71250fc9c8219efb87953422115472ee2fccc7ed7b63d70954312c5df5490" - }, - { - "block_id": "000f4291bf0973dfabb7777d7149c53cab194f6c", - "extensions": [], - "previous": "000f4290b64f1cd702985e078f36ab627804a3eb", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:16:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f5aa938fde145a354341e49c106a371ea3aa4201aff510ce63af746985d4040e749bbee833408bf5623cfc349a6853e12e35515cbddaea8dd4b13dfac74a6ba67" - }, - { - "block_id": "000f4292cb823bc4cc1b975fc22220cac6f0dc37", - "extensions": [], - "previous": "000f4291bf0973dfabb7777d7149c53cab194f6c", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:16:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "203e6513c7c6c04a5fbc7d88dd25bb65ee0c186f9a87c50084af5d9a5b546c5aa23adee421c31b38343e2a3aa8da3a890a530d5ce67c768853de8d0f33656b93f6" - }, - { - "block_id": "000f4293bc551faac5322b327d9900185d38bef0", - "extensions": [], - "previous": "000f4292cb823bc4cc1b975fc22220cac6f0dc37", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:16:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f5242c6c41ba73a67b4bc1d0d08bf9d4cf1b214001d56fe171af3ccc9d49e38036e54e827f48a9a91829eb1a550bba2ff2fc9e09c7d13889a15296dac145a035d" - }, - { - "block_id": "000f42949067ccccf867ed49606bf79ec7d3952a", - "extensions": [], - "previous": "000f4293bc551faac5322b327d9900185d38bef0", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:16:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "2031dc2ebf89938bdde8ef6b02e0c7ac569a3472c786ce4eb115463bc14800147e097f9ee95122127fa06b0effc8234c4da7a7f22edc445c40cf283ee88b0dec7d" - }, - { - "block_id": "000f4295b018157b0acce8e70befe7bf86f814db", - "extensions": [], - "previous": "000f42949067ccccf867ed49606bf79ec7d3952a", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:16:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "200b95e3f4fa697e478bdadacc4ed6eb166b4e24c7b491b543c361fed641fba47e2e8196fb70cb92f331bd826f9ea3bc7e8164e8a81d7b6a1410040821c5fa1a51" - }, - { - "block_id": "000f42966062085760c27938c5f443de1d4e1bfb", - "extensions": [], - "previous": "000f4295b018157b0acce8e70befe7bf86f814db", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:16:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "2044bb598200eaeeddcb1d859bcef19fa2c30837d2c6f38bc9c4dbcf21c7bd7446031bd7fc2860c45c799a18245e7e0c9bf812f0f76b807435a175fafc3e35caa9" - }, - { - "block_id": "000f4297933eab45b74e8392b65041238e7ca29a", - "extensions": [], - "previous": "000f42966062085760c27938c5f443de1d4e1bfb", - "signing_key": "STM4y2uqBfLmtcDcJXuCdKwXPyytim1kiC4kTt6pjpuBmQmqPuLzH", - "timestamp": "2016-04-29T04:16:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "vella", - "witness_signature": "201798c2bb1aef336847d8b9f0839faf0861fe4aaaed1b1c669430aea3409269504d781d2d37613ff67f09f93d314a70d133f810efa1e2b615768f9da0444cfbfb" - }, - { - "block_id": "000f429820f8adcc28fa718ef6999813ae73d02e", - "extensions": [], - "previous": "000f4297933eab45b74e8392b65041238e7ca29a", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:16:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f2e90346e7c973d0314f825cc1d5cc3dffb8b0cbfc2ca793919a0ec4cf7cd746b28870a6753ec2b6a50d647eb665db40fd159a3db594f26214e7542ebec8be72f" - }, - { - "block_id": "000f42998b3a7cacfd86f4e73201ea613b640e22", - "extensions": [], - "previous": "000f429820f8adcc28fa718ef6999813ae73d02e", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:16:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "20553e7a9c7e29ee171670d1e9ff862d53bb7b8a02034aaa325e5ec548050e21b50b7c1c4f4057289cd7acf42495797ddce26bae629caad30217cfbb76a7d390d3" - }, - { - "block_id": "000f429adae777797c1fbc0367f681ae8606807d", - "extensions": [], - "previous": "000f42998b3a7cacfd86f4e73201ea613b640e22", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:16:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f644d35f0fb77f3cd19597080ff6ab2085855c9e5f8e7201f47bed3ed454b4884692f6130d74021f3a59fa176d41dd5b1aee3f014ee29d9cdbac8ee75a808905c" - }, - { - "block_id": "000f429b674dd0b983ad160c4718c755a14be84c", - "extensions": [], - "previous": "000f429adae777797c1fbc0367f681ae8606807d", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:16:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "20644666f1198b69573e89d343ad04044c9becd2a6e8a024f780ec12ef2911f9a55a58030101c708283196ead7cdb2f458bc930e2b4157bd6f0c7a903d36beeeb0" - }, - { - "block_id": "000f429cf7db6032459e7d6d06ff9d97277ec7e1", - "extensions": [], - "previous": "000f429b674dd0b983ad160c4718c755a14be84c", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:16:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f5a6663b2b807f679e0bc36091b448d45a5fccd7408d5ac62d870428788f4291968ec0ca922a961496431824602aac1c882d939af5b6bc7793bfc5fea97b9cca9" - }, - { - "block_id": "000f429d231c344817ff7d910f6e458c304625dc", - "extensions": [], - "previous": "000f429cf7db6032459e7d6d06ff9d97277ec7e1", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:16:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f144ff5da357c860186907b65a36673f80426a1f751727708ff25432c2be9624d72ebc8598e6556e95e8bb6ac907cd5a93392160f3fa950040ec4149df84e7d36" - }, - { - "block_id": "000f429ed26fdd27e69542ca4485e06445b3fae4", - "extensions": [], - "previous": "000f429d231c344817ff7d910f6e458c304625dc", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:16:42", - "transaction_ids": [ - "bbc566d661674310d5780820a533f7b0014c8a95" - ], - "transaction_merkle_root": "d926fe9aa0987dfdec6a95822a9fee6ccf65f2d2", - "transactions": [ - { - "expiration": "2016-04-29T04:17:09", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "441", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "cyrano.witness" - } - } - ], - "ref_block_num": 17053, - "ref_block_prefix": 1211374627, - "signatures": [ - "1f19d9ba590ac7a82cdfeb36667ffe88265c41a73ba0de66e7ea5771ba9fa70a52762c435e1b0fc21eb621e86170545a76c6631a92d9fd282e5a4cf5f574891410" - ] - } - ], - "witness": "abit", - "witness_signature": "206c458eb65594e2d5168a2866e6784a83a58f6fad708f73b441694f7ea00f5ff670053b62f206b9efed6894bb49c186ee4703fae9564101809a5847009ad19f99" - }, - { - "block_id": "000f429f21ea3aded0eedaaf1816eb476b97960f", - "extensions": [], - "previous": "000f429ed26fdd27e69542ca4485e06445b3fae4", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:16:45", - "transaction_ids": [ - "d99a05b39cafa7d53c321b1717bdc83fb77a9986" - ], - "transaction_merkle_root": "27f5689e10fc157111d56ff40fc4e78b47b09a43", - "transactions": [ - { - "expiration": "2016-04-29T05:16:42", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f429ed26fdd27e69542ca4485e06445b3fae4", - "nonce": "11247879270196572684", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "a3c346b5f007321f55cbf7c987956fe74012ec15a44fb68125d969ea83c14e42", - "signature": "20edf3708067601f7c72cc04e4365abbc4c913e828810e83ff9fd837602d07723902a3e109e73678786f5d9d883c92b98c5924f79941dfa34de0f6edbf2525a11a", - "work": "00000016adece34a2c363b57e7afd3698c8c399074e961108097e295b1408028", - "worker": "STM6UDQwi95eq6NEmQXoDafPqcS1NzgajL1CDPQ3ZbdChgkarfsfA" - }, - "worker_account": "fontas" - } - } - ], - "ref_block_num": 17054, - "ref_block_prefix": 668823506, - "signatures": [] - } - ], - "witness": "datasecuritynode", - "witness_signature": "2039fe13b6050d6e2e03e8e368029d1eac012364fbaa863926c5fbcddddba50cb93176de93f41bef47e51d8258389b21f6476ec7d32b7914bd8b1d5239b33ee7b5" - }, - { - "block_id": "000f42a09af4ddc16eae8319c66dcb0ff846b099", - "extensions": [], - "previous": "000f429f21ea3aded0eedaaf1816eb476b97960f", - "signing_key": "STM6kzHDuHQYGKGUUoPdhmPcHgFFjpq4uycnLK5mUBPPqqn6qpiaN", - "timestamp": "2016-04-29T04:16:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "boatymcboatface", - "witness_signature": "1f3ff272a93a230df4ac8befd58f648ab0a9e1a2d6eb6c7180566dbe07eaf35e5e2dec3cd78743b5d0035b52c8b73204ce2c173bfc1ec559ff52e72eb91f6df1f5" - }, - { - "block_id": "000f42a1b288339a758f609baef473e38f74b99a", - "extensions": [], - "previous": "000f42a09af4ddc16eae8319c66dcb0ff846b099", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:16:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f14d0650f5405b5f1af24ab052e0bc123a95535ef5d3317520c693c5edf35d2c93d9551fa5961bffc02f7b40d08e90b5c86c8dc1625e2f0bc847e8d39b9150c1b" - }, - { - "block_id": "000f42a22d9dc7612497da58034b80c8d3346011", - "extensions": [], - "previous": "000f42a1b288339a758f609baef473e38f74b99a", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:16:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "201c9098950aa77df21abd108250b47623c4756fc947c2a134d0ffa3ef53eb35ce1027868b729c1bb1dba1a0f1afd8a39c53a353646bbd7d76891fede8898a51bc" - }, - { - "block_id": "000f42a34bac8532ae612ebe74b7361d274304d1", - "extensions": [], - "previous": "000f42a22d9dc7612497da58034b80c8d3346011", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:16:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "203021fb0e1b7921a317170f5de336877b5cf30727af37d5fce8685f3d6fb472f860b2f696b9e36b4eeb156fe8f2de7effc8ba1a6e9605a388358ed5ed549a7744" - }, - { - "block_id": "000f42a461e316728de737c48f9084d2d2debdcb", - "extensions": [], - "previous": "000f42a34bac8532ae612ebe74b7361d274304d1", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:17:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2004676027d4e1ed75a206b404c1ddd40803715dc356b86c3dcabec8b1da1b8b0c149502d25a398b916c5b9bb0f6a44f9624f1c85bf0fa4e29698afa571ad277e6" - }, - { - "block_id": "000f42a5341546d901216807c6f8fe017f5e80c9", - "extensions": [], - "previous": "000f42a461e316728de737c48f9084d2d2debdcb", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:17:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "204711070e55dacfacbfe6577121be0a2650ad98c0a083cdcea676071fa625578f285e8817dee16b693dc5dde7ddb7edf1777cb0e4d7d7cf22d0ed86165192cd09" - }, - { - "block_id": "000f42a6c058390e67d278cd9ccc7bfc3d96f16b", - "extensions": [], - "previous": "000f42a5341546d901216807c6f8fe017f5e80c9", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:17:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "205fe5973ee957b14a66c9088f930204eab70470ff1955dcd0679dce27897f72012a9d3a2198a521bc0f189d8ca7e6d94d15b1a4f55ee26355f98b93f34d232564" - }, - { - "block_id": "000f42a75f117bf993883458a9a7a760c5eb9492", - "extensions": [], - "previous": "000f42a6c058390e67d278cd9ccc7bfc3d96f16b", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:17:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f1efb1084e465e2b84770bfdfb473b1f52879eebf708345102932ab94aa4466957983f023aa5cf3c034a2186e68df1d6e55a13daef4542926ba143763182fb202" - }, - { - "block_id": "000f42a85faa40bce8dc17454ad834c74e01c03f", - "extensions": [], - "previous": "000f42a75f117bf993883458a9a7a760c5eb9492", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:17:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "20039876f5f7e0212267982253d1f153afb75c5d7f5fef7ac858ae0da6b43424e06dbbebabaada7d12af1326ad8dbc27251c3b612e68181e30100e903ae82e48cb" - }, - { - "block_id": "000f42a9e91d0319ed117332975ad505f74c3ac1", - "extensions": [], - "previous": "000f42a85faa40bce8dc17454ad834c74e01c03f", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:17:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f6af517e8984b04b05660b9257b30f31ea08072ae7807ad3ca40cd538db62956321a1dde9daf07cf5dd7dee34a057eec7f82e67158d7cc90c79fc471df34520d8" - }, - { - "block_id": "000f42aa78283c4a37f8c08f4199ae5b6a0a6489", - "extensions": [], - "previous": "000f42a9e91d0319ed117332975ad505f74c3ac1", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:17:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f7dd4480881af1e04d37972ff2a085fa7e1a622c9e7f4f165a41f38558a40f967588c4d9cbdcb847a8d7c3478a1b47690de7e6c856cdf5cd60df069aca68c55a8" - }, - { - "block_id": "000f42abfdfca6c29f7c6d9399c11f474a31db5d", - "extensions": [], - "previous": "000f42aa78283c4a37f8c08f4199ae5b6a0a6489", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:17:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f3fb6f86dd97b58ef9c9b2fe5f861c2928d9725c12bd7f2e4b35a82310ac16f7015f19620d19b00b7213df5b3679be51a253f135a3f62abf9c4d3606b8cf55866" - }, - { - "block_id": "000f42ac562fe20db46533fdde21fca2f4b88aca", - "extensions": [], - "previous": "000f42abfdfca6c29f7c6d9399c11f474a31db5d", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:17:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f729acaa18f46e134d58c745f131b6429b8288e474606ae3691da6eb96e7d4caf6161da0dac8828b73234cf051eb07fe5997eb33654426c032d88e96851a9f04b" - }, - { - "block_id": "000f42ad2dae880cdf2f58f267d4564558e03c1b", - "extensions": [], - "previous": "000f42ac562fe20db46533fdde21fca2f4b88aca", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:17:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f5f6e2384116c2c8c528071b52538079344a871c4bfcbc6caaf50473bc60b940d119d1ed9758dc216a0d2c262e6cc1d506855c0711e9d3f241277e2c8a330e4d7" - }, - { - "block_id": "000f42ae7bfd731d4aec8bd98778ee50fd3c44ba", - "extensions": [], - "previous": "000f42ad2dae880cdf2f58f267d4564558e03c1b", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:17:30", - "transaction_ids": [ - "7fa3b5797381a5d037dc339c2d847f3e30b75ccc" - ], - "transaction_merkle_root": "4eb7fdbd805b6d85ad1a1a5bfe79a9f2389a8a07", - "transactions": [ - { - "expiration": "2016-04-29T05:17:27", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f42ad2dae880cdf2f58f267d4564558e03c1b", - "nonce": "12021481535921371793", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "b7c37940c87bc909463f274952fa768587e87c475f6db0fbe87a45d23d86f0c2", - "signature": "20899f668daef369483de2b7cce5898f829276322f52eb3c041c55488d69d2f0f86aa098ba59bfedeec00004d806a58fbd65b2dd9cafdb4706c4bdfe3bd9b64f18", - "work": "0000000ed065fcab8a2703c4cc7a972aede58091a0fe889a9420d429114363f7", - "worker": "STM7qCXPrH3nrCTmeSdVWoQwH8q5HfS7yQYacrZLosfZUewMnKayA" - }, - "worker_account": "hr203" - } - } - ], - "ref_block_num": 17069, - "ref_block_prefix": 210284077, - "signatures": [] - } - ], - "witness": "xeldal", - "witness_signature": "20624ddd8fbe1f28cc0c518c41ae1307abccbc46a46433a595009580b519e81848274e586b2fdb920fed9282bb81168ee9afffe8c3d9e57bfe74c36a3380518f59" - }, - { - "block_id": "000f42af56c7f866bf9c4b2aedb5fe13a934127c", - "extensions": [], - "previous": "000f42ae7bfd731d4aec8bd98778ee50fd3c44ba", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:17:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "2076cbf016faba7a1c0c6bbde574343842bead31d54db17be7c9237ec7038824df212f92a9d58894b780dbb1ee2b45769de4bfbf1f87b3dd58e250ba42c23de360" - }, - { - "block_id": "000f42b09e9dde1f296012c01e187bc30ece70fa", - "extensions": [], - "previous": "000f42af56c7f866bf9c4b2aedb5fe13a934127c", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:17:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f6d5897a1620c073eb00bfc57ac27bb11a353fa0eb4666777a0c9121ecd661b2130394b947f2e054585f2803131f232f7f46c6ab746adf17c5a366463b8cc45ab" - }, - { - "block_id": "000f42b12a42260f0c3664198f686abc3f41c7ce", - "extensions": [], - "previous": "000f42b09e9dde1f296012c01e187bc30ece70fa", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:17:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f76fba5bd1b5034525403c43d10a32c82fc7baa7b90741f0c5256ae3a6783e84e53ce5c8aaa2014686bc8db662a7c192596f9283cb7b160dbccf50f948ce83ade" - }, - { - "block_id": "000f42b2cb65eb92977274dcee9ecc9c5792f160", - "extensions": [], - "previous": "000f42b12a42260f0c3664198f686abc3f41c7ce", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:17:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f1c236a26ef291a4068bc3918497abe5b5bfbaea706c367b5add0fd8c0634008a510703558ce501db74512f7c5295ee7dea48e807a21a05742676e36a606aba15" - }, - { - "block_id": "000f42b3513673fbbe2d14c7cffffd96ee2b7c63", - "extensions": [], - "previous": "000f42b2cb65eb92977274dcee9ecc9c5792f160", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:17:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f700ce3a96bbbdb26b34cc33cf0e0cf3312d3697b1d5d2b2ad94c4413f68445690ba5a0a6069d649a5669e93339c03db8790957d4305d14944ea0f3f1f574f2fd" - }, - { - "block_id": "000f42b49310cf5cdcea7da21e27f46d05b13d5a", - "extensions": [], - "previous": "000f42b3513673fbbe2d14c7cffffd96ee2b7c63", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:17:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "20427fed326c2576b80c1b9c9d57ce5e2fdcb0a196adbe3c0832221c26e7c46f1b0cf7037f69c7c09572dccfe7455eae15a369f83f27b3bc6d8f938fa459dce2a0" - }, - { - "block_id": "000f42b52ba19b3e64de99ae4cb804fc5c906cf0", - "extensions": [], - "previous": "000f42b49310cf5cdcea7da21e27f46d05b13d5a", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:17:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "207d4bdcfa0ab05a7f572ccd65a23206a511a0a1b9527ee1543d6a1319083b007b578341ef0b27e40c67bac96003fa83f1ec773140c59067c4519e090c4b610bf4" - }, - { - "block_id": "000f42b6b0cfb43dff0a0405c8ff5579be5ccb2d", - "extensions": [], - "previous": "000f42b52ba19b3e64de99ae4cb804fc5c906cf0", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:17:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "207ed9e3560cf030e5750e831c004e1d8c879c99f4345861a62f9e091d993f09067c736ed436c7e5fb49fbd5a596680fc054f98e633ff29b2e369bee25a0a50792" - }, - { - "block_id": "000f42b730f4a02d7f705a4274d93bbf334709e7", - "extensions": [], - "previous": "000f42b6b0cfb43dff0a0405c8ff5579be5ccb2d", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:18:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "2032746a91d27c1ea69d10a94cf7359efad2e7229a43f644c04a2d29ed7e39d0e00ed5e1e5f3fe36074ec9254a3ad82eb0679b4dc2d535d750be8d606857c36b41" - }, - { - "block_id": "000f42b843fd2d4757c3bc972281bf4479d2222c", - "extensions": [], - "previous": "000f42b730f4a02d7f705a4274d93bbf334709e7", - "signing_key": "STM4yQG8BiozEtiBErWJG9MhGipmkAT6Bp7cWDnwSZkyUnMciVUyt", - "timestamp": "2016-04-29T04:18:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr103", - "witness_signature": "201b10d7e35da0c91a451dd1f6f7f53609c9362dbdde32b4d547ffcf301da473f1013c60f05f3443a20fbca5cacbc3159c591f94fb9538a941afba3614e6c9eb1c" - }, - { - "block_id": "000f42b95d58998217b43d27664ce410f819bce5", - "extensions": [], - "previous": "000f42b843fd2d4757c3bc972281bf4479d2222c", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:18:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "2076bcb84ef7086514a34e9147023e44e53f4585c9c01d351bb8c8993335d352ba6fe0487faa85d49a877fd7923f525b9456c121ab59575478bd836de5db0ddbf5" - }, - { - "block_id": "000f42ba6576bf693bde372b9d13e1a5194ef54a", - "extensions": [], - "previous": "000f42b95d58998217b43d27664ce410f819bce5", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:18:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "2046aa502c20d83cb28f6476788e8d865a236a5c8d3c2eb7574eb8c308892e766308734b63ad943520b492380e813441ea1ae39ec9c407a18842b2a9be727c53bd" - }, - { - "block_id": "000f42bb582bdcff0e2b625f8895bb824e120e42", - "extensions": [], - "previous": "000f42ba6576bf693bde372b9d13e1a5194ef54a", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:18:12", - "transaction_ids": [ - "9c8238e421775c1d606488c7c7873e3b92c66ada" - ], - "transaction_merkle_root": "79cbcc98ee210c85585da637e3655adbe0077634", - "transactions": [ - { - "expiration": "2016-04-29T05:18:09", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f42ba6576bf693bde372b9d13e1a5194ef54a", - "nonce": "18170171114775118478", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "a993f964b2c0edfc3ad7c2b210fec1e4b919691892f8d04ce26ef6b2bca4bceb", - "signature": "2012deef3931b14fed70946a3f42a66bbf811eabdc1e4200dacd836376113b06a47a05b88bc9d2dd7843ba74395d5d1739f58de94081cb31a9fd191c5e3d750a2d", - "work": "0000000c11955d07eedd15ba443b1e308a1c0b9e26415d8a01c5e4542b9efdf1", - "worker": "STM6ChCh8ryiuwfbzFfViZvh6t6gAZzSg8BubWQbqUBviHCSefFkd" - }, - "worker_account": "hr704" - } - } - ], - "ref_block_num": 17082, - "ref_block_prefix": 1774155365, - "signatures": [] - } - ], - "witness": "bhuz", - "witness_signature": "1f352f275fbc1637d832f6c50b21f2d5e4d1b0f3b1d6965d5f62f1cf9e665c57cb71a4c32201c667a1bba14603a67198a59843c603176e0bf72fba09a588fd5a5c" - }, - { - "block_id": "000f42bc7ee54f0aacd6c18edce39370837c04ca", - "extensions": [], - "previous": "000f42bb582bdcff0e2b625f8895bb824e120e42", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:18:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f1f5c1be6c6ca78a9da20ae14370ad26910c2766e749a8bae369503384f3a0ea24f8e7039bd78e0b63a2114f851137475028de318699c231d3559a7634b8a837f" - }, - { - "block_id": "000f42bd5af18782322210cc28397081493dba88", - "extensions": [], - "previous": "000f42bc7ee54f0aacd6c18edce39370837c04ca", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:18:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f6c8e6198f4fc2464e61ebee50e3758ab412e9f1ef81676193fac8c6e5487524c15d86514898aad781d61313c9762f524d5b8648648035e6bc05a39893168b6c1" - }, - { - "block_id": "000f42be279d4f30b5f9b15f72d252a79527d37b", - "extensions": [], - "previous": "000f42bd5af18782322210cc28397081493dba88", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:18:21", - "transaction_ids": [ - "c80a4e33cc9c40258d530d7e7cc1960c921ee491" - ], - "transaction_merkle_root": "9f750269624ecf8b51c0a5b207e384282cc4a530", - "transactions": [ - { - "expiration": "2016-04-29T05:18:18", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f42bd5af18782322210cc28397081493dba88", - "nonce": "867701826036134498", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "35a0428f752aca4e1c74b4319f5faabaceff4afde853225a6217f8c64c5aef87", - "signature": "1f2e3652e067b45e5e5db8f725ad6e493ef63053a6ccf7b76b12cc04aea4a2726719a6ba54bcb6a114d3ad9d7e1648c16e227b2b634545c53c17c7e7370735809e", - "work": "0000000134e1df8b3d93aad2aea144a71a27791b7e6e114ccf85824647e32ae5", - "worker": "STM7nhQdwXXRQnzwqURG4NkwSmb89g4HuLbistFdCam36YHZZpS55" - }, - "worker_account": "kolby" - } - } - ], - "ref_block_num": 17085, - "ref_block_prefix": 2189947226, - "signatures": [] - } - ], - "witness": "steempty", - "witness_signature": "203f96453ba74bd436cfc02ffa9183ee1313c42982ab13d7fcfbd3b1f52b4b186b4a832f357f3a6c08c8c8a856e149dfee66dd8852e7464c7193868bac8d795b75" - }, - { - "block_id": "000f42bf2fa83ae6eba2a8a0265552e1656c8fc0", - "extensions": [], - "previous": "000f42be279d4f30b5f9b15f72d252a79527d37b", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:18:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "2052738925fb44f77950b37e0b6cd27c6a7a71a7b7f0c8f9009e4fbcdb722add5c03b7bc4d381ab9aef90acafd2a0c2b7719d18c9b4937bd9bc08a7792d345533b" - }, - { - "block_id": "000f42c0c769e50c878d8d7c9e5b4a1ae427882a", - "extensions": [], - "previous": "000f42bf2fa83ae6eba2a8a0265552e1656c8fc0", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:18:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f07ec392f0202e731e7082717011aa5a1e4a5a70706ba49fd8ce374b7c2473e2c06d0e3cfa94f7ac941d1a08c58d30185c53f4dbc0e39b96d6cdedf8962a70a14" - }, - { - "block_id": "000f42c11a5724e5ce5cf22139cac7db42cf2dcb", - "extensions": [], - "previous": "000f42c0c769e50c878d8d7c9e5b4a1ae427882a", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:18:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "20381116fccb30e26d86129b27c932201c020d29ace016b69692a89390bed3996e78f1ddf9820cdfd6d057c8caaea6c48773b22245c851a3e5f08de1a03a66ea61" - }, - { - "block_id": "000f42c239c12da0189c60f4bec67c92b889c4a8", - "extensions": [], - "previous": "000f42c11a5724e5ce5cf22139cac7db42cf2dcb", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:18:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f0bafc384b54328d6e17d9cb6022b3312521e6b563cf1781b336615a6e6243bbd2863cdba23e7f551790de1a71077c3db10baf72f318fa10ac97e602f8129e62d" - }, - { - "block_id": "000f42c3d394b18aff8fabe86f6026658221e3cd", - "extensions": [], - "previous": "000f42c239c12da0189c60f4bec67c92b889c4a8", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:18:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f33348a4648e56cd861549d170322d31647c0db4e6a9179db1dd5d37abb8468604bdb228a48fe4833381b856f473f9997b54147332c740b48c5dba18b17a47699" - }, - { - "block_id": "000f42c41719df2d52f14012aef8468aaae80c7d", - "extensions": [], - "previous": "000f42c3d394b18aff8fabe86f6026658221e3cd", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:18:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f326517af80b5a7b5a0fe8a09e0aa636a3b48e87c4c717b78c650c797f624fd486d1ad91c9437113a9f906fdc941806efdcc0db4bb5e5dff6b39aaf222568dd09" - }, - { - "block_id": "000f42c56a16ef8ff2328c9c641b6b84b203c2e3", - "extensions": [], - "previous": "000f42c41719df2d52f14012aef8468aaae80c7d", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:18:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f16cc3d5d0ea104d2060e5b96aa5792fbab4aca6d36d7f2bb393e82e785a7e07160b36faf0d39dc1d0ec2cfbe330f7f6ab412542033e2e28a2a6c3081db1e5fa5" - }, - { - "block_id": "000f42c6cb8fe3c350fad3226e6f76347ae5d617", - "extensions": [], - "previous": "000f42c56a16ef8ff2328c9c641b6b84b203c2e3", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:18:45", - "transaction_ids": [ - "3dbd659af7e97b357eca1afd63b9ec857b350e64" - ], - "transaction_merkle_root": "7c24eb6927501307ec1f9ba7f827e6da3d95ea45", - "transactions": [ - { - "expiration": "2016-04-29T05:18:42", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f42c56a16ef8ff2328c9c641b6b84b203c2e3", - "nonce": "7686201120965679886", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "33ce5b68f37696a8703f6b601035adbcc7d801eb1d1b56ce22e3ce8f58b253d3", - "signature": "1fa80765323e17f626f1753b2c17db862e498e2347a0c16c54eb814bcd4fdf478c14eb35b02793a5725ec4075db6f2483c5ab520a334eba4193d2bb8cd510e9cd6", - "work": "00000004c44bbee05d2c11727184631864718eb58481fb3e2ce54f4106f476e0", - "worker": "STM7ecWzLZc9S3Ema2LVzQzBPyEYGaSAswqG8NrLJTjynEMFqZE4p" - }, - "worker_account": "thorium2" - } - } - ], - "ref_block_num": 17093, - "ref_block_prefix": 2414810730, - "signatures": [] - } - ], - "witness": "dele-puppy", - "witness_signature": "1f71b427c8ac9a2cd7af347e782731dc353f49783dd09dee6c661cfe3b849853193f81823173c8fba8fecf54073b9da4ff7d317b720d36ebed217adcb4320ce095" - }, - { - "block_id": "000f42c7a963a78de5c2d3ed6722a8e9a6ab1994", - "extensions": [], - "previous": "000f42c6cb8fe3c350fad3226e6f76347ae5d617", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:18:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "2041dcef40d0ed1356973f095278c424411b227f56030e66c2e0f7109da11e4dde423c69195513c3055e6f0040ab4b6a1560ee7d8400bbed445698a337875f2ec5" - }, - { - "block_id": "000f42c83bc91e85c807d58bed20a496c8e16153", - "extensions": [], - "previous": "000f42c7a963a78de5c2d3ed6722a8e9a6ab1994", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:18:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f232837c16569d262c7c686ca777a4e64e9a1dee54026613e94683a708db521b6226ac0993b158899223593c9d8eb6f437bd10c55e711dd56d2acfa93b153dc1d" - }, - { - "block_id": "000f42c987af60b38e07686bbfd30780769f36d1", - "extensions": [], - "previous": "000f42c83bc91e85c807d58bed20a496c8e16153", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:18:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "203fa0a46546aae4c4f6a56c731e061da7be240051334442473d4a4e6a996f53330b428904cd39005cafec4d4340a012f1dd88a534aa3b3f9c1cca05a3b1487db3" - }, - { - "block_id": "000f42ca4eb200743d5f90f5d4c7ef0c1b9ab39f", - "extensions": [], - "previous": "000f42c987af60b38e07686bbfd30780769f36d1", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:18:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "20531b5cb24b75f0f55fc268ea8b44ed50eb70ba701648699ce9cdab844f01f6744f53e48652797aa9b4ebd847bb67587039e1cc2559e4598f2d7747ef405d6c2b" - }, - { - "block_id": "000f42cb426731055fc57f9ec27d8880474f968d", - "extensions": [], - "previous": "000f42ca4eb200743d5f90f5d4c7ef0c1b9ab39f", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:19:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f14c7a85e20e4bb1b5f592ca19eb4499fdd2c9b1967b40eee60aa84903d2dede03a75adb2b57a293051ed2d6b8e2b701a4796b130d836464e63f40ca6d8ef612c" - }, - { - "block_id": "000f42cc773344c508c6256980bd0b28f72afffb", - "extensions": [], - "previous": "000f42cb426731055fc57f9ec27d8880474f968d", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:19:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f5a462571db1c2ecdeac89f34d039493804c2c2b8936dd66c41c4c86d1cc0497e651e17150a40b96162246fe91f8cb731e64b654208f2b9967bc8dbf77c85485a" - }, - { - "block_id": "000f42cdef3726fdccd3ec896381b0e4199a43e4", - "extensions": [], - "previous": "000f42cc773344c508c6256980bd0b28f72afffb", - "signing_key": "STM5Vr2N9owagzJ1KYDDuAdzp78K7C8qE2MDAriFaWiGqn1R3ex1P", - "timestamp": "2016-04-29T04:19:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "virtual", - "witness_signature": "1f289e51f60d5c95e8e1a25b6a938d4e33e1211d1a9f79a8e75dc4b9b2313139ec246f219835242ba6ba8c3946312fe9d608618fd60f389de714f86a97c13c91ff" - }, - { - "block_id": "000f42cefa0de6e3f69b71b6c103699947e5089e", - "extensions": [], - "previous": "000f42cdef3726fdccd3ec896381b0e4199a43e4", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:19:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "204dda924601b4667f3dd576fc880b8f982a28e8a76535061217674581cc29bd516ff5a2374b88abfa6c9e0246c15d7fa79b11b84d02c78d7c94fc8738744fea69" - }, - { - "block_id": "000f42cf476474c99fe62e1bd208c8e87048882f", - "extensions": [], - "previous": "000f42cefa0de6e3f69b71b6c103699947e5089e", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:19:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f24dab18030e86b7ae542e4707e09f8dc62a8ae1d2f7d0fb8fe7dfdfb14f1ecc5789271bdabc3007b7ab0421e7936084df0b9b6c5ddf21ef2ee8ddf6ce44e8221" - }, - { - "block_id": "000f42d0c6a2efaf61804c2efa7aee437367fa2e", - "extensions": [], - "previous": "000f42cf476474c99fe62e1bd208c8e87048882f", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:19:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2006a7602cfab079ed9b8a235dfd1ff6522e4167331d59883f27f396cd98db3eb33656c283f27d17784bba6c0f2be7eb069ced3940eb0d29d2ecdf547fe3ab07cc" - }, - { - "block_id": "000f42d14d3fc9574f18858c021a64e11491846a", - "extensions": [], - "previous": "000f42d0c6a2efaf61804c2efa7aee437367fa2e", - "signing_key": "STM5742TmF2wyuStKtgETi1KDycZ56NNvgJhAt6xWA3PA1Rx61FvU", - "timestamp": "2016-04-29T04:19:18", - "transaction_ids": [ - "ec6cb7706190bcfcc70c30bba965fa08d8fcbcd1" - ], - "transaction_merkle_root": "d387dfe44cdad36f9efe8bd0afff2f14cab86923", - "transactions": [ - { - "expiration": "2016-04-29T05:19:15", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f42d0c6a2efaf61804c2efa7aee437367fa2e", - "nonce": "5296779896958626247", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "39f8cb1fdbc24a499e279a2e22371939648d2c7ab1f0444e8166fd6f6ce39471", - "signature": "208bee2cdb351e866eab4bbdbe314a37f3f7c147b9317d00b12a9b3835495bfe72134976b7911e2169f84a7383280e0d0bab27d4654ff3de198f9d7c96880b528b", - "work": "00000005d7d86033767e6f7178336354b2b3678e01cf3be76e2a26723f6c4d62", - "worker": "STM7W72xmZTzVd3duKHNhK1c7ch1GZUX5MErcWrV1sBqyBbRn3KJg" - }, - "worker_account": "lee3" - } - } - ], - "ref_block_num": 17104, - "ref_block_prefix": 2951717574, - "signatures": [] - } - ], - "witness": "cyrano.witness", - "witness_signature": "207ac0032a90a6fd99d07ae7a944c1d854ed39a45c9a144ec7edaae1e1a581f039435239e02290e04f9ed77a6e07db858713878591076060ab16464957900489ca" - }, - { - "block_id": "000f42d267e6c866a21537cd9dbad31591ba790f", - "extensions": [], - "previous": "000f42d14d3fc9574f18858c021a64e11491846a", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:19:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f63c06c510dd115dabfb573ddadb847db359fb7ed09edb8db63fd9fcfc11d6962045877a0cebd40bea176b753431f66a354415951c1b462ceb2c31bfd00ec630d" - }, - { - "block_id": "000f42d3127ba09aa8aa1521bec95cea483042d2", - "extensions": [], - "previous": "000f42d267e6c866a21537cd9dbad31591ba790f", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:19:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "20160b6050726e50687b30d70a066164c671b76dcb92b8e7d64cebb2cbd83fbc823f5a4135b08ab533c0130cea957afc6169ad801186abfbed668df0d4da4f6e6f" - }, - { - "block_id": "000f42d40ebac69730548ac5e374ae4b52e0b461", - "extensions": [], - "previous": "000f42d3127ba09aa8aa1521bec95cea483042d2", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:19:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f16c80b0466e1073fbdf2f33a6529aa5c2ded7578501105d31857647e588e05c174161309a9c0253ede4fc7eb252f7ba9e83a31d0b0139a58428fab8fe52ae69b" - }, - { - "block_id": "000f42d5a5a63e0c8d467800d5faa5eef5335c0e", - "extensions": [], - "previous": "000f42d40ebac69730548ac5e374ae4b52e0b461", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:19:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "20569477a216fe747e6b07ef891adf6c6aea801a35d4ebbe148d35610e948492346813d5a27668a116b3f44385f6d4feb717f50784523e9475f1e8fbf8b5929c15" - }, - { - "block_id": "000f42d62b80585b3b4e273ab4c31452716540d6", - "extensions": [], - "previous": "000f42d5a5a63e0c8d467800d5faa5eef5335c0e", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:19:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f4f2af8b1fb9c6d910c057f2225700a5e5703c06ff29cf632052770d0be417e310fdb18f00f8025f60fc03c1912f968cb40865e3f2b7e6e5ac3fd748b45fe0224" - }, - { - "block_id": "000f42d7a6e7af4e50a8267797888914a6eda5e0", - "extensions": [], - "previous": "000f42d62b80585b3b4e273ab4c31452716540d6", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:19:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "2025563e9fc4c12550acb98a19335730ddfb7ba002badc64d6ad1eb4c6190ba3601d47ec344d7a271422d184b72ca36c8c2f6c1593305184d9b3d63c51e38af17a" - }, - { - "block_id": "000f42d8cafe138d632abad2d8f8a02bd5123705", - "extensions": [], - "previous": "000f42d7a6e7af4e50a8267797888914a6eda5e0", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:19:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f1431c237ae05f6ee43b08a76dac9af6ff5b4921d1009fb306eb33076e8674a5f16a4347545d4fb8788734ea7d3cb0d3a4cb3e183addfbfebc335148784a1abb9" - }, - { - "block_id": "000f42d9bdab4e66eb5346c1309a3997627f099e", - "extensions": [], - "previous": "000f42d8cafe138d632abad2d8f8a02bd5123705", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:19:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f24d2ea54a4fa8389fdab29e8cd5edd3dbbaf84b1faf7ab9a5972a0610bf621b35d17154ac11128a853a476345e1c7c9a2c1df2f91ee4a30d1867f636b31b76ed" - }, - { - "block_id": "000f42da64c0a379b731b3ed8a2c5c54f2cd542a", - "extensions": [], - "previous": "000f42d9bdab4e66eb5346c1309a3997627f099e", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:19:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f4436eafd650c399e72f68089fecacb1043c18e8b424e4290465fc59e690e72db4cc72c2f7a2d8ddba73aa2cdf187175a4f030337aeea57fef18def785f345ff0" - }, - { - "block_id": "000f42dbc2d31cf479abb580930945a23134dc79", - "extensions": [], - "previous": "000f42da64c0a379b731b3ed8a2c5c54f2cd542a", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:19:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f18204c6effe453cd224f3a8c0ff5b8aee4ecc9af912f7ab79d44751d47e69afb6d4b682488ae6438dcecbd4bdeec26e2976d30d25f3506b7adbe5f425a4ea74d" - }, - { - "block_id": "000f42dcefb546943d02ef7c930d6248003070c8", - "extensions": [], - "previous": "000f42dbc2d31cf479abb580930945a23134dc79", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:19:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f030ad2dd12da640e3e4a93afd77d706743a5b23887425a6fe5992bd53668c09c52f4f1c5e4162a489518470b3cd34e0b87f5b55661ba4814a90bec59e4f26b38" - }, - { - "block_id": "000f42dda095d5c9acfc8b159fd6317cc116fafa", - "extensions": [], - "previous": "000f42dcefb546943d02ef7c930d6248003070c8", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:19:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f12dedf7944f3c3e1db98f6c3bdbaf88b20cf69b2dfb28261e7015279c5bf9253669558f9659796d2db4eb7dcadc7882d8bcee2de7b3a461c6d30835f5b0377c1" - }, - { - "block_id": "000f42ded9fb9072cb181a393bf78be558a4128d", - "extensions": [], - "previous": "000f42dda095d5c9acfc8b159fd6317cc116fafa", - "signing_key": "STM7D7oC3o7YYLoVVd47qXr3tMaABtGGsvWiraQQt2jtnX8gZAjdB", - "timestamp": "2016-04-29T04:19:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr801", - "witness_signature": "20701934c8ec755a8d067ee887f8a28cc9944c7b7979c3d24b908093c7e5f3bdae1d833e5de7ec78152ec362771c4a39ec80b9833677c1591a522a99a603b30d91" - }, - { - "block_id": "000f42df476196dab04cc69653b9d8c67ff33096", - "extensions": [], - "previous": "000f42ded9fb9072cb181a393bf78be558a4128d", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:20:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f1d8e52f44c73986a37d100a4f047690f95f968a273cb33eef7305835adb473a33dda4c822ff2cb17bb862cd5765c5b088f19dcb474c7f499c92f94da609a02e2" - }, - { - "block_id": "000f42e078d4addd3d7ada1ea9136cfb01166e0a", - "extensions": [], - "previous": "000f42df476196dab04cc69653b9d8c67ff33096", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:20:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f73ba36bb9bbb2e4de2197e9664db75b8c411aaad4d2b5cde12bcfa00bc888e33249a6d369079a1acc7fde135ce63ed01d6e4860cfca6ad3b52b9ff06e329e8b7" - }, - { - "block_id": "000f42e1e1573e4b0d15898d77e7840745901e63", - "extensions": [], - "previous": "000f42e078d4addd3d7ada1ea9136cfb01166e0a", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:20:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f5c89104e3ca8458c60225088095f3411165e7b8c25f692b597efde05e23908ce36bc23c62c154c158f98728562faacd957fd7cd61c0f96e54dd3698a42015079" - }, - { - "block_id": "000f42e23770678a1a1d10d96db5a0b72e803a96", - "extensions": [], - "previous": "000f42e1e1573e4b0d15898d77e7840745901e63", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:20:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "2032a6c40bae64f05372b7c11eecc587290b3f2fc69eb1a51cf116d649020caeef2d44a6b197ce9cb76a18df597cb4266694b3f6a0ede80541f956e2e16bde7d9c" - }, - { - "block_id": "000f42e39746f2637bfbd8d6a53f1a886ce85cf5", - "extensions": [], - "previous": "000f42e23770678a1a1d10d96db5a0b72e803a96", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:20:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "203a55a20bc84be8337947ead9cfe8e90f2e906c5aca1c5bb2c6ccdea410a7d4506868a25b6fb2da79af0a27cd7b26af01f1555979a06aef3aa28ce0f11b1ea21a" - }, - { - "block_id": "000f42e49a80fd0419b6f33bd6b06c1de4c36191", - "extensions": [], - "previous": "000f42e39746f2637bfbd8d6a53f1a886ce85cf5", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:20:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "206e869a46d118e3cecf455363b5b05048731557996d904ae656c1b5602df5f1ef7dbf2db3c4fc27796e3765273a75989e86b54a6073a8985c9d000f004ee790f6" - }, - { - "block_id": "000f42e512ec35aad29234e6e8d9ca13eea42a7e", - "extensions": [], - "previous": "000f42e49a80fd0419b6f33bd6b06c1de4c36191", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:20:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f43dc0144ce2e63ed87f2ae030dca88345cecd02ec539fb80e41417fbbceef979477326b97fb7655b5c1a2b17611e05f2aaa9c3867be270a6fbad3168b5b13f77" - }, - { - "block_id": "000f42e655501498ffb96c4c6b6d0502b6aa8ac1", - "extensions": [], - "previous": "000f42e512ec35aad29234e6e8d9ca13eea42a7e", - "signing_key": "STM6Kq8bD5PKn53MYQkJo35BagfjvfV2yY13j9WUTsNRAsAU8TegZ", - "timestamp": "2016-04-29T04:20:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "wackou", - "witness_signature": "1f2f60f8a218dbaacdb98c62b62c689defb5e908a31cf3ccb9e1727fa5e314763955b0ce4633aa59c92feb67f5024ea7d08128d7e16323756c466d9a807ff8dc40" - }, - { - "block_id": "000f42e71a69214cfd939d1d2d6f790342fe718c", - "extensions": [], - "previous": "000f42e655501498ffb96c4c6b6d0502b6aa8ac1", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:20:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "200ba736994566192cf2f94e5df0742ba5e5e8fb957714e16c55e020541605a05a7ffd29bdc4431b399dbb5996d36ae7209731689937f1f42e0889f39ed253b913" - }, - { - "block_id": "000f42e833c5839dcaf2976a254cbdb9eb3163dd", - "extensions": [], - "previous": "000f42e71a69214cfd939d1d2d6f790342fe718c", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:20:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f14a06d2a36f79f5c00c0df1d01a808c7ad0f45514e69cb4aab536c6f9917a2504c6ce21f32cbab20dc4048333f3354466b6998b7a3a8e4e624959c17d65d066b" - }, - { - "block_id": "000f42e972c26069e9b408818a0b8101bcdc90cc", - "extensions": [], - "previous": "000f42e833c5839dcaf2976a254cbdb9eb3163dd", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:20:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "206285396e4d782f958e779c063faaeec0f2f21a08e65339a367826274a43f728d454f6cf04d0c52a3364da40d7549ff66297800317976f843dd3c7ff660b6e533" - }, - { - "block_id": "000f42eacd9341b2ef615118856e8c68a991483b", - "extensions": [], - "previous": "000f42e972c26069e9b408818a0b8101bcdc90cc", - "signing_key": "STM6emoLSjgfeKDoPPGm2HRe6uotTdHA1gkYYhjVZWFzPaq6x1aVB", - "timestamp": "2016-04-29T04:20:33", - "transaction_ids": [ - "54d837f44e5428124b99482352a6860b69bd251a" - ], - "transaction_merkle_root": "d32e9d6e71c887e775517f4f3613fdf8777167ea", - "transactions": [ - { - "expiration": "2016-04-29T04:20:42", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "steemit-news", - "permlink": "its-time-to-end-the-federal-reserve--john-mcafee", - "voter": "rimantas", - "weight": 10000 - } - } - ], - "ref_block_num": 17128, - "ref_block_prefix": 2642658611, - "signatures": [ - "207d001e406c7c3a192d522b361ac92f3e01a4bc6d39e48699a0b6f407c7eca7ca17a13db00354d2cb65c264f3845b84ce1a4d188ef8cebc90596987f181b9f13a" - ] - } - ], - "witness": "smooth-m00", - "witness_signature": "1f08b5eabd2aa6d88364f5b8324e47316ffd2e049b32ca905efa67ba5767ecafd2295ed7e4f8e6a646afc234d2114c2e7026ccf0b1b16d03562b6b7f451262333b" - }, - { - "block_id": "000f42eb33b7702e4d232bdc9bdb1f820a37cc51", - "extensions": [], - "previous": "000f42eacd9341b2ef615118856e8c68a991483b", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:20:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "206c00afd2308795469b476064dc62bba96251bbde93d4cdeb89663233893f4d6e2bb4ead5b04a4a44c0173f42cd285ebd0c0ccf0cce02bd171b47080dff4735e6" - }, - { - "block_id": "000f42ec4548d428c9d8526737d79a1d3d07eb75", - "extensions": [], - "previous": "000f42eb33b7702e4d232bdc9bdb1f820a37cc51", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:20:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "200a7304463f0511a00f881ae989ffcee7e4285bb9a28d9840ee61bfed99b282a53f822ac003e12bb7a8f186860083730cd4d315dd2fe26aa095f6e39a2c9d95e0" - }, - { - "block_id": "000f42ed05e815796d88cbc2e903c20d0f5d1507", - "extensions": [], - "previous": "000f42ec4548d428c9d8526737d79a1d3d07eb75", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:20:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f158dfe6b3e3424fbbc51ce69a9ba65df6998d6eb0c3d1187763a87dbc25b8f3b59cec4abfe4d2e1950ac84354fc60245a9b95f1d34b57998bd844504b6fe745e" - }, - { - "block_id": "000f42ee06fe0970f1a43425bc682fb4a2dfc1a0", - "extensions": [], - "previous": "000f42ed05e815796d88cbc2e903c20d0f5d1507", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:20:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "203c202932a39703a681968c8989a6570e3639a6067f429e4a9d4b6788513ae06c364e3fb0e6c46078d0470213cdbbad29bcffa479d7f6d2c2276f0ad6c07f188d" - }, - { - "block_id": "000f42efa0fd3dfae9d71772f4939c785f249d14", - "extensions": [], - "previous": "000f42ee06fe0970f1a43425bc682fb4a2dfc1a0", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:20:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "2050acb7868e7d751cacd5d9ce0a83243cc20a0f2b28aa97e00c685b4ddbfb21432d5c96cd1860ad610b743d0380cdaaf2ed974d0855a294c474d73fca604ee09d" - }, - { - "block_id": "000f42f0a37ec695a71e551d4396f028bda57ad0", - "extensions": [], - "previous": "000f42efa0fd3dfae9d71772f4939c785f249d14", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:20:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "20182e68a7a557307341be5917f777e291a9059b2dd51e243545df1c55c5cff6e74f435e762ca2d1fd94e36a69a316cadc05054715e18c2ad91257c549f6edd1a1" - }, - { - "block_id": "000f42f10a9b06ad37a11d8b0b0a5f97af89b0a7", - "extensions": [], - "previous": "000f42f0a37ec695a71e551d4396f028bda57ad0", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:20:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f22d32faf6c8268cf3d9fea59fb6bab289929805115540e7bb52c4dcde5c00e4536f6200e9ee289bf74ff75324f9583a0479d5abe8d44365c50efd0b68fe9c9ba" - }, - { - "block_id": "000f42f25248a7b9698e9dcdee4a3d0daef85364", - "extensions": [], - "previous": "000f42f10a9b06ad37a11d8b0b0a5f97af89b0a7", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:20:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f6fa89e6438feb4e69145a78fb0c61cd6fdb8b6bc3b349e9d924764db6bd836267954bb7a4d993e4d2cca4415847b0f37deaa36bb439d7978a73b1423430bc2cc" - }, - { - "block_id": "000f42f3093e19cb9df996fc331dcd0f19941872", - "extensions": [], - "previous": "000f42f25248a7b9698e9dcdee4a3d0daef85364", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:21:00", - "transaction_ids": [ - "0c7eeea18974f47b90e477436471014bc0368398" - ], - "transaction_merkle_root": "cc32bd7de976d18b9a76d79af4754adae7be1560", - "transactions": [ - { - "expiration": "2016-04-29T05:20:57", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f42f25248a7b9698e9dcdee4a3d0daef85364", - "nonce": "8830300102805972786", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "ac5a56af5f2256569f7cd1c0c1cf3c471d7557c6d1189eeee702628dcaa7eeac", - "signature": "1f0587238976db5eec11c7d79add22602cc33c92967a0059bf6a7f2a46aec8ccb17efb714101b1f1458743a0f98613c316241a0a6efb2b2368b59091b20c4ea893", - "work": "0000000b4c27149009fd8876d9ebdeff08b0797ab45ed1d1c23f4984f672f141", - "worker": "STM5qfXwkTZBD3HmLpssvMmi2CtQs6oAffjZcbjaUJ4zNsyMfMoTf" - }, - "worker_account": "orwell" - } - } - ], - "ref_block_num": 17138, - "ref_block_prefix": 3114747986, - "signatures": [] - } - ], - "witness": "smooth.witness", - "witness_signature": "2038bea09796654fa12ed43c4ddccd0a394b1e6e39303a644ec1d6214347626bcf124cceb3e3c314ab61b9de2b55a7666de91d42277c4d2885c6bdc790f9c1819b" - }, - { - "block_id": "000f42f46c79f27dbd4feb1ecce3580a74603f26", - "extensions": [], - "previous": "000f42f3093e19cb9df996fc331dcd0f19941872", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:21:03", - "transaction_ids": [ - "71f5728e77c4541fb5f7dc1227a4d807282da028" - ], - "transaction_merkle_root": "11d7a78afee01aff7082eea8075eb17f5dadedbb", - "transactions": [ - { - "expiration": "2016-04-29T05:21:00", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f42f3093e19cb9df996fc331dcd0f19941872", - "nonce": "17063973574701695350", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "845383112103e7dc0c570279a255651a4ca0f3c3b4f1c9ec39197860e4a87146", - "signature": "1fbf32b53773d88c78c889cb0e87ea9fea530d96bbb64da21e54f3291b84e3c1bf65aa6d6ec8362a56e1aa1d89c772ab595e70fe8bfab3db9e1c3ae3bfad6faf66", - "work": "000000002b70c8f33cd07e60fd5e7cb50b45c1722ecaf2d5b27b101f9a1f1ad2", - "worker": "STM57RKLJTbg7wsE8ecbhvqsjj1gLVgJwQm72YnspLeWPrwMaWT8t" - }, - "worker_account": "smooth-m04" - } - } - ], - "ref_block_num": 17139, - "ref_block_prefix": 3407429129, - "signatures": [] - } - ], - "witness": "au1nethyb1", - "witness_signature": "207553a6afa4a361d2c2872bcfc308623d2c79c816a95e6f63d450469ea85e239d4fb380dd9a5fd77f23aefef340f5ba7b38aaebd664202635ba86217d54e824ef" - }, - { - "block_id": "000f42f56f543990e27fb22e50306050313d8891", - "extensions": [], - "previous": "000f42f46c79f27dbd4feb1ecce3580a74603f26", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:21:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f45ca34a116a7ec83cd4b59e5e154a1f7fee92957bcfb2e5216b15672d4f4fe38135639c1a113c84aca9f85885f9ba634666786deae38367305f8604c4099bb19" - }, - { - "block_id": "000f42f6a5d48c99a1b82f61b6b63fcbedc52492", - "extensions": [], - "previous": "000f42f56f543990e27fb22e50306050313d8891", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:21:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f395b000c9136cc5c6c7b422e91b341bda127c3c82c26793e298d81cd6ccf1a9810a8ecd16eff943711bbc53315f0b36107bafe4df0ae98c5273b899b9142e1dd" - }, - { - "block_id": "000f42f741d85024907f1512154c88df0f9f615a", - "extensions": [], - "previous": "000f42f6a5d48c99a1b82f61b6b63fcbedc52492", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:21:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "2049a9688f957ec192ee25e6d0bf3a5ec025611675bf90d12d14096b6710c745110933f537cc0195344623228a60f7b99a87c2d1cb7fbc951e8958436d45620e25" - }, - { - "block_id": "000f42f893e92080cb2de523ef4b3cc77a65bda9", - "extensions": [], - "previous": "000f42f741d85024907f1512154c88df0f9f615a", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:21:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f05b395d6922e5625b61ca9d4ba762b1f16784edd6c0c072df22ec2e7fa83d6a52b02c12107d717b98d329560e55f04b46253a013aad1d449b9f67c79200e37fc" - }, - { - "block_id": "000f42f9dfea44ef81eb420d513b168fcc1d1ef7", - "extensions": [], - "previous": "000f42f893e92080cb2de523ef4b3cc77a65bda9", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:21:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f1c6da766b641442ee984ada3fbbb852f84771c85d914af5fba8a34d34b7f677454cfd03fdfbbb1d23ed0897a3d15f592ee5e2e29477642bd62b04daaf419c1a5" - }, - { - "block_id": "000f42fa5c8005d23ced28392c846cd80f271584", - "extensions": [], - "previous": "000f42f9dfea44ef81eb420d513b168fcc1d1ef7", - "signing_key": "STM8aUs6SGoEmNYMd3bYjE1UBr6NQPxGWmTqTdBaxJYSx244edSB2", - "timestamp": "2016-04-29T04:21:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "ihashfury", - "witness_signature": "1f6afc656b35078969f3b0a32920235311675e57ae0e1631dbaebd37961b1750ae4392242afd132019750906fb01dade95e5268e1818a86924d3feea6da11f0024" - }, - { - "block_id": "000f42fb5bd3fc3e4b9ad86949bb61c6a6610dca", - "extensions": [], - "previous": "000f42fa5c8005d23ced28392c846cd80f271584", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:21:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "207feff7f5cdc381c72a5151160c5d5c83d217e1337b3d8575f7f209c09e190b650ec0928ed4869e5f1d10e57d4f4be4e9d217fee1490f8a509948b33fdfb39ba0" - }, - { - "block_id": "000f42fc7f0fc442c55da3a574b22085c2c89bb4", - "extensions": [], - "previous": "000f42fb5bd3fc3e4b9ad86949bb61c6a6610dca", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:21:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f1b719609fd8adbe034d1c5057d35f768013ed4910f4fda95e112b87b1cf449460812a872cb5af54fb12b544dda8262e5fc5240cffd03d983589bf02967098b48" - }, - { - "block_id": "000f42fd5f898b3f30c4f7ba878ca03c637fd66d", - "extensions": [], - "previous": "000f42fc7f0fc442c55da3a574b22085c2c89bb4", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:21:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f1a922047e2fd9604b408bf97e4789c9cea6bba508e8e9c047cadf9c57a4c215f7ba20552f7d7612ca5b92ef5ab188aa505c05f730e7651145bec6e42cf165a52" - }, - { - "block_id": "000f42fe0ba9495069a4f59a805e7591c22f0529", - "extensions": [], - "previous": "000f42fd5f898b3f30c4f7ba878ca03c637fd66d", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:21:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "205d7d746d9c9cf13d298e6dde10388c3ea5ab43440d183a107ee190c721baf8c24e4ba4f50012e9b62843d9aa2e70fe8e9d9c77a5b1cd0bf4edd9bf12e5f0ab9d" - }, - { - "block_id": "000f42ffb4e033c3fad135ecb1fc419f22475add", - "extensions": [], - "previous": "000f42fe0ba9495069a4f59a805e7591c22f0529", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:21:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "203b9653ceabbc3930e58befc55cbc201fa7a3ba3682f473ba6310a25cbaa923a3147baa739750f1f90fd77f3ed111bcc9de75dc88caa703c30b5fdc2422989555" - }, - { - "block_id": "000f43003829fd774203481363b2b6e4c1c89bc5", - "extensions": [], - "previous": "000f42ffb4e033c3fad135ecb1fc419f22475add", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:21:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "2034095797b805f791d38c63ef5b1a736143fe6601fc60b7e1dec784af8f8b5ca56dfaae592651b0fa2ee4959a34d3a96813a6e02bbe314eed2a65d9bb8ab9126a" - }, - { - "block_id": "000f430155ce5b5625fc2b10b2f512a80a5d0354", - "extensions": [], - "previous": "000f43003829fd774203481363b2b6e4c1c89bc5", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:21:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f6127da56ab7b504893a90b7aebe482d1fa163395412bcc0f3a9b61b4e7be14461e043f0da08ac6b4d6841d6969170c8482e64e3cc2a223e7ebb191adaa264b95" - }, - { - "block_id": "000f430213c52ba86a1edc70434557512c836942", - "extensions": [], - "previous": "000f430155ce5b5625fc2b10b2f512a80a5d0354", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:21:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f3ca60809876bcb8363fbe112ddcaa88262a1b04e40a9dfe0643420b8e186595416ead4eb4e074dd3c83ca163ab4cbe1863cee9ef61ab9696b02bf3c2a5ab4637" - }, - { - "block_id": "000f43037cc4fc55b17ebe59e74d9acfa737ac6e", - "extensions": [], - "previous": "000f430213c52ba86a1edc70434557512c836942", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:21:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "201980918c8e4cfcf6e011ded4e6e09356e0878cce6a545808f7b2f93eb765f44667fb9a044aece2c544c1cc3522f5241ea22d5139bc1f61dbc4516c0d83722d4c" - }, - { - "block_id": "000f4304719b484dd32f3f5072c89c379d5b4ab7", - "extensions": [], - "previous": "000f43037cc4fc55b17ebe59e74d9acfa737ac6e", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:21:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f27f96ca65ec9cad2f62b1f5eced44f3d34ea9228a034648235a44431d3d58cc86dceb706dd563d643778c35f6137e4b352a9ab7701b68a69022270151e6a3deb" - }, - { - "block_id": "000f430599d66da7b53aa0c461e955c9a30f86ef", - "extensions": [], - "previous": "000f4304719b484dd32f3f5072c89c379d5b4ab7", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:21:54", - "transaction_ids": [ - "70f63bc14982502b4ecaa0501762f76978f7f216" - ], - "transaction_merkle_root": "679566456e5714dde56650a802ca992f298876a8", - "transactions": [ - { - "expiration": "2016-04-29T05:21:51", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f4304719b484dd32f3f5072c89c379d5b4ab7", - "nonce": "5566925113366570490", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "429675d360f1a30de9b1cee8575aa633f80b6021099d20eed543fe0248874ce0", - "signature": "1f6f2f337f5f9d8acc624d8b7a70bbfa0c34c43ca6f53802a2522531b8d12780cc02a2847fe4c1a265672d285b9090229a0cdb8be35bb13f7b2abc62ce3ce2a16c", - "work": "0000000e7b25fba35d5c005ee75f58bb0ddfed104ea9171ac27802b1ee872cd9", - "worker": "STM6QdeULVBq4q49STGGWkfbUk7dmGSCkwxyApRW9zELGu31Gpe4X" - }, - "worker_account": "jamie" - } - } - ], - "ref_block_num": 17156, - "ref_block_prefix": 1296604017, - "signatures": [] - } - ], - "witness": "steemychicken1", - "witness_signature": "1f7319812d7df9e58fb3dc8817b82215514e665026b55da47e7b1bf38a8ac4340e3a59c374491d4df61179b03b403988d7c09d590f8f5bf6d3ff7001d73d10cf1e" - }, - { - "block_id": "000f430650ba950aa10a88723bc28c59effb9ac8", - "extensions": [], - "previous": "000f430599d66da7b53aa0c461e955c9a30f86ef", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:21:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f49142d2b6ad74879b64596711eeed0108e20e8c5d6f5e30139a1035deca107a0669f79086998f36b7446c216c7a3d1aa94d49e4e10a0915654768529488962ba" - }, - { - "block_id": "000f43079dec04c97f45d7d90a950d4bfca6e2cb", - "extensions": [], - "previous": "000f430650ba950aa10a88723bc28c59effb9ac8", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:22:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f39d744c877bf8915c0391a6127865dbd6e77c67a86c4e2cce8daad2c185d88f521bcaa683e3743eabb24d3d03b3efa7f7d3fcdb61fa0958901896ad42ac78f0f" - }, - { - "block_id": "000f4308f1022e2482e3db92610b17811d16cb68", - "extensions": [], - "previous": "000f43079dec04c97f45d7d90a950d4bfca6e2cb", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:22:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f6970d260cf0d853f1c81321049cfd78ccabc053ace78499576e19c21e488e0d31a2692d17952be706d3eff2c87678deff8fa15fe6ecf21e8c54b4a1dbf5a723d" - }, - { - "block_id": "000f430916035b8a65d0fb050a4903fec8183cda", - "extensions": [], - "previous": "000f4308f1022e2482e3db92610b17811d16cb68", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:22:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f33d99b39638eb2d00e605dfad698113fdb0b9773a308f65b8b629881ed568e422059b54a68c33484ceb1db9d86391b650d5d7ed45bfa0b54164765d0d2bebdd0" - }, - { - "block_id": "000f430a5599bb470c0803d08605422dd0367f23", - "extensions": [], - "previous": "000f430916035b8a65d0fb050a4903fec8183cda", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:22:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f69f8a1f8d159ee325a86ac9a339a073fcbf357a4a56616a4a330e3f3fd487e1d4097c8c782a0ba5f61789f44e43fc8d103d442ef688003d0ef948ca61a346717" - }, - { - "block_id": "000f430b56beb3ed2d59318578472564babbe89c", - "extensions": [], - "previous": "000f430a5599bb470c0803d08605422dd0367f23", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:22:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f462192a929e2c9414ecc8b2ebf43610b3119b48d8ed0db06506b7022d92bbc027849504143da8405f0961b0cee8c55588459c50f77cd922d52a43b4ba4f7fc17" - }, - { - "block_id": "000f430c703aa170e0eb1b2ea835a41d4fd6dbe0", - "extensions": [], - "previous": "000f430b56beb3ed2d59318578472564babbe89c", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:22:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f50d65a8c8eab36e6ab2175a20e104ccb4cd590cb961b427b01521b2fd42411ef507f6f0ee2f314deea4d3219a19a0b8f764d585e3f700eb9c96f27bc8bfe10de" - }, - { - "block_id": "000f430d448313bb96a255cd54041fde6b5d9fbf", - "extensions": [], - "previous": "000f430c703aa170e0eb1b2ea835a41d4fd6dbe0", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:22:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f01dd3e03bcb05f1ae0423b125d423217cba3df1d49348c54934744c5baaf566434eda1e8034b35b507e72cc648e3ccf7e783457749ac83509471a8e799499f43" - }, - { - "block_id": "000f430eb07d50045bd738b7759a9b2fef65cfa6", - "extensions": [], - "previous": "000f430d448313bb96a255cd54041fde6b5d9fbf", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:22:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "204ce2aaba3abda1f2c430be95cb529f985ddb2c71c5afc409cacf2bd6083423cb72f320b869d96b367e52b5f87ce61fcdca1b35e72bdd2d1f2d7a1f98bbd4e647" - }, - { - "block_id": "000f430fc017eaad28067ba3751901549158b8d9", - "extensions": [], - "previous": "000f430eb07d50045bd738b7759a9b2fef65cfa6", - "signing_key": "STM58QMgvDU4W88PtpEpy289EPKqUC2JELXeNGm4Vm8EgzUP43DHn", - "timestamp": "2016-04-29T04:22:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "delegate.lafona", - "witness_signature": "1f378e09201f89e85b0704f7b2d44155c40210c45bc3bcbbdd4b5d74b431340ca24e43fd88eee6184c6b71c67297db25486889766d806d098badc14a7b3a59f48c" - }, - { - "block_id": "000f431090e3b497671ba2e0038ec4723c63badc", - "extensions": [], - "previous": "000f430fc017eaad28067ba3751901549158b8d9", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:22:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f3478f3a20e3978442d5fe9cb8e5ad632ee9f7c98c2e6bf026a75ef228a0e501726ae9da9706c3b09a08a4c19902c8a7fa9c3abc22f02dc9496a86f0d4ca65a17" - }, - { - "block_id": "000f43118dc8c38529125862a354584efbab1a81", - "extensions": [], - "previous": "000f431090e3b497671ba2e0038ec4723c63badc", - "signing_key": "STM7rRERTkwbmGY3QhJfrjXXpmqjaYfJeqeQTMzux2sUQzy74BLue", - "timestamp": "2016-04-29T04:22:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "self.esteem", - "witness_signature": "1f4d51720c2228db93a1f9e5e23741bc8ea7ded91fecc5a83ff486a18981016f4d0eed16f89363f947bb63d54ed0c60e6d0b2e54c6c1bddf97c64797b11daf92d4" - }, - { - "block_id": "000f4312581c8a099cf8d411bdb99fdd527b4dd6", - "extensions": [], - "previous": "000f43118dc8c38529125862a354584efbab1a81", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:22:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f5e6da52a54df1e1c43231566d2e75da0ecc14d212716dabd797a05dedb9a287d63b5fd3aeebdc7dd8ff0395af34dd860ee06ef11f42d2253e7a11f04ab37e76d" - }, - { - "block_id": "000f4313b146a6247682f08471fdaeca1bce0b5c", - "extensions": [], - "previous": "000f4312581c8a099cf8d411bdb99fdd527b4dd6", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:22:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "2053fd09e76b0f89fa82e48b070fa51dfcf71ec3f3af52c8c82c167f36abb4c839459ba8e563c2769f0d5e70af9a7c33ce53e5849a9c30eb909197dc0fb741d528" - }, - { - "block_id": "000f4314a199fd40a1f9de53dc9cf5129f876dad", - "extensions": [], - "previous": "000f4313b146a6247682f08471fdaeca1bce0b5c", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:22:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f0c44ba47f30acaafb5f379d3ca0d0d6b5f73836b1397672d97053ed40c85d2aa73033498dde071757fa39011a13dc80218b33e49c4192ed265734fac9b23a9b6" - }, - { - "block_id": "000f43158630d31a99fa30dabe72d1c9aebd5755", - "extensions": [], - "previous": "000f4314a199fd40a1f9de53dc9cf5129f876dad", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:22:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f70854c7f81922601658f39d8128e002039b15fb106bc2d5252401373df9863a50abbe7253628541b15362cd218fb3661d4b80d1557cce7d5405b42bef73a86bc" - }, - { - "block_id": "000f4316bca38253a329a771cc894f55030ab6a2", - "extensions": [], - "previous": "000f43158630d31a99fa30dabe72d1c9aebd5755", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:22:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "2043564c10ac2aa129f5965d1edaa1ea3ad1309c96fad14707146f17f3854428e009b90ce99bcee91e9b3e23ff2273c2ff20cc7df49f5828c97d505064f6f2d81d" - }, - { - "block_id": "000f431772d380ca9c212705b56cfe3f0425a071", - "extensions": [], - "previous": "000f4316bca38253a329a771cc894f55030ab6a2", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:22:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f1dca7006db4e4d616e7d2c45e967610b05a1abfb72b2d221b6422f55df512d644a60ae6faf0d5605562787336ce7d28a3deb5cbbcdd8f6dec80881561e1e4030" - }, - { - "block_id": "000f43183ff2c79bc6f34e9db7d7fa3421af61e2", - "extensions": [], - "previous": "000f431772d380ca9c212705b56cfe3f0425a071", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:22:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f69f18f6260e60da637227d8f2465720be1ab7761b33d6c75ee7c1a5b8a95fa5e0b8f6f61a581eab697a3bfba0ee4496f088a63a9c107e3bbd4153dd86683f210" - }, - { - "block_id": "000f431980b2cbb4abfce29cf722987af1690dcd", - "extensions": [], - "previous": "000f43183ff2c79bc6f34e9db7d7fa3421af61e2", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:22:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f0f835f581b22c9e13a6c4f1133271d1fc5bc081ad616fdfbb65413ebcc1a3e853d08ceefdfd7598e9d992f6ebfc30ac7f12af5cfa90e68a24e23e7eff870431a" - }, - { - "block_id": "000f431abb4f1a4e969c244a8e6a27b14d322f85", - "extensions": [], - "previous": "000f431980b2cbb4abfce29cf722987af1690dcd", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:22:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f5376215b4c143d42986f2e5dc4407aa2a3027f358fbd60315528477a016ad27b7bc346c2a7262cc70168b66d4b213965a80767ae4eea86a7f1f422ba668d1d83" - }, - { - "block_id": "000f431b8a723f9d98daa15fdc3692cf32673216", - "extensions": [], - "previous": "000f431abb4f1a4e969c244a8e6a27b14d322f85", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:23:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f5c26aad0b5fd7bd731bd69c71d969f3ab17bbdb58ba3dad3d6f2afe41bbe5649159899a742218790d7e628843e3b61261947a8a4d480c756e440f4288803ad7f" - }, - { - "block_id": "000f431c3440276cbf39bbd39f4fa95e6b53cad6", - "extensions": [], - "previous": "000f431b8a723f9d98daa15fdc3692cf32673216", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:23:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f583dbdabaa6a7614a095075b860976a6b9a8369ae45e7c8a3ec5217739396d280ea1c2ff206f3b105b9b9c865e5e4cc303f029858ca4a5eac4a962f734a95d08" - }, - { - "block_id": "000f431dafde5588c49be140997a8a6e5efc3d39", - "extensions": [], - "previous": "000f431c3440276cbf39bbd39f4fa95e6b53cad6", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:23:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f023bf1db3347997d9a163887c7cf9e0dfa14f72a686fd6717060ac67610d70b107a0b3109389a37d645cb075ba717c0e6771618f67455723c8ab6b3073c20820" - }, - { - "block_id": "000f431e3806879f45daf31c98864b06e4bbfff3", - "extensions": [], - "previous": "000f431dafde5588c49be140997a8a6e5efc3d39", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:23:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "2016c0d92b06fca187b56c78c698ac9d27fc3bbff1ebc4f93934bf8a115471626f6eda1431bedba49d73f47e8877a02582a97d52e63b763d2da85e1b90102cbe40" - }, - { - "block_id": "000f431f5f4c93acd875ac13f4b5109ddc1fb653", - "extensions": [], - "previous": "000f431e3806879f45daf31c98864b06e4bbfff3", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:23:12", - "transaction_ids": [ - "00b002a5a301ab89d46ffebf694683c5d3dd965c" - ], - "transaction_merkle_root": "ba3dbc6aa8d493b61882936fbb6cd8c492a22fdc", - "transactions": [ - { - "expiration": "2016-04-29T05:23:09", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f431e3806879f45daf31c98864b06e4bbfff3", - "nonce": "9476320701278900471", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "f92fd064e5aa64d9d795dc88d1c8853180cc6b03c710efa77fb9aa970d77b9a4", - "signature": "1f203a0a110a3c23be0b03adf0efeba198782d0e3d9bf023d0db83e4d188c026c37b69e2d79eec56c591afd082d87e0cfa528027b8a863df7f344e2cb108f89a95", - "work": "0000000f53e26391f89bdeb4177d6f37d45c2e0137d072f2952021a27116db7a", - "worker": "STM6Zaze2vwwqzgSeop7PUCKvpDyDowVbr99XPJKJgAYTUuGLjv2v" - }, - "worker_account": "ocminer" - } - } - ], - "ref_block_num": 17182, - "ref_block_prefix": 2676426296, - "signatures": [] - } - ], - "witness": "steemed", - "witness_signature": "1f4e8424885f81bc0a0ff205a5678b9a177462fe6801fc53fcced7deeb59ffdc22077feac37b9e2d96d7b4fc9d3dee0ff3d8d431fe79eb748392d1f121f34515c1" - }, - { - "block_id": "000f4320f5512faf4d792b4a96c1d517165ac241", - "extensions": [], - "previous": "000f431f5f4c93acd875ac13f4b5109ddc1fb653", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:23:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f3ea741e0be6c77b28e427e8898ea14b513bd52053e52dfef7d713b697c25a9553b97faebe9714ff0a709cedc6e57ba8a71ffcd2265beeb63bfb4c1035d3fd9a2" - }, - { - "block_id": "000f4321defaa3cb1e0a14793a55877c192dc826", - "extensions": [], - "previous": "000f4320f5512faf4d792b4a96c1d517165ac241", - "signing_key": "STM7HR2jZiuaVi7xJx8DnkdL1SvTVEDTP1pQZdmaTLGAnt4EqnscW", - "timestamp": "2016-04-29T04:23:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr902", - "witness_signature": "2065bc84925c7b8ddd8f190af5385dea8f88d4eacc04253d1f3d4641c643c629996ba034fc44f4cf02a39697b0318920a135477891a58c22719315ed04317d5ee4" - }, - { - "block_id": "000f4322bbcaf9186c09bcf16f91b2188b262c73", - "extensions": [], - "previous": "000f4321defaa3cb1e0a14793a55877c192dc826", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:23:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f25ea6954e6690d7b323d4d020cb2fa90bccc57d2c403221815709ed04e1340ca16f493fb4e2c86afa17427758c589a968c50c2ab1ce617078039d49e9585e8e7" - }, - { - "block_id": "000f4323f6e5a448a602b9e83d0053c800fe6417", - "extensions": [], - "previous": "000f4322bbcaf9186c09bcf16f91b2188b262c73", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:23:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "207aad5034029366bb151e6774c7e16061cdbdf30b1e31f7fb63498a34187089e21a58ed5ae5a92122514e6ef5602b978a0d489fe8e07c35432b04d2d7b3f1d477" - }, - { - "block_id": "000f4324aa0ea52c92af8f0b351c04e47dffb51e", - "extensions": [], - "previous": "000f4323f6e5a448a602b9e83d0053c800fe6417", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:23:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f26895a3e9a742cc3a2763cfc8e68e57f89a35b16f2a989bf19e659a1272ac8ae225fe12e0aac73022a75b04abe8497b4db9f1982c978ed437330eeb2690f1a32" - }, - { - "block_id": "000f4325cb7829b8893bafc6928cbd2fa8b28adb", - "extensions": [], - "previous": "000f4324aa0ea52c92af8f0b351c04e47dffb51e", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:23:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f79eaf11d7ed7595adedb46762ab7beb5755ad7443995480dec890502122b3df23022444eb3d7bb1e11c059df14f0357c65d4666788cad953d842b811374908a4" - }, - { - "block_id": "000f432672b80137cb4e66bdcaf886dce2a897ca", - "extensions": [], - "previous": "000f4325cb7829b8893bafc6928cbd2fa8b28adb", - "signing_key": "STM73XgqmDfLgXVF5VArK9rvHbUDbbx3HtfCU81Fy9MqVNxLYMpay", - "timestamp": "2016-04-29T04:23:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "jabbasteem", - "witness_signature": "20126ce4b1469037928175db03bd19e14ee45e38434c9683b9b41558d6e046f9102f6cbaf9bd52c22f5ba90da19a021f7d61ed0d86c3427d244c6e7d0d9625c8a5" - }, - { - "block_id": "000f4327eae472d133e668d1775b307bd5e934d7", - "extensions": [], - "previous": "000f432672b80137cb4e66bdcaf886dce2a897ca", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:23:36", - "transaction_ids": [ - "e575e0be388c5222a69d118d0badea5c1d733fd7" - ], - "transaction_merkle_root": "7179e95080670e26268794e988963fd6dde75d9d", - "transactions": [ - { - "expiration": "2016-04-29T05:23:33", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f432672b80137cb4e66bdcaf886dce2a897ca", - "nonce": "16041602454671123853", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "d531dcbe57cb443b88c88e86a6db769526fdddf4249fc68d9c28f8487cbffc61", - "signature": "1fd7b070426f925bf2ec18af93d349cef8d7f4095a7638970d3323c6fba8715c0f7a1e4fcf761c256da57d3308b82e082e7a9d19c291a91b8b8db0f23daa7f3e64", - "work": "00000006cd1e632f4d2f7f51d125983d670bf3ae4039c6c12e8bdf5238ba947c", - "worker": "STM6TdSaghf8561PAvaQxcXsqYsdZfLWGsEaB3wMofxdWj4PYWLZk" - }, - "worker_account": "peppers" - } - } - ], - "ref_block_num": 17190, - "ref_block_prefix": 922859634, - "signatures": [] - } - ], - "witness": "dele-puppy", - "witness_signature": "1f3ba3593e9e9f10030584a3cfe6903e226cfc8a2d7032e8aae481818466bc962e78c3a729f211a2ba5539e5272f68c7354955d73d24d8bc448f7666b4a9d27f91" - }, - { - "block_id": "000f4328f262455c166a5e178f922fa8c1b7a3cb", - "extensions": [], - "previous": "000f4327eae472d133e668d1775b307bd5e934d7", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:23:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "202432120ddb9d88652febc137e672918e19d4d059036e35fb697588c8ba82cbab28931f3fd37ef78ff406b70cbb5ef0b79ced829a2a09a25d8bfa8f9ac2f4ef51" - }, - { - "block_id": "000f4329e632ff347bed8b6d9945937c5b626675", - "extensions": [], - "previous": "000f4328f262455c166a5e178f922fa8c1b7a3cb", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:23:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f1f9f2cc90aa3d88e7f866603cec5f8d7487834695625950b55b69940b02453fd2bdc711676c51889657d35c0e72ee20b26311de5e9ccaab29cc07928457136b2" - }, - { - "block_id": "000f432a8301379948ced4a698ece781908237af", - "extensions": [], - "previous": "000f4329e632ff347bed8b6d9945937c5b626675", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:23:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "20559fb8a6b3f2b5f791d6707f4eecf4525dc183ddc080379344c2855d7548f0546e30eaf04584bbf0c7ecf6b5ceb55664d87c41d4a85cc85ba2923199e4b970b7" - }, - { - "block_id": "000f432bc72e8f0399e73c3308525b7a6515e2c3", - "extensions": [], - "previous": "000f432a8301379948ced4a698ece781908237af", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:23:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "2016c8a705c657296aa415cc947f0b27ebe5ece75dacf9c9bea4a1bd330b3c8ec607f5bbeebd52e2b524288aa3ffd6b3d34361a3ebcea353256b4fbee9c574f064" - }, - { - "block_id": "000f432c6376b6865c3006344a98c6c9c2dd414c", - "extensions": [], - "previous": "000f432bc72e8f0399e73c3308525b7a6515e2c3", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:23:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f02c912d0727b4cddc8e3fcd37680752bb5a15b381d06ce94a8ce8aaea0eaf8e71e16e18fd02d346bb9d003c05aa237204d9ba5e5750256e7cc40ff8473612ae3" - }, - { - "block_id": "000f432d24e0672451246b5f65eb9b8d98869e03", - "extensions": [], - "previous": "000f432c6376b6865c3006344a98c6c9c2dd414c", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:23:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f5c57dcb3061be988984f75357605c24b230dea5a72fd79961c914056ac7e72f739c6ddbad21bd2c2078c1b533cd73f80dec852bc6d2b380a978a2c45f794b6fc" - }, - { - "block_id": "000f432e80859f0d294388738d1a5cc80be4de53", - "extensions": [], - "previous": "000f432d24e0672451246b5f65eb9b8d98869e03", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:23:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f0a4724765a2836bfdb8bfdc4c0674d53b180945be4d44537ae0814686cbafacb3901535fc8e60fa6a1593024e692613f87455fc47833cadcdbe4bbf805dd4022" - }, - { - "block_id": "000f432fcfc35fa7c5b711cf164b793ec139b255", - "extensions": [], - "previous": "000f432e80859f0d294388738d1a5cc80be4de53", - "signing_key": "STM6BJYGHftujnbttFFKX6YacnvsMd4sbJrbucg682GiU4vmXHTik", - "timestamp": "2016-04-29T04:24:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "riverhead", - "witness_signature": "1f54a4248bdbb6ef19c9b337cf86359f140492e91e142d8f1498d93aa83f76a9112435ed919d6640fbd58c475710f7d42360ccb5b82cbe0e3f30c78605beb4e2bd" - }, - { - "block_id": "000f43307569414e895cdbf0a4c8670cc273fdfa", - "extensions": [], - "previous": "000f432fcfc35fa7c5b711cf164b793ec139b255", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:24:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "204c9752c4b82bf43ebc2d92e399abf7cec81258fcbd12f0901804e2797b419ef05fda420ca43ab1fda8dc6a8d02d94b6387460ec07e2a73a5ed2ea53dfe864f99" - }, - { - "block_id": "000f43317bf1e20d1268a61010692e4128ce2339", - "extensions": [], - "previous": "000f43307569414e895cdbf0a4c8670cc273fdfa", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:24:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f5080a9f85e4ad76accbbf1a589a15ea842d6f587d215f08ecb2dc38d066c97f2380d5233bd59ec11c0934aa2035b87a30ce1c9f5138e9dd035e9de4c68bcb9f3" - }, - { - "block_id": "000f433299c22553b76c3a9d9d2b080b62279421", - "extensions": [], - "previous": "000f43317bf1e20d1268a61010692e4128ce2339", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:24:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f0b17566bb1f8e59dba9a33b67ba99b4af27c500ce8a5ab87f162008d93269c7b36e137282ba5baf1850135d4f251450cdfd0da960e9cbfa0284fcda5e19b14a7" - }, - { - "block_id": "000f4333666980a995d494fcb0816c98c8cf5b7c", - "extensions": [], - "previous": "000f433299c22553b76c3a9d9d2b080b62279421", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:24:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "200908da54129dae445a192fa9f90a1c38c3d1973beb1053e6cf5d6e26ef6e14771e44115b37fcaad80a6a277dc0504058dbf1f82a5ecee219e761e43f26eeac40" - }, - { - "block_id": "000f4334af78dd78e78570f226d9ffac227e0fa8", - "extensions": [], - "previous": "000f4333666980a995d494fcb0816c98c8cf5b7c", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:24:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "203f443b8080a2fe7b2bb64b214855b83120fa32dcdc4df473c8e3caca7ae59cdc19330a14856d921183eaba3f8abb35001e29abdf405c0be8b9ce7e93a0c2e453" - }, - { - "block_id": "000f43353fae478d8ea0be7a5140191676b93d04", - "extensions": [], - "previous": "000f4334af78dd78e78570f226d9ffac227e0fa8", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:24:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "205869aac7aeda28c52e7cc95fb18d5f91e89444bcc8ef23dbd7428e895f8adfd538c5b42f3c7c455137b0048b213f13f19f0ac6b847b4b43095dfac5ce7cb99ef" - }, - { - "block_id": "000f4336d399cb3c6df88b562cd27daa53615301", - "extensions": [], - "previous": "000f43353fae478d8ea0be7a5140191676b93d04", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:24:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f0abb8de63078504f5d5496658310974a2e8503f00373e711b5f0edde21eab1b36541ed69a10734f55313c6c19e832d5252d86100e85032db1c486e99c5bb059c" - }, - { - "block_id": "000f4337612bd82e4966f2fb72a091336cebcfd9", - "extensions": [], - "previous": "000f4336d399cb3c6df88b562cd27daa53615301", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:24:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f73a2a856317f65cc5d9f1a87f8bba3854369853753ed1488a12500906037ae3e3a06caaa2068ce21addc24cd62d017fae23a40fb55d57747080bcb17845bb366" - }, - { - "block_id": "000f4338a564c99aa92d834623b834b9fa64983f", - "extensions": [], - "previous": "000f4337612bd82e4966f2fb72a091336cebcfd9", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:24:27", - "transaction_ids": [ - "9076f4aa94b6ad073edaad1e4656d59d24c8fc37" - ], - "transaction_merkle_root": "f4b61d49e22fa80c0e2a043e26a6fb5d520b7f90", - "transactions": [ - { - "expiration": "2016-04-29T05:24:24", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f4337612bd82e4966f2fb72a091336cebcfd9", - "nonce": "12699203331776964407", - "props": { - "account_creation_fee": { - "amount": "10000", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "5b35e35e9b85eeee40e4827df6d3780423369cc7878ab561e16ec9668714096a", - "signature": "1f164386d61ab7363f6ed18ab82c5894e7a0dcdd5f0fe07e1fd3c046e11b613ee627a51fc65de8b1d9a30bb29b0938c383b10f3082eeedc04403999a8f04f57b72", - "work": "000000063799754e49362abfa01557dba3a06da11867d1f1f312804c1b53a298", - "worker": "STM8iDmFbqQYvoapLqxZUhUqmxXUZTAkMdzqJGCxdm833QY4JSPwn" - }, - "worker_account": "riverhead3" - } - } - ], - "ref_block_num": 17207, - "ref_block_prefix": 785918817, - "signatures": [] - } - ], - "witness": "nextgencrypto", - "witness_signature": "207c1bf4076db49a5d39a7a8bc5cb5186a2417e17e9337b82e7139d4fbf2b8dcdf1e184dee77f0929c598c4c787be410fe5945658b2bf42037e294800f45edc8b0" - }, - { - "block_id": "000f43396f3f08ff869c385fb36a44cf17bc072c", - "extensions": [], - "previous": "000f4338a564c99aa92d834623b834b9fa64983f", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:24:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f12c250b7c8ed3570e34d9ce70435a7b14de7e63437b5f1bec9ac7a69789bfa004c78302a734d2b504ffc25e95711abed04901c2724487974452b0a47a97459a0" - }, - { - "block_id": "000f433a8a7359def77d2ba828a3e872c7c5f232", - "extensions": [], - "previous": "000f43396f3f08ff869c385fb36a44cf17bc072c", - "signing_key": "STM5UVXLGQA3pD9SRxsdtQLrAKrYDtMoe5qSU5innKqxvjQ9Kzd64", - "timestamp": "2016-04-29T04:24:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "books", - "witness_signature": "204ae5c1343eb346181843dd674ae7d75500388f3e2d483ad4da6745673681e76d3476d86190b96584e77fd477d84f76f6c82bda0fe9bf3ab95af6151efe556ecf" - }, - { - "block_id": "000f433bd5b3aa2f2a6812c43fe57e278d7d23d4", - "extensions": [], - "previous": "000f433a8a7359def77d2ba828a3e872c7c5f232", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:24:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f02dcc6e26505fd3b47129675e824c75aa463e436a0ee9e029f6af368408a44106d34fbff60c3ead571b202e787c619cf5b93cf60353654098fd5d6c5ff6b2540" - }, - { - "block_id": "000f433cadc2cc01af8b02c0f0dac6229a5dd956", - "extensions": [], - "previous": "000f433bd5b3aa2f2a6812c43fe57e278d7d23d4", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:24:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "201748dff7c49f6f6ab69a5e874650e42bde3a3f3b8a72cbfd877ec5484c7d48684e8427f5f5fde8a905f416fcba6d6818cc72bc373384268f796d6c83102706f0" - }, - { - "block_id": "000f433dec6aa0f30331a6b7b9a5c67754fe95b0", - "extensions": [], - "previous": "000f433cadc2cc01af8b02c0f0dac6229a5dd956", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:24:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "207af069579b8a73176d1ac2d009f70c96dcac832f6d9cc00be209f124d667089078be684c51411bd3efbf71f40f65cdf1dbdbb10859c79f3559ec56ed4ca69a8f" - }, - { - "block_id": "000f433e14cdd36d87df6b3b098ccf1e51c0142e", - "extensions": [], - "previous": "000f433dec6aa0f30331a6b7b9a5c67754fe95b0", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:24:45", - "transaction_ids": [ - "d536f8a2a66acd444d8bf9da7bd6af1fd4ddb366" - ], - "transaction_merkle_root": "b962b1a54bb010b532c62004bb04c4cbafdd54db", - "transactions": [ - { - "expiration": "2016-04-29T04:25:12", - "extensions": [], - "operations": [ - { - "type": "comment_operation", - "value": { - "author": "steem-id", - "body": "I think its because mixed content protection on steemit.com. Firefox automatically blocks insecure or mixed content from secure web pages.", - "json_metadata": "{}", - "parent_author": "dantheman", - "parent_permlink": "re-steem-id-re-dan-re-steem-id-steem-seed-node-list-20160428t165903001z-20160428t235939283z", - "permlink": "re-dantheman-re-steem-id-re-dan-re-steem-id-steem-seed-node-list-20160428t165903001z-20160428t235939283z", - "title": "" - } - } - ], - "ref_block_num": 17213, - "ref_block_prefix": 4087376620, - "signatures": [ - "1f3270718bfbac6bfcda9305559943685507a734bbb707ea45c9c67f5dcb2e61c86fcd3ec71e746eb7047dc5011e3d13438b9bd2e849f975f78143b071f13ee67c" - ] - } - ], - "witness": "arhag", - "witness_signature": "1f51f63a529c0cc089fdd51583731ae490e3632b472d3b58208c015b051d6c703508f97d472af9ed0b78604efb7dd35610c393a79680bb3234c0bbca93f1996b17" - }, - { - "block_id": "000f433f642be1aee5cd4492fdf048a0fd8b9914", - "extensions": [], - "previous": "000f433e14cdd36d87df6b3b098ccf1e51c0142e", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:24:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "202a19e17cd3ba80ff2d950158ca230f765389340c6521f1ed6b8522bde0e6ff494172cf266de12d065af7cb853107a30c41ff74cb047b9cfd972777eb726559c7" - }, - { - "block_id": "000f4340e038f1a3b4b049d1c226efc3507f94c3", - "extensions": [], - "previous": "000f433f642be1aee5cd4492fdf048a0fd8b9914", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:24:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f381244216147a1f3095dc0186f6bf2d642319828b7b11729bef7e8cad379582169c447fdee936950165ef6244fba601d253ab1267c43f050d44301f98a46abc3" - }, - { - "block_id": "000f43410384198c801e40a1b1629b92404158bd", - "extensions": [], - "previous": "000f4340e038f1a3b4b049d1c226efc3507f94c3", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:24:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f150ef923961065e2032a94c50c83e05367f3641b437e2665cac6dd673fa124da19e94f810f59e4f60b5028373b47d8fd72849f4c8e8b952c72e49245be7cf1b7" - }, - { - "block_id": "000f4342a084a60c9e8ce2b2106c1e115e72b754", - "extensions": [], - "previous": "000f43410384198c801e40a1b1629b92404158bd", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:24:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f2145fee771ad2e3ae41b74f9d5815e6521784e546e571ff402775c3e89a8804b561664bdc221bec6bee2628445b37d222094915631eac057e0a65a98732b7981" - }, - { - "block_id": "000f43434d3b206ec991977b59bd5c6ec76de728", - "extensions": [], - "previous": "000f4342a084a60c9e8ce2b2106c1e115e72b754", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:25:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f1d2a0ddf403b9139eff720367ca50f7a818f992b0f785ea1526c311ea6f35e366147b3920c32a92c5b3c1868b85722223b48edeef9d3c2a7a22dcd61acd19419" - }, - { - "block_id": "000f43444910d731fb4331c8b770a5d02c80b82f", - "extensions": [], - "previous": "000f43434d3b206ec991977b59bd5c6ec76de728", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:25:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f49d0df61d9d572754c9ef49bb45e7fadaf0549277fcf3141c8c86f06603b136103e03eecfc38153afbbd5535fb8e460dd671dfbe69e6e39a47e22645e9cf9373" - }, - { - "block_id": "000f434592abe68c4819c54437f5f90c3eb0e3c3", - "extensions": [], - "previous": "000f43444910d731fb4331c8b770a5d02c80b82f", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:25:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "20094155c7a4efe6f41dc00191151bd6a78962c7af1843bf81d39f29ce744f80c91d7f5616512ee7d1020906ad63c0abe93379a64e92a76ee0cc3e3e4d4261f0d8" - }, - { - "block_id": "000f434681546d3b57dbfa2396c89840415543b2", - "extensions": [], - "previous": "000f434592abe68c4819c54437f5f90c3eb0e3c3", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:25:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "20253b110c7c5d0ea0f15d182129090d6526ea2fb281a053b320794ad9196df701059ef202c77747fca9cce2bc2221350400ecc3ddfa5f22eae59facfae76542ba" - }, - { - "block_id": "000f4347d3e5c2f333af408344a3b621480a3eaa", - "extensions": [], - "previous": "000f434681546d3b57dbfa2396c89840415543b2", - "signing_key": "STM4vhtognZwHz1sNX2efiySFap35k5M3g186nyjBAnbwPkTHCBT8", - "timestamp": "2016-04-29T04:25:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "joseph", - "witness_signature": "1f6cbc27a18037c4813e7be6534439b2b389d03064436a49eda2e45961919e986102896253bddd8269db713d05f137479ad51958f3f02a15035936e23bf9b9a2ad" - }, - { - "block_id": "000f4348a3be97e47b64435b506d48b62a199d29", - "extensions": [], - "previous": "000f4347d3e5c2f333af408344a3b621480a3eaa", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:25:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "20402f43c9bbb7a4216a4417454f38c3ce8b7a786a036d59d60228a596d4bfa3d527718c84f45a91c02d40095908411c246c66aa9f91e6128a4536738d4455637b" - }, - { - "block_id": "000f43492103dc6bc100ce5fd52f07cb63d01f16", - "extensions": [], - "previous": "000f4348a3be97e47b64435b506d48b62a199d29", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:25:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "20085fee1fa2e3aa8fcb5a555735b284c6ceda1780c1c32d9462fc143f8246fe6d1e52fa19ecda1cdbe2260e850e159983c8be7a46e7c883867a4fa98694311d82" - }, - { - "block_id": "000f434a0c01423093b7ad7efaccbdaaa32b6291", - "extensions": [], - "previous": "000f43492103dc6bc100ce5fd52f07cb63d01f16", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:25:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f6b6401e2b1454b0d679374105b1a1c822d6cd49f0d1382faf5851412e8e561ed2761b1771d230a18b8194e468c33a7c8a6e9b74ce8b2e3c47f4f7fb26e990592" - }, - { - "block_id": "000f434b9b719e0f6e7bba32d2542871a168ba05", - "extensions": [], - "previous": "000f434a0c01423093b7ad7efaccbdaaa32b6291", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:25:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f0e95f4c71b78acc18523023073efc130981195f92e79361cb63a54fe73cdb2a6498512a61f5cf13a0d468d96ae926e6bdace569e2eb7ca4956cba9019b9d5987" - }, - { - "block_id": "000f434cd5fd147e8ee9a77907f2aec81a77c1f2", - "extensions": [], - "previous": "000f434b9b719e0f6e7bba32d2542871a168ba05", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:25:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "207190666c3815e6eab1f16d9ee4d1f20aee7dd11244220d84101bdf75061498f65d6c043bad656b6621ad886a27216f2d32af28fe520477ae671e945ac07864cd" - }, - { - "block_id": "000f434d5858ba4eb771c64a6f83d12c5651b64c", - "extensions": [], - "previous": "000f434cd5fd147e8ee9a77907f2aec81a77c1f2", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:25:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f55baee6cdecff0340603af0d600571877a796a4e8e1fec7d92dae9011a203a8f7c3700cbb11fe5655649d6f4279816cb4aaa852f8557cc7841b8679c5dce32ee" - }, - { - "block_id": "000f434e660bfbeef21ed851d8f504b16246b1d3", - "extensions": [], - "previous": "000f434d5858ba4eb771c64a6f83d12c5651b64c", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:25:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f72168bcaf48d200e324d587b6d3c09c81d352db5626ae5a611491ab3851cadc30e6e51eca6e8520021bb2b33693840439673bd350b1d78378a8c04aa1da177bf" - }, - { - "block_id": "000f434fc6e8634ec50d6f45523c235a3d0a01b3", - "extensions": [], - "previous": "000f434e660bfbeef21ed851d8f504b16246b1d3", - "signing_key": "STM8Mh5ZPS3EBD2YWpXtaeE94quqJ8XSG58ZudFyJBenrJ3y2MX1B", - "timestamp": "2016-04-29T04:25:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "turbobit2", - "witness_signature": "1f3f5610c5ee23df87000a88efe466249edc33e3d88bda76d17a6bafb120f82a553444e1d6dc404aa825e2feb22b2b70d2280b95f82c8edc62e38f249345700b02" - }, - { - "block_id": "000f43503fbd5edfaf3a517fbf40e51f3de89053", - "extensions": [], - "previous": "000f434fc6e8634ec50d6f45523c235a3d0a01b3", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:25:39", - "transaction_ids": [ - "8c29553a4b2f99d47a234ed719351730d42fe460" - ], - "transaction_merkle_root": "e2c6c5f79e3157074fd775b2efb06cc40d359d48", - "transactions": [ - { - "expiration": "2016-04-29T04:26:06", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "441", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "delegate.lafona" - } - } - ], - "ref_block_num": 17231, - "ref_block_prefix": 1315170502, - "signatures": [ - "1f2953a6f681497fe63581b826e0e03dc7ff01baccd9a5cfddf447e31fd17aa85e32f439988bd459f7b62bd6f5e76f1f2ca2388a248c7812f6813f1df1c6f878ce" - ] - } - ], - "witness": "abit", - "witness_signature": "204b42947f74ddd9fceecfe7fbc1b18c24fdfdb89970bf195a4b0070fb3850d058704093a4c5068262964afe051029df3c5d6385ecd06f0e00a6faffd8a0261b7e" - }, - { - "block_id": "000f4351f4d7b504ef8633021fe02b09d1591172", - "extensions": [], - "previous": "000f43503fbd5edfaf3a517fbf40e51f3de89053", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:25:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "202f25ca052fffdb6e39d04971b71e33e645d2f29d35a9dd405c599a045b1a1b5a379950d3ad14d2a83bea439ebc93346260b3cdb45bb58110d82b58a96aea807f" - }, - { - "block_id": "000f4352aea75e8aeaf5d5c4ded454d7ce3a47cd", - "extensions": [], - "previous": "000f4351f4d7b504ef8633021fe02b09d1591172", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:25:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f3c63279a3a69463fec0146da35347eea5debcab5301dcab7b7e4b6212e4fd0e1752b1bb7cd0903b7380ef62778fdd3ac63019e8f71ab35fd920ad726c1b9c900" - }, - { - "block_id": "000f435393b323e3aff5ee7bb69e989dbb9c1734", - "extensions": [], - "previous": "000f4352aea75e8aeaf5d5c4ded454d7ce3a47cd", - "signing_key": "STM89KiUX8J2QJJcU3EP49LPjsEzQhkNgjQHwhjteitPcH4XBRNaJ", - "timestamp": "2016-04-29T04:25:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steem-id", - "witness_signature": "1f35effe0fe12f416ea7a327a0f4c7ade97a3b24688274d7ae934cc07add9394c051f93f7e1d563f778535575d4ba35a8873da9470f9e181fda921052961b37afb" - }, - { - "block_id": "000f43544f26957d657a9cd5f0e9f857c30ccbd4", - "extensions": [], - "previous": "000f435393b323e3aff5ee7bb69e989dbb9c1734", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:25:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "204ec896fb128892b1d6ecf7ed0979c11159a448549346b1faa9f0fc3a27d9ebe3127b14855140a19965d361598d2611818925403cc967ebf55d303f9c9726197d" - }, - { - "block_id": "000f43552eff9fec8a188bc479649b1d4f7e483f", - "extensions": [], - "previous": "000f43544f26957d657a9cd5f0e9f857c30ccbd4", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:25:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "20695894b9777c0eda8a86135d2838dc202edebdbcf52163f7f6063bf193690ece3e14f2a4a6c7aec620d18404fabe8f52055b55c3e7ebc8b5562dfd11ceb1e3e2" - }, - { - "block_id": "000f435618ecfdf748906ac51c249c8c69e0e9f3", - "extensions": [], - "previous": "000f43552eff9fec8a188bc479649b1d4f7e483f", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:25:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f3b8e12e1f3d72df9dabb3c6bb0f010d7242d25af8b613bfea8e08a5eb844375d29ba453b44cad5fd9cb88d2fb86df0b8ad466bec662293bec7f7c4dac5649b82" - }, - { - "block_id": "000f43577afd30eecd7c04c4e97cf42c4bc61547", - "extensions": [], - "previous": "000f435618ecfdf748906ac51c249c8c69e0e9f3", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:26:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f23577322f1f822f95b78ae7c1805b6a1c3969eacf38f6bee48c26cee8fd780701cf47365a62195c271805f998003df683ee0355064795e50a4f117ff16bc2d86" - }, - { - "block_id": "000f43585fcc4bef523887e83b7108430c873c86", - "extensions": [], - "previous": "000f43577afd30eecd7c04c4e97cf42c4bc61547", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:26:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "203f8f1233d309cac324da130840726111c339c01b504844d23d1f23828845b03f22008943e25d81ecccfa628ed96b551fc1f0d4d2aeefca0ca3cca7569806bed4" - }, - { - "block_id": "000f4359b56e972674dc463a1d52431dcf461800", - "extensions": [], - "previous": "000f43585fcc4bef523887e83b7108430c873c86", - "signing_key": "STM4yQG8BiozEtiBErWJG9MhGipmkAT6Bp7cWDnwSZkyUnMciVUyt", - "timestamp": "2016-04-29T04:26:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr104", - "witness_signature": "1f10230330e51f674ff1ebd6f7ba5f998bdc06df8070fc05f0a875df701a59a9e444e1d5ab3c29df25688ad92115fcd519d22742bfb43acf9a10ca973085adb8bb" - }, - { - "block_id": "000f435a4e5a20f8fca3b7e67770c9d9dbe84a9c", - "extensions": [], - "previous": "000f4359b56e972674dc463a1d52431dcf461800", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:26:09", - "transaction_ids": [ - "7220f5be2c6028a9219c8bda0af4335e7c00d6a3" - ], - "transaction_merkle_root": "a48b7306a3c2b7b89ca119df8c9b232ba8c093c8", - "transactions": [ - { - "expiration": "2016-04-29T05:26:06", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f4359b56e972674dc463a1d52431dcf461800", - "nonce": "4844898314579508458", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "d86613563e45e3dc9bb2a50a32415704521fb86345bf3b877a20d549614e5ab7", - "signature": "1fde0990925a5d1ec8338eb936d475d16cfbe3d9e850cfe22563124ad8308dc0d26b23798ad298e88991733d7553eb9a12ce08b8fb62dd7d064783b2d9c97d085e", - "work": "0000000a5d2c16b1ef43632bd68d908f797745d8b8dc9b9d0defb7b5b7ab89a5", - "worker": "STM72ZRPaBjxtrEyNx7TsXvFp36FHV49M2qWE4tuSCUBxGnYBUeBZ" - }, - "worker_account": "chaosakos2" - } - } - ], - "ref_block_num": 17241, - "ref_block_prefix": 647458485, - "signatures": [] - } - ], - "witness": "datasecuritynode", - "witness_signature": "200daf5f5cb175efccfa84eb048e4ac6d4e8ef193d9f61debc570c4cfed7ae714574e22fdcc7ba36df9548e60b3c0eef17ff29a386d72d7765512432ee87e36726" - }, - { - "block_id": "000f435bd398931a9b258a2e5703bd82024561c0", - "extensions": [], - "previous": "000f435a4e5a20f8fca3b7e67770c9d9dbe84a9c", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:26:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "2055afd1b7f37922413b3537545341ccb3e067d4d15d8fb98c9974599dba13298d070bef018b8fc3a302bfb043c80e1ed95f4850d036e4503e5e2b80b59d8cd7f8" - }, - { - "block_id": "000f435c035b3b465abe27cc5bfc1070d92b5a4e", - "extensions": [], - "previous": "000f435bd398931a9b258a2e5703bd82024561c0", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:26:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "202588c1eb1c8248b2c67fa71e146b6f7d2d98fb9da4fbd7141c092e692fd5c850238c34694e45e082bc515f5588a766898a6df87be67b42f8c8dd530c5dca4775" - }, - { - "block_id": "000f435df1e6a790fd1b270b9ff98e2cc75ce159", - "extensions": [], - "previous": "000f435c035b3b465abe27cc5bfc1070d92b5a4e", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:26:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "2017094362076ed0110ce406921fdd1972b7fd4accf2f619c8c1e1750e78ba80f640a20d603343e361e7a98c29aafc63b12dad17ed0519c60e2b9aa580352a60ce" - }, - { - "block_id": "000f435ee5f87830e68cad9644819683dcbe601f", - "extensions": [], - "previous": "000f435df1e6a790fd1b270b9ff98e2cc75ce159", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:26:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "205eb3de2197827785c2b78ef468607e866ca4c5e6e073964a1dbb23cf97cae3fa0e7db190c58b7e2ca6b85090d6e19d85fd9538330204050ae20d2b28dbb78570" - }, - { - "block_id": "000f435f9a6ef355201ba8a8f302ffdfa6678fd2", - "extensions": [], - "previous": "000f435ee5f87830e68cad9644819683dcbe601f", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:26:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f3e407c54ba292d97fc519a1e22aa332590655120708116954071904ece0b5b34416b5600021a9fa9f2be0e97e42f8e4e13be9fc24ef8f9898fca2c98d97507e6" - }, - { - "block_id": "000f436065f558e0e1fe6829b49fb7c4e454478b", - "extensions": [], - "previous": "000f435f9a6ef355201ba8a8f302ffdfa6678fd2", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:26:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "205defc1c7597b7e6523c7afbb6e22b55c4d7877f90963338e1709a8946593aa71018bab118f6ee2c8b8cbd196bd74d7df53a5fc26d77408785062c6c8662b4045" - }, - { - "block_id": "000f43614a4795990578dae402db89eedadb1551", - "extensions": [], - "previous": "000f436065f558e0e1fe6829b49fb7c4e454478b", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:26:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "201f9ba0dcc9bf2479660448521327ce2f787f9ba5c247043f8405bcf88327f90f56653b3daeda36deeb4cfc180433a311ba0e8b7d7d7165438cb405010ebb6bd1" - }, - { - "block_id": "000f43627e11c482fbb169e7e90f20f881a2eace", - "extensions": [], - "previous": "000f43614a4795990578dae402db89eedadb1551", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:26:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "202c6329d9d13fd3bd6deca5165b7988d566e8bf7916fc815037e4c69580fdcb2a08358056655cb309986bfe7cb78e69f0fd83ec3556d6c20928d2728a09af1f12" - }, - { - "block_id": "000f4363c87172b4b4665b4db1081d0c7d3844b3", - "extensions": [], - "previous": "000f43627e11c482fbb169e7e90f20f881a2eace", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:26:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f47ab274a0fc25867845e5094994e656f04836086b6b839b233c64a1f74d4c6336bc53d98604c8cf636a75881ade078c4e8cde3f4f7a80d2e776ea84aef8cd0a5" - }, - { - "block_id": "000f436433c074fbce40310d990e05e012edca23", - "extensions": [], - "previous": "000f4363c87172b4b4665b4db1081d0c7d3844b3", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:26:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "203a90800dc53405f584bcb5523d360980c99449361974bfeef5c898d9db9be10e712c89f4f356cc55d23b94b08b3480cb5bdf746b2fceb4d090b5fb667960d095" - }, - { - "block_id": "000f4365256b83a1b15459064085ff030bfed0ef", - "extensions": [], - "previous": "000f436433c074fbce40310d990e05e012edca23", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:26:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "203dfc03e82face0a23498c3fc9b117e0d635b38df9381289bbc15960267996f6e19bf3eaf0fd180ea3cf0b4cc800e5398e0ca120e557977353a3de31a1334a172" - }, - { - "block_id": "000f436636b6f72817194083d59721ab3f6e2ea3", - "extensions": [], - "previous": "000f4365256b83a1b15459064085ff030bfed0ef", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:26:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "203971886eb03a0d328c2c52a92629bf265c1040516df0f482fa071e902b310bf448d6abd072c985a09bb32f54beb33d952b15d92c197e99a5ccdd793727a8674d" - }, - { - "block_id": "000f4367a7afe7048678cb73837d01cfcb7a31f4", - "extensions": [], - "previous": "000f436636b6f72817194083d59721ab3f6e2ea3", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:26:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f4c4359a4773a039544fdc51e812120e7fcadc0ba3b1b6f8dd3443474bc6f89082c1cecb16ed00b1202fe17a8cc06358f0aefb37ac95e03ef85d107cf256563c1" - }, - { - "block_id": "000f4368a44774d2e3a274d7365c32b695eea561", - "extensions": [], - "previous": "000f4367a7afe7048678cb73837d01cfcb7a31f4", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:26:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "203d988133c1f3b2279a3129963cbd1dd5d571aa989408bb8250b948562ae9b9020221f47d35733a31e39552c4983863686dde62eb2bb2816f7d62acb1bc7c3cd2" - }, - { - "block_id": "000f4369569dbd9563e9605c21df939f0c1ac775", - "extensions": [], - "previous": "000f4368a44774d2e3a274d7365c32b695eea561", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:26:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "2011950e1e1712db2db33ddee04a634635a69103f50181eb26b5443b52b198131249fe4c055b578e9401bbc470e8affcfeced2aea0518e071351cca39cf675253d" - }, - { - "block_id": "000f436a2cd78035ae77324f603fb2b76ad3a53e", - "extensions": [], - "previous": "000f4369569dbd9563e9605c21df939f0c1ac775", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:26:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f14cec8bff043dcf685d47ad6dd10ca0df6aec307a4746d9703f2ae322e178f5a34bce690f54e5400131a8f65bebc4621bd34c4f307840cdc1ac74dfe9af51205" - }, - { - "block_id": "000f436b83e536d64c8849d4c0118188fdef1d92", - "extensions": [], - "previous": "000f436a2cd78035ae77324f603fb2b76ad3a53e", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:27:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "2026c79457df29aa96816c828abca2d12be93f7585463119f9ef0da3c95c44ec34541260ee25ede39a736e01ab176debfe4214ccaf79fd58c39d104d178292138c" - }, - { - "block_id": "000f436c378bb723648a71e7869b2790b356e186", - "extensions": [], - "previous": "000f436b83e536d64c8849d4c0118188fdef1d92", - "signing_key": "STM7P7TMeUofSum3cdUFfEZc3e78axUMcHrcSLtMu1j4BPW7sVxuZ", - "timestamp": "2016-04-29T04:27:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "masteryoda", - "witness_signature": "20325bc066926c5bed3d3a766264d78fb30d70d181b276453bda241b9655be947d3369f7385bd14c7ef8ecd67fda1e50bae181d8b3e617402d3d52b601e66936aa" - }, - { - "block_id": "000f436dc5482ce351b4aa11388bd71aaa016428", - "extensions": [], - "previous": "000f436c378bb723648a71e7869b2790b356e186", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:27:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f23365d66943d2638b2eaa3bac9094f8f8039bb71997dbd651a1a255accfc92a672c30d0d14795fa647150cbeee500e5c4b6200f42566f9092edd556c7ce30b5f" - }, - { - "block_id": "000f436e3e75202973139bbc4061cf73fdeebcd1", - "extensions": [], - "previous": "000f436dc5482ce351b4aa11388bd71aaa016428", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:27:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f2bb421135df8f5705322d53de288a46461419a0ac29b157b48b0f83038a60ffe60c770b730b83dab63bfe5a8ddb2130f21beb0edac3336f60a4c42930f138686" - }, - { - "block_id": "000f436f7bcc29ea549e40bdb9b090688ed9851c", - "extensions": [], - "previous": "000f436e3e75202973139bbc4061cf73fdeebcd1", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:27:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f3e6409653d625c992dfb5784bac04989513e7a9f12a66a1ee1c3e1100e23a33309f1d01c8df1b671e3ddd734fedca470eed9b931f5a363c6e1c1bd2dd815944c" - }, - { - "block_id": "000f437001027600fa56c63b14df797a8530a8fe", - "extensions": [], - "previous": "000f436f7bcc29ea549e40bdb9b090688ed9851c", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:27:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "202821a9241929d2af2651a7d804708bfb8d9aa90895cbf16ee4a0b80ca785853a5ae7bdcd6652269c5c7668cc789a3e15df8c105fb79881fafaa10eaa5c2a42c9" - }, - { - "block_id": "000f437137485f201ac4f22b6e64280fca1386f6", - "extensions": [], - "previous": "000f437001027600fa56c63b14df797a8530a8fe", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:27:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f71c9c4ffc6af04cf4f569ca1da37040ac243b28b92922eaa5e3c55586b75dbb9343eff7562b0185f59ad7aa727727582665918e1a1a16ae048559e18a6ea2b92" - }, - { - "block_id": "000f4372c35aece3ba16f02188e706d847600625", - "extensions": [], - "previous": "000f437137485f201ac4f22b6e64280fca1386f6", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:27:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f288a2a7626f11719cd8f6f0d753199fa76da2a6d1ccce14899108904a063137a2dfe9f217d2bed9e46755653fc2babdcf32841648ed2cbcbe0f6bd207de45954" - }, - { - "block_id": "000f4373d9ec10f67c9cd1f853a4fc9ea7d5588e", - "extensions": [], - "previous": "000f4372c35aece3ba16f02188e706d847600625", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:27:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "200a084866ef974d3ef8ed671b6d3cd69d1d44edb3dfa4850366d5800faab9272f77ec039560f505d39fef1e2c13194832905168d4f334c1571b65607eeb96d525" - }, - { - "block_id": "000f437474cdacc0cc1e8fc8f1982c462af5c9dc", - "extensions": [], - "previous": "000f4373d9ec10f67c9cd1f853a4fc9ea7d5588e", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:27:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2050d138ff8c8598abb2ef8e9d2287b3b1adf7aec3863afe3e5b17f3c1312e58b3079d158dc6ae98cf2bf2de5d376460f19b577d773477b453cff83bcc2110eaf8" - }, - { - "block_id": "000f43755de975e851fe8bc72ac4343941fac9ee", - "extensions": [], - "previous": "000f437474cdacc0cc1e8fc8f1982c462af5c9dc", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:27:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f5a452e26a5b2a01951f0f93286700f27058283f776041c2c07a58f070ced3bc32a7d6d3ac9b715b76bf5e7f36337c7452a86e143bc0af1f4545a173766c52c29" - }, - { - "block_id": "000f437659ce97fe4d72ce7e742945525bb3bedc", - "extensions": [], - "previous": "000f43755de975e851fe8bc72ac4343941fac9ee", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:27:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "203b75aefdcad8e65e56195ad5729b3fd64e807be936cd889980642da64cd40bbf3f02b7e4923d43ddb1707f97d98c2cdb49b0b01cfaf9e07a4acb7ff401a0e4cf" - }, - { - "block_id": "000f43773f9fb62c6f62f4465bccd5eb2fda0f4b", - "extensions": [], - "previous": "000f437659ce97fe4d72ce7e742945525bb3bedc", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:27:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "206c7280c02783ddb941604fe5ee162986d72630004531a4a6dcd2e6436f256b5f5353d2a90f84cdaebf767b1a326c6d837f536eefc139485bd1e010b3ead84605" - }, - { - "block_id": "000f437852f833c130a95e5018a7e887c4d18b0b", - "extensions": [], - "previous": "000f43773f9fb62c6f62f4465bccd5eb2fda0f4b", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:27:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "202401208e734748b99163dc1ab97204aa4cbd2467e3eaab50a7f852af091d26802cfad35177758452937015eb9b6ee4766cdc8c2d2170ea02c0c3753f23e7eccb" - }, - { - "block_id": "000f43792cec2c38eebd92de684ba1ed03cf6069", - "extensions": [], - "previous": "000f437852f833c130a95e5018a7e887c4d18b0b", - "signing_key": "STM6R5B5uF4hdUvFJFtNJbHd4CHmpgSFN3EA7yzaM9uF7HeNPwRN4", - "timestamp": "2016-04-29T04:27:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr601", - "witness_signature": "1f4eb4bf6bdd504912c0b129ee866ab6c7bf25e1ebc51d595611c7ca8e03d0eec47d2ce906d4e5d9e1197ac486f1b84d5d9fb0d10e173e81bea390494f13da3f98" - }, - { - "block_id": "000f437a23781f8ef8d00d8848557ab6674c14ac", - "extensions": [], - "previous": "000f43792cec2c38eebd92de684ba1ed03cf6069", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:27:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f41112f64b5408be1ee9dc6bf347c081f337e9f1ac51fc5f0d419ae4153888626103caf54f5283677eeaa055872748090ddb5efb06cb109afb1b4cd2fa8fbf5db" - }, - { - "block_id": "000f437bbd622b4417cb18bb7e46dad1b35c28f2", - "extensions": [], - "previous": "000f437a23781f8ef8d00d8848557ab6674c14ac", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:27:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "20494cfb00cb4a10deabb9a962ed7cecbcd59e7662a2981b81c128c98daf671f505814723ed7aaf342e0273780aea3eb83c9c1ae198722e9b4fc071f8ee7f99fdb" - }, - { - "block_id": "000f437c23e74df4cd4c3042a0bb9e8d062bfc00", - "extensions": [], - "previous": "000f437bbd622b4417cb18bb7e46dad1b35c28f2", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:27:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "20725debb448ff769c87408e7a8f7adbecc92df8311d9e53ca15186be127542485479735aa88bce1da2662b103cbbb1c164bcf6ef815c061ea8ae21a2afc2fae1d" - }, - { - "block_id": "000f437d8a5c25cc3f9cdf4d514b6fff648fec57", - "extensions": [], - "previous": "000f437c23e74df4cd4c3042a0bb9e8d062bfc00", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:27:54", - "transaction_ids": [ - "ebf98725e80e80131eb36237f56fc5eb2be08b8a" - ], - "transaction_merkle_root": "be17067237ce7644bd3669f68fa92f46455e7d01", - "transactions": [ - { - "expiration": "2016-04-29T04:28:21", - "extensions": [], - "operations": [ - { - "type": "account_witness_proxy_operation", - "value": { - "account": "smooth-m00", - "proxy": "smooth.witness" - } - } - ], - "ref_block_num": 17276, - "ref_block_prefix": 4098746147, - "signatures": [ - "201d72e02c56e7845ab9c622a5c806e69100f2c433f5d158deda3d6284b141dfd47abfa78e5ed87884c562dec73b816d0d3a493f2098d3d0ecabd106a252896a07" - ] - } - ], - "witness": "pharesim", - "witness_signature": "1f43d917acdfad6d1e0356b586043016fcfd12db1503d9f3b5b5ef53bd3e310fbf2185dbe72d4c3fc2e501139c5498fa2577cf52c1319f213498ad4bee07d0b1bd" - }, - { - "block_id": "000f437eef0208617e86a5a0cebe49b83cb1d9ea", - "extensions": [], - "previous": "000f437d8a5c25cc3f9cdf4d514b6fff648fec57", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:27:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "202dc86f170368850eff8d30930b70c77c1b6c2f3b6d5594e5c1068a86d82859de0c75dad861e5c3ab059856f2e54e9cf4b99072f21a7b13bcce5c1abf8a926545" - }, - { - "block_id": "000f437f821a59b10be8c534387d5350bf01682a", - "extensions": [], - "previous": "000f437eef0208617e86a5a0cebe49b83cb1d9ea", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:28:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "202f73b1be5ff9e3561e8d4427f1cf6fa0fe8b2528c61ad8fe6dfa42e79581d2c13db8ef729aa094b0f80d9b8e67188ed16873be252659e74c1fabb6586775ece8" - }, - { - "block_id": "000f4380b887c1a4d1a5345e67990e487740a0d0", - "extensions": [], - "previous": "000f437f821a59b10be8c534387d5350bf01682a", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:28:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f47a864768e56f5509cd1e1ff2d0bc7f1b557f75342a14971f64cc2350774dd9e0642cac419ab440a78949e0c8e898732e66ee7fab9c5a3c48db1d964a197d471" - }, - { - "block_id": "000f438186875ce8adabc09a567347eb4185d742", - "extensions": [], - "previous": "000f4380b887c1a4d1a5345e67990e487740a0d0", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:28:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f010b786de90b00da99b076e8d92b72b60fa1ae0523d87e22250c62c93a76436f405bae269df56b043fd717a48a699673cb1143e714092d2069ced0cd05d64a21" - }, - { - "block_id": "000f4382e5c0d05b3ddd44b7f716820ac27cf1ca", - "extensions": [], - "previous": "000f438186875ce8adabc09a567347eb4185d742", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:28:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "20116847cc733b7f64ded03b30b33cdeda83dec4f7ff088c4bb1a3890a87095d9b24c6843a609a700e75a4ef2bdb9073d072788fd86333509158df4d5d2186ad01" - }, - { - "block_id": "000f4383b32e3459f6cac73b188e82bba9ae6070", - "extensions": [], - "previous": "000f4382e5c0d05b3ddd44b7f716820ac27cf1ca", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:28:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "203af471fc9bf72b03573e129a38cae1ca72a576a3cb89d8e5b756fbfc35c1c2976fdb84f2cee1f3d9d8e45b24c14e452c24802acdc9eeed13b9de1db68b4c42cd" - }, - { - "block_id": "000f4384f9fbac15b9f17655b78d69f60f468fe8", - "extensions": [], - "previous": "000f4383b32e3459f6cac73b188e82bba9ae6070", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:28:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f7b9befd5ddccc069347f166e8e2a482f7feff8e7be5ac4a43a53a085d704753d2a9fea778e2d342a38ecd36ae9437a341c53b213072190e976cb9cc2c47f772c" - }, - { - "block_id": "000f438551d4c3d951024b73bc9bae5bf77084b4", - "extensions": [], - "previous": "000f4384f9fbac15b9f17655b78d69f60f468fe8", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:28:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f6f9f27d237ddc784586f429614e37b9bec2168815078ae5d56c9555126ce8d451e57f07e6b2deaae3ec65740112764e749340405218e48c491ce060062add2a2" - }, - { - "block_id": "000f438675f2cf87b71587acc7f04ffeecfe77a2", - "extensions": [], - "previous": "000f438551d4c3d951024b73bc9bae5bf77084b4", - "signing_key": "STM7q6ZRH8giNQSMD9EM1z6cUbSgcAfE1HmRrwF1T78zsvpAAN2hv", - "timestamp": "2016-04-29T04:28:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "copychicken1", - "witness_signature": "2046cc39ba3ce1736adbeccca119edd175e42adca363be83ad8abe43901501ada640e0e84ac620a2638592a575c13d7c534c2d353166c7fb75506f6be41b3528a8" - }, - { - "block_id": "000f4387e5f49cf4ac4b93a56b1e2330b1cd702a", - "extensions": [], - "previous": "000f438675f2cf87b71587acc7f04ffeecfe77a2", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:28:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f6c352dbe3c20663b420861cf1de76d2f44aeef691a3aeb61be7d2b7dd7dd517264e280608be602bbd9df4eab5c2a670e5a0a763bcde044af1e717452dee30c10" - }, - { - "block_id": "000f4388cd128ae1dcce071ea361ccc2cbb1bef5", - "extensions": [], - "previous": "000f4387e5f49cf4ac4b93a56b1e2330b1cd702a", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:28:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f64113200a22140997e0eb04879d6c74da56e443f3475336369a0363697164a9b61bc572484bae85b25524b1479bfc5ba37212cd8e9e3aa66b92952d169850e1c" - }, - { - "block_id": "000f4389f4a2a87802577433a07dcc7682f79685", - "extensions": [], - "previous": "000f4388cd128ae1dcce071ea361ccc2cbb1bef5", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:28:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f05c3aab1d9b4397d5546122c7717fd88922b31dc39958c1e7c2a14b829f3769337cc43ee9d13e719a03cefe8b19f224f2cafd61e423f63ff99186ee22a19806d" - }, - { - "block_id": "000f438a8cf531d0b3d624be4bd98ba1906c234e", - "extensions": [], - "previous": "000f4389f4a2a87802577433a07dcc7682f79685", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:28:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "2020aa70475021977f3d6b2e7eb2728b703e9c7142c1d70b6d2f6812a4ff1afee6319edc744aa102e42f9b83f5538e4a6bdcfda73d150bf035250de817808961a3" - }, - { - "block_id": "000f438b11e8f2d95a8aeaa2b9608afe9810b39d", - "extensions": [], - "previous": "000f438a8cf531d0b3d624be4bd98ba1906c234e", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:28:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "2057c078db28cc43f1238dad178caafae82547f018ceda21717104ddabe2dfc73f1cdc89904e9128f876c6d9f2a25a4937078f36ad37c742c2f99cf5c6dd69e511" - }, - { - "block_id": "000f438c00bac2b05b17da593349153913925833", - "extensions": [], - "previous": "000f438b11e8f2d95a8aeaa2b9608afe9810b39d", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:28:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "2044c77b5924691dd6e5b96418ddefd73de91da2f5323e22b9c587ef9c8ce0f32b52b16609292f6a14f161500119ce739d3508ccc4590bb170124a78120b4bb8d9" - }, - { - "block_id": "000f438d2b309ac13b6049f771c5735dde416101", - "extensions": [], - "previous": "000f438c00bac2b05b17da593349153913925833", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:28:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "20067fa8f9e3005e54c364e9cba9ee29986f0a72845d85170aa38b14b990d7e12f60e02848974c23972d896afa0e21ac593224cdf34dfda14616e5899c19858a60" - }, - { - "block_id": "000f438ebe44993fdc1f485788b9b60ebc752829", - "extensions": [], - "previous": "000f438d2b309ac13b6049f771c5735dde416101", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:28:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "204a58eaa9138e4e09a1d40b31a19f681886c19ff44ce75338f46cdb9fe9422445237c221e983653aab2081ff77b7bc476f418553ee7b5835fd50d3321c658bdeb" - }, - { - "block_id": "000f438fdf236ce061369613929bf89e143da090", - "extensions": [], - "previous": "000f438ebe44993fdc1f485788b9b60ebc752829", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:28:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f3376a4c1299a3b1a89edf280a31fd36d9bd60f4a9f40f6d27c8fc1cc31d81eab7feb9772a7cda8924bfc56b7da0c6863620e267a4bcb9b415a52e19d5b0a8a7a" - }, - { - "block_id": "000f439020affc984551ec1b6169845926ed2a17", - "extensions": [], - "previous": "000f438fdf236ce061369613929bf89e143da090", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:28:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "205360f7eae8d90a41dfa1f2051048f8cc15b4b9c9bb4afa215541cb5e209164a978020fc6697ca8970cb0fabd83af3c8114c2cfae2581eda559813a7c5e79b323" - }, - { - "block_id": "000f4391179087865acbe16fca3ab13c605d5275", - "extensions": [], - "previous": "000f439020affc984551ec1b6169845926ed2a17", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:28:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "20783a30090fe4d6a82f5a1b138ddc0913f6a48e066fb269a762f21e2a0b24b73267e68187908857da400523c6cbd2edee3fdd611cd48576cef162d78c6c76fc11" - }, - { - "block_id": "000f43922bf2abbbc65fe65759934d0ada9ad176", - "extensions": [], - "previous": "000f4391179087865acbe16fca3ab13c605d5275", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:29:00", - "transaction_ids": [ - "a537037f3d966bb539b312aa1b07b3808316d24c" - ], - "transaction_merkle_root": "eb522a6b635cb8b3d323bf48b0b9a0ea76e87c41", - "transactions": [ - { - "expiration": "2016-04-29T05:28:57", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f4391179087865acbe16fca3ab13c605d5275", - "nonce": "15658925604317528114", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "0014b167a673db389c9b18a67283f321fd1d048b1a53ee03b25bf72558b655ff", - "signature": "20d4ea2f2f27a5a17d6d366cedbb62879648938b6fa3a0dceab2964b7f56a190605e2c8ce3ebd253c33e7cc05f7efc77e3cc297734a2f6e418a869a121bad749b1", - "work": "0000001bfe947371a7f0f1f42819a81f5f01d161eb085157d3af8bb94e549b54", - "worker": "STM7R1ppiYYPozzpRPgZnCUUaCsLXF78HV2ujXALePfgwhN8cfFP4" - }, - "worker_account": "bmw" - } - } - ], - "ref_block_num": 17297, - "ref_block_prefix": 2257031191, - "signatures": [] - } - ], - "witness": "silversteem", - "witness_signature": "1f697c84860c868c989eec93083e53b1095431fe066433a2534e0388b55f78b7ae714eaf3fe638600bf756492db24445a0265edb18b4759fa66964042ca2c37587" - }, - { - "block_id": "000f43930ac60fef36a5ddbffd22a8f3af21a299", - "extensions": [], - "previous": "000f43922bf2abbbc65fe65759934d0ada9ad176", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:29:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f23b6e90628bc99f2ab958597146f93ff70d816a354cfa266cf9a07af912af7b006fae142558a0a0afab991e1dc6c9511fd1606a4298efbfd57fa622d0f73a765" - }, - { - "block_id": "000f43949674d60391d31120fd4fa0d75a881f18", - "extensions": [], - "previous": "000f43930ac60fef36a5ddbffd22a8f3af21a299", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:29:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f647b38bce4391620db5c138c1871c34979bb49b68782b40f9090c29552d581536a0b34971c09f622f8441e423b056482ca24bd7ffa0dc97936d5f2ff49ab3861" - }, - { - "block_id": "000f4395ba9054b4b6cd363f70d668200f24636b", - "extensions": [], - "previous": "000f43949674d60391d31120fd4fa0d75a881f18", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:29:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f141a04705548aab2ed59df8f3d32c639bf485fa2f38f12b0269aabfacb0300d81ea78c75a65b80315993134956799a5b1c3af8e41fe81891f805f0bbb86c94c8" - }, - { - "block_id": "000f4396e0fddf6365469e7f2fe3a75cad28e740", - "extensions": [], - "previous": "000f4395ba9054b4b6cd363f70d668200f24636b", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:29:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "205a749797c2149a9fa8ebe9fabe488b5b2bc196db28b0443c77f15e2641b2f46f224e61c4310f28d0790c610e1ae81f4a748df5af39f71e508d944cf186ad6db6" - }, - { - "block_id": "000f43974f0f1e2556885300189f29377a089e2c", - "extensions": [], - "previous": "000f4396e0fddf6365469e7f2fe3a75cad28e740", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:29:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "203b0ea7fae7a376e0cec8014a474c5450477d0331671b4d4d6cffda34dcaec09a296afa055feb04b4db6bc5f63ce3d47e01b03d6adf329d344a876683561ac9cd" - }, - { - "block_id": "000f43980f2f370043cc6d2acb5a97782586bf31", - "extensions": [], - "previous": "000f43974f0f1e2556885300189f29377a089e2c", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:29:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "204b486db5833743ea6760d519d6e18b206afd502ca2001d18861469d4f74674474ded7d9ff75f7e8852f973aaf492559e576050f8ae57b62a84340936bc199972" - }, - { - "block_id": "000f4399935b27e23b8d9bfcaae1046f90691fae", - "extensions": [], - "previous": "000f43980f2f370043cc6d2acb5a97782586bf31", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:29:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f743018a8041a34e3901eba2fdaa8891f3f26140fbcdd3f49b32741b42291e20c3d1d0196b5256037b2a91206b8db73e2b53e8b74c8243b9186f33d8b753f52bf" - }, - { - "block_id": "000f439ad43695ad6e2f3ae39ce501925d487cee", - "extensions": [], - "previous": "000f4399935b27e23b8d9bfcaae1046f90691fae", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:29:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "2009b3d93e6ca695ed970c717003c6dcd575238867c7c1a1beca52428ca257567f03d710fc4ee75bd6d9cb269e1627b9525acff38208ca50e45da50c40a37eae14" - }, - { - "block_id": "000f439ba2805db5b0a70758d188e642651335dd", - "extensions": [], - "previous": "000f439ad43695ad6e2f3ae39ce501925d487cee", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:29:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "204c9130d2075b350d86aad7c68175b6d14ad615b84bfd3cb2b44c0c20f8ecb71207a8ec0a9d70d43feb84133bbbd917ca0340f3ef580eebce99e27b8b9b05dcc3" - }, - { - "block_id": "000f439c471820750db6fd4f1fad3d27a845b366", - "extensions": [], - "previous": "000f439ba2805db5b0a70758d188e642651335dd", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:29:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "207c71c9c1bd8991f1f8c25c0018dff7ce9d7268ab615743c352f62efb3fb623201960663796d4a8307ed3e9a1b50676d380af6327648dd3af2d5a7fa8fcb5984f" - }, - { - "block_id": "000f439db467ad4694dec374467c27df26198ec8", - "extensions": [], - "previous": "000f439c471820750db6fd4f1fad3d27a845b366", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:29:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "204f3750ed496c702fe80004fab5ab18e1a1b930198f87b567bd47ca9386a537014ca37e334bc06c16fdb258327cdee382512c8e05ba2eef2f748188e0be4165b2" - }, - { - "block_id": "000f439e36405b5eea1ddb26127c251504e06ada", - "extensions": [], - "previous": "000f439db467ad4694dec374467c27df26198ec8", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:29:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f777e9cb6eba90564bb241e453ee90d9e49db9de6c8e294cb0caf5456203b84b3029e0cc1c89d39c893a7f28d59f5f074e55de24a07a26e89ba501a3f4a2b003c" - }, - { - "block_id": "000f439f2d2f572ad8d76a343bcdc153bea6ed09", - "extensions": [], - "previous": "000f439e36405b5eea1ddb26127c251504e06ada", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:29:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f23bfedad38565d3dd004d82af834252127bd7f922eeaf8e2b78bfc9e9c8497d154ff93ebefaea1ac76395c4429076bfe80ebaa046360e3a4508f53fa34d24b27" - }, - { - "block_id": "000f43a09d54331aa9fdc2f44f628428b94f04bd", - "extensions": [], - "previous": "000f439f2d2f572ad8d76a343bcdc153bea6ed09", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:29:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "20277afc3b06b84eac54f1909e88d3644701a3b0994ba42b7c66a4ddd29b2f2964797fd41f8ac5c71aa4ff856de163002ec65604663ea6150d5f785da819bc8219" - }, - { - "block_id": "000f43a1e70c91329b09a08952e213a7b98e0926", - "extensions": [], - "previous": "000f43a09d54331aa9fdc2f44f628428b94f04bd", - "signing_key": "STM5742TmF2wyuStKtgETi1KDycZ56NNvgJhAt6xWA3PA1Rx61FvU", - "timestamp": "2016-04-29T04:29:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "cyrano.witness", - "witness_signature": "204473f9a6cea6b33680f81149474fd662ba5605144a1f08fd09a430c03fed067c72214d3a875aea9c59f020d064649edcdd247bcfca6ffa3d82840280f1ba344b" - }, - { - "block_id": "000f43a221655de9ec9172d8edf29adf387bebe2", - "extensions": [], - "previous": "000f43a1e70c91329b09a08952e213a7b98e0926", - "signing_key": "STM8io6LPUoB89b9iwBh1qoN9nKQSmigbq97VvufoSWhqZkc12nLW", - "timestamp": "2016-04-29T04:29:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bitcoin2016", - "witness_signature": "205e4a507a68da7e0f8ec9cfbf9bf70f8c1d746b0498a89c3a9f4d79025879d7b2789de325874f38ef1430814cce191bb4a4f72f3312e74931d8483fb8d385afbb" - }, - { - "block_id": "000f43a39118c1b2f5a4c0b9339aeaece3de9913", - "extensions": [], - "previous": "000f43a221655de9ec9172d8edf29adf387bebe2", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:29:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f07a4a49f3abeca58b8f0de57899e357119264d689a25c75d6d8cde4dff93fa4e22c1ff84767c93af1bb21e5d6326bf751cced171947341f99a3142f35529f826" - }, - { - "block_id": "000f43a48103ce5c6b7eacec782d77043905500b", - "extensions": [], - "previous": "000f43a39118c1b2f5a4c0b9339aeaece3de9913", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:29:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "20624fc1bedfcbc346b58224ff3dfbf3cf75fb1f38883a598e5eed67137b7782df5ead5517a396ba2428fdb65920f1be6a51bf6e6a0be6c880fb3c9887f1fcc393" - }, - { - "block_id": "000f43a5d54286ab258360d4d52dde33854f616e", - "extensions": [], - "previous": "000f43a48103ce5c6b7eacec782d77043905500b", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:29:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "200fb8273ec6d21c300c23fbda11086fbc8c1042084fe8b904fbce5f1d2d65eac3143e5f260300f304037c9dc0768a1caa8863cf451adb9077afac6d63193f0619" - }, - { - "block_id": "000f43a6aa459fcc8d9dc487af122ab3dd7a4977", - "extensions": [], - "previous": "000f43a5d54286ab258360d4d52dde33854f616e", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:30:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "2061df9c6bc054cbf746a6af9dc8670585ca61ac6117a270d02e71e73f90463fb37a3ecd1defd291b95ced2ef418c4e989fb86cde9099b48fdfcc991fe145c93c6" - }, - { - "block_id": "000f43a71197499748624ceebf0dfa46cc031c16", - "extensions": [], - "previous": "000f43a6aa459fcc8d9dc487af122ab3dd7a4977", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:30:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f15245d46cfaa1447ebe071914948ec17f6ddd9947141e72f182e85dcdaf8219e3ecddbd1b0aa7384be22ba46ceb2dbe9fb5ead63b5589c34279fee48b820ff59" - }, - { - "block_id": "000f43a8a9890b0c52424ce9720940c903bb17df", - "extensions": [], - "previous": "000f43a71197499748624ceebf0dfa46cc031c16", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:30:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "20517252eece56d0de582421d399786b99f18c28b702f4e1753154341711f4acd445d71e837db7ce16f37782677f40942511e0c65db331f03c8569ac753572cb19" - }, - { - "block_id": "000f43a9508d9c19c966080a84c86103e8914f08", - "extensions": [], - "previous": "000f43a8a9890b0c52424ce9720940c903bb17df", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:30:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f1588025f2c3cdcaa76e7c8fd7dc00a367aeef62dd8d582a17887f5c65568a90434b8c6219878e12cff61529d835d6673d911b63af882e60be0967ab8305fbc4e" - }, - { - "block_id": "000f43aa6db92fad69691a2af5849cb199185965", - "extensions": [], - "previous": "000f43a9508d9c19c966080a84c86103e8914f08", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:30:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "207ab27e2cdeb11c3f77c5488585f4413bf02bb24a7d9476c0783d5a73fc15e9940ac42fd880e9778fd9921ddba96b062e2031ae1856c60bc7f2c7d0230b01a35d" - }, - { - "block_id": "000f43ab5fd6134a8bdf10225cde1f5338b8df27", - "extensions": [], - "previous": "000f43aa6db92fad69691a2af5849cb199185965", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:30:15", - "transaction_ids": [ - "8b0ce090e4ce097743dd6ca0debb5822ba9b5e35" - ], - "transaction_merkle_root": "9719e0b008388fd9ee5a63d56c17bd90362f56ef", - "transactions": [ - { - "expiration": "2016-04-29T04:30:42", - "extensions": [], - "operations": [ - { - "type": "account_witness_vote_operation", - "value": { - "account": "clayop", - "approve": true, - "witness": "joseph" - } - } - ], - "ref_block_num": 17322, - "ref_block_prefix": 2905586029, - "signatures": [ - "1f2122c2237f0fda817cb9c6173fb002d8666a6e4ef9cbb923df1f4cb3cd27b841591a27433dac44a47979ec53400c874c85e1cd931acdf398a0f811083fe12694" - ] - } - ], - "witness": "abit", - "witness_signature": "207dd5ea58af42a25206921ca276f8ce197e419292e68c1824ad0238117a8944030b3d354887866587367afcf13a9e537af0ddcca99ec1ac239a409685cdcbcab7" - }, - { - "block_id": "000f43ac972f0543a2b6310ae7aafc48b1d8f882", - "extensions": [], - "previous": "000f43ab5fd6134a8bdf10225cde1f5338b8df27", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:30:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f2c221f27c88a82759359632ff3a1156ab7170361da9da241c973a732d6c8ed821fddd753e1f03649c110e94e525078f0f4f177b59f357427c4d07f65e67136af" - }, - { - "block_id": "000f43adb4ac6350aa0900e2adfc67b7ffab7537", - "extensions": [], - "previous": "000f43ac972f0543a2b6310ae7aafc48b1d8f882", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:30:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f17d5d816542324cc1c61da7abeb7a8be2e91c11d8ad2943ddeedc4c299111ed92c3fc0df56cb621c00ebea6d6d74843be7224e72e7701e41adf56ceede057378" - }, - { - "block_id": "000f43ae089c1dcc2e161b7dcc3b936dc8a8c09a", - "extensions": [], - "previous": "000f43adb4ac6350aa0900e2adfc67b7ffab7537", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:30:24", - "transaction_ids": [ - "d3f0d1e341b9f277ab3b0be7f950ff81476ed1ef" - ], - "transaction_merkle_root": "32aadc032da57e9622cebb5f2db4cae09a07bf97", - "transactions": [ - { - "expiration": "2016-04-29T05:30:21", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f43adb4ac6350aa0900e2adfc67b7ffab7537", - "nonce": "2752193280839846667", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "7e7b0aac4b806758bb53dd69ffa2012b9306418e119f26f85fad4e70960d4ce0", - "signature": "208f49fcc2d5bd5e41e41dd1ae5ca18abac946d5b7325c1950486dd6f6be07f49f44fa22a42d9572ff0c6c88029a8a4d917f9dac38a585962526d05beece268e35", - "work": "00000017edd3dd394aa8afa6b72ce29b51c1706b47e74275bd799dc38cec38eb", - "worker": "STM7kDvpn5r7j42kEdu4bjbUonQtES3xQiWex9nAErBvKMdFuqWNb" - }, - "worker_account": "trade" - } - } - ], - "ref_block_num": 17325, - "ref_block_prefix": 1348709556, - "signatures": [] - } - ], - "witness": "steemychicken1", - "witness_signature": "1f384abcf64577e2005b04103c338db3c618636e67943055877b6bfc7ef547569077ade9ca11695826b4534cecb683a4a96a967e13f625e9280c899c907c42e5c6" - }, - { - "block_id": "000f43af12468029b8951cd3b6a0ba9faba9992c", - "extensions": [], - "previous": "000f43ae089c1dcc2e161b7dcc3b936dc8a8c09a", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:30:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2015052140aa068172057516136bdcec5cbaac1ba7f5274aa91028bce53127012207a62792093414f9de5a70067147856b73b41daf7cc4a0050e2d110644971da6" - }, - { - "block_id": "000f43b0168915e44d7b3cfb950d318809b76a6b", - "extensions": [], - "previous": "000f43af12468029b8951cd3b6a0ba9faba9992c", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:30:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "20015141d08fc0424cf964e2425624cd8aa3a49eb59bac18af2b1a509f3841130340520acd1766e89eda5931ea5130b62de669178ff641f473ee3f1e28f5578e4a" - }, - { - "block_id": "000f43b1e53b6119f9c4c57a09071a9908eec018", - "extensions": [], - "previous": "000f43b0168915e44d7b3cfb950d318809b76a6b", - "signing_key": "STM8AnYzmrM4H8ZR19qwkXCAhcbLKK1hUjGmFLEQnx7ukawZLTSSN", - "timestamp": "2016-04-29T04:30:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bunkermining", - "witness_signature": "2051bd347e3b0a954234fcd6506fb99afeaeca79e1d24cba8182d0a2b7ecdf9bc75999f63bdbd1add14cd54a208dff003fcc11148c145c96576660b78b1635cbb8" - }, - { - "block_id": "000f43b24aabe480196b303ad77e50bdfb2a267f", - "extensions": [], - "previous": "000f43b1e53b6119f9c4c57a09071a9908eec018", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:30:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f3d048a1ea6d407890c10a280ed55f96d2d3c6359ea50ac08a8f561d5e37b873d20f6cc7c161fa9efc4f28f15b5aba8e1a6b367928a8cee0fcceb8cbcff63b95f" - }, - { - "block_id": "000f43b3cadb4111f38aa7cf3a387a9ce729e445", - "extensions": [], - "previous": "000f43b24aabe480196b303ad77e50bdfb2a267f", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:30:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f4ed203faa42ed6e3fc314ea10fd58eae1b453b8a610afda29e0d00da4e72cb1e14a55ea762131e715afa87499705e0d6301a53f6111eba5e52b690cf127c81ee" - }, - { - "block_id": "000f43b44cf7d472bda7f08b345bc8d4a2a8c5e3", - "extensions": [], - "previous": "000f43b3cadb4111f38aa7cf3a387a9ce729e445", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:30:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f64abff470cbf1f020fcba98000431c7489ce4a5cde359a10cf28f2eb74dc8d7a3c8ed7c285d9bcc48e418b3e519cd935cf264e6dc74c0ff476cc436d3d0bd25a" - }, - { - "block_id": "000f43b53ca3dbdd44a2dd38447402feb2b067f7", - "extensions": [], - "previous": "000f43b44cf7d472bda7f08b345bc8d4a2a8c5e3", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:30:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "202bcb4c09ae4e74367687ad7f8204e9ee411790157be389a656c5f27bcf2576cf7572958d1b93e3e57a968f30caf9db0f396ba9ff30faae59838dddfdd7dfcbab" - }, - { - "block_id": "000f43b6efef515150bee975475ffb7c7639baad", - "extensions": [], - "previous": "000f43b53ca3dbdd44a2dd38447402feb2b067f7", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:30:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f1c4f4db02f584876aeacf9d6abc31b2ad02e2a1e4d80651e5fed5e9e867186e16e9c7e2c344bee4dc0913e558cd6fe9da45e0203c4c8cc01f75ff72291f5d7da" - }, - { - "block_id": "000f43b7fc4f2e39bbf4c31cc915aaa042bf584c", - "extensions": [], - "previous": "000f43b6efef515150bee975475ffb7c7639baad", - "signing_key": "STM7zCGUYKYNsJEDnrwCEU2BwPdNQmFj8NQ1TEhPdVSUgneNMRUEU", - "timestamp": "2016-04-29T04:30:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bue", - "witness_signature": "20503ab7172ae40182bdf763590f4cbe425738a74a0192641ba4b621d32f8f54ec7b914bee7c8f814b05e7899c294fa9bee7e27ca3928e00c2cc3a353524da2e5e" - }, - { - "block_id": "000f43b84a625a39ca16a6cd6b1ad74125d1a8d2", - "extensions": [], - "previous": "000f43b7fc4f2e39bbf4c31cc915aaa042bf584c", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:30:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f776315c8a0088d3dfc42499e039ea3a62bbdbe824224ddd2cbf61cd41699ae12567139100d3bfefb46da6bef0c9a537954648dbfad883804526d492f268461c0" - }, - { - "block_id": "000f43b94c079787f332c7cbc93dc3ce84bb30f6", - "extensions": [], - "previous": "000f43b84a625a39ca16a6cd6b1ad74125d1a8d2", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:30:57", - "transaction_ids": [ - "6bb353421840356e973abd1296743b93b8a08ec0" - ], - "transaction_merkle_root": "ad0aa02665bc47b97e53d5f8a164b22b1e9f98b9", - "transactions": [ - { - "expiration": "2016-04-29T05:30:54", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f43b84a625a39ca16a6cd6b1ad74125d1a8d2", - "nonce": "806255817238555383", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "579022fe3ccc9730ff35d571b1bc740c0cbeb0c2e92b1ce6c3218d95028b65b0", - "signature": "1fa6103e395031b04f9df9ca6f057c39e0a2d6908bf1269b204956e9975b8c6a9e522f38dd4af29950a029c8775a2f53d1c9a2400cb9eaf15b79f3a4d9c5c6dd82", - "work": "00000007d4a3e3cfcd26958d2db64d20f11f5d82498510bd5ad3c6534348b822", - "worker": "STM8RVh9ZnJZhULNSQwRUueSJSKtJUjNMPbnyRdWmEJAVCTJZ5AYT" - }, - "worker_account": "hr402" - } - } - ], - "ref_block_num": 17336, - "ref_block_prefix": 962224714, - "signatures": [] - } - ], - "witness": "arhag", - "witness_signature": "204ad45256c8c8ee6b5ec2ef7323d677353589d2c10ba81f12b4f5dd950b0210ab46b5aaca316746ef46cbfb145720821aac58ded2e36c7adc718344cce8f34ca3" - }, - { - "block_id": "000f43ba67165bd418dc630752bd69b2cc54c1da", - "extensions": [], - "previous": "000f43b94c079787f332c7cbc93dc3ce84bb30f6", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:31:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "20273ddbbe528c6fcbe95922f43eeff5ff8536896fd4765e259f38416863ac20e83604bbcba40f74501ac1126f4707a51be12bc537bf1bc5b1c7d7fcee6952a623" - }, - { - "block_id": "000f43bb506caff1dab6d28747fbb284fc4d8165", - "extensions": [], - "previous": "000f43ba67165bd418dc630752bd69b2cc54c1da", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:31:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "205a7c48c965d4a56e1109ade26e3f56c3e774765bec9627452563527543a545f250e53c6ee11db74f8b43a03387343dccdf73e8f543bdd7f753b5a4083f1a46ed" - }, - { - "block_id": "000f43bc2619f46ff854e2ba3bcdee558e4f5b7f", - "extensions": [], - "previous": "000f43bb506caff1dab6d28747fbb284fc4d8165", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:31:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "20756138b505b6658fa9dd6cecf65881e3b160d13ef5b17684a70ce00485729d7a5e8ab74876783691e59c92f38ccf7393c65362d92e604a87b9e1aa830bf1e85d" - }, - { - "block_id": "000f43bd8ba83c30f1b2610c8f3b1125e769ca77", - "extensions": [], - "previous": "000f43bc2619f46ff854e2ba3bcdee558e4f5b7f", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:31:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "20778b293ee104c3c2e272de1026ade32859cc90c747eb91a5f5108f08995c50280b3c264f0a5b28b49d8619a9bbb99fe9ec485a786b5e2e1873ad5fc5f8583969" - }, - { - "block_id": "000f43beca4f03c74af6bef1a8839217e81812ef", - "extensions": [], - "previous": "000f43bd8ba83c30f1b2610c8f3b1125e769ca77", - "signing_key": "STM6J3NVGh67YVF2M7VHkwKPSaTz2PJ9uMYnixM36JMP4rxhagT8v", - "timestamp": "2016-04-29T04:31:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "rossco93", - "witness_signature": "1f08c5799c80482dbc7c4b34d358661aabeea2adfdad1da322c36f62d3a0b0c33a6277c9289466807747ce39592974dab545296dd4f114dc0582ce8620dbe04ff2" - }, - { - "block_id": "000f43bfb7015440e5d9b1d883a3d7e6d20176f7", - "extensions": [], - "previous": "000f43beca4f03c74af6bef1a8839217e81812ef", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:31:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f19422235c5d26c90f6d27b94cea826bb970f1cb6644c679af703cd0b0914ed8e2429c026aa1d27765258cfe05ca0b457fdfa76399c72dab9656bda7e5f27069a" - }, - { - "block_id": "000f43c02deae02fd393ac23a5f568b098125dc1", - "extensions": [], - "previous": "000f43bfb7015440e5d9b1d883a3d7e6d20176f7", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:31:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "2040ac9aef12bc7d1cbd8a0294684eefbb04308ab95c41371cdd5dee6bda11164b552bd37be3d593ae5c18d5bdbdec92217b037a28e325424695cccdae258440d7" - }, - { - "block_id": "000f43c1bfd3801cd97ab3199928d206b6dee703", - "extensions": [], - "previous": "000f43c02deae02fd393ac23a5f568b098125dc1", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:31:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f7501d3384a8f627f1cbad737f7c88175c60814a71dfe45d6bd9e25a476a89abe6138201f59d8471d2ffd275d63ff50077fdcba89482c6017c14432d2e9208f6d" - }, - { - "block_id": "000f43c24d27872fcd74f8e6193b8bec2307f035", - "extensions": [], - "previous": "000f43c1bfd3801cd97ab3199928d206b6dee703", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:31:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "203ac14f59e0d30584638ad096686f8897adfb316950ee228877fffebc2b750e8303cf846012b5365de6f69e54a9fbaa4b9b4783caa5fc92e0882263940c0bdb34" - }, - { - "block_id": "000f43c3d5e10a32678e57adf084837e90a23110", - "extensions": [], - "previous": "000f43c24d27872fcd74f8e6193b8bec2307f035", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:31:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f31a744840ad770b49c774bc64a9061ed49d58178b9ca1728645e94362b132acb43b2a047d6034a1356533df0f8a8eac35b9fb3a2cc968aa0465da192d7180f8a" - }, - { - "block_id": "000f43c4d0aad3e7b89fe79e1b0497a3f8946533", - "extensions": [], - "previous": "000f43c3d5e10a32678e57adf084837e90a23110", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:31:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f5cb1ba583fe503bf1b59b7b3a4e3c840f2262224a212fb4a20aae4a8843d34a9381fa6baef038abe5f5652409e8370e5d85ec53324a07109064fd3f8ee32179e" - }, - { - "block_id": "000f43c5a951f5888843b149f6e56640f059c9a2", - "extensions": [], - "previous": "000f43c4d0aad3e7b89fe79e1b0497a3f8946533", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:31:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "205a0bf3e9017ae017ad7a442fdebc5b04e4bdb86a04064ce69478cbb3c1a8ed487af451b58d423593ce0a3417dd676e3d2af173bc24f569ea974d79bbf9276245" - }, - { - "block_id": "000f43c60d6293b2adcd665a2777e37c4ee9a749", - "extensions": [], - "previous": "000f43c5a951f5888843b149f6e56640f059c9a2", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:31:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f734d9213283d4d57387539d3c61f55106b95b48e783d4415c28d9b1c23d2d8d23d26f3d2e28ecfe18a13b580a95417bdf84759ac69a2962c833cc98f3ed87878" - }, - { - "block_id": "000f43c772013f1ea264a111b5fac30824c1d4ef", - "extensions": [], - "previous": "000f43c60d6293b2adcd665a2777e37c4ee9a749", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:31:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "2046bb7396d8e8c9dca154a18e357ad4d32afbe99bda4054516514e752733cbed40b2e00ec917880154c40b94e55cb0d6bdeeebf55ad85efda5fa100726a2b5e25" - }, - { - "block_id": "000f43c8b05bc320d025a8758775145da08215e0", - "extensions": [], - "previous": "000f43c772013f1ea264a111b5fac30824c1d4ef", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:31:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f02b5f2990cab8d1efd5e8bf1b9ec924d6fde7e24301ee959861867c363dbb7147bbad42a97c001104f0d6e0981bf862ae4648a600261bed7cac680144e807e55" - }, - { - "block_id": "000f43c989ea47cf257732ec9460767fe1ad842f", - "extensions": [], - "previous": "000f43c8b05bc320d025a8758775145da08215e0", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:31:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f5db1c63873cffc97c74886713d901d6b8717d98130aff35db2e33ee94da123c5375269b8bc6d1eda68e282b8192a16fc2e17efcc28a4f27821a5573f5522bf91" - }, - { - "block_id": "000f43caee32f4e2029af7d9878dbf0bab6f9a5f", - "extensions": [], - "previous": "000f43c989ea47cf257732ec9460767fe1ad842f", - "signing_key": "STM6Kq8bD5PKn53MYQkJo35BagfjvfV2yY13j9WUTsNRAsAU8TegZ", - "timestamp": "2016-04-29T04:31:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "wackou", - "witness_signature": "2071a282ca287b6c51b202b2c8a5d1e051d554e89d01c3b76d9301cc66450c74721611773e523410ee44232f82288052adb5c4449150d1a91fa6a06b50410e2a78" - }, - { - "block_id": "000f43cbd2386aa996a62c4f08b62f2bc66d3463", - "extensions": [], - "previous": "000f43caee32f4e2029af7d9878dbf0bab6f9a5f", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:31:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "204ff65f6e13ff21f87b75d2fc4d1ab2f6cdd9106a86954f072b685573796d965932ddb13701d47479e1b4b87809b17c6062fefd8142e85f31432a34cd4eea6458" - }, - { - "block_id": "000f43cc46e613cc1ce9a84b89e283457333f54f", - "extensions": [], - "previous": "000f43cbd2386aa996a62c4f08b62f2bc66d3463", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:31:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f5c4a1fba67c735303511a69e70c05c2607af41aa579cc511bd8e89267ad5aef87c417a51d9a1190e07874a17ecbe3ac5c13d34c11f53ddbc3bfe9dbbcfbb80b6" - }, - { - "block_id": "000f43cd2f88a8e0b310fe44195c51f95644486b", - "extensions": [], - "previous": "000f43cc46e613cc1ce9a84b89e283457333f54f", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:31:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "202585e5d8334d7ccdcf2ec209127260aa972851459d8c30ec56c8d8d9a0184d360d119aff1baa303cc1e92ce7aa5b9781a05e8b1e2fb522a089e8a6759d0b6319" - }, - { - "block_id": "000f43cec297b96acaaa2279fcf4bc309c353e1a", - "extensions": [], - "previous": "000f43cd2f88a8e0b310fe44195c51f95644486b", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:32:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f1391b9e1f44d9865b63d2b48fb6eb5e6242f9e98df0ed402d874aba7683ffc18092889af980e4a1e326db8324e733113d25d5d77e768b8f3bd5e3b4a140c4788" - }, - { - "block_id": "000f43cfe183d5611457cb9098297e54502c7122", - "extensions": [], - "previous": "000f43cec297b96acaaa2279fcf4bc309c353e1a", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:32:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f347b4f5d932ea3b74ceaaf26c18570368fc81afb2125fd3cb9e5f6e53ba5512625b2f5c617c271c4eba6f2866edfb388d12d8815a07b57f0f0b682c33669172a" - }, - { - "block_id": "000f43d02885b87e3c4a0f650c03073e99cff4f2", - "extensions": [], - "previous": "000f43cfe183d5611457cb9098297e54502c7122", - "signing_key": "STM6QzpMj54ZsMkDMSv7fV1rPC3HEW6sorumfpVeDd9YnecSBf8AF", - "timestamp": "2016-04-29T04:32:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "mecon", - "witness_signature": "200e21fb91c4066a4d9694a01a70fb111202ba8c2f93853a342851b656480582e50ec35a66f1e27c89471c46692a5fe62f593fbe8d7a710d82a117cf58e29e068b" - }, - { - "block_id": "000f43d169c8655089826152d362717d3fb4ebb5", - "extensions": [], - "previous": "000f43d02885b87e3c4a0f650c03073e99cff4f2", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:32:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f7590340de73ca092b3b65469c82f4bb3c5a34e4c89853547af35efae4c0927d74d2673520b79057679546c2f1167ab3ff7a807a25d6a9a41d472de21d87a658c" - }, - { - "block_id": "000f43d2ac996c7c3b2c24a3305e37bee9ccb939", - "extensions": [], - "previous": "000f43d169c8655089826152d362717d3fb4ebb5", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:32:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "207c56e57bf05f991106b466e6fd1026807c275196b64a72bb3e1d783e947aed9267cc0803c9bb6d5bb79a74fdc88688e5588b3c7e5014a74695c8fbc0e795f5b2" - }, - { - "block_id": "000f43d3a29cd0bb7fc2e3eebbc8d29ab5def2c0", - "extensions": [], - "previous": "000f43d2ac996c7c3b2c24a3305e37bee9ccb939", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:32:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "206769ca4f1c0c5e7b9504bfb843972d924b7d23ae6d5b9f51731e0574f15b1feb7b159a0090abc8980f422b41a1ec826dbb1933383d91c107d9ddc4c29ab29f32" - }, - { - "block_id": "000f43d45adfaf5556f443ca3bb2cace47364490", - "extensions": [], - "previous": "000f43d3a29cd0bb7fc2e3eebbc8d29ab5def2c0", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:32:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f08d89f7513ae63ead96fad43c31bd3b5d659ddcaad4b1418afb049ed810236da1298e1f6ba33873a52eca871f8bcfd01c6cf91bdefacb52c9f58ed284bad7128" - }, - { - "block_id": "000f43d594b9501b0bebabc050a60dae3504715a", - "extensions": [], - "previous": "000f43d45adfaf5556f443ca3bb2cace47364490", - "signing_key": "STM5wf7YDmZdh6L6f5GDDeB239f6WzrLWcrueNywDa69zX8zuXRkA", - "timestamp": "2016-04-29T04:32:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bitcube", - "witness_signature": "201d816b729fb58771e32c280922804bb89ea7031980b594cc6ba91f056bf34b044432938e95becbd7ae2ee26c9f8a907f2767a133cdc1a77b9b78c94feee0f6ec" - }, - { - "block_id": "000f43d628bdb4816b236de7405d8e5fa3ae8702", - "extensions": [], - "previous": "000f43d594b9501b0bebabc050a60dae3504715a", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:32:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "2005f36b46038eab35cf49eeac375e4141ebd6e84871a3ce32b3e76bef2eb11a4a1dc8b369802aaf3bd6c9cfb7d29d55c1e1f926d8ca3079ea6ceebbb69e8ce395" - }, - { - "block_id": "000f43d7e8632b4980fa48afe965f429a50908d1", - "extensions": [], - "previous": "000f43d628bdb4816b236de7405d8e5fa3ae8702", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:32:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "2018aff039b1abb38f0f308f5e08c5bb5e902deb7714cf58c36f6922bd5c0eefa8740e234163fe7f9ae97084413023b924d0b57981f103137d671b0a4af1f303b5" - }, - { - "block_id": "000f43d82ce1b688155b1aa29d67f529f6f11862", - "extensions": [], - "previous": "000f43d7e8632b4980fa48afe965f429a50908d1", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:32:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f14daba4077b144ecb1b5fe93794b9fedf25763556bd0bf2e372c8a0f6e65e9ea7a4d5961b598fb3729b0af08e4546abdc567796228e288d410e15e5f8e818f16" - }, - { - "block_id": "000f43d9f5cc42bf3277b97c49533f1cfe1afe9e", - "extensions": [], - "previous": "000f43d82ce1b688155b1aa29d67f529f6f11862", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:32:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f2eaa794711999915ff44ce0ffa0059a97dc3972d8f8793a3406d2f4e5b0dd93652cac57c7d61a30809db8917fe9c343b2edacbdccb2201a662c293987030f259" - }, - { - "block_id": "000f43da11142a8c0195ec27a419c939ac65c7a7", - "extensions": [], - "previous": "000f43d9f5cc42bf3277b97c49533f1cfe1afe9e", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:32:36", - "transaction_ids": [ - "b3c7f1c5507d381a724818a70e79a1abd9ae6154" - ], - "transaction_merkle_root": "028436dcfe3328423c4955c5241751f6d3a5db25", - "transactions": [ - { - "expiration": "2016-04-29T04:33:03", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "434", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "datasecuritynode" - } - } - ], - "ref_block_num": 17369, - "ref_block_prefix": 3208826101, - "signatures": [ - "20632d98f67dd68d20aa006034e4f7c05a20684796d4655096ac5037b8b8f07ee46809f32fa47718d60affdcfc7189610ca907132ab9c35dbbca6faf796f323897" - ] - } - ], - "witness": "arhag", - "witness_signature": "206e62142efaf433fd5bcb1095598d6207ca76740a8a783560cf7afd6b27146eaf6219b7e2b82bb50961115f3b8a6a24941f87d77c6a44040962b8baa2700a449a" - }, - { - "block_id": "000f43db87d97f9b98342dbd79868dc1de293b57", - "extensions": [], - "previous": "000f43da11142a8c0195ec27a419c939ac65c7a7", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:32:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f26e5e068347666d2b747f07cec62ce8ac2eba13b53764f0dd94024e72519959b2738d4f5741a2d29224a625328c1d13a7a085fb7ad85d954ec1fd5c749e4bc58" - }, - { - "block_id": "000f43dc653f96861768e63a84234755fa369098", - "extensions": [], - "previous": "000f43db87d97f9b98342dbd79868dc1de293b57", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:32:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f69f2e91fb5fd0f5fa47f90f6b79f9a379b821237c4bf26790db7a6b6c30dc9af6af103152f41e2f57514bde50a18d1b8af86a41e88843c4e911ba03f548affab" - }, - { - "block_id": "000f43ddfd7ffeb4be1e06236fa060b7a5630ae6", - "extensions": [], - "previous": "000f43dc653f96861768e63a84234755fa369098", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:32:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f2699e2707b738d05ce05e6a4a027d6bab0727cf2c25549c257afac00a84ae2ec45f6a57b04a156cc9cb43c16114bf127861f8caf5dc277aeb1ac9f1cea4b8dd6" - }, - { - "block_id": "000f43de07cf8c86354cba90f67cddf7e1e2fe19", - "extensions": [], - "previous": "000f43ddfd7ffeb4be1e06236fa060b7a5630ae6", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:32:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f7555350bb20a46ae2fbfe3a31df489401a4220bd226dcd5053e8b74fc33e90d70d74549f598dfefc5555ce2bb85361b7662fbc7cfadf77f7d250ae0abe5f5ab7" - }, - { - "block_id": "000f43dfcaecc0978ca89ebd6d4ad35ee1b15a72", - "extensions": [], - "previous": "000f43de07cf8c86354cba90f67cddf7e1e2fe19", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:32:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "200f3ad42f782de16e9165c48e5db26a7e9181d23bf8f8b6895624dbec480861a213c6a0460acbd51ad0c1f4e2de8dd0ad2404f3140935799fd4852491eca11619" - }, - { - "block_id": "000f43e0f282ab22aabb619dcf768fd80bd9f8ed", - "extensions": [], - "previous": "000f43dfcaecc0978ca89ebd6d4ad35ee1b15a72", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:32:54", - "transaction_ids": [ - "61b4dc2377f68d2f861bddc362ed9afabc06fdc7" - ], - "transaction_merkle_root": "946c951ad44b251ab2bdaeddfff7cb0c6004144e", - "transactions": [ - { - "expiration": "2016-04-29T05:32:51", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f43dfcaecc0978ca89ebd6d4ad35ee1b15a72", - "nonce": "10126926471287149973", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "0abaa532475bcd7f12cc029bef8df3ef93facf28795d8813fbceaf915389f81d", - "signature": "20bd66ad0896bd283c0ba84b65e7755d7493539bf772a62baf082d0a134804b6615eb2b021600d97a424bbc7db0203e010380e4729ce56d2010de8256e008d655a", - "work": "0000000b2ff1d1dd447d71d0aea161d080cb0986e20ef6c600d52d2767dcb5d8", - "worker": "STM5Tz7eGU5FXw921xJpXzSuaFCUeudTWxKPyPhyURYEkZVxofQNW" - }, - "worker_account": "sammy007" - } - } - ], - "ref_block_num": 17375, - "ref_block_prefix": 2546003146, - "signatures": [] - } - ], - "witness": "silversteem", - "witness_signature": "1f1dd818a5800ca273d3199c95ac4484242b0228d8b3b9509e6a3a1bd3830a18c32ee70eef8048a20e830ca1925affffa23249f8fd52b96850579e352ef6e6bcb2" - }, - { - "block_id": "000f43e1155d7198b9b482a36a528a9943576337", - "extensions": [], - "previous": "000f43e0f282ab22aabb619dcf768fd80bd9f8ed", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:32:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f6ddd4f889b1429d639a1a1a59b137d56407e45563a5a4042ad2efa4697112d9801b84bc2509dee7b1485c8a28b9b457e92965a13f4222693e8ce7f4428e954af" - }, - { - "block_id": "000f43e215d80b71a3089b864ad8135c3472bf3b", - "extensions": [], - "previous": "000f43e1155d7198b9b482a36a528a9943576337", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:33:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "205a27cdab2cbe54ab02504672f8d85932bcb7a5f0638ccc74ef4c17a3322807f011827cfb793ec72fdec12ffdfd1f3f25dd958bcc04945e920fd244ca65ae00d8" - }, - { - "block_id": "000f43e336e7ca6028cd8bd7833580e9906608ed", - "extensions": [], - "previous": "000f43e215d80b71a3089b864ad8135c3472bf3b", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:33:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "204a6b8a47dc4367d551350915fd407e64efad8935b2a81ab55e2989b05cc848c46e9d93dc3989a05960be988f16e24d098471956f39f37df51261b64eb41786d1" - }, - { - "block_id": "000f43e40acc5fb612e14e9f974af2ce36341398", - "extensions": [], - "previous": "000f43e336e7ca6028cd8bd7833580e9906608ed", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:33:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "2062eaf395e04a9637950410972f15a8be008e3f9997a88830743f99d78f5179dc4cb620a8fb1501e63407872a5e461c744338b5d4654de3cdab532340fb445601" - }, - { - "block_id": "000f43e56cbe957b4a84330c5d146ed2abf021fe", - "extensions": [], - "previous": "000f43e40acc5fb612e14e9f974af2ce36341398", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:33:09", - "transaction_ids": [ - "5bed6b81acddee503c4ef7b1343dd4e9b20a482f" - ], - "transaction_merkle_root": "5ae6c12676478c50cfe4760c6d197806e0003484", - "transactions": [ - { - "expiration": "2016-04-29T04:33:21", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "tuck-fheman", - "permlink": "how-is-voting-power-determined-in-steem", - "voter": "clayop", - "weight": 10000 - } - } - ], - "ref_block_num": 17380, - "ref_block_prefix": 3059731466, - "signatures": [ - "1f1a5b65eb6b87a254fa3ec4f1a3680ea461ccd64ee6ac263fd0055cc84f62dfaf2e233a28de96fb7124d47a5bc0bcd0d204ecf888e75e9503f929c219d1037b85" - ] - } - ], - "witness": "smooth.witness", - "witness_signature": "1f592d769a010d401cd98d8de64cca6781dacbde2c82b2b6bcdace6d0724e62aa0347c978a682d261e21379928b7085fabe9f5f9d1e29924aaf4dc661ef72fb646" - }, - { - "block_id": "000f43e653481bdb2e98c3e37b13d8c9274cc9dc", - "extensions": [], - "previous": "000f43e56cbe957b4a84330c5d146ed2abf021fe", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:33:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "201af932d697e492975f851e135259bb4132e46ca48d9e15b416f15c5bb06ba5fc56fb500d2658d2b48fdbaa879b7ffe617e95d788e05f6fdcda6895cfc9bba5fe" - }, - { - "block_id": "000f43e7094963daedade526b5123c113923e4ab", - "extensions": [], - "previous": "000f43e653481bdb2e98c3e37b13d8c9274cc9dc", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:33:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "205cc0fe23684c628b9c8eb133b6190e4d1f586e5d000ff1210ccdc549c263b2c7425458aef6587169d914cc967d829e00d597c494feba67d747a0670ecdee0dc9" - }, - { - "block_id": "000f43e82ab67070576cef1171e1ccb463d476f1", - "extensions": [], - "previous": "000f43e7094963daedade526b5123c113923e4ab", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:33:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "20752feadf02e95f6419351da961c78d7a7907df135c3011ad57e28204f7716709528a8cdb6918545a3eb5e0f160d5b8cfdc0f40463d5f70dc2c1f0c5add956ab4" - }, - { - "block_id": "000f43e9b8a2938c427a6326bd70d7578f51f38d", - "extensions": [], - "previous": "000f43e82ab67070576cef1171e1ccb463d476f1", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:33:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "204feb237e883702459f8229a1b40a9024e45d687fcddb9da6f0320e8ef91f975a56924dc25ef26ee0d903958fff2e958ba78b603e9c0f7a8e71e39d086f998532" - }, - { - "block_id": "000f43eac7e43e768d23b2aa85f647ed539cefec", - "extensions": [], - "previous": "000f43e9b8a2938c427a6326bd70d7578f51f38d", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:33:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "207c1454fccbdf496498d856bc38ac89120d348f57055919c1ed75473c5e2902c9261083fd76d371b545056f880dda97fb2fac264a461807a8c0405a3736bd3c29" - }, - { - "block_id": "000f43eb8bbb4b929dd9dae39b7a07209d9f148f", - "extensions": [], - "previous": "000f43eac7e43e768d23b2aa85f647ed539cefec", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:33:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f35df64528f93a5fe2e3e8cb069a6022e13155ac2de61ccca432773482b470d2a1f2062d946db950f6e8a431eb01b2dbc9b88e202b1304ba82811e9c97fa56f79" - }, - { - "block_id": "000f43ec65b15391668cbae5de0f15e10b3e19d8", - "extensions": [], - "previous": "000f43eb8bbb4b929dd9dae39b7a07209d9f148f", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:33:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f49741d35a28d362fd7ca3bb2b47132566867eb8d7f869fa48c88039a6886e49d5b81a7c41f7097191e016563e891479e0be1a8827bff215dcc5baf83ed8fedb1" - }, - { - "block_id": "000f43eda9149ea6aa9067701fbecdc5c27c4ac1", - "extensions": [], - "previous": "000f43ec65b15391668cbae5de0f15e10b3e19d8", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:33:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f2e46df90d8bdd7c19d198519fe551207fb6005e81dceb6068fcbe705c41497e41305b8ff15ffafe81206536f05694247c34027138e1e9591e59d70f0f56dde7f" - }, - { - "block_id": "000f43ee7883039cad4c25a41e29deafa9109ce4", - "extensions": [], - "previous": "000f43eda9149ea6aa9067701fbecdc5c27c4ac1", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:33:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "200b0cbd337c2e58a29e5ab4eaece7a1f7079a5ccf7aaf654ff034a474d58ed1077a932cfb29bce88d13d40ac9b3ab691c5cefc26025fed9cdd25c056f49c9433a" - }, - { - "block_id": "000f43ef437f91852f6274cbf691eada67e7acd3", - "extensions": [], - "previous": "000f43ee7883039cad4c25a41e29deafa9109ce4", - "signing_key": "STM8Uz1PaiWG7rByBGdfkmLtLdi1GVC2X3dGNnVCY82QKZAnGhG13", - "timestamp": "2016-04-29T04:33:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "salvation", - "witness_signature": "1f330e47146755e14f80d99e4227829153aa0f1fa883fb7459e9889194398701192347ec368a14937b24c91e353e3526b87ab741111ede74956daed5c5a86e3777" - }, - { - "block_id": "000f43f044bd6d92710bf647efe4974e9eba7ff2", - "extensions": [], - "previous": "000f43ef437f91852f6274cbf691eada67e7acd3", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:33:42", - "transaction_ids": [ - "4f80861298f1544154dd04140c975c99993086bb" - ], - "transaction_merkle_root": "5bc278082b780e07829c7903090fbe2490bbfc7b", - "transactions": [ - { - "expiration": "2016-04-29T05:33:39", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f43ef437f91852f6274cbf691eada67e7acd3", - "nonce": "8624443040766038969", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "cb2aa81223efdb8dacc51e128cc748402db678d90edfa6c312738d9b763fbf45", - "signature": "1f7dbdeeccdac260f011ce7764b615d0fa55f80d3061d5c7f8fbf034f45709f4aa1484ef21f021231c325cf9415d60fe531f0f4b09f4cedf6e22fb388bb6822d8d", - "work": "00000006ca5920740b1ff7984fa84b6272218d466966af3433be0ca0fbc92f10", - "worker": "STM7wNAfF58SNaKUKNJV7EJg8AMNz6c2W9WXzVKHEgvzYLDasLse3" - }, - "worker_account": "ah1" - } - } - ], - "ref_block_num": 17391, - "ref_block_prefix": 2240905027, - "signatures": [] - } - ], - "witness": "clayop", - "witness_signature": "1f50aefc33f0867fcd41c5169559a2e35a2da35c940a5d7fb23e2e4a31842ef3dc7f15996d217188fbbe1cbddf07d40bb3616ed87bcf9d39ce442db0f62824add8" - }, - { - "block_id": "000f43f1596d529e7ed2b0dfcf20e27b68b21590", - "extensions": [], - "previous": "000f43f044bd6d92710bf647efe4974e9eba7ff2", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:33:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f05a056d360b68efa2bb940ce33f09d6828ff3efb73c6735cbaa268f9afd1130a6da8ab38f922620930ed002462918d69f0ee8cdf2c6f82b7d1a5c6d1c2ada5a2" - }, - { - "block_id": "000f43f264694aa2cb450d54fe038004076e3996", - "extensions": [], - "previous": "000f43f1596d529e7ed2b0dfcf20e27b68b21590", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:33:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f238ab3450ed5e0c26490a25ef913a5a2e664078ec0ed3cdf5bb465dc2308426513b4a684b5d124f06f7f5ff39e5386b9de2585a59fa4d6ff8ae9812af366ece2" - }, - { - "block_id": "000f43f3664ebd8d681029bd6a7e85fa1437be18", - "extensions": [], - "previous": "000f43f264694aa2cb450d54fe038004076e3996", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:33:51", - "transaction_ids": [ - "23f23b034f667d3f266bb3fb5cb385321e280854" - ], - "transaction_merkle_root": "d462be013664bc3f439b8288bd96730d493db667", - "transactions": [ - { - "expiration": "2016-04-29T04:34:18", - "extensions": [], - "operations": [ - { - "type": "account_witness_vote_operation", - "value": { - "account": "joseph", - "approve": true, - "witness": "clayop" - } - } - ], - "ref_block_num": 17394, - "ref_block_prefix": 2722785636, - "signatures": [ - "1f0bf9230f8772ba6107ac22ce8cdee1e66afe3ced6e146e83a31da089bbbe4f9801e11dca7a6e7e17189abea9490afe2a23af7e5cdab1d053b72dee002c8662cf" - ] - } - ], - "witness": "datasecuritynode", - "witness_signature": "1f3121264972c125e0efe7ea9b1214269f400ccfa80fc433721ca2e8aefdaff1e97e98db73c972308d92c8e208eea900689677b07e29799e12e736bd74aca626f0" - }, - { - "block_id": "000f43f4a8c903ef8d5dfab7a8018bace1b1b619", - "extensions": [], - "previous": "000f43f3664ebd8d681029bd6a7e85fa1437be18", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:33:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f4f2e98b45655b5ee637405206eb7236ef9fe13eef0cc0e8c53328521b890d4a17c4f8d0104d8f236a28d8ac9ce8b2627a62900c00847b40149fff1183ae76b5e" - }, - { - "block_id": "000f43f56dd916cd263d4371ae88f9f13f2c9cee", - "extensions": [], - "previous": "000f43f4a8c903ef8d5dfab7a8018bace1b1b619", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:33:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f5da412436ed99d1601724c7e477211992e0132a9a4222a62dd66ead68727468648b6362da4723af37b759ad2beecbb5d08d847de178f03f6883e9b482aedd563" - }, - { - "block_id": "000f43f6730be935a32027aaeaae4c39f3fe4326", - "extensions": [], - "previous": "000f43f56dd916cd263d4371ae88f9f13f2c9cee", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:34:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "204ff3e8dd728deca12e9e63717bc189a0bd93319a53af9968a19af33b3e6fcc49378f12e9a41fc77937e50930a697ae2527fd35e6477855893e4e05e79a489114" - }, - { - "block_id": "000f43f7899692ac6c3990b70531d9ed19fa1bef", - "extensions": [], - "previous": "000f43f6730be935a32027aaeaae4c39f3fe4326", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:34:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "2064b8a0c995c4f3c11ab9a13bbf67c467be29c98f50026b1663aef899346d01e2090b18df54d311be9f2d05709c06db6affb156dff30f00bfeb08f9cf9d773f99" - }, - { - "block_id": "000f43f87c014180852e8060e43655e1f0c01122", - "extensions": [], - "previous": "000f43f7899692ac6c3990b70531d9ed19fa1bef", - "signing_key": "STM6xqwMyEhWBWepcNk1mqvnTXVpz6Ntct8jxQ519Qijo3pQKu6dv", - "timestamp": "2016-04-29T04:34:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "rabbitminer", - "witness_signature": "1f0e578af4465fad27da2313e32964280aed1bed3660c8626e5a630a96886cf07432b8f70b730cd4c62fb3ee6e4719bbd9ffb21142e9d9bbaa9347eedb6b4e5b4d" - }, - { - "block_id": "000f43f9c2bc8be998f6a72ea4dd7d320d5a7b27", - "extensions": [], - "previous": "000f43f87c014180852e8060e43655e1f0c01122", - "signing_key": "STM66crmnX96YMyWUPnFzSkv7DsGESbWaoSGbmoNq4EWDYq2wnwPf", - "timestamp": "2016-04-29T04:34:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "liondani", - "witness_signature": "2056567363763a0fddafc14947d75daa024d2a1d5323c3e8dccf342b64b348c2175ea5e7241390cbb3e7ca85c569e43332cbd348fde0bcc9d7bdc0d240b93ee8e1" - }, - { - "block_id": "000f43fa674e58f9740109ee6a02877c020eb990", - "extensions": [], - "previous": "000f43f9c2bc8be998f6a72ea4dd7d320d5a7b27", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:34:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f15be298cb1a03f6896583620e2b1a90a8ef4a82f32dde54f2b7509f080a4835a422f93474141606f013834cdc62b1b1f7eb7fbffa9da6dec4debfa2015609948" - }, - { - "block_id": "000f43fb118f24ab3c57c740cd142de03250977c", - "extensions": [], - "previous": "000f43fa674e58f9740109ee6a02877c020eb990", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:34:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f1012d97409686edd883573085d3970ee8d8c8e74dd56cc744c32e5fc23275a702538d0a4a39dfc931bdf93f136deb1c1373d3f51e13911bfb781d77b75a93754" - }, - { - "block_id": "000f43fc74adf26823c89c7e6217f45b90bfbd58", - "extensions": [], - "previous": "000f43fb118f24ab3c57c740cd142de03250977c", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:34:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f59e73ea595c5afc2e66d8be991a758e72f85eab6cf9f8ff0f254c853095691b0626fb4ce724df43f59354bfba066861e74571da6a1f8e4739632175f42d53b45" - }, - { - "block_id": "000f43fd5d957254dab46c2e6b8723cdc6051f8a", - "extensions": [], - "previous": "000f43fc74adf26823c89c7e6217f45b90bfbd58", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:34:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "2046219fe17becf19be522a86b555d4daddad480a46f872cb85d5a830ccb72b6d877835bb55b5db7bb630d67491953d4cec7e2a9aca49e7c01ac0a6d8bf4d4a09a" - }, - { - "block_id": "000f43fe82720a6b3ced2e824364d9206c605d55", - "extensions": [], - "previous": "000f43fd5d957254dab46c2e6b8723cdc6051f8a", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:34:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "20158f5b77189c759411bb65850f9c2587b909d88b1674db0652c9ca5c156b784e7a285eae7c272f78006d5631226f79da43e2a0325a449c0a359fdc6661bf50c8" - }, - { - "block_id": "000f43ff5d5509892a75ccd57dff695ea10f2086", - "extensions": [], - "previous": "000f43fe82720a6b3ced2e824364d9206c605d55", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:34:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f65bcdbed8ee58109ebdc66daa31c5be17932afbbd3614dd0348407c12938f8c260e12d12179400f0614f96e275955494004469a2f81e06118edab82dd643d01f" - }, - { - "block_id": "000f4400ae1b6faf4f59aaeff0d4569133caa97a", - "extensions": [], - "previous": "000f43ff5d5509892a75ccd57dff695ea10f2086", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:34:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "201401827b235a32563158dc4becdeaf43ac8fad44146fe1576fe6bbd83c96f959127ba1ab4ee7210554b5df3748ed7a158ab97489f7b2fc6d72ee1f12a88f6f74" - }, - { - "block_id": "000f44013052226253c1ff3cba72849bf10cf9ee", - "extensions": [], - "previous": "000f4400ae1b6faf4f59aaeff0d4569133caa97a", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:34:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f2d346608435afe19aa9ab1ba93ee7eab28d9ad4075c18fb6e21419f63c8e665b05b2417fb88a5393b4003b5cc699446a210059758f0a0ab9171812d7971b3956" - }, - { - "block_id": "000f44022c85c6998519e92dfbede37506f5abd2", - "extensions": [], - "previous": "000f44013052226253c1ff3cba72849bf10cf9ee", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:34:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "202f2255137a07d5add7bb0c12dcc473effb3c1760a28dd4b2c1747e964a6fc9c751a49bf35ed58321329a442059604823c9e40815353b1d24a36d806652787857" - }, - { - "block_id": "000f44033dcdb7bc45c5097b0a5a00f53abbf03b", - "extensions": [], - "previous": "000f44022c85c6998519e92dfbede37506f5abd2", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:34:39", - "transaction_ids": [ - "1629669fe614bcfdce41a0067d937248f1af1fa5" - ], - "transaction_merkle_root": "a9d595e4ba2a4c887731b327c454060c93de89a2", - "transactions": [ - { - "expiration": "2016-04-29T05:34:36", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f44022c85c6998519e92dfbede37506f5abd2", - "nonce": "911740918991173438", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "6df435876be8a6d6711692a0fb97966e7e606b41fbfada11747e14865251a7a4", - "signature": "20af5f7c2cba313b49a5a834287bbce8f04677d4a878f2325b9ac39407cd09aea45c7ad0bd0ddc6229a62cc7458232b9adb2e8cdff99235ff0598c13afcd742163", - "work": "000000198e1568a1dff0d22696e3efd0b0a0ac2912a2d536a2e347a49122ffc5", - "worker": "STM79MBtZRHzawavWSBSVYNRAy7WkwM2PMPDEnoWH547WLzXP5CkP" - }, - "worker_account": "homer" - } - } - ], - "ref_block_num": 17410, - "ref_block_prefix": 2579924268, - "signatures": [] - } - ], - "witness": "arhag", - "witness_signature": "1f5ed0f583380d472cd5c4d55e246131dad5773d7287ba171d8575613822668a4f76c848cf7b5bc489c5e79b8b58f24e94974d34be38ff62957a70f9ebd7e7e38a" - }, - { - "block_id": "000f44041ce4c16abf6d15db20b7da1663a3da3c", - "extensions": [], - "previous": "000f44033dcdb7bc45c5097b0a5a00f53abbf03b", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:34:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f24a225eec7ecd3f35f6eac694bb1805494772a1041d1c08e6a9ea673b92761230b05b2c271291b24536883adcccb95873d1ba7c6677f7d0545475fb6de97bafe" - }, - { - "block_id": "000f440531cd71649c926909059cd5711fc7d97f", - "extensions": [], - "previous": "000f44041ce4c16abf6d15db20b7da1663a3da3c", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:34:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f2e6086cc80fb5d700642fe261d877c763066508cd124129223b515362f66119a42f3e0c5467af8bec588318d1c47a94419b5f6527301298f38c8c20b7639ad8f" - }, - { - "block_id": "000f4406db3bdfd34f15b2bcef25f7e165ef5f55", - "extensions": [], - "previous": "000f440531cd71649c926909059cd5711fc7d97f", - "signing_key": "STM8NcmR4vQ5x2x7MBBF3wGqvqarEceCJnCMHBNd2XahZe2TFWGMR", - "timestamp": "2016-04-29T04:34:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abdul", - "witness_signature": "200ab0e1395dca5fb2a228b013dc80786997e387770bc97fe82ff3f516fb5bbb6430e55ca83d2e945c7a68bd1e6fb016c5ee6c5aa56e5c0bfcf5374227c8f20506" - }, - { - "block_id": "000f440704a803b101a23008d39a99b719e5b278", - "extensions": [], - "previous": "000f4406db3bdfd34f15b2bcef25f7e165ef5f55", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:34:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "2079e3cc531e2787a1281c63cf062778bc1f4dcaa29299803ab695c476e26703f35390c98c0b0976d8eb2a589a408fdfb24ee8324ee29b3bf7650424eaeb78494f" - }, - { - "block_id": "000f44080f0adebad6f3ec7136c62c78a2dfb415", - "extensions": [], - "previous": "000f440704a803b101a23008d39a99b719e5b278", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:34:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f18c6698d9f2fe6eac8fcb1933431015ceba9b34213b59d8e7433be6ce331f0f421aa5b237e7bb744e5c69661c7a8ede0d88a34680a3971a17a92c9c5bd92f7ca" - }, - { - "block_id": "000f44092e3e97a5ac4b59282342ad7601f81cbb", - "extensions": [], - "previous": "000f44080f0adebad6f3ec7136c62c78a2dfb415", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:34:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "203beca4192169726a7ad5829a7d4f7dc3d4f6fdeee6e6c62905465ce382f7d07f0d5e2d755eefd2f38ca1c8e6b596a19b3f0e7773b1a7903c929deed54859868f" - }, - { - "block_id": "000f440aa006803606c9d7127247701f0b20730c", - "extensions": [], - "previous": "000f44092e3e97a5ac4b59282342ad7601f81cbb", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:35:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f1a961e63a43f029146c82e2a3e1621e35715bb216e2f5389433f93a0f3e7683232cff4466ad50003e63505f4236db8342a6e30144172e42aba3edd3b475e5ab8" - }, - { - "block_id": "000f440b30ee1319d935703c1f6cdd69e98767ea", - "extensions": [], - "previous": "000f440aa006803606c9d7127247701f0b20730c", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:35:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f011e8e005f8eb4c8e8a9ed3fc47917c2bc301a783f794d3f539fa983b14d37602556dd2173714739477c3470a82704630db6dae2d3ce872f5cd74b62f00ce367" - }, - { - "block_id": "000f440cfd577e621c3878295da7781c81b2a7ba", - "extensions": [], - "previous": "000f440b30ee1319d935703c1f6cdd69e98767ea", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:35:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f13e1e4a7efe9909aed18a2ceb796611fb2f5bf751f6b459d1043f3c9b83e17ae07334b1d68ac010586db365792a61e7f6eb6d774805bae0471628bee5c93bd26" - }, - { - "block_id": "000f440d95808bb25b9303687c44749049ada67a", - "extensions": [], - "previous": "000f440cfd577e621c3878295da7781c81b2a7ba", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:35:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f3489222846bac8739f498637b535c985231c7ffd6d966b07a804ab647b5756b630d1b1358e0f8c7eda3f97685702a1b43510a154ecfc88f5a0e9a80faa8d035e" - }, - { - "block_id": "000f440eb33785db854588b0d77abf542e0a25b0", - "extensions": [], - "previous": "000f440d95808bb25b9303687c44749049ada67a", - "signing_key": "STM73XgqmDfLgXVF5VArK9rvHbUDbbx3HtfCU81Fy9MqVNxLYMpay", - "timestamp": "2016-04-29T04:35:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "jabbasteem", - "witness_signature": "203440b8a431784adb1338a4dbc85f9c610ed0649ab4d59fbe3321b9f6e7a098ae7c34f7ab4a703207af7db017d4f2c5b26c393e3c0e8c0bb08990c84ca8b00c6e" - }, - { - "block_id": "000f440fd4b309b8ecc63b99adf120c14af30b08", - "extensions": [], - "previous": "000f440eb33785db854588b0d77abf542e0a25b0", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:35:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "204639e2f14dffc56a7e7e4e140c444047052d5053b985f617062afa5afe8968f8130283884cf52c348d14365996f45e41cf88ce3e578483de35193825bda72059" - }, - { - "block_id": "000f441042001df29e95b3afa1334a72a6c8ad73", - "extensions": [], - "previous": "000f440fd4b309b8ecc63b99adf120c14af30b08", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:35:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f3d0a38a90566b3556f386a950441fd7dda976bd3d050c41b198ceb77e797ce87216b966fd7775e200fca4d8204e75bc95150bb41a6720a1dcfa77591c058b389" - }, - { - "block_id": "000f4411f68371c743ec834fdfd83c2631239ead", - "extensions": [], - "previous": "000f441042001df29e95b3afa1334a72a6c8ad73", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:35:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f52644e44d36c9ee6cf530b0699a8505245d0a50098a3712d1096da6343373bf8203affc50fb264482ed4ee2f4c63c8e90d06d4aba3bb2ffd74e200807e643584" - }, - { - "block_id": "000f4412e0a037fde52ff5d3494547d579544a0c", - "extensions": [], - "previous": "000f4411f68371c743ec834fdfd83c2631239ead", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:35:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "2025f31c37cf1bc817a16ccecb89f2f15df5187ba86dc6c3f3425ee717fd7b90562e17affd236df441fb6741686bb73ca97054e5d258ebd9ac2cdba41f5d9a5c15" - }, - { - "block_id": "000f4413283c74cdbcb29d23a671c1e5c2ad7d41", - "extensions": [], - "previous": "000f4412e0a037fde52ff5d3494547d579544a0c", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:35:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "2004ac71e0eedf37f8c73ad82e66d67c712af3c22f87556a88f7802a53a3d77c4462d58bcf6c8f25f11a580fcda2eac1de16928a3e941fc38e51d1ffa9bbdb6758" - }, - { - "block_id": "000f4414fa83495516d8324ac3f26ed6a1eba3b9", - "extensions": [], - "previous": "000f4413283c74cdbcb29d23a671c1e5c2ad7d41", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:35:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "200cb750d70ce303f1d376b49169a998153d5ad1c90bc7963bd425e67a4bb3ba8a52fbfd1c3d88acae9a6a71eac633622562c6360395a591910f91d97d8a08b87a" - }, - { - "block_id": "000f4415f046202c4ab9020eedae5e419fd624d2", - "extensions": [], - "previous": "000f4414fa83495516d8324ac3f26ed6a1eba3b9", - "signing_key": "STM5jPLUBiq7xf3hGLa7ckKgVHkgLbeeUd6u8H1nxB5LUotwxSmKd", - "timestamp": "2016-04-29T04:35:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth-m01", - "witness_signature": "1f7afa983173e6e884ee20841c3ab501a252f09ce2b7d480cc1bee55ae69285df67f86483bd78819d9ae7102ee80778cfc1abbb748f1fdecab6c14f31560a19c34" - }, - { - "block_id": "000f4416a3c714459b07b1b4f66599cd94a91f7d", - "extensions": [], - "previous": "000f4415f046202c4ab9020eedae5e419fd624d2", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:35:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "206f4366c60cbaf3fe3bfe6989b0c5d5a9027e56ded3c365540df5d360d93700b01d1d13d1ac1aa295cf5c95ab562f6595b45ed996edc9ee25d52a5ef2c40a2d84" - }, - { - "block_id": "000f4417e8a8f9bd0009da0617b4ddd2914442c1", - "extensions": [], - "previous": "000f4416a3c714459b07b1b4f66599cd94a91f7d", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:35:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f52f754e944e31403c7a5f6c152d4886c9b5b166e80e22f7878274dd5925e467f70327a80efc7308daaee19c999dfdedb0ce3c9a5cc6b364fc107b1f0e6e92ffd" - }, - { - "block_id": "000f4418c92c93282a9e54a9dd1428ba6b09cbee", - "extensions": [], - "previous": "000f4417e8a8f9bd0009da0617b4ddd2914442c1", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:35:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f3c2fe9243c5fe8c3c998e41575904d5ee9380ac2d18376abfb99b518d3333ae5138d97f255fca247a86e6b3bbf1f6b82dd49d4f01ccbde9db704cbcd1ab7e88c" - }, - { - "block_id": "000f441916fc305d721099ad6e89dd9808a14665", - "extensions": [], - "previous": "000f4418c92c93282a9e54a9dd1428ba6b09cbee", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:35:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "20682d6509eee9d9dee92fc01e9a2f155270df84621dabdce3f488fda50ebef7d04f2bc82a9c8169bc93a96b9a26cdb5824e4c9937eb2581bf8915a0eb8d728b3e" - }, - { - "block_id": "000f441a809de5105b78a083beb71dc2e5a155dd", - "extensions": [], - "previous": "000f441916fc305d721099ad6e89dd9808a14665", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:35:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "207f9365db9de478ce9bfba04dc0a6f55dc43e019122861a37778a62c1b2ef2b75730106b3bbafa8c1a171b184bed1f7b238b5f67a5fb2296b97f3902a6b7f61ee" - }, - { - "block_id": "000f441b9f7927ac449d1e12fbf5662574e5dcf0", - "extensions": [], - "previous": "000f441a809de5105b78a083beb71dc2e5a155dd", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:35:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "2038d6ed43e945a72f6c9bc024722da5ab24e6ad0aeae198c796d5bf2b2816be65452d0c8cafdeaee3a5fb1f25853a421af4c4070450f4ce9953f39dac67ce404e" - }, - { - "block_id": "000f441cc8086a6ec1286e361762ab3e11c1e6e5", - "extensions": [], - "previous": "000f441b9f7927ac449d1e12fbf5662574e5dcf0", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:35:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f0133cdb25fc27714f99cf40439143d3782a6733687f6fb785a9375bdcd8f3cd6539db5659305293266b8269720fb909ba8eab53d8b66b7e378d5f6193332a1ec" - }, - { - "block_id": "000f441dd7273d3ca7b185c32f7ac3bef770848c", - "extensions": [], - "previous": "000f441cc8086a6ec1286e361762ab3e11c1e6e5", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:35:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "204ec550f8f778faa862f6f71acb029fb558aad0dfb166adbd6f8c0b8f95bf84742acf7f4f957ed6ca1dcb6760c93f794eb90f32d39db5e980342b5b954b7c8626" - }, - { - "block_id": "000f441e400c6fdc1cc2abc863c8122ba8897f0f", - "extensions": [], - "previous": "000f441dd7273d3ca7b185c32f7ac3bef770848c", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:36:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f4c2ce6869ae93868c5dbd71a4854cd0196815b0a8701667c1c95842c2e6ecc531704aa3c965fe1dab7e391f08591cca22a31b0f2ee885043a2f0e0ac5d9b275e" - }, - { - "block_id": "000f441fc92a4968ab0aaea4edfee53832be6ca4", - "extensions": [], - "previous": "000f441e400c6fdc1cc2abc863c8122ba8897f0f", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:36:03", - "transaction_ids": [ - "c5c3459e9ca8c648b37c7334d905b43aa1ea9e80" - ], - "transaction_merkle_root": "d5029bae209b60423fb7dde2a4d3c5ee45440347", - "transactions": [ - { - "expiration": "2016-04-29T05:36:00", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f441e400c6fdc1cc2abc863c8122ba8897f0f", - "nonce": "15658925604317763207", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "0b0304c9916e576b03f36e81593498fea9f9f12767f9f7eb3fdc23275c705215", - "signature": "1f87e4501064aa0e0ffd9ffab462494959c67fb324e3ed48160e7a6c06736681394879aefb0f19f55ed6273dac21286794098b2b0db13c12f84f6bb72d2760d886", - "work": "00000017fe7b031fd48f5dee24ff02267bed77796f0dfe6bed9a8d92012ca5ad", - "worker": "STM8io6LPUoB89b9iwBh1qoN9nKQSmigbq97VvufoSWhqZkc12nLW" - }, - "worker_account": "bitcoin2016" - } - } - ], - "ref_block_num": 17438, - "ref_block_prefix": 3698265152, - "signatures": [] - } - ], - "witness": "complexring", - "witness_signature": "1f743e1a5bfd5309588dc79b5f51f59d667b94ae2ca99edc22f8d12577c600b1ff0b9e2b7569ca3d9ef37600b82ec61126b1f8691f45db5a0377478147baccca6b" - }, - { - "block_id": "000f4420d4af279093da4a52c8900c48540a6497", - "extensions": [], - "previous": "000f441fc92a4968ab0aaea4edfee53832be6ca4", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:36:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "20771d9592560c0c996f6c03b4d560b412315469ba910f5d0f785bcd97cf8be8127ab17cdd8297d1a7e647b65699051fe46e72b80fc7b5199956ba1c9429e89303" - }, - { - "block_id": "000f4421e0b474f8fef5f6e0bc0f55391661f246", - "extensions": [], - "previous": "000f4420d4af279093da4a52c8900c48540a6497", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:36:09", - "transaction_ids": [ - "bbeb0a3ebf040b09c23b3ada08b512b8e76e89bf" - ], - "transaction_merkle_root": "34be93968803a9eb46a9e47ab7b3aba0c75eb7fc", - "transactions": [ - { - "expiration": "2016-04-29T04:36:36", - "extensions": [], - "operations": [ - { - "type": "account_witness_proxy_operation", - "value": { - "account": "smooth-m01", - "proxy": "smooth.witness" - } - } - ], - "ref_block_num": 17440, - "ref_block_prefix": 2418520020, - "signatures": [ - "1f0c36274882fc696d604bb7459f871ef2b21693b6fff02a2db078b70bf72809c6372f856a58a44e6769dced374f44427583c605bde1bc56b19ff7888a6d533640" - ] - } - ], - "witness": "roadscape", - "witness_signature": "2027d2995536da49a08010d6f73c66d57605b0a6783c84b146d02e39c1fc2776ef7b6e606022d720fa0d1a56a2b4e54080f88499979e1b055dc1a082db8926ab17" - }, - { - "block_id": "000f4422247590820f7e43db2d5a6b21c260bc3f", - "extensions": [], - "previous": "000f4421e0b474f8fef5f6e0bc0f55391661f246", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:36:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f7d5dabbc85bff631002adbf6d9154dfe01361f37ef7065f3441cdb61eadae49e7f89f5c35eb18f7b06b39d32f64ae919aa230015f52ad29ee664ac7e0d0a864e" - }, - { - "block_id": "000f44236233189cfc6343920ca60505deaffa51", - "extensions": [], - "previous": "000f4422247590820f7e43db2d5a6b21c260bc3f", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:36:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "20613568b2a3831b90b2b08f25dd7efc22a45973e5543fa732fdcd1c12b08497b75aa305d385fd3868e1b1c44f3525773fdb1fc0b3f7974540b41d4c3b9f78b019" - }, - { - "block_id": "000f4424fe97c8cc230d750c4e026d0f8eab345c", - "extensions": [], - "previous": "000f44236233189cfc6343920ca60505deaffa51", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:36:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "206f2aeb9dc9095da015cc39822eddee68fcd4375800cefaa67070bc1334f15ffa53bea30e8922d3e32c4fbb9ad65c24f0f934dbc37a0837d64d84642e5ded95ef" - }, - { - "block_id": "000f4425c82fef95945aa6f153e1f3fc173b9239", - "extensions": [], - "previous": "000f4424fe97c8cc230d750c4e026d0f8eab345c", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:36:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f1ce7eb65dfe0ebaaec94bbcf40389c29a872b1dcedcefc498edbfb205959ee167e4d7847028538d62b5702ea9f70a3d7c4de0d27825a9c7fd7c57b544da854b9" - }, - { - "block_id": "000f4426b06dabab32b8c3a7d8fbd49eaa811d24", - "extensions": [], - "previous": "000f4425c82fef95945aa6f153e1f3fc173b9239", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:36:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "2005d858b0377171e9aff659bffb59ebba44b65fc1c070112f7911c17d7d435d1f77f6700379ce0413ffc515b64a7818d72487b51a94eed77f0953de56436a34d2" - }, - { - "block_id": "000f442793a66ed25f3e3d6bb6962a958e3aeb50", - "extensions": [], - "previous": "000f4426b06dabab32b8c3a7d8fbd49eaa811d24", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:36:27", - "transaction_ids": [ - "ce0db09d39c06c8f59a5e695ed134035a9bd64d8" - ], - "transaction_merkle_root": "45e7a768f4fd29f7de2d216efb0375dbd522072d", - "transactions": [ - { - "expiration": "2016-04-29T05:36:24", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f4426b06dabab32b8c3a7d8fbd49eaa811d24", - "nonce": "806255817238683288", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "18d342e1ad6593a411b066d06fe3ef189efb15c3eb69d06589b451bce43dedd1", - "signature": "20b6e0f2d0e1a9cb20bc791cca34418873bc5cf97518eddd7bcc87c3fac00ecbb519a3a85b754dc50b8d36598c82538ff99bcfc32ce8d79599a83000cffc2be335", - "work": "0000001dcd2c7aa43ce0ac661497de6722b6c3cac524f23e1242053eb1b6d2d7", - "worker": "STM8RVh9ZnJZhULNSQwRUueSJSKtJUjNMPbnyRdWmEJAVCTJZ5AYT" - }, - "worker_account": "hr404" - } - } - ], - "ref_block_num": 17446, - "ref_block_prefix": 2880138672, - "signatures": [] - } - ], - "witness": "abit", - "witness_signature": "206746035a3a0ef65facbc7e19ddc11ad62ab3464dd1c9020db516001c6ac8e2f249da3b00ca78f55f1b8b32ccd3ad09ab72ba5b9a6c3c0d50e4eb64b1b62c5cda" - }, - { - "block_id": "000f4428489a920fa06160574518a219d57610aa", - "extensions": [], - "previous": "000f442793a66ed25f3e3d6bb6962a958e3aeb50", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:36:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "203bfc06f8318c4e7dd9ccea2cca1336a8a82ab3c433e2a3e667875dc91d358953626b1ae166b22bc5d6dbbe1246863b832e52265c872d3cc97ff9201285126679" - }, - { - "block_id": "000f4429439285e7d1def4fc78ee2589d53c0c85", - "extensions": [], - "previous": "000f4428489a920fa06160574518a219d57610aa", - "signing_key": "STM8kQi4yD7Ho8gkwPruaGU4oKuu24Lv1qXxxVi51ByaNHnw8Eydy", - "timestamp": "2016-04-29T04:36:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pumpkin", - "witness_signature": "207145da1c32d7ce73ff11bba5c5f694b90958c978a8398fe91ea2cff1ef7bc3ae08266de96c61065b66349e4442da9a6ff5152c9905f23eca1e3c4538db3bac76" - }, - { - "block_id": "000f442a2a66066f2a6be107e3ca9daf370c467e", - "extensions": [], - "previous": "000f4429439285e7d1def4fc78ee2589d53c0c85", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:36:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f0e0e8974b8d8feb24223ad1816c54e0a53699e40d6197d9bf4b9840356cd5cef476d398045edecb32faf0917b5299c5e0a433260c6ed087e0c7e00ff04425dc2" - }, - { - "block_id": "000f442b842a78bc6bb1fa12ffa093c5c6f9d613", - "extensions": [], - "previous": "000f442a2a66066f2a6be107e3ca9daf370c467e", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:36:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f2e9d32b97864dda81baab58f22272614d560b1d8383c31918aba639db47e4cc856db2b1898dda109af45a0142e30b7b1bec8bcf7147b6475aed8b9a402f3d7fa" - }, - { - "block_id": "000f442c0b47fbd0baf55b94ff7f346603af29d2", - "extensions": [], - "previous": "000f442b842a78bc6bb1fa12ffa093c5c6f9d613", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:36:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "201eb5ce4dc1c32a0f250209a3bb2760be69b72a46c7baf27544c6853a8b6d0eee18cc32a7d86c4bee2b8ff63437576b4d5293754890b283422656a31f2100c436" - }, - { - "block_id": "000f442d22e82fc6675b90b5e6d6ccfd671deb22", - "extensions": [], - "previous": "000f442c0b47fbd0baf55b94ff7f346603af29d2", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:36:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "201552523f7fd4dc24cfc8daa99b99ea278272500deabb0480b58e76a80671c4fd4f8ec5ab1d77b9bb52ea178c4a6130626247656745bfe7868f6148cdfbba92f1" - }, - { - "block_id": "000f442ee92ee83f6a898996d9540a5e74ddbf7d", - "extensions": [], - "previous": "000f442d22e82fc6675b90b5e6d6ccfd671deb22", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:36:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "207401c545df84ceb7d30d835889adf1d4e164f01df28af38b4a5c0a69826c3e7549ad033becd9cee44901c7b8bd8a96e75046b9eeeb5a6fba7fb5b6b0896b9456" - }, - { - "block_id": "000f442f68e46f1d3593812f5ca3e2fbdfc18ba5", - "extensions": [], - "previous": "000f442ee92ee83f6a898996d9540a5e74ddbf7d", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:36:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f11d6992052e5ecac5fe7e119e151822202bc47b9dd846c69665abe2533e1c06d5e8ccc58ca24984b57ae68456b3f0f989ecab669fc7e24606e9663d883106ac3" - }, - { - "block_id": "000f443067188df978c8b2d36a93e43230f5c646", - "extensions": [], - "previous": "000f442f68e46f1d3593812f5ca3e2fbdfc18ba5", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:36:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "205fa83af4fa848370a32076542daee5fab3e9ddaac62891a740115018a04470352afde437e183d3d55750441c4744302712e3ad1188affcb9a72c9aa4550818c5" - }, - { - "block_id": "000f443100665e8a5cb5595767446898b9893818", - "extensions": [], - "previous": "000f443067188df978c8b2d36a93e43230f5c646", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:36:57", - "transaction_ids": [ - "ffc77551230efcf92e3bda9d4e5023b3c4eb102c" - ], - "transaction_merkle_root": "15629057d549d7403348a173a3305e257b42dd73", - "transactions": [ - { - "expiration": "2016-04-29T05:36:54", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f443067188df978c8b2d36a93e43230f5c646", - "nonce": "17063973574701914533", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "51a1644d9b661778edd5157d823a77b7471cc4e3d2e9fb4e662b309504b2baee", - "signature": "1f000270353a6fd35d4d498653f5d1e4cf6b5ae2b2e1b2fb7deebbfbe9487089bd25e87cfbef2d1e515e3740c114bddf68d801296ae47274085bacb2d089a3ca8e", - "work": "00000009d3e2c2db25a842cc2e23141f390c19b83d88f9b29893a05f78462f1b", - "worker": "STM6emoLSjgfeKDoPPGm2HRe6uotTdHA1gkYYhjVZWFzPaq6x1aVB" - }, - "worker_account": "smooth-m00" - } - } - ], - "ref_block_num": 17456, - "ref_block_prefix": 4186773607, - "signatures": [] - } - ], - "witness": "kushed", - "witness_signature": "2062ba46a254cfe36fa4abc73b3404215a3b7e58848ffff95563c259925c6863db37a5df1273800309bf5ec4349f2a11c423d93ef855c8d1e43a5807c13e5f4adc" - }, - { - "block_id": "000f44328e2ec20f06850f69067db1f5d597d185", - "extensions": [], - "previous": "000f443100665e8a5cb5595767446898b9893818", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:37:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f1c2163bd06cef46c4cc9472500d28637862aa9a62ef6a714c9ae787630564af3641d26035996c8d178aa490869e06fa7bd7061ff23e7973573fbf05a2c2316b3" - }, - { - "block_id": "000f4433153c203fd540239c57680c03e1cdf896", - "extensions": [], - "previous": "000f44328e2ec20f06850f69067db1f5d597d185", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:37:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f6a70ba63e6534e3cae10d4621ee253d4abea897e2180eeffabe42d1363ce84a82bff50f0059bb84280b185fcf9b9f4d757ab10d012f83d13d05c6931316b59e1" - }, - { - "block_id": "000f443485364553475800abb52869d8a6fc8180", - "extensions": [], - "previous": "000f4433153c203fd540239c57680c03e1cdf896", - "signing_key": "STM6ChCh8ryiuwfbzFfViZvh6t6gAZzSg8BubWQbqUBviHCSefFkd", - "timestamp": "2016-04-29T04:37:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr701", - "witness_signature": "1f3545a9683e4c89ef5121c498d0510ba1a95a8eb38d81e22c65dfb80b231d503d6e5b2b121c3bf723981ce0a8a49ca1af992607db7db225558345f1d46c874e7c" - }, - { - "block_id": "000f44352009bbdaa9601aa77a981d2af5fca3a0", - "extensions": [], - "previous": "000f443485364553475800abb52869d8a6fc8180", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:37:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "206caabfe7ddb6cdca30b7956162ee68e91b7740a411283815dc421b047af61cb62044903d7d6d8366ca366b4a8d2282787601475bb4cb1aa7f67186356649d6c5" - }, - { - "block_id": "000f44361054e067ea4eac66d2e75e35600d9caf", - "extensions": [], - "previous": "000f44352009bbdaa9601aa77a981d2af5fca3a0", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:37:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "202bbcdc72844ea1130c16b2ff32298c5cef96f3428d9e8d7881628d6305d510fc328d9403edc3a756da9d5b5cbcbad4d9b41478ef376b4cd47daea04d762a9cce" - }, - { - "block_id": "000f4437e1c4bc0d82d14eb3ac66767b92eb0b1a", - "extensions": [], - "previous": "000f44361054e067ea4eac66d2e75e35600d9caf", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:37:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f17992561fccd93d74ef4c339a83a227b3110cb4774a08403a8655496528733452bd94503336ea8689c0a93a73b11bdfddce06c6d9c5063b46c8d8a1a5dc9792b" - }, - { - "block_id": "000f44387a5619ef5558aefb8e88bc4135a77593", - "extensions": [], - "previous": "000f4437e1c4bc0d82d14eb3ac66767b92eb0b1a", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:37:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "204e353c16c69d79749d58098beefa68c4814f5aaa3752e48ff94fef83554a36b45e78636b5168b917cdf2f24410d1ad7d0ce7e0b0654a45a900c934b643a2e211" - }, - { - "block_id": "000f4439c69dcb4292d90b8371835e91d2780121", - "extensions": [], - "previous": "000f44387a5619ef5558aefb8e88bc4135a77593", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:37:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f63f30ae2bf94a3ef8f6ba36f849d74b4f9a1af6d7182beea329d4a580dd85b9729bdc4193d773f02db99306783189d4973f615b6d0d651735f5fcbc37cc77d54" - }, - { - "block_id": "000f443a8da79f453c5c9cb88c9170b16c37a42e", - "extensions": [], - "previous": "000f4439c69dcb4292d90b8371835e91d2780121", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:37:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f3b91453c3ef365d21c77ab7ed208dd5c20f82d5ca18b0a2f9e8c0ea412bf2c4b702275ba78c93d33a7eafceacba3b4a24513237950b6fdfcb3d6e82b032d91b8" - }, - { - "block_id": "000f443b95c52b5af61594e491bac7cc988fde4f", - "extensions": [], - "previous": "000f443a8da79f453c5c9cb88c9170b16c37a42e", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:37:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "20565e8380688917d8d313c4c15b7614c3a650c1ec10e143de7a35c1b920bfa6f57f07cdfd8d6e6f0bff15349261de7e1e05b5940ac9ceaaaf4f7966ef602e0b38" - }, - { - "block_id": "000f443cdcaf767b60339e6dea99a2934be55000", - "extensions": [], - "previous": "000f443b95c52b5af61594e491bac7cc988fde4f", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:37:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f7e33e3c66596188337898cbed0ad2b774bed31cabc001ffafbdc369385e6c3a938008078fd594c78124a779ddaef4d1d2639f9f001439f4a8d0284f294f717f5" - }, - { - "block_id": "000f443d6d131b04d80bca1a21901e2f2eb450d5", - "extensions": [], - "previous": "000f443cdcaf767b60339e6dea99a2934be55000", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:37:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f75306af59fb2ec53983e6c4c76bfe145a827c4b86feffe4689a75acc4906eacd124d7bab9bc92cb417357e514a15e9067118002ec9ea519fa40d0b76e7b556e7" - }, - { - "block_id": "000f443e5c161b82e9cca26f0f2e7fbc1cee7617", - "extensions": [], - "previous": "000f443d6d131b04d80bca1a21901e2f2eb450d5", - "signing_key": "STM8KTqm5g3i7wRTpwPsDdxUTNBa7CTJUtjuX7pAWUELBZjBQfwK2", - "timestamp": "2016-04-29T04:37:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dario", - "witness_signature": "201530d492682592645ae04feae1c100b22b3ca63e474ea0c922c1c8c09ae676784f8c08bf52dcc2ef3a4106ada1d10934be64d1ac65a70ee44ad74211936b3543" - }, - { - "block_id": "000f443f7186c43f4d5f7f8c1b00b994a067fee1", - "extensions": [], - "previous": "000f443e5c161b82e9cca26f0f2e7fbc1cee7617", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:37:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "2019908d234f846529beebbdab24bea05f6685c088ca3d970992c4777fae82de97403a199828c3201e029d3a596fb9705b37af4935503fed8c48301543be627fae" - }, - { - "block_id": "000f444008f7629cb3ade59a30066db045d38a5c", - "extensions": [], - "previous": "000f443f7186c43f4d5f7f8c1b00b994a067fee1", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:37:42", - "transaction_ids": [ - "60b552ee7447378ed8f92e6ec6b2689f2569babd" - ], - "transaction_merkle_root": "9749f226ac8562fbdf19df84c3ac7f4fa219424f", - "transactions": [ - { - "expiration": "2016-04-29T04:38:09", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "441", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "joseph" - } - } - ], - "ref_block_num": 17471, - "ref_block_prefix": 1069844081, - "signatures": [ - "1f4546b0a29059e52a69d4411289d2dbdd8e832df1b2c2086f9c702ed5ce5024700439fabd65669a2f0e7ddeffd01a3bc2530454bddae60a4f1c57068af1ce67f5" - ] - } - ], - "witness": "abit", - "witness_signature": "205075861a38616b56d7498d28ae302902dcfab620fe40ccfc1f0d54c22f2383a8420acc38406b0b18ea29c063f23f9e8c41fc3e050c6b3f9b5c048bacb44d1622" - }, - { - "block_id": "000f44417fe647c9171290c79ff6a92da4453c56", - "extensions": [], - "previous": "000f444008f7629cb3ade59a30066db045d38a5c", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:37:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "2042f1d601e99e30af35c6a1c2681c84830b949410394eff2fa819bc903c5d7acf5bc6923a0a1117ec81176a8da933dba2f642de377ef50a4f4a46f497865e2095" - }, - { - "block_id": "000f4442005d9b9f8169b670a8624f83aff6e006", - "extensions": [], - "previous": "000f44417fe647c9171290c79ff6a92da4453c56", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:37:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f246089df41cb70f2d7f0a99f27f661e7d2750340364a5b34ecdbcc3a5c5790042ae831d73813a10fcb743ace140eba68327d3840320c0d12d1faf295c4d0add7" - }, - { - "block_id": "000f44438b3515ee5425b719c1f218471ead2be8", - "extensions": [], - "previous": "000f4442005d9b9f8169b670a8624f83aff6e006", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:37:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f396a0caf785ad37a0724936728bddec3e6c1986374b2cb489005d1b3f74ddc3753eef8e39f1b8b5a00eec7fb6288d2a662275885833ce5e3dc3642a70f846c1a" - }, - { - "block_id": "000f44440fda9296f958fc509385f5cfb61de18f", - "extensions": [], - "previous": "000f44438b3515ee5425b719c1f218471ead2be8", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:37:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "200da2834e57267b2ec6ae0d5eaf8fa3f68ebb8284b1b96faec4f840ec948c23632827152ab4ea8dcc4050b854767b3f03a5ee7c51d346bd1a85ba7607b81bf588" - }, - { - "block_id": "000f444559094227a2d2dc996e0707f3ff82cc44", - "extensions": [], - "previous": "000f44440fda9296f958fc509385f5cfb61de18f", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:37:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f4d37d08923ba0c85adb796092b38417b4a096c689c7a9dd9de86ca2bd2ad7b945a3f6249d732021f4d5d1ccd3dbab295cf330f9b1162b5c772fbf12e0ed08383" - }, - { - "block_id": "000f4446ebb1626ea9ab3901f59c3ff70234d786", - "extensions": [], - "previous": "000f444559094227a2d2dc996e0707f3ff82cc44", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:38:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f667bd02f18d4b649d1ab4ae22b0789fb60628553242aa89a4228cd9571706c8e73b9b4670fe70e5e4135259ad9e34bea3e54d7085f1dba6e291e4faeb5820016" - }, - { - "block_id": "000f44473397af3811a3cd9e0e9423cef9200adf", - "extensions": [], - "previous": "000f4446ebb1626ea9ab3901f59c3ff70234d786", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:38:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "203e68e162b5ee20a6fc1bbd5af1fc3d336108cf766f34fb866b6d1db1205827f42cadaac73273fdfb5c62f8b0a5217fc31798b3ebfe6940eede6b9df98c76a03b" - }, - { - "block_id": "000f4448d09f5735aad022230c6e1261ae2c9153", - "extensions": [], - "previous": "000f44473397af3811a3cd9e0e9423cef9200adf", - "signing_key": "STM6kzHDuHQYGKGUUoPdhmPcHgFFjpq4uycnLK5mUBPPqqn6qpiaN", - "timestamp": "2016-04-29T04:38:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "boatymcboatface", - "witness_signature": "2013ec9b4ea74ab7560f9b1656de75bdb220d1f6ede68204c8f1b52ff94db15f5131592b7f7f67ca72637149d64d75763a7c8f5c7a8b1eba63ea16649d1037d387" - }, - { - "block_id": "000f4449768d51d4ba404804cde33a52c262fdf9", - "extensions": [], - "previous": "000f4448d09f5735aad022230c6e1261ae2c9153", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:38:09", - "transaction_ids": [ - "3334ac945ef6f512c3e476c9b844fe503e68848d" - ], - "transaction_merkle_root": "60ce717fe9d9b9d9436af97a9e3c788b5080f833", - "transactions": [ - { - "expiration": "2016-04-29T04:38:21", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "dantheman", - "permlink": "re-tuck-fheman-how-is-voting-power-determined-in-steem-20160428t213727572z", - "voter": "clayop", - "weight": 10000 - } - } - ], - "ref_block_num": 17480, - "ref_block_prefix": 894934992, - "signatures": [ - "2012da25d7ca2c5766a3699b338590ba1b1974367234a5b16a0c170147a0b1a98942837438fcc45b279a9e8be6bb2afb1fdba03323725f35f8cc0d7a82fb65c2be" - ] - } - ], - "witness": "steempty", - "witness_signature": "20065d7d064719d777f83f9ff04b74d2dc0fe7cd949ba495120edbf351a20c021e7d9182049302605d14c78de3aa43f68375bbd60f1bc310791a212493537259c5" - }, - { - "block_id": "000f444a3582935b9bec81ff7cee636b346be060", - "extensions": [], - "previous": "000f4449768d51d4ba404804cde33a52c262fdf9", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:38:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f63acbd67e6a4d8fbe3b20310497e86b966b91ce1a16106ff364528f1417a42295f74aea9c52f27e448d4990063e80182086e53bf88f58b7bb186092ca3e700f9" - }, - { - "block_id": "000f444b0666ac91fbadb5ea798115e115f89dee", - "extensions": [], - "previous": "000f444a3582935b9bec81ff7cee636b346be060", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:38:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f04c60794773e229109056278b5387ac90d93c19e4186c4fee523d48c56ab09703dbac6444ad91656a75c975a5247d163e0a1d27ee64c3b61b61618ace0dc78f3" - }, - { - "block_id": "000f444ce45ee8b2b33dc761205f6a58cf25e10b", - "extensions": [], - "previous": "000f444b0666ac91fbadb5ea798115e115f89dee", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:38:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f70b2421265d3bfa913c4be38b570bd8df2e7706a5002f93716edfa7ee52a554550179b0bbdc65b8cf7971bc4945bcf12c6837b8050fa6ee223efdd6b72548c3a" - }, - { - "block_id": "000f444db9bc06805e4d7f77459757937b64754d", - "extensions": [], - "previous": "000f444ce45ee8b2b33dc761205f6a58cf25e10b", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:38:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "2042867290e06a71735b177b88b93b110216100d3cdb61df4b461b764438c7448568b27d91b6072ddeae936fecd7cf49fd720564e68c1fea6a5ed889165eee8455" - }, - { - "block_id": "000f444e476dca3de27d06b2b85f6615353e04bb", - "extensions": [], - "previous": "000f444db9bc06805e4d7f77459757937b64754d", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:38:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "2057043f408b688b90133b90bdf5809cb9840c188e91663b2076d03d262bfae60f704bc3205b4102af0350379ad692ed4beee574219e94515d2b014b1e1554c98e" - }, - { - "block_id": "000f444f0441f277b3016a1ec1401dba7674359f", - "extensions": [], - "previous": "000f444e476dca3de27d06b2b85f6615353e04bb", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:38:27", - "transaction_ids": [ - "0c928d159c53afb4f73267023bd6fa8a6804e864" - ], - "transaction_merkle_root": "68a7bb571a0865e58aa92dff71611edad09cdbb5", - "transactions": [ - { - "expiration": "2016-04-29T04:38:54", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "444", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "steem-id" - } - } - ], - "ref_block_num": 17486, - "ref_block_prefix": 1036676423, - "signatures": [ - "1f3b4b455b3f53e821d5b4c52bfef23edac791d8d7e548c9458c5bc170bf21de4407625b3d83a75dcff4009e042568ce8ad24c6b2a57a20e313f42d7452c130f7a" - ] - } - ], - "witness": "au1nethyb1", - "witness_signature": "1f42ef69efe850a0a519272c16485e781487cd3e13f877a7fb7e9bf79f179308653740e34f8cd94ef8fc5256b867bcaa05954a76b9ca908dd6bb85040be96d2fc3" - }, - { - "block_id": "000f4450e5b0d068e0a6e247569346f6716f78bc", - "extensions": [], - "previous": "000f444f0441f277b3016a1ec1401dba7674359f", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:38:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f578ca8715f6bb993e47fd22040a4a1a530b6beeb26a13cc954ad03a922c6e5d96db216e883f6a067d39a0ee6b9831ca78e645b4d376a26df1a880b9a7c88b833" - }, - { - "block_id": "000f4451f5c5b4c500e12e1cd75bfac575d28f5f", - "extensions": [], - "previous": "000f4450e5b0d068e0a6e247569346f6716f78bc", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:38:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f22c1499812c35e03a5a02b6a9ab441b24d0a4f141117cac3e8b1abe89ec142e412d01aed01ce36906cdd693e2751719c66bfee85535e6d72b30b4a47dce0bfa1" - }, - { - "block_id": "000f44526b92ce051104f2a0667d051b0f181b0b", - "extensions": [], - "previous": "000f4451f5c5b4c500e12e1cd75bfac575d28f5f", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:38:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "202b291c1154942db3be323fb6b1fe924d927dfd1342bad282d41daf483651e78b589762809e4cdd5a01373b26e3e0e41b49d983d275e9520092c76fb3d78beacf" - }, - { - "block_id": "000f44530d0b25102574ba09d6ee60313baae9c3", - "extensions": [], - "previous": "000f44526b92ce051104f2a0667d051b0f181b0b", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:38:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "203c2225373a7b962c6ec25c2793474081b5eaecd629615237eb0381026bcfdd6b57dd29fe8d0179871dfd37cf7ad252b2f10e00fc9b1e9b38d3fcbc96be143326" - }, - { - "block_id": "000f4454d48dd2f885ee80c396c13e17e8e007cc", - "extensions": [], - "previous": "000f44530d0b25102574ba09d6ee60313baae9c3", - "signing_key": "STM6f1wh7wNG6t7KbPuRc2AvXPH3AE3erkGRg7TnqiAd2iEAdQ56p", - "timestamp": "2016-04-29T04:38:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "testzcrypto", - "witness_signature": "202ee34b786eb8877771568807e453c422af7fbef4d688ac3daf439c4ee8a230910d3bcedeee391361553056ce90bf18d6c81139e3904d7a8fd1c46a21ed29d517" - }, - { - "block_id": "000f4455a8620b12d22934bae6947a9e2feb1f38", - "extensions": [], - "previous": "000f4454d48dd2f885ee80c396c13e17e8e007cc", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:38:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f6169d5ba8acafff935b2128cd0f174b65f5bc3beed25dc6f60964aec967ed90878da4e3d21c1166fe74301b5a4533534342893764ddba26ffab32c3d36c5e9e0" - }, - { - "block_id": "000f445695b6accc102a95cd90242be9f1cad3b7", - "extensions": [], - "previous": "000f4455a8620b12d22934bae6947a9e2feb1f38", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:38:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f0c78886a5542d0b3a87c27e8915437a69e928129975933c0c6987a7b398e7d6c5a9c01750833e5bd073fc846f13700f022b4dba0cfd4d8f1aa3b0f2d32508797" - }, - { - "block_id": "000f44572f8c9edcb55f5b99ee4dd6015f3b1770", - "extensions": [], - "previous": "000f445695b6accc102a95cd90242be9f1cad3b7", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:38:51", - "transaction_ids": [ - "607efe482fd535dc6680d5a4fd5da88385be9493" - ], - "transaction_merkle_root": "0555c04d6503c0577d9345ca44d42dc5af6eb8e1", - "transactions": [ - { - "expiration": "2016-04-29T05:38:48", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f445695b6accc102a95cd90242be9f1cad3b7", - "nonce": "11994519578494274919", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "d376114b1c5755cba07c068cbdae4381415cb5d5a74124474e7a2a64a967a30e", - "signature": "1f2a7eeb5abceebf92831df0d52b0befcb9b3663f96dc24fc2c1950f3e1a9573a857983cf0a4e3913c8fb9ef50a571ebb825f2b907985253b08c545299da7393d8", - "work": "0000000925b13151a4d13ac6d561da9bcab53050f857af197343467f426dab08", - "worker": "STM4yQG8BiozEtiBErWJG9MhGipmkAT6Bp7cWDnwSZkyUnMciVUyt" - }, - "worker_account": "hr103" - } - } - ], - "ref_block_num": 17494, - "ref_block_prefix": 3433870997, - "signatures": [] - } - ], - "witness": "steemychicken1", - "witness_signature": "2010e9743b028cc39fd4eff6be34e9ea0694d188ffce62b237bbf6019a6ea5952c5dc4a3ab190d9663fb5b6fd21bf13c2d5c1ad22709800d6943f2deae4d88f8d1" - }, - { - "block_id": "000f4458aa5fd73f6580921329d400a4c5bdcfd8", - "extensions": [], - "previous": "000f44572f8c9edcb55f5b99ee4dd6015f3b1770", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:38:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f249534cdd07bfa22219b5a2ea78b7eb430c81316f1464d6b9a9df33af3adfff972db2792b16058c35e8e65af0a1c79f062d37e02463eb0f7c9a4095944ec9f09" - }, - { - "block_id": "000f4459d8836895e956af7e4f8b4a6d6feda4dd", - "extensions": [], - "previous": "000f4458aa5fd73f6580921329d400a4c5bdcfd8", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:38:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f1b00b21260d86e156722ca0ddf5254c4e0da414737356703433adc83c396cfc95e299b37e454655cff274285ce92008ac56e45cb07ca035f5ed24f44ac3833ea" - }, - { - "block_id": "000f445ad5e10ff16b26ec78dd58ebe409853ff2", - "extensions": [], - "previous": "000f4459d8836895e956af7e4f8b4a6d6feda4dd", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:39:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f4f336c9544a9eab730d91398909edc67007acaef912d8e9a5a507ca3007052f07f47d512fa946e36faf7c772cab94789c24ed16ac27ad7b7ed6740e443c94e71" - }, - { - "block_id": "000f445bff091ed6fd704e2736c0f522a25bc882", - "extensions": [], - "previous": "000f445ad5e10ff16b26ec78dd58ebe409853ff2", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:39:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "201e34e79179ff0e2c0d08a79a206bf16ab6cf40da947fe41c999c72fc97001ee413ca8c59cf08d489db8c04f1e56145b11182488c18ccd71087a1443f15bfd184" - }, - { - "block_id": "000f445c1d9ab1381169abbc56e011c39a276e6c", - "extensions": [], - "previous": "000f445bff091ed6fd704e2736c0f522a25bc882", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:39:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f5eef49f43c21a2faec097b33ff3b267ee51ff02eb60b338fd592dca8ff3ff2782162c347aa4d467fef32bd018422a3548c87df44b349f6d21d837b4ab0a54b5c" - }, - { - "block_id": "000f445d47ff8adc82e21544c4e2de8f219e7195", - "extensions": [], - "previous": "000f445c1d9ab1381169abbc56e011c39a276e6c", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:39:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "2078ce85026e497bd835dd6addbf69758b24209e5bfa7fd7b786d99bec3118febc7fc89063364998d723da5ef6fbb57ee1d7026b9feee667b5eaaaef34c7f54cde" - }, - { - "block_id": "000f445e281beb80a2699142341d92754ba21a24", - "extensions": [], - "previous": "000f445d47ff8adc82e21544c4e2de8f219e7195", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:39:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "204cd1a186cc00759870f22ab23c3543be8652fc895cadcd4b07e0f17c4d348450232e73a108446a43454e7221f286db5df8618285e84c8b2d093eba0e3dcbc8ba" - }, - { - "block_id": "000f445ff27765c97e452eb28d90cae6c34f36c0", - "extensions": [], - "previous": "000f445e281beb80a2699142341d92754ba21a24", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:39:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2058dea22ad4d410fca6f7a94b667dd643d8c6e13f5be4c6d2eb858f7837dbc5f57b2ec98d7e9a18656c61d39004eac9075291109404870f8a8206aa0d0d048f1f" - }, - { - "block_id": "000f44600aa1c89d85a4dd56eb416406dfb8e972", - "extensions": [], - "previous": "000f445ff27765c97e452eb28d90cae6c34f36c0", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:39:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "2004c68de671cbd85d8cc74e15a1e2958b590c9918d36e3334018a492a61f82146019ee11c381392628f9ae743483af8dae1f531e144030ca8f8c601aeed260097" - }, - { - "block_id": "000f4461fb88bcbe925c144416fbdab80c1f90e5", - "extensions": [], - "previous": "000f44600aa1c89d85a4dd56eb416406dfb8e972", - "signing_key": "STM6J3NVGh67YVF2M7VHkwKPSaTz2PJ9uMYnixM36JMP4rxhagT8v", - "timestamp": "2016-04-29T04:39:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "rossco94", - "witness_signature": "206a0da50ca4bfc42d8fcf79ec419c40efa1b9cd4b6ceffe5760758ed28abee89e029bf2cb969585173bc5b8cf64ea6120d95dd7bdaad003c8956af132998bcbfa" - }, - { - "block_id": "000f4462876b712108b6ebe85f602a0b7f29abf1", - "extensions": [], - "previous": "000f4461fb88bcbe925c144416fbdab80c1f90e5", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:39:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f0739d90319fda7e05ec3c7c10ba1166ea3a5fbcae7b46d9ccaba2cb769ccb04711165aa0042bf2ffc15918a95df0f82b7fb6dd6a0568844addd4f0a9667f2627" - }, - { - "block_id": "000f446312495f3b11a3d0b763549a94f80ead72", - "extensions": [], - "previous": "000f4462876b712108b6ebe85f602a0b7f29abf1", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:39:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "20535eb5c2f546864162747ccd6abdf02a4ac05235c9de84876439878acb0659495a0fa35135af75b9b8f5a17567d644701f02a1e4d34355e6cb75960aeaf54b75" - }, - { - "block_id": "000f446410c766f9343954fe23e37c2d0fc2c5c4", - "extensions": [], - "previous": "000f446312495f3b11a3d0b763549a94f80ead72", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:39:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "20198e5e63c6bcae5a8be24d51734b753558820f9f690e351a6966ddf71ebce24f25efec4ba623c84b61be9b41777433bac15f26488e7134d17fe1dc4c1e1c9124" - }, - { - "block_id": "000f4465a44317d7b83a27e24ef358f97c0421aa", - "extensions": [], - "previous": "000f446410c766f9343954fe23e37c2d0fc2c5c4", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:39:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f3dd8bd237c387df06016959d339ef182ed09f2c412b898eeb3f11335e91906013a8d3e9bf340edebbb179a830360b5db585250af865f73aff5f96a8003307fea" - }, - { - "block_id": "000f4466c0929040e17d1a946f13306fe505ce34", - "extensions": [], - "previous": "000f4465a44317d7b83a27e24ef358f97c0421aa", - "signing_key": "STM8UYGESsdnLecTFg7YaBm5tM4uQiUeUL7jj9AP2VQXDePj4emUR", - "timestamp": "2016-04-29T04:39:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "zzzz9999", - "witness_signature": "207658cbc0530b09ae900032c3139b5ee3d419a1029003fa604b336efee3c964e814e56563767182edd7f01279bc0e12cb9d4018ba43f11c986775dfeb877c9b66" - }, - { - "block_id": "000f4467518f6402507e7ff0ca49f2c511ddfc7f", - "extensions": [], - "previous": "000f4466c0929040e17d1a946f13306fe505ce34", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:39:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f65ac593b68dd0c42e288de7b0df8ea3e64d0c6d7873b474860557d77a6bb1d151e069630e2377d10de0a6aba56c2821c988176d1021f0a810f2668941e87ac68" - }, - { - "block_id": "000f4468e5e93286d4488a46a5bb373f059fa23e", - "extensions": [], - "previous": "000f4467518f6402507e7ff0ca49f2c511ddfc7f", - "signing_key": "STM4vhtognZwHz1sNX2efiySFap35k5M3g186nyjBAnbwPkTHCBT8", - "timestamp": "2016-04-29T04:39:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "joseph", - "witness_signature": "1f0cec671ec26f79d94ab80411fc4803fb490f103a74931dbdf08cac68abbb32c340a12124f5379ed307a9345b0bd98de325aa22e462e2037dd8599298028d5971" - }, - { - "block_id": "000f4469416f2b1442159713053d6dd27b5ee6a4", - "extensions": [], - "previous": "000f4468e5e93286d4488a46a5bb373f059fa23e", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:39:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "201fc42e24e164c3c32df78f42ec6bc3c08e4a676af4cce09d5cc34a38698bb20f45021a62d9fb3c0a70d974c0af044d5ba6f416847c0be10274e47d3b3aeb7a02" - }, - { - "block_id": "000f446aef79ddd26254e0d16f8a83a132d8f579", - "extensions": [], - "previous": "000f4469416f2b1442159713053d6dd27b5ee6a4", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:39:48", - "transaction_ids": [ - "e0049f6c5a57f0ae96b1c0c704e36f9aa831532e" - ], - "transaction_merkle_root": "cad89d43691f2d0ac25d53c23dc6577d1de68e5b", - "transactions": [ - { - "expiration": "2016-04-29T05:39:45", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f4469416f2b1442159713053d6dd27b5ee6a4", - "nonce": "8194284927866352513", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "3df50ce7ee0fd8cd7897350f2115fb676310e57a5258102c653028f5836633a4", - "signature": "20678cf4104f6c5613902a91b97d344b4968aee5b29c99d6ffadfea40978da42ac2a4fd057d3c67786288c6898dfeb8112eec2e8ae36d60038d335efcb3c82a667", - "work": "00000006721debd6a64dd2d4cffc1fe7368f5f65dde68a99cb684bb238d323cb", - "worker": "STM6P99QuwgzakzYov6N9b5sQrBBzK22H41Rgs6ydScCaNQnfpJ7X" - }, - "worker_account": "smosh" - } - } - ], - "ref_block_num": 17513, - "ref_block_prefix": 338390849, - "signatures": [] - } - ], - "witness": "arhag", - "witness_signature": "1f166a3b4ceb5d80fd6bd2e62bd1b416106048272521047b95356254cc3f1b41355d98c8c06901b56489d2684e24ac9958ef570db1204665b51dd4ed4481c9a291" - }, - { - "block_id": "000f446b4446a58ae8ca3855a5ef90dba0d09784", - "extensions": [], - "previous": "000f446aef79ddd26254e0d16f8a83a132d8f579", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:39:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2014399a9a537a81365635b1710c62cf8c290da4df4d0b7c426e9b15157747e8056e4185955c2817fcbc59b14f246ec88f9f3a128cd4d9e0e9997e2020c93810b2" - }, - { - "block_id": "000f446c0b7e7f96ed3f2843b289820a0a488f33", - "extensions": [], - "previous": "000f446b4446a58ae8ca3855a5ef90dba0d09784", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:39:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "207929125259453aa00fd5266a7777019d714d21807cb7b2cc812e79904d1a59af159c5ab4768130aac5b20fc1a17cc36f16350c3d2daf9396769dbe44dbec43ad" - }, - { - "block_id": "000f446dab756c55bbf21a7655f74f3cea358702", - "extensions": [], - "previous": "000f446c0b7e7f96ed3f2843b289820a0a488f33", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:39:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f5f8e866a6c75b0e11f00494fe31a5f05ba5aa84f80dc3e25b9dcf0dfb18274671cba79671cc17b3b76bf6a260bb02d2bbf5db2d68606759cd200eb3bdad14622" - }, - { - "block_id": "000f446ee915530270273b8d9cc7896b67d6c80a", - "extensions": [], - "previous": "000f446dab756c55bbf21a7655f74f3cea358702", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:40:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f41eb4ac1097e0bc8ae8d44bb46d19c2e4b538752bd2657f678bd60bc82d675303c1a24601678f2348ac78ac40bf0df02a784b52f3dfb603be91b948504b71f6a" - }, - { - "block_id": "000f446f94b8ae707b2a197fe19f575eb157c998", - "extensions": [], - "previous": "000f446ee915530270273b8d9cc7896b67d6c80a", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:40:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "204337766cf5d0d72185bb31fc88480a83cb4233c894ae9f4fe3a7b5c9909270fb08fe9ef806fc8bb0c197bbd2264a64d85ab43e284d4a7a8bb0ea2280101c41cc" - }, - { - "block_id": "000f4470a8b2cf819968768ff5393c5b46dc8220", - "extensions": [], - "previous": "000f446f94b8ae707b2a197fe19f575eb157c998", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:40:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f06c59c478ded02c5faa8a380d6e5aadf39b3193d89ab08efb20f6c690da3c77604a6d72af8a51c567358e633dcbbd474e915076afd1d174900637934cf8502cb" - }, - { - "block_id": "000f447164ca603cf72091bc3695430ffa762906", - "extensions": [], - "previous": "000f4470a8b2cf819968768ff5393c5b46dc8220", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:40:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f122daa8fb31f5d83abcec690b0eb4303e9f78188e02e25972022d44934b1b4d023035841ddf130cda9b3f4af206b5aafc0f6a72ef6abd79e19034515e52f9ac6" - }, - { - "block_id": "000f447204baf61bfbfa0da0de76fbd9ece046c8", - "extensions": [], - "previous": "000f447164ca603cf72091bc3695430ffa762906", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:40:12", - "transaction_ids": [ - "26c05e5065a772e1ab24f770cea2054b5bad307f" - ], - "transaction_merkle_root": "d62ccbc13f6cd18fa5fb32e82ccd9f5c2faca173", - "transactions": [ - { - "expiration": "2016-04-29T05:40:09", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f447164ca603cf72091bc3695430ffa762906", - "nonce": "911740918991242232", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "0ac3eca010cfed98aba883234721d306e7b7d67c2c48a8374e8b1cf5cf47f74e", - "signature": "1f7b24e4ac27b2e4a45e26b0b27e0000cc33fb0210d6ece0a8256b60fbbb09f51c0f32ec91fd1061550a6b675112039ee6b8df672dd4e471b7d2626581fc7ce698", - "work": "0000000184b5927eca7fa08ff2a22b493de3ef9049abeac8b8f02cf9d7f83175", - "worker": "STM79MBtZRHzawavWSBSVYNRAy7WkwM2PMPDEnoWH547WLzXP5CkP" - }, - "worker_account": "hosea" - } - } - ], - "ref_block_num": 17521, - "ref_block_prefix": 1012976228, - "signatures": [] - } - ], - "witness": "silversteem", - "witness_signature": "1f5f876472fa03c74aa295c5ecf5c15de143344a064985118935f8ead7767411da1571566d514e8263e728d025aaa561612ce32a9b1e409b2f76c5c6f8737df470" - }, - { - "block_id": "000f447375d2a89f1e8502fae065c94d2a5cd44d", - "extensions": [], - "previous": "000f447204baf61bfbfa0da0de76fbd9ece046c8", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:40:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "2062160fd83e25021474e33af4ee88939a7af60d3f1663eb14c452baa8c763ec925516f1b305bb54eb613ec0c10d2cb5c3e551be4e3226b0e4b32bef023271fffe" - }, - { - "block_id": "000f44743e622bffad39c2fc8ec71b1a06162495", - "extensions": [], - "previous": "000f447375d2a89f1e8502fae065c94d2a5cd44d", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:40:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f394d4b4c6e3e9843351a4c01d7065a3685abce7d700fd91d60c60cecfcd8bb7623714741dd64bee71285de0208bb22d288915eedae6b0cf2dd99912e8c295c1f" - }, - { - "block_id": "000f447567623081ab96b5995cbb54e76d2e3d99", - "extensions": [], - "previous": "000f44743e622bffad39c2fc8ec71b1a06162495", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:40:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f52bebbed4e9c7b3ff8af9885f97b752c86e55e1a7d706717218a572aa79c87821ffe8c5f54711e6b31eee763b35cae8c3c624b070dddc1440c3924f2c86e0866" - }, - { - "block_id": "000f4476fe1868896a69df063e2c2716c827ef43", - "extensions": [], - "previous": "000f447567623081ab96b5995cbb54e76d2e3d99", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:40:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f6d19864ff51e4a6861594a38ced24d98c526c2ded594cab53c1811b6e3d7482d59f47d87ca0a45be0a2a7ce2ea7dd4313338c7593a216bde7de861624fb4f714" - }, - { - "block_id": "000f4477b0341016043b83902ac8cd2b0c54c162", - "extensions": [], - "previous": "000f4476fe1868896a69df063e2c2716c827ef43", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:40:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "200a0b56f668adf0b33c8e95ddbef2f9ff8d0cd31955fba40c5d7f8bf42fc02028388dde5a7217d60514ad56bddfd4afeae31665a5e8d229d480126ca35f8e4418" - }, - { - "block_id": "000f447898638651d150cb2cc2c3a73ad6af07a7", - "extensions": [], - "previous": "000f4477b0341016043b83902ac8cd2b0c54c162", - "signing_key": "STM6BJYGHftujnbttFFKX6YacnvsMd4sbJrbucg682GiU4vmXHTik", - "timestamp": "2016-04-29T04:40:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "riverhead", - "witness_signature": "201c1d280b9702d81c6d45e5cae02d7e24e3b376e79ffb31ab261d8b84210d97d265cd0f994dd2a7947da1d7838aff272cd24c69a7cb5182beec3f5839239f8ad9" - }, - { - "block_id": "000f4479933e4e2094da80de5fbd0fe9c41d3489", - "extensions": [], - "previous": "000f447898638651d150cb2cc2c3a73ad6af07a7", - "signing_key": "STM6oyQdLfXN24awGycHsfv2FU3YC96YccEtim3bnxtBdJxoz8Q2u", - "timestamp": "2016-04-29T04:40:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "give", - "witness_signature": "202a357ce01b92ac6040152d9eb32c4d6f4f30d95e867d0b8157ef3580515374e307366fde39eb582bef86e60bf12f9e8999c96c4210be118369633f5a6da9a0a1" - }, - { - "block_id": "000f447ab13942dcd182a70fc5747f5b68c6c72c", - "extensions": [], - "previous": "000f4479933e4e2094da80de5fbd0fe9c41d3489", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:40:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2060452c06aa1b11aa1ffd4f4643e2027cd6499eb63791e088cf1e546e8675f40a2162eebe59e69c3a24012e5c0c6223b4908ea9a613fdb21872a9d6258bee976e" - }, - { - "block_id": "000f447b18b968f2ed981c48b89058e6d6354f02", - "extensions": [], - "previous": "000f447ab13942dcd182a70fc5747f5b68c6c72c", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:40:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "20777f27af94774f4cb2feb2615809fed57a12ed82f2f1075cb748347c5a8eed9202d99a8d4975be05fe66889be24c3b3d09cd7025e90d31ac25f6f0714ffd9a29" - }, - { - "block_id": "000f447c1094941b540048852ae22bf742801901", - "extensions": [], - "previous": "000f447b18b968f2ed981c48b89058e6d6354f02", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:40:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "2044143c0f4568f5725af7fdc3bf3ea6cd5b2014bc2aa7b4e4c618a66bc1799cdb6b9b13b52e5ee31c07d5adde6e98191f9d82bb406f23859811dffa5f2a303aaf" - }, - { - "block_id": "000f447da567377c95043a84f8b4549540064b72", - "extensions": [], - "previous": "000f447c1094941b540048852ae22bf742801901", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:40:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "20634d4e69d8ab9eee503d9a3a6718d0356cb36aae077bd7b9fd53f9b88391a56f3498596ba56da5173d26adab8759eedb638d88beab195633f34cb527b7e2ce32" - }, - { - "block_id": "000f447ef7a47252b2439dfc6f4c05c1484254ee", - "extensions": [], - "previous": "000f447da567377c95043a84f8b4549540064b72", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:40:48", - "transaction_ids": [ - "30ddaea0248609a5420e2e967f8e8a2e6e6dc68c" - ], - "transaction_merkle_root": "e68ad5386b3c67a86c80ae8be4f4384ccfb83b1f", - "transactions": [ - { - "expiration": "2016-04-29T05:40:45", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f447da567377c95043a84f8b4549540064b72", - "nonce": "11390183059392089875", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "d9a2977549702d76a03070ac770a1f810369740b42a4ee7d13301fce24f9930d", - "signature": "207faf52fba4fbfe6850ce22bf4192509ba2abe81dcf48ba23dfc8468d321459b3502568441f5816b2faed35ebda7333a8bf9605dc44096e86f8afc9fbf0a922ae", - "work": "0000000b7dec36b0e36bb41a9020c03378016ee4d533986d927d13d5cf40697a", - "worker": "STM52u818cFy9oCJsqPK2YCgtbdnmNnwN9eYAQNMSf2C96eTiyoW6" - }, - "worker_account": "phd" - } - } - ], - "ref_block_num": 17533, - "ref_block_prefix": 2084005797, - "signatures": [] - } - ], - "witness": "abit", - "witness_signature": "1f6357de21ff8c33355f2456005d89d872fe131c8d194c8416ff29483f0e8350ef7c37221090c5a7342aed9a08d60bd04d4a39c40cf88656880de0112812736597" - }, - { - "block_id": "000f447fe39fb79155ceab046344129a01574241", - "extensions": [], - "previous": "000f447ef7a47252b2439dfc6f4c05c1484254ee", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:40:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f52bd8d028db3558961f24ca38a34a89c424aaece73efeb658b1e77af4191a9731c28e8ac094685d6338eb0e513bc3a9055dff4ea69acd3bd1ff1b45b2043f3e1" - }, - { - "block_id": "000f44808f7f46efe119eabdc4c430acc76d2d39", - "extensions": [], - "previous": "000f447fe39fb79155ceab046344129a01574241", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:40:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "205fd6f80e2e5a28f1982e19939cc9cf472247a9019f6ae5a5eea3d48468934f9709d94ae8684fd8928745a8664be22926c239b14b4d6fa4010d90e4d4ae3390b6" - }, - { - "block_id": "000f4481b00f18e2f2a51fd213662b060417a92a", - "extensions": [], - "previous": "000f44808f7f46efe119eabdc4c430acc76d2d39", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:40:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f57e4d70c23cdfa0bb5ec72dec94084c887763fae7e0f06937a2b2f4ebae247b833db38845ad64c5c149131d05d3723771615888e6ea5a7560c73c43f54d0d5ba" - }, - { - "block_id": "000f448242e4d3f26db186e67f2eee5b3c5c0eed", - "extensions": [], - "previous": "000f4481b00f18e2f2a51fd213662b060417a92a", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:41:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "204bbef1a62e6163e618e0d436fc86c07c5e2e84fdb55358a46dab55570761b4c13e94a81edb6393e426d6b3f486bc9885824ffddfd0f1a0ad9568964a54675573" - }, - { - "block_id": "000f44837ac6bd1c98cbac8b57832b669571175b", - "extensions": [], - "previous": "000f448242e4d3f26db186e67f2eee5b3c5c0eed", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:41:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f647348d1c6e7b1d3eafa01d7bca2538da6e5f30d847435bf31f82db9395c1bbe07054c0c014fb7a2394b9c69d0d6b7dd744ef46757c82efc632dc228e8998eee" - }, - { - "block_id": "000f44841a7d20d0ff47772c3bdc24daef2b6496", - "extensions": [], - "previous": "000f44837ac6bd1c98cbac8b57832b669571175b", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:41:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "207cd373d9e04b8b700d6c52c55c82a80f08cd878968b798d3a75d0226ba3eb5da553b755b98007403c8e98b7c8f1479e8f6cb29f31be77e03f19acfba6daf5b18" - }, - { - "block_id": "000f448589e561573a6c5c861f7e3f91772b5ec7", - "extensions": [], - "previous": "000f44841a7d20d0ff47772c3bdc24daef2b6496", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:41:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f1a58f6fb9c1934f00da5ae730c6c150271d1db4cd3308401420b66331b5fe32947fe9bd42f822ccb5612c63cc17716b90ec9c5bb441f080ba16a56ec97857e5f" - }, - { - "block_id": "000f44866df0121b45167af28f4150be3a23ce13", - "extensions": [], - "previous": "000f448589e561573a6c5c861f7e3f91772b5ec7", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:41:12", - "transaction_ids": [ - "09852d47137d4ae5970c24eedc51d52f009b7ee5" - ], - "transaction_merkle_root": "e6af719df30c443c8fd1f2d00c8de75098c8d36b", - "transactions": [ - { - "expiration": "2016-04-29T05:41:09", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f448589e561573a6c5c861f7e3f91772b5ec7", - "nonce": "911740918991176605", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "2b7ea2dd23c026b0cc1a97346b98e0b0f3945e0584cf6d5c9f456d56fbb8acb7", - "signature": "1f99e3027eab0df2241d4e4455acae7a39d85f6ecbc1bca106979abb09a6051f534931935a009786950ef27539e6b7a79d6bebb34820074f4efa8d118acd4dd9d1", - "work": "0000000b698fbcf8dac66301b5e01f7915d2db0fab38771b016f42296ff1613c", - "worker": "STM79MBtZRHzawavWSBSVYNRAy7WkwM2PMPDEnoWH547WLzXP5CkP" - }, - "worker_account": "hosie" - } - } - ], - "ref_block_num": 17541, - "ref_block_prefix": 1466033545, - "signatures": [] - } - ], - "witness": "complexring", - "witness_signature": "200a4d997eae7c6ae22302ba3afd237b93f5f9f0bfaacd43a69e0aa1649e7ee0f675f3a0a9460703f3181aed615344d8ffa49f887000510a0ef64332239d397f41" - }, - { - "block_id": "000f4487f4acf0bff517e6dab88880e804b841a1", - "extensions": [], - "previous": "000f44866df0121b45167af28f4150be3a23ce13", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:41:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f78fcbc6bddc336839a75ce56cb83c7650a20d5c2bc3a5b953fa46a618cd8dde975913dd94fe0a23dd24f0be32723fcbae6ba66b4bb63acfbe00c64a4683bc527" - }, - { - "block_id": "000f4488045aa98e035d30142ca72918c1aa5135", - "extensions": [], - "previous": "000f4487f4acf0bff517e6dab88880e804b841a1", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:41:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f153d6b21e92b07da01c7500e754a840d564e04631ca935523ae3ba6af3b20b592878c17d9721861bcc210c61599dc4f483182c4ccd2dea73b55dcef49e22d916" - }, - { - "block_id": "000f4489d25d956512e4b2455c4b9a965ace6c55", - "extensions": [], - "previous": "000f4488045aa98e035d30142ca72918c1aa5135", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:41:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "202e977a370c59a8e15e4b69f4e2a7917f396a84f00d70f38363d4439e7235cbb353204c35d81eb5423acbdbe61f3b144e0c549544cbf9c3144bfc9f6dec09b8be" - }, - { - "block_id": "000f448a74852349b8b320f04d169e35b8bd903a", - "extensions": [], - "previous": "000f4489d25d956512e4b2455c4b9a965ace6c55", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:41:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f157342f7b53f45129c3afb8d461e596f747493a47fec947badb1ae83563db11039830b25564ea87c40ac77a1f5f73c30a189a3d9fedd0fc78b558d094a40bf7f" - }, - { - "block_id": "000f448bd997a1d499983c398d47e48eb02c57f6", - "extensions": [], - "previous": "000f448a74852349b8b320f04d169e35b8bd903a", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:41:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f53d5d82dbebbb9136a7fc17295d6b3cc40e9c10a627546148de19ce6b8c327b058e3c38591f81f8419ec34c529475147998940ba0d0b0107cc6dfaccc5fa10e1" - }, - { - "block_id": "000f448c88c6951943cc42523e9f9372fc90b750", - "extensions": [], - "previous": "000f448bd997a1d499983c398d47e48eb02c57f6", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:41:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f39f7a697e613bc22690ae94223d1c30bc0db5991665a5c89f083a29649b5676c4e2286c1ce981734feef383105c3d03516c8ca28d910d9ade197b8cdb2c16058" - }, - { - "block_id": "000f448de6fcd8d4768b40900a85d5aae770dd80", - "extensions": [], - "previous": "000f448c88c6951943cc42523e9f9372fc90b750", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:41:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "2014754030dfaa12d2501b0c43b5e9ec88651a7388104e05dc4b61193f285669cb0d8b09e6ff44363768696df3e9baadb250a0c70010728f621761b68d6eece123" - }, - { - "block_id": "000f448e1c8566d205fb72b8bfb08c74a39da448", - "extensions": [], - "previous": "000f448de6fcd8d4768b40900a85d5aae770dd80", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:41:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f784a115a11f8081bf1c04133ccdd0b000a557e56d8c88e4625d4c6a7107a23340721b9787964e296f928e0509a2ee15f5ba746ec3f3c6b5dd94c0a8c6920cc2a" - }, - { - "block_id": "000f448f3aeaf1237a9bba50dd752f98132446ba", - "extensions": [], - "previous": "000f448e1c8566d205fb72b8bfb08c74a39da448", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:41:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "2003692d8b35a05d885c619eaa3b0547f82b621e896cea245d7ac93a28a66240395d6d57e537aef8ef8e9411aa0f43d32b93ff02b2223296a879c1cccef956fb2c" - }, - { - "block_id": "000f44908831b9c6328d66c9a7eebc502562f335", - "extensions": [], - "previous": "000f448f3aeaf1237a9bba50dd752f98132446ba", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:41:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "207ea60c6fbb119d0467da5e71249e3d6d1862b2216a96f8d490c63fc4796241127b8962128c416ba3be523e5494ad1f4904024224e38728fc34ab12293ef4de7f" - }, - { - "block_id": "000f449181fea0ed1123efaa757f70857d54b3a4", - "extensions": [], - "previous": "000f44908831b9c6328d66c9a7eebc502562f335", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:41:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f6064ff99d995d33da29ae8c5de4b24c097e519da92e1348a57101fde67d0309124c45119be46b1bbc8a6156881f526afe77bb8209d66eaadf5a737b014d44538" - }, - { - "block_id": "000f4492b7924711d8e35b7bfe6ec8f669f03efd", - "extensions": [], - "previous": "000f449181fea0ed1123efaa757f70857d54b3a4", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:41:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f210f890373fa9d26bd6a002ab4f1ccef00e0041c15d15d3c342ca60f1df8350a294fbd711f398758d44c27024d88438379bc37508979d916783b815c40f9ed96" - }, - { - "block_id": "000f4493d70b94305467eb18769a763826ddd21b", - "extensions": [], - "previous": "000f4492b7924711d8e35b7bfe6ec8f669f03efd", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:41:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "203c849b361c6352e813bc8cdfcd65435bc8740d21c1db9c626e99e1dcec69fd1c4a2b69f19089be88c39d00bd247cf606f862766981be791d1b97d6196e347cc9" - }, - { - "block_id": "000f4494d28be510f52f15adaec417994ff13b09", - "extensions": [], - "previous": "000f4493d70b94305467eb18769a763826ddd21b", - "signing_key": "STM6x6XcFUTNgJcip2AREjVGtr4u2YMKVLiJm7VgY9UeR37enhesJ", - "timestamp": "2016-04-29T04:41:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "medialka1", - "witness_signature": "1f07e5097612a0b626c3b29be35d9cb59a3d6a63e05e9965d92b1b770040bc9d4b7aadbc1e6c0086081311c9c07886d3f1dd9be7524d0114592e4d71003cceccf6" - }, - { - "block_id": "000f4495ddc742afb21b7258c5b5fcbae8716307", - "extensions": [], - "previous": "000f4494d28be510f52f15adaec417994ff13b09", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:41:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f7a313deb921583c0d2dbcae5147fa0ee508e2f21ac25f4194e265c1b748721157fa4a389d587d0ef0df7fca04122f1f922f04361f73fbb80ef16ae4cd3f43b92" - }, - { - "block_id": "000f449623268889b311eaab0cb9c31bc9950744", - "extensions": [], - "previous": "000f4495ddc742afb21b7258c5b5fcbae8716307", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:42:00", - "transaction_ids": [ - "1ad4fd0c345c0acda212ebe223231bace501607d" - ], - "transaction_merkle_root": "a83440d9e049f327a50d9eca16ef6e74f087953c", - "transactions": [ - { - "expiration": "2016-04-29T05:41:57", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f4495ddc742afb21b7258c5b5fcbae8716307", - "nonce": "2160135066759250815", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "6c8bf162d210dce6129b2f6e560bc2782012fa1da280e9f3a47ff6703e5fd1b3", - "signature": "1f379fc378e27de9cfecbe6ae57cd2fda9e0795543f65311e56b85e9ccc7fd4cee2c379b1474707085d8912e7d6d5730c5c823afb6eaad386b6be0363c40330740", - "work": "0000000489204f846acd3b76a8357c415566f3ebf3b3ab03de61f3dd39e2366e", - "worker": "STM66crmnX96YMyWUPnFzSkv7DsGESbWaoSGbmoNq4EWDYq2wnwPf" - }, - "worker_account": "liondani" - } - } - ], - "ref_block_num": 17557, - "ref_block_prefix": 2940389341, - "signatures": [] - } - ], - "witness": "steemychicken1", - "witness_signature": "20752e922a619b8e5e9d2ce7d66c68f6e0e8f6f2299d763cf78d219d1dcf0b6ae024feb3dfd5d06e8a4ee5c73c5ffcc727fa40f24d5aa4b4a20cf007d46942be3c" - }, - { - "block_id": "000f449770a18ddca017488b2c164c33e25973e4", - "extensions": [], - "previous": "000f449623268889b311eaab0cb9c31bc9950744", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:42:03", - "transaction_ids": [ - "4418e2f3a8cfa181664b4d24eb8bc71a3b759575" - ], - "transaction_merkle_root": "c857aa64042c07f3c93fb22fda173f2c9f5dda1b", - "transactions": [ - { - "expiration": "2016-04-29T05:42:00", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f449623268889b311eaab0cb9c31bc9950744", - "nonce": "806255817238575234", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "2b20010212e16d96d26357a48f171872b043c9218ad6208fc6e1796f802f270d", - "signature": "1f7cedcc7713ce052065ea92f051761529c4091b1c2ab166429426d30a7f277e482f764adbe454c8a5f766f69176509fdb78fcce0c0f96c6a1a795fdfd0238f113", - "work": "00000009091fe3689b2d785afbe92e8e9f3cb2c25d6be350d7fa399d9d277ed7", - "worker": "STM8RVh9ZnJZhULNSQwRUueSJSKtJUjNMPbnyRdWmEJAVCTJZ5AYT" - }, - "worker_account": "hr405" - } - } - ], - "ref_block_num": 17558, - "ref_block_prefix": 2307401251, - "signatures": [] - } - ], - "witness": "abit", - "witness_signature": "1f6252b7a48509d750784cf80cc81a7565d39d5223a3588ef7c7961942975fcef9285c2c84099e6a4459422a8b5b9430bfc21de1b838b3e0352c805288afabc17f" - }, - { - "block_id": "000f4498327c4352bbe6809d94522d76979c2a4f", - "extensions": [], - "previous": "000f449770a18ddca017488b2c164c33e25973e4", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:42:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f5e9476394d560b7bf99b353d73621661b2c7bd8357eb1dbfd5aeefae38c13a054b4286344bd139fc16e338396bbe5791f909312862c36f7b380da1bcdffff7a7" - }, - { - "block_id": "000f449955a147e9d8e38def4447a8872a72ab9e", - "extensions": [], - "previous": "000f4498327c4352bbe6809d94522d76979c2a4f", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:42:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "204aeb15e16e2ac3c8858072501e505e5ad6abc4dfa7cd263185dec73e32da6bdd49a433f34f9d8bc786b5ab3f28460818350014b7d37e1231ed032615d512c6f5" - }, - { - "block_id": "000f449a2f1ad61e63a5937a68bb060c35c4f526", - "extensions": [], - "previous": "000f449955a147e9d8e38def4447a8872a72ab9e", - "signing_key": "STM5742TmF2wyuStKtgETi1KDycZ56NNvgJhAt6xWA3PA1Rx61FvU", - "timestamp": "2016-04-29T04:42:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "cyrano.witness", - "witness_signature": "205a6d381e8a63a947ec1e4fd6d95d24bb1504f6250c131ef516e3e5c411f88a195e3b6b2ba6c65d600e4b71a487bdaad9e982afe66cf0f14c21efecd8b573fe4c" - }, - { - "block_id": "000f449bc0daf7d78392f57facd59020a0c1f9d5", - "extensions": [], - "previous": "000f449a2f1ad61e63a5937a68bb060c35c4f526", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:42:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f04bf764cd220b3911c0a553fe81ec534bb3f4f671a093b3e299156f8bf7a07162f7e700498c542c48a4f54e09255417bd7b02e94fc02f276ba1ff8c7503bd431" - }, - { - "block_id": "000f449ca4ecfb6ddbb2636df26a29d3ccff49e1", - "extensions": [], - "previous": "000f449bc0daf7d78392f57facd59020a0c1f9d5", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:42:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "203bdf1e23f02b77ab6895bc28536515676064d6ef5f69df3156377ae64e00ba9e3cd9c9ddfb06dae923330a422938515f22b260677cc7c3b325e9bfc60bc07cb2" - }, - { - "block_id": "000f449d205a36c331a459b22a5448ca4c639ff4", - "extensions": [], - "previous": "000f449ca4ecfb6ddbb2636df26a29d3ccff49e1", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:42:21", - "transaction_ids": [ - "b26a81e8817bc5b22a2be85c39ac056a68b7ea76" - ], - "transaction_merkle_root": "085c28ba3a1d3efd2bb220d8b86be45d2aef8ee5", - "transactions": [ - { - "expiration": "2016-04-29T05:42:18", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f449ca4ecfb6ddbb2636df26a29d3ccff49e1", - "nonce": "3352594912243849421", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "1858e042b3a0ed70b3bb70df16246df0db65deb961294c7d60267fdf6f29de20", - "signature": "20801143434bd29bd86a6498371c9ea8bf2f9c8096ba5c78bbdabe9b286dcc993c56acee35ab10a60a118ba7897860271c120b3ff796fca9c06f42adec407e460e", - "work": "000000016eb8b0c78c5b3ce63bf10a351c8e7347f1cba140046399615fd654ec", - "worker": "STM7HR2jZiuaVi7xJx8DnkdL1SvTVEDTP1pQZdmaTLGAnt4EqnscW" - }, - "worker_account": "hr901" - } - } - ], - "ref_block_num": 17564, - "ref_block_prefix": 1845226660, - "signatures": [] - } - ], - "witness": "roadscape", - "witness_signature": "2020627f6d2efc7e255d54c7270b09e9e1fb5ef99b73671d61ea24a96ce5e3097279cc4d0d657522eecc924cff5f45672ad63043b06c2a75c11400b36ab329269a" - }, - { - "block_id": "000f449ee14d1742a7207c20eb9676a4d1a1a1d7", - "extensions": [], - "previous": "000f449d205a36c331a459b22a5448ca4c639ff4", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:42:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "2065970dc1e09e621c342301575dd3a0dbaeaed516cb715e6fee0cce276253c128724468fdab673e2824070a7b19d18b122435f5d3b7afe2270432bce5e0ae48de" - }, - { - "block_id": "000f449fcede27c221910f827ee5dde93db95a33", - "extensions": [], - "previous": "000f449ee14d1742a7207c20eb9676a4d1a1a1d7", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:42:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "204570257152a8f2c4488ac21682ec253960ee6b6b22454bc01f09f3910ef6550222fc6afa11082f43bff2168fe8cd1a9f0e8abeb784ce60d21f5f7bb6e84ec900" - }, - { - "block_id": "000f44a04dab0bcce62df8cab145ed360fecea84", - "extensions": [], - "previous": "000f449fcede27c221910f827ee5dde93db95a33", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:42:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f7346ee3c3780b275cd00a475a2da713dfaca930face7135fc797b019182369e05ee2238470226a1daa436b7097008d662589513d3de5b5c7c0b6f8ea333cb5cf" - }, - { - "block_id": "000f44a13260a697da0e9cf246d57001151b69c3", - "extensions": [], - "previous": "000f44a04dab0bcce62df8cab145ed360fecea84", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:42:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f74b6bea11c071d75af4b7a3da71ad377e7125f724b8a4f793c1a9cb4dde651c23d2794eb6bf11a0e2ca7e2501dd24fb737c3836a364f84e54b5430a17c20a9cd" - }, - { - "block_id": "000f44a2af496ddf9f021a4e9bb87e0d96a22688", - "extensions": [], - "previous": "000f44a13260a697da0e9cf246d57001151b69c3", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:42:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f016cf4b07176789d5e5ea5461c0c774934e3914e2366d3556fdb7e26fc0d841a4d8b3db14c6cb2b602ff7283aba1493cdef14a0b91035d366a4263cfd7f7f065" - }, - { - "block_id": "000f44a372de20bf1eb70ba4ac408d942e5463c4", - "extensions": [], - "previous": "000f44a2af496ddf9f021a4e9bb87e0d96a22688", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:42:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "20442c8fb3427be93b611b8b1b00437c47e8770c97470263aae437a22197e0062707197c0a2d6abddb804eb4658dfba3e93991de42bf27c8a1e374c0e5c5a49c7f" - }, - { - "block_id": "000f44a4ffc7305eb1f58dc750a3361c7aeb6fcf", - "extensions": [], - "previous": "000f44a372de20bf1eb70ba4ac408d942e5463c4", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:42:42", - "transaction_ids": [ - "ff3af3c61349ab604ccfc415b9c7ab227a98c37c" - ], - "transaction_merkle_root": "2de82b695524a4d7546123dfc355fc7529217553", - "transactions": [ - { - "expiration": "2016-04-29T05:42:39", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f44a372de20bf1eb70ba4ac408d942e5463c4", - "nonce": "7947420156751865554", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "c21972144ef5e1ebab88112078cf48630b00bb2d7120fa44560e1b81700fef59", - "signature": "20271433ffe0f1126a436582a15e81716896d7292d1bc2e59a7e5e79fb6edfc0ca66bc6dd6922ba5779b99e89f27b0de593fdaba99a82a15146c82cf31b22fff70", - "work": "000000052918ca7a7ebdc48bee9126c2a187c7ad4a8772328859bc9146b94a68", - "worker": "STM5XoTf4uvGDDxWYMT9nR4BpM85CqR9spNbnJkVZufEqYztnbqYK" - }, - "worker_account": "hr505" - } - } - ], - "ref_block_num": 17571, - "ref_block_prefix": 3206602354, - "signatures": [] - } - ], - "witness": "xeldal", - "witness_signature": "20118a49b5f3a55aebbe00f91e17ca95312cb7e1d6f659e4605b8a8af7749e7ce267f3b46417df40bb897ac6751938851fdb7dedeb09ab6abfc553bee57d00e583" - }, - { - "block_id": "000f44a575259c980c5054aa7cc65d29631d9b59", - "extensions": [], - "previous": "000f44a4ffc7305eb1f58dc750a3361c7aeb6fcf", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:42:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "207e26258ff5a6bfbd8c2920748b91c0158bac7a216cbd3c5de5795b301f40648e0c711cab70894d53ae559af160a01b28ffbc70d08aab2bb6ff0d078023a52fc5" - }, - { - "block_id": "000f44a6640e4e94e718264cd5acaa99620c5d1a", - "extensions": [], - "previous": "000f44a575259c980c5054aa7cc65d29631d9b59", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:42:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "20756633d841d1bc6b981895d26416d69b85102c5262ff50ab5a5478650e9185346ea6843359c016278cdac3040585255c2d3fa48fd4333a23575bb81b258e75d8" - }, - { - "block_id": "000f44a7741941a7df612d3f895b7047dcc9f3ff", - "extensions": [], - "previous": "000f44a6640e4e94e718264cd5acaa99620c5d1a", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:42:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f7789544ec48466f9b763562e71f98b8e297f45b453e3f59aeb84041898d80a2a33a592e599ce672d9b6a7a47f4a1cee8d93304e69aa47f957831afb3d318fdb9" - }, - { - "block_id": "000f44a8bc65891d72a609a7f46aab1e4a3620eb", - "extensions": [], - "previous": "000f44a7741941a7df612d3f895b7047dcc9f3ff", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:42:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f24e3ec5794380c92899946d7d79e9b7493ddb6b3315853662f378a22fc2e591109236814a33f8ba408f29e6fcf289fa74f668d97ab0d0b0d21ec08a760a96254" - }, - { - "block_id": "000f44a9488fca49de8a71eaa16a55afa79f6dd8", - "extensions": [], - "previous": "000f44a8bc65891d72a609a7f46aab1e4a3620eb", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:42:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f0da8441d502fc9b6a6f1fc5960fba4f16e18a5637bda5b676c322674e16eb7f54ded80be13da7e0535b9fb2e92cdb1707ce510f161faad870aacb2d2f13a6328" - }, - { - "block_id": "000f44aa5e15fa6f9d6e801c629e6b42a4140a86", - "extensions": [], - "previous": "000f44a9488fca49de8a71eaa16a55afa79f6dd8", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:43:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "207dcc7454b391865fd9d1adb5ca9154b3e8d5f13eafe432c7f001a5a2ec16b6d53f86730765c6523d0ac386df05263caaca23efe63d140ecda6731ef235f0e105" - }, - { - "block_id": "000f44ab6fa6d2474efc7c11db987fe6fbf12c48", - "extensions": [], - "previous": "000f44aa5e15fa6f9d6e801c629e6b42a4140a86", - "signing_key": "STM6Kq8bD5PKn53MYQkJo35BagfjvfV2yY13j9WUTsNRAsAU8TegZ", - "timestamp": "2016-04-29T04:43:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "wackou", - "witness_signature": "1f021115b64701cf3b15d405e9f0ccc9723b3957fdbf9f4bd12375221bbb9d2eaf3f4ff69d16463b6b8240f330baa654ef4f96ae83439e711ae4a5ffccc3ce83ec" - }, - { - "block_id": "000f44acf383a457573e9e030270ab9143aac4b4", - "extensions": [], - "previous": "000f44ab6fa6d2474efc7c11db987fe6fbf12c48", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:43:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f40704b0c2b8adab4ae7f21b85a93d8d330486cd896ea30cb4eb157d8c15970ac163ccc82824c7958d3dca2401e8d428c150283049530c88b867c83c0a2eab1c9" - }, - { - "block_id": "000f44ad1a232ed29b6599d451d20a81a8deffa6", - "extensions": [], - "previous": "000f44acf383a457573e9e030270ab9143aac4b4", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:43:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f4426f6387d4b6e2b5f608878eb1c0d370b15be583158989d0ef1a5c9cf66e28512c6897be0ef45946af5d4ce4b65335a953ab01a0fdc08db9ffb919cc8ab2e4d" - }, - { - "block_id": "000f44ae6f7a76c946adb801b018ed9386a1cf7a", - "extensions": [], - "previous": "000f44ad1a232ed29b6599d451d20a81a8deffa6", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:43:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f7bf1a338101ea817f0e757cfd7078958ab8f562228eaae176d9a145da24fafbc357e161db3231473431dd253ee0d7c78ff1f74aface1b505a69f3cc5c2509092" - }, - { - "block_id": "000f44afa8a61fcf313d1c73f8e3203125512942", - "extensions": [], - "previous": "000f44ae6f7a76c946adb801b018ed9386a1cf7a", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:43:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "2041f59ed2fc694f13f808a1a3a0c450a9e082d13a0624cbf25a2cd8d6ea39de097001e97b8e5f39f5ac1496829bdaa8602402826aec5604caab7460b4ccd8a4ec" - }, - { - "block_id": "000f44b0bc91d26f1b492fa40e45a8e4e2cadf75", - "extensions": [], - "previous": "000f44afa8a61fcf313d1c73f8e3203125512942", - "signing_key": "STM8YQcoXk8V58tBsrrhixP2pkHM5z6tNbQu7Z8KfHSx1jCJnN82E", - "timestamp": "2016-04-29T04:43:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "troglodactyl", - "witness_signature": "201522cf73d7999b9324f7cf1d1f802b9345a1988ea5641e16041be39160ffc10864509eff4ac7533d9043dcedf1b3b61a6061c2d37584a59c7929f85b330e571a" - }, - { - "block_id": "000f44b1750b60d51ca174539b8db1540974e5d5", - "extensions": [], - "previous": "000f44b0bc91d26f1b492fa40e45a8e4e2cadf75", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:43:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f45feec720c0930b951ba5c9570671c47da02e1ad22f0eba06e2016e4855abd352ab1475ef36788fc3c3c6e93584e658ee223093a29077cdb70d72fa8d6ebb8fd" - }, - { - "block_id": "000f44b2f0c8a62bb02a718f003c7ca7c731d082", - "extensions": [], - "previous": "000f44b1750b60d51ca174539b8db1540974e5d5", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:43:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f1b824d59f79589012e0b9c0ea1bb03692c785af8cb939921511a636d40908fe71f32e098d71209222010fd59b5f347f78235e6580aaf0190c037125edf6e89dc" - }, - { - "block_id": "000f44b36770b3cadb6fb8ee6b6ea68e70841ffd", - "extensions": [], - "previous": "000f44b2f0c8a62bb02a718f003c7ca7c731d082", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:43:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "2033945274ca837dd37889a576a89c4e5cc6d2decb5960f87a26a54514086672d81ed15fceb2716da33edfeae8b8780add2d46f5165ef434e1e67ffcc789287a16" - }, - { - "block_id": "000f44b4cf0b1337d649b8a4b332cba9215c0bc1", - "extensions": [], - "previous": "000f44b36770b3cadb6fb8ee6b6ea68e70841ffd", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:43:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f16bc443324da5ed542ae50ffa1a756aecce5c362b404d04243b7a73d06375682366629a15eb9a54a7dff626a1f14e7312c139f9a93d1d93c1f15e8e92cef016a" - }, - { - "block_id": "000f44b5823dc4dd50acaab570b0051dca9483de", - "extensions": [], - "previous": "000f44b4cf0b1337d649b8a4b332cba9215c0bc1", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:43:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f2bccbaf5fb28906c147032dc9ca3120dea08f3264136c6de3004b25e1deb59f25f1e2396c19e87f999a3ecc999925dfd8730e43c926ae74bcd8cc3838e703ad9" - }, - { - "block_id": "000f44b6d32484b1920d2da7107cd5e82e08f580", - "extensions": [], - "previous": "000f44b5823dc4dd50acaab570b0051dca9483de", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:43:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f252c0a4d03671efe882df16693e54235c938f158e844dcf9f31dcbbc8aa76a20118ee991b7db5e500a04029f78bb1c7daef2a57e429aac3c2b5082961f24272c" - }, - { - "block_id": "000f44b764e0d945e9517f1ac677bce63da93ae4", - "extensions": [], - "previous": "000f44b6d32484b1920d2da7107cd5e82e08f580", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:43:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f3b678c6987db1c740b6bcf1319cdb5b64205c9f366c4a7f2d7a270d7d257abd353ae4c63fe7ae35eb07a25b67e62d1805232e615e004cf1aff1c4c301a8c21af" - }, - { - "block_id": "000f44b812ed4d6ab95d7d2c2a3a3bdf9625bf97", - "extensions": [], - "previous": "000f44b764e0d945e9517f1ac677bce63da93ae4", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:43:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "205afc87c40cd9f7cba6d91db97450258f193a627a5491990c9e530f3784dae3321df66bb45acff128910b370bc3d90402d28fe7b826f732881dae1ab24c1b6157" - }, - { - "block_id": "000f44b9b31327c080254b6142f1b01f5d2ea0da", - "extensions": [], - "previous": "000f44b812ed4d6ab95d7d2c2a3a3bdf9625bf97", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:43:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f5f07288477dd23ba60ed61d6d13e415e134d75ad379a090b82425f6521abdc9c7178d45966ce0a1311eeb7d4e132e4a4a83d0d27d125d2a0d7763af413d784e6" - }, - { - "block_id": "000f44baea1173eec4be598c9cb813c7c0ff8f06", - "extensions": [], - "previous": "000f44b9b31327c080254b6142f1b01f5d2ea0da", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:43:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f5f8c790017f7393a93a76c7a208f40f06545065a7b585e129add6dc623ee81d94f8dc5686a7346f7d32ee33dba856813f1d83f32d5ba94ba7f91e756d1b8857f" - }, - { - "block_id": "000f44bbfec074aa2911aa741abe482653a3e5de", - "extensions": [], - "previous": "000f44baea1173eec4be598c9cb813c7c0ff8f06", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:43:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "2016ab84ea2e66f8a0e67ff3b90a9086979f16b1159896c82e6ace890a8c0e874274d57baca9b247911070b13c1120e17d9050380328f9065f7046d15abfe4b379" - }, - { - "block_id": "000f44bc5621a937edcf211890e32ba0e830c306", - "extensions": [], - "previous": "000f44bbfec074aa2911aa741abe482653a3e5de", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:43:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "206fe35fadf9464309df3729cd683af61da0e049dd66633bc9fea14795fdd8cb722dead7d9935be5acce0c33381464c4423f313a389e25f300f1c9a3e563d4b74f" - }, - { - "block_id": "000f44bd8502fe345457274bda66d9c18a0af761", - "extensions": [], - "previous": "000f44bc5621a937edcf211890e32ba0e830c306", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:43:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f2b545e6f3455e65ca26318c68f2da672a088b1de4100040b863241366f3fcf1d7ae29204af78b9162518f6bb447614bef9fb1f7d0c12fd9a39847617529ba79a" - }, - { - "block_id": "000f44be26c4e2338b15f3b444d77ac4ab6bdb11", - "extensions": [], - "previous": "000f44bd8502fe345457274bda66d9c18a0af761", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:44:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f13d3b37b2f9972209fa1ce2f2107e2defac8677ab490f9a7f7c5751f2810a19647d1795991d0a2d6bdba7e6bca75700e5d391440b776a14f27a423ef5cf3da16" - }, - { - "block_id": "000f44bf5a738dd85cb03c8292510150110ba8c0", - "extensions": [], - "previous": "000f44be26c4e2338b15f3b444d77ac4ab6bdb11", - "signing_key": "STM7iLmTyCkA1vFsPEXE9PX9up2s8mVNeQKjqJi254zTPbj5ndAUE", - "timestamp": "2016-04-29T04:44:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "tipbot", - "witness_signature": "20321942a698bdffad968c1de2a7a5f3b7b8678fb8dc75410f0d9b4cc5ced758283339895d126a22cdbae2535006ff78f792b8942f8883a2f1914af77ea98d1ebd" - }, - { - "block_id": "000f44c065773e61b269b034978c7b56738ae8fe", - "extensions": [], - "previous": "000f44bf5a738dd85cb03c8292510150110ba8c0", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:44:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "2028945dcc1f432e26dab0eaa93b3bc02167d957767af0030a5bf8833302df461e7c5626032478342855354609554d69b6408de852de9966bd5b333ac0d40aa589" - }, - { - "block_id": "000f44c194a34c607134c8b8bf233e0aae333db5", - "extensions": [], - "previous": "000f44c065773e61b269b034978c7b56738ae8fe", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:44:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "201fa009bb58dd9bc794464f6468ff333b4832ec5e3946f23d28caf18e316cab1157119d1de41aafd2a8ce76e1d57af1b49cf44a2fc71e4df48c40e41906b14a8e" - }, - { - "block_id": "000f44c2c6d6aa3783ad7053a94c8615dc9cb307", - "extensions": [], - "previous": "000f44c194a34c607134c8b8bf233e0aae333db5", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:44:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f068d0f2cb1f9abef7c6534838e61c565ed6938ac3277efc7f785635a6c098e592c167d0859b66e2768b2fa01f779c3fc69559d7de6146eeca471d689e6ae1106" - }, - { - "block_id": "000f44c3efbefc8a53abe6e6d02e1e59555d0735", - "extensions": [], - "previous": "000f44c2c6d6aa3783ad7053a94c8615dc9cb307", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:44:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f0e287fa12c93b0aa3777381eb807a10e8300228ccf2356f110b69ff11c4ad1861ab492770164c581bf0c11b9281b3debdb9e31eedc0aa05fcedababfeb5d8345" - }, - { - "block_id": "000f44c4403c4bc7779454f9de58cf7f38354d8b", - "extensions": [], - "previous": "000f44c3efbefc8a53abe6e6d02e1e59555d0735", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:44:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "201ff16f432d081a05849709dee277182dd5694c2bff9cfd592e6052a50e0ff9f441a6ccac427178b36d41b1c89dd57dbc23a5e0e5798ebd02f5675aba37bb9d6e" - }, - { - "block_id": "000f44c56db744ffd5cb5de604c4be80db289995", - "extensions": [], - "previous": "000f44c4403c4bc7779454f9de58cf7f38354d8b", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:44:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f2c318ca96acddf70f055b7e70531c4e74f4bb86358ad9bed931a454cf69cbafd7a3cef4ba5e0f5fd62233a93c026da35f2a734aeac32780f2d3c9f12a5cac9ba" - }, - { - "block_id": "000f44c64772999c8a7b0a07f1a2b21b019239c5", - "extensions": [], - "previous": "000f44c56db744ffd5cb5de604c4be80db289995", - "signing_key": "STM58QMgvDU4W88PtpEpy289EPKqUC2JELXeNGm4Vm8EgzUP43DHn", - "timestamp": "2016-04-29T04:44:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "delegate.lafona", - "witness_signature": "1f66a0004af24ec06d55bdb6502ef1d0e360e6c9e3fe50c009393d5d0150e22d47776c0d1bee59d1d4dc6295c9bd9c997ee988050dffaa33a625fb5a9654529300" - }, - { - "block_id": "000f44c7387d63ae6c9e060dd0a9107ab81fcbe9", - "extensions": [], - "previous": "000f44c64772999c8a7b0a07f1a2b21b019239c5", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:44:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f54d9b7303d821d8811d3c7c2cc4b1e71f9f39524827a036b0777244a6d767d1176412f1fc7e282cc9adf56da2d3286b65449e805f727f036d7276c4ac2bf9be7" - }, - { - "block_id": "000f44c8e9e911600570c9f9e210e366c3c00a9d", - "extensions": [], - "previous": "000f44c7387d63ae6c9e060dd0a9107ab81fcbe9", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:44:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f3b4954b0f8f5e7e4dc4813170f696179beb551a3de48d425b35acf9ca8f304795df44c1c46107df281ceaa286d372f21d805203337fee2d682c9eaf81e0c1b0b" - }, - { - "block_id": "000f44c93b56309282f21a859d3dbf70c22c57f7", - "extensions": [], - "previous": "000f44c8e9e911600570c9f9e210e366c3c00a9d", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:44:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f15915edcbfa73706b71132af02fb984c679d31c0445632dec148f3ac62d210ce564871e9b3e5232d18ef0a7f882ee986a3810c889fa8c23ed764164303ad3576" - }, - { - "block_id": "000f44ca7b88626b3e3977f1ddfff9b975290828", - "extensions": [], - "previous": "000f44c93b56309282f21a859d3dbf70c22c57f7", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:44:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "2007e6310b3a7c310c7f9bf67e62009f1178a1e37d5d8efba298e872cbdb1e6e61723389f7fe838cbc805bc00a49ad9734d600f67cd52fefcf40804aa3ec03b454" - }, - { - "block_id": "000f44cb04e7940505004154e4ae8e2b8038e2a5", - "extensions": [], - "previous": "000f44ca7b88626b3e3977f1ddfff9b975290828", - "signing_key": "STM55Q6YCSqAE4F447VZqYARBYGJHEzyNTbxWMbTU43qs6dNeHuWA", - "timestamp": "2016-04-29T04:44:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "butterfly", - "witness_signature": "20159593ae558848592a0dd04a13163c80e2d63e0d9ab8f7cbb986216849df4edd653cedf65125866d3ba0c82fbf97c338f9f33eee199ee947e35c82821d5e7a0d" - }, - { - "block_id": "000f44cc0c853b755988964d9e655a6456261b8a", - "extensions": [], - "previous": "000f44cb04e7940505004154e4ae8e2b8038e2a5", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:44:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "2058e7612c45ea4a84502e0f7051bcfde74c330479d43acfc69adfc445decbfbbc77381fd37a6881c65230e1a71959b0ddecee6291b1fe29f72b62e2c10e8d41f6" - }, - { - "block_id": "000f44cdcf2d8c6d65b22d7a8be1a9a187e7f382", - "extensions": [], - "previous": "000f44cc0c853b755988964d9e655a6456261b8a", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:44:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "202fa48b35dc152645bef0a6c053c372191ceaf706a354982d8704cd614c1eead14126cd1681a5b3b4e2e4b7a7e41ac32bac31ccbe7edd09a1a916aef42468b2a9" - }, - { - "block_id": "000f44cefe9e2f8f6b384731670ff301e0379e97", - "extensions": [], - "previous": "000f44cdcf2d8c6d65b22d7a8be1a9a187e7f382", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:44:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "205b8ed39143df808e8fe88d7e0dcdc298273643e9802ecc66a11579f7e862fb931b26dc8f0fec916359b1a1de80fc72f28c02aeb181d24d3c69724404c3265a74" - }, - { - "block_id": "000f44cf6504e496d58affee25e7c95df52c5cf2", - "extensions": [], - "previous": "000f44cefe9e2f8f6b384731670ff301e0379e97", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:44:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "20232d254f2443767ba5afad0eef95ccbecd01b80c0103ae77e9605d4688a316180f3f29ac9820a550db6c8573081db7c142a06bf4a865e5c2dc931fb4b573ea14" - }, - { - "block_id": "000f44d0f6db3ce14b0cb464751497654376a53d", - "extensions": [], - "previous": "000f44cf6504e496d58affee25e7c95df52c5cf2", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:44:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "2016dd11c99d6a6aaab0c59557c03358f43a458400d30da1c7975f4a41eb6010286a37e82848fa2134c92937bfd8a9c09765643e5ff3609f1f45bc5b3918cc5979" - }, - { - "block_id": "000f44d10b953de216522516c1146767a1160172", - "extensions": [], - "previous": "000f44d0f6db3ce14b0cb464751497654376a53d", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:44:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f3853e003fe2eb5afebe0454d61f0b611ba42f2758be2c80a48185dee2b8ce21a7bffff129f3b84977492b7bbcda41cbaf2ef8931a5de49b61f9fb9162296d8eb" - }, - { - "block_id": "000f44d2b9b32b092dc349eb0e2f75085bc48828", - "extensions": [], - "previous": "000f44d10b953de216522516c1146767a1160172", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:45:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f6b09852776ae7f7621fe2dfd9e14c4b4fa2a3df2e9fa7b874c9cce7eb5c30d7c166204b17f805227c098d1694a89a9283b18f437aa7bf8ca7a2a42f82fb669c7" - }, - { - "block_id": "000f44d3fcf84f578b93554e688308a1744e6fd1", - "extensions": [], - "previous": "000f44d2b9b32b092dc349eb0e2f75085bc48828", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:45:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "205e637d60951af170aefe64c016016de13b615c70a09b3ed93e6935b29ef0565b40920aaad07de23c02447a47b1e7521e54e842e1b6c971033cab668ff1205ccd" - }, - { - "block_id": "000f44d4f379c4f579ea5865bb66322d9e38b219", - "extensions": [], - "previous": "000f44d3fcf84f578b93554e688308a1744e6fd1", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:45:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f622c7f911f7cff743b15fea1e1659211db7cc2a6ee044ee82e408ed17c149bdc11de44dc109b67b89f2d4a4c728620a633f4666a446341b35d4f6b7ba3e313d7" - }, - { - "block_id": "000f44d5cfdcf2d799c1fcbdb3d11ba1c3ba1f21", - "extensions": [], - "previous": "000f44d4f379c4f579ea5865bb66322d9e38b219", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:45:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f42e5fb2a11080412b89c375c368ec0d45bbb07461060ef4f26bf5d4c13d6b289525d3553e807307530bd72ae7610dced61465c748c713a581d897cfebb81239b" - }, - { - "block_id": "000f44d65b5f54e77bd6ab9e039e754bf84db01f", - "extensions": [], - "previous": "000f44d5cfdcf2d799c1fcbdb3d11ba1c3ba1f21", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:45:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f2932ec24d0554c0ec08ab34489a0fb7565ac5286f007697c767e02f2c4504f1601b9c357412b8ff4181eca044b9f06f33b4a76bc44622baa8bc2f6837a0f3a1a" - }, - { - "block_id": "000f44d7be409c66d33d94a9b848ef8002b25f25", - "extensions": [], - "previous": "000f44d65b5f54e77bd6ab9e039e754bf84db01f", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:45:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "2073ad5671cafdcf7c9e8b32d4cc91330acba5c8f49b94e301aff5039760ea7eeb25918796d5b03bd3fc9cbc0e0bbcf14972a235951e466204107de3003109f3d5" - }, - { - "block_id": "000f44d83b82bf1e5db0ebd0adf61b66d14273c9", - "extensions": [], - "previous": "000f44d7be409c66d33d94a9b848ef8002b25f25", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:45:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f7064e7e9fa0bda56f4509a07c9491b58d25a4045ff86fcffc6de144d38adf2f3701cd48de25ca91d443b5f1e3889eaf4185f62c93eb7ad75b289f2aa870f9387" - }, - { - "block_id": "000f44d9d0056fdcc4e8164fb2507d999bad3c72", - "extensions": [], - "previous": "000f44d83b82bf1e5db0ebd0adf61b66d14273c9", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:45:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f5e76130015bca0b659f67b05873555928fc1f94c093b209bd5a96e04bb491f6172ec495b99eeea38b70d1458c698402bf94983c31022767388a16558c69f508b" - }, - { - "block_id": "000f44dacec3dfa1f7299733519ec00dae588fed", - "extensions": [], - "previous": "000f44d9d0056fdcc4e8164fb2507d999bad3c72", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:45:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "201c2d108ba5084c1843070294b41ff1a188a43130d684786462686c4fce04644c432700fa6829ed4a381596a35dfef9830150c140e96e25405da03dab1e787c08" - }, - { - "block_id": "000f44db592cde442e773f68f0f37585783245d0", - "extensions": [], - "previous": "000f44dacec3dfa1f7299733519ec00dae588fed", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:45:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "2003b40c466312e75bc6bc5af2b7c3f3703e03fd504b5afe9d67f535cb7c90e31c622b9c9a37997efc264466fb3cd52c73441ec62b9640b92e22643d81ad466d25" - }, - { - "block_id": "000f44dc1f5ee48ca0a5cbc3ea6db5b1a8c7d83e", - "extensions": [], - "previous": "000f44db592cde442e773f68f0f37585783245d0", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:45:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "20031422dbf83058d9a2c543bbf22c053177a98f245dc608002e043feb74d80ec61cbe8659f0a66589eb3b7e63fe1be7114bb0e6d3092b4d3bf93d8d5637bbe69a" - }, - { - "block_id": "000f44dda254a62a92fae6ec2c0f19da806660d8", - "extensions": [], - "previous": "000f44dc1f5ee48ca0a5cbc3ea6db5b1a8c7d83e", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:45:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f13cb61a35cfce0e5a80c62dd83f95dfd8e09c768075715a2bb862343dcfd19ce4d6350ac7ffaa3f298ba05a79c2131cc5c212bc595f2122e1cccc5608796f81e" - }, - { - "block_id": "000f44dee934040d841a8495a6642e7ee0c045bf", - "extensions": [], - "previous": "000f44dda254a62a92fae6ec2c0f19da806660d8", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:45:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f43820bb68b237af42620b8cf0731e7f8e1e99ee2c7a4690882bbd29b2aaa3403680e64a150d49ac900b29991735123c5d8b1c307727cd0663df5deac32746b56" - }, - { - "block_id": "000f44df6fb139c6e748c512a485aff702bb6ec0", - "extensions": [], - "previous": "000f44dee934040d841a8495a6642e7ee0c045bf", - "signing_key": "STM55Q6YCSqAE4F447VZqYARBYGJHEzyNTbxWMbTU43qs6dNeHuWA", - "timestamp": "2016-04-29T04:45:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "butterfly", - "witness_signature": "1f52d61d8b56a17812b02cf683d43e34462392360a51a8d9e9cc26b7dd9e68ba71334930f7a41ef1b1681370b033dc3512e3d14b564f46b8c75589f52d754a7066" - }, - { - "block_id": "000f44e0d90eaa9b34168dc4cc04d4b032af1227", - "extensions": [], - "previous": "000f44df6fb139c6e748c512a485aff702bb6ec0", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:45:45", - "transaction_ids": [ - "a5da0cef5e747dc676c56039ec5bcec0ef440865" - ], - "transaction_merkle_root": "cc6b9df88bc6934eeb28a2a184c3a60246131a78", - "transactions": [ - { - "expiration": "2016-04-29T04:46:12", - "extensions": [], - "operations": [ - { - "type": "comment_operation", - "value": { - "author": "steem-id", - "body": "I think its because mixed content protection on steemit.com. Firefox automatically blocks insecure or mixed content from secure web pages. We need https image proxy similar to github [Camo](https://github.com/atmos/camo)", - "json_metadata": "{}", - "parent_author": "dantheman", - "parent_permlink": "re-steem-id-re-dan-re-steem-id-steem-seed-node-list-20160428t165903001z-20160428t235939283z", - "permlink": "re-dantheman-re-steem-id-re-dan-re-steem-id-steem-seed-node-list-20160428t165903001z-20160428t235939283z", - "title": "" - } - } - ], - "ref_block_num": 17631, - "ref_block_prefix": 3325669743, - "signatures": [ - "1f087475c5b773eb5771bc95e72652053305a1d95d564107158fe954dfe0040f816a6db2b2d80d6b5a1e10ae4ee9ada8c1916c13e214925135a8a1b91bdb41df40" - ] - } - ], - "witness": "steempty", - "witness_signature": "200aafdaca1464672f17b967c3ad863d18904c0e4c89875518ba696ea5197d131d658f075ce18aa7571a85449853cd7547e076b1c1b45e8003288030ec927e6c77" - }, - { - "block_id": "000f44e1cd8f47533f57144554f816593f93c1bf", - "extensions": [], - "previous": "000f44e0d90eaa9b34168dc4cc04d4b032af1227", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:45:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "203d8dd86afc3aac0bf8b59381d8180dd2faf31e47ce687cc88d7fcd9570ce6c711a810745fcd2b3852e030807ce187e8c94230e1ea519995e9a103b0c4eb11adb" - }, - { - "block_id": "000f44e297db32a06d39c4c8dc8725517b91d2fc", - "extensions": [], - "previous": "000f44e1cd8f47533f57144554f816593f93c1bf", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:45:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "205ba3578546143c3f985faf134ab42699781df0792ca9cf5b91058c4962a544480af66e24c8fc7d6f1f23b70203e2828f9f784038ea9ba90f1e75bb85e63eecc7" - }, - { - "block_id": "000f44e3fed2a6cff44d8cb206e73fd989adc89c", - "extensions": [], - "previous": "000f44e297db32a06d39c4c8dc8725517b91d2fc", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:45:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f5f7ac60667f3cfc2b763f013811240fb438c3a7893335cd32860bc1e2d185d7f68ab048606bbad813fd8ba24e3b0499617f94682204b8a0a7ebc01d02b2100ab" - }, - { - "block_id": "000f44e4cd39441b1505a98acb3eed88898224c8", - "extensions": [], - "previous": "000f44e3fed2a6cff44d8cb206e73fd989adc89c", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:45:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "205d7648f418c2401ab040a3f9dd89f0bb9984a8d4d01ca4fecfaade069a0586a933ec2833cf013c70ee742056358872f21966e85e41aff92620802170fe9c5705" - }, - { - "block_id": "000f44e5b1219df1a19ab991b4ddcd224477dd95", - "extensions": [], - "previous": "000f44e4cd39441b1505a98acb3eed88898224c8", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:46:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "204a134b2b38d24a47dda61b8ddb6d532b8d4c751498594e1966503d116460e7b700af1da74ae73fdbfa9c6899c17092ce64fc2616240e07cdd00ef24706e4c7c0" - }, - { - "block_id": "000f44e6a23d6bad800138b39849cb42b064b241", - "extensions": [], - "previous": "000f44e5b1219df1a19ab991b4ddcd224477dd95", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:46:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f717a6bbe577465ef93c07c3ddaed879dc3d93362804c21ecedb896eddd0a1622362e90fdd10e763ab3ac7b41d1579e95c11ffef63f7e5f3c57c73d97b04423cb" - }, - { - "block_id": "000f44e7ad117fed7a9dda30149a81294c12fe57", - "extensions": [], - "previous": "000f44e6a23d6bad800138b39849cb42b064b241", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:46:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f4744404b64b45bd318b0b6f2d27b2b284dd093a6887e7f2046810fe852733e952a0af41c4c5e7b4b4c77e6fcba2ba7777b0c366b81721604a2950d9d7b6892ce" - }, - { - "block_id": "000f44e822cd218345430072a4681a5a763c42b6", - "extensions": [], - "previous": "000f44e7ad117fed7a9dda30149a81294c12fe57", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:46:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f24c206c3143fe0006cdc135c28cf6048ca9030ebf0df56a563951e4ef95f97ce26adaaa78dc950e9561e308bac3cdfe7f499b6cc4799b77521e39facc6f6ef2d" - }, - { - "block_id": "000f44e96ab34cc703deafc4a80b1680e3229c47", - "extensions": [], - "previous": "000f44e822cd218345430072a4681a5a763c42b6", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:46:12", - "transaction_ids": [ - "55f7cff00768f808e24af8c28d9893574454faef" - ], - "transaction_merkle_root": "5c2c7f2629630969f2209151c63bf550b97f982a", - "transactions": [ - { - "expiration": "2016-04-29T05:46:09", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f44e822cd218345430072a4681a5a763c42b6", - "nonce": "6280519643775766609", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "3213b1fe26620f1f9d0a0b412d91f4d781689b6022d82a031839f15b64f7444f", - "signature": "1fa13175bf89808ada480349f0ebedb359f4dfc9189bcef2ad7f8d680af90ec8983c450e08ada6176b9b41eac5ce2b5478f4ec2d8e7b917f35a953dbdfe815102b", - "work": "0000000987076a3c54dca4283dc35ab5fe7a0a4c0da37e926ebcf523a188f674", - "worker": "STM5UVXLGQA3pD9SRxsdtQLrAKrYDtMoe5qSU5innKqxvjQ9Kzd64" - }, - "worker_account": "books" - } - } - ], - "ref_block_num": 17640, - "ref_block_prefix": 2200030498, - "signatures": [] - } - ], - "witness": "witness.svk", - "witness_signature": "1f56c3074791dd91005b878305afd604bb14a539c57fe0c1668ef421777394a9806df8e6cebec1e007c2871626be44bceb82f7b95e090c594a018147f19b4808e5" - }, - { - "block_id": "000f44ea6c9f47073479f32cd38c49792aa2af88", - "extensions": [], - "previous": "000f44e96ab34cc703deafc4a80b1680e3229c47", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:46:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f40e9963d252ca158377edcd839c7a3bd213fc7af13b064e731bee60d631babf26917a6494121102e788b18675f61f864d4b4ce34d72764a6bd6b3c623ddbb53b" - }, - { - "block_id": "000f44ebf8fc2c685b46d18b480c06ec9bd41538", - "extensions": [], - "previous": "000f44ea6c9f47073479f32cd38c49792aa2af88", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:46:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f5925a4060da6762e2a9bf705421a4fb8b1a7e06971dcc36e5c04eddde9d6bc3154a52a49aeaf9c195c3cd4a7a4d017294d4b4d67d8ac6363fe732887afa94794" - }, - { - "block_id": "000f44ec5cba8a1fe84e604dc835fceb927aa336", - "extensions": [], - "previous": "000f44ebf8fc2c685b46d18b480c06ec9bd41538", - "signing_key": "STM7zCGUYKYNsJEDnrwCEU2BwPdNQmFj8NQ1TEhPdVSUgneNMRUEU", - "timestamp": "2016-04-29T04:46:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bue", - "witness_signature": "2055a5fef1e578b841a9c644b64224d7096a7fdc11d95848cca63f6f0dbab0b2f62e9f0df438327ffceecef08877ca386845c56903c1d50b4abb5ef42f73227e4b" - }, - { - "block_id": "000f44ede47f9b92d99471631e01edf6b1d6e31e", - "extensions": [], - "previous": "000f44ec5cba8a1fe84e604dc835fceb927aa336", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:46:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "20191c07f654a735593ed8f48cb7314c99929dd37fe45da0fe1be34153804de9940c313d192432e68f3c914c98dd1332dd1963a8f2516ec515fbc023b8a9f99e39" - }, - { - "block_id": "000f44eeb15d0ad0a17174ddf553e5c274757eb0", - "extensions": [], - "previous": "000f44ede47f9b92d99471631e01edf6b1d6e31e", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:46:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "201041643b58f303ef1f9c511b0348430bf806ade6355e239ccb5d3476842c4ec42cfe5ce4d29616238f401d111090615c513890b7df186855c7b09a4428330a81" - }, - { - "block_id": "000f44efca4c2f39dd1c9950efc8e4f4a6c7a22a", - "extensions": [], - "previous": "000f44eeb15d0ad0a17174ddf553e5c274757eb0", - "signing_key": "STM7MywNwp43UKXTv5pRWdNB4oQhZ4Tne9LAKDbhVju2vv6xUiLzW", - "timestamp": "2016-04-29T04:46:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "adrian", - "witness_signature": "200f88ad1625561e2a044614bd70b27858acaf20adb57c6cd1740ebb1eb1b985ce764b03f2931896dd2c141e5476364b8b872cadbac293c2c613dbf65169ffa137" - }, - { - "block_id": "000f44f07ba56ce198cc4bb169682238d243a21a", - "extensions": [], - "previous": "000f44efca4c2f39dd1c9950efc8e4f4a6c7a22a", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:46:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f5fc3214e0145ff358c5605b44457904ff35197f1a843cd94e5cc15c2e45506ed67c437bf12696a5af9d031f0fa969d13d7aa98c78f94b4c175504176a38ecaf4" - }, - { - "block_id": "000f44f1828f116ffc56600b700057dd3e4687a6", - "extensions": [], - "previous": "000f44f07ba56ce198cc4bb169682238d243a21a", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:46:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2056ac3585b4d4336df60125ae880abfb03410967b60caa4aa1c299f75b86138ca122213058e8029b5bb692b360c52e1bc1af5a28ef4f33c81b43037a8af727271" - }, - { - "block_id": "000f44f24e0e771a1ac4697a689ba239b51b408a", - "extensions": [], - "previous": "000f44f1828f116ffc56600b700057dd3e4687a6", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:46:39", - "transaction_ids": [ - "ac63034d6e6e9fa4e9c38d8fd26201e25741ce98" - ], - "transaction_merkle_root": "7a2abe2315efb890fc7fb1c68614a156fde27788", - "transactions": [ - { - "expiration": "2016-04-29T04:47:03", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "429", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "dele-puppy" - } - } - ], - "ref_block_num": 17648, - "ref_block_prefix": 3781993851, - "signatures": [ - "205c2279a070e6fc0bb40e4b254ce2c3e234eb370e6941a6e041ebeacd930e5bf32052003d8ea2f8302cb14bcc3bebfce165e3ebf6d007d43dd1356e883f78ac34" - ] - } - ], - "witness": "kushed", - "witness_signature": "1f196e02149f24ea09eb967ec35a6b9d0912d0895db865b982997803d97d9ed9ba5d9bb5dc6a23938173825040b191ba1455b16e904ace1b4dcbb93270734a2eb8" - }, - { - "block_id": "000f44f33111a74a509c506432b9ac8fffac8ea6", - "extensions": [], - "previous": "000f44f24e0e771a1ac4697a689ba239b51b408a", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:46:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "20056e329f081a7b7f901f787b15c1a23de132e5abbec79bd0461cf5e361a8a79579c95adc573f153a91c32a9bc5bec640321065ab61bc47fef71794493cb0e3be" - }, - { - "block_id": "000f44f45af1fa68ed2eddecd879b6f730d75ecb", - "extensions": [], - "previous": "000f44f33111a74a509c506432b9ac8fffac8ea6", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:46:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "2057694fe0cd54562576e927447700537e5d1abc33f85adfd96b40305d01a59a122b8f580627c8e3cd0c0769ca8a7dd87a6f00c52634c46f5896a7782d18d8f36c" - }, - { - "block_id": "000f44f54bb5c5bef9adc55274986290386d4848", - "extensions": [], - "previous": "000f44f45af1fa68ed2eddecd879b6f730d75ecb", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:46:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f5604910e77947553b5ea1a50a1d7e352ce1ee29d93fd21342a8480371728e54643cb1c3fb49401a5491d1c8f0e9bcae8e417fe4845e2ff8a1613fcdb4d4696e0" - }, - { - "block_id": "000f44f65a00b93ceabc35fbca4420ee56802c67", - "extensions": [], - "previous": "000f44f54bb5c5bef9adc55274986290386d4848", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:46:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f16f32a6eebd8c9f2eeb37a8b746f7b02518746b094efccbd4bba9115b7ed238952dce67c4e6b5c0a3fdc56c1c406ff9f710ea9151e2ceeb333b0fb028e4cc186" - }, - { - "block_id": "000f44f7801b00da554d78ffe7e54593fd91d0eb", - "extensions": [], - "previous": "000f44f65a00b93ceabc35fbca4420ee56802c67", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:46:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f33eec63c06ac907f2cd343b6e796c72bf42b93966e57aeaabadacc4231799c4359c3b60fccf502e9bfc8fcbde73725ebc67aa4b59d9dccc2389e0fc4086dc93f" - }, - { - "block_id": "000f44f8976532a6a8d64edc37d5ad34258196e8", - "extensions": [], - "previous": "000f44f7801b00da554d78ffe7e54593fd91d0eb", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:46:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "200a5beb55d7f08112d30bf8b97a8a76441be1324a12ab0e780a6685d73a92450b34f89749deb5f6bd72fec02388a445929a692418c08f549654605c76ae24b985" - }, - { - "block_id": "000f44f9a3fd5f50156974a3dc18b953b43f5145", - "extensions": [], - "previous": "000f44f8976532a6a8d64edc37d5ad34258196e8", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:47:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f16147af733cebf29e1e2b6b00303641ea94d8162a6166c4e60ca07a4b28f03f755a5ee748868f391e20479dc809b2ca008417a32de0977d887d94178fcb959c7" - }, - { - "block_id": "000f44fa6d0937137f251a7ef6382313d9cafa45", - "extensions": [], - "previous": "000f44f9a3fd5f50156974a3dc18b953b43f5145", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:47:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "204b82fcec0f0a2180b8d0bd66da7bba8de269e7860213333f81a5e162c943f66b2438c8c0ce63dd52b257dc856291eb75620be21a38825b9ea0694ca25916934c" - }, - { - "block_id": "000f44fb9b043304b42f592d440e1ea4c8d7e239", - "extensions": [], - "previous": "000f44fa6d0937137f251a7ef6382313d9cafa45", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:47:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "204712f227cfb04dcc70db3173720206e305fb1afe4977052f6f32dfccc1fe43c538adba72c40076468d6656f4d85b14a7831ed03ead75cdaae0b901bb1ad3f22f" - }, - { - "block_id": "000f44fc55b91c3e16aebee974f7304e20427258", - "extensions": [], - "previous": "000f44fb9b043304b42f592d440e1ea4c8d7e239", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:47:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f0d03a04169563cee894784e296a126655a7cda19da6f2ad34a8792a3585ccefa5b876f2edeb1433f77fb0c9a5525eff14ae088cbe180528bd5b6c80985a7d0b7" - }, - { - "block_id": "000f44fd5ed1b26f008dc7c03b29eacb2b880d89", - "extensions": [], - "previous": "000f44fc55b91c3e16aebee974f7304e20427258", - "signing_key": "STM6R5B5uF4hdUvFJFtNJbHd4CHmpgSFN3EA7yzaM9uF7HeNPwRN4", - "timestamp": "2016-04-29T04:47:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr602", - "witness_signature": "1f1c6acdb33717dfacbf5e8fc422bebfb4038c9629eb97589084aa05c7a0ce706b65b79028e9deb6df340b2ebf3bd996b96a1490dcf5f1ba2b85eb1e98ca2219a5" - }, - { - "block_id": "000f44fe2004878928c56e163b47b363508a202c", - "extensions": [], - "previous": "000f44fd5ed1b26f008dc7c03b29eacb2b880d89", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:47:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "2020fc2513c7043d510a368f3aed6332195c3e974e1e62ac974a7dc3f4ccf1c3797437345e14a6cc4b67e960c64ee3f800fa94b57630c5f50156f78610ad8aa20c" - }, - { - "block_id": "000f44ff974baa548a89e5b55f298c13ec9c63d2", - "extensions": [], - "previous": "000f44fe2004878928c56e163b47b363508a202c", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:47:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f1cdfe014a38835e1a45011bef9a5ecd14abbddceca1258582f3fab30e68cf2bc5559d765288f5efad38e3e5c79f68ce2368c6f8fa477e9df3a21a9d0bdfc6186" - }, - { - "block_id": "000f4500ac9bc9c4e2e5bcffe9d8d0de1353c8d2", - "extensions": [], - "previous": "000f44ff974baa548a89e5b55f298c13ec9c63d2", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:47:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f4dbb92d59a4b4590b4a116371ef688e469cdfa691ff60024b3eace3e6ce3a45821f59080fb6f2383fd484e3e320e8665b0ba11edc475c45e7ea45c12bdc15757" - }, - { - "block_id": "000f450109235794b674f65f28001e3d11680931", - "extensions": [], - "previous": "000f4500ac9bc9c4e2e5bcffe9d8d0de1353c8d2", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:47:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "20789e255f269d66319b503211d8d3ec4db24d76355ced8f8bf1eb1a2f829541c57b8d6d597b09e9f662812f4419e6f8f9c7d143967be4160bff3b8876d669524b" - }, - { - "block_id": "000f4502c63d62c18eff8e30341f70b0d52547ce", - "extensions": [], - "previous": "000f450109235794b674f65f28001e3d11680931", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:47:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f7107bbc942bebb4f595e084649be3f8f0cbd9c08f2945c43438313c12631d37075dbda877580d30382602b5f8db31eb79305f2fd199c28826d3f8412f7cbb1d1" - }, - { - "block_id": "000f45035f2aa1c64066b2ffb2eef56607c96961", - "extensions": [], - "previous": "000f4502c63d62c18eff8e30341f70b0d52547ce", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:47:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "203455659f8c23b6d26df58a9658b68881e064da3a46ca021c5fdc4cb3cbeebfd96108abe369ec7e91906df277891406e74937e108a8a57837bc623a0a2ec394e7" - }, - { - "block_id": "000f45044e020845d17fbcf20f8cf86dd2b94510", - "extensions": [], - "previous": "000f45035f2aa1c64066b2ffb2eef56607c96961", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:47:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f114a27b30a9308f3582f5b67593874874b21bf0d28bebec9f09b2ed899cab74a55af07465f6f18206a726160d58bf77f804559a842f320595d79555efdef0bf3" - }, - { - "block_id": "000f450506cd8c686f4bd0f02f36cbd798b9586a", - "extensions": [], - "previous": "000f45044e020845d17fbcf20f8cf86dd2b94510", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:47:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "206d4c8215a13b4e0be3910903a6d9561a97cc8ba4879abe5dafb692c34857af3d75a5eb5a46dbc7e55b361df8cd8fdd0f359acbe5a56bf169877ea7625acc3b3b" - }, - { - "block_id": "000f4506bd41443bb7fd6e0efa9c5419dcb474c8", - "extensions": [], - "previous": "000f450506cd8c686f4bd0f02f36cbd798b9586a", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:47:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f165e1acacacf0ca1f073ca21a4c7e11dbbddbb446e9f48ce2b7b0a7fcfba69d71efc66a6dce6c7f921f0d7a967576b969d998e3fbd980148dea9c9ab6f0e7ea8" - }, - { - "block_id": "000f4507c8128166eab1b8cb8fdc84f079807754", - "extensions": [], - "previous": "000f4506bd41443bb7fd6e0efa9c5419dcb474c8", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:47:42", - "transaction_ids": [ - "a357b2964ce64dd6de604870f8fba23470ce4791" - ], - "transaction_merkle_root": "775c072746df6a79c36a40c6e64be6fce049b0c6", - "transactions": [ - { - "expiration": "2016-04-29T05:47:39", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f4506bd41443bb7fd6e0efa9c5419dcb474c8", - "nonce": "2389133005985303354", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "7bdfaf0abdcfb8155643a5da3f93a8317ea28e0820eee83afa50e191b95690c4", - "signature": "209115e7c2bf055cbdc8f534dee534114d6e228b6c3fddb49399e0d48c6aa096c56df6f1a86b5382cefb4e31f2fc7d5ada6885d9b14880e46a9bbf5328b770394b", - "work": "0000000fed7a02ebda1d6e41dac51e6aaae8c60eee41f9d719b5e093ad60b04e", - "worker": "STM8KTqm5g3i7wRTpwPsDdxUTNBa7CTJUtjuX7pAWUELBZjBQfwK2" - }, - "worker_account": "daryl" - } - } - ], - "ref_block_num": 17670, - "ref_block_prefix": 994329021, - "signatures": [] - } - ], - "witness": "kushed", - "witness_signature": "1f38c48d1895c430e09073008be60e92e95acaff89ef376859aadd8679d3d5b9f35ba750101bfb053f0a9a9fd5714ecfcebd4d25e148e4bdae92dc3b7aeeef8764" - }, - { - "block_id": "000f4508b0d508a031eba3e074693c8dcf26dc1e", - "extensions": [], - "previous": "000f4507c8128166eab1b8cb8fdc84f079807754", - "signing_key": "STM73XgqmDfLgXVF5VArK9rvHbUDbbx3HtfCU81Fy9MqVNxLYMpay", - "timestamp": "2016-04-29T04:47:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "jabbasteem", - "witness_signature": "1f3b1fbb910e95064cfa8935573d0106ee24adb5a9dbc0911bc00cd3352100a794550a18315ee703152385494849f7b6f8578b7ba5505474d758f83d7341fec11c" - }, - { - "block_id": "000f45098c2779d84a943e55cc37f65702901922", - "extensions": [], - "previous": "000f4508b0d508a031eba3e074693c8dcf26dc1e", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:47:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "205a098b08861dd04590fe4be7afe11477ebdcbcb4c5d3e8752ddaf37fc5478899188c1385c28552946451920e117204588e37c0d247549c28362d9ab7f37653c2" - }, - { - "block_id": "000f450adc9ca9e5681771b9fd61c6062d4d8250", - "extensions": [], - "previous": "000f45098c2779d84a943e55cc37f65702901922", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:47:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "202fe1a66a3fee930d430a71b880413095d7e68c35f2399a7b5dfda5c448e5faf93218444d66614d5284e82514f9066a90a1cec7451f7b45f2a0099999dd953b1b" - }, - { - "block_id": "000f450be5efdc981d2c44a838aa702d08662987", - "extensions": [], - "previous": "000f450adc9ca9e5681771b9fd61c6062d4d8250", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:47:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2056d6c7b6a26b686f7175f939c66b77a210e4a835f9e211d0b3ed882c1467d10f3f4f5f96d028ced0f448b73dd223aaf402cdc1d25f764ff7a382ad0c35a64686" - }, - { - "block_id": "000f450c3c18f6913465164c5d4c7267c64416f2", - "extensions": [], - "previous": "000f450be5efdc981d2c44a838aa702d08662987", - "signing_key": "STM8aUs6SGoEmNYMd3bYjE1UBr6NQPxGWmTqTdBaxJYSx244edSB2", - "timestamp": "2016-04-29T04:47:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "ihashfury", - "witness_signature": "202be2ecbb65152b129ec76ac757c257f7bfc8198f36ce6a329b27bbc4200c77550dd4f4633c34a24e6ee757dc77179aa9a6c1a2862bd90a993a9bdb75b6ffab86" - }, - { - "block_id": "000f450ddd4b1d7c8a71896b94f6ff4b3df86b00", - "extensions": [], - "previous": "000f450c3c18f6913465164c5d4c7267c64416f2", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:48:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "20471db53463c3b9e93d7d513dae74c47898e489dc9b5bacdfa3f6eaca82f8f1fd4b202dc6f56cf8a2b7ef53c1d0d235bf50f45ff709846dd8204ae0f7415ffc65" - }, - { - "block_id": "000f450eec6d983ca157b056efe0073a407dcf90", - "extensions": [], - "previous": "000f450ddd4b1d7c8a71896b94f6ff4b3df86b00", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:48:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f22906afead1b61dd935f6a39fd14f8934bcd8995ad85733b201b673cd7922e3d3d0d15408cff5afd26633aad7d38aee5455f7ef898beabaadcaeeaa408b5ab8e" - }, - { - "block_id": "000f450fff7588f33185e44d97a650d60ab00106", - "extensions": [], - "previous": "000f450eec6d983ca157b056efe0073a407dcf90", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:48:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f79a7b77c5fb4c2807224f9c01b39a5bf400275c878d45b8bbdeff433c15a97172f929b222904ec1a51493eb1b192cc53684b81818c52f73df7d0ce2c1f25653f" - }, - { - "block_id": "000f45101e92585ada520d4dbde4c4b536d7c86f", - "extensions": [], - "previous": "000f450fff7588f33185e44d97a650d60ab00106", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:48:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f0902c7d99a1d0ba0b9b6c215e8834eb9c4aaeff8a6af8c31ae1b73cfec38f895093c353cb311439c7109b4f85fdb9930204cf644325508807ad6bc983a76cd3e" - }, - { - "block_id": "000f451189ecafebe479c0e6ed83469c1cfa8365", - "extensions": [], - "previous": "000f45101e92585ada520d4dbde4c4b536d7c86f", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:48:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f5581de9d1ea6376ca752800e53aeef4b052876082be0cd7493348ab6a3fad0be33193ef98368cb3f378a3b9a5ace8b25bf587a17d015d9614f52000e9d693003" - }, - { - "block_id": "000f45128f9ff187e4cdfd19b8112c43a7ad962c", - "extensions": [], - "previous": "000f451189ecafebe479c0e6ed83469c1cfa8365", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:48:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f3919243494056c6c8940f37fc9689f576bb9fa760577a1676fdd92444a112bfb39ddd88b92c0630c7f8bb95c09582180731c9b93d9a0862fc3cc6fe5185e2f37" - }, - { - "block_id": "000f4513e73c5685d26ac6de4c2fac1b563657bd", - "extensions": [], - "previous": "000f45128f9ff187e4cdfd19b8112c43a7ad962c", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:48:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f06ea55f1d3b701ccf72d941848f3e161c6aa6491e085b480ec2855edc036e2b566d4a48001f4c11fe5aca3189aaa195b09e1e73f2f676e92bea48d51ba25eea4" - }, - { - "block_id": "000f4514e89486c121d7e0a0f26b92785d1d4a57", - "extensions": [], - "previous": "000f4513e73c5685d26ac6de4c2fac1b563657bd", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:48:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f1d00d3654529d42646c43cac9e7aad327a8a737a2a57dde72f0d275dcf86c19a7534310538160b1bbce5e570677a12348361f19326d25913d22b1d47e8c1625b" - }, - { - "block_id": "000f451556ae90407ab6048cba8b5fbe01719360", - "extensions": [], - "previous": "000f4514e89486c121d7e0a0f26b92785d1d4a57", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:48:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "204d0abae05b8f4f18c85824193a96d84a6d213aee1782ebac3c59bf49c506f7387eda945394b51f5568318de0adc77858aa83dd331e99b755a99ec41d2f196f4e" - }, - { - "block_id": "000f4516d5efd0934bade46d28b03dcb1933c13f", - "extensions": [], - "previous": "000f451556ae90407ab6048cba8b5fbe01719360", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:48:27", - "transaction_ids": [ - "4a69ac2aa0610ed959907ce3a3420b26fec5e756" - ], - "transaction_merkle_root": "f4cf929aab5c72cfba5f371ad484764141f27e43", - "transactions": [ - { - "expiration": "2016-04-29T05:48:24", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f451556ae90407ab6048cba8b5fbe01719360", - "nonce": "17425324765893191282", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "d6f653644d95c1cdbb9e1b8f0179ff90c0999b6acb17b295c31048f90c11d9f9", - "signature": "205dc0469c7334e16f668a19dec05f6e8540d0bceae93d08b434528bc7babaac2f11dfd9d471005b695cd91f438ac4cc9fc5abc0a67a6719243fb8d28009ce1afb", - "work": "0000000c93dd23be6e495dc668510666bb8c73efa53751e95ae174ffa325d81e", - "worker": "STM6qYRfDwiCYeGAZ5SkxkFxmceAbobHsKAB4RCyh9Scd167x6hXS" - }, - "worker_account": "dragonball" - } - } - ], - "ref_block_num": 17685, - "ref_block_prefix": 1083223638, - "signatures": [] - } - ], - "witness": "arhag", - "witness_signature": "1f7e2796c6412b57842a3887668ed4cbe54af00b209580a16aaad8181307403c5b2796889dace136ea0421ea19ea51627792263afc22344e8c12997d6c83ad2831" - }, - { - "block_id": "000f4517742640d9a6e86e1993109072204a176b", - "extensions": [], - "previous": "000f4516d5efd0934bade46d28b03dcb1933c13f", - "signing_key": "STM771iHQotSJcwqN931RvgaPN6kTVqM6HJM5UT5A9DtTLNbWtUT1", - "timestamp": "2016-04-29T04:48:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "liondani11", - "witness_signature": "1f17f2e07c1f0786701dc10f63622c66e6403ae8ce39f9fd1b474af5f4f2a1f48d110c136478b9934506fd29657195b1f24a274c65e9894b28efb41463abac936a" - }, - { - "block_id": "000f451803c7a786a0407bc9b430ddcb332d1c64", - "extensions": [], - "previous": "000f4517742640d9a6e86e1993109072204a176b", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:48:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "204e1ad8b876550eb9535989645ba428d6fdfecbd0a20ce61aeefa38910e501f6151fa60c183c5273475dcf5ba745e870dd9d93604a7284e7dbd410cec2b1b0a77" - }, - { - "block_id": "000f4519b6ff75a7586bed3b4abe4405724e8989", - "extensions": [], - "previous": "000f451803c7a786a0407bc9b430ddcb332d1c64", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:48:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "207e03ebc153858ad0e9c1b032aca9f3b861c4cd03839675798586bce6b809da2b719554c33d47a0eccd091f53520627156caa34ad479de8fa23a83123c9378c43" - }, - { - "block_id": "000f451a102c83639d617d57b1c429e1d2c267b1", - "extensions": [], - "previous": "000f4519b6ff75a7586bed3b4abe4405724e8989", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:48:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f700f34490441049379a2053cb30b19880d58ee97566aa8490eeb36411379251d3ce58c97d88d1640a46aa2902b9d1a2f314b94c0aa8de75daf7c6ec58b31d0fc" - }, - { - "block_id": "000f451b656f926f7e596ea59610d433c842516e", - "extensions": [], - "previous": "000f451a102c83639d617d57b1c429e1d2c267b1", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:48:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "200a58fd2da39e0b51468af66955df51ac27e4d4ef108f0b3daf8fedf817b801fb41072b17488edfd431a72d90252bcad9cc1bc2805001d1253a50433d4f4cbf35" - }, - { - "block_id": "000f451cdb0cd4090266fa26d3ff99e7d4017b3d", - "extensions": [], - "previous": "000f451b656f926f7e596ea59610d433c842516e", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:48:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f53df91c4bb8d1da6452fe1556619c88c4aafe2b75dd098ad4cf4d30397e5c6df2a1f3e5f2af68d62691c2f34bde2b91702aab8c3af4b0418c6fc05737c02ed45" - }, - { - "block_id": "000f451d742c7dab2cd45173457e01de5263d9f6", - "extensions": [], - "previous": "000f451cdb0cd4090266fa26d3ff99e7d4017b3d", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:48:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f5d1ace13f02ed245f3df301f0f12a24a1dcc3fe9f13d2ef9f5b64f9bd9f5972f03cd64c80fd85ab168e065fdd915f544a9ba7357d21aa93e20c9346f6cdaba7b" - }, - { - "block_id": "000f451ee1e78d0d82c42151bb04ccdcf9acc405", - "extensions": [], - "previous": "000f451d742c7dab2cd45173457e01de5263d9f6", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:48:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f43a200f4f639dd804c69b4b136ec65637e402934a10f1b76e3593098921e69bf163ce83d1e1ecad390bf5307da0fd3d966afb120b184835ee88eb8f6c6440420" - }, - { - "block_id": "000f451f2c5e561676fbae1dc858ab5f04134861", - "extensions": [], - "previous": "000f451ee1e78d0d82c42151bb04ccdcf9acc405", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:48:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "206f394d6afc6414c084cc62cef2cc9ee36b1b6168fdf42be93679630799addca34b63d556f78ef18805e6569e79e9b4125bcb7f07228f6cd5262daff0440fc66e" - }, - { - "block_id": "000f4520d77af5023079e3e6ba2f6b773280ff07", - "extensions": [], - "previous": "000f451f2c5e561676fbae1dc858ab5f04134861", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:48:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "207921eaa6ecce773a312de73f4decb9b17185a1b3b2964d48baac1497dc2dc49b1e0be7c55e776193df1b3db21f624a32f1ed85df89d90d675ba1f02d76023367" - }, - { - "block_id": "000f4521059748c3511731ac030d9a58f6aa8957", - "extensions": [], - "previous": "000f4520d77af5023079e3e6ba2f6b773280ff07", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:49:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "206c13d8db2d10cb30452bfe80e253b1c5007e203a44add21039b3511d39f6393a245db1e04135b2c373b18465e6bc048c2447f83b083690d748d89cf1de1a0e61" - }, - { - "block_id": "000f4522bddcd3e29f95528d6b7353c22d2d62a1", - "extensions": [], - "previous": "000f4521059748c3511731ac030d9a58f6aa8957", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:49:03", - "transaction_ids": [ - "643bd008a8f0476d8d7ee4467c84ec08561f0f7a" - ], - "transaction_merkle_root": "e60489db7ff653952fe6aafc7c11f970320b02df", - "transactions": [ - { - "expiration": "2016-04-29T04:49:27", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "441", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "bittrexrichie" - } - } - ], - "ref_block_num": 17696, - "ref_block_prefix": 49642199, - "signatures": [ - "1f3cfa2dde1641d0037d9a8823769b182aae3d98aabef3eff6092bc52eded4bdbc1adf4bbe0fc8bcc956b8334e61c5995d40d6a4aafaa20ad972638fe7aa78e80c" - ] - } - ], - "witness": "xeldal", - "witness_signature": "1f3a2d83faa00224b13a6a87c2520af75d7bdc05c61f6452666c70c31c2e234c7f3049fb75cdca6b63bea4cbfcf11a2ef7093b65e759cc8433d9e55a305a91322c" - }, - { - "block_id": "000f45235f32dc9a85ee6c67691c0e24ec3e277a", - "extensions": [], - "previous": "000f4522bddcd3e29f95528d6b7353c22d2d62a1", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:49:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "2004127d33d07ed8af1f5a6a10d40996aa01e8ddedf1a48b7bfc674c351c6fc8150899c7a3b7a557d8cb2e7f2fa77efc5e293be919e87b26183db30b98a5f8f8f7" - }, - { - "block_id": "000f45246a363d7df803e3808583b267ea223d31", - "extensions": [], - "previous": "000f45235f32dc9a85ee6c67691c0e24ec3e277a", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:49:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "203332fddab07d3636a62ba53529678b59ba9f8d9c60cfd2cfcd0ee0a8b3eaf1ba7ec439993e76f7238e6b03ce2311bf5ada4c7a76e1d3f77df447af18c90e7dce" - }, - { - "block_id": "000f45250be3bfd9b1063fa26068eb673b2df364", - "extensions": [], - "previous": "000f45246a363d7df803e3808583b267ea223d31", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:49:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f79e5b5c4aac1b514aa2d6bc0d01bf67625f0e8e61517ac92c7ed266923bff14a6a1dd8f17af3a61abc65ac4e46ee02d8ec4a267a910fe9b6efd2c2a29760a06f" - }, - { - "block_id": "000f4526ebcfcc5cbb6dceb0c633db4fdcc2c75f", - "extensions": [], - "previous": "000f45250be3bfd9b1063fa26068eb673b2df364", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:49:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f793c62efeb653454b650645408ce27e934acd13a08c9250f0cab6e29e4e73f8651951f1b99509db664fa47e32266f45c2b3dad50b241d67560ec4dfb212de993" - }, - { - "block_id": "000f4527d1a6f1b5ce3ed6da109657ab4d8e82f8", - "extensions": [], - "previous": "000f4526ebcfcc5cbb6dceb0c633db4fdcc2c75f", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:49:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f071542dbd491f98181db99055f60bb2fa216a7fa13ef791073a7f9fa366e4e794f6230ff68e33091743875bfac0f80f0c4c548a54ef28889e1d35f0b8e382f80" - }, - { - "block_id": "000f45280cdd7237292fa68fa59fa7146ed49062", - "extensions": [], - "previous": "000f4527d1a6f1b5ce3ed6da109657ab4d8e82f8", - "signing_key": "STM8hJVrB1oGRZxoVCS9NzE7WtovMcNLeNwq7Pd7miVZLP8Yj4sxZ", - "timestamp": "2016-04-29T04:49:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "phantas2", - "witness_signature": "1f4f0bd6248fb7a47c3e44aeece15b08f0c8face91e4f91a86c7f1e667af2a2a517ed0879e25c2365b184bb25dd41e4944149df6cfe886b6ac039c3b92dacd5b1d" - }, - { - "block_id": "000f45299c9788c11ce307630fef4ba7646348c3", - "extensions": [], - "previous": "000f45280cdd7237292fa68fa59fa7146ed49062", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:49:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "2002b7debfc7bae38cd913de27ac1f43c1e4e59d52c6bb5aac4329e4f06674f3da68beff5f17b0650465ccf003e380fc4594c8a6fff5b5f3f5b15b9877a66dd919" - }, - { - "block_id": "000f452a8e6377f7d092678a4ed083a9f6d5ec4c", - "extensions": [], - "previous": "000f45299c9788c11ce307630fef4ba7646348c3", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:49:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "207c89977b5d9499fabeb83c284366e756f5a31abbc1877e41c8bd8c7f431f175102888b7fa3681e67d6e96562e97a78608723007901e28111ac5bae29be7ba2b1" - }, - { - "block_id": "000f452b544993af546b7471d814a246fc8c70fa", - "extensions": [], - "previous": "000f452a8e6377f7d092678a4ed083a9f6d5ec4c", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:49:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f7ae8fc32b872929c65665af8de4db7f4ca69ef061aa209134598a753617d86b434166fd614ba3a534a30e83744e9cf8a57a73619bca586fbbff5e25054c7376c" - }, - { - "block_id": "000f452cb1e63e41ecfeefab2889f6f85cf78265", - "extensions": [], - "previous": "000f452b544993af546b7471d814a246fc8c70fa", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:49:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "20497509d4cce0615949ef319ca0350fa5edee0f40214884d3b5a3491a76d8fa64417281e9a9fffc786e922f88fc8cabfbf75a934b3765956078ac2da593c8d270" - }, - { - "block_id": "000f452d0086e7422fff55932271c08b97dcac40", - "extensions": [], - "previous": "000f452cb1e63e41ecfeefab2889f6f85cf78265", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:49:36", - "transaction_ids": [ - "43676938c31fc1c7c312f49e255fbd87819065d8" - ], - "transaction_merkle_root": "ab2b3e2bb3fbc5444add86f4518e94ca5d4ca2c9", - "transactions": [ - { - "expiration": "2016-04-29T04:50:03", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "441", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "idol" - } - } - ], - "ref_block_num": 17708, - "ref_block_prefix": 1094641329, - "signatures": [ - "1f70f219206bf49dfc155bd0b805420627745dec845287ff9cdbd3ef4367a5e22e1cac3a9f330f20bb663e42b52140b455913156a0f04355c38ffb8536525831cc" - ] - } - ], - "witness": "smooth.witness", - "witness_signature": "20383a862c70087735b4191e995be9b9a274515fdc8522003ea06c0518cb6aec961b25aa05f66adc25bfbf79a9f330f7876d7db8a7285135ff77f7725b2ac469d3" - }, - { - "block_id": "000f452e0ffccbf1a0da67ba8f61358f2b7b0d4d", - "extensions": [], - "previous": "000f452d0086e7422fff55932271c08b97dcac40", - "signing_key": "STM6hm3wLsDPmx9GrxZu4F4cGHEUEofmxgeco4gCYhkiBhaGTXuyg", - "timestamp": "2016-04-29T04:49:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "mrs.agsexplorer", - "witness_signature": "1f2fd7d73037f6fcb0de10f54d526544aaf1060c3f2539cf561e8889bd728a442112b9957f6d23ec4a0c46dfcf06c82906fcc399858001299f98eef7bb06c9f9f6" - }, - { - "block_id": "000f452ff9a345465e1830e5ec164eb2871b4c76", - "extensions": [], - "previous": "000f452e0ffccbf1a0da67ba8f61358f2b7b0d4d", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:49:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "204df7fef869685e96601fa46df498d17655bbb112b881ed12933d7d8cab32bb3d6d631a2650981756cbc7512635ae46076cbbfa5081cfe2d5427fadb478a20148" - }, - { - "block_id": "000f4530826937212ac4a0f00c97d9678e22a763", - "extensions": [], - "previous": "000f452ff9a345465e1830e5ec164eb2871b4c76", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:49:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "207786a78b549c395245d4b783a0110435110ffdc65823957e8eced056f30fb4de47ab6979924f57572e00b93a30549dafe7ffc51e6313edf60a7af934433558c2" - }, - { - "block_id": "000f45310008d9668857e052e10b16ca38de3ede", - "extensions": [], - "previous": "000f4530826937212ac4a0f00c97d9678e22a763", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:49:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f61057be03948f5d4f5e7fc24b999306ac865a6b34d6c62c321d25dc057bfa8dc6590a6f8b83629cc5db7148c97dca7752eef01a4e4778eaf114e6d92114cc9d9" - }, - { - "block_id": "000f4532405a13845abfe979c3cf6ac97a9954a9", - "extensions": [], - "previous": "000f45310008d9668857e052e10b16ca38de3ede", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:49:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "202d62b88a3a72533401c1e655c800d182d32c4f6dbc4464e7cf1ec20cacc453ae5db43e3eeefceefa706b0ee17ec0698e89778f20cfbbe4c3bef087a11babcc1e" - }, - { - "block_id": "000f45333fe0cac30959140dbe6418287f8e7d17", - "extensions": [], - "previous": "000f4532405a13845abfe979c3cf6ac97a9954a9", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:49:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f2329bd291af1afbd0c21052db4fe2707b85dbff542dacda29c2446064f97284049f0163be84f0183416cda5703c5ffffff649b5896840217ea77284a2e66737a" - }, - { - "block_id": "000f45341913d3c817fc9208f194784914f6539c", - "extensions": [], - "previous": "000f45333fe0cac30959140dbe6418287f8e7d17", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:49:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f0e6179bd72ed96f3bd89cb6373a51ddeb43e1e7107b5e71b440e629dbae9037545344342ce6b6cad7c29125d0f0dd49e0368462025ade9589c58d24526bcd836" - }, - { - "block_id": "000f4535ee74a096c9e805f3ec9d1e4fcc9a4790", - "extensions": [], - "previous": "000f45341913d3c817fc9208f194784914f6539c", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:50:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "2069b986ad2b4a4f2e28786973cd80efa9fae0a211b92693139a8f73a6ff1ab7d525143ad893994287fb95946749f6767882bc952422efc787e1b920392313ee9b" - }, - { - "block_id": "000f4536ca33e1a4db14341b637a310781971557", - "extensions": [], - "previous": "000f4535ee74a096c9e805f3ec9d1e4fcc9a4790", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:50:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "206558b6bcdce4fd329aff8b0606b38c01284a28de7b0f8f1a15f3a440444403f03f7ffcf6757584c38be09d81c656f2a73277f33f59dbb40381380f26bab6b8a0" - }, - { - "block_id": "000f45379efc66f52c73e4909e169ad7b8f001d8", - "extensions": [], - "previous": "000f4536ca33e1a4db14341b637a310781971557", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:50:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f6dbc06ef23d6cde4a221fa336819a7838f9757550f350f23fd6e027bc6280e730d3ecd2bc2c7de5409629d9de87b621d0ca5fd9d1022bff1ffdcc660dad4a24e" - }, - { - "block_id": "000f453859fe5885b0fcdea49f4cda4e6eefad7c", - "extensions": [], - "previous": "000f45379efc66f52c73e4909e169ad7b8f001d8", - "signing_key": "STM6k58ygyZRmunSctdyD5F7gmJuQbm9kedCpHMvzKX7N1YjSbv85", - "timestamp": "2016-04-29T04:50:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "commedy", - "witness_signature": "20126c36357aa5d1d294da58b83249b07b16d46c7c321b52c8041bbe6da6cd7dde68141fe9be4f64ed2acb047e157c49ffa79282813916a13d2722dcb182548be3" - }, - { - "block_id": "000f45394d6753e8c00a6ce5ce94e6424993385c", - "extensions": [], - "previous": "000f453859fe5885b0fcdea49f4cda4e6eefad7c", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:50:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f6778579d110a1586ea5a6f42c0eeb185912246f28bd8f9439e8065bc66c1711d566b35808f9a755574a27a9861a3f6675ed381793dd1119c38c7d4f3eb5b950f" - }, - { - "block_id": "000f453a076219f892f1fc5092d28fb480696fa7", - "extensions": [], - "previous": "000f45394d6753e8c00a6ce5ce94e6424993385c", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:50:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f390e7874b42f1b0f0156544c2969a51b657b235e2c6507737f5dcaf4f37bd83043a9e68fad7b6d908aee963b42db6fe006ecfaf7d56718ea937a4a1b8f6e47eb" - }, - { - "block_id": "000f453b7b2132bd19500a243d279a5d5cc7832b", - "extensions": [], - "previous": "000f453a076219f892f1fc5092d28fb480696fa7", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:50:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f131c7de977d4214b260731d2661a69524447718be5e01d4e9c0fdd1ca5a20d566d6a180d3d61c9d31f871b664d8d7e2fc817ae70e6a9706f0e9413f8525995b1" - }, - { - "block_id": "000f453c29f6c5e92c427fcaa8bfed4548c2b2f0", - "extensions": [], - "previous": "000f453b7b2132bd19500a243d279a5d5cc7832b", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:50:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f6be999be6356c78e1eae1d31a5eac306e0429437637a2bb7935f63c2aef15d74520518ee9464a3f062ea97b7412eb13f535979ef0b75831086ab1527518dc500" - }, - { - "block_id": "000f453d43ea3bde77ebc61cd00d6df08c887e61", - "extensions": [], - "previous": "000f453c29f6c5e92c427fcaa8bfed4548c2b2f0", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:50:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "20692ea475140452df74baaf45c4148bf5f865464c48c7c013b1ce3b34d1dd547f03107c78841b9ea50814e00cda5f4aa311465ab2a805dd87fe3d47e7e0a1b22d" - }, - { - "block_id": "000f453ee4f9d461a1e961a66da8ca535048609f", - "extensions": [], - "previous": "000f453d43ea3bde77ebc61cd00d6df08c887e61", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:50:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "201cd9699996f1bb8578292aa31c4e2518dca6432c500763936381b53204f1edee481a8253d6136d5f1aa18f66e718d6c76c204b36cb2557523e68682c75fd40b7" - }, - { - "block_id": "000f453f6f0005823218ff8a9eadaa2dfb1953e2", - "extensions": [], - "previous": "000f453ee4f9d461a1e961a66da8ca535048609f", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:50:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f24d17e76d5b395ebfc83e8bed38a05685367dd8373605a4d705f4508f1c7db814f8f9d3d0dd1522381c602fa638b5a25a04849c31110991552e604d52073e67b" - }, - { - "block_id": "000f454073a29bedcacafdab77f21229dce822a9", - "extensions": [], - "previous": "000f453f6f0005823218ff8a9eadaa2dfb1953e2", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:50:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "203652a4993b497a60fc12bc8d0067b350f0fffa2d003262acbe114a5f1045a91a291021cbbf04a31b6bd79d4b277192f41b0db7bddfab7d560e4e74925c19b741" - }, - { - "block_id": "000f4541fdce3dfc737c8bffbf34703ed3d6cfd1", - "extensions": [], - "previous": "000f454073a29bedcacafdab77f21229dce822a9", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:50:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f4a120c02bb369fa88c845e0b4217b1c6a1959eda84e2ab54116aad9a2b64860c5722bc9d45b0fbe087abc05142c9b6b1b354f4be5cadc314ae53927dcf42d1a1" - }, - { - "block_id": "000f4542da4568224cc452a5f398364de2720883", - "extensions": [], - "previous": "000f4541fdce3dfc737c8bffbf34703ed3d6cfd1", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:50:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f3d7e2a31bf3aa13f70b9a254b236e94387be34e11e41be9d1d1f4965797c3d6f7a2ce3920aca45f18baef540cf603c00a50ec8370716dcc6bd07d6502471c8b8" - }, - { - "block_id": "000f45430a829fcd8e83584f668de8617469227b", - "extensions": [], - "previous": "000f4542da4568224cc452a5f398364de2720883", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:50:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "20661123abe18a2654b217428889fc177c616e1fa2df8d8ee5ad23ff275eb3c81a348749921824c698b9251dc5fb49d8bb818c7c4e84d2d955e288324610633fad" - }, - { - "block_id": "000f454464490a0efbaa182c8513876c7f130dcd", - "extensions": [], - "previous": "000f45430a829fcd8e83584f668de8617469227b", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:50:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "2064de6efdc9dd5a20b9f3f9da72a0ddc264b9ce71515fe7d187f757c335b5263f03a65a191d9ad772326d0aa08e5d0533b78e05d54756ccb8f3fcbc31b326e940" - }, - { - "block_id": "000f4545b946aba2ce7988cbf010bff8d8007eeb", - "extensions": [], - "previous": "000f454464490a0efbaa182c8513876c7f130dcd", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:50:51", - "transaction_ids": [ - "b57fe4e45bb6f61c7c28d28771b6992c59c79c70" - ], - "transaction_merkle_root": "c730b5ac494932e719fb7d84f86ef971c11f3c5a", - "transactions": [ - { - "expiration": "2016-04-29T05:50:48", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f454464490a0efbaa182c8513876c7f130dcd", - "nonce": "2752193280839810334", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "2150187af947ab1ace957173932ce0a6f5a04e86f169055a53d3e0d15317683c", - "signature": "1f68b98c8977b836f8ada4e6289ae6d911e987d25551e09634b5744c15c99d0ec60a967f892e5a28f279ea223b5414d1867e24c21922f6d073c2c05987d2ac38db", - "work": "0000000c4ba1d1ff44ad527a0b9bb5bc1d6992c2e3f562579786cd2e98983cf5", - "worker": "STM6T8rz9SuMeCnfnjYCMszrZcek4Ug45GKf7BVzYTuqH8ZZoSrPz" - }, - "worker_account": "viv" - } - } - ], - "ref_block_num": 17732, - "ref_block_prefix": 235555172, - "signatures": [] - } - ], - "witness": "kushed", - "witness_signature": "20621a41bf378ba61181b9e25edb5cb984efd14249676d6dd2967a81b4d2172d95242978b037316bc84864690578027fd98bee9a0dec2830ab02791721203debba" - }, - { - "block_id": "000f45464d491c0a7fbe514d60e1382caab50978", - "extensions": [], - "previous": "000f4545b946aba2ce7988cbf010bff8d8007eeb", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:50:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f0997ddb8d194fb8dc821d1e1f75d41b3b36852b34761d6c7918989be000d36596da33ae50d1b58cfb66d9b320f9990e8dd295cf742219dfd0e00f8548ce4b366" - }, - { - "block_id": "000f4547816ddb733865d436e2420c872c6c5798", - "extensions": [], - "previous": "000f45464d491c0a7fbe514d60e1382caab50978", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:50:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "201d00dcd95b9f58446b315529112c10637de7edf43dc8f3c29d14fd3aa23dd75f3c90f2be7056ce61bda0bb18913caa5429089a085c14ef2fe96e14106761be09" - }, - { - "block_id": "000f4548fed4eaee5c9a728f60b2d825d7bd9b84", - "extensions": [], - "previous": "000f4547816ddb733865d436e2420c872c6c5798", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:51:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "20648d6940eb6b0989fe1cb77446aed04fe7939f9e59a951ef3944430a365ba67a15fd18fb6f6088dd5f46f5185abd32a6f1cc81f9eaffebaa5a92a4d0c06e40bf" - }, - { - "block_id": "000f4549f6d5fb10a8b372ed0c20dbee4da701dd", - "extensions": [], - "previous": "000f4548fed4eaee5c9a728f60b2d825d7bd9b84", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:51:03", - "transaction_ids": [ - "7f6287914e5ba2b6a0cdcd775b2bd2d4cf3a47f0" - ], - "transaction_merkle_root": "6aecd497a138f1439c02a2befe1c56ed9492501e", - "transactions": [ - { - "expiration": "2016-04-29T04:51:30", - "extensions": [], - "operations": [ - { - "type": "account_witness_vote_operation", - "value": { - "account": "steem-id", - "approve": true, - "witness": "riverhead" - } - } - ], - "ref_block_num": 17736, - "ref_block_prefix": 4008367358, - "signatures": [ - "202495ee9ef353d4cdff8d56e3b9a3cf3f36e9d8b23413ebf2745f7dcc272d9f7e5ab26c6674fd477f30ff31f632951576aec3885192c5dad8b7c50a012df1809b" - ] - } - ], - "witness": "roadscape", - "witness_signature": "200810327af9167ca9dee3f2af6296d81186de1ac642871043efbba8e1330e61342018a029b2f4300ec7393000abdf2bd70d9d665e2085cfe65cd19e9589977130" - }, - { - "block_id": "000f454ae1b6b81eb1bbe396ca8e484d6ec3d29c", - "extensions": [], - "previous": "000f4549f6d5fb10a8b372ed0c20dbee4da701dd", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:51:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f117f8bd9fb1a213b94a42c81200943c6f8bdc6afd8d279d5e63f371cde053b377b75540848c03b53252b23f6c330a967dd5bb23cf593051f1bda88f9cd311fbf" - }, - { - "block_id": "000f454b74dfb3bfa53513f0e48ebfec03cc19ff", - "extensions": [], - "previous": "000f454ae1b6b81eb1bbe396ca8e484d6ec3d29c", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:51:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f0600401fc52184e8d643ea58235129fe8a8ccb608df5f3f917798e1ea525c73670440593f2e2e54f185450ccda4f39842353b6aac51081356bf4e5d6d98634f9" - }, - { - "block_id": "000f454ce31c1f22ddbddde9f409995fdd35c38c", - "extensions": [], - "previous": "000f454b74dfb3bfa53513f0e48ebfec03cc19ff", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:51:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "20391c643f5f46fa460b9151fa45d3f3634668015407abd4281f2562839d7b5d20741ab6a6f386bcee9a71de5f2cf71b9dd9f6187e756771910f1cf480211590f5" - }, - { - "block_id": "000f454d6aad7e7229c46db7244af0ee4656fa46", - "extensions": [], - "previous": "000f454ce31c1f22ddbddde9f409995fdd35c38c", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:51:15", - "transaction_ids": [ - "5dd9f574fb0028342f5aa016f26d38dbfeae9693", - "4559e2c6f87c3b4f3dd92de95608414efbf5faae" - ], - "transaction_merkle_root": "27d9995782dd06389017cd36810c303cfa4c8604", - "transactions": [ - { - "expiration": "2016-04-29T04:51:42", - "extensions": [], - "operations": [ - { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "10000", - "nai": "@@000000021", - "precision": 3 - }, - "from": "openledger", - "memo": "", - "to": "register" - } - } - ], - "ref_block_num": 17740, - "ref_block_prefix": 572464355, - "signatures": [ - "1f5dc29353fa1366a4662421d73913212919e06064b06f5913cac1f27058726d15613e092021fbcb73e91cf16373abb90b5b1558705ecb442f81bb46b9d8b13cb2" - ] - }, - { - "expiration": "2016-04-29T04:51:42", - "extensions": [], - "operations": [ - { - "type": "account_create_operation", - "value": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM55u5RJugcyLCCwutKNE6a5B6wKKUG4NyNUiw9DVaoYikY55a9Q", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "register", - "fee": { - "amount": "10000", - "nai": "@@000000021", - "precision": 3 - }, - "json_metadata": "", - "memo_key": "STM55u5RJugcyLCCwutKNE6a5B6wKKUG4NyNUiw9DVaoYikY55a9Q", - "new_account_name": "asabove.sobelow", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM55u5RJugcyLCCwutKNE6a5B6wKKUG4NyNUiw9DVaoYikY55a9Q", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM55u5RJugcyLCCwutKNE6a5B6wKKUG4NyNUiw9DVaoYikY55a9Q", - 1 - ] - ], - "weight_threshold": 1 - } - } - } - ], - "ref_block_num": 17740, - "ref_block_prefix": 572464355, - "signatures": [ - "2042bc243b7f77f4eab707c4adadb700904b182259ad312eb46062cd2ab246e3983e903d7fb038e342c3b7114e35eb39b46c20ee59d08aa7d71f4129f6e97271ac" - ] - } - ], - "witness": "clayop", - "witness_signature": "201f64549483c66094df35cfadbc69988ffb36e5fac964ad24f0eb39677939eee87157f000505ccb4ed87762b2e75347af3d8d1753279be14042b2140a278296d8" - }, - { - "block_id": "000f454ed2602feea7b0267a1bd41791ca5958f7", - "extensions": [], - "previous": "000f454d6aad7e7229c46db7244af0ee4656fa46", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:51:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f173fbbbcc3e58855f7bf06b0ad84509d24af8970af6ab28de10f3f9be7bcfa7d54ea00a111b90adaae65d92044567cfeedb207aadfa6429822879b6abec71851" - }, - { - "block_id": "000f454ffcdd3f14f289b574d912ea7bfedb6d56", - "extensions": [], - "previous": "000f454ed2602feea7b0267a1bd41791ca5958f7", - "signing_key": "STM7P7TMeUofSum3cdUFfEZc3e78axUMcHrcSLtMu1j4BPW7sVxuZ", - "timestamp": "2016-04-29T04:51:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "masteryoda", - "witness_signature": "2020cc51227b2b51c9e0eebe51f3d60f6be38dbadb6631428b2bbb895691bae6835e4b58e3fd8a41b2b617c57c34234439b8cbee66357737ce171f4ed3f681747b" - }, - { - "block_id": "000f4550b71f74af9f63cf54bedf54888453ad50", - "extensions": [], - "previous": "000f454ffcdd3f14f289b574d912ea7bfedb6d56", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:51:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "207f19fbd5fa8ef4c545fefb368043aae25d5b06f98fe79a1eaf65dba1f21e3c2a6477f125366e7b474e017b361b3349f08da7380c8de8194cac3a747ecc2b9dc1" - }, - { - "block_id": "000f455172fc830f5a0ba53617c8754d24364af3", - "extensions": [], - "previous": "000f4550b71f74af9f63cf54bedf54888453ad50", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:51:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f25ef6e7d5f0ec0df5e94239dc8f3096b4acad9dbb7fdf94f90ef75f37d115d7b0647aefc492aa17e30aac70160b18559d8512203db50ce2db847901c87c3b0ea" - }, - { - "block_id": "000f455282183ab7870683ca7170dd8ff813610a", - "extensions": [], - "previous": "000f455172fc830f5a0ba53617c8754d24364af3", - "signing_key": "STM79MBtZRHzawavWSBSVYNRAy7WkwM2PMPDEnoWH547WLzXP5CkP", - "timestamp": "2016-04-29T04:51:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hideo", - "witness_signature": "200b98d62902834b526fd5d46c913b408efae669428531c8487f5846ad06b6a0d325e62b9cd93b295ff0882087e9a334b1c2a990b09f5f325cc21c06eaaed7de35" - }, - { - "block_id": "000f455308c9828cb76a424e411221882672dc39", - "extensions": [], - "previous": "000f455282183ab7870683ca7170dd8ff813610a", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:51:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f43215ca1f5aeae6df69e81669abe3add170ddd6b5132598ceeacdbb88edde7f209d6a0880d956e44a7ad5957fb4a844ff1f8d596dc4f55ada84a90c8efd1df0a" - }, - { - "block_id": "000f4554f1d882f1d39e935530ef407b9d3b8853", - "extensions": [], - "previous": "000f455308c9828cb76a424e411221882672dc39", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:51:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f7594394e2edeb4626a3b0246727324e86c8bc7ba217aba3a679c0587fc5567ac225b3fc3e4c56d1cb49717af8e39c1dd7b430b33bf819da3eedb818dc0c07202" - }, - { - "block_id": "000f455549f7efc4c076c5a0707f977df0c0b21b", - "extensions": [], - "previous": "000f4554f1d882f1d39e935530ef407b9d3b8853", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:51:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f2e10e6c3de91f02b9c778fa01ffd612d05591556ca8675a79dc383911ecaa60e5965271805132ea3b8a602151015acc764e5e91e8d3e602d1971f2e2e611193a" - }, - { - "block_id": "000f4556c467256e2b2bf25043c6535549e06218", - "extensions": [], - "previous": "000f455549f7efc4c076c5a0707f977df0c0b21b", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:51:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f4c86f2821b16843c881579c1a31a76af16f726f4145018c0ecb46da96f158b94177dc833716b17feaf244eb1b1d181d56e371a33a9cbde814c7df37d2a6740b0" - }, - { - "block_id": "000f45571641522fb31828f71325578e7ef6e0b5", - "extensions": [], - "previous": "000f4556c467256e2b2bf25043c6535549e06218", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:51:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f73d4cee53b675d2edd2bb851106d138e0654667070cf3061db374214a185159279cf875c74e2cc7348acb23ff2e63d20b75ae5779ac62fd6829f31464bfa96a8" - }, - { - "block_id": "000f4558928d8e28ddf6540e304382328ba0c024", - "extensions": [], - "previous": "000f45571641522fb31828f71325578e7ef6e0b5", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:51:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "20473d22736b7e844c014bf5e3995f505766b14ebf75067d4ba21566d809a0f58a1ce7af6e6aed205b538ffbfa46db5f8d895f2fe5d67e2ca7b070b363aba1fb32" - }, - { - "block_id": "000f455962ee099f57228b971779c35e2e25e56c", - "extensions": [], - "previous": "000f4558928d8e28ddf6540e304382328ba0c024", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:51:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "201a792e245cf9117dda4cf31cb6dc036c07cb63961cd784857a1c715a8fc6d0a34e745841da7cbee3e057e1c02fc5b346dbb45f9f172734f8476b5a79eb845e1e" - }, - { - "block_id": "000f455aaed4342aa63741a4f000d4bd35934f21", - "extensions": [], - "previous": "000f455962ee099f57228b971779c35e2e25e56c", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:51:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "2048b1578f4b6a962d55962ea3825f4a35516ddb369e396495d4f24331ff4871e51474b990f7db797e8544f360e80946f321d77b18893d981402b5283ed522a2fa" - }, - { - "block_id": "000f455b4bed7ea727ec005c4bc668262ede291d", - "extensions": [], - "previous": "000f455aaed4342aa63741a4f000d4bd35934f21", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:51:57", - "transaction_ids": [ - "d61e341eb7a4ac077c4febe1f44d38df6ad2a5b2" - ], - "transaction_merkle_root": "3798840bc210806e378146a5ce6dbaaf6bebd2b4", - "transactions": [ - { - "expiration": "2016-04-29T05:51:54", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f455aaed4342aa63741a4f000d4bd35934f21", - "nonce": "3880213603049396950", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "90373cc6196fad92d67c53d0808d424195510f8a16ec8b1d758f4658debffc8b", - "signature": "1fc8924364b63b9aa4d8eae719e3e1c2de48236f83cb78f90a0ca46ed35119d9db216e8cd7ceb14f087280a764df3c7b678b209f7fb28a4c803ff44ea97363b41f", - "work": "0000000192a7e64b82d386defb3b942318093ca2143e4505842ab23a09386f8c", - "worker": "STM55Q6YCSqAE4F447VZqYARBYGJHEzyNTbxWMbTU43qs6dNeHuWA" - }, - "worker_account": "butterfly" - } - } - ], - "ref_block_num": 17754, - "ref_block_prefix": 708105390, - "signatures": [] - } - ], - "witness": "pharesim", - "witness_signature": "1f12bd6222242fb3b919e6e124c3004234dc64262ff206960e6f6a52d930309c5058c447f9d281f7090869c7ee2cd933d8aefcf31eea2239b3a54359f39d3e20a7" - }, - { - "block_id": "000f455cfe18a0eca36ed6d4a7809e4ffffbd21e", - "extensions": [], - "previous": "000f455b4bed7ea727ec005c4bc668262ede291d", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:52:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f3b455f6548014831aef3f3670c5e48b1b4b9b7be4da42c858a6c2536e782f32e2a133269b3e0b6b506709902af88e8ab47b9b4b90a325723c236effa3eae8617" - }, - { - "block_id": "000f455d92cb1a25e432b1cd1ea2f1b39e405357", - "extensions": [], - "previous": "000f455cfe18a0eca36ed6d4a7809e4ffffbd21e", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:52:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f6c6377bd312c5672c0ca15fcc82f38ab87fd778470b4d0b839921333cdcc6c8669e69cc8d9cf2de474ec8990616e9da0a368cb23c9d2807f44b4d9865979ea59" - }, - { - "block_id": "000f455e98be45441a549ace6c464861e9ee7296", - "extensions": [], - "previous": "000f455d92cb1a25e432b1cd1ea2f1b39e405357", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:52:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f1dfa6db6b02f1cd6f6debb52f1d760cd92a7fb6c8f260088b23ecc5078c63dc71c0e374d98a601c7a2401c0893f83bd4363ceddec9a76741cc2b4685da340e10" - }, - { - "block_id": "000f455f1909c0f806dead4840e8482b5e05b13c", - "extensions": [], - "previous": "000f455e98be45441a549ace6c464861e9ee7296", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:52:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "2037a37f99f78a2d911b656de06fb8e946404c6f682ec223164996a95d87f6e3ee67181cf3e17712e73f691ab829adf118f5d89fb64a54f4ab586f65b913588399" - }, - { - "block_id": "000f45609bcd01b4633bfced47bb7595cb1c5547", - "extensions": [], - "previous": "000f455f1909c0f806dead4840e8482b5e05b13c", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:52:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "200df0c72c9ba3b3aeb1f2b059827d1417daf179734fac3f4a098cea756bcc6d2510f8402f8f5c3fb1c466e432431164c31c7c7e479a8090b4345a1d5049f22e2c" - }, - { - "block_id": "000f4561bdda2bd24277c6dda5ee8e3da76af5da", - "extensions": [], - "previous": "000f45609bcd01b4633bfced47bb7595cb1c5547", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:52:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f0440fd07f0a46814e9259c94cf23e09c9b3d2fb2a213c894096b7c67c3ffe6c370601a37fdfca4b35fb89a27e099c426e272fcab4decfd11ad5dd0ce1f3ff1bb" - }, - { - "block_id": "000f4562d981e0bc148b0cbb508939132a1c05a3", - "extensions": [], - "previous": "000f4561bdda2bd24277c6dda5ee8e3da76af5da", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:52:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "2034a294ec2c1c24be4faf5a1f97830990d80eb29caf144af2ef699957903357a1499d7e54584efe2299b00836d883ffc79381aa920f389a18377c4de0273c20ac" - }, - { - "block_id": "000f45637a806e38d4e7beff5710ad94d3e68ec8", - "extensions": [], - "previous": "000f4562d981e0bc148b0cbb508939132a1c05a3", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:52:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "203f3a4f76d837c493eedac1181f71baedad651e5931034c54bf358a42595b1c45647ace30aa80332935a96f09d04813822f690680486c62e1110eb179b042e9a0" - }, - { - "block_id": "000f456490bfaa4e54335c0c771d375bf2996009", - "extensions": [], - "previous": "000f45637a806e38d4e7beff5710ad94d3e68ec8", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:52:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "20457f5d13c5c3fae76471e98bef39c4ab3493a1adc9dedadef5d66e52c47caaec0988787966ab32fce7455fda862a5aa88a139e04b13d07673f09edb9728b9b54" - }, - { - "block_id": "000f4565f9318d8740338d1bd2127375225ee7ee", - "extensions": [], - "previous": "000f456490bfaa4e54335c0c771d375bf2996009", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:52:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f1dac443196671228c9a89ef6b7d9792898847e00df6dfc4dccd06c6493216a964b850d11a38524c2ba278ba020a0b6324e3c55c9971c3750a7f59914ebde5c1a" - }, - { - "block_id": "000f4566b22719cd0ebb6eeefadb59d255a22810", - "extensions": [], - "previous": "000f4565f9318d8740338d1bd2127375225ee7ee", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:52:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f72e694e2b1129918e70dae6dc382cbf1eff5a6486466bc4da254a252f8fec55877e5fdf233570d177f0b4cb4eb2d6e8c58141101a4ce3a732d94515575b2623a" - }, - { - "block_id": "000f45670cec413405d096fc3524a5606c0254ca", - "extensions": [], - "previous": "000f4566b22719cd0ebb6eeefadb59d255a22810", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:52:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f05dfa420e83795dc77131aa8c9a18ce9af4c1f37e92a2e8fb1bb8386e609615e46d7c4e9bd7dcdb9001e40c44225f9ad38dbee82df18190436fca8d6997699d6" - }, - { - "block_id": "000f45685d8f5aa160e49829ae8d6e1af8664998", - "extensions": [], - "previous": "000f45670cec413405d096fc3524a5606c0254ca", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:52:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f06cc9bc686b825aa4fdb70308b8efaffeab25637e38bdce7cf48c43b44b35a434d55104d7c26b5a2cddc05b9fd052b0b47abd714383884390afdc8a3ed675292" - }, - { - "block_id": "000f4569d9bc5da71f86ffba1e190ab8bda96241", - "extensions": [], - "previous": "000f45685d8f5aa160e49829ae8d6e1af8664998", - "signing_key": "STM5XoTf4uvGDDxWYMT9nR4BpM85CqR9spNbnJkVZufEqYztnbqYK", - "timestamp": "2016-04-29T04:52:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr501", - "witness_signature": "20471b604cc376c4fac99fc2ea8a3a32e4b3db63217ad3b8d025fb77cf0b61aed3138f88632602993053d86ca57910eb1c38b6818262b25987ff6f65a1667f9d84" - }, - { - "block_id": "000f456a52a21a2fb6eb2db06041785360ee6deb", - "extensions": [], - "previous": "000f4569d9bc5da71f86ffba1e190ab8bda96241", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:52:42", - "transaction_ids": [ - "be04e44f49211b5771fd61a23a5ec90a5adf7ba3" - ], - "transaction_merkle_root": "491456373f07da08b8930190cb06310aa1672776", - "transactions": [ - { - "expiration": "2016-04-29T04:53:09", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "riverhead", - "permlink": "simple-dashboard", - "voter": "steem-id", - "weight": 10000 - } - } - ], - "ref_block_num": 17769, - "ref_block_prefix": 2807938265, - "signatures": [ - "1f7e3a0ecf13c6019a63beb9074474690a5ad98365e2959f0a918849558978bfd928439de6e1a67d385132a9e71d4d624f2cd1a18ddc6576a7f48800e27a86c11c" - ] - } - ], - "witness": "clayop", - "witness_signature": "1f3d7a94eb18b3a2442951d038f25e22439f1619582db41142a9596e800dea002a20cb9e5e1a412029c93e71482e3229afc7e47513e0df67dbad1c45e31083cbe1" - }, - { - "block_id": "000f456b25fad9d086b842c0f66f070a7378feaf", - "extensions": [], - "previous": "000f456a52a21a2fb6eb2db06041785360ee6deb", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:52:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "205e51f78d802669aa4b6668a70d3701f277f40535baa87b9505014cfe9f3a814d2e7a9ed05f3ee2d0cb7b14d770f5f6f416916b581cb1c453320ec91847aab1e4" - }, - { - "block_id": "000f456c7994e6a65cd79e40ae2c51aaf1890c04", - "extensions": [], - "previous": "000f456b25fad9d086b842c0f66f070a7378feaf", - "signing_key": "STM4vhtognZwHz1sNX2efiySFap35k5M3g186nyjBAnbwPkTHCBT8", - "timestamp": "2016-04-29T04:52:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "joseph", - "witness_signature": "1f4fc10c04b92e5ab42b676208ca55b0d333b3ec674b39223e99df2432e0ff1c8418e8a0cc7cefdbe8667a1b4f8492c7dc7a33c43baea9c7c7347ce88724727a07" - }, - { - "block_id": "000f456dcf83ce7957e64492d8c802543ccec190", - "extensions": [], - "previous": "000f456c7994e6a65cd79e40ae2c51aaf1890c04", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:52:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "201e5f945846ede664675140b4ba4dd8ababa8cc8ed0679758f278136155be4d831ba3939a066d4a02ce2aba1921cd2066f36bdc226b4be1e00a363549a4017cc5" - }, - { - "block_id": "000f456ef5fb1aab7cb791250e57036df8cceb06", - "extensions": [], - "previous": "000f456dcf83ce7957e64492d8c802543ccec190", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:52:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f421fe832289aabfda99ebec6ae6004a1f04784f9551eab7a421e843b85c6b06956ece3057f4c22047062b106b82e8ad3f9e6f251f4330fa71d86ca7bd219eb1f" - }, - { - "block_id": "000f456ffd6b654a8457a36edd6f6a6ee1fb4ee9", - "extensions": [], - "previous": "000f456ef5fb1aab7cb791250e57036df8cceb06", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:52:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "2075860318c5a7f38825111a2885897f60d5002b37b117a192d6fd244dd0ec0671080cfc721517b4b07e284ad3b81fc17118c1a0b95b7442a14073aef20d032a3b" - }, - { - "block_id": "000f4570594e7de7e726b461dd6cdb13181e483b", - "extensions": [], - "previous": "000f456ffd6b654a8457a36edd6f6a6ee1fb4ee9", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:53:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f5eebf6d857ad003113432b88f05539cd7b18910c77060ff6ae885e8a9168adbf20202012eae9d9d01f6bb4944b2f899d2a4838135ce5f5d5feb0c8da9811348d" - }, - { - "block_id": "000f4571934e76492d70f88b9e7c340294007c2c", - "extensions": [], - "previous": "000f4570594e7de7e726b461dd6cdb13181e483b", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:53:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "2055592fd4b11923afc46698da944164651491dcad82e5241bd1ad9e8b8b3b71656f3bad47b52b6150a3aaa40c59672953e80c17c4d6fd749dc2fecbebd418cd29" - }, - { - "block_id": "000f45723be2813d3fccc8af4add8f6666364a4e", - "extensions": [], - "previous": "000f4571934e76492d70f88b9e7c340294007c2c", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:53:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f64886fad1dd4aa4a9c9ace58eded1e594d805f3266ae03972a9d0751ff33c5eb5cba7d2d53ec7515d499cec2a95392e230b1bb8eb9a70f8a9ad550e23e6cf5ec" - }, - { - "block_id": "000f4573aec75272955d192b7f091b35e055fe46", - "extensions": [], - "previous": "000f45723be2813d3fccc8af4add8f6666364a4e", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:53:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "2079ca5ac764f51b6f26e257830de434e13f47f5bdc14cb3d7ff0a2b97b80c04435ffb29f8189c5590a72e46b6cb35de574305a845ec4cb0b6fe99bf316d3548de" - }, - { - "block_id": "000f4574afb7853faf8c42db9e8a946596e81714", - "extensions": [], - "previous": "000f4573aec75272955d192b7f091b35e055fe46", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:53:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f1a9b5f93a3c4577743b6ddb0b477745ab68c8081a53fde17812d88a5b508cc6514ecf6464364160d9ec71798105247d119aa7e99be5d78244cbc190b559a3018" - }, - { - "block_id": "000f457504bd8f076d019837315f88d18f750e2b", - "extensions": [], - "previous": "000f4574afb7853faf8c42db9e8a946596e81714", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:53:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f77d73f1a04d6ceb78ffe680a098a5d8f406efa68b537fe922a8e2e18f36b0feb1c1a2326203390b908eae103237c7431398688fc16d6691e24cbab0446506205" - }, - { - "block_id": "000f457697d3b3f4b56d27fb2f0c5ec31315dc68", - "extensions": [], - "previous": "000f457504bd8f076d019837315f88d18f750e2b", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:53:18", - "transaction_ids": [ - "bc07965d09d0deec05f2cf00a7bf25f76f3ef38a" - ], - "transaction_merkle_root": "97410b94f555c8fa5b17a803c46ec3c38437710e", - "transactions": [ - { - "expiration": "2016-04-29T05:53:15", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f457504bd8f076d019837315f88d18f750e2b", - "nonce": "7402656884829379964", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "9320faa917a92d0551aaac7d8168adaedd5734a9268db345d2194f7b1e9fb00c", - "signature": "1f8b0331e1dbf7564544eb307f34baea95c429974a6ca1da9c892a364634e8dcb34e1606891006082e723b14525fc8faf0d97e6b7d29d72cf509b64f50ea2450ab", - "work": "000000140a86d5c68c58ceef86d58d052da92835bd8c0300662d5c83b9ddec46", - "worker": "STM7D7oC3o7YYLoVVd47qXr3tMaABtGGsvWiraQQt2jtnX8gZAjdB" - }, - "worker_account": "hr801" - } - } - ], - "ref_block_num": 17781, - "ref_block_prefix": 126860548, - "signatures": [] - } - ], - "witness": "bhuz", - "witness_signature": "2047933fdf88307a02e276efbe93a671ba1f31b971dd34a4845640c3b07f65a1b101ebf4d889ad88aeb0841c34b5537f6c659128c83507ae046da1b7539fb41e42" - }, - { - "block_id": "000f457777bde56a4828db46582019fb52165fea", - "extensions": [], - "previous": "000f457697d3b3f4b56d27fb2f0c5ec31315dc68", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:53:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "200fe94594d233cab7b974b6fe416cdd3dafe6a57143dbee3ce8392a9ecdfa2ee476c69c817a1d8ed27789e76dc4d05256f074b81fb7f56727155963018a5cf86d" - }, - { - "block_id": "000f4578f5dfc8db00e516b20cfff0a1d478f161", - "extensions": [], - "previous": "000f457777bde56a4828db46582019fb52165fea", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:53:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f48c329b09b7389ae544af3164eae7df365e1367583cca55051649df6bbfc6eda0d3418b8102e459c4dae34264b714e7154776c60d6faef3900a7646062a2fc4e" - }, - { - "block_id": "000f4579d428426ab8e0ae839c55f79d142d7b66", - "extensions": [], - "previous": "000f4578f5dfc8db00e516b20cfff0a1d478f161", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:53:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "207f44c787fe0c5ffe704f472e6c87c6651cb2867dd8d419273087fcdcbbf319904c218be57dbba6137ae7fadc328ec7c0ab91153196fbabf3d52442f048aeef6d" - }, - { - "block_id": "000f457a85054fe2a25fedb27a5dbe3b554fb4ac", - "extensions": [], - "previous": "000f4579d428426ab8e0ae839c55f79d142d7b66", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:53:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "20536f5668c5225a4b7d0b8d28d55fe2446016801ac8fc8370dd98a499f9fb22de50e5d7312ee2eea3a7de6408779e14c497438a3ea812dc2bdab55bebdccedbbe" - }, - { - "block_id": "000f457b551420181c1f7a794ada19623faefe16", - "extensions": [], - "previous": "000f457a85054fe2a25fedb27a5dbe3b554fb4ac", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:53:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f3eff16ae290549cf32d19c33b80f2719e9eb5c73cd1102d919f06253ad6bbff615c519c0e2d3a2cf3e6866e00b630e75d950a029cc6e5fb1611c60fecd58ab9d" - }, - { - "block_id": "000f457c554971f73868131b48e707cc1278281c", - "extensions": [], - "previous": "000f457b551420181c1f7a794ada19623faefe16", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:53:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f7aecd42617b11876e5f6c3cb4091fc034c38f7ffe2027dc92c9a7e1b12fb3e8e07038b661a036c2d94a8279c8dc1baa0bac24b92dfd3cc2826c1a2c8e8c3da99" - }, - { - "block_id": "000f457d824fe873df733c908e10629202d166af", - "extensions": [], - "previous": "000f457c554971f73868131b48e707cc1278281c", - "signing_key": "STM5742TmF2wyuStKtgETi1KDycZ56NNvgJhAt6xWA3PA1Rx61FvU", - "timestamp": "2016-04-29T04:53:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "cyrano.witness", - "witness_signature": "1f31750defe329ab4e9bb004ec4e4578966dfd700388314f3ce801cbe7df7ce8ca15c3a37b93fb411b994739f5762fd735ded2d84eff71a66074e2cb209f70e929" - }, - { - "block_id": "000f457e21fe74c01ab02c18cd5c3864fa307508", - "extensions": [], - "previous": "000f457d824fe873df733c908e10629202d166af", - "signing_key": "STM8RVh9ZnJZhULNSQwRUueSJSKtJUjNMPbnyRdWmEJAVCTJZ5AYT", - "timestamp": "2016-04-29T04:53:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr403", - "witness_signature": "2042a774ecc6d5e41d26314ad2154112cfb8dfada4e3f7c5aa32ed1024f6f3c1822c010d4969de77bba2558a7b127c2f2fb13b44a1b377892890e6d3d2e1c79a98" - }, - { - "block_id": "000f457faa1e1fa5de920bd659aac0dc732ada63", - "extensions": [], - "previous": "000f457e21fe74c01ab02c18cd5c3864fa307508", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:53:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "2031e107a90b76dbf1be99d0f2d7e549feacd3c55894417142e495e6f26e7c95973a8147c36f1e0ab412086f61f33e8a2e9680c08baf1b7e77b5e82f0c50eac966" - }, - { - "block_id": "000f4580c0f5c441dc32ef689769ffe4961be3fa", - "extensions": [], - "previous": "000f457faa1e1fa5de920bd659aac0dc732ada63", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:53:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f503657da82a9361205ba8168dec03ff053c2533707567c41a73b7d9052c493b54d28e8c1197e9a4dc7461005e44b6bc8f6b61b3f503fdf2bd508b0b0d23861a7" - }, - { - "block_id": "000f4581e35bf3b73c09215a976111a880209fea", - "extensions": [], - "previous": "000f4580c0f5c441dc32ef689769ffe4961be3fa", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:53:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f677d6bd803395f7e2032c559c9fb2d5e6ffb0b1ebdd9cf41555ecc35e6ae16d91b510f45b2e7ee156156f9a8ae52e340fe1c12d34fd2982dbed3244fd4199aef" - }, - { - "block_id": "000f4582720e083bd603457121a807d2e9161dfb", - "extensions": [], - "previous": "000f4581e35bf3b73c09215a976111a880209fea", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:53:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "205ea8a42a9d6e31262647177a1ee5458c39285207b2989c360e2842d27a7c50ad217917dabd7b1daef2d01f2df2ee1a2ecc8fe673c801d6c93c10267100fe1819" - }, - { - "block_id": "000f4583262fc7090936836f80d2295870525891", - "extensions": [], - "previous": "000f4582720e083bd603457121a807d2e9161dfb", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:53:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "2009476ef15453bda17924f161fb402f8cef6bcb498d0f15e2e6985dd33c3c2f1d71673f7b43503d2f470555fbcbfad753782fb73633afa36a525787f504fc0cb6" - }, - { - "block_id": "000f4584cb1baab98d98b7ff662437f0f5709031", - "extensions": [], - "previous": "000f4583262fc7090936836f80d2295870525891", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:54:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f6d9db94b8a26122dacb7bd62e49e9da6adc628c56480920497a2cd1b5a52039f64250083d2d109fc8621ff7d9ff69986175b320639cd221904f073bea114841e" - }, - { - "block_id": "000f4585a42962bdb9731c29875bac7d4e836c76", - "extensions": [], - "previous": "000f4584cb1baab98d98b7ff662437f0f5709031", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:54:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f41782246f7cf8fa8df6ffaad2584228fd648ed683983a8fc7d09a4c6f6a3e9111efa1ffb0f3e8a024a2e958a92e03aa85c331e777f68db683e1bd1aadf9e2cd6" - }, - { - "block_id": "000f4586b828449d9fabd1e2239a582ef96bd848", - "extensions": [], - "previous": "000f4585a42962bdb9731c29875bac7d4e836c76", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:54:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2017029a31a2fe44ffaabfb798e22199d949fc347fca1eea830105da7236a3101f016eb4df6fa93a6bf5be0dd53a1a5743d1ca9a5c02db92ad1706a65f7821bd28" - }, - { - "block_id": "000f4587fdf5d1f74ddec2d6bfef7280a78b7bb4", - "extensions": [], - "previous": "000f4586b828449d9fabd1e2239a582ef96bd848", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:54:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "205cd54804068d2e78495b4e11c9c437776bfeb50883a104f417fbfa86a766613350e319137cc0f6c13adfe4643ea62fdbea01d06254f4c7d4a24c22ebb6b83d2b" - }, - { - "block_id": "000f4588fbdf8b0d734951e30e28700b580cea08", - "extensions": [], - "previous": "000f4587fdf5d1f74ddec2d6bfef7280a78b7bb4", - "signing_key": "STM6ChCh8ryiuwfbzFfViZvh6t6gAZzSg8BubWQbqUBviHCSefFkd", - "timestamp": "2016-04-29T04:54:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "hr703", - "witness_signature": "202883413385f0b86203f3524be191a24cd4290afeec51a88d4f786e1a7ac77b6855eb6e09803ab9e478e592238e00cfbd14f1000d3312e138d5fe8a595d8a4197" - }, - { - "block_id": "000f4589207fe6d9d784f6d3d4abdf458dd0e7cb", - "extensions": [], - "previous": "000f4588fbdf8b0d734951e30e28700b580cea08", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:54:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f3327fb452aeb22401102e878ddf6f0c95f8c29646ebe6cbff157704d308a165e4b7fb850606b47beb0b4dee29d16570fbcaaebf630d891ebb5094db349712ee1" - }, - { - "block_id": "000f458ac8749e7c790860ee072b248cadf6a4e4", - "extensions": [], - "previous": "000f4589207fe6d9d784f6d3d4abdf458dd0e7cb", - "signing_key": "STM6Kq8bD5PKn53MYQkJo35BagfjvfV2yY13j9WUTsNRAsAU8TegZ", - "timestamp": "2016-04-29T04:54:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "wackou", - "witness_signature": "1f00b96c6b3f666b35c1a186407d0130ef5bf01924b9668077c7ceb4e4aa0e410b332944c107ac5c8a1dec1d566ab8fa5ddbbb106b3b87232164c66f4e04a173ec" - }, - { - "block_id": "000f458ba837953c2a82c6b2b3a760c7e6726a5a", - "extensions": [], - "previous": "000f458ac8749e7c790860ee072b248cadf6a4e4", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:54:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f6a8aa95004dc1a14886a69895f722c8e508ae7441ebab9627cffa65291b91b9823b104010ef457ff605f6e38b2a6300d002175282402144d2860679ff927d6f9" - }, - { - "block_id": "000f458c018de054fdef2020d62912b9bd0ea5d7", - "extensions": [], - "previous": "000f458ba837953c2a82c6b2b3a760c7e6726a5a", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:54:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f380b06bb3ed047459b5da5edb8050d46afcb8a6451501006eeebc9146321b4d40be805b65f61bcbc069234bfae54d76003744626cfc0a13de3b44e6783585778" - }, - { - "block_id": "000f458db7360149281e4a552f93874f76e21fa9", - "extensions": [], - "previous": "000f458c018de054fdef2020d62912b9bd0ea5d7", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:54:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f5cc4bed0f76e100dffe80975eddf50ae043d742e4390cfb67b9fa363a01bee7635d88d1cad4ac62afb1281260cb28f787a97d144152ec45f93d3b086565c4d55" - }, - { - "block_id": "000f458efb5312a8ef8b74671c55092598cffea0", - "extensions": [], - "previous": "000f458db7360149281e4a552f93874f76e21fa9", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:54:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "206093f9e7ca4566d31952abb9894b8e78d7e8105d6bbd0ae386557097ccc4934938715770e17eee57d7c0163ef45d7584139a8524801b181e526072504222379b" - }, - { - "block_id": "000f458f7e511abe1efe158cebea7dbc080b6545", - "extensions": [], - "previous": "000f458efb5312a8ef8b74671c55092598cffea0", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:54:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f3ec292dd2c93686671db4eb4bb33776d8f509b66612ceb5d76ff38db10fbb0c000ed5cb73e4cefe0a7d10cec15abbab64cf31b30da1fc81ae06a736fa76ff133" - }, - { - "block_id": "000f4590062acbad3d300cd1122866073dcd95f7", - "extensions": [], - "previous": "000f458f7e511abe1efe158cebea7dbc080b6545", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:54:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "200b6fa49bb98b76aefa5cd14c8790f17b87a7709627fea016862cdb0a7c8560a637f56da81c92ec36161a23f01cf1709a2b7dcbad1a194feecfe99c01efb45583" - }, - { - "block_id": "000f45919db144ed2f81364d716b16b547cf8426", - "extensions": [], - "previous": "000f4590062acbad3d300cd1122866073dcd95f7", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:54:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "206492dae74b09f662d4be1f927cc45630d62825c7469420663a1c3bdf454dc9612009284448e7961f5f010c82236fcb31c90b431d3c762f2d282deadca2c15c5d" - }, - { - "block_id": "000f4592cdc1e99763a9e31ce40baefa4bc7082f", - "extensions": [], - "previous": "000f45919db144ed2f81364d716b16b547cf8426", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:54:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f7e93a9b66be4912c2a2ec9e8f43e4631da1d7c20c218ada9ca9f6ad0b7a9654f617501727c2ca01cff79c05ca34a4007c2bc7f4bba3dde361f830045565ee5b4" - }, - { - "block_id": "000f4593ea60cc8137d00dab644b5701427b1010", - "extensions": [], - "previous": "000f4592cdc1e99763a9e31ce40baefa4bc7082f", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:54:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "201eba2c0ef36fae78cdb447ff4deef0dd399f45bde4f6b3796b103fef51edd9e145040475ea93a0f4d32b53dfc38d8285b5f94f13a4ae25c4fc9164f5cfd06ae2" - }, - { - "block_id": "000f4594e2d56a08cc573316856f07e3752bf1f0", - "extensions": [], - "previous": "000f4593ea60cc8137d00dab644b5701427b1010", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:54:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f56bd9594f40c6e842acfa409f8f237d303f95f8c2f532efd8fe2069e0cb8cc2540474343d3cab0ee622245bb4d3dacb216c0342d5b7a605a1b9b156c50b0daa1" - }, - { - "block_id": "000f45955497ef3b1edeffcbcc703185c7ce0648", - "extensions": [], - "previous": "000f4594e2d56a08cc573316856f07e3752bf1f0", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:54:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f44ddc6a98bdfb2148b4523349e97f6284f6adc32ffeb68434cb16fe11622f7a747af0ef53ae553510787736ba97fe523e20673e865a18ee476ca967341673484" - }, - { - "block_id": "000f4596b17479743269d5fe35b312a02e637e31", - "extensions": [], - "previous": "000f45955497ef3b1edeffcbcc703185c7ce0648", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:54:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "201914aa5cd110f59eb7b3c9b7f572ac99864e29129cf54f2750a915d55c4e250c23efb38f69e4b1b5b8e5d9ecbcc3af84fbae9d09814a2de9ffdef4fdda644ee0" - }, - { - "block_id": "000f45978e172793f986f95ab0ff045018240827", - "extensions": [], - "previous": "000f4596b17479743269d5fe35b312a02e637e31", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:54:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "2015fbb40f4e300b05bd862bc8dda354773403b3444881be475d8ca7bb00bbb80831c5bd5e8115574ea26f92243685dc767cc87f3a8ba437d572736a4b2e7eff88" - }, - { - "block_id": "000f45980cfa999000c723d89f73d10c7fe75fed", - "extensions": [], - "previous": "000f45978e172793f986f95ab0ff045018240827", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:55:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "2058842df7f3f9366a0d64cc096c3d4ef5b8121f15bd5a9383f7c17f2e640ea4002e61776f9bc46548a0d4357d0b13706e39b617ffbd55e4de06fcad50e86f569f" - }, - { - "block_id": "000f45993ca5ef6db9d6b7d1b3e8fb8698e6afce", - "extensions": [], - "previous": "000f45980cfa999000c723d89f73d10c7fe75fed", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:55:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "2041546ccb2dd703e0a5aca1a3e68df45704a58d0a633fa274d0ab32bd6d60b43d3f275af75e4a532d1a9e06d030b5edaf86da8d380413027212c866cd8a8c93a3" - }, - { - "block_id": "000f459a63e8f0ecb6d6c3e1efbe175604fddac5", - "extensions": [], - "previous": "000f45993ca5ef6db9d6b7d1b3e8fb8698e6afce", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:55:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "203cb7d33cfb9ec32b307b7bb846052e0b923548aa6185b6274c55399fa8f4dad02f133f01d0a15e20393d1e44f13e77e1cf13516de27fe54b3ab40ddc679922e3" - }, - { - "block_id": "000f459b00c78d404784ef7f7e6955259900c69b", - "extensions": [], - "previous": "000f459a63e8f0ecb6d6c3e1efbe175604fddac5", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:55:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "20247d6d832fa7f9bc71381b7e33b03f50d49c7bd051ec6cbdefb7dd9a307beab33271543dd85fc4de41ed48629082c0830aeaab3f3f245564b01bed3e5fde32eb" - }, - { - "block_id": "000f459ccd064009465c61c8ee6eba32ccffe463", - "extensions": [], - "previous": "000f459b00c78d404784ef7f7e6955259900c69b", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:55:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "206018e727ec3345fe613a02f5775581beac56114cda8d2bdd6a7f547032a7909746cb205d36d598142877e68610780af111c7b75f6a591a1612aeaaf9202437b6" - }, - { - "block_id": "000f459df758c0078c950ff94e9bbb86624cb1b2", - "extensions": [], - "previous": "000f459ccd064009465c61c8ee6eba32ccffe463", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:55:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f1ac8e0447b31484b7eb7cb249f567f7952b6b136e99e1aaf8a843c73cbd386c028704d85e158fec5b5632b9740fd53cfdfcb3f5189d78042297dd7d1f6eb723c" - }, - { - "block_id": "000f459e86ad0940be1bcd726c0457ae28ac2d64", - "extensions": [], - "previous": "000f459df758c0078c950ff94e9bbb86624cb1b2", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:55:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f78a08c79380b65f2e37da868b7c6942822c89b2267e43306e005ffd73d57370b59c4bd83f0a92bdc218c8589f2ee3ba43592ed4c59afc96b2f2f36627ee96fbe" - }, - { - "block_id": "000f459f447a830c9535e06288136bd6047672f9", - "extensions": [], - "previous": "000f459e86ad0940be1bcd726c0457ae28ac2d64", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:55:21", - "transaction_ids": [ - "644e1bdd6d3fabcf78ca87c909722890bad38718" - ], - "transaction_merkle_root": "7e7747469c1850a3a493eb6d1e9515de44300838", - "transactions": [ - { - "expiration": "2016-04-29T05:55:18", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f459e86ad0940be1bcd726c0457ae28ac2d64", - "nonce": "5402191577726870504", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "2e9078f21a216072703b801c28bcc2d0512fc4c72cbe2136dca3fb47e1f136d5", - "signature": "206832d648b1b1fd80e8acae40cc17a420b20dbaea2ef6202ab25e2512f01f4bcd06b57a4b3c214cb3ea36c3b145b206b8a06b07a51fe28c4c906478e58b7978d3", - "work": "0000001871d6e5e5308f747f395fb72ab7c653df16b99af5f963271391862783", - "worker": "STM5ti8pn3E88w7ANNYmubYaSumfXoqSpE3u1yxYyovtPUdUGZZMJ" - }, - "worker_account": "peter.conrad" - } - } - ], - "ref_block_num": 17822, - "ref_block_prefix": 1074376070, - "signatures": [] - } - ], - "witness": "kushed", - "witness_signature": "1f07eaf3a6ffa04e611c979463e3775fd5f9062c80e9b64e3eeaefd44075fec10c10eaa69e1ba0dfc48248a68dad1a0b322a6097bb1ac6806ddc274dac2ce22b96" - }, - { - "block_id": "000f45a094f6e1eaecb3b993f496c9f6699ff91b", - "extensions": [], - "previous": "000f459f447a830c9535e06288136bd6047672f9", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:55:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "204578962607de2eb1fc24796e4197ae1051a88174a7f2ca759303c6f845cf471775ca0e008e69730ed0d750540e1cacf5f2bea13df8940ef409f00d47d34afc91" - }, - { - "block_id": "000f45a1fc676e2699459de7e8e8a3d7c2a73b34", - "extensions": [], - "previous": "000f45a094f6e1eaecb3b993f496c9f6699ff91b", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:55:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "2029b84edb93c07657bd80bb61405c717c8927aa5b7371dc510546c9bdacd405ed465051b0a050c1b7fff8d15d0f744ed28f5253576820bf912f6ac26fa26ddcb6" - }, - { - "block_id": "000f45a25ebe50f2ea2a3bf5d6f2ef6ab5dd343b", - "extensions": [], - "previous": "000f45a1fc676e2699459de7e8e8a3d7c2a73b34", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:55:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "2073a2392ad6e6c168bd94bf1d4fd86984baef75dfad39efe1bd3966e68c08d4507f681cbc35e9a64a8c0a01b894069178fd5248e98192504e2eb55a358f6dbc5a" - }, - { - "block_id": "000f45a32557cdbc4a295583edafd5781b2bda43", - "extensions": [], - "previous": "000f45a25ebe50f2ea2a3bf5d6f2ef6ab5dd343b", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:55:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f5a4ce7f42ac54bcaa9676c5c5d97032761f816fc383f77508b3113867e74e0df48b5cf71673a0e08f01ad81ed8ad2ced674de14b10949d5e3b11cdb3681e981f" - }, - { - "block_id": "000f45a489432fef4b0be36871aff37f015f035e", - "extensions": [], - "previous": "000f45a32557cdbc4a295583edafd5781b2bda43", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:55:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "20794daea724306490224fbd5ddab9e8c9fab752963215b25d692eff2e0bd29694718618ff5cd6139865b3aef7407167f09489ee940d6086baedbdce977d9c64f1" - }, - { - "block_id": "000f45a5312333f4e0d6a5ddd9e9770d84709b01", - "extensions": [], - "previous": "000f45a489432fef4b0be36871aff37f015f035e", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:55:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f731c3fc71c189fe4b3b383ca5ff6298ad9b0159ff676f14f528f5a0d7836aa865cac17f12beb8b736e926e20a1e4b6a146195e6e2c7f21fdd225f6568b0ed0e0" - }, - { - "block_id": "000f45a6549f8d36fa5297372b3fab85110808b9", - "extensions": [], - "previous": "000f45a5312333f4e0d6a5ddd9e9770d84709b01", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:55:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "200d57a2c6d1baf07fd6fc611fcac239e4201fe246a0aa46e82cf8ab6af4acb8dc51baeabdc38d330cf79694d8db1e0669c3c274c7f1127abb9ac6cb3f54e84d7e" - }, - { - "block_id": "000f45a7f1ed88da9a003047904be32989165d9e", - "extensions": [], - "previous": "000f45a6549f8d36fa5297372b3fab85110808b9", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:55:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f4e8b0575c3a874130991f39a8eb6a655b76b1e658f0ec42961ce985cae7c058105d152d205dc0eea2d1a957b972cf84da81ad0a2c78d40ff247d88c93c04992e" - }, - { - "block_id": "000f45a81696bafe4033b7811aaaa11680402aea", - "extensions": [], - "previous": "000f45a7f1ed88da9a003047904be32989165d9e", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:55:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f631b1e9287ab1dacd3bf781a5ec78b961dee745655135a8fcb612abfc3488aec771b18cfb351fbaf1740366b15da0c696a3fe195571360a69a688565233a0782" - }, - { - "block_id": "000f45a993fe466b62bb9d44e868d59c0011b810", - "extensions": [], - "previous": "000f45a81696bafe4033b7811aaaa11680402aea", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:55:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f5a2bae22b9c478edfceabf9252cd66f587af5ff93ee22aea56c08bb820b5721328763603d0ae2dfdc3b2b83e8556ad07521ee8e59f79fe5e71227791d37bd183" - }, - { - "block_id": "000f45aaecb6f80e74824b26a0cc1b384c1f585d", - "extensions": [], - "previous": "000f45a993fe466b62bb9d44e868d59c0011b810", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:55:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "202e529ac01f9875c3b6635699014d9bb3e8cf58c9b8b317715b004b9e4f1e905b7a18cb124825237d6f3ab91931b8bc7e0beec89f9c3d0f0907338c047a61dc90" - }, - { - "block_id": "000f45ab4a71fd10ed414e91a44306e48c9089a5", - "extensions": [], - "previous": "000f45aaecb6f80e74824b26a0cc1b384c1f585d", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:55:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "204a7cb379aa3c1dfa0ce4572f5e641a5bffea85e96e9e90797b38a3fb325cda43106019cec11e4b5befc4376e8997280555ee1316519c0032de57c528b20739c2" - }, - { - "block_id": "000f45ac55779586a88aadb8fd9be9e50acd53fa", - "extensions": [], - "previous": "000f45ab4a71fd10ed414e91a44306e48c9089a5", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:56:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f38489093118ead563756937cf948b8a72737b4482a3a348b7159c75a05b1d324036dd5c0b7d3b2d2eb1e216d611d382898f6057274a30786d6d47c23bee5a9cd" - }, - { - "block_id": "000f45ada2e8bcd19c58bf625244dabbdef7341f", - "extensions": [], - "previous": "000f45ac55779586a88aadb8fd9be9e50acd53fa", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:56:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f16ee2b09914beb3b8840c06442679aeb3372c77fa043215a26884ad356fe7eda117a99541a2d15f6bdacb143602fabfeffbc323d8ef31ea13237e2c8577da003" - }, - { - "block_id": "000f45aeb6c87f80b547d518617ba9f60a1c5b67", - "extensions": [], - "previous": "000f45ada2e8bcd19c58bf625244dabbdef7341f", - "signing_key": "STM7YP2em2Cwug3Wnq6QjuSHkfEiXYh7PapeFDVWkGXR8Wmi6kKVc", - "timestamp": "2016-04-29T04:56:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "alphabet", - "witness_signature": "203c3576cab4202a15676f99d7b4cc1056bdb71378b89f361c035d8e85d7220546187d84917ec41dfd3ba223919bfdebc485aa75fe587ee0979e06d91e571a689d" - }, - { - "block_id": "000f45af9cfcb7aaf65e4fd471cb6a2f72c7576b", - "extensions": [], - "previous": "000f45aeb6c87f80b547d518617ba9f60a1c5b67", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:56:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "20725e6b7126dc0a08d8aab8308bc8bf8a84f4b2c2376c0f129bd9a242a6c8c882731c189aae597f3a33a288cb6baf1e32a359a65fa9e2a942feed5fc0ce4e9bd3" - }, - { - "block_id": "000f45b0a214c8e7fb2b60499479513a5a083c96", - "extensions": [], - "previous": "000f45af9cfcb7aaf65e4fd471cb6a2f72c7576b", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:56:12", - "transaction_ids": [ - "55d7171dc611fb7dbb83f00fdc1ced6368301b7a", - "dd321bd8f1ee8a5c67c94284b68fd8f69f6a0b85" - ], - "transaction_merkle_root": "5356ae2df8ee6462e24734e06370f6e5b0779d84", - "transactions": [ - { - "expiration": "2016-04-29T05:56:09", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f45af9cfcb7aaf65e4fd471cb6a2f72c7576b", - "nonce": "9533764316779686410", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "dc24e39611dfe7f7c47f14451f4f0f3fe65ceed801a7155da8d91700759ae0ea", - "signature": "20aaa3e221204557dac5e0bd8d5a4a64f3dafc90ccca2710f419768f0cdbd881b96dc8975deca072901d12261144383cef90176652a657bfa8f3d8651624ef3784", - "work": "0000001d970bfffbd4f2c1350cbf48028eb72cf3ca74750103ee2651c0da0b26", - "worker": "STM6k58ygyZRmunSctdyD5F7gmJuQbm9kedCpHMvzKX7N1YjSbv85" - }, - "worker_account": "commedy" - } - } - ], - "ref_block_num": 17839, - "ref_block_prefix": 2864184476, - "signatures": [] - }, - { - "expiration": "2016-04-29T04:56:39", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "434", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "au1nethyb1" - } - } - ], - "ref_block_num": 17839, - "ref_block_prefix": 2864184476, - "signatures": [ - "1f49c16fecf53e9546ebc7f522de64f94ee252cf21ac00b860983a00265b1e05e35c96ff8474e65adc911cb9da48b0e086b4ec1bff12dd8b0b4fe57808ed74692c" - ] - } - ], - "witness": "datasecuritynode", - "witness_signature": "1f1b0f8bf3bc85f3beed7e88e17b7a49631b20e3d694ffc048803bc60ce1d0219a05c52d22ad0e3b6ddc21c9a69f6dfc2aca759c3663dd8e4e8ac3e22b2e1315d1" - }, - { - "block_id": "000f45b1b345affedba147b2ad3965103177125e", - "extensions": [], - "previous": "000f45b0a214c8e7fb2b60499479513a5a083c96", - "signing_key": "STM6BJYGHftujnbttFFKX6YacnvsMd4sbJrbucg682GiU4vmXHTik", - "timestamp": "2016-04-29T04:56:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "riverhead", - "witness_signature": "1f6d7d61e0c8341d94f95202c32b6edb0f89d47aa0f26a80c02197af3e539df6fe5b0f6b99dc444a428dde0bc3f576b8341e0b5d5356da42fcdd5ca02ee4c7f803" - }, - { - "block_id": "000f45b26453522d975e731c5c84a52c62fd5ac2", - "extensions": [], - "previous": "000f45b1b345affedba147b2ad3965103177125e", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:56:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f35114b7be300ff053e51027fcc594c3a11a5db460581b5e65f3837202817415c17873bd535a95384103fd5d75d232875bf7f78ab8e5fd4085c6a7b52032a9503" - }, - { - "block_id": "000f45b38eeeb0f798a45a1a6c5e26619b64107c", - "extensions": [], - "previous": "000f45b26453522d975e731c5c84a52c62fd5ac2", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:56:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f1dcedb9c67523df46e4911dcff3e92d6a0f1700074f8bf93395455b1b6aa73ab35beaa5bf10c4fd246b6c1f0e01e0e85d508bc21e967878ac61a2b65ee90cb23" - }, - { - "block_id": "000f45b43f4b282696005f9f0d21b91b3b2c6f1e", - "extensions": [], - "previous": "000f45b38eeeb0f798a45a1a6c5e26619b64107c", - "signing_key": "STM6joyzLxjK3M4zpExQXp4BNy1jQwaErNoBuGPAdkx7MKWw3QgzR", - "timestamp": "2016-04-29T04:56:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "tippero", - "witness_signature": "2009a440e944d0e16f6f451d5dda6e3967d06b4f2752daa2ebedbee4a54c9f7f5208f98851373dbc759cd1bec5c24f4c83b094987453cc1f2ce61418f845f1f6f2" - }, - { - "block_id": "000f45b5dc123277efc4a78d725b1b45a83097b0", - "extensions": [], - "previous": "000f45b43f4b282696005f9f0d21b91b3b2c6f1e", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:56:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "20049b3129fa7428695e50ea25bef80fc3d66d663666b949b44db0db5b1d5f9319570111a186e27953013208b3069a9bb39ae191c0995e2a7a41e14d118f41c133" - }, - { - "block_id": "000f45b6e40116d5423ed2bf55450298e4b114ca", - "extensions": [], - "previous": "000f45b5dc123277efc4a78d725b1b45a83097b0", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:56:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "200903fb807fe0906627df8c2b94ea5a99598062501a03e7fc77252205c61deb763e7ec3e851a622bc805d134bcadc93f10d2a3b3fb6d66f5ff5305dbf89b167d1" - }, - { - "block_id": "000f45b79ab8e33313a99273e04f55d42e8024bd", - "extensions": [], - "previous": "000f45b6e40116d5423ed2bf55450298e4b114ca", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:56:33", - "transaction_ids": [ - "9e7fe362e076056f74f3466dc24763bbcb381acf" - ], - "transaction_merkle_root": "69421e257064af8465fbae58d08ab90fe3cb21c3", - "transactions": [ - { - "expiration": "2016-04-29T04:56:45", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "riverhead", - "permlink": "simple-dashboard", - "voter": "markopaasila", - "weight": 10000 - } - } - ], - "ref_block_num": 17846, - "ref_block_prefix": 3574989284, - "signatures": [ - "20552409a84f0af0707c5fd658d72a7d1c02606373b29e4be6a6f392749247c1aa3a1503522ff14a11b048865e81f891009a340cf64109c23fbbf3d09c9a1aab31" - ] - } - ], - "witness": "kushed", - "witness_signature": "20552bc0ddc2244114d7b6928fff673aabd889d59f288989d7ac8949ba057f8e0442ca3c599eb4880664514942905b96dcc56cc13f50ee075a15a6d69d339eba89" - }, - { - "block_id": "000f45b820dd34b07319d5400dc5863fea9d3330", - "extensions": [], - "previous": "000f45b79ab8e33313a99273e04f55d42e8024bd", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:56:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "205ba034ea5561aae64353f433e825f5d1f4520bbb211b93a457568f26bcfa4d502821733be8a76c6cd314285d932431d2086b9befdeac49e5eb972d5aab8f683b" - }, - { - "block_id": "000f45b9e0b23bc02834dbc7fbab7867ff4322cd", - "extensions": [], - "previous": "000f45b820dd34b07319d5400dc5863fea9d3330", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:56:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f01adfa6c6f6508eea1adb61dba07e2f6555fe78977f3d184e1e273afe37f860d4a6e0e9879f8ba78dc1653beed38316b1d0031e94341af7815b91b9e5f6575a2" - }, - { - "block_id": "000f45ba80b573636ce13cf0805d196e6f8821b1", - "extensions": [], - "previous": "000f45b9e0b23bc02834dbc7fbab7867ff4322cd", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:56:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f29404d391bc02135290c81d9656b1b3b1cbf12bdb9a5961ed6f253712225f05b738ef20a34e56d9f42a0fcf335ecfdf484480e4a1c0dc3a2b69f878816c32e7d" - }, - { - "block_id": "000f45bb473dfe5ea5f710eeab0d97eea7ff749a", - "extensions": [], - "previous": "000f45ba80b573636ce13cf0805d196e6f8821b1", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:56:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f5f9831c636902d7dcd2059754cacc6f1c736f7f968c1d46cbee9efef3f024a12414646ad1c3437d5c5a545187d28be76dcf0b421344185ef65486f222f0e5ba6" - }, - { - "block_id": "000f45bc97b9b313bc22668f557f85c4199406eb", - "extensions": [], - "previous": "000f45bb473dfe5ea5f710eeab0d97eea7ff749a", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:56:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f42ca2b396114ad39bdf9cd1690d4d8df17afacc3e89bc28bc14c62fa269d598a12f0c59218df1dba6df47114392ac626d0348066e856b46462e44dfb2f9e50b7" - }, - { - "block_id": "000f45bd1accc8086eeaf607677925776a1675dc", - "extensions": [], - "previous": "000f45bc97b9b313bc22668f557f85c4199406eb", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:56:51", - "transaction_ids": [ - "963920f857a76029057115ee98e0ada5d3cff2a8" - ], - "transaction_merkle_root": "44b2b66e05930e768a484096da0366919241928b", - "transactions": [ - { - "expiration": "2016-04-29T05:56:48", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f45bc97b9b313bc22668f557f85c4199406eb", - "nonce": "11994519578494225272", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "7b32b4fedfc3a371cf3cf6af2d8dab5bd2e4e32e20e8946f52f27869004dbcd7", - "signature": "2031eca0f62089afe3dcb5c3463f372c94c4148cba998eb1ab3d2a1b1574625481147bb95443af3a1478aecf4cf48a345ed64e47c1de0f91c661e9d0ec6400a63e", - "work": "00000003a082c887e9cb8d79768ed93bcd3b529f90902e9585d35f447ebef5cf", - "worker": "STM4yQG8BiozEtiBErWJG9MhGipmkAT6Bp7cWDnwSZkyUnMciVUyt" - }, - "worker_account": "hr104" - } - } - ], - "ref_block_num": 17852, - "ref_block_prefix": 330545559, - "signatures": [] - } - ], - "witness": "nextgencrypto", - "witness_signature": "2053208435e24f2b253b5c706be0a17b85a08321cd5244262e2631140d22b8c28f21249851058e12e167d82c5260fba31918bbe0be76e34c7e5c80eec0283265fa" - }, - { - "block_id": "000f45beca671987c2c14e4a208524d32fda5fa5", - "extensions": [], - "previous": "000f45bd1accc8086eeaf607677925776a1675dc", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:56:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "2040f42b22c957e01a05690e10513bebd511ae08e25b0050e8c5d55e7de9e16f1862d0026466cea6a78c363606ffb16408c6e40eee7b64e75df1fb3e0bea1f537b" - }, - { - "block_id": "000f45bff73c75ee6276b7954b974618323523fc", - "extensions": [], - "previous": "000f45beca671987c2c14e4a208524d32fda5fa5", - "signing_key": "STM8Uz1PaiWG7rByBGdfkmLtLdi1GVC2X3dGNnVCY82QKZAnGhG13", - "timestamp": "2016-04-29T04:56:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "salvation", - "witness_signature": "2003b94fd1b10716c2e10a64aa3dbcd3dca534b4f1702a801d9887420e80cffabe7591f34dcd515efcc3f2776fbd907fde32d23938a77c14f32005b8c212e0f985" - }, - { - "block_id": "000f45c0a3515bb84dc8f6dcf411ffe8d8e15bd7", - "extensions": [], - "previous": "000f45bff73c75ee6276b7954b974618323523fc", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:57:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "200e961e49d43d1de3b2ad974d5f8b104d1ed7d8ea53e6b71d99f5404ba054c26f0fc7ed0aed4e0b4551be0bcf03df0ec1a7cac15e51c33947f4fd9cbf201e2b76" - }, - { - "block_id": "000f45c126db4e40214acef89de23d30a29a0425", - "extensions": [], - "previous": "000f45c0a3515bb84dc8f6dcf411ffe8d8e15bd7", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:57:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f26214083a858a987da9266c0dc49e3b1bd0471a9cab6f9b91fc2b12e41204e6d15239deff770f0c100edceb2455343c5bde0aad335fa0833af9989c3549466ab" - }, - { - "block_id": "000f45c2076947c3941a5bbe3cd17832a7b72366", - "extensions": [], - "previous": "000f45c126db4e40214acef89de23d30a29a0425", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:57:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "20774b6e13e59f2dc4b04e294097421cd1cb63973c0f8299d27d40fd15900cd54c4441581b1e11aa0b4134f621a13f42fa517827d79c4475f4fbb886ada4e35a64" - }, - { - "block_id": "000f45c3454cd16131019971e32ee270cf63888a", - "extensions": [], - "previous": "000f45c2076947c3941a5bbe3cd17832a7b72366", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:57:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f1b4c7cb77f5ffbbb8eab34d9e0245e00a15a83cc2d1038a46a952af94881ab9d0705d0bb9783284894aa5a5b66b439355bc600d197692486208305a298b12494" - }, - { - "block_id": "000f45c4e050ea8ee71502aa1740ac66acefdfab", - "extensions": [], - "previous": "000f45c3454cd16131019971e32ee270cf63888a", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:57:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f038efa919106a64b1b35a4dcdb4e7d405c635fbc0c69de176045a36cb09bde7f01f598ffd768efe603564dba3626698fa19463960e5f537ce56b4b3e33337432" - }, - { - "block_id": "000f45c53805212707925316733e02cfcf27985a", - "extensions": [], - "previous": "000f45c4e050ea8ee71502aa1740ac66acefdfab", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:57:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f20609dea5b0cf51f0c9ac926aab9ce5e51e6d43195887e2e583cf11e2212a1ed39421a78bf6cf678dff84a5a297b36f11470c19ea45a8937c6a388c6a160d0b2" - }, - { - "block_id": "000f45c659d59863bdb2f5f5be4865bb3ca25264", - "extensions": [], - "previous": "000f45c53805212707925316733e02cfcf27985a", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:57:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "202052b8f367ef0862584288a3f5e0834be0720f7ae79102b0260daaf49d51a88f758d119718ff8b2cdb767266d7b7286f1d25c5605d50ee37c79d10c2cece7b4f" - }, - { - "block_id": "000f45c79342e445a467716aca215be05d600124", - "extensions": [], - "previous": "000f45c659d59863bdb2f5f5be4865bb3ca25264", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:57:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f6926a8fdd4620a151219ad757a4c6233560e18fb74db294826ab1835e8271ea05a1706c7164802e3783132f48e35a266b078ffb7bba2fc13c5293c5133cab887" - }, - { - "block_id": "000f45c8abd460776e9cbae24bc5b83a41035342", - "extensions": [], - "previous": "000f45c79342e445a467716aca215be05d600124", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:57:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f5de0621bccf2500dea9ab4c2db5a48a4c190bd15fe856051b9190b9a841ead47727dc9489ea7ac75e3248a3ef9f86c8421eb39d96d873e7f73f3ae7e7f9a434b" - }, - { - "block_id": "000f45c9e94f9b93cb69b7fdeb964ce1e455d13f", - "extensions": [], - "previous": "000f45c8abd460776e9cbae24bc5b83a41035342", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:57:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f5350752bee5c5befcc1cf4f320ee7bb494bf4f1372ce239216ff28c9681deefb7bc87a246f10e30b02da9d2aed1d75f1b43651ab33c1d47b8b4e921885c95317" - }, - { - "block_id": "000f45ca19034c87d87b1bdd5489d2e2ba9f161b", - "extensions": [], - "previous": "000f45c9e94f9b93cb69b7fdeb964ce1e455d13f", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:57:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "203da7638b30f9e65cf941865ec5465ad944aa3e0018dda5ba4da907f6019d289536efd2849f0cf7b07357f7e6f7c5d780fce6cf59286da326b98a12e2f15e3f50" - }, - { - "block_id": "000f45cb17e0b501270ade039a9d952401ccde95", - "extensions": [], - "previous": "000f45ca19034c87d87b1bdd5489d2e2ba9f161b", - "signing_key": "STM73XgqmDfLgXVF5VArK9rvHbUDbbx3HtfCU81Fy9MqVNxLYMpay", - "timestamp": "2016-04-29T04:57:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "jabbasteem", - "witness_signature": "2013a14980183e4abee7b395b7c6ee1b2210b5ece7ea692724017b5d2c557e26e8433a13e11b0688770f2ee95a839dab3403c476e694beb88ab25768d11a299126" - }, - { - "block_id": "000f45cc22d7c6f6366f1e93eee28d51abc67a9b", - "extensions": [], - "previous": "000f45cb17e0b501270ade039a9d952401ccde95", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:57:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "1f14e5d57dd322f92756c4ba6c8d823d3653e3bcfde02407962bfc1d018d9664c91eaa55da4591f1ff0766cb0aae19712652103033247c2fb5b933c016fc613584" - }, - { - "block_id": "000f45cd1f8623f4be3a59881a9e478a7d442c64", - "extensions": [], - "previous": "000f45cc22d7c6f6366f1e93eee28d51abc67a9b", - "signing_key": "STM6rkDXv6nR1wUcqeeEJb7SLu6GPXirvHCm9v5Hr6drXE4Z2Vjgy", - "timestamp": "2016-04-29T04:57:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "penni", - "witness_signature": "203420c2e84cd470f258697be96d5d4726211fbe79b979b40afa51bad2f11d35d23f47e98a85507b33d7799310d5f5906ea14fa126b7eda5b0f824924f19061589" - }, - { - "block_id": "000f45cee9baacc5138fecba35f1761aae097789", - "extensions": [], - "previous": "000f45cd1f8623f4be3a59881a9e478a7d442c64", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:57:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "1f7fbd1ff143f30fe8f0e55f6870541333a3d424762325c30cf7c9b1096826d30a212cc732e0a05a5b0342ee3d15ef219b51c1c3729504756235e8258e9cf30f46" - }, - { - "block_id": "000f45cfeaed4461703c4a9b0cca831f33d0b57a", - "extensions": [], - "previous": "000f45cee9baacc5138fecba35f1761aae097789", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:57:45", - "transaction_ids": [ - "e9e474fa68a73e1749f37ede52204f5fdad47cb3" - ], - "transaction_merkle_root": "aba2f9a2c9a2bc6f3801a1801de0e9d4639ffc4d", - "transactions": [ - { - "expiration": "2016-04-29T05:57:42", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f45cee9baacc5138fecba35f1761aae097789", - "nonce": "12956217215122769744", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "bacf8a1ec1178c6cbe9e418375159ef2e73c0083c6b023703176e3ce49594a30", - "signature": "1fea0362334f11f7b6172243a25d68e9047b24cad50a6acb9f3b198592b23af51110919e0c86cfd8e00951c3974e5c73a75ee992f031c1f99d83f8ead46783062c", - "work": "00000006e88c627182ecf66ab460e64eec270697395dddd2a0ad3bfb656e2133", - "worker": "STM7q6ZRH8giNQSMD9EM1z6cUbSgcAfE1HmRrwF1T78zsvpAAN2hv" - }, - "worker_account": "copychicken1" - } - } - ], - "ref_block_num": 17870, - "ref_block_prefix": 3316431593, - "signatures": [] - } - ], - "witness": "complexring", - "witness_signature": "1f60812d3071853e1763cc619725aa1d0d3a219054fdde0b311d9d6206e3207a3c2883022fc26d30747c745dec381a3156a5685c6f8aaaaafa2af2b1d4c20557fe" - }, - { - "block_id": "000f45d0a28c065554779c7a6007c1a94c271541", - "extensions": [], - "previous": "000f45cfeaed4461703c4a9b0cca831f33d0b57a", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:57:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "20414871ae66a275586c80ec9760b1b2ae04bba104c594fa2b6bc0357c2535bc17755baa56123d93532c8b4dad7203a06a49d0124eb42a076db8591500e9fc4d71" - }, - { - "block_id": "000f45d17eebafd7e8ca8918e316bf7455cb62e0", - "extensions": [], - "previous": "000f45d0a28c065554779c7a6007c1a94c271541", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:57:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "205c3c32b97e2f803091ea4d54e90830f60a500c09f25b4a4ce1f0c6b77f9cc5732266caf7981352ab65e554c7d057f783db5ca3d9282dee4af522b801982f2281" - }, - { - "block_id": "000f45d280c174df5e00c0ad2fd7e83324ce896f", - "extensions": [], - "previous": "000f45d17eebafd7e8ca8918e316bf7455cb62e0", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:57:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "207ccb07dff1c9014d389a9a08ba7a5f95bf6bbda38dcc200604abd2d44793ee7d2ef0ea54a3f724a75a45e7643acacb3b7055c80297a68f268bd5d8caae2955c1" - }, - { - "block_id": "000f45d318bff7bc4aee3f74d941ad51d9179efa", - "extensions": [], - "previous": "000f45d280c174df5e00c0ad2fd7e83324ce896f", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:57:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "204299a4c063ba7365da6d1b45f4b938d846766d83b5d3a26afbda114c9ea4671211e2e476e51eee499dbad09090f47094604cb52a65079829c5125b7fe91e48a4" - }, - { - "block_id": "000f45d468b402e29e1a39fec101ee39f7e774dc", - "extensions": [], - "previous": "000f45d318bff7bc4aee3f74d941ad51d9179efa", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:58:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "1f3a96ec10a81dd7e61be44ee6811dd79b031c64c43a6993088f9a9e3f3bd94f9c25446d245fc818728b8083b0047c1d8da84a3505041bbb3dc0d9f9a3cf0b5d3c" - }, - { - "block_id": "000f45d56008f2d928ca31e970ee19c04413552b", - "extensions": [], - "previous": "000f45d468b402e29e1a39fec101ee39f7e774dc", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:58:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "1f1b027ba789f1e236c6a036487596b9a1529d1ae818682726d6903b6e294ed54c22cd70b6e386178ff15fd1c590c4010d1efeb85064115cd4a99d9394e203e683" - }, - { - "block_id": "000f45d6c03dfe02d7cc407ed81f3d04226574cd", - "extensions": [], - "previous": "000f45d56008f2d928ca31e970ee19c04413552b", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:58:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "201f0351ffac3537f4affeeacc79464b10de92dc94f25688bd88a73d2981959f793c54caddf45b599d33283d4719d51210f2e3495866c8409b738f032d19b0f2cd" - }, - { - "block_id": "000f45d7c73d140266368c6a36ec3b613f85ff5a", - "extensions": [], - "previous": "000f45d6c03dfe02d7cc407ed81f3d04226574cd", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:58:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "207cafbae3cdf5dc568e06ad505e54f22cb1133059e65ded510e1beb706dd1befc7950110dd5955ec55ca5e85a159ea6d63621cd104d7d58fadb4e780f819be332" - }, - { - "block_id": "000f45d85cee98a5c3135f070ab69d0be2fec4f2", - "extensions": [], - "previous": "000f45d7c73d140266368c6a36ec3b613f85ff5a", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:58:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f13c24fa76bc0da8f992d718cadade72803e6158ff717e356a79315d94aec98484f330466142af0697a4c63858136178d7279fed2876cc7a2df29a6f01b39ef56" - }, - { - "block_id": "000f45d99c113ec00249ca54c3c4d9241f2d3222", - "extensions": [], - "previous": "000f45d85cee98a5c3135f070ab69d0be2fec4f2", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:58:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "205c031168bdb305a9075f1dcb1c29b3a280ef9706d90e87bcf715696ebc0eb19573bb891fac770b302f61db32663c84464533ce946551c915602f28c493a47224" - }, - { - "block_id": "000f45da94ddf9c4040c76750705376c4da89901", - "extensions": [], - "previous": "000f45d99c113ec00249ca54c3c4d9241f2d3222", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:58:18", - "transaction_ids": [ - "7c02171cf3509e0c5f834864fe0c3288bd67b27d" - ], - "transaction_merkle_root": "633924e6658e0e4615d1cc34f2681049e9c6c8dc", - "transactions": [ - { - "expiration": "2016-04-29T04:58:30", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "steemit-news", - "permlink": "ever-wondered-what-a-block-on-the-bitcoin-blockchain-looked-like", - "voter": "steemoholic", - "weight": 10000 - } - } - ], - "ref_block_num": 17881, - "ref_block_prefix": 3225293212, - "signatures": [ - "202c7e05f71bc30bf40d6ac09859e32112c45fb010fb4af71f184bf8f7f32cac2e61a5f040fe8b5b96fbb39cfa36cd43a1243ade280d9af97bd6bfd4688ce04697" - ] - } - ], - "witness": "clayop", - "witness_signature": "1f4d1e1f21084ec0fb77132a3efabe857aac3e951a7d51f06d54615ba57906a16c041b2880ee1bbe9171bc07f600bbdced6cadb56afca0d7962a7683f8aefa9fcb" - }, - { - "block_id": "000f45db61644be4eec758d1375ca6d7465db96e", - "extensions": [], - "previous": "000f45da94ddf9c4040c76750705376c4da89901", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:58:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "1f406cf1e4f7ecb8f015f241267a4fe4788d8a1c911a7d037c2cd1f5d2b7284eab07ed589c452a6228ad3de7ae017b69caea29f3a9c722e0e446f9a37b873cb6f7" - }, - { - "block_id": "000f45dcc5854e510807d32a9b23f6c7cc39ace4", - "extensions": [], - "previous": "000f45db61644be4eec758d1375ca6d7465db96e", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:58:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f06f2b726519e3216b5fc20b7e135d867bf812c0901d102a359b17725de45c8000de2a867a32da4f8e8bc157d0ead66e48a7ee87ff2d0771a4b05701e2f7d4c33" - }, - { - "block_id": "000f45dddd8cbe8d4c20fd37cfcd3d12a4dcec45", - "extensions": [], - "previous": "000f45dcc5854e510807d32a9b23f6c7cc39ace4", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:58:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "201b14d87a84f23b72a00b639a522b9e4de92f22e6da5b52259f83572205f9cc102024dcb4fc1159892a3ed96ae5b30c707de452effe918fc65cade2a8cc66596b" - }, - { - "block_id": "000f45ded8d529329685076ff462812d87625c3f", - "extensions": [], - "previous": "000f45dddd8cbe8d4c20fd37cfcd3d12a4dcec45", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:58:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f7fd1e130e99cb71cd4101e0b8bec8fc19c983285c1d579ed24a817e3a4816c5c0f880e9f1638d622de4c4b52b6a3569a9eefd4ed965cce7d0722b23551ec6c91" - }, - { - "block_id": "000f45df9814e3b2c36e537d279e7008da2963a1", - "extensions": [], - "previous": "000f45ded8d529329685076ff462812d87625c3f", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T04:58:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "202d5c1d67b4b67fc4af7471af91b0b98efa5a132014585f2746254f9cc50e55e848ff40f551f583a93426dcc137f5146061462c557d4bf410519bed679c1a16e8" - }, - { - "block_id": "000f45e09a82a9bc8def357b378083e6a91113b2", - "extensions": [], - "previous": "000f45df9814e3b2c36e537d279e7008da2963a1", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:58:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "2060ba47e79dd2a919991246c1737a0ab98348b9fe573ef810daeb67ac24a630ed6b5898654a189b14a40a856463e53571046c25b9b4af2300285895690d55f6f9" - }, - { - "block_id": "000f45e15346bceb384cf94aab1d859bcbf67910", - "extensions": [], - "previous": "000f45e09a82a9bc8def357b378083e6a91113b2", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T04:58:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "1f1b85a35badb6dff9b00ded1d95acaecf151a00370e4ef08fda9acc6c499883c1094bd116283d221b2655ed5d37a9b1fd13d851cd2104afb3f15bf9868376c8c9" - }, - { - "block_id": "000f45e28a479a956a31ba7808dece998258d487", - "extensions": [], - "previous": "000f45e15346bceb384cf94aab1d859bcbf67910", - "signing_key": "STM6Eac3W57DXnNEbc5w31NsjUiKTqm5WtQwWaqVjH6uRxEiJmFuT", - "timestamp": "2016-04-29T04:58:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "diaphanous", - "witness_signature": "1f625286e36e98f6bd3d9d29ebed4eb0a56d762ae0f73f7b8136a12fc04e347fca12f5248b1ee1d466e5d36389ae4c8adf6b715a8db0a5930e214aff798087ebf5" - }, - { - "block_id": "000f45e3c03d261a0a0253bccf0601d3b84de921", - "extensions": [], - "previous": "000f45e28a479a956a31ba7808dece998258d487", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T04:58:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "201ec406fe7dd61c8766a933b2fad62ebd2337b3167822f0338ce7eb10c93fe48601cb0a12ff9d6f028870f3bd91922851fba9e49f05e5ac13813aeea055ed9fc1" - }, - { - "block_id": "000f45e4f98cfb0a4878a5706f5663fad1873f6e", - "extensions": [], - "previous": "000f45e3c03d261a0a0253bccf0601d3b84de921", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:58:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "1f77f8c69c81d58758b28a1951fc68fde882d7692e75524a2b4809e7a7c6e240731f8b79416dd3499ca78158fc24fccb1a40e880df8f90e190b71d81fdb1e78932" - }, - { - "block_id": "000f45e5d6340ffad6d490fe005ce8f783bc3980", - "extensions": [], - "previous": "000f45e4f98cfb0a4878a5706f5663fad1873f6e", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:58:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f5c456441f8f4a59bcc0018776914e76fab85a2c43c9813c6c8b868a266b4477e74b60b52b0edab17817e7714d477cee3b05105993d159c4d0b99224b43760568" - }, - { - "block_id": "000f45e677d5f2ba58c30bb1ddda1e4f684e741c", - "extensions": [], - "previous": "000f45e5d6340ffad6d490fe005ce8f783bc3980", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T04:58:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "2065c9bc8240363aba2d9134cba2c08321a64f5f52b0816981df0ff6afc7538e9e7930717ad2ecb5fedb044edeba0617bc6fcdd5b75c055526dd5b1067a17b53bf" - }, - { - "block_id": "000f45e7aace856d76b42e4574c778136aebcf6c", - "extensions": [], - "previous": "000f45e677d5f2ba58c30bb1ddda1e4f684e741c", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:58:57", - "transaction_ids": [ - "a2a0e27112bc99980ad485b6a1b1aef1461dd433" - ], - "transaction_merkle_root": "3d6f15116e453b01823f53de2a405de5fd511f45", - "transactions": [ - { - "expiration": "2016-04-29T05:58:54", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f45e677d5f2ba58c30bb1ddda1e4f684e741c", - "nonce": "9533764316780074896", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "7dbf4fc1090b87c289c5be70e98bacd12c5a26f1abe3e7081b3b0a45b08f08fe", - "signature": "2012e6368d112c5275c2ca1f14d4c6e064e5a8606678b48575d3826de78ad954424fce81455d536b41a63f04a8edb7191b26fe9150a9f4e243735b91382d2343f1", - "work": "0000001495728ce73c9b3b92f049b6fab4e6e0c1bf284bbb457eb0789ef721d5", - "worker": "STM7YP2em2Cwug3Wnq6QjuSHkfEiXYh7PapeFDVWkGXR8Wmi6kKVc" - }, - "worker_account": "alphabet" - } - } - ], - "ref_block_num": 17894, - "ref_block_prefix": 3136476535, - "signatures": [] - } - ], - "witness": "roadscape", - "witness_signature": "1f6799bf1d2d23b4b27ecfbef2df73032f74b1466a5ecb585ebaf21201075a026e117be8cf2197f1fc2296dc5eee4dcea649ee53cb9f0e768cac457f3df67d3c4d" - }, - { - "block_id": "000f45e8580d19b25fcb8019b4eefae4a8af2abd", - "extensions": [], - "previous": "000f45e7aace856d76b42e4574c778136aebcf6c", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:59:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f03e61e822d6a922d5a3527e6360326738db59c4406339edd5576c789665cfed5703bebcf471096b2c10a3a6fae4a3959c76b9ff66939174b407d1d91ab3328fe" - }, - { - "block_id": "000f45e976fe1e05c9774da249567c213e217a18", - "extensions": [], - "previous": "000f45e8580d19b25fcb8019b4eefae4a8af2abd", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:59:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f6ab09c9a0ac2d84430f6977446d42592f88aa8c839220daa2f325fc8ff7f8d3736ebfd6a140052c3c39855da1edea67cae40d8dc7c473e1e874bca8405e359b9" - }, - { - "block_id": "000f45ea866fce417bf3a31c419a372acac1c4dd", - "extensions": [], - "previous": "000f45e976fe1e05c9774da249567c213e217a18", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T04:59:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f065ce66ba9b0482b68e21bad6710ac8e2601e976db6a237042c2c921c7d8fade14a6e2ae1826d4055a5ed4609a5d65a7276d8d6d0d644f2a419870415f24bd57" - }, - { - "block_id": "000f45eb381e6803a3a044ef1d9e126e980ad194", - "extensions": [], - "previous": "000f45ea866fce417bf3a31c419a372acac1c4dd", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:59:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f2dbf032aaa6fc90e6a662978b091999e549b50b48b295f0722e9f5c7344d7b791d51bdfb5468225e6d983884ccd05218ac8c0becca44d87e1145e51e2f9aa7c5" - }, - { - "block_id": "000f45ec968de16b33cb545800da772addc607a4", - "extensions": [], - "previous": "000f45eb381e6803a3a044ef1d9e126e980ad194", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T04:59:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f1fcabb9cc350f38dc141f9f76afdd5265509afd2b12b50cd1b32811cfcc56a7d3f1e88fd8a48687a13c0700ea95fdb406d8d78cab517f23c03d865800d3b2511" - }, - { - "block_id": "000f45edd4c7745846bcd36bbdbaf7239cc6b40f", - "extensions": [], - "previous": "000f45ec968de16b33cb545800da772addc607a4", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T04:59:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f6c2960c5173255c999dc70d1169551ab578f8df6eca49e79e028c22bff7da297585ea7a3b9bb9f44ea09ef71ae33a23348c830b2a45079f4d67d0316b7a63037" - }, - { - "block_id": "000f45ee69b32490277016387132ab41ba76a146", - "extensions": [], - "previous": "000f45edd4c7745846bcd36bbdbaf7239cc6b40f", - "signing_key": "STM8kQi4yD7Ho8gkwPruaGU4oKuu24Lv1qXxxVi51ByaNHnw8Eydy", - "timestamp": "2016-04-29T04:59:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pumpkin", - "witness_signature": "202c53ecabed963f7dc7c29d2c613c1244fe748624f957b23043a93094515d795d1c41392cd481bef24916984ea0feadc67ff6576a2bb81f7957e4e15d7d36f86f" - }, - { - "block_id": "000f45ef20e71f278d0a28871f33e1da20fa24e8", - "extensions": [], - "previous": "000f45ee69b32490277016387132ab41ba76a146", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T04:59:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f1792f8141181f8d2139aebd27c11cbee222780242e66d695ffd3c4390c4fd705440a6ccce015182cdef04505ad6036859a2fb848299ace153ff52fc5c6ec6f01" - }, - { - "block_id": "000f45f0993fea38b2ecf292885420b4aa6841e1", - "extensions": [], - "previous": "000f45ef20e71f278d0a28871f33e1da20fa24e8", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:59:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f7dafaa52be4a3ae16d254e62f87dc4925670dcb69d0573d0c49b10523ca4ddee4f501dfd62eecdd9e79293f6312446900773b2e6e63a0e7bf1e89cf85edd5d6f" - }, - { - "block_id": "000f45f134177765c853137b06bdf6ecb52a99f6", - "extensions": [], - "previous": "000f45f0993fea38b2ecf292885420b4aa6841e1", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T04:59:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f51df31a0c37a9acb955935d10cb0c653ba0e27a9fc4bfe454e5e5e411bd2d468443cb639d507750b9ae3ac1ba623ed7d7ebc2fa51d31f38f4cefa044fd72365a" - }, - { - "block_id": "000f45f2a8978836060ed856a373c70deff38372", - "extensions": [], - "previous": "000f45f134177765c853137b06bdf6ecb52a99f6", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T04:59:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "200574d85a09eede84d0edfaf9bd07a3d19a8fb77597c50db4baf3bcaf9eda244020d58349e468177ac9f7872ca3ece3bef4c95a3d4d8d19d925702fd834f47740" - }, - { - "block_id": "000f45f3684385a532c238ed69e8e6a919f958e5", - "extensions": [], - "previous": "000f45f2a8978836060ed856a373c70deff38372", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T04:59:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f6392e886dd03b3532414ffbca9c698ed4f77a6ed8e516928345720975368beb17e2fec08479cc0dd6a70075475d18ef7ecad76409c6f6c733988b1265b09f690" - }, - { - "block_id": "000f45f4ff78283d48412f9e02da36893bdd5903", - "extensions": [], - "previous": "000f45f3684385a532c238ed69e8e6a919f958e5", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T04:59:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "203d9d75319323919090503fc128514c2c4fd4f8a9774cfb4068caba7ca6700324209dd87c236ba53f2aa72fc2c3bb04dae38ff813c17023fb9e584d7467703ca8" - }, - { - "block_id": "000f45f5d6bc066c98420002dd04e862149227bb", - "extensions": [], - "previous": "000f45f4ff78283d48412f9e02da36893bdd5903", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T04:59:39", - "transaction_ids": [ - "dda7c0382efb5a5d5878dae0e59649c7f003d5a2", - "2bc526140694694c483b71b69522e3521beb0027" - ], - "transaction_merkle_root": "be62d47ad1f54290661bc7a969970302f505ebd8", - "transactions": [ - { - "expiration": "2016-04-29T05:00:06", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "433", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "clayop" - } - } - ], - "ref_block_num": 17908, - "ref_block_prefix": 1026062591, - "signatures": [ - "1f3746f3c1d89adfecfdae867134d3c2a95f2708bae3b179bfd65d2220cebadfa01ad8a7c0f486f5565f403c370d790583b1f2fae3c0d6bdc9d779a9f398e45f0f" - ] - }, - { - "expiration": "2016-04-29T05:59:36", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f45f4ff78283d48412f9e02da36893bdd5903", - "nonce": "7402656884829328823", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "0eec985d1dd42092e761781ccd85ac5a07ea55d0c986cdab818b26ec38039b8f", - "signature": "1feebb48e0597b7cdfdffaad6c6b7a76ae11bdf6e80602f4b006bcf44eadf454a0313fcf0ae789c3bfe3e314603533c7f2ee1eeb3b660683151efb1a377985c22c", - "work": "00000015e51f1f1535d81eb0f5317ce386f25bcc12bd027e15a5d27b7e209111", - "worker": "STM7D7oC3o7YYLoVVd47qXr3tMaABtGGsvWiraQQt2jtnX8gZAjdB" - }, - "worker_account": "hr804" - } - } - ], - "ref_block_num": 17908, - "ref_block_prefix": 1026062591, - "signatures": [] - } - ], - "witness": "steemychicken1", - "witness_signature": "2021af80ff815c91da0a5b8a939288c66649396791b3519d9cc526e7b50a626b0a371f7e7028eff2578039b62b6721e9159fa668b00659f7ab2cbd6c75c7167fda" - }, - { - "block_id": "000f45f6c87bca70dacf90c3a2776c24a21f80d3", - "extensions": [], - "previous": "000f45f5d6bc066c98420002dd04e862149227bb", - "signing_key": "STM7HR2jZiuaVi7xJx8DnkdL1SvTVEDTP1pQZdmaTLGAnt4EqnscW", - "timestamp": "2016-04-29T04:59:42", - "transaction_ids": [ - "558e6328481c5632905973cad8ed044ee6ad0205" - ], - "transaction_merkle_root": "692142277a4ac78a624b988f69476b5e3c8c3b5e", - "transactions": [ - { - "expiration": "2016-04-29T05:59:39", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f45f5d6bc066c98420002dd04e862149227bb", - "nonce": "2752193280839864714", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "e51fc11ff514e1f34500c4cad3a45528c5461fd864dbc98e273ef222af1cddec", - "signature": "1f40fc18d9d7c286832489f7debf1cf61da4c60efc15e3c9d9c5495484f9012985519ce1c535f4eeb62a246a0314f76c1c192bde7995a80bd5dadb964f8f5a6d2d", - "work": "00000008da76c44f9c1740cc888d29dfa560e7cc6727591fe7990a85f3cb87d9", - "worker": "STM7BfmM9dRpxKpckxBthNvaS7JZhkTeAX8QQUtGwbgctcFfwnRrm" - }, - "worker_account": "wumpus" - } - } - ], - "ref_block_num": 17909, - "ref_block_prefix": 1812380886, - "signatures": [] - } - ], - "witness": "hr903", - "witness_signature": "1f4b0d4c5b67587ec2b558bc7a035f7fdfeb4e7a6ad28a85717e663e3f2c3143457a57631d17c47397e0be7a1d3a49c893e2400079735d9a70e1f596b2ddd2616c" - }, - { - "block_id": "000f45f79484589d7234e1da32f16c36b825e2f4", - "extensions": [], - "previous": "000f45f6c87bca70dacf90c3a2776c24a21f80d3", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T04:59:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f2d1810c122b19dc7dd9203dcbcfd11bf29f5438a02817987d9e31074b6532682502272cdb6faaa1bb01b23c0a8880c711c7ce90e091c94b6493d0d8fc4b194eb" - }, - { - "block_id": "000f45f88e8e1b028f72d74812a87b74105a47da", - "extensions": [], - "previous": "000f45f79484589d7234e1da32f16c36b825e2f4", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T04:59:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "200fa801c9a3bd55f297f9aa931134d3fdc66874f963e10c1862ecb06260849c064a4c4caebbfe25505b6b68893ebc430076fe2c9c11c0c14e192595e4ba8f5313" - }, - { - "block_id": "000f45f9416f98830129ce23bd8981e9e49050f7", - "extensions": [], - "previous": "000f45f88e8e1b028f72d74812a87b74105a47da", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T04:59:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "206cbf3ad1ffb45962e4805143ce886d99505b4535385d179afbc0df59e5f727b85a09f7fa6af294df70200d17baf541e808f67d2b291de5aef63732c2c7629ab1" - }, - { - "block_id": "000f45faae4a068edfbe586eb09ec7debdb13714", - "extensions": [], - "previous": "000f45f9416f98830129ce23bd8981e9e49050f7", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T04:59:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "1f2aa6729fa8f817cbd6b2d5e1e0f32210075deb97ff5f9e3de782f687f4350fa02abbfd6f30681ca986184f88911b55d1c8729c7f4fe71ec94ffae4aeaed83edf" - }, - { - "block_id": "000f45fb5b57c6c68e10e9c72457f00c92ade7a6", - "extensions": [], - "previous": "000f45faae4a068edfbe586eb09ec7debdb13714", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T04:59:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "206158410a7c0c0ac960adce992fa8e24eff7bbc2c9eea3de82ecf60335fb9e1d70f3f6a40473a72a9a901f08f91dba2ba4360c261acafb336156d8b9ff3f255c1" - }, - { - "block_id": "000f45fc663c38a4a87d13df3dd2f42b128030e9", - "extensions": [], - "previous": "000f45fb5b57c6c68e10e9c72457f00c92ade7a6", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T05:00:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "20086e3398b4ee56c6a76c28963eb1f4cd4c9a26c13f2d8c87e394eb5d7926ad57779c922145a34f7d0c57cda6dcf3a045832ba5ae57e036e60334729760ca1098" - }, - { - "block_id": "000f45fd5d8ac90b848c5cd503b99bc16ac95d42", - "extensions": [], - "previous": "000f45fc663c38a4a87d13df3dd2f42b128030e9", - "signing_key": "STM6kzHDuHQYGKGUUoPdhmPcHgFFjpq4uycnLK5mUBPPqqn6qpiaN", - "timestamp": "2016-04-29T05:00:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "boatymcboatface", - "witness_signature": "20258d37cb07ccb53cedc04f58db3473fe41491db83d10e0c402449838c220668d0a859955b945bdd6cc7bb877e33047669bc55dd5481911cbec9f47e8e085dd87" - }, - { - "block_id": "000f45fed5b474586fb3eae7203bbeb02f611737", - "extensions": [], - "previous": "000f45fd5d8ac90b848c5cd503b99bc16ac95d42", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T05:00:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "207b99b9d8dbe9cf7e9697f58d1d353a27e257950110e828fdcf1d64faf713ad10713db50058f5aad40f2837533fab63d8e01a14eeaa5a01de867a72e65e802005" - }, - { - "block_id": "000f45ff761dfcea85c889f481b73f9d1fa7d086", - "extensions": [], - "previous": "000f45fed5b474586fb3eae7203bbeb02f611737", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T05:00:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "1f03cb7e8cf9eed51b296445039edc13c7339bd5b7e442fe4daee23d214b7099db5250744d0b417329f0567c64e073dcf78217adf98269dc2fce6ead167d6e97bf" - }, - { - "block_id": "000f46008cf887d026e2d86592657a0229557a4c", - "extensions": [], - "previous": "000f45ff761dfcea85c889f481b73f9d1fa7d086", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T05:00:12", - "transaction_ids": [ - "d578b80696f4508df57fe9cbd4799f026ac0451a" - ], - "transaction_merkle_root": "6f8b8ed4de0f9cf3e98c7aaf850ab499dd45a550", - "transactions": [ - { - "expiration": "2016-04-29T05:00:39", - "extensions": [], - "operations": [ - { - "type": "account_witness_vote_operation", - "value": { - "account": "idol", - "approve": true, - "witness": "idol" - } - } - ], - "ref_block_num": 17919, - "ref_block_prefix": 3942391158, - "signatures": [ - "1f48cd63f358745b29f24061e81607b00ba2db3c635ef7d08a5e900739511cc19460f7d6ca5073b636c5aa1f08c1519f277842301437c18257811dd1e67ac57e46" - ] - } - ], - "witness": "steemed", - "witness_signature": "2041c50c2d1f2084f9948b2191bbf183f2dde036b9cc2bc0e9f5b222b3b74063555c53d3e5d21b1d7c7f985e445d7221317895f90dcc031b915ebfbbb113d6decc" - }, - { - "block_id": "000f4601ca4a666d2bd5fdd623abf1b421cba134", - "extensions": [], - "previous": "000f46008cf887d026e2d86592657a0229557a4c", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T05:00:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "pharesim", - "witness_signature": "202c7b39fc577bddfb9b665484a6cb529502a5bedcdd941ed1f57555bd90af5e672675a546f0c40760a73c94a47e4b4011b6c3c3accc4e692723e31c267bd1879b" - }, - { - "block_id": "000f460208286266ab373f5a4ce91b11db2accc1", - "extensions": [], - "previous": "000f4601ca4a666d2bd5fdd623abf1b421cba134", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T05:00:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "1f0a73df5819852e3b5ed397e93cd6d098d07cf87d7f8ff18c8e1fb2ec51abaf2a521e14db320b127be6e259609bd63a684acdccbb28bb7b7843f87807baa2a7cf" - }, - { - "block_id": "000f4603405fc6a85fc48df62b7242fef4201e93", - "extensions": [], - "previous": "000f460208286266ab373f5a4ce91b11db2accc1", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T05:00:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "20581269dcac087bf7b0dc90fb605072c7985fa07b5a02ac94df1818afb685ff274ecacbb02debebcef4c63a8a5758dc74f57a0b2f9a289ff74b8dd3313a3b1f5f" - }, - { - "block_id": "000f4604365d098b4a141969ccd262d23582a1cc", - "extensions": [], - "previous": "000f4603405fc6a85fc48df62b7242fef4201e93", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T05:00:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f5e6f89a026c776fc0036e3e4f02e47a1aa8d122198d9511fcb6e168145b0eec309dadaca24e8fc516727f9794799d722e810227ea9e0300cee257e8bd355ecce" - }, - { - "block_id": "000f4605f00050fcea8ba3fed8eb1c0e0cee8bdd", - "extensions": [], - "previous": "000f4604365d098b4a141969ccd262d23582a1cc", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T05:00:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f197667838f527f653d0e5b12e60f96d23b3c4d4a4303ac17bf6b2c0896592c1e154bba30554f28a540cbd645a002daad1d749ab011c77f043a6fea637885c89d" - }, - { - "block_id": "000f46064096cf1dc45bafb8a1fbe372ae023e2f", - "extensions": [], - "previous": "000f4605f00050fcea8ba3fed8eb1c0e0cee8bdd", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T05:00:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "202b1734eb6fe9a23ac91e420faccd2b78cec96a2fd5dcadfaec1bfd40bbbde0a13c60cd5a207cb47ceafdd909adad58c251581801dd6b9a7082a35847c7e17778" - }, - { - "block_id": "000f46079f74d57d9cfcaadc05465671171d0612", - "extensions": [], - "previous": "000f46064096cf1dc45bafb8a1fbe372ae023e2f", - "signing_key": "STM6Wf68LVi22QC9eS8LBWykRiSrKKp5RTWXcNqjh3VPNhiT9xFxx", - "timestamp": "2016-04-29T05:00:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemychicken1", - "witness_signature": "202b18bed8d1d3d4f59bdb566319cde0081b7d7cad74c7de91395b55a85503216a6d43442891914de79fa536b2721184c6c463400e496b1b1837ac6fee4e3ce676" - }, - { - "block_id": "000f4608c25874ce495eb00db9dcccb786c5c322", - "extensions": [], - "previous": "000f46079f74d57d9cfcaadc05465671171d0612", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T05:00:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "silversteem", - "witness_signature": "205a25eb887916e64f7ea973516c952cc2082167d9b13408d49053ef896ca383b34ae5b3517eab475377c10dd2197ec2483bb3dd37f5deaa02c96c00de9e08e927" - }, - { - "block_id": "000f4609f293a0503accfa5614cc023adc47b4f1", - "extensions": [], - "previous": "000f4608c25874ce495eb00db9dcccb786c5c322", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T05:00:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "2079fc1f98cdf42be7d542def74eb96e18b2601d5d4f629a0f54967c2a0130da603880869d97e9ecb0787e743d9fe5abe08cb374e9bca02b5c7063634fe522c258" - }, - { - "block_id": "000f460a9742bc9b98fb90058685e79a9b29de30", - "extensions": [], - "previous": "000f4609f293a0503accfa5614cc023adc47b4f1", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T05:00:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "200c05c1c60055a563289308b730eaaae66a895d65fc4d43f54b0c0f54cca31847060e599df6d8c69713a499214a775957ca5fddaeecb7944e44ffcdcca28aec60" - }, - { - "block_id": "000f460b67e9702c23c3dd692e9874aa3423bdd1", - "extensions": [], - "previous": "000f460a9742bc9b98fb90058685e79a9b29de30", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T05:00:45", - "transaction_ids": [ - "737705381f639c98982724452e8d972229bab8ce" - ], - "transaction_merkle_root": "f0c50b9ed58931c32fcb83012d2c6012b09f1f39", - "transactions": [ - { - "expiration": "2016-04-29T05:01:09", - "extensions": [], - "operations": [ - { - "type": "account_witness_vote_operation", - "value": { - "account": "bittrexrichie", - "approve": true, - "witness": "bittrexrichie" - } - } - ], - "ref_block_num": 17929, - "ref_block_prefix": 1352700914, - "signatures": [ - "200f4f749acbbcea945f292ad8891c2d7e172e0bff87249d272f30e105b4b313d35afa1ccc4eb6ed95e53c1d7fe3c3073de180695e5546794ca195b022e2f36fde" - ] - } - ], - "witness": "dele-puppy", - "witness_signature": "1f79c8327ec4851ac34911a095a2e4d236bd8be564be5f64af40b19ef9df9052f75a29a3ad97ce341d270dce26d8717734bfd663ea0783761d47baed3fbc865eab" - }, - { - "block_id": "000f460cb382676dbd12ad24bc473536d161d98f", - "extensions": [], - "previous": "000f460b67e9702c23c3dd692e9874aa3423bdd1", - "signing_key": "STM6jGoakU6V1Nb3ZW1CqsEMd38grp81C9BKRkko3J2WrAXyzava7", - "timestamp": "2016-04-29T05:00:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steempty", - "witness_signature": "1f22a472055d2e901fb0d5a5b7b74c5cca3cda70a3f45a002374d064e0aef028a22bd5989f363224a175412a6f494c8ba61755bb9d2505b2dbe730c0a1f206b365" - }, - { - "block_id": "000f460d4377cd1a21da5019ef3f40bbbf015dc7", - "extensions": [], - "previous": "000f460cb382676dbd12ad24bc473536d161d98f", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T05:00:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "204ba7551b7cc6aff7d82a8d7b40a90eb71aaeef9676daef6addf55daab8eb94e62c0f1becf7a3db6ae8d4b08298c85618504bcce6142a06b88f57065153fe3fbe" - }, - { - "block_id": "000f460e33fa5c043d1ac88f960f3a01399773bd", - "extensions": [], - "previous": "000f460d4377cd1a21da5019ef3f40bbbf015dc7", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T05:00:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "roadscape", - "witness_signature": "20748235ed701a76e80be52e3650109ba8006058baba61520fc03b2eb4eecfab3c37cc1ad44630ff687acaab7a37974cf880f7fcf3832e17882d737f1da6d31c7d" - }, - { - "block_id": "000f460f524986e7adcc2541be1ed0d8984dae4f", - "extensions": [], - "previous": "000f460e33fa5c043d1ac88f960f3a01399773bd", - "signing_key": "STM8jqLo66hWA6G7QK5FcQZQdJW38tq161QZW6kh1XK6RESzPKWXQ", - "timestamp": "2016-04-29T05:00:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "complexring", - "witness_signature": "2052b99484b7389eded16772cb23b566f341a0a6f6d10b886cf4ae61d971a055662fb4a2b2141480796cdf8ec78660e2dec56b4557f70bf57e90c891fe4e4fae29" - }, - { - "block_id": "000f46101f57d4719bc87d335aca2f55c2591f8c", - "extensions": [], - "previous": "000f460f524986e7adcc2541be1ed0d8984dae4f", - "signing_key": "STM7zCGUYKYNsJEDnrwCEU2BwPdNQmFj8NQ1TEhPdVSUgneNMRUEU", - "timestamp": "2016-04-29T05:01:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bue", - "witness_signature": "1f188b7d0229753d701d0e1a17b662aac3230000bd89460003c649e59eefde5e9a2a22068058e4a7b3f382d1fcb9deadedc47e0ceca6878f8116e053de5b39691e" - }, - { - "block_id": "000f461106186d1f3375c5fb773284599f86cce6", - "extensions": [], - "previous": "000f46101f57d4719bc87d335aca2f55c2591f8c", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T05:01:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "2069bd7a6bbb4131ff9db34263c8488f8d6dc1521c09ad68fe76dbf4ede615fea413c2c5787074f9124809581cbac72092d861c4c8de47a49ae9e32c484f5572e5" - }, - { - "block_id": "000f4612768fd7dd5d2c559fee91268837c6e06e", - "extensions": [], - "previous": "000f461106186d1f3375c5fb773284599f86cce6", - "signing_key": "STM7ceQk9K5xR7WvTgiY3NSGQBuiGKQCd3ewZM3QLny2Aa2iPmJiz", - "timestamp": "2016-04-29T05:01:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "disney", - "witness_signature": "204da90fc1a28ad470536b7e154403648b6d19b402aac8344c3540295f0bf2a8381b4f635bed1b2b8de1622c22430aaf011881fea67e90a20c17cd3cf4076d27c4" - }, - { - "block_id": "000f46138d30b253df7a556862d5a7e8918da194", - "extensions": [], - "previous": "000f4612768fd7dd5d2c559fee91268837c6e06e", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T05:01:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f2b98527434b9d0678cf6fde8a4e5ea2044453bc3fd174489151c7d70014bcaab32a1ff49e87aa89ed072e2a26af70090d32f346d81017d53709405b5faf782e0" - }, - { - "block_id": "000f4614ba12b23b94705fa0b6927955ca1e4738", - "extensions": [], - "previous": "000f46138d30b253df7a556862d5a7e8918da194", - "signing_key": "STM8RR6DMdcehSLgvbDcWsCaYowkvKGYv4EVaubtNZyb2gBst7kS2", - "timestamp": "2016-04-29T05:01:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "steemed", - "witness_signature": "20252203c10c339e830adb6a675bc63cc79d061da5c84b8a2471e62aed3fdf3299260162ee0c968b18729b0344c7dc70cfd50bb96c3155051395f8568b4392662e" - }, - { - "block_id": "000f4615654731720887539a2c9b122d0876e9d2", - "extensions": [], - "previous": "000f4614ba12b23b94705fa0b6927955ca1e4738", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T05:01:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "20642051fc8073212537cad5b637e9bc18b68810e51d06b05b165955778f777db4281ef13e71a01b38b1f86ac2b9497ec75c73101812964817d44f47ee956a195d" - }, - { - "block_id": "000f4616f41e31a8bc24f9aa2b1187e766aeecd4", - "extensions": [], - "previous": "000f4615654731720887539a2c9b122d0876e9d2", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T05:01:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "2009257fe2e65a800fa576ffb3010dc85119fcaa9ddb82229671cb25994836c976475ed01ce917db455e1ef189e1df4b269a6598ac8af927520186627b17dfa6ea" - }, - { - "block_id": "000f461788d230f0484f3e15f068f9618260c641", - "extensions": [], - "previous": "000f4616f41e31a8bc24f9aa2b1187e766aeecd4", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T05:01:21", - "transaction_ids": [ - "731d8e99e5421d2f86f11d9a5c493b0c0de3c066" - ], - "transaction_merkle_root": "80e6caca60f441067936ae412cf26bbdd062fdae", - "transactions": [ - { - "expiration": "2016-04-29T05:01:33", - "extensions": [], - "operations": [ - { - "type": "comment_operation", - "value": { - "author": "steemoholic", - "body": "> Why would you ever get out of bed?\n\nTo reload on condoms.", - "json_metadata": "{}", - "parent_author": "thechive", - "parent_permlink": "kvbjx-why-would-you-ever-get-out-of-bed", - "permlink": "re-thechive-kvbjx-why-would-you-ever-get-out-of-bed-20160429t050109605z", - "title": "" - } - } - ], - "ref_block_num": 17942, - "ref_block_prefix": 2821791476, - "signatures": [ - "2033ca939941c65a3c07bf1ece3d3c2b9d8d866d6b30acc9f996959efa2c4f5f10715da4134682f18c92bf2a714392fa1b1065c46aadc5fa4f7d46d23ffaf3e064" - ] - } - ], - "witness": "au1nethyb1", - "witness_signature": "2051474202f91462568f62f4ec6c1b5e2b45aab21e4bb1e1cf534b838adbf4423858a2da69578fd71fab87c724f12dce56e6a15a6ea40c8666ff1e95c4943fcf98" - }, - { - "block_id": "000f4618d7d76a4a8b1f002da82115be78a8925c", - "extensions": [], - "previous": "000f461788d230f0484f3e15f068f9618260c641", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T05:01:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "1f0a1c3fe4218f5a3c26065b7666b18db43d7102343a3490b836aa6cc2cac794494ed6e9d02871bada47770284d3d00ea533bab4e320bbfbd573b878ce51fc1c18" - }, - { - "block_id": "000f46199079d91c5032155e84fe8fe2f365c213", - "extensions": [], - "previous": "000f4618d7d76a4a8b1f002da82115be78a8925c", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T05:01:27", - "transaction_ids": [ - "21b424cecab3045ad67fb4d6c21d47da6ef40ae2" - ], - "transaction_merkle_root": "26851c3c08b4259b0c303c2cc30d5068dbe5961d", - "transactions": [ - { - "expiration": "2016-04-29T05:01:51", - "extensions": [], - "operations": [ - { - "type": "feed_publish_operation", - "value": { - "exchange_rate": { - "base": { - "amount": "434", - "nai": "@@000000013", - "precision": 3 - }, - "quote": { - "amount": "1000", - "nai": "@@000000021", - "precision": 3 - } - }, - "publisher": "boatymcboatface" - } - } - ], - "ref_block_num": 17943, - "ref_block_prefix": 4029731464, - "signatures": [ - "1f021e7a36e56fd8cc8657c3f5c81d55bfd8416f203f9b33bfe0283ab9473a2bc11879863942b588309fe57160a9bd4b0c6f3503ad183bb9d758efd911831ff2ca" - ] - } - ], - "witness": "pharesim", - "witness_signature": "205ef0469c8e74c11dd66a4039a86f5533c199f7dfa46b4590257deef433e320557933ab99e30a66cac69b24663c8d74e42fc3165a187a74c95446e58498e69dbb" - }, - { - "block_id": "000f461ae0bbd41753d55683c60680cc9026011b", - "extensions": [], - "previous": "000f46199079d91c5032155e84fe8fe2f365c213", - "signing_key": "STM8f8i6zPGwkvbpUsRaQdcyKhpyC7TWRKf4S8JSkDsyWTGMnjVKQ", - "timestamp": "2016-04-29T05:01:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "smooth.witness", - "witness_signature": "1f7c3419f41c8fa1f5a5984b80d29de7d7bfa40a643c587e714a8bacc1406866ca161a46cb6d53629adbacf05e58379b713f6d72cfd30409c1c7a1ab596db001eb" - }, - { - "block_id": "000f461b8b0634a9049eb4541ee55c5c45f40e93", - "extensions": [], - "previous": "000f461ae0bbd41753d55683c60680cc9026011b", - "signing_key": "STM7tEwjpPZqx9MAqv715aAqQJqYGa7VAT64VSw66BDLJkGD2nXii", - "timestamp": "2016-04-29T05:01:33", - "transaction_ids": [ - "cd1ba7f8d2b0c50c106e57b0fbf30c396825b23b" - ], - "transaction_merkle_root": "5469984c3dbe152f2fb5cd264501b0266576ffa6", - "transactions": [ - { - "expiration": "2016-04-29T06:01:30", - "extensions": [], - "operations": [ - { - "type": "pow_operation", - "value": { - "block_id": "000f461ae0bbd41753d55683c60680cc9026011b", - "nonce": "3880213603049724233", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "7a928933cfb036cbb17321393e2389834d068aff25e2b4cd293350a754733bcc", - "signature": "20b554eea5992e7672755fa294cf26fd2be781276ccad31bdc376e34db39d67c8e2e76132ea60a1cf2bbd282b77f08a7e41e670233a277400863e323a4aaa55130", - "work": "00000018bf9bef76168e1d71dfcef61b7d05a78bbfa0c97dfe9cff19974d4131", - "worker": "STM6Eac3W57DXnNEbc5w31NsjUiKTqm5WtQwWaqVjH6uRxEiJmFuT" - }, - "worker_account": "diaphanous" - } - } - ], - "ref_block_num": 17946, - "ref_block_prefix": 399817696, - "signatures": [] - } - ], - "witness": "silversteem", - "witness_signature": "201cc09a23bf5ba0c7b9b3d8adedd4d1921f2ec75ae3e2e8404a0ddcccdba051e51fe1db46c132b3ac4f1ac2168a92555803c7d034ced138cb8998d6b4dde93df4" - }, - { - "block_id": "000f461c29cb0a2f2e3fb94a5d5233acb1373053", - "extensions": [], - "previous": "000f461b8b0634a9049eb4541ee55c5c45f40e93", - "signing_key": "STM7xxob4v4X99qiBNpwpd6rTFqh2JpXER9DC4EYqr8rAH8J2QUMR", - "timestamp": "2016-04-29T05:01:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "bhuz", - "witness_signature": "2032efca2bda457473ce1c6a76100108e6b5c6ca0219a3c41b692ec737e7bf6d5a6bf66c612df6bb8dd0a00797ffa794f9cc6269a53cfed5e559a48487a0933175" - }, - { - "block_id": "000f461d49a2d47cac18ca7955874280c575c43c", - "extensions": [], - "previous": "000f461c29cb0a2f2e3fb94a5d5233acb1373053", - "signing_key": "STM6Bsi81GxkyL8QRGsigSTgUP8cneGBrqAbNeMzHrF6TyTHRR4tN", - "timestamp": "2016-04-29T05:01:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "dele-puppy", - "witness_signature": "207ce1b9d4818258830638c56aa3f347a088c58df7b78c806043b0739394a835ad6844bb92c7faf0ef48d445203a7ab779fd57d24c88894219bc63f1a04525658a" - }, - { - "block_id": "000f461e776067c847746bcbe1909f02f54990b0", - "extensions": [], - "previous": "000f461d49a2d47cac18ca7955874280c575c43c", - "signing_key": "STM7xK6BztrGgYRekpBzLaboXEZsXWH14Jigr3pX2CCRf8hU3bXYC", - "timestamp": "2016-04-29T05:01:42", - "transaction_ids": [ - "68fbf47e19316339274b4d908bc207da90c0beec" - ], - "transaction_merkle_root": "5a5381ac42b47b6205096e4d3ad96d6c06b943d6", - "transactions": [ - { - "expiration": "2016-04-29T05:01:54", - "extensions": [], - "operations": [ - { - "type": "withdraw_vesting_operation", - "value": { - "account": "rimantas", - "vesting_shares": { - "amount": "1930906268865", - "nai": "@@000000037", - "precision": 6 - } - } - } - ], - "ref_block_num": 17949, - "ref_block_prefix": 2094309961, - "signatures": [ - "204777ee322f07e89053c8a33ef30497167b1f0d5bf8ecfea450d8643dae1e70a22c0184eb0b411e1180a82c0d48f91e86a892deeacb0484c4d84126d11d303954" - ] - } - ], - "witness": "pharesim", - "witness_signature": "1f746ab9799ed6333de1369fdc38cd569fd85216b764ba31cca6ce3f5921c27bfe3063fe1c2da07557752840925f446ee31419c0d0c100e4b677671caf471dae50" - }, - { - "block_id": "000f461f30392f361a520ce378a7d48b9b6941a1", - "extensions": [], - "previous": "000f461e776067c847746bcbe1909f02f54990b0", - "signing_key": "STM62tPR1TZhdasCBhh9gChKLUsaFD6hFoyLwdvVrxjdcSoprUhu9", - "timestamp": "2016-04-29T05:01:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "nextgencrypto", - "witness_signature": "202c72b10596ee2eb92209e236ee7afd782542ea2b540b76325acd3691519f39d23058c67ab4e8adbdeaa2eb40a521de6f842ac6774b095e9c929e0080ebea0a7f" - }, - { - "block_id": "000f46207ff3d9ee48ac0fbcb7c6a09b9fea4f72", - "extensions": [], - "previous": "000f461f30392f361a520ce378a7d48b9b6941a1", - "signing_key": "STM5P5sgVcp8z3hYXVHsTZnZVLtyp8Hsrjsotan8XLsheVMicxMhS", - "timestamp": "2016-04-29T05:01:48", - "transaction_ids": [ - "68ba24d27a53b05c73ac86d1e0f2f52eb01657cb" - ], - "transaction_merkle_root": "ece1c375c782d3668694f62439fbe3786d1ee36a", - "transactions": [ - { - "expiration": "2016-04-29T05:02:00", - "extensions": [], - "operations": [ - { - "type": "withdraw_vesting_operation", - "value": { - "account": "rimantas", - "vesting_shares": { - "amount": "1930906268865", - "nai": "@@000000037", - "precision": 6 - } - } - } - ], - "ref_block_num": 17951, - "ref_block_prefix": 909064496, - "signatures": [ - "2053f02c49f4ce898f6a0c84417174107b19b2dc4005723d5702ada5042c8ee5d0505e9188f0e2e8c8edb9fa1099254000da5f8ae1c3dec054ff08a6b98a3fcd5d" - ] - } - ], - "witness": "roadscape", - "witness_signature": "1f0e7205f6b3e0e7fd48c2be96e29980e237e139bfd703a661db7da1db7ed7898e63fe35e23cf17fb17f6b1df38b9a6379c396f6912b891dd986400365de06a21d" - }, - { - "block_id": "000f4621bd0233435a8da8ef792d194280713ad0", - "extensions": [], - "previous": "000f46207ff3d9ee48ac0fbcb7c6a09b9fea4f72", - "signing_key": "STM8B9oiMVsspwSAVbCsddDNftN7KvRjex1YsAnrXeojq9LYzygjb", - "timestamp": "2016-04-29T05:01:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "witness.svk", - "witness_signature": "1f384270afe0d1075c6b03c7f85403800aa5c2ab32da7187ab2ac9f6d327cc162f7d8ba97de31197c6c59fbf97c6df659cb626a5b47a266209086f7ef0688f81de" - }, - { - "block_id": "000f462208ecbb5474308c242ea0a9f44c44cb17", - "extensions": [], - "previous": "000f4621bd0233435a8da8ef792d194280713ad0", - "signing_key": "STM5SZ8DRytVTmWTbhVEoVwcj8vS4NcpvuaFi5KoLaRVSTCwqtCdy", - "timestamp": "2016-04-29T05:01:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "clayop", - "witness_signature": "1f74753b16ee75144f1654786a32bfbc016d8a004b4a485f10be67ad991fbf7e4a40f91bd7d9ce08fb16fa03416ea58bd05f23bde9b3d4e6dad8d52c53b95820d2" - }, - { - "block_id": "000f46233a993d6b388882ef1feef9956f191610", - "extensions": [], - "previous": "000f462208ecbb5474308c242ea0a9f44c44cb17", - "signing_key": "STM7UfD2maqjD3xmUCthokLAdDnb1ph2gNEMqe1cKCZKjGgzHy2Ce", - "timestamp": "2016-04-29T05:01:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "xeldal", - "witness_signature": "1f7878bfda9544bbb65406c8f62fb2e22ca54228d282bf44f476d3bb32ddf3a04b623c2fe52f7c3100ec4bab3db8a9ab5c7b77011b3f1fbfdf9b5257663a2cca44" - }, - { - "block_id": "000f4624f1f992d7e91fbd6f330f53203c5d2812", - "extensions": [], - "previous": "000f46233a993d6b388882ef1feef9956f191610", - "signing_key": "STM5zo37Am3hyCgGWjMVNbacRohRRrQvjodfZWnDYL3rPaGCVjKFU", - "timestamp": "2016-04-29T05:02:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "datasecuritynode", - "witness_signature": "204dfd7fb56d53b2ff57c7b8cf926697fd5e983047c59a50a84dcd94791230f38f4421d25de5bff74656d669614b0c0cb56fe28f376f336ce6a1a8bde4c79a797c" - }, - { - "block_id": "000f4625f24907ea0f0b63e8a6dd8240efdb62ea", - "extensions": [], - "previous": "000f4624f1f992d7e91fbd6f330f53203c5d2812", - "signing_key": "STM5UhCTuUwNq2FAWqu7BkTk68BFDSjg2nCgyAj2A1bcWW7rZ7E6W", - "timestamp": "2016-04-29T05:02:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "au1nethyb1", - "witness_signature": "201cbad652c485e85b957a2528c0aa4b927c1d7f637c6ea83b93249a8ff3240b0a1598268dfa139d879db1d15f18f4aca8621b7fe0f8baba2eea80422bbe5cc31e" - }, - { - "block_id": "000f462682f4e929a637ad4790178c6c43b535bc", - "extensions": [], - "previous": "000f4625f24907ea0f0b63e8a6dd8240efdb62ea", - "signing_key": "STM5VNk9doxq55YEuyFw6qpNQt7q8neBWHhrau52fjV8N3TjNNUMP", - "timestamp": "2016-04-29T05:02:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "arhag", - "witness_signature": "1f5b3fb2e133c9001af679f0a6828be1f7b10fb447e1dacfd833a5fa42460d46a06541b719ec995d59e70ac1f504af941a5301db07f6b0f9b4dcfd23c59003efe8" - }, - { - "block_id": "000f4627983d86efb4ae04d1d677f38db1b1c3b7", - "extensions": [], - "previous": "000f462682f4e929a637ad4790178c6c43b535bc", - "signing_key": "STM6K3FYABHcc6suPLU2EMAJx9uD5M9p6k7StAcKQF1W41DhubGhe", - "timestamp": "2016-04-29T05:02:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "kushed", - "witness_signature": "1f46df9c254231aa43d724e66cb444f6a402077e5519f02bbde251a2150ee688e4032a2d13e91bc5c2c84921d34914784043c0518d144f7127867f3c58e59eb7e9" - } - ] -} diff --git a/hived/tavern/block_api_patterns/get_block_range/milion.tavern.yaml b/hived/tavern/block_api_patterns/get_block_range/milion.tavern.yaml deleted file mode 100644 index 454164f89f3feaa5770b59ce87a4cae0f0279aca..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_range/milion.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived block_api.get_block_range milion - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block_range milion - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "block_api.get_block_range" - params: {"starting_block_num": 1000000, "count": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "milion" - directory: "block_api_patterns/get_block_range" diff --git a/hived/tavern/block_api_patterns/get_block_range/non_existing.pat.json b/hived/tavern/block_api_patterns/get_block_range/non_existing.pat.json deleted file mode 100644 index f2ea7c6b71fbcf836fc6de12221930b7778504b0..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_range/non_existing.pat.json +++ /dev/null @@ -1,3 +0,0 @@ -{ - "blocks": [] -} diff --git a/hived/tavern/block_api_patterns/get_block_range/non_existing.tavern.yaml b/hived/tavern/block_api_patterns/get_block_range/non_existing.tavern.yaml deleted file mode 100644 index e81c762b8043b1bab468be52ee62e89e94e0aacb..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_range/non_existing.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived block_api.get_block_range non existing - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block_range non existing - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "block_api.get_block_range" - params: {"starting_block_num": 1000000000, "count": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "non_existing" - directory: "block_api_patterns/get_block_range" diff --git a/hived/tavern/block_api_patterns/get_block_range/one.pat.json b/hived/tavern/block_api_patterns/get_block_range/one.pat.json deleted file mode 100644 index a0df2d84a0db2cf80bb5dda1a02bb228000e01ee..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_range/one.pat.json +++ /dev/null @@ -1,12004 +0,0 @@ -{ - "blocks": [ - { - "block_id": "0000000109833ce528d5bbfb3f6225b39ee10086", - "extensions": [], - "previous": "0000000000000000000000000000000000000000", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204f8ad56a8f5cf722a02b035a61b500aa59b9519b2c33c77a80c0a714680a5a5a7a340d909d19996613c5e4ae92146b9add8a7a663eef37d837ef881477313043" - }, - { - "block_id": "00000002ed04e3c3def0238f693931ee7eebbdf1", - "extensions": [], - "previous": "0000000109833ce528d5bbfb3f6225b39ee10086", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3e85ab301a600f391f11e859240f090a9404f8ebf0bf98df58eb17f455156e2d16e1dcfc621acb3a7acbedc86b6d2560fdd87ce5709e80fa333a2bbb92966df3" - }, - { - "block_id": "000000035b094a812646289c622dba0ba67d1ffe", - "extensions": [], - "previous": "00000002ed04e3c3def0238f693931ee7eebbdf1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205ad1d3f0d42abcfdacb179de1acecf873be432cc546dde6b35184d261868b47b17dc1717b78a1572843fdd71a654e057db03f2df5d846b71606ec80455a199a6" - }, - { - "block_id": "00000004f9de0cfeb08c9d7d9d1fe536d902dc4a", - "extensions": [], - "previous": "000000035b094a812646289c622dba0ba67d1ffe", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202c7e5cada5104170365a83734a229eac0e427af5ed03fe2268e79bb9b05903d55cb96547987b57cd1ba5ed1a5ae1a9372f0ee6becfd871c2fcc26dc8b057149e" - }, - { - "block_id": "00000005014b5562a1133070d8bee536de615329", - "extensions": [], - "previous": "00000004f9de0cfeb08c9d7d9d1fe536d902dc4a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f508f1124db7f1442946b5e3b3a5f822812e54e18dffcda83385a9664b825d27214f0cdd0a0a7e7aeb6467f428fbc291c6f64b60da29e8ad182c20daf71b68b8b" - }, - { - "block_id": "00000006e323e35687e160b8aec86f1e56d4c902", - "extensions": [], - "previous": "00000005014b5562a1133070d8bee536de615329", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6bcfe700cc88f5c91fbc82fdd46623fed31c95071dbfedafa9faaad76ac788527658fb11ae57a602feac3d8a5b8d2ec4c47ef361b9f64d5b9db267642fc78bc3" - }, - { - "block_id": "000000079ff02a2dea6c4d9a27f752233d4a66b4", - "extensions": [], - "previous": "00000006e323e35687e160b8aec86f1e56d4c902", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5202b4570f1b0d8b197a5f5729389e762ca7e6b74d179d54c51cf4f79694eb130c2cc39d31fa29e2d54dc9aa9fab83fedba981d415e0b341f0040183e2d1997c" - }, - { - "block_id": "000000084f957cc170a27c8330293a3343f82c23", - "extensions": [], - "previous": "000000079ff02a2dea6c4d9a27f752233d4a66b4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2050e555bd40af001737ccebc03d4b6e104eaa9f46f1acac03f9d4dd1b7af3cf1c45c6e232364fda7f6c72ff2942d0a35d148ee4b6ba52332c11c3b528cd01d8c3" - }, - { - "block_id": "00000009f35198cfd8a866868538bed3482d61a4", - "extensions": [], - "previous": "000000084f957cc170a27c8330293a3343f82c23", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2044cd87f6f0a98b37c520b61349de4b36ab82aa8cc799c7ce0f14635ae2a266b02412af616deecba6cda06bc1f3823b2abd252cfe592643920e67ccdc73aef6f9" - }, - { - "block_id": "0000000aae44a2f4d57170dab16fb1619f9e1d0e", - "extensions": [], - "previous": "00000009f35198cfd8a866868538bed3482d61a4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6ac53a8bb6ca885e988baafb1363b98e8807f62e6256462269b7288c568010096402d9bd2d8a69549568477f79570e8a41474daee2b7d29c623a0b5649081417" - }, - { - "block_id": "0000000bdf319c4c568d696ab4e58b935a20d343", - "extensions": [], - "previous": "0000000aae44a2f4d57170dab16fb1619f9e1d0e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4deed32395e69b61df58aeee6fc0aa0a7025cbe78c8c45b8b0d59fa154fbae585831579727b315292fa81aba7b624f463894eaa67bb2cba105516abe3a9a2686" - }, - { - "block_id": "0000000ca60eb6a46126502eaf50eaed19d3a6ed", - "extensions": [], - "previous": "0000000bdf319c4c568d696ab4e58b935a20d343", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6a4896277d9b15d7f027ba6846b320c75973c63a73901d5ca8217f8f851af2ec4024f1f14a2f3330f5bd7e1a1f0b1b49bb1adf4785b6a129fadb77443c51dc37" - }, - { - "block_id": "0000000dee90bb7feb94b7c15a6dcd84e79e8706", - "extensions": [], - "previous": "0000000ca60eb6a46126502eaf50eaed19d3a6ed", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1505a740f3c5fa2559a3213aab9b60e26b929e5fc6b5c21ea052f64d33a7f6026899051fddd52d57cad1a1806a55f1b71a4c1783a49dd9208e00b3b9fe1ba653" - }, - { - "block_id": "0000000ecbc2984c4505a1957e55de29facbc7f9", - "extensions": [], - "previous": "0000000dee90bb7feb94b7c15a6dcd84e79e8706", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2005cfb2f3291102daa5d73bc524e1084b1743a2851f762d0c417db2ec59e53400715ec2de64953e8cc3487b963465adbbe388a367207bc36d6c4139973fb0a558" - }, - { - "block_id": "0000000feabd7814909670c6c166c2c535b1be55", - "extensions": [], - "previous": "0000000ecbc2984c4505a1957e55de29facbc7f9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204fc34697dba2f7082ee6af736a12dbbcc22d9adcea54d4c038407102c73fe2997a08204d548bb9c1bd43bbdfd3c377dab1b328a0223197f7911154a3dfb10a54" - }, - { - "block_id": "000000105ae15ac3352ef72102bb6d8685ada410", - "extensions": [], - "previous": "0000000feabd7814909670c6c166c2c535b1be55", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f420e04824a7fda51354f712a899afe1ee5f1f8519904b252f2dfd9ae755a10880cebeb9decaf1fb3fd7401b7366805a11a1ebd0ef5aa39435f0e58489bdc1afe" - }, - { - "block_id": "00000011cb45000fd0731cb5031e8cf91b894475", - "extensions": [], - "previous": "000000105ae15ac3352ef72102bb6d8685ada410", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204caa902b1992aaf152e8f6d4df8e6dc05f11cafd6ae6dae2fbaac4d747f4e32f38b11d10f7494a70c32dd530003ce98f463f7b71ae540319d54f6659454389be" - }, - { - "block_id": "00000012ae4e30d0f532ad03430e939306a24caf", - "extensions": [], - "previous": "00000011cb45000fd0731cb5031e8cf91b894475", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0166390c118aa17414acce47f076acad1f3693d290b04e4b17f6edf78558f1134a7518a1fa4c3964660943363693e7b63f061dbcb60b24927f22f5c0c6e7cadc" - }, - { - "block_id": "000000138828e4c4633cc0f1c9f5eb56b2988c54", - "extensions": [], - "previous": "00000012ae4e30d0f532ad03430e939306a24caf", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201b7fc9347546a6631385267c45bb836aa0fe80f33a6fd53fdf773c6dfd98c9ec4754297afd701cae2779ec735f996594da5588c9d188490f237ddba6133bcfbe" - }, - { - "block_id": "00000014d3da0c77ff9f19abbba6a7e6b7a91240", - "extensions": [], - "previous": "000000138828e4c4633cc0f1c9f5eb56b2988c54", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3c677d45038e42b9d28cb12cb9d058ebd6726d31fc0a0cca0ed71f07e5cef83a6979fdf6c85648790ea111eb65653347abe92e9240435f9c491bf651b2251765" - }, - { - "block_id": "000000155f87b781a20025dd724892e192f0149d", - "extensions": [], - "previous": "00000014d3da0c77ff9f19abbba6a7e6b7a91240", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20090ae1848ef47570507774e450dbf7ae7a25bab25023007d6584b3b35cecb2ec0a3589d10842f866785804e7f7a08e150fa29ed9c3ac8911f86be47f986a361f" - }, - { - "block_id": "00000016d710f59ab6f922bd85492166e9a4d62a", - "extensions": [], - "previous": "000000155f87b781a20025dd724892e192f0149d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203a0c171d09464a3f4bfeaa5874cd09e32af7b2f3d1eabdc909e1542f278e32f45e315ee7d1ce73ae8f02cce5d57f8e51b41acac900fcd6f132b855586e7c5bdd" - }, - { - "block_id": "000000173b3c916f608e99bf6f390af66be880e8", - "extensions": [], - "previous": "00000016d710f59ab6f922bd85492166e9a4d62a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4a32015e0bc3e2a664c7847eb3dc8049445da3bdc78c0f5b9e4cd162851f1ef05104f0b00ab4c50b9cc0579d28941493d6a2f756718d43dfb81a7536dffc5678" - }, - { - "block_id": "0000001896c3e068861af5178cf506788329b492", - "extensions": [], - "previous": "000000173b3c916f608e99bf6f390af66be880e8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201f4984a09b4e38e832db301969d439dd4f9627374a16b7c4912c762e766df51314d5f879bdc4e779a7576f5cafd16d07882953e60e1a6b53b47790ab947857bc" - }, - { - "block_id": "00000019f7ec4cfda1fdef2327615fe8f71f57a4", - "extensions": [], - "previous": "0000001896c3e068861af5178cf506788329b492", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2079746f73a3b1394cd67735786cc98f11a92594c62bc6e56d6e3acbc30f2332cf5ee338b9bdd59cfadf630aac88f94a1ae5ccc5f6cf563cb7a25fdb7a51b0f06a" - }, - { - "block_id": "0000001a47b83bc9cec470524cac9673f9f11110", - "extensions": [], - "previous": "00000019f7ec4cfda1fdef2327615fe8f71f57a4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5859ee86ab22b14c2c327d3d63876cd44b21b8122068b77840f24e6a9dedb1494ea053128e9c8aa0a2f20d18a9c900b4c3ef0f5df406009e2bde2e1822c7ea1b" - }, - { - "block_id": "0000001bde5d2e6a01aa0712aa852024790de528", - "extensions": [], - "previous": "0000001a47b83bc9cec470524cac9673f9f11110", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2049c203820c3d05f06f7a95604fabdd122f1526e332e68dd8057ff3dfe9298adb0db616bb20e1f9ebaca1e2328e468c6402de8c7c9fa2bbdc2a17b5e63307c546" - }, - { - "block_id": "0000001c6a4eae1efcad5905a1303a4f8266b61d", - "extensions": [], - "previous": "0000001bde5d2e6a01aa0712aa852024790de528", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207a2259f3e9e4271fedace00e4873f8046b3dd77d0ae6ba98f5d9d8a096b9ad40427e12ecc99cfcf4bb7559dd21cb62dbe633fe853761c99e52668d81351b8fca" - }, - { - "block_id": "0000001db08e75bd379e402cb2e66a6fe3986371", - "extensions": [], - "previous": "0000001c6a4eae1efcad5905a1303a4f8266b61d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:06:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6a5fcc78e38bacc53d8272b15fc73771101d44de90b21f7b1ca9257d2e4375fc7c67d34096a4a079a2fc843e8287e6caba2ee08d8970ef392d3660c5170b6d5a" - }, - { - "block_id": "0000001e24bd0a68380ff25828a1ca3e91c12c3e", - "extensions": [], - "previous": "0000001db08e75bd379e402cb2e66a6fe3986371", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207da43b4e3749aee64bea82cdfe4e38ab2692b09770694794b21f96df17d2f47c41bb2e3fb3f3da618c5c0772fcb79831bf9dd3245f566892e270f7c98e93729d" - }, - { - "block_id": "0000001f6822f531883cc35cb41f15aa36db59d2", - "extensions": [], - "previous": "0000001e24bd0a68380ff25828a1ca3e91c12c3e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1d975cb9a50886fec9201dd45180681c9a49cfee4af9ded447b94d4a3746c8591cb7b239a49a08710634ccca4afc1bd7978cfffa561fc29d97d4ec2629622dc4" - }, - { - "block_id": "000000207bc6cb67dab285a5cec3c39040731030", - "extensions": [], - "previous": "0000001f6822f531883cc35cb41f15aa36db59d2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201978ce881789eec2e497d51bc516030c324627f2c840c8d870ebb7261ccf9d6743a61281b9ac25c3c9e036d7598cb69ff2d3df3170fed8433a8c8a2e6adb76d2" - }, - { - "block_id": "00000021771d33c2fcb26c5ce0373224db5b5390", - "extensions": [], - "previous": "000000207bc6cb67dab285a5cec3c39040731030", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f60ae9afe11b72394ba6025315ee314df70b6d1bd31d6078e9096c90c042c74af138796aeaea320e7c0df4c1fa6667a4be85fe5746fb6f0c148690b19fd80a8e0" - }, - { - "block_id": "00000022b7ea755d7c0e2a1f14b8c6a13ca4c216", - "extensions": [], - "previous": "00000021771d33c2fcb26c5ce0373224db5b5390", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200599e4fb0be109494b1f1da1d642b9c8d77462298d0dcdc5fce21b088f7e822b1a2446f6e63cc53fc211c54ee203f7782798be232f2ff01d89ce5742532c87de" - }, - { - "block_id": "0000002306d8a911e57648148346fd72acec1508", - "extensions": [], - "previous": "00000022b7ea755d7c0e2a1f14b8c6a13ca4c216", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2f7c5c33ac43847bb46eab694f36dc55c69efba42230e13b63984bac48b133c271f344efc7bca9a42e9eb6d217b81536ff413b42dc230ad53f2b3f0f6ce0d09c" - }, - { - "block_id": "000000248dc750abbabc5ca2dec235d5e994da7f", - "extensions": [], - "previous": "0000002306d8a911e57648148346fd72acec1508", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20544e49837f5167f250c3b9b31839506554ae58dc5c9980e624ce38265db91b2152c8ab42624115f6c040ec4ddadd094738367b93dd367c697758dc0d90536e1a" - }, - { - "block_id": "000000255d5d91b592497a1f2a0f49be58c25429", - "extensions": [], - "previous": "000000248dc750abbabc5ca2dec235d5e994da7f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f64298cce1c6313d0abc3717125d094cc0d5a9b172109865808955c250c822ed30544be14ef91a3fde6ec19fd88630d949c2bc38571a2989ebb8e7dafd2601542" - }, - { - "block_id": "00000026cafd6b749800d3f7fbff84ef2ebe0063", - "extensions": [], - "previous": "000000255d5d91b592497a1f2a0f49be58c25429", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202dda8f9ad4cbf2861d88a2611ddb1d6b72cd44b022088cca89783749a4d1d5d06f6386828d3fccc5e2918ce8d3aeec2d85f198e38a357acc9febeef4c261c473" - }, - { - "block_id": "000000277ec13d304bee7bef0dddf279806fa947", - "extensions": [], - "previous": "00000026cafd6b749800d3f7fbff84ef2ebe0063", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6bf80186cadeac8b288cdccabaa6cc0971931a5d4521329f575c299c3a73a23e1961d9fff8dd420bf9b7b9a77048586843c7880aaae93c22ef5e4689cc808d13" - }, - { - "block_id": "000000282597a87b825d57a2af5709586e3f1905", - "extensions": [], - "previous": "000000277ec13d304bee7bef0dddf279806fa947", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2039290eadef375fa522141a072d58c26f153f7d1f068555ea91fdf20585ac09057ea9def97f4b820603ddf43a5a9dcacec64cd9402a7a54b0c5dee5af5301cd30" - }, - { - "block_id": "00000029684af317654a6e5c544e319c497bf7e4", - "extensions": [], - "previous": "000000282597a87b825d57a2af5709586e3f1905", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f659efffe91c271cb457fb65f915f5616e2bd6963cff476856d7c7c07c5188f7775479446508e7c4f2ac1632d23d9b9ac38174f04096290ff9e491d2cfa74b3c9" - }, - { - "block_id": "0000002aca2af268c86d408ded4d5024e30f65f5", - "extensions": [], - "previous": "00000029684af317654a6e5c544e319c497bf7e4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f66033e8938d37532aa09a88a62ac2b39f6f2f164773b78cb5f49edcdcde216dd486b45b4c396d4ceff1999399147651ae24ecd0558a67879c3e9f8757b7e7925" - }, - { - "block_id": "0000002b0835be80bc366265a77e3aa15bb3d5a1", - "extensions": [], - "previous": "0000002aca2af268c86d408ded4d5024e30f65f5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1e5e062ce8c891ab45db17294fb5c82b5da972141285ad7adea40cb2f94972fe4b619d3018b4eed462277784102b146cce36fa6f06ef12e8d7ef8df0f0627ecc" - }, - { - "block_id": "0000002c077f84609c9b0843c8e97a1747d96e49", - "extensions": [], - "previous": "0000002b0835be80bc366265a77e3aa15bb3d5a1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f28fc66c0adcb9ec0e296b2da74d9b36f447f05023620bfac1b18172f71d6a82334b4b3f2610913c6f11b96f0939b124dc1c07fce6335b55caf5e49cccfcd6966" - }, - { - "block_id": "0000002d3a46d825d23e0a70b7410317ac00ccbb", - "extensions": [], - "previous": "0000002c077f84609c9b0843c8e97a1747d96e49", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6bceeaa36e7837af9fc8f782ddee6a6a633f8e837a7f19017a708df0a6f9f2ee407414b79a3a67e954ccae61ae0f54ad983a5d88fe0cd3aaca394f0a7f2bc96a" - }, - { - "block_id": "0000002ef5834de762e76bc890f9e263a26df41e", - "extensions": [], - "previous": "0000002d3a46d825d23e0a70b7410317ac00ccbb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2017bc5d4db80274703047e66b5f5f4786947d2e22eedf52c03d92b1bd79687b1b1ee5999caf45916ace7064b95a1174a9b790d52e75bd874517d5199e1a904ecf" - }, - { - "block_id": "0000002f683a483b9a194cecd014b9d061e4691b", - "extensions": [], - "previous": "0000002ef5834de762e76bc890f9e263a26df41e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2a632242eef00d5ac4c7d4ee315aea75405c862b9d22f74181c54cb66fa6013329391325d18557de6020c299881a19e92c50a1d0d012fb38a6e85cf77c65281a" - }, - { - "block_id": "000000304084835f85806a33f12fadc9afc6c813", - "extensions": [], - "previous": "0000002f683a483b9a194cecd014b9d061e4691b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5289d4b5622d0dfbc24a535dc9bacb245f382ba8a7ccb7941ac2b61c71fcfc755542b82d2d1602e85e9800842ac9efaf4a0a6ecf7c6eab5afe093f61592040d1" - }, - { - "block_id": "00000031ffb9863574038c8fdfede35ae20eb376", - "extensions": [], - "previous": "000000304084835f85806a33f12fadc9afc6c813", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:07:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3944e1b119d50dd1d5b97d1047aada9046b5f984c30d21de4a5ae55e04b8254449de5f9f374e8f4c1ac98bda9f9cb101f9bca692a8b56d5824cc7035518c6291" - }, - { - "block_id": "0000003263d5d971caeb20cc64d54718e6893954", - "extensions": [], - "previous": "00000031ffb9863574038c8fdfede35ae20eb376", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5ddf6c7f59d98a1678faa6c824a9266a469e5446f50dd32249a249c5b9d630ed2aac22e4a92714a5a977b61f3217961cdc9053fad396bb5a2a5a76675db9a679" - }, - { - "block_id": "000000330802741ae4527b6f4773abac9f8763e4", - "extensions": [], - "previous": "0000003263d5d971caeb20cc64d54718e6893954", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2025cd5735b6eb0a6380204ed01e650343c2c0c53b29de44b83485a381b61ee4572ded2c54bc599f167b29bbe29a9006b13778eaa426f368bd48fa0c3ac041652a" - }, - { - "block_id": "000000349fddf9d2082c2cb8c456044e248b1aa6", - "extensions": [], - "previous": "000000330802741ae4527b6f4773abac9f8763e4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f787e49bc4d9d97cfcfd125dbd30ff697de88315b498344cdc4ca2a1bde6fc7cb00c97b6b99194a4778e6f3055802d5a07992074b6d54b7a0368ff18355f59e3a" - }, - { - "block_id": "00000035dcbf4a38d74f3ff1c14501f4c4fdc17d", - "extensions": [], - "previous": "000000349fddf9d2082c2cb8c456044e248b1aa6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202fa6e81dcde8b1cf6d06df35d5d5c70f7b6573de6ca32d36c7853ccf5c8a32b1699bf6fac602c7287d46e4d79ebc226942d1aa64f3571b02e5dd1bfd50c442d8" - }, - { - "block_id": "000000364e0ac0eb0059688a0a5ddc0400e9029a", - "extensions": [], - "previous": "00000035dcbf4a38d74f3ff1c14501f4c4fdc17d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20305cd91f6d37af5701b6a15e129c11f6ad29cbfff64886e9a9780537adb50bbc1ff110d02ab5e5e56be56ab354eff37ea4b5683292a8895d2715ac0518c920e9" - }, - { - "block_id": "0000003728c920e3b35d3ce7817dfd8bcf1254d1", - "extensions": [], - "previous": "000000364e0ac0eb0059688a0a5ddc0400e9029a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3549720f13d2ee9c248a27848d53c7003aff7dc8dfd6177f5a8f605f040bf25d25eda4cd73be6d3eb68b8ea167aa1784bded0233dcd7ac71b9b4a8c65a892b44" - }, - { - "block_id": "00000038e1a24675564758cc85e45a6ee591e64d", - "extensions": [], - "previous": "0000003728c920e3b35d3ce7817dfd8bcf1254d1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2056660a75795b1a22adfbca14006350b5e0f099efac3213be0cb1f28c536fd4b442051c231b3aedde078fd98d253f3f2b0d39a8c94f9d144b3193998cc7425cf8" - }, - { - "block_id": "0000003995f3e59dea8c5be31d7254893cbbbe84", - "extensions": [], - "previous": "00000038e1a24675564758cc85e45a6ee591e64d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203173077bc67bac2217286e6f6095238080ca3cce2f8e3daa89f74198b8a5c70247b3aa345ed2b8a8129c3b023706e9a8325fba978000b3aac1aaf5cc2b3ffd96" - }, - { - "block_id": "0000003a87c5fc362e17d1f5cf1853da23f03ced", - "extensions": [], - "previous": "0000003995f3e59dea8c5be31d7254893cbbbe84", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20013fcb202d8a6bb5ee3bccd5fd94b9ddc851d8dfcb59760d9c5a54947ff5885e59e8bc25fa923938d7e671e2ee3996ad42c6d5c2d3163596a6c7e2cab011130c" - }, - { - "block_id": "0000003b43b311a45690987ab2cca27b64d08c37", - "extensions": [], - "previous": "0000003a87c5fc362e17d1f5cf1853da23f03ced", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2eea22474cd42c78e16bb69266855a25d88c19a871a4d8b2f1ef59ad42b208077aa0d4ff0b7eaa2de9d80241c75955973651b46b7f4172c8f5d401dc35f90c1b" - }, - { - "block_id": "0000003c68fa6646c7ef94f42aa1c3667bbe13c5", - "extensions": [], - "previous": "0000003b43b311a45690987ab2cca27b64d08c37", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0fa646d0da1900f605a86d3c19de2219d507e3baecb4f05372fb4713058e588a0fcb1d508df2a5233a53582a3a90573c2106f370d680d0bc9a575176cc9470d3" - }, - { - "block_id": "0000003d363422d78ee838b2ea5fc3fcded600e1", - "extensions": [], - "previous": "0000003c68fa6646c7ef94f42aa1c3667bbe13c5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f63cfc42af6028f1606e82ba9d81c8c8758ecfff4f9e433a7307fa664f5ff232f10f5e3464bb2dbdd33f994dda49f3d49173dbca72cb98ea9b4f6cfbe38730719" - }, - { - "block_id": "0000003eb3439ef5864448a93cb2110af9677dac", - "extensions": [], - "previous": "0000003d363422d78ee838b2ea5fc3fcded600e1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1c2f49bbb52b5fa1cb2b69cf7656c54116b0366848cc0ddee78c6f617ca37b5a37f8402a3f7ec7846c2e96a57a683bf16a3439e362dc66ca5e2ae190d92b4360" - }, - { - "block_id": "0000003f42157fdc2139390b56d89e8c0438afd0", - "extensions": [], - "previous": "0000003eb3439ef5864448a93cb2110af9677dac", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202c145c443e8eb503c0de3042da5bbdd588b81a5b626d9c7bc2d5f5c047c51eff565f5da7b71bb4fd2300513513c510b40e9ec0107990f7952087c24273a1d13f" - }, - { - "block_id": "00000040fbcab22a36b376ab2d91965ff2cd04c5", - "extensions": [], - "previous": "0000003f42157fdc2139390b56d89e8c0438afd0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f41bc864a3433d85e823a7c10fbf9202b382d8a6a556eba5d9715cf9f40a0f30e35a22bb8598a4c358b1da32e699611b4fb1db2f61a9d9ca31fbbd1ba2cbf9291" - }, - { - "block_id": "00000041116d46d916798ad3a9d63aa4d422da66", - "extensions": [], - "previous": "00000040fbcab22a36b376ab2d91965ff2cd04c5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f41b34fbfaef9505ac554af6be04bd9f33fbf52f589d0d84db0cc1d0ab4f04720590472d4bd7db243612685a71ceb313da4b9f916439a3e536e622de9ea98a7da" - }, - { - "block_id": "00000042acf0719025f937a1db992a699358043b", - "extensions": [], - "previous": "00000041116d46d916798ad3a9d63aa4d422da66", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7dca312ed73505891d8e6a4b6736c8b455db993f7c8f03a0aaed302de63ac95135663975af020ec457871b0c5106f89b4a2a04b0e51284d0d4e2f505e68c6dc3" - }, - { - "block_id": "0000004321db65105cc75f309005598b086d4d0a", - "extensions": [], - "previous": "00000042acf0719025f937a1db992a699358043b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201a8c86eabb4aef8bc1a1c6d44b7d97df97ffcc8851538f9632393b88c2dbc11052e7ce6c504bd5bc1d4b5a68e9c8900faade3ab015be95a04f8a4b54c8bcad32" - }, - { - "block_id": "000000443482d4dd1732bf99a22e9d585a251793", - "extensions": [], - "previous": "0000004321db65105cc75f309005598b086d4d0a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200ee809ea4425fb5b4a7fe04a7bfbf1712cd30601d49547dd2cfb70362dc4e6cd649d8e759d3932b0fb1f5ae71577d8661cbc551531ffb3350317001f9109d0d8" - }, - { - "block_id": "000000451e77c8ef5d57413ee0a11dc6b670ae71", - "extensions": [], - "previous": "000000443482d4dd1732bf99a22e9d585a251793", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:08:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2036cbc4f73ae23cb0df4e41531a94e8e387be3d5b05edc4d62c7031a92585e96f00f9086eaed0c0c045e7e6ff944873d6cf7c8075c552b6a75c9d5de76cc3e218" - }, - { - "block_id": "000000462f0f6cf029f5057135845b23381a665d", - "extensions": [], - "previous": "000000451e77c8ef5d57413ee0a11dc6b670ae71", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f54d00e588a90c1af190ad563a8e53d7b7906cb0cd930dc049e11bc36c3acfdad2d7823be8ba3b32106334e97b966c730e171b873aaf8f27ad39e35e6ed9b7b05" - }, - { - "block_id": "00000047ec5523e3835f50079609f99da0bc2fb5", - "extensions": [], - "previous": "000000462f0f6cf029f5057135845b23381a665d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f58ea8bcbb2523b32df5963fc19ae2880fc9c347587463975c3078e1eb0aff56c7efc924d400457c18ea658a6e40aa8c4da2633fa87a3a03423e6b81e4d2bfc7c" - }, - { - "block_id": "0000004897e7db06bd9bc3c7639bcd9fd1ac5258", - "extensions": [], - "previous": "00000047ec5523e3835f50079609f99da0bc2fb5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2044bb6942b0b4a836ad35282da93a04fac33343349ac7a514460733ab220f3106336ba646175cfd45fa7274a93dafaf58a1a108e0668b77cee1f734aaf5e03b66" - }, - { - "block_id": "00000049f65c44f1c67c52c7688e5b0f8232702d", - "extensions": [], - "previous": "0000004897e7db06bd9bc3c7639bcd9fd1ac5258", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200c2cb7d4601f799e34b25863afd4734a5bbbd740672b74c297f2c9d78759127368e4a2b38ab352b4f78122b145592ddf7097e17b83760d856a2ab323ebadb695" - }, - { - "block_id": "0000004a4d113fe3bae711e94c8e2b4ed0f34d59", - "extensions": [], - "previous": "00000049f65c44f1c67c52c7688e5b0f8232702d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206d15ef3d9cceabcb2f096f1bd47e4a9ffc6f9f65e474bf0af68437c4683752b60dae41afd64d5f6fc49b5daacad08296133963c634bc8ac0c98a61c7e816c4d3" - }, - { - "block_id": "0000004bc692e20575a27c80115d90b995ffe8d0", - "extensions": [], - "previous": "0000004a4d113fe3bae711e94c8e2b4ed0f34d59", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20679e44a11d4ce15f8d6b84bee4c48a426e5941f94135ad551ddfeb3d6be9c5267a2578e1321a6424ea10a72185cac41a684ea3ddac12944195e5279309a8f9e6" - }, - { - "block_id": "0000004cf9d78e0a325898b98946cc7bbef97b90", - "extensions": [], - "previous": "0000004bc692e20575a27c80115d90b995ffe8d0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206f37b49fb935b08748f1370f0c6880d56ef6f2968e8cce7da0b7d93610114d9d78571753eff8472aa11cb66c5828b2e404878206a39a80b75f67f4b4c0210365" - }, - { - "block_id": "0000004d51fccf31f1a0b2253ca47842676ffc08", - "extensions": [], - "previous": "0000004cf9d78e0a325898b98946cc7bbef97b90", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2053f1055e983e8a2c95d465c31401a302fb4b78a58b8dcc6415b7b05a0aeffc4917dc04011cdfe12f1265e45a178730479add019aef847fe0f23db4b6d9f54996" - }, - { - "block_id": "0000004ec91b76a73599c71f44953fbc574828c6", - "extensions": [], - "previous": "0000004d51fccf31f1a0b2253ca47842676ffc08", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f641d1fe530f3301fc227cb59c7712ee579cbf7e651a67a7bccca14d335456c025eb9a33c44a487e0989d4fd2b7e6ae05fe6e3a0685b9990aa84ffbe2f13bf45d" - }, - { - "block_id": "0000004f99c3f0fecafd668b58f490276c36183c", - "extensions": [], - "previous": "0000004ec91b76a73599c71f44953fbc574828c6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f14f90c2b1dda91f3feb57558490ec1b1e05cb73c475db06722aa7f90fbc454a135539098246eb9faf836907a6b039243f6613b82b96edcb4f13db6ea67336b0b" - }, - { - "block_id": "000000507017c6b2fb0d333c103e6c127824494f", - "extensions": [], - "previous": "0000004f99c3f0fecafd668b58f490276c36183c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20010fcb6802b6ddbe607515f103a82bf8fd61a397457ffe9731deb9e68c9b28d0164c1fd4a91f1a6907aa1f07420381c62c07a78175dd084092d04cc047721df7" - }, - { - "block_id": "00000051f0214e256745c68a51a941afab59838b", - "extensions": [], - "previous": "000000507017c6b2fb0d333c103e6c127824494f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f10a2948aaca8895620970e3d97c7c2440a038a44bab45ec4ae1eace60502375511dcd751844da9f7615f81e6f7da33cca0dc320572e3e3a50914d27ca98c35ee" - }, - { - "block_id": "000000529c66459a03258f607aba50537d432e73", - "extensions": [], - "previous": "00000051f0214e256745c68a51a941afab59838b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202411777bbfe4eeeefe9bdc53ab1a7121b73a264c1d0d576580fae0c11bfa913c7be45f06199dc65bedc93b58ae6cb2174c0a787944d5d43cf17067c5d5851b93" - }, - { - "block_id": "00000053787b029599f320d86ce313b5d816192b", - "extensions": [], - "previous": "000000529c66459a03258f607aba50537d432e73", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204f00603da5ad38c98063ce3128d22d6767a22ae7b9171409348fa2b44d5e9cf300ca45da54971fb97cd2191b258b08ff297e782ea853826ca81dc3523e3246e9" - }, - { - "block_id": "0000005412bb8d65b894c4e8b26fb2f8e53182f0", - "extensions": [], - "previous": "00000053787b029599f320d86ce313b5d816192b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3aa7e599198065d8a59343fd466dc1e2c79c792436f1a833d742994fe349a3181f3969634df25b626cdb7ee8ae4d7243f52443b4efb64a4b7c41467263a0facd" - }, - { - "block_id": "0000005557efc97bf3a40854c6bf7e1b1ae2f1b7", - "extensions": [], - "previous": "0000005412bb8d65b894c4e8b26fb2f8e53182f0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2020d2b6239557ae2f25878b9bd70d528a8abe75b12daaa5dccfdd8278589e9eb775b91ecb4ec2cd7a7dc11fdf066f7a5c9135912830775254c8a334fa6b102ebd" - }, - { - "block_id": "00000056f12344886fda061e2ceb32ce084bbc08", - "extensions": [], - "previous": "0000005557efc97bf3a40854c6bf7e1b1ae2f1b7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f12dbc32cbb9f5ba237a2096692c4a22eef23ad6e7d28b9bb8574d2707626f31a45938ea27148bbd76c8e36443b4f8965719a77bbf60eb7666250a82b29dd1628" - }, - { - "block_id": "00000057f00955e1572f4630616b339bffbe4c4a", - "extensions": [], - "previous": "00000056f12344886fda061e2ceb32ce084bbc08", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5afb7de3928751c37a499b54372f3b2694248a0ffc9bc73e7f8307673bbd8c4b3e7c9d2e2ce2d0dfec9a4e97a3983809bb414f335589849e0d758606c559940f" - }, - { - "block_id": "00000058dfda63e6ea6344c388b618b7307b8e9c", - "extensions": [], - "previous": "00000057f00955e1572f4630616b339bffbe4c4a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2044e124c91a5b1888aa02289d652105892ae0e7490df515042b63e2032ea97dff2308aa3003f06a7a9e659b173dd83df0a9b73eb5be176cc56cbefc1f5946c34b" - }, - { - "block_id": "000000595603e68305d5fa638cfcb00e220df321", - "extensions": [], - "previous": "00000058dfda63e6ea6344c388b618b7307b8e9c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:09:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f19a39fe3e71cbe392edf9672e5c1f3a5f258bce14dbf8d5332c8893b078df3c00167b6086a1195cd25d747465a6f7fe14186b8ea9529c5ad32c00f5c23c41743" - }, - { - "block_id": "0000005a3111b50cbf01da7ba067c25b586c4035", - "extensions": [], - "previous": "000000595603e68305d5fa638cfcb00e220df321", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205a891351fea958a939c2557679a0b2840ddf91fd540c3d9ab9e5311f8df1fafd7248d74190cdb7bce517fb8bcf384f035b433fed21c21b8dd22cf4b2b65a8f48" - }, - { - "block_id": "0000005b49e8194476cafa1fcae6d963dab045a5", - "extensions": [], - "previous": "0000005a3111b50cbf01da7ba067c25b586c4035", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207f115bab70c4211a11258d8f502842b34162745d9ede319b4e073b113d8165914951529c68acfc3f5ada46968c8162e3e16201301b8bc1c410bee462e67789c1" - }, - { - "block_id": "0000005ca7d04f680b4dc52b154b2101292e1b84", - "extensions": [], - "previous": "0000005b49e8194476cafa1fcae6d963dab045a5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201cbfe90fd5bcf05eb295c02f3891d63b580e88d6fa42dd88e707e14e0f6a1a8e4e60507343a258a69f7147526c2df2ca30175b747fe10d72c50c790099ed13a1" - }, - { - "block_id": "0000005df8adf199b9d28365c5bc19e59fb4f8e1", - "extensions": [], - "previous": "0000005ca7d04f680b4dc52b154b2101292e1b84", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200764ed7179166d1d294e6ec83b74a6d1a8e8ac4f73080fffd971b2e1f9e306d613c9689b6f24c013d8534ddda5198006ed78cfb3c5d1d65467392e8befe8777b" - }, - { - "block_id": "0000005ec36fa5413af6bf3dddd717223c119d75", - "extensions": [], - "previous": "0000005df8adf199b9d28365c5bc19e59fb4f8e1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1f800821518da1966fede7f957aeb7c1483a461d21572297225524f92d7f91f40994a93a740f2fd97d0cbf7a6c9dafd9205828716eef9894f4eae1ae5327e25d" - }, - { - "block_id": "0000005f6283c21d3b4a5d918d7c1fb9fa1d02cc", - "extensions": [], - "previous": "0000005ec36fa5413af6bf3dddd717223c119d75", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f71b790d79b0ad1d2e63f073bb9ef5afc797352bb6c4ebbbbf2d0a4c7ddd7bd29512dfa6a5200a6bd32f317c61f358a523ee906588a0aa70f1ca108e05256cfef" - }, - { - "block_id": "000000605e0904bd227f264695c3f2e94606e240", - "extensions": [], - "previous": "0000005f6283c21d3b4a5d918d7c1fb9fa1d02cc", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20556d8f8a2075bc893ae5d10384506ab64dee6c4678664122d8578159d601e1191a31ba3c13730e446b097036fd99905edfd75ea60cd030e35bd9a1504c61b6ff" - }, - { - "block_id": "000000618d94aebee3358f236408bde4374ce8a0", - "extensions": [], - "previous": "000000605e0904bd227f264695c3f2e94606e240", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3047ce25db393d4a84b0c0680d50863385dfa971bd55c163f6237218cf8acbfd561cce28ae8c2521f57223b051337c013df7de3caef369a05db73b66854635aa" - }, - { - "block_id": "0000006248f134582a7eead2ce03724d910701df", - "extensions": [], - "previous": "000000618d94aebee3358f236408bde4374ce8a0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f676beea1b719feb5c4126151a662ec4b15328e560b403686e103380c2de509aa1df22232a442e4426d79bffb249a1044de56a9add426071991c0a9e7a150092b" - }, - { - "block_id": "00000063a3f80fc328722f60d1a63faf5e14f154", - "extensions": [], - "previous": "0000006248f134582a7eead2ce03724d910701df", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2077cac08d683280a2bc25d1fc6bd484ebf84c5d57038df60665a99163676718dc03fd720c70ccc922cfaabf29d012469b566a71845dfc1d61471e7e47f01a4731" - }, - { - "block_id": "00000064419f3740f755558a6b3ee7b1de82f0cb", - "extensions": [], - "previous": "00000063a3f80fc328722f60d1a63faf5e14f154", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0bab4ee04e5445ec52b2aaa05213896eedb6552a99aeddb0ff74d8a925ce4f5a1ed027646f93393946648a4905530829cbd6e3953ebeda9820c3f25ac664fa5f" - }, - { - "block_id": "0000006591dcf70e2564085de9c7795977f6fa2e", - "extensions": [], - "previous": "00000064419f3740f755558a6b3ee7b1de82f0cb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f166d2b51f6cf40c5a8700878507b729513e62d53c02f8eb369bcfbd57f511f9b73b077ae358f45365014e90b5ed9911786ff4faca49016352b8a34d62b070755" - }, - { - "block_id": "00000066ec0f1d672a7c5fed9c57549c7a4a8aa3", - "extensions": [], - "previous": "0000006591dcf70e2564085de9c7795977f6fa2e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f78f20bec294a5eaaf63b09c43470585d3948418d23af3e3554f8d5c2b91c1d91375092053833e869d60deb17823f0b7357d651002c91ecf9766e459114a5dc72" - }, - { - "block_id": "000000677afd22eabf6ab520864b41a1f18d8cf9", - "extensions": [], - "previous": "00000066ec0f1d672a7c5fed9c57549c7a4a8aa3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204e248d2a5b0b73d5b84b441dc32de19090a89bbb69de2d7f17b2ca48f038ce8d79c7073e51d6771e021b09b49807fac9981b736b616ce15da1f68c6e94ff8c61" - }, - { - "block_id": "00000068f39c72d402cdaec959bb6ad7d72fc385", - "extensions": [], - "previous": "000000677afd22eabf6ab520864b41a1f18d8cf9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0426bcfd30d47d2439bdbb9f65fcfcf072029d04223e4c6410c58c9efc593be21752898f6f3bf4f1bce3dd44f3deb59de961ab2a38d3bf8c13c764814fc57033" - }, - { - "block_id": "000000699fbbd587633fed693e786ba0f1649a3d", - "extensions": [], - "previous": "00000068f39c72d402cdaec959bb6ad7d72fc385", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f650a56e8991ad7f8de428ba6957ffa57b0d84c3e28fcdfc4e33085ddf71a4d954fa310a1d5ca374a0429ccee1bb51ec7dac1a70f704db1fc155b824d8c3693be" - }, - { - "block_id": "0000006aceb360778ba1d14c32786ccd6419f2ac", - "extensions": [], - "previous": "000000699fbbd587633fed693e786ba0f1649a3d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f46271840df53715e59fc935fd537ae7f663274825d607ba9d9f5004907b38e3661e2d91ebbcf95350d3f261e2ac1c536014a03fdf2c432def5d29f14bcf2eecf" - }, - { - "block_id": "0000006b3f5e92949385f486289f9e56f0715f86", - "extensions": [], - "previous": "0000006aceb360778ba1d14c32786ccd6419f2ac", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201e6d730d90e5ed2199352851059f4e2efe98233721eb1d02c56bee93bc664bde67b504da80cc98a747bafcf1fe556879f4ff30a42ba0d819f37125872e4686c4" - }, - { - "block_id": "0000006c497d236bee9ef073aadcb894b7bf7995", - "extensions": [], - "previous": "0000006b3f5e92949385f486289f9e56f0715f86", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f100fda9dd54c7e8075e08bde10ba5742aad95c718da009f0020504a243bbbc1240af34f770d57d82b0223636056f10b83ecaee8dd32cf96ea477dc683eb040a6" - }, - { - "block_id": "0000006d78716d94f2c60086355fab6ee35b3f8a", - "extensions": [], - "previous": "0000006c497d236bee9ef073aadcb894b7bf7995", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:10:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2abeba58e2f7008df15a7c720029a3fc6157e139929fd4dafc8479c13a4b072f6c43fc4f080ccabe6bb372c85ae4baf98f6bff2df8403785ae69aacae53cf0a1" - }, - { - "block_id": "0000006e84ac1435555bb8d1be414161207c1d14", - "extensions": [], - "previous": "0000006d78716d94f2c60086355fab6ee35b3f8a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202caffc044d322fff1094e6c82726be79aecef0b91df0f2ac2dbbdcacf5c5a967348d5a4c56f23040aeeda8c06d1dac3b8f5987325837584344d73adece2fd9cb" - }, - { - "block_id": "0000006faaa03ef381c27d43209e80656d591108", - "extensions": [], - "previous": "0000006e84ac1435555bb8d1be414161207c1d14", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f34e15899aaf1d907c08c9889f1136799f22d1a069a99667d9bf5b3c81012f74b3e1d92d879903a0298c405355dc70ff707f9cca22be103e1e383fd5dda7d0d83" - }, - { - "block_id": "0000007055ff0e93407f8e72cb612ffcf4d8bb23", - "extensions": [], - "previous": "0000006faaa03ef381c27d43209e80656d591108", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6f63d23beca1da56467145b704035441b3a02e569303f6da247c7247688a834a2bad040ff7f3a5fdf3620d23aa92ee4db000d1aed6265e57e00fd932c298ed8d" - }, - { - "block_id": "00000071dd1109cb5d7f8359aa70770ad22c5113", - "extensions": [], - "previous": "0000007055ff0e93407f8e72cb612ffcf4d8bb23", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20101f4be4c354d8c7e28c0ed5e8f21a5538764584a5607b152a88517ba7fcf58638eaa41e6bd8c7afb8dcf0bf5d9c652e6d486fae4272eda746713264163ee09b" - }, - { - "block_id": "00000072e83d59bc9562870aac7d0539f410d396", - "extensions": [], - "previous": "00000071dd1109cb5d7f8359aa70770ad22c5113", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f22b9179d3eb1b4a9bfd9ac9869feba356e1d4fe9bb7162a0a4791d14831240e43df2769ebcf0ed46a025db9ee864209a74740c96ac7784aa471a6200a7ce1914" - }, - { - "block_id": "00000073c29f27bc7eefa727a9ef655a7c0fcf16", - "extensions": [], - "previous": "00000072e83d59bc9562870aac7d0539f410d396", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2044839129132c933a3bff5067613b3d3493400d0f06e3d673699551c5896a46d245a225550cfd223362ca99e8dfe6041f2e2ad84b4b2e9e2c1d37243996df8ae2" - }, - { - "block_id": "000000740f3b5f49af534268535592e59a03b43e", - "extensions": [], - "previous": "00000073c29f27bc7eefa727a9ef655a7c0fcf16", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3d4694c95fd9f68d14e7f9f541c49d6fac528eb7919d34d523314849cab4c0682c7b22f7b877add17165c53369dee177377f92b11d6502b7ca3300980cee70cb" - }, - { - "block_id": "0000007506bbd08d96c0aac1bb875b5d6264b406", - "extensions": [], - "previous": "000000740f3b5f49af534268535592e59a03b43e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20236b70ca99b3c82bdad7ca812c92f9cbfebfd89ece2800f34a9fa7f10e9eb34c445ec5df6d61e7d3fa1597a5b23e4479fbf058cf73b0060a64ccbd1709204d95" - }, - { - "block_id": "00000076a0ee0f230cf63ab071a6eeda8ede0c94", - "extensions": [], - "previous": "0000007506bbd08d96c0aac1bb875b5d6264b406", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20693eec896687de75928ddec6080cc959ff6237085167155255188b56e86f2fc406cc7dae2e31e526e6ad71d95e1944bb004ef55a9b1251874aadcefb762b650d" - }, - { - "block_id": "000000773cf65d2b81aa54fdcee69cdaa208ed9e", - "extensions": [], - "previous": "00000076a0ee0f230cf63ab071a6eeda8ede0c94", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202cf33b0bff2e157bfe8b138ef5a929c2c354620f9ee620912035ab3dbbb3352941794e33ce54e1865dea7bb290669fc05b7114965c9be062cd9e3e03418c1512" - }, - { - "block_id": "000000781576e1a2c23d2f296c4d1f813b307dfe", - "extensions": [], - "previous": "000000773cf65d2b81aa54fdcee69cdaa208ed9e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20215704d7ae3b539dde7a4df905f8693ac6aab2e180fc4fb9517aebbc1762f02e4a0599e53f3dfd982759f4f57f485033cd5fbf9cb17cb7c3f3f3fa51b9c67182" - }, - { - "block_id": "00000079e84af5ce255066688fe2abfde1174927", - "extensions": [], - "previous": "000000781576e1a2c23d2f296c4d1f813b307dfe", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205be55cbb4c1a31f3e70d0520568489b52399760b28f69d04b976e3d267c0c8c12fe340b8d1957fb1695f7ac677d0a2a431d7767c44917a3f651e16b24a5ae2df" - }, - { - "block_id": "0000007a514fd4034a39ff8bb4225760ccf61154", - "extensions": [], - "previous": "00000079e84af5ce255066688fe2abfde1174927", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200cddd099fb05345588e756b2988e37fe2b6eba9b0f27617fa48a8889872f49a26c3d8c9eda4ff70db4b2ce645b0438e93f024f14d56c23adaa8bb9c42719ca34" - }, - { - "block_id": "0000007bf0fe8bb621384e71bca4aa785e2fc369", - "extensions": [], - "previous": "0000007a514fd4034a39ff8bb4225760ccf61154", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f679f4c270150fbc880d5c4f83f12b1e54f9bf72862b9c4f9f1bf11dde2062566149a96de894e79544a852c37f3917063b965a662a5a412c83144898e7fa8bcf1" - }, - { - "block_id": "0000007c86dc5a46060b0590fcb61fd0e3de35d4", - "extensions": [], - "previous": "0000007bf0fe8bb621384e71bca4aa785e2fc369", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2028c4e03701d36ae9fb90ea237b36650b0d24778040f6ddba532f414c7c1ac3c329b7c02481438c36a3fed58ee8180c53e472cfb3a359f012833e6afe8e09e3fe" - }, - { - "block_id": "0000007d1d0c4ae8538aa25195c6d4fb82f0c10e", - "extensions": [], - "previous": "0000007c86dc5a46060b0590fcb61fd0e3de35d4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4edbd875c5601e2c412fedcee21cacb07fee38e7deb12e0606ac0ad016bc8a503c88da54004e97871949a026ce4fa2abec50d57b52147601d95270f2abcbb9a4" - }, - { - "block_id": "0000007e57c38c79a274120d94ce453f84cf480c", - "extensions": [], - "previous": "0000007d1d0c4ae8538aa25195c6d4fb82f0c10e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204b628a16d6b54b662af6eb669178140178857255bbde27569bd8e8ac588ea46c2af38fd729661790d4d166008b56897bc6c4466e1c57609523e1e6d94ec5820c" - }, - { - "block_id": "0000007f5a36c76f7f7725a8301c7045598ced71", - "extensions": [], - "previous": "0000007e57c38c79a274120d94ce453f84cf480c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0b4c9a345744dbd55efdaebd1ad810dff614ea2bd7dedd888eeaae97397457640c634637c4a6f2b3705be779f45bddd7526d3082df33a19b57a12b3c33f9f15f" - }, - { - "block_id": "00000080c7835d84ccdae7923b5f3b2d080b8f32", - "extensions": [], - "previous": "0000007f5a36c76f7f7725a8301c7045598ced71", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2b3bfc76d944f65fa967ce33b452705b9a9c0c2cd84980d91cb9c96a10c60a0b23a510b969b3004a7ecad75bc956b4565979280d31cefa6be6ff372c3074b093" - }, - { - "block_id": "00000081aff8010aa3fc988e7ea6cd9b4ecef082", - "extensions": [], - "previous": "00000080c7835d84ccdae7923b5f3b2d080b8f32", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:11:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202267b508200c51f6c443ac448c426636f3f7ec6bd06605ea1f432b48f15c60597c9bdcbf8c1644501dedeb8642673b3d7c89ebaa809144beb99eb0ed58c5cebd" - }, - { - "block_id": "00000082a7c19ac51689799c53d35286fab2880e", - "extensions": [], - "previous": "00000081aff8010aa3fc988e7ea6cd9b4ecef082", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4878583d27719b323d8d35c5fa4d19129148145b9bff07456240ac4a87739a6441c9673292c34887b273ed1099da5765df9e3fb455da6ee08c59cc84952fd8aa" - }, - { - "block_id": "00000083435b783866f834af7f2b7e8482b11275", - "extensions": [], - "previous": "00000082a7c19ac51689799c53d35286fab2880e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207b9af3805532a88d9d4bce1f32ec0340be24575bbc170ab39e48d8cb0d22b06c4c8f4a8d536af848c399585788b982f6f9d4feea7ed5c514dacaea6584beb07e" - }, - { - "block_id": "00000084552dde1c53cec0e1ed72d1fcdaf89900", - "extensions": [], - "previous": "00000083435b783866f834af7f2b7e8482b11275", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f09f0d764ca0225cf9a5f192fe5b6c629ba854d727effe00929844b65ac7aa4383b08e97f13f2d31bb42c5942f5264f1b74375f8ded0ca8bfc19b1fb2e2744206" - }, - { - "block_id": "00000085cfbd79d146e239fb08d99d203403c09c", - "extensions": [], - "previous": "00000084552dde1c53cec0e1ed72d1fcdaf89900", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204035165dc850cc35cf6e91137b0f99accdbfef969c6f412e10bd80c8d5569a2648b2f526e8ada340e09fdc62d0d7ac945eff385abea3a52e0e6505a5a1d8810a" - }, - { - "block_id": "000000864156bd943442d11d4b660a9b03e6d415", - "extensions": [], - "previous": "00000085cfbd79d146e239fb08d99d203403c09c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204697ce391644413d0e0f51160f5cbf3bee07da8fb64f1093829b175beb7ea8f125caa6d1b99b9e535da6ae62b82a51aa821ee47b529ce9b274f673f399ca4748" - }, - { - "block_id": "00000087a0be955702205b6ccd1fc0d2aef0706c", - "extensions": [], - "previous": "000000864156bd943442d11d4b660a9b03e6d415", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f09b5c9a9849f17c9c33a6a41874163381d37c1c97d069caad28dfdfd6b30dcc502fd186396a972bea103007ce25a0fb5cd56816e836e614f2cbe55a5bfc46589" - }, - { - "block_id": "00000088f09c3639bffeeba5a32933cac8e60b5b", - "extensions": [], - "previous": "00000087a0be955702205b6ccd1fc0d2aef0706c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2031fa95e1aefab320b1b5030ba30a81c96e7616a0026695fb2390fa1c57e41cbe6bac3a11c515c89b2911fc88caf348a7ab4af074a6743961f022ec6a07827aef" - }, - { - "block_id": "00000089fe0919e06045d78beb9e5eea0db76b2d", - "extensions": [], - "previous": "00000088f09c3639bffeeba5a32933cac8e60b5b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f22c7c86a0ef94904c9dc24984036dccfbb8cd0a8ac044059bf6d70ace37edf1c53ebea8dbebf633c4b5ba2ba21eed50579a00f0b83c789c6556bf58737fd6d4a" - }, - { - "block_id": "0000008a5c95a4d46ec295a32b091c9fd0d627aa", - "extensions": [], - "previous": "00000089fe0919e06045d78beb9e5eea0db76b2d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205e9cdcad4a7fbf3f69f67ce3790a78fe2e2330cad183c9d2406c4142ed857d6510eaada73067ce4be9517cf60a518864597e38260ccd159bdda4fd3b30951223" - }, - { - "block_id": "0000008b32d6882c17d274c23f4663af5e15032d", - "extensions": [], - "previous": "0000008a5c95a4d46ec295a32b091c9fd0d627aa", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200ae70a387ebc55b00b4aae673018836fd2ac85da2a58e3cf31eb7d320dcf06aa75c3b3060487fb18befb26066ce19488a5108d213875b254757b5fa4a7d3a667" - }, - { - "block_id": "0000008c3dd4b1448cdd316e5a238352d673399a", - "extensions": [], - "previous": "0000008b32d6882c17d274c23f4663af5e15032d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f735e6935e9750fc2af879de7612508aaa153a228a8a7559d619bf63017f0757e169586943920a05ea6c4dbb9c09a5b3646aaf88f370fc884d0253152fcf9de71" - }, - { - "block_id": "0000008d4cf1e3788410208317ceeaae7508c4c0", - "extensions": [], - "previous": "0000008c3dd4b1448cdd316e5a238352d673399a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202c437a5683e36aa17aac11f583106516c2cd47a7ec97d3e6c54a141899dc8d127ef6dd23c046e0f42d1460708735f4609ac74a42de5d2d958178181e94c0e0b0" - }, - { - "block_id": "0000008e655dd4d896a049824f4fb45fc8404b64", - "extensions": [], - "previous": "0000008d4cf1e3788410208317ceeaae7508c4c0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20193cc1f21069c612b475e1c8e3fc5b7f60536ea59ed5a19a9a56df258b85083425b7cf627a9ded83bb4760766722d9be5004cf86f45fe57bf9e000cde5fa6db5" - }, - { - "block_id": "0000008fbe5bdd6ab8660386c29c3a8ed32098fa", - "extensions": [], - "previous": "0000008e655dd4d896a049824f4fb45fc8404b64", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2018823f25656ee541374d067fe02349fd2cf339790e018bf1b0eeef846e5aa0be04e5dda33866f521387b4dd1bd9db8202e05d1c3a63de386a011d6920424ed10" - }, - { - "block_id": "000000905dd40072494ad05628bdfbd331312435", - "extensions": [], - "previous": "0000008fbe5bdd6ab8660386c29c3a8ed32098fa", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20643c9ad423d8744b6997cd446e437752498de733f78ae7bfbb2add4fa4634a67572fb68051ca3f73da35159db08615c1098fe509a8717ea6c9ef782cfa611a65" - }, - { - "block_id": "00000091b3b5f62f1292ac66fcaa74ec601cd052", - "extensions": [], - "previous": "000000905dd40072494ad05628bdfbd331312435", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f50a834425dd908e15dc997d223a144e10a5eaaa429ba486acfaae8c90138607c7e6529d7a0b16cab6fc2d7968f5bf6980f4462a552416c76a569c5ec266ba5a9" - }, - { - "block_id": "000000925c52ed132bc610ba4286b93a76819234", - "extensions": [], - "previous": "00000091b3b5f62f1292ac66fcaa74ec601cd052", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f734874e7e8bb1407bb0537aa6cf9a4c4b4d9b7bf56ae6c8bb7fd99dc60393f717ef824d069c5d6059863813d7e4c4e8c94a7c555f77c7525e60ac102f59d44fa" - }, - { - "block_id": "000000933b232842c3c2135416d497a1a069cd28", - "extensions": [], - "previous": "000000925c52ed132bc610ba4286b93a76819234", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205bcd157b84c71ac01e8cc29c35ee5fab63af2401c621d8ae4dde627ad1e3d32f33fb74790f2c252125a2c09f05a858732611736d1e1df42fd874f3c3bcd7b198" - }, - { - "block_id": "00000094afdb8846404f5f768044420e4eb066b7", - "extensions": [], - "previous": "000000933b232842c3c2135416d497a1a069cd28", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206b52a96896c8ab8dd7a80c2a25a72c4b609809485f0480f2298966f8f6ad3dfc423ce112058a3a9cace2f2d147eaf6ac49297bcde4107af5e2c76c4a0809a2cc" - }, - { - "block_id": "00000095a85c390d541d98f041f453ee8d5af3c3", - "extensions": [], - "previous": "00000094afdb8846404f5f768044420e4eb066b7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:12:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f05da86ee8eef84d3149365009edeaf364624ca03644bb340fc50f845133e0b7a2a50c7ff4719f1a4eea2dd96de02d03b7c9a3d85849dc6c09ae37dcaeb4f9711" - }, - { - "block_id": "00000096d032cada46ba4d47318df0a8270d219a", - "extensions": [], - "previous": "00000095a85c390d541d98f041f453ee8d5af3c3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204380a636f63f01978fb1a788162bb3e3f58c53df61c1676c3ed2a6d53e2b48cc114a02214c058ec269e137a553cb8e4cb1f6d585873b729b61f62132cb389f9b" - }, - { - "block_id": "00000097a6de1f5c96d0d72c0a4b7a35a733443a", - "extensions": [], - "previous": "00000096d032cada46ba4d47318df0a8270d219a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7499ea5f2041eba1692daf8374f4b60bf118721f011c797a1826d4779c68539a0f6535eeac6c0f87615aa6e3e831b0366ca2a1e75de985ad5730e6003fca699c" - }, - { - "block_id": "000000980e39455ca633eedc5fe97eed0f2e6c45", - "extensions": [], - "previous": "00000097a6de1f5c96d0d72c0a4b7a35a733443a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3d48279d3e4f466f8a398f86279fd42d8172a3a70917c9f91bd189a5440a5dcc6935370bb1d4af3cfc7de30a0945c281891dd3959000f61c493f58b7a9a7bd68" - }, - { - "block_id": "000000996f46a5630490c2eeebd2e366cefd7e7e", - "extensions": [], - "previous": "000000980e39455ca633eedc5fe97eed0f2e6c45", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2068baa04d189b60ce1a11e5c1a93831be56dd5feb2403e494082857ad30316c6d7d45695ef96a5859f04c542a63932adbcdb102c4f914b708176a05b0548c85b1" - }, - { - "block_id": "0000009a648a07ccee5238b9025ce62c36204ab3", - "extensions": [], - "previous": "000000996f46a5630490c2eeebd2e366cefd7e7e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4d1849ac0956a831f6869ca840fcf40829f042e1aec1aac9406adf32e6b4d28041ff10cf7c83c88488f31475406db53d8ea24b1cb277c19d1f36b240904669df" - }, - { - "block_id": "0000009bb4fd9f9896503f49d9c5d1e42c3c6504", - "extensions": [], - "previous": "0000009a648a07ccee5238b9025ce62c36204ab3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7bfd7de6ca4b51594ddd6df5cf6d44702620ab2595acbfd125189c5e30d726944e0bf6dd6557d7a86a04a45b48a6dbb4255d9cab0fdf6072c2ff561483315a6a" - }, - { - "block_id": "0000009cf8d639784052e62ffa878f0904a4d657", - "extensions": [], - "previous": "0000009bb4fd9f9896503f49d9c5d1e42c3c6504", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20090ad28d3597e8a06f3c0680ee88813e3ba83a4b3636c41caad9d7757889add9590a5a873b6fc7f6dc7d2aa952bf01fe18bf19349fccb4abf91591438551695c" - }, - { - "block_id": "0000009d2aada6a6736e7758129d2ae490a5cb12", - "extensions": [], - "previous": "0000009cf8d639784052e62ffa878f0904a4d657", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20562ba53d3798208523f89a75dedce19ebd497f1b82aec5605099e54dafd9b99c1ebacfd5ecc0a6c10fc07dc4b36c06077aa87c9dbbcf8b4c29f0b1cb29fa0fd7" - }, - { - "block_id": "0000009e4a71565e9cc94b0e8c8b51ecaf5fc3b4", - "extensions": [], - "previous": "0000009d2aada6a6736e7758129d2ae490a5cb12", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207e57f1bba277f71bc65e4d098b1e9b7e13393fbf71d22294fe8b0c84eda7fda1011dae24ebc5b8c3b52a22811f71b30db5bc3b5cde23b30b6e6f924ae4e214e2" - }, - { - "block_id": "0000009f62c47ab45a1311d47d0b387cf5caa20e", - "extensions": [], - "previous": "0000009e4a71565e9cc94b0e8c8b51ecaf5fc3b4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2030f932fde4a7dbb8aab66f331db06494a4b9c8727701d68a8d6535ff43794813061cdbc208dd67142e2ef9ca96f1225a3431824eab5f93c71ed26bc0aaea1c3e" - }, - { - "block_id": "000000a0f88621fa8e7424b17c6a290c9e8daf41", - "extensions": [], - "previous": "0000009f62c47ab45a1311d47d0b387cf5caa20e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20401a4540310bb64bf5df3f96f8feca0e9efcb8eaf0bfd13c48fa0e6591f2ef661ffb06975ce80e04305325708ad360fd9eec7f3e7345ef4a78da3a695e472e19" - }, - { - "block_id": "000000a1e719941f65c0f941508c16fef4f55a70", - "extensions": [], - "previous": "000000a0f88621fa8e7424b17c6a290c9e8daf41", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2041b3fa5af01cdf366e4a0b0ad79da5f3a03b1e1bcb72c52d7ad5570b9f8b44cd2c92c6ac2c1a4e0d08fdf066a447feb813dd0e109b67f31654d4ea06fa966d22" - }, - { - "block_id": "000000a20e5baf62f9898276ce635f78df4f85f1", - "extensions": [], - "previous": "000000a1e719941f65c0f941508c16fef4f55a70", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f193a4136032b7e94c8d0ac57a17ca31d32c1374f9adc3589b6f3678dcbadb5ad64eb115f9a38d3c60abda5c3a0b45f88d75d4b62fa73e439061596d98188415b" - }, - { - "block_id": "000000a3befd2950e3c5a7994d98288eb8b1af64", - "extensions": [], - "previous": "000000a20e5baf62f9898276ce635f78df4f85f1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6399d9f3b700cf92055c795cdb25229f444353f3876f40af45beac56dcb4a81f6cf05f41f221023cebde54e113e0295619d81a77ee9fc9f67e850fb7166b4da9" - }, - { - "block_id": "000000a4e0174a8ff89a32dc3577f0e68d9a103a", - "extensions": [], - "previous": "000000a3befd2950e3c5a7994d98288eb8b1af64", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f02556c255bf1020347fd3e9c6eee13b26c0b7bf25291f88e61113d2f42001420391c72e38a1f18a8f754ef3116b2a65fb414d94869cf31d6feb94c0edc254de8" - }, - { - "block_id": "000000a5e2458c5191688a472515fa9b362c5121", - "extensions": [], - "previous": "000000a4e0174a8ff89a32dc3577f0e68d9a103a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6adfe72068cbd04a9f75e9dd113a76898ed6b5d1a9e2b93714fb5f85e779d9647416521280e2cea3757804585704f56bf654fdd122ed03669a3a3cf1cc6e43c0" - }, - { - "block_id": "000000a60d909964774b49f9e6e96823f31842e0", - "extensions": [], - "previous": "000000a5e2458c5191688a472515fa9b362c5121", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4bb7c0343cb701c34bab025d3dca48772b565640084394e24fba7afc168658181ac32a90cf41c7c02f60301c5bd56843c0e413cda0e73ef257250faa9d2d02fe" - }, - { - "block_id": "000000a709160d801214c72a2a4c7213a677f03a", - "extensions": [], - "previous": "000000a60d909964774b49f9e6e96823f31842e0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201ebf65378227119a17e26fef3de868e044da5d4846cb5af582a1ab24201277ae5b55b5f7500a2952ca398af64f40a466c9e5c5054aedc88386ec76808f3164d8" - }, - { - "block_id": "000000a80320303f09562100484c2d7f1f32afaa", - "extensions": [], - "previous": "000000a709160d801214c72a2a4c7213a677f03a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203d73ec51348acc0a7b9c3f0adf8d659786779cf169d0a649dd3b9f05e6e294474c3419d92a93a27cc1e91b49d625d8cfe4c055a5f8e509c252ce99dbcd02c746" - }, - { - "block_id": "000000a940e75e88521342f81bd9ff3b486810f0", - "extensions": [], - "previous": "000000a80320303f09562100484c2d7f1f32afaa", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:13:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203bc2f797fcc23353d78a042b961d4f02cd8249ccd06bf316212b058f15739a231d2f8aeeb8243d4770d401eccf2dca4f14e9e5215df87c4da028c9c12d46050e" - }, - { - "block_id": "000000aa0fab32632653cb71d7f6eac2c2aa495c", - "extensions": [], - "previous": "000000a940e75e88521342f81bd9ff3b486810f0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7738075050ce394e9b940ee322d61c05568d30514f1f1f47d9da34556a9d38506d93c6f5efe441ba2efaad0e4f56d5d493c0a9684b0969f5a2c4e3557f105b22" - }, - { - "block_id": "000000abd9f5b63354e2fa55c15a81bf78ed258b", - "extensions": [], - "previous": "000000aa0fab32632653cb71d7f6eac2c2aa495c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f51a520ba2cfc9f9c323b2ba0f471377749ee37bca1a93ce8af9a998876165de710a9c24eec87e097bac50e6ee391a91d0cdd6249dab8742c1fc972ae70572c09" - }, - { - "block_id": "000000ac4a9ceb8f0ca85b5eb193a06b686ab336", - "extensions": [], - "previous": "000000abd9f5b63354e2fa55c15a81bf78ed258b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2052537ed8281428769d22d4d98c07f0366fccf782395ad6e5fa25856bce4983bc5c8547f7d785f652fb5d68555c7ab22aec5608b46faf21219b798669662554d8" - }, - { - "block_id": "000000addbbacca24ae0df39dd49635b1215e097", - "extensions": [], - "previous": "000000ac4a9ceb8f0ca85b5eb193a06b686ab336", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3ea09ba2a29223e3200899878103fd908c26fcf9af21491d9f926bf4fa483196020c0105832ea5f87658f9e19b7cf833706ecec18a747ffb3a80cf438c1eceda" - }, - { - "block_id": "000000aebfa288d029ce2aa93fd82b16ca2db19b", - "extensions": [], - "previous": "000000addbbacca24ae0df39dd49635b1215e097", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0f64c25338bcdbd13022c7a9dddc991d0c1f3ef36cf5bafdeb460fcdc10a156d522c9ee8578c55a48ae86861a699376187cd642e454b990e12957a1f0f39c18b" - }, - { - "block_id": "000000affb10784c76d037b143ece6513a6e915e", - "extensions": [], - "previous": "000000aebfa288d029ce2aa93fd82b16ca2db19b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4a70376ae68183772c3ba8afc7e240ec5ce364cfd346ecd3f3b8ace7f33a4395282bf79e3ed921183b798f804514b5a0b73d3bbb5a991ca85582047153625bab" - }, - { - "block_id": "000000b0c668dad57f55172da54899754aeba74b", - "extensions": [], - "previous": "000000affb10784c76d037b143ece6513a6e915e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f46b3eccb9c36e637edf14dfde246c1b0d9a82c0d15a2ebde2d6812f5b30ffa1762c6e6f653bb8a845f8e076bceedefdff22b6773c8fe403a655df5a5447239b3" - }, - { - "block_id": "000000b13707dfaad7c2452294d4cfa7c2098db4", - "extensions": [], - "previous": "000000b0c668dad57f55172da54899754aeba74b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2036fd4ff7838ba32d6d27637576e1b1e82fd2858ac97e6e65b7451275218cbd2b64411b0a5d74edbde790c17ef704b8ce5d9de268cb43783b499284c77f7d9f5e" - }, - { - "block_id": "000000b281f1aa2c692b024bf6fc0d8ab7f3ee69", - "extensions": [], - "previous": "000000b13707dfaad7c2452294d4cfa7c2098db4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f258a2b3176a29321b2771d0def4ab1fcb72e5e8d6956734ed492ab354a7ce95e3071e63e390ccffb26114699e031630529e82f24280e840650f5c3bba5c76422" - }, - { - "block_id": "000000b3eac7c8e3de2b029f8e9a5f68a90f3b3a", - "extensions": [], - "previous": "000000b281f1aa2c692b024bf6fc0d8ab7f3ee69", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200b5649ca3120dc6806eabb2bc211484f078275dfccce4d89d5aad3ab7b45abce548eb28d06afb0ceeefea7c19704374d06ceb022edbd0a4b69bd8fa2298d9b92" - }, - { - "block_id": "000000b436bb1376d62bbef11feeaa4860d9900a", - "extensions": [], - "previous": "000000b3eac7c8e3de2b029f8e9a5f68a90f3b3a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2038ba4f48289d836fd0cdde803b7ba5fc9cad9bb5765b307d9020a3d33fe1317b7a46c8c5c85ade824189eccd5d20f4d0c0623c37f4efc0687096d45d4006ac94" - }, - { - "block_id": "000000b5aff6db1695ca80b92665ffc6d09b8c9c", - "extensions": [], - "previous": "000000b436bb1376d62bbef11feeaa4860d9900a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20524a11b01c04552f3dae391c5276b784eff743459d13a6de4a665ff6e280d4725b138686ff0956ab7592cf2fed965c69bb1043e68bc073b96973146b98658990" - }, - { - "block_id": "000000b607041686ab899f74bdc0fc262bdacf3e", - "extensions": [], - "previous": "000000b5aff6db1695ca80b92665ffc6d09b8c9c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20347012d193c97aa5b12b310e6e66ee4fcf6a863969b0d0ff1c889068d0eeb77714657dc202cf552fbc8f1c8a3ef97aa947fc64af1f26abe23fb32e2e3aaa1e8e" - }, - { - "block_id": "000000b79d36828c4c930a5112416fbbd35d6073", - "extensions": [], - "previous": "000000b607041686ab899f74bdc0fc262bdacf3e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2000ea50ea2fb9c2023c2217b022cdd49bb520e718add516e0ce7fe5e70ca64d1301fdcb7d87a66fddd13511d79ff6bb4415d7cbbe947f80a58e4c0a855eddfbd7" - }, - { - "block_id": "000000b82bfb22d0773ab3d11dc72b61e66f97fe", - "extensions": [], - "previous": "000000b79d36828c4c930a5112416fbbd35d6073", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0b3c7e940168f12a1a3c4bcc9e4a7d5b7d8282af4e2a4390e31dde04cded418129b9b6636d600076481565d86e764f6697aa4736c1963e0427d639c3a6e75721" - }, - { - "block_id": "000000b92e67f8bb2b2396757cba17495be49d76", - "extensions": [], - "previous": "000000b82bfb22d0773ab3d11dc72b61e66f97fe", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20667db80d9cb331d8efcf242ab374788942ea34e409435cafb772479b41a4fa4d7fa7ca0dc4135307e554e180e2947d322c02ebb3080badfa7f79cb9332bd8a9d" - }, - { - "block_id": "000000ba749cc7981af2cc1b5f2b7fb19c003c03", - "extensions": [], - "previous": "000000b92e67f8bb2b2396757cba17495be49d76", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3716e545bdf0c7d4a144a358a147954dce387f9a6238a50ee286198a7ef9c49204fb51a6e5d877494fce4ce470918c815ecba21fba6ea9f5704df4b85d0bfbb8" - }, - { - "block_id": "000000bb0268c76b8ec70f839e29f0e2ce10be99", - "extensions": [], - "previous": "000000ba749cc7981af2cc1b5f2b7fb19c003c03", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f61854ada7cd1653bc4b397c3d1e6d528047d5f9cd33003c9f61b466891c47721106510d7f85587ddd6e593471a3d0a3580dea172f3a21c6bef76139e0b3b0fa9" - }, - { - "block_id": "000000bc224fd8eb318aff577859072bc5a2a1a0", - "extensions": [], - "previous": "000000bb0268c76b8ec70f839e29f0e2ce10be99", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f642a00d9826f1133712e00bfbfb2f27aa41d7942f7682208a949e5b7bfff6f4763c5f99a5f1722d865fd6e742362379a18b8b7e15cf642897329c9896214c2d3" - }, - { - "block_id": "000000bd227d3a042ff85b51b6ea97e13a8772d9", - "extensions": [], - "previous": "000000bc224fd8eb318aff577859072bc5a2a1a0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:14:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206792f4dfb236053d02f161e5305e41581e8c062d2c5716aa449bbf583559d9ae3f49827cb40062445926ac05f76df5024efe9be58adf6dcb3b261936414c7525" - }, - { - "block_id": "000000beced747fbe5768d79f6840f1a3b7ad254", - "extensions": [], - "previous": "000000bd227d3a042ff85b51b6ea97e13a8772d9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3b847a16efaaef8c41128a799cb0c507f81aeb99650313167f59d1b7063e61c56fd07b26ab1c1a1c8937fa920e00907f6d4f0d498155f9b747e1e6f372d10f19" - }, - { - "block_id": "000000bfbe17935034a9cd9be9ca496a08147265", - "extensions": [], - "previous": "000000beced747fbe5768d79f6840f1a3b7ad254", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203fcbc5950cd0a66aeea2e672479bc07cae12d6f3a0f6e196faeed7429830734439a98649e6865b553240bac11666f90378e6f5e0a40707935c2f6e5f1a8e27cc" - }, - { - "block_id": "000000c087a73d7ec0eb3eeb3fbab2081d976029", - "extensions": [], - "previous": "000000bfbe17935034a9cd9be9ca496a08147265", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20611750ce3d2fa9c2219aa5ae9d8af59b7078557fbe250b7065d913ab5bbcbd2137daead216d299af7c27c9b460795f23c1484508f4c866792ddd46dccb006916" - }, - { - "block_id": "000000c19152d333489307936179dca6a1359304", - "extensions": [], - "previous": "000000c087a73d7ec0eb3eeb3fbab2081d976029", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6184694f85ab4b2b794c0abc584450e8363a47acace374403c9906d130e78d8c627d2e64a1d2778322a96110f95c974ea32144fc64130efc78f775ceab4bb36e" - }, - { - "block_id": "000000c22274f75c8ed8b4f5ebf41480480752be", - "extensions": [], - "previous": "000000c19152d333489307936179dca6a1359304", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0764772166ab62a45a3c1117b1cb73fc61397de114343bcab17bd046607e8e9355972943db2185642ed68fe45551656194d0920e3da008b6ce9c60068dc255c5" - }, - { - "block_id": "000000c3ef8cbf06b5975ccb8c24cb9da318be33", - "extensions": [], - "previous": "000000c22274f75c8ed8b4f5ebf41480480752be", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f51628e9cef7aa19a9a112a2a97a95eb7e4decb8459f9bcda96422cd5ea89b4ed57c8ca8065daaa0f2585b3630d37c73977d06470804d1c620dc5cc2d43450c7b" - }, - { - "block_id": "000000c4d9198449cf5bc1528e1e248a25d2544c", - "extensions": [], - "previous": "000000c3ef8cbf06b5975ccb8c24cb9da318be33", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f278324f1bf5b7e9edb409f195c4a2755d106c44765e7ff8aa8ab1a757418d2f04764078330054fc20da8a53b721685f7365bfb3e98edd012b5fcd5a20c440a24" - }, - { - "block_id": "000000c59d283f2f486d08cc635719ccd6ecb991", - "extensions": [], - "previous": "000000c4d9198449cf5bc1528e1e248a25d2544c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6cb1fcb1b88f257d2b191bfcb734a89906ac118c81c3caa916f22d24fb38c55c40604c24c931bc45485a73a4c257f5dce4e4d866711cfcfc2cf3be1d8fb396ee" - }, - { - "block_id": "000000c60b38cd9b4939c815faf78c93b371a58e", - "extensions": [], - "previous": "000000c59d283f2f486d08cc635719ccd6ecb991", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2009e7c5ce0a37ba038f3176ab6639e0ef344a92afd9297f38f0b5c9ba87e612603c7f0695b5fc2a3dfd6cce5762e5f894ce1614e8712caef5390641fddd524e49" - }, - { - "block_id": "000000c78dd942bb3b207f3a25ca798330f47cb4", - "extensions": [], - "previous": "000000c60b38cd9b4939c815faf78c93b371a58e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f427a73fe982b6d9d21817b97f4259f87a7c9ccef3374c7a4db72a31838a675d94b259a90444b274192e584b27b067097f4c339d9939ed496ed34cc2bffc85d0a" - }, - { - "block_id": "000000c811143b04d5a25a1dae5c1e6714745d9a", - "extensions": [], - "previous": "000000c78dd942bb3b207f3a25ca798330f47cb4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202df59b25ce8c41c8027c676961dbad5a7c59921cbeb402c96ddeb800aadaca4d03305911e59e40abcab801005e6f173ff5245f598446f56b07bc6b016becc35f" - }, - { - "block_id": "000000c9a117e8bf8dbaa930192b421900cbf0b6", - "extensions": [], - "previous": "000000c811143b04d5a25a1dae5c1e6714745d9a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1af9b5be643c0487f423c3a4db475ead348c6687a0838a70cd3a67b9263f59e42442798535ecb3e250fd9f0d4a30e1e040df20054576bb14d4e422e3a6e3dfed" - }, - { - "block_id": "000000ca9260390e31ca63055a824b6a0c021342", - "extensions": [], - "previous": "000000c9a117e8bf8dbaa930192b421900cbf0b6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2042c5de0edf50035209b21dce859f4ea6c2c163620ced892082a17cb905ad881c6d85473c53a125fe423c38f143f8853c6d9d81ed5e55a009ee104def017316f1" - }, - { - "block_id": "000000cb321e4e1efd1316ce0b8ed898f0e8b4b6", - "extensions": [], - "previous": "000000ca9260390e31ca63055a824b6a0c021342", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20434e8dfc0e5cd78505deb369681aa3ea24f74f91a66ad7af19e51b27635dff113f03f8eb7c9446adabb39689bf1759618a9e8d13e27aa538befb2c7c7e9d6ccb" - }, - { - "block_id": "000000cc813ee790e60863a1271d1db9d11e2e13", - "extensions": [], - "previous": "000000cb321e4e1efd1316ce0b8ed898f0e8b4b6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20676795063d396b74ceea0b966ee522349b340216cab81bcc2a1e50471dae762d68289019ee6a7094bb9875b00494cdd64774e415fff9af8da212fecdf69101c7" - }, - { - "block_id": "000000cd7a11d94c36314bc75a9b830ea362d97f", - "extensions": [], - "previous": "000000cc813ee790e60863a1271d1db9d11e2e13", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206c230f682d4fa918267fc6c6c65a8b65dbbe355016709382ae6392a34b31b3181cba0c635fbe252fb21ff582c2e47ae3b1610a223a067179fedbf831ae197a75" - }, - { - "block_id": "000000ce83a469ab5514ef4c79e08620af8ecfad", - "extensions": [], - "previous": "000000cd7a11d94c36314bc75a9b830ea362d97f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2019555bdc38947f6362096840040d2eb881d3912426404232c68ce2237d7d2e14508c22609d2664f82be7f98b82175c47bb7f0028cf5abda024a3a117b45b245d" - }, - { - "block_id": "000000cffafe83f440743a31776d519c5b61b290", - "extensions": [], - "previous": "000000ce83a469ab5514ef4c79e08620af8ecfad", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2051d3ba75802ca2ca2bafb092a185c54811a9b6d532afa1bd5250409a133190fa1f9a4aea58d5a8a17751dd1f860055bb3062770cd62d466fd7241dc03e2afe4f" - }, - { - "block_id": "000000d06db368a46b59d5c1fc4946f6d37640ae", - "extensions": [], - "previous": "000000cffafe83f440743a31776d519c5b61b290", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20516cf195fced3d37db3e94959aedbba50b3d2ca10d0be964e09b73d313a61abf782883b5a7b366f644d4df2af87c5450c229da2a4305f446021b9f6b023c6bfd" - }, - { - "block_id": "000000d189c5d3d17fddd4be19dec3de365193b9", - "extensions": [], - "previous": "000000d06db368a46b59d5c1fc4946f6d37640ae", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:15:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204e7ccdcc9c9aa9e6fccbba24c9221db4b958203c7eb13f62d13bff753e7085fe0e2ebfd782b026be874f8499c00a3829f8a1d378e9ce81c0061db924c999bf80" - }, - { - "block_id": "000000d25f45cee407d7d1fec5600dac0c9cba24", - "extensions": [], - "previous": "000000d189c5d3d17fddd4be19dec3de365193b9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f562f46e2afddc211950b4bd23feee20f06b95d1dd444f5e8579b237cf2715b9e33a5bea943ed1aabe9e60b39daad42c5c4ea5d0e736ef1db06bfa33745b5d42f" - }, - { - "block_id": "000000d31914154eef0b5b45279bc17147a73f6e", - "extensions": [], - "previous": "000000d25f45cee407d7d1fec5600dac0c9cba24", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20378098f17f9e411c5f74b64d42dff260a510a808c9c2870c0707d04b3ee4f5d21be01c6246a3517470c6e843803ba9df4a8ba36446669c2f11507d7cfe5bf834" - }, - { - "block_id": "000000d41df88a3a7aac4d8e8fc28bc904977d36", - "extensions": [], - "previous": "000000d31914154eef0b5b45279bc17147a73f6e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f79013878d2b40fb074691353603472d7e93d3e4555bcc636f6943109e53d685d5fe5e721b1455d2997fd2eb35ab470fa4f487320370d19ea2db22ef7fad3e80d" - }, - { - "block_id": "000000d592bce5ae675dad0f68d881fefc3b0da9", - "extensions": [], - "previous": "000000d41df88a3a7aac4d8e8fc28bc904977d36", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f410f7d8c61605d4f3470ad54432813083edbeffd1e41ca9c0805fc22dea914fd390ea4b1fd9f55a6800f5a295e7b3dcc8b980054ba8d3f6728ba3a43e041b225" - }, - { - "block_id": "000000d696b309e1e1d472f6c17e07f8e0a2aa9b", - "extensions": [], - "previous": "000000d592bce5ae675dad0f68d881fefc3b0da9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200b5c6ba621e3a4a525e16ee7dc43c2d3e5b4d13a8296a429dce186d79a69ff407492fb6133b6837790423376776c456bece3e0d050265e56fbecedd9c9270954" - }, - { - "block_id": "000000d7f6e7575deb1e9f26a1a5308457d406a6", - "extensions": [], - "previous": "000000d696b309e1e1d472f6c17e07f8e0a2aa9b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201894b85103d123e19572dc981de554fa138e6cf732c2d5e1240fe937fda500864524d904e6915590593ebd1bc17715e584171070441ab53f2289b82bda6fef03" - }, - { - "block_id": "000000d813e01c21d919ebd1ad642bc00e766469", - "extensions": [], - "previous": "000000d7f6e7575deb1e9f26a1a5308457d406a6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2008ec24322c9a3bedcf0dfa04d608f5cf865a1f5875f981cd45c07d359e999afe23c037ac075fa093b682c357c40e19fc07ae3ca974f53079d86262631275315f" - }, - { - "block_id": "000000d982cbff041ae95f3b8f4ae432e007b638", - "extensions": [], - "previous": "000000d813e01c21d919ebd1ad642bc00e766469", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20264be76e9716a7a656f9dd73af550a1bdf38ef8dfad3a6ec7318d54876e5a04126dbcfbc5956e71555b9773f8949363879eddca0ee88b04ad33a2aa44dd5d2a5" - }, - { - "block_id": "000000da3c16a92840be4b9bd4dca08a6dca6251", - "extensions": [], - "previous": "000000d982cbff041ae95f3b8f4ae432e007b638", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20580cec3ca3e49257b788dba1eecb342a88312fb96df848810a52de6f6d296ce9663372417275fb07378f6e0621e2c3f80b7452e0b5a9f32742912c50a4beabb5" - }, - { - "block_id": "000000dbcee3cb9f6fca052f31558fda3eba4f18", - "extensions": [], - "previous": "000000da3c16a92840be4b9bd4dca08a6dca6251", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2cb3cf415e8faf88a09931e7f2a75a0985d3bc1c900a30a70a7205ca70a5f754081d9c919940cdceeb912a9c841aee929aa0b913581909253c6114e14429ad46" - }, - { - "block_id": "000000dcd2b83d9dd4a375d9b866c3b8a12cdbd5", - "extensions": [], - "previous": "000000dbcee3cb9f6fca052f31558fda3eba4f18", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205333e3f22c455bb329a8f69b87b7e82a105a0c92be8230bb14abbdab2e5120176c1d98f57959337efee30cb84b770bb87ebfbe9125c7f970beb1cfe15e440030" - }, - { - "block_id": "000000dd5185b81a75f736eb567abb4ec85a3965", - "extensions": [], - "previous": "000000dcd2b83d9dd4a375d9b866c3b8a12cdbd5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f51c0e6dc01ddb3694f1fd34ddaba1580b8ce6e7fb9fbb4f0040d5a4ddb5948b85752f1d533638fcc2fb26bb3c70b2ee9d6129a113a5653dc88febad4d6ed4ccc" - }, - { - "block_id": "000000dec728930fe8f7f5a98fef737e0fa1445f", - "extensions": [], - "previous": "000000dd5185b81a75f736eb567abb4ec85a3965", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f793710fa98800990a313b96ac1905e19826ad2468fcebced2579f4ebb80d0120470e1f01d45655035aa171d72b37fd1c1be5981fabf4e732386904dd15d3e43d" - }, - { - "block_id": "000000df28c4bcedc300d65dc6814c0461f19b24", - "extensions": [], - "previous": "000000dec728930fe8f7f5a98fef737e0fa1445f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f448c0f4925bbfd9b921ddab6d044db2c7552d9eb91022e7f8bc3f6949b90aed26588f60717a4221665846e30702a5d473f54837235cc5ec825651ca8805216e7" - }, - { - "block_id": "000000e09ee209f9b55f11549f42b7a98c994534", - "extensions": [], - "previous": "000000df28c4bcedc300d65dc6814c0461f19b24", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f18c30aa6a422f1ae2d1a1c63fc92544ccbca94f4b8e905c8d772b3e51ddc86bc1778463de19d60e958d5864a19691f78e1be7bdc6eb516d112f909efd9f1a243" - }, - { - "block_id": "000000e1272f68eb06be206fd11fb74684295d04", - "extensions": [], - "previous": "000000e09ee209f9b55f11549f42b7a98c994534", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3b056b4ffb0706474cd1e1dfa080fe185234fd2e33c32e41d15d03c9fd512b6b2eb91d24e8fa8c2d5233d1e50f8934f0d0e156fd6ee2612d78da11ea15625d7e" - }, - { - "block_id": "000000e22c6a666019e539cab783082acdcd215c", - "extensions": [], - "previous": "000000e1272f68eb06be206fd11fb74684295d04", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200427818760227db8f007fd28ce344f17ddd154d494386cc31ed69a8680493a3530f9dc686ee606ad1594d88c3287983df99ae5c58e711d34edf40741a571b5aa" - }, - { - "block_id": "000000e38684d9f4338a09a6f1066a4427a719a6", - "extensions": [], - "previous": "000000e22c6a666019e539cab783082acdcd215c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5b3a9296bb4eae6fa4ed4985c43dc46ed3eb2fa06a203e2054b9a6b2b08bf02647b5706a39d74a951ef43006252ac28a1aaa6457613d27df8bdebfcf5108149e" - }, - { - "block_id": "000000e44f95ff053f6e082811978cb545951231", - "extensions": [], - "previous": "000000e38684d9f4338a09a6f1066a4427a719a6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f50d5aee634e509196fe4fccd5bbe18ea4df1ffd8d767e0c4d2775d6f1d28cde858c667d0f5397168d84059357b6775f0d1d17149e1b8f19c1ac8d3768d627fa1" - }, - { - "block_id": "000000e5add46001f0b6dc04c3cbd1887edda5ea", - "extensions": [], - "previous": "000000e44f95ff053f6e082811978cb545951231", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:16:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5dbcebb73ef4116508c146537fa6ffb79f8a8fd9c917e19a184973e564bd797d26ef077252505951489138c8724a83beb9296e771ed304bd08b4b444c1fa36df" - }, - { - "block_id": "000000e63caff25f8dcc6c8bc9b2bfbadffd923e", - "extensions": [], - "previous": "000000e5add46001f0b6dc04c3cbd1887edda5ea", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204aaf020c4f480952ed649a401dece9a338a79a0b86bfb363235676b25b1787691970b3b9e314b05c2548d21a8c98a33e1490a6dc7e961fd3cca7693518dac346" - }, - { - "block_id": "000000e76a310e627604279f00dc2d97e461c22a", - "extensions": [], - "previous": "000000e63caff25f8dcc6c8bc9b2bfbadffd923e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6f594d1dfab122ab7b28affd5c99961437a60b6a923f00fc48575df75405912752535d960d00646bb76d45a03a31082a35d17c1454b3fac679557df54b8f3aeb" - }, - { - "block_id": "000000e88163a8c3a717fca60266fe04f556031a", - "extensions": [], - "previous": "000000e76a310e627604279f00dc2d97e461c22a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f534da2078f2f02d5efedb31e549e940e086f442e6577eeedfd5631bf81f58a3824bbfc506b19a607ee266239a28cf905cb012082b1b2909922418afcfe2b3921" - }, - { - "block_id": "000000e945ee2873b8d015cd71622a0ce537eed7", - "extensions": [], - "previous": "000000e88163a8c3a717fca60266fe04f556031a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5512ee0e6fb77a4600ee80d9135f0b2ecfe391c0700bcd8d9399979ca095205b0a39bb2b9c574ef0e3a4033c718a41a83ccb845d5e25ed6c76801f8c47c637a7" - }, - { - "block_id": "000000ea53cc83e02cdbe70df58c956266ccd97f", - "extensions": [], - "previous": "000000e945ee2873b8d015cd71622a0ce537eed7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205976562019c17cd6c867fbd89e55d7bf125c99dceb663e4ed95e89bc4eeca88c58d897c71e63faa33621a151fa2cbaab5d1e63296ba0ecd93cd535df5c767244" - }, - { - "block_id": "000000eb6d7500f853017fa5c8bece514981a902", - "extensions": [], - "previous": "000000ea53cc83e02cdbe70df58c956266ccd97f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f63e299744f91d169571bed5c922d8ad2419d45d680b6e6b147a54958f78a2c69127c90cb46a6625f431a4c2e8e66bd14ae74543bcc22e8bb9e0f964c9b1f9865" - }, - { - "block_id": "000000ec92c58ee30da2542fdac1fe4e713c0972", - "extensions": [], - "previous": "000000eb6d7500f853017fa5c8bece514981a902", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5fb3e7c07067af4893ba9491221670fc93045c0e8890fcb3a705b9b3b0154b722ff88d0ab4c60333367139ef1569b45e3afea3fc275ce8b04e2c9fceca9f25be" - }, - { - "block_id": "000000ed7c9f6922e8002169a24d22c30f2e6790", - "extensions": [], - "previous": "000000ec92c58ee30da2542fdac1fe4e713c0972", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4d3a7c2358378e4a541608d5ec72ef1c2f80e3c7ac5f3f2daeb52b5a2d4a72f7238c93f414b824f83bc3cd1ac0f376a218088d240cb906f55d49b9c6711176eb" - }, - { - "block_id": "000000ee0fa56df6b800798864eed965ec323ba8", - "extensions": [], - "previous": "000000ed7c9f6922e8002169a24d22c30f2e6790", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6d39d3230d049edc6053896b36e5d61ad4e60f73c2900ec40b26ed54721fddb32112a59d7a512128ce3462bb84b862fb2a737e5c310e07cbfc56cf6893695ccd" - }, - { - "block_id": "000000efc53b26de3f9a6e6ab28ad515f27823de", - "extensions": [], - "previous": "000000ee0fa56df6b800798864eed965ec323ba8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201ac9b66aaa7cc1cecca838571126b052dccff140eb08f30c4d7d053efab0a0fc69e0058cc795374469855c76a58813cadc248c3290163f5c1324ef45ced976ed" - }, - { - "block_id": "000000f06c2b8190263ab85f1a2da4ecb2d473ff", - "extensions": [], - "previous": "000000efc53b26de3f9a6e6ab28ad515f27823de", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0bc80c1e7512fcac4abafa0d21c992c816f63c2d423b8eb8991a3fe27817749321c883aa0657560224ba3c28596a34a35607ec5f43eb728be3ebdb28b6059f16" - }, - { - "block_id": "000000f1cbc736a47621a188af9ba61853b36e95", - "extensions": [], - "previous": "000000f06c2b8190263ab85f1a2da4ecb2d473ff", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f62e26b9d62c11e2080f8aee7b48a7e8f1f005446ac15d6240a1bfcebaf802b604fb775686409c9fba0b7979543c322ee16de76713011b35851ae4e24f294ed7b" - }, - { - "block_id": "000000f22ad05eabdf381207f64d711b4e197fd5", - "extensions": [], - "previous": "000000f1cbc736a47621a188af9ba61853b36e95", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2006e278d8bf6714f6f8f0e2edaca913f63e859182bb0ec0c44afa44a967f500420e0a44812825502551bf9fe86d2846dc3e746a5e4a131406cbdb450d6cebcfd8" - }, - { - "block_id": "000000f33ebbbbbc4ec4a9c0d4a68e4b8f953cf8", - "extensions": [], - "previous": "000000f22ad05eabdf381207f64d711b4e197fd5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f63e22dc4ad82f75a0689c5bcc549ace9fb38d622531d8c6a6e79b713a517d7676a45d054ea171b5874192f5b0223639c75e3858162abbce32ce93ff933b066e1" - }, - { - "block_id": "000000f4d9d09c3ebe812f4f096d7a1b7ceffcc2", - "extensions": [], - "previous": "000000f33ebbbbbc4ec4a9c0d4a68e4b8f953cf8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204b77682114a14bf59e7e9ac5e442cffb8d795e17cc5bb9ca8816639f5cc51f0536ecb8afa7f8e377cb64f5ca0a35059a464985b28249d603fc2897461cf7bc4e" - }, - { - "block_id": "000000f5aeedcec1e0561471e6cf17b3dabf052b", - "extensions": [], - "previous": "000000f4d9d09c3ebe812f4f096d7a1b7ceffcc2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f198408d7384821b40623aa5ef9dfbbca65cda91099a8bb7c51766f6e61e4b7ba38bb1d1b0b38fc18094c3476e986c519f12ac0bd31971573a3ea5d5300cd1040" - }, - { - "block_id": "000000f6ba33465e8a5ff07c676dee3873461346", - "extensions": [], - "previous": "000000f5aeedcec1e0561471e6cf17b3dabf052b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f359598f59a832255377d67b5e16249a80b1ec73a5935d8d604af3b72787c9e393eefda3dc8baa02578dcd22e8265fd0b603c4995b2057892877965704df10801" - }, - { - "block_id": "000000f718d66647d74bd92c366ab160a017107c", - "extensions": [], - "previous": "000000f6ba33465e8a5ff07c676dee3873461346", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2027257e7b91bb63138cfb2038403e62a429c081c112f254d524b420218293eebd3607d908df5a4e88347babdda587140f94ae6e88b6e7b2447a98d674cef58cf9" - }, - { - "block_id": "000000f87db3f6c5a484ca943cd6a73b243914a5", - "extensions": [], - "previous": "000000f718d66647d74bd92c366ab160a017107c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3d9a6b3765287d8463f0ab166fd4673326322da02cf36bcd83421c3853bfe23a5b9c521dc9ca2f5b9554889cfa35348755a54186163222030fe70411dc1ea3df" - }, - { - "block_id": "000000f9e560b0d5a3936a3a8de858adee2d9295", - "extensions": [], - "previous": "000000f87db3f6c5a484ca943cd6a73b243914a5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:17:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6eb1cfb47235be78dae7ba04b80c816261ccd4c14911a6bbd025c4c1677dca772262c606ae1abcfa2d3649b5f716d3a5fd8aa79d2fcb873cb7b544729672089d" - }, - { - "block_id": "000000fae9d4f023e0d0450dcd4874e12e7042c6", - "extensions": [], - "previous": "000000f9e560b0d5a3936a3a8de858adee2d9295", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f407691e606da715b6b96dbd56d4007f31a27be0492ed350891427b4cd7274f8a158901ef550930d81dd5d21a890422a7534e7a13ea138c3edc11277d4b27d68f" - }, - { - "block_id": "000000fb01eb7242928bb3d420d0bd8d4bfaa39c", - "extensions": [], - "previous": "000000fae9d4f023e0d0450dcd4874e12e7042c6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2022ed33903915f0d04d12bb0c7d9efe57b3e0dfcc76c379c4ef3438ccc4ccacfe206ee4b7da105a4ee3ee3e91586a4eb6b3192aaf771425efe46f43e2df96f8a1" - }, - { - "block_id": "000000fcd618aade3a8fd322fb3b24e90766e906", - "extensions": [], - "previous": "000000fb01eb7242928bb3d420d0bd8d4bfaa39c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2057511909ea5bc6853750b46f0e6d9a23a80800405a7e785032b09cf030683fba378cd83d7df2eb8daecfc2fd4ab5ca73c7cf878e291a006822c627628af140d1" - }, - { - "block_id": "000000fdd728cfd2bd5bf54f83cac957c97953a0", - "extensions": [], - "previous": "000000fcd618aade3a8fd322fb3b24e90766e906", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203fdf088c2b47bb0c987ee7425187de02e0f8e4940a0698ffd40536a4af63e1e30956c4cab22957bd5316b1265e1a0ade7d561be72dfe08564f42ea4d4e2afc51" - }, - { - "block_id": "000000fe607c4a7040bacf1923ce44881e73e0fe", - "extensions": [], - "previous": "000000fdd728cfd2bd5bf54f83cac957c97953a0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20126d832b9f4ec86634d29fe5e1b99a4c2f31dd9d75a68e73429681c0a96f9b832b85074d9ceedb7c572f3ad5e8ed4cd8a69a608e46865940e0f75703fb7ee48f" - }, - { - "block_id": "000000ffaeece49992a3abca559ccfcb5b7f868f", - "extensions": [], - "previous": "000000fe607c4a7040bacf1923ce44881e73e0fe", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20460cacd5ab1e643302ada39f6a158da851738a3daf15215aba6ce245019b2f0e5cb922d14fb2a54cb3516cf4cb1d469a21f1b52a020720986bbf9357ec145b47" - }, - { - "block_id": "000001006c83a213626718dbffc6fc375678bc41", - "extensions": [], - "previous": "000000ffaeece49992a3abca559ccfcb5b7f868f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20098bb495580c454279ba577d332e205a690af89de087af5d6aa3961c432a9878279746005cc2f1aa5e47241c33a74ec24c7411aafbabab0b312b1e4589dfe40f" - }, - { - "block_id": "000001014430ba3c2d6041241c1565ff409bdce1", - "extensions": [], - "previous": "000001006c83a213626718dbffc6fc375678bc41", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2001e45cddfe98f576adc8c11e455c9225afe1b48824908685996d655f596afbf75e0c9ce1e805f4a165efdbab9549440ca931bf34d2cdc0887998f30629be5bb8" - }, - { - "block_id": "000001021d85ae553b64b0edd03d3666c5441451", - "extensions": [], - "previous": "000001014430ba3c2d6041241c1565ff409bdce1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206d860a0ee904a037c3134fc93cb111975f731bbcfcfcdc917125d319c3a8abf12f9f926786cfef87da6b2a7314e5287c2bd392a978083af37ac8acd4c257d18a" - }, - { - "block_id": "00000103b6aad9a6b31af6faae377d6dc71d97bc", - "extensions": [], - "previous": "000001021d85ae553b64b0edd03d3666c5441451", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5a9a8f7cf34aed437d7082aa7688ec7eda165be2a80d04994f5cca3641fef9852dd3c28aa767c8c0131ddbab9bde2c80c473be6e76e1fecb50a273bb2e56b3a3" - }, - { - "block_id": "00000104a6dfc109af2f93b98dc31a20bc2c8203", - "extensions": [], - "previous": "00000103b6aad9a6b31af6faae377d6dc71d97bc", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2016f893a0591799b86fc6f0fdeeddf77bf178d399cdae1e0d4d9a4adbf6eb2b75574d1306d78ae1a5564ee8f35f6ba60a2712713ee5a588253cc7ce4293bb63c9" - }, - { - "block_id": "000001053f15ad4b66353cad1cfe250ffb8a1112", - "extensions": [], - "previous": "00000104a6dfc109af2f93b98dc31a20bc2c8203", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f341ea1ccbe09afd58cf66b36f72a36ae89bfba2f63553e4db81b8620a261a712521e2c8312d585528940449d85cc9fb7ff036b6729bed8f3ccbbc8e23a32b527" - }, - { - "block_id": "00000106a870aa5d8fcdfa73e2a821eefdffbe63", - "extensions": [], - "previous": "000001053f15ad4b66353cad1cfe250ffb8a1112", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2022732738b4bae440765dfb4057d381aa7b377607cad66efd0affa7ddd1af4e954dd6e9ff9325de1fd9e5343b3577db2fa1974f396522d5106eb76c7e56996f58" - }, - { - "block_id": "00000107747bafecfbce0c0436ab700dd3a58d00", - "extensions": [], - "previous": "00000106a870aa5d8fcdfa73e2a821eefdffbe63", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f61d1ce559d02e4ab535fa9806b826cb3ae77b0fa007e51d140a0e4ff05f9af8f3d7fe42ec46b42d3dcd4f628c04754360ee487959eadf038b718e5c22f1bab38" - }, - { - "block_id": "000001082a3de2501608751ee7a67d1b5161afae", - "extensions": [], - "previous": "00000107747bafecfbce0c0436ab700dd3a58d00", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7e67ffa04bc420111a75ff66c3fe0b6e29ca338e05de55c84ff5996ebaea5ef23cd7d3acd28fcd72b99680069f6623641a6d6eb5c591edeb57fc0c88cb0ad48f" - }, - { - "block_id": "0000010928b175956d5c336a2fe5838317e5e434", - "extensions": [], - "previous": "000001082a3de2501608751ee7a67d1b5161afae", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5684e99a60fc282932332ff5ae40aec1b765e97c323aebb4dfd3d80eed479e5a429e1655ded4d840a7c5beec15e697386646d857e6ad42739ef129802fdcc1f6" - }, - { - "block_id": "0000010a2693375d3dcce92b16ec4e006e00eb40", - "extensions": [], - "previous": "0000010928b175956d5c336a2fe5838317e5e434", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2024f6c030a20a656960e0415ffc640bfe68b6f6592b78d3f0befada8b7272ee1950249bef0001f7b81b4522ef9918a38acb612c1ee7a61600c479b6b7d411671e" - }, - { - "block_id": "0000010b2f27eb7cc85af922fefe767afd8c9e29", - "extensions": [], - "previous": "0000010a2693375d3dcce92b16ec4e006e00eb40", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20503c9c9cb9393d5c3153c8c510e5547fb6e2dca02e424579658c9931c2c3cb4f71dc41114b40154108270810592b52365dc51d0b7e5fa492fbfb5a9d0e90cc3c" - }, - { - "block_id": "0000010c8d3f776b33b8517f744b919e55a0c826", - "extensions": [], - "previous": "0000010b2f27eb7cc85af922fefe767afd8c9e29", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2067fd63f2b3e35f05357434b316e7c0e45c8b78490fc4be14103ad3f5c499702f351a98f5af6a86d732c6948f4fe13bcd847863f0a7d9c2099d55ac5573c0e7ab" - }, - { - "block_id": "0000010d4a5f5812119a527afa7853d9d9023c91", - "extensions": [], - "previous": "0000010c8d3f776b33b8517f744b919e55a0c826", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:18:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f359c87da08c19c237efaa1171363bcec2def02fafc5fad328264d39d8967ac1053787e5d4e64e0b973ba5613b9826ac923fc306bb67ed9937575ed88d797e3e7" - }, - { - "block_id": "0000010e10b0fae159093b8264285fe7873625c6", - "extensions": [], - "previous": "0000010d4a5f5812119a527afa7853d9d9023c91", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205509a6f2616fe6a39561002fb3c0c513fd42a154f8a3ab66efd3ad45774604944b8cc655d05fa08de59fcda3dd5934b1b78c126195f9dce79c4363b62451509c" - }, - { - "block_id": "0000010f914a8e6c2cebac0cf25563e93fa4d5a6", - "extensions": [], - "previous": "0000010e10b0fae159093b8264285fe7873625c6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201e87951eafd2b191292cde61c65cdc0cbb4c11acc8b77e259fad53146680bac32960fa04bae7863c90043b8970a6aed4431873d7fb7bf5a6b769efdb6b60470f" - }, - { - "block_id": "00000110d3c37332ae8c2388d0e772a03a828fa3", - "extensions": [], - "previous": "0000010f914a8e6c2cebac0cf25563e93fa4d5a6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f187a067f6b597fda3ef0221bec04122329b82e6264cfaf71f7b5db6e15a860ec786c350e77a1d19491717c1513afae7960b28d7dc6779e04f41d855b5afc965e" - }, - { - "block_id": "00000111cab3c0efefc58db5560e784bf392fe0f", - "extensions": [], - "previous": "00000110d3c37332ae8c2388d0e772a03a828fa3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2076e66d617adc21a45353563ae800cdee11d028a42a6166749d585f59675b5d504c687043588283d74b4ea60756778e44dee3967bd949d2799fae447efae442ce" - }, - { - "block_id": "0000011288e6c6dc9ca765bba4c0db23b9b10961", - "extensions": [], - "previous": "00000111cab3c0efefc58db5560e784bf392fe0f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f01a8bcc815ea3276c8c43200df620d805347ae7f8a93dc143fd902f22348a8250cd138b2ff43cf13e12c381a76ba8415cc4654a26527c27e7622d34f18918b4d" - }, - { - "block_id": "00000113ab367f871817a56fd90e55e095687fed", - "extensions": [], - "previous": "0000011288e6c6dc9ca765bba4c0db23b9b10961", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f11515c3ceb48804f5532207938bc416139a59ac78a64907b55b8405d1abc67f74b7daa8010186dc7e0d697490b27c76baf80a6e791875db56e93ae94450e88c1" - }, - { - "block_id": "00000114c024b740a653053f82705f1514cf1c75", - "extensions": [], - "previous": "00000113ab367f871817a56fd90e55e095687fed", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7c19c508aed027058da5ab80e9bcb1636fe04ef8de7ec55fbfe4c29ee7383b5742db0128f3fd32540bf7696edb7ad7d23a5818e9fabed2104c36fafa7eed024a" - }, - { - "block_id": "00000115d003dc08b7e86c90d953cf534ae9e5e3", - "extensions": [], - "previous": "00000114c024b740a653053f82705f1514cf1c75", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2030cc91d720eba3c350ca0ff1e833368ff8ab33d3fba48f6c49e120b5a0de39b72d8395f52097615b83d94a7b1553d001d8cb5ace9f63a051974fb59821965096" - }, - { - "block_id": "00000116100b1baf6849b8c4f5e4fcd1623fd6cf", - "extensions": [], - "previous": "00000115d003dc08b7e86c90d953cf534ae9e5e3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1284e700b33d86f8289637eeb357aa75e556062e0bfbcab2a1f4343b93ae4cb473c3bc8a2d72193e732495a23f0034d2fb9d956e5d356e252dbf75044edc6f57" - }, - { - "block_id": "000001171dc3909ed8e00d352b0c49e5f40a7bd7", - "extensions": [], - "previous": "00000116100b1baf6849b8c4f5e4fcd1623fd6cf", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7f442bafa9d21634d77eba04d0f88acfa71ea75e05588d0562821520e685a5532ffb6897f9c1fda4b0ce715bd90bf98d81e8a07a76082c8e12784b7cc5c13096" - }, - { - "block_id": "000001183c49dc974146dda6cfea483e49f2a13f", - "extensions": [], - "previous": "000001171dc3909ed8e00d352b0c49e5f40a7bd7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7a988899eb7121db9ba3bf800b7eab03b9f676e66fbb5979f1890567987b860f0f628c1f7e01906195600c60d94ce7138751c46edc15c4db5bee2d3a113579cf" - }, - { - "block_id": "0000011962f018fa5ba2ad9299696a019eb99fa7", - "extensions": [], - "previous": "000001183c49dc974146dda6cfea483e49f2a13f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f45a9ce97f45e83569dc99842cf41be96cc58968e2155046bfcd7bc7d37bf74a46d8b38389846e322de6e14c6e8bfeee603a6663f338a545c4b1f20b2e6dbebeb" - }, - { - "block_id": "0000011a2e76bae7c06b2f9d0b72b893adac4a1c", - "extensions": [], - "previous": "0000011962f018fa5ba2ad9299696a019eb99fa7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3ffbfbe878cc23c5dfe0afceceb96fa4c675ad98d885e56f38ad93a20726154d4ce374fb0bc5841fc0ef8bcd89866dd95d75df2097b66a489dec41db9369fec3" - }, - { - "block_id": "0000011bc6db338eb7e38cba8374004f76ba6ad7", - "extensions": [], - "previous": "0000011a2e76bae7c06b2f9d0b72b893adac4a1c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f30291911d10f295f35897e6e59fa60a9d06c6df274c38b565c09e355b40cca326ad923f56dc42a322d5b619468efa35d9c836e74fa774da9cdb36fbc893ffb13" - }, - { - "block_id": "0000011ce5e2339ee1b3b64e3fcfd8a9a80dfd3f", - "extensions": [], - "previous": "0000011bc6db338eb7e38cba8374004f76ba6ad7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20038835b479a542d91dfc8af2bdfdb88e297127b1746b3959c12b0c71aa4dcaf42034d697e528c06dc1aa41d08405a1a935155ff6e897b594f20bec772810542a" - }, - { - "block_id": "0000011d087084e1028258379b75aed4a448193d", - "extensions": [], - "previous": "0000011ce5e2339ee1b3b64e3fcfd8a9a80dfd3f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6cc38d85076685a169e2ce4d1f485dccb3eeb0a6c8fb063b50002258b16e37aa4882e5bdb7304e8e3ee3aec43f4f5fd12a4367a03e746b58d532105f84826adb" - }, - { - "block_id": "0000011e25f256d3e40f793457fa0bc49b7617c0", - "extensions": [], - "previous": "0000011d087084e1028258379b75aed4a448193d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f777d9574092709898dbc75aec3658ea65935c81d17b5b6655926245a341962a00649380cd32f20daef321eff25ad8f021c888f1db1b108b5ce6a61372faa76be" - }, - { - "block_id": "0000011fa926c6732b6b06aa408ab5dbc516576d", - "extensions": [], - "previous": "0000011e25f256d3e40f793457fa0bc49b7617c0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205bc6ec992eff86a4a7293b4d663643ac4162a94e9553bdba31b6c7229887f597388f18c69732df3ab7e0788b42fec991f7f20ab6d30fdc5c8584262c6404da29" - }, - { - "block_id": "00000120b1470820bc716216a9dcd39c3f4a9d89", - "extensions": [], - "previous": "0000011fa926c6732b6b06aa408ab5dbc516576d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2044425a455aad0e90f4fdb275e5e960d239903d44db38b189dba93acb886b3e7f3f831850eb9c3b9c094e8f92e0fb3cd2081dc6b348d625a5dbb953f179e406aa" - }, - { - "block_id": "00000121ee64de170eb0e14852e7758e265a22b6", - "extensions": [], - "previous": "00000120b1470820bc716216a9dcd39c3f4a9d89", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:19:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2074dafff1e6a9acd535a43cf60f24ac867ff1a6ec7ac29c8233f7cfed9bd0da6159473f6e1347921d9720e567faf83971a4bba1ba57c3dbed73a27c135f8b5d1f" - }, - { - "block_id": "00000122b3927cabd68d0798375366c1f030bfa1", - "extensions": [], - "previous": "00000121ee64de170eb0e14852e7758e265a22b6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5b65c0b2900b0e6d9ba3fa501f022b1dc916fddd87cc1ad5b05d19df422925a600ded3287c0b861070c5484db47b5c94c5f7dded2a17aeba816827edda87149d" - }, - { - "block_id": "000001239d2c2ad47e7aaee8739360d06f70d65d", - "extensions": [], - "previous": "00000122b3927cabd68d0798375366c1f030bfa1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f37dd95a4af0926c16fe02c380babc51ee4f877aef096e7507003ed31831fc8da058663964ee32334051c1bae97d01b2068f7d95d2e3faea3edfb4f31cc799064" - }, - { - "block_id": "00000124fadfcc8a18e62a3d478d63d84d8b080f", - "extensions": [], - "previous": "000001239d2c2ad47e7aaee8739360d06f70d65d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2057a2f416b57314b46de31ed9fa312b4f605ceb03383a9bf76c7072fa0b3dfb32552e948b8f012e05f95963fd9a3eb91c84d9f6567a954906f084c10187eb55d6" - }, - { - "block_id": "00000125e232a7f0d2b2adf3cf94e406b8b9ad80", - "extensions": [], - "previous": "00000124fadfcc8a18e62a3d478d63d84d8b080f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f117755bea9e047f8254e321dbd94e2464f34bc72feec9f81ffb9ecc64ca6005745a07f484ca59878a88beffd9b9107b218bd5cafcc11277d2939cb30dcdd5ccd" - }, - { - "block_id": "00000126fca9a2d27ac97ee70dd7bd9c693af835", - "extensions": [], - "previous": "00000125e232a7f0d2b2adf3cf94e406b8b9ad80", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20628d9ab8b7b67a49f1b5305714d2220ebf1640019a9d4dcd715f3b058a66c66a7182c5428adde06ad598cff42a8f0f4b2e53d389b7d20177a522b9e30c0af2d5" - }, - { - "block_id": "000001271c40dbc182c5c3dc73cf2f71449ca235", - "extensions": [], - "previous": "00000126fca9a2d27ac97ee70dd7bd9c693af835", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201a4c7fc720dad2255671f6a6a15282b8997b7a47d8ca411168bbeb68ec49a89c667d817f754968f7ac0ee1e4dbff4a02ea8a82cd3150d3bf81ac0ad6b6670cf5" - }, - { - "block_id": "0000012884d5843d384a7ba0e7bef53935394fab", - "extensions": [], - "previous": "000001271c40dbc182c5c3dc73cf2f71449ca235", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203d5ff738f56e7d08aa8ed3a8961be6cb6823bf95637f56a0b1c99ac695be9aff52812b1f19c17cc7934ecf7d1ae7518a2f5f0cf801ff06b9a856e03ef3053377" - }, - { - "block_id": "00000129dc74fce0ac6ee2257a7072cb93012e00", - "extensions": [], - "previous": "0000012884d5843d384a7ba0e7bef53935394fab", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f37c5c164c5141132b28c96fd99c2844ea76f0ec0bc8cb87f68e03de4eddd76ae190d31c2c850ff700d1fd628ce0a12cdefe12e46b57531f71453b45267d66cfc" - }, - { - "block_id": "0000012ac669463c3e350cf5f11fb5dc5b5c7a25", - "extensions": [], - "previous": "00000129dc74fce0ac6ee2257a7072cb93012e00", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f08b5f01620720ec0ddd6fd66b1b2ce6a166cb5a3cc334f05cabbe21a5832bd0a7e956cf04dac6e78decaec877f4ddb7b9402746360eca012471b10b9810b2492" - }, - { - "block_id": "0000012b9edb72ce6ee6742f93a692d35edbdb46", - "extensions": [], - "previous": "0000012ac669463c3e350cf5f11fb5dc5b5c7a25", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200ecb997a469413e9cf5ff78b21801a867c94ca73e71d8c8810ad0ade44521f783eebe330bdaabbeeca3e7c53a8ca4a03716321d13c6a09c23f7927916f7700b7" - }, - { - "block_id": "0000012cd1d7cb9cfede098a07cf4981ec5506a0", - "extensions": [], - "previous": "0000012b9edb72ce6ee6742f93a692d35edbdb46", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2041f3fea5dde9df8879f26df58e97b406d098a569e97a6daaf17ae9a7f6b0fcfe6e1aa74c8b469dd2e337d3d16b71d9d865513199fc79f08a9c78ff9e2f040e03" - }, - { - "block_id": "0000012d119b2e0dc72374f3b401ce95bed6a237", - "extensions": [], - "previous": "0000012cd1d7cb9cfede098a07cf4981ec5506a0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3bf49e0553a903b0750794bf7990ce450f9aabba125db0fd143f403e3295df27393c89febbb08d6d45201240c8f07e624108c79f796c82a052528d2d3e5d812d" - }, - { - "block_id": "0000012efbef7036d91f87e771c1319c71b41f1e", - "extensions": [], - "previous": "0000012d119b2e0dc72374f3b401ce95bed6a237", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204ee80f6b74910b1c089e6ab336b478e3dc39b9101542071562d63b4e320e7994085d1e55b042ed8d1a33f0cece11db905ea66308d045a6ede56678a37da11fa9" - }, - { - "block_id": "0000012f71a6949ac39016748a3be3d244d5844a", - "extensions": [], - "previous": "0000012efbef7036d91f87e771c1319c71b41f1e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200fc79082bcffb68a79e010c5841b5747884545aaf6544d254f848527f58977cc7ea48c427e3fec93d3ffa0f2a916901ee9add8c42738333d41365743ebdc3ad0" - }, - { - "block_id": "000001306fc6beb1da1cfb6ccfa43704d84cf19a", - "extensions": [], - "previous": "0000012f71a6949ac39016748a3be3d244d5844a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203b69d2465c37ff3dfa57f5efb520fc2e34dfd9de1220d8bd8dd9aa801e67a4de29843e4d8dffa6cad00a5d61facf88b0d3526fd8242f3056a07b20bbc44ceb53" - }, - { - "block_id": "00000131704e5dff14ff527337e6023f7ea223e8", - "extensions": [], - "previous": "000001306fc6beb1da1cfb6ccfa43704d84cf19a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205aa6363a13541497877fd091d823593ac3503656a35a279ad0b1c552671647755b69ed4b59035e8c00a895f43f017ee15d5c24e929f646eb28e7c0e8bf6dc63c" - }, - { - "block_id": "00000132bd1fc9dfd952579b9b5b097629ff043c", - "extensions": [], - "previous": "00000131704e5dff14ff527337e6023f7ea223e8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20366507c9bb2a64fb4790a811ae6cc34b72fbdb672c80564743ae95f92fb43cdd4ed0bc3668a2035991ea60f6d4ea772bdae30083de63a2aa59a95f7ede31acc0" - }, - { - "block_id": "000001335c04cced3d2ca854503cd6692490a4d7", - "extensions": [], - "previous": "00000132bd1fc9dfd952579b9b5b097629ff043c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202c4ccf5edd5f1048c6c0a37c2ff4d8e57565ca1adc7281251357e3505f4a0f40554cb1f24ace1b8a81cc32de6609b22f5bd95115df3f21081fe4e9f9d0624005" - }, - { - "block_id": "00000134bb6586d902db5554bad543bdb66a98e8", - "extensions": [], - "previous": "000001335c04cced3d2ca854503cd6692490a4d7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206d6d7f6af41ad502c2cbda2a6e1e0bfe63240ad9a5702061bfa8d12e3f975b7c6a78b738f6ef79357fee77b2d36a0da657a6ca7ab6fa39826fa6cc3135832c47" - }, - { - "block_id": "00000135be2b0ea7c906121beda95c83a956260e", - "extensions": [], - "previous": "00000134bb6586d902db5554bad543bdb66a98e8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:20:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2025de0cebc43f6ef877aedd08490b292ce86883bff00659c0ea2db5584b833c90622005ada43e435ebd87269702eda4d89d1e9afc9c889d53a4ac156cc1a4bebd" - }, - { - "block_id": "0000013625c56c7cf6417b07fbb91df52a58d57f", - "extensions": [], - "previous": "00000135be2b0ea7c906121beda95c83a956260e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3a8b755053fcf68a9242dcd8f97e377920eeffc0b015d8382692a2bba16f7a0c40bbfc7c00c14d5e98ade93fb6820bc79d9d7bafe15cebea9cebb69be08ae10e" - }, - { - "block_id": "00000137450ef7ac5eda0f3114ca888168a6450e", - "extensions": [], - "previous": "0000013625c56c7cf6417b07fbb91df52a58d57f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20606c227c997e40ddb7d5dca327e90364030f6327794eea6cc2b06ca1898c88d0068725601e73d5e93b683c6346964449efaaf6414432fb65e5f38ca9f959783b" - }, - { - "block_id": "0000013868a41f072655dcf35b5be71ad7928573", - "extensions": [], - "previous": "00000137450ef7ac5eda0f3114ca888168a6450e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20751e2bc83232f6be76f933b2b24fa183414058b372382fda4f32143cf3325063781e128de93b9f73cae905a4bbed7999024b742a36f7486e6b29882e91b429cb" - }, - { - "block_id": "000001397bc74d71a1a490d6eca7f7095a324403", - "extensions": [], - "previous": "0000013868a41f072655dcf35b5be71ad7928573", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f112624274b2275605a923e4618f2e63c0a293a86cc6c81f76e266ad992525ac725a2eaf5d5d608e533c1750c87e210e037a3a004dc1c7bae927488c9da845059" - }, - { - "block_id": "0000013a70c17d772dbebba587bf5deb8f3eba72", - "extensions": [], - "previous": "000001397bc74d71a1a490d6eca7f7095a324403", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2013f7799189103eb1b5a91d2bcdf5addac8eccc7f7e9adaef96113c04a6357b372bd152a6f19d577081b90eb056488343e5bfd173fe81d2f411c92592f6102795" - }, - { - "block_id": "0000013bbcf1d015c8bf7311e97561a4945d6d24", - "extensions": [], - "previous": "0000013a70c17d772dbebba587bf5deb8f3eba72", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4fccbd157cc89b46c3fea99e738e6453822b7983eef19ea79f49a95727f0a4f737031bea4e0222fc7360865c4272f83739fcc18cfd409121e5055de9bb5417e3" - }, - { - "block_id": "0000013cbe46afd51491387e630c4a4f8f9472c7", - "extensions": [], - "previous": "0000013bbcf1d015c8bf7311e97561a4945d6d24", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20336aebcb611cce63feeb587104716d74841eb89e2218074627b09ab0521165a2370af5322f748153353292524dcda153381fe31817ec770e7e2928cd939f6cca" - }, - { - "block_id": "0000013d377e3a6683be53ed1949e618172d8059", - "extensions": [], - "previous": "0000013cbe46afd51491387e630c4a4f8f9472c7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206329f0d3d7cf060f464fa227fe1dcab83f833072b90a22e51731278c5d6f6c057aa5ed69e48fa0f5c0c5a47ee3fce4fde89842f2657d34b971493fa056c45bb2" - }, - { - "block_id": "0000013e782bf049f9582c65d938a6692e2e6a0b", - "extensions": [], - "previous": "0000013d377e3a6683be53ed1949e618172d8059", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20190d1908b6b92d5a8b1a1e57276a6102abf4e7d6e8666378d69fe4222d6fcea625e67da4b54185892b783cf32fde3fe8c196ededbf010e15e4bac02ba190c071" - }, - { - "block_id": "0000013f3b4661995839cb53197dfb551e43df68", - "extensions": [], - "previous": "0000013e782bf049f9582c65d938a6692e2e6a0b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1e09e29f5a27390153d44e9672056ebe31c789fd564e826e713764904cd3fbc2531b95d381259a0d85e07641626423b391c03a30889b4bf1ac40ea4310477e0e" - }, - { - "block_id": "00000140f2c9546f4b0ba86e408b375df9bce067", - "extensions": [], - "previous": "0000013f3b4661995839cb53197dfb551e43df68", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4e847f24d0689ad9e8ace0bd3c00171e5f1bfa3872d7598c2444b3d5076d23bd73fbe91692ce1058e5d168b00f924862125d8497e51585ddfe6b221663b97d6f" - }, - { - "block_id": "000001412e6934c47620e626fb6662aca495e0d5", - "extensions": [], - "previous": "00000140f2c9546f4b0ba86e408b375df9bce067", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20070b19fcb7cd18f85ca020d2b4836c7e58b4cd14ad833f141b5d5a50c363a25a5634bbefcbc5e983f8b4a6217a725a6d644bbdebb08f592cb69db6391af55d42" - }, - { - "block_id": "00000142ceb8d548c967df2355f31701c8db9616", - "extensions": [], - "previous": "000001412e6934c47620e626fb6662aca495e0d5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f781eeb62d756c79b34ef6eb755344235e52b8def5dff07e83572c7546d0dfc494cbc5ee3a6e87210502c25765eb70744040fef59c592f6171a30e968f79f39b1" - }, - { - "block_id": "00000143070b11e853d3995c09a7f1e37e28491e", - "extensions": [], - "previous": "00000142ceb8d548c967df2355f31701c8db9616", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f263e1f4babaec51be0a9d1781af6608a034dec6b906159c3702142b7a1859fab677ab45584c0ebc7f1f526fc545e9124e8c7c41b96ab182ce3e8b554159e55dd" - }, - { - "block_id": "000001443f21c329f297d7e534050830fde4148e", - "extensions": [], - "previous": "00000143070b11e853d3995c09a7f1e37e28491e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207f8bb14cc7b397795525f9d6889ff1a3e0b33ad130077f940eff8af63812140445871e074a920ad245caacf1172a47f6f2cb0740312f1b55c248767e0564e325" - }, - { - "block_id": "00000145a5e05c77f013e44df94233d5a80d5dd9", - "extensions": [], - "previous": "000001443f21c329f297d7e534050830fde4148e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20672c13964fe972343ba9452c3149f1032445f9a321229d5506f373a242280ffa037e683061160709beb99501db4a4bb0f595d4765f4d0e4fd45f08f8e8efbdcf" - }, - { - "block_id": "000001467773ee390e1e1de8cf37841008cbfb3f", - "extensions": [], - "previous": "00000145a5e05c77f013e44df94233d5a80d5dd9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20277fd72673c3dc58670c6b7e27404407b0742c0d3c8f91ef6e25fa4aa4fac50f48e21c04f21540fc76bf1dac85e08592d8dc553cbd514f24a1d0d622b8b506c0" - }, - { - "block_id": "0000014713ad8b08499a2cf6caaa5f69119d3abe", - "extensions": [], - "previous": "000001467773ee390e1e1de8cf37841008cbfb3f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f03e176b2c4396f8d3eeb5c8fde36a424638b6a446d53abd9d4f4a5535ae713273f13fef0038a67827868f19c6131a01c21bc9ebd85f74d356356c7789a0d69d8" - }, - { - "block_id": "00000148a3fcf40d57e69b809d2097c3322ee5c6", - "extensions": [], - "previous": "0000014713ad8b08499a2cf6caaa5f69119d3abe", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205718ea4e5b4cfba7aaec64fa23ce0727370a346be1c3cf37bb47c88c1876cd852a1133842874614a6cb22c6e0f8c2eb097de3de2a8b85d22f677cfb72e64c817" - }, - { - "block_id": "00000149c16d14366f1cd31caff3fd942e8afe42", - "extensions": [], - "previous": "00000148a3fcf40d57e69b809d2097c3322ee5c6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:21:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f618c7b9c666438a08e222fc3a17493768ee4ab2f50a95203820698415b3d6dff3b81de2c41f8235c91c038499e00aab4d597742bd25ea02adf41d9df0e94fe66" - }, - { - "block_id": "0000014ade9b2a0ceca0371cee92b3ef164c098d", - "extensions": [], - "previous": "00000149c16d14366f1cd31caff3fd942e8afe42", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1a561706e6e622cff747b6e64551dea79a8a678533d1de3d6f092e4a108375ed4265c6a41e2ace592dc3e3057580a1d986d24b6ba49da0f40dde65b8e8261b11" - }, - { - "block_id": "0000014baa33c55b8e1bbabf1be02b261e7a5432", - "extensions": [], - "previous": "0000014ade9b2a0ceca0371cee92b3ef164c098d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f359ee6d1d5bfec7afa99ac819716ff17ea3477f080392661de7d2a11e5a7f33e522045ad019ef5e823908e87a730d9cda09bb04b82436f8f4d9ad5b8a333e42a" - }, - { - "block_id": "0000014c1e40a6dc56ba4a25f21438375b9579d7", - "extensions": [], - "previous": "0000014baa33c55b8e1bbabf1be02b261e7a5432", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2006e3aa3e7d6694b86ff4d1e4cdff4aa3c497ff147793fb192a9a04a4bce723912511062fd896e3c6f06d74ed388ffc480de8d6ca4ad45ba0cd718b550fc3d2df" - }, - { - "block_id": "0000014d57fe756eed86e5c178dc1b4e142271ba", - "extensions": [], - "previous": "0000014c1e40a6dc56ba4a25f21438375b9579d7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2575d312c9daa803c131e4722c283ff32e82b9c37a5947341b04f186202bc5e454bc46f192e51c277302b2783295bb397001b501b77de59f271d6aa7cd0c9c98" - }, - { - "block_id": "0000014e7a1862d613f20b69281b6f896a00024e", - "extensions": [], - "previous": "0000014d57fe756eed86e5c178dc1b4e142271ba", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f693a00af490b12efd67b6c6ab00b97cf32c6447ad068cd61f8996d7f66dff61444ffcab644b644a4de684782a520285a325f151103878b4c27b050527dec3eeb" - }, - { - "block_id": "0000014f3cccec87d7b41aa73170b87684a3b8ef", - "extensions": [], - "previous": "0000014e7a1862d613f20b69281b6f896a00024e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3de6da9c74b29ed9e68e39fbcbeb6e9c97fa8fdb9f634f0b365df25800ae513d520bcace180dc86860d8296d670374792d3cf92159584b6a25104ef7c23d8e4a" - }, - { - "block_id": "000001500049d0962e51b8b03554d406034dbb64", - "extensions": [], - "previous": "0000014f3cccec87d7b41aa73170b87684a3b8ef", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2033055ca23f3a4117ba1d1b6e46bd5dfc2a7a0ca96b99c2d18f35e172e5ea6f813fc76fea579ef38ed7bd47dfe8b45a988ffdc87220b07283797b36242e7be829" - }, - { - "block_id": "00000151dfdcc03313119b34087f2deaaee5a47c", - "extensions": [], - "previous": "000001500049d0962e51b8b03554d406034dbb64", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2e4ad20b24bad0b7e43ef87a9f5f7cfb1dc456b5b22d10104e5926bba72f965265f63467d3ec6e96a46d328678bd8f9c4774fc9615805a168b4ff19868327e6b" - }, - { - "block_id": "000001529b9d8d8b44b7a3b79154749aac914b39", - "extensions": [], - "previous": "00000151dfdcc03313119b34087f2deaaee5a47c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f04942a7b4295712c88a21cad67a5b5f79534586a024853369b677482708d86a6142a3e5fe85b4f65a9445461afb526bc496a8076e25af8435f122bba0fd723db" - }, - { - "block_id": "00000153206a7c47b7fb037b127f3588f088e361", - "extensions": [], - "previous": "000001529b9d8d8b44b7a3b79154749aac914b39", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2349c53de5c87e692d2c3dfcd06e95eece715dd1af71c252098479873cdec7475b731c39266bcc44d6c24b231d61451835d1a7c8520001f6e25c462f234121ea" - }, - { - "block_id": "00000154d9f995eb7ab0890b48c74e14bef07064", - "extensions": [], - "previous": "00000153206a7c47b7fb037b127f3588f088e361", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203ca7ce99b649ffc7831f87c390dee05054edf41d373f03ff0686507e564378a2444e87d421e51eb6414f3c7781dff4f5705760a6dae002a23b038729136ec55f" - }, - { - "block_id": "000001555e81e6ca971a4964af2f30b233315e05", - "extensions": [], - "previous": "00000154d9f995eb7ab0890b48c74e14bef07064", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f72341b6ef5b3a8cecded958d4cd5ed82637daa92f6acb96b151657f6e064f314134ac01d78c83edc83dc156f547303441ea84a2c5a9cecd0aa5db59475c1554c" - }, - { - "block_id": "000001566cb08e203d20a6f01bf8f663ea89f72e", - "extensions": [], - "previous": "000001555e81e6ca971a4964af2f30b233315e05", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f27ca2c549228288650e6444a60306b0c1b376f45d93210546c21aeea3a5735710c7968b0fef673a5f23ff1a22e5e340465b0dbbfba0916d6e3d7e68b6ab52338" - }, - { - "block_id": "00000157b608f02562059d40bdf3865cc52d7936", - "extensions": [], - "previous": "000001566cb08e203d20a6f01bf8f663ea89f72e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2069edcab7b4bb62c27e314f64c04b6bbc153d75d8a96c1b1eedcafe13d1645506764d771e280c0202b8799e35223c589d02b4edc50f525e3298e055c73d890c09" - }, - { - "block_id": "00000158ba0c37925ab0d20b5eacaf1e34a12c48", - "extensions": [], - "previous": "00000157b608f02562059d40bdf3865cc52d7936", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207a3a6a18d881aa86d55801515cfa73cd6215122a0fcffd4538d8eaa093be79f26e9d45308d284407d9f0a7932fc5fe27a81d7071adafead3903ce585214dfd43" - }, - { - "block_id": "0000015900f198e916e07af73f17156314fa65dd", - "extensions": [], - "previous": "00000158ba0c37925ab0d20b5eacaf1e34a12c48", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2058c1d3248c6787b200081ff2c7861b571a0b072f26fc6ee6d8e1dea648dc6ea260f4d7413f4aaf04d67e14a88a211309f68f5a113e3d1e9c0e996ff51010399e" - }, - { - "block_id": "0000015a02fd4a21abe0471784be0d35c14d1ab6", - "extensions": [], - "previous": "0000015900f198e916e07af73f17156314fa65dd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207cddcd5d1524a972c5931d68b53d049dca6ab292d5e28a95271d6f5fe21d7a7d2be5898df7b97a15ad5e9d633be92fa4cd5deb9e41ce7ae3029529b3487512a3" - }, - { - "block_id": "0000015b0086e3c0dbcc9a13bfb8b178ea0bc207", - "extensions": [], - "previous": "0000015a02fd4a21abe0471784be0d35c14d1ab6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f08a4adecc5aa29dc7a9765bca75e334247f129cd378f9cd72bddbf46d175718026bd25cdc24a88060dab4374e03f607f7a47557aaf6ee520bb1170a5e98dd627" - }, - { - "block_id": "0000015c2f695587cffc86bfad630278a39ee43a", - "extensions": [], - "previous": "0000015b0086e3c0dbcc9a13bfb8b178ea0bc207", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20156fca102b70ec16a92be652fa9a74bf1d97d8f66b4641dfda452c0af46d182d7621e07d2d7c8287f815aced6c4b21ff64d7f6eab25cd88f574a3047a16fe70b" - }, - { - "block_id": "0000015d3ff0263413cc000ef783b6404331d771", - "extensions": [], - "previous": "0000015c2f695587cffc86bfad630278a39ee43a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:22:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4135135a5993790f07fabcdbeb699a0cea8402f76ddfe27c38b2ed5d3816f528748db348c27e87a8cb7cf4835de81ee30f09741dcfa387fe0a2e343a1e500959" - }, - { - "block_id": "0000015e585e893bd5130cfa876683b22f1f810b", - "extensions": [], - "previous": "0000015d3ff0263413cc000ef783b6404331d771", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2041b85bb455f99a24d02b6cc38f481d83bfb3c484d53616d828bdfc4c139f75332d68b3afc657ef27146d0fa2e73e120ca8a675bfb0d9335c00d49a38c6d4ec16" - }, - { - "block_id": "0000015fedb0684f74b4ebfe236de8b67f0d370f", - "extensions": [], - "previous": "0000015e585e893bd5130cfa876683b22f1f810b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f64df30a6bf3b899e483fcfe4f6d1e678e948ed14b656169dd240c6d642088042197acaaa73360d2a5df842219b739b0998e14d7382ec7e7bc81d72572577c476" - }, - { - "block_id": "000001601db1958a555d5da15f7029a6f401a662", - "extensions": [], - "previous": "0000015fedb0684f74b4ebfe236de8b67f0d370f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207f6ad953c76c94f31da65af98d2d14b5c76c9ce0f89f763655430adc67008d623d297dda91844697c1df0c246486da98bfdfbf5b768b0d4118b2ff853bb9b0c7" - }, - { - "block_id": "00000161b7ab2f1911afee301a5ecb6a249125f0", - "extensions": [], - "previous": "000001601db1958a555d5da15f7029a6f401a662", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f47529130480c7eb59805feeb996527876a3cbaab8aa9099323db93efd25d68e47464fcea278a7a663ac30d18d06401b6b6e245fac827b0c21de9c99afa0e638a" - }, - { - "block_id": "000001628f5a4d6266dbf39e1886adddb9682247", - "extensions": [], - "previous": "00000161b7ab2f1911afee301a5ecb6a249125f0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207697feb9074a91000437dcb324a13591ad6669f9e8ee95a28cb48a911771a6b0165127bc5c6e0d5f1be0461d8eee4a9792041139f198d52fd73000cbc857b56a" - }, - { - "block_id": "00000163e5e52219fc8ea7b7d03857ac72844b00", - "extensions": [], - "previous": "000001628f5a4d6266dbf39e1886adddb9682247", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2030fdd6ba4a26b6edbf70d1b6de0e8208a3889a0f6ed751f6454655b5e4857f4c048004443e3ec64628af09d2fa3a8be4022f31df69d8383b16fc86ed478adca8" - }, - { - "block_id": "00000164042fc33b9133a555f9a578dd4e97c3f3", - "extensions": [], - "previous": "00000163e5e52219fc8ea7b7d03857ac72844b00", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205b384ecae7349085a7ff466029913cf957439d0c9e8221acb84d347a7d720ba20e672b2f4e432732c7b3a6c75349b1129cf1e13217a71f83c72a05eb6c215a56" - }, - { - "block_id": "000001654393c0ddaaa7d904add293f1cdb22725", - "extensions": [], - "previous": "00000164042fc33b9133a555f9a578dd4e97c3f3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2001b1e0eaf40c311f13edaf5552086e807a869a09954f951848e57cd2c41cc5a53c51062a04cd9e5930ae49feff38c9d9f2b86cf979c3261ae603021aadc7ff05" - }, - { - "block_id": "0000016610e0508193b88b8797cbfc7ebc19d828", - "extensions": [], - "previous": "000001654393c0ddaaa7d904add293f1cdb22725", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204b3ccdef454b4996e4227aeec1cfc994aa2c54f8b7b818c3884f53611c06b71c1573a3c6ba7ddac481c1466cd72bfe6361bb6f1646c1060f5ecd35af747f381a" - }, - { - "block_id": "000001672380ab6af215c4b7d5b45fed1d1c9484", - "extensions": [], - "previous": "0000016610e0508193b88b8797cbfc7ebc19d828", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207af421d5f62832f694fdffcbe1b4c6de6b95a6aeee2c7fbd8a7460142dc832fd316a60b5c2b9a35d7ececcffcf0511d873fe393940abf85d21a41b8a344c76f4" - }, - { - "block_id": "000001687243752f645e9a88530727aba12c75fb", - "extensions": [], - "previous": "000001672380ab6af215c4b7d5b45fed1d1c9484", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f54ffcf9c172f7276acf2621bacd38a3b70211aa86aec307d19bd3f1a98cab7e6322607e025b24a5c60c025edd4b47619e9b8feacb0faaa7ef77aa2c5d7d61a7a" - }, - { - "block_id": "00000169feb3aeb4c1089855c58e6ccbc5f5719d", - "extensions": [], - "previous": "000001687243752f645e9a88530727aba12c75fb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f43478b15921d211c23e87c7bfff2c3cb38c68e2ccd372c84d3d3083acac1033f4a286e1081a1c3515322c2b357ccb203c2b97a4fcc60919b753df14e1bf80556" - }, - { - "block_id": "0000016a88bd318a4824a0f75f5ef8fcf16e3b5c", - "extensions": [], - "previous": "00000169feb3aeb4c1089855c58e6ccbc5f5719d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f63a556ec1b51670ae06bb3df9fb29d113cdf35240147b483684fdb2abbe3eea238fc2364dc55318428fe6c79d0901580ebf9fcd64987d4d79741aed371f58a8c" - }, - { - "block_id": "0000016b2d5b8ff86f5da126ecd3251805a556c3", - "extensions": [], - "previous": "0000016a88bd318a4824a0f75f5ef8fcf16e3b5c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3cb0a895413fc97b56e058c17797adee9877580988a453ec2f34b7b3c93bf9ef1f22d28811ab4f229e22a640a0986550ea204c83b8166c69b299610cdf2c92ba" - }, - { - "block_id": "0000016ca8336027f1808863af99916340d37fee", - "extensions": [], - "previous": "0000016b2d5b8ff86f5da126ecd3251805a556c3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4536eb1e2363cda66e9253ebd1bc1aec059798ff352bd547cb712d7c444c643653a8e573265b019e1a9a59a6e062d6a5b8b415ecab4ce2aa1cb72cbabfc51b23" - }, - { - "block_id": "0000016d7f39002546e7bd22a5207f752e858ace", - "extensions": [], - "previous": "0000016ca8336027f1808863af99916340d37fee", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f632e42d02b2eaefa38cd8ed79d64d4a8e0c30b8ed491e8356ab45e5b2b015abc70129a28eeb42767ef5ba8fda8dd9ae8871871d46dadc4073d7d0e33c78e15f0" - }, - { - "block_id": "0000016e7fdb492d82be47fc7342496eb52ca54c", - "extensions": [], - "previous": "0000016d7f39002546e7bd22a5207f752e858ace", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2d79f8c1a820b1c2558bdf09e29de4488f4b661d4796add55cd92c02eff0f3116191f5c4cefb25d936d490f35b791042c20895b10127d9d8bbc1bfa4fba5cbe7" - }, - { - "block_id": "0000016f316b1f2ccbc432be862c766554bab872", - "extensions": [], - "previous": "0000016e7fdb492d82be47fc7342496eb52ca54c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203077e0789f5d9ae0b13e3bd02b29545af489d6dde634dcccf6c75581bef9b51868ea27e5ce663e3600af28f23bbe9c6409b7c548f32c1837e5363bfef30e9c92" - }, - { - "block_id": "000001702a5f1a87c4f1a15a51917220cd45c5ac", - "extensions": [], - "previous": "0000016f316b1f2ccbc432be862c766554bab872", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f48a9350b7d1471d978ae72408c233403c010e61e7b16fa75ca361f87555b596c00cc220f9c7dfd23c490a976e045fcab6df01002b249170dff77024640d52792" - }, - { - "block_id": "000001714ecd8b0389511bdd803025affbca21d1", - "extensions": [], - "previous": "000001702a5f1a87c4f1a15a51917220cd45c5ac", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:23:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f27ccdcff8e0c3e584d5d3c777f4dbddb6e3c4904d08b432ab02c88a27bc1966173af07a7f104ba11d1804287d0d9254d6ba1a2f6df440c274c651c541f309452" - }, - { - "block_id": "00000172bf26d43fa228090a0b890c8a5996a61b", - "extensions": [], - "previous": "000001714ecd8b0389511bdd803025affbca21d1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f44cf5d852534c83cbf87f48489e25350062b1212216202ea168fc7eba21f65095fcd89dec847b413d85c44eb3883215678e35245ae5f8d77a87ac3d122a2fe56" - }, - { - "block_id": "00000173a461dfabd5d672225f7cd88c6fbcb60b", - "extensions": [], - "previous": "00000172bf26d43fa228090a0b890c8a5996a61b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f18848b4f0cddf58c3bed75c62a701c7389a3de3d55e83a086b93f680e32b257b5fcc323cbe88a0932e473d9f1c1e4efd98590e5932de13d812f4e3392778a671" - }, - { - "block_id": "00000174f8f006ce924a02e48b447fe5ef63b3e9", - "extensions": [], - "previous": "00000173a461dfabd5d672225f7cd88c6fbcb60b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0494f3b2fe3e0a7399f2717f1f950911b474aa4cc01f1725cb72930d0a2711605cb99fbd7bdda876ad4447ab18bee7dc5cffa7a8df741611c674573a8db414ac" - }, - { - "block_id": "00000175818da68d024dabb5086065c0c84def64", - "extensions": [], - "previous": "00000174f8f006ce924a02e48b447fe5ef63b3e9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f14535a74c72c467988a665f00ceb9568f295075ac2ee6125cfdf237c46277cee1fb2c076a56dc93dcf7850d2fbc882e6d5d7a802f0ce9c2276b1de3036f4f848" - }, - { - "block_id": "00000176ce6090a325ea32d746ea46f436824fe1", - "extensions": [], - "previous": "00000175818da68d024dabb5086065c0c84def64", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20450c1b17d146dd950cea901d57c5f27a6a8639004911242da40235025c7bdd5b6120536487fc3b4d4a9253d927494b23fa0e789eee057cf75f191d600ed19e63" - }, - { - "block_id": "00000177e34b8ec81fab667530210189e97a1b84", - "extensions": [], - "previous": "00000176ce6090a325ea32d746ea46f436824fe1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f038905646680ed711ed2a3a16f5aac07f23565ebda45ee91b6b0e55ec7f9a6a4626715f38487e56ae0172f6ab527b5214f0e4f554d3aba2d46c28cb741cc56bf" - }, - { - "block_id": "00000178346ed379901fa09b5f048718f33bcbeb", - "extensions": [], - "previous": "00000177e34b8ec81fab667530210189e97a1b84", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f14d5ed0b5dc6916dd294e378534c6085730d67be54f61aba7e3603d580f0ee1a6e3a235ebd5ccbf08f7a74447c340236a75beaec6bc7e595cbf894d5cd8b7ec6" - }, - { - "block_id": "0000017900b3597c9bb40d05b9192da6ae0573be", - "extensions": [], - "previous": "00000178346ed379901fa09b5f048718f33bcbeb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20476599329722c669653d8c358adefd091adc0e8ac0e569b68c83a65d9270b2344aaada278c085316e6129761e8ee8c4ce7574a7ed639603a0898df6daddae6ac" - }, - { - "block_id": "0000017a03f870645d78e9f1190bdafe42438466", - "extensions": [], - "previous": "0000017900b3597c9bb40d05b9192da6ae0573be", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2009b014d15eb80ce66a159fffb01b7cdeccb3d6df25f6eecc5b70d57a85123a041a2a1c9a9fe72cff0e4e864275f9d11ef52ed12446dc50a8bd2cd8e46f9b8530" - }, - { - "block_id": "0000017b777b4355c97b3fd041e8ba06b3d29f92", - "extensions": [], - "previous": "0000017a03f870645d78e9f1190bdafe42438466", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6c83460473f699a4d6f7725902fa78f81dd51bcd77fb770e2d64af6013818fb17741f3f9062cf2bcd4df4cb9a6c95eb4841cf8aff6760693f49e779dabe51213" - }, - { - "block_id": "0000017c868e92386597d4e61f6e57d1ddbfea54", - "extensions": [], - "previous": "0000017b777b4355c97b3fd041e8ba06b3d29f92", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f094de26fa372ae88ccedee0b875a21b7dd84e3952630f0b95d53a757cccdc835325a261826b22655b0273fc4bded4295f1f90c1e3efa59f5ea7e03a19c56e414" - }, - { - "block_id": "0000017d936a9213b4816009f6de4a60fc565f31", - "extensions": [], - "previous": "0000017c868e92386597d4e61f6e57d1ddbfea54", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20304e7651970e5dd6c79be7a8a12b6f8e69b2283911168ecc252b0f9f4c386c8b73b6bdbc3ad396b484997b5400645ea16222be48c475bc4eae3328dd90f1da5a" - }, - { - "block_id": "0000017eea370006ca81dde00880335558b39795", - "extensions": [], - "previous": "0000017d936a9213b4816009f6de4a60fc565f31", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f308d56bc60b456082be19f4297716a7df97282bd16b4dfcaa31d722eb769b9712da04c93cb02e52d74b005f2b3a57e5adc81570baff487237d10e400d7c4062d" - }, - { - "block_id": "0000017f0799417ee99af7448a1e4e1ececc3e91", - "extensions": [], - "previous": "0000017eea370006ca81dde00880335558b39795", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2033d7f80d59a7e2545f65e2562abdac03a502af1b7c77a061d0ceade48ae2738e04497040957c5a93692d7b52f53e053ef422140d76ae39e4c979187b3cb2fbfb" - }, - { - "block_id": "00000180076146e1f5397dabf8fa80eac3b8f1c9", - "extensions": [], - "previous": "0000017f0799417ee99af7448a1e4e1ececc3e91", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200ea3c6c6eb2460a26389a313f8bbc8ae1b14bce50904d281df354adc68417b0830e864864f9a074c5b6a379e1b9aaecca9a55adccc4aa80c3fe4704667331095" - }, - { - "block_id": "00000181b6b3f62e8b1269805aad97fa4b0fdfe8", - "extensions": [], - "previous": "00000180076146e1f5397dabf8fa80eac3b8f1c9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0cd6973bf38680dfd5f607bd5b9302b4c7750cb1c44ab3845d3aea7cce34fa3363ff43caf9faf3b8a5c2e18a631e31392507beb04ec85b5ee42226e22ce4bbcf" - }, - { - "block_id": "000001829268887804e2b47b379d516ec50eed9d", - "extensions": [], - "previous": "00000181b6b3f62e8b1269805aad97fa4b0fdfe8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20603f24adaeda35dace606c153bae57af23d536b853372c7b520b33056d8a0e523a393c232a6e040f502b2ec447436f8b55264b940b5cff219acf27cca85d15ad" - }, - { - "block_id": "00000183c40d8d8bda09f4da52672c04d434ae99", - "extensions": [], - "previous": "000001829268887804e2b47b379d516ec50eed9d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f30f2603071265ea01e31c1291bdf525df58a7ec99db8d951991e52998a37806367bc286aec8c85e278e50e7d0489eacbe71c78f6c7b34c8f282b54cb0c6fd163" - }, - { - "block_id": "00000184a9982ac2ecf8fffbcbbcb26d9dffa8b8", - "extensions": [], - "previous": "00000183c40d8d8bda09f4da52672c04d434ae99", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1458296705b0c9fee666cd14570315e01c881a1ace4b39b415d16f652992d3780e299412ffffa5ec5ef9cbcd780366e140fad92f5d953bf19c818c29bc17b79d" - }, - { - "block_id": "000001859d9b1ec29c38ccd2ced491e99e3be205", - "extensions": [], - "previous": "00000184a9982ac2ecf8fffbcbbcb26d9dffa8b8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:24:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20774311da6e30b80b7d786b19407473b0dc00a3da191b539cbc23681402278b876c6c0b827191a31f5ac6aff20ebd99985e2eb034617b57c48d3ba56926467230" - }, - { - "block_id": "000001862a5e2bac97e0cef54e6f94dd2f096537", - "extensions": [], - "previous": "000001859d9b1ec29c38ccd2ced491e99e3be205", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f02b2866bd548f60534e21af957cdceb348830874bb1b5b10e0fb3a3d5ca756436bdc4cfa3346f766be5025c6996dc4c9901ff81cb4aec8853681150bbbd788e6" - }, - { - "block_id": "0000018787c665357b827ffb0817d9aaa6e4e8a4", - "extensions": [], - "previous": "000001862a5e2bac97e0cef54e6f94dd2f096537", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2d26efcbe1b20f7c05e2e9649f1de6d312b9eb00c3d7c6b6f0dc8918b41406ba3992c954de0ca1a275ae612d066c459730c64101a2ca0a91f8a38d0f7cd82818" - }, - { - "block_id": "00000188195b7d55bfa3586664084efe552cbb5e", - "extensions": [], - "previous": "0000018787c665357b827ffb0817d9aaa6e4e8a4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6ce6dcdbe64b32187cc44641db1ec691bb850b0eaa9fa1cc09ca860ba6d3d86d298e63a87bbcf4875293dcc9d501d68326a70d4ecb95c415e354235cb0ce7b4c" - }, - { - "block_id": "00000189132fcd87cff6181345d5fa227e161fb3", - "extensions": [], - "previous": "00000188195b7d55bfa3586664084efe552cbb5e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f59748a8032d97f0cb2f554f250109397e0c240fa6127eb295f7970aa529d43ec4ffd3daa69840da38461d719ac123bfa514bd08ab29f486615183bbdb4fa7aa3" - }, - { - "block_id": "0000018adc64fee170bbd2a002a22b98684d80c6", - "extensions": [], - "previous": "00000189132fcd87cff6181345d5fa227e161fb3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f03c7c4a4d4929b2e96708269076147f6367f23d493072f46201d73376d6a2a377cae619cb339daf5f9de5e52dfa6fde026c5226c277094f508a8ec0cf641f492" - }, - { - "block_id": "0000018bfcb93eb5f732680a6336204df3d06e37", - "extensions": [], - "previous": "0000018adc64fee170bbd2a002a22b98684d80c6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1d5013ecf29b449348695152305317704160cddaf69204738f8970ee1bd04ab77a04f610b6bc9224b9171992a9e2bbe8133e1118cdc65c1891f16baa66ae2688" - }, - { - "block_id": "0000018c7bcc27b94d4e4129c490ff0b7354c2a8", - "extensions": [], - "previous": "0000018bfcb93eb5f732680a6336204df3d06e37", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207f3430cacfe6d979b1c5a4d4bb9df1d05d1a9309ba687f5fb2e8e326734b4af57622a7a132ccb64bdcd11ffbd190b4abad36efcb5081ed0bdd360dfdb3cae73e" - }, - { - "block_id": "0000018dd9a542eb0f55896ae9b0504fb506ff6e", - "extensions": [], - "previous": "0000018c7bcc27b94d4e4129c490ff0b7354c2a8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2034610a05dc27ff3864ccd3956146eaad69b33938f9c782dd5ec08c4d552725942c36455eef678011146db2f44fe4effc1d6e67945e150d16e3800239216f5487" - }, - { - "block_id": "0000018e1ae22be5391b415d2c720f492389450c", - "extensions": [], - "previous": "0000018dd9a542eb0f55896ae9b0504fb506ff6e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3bd84ba365e46295cff7861e3d5eef7e24d09883fbafa23cd7d6d34de27dd7933a3e0fc32105ba1147c84d1d7d7641e8af89879853a2d7f8c215dbbe6de21fea" - }, - { - "block_id": "0000018f43552fa1a380af5706a3aa2058e66137", - "extensions": [], - "previous": "0000018e1ae22be5391b415d2c720f492389450c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200ac07a99385e01f0b8a356db399ecd3ca9db7faf14279ff49022f6008ed4fe4251bf647c4ce4eef404793608a5c933c7768b311bf0b130688dac99650ef396f8" - }, - { - "block_id": "000001900c637d6fda9e8b18fe7cf4f612feec43", - "extensions": [], - "previous": "0000018f43552fa1a380af5706a3aa2058e66137", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2c5fcb9ca261392f27de3301cebd229d2c4eed7944f5c7236c78935fa37ff0c65a1047ecb4ffe82b1fafe4f0c5ba504cb7f69bbef8e72c378bbd4a053c4d7f3f" - }, - { - "block_id": "00000191e097c86210ecf3cf16b9fcb0cfca7d82", - "extensions": [], - "previous": "000001900c637d6fda9e8b18fe7cf4f612feec43", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201f03d105121f24a89594a17f7b0c5f72f62cdc84b26a9691112d6f02a46a917545076d8aa1081ed59fb1d2c37bb4da8c2c7e972e5c564fba9bdd728f9a76e7d3" - }, - { - "block_id": "00000192ba5e888b503e9abb6887bdfaee87ba2b", - "extensions": [], - "previous": "00000191e097c86210ecf3cf16b9fcb0cfca7d82", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5c19a4b2894707446ba5955589923351a8c3fe437da4a5671ddd9af30797cb524f5cdef16fc1457973823b53ab93a3826dfa69e2c9ae1f99a4720c6d78bcc763" - }, - { - "block_id": "0000019316590295e71c4cda1890b9b1701b1330", - "extensions": [], - "previous": "00000192ba5e888b503e9abb6887bdfaee87ba2b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5f7b9c90fe87e648d7962cfb019d0e66f0d13603cda7584fafae9b1ac17029e534558456028b723a70937ce4c1ec3601a6c49350e877fefd736c46cc7e5b6221" - }, - { - "block_id": "00000194dd4bcd07d333220e6497fa885f967c0e", - "extensions": [], - "previous": "0000019316590295e71c4cda1890b9b1701b1330", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f44698b74d3f5884a97d330b9dbdb701cf11320803d5a72e4e357692d6103f9dd4149b66a5b5a6e5c3017aa4db06e9ad3e441f5c2b660aad7505afa4840e399a9" - }, - { - "block_id": "00000195cdf709c539ce67d19b1470ecc7260dd6", - "extensions": [], - "previous": "00000194dd4bcd07d333220e6497fa885f967c0e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2052710bb44ebbdc201976873598ca08c2aece1ef59e1e29ea2c7a55ce7148e6d611381242af71df2c0de6cf878b619c0d2d16c7af66c4e4641d36aac331041da3" - }, - { - "block_id": "00000196451ced7c1af1ff2d89f117b5a2d2b151", - "extensions": [], - "previous": "00000195cdf709c539ce67d19b1470ecc7260dd6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20111a3c3dc0aa858b49235d30f90daca4a88d0194f4eb8b348bad4e6e12155be94651fbcff4c50d78e087b8de1c83ed06ea6b1cc54815142e79cc8f1a3981d480" - }, - { - "block_id": "00000197d9c7c9e52ca937bc4c4d79820462af51", - "extensions": [], - "previous": "00000196451ced7c1af1ff2d89f117b5a2d2b151", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f60700bc0243ac6439267f0b55ec7b59a2f90cd30f4ba6dff428add8cf71893cf1514cb5dd922efd6e34f478fee0cad8e9b6b125fa6fb547cab00a8824379adb6" - }, - { - "block_id": "000001984f5efb12055e535afb43de8a1f05222d", - "extensions": [], - "previous": "00000197d9c7c9e52ca937bc4c4d79820462af51", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207b6eb2b50b9210133aaafc0f5fcb8ae3aead327b9f7266300d24af8cd288aca54c84a74342919b85d300d7f0f7275520a9d46b1e8a66210f652ad530717072d2" - }, - { - "block_id": "00000199597049a6d09a9fd74a629e3059bae403", - "extensions": [], - "previous": "000001984f5efb12055e535afb43de8a1f05222d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:25:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20773ff19bb68b9569f2aad1c13dcb285cb3d24ea29af6f78691c493cc683edfca46d385efa604070405bc24f69a29cedaf129eabebe4c1632d630f61594324891" - }, - { - "block_id": "0000019aafdf2da57df0b7f27c731581f436961c", - "extensions": [], - "previous": "00000199597049a6d09a9fd74a629e3059bae403", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2065a714526fb83efde1db9e72b4838f0033765c9c32c965f7dfa8522e3869b0d31bc010e3455ffd09845c5759ea69ca51ebecb07ab3c7a0d675a7808939812b7b" - }, - { - "block_id": "0000019b2d1f3f09e2c14326860d5840782fdd84", - "extensions": [], - "previous": "0000019aafdf2da57df0b7f27c731581f436961c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f06e91474df4ff447192cab301acb259f3c6aa2c675694ca2dd4892a82073bf3a709a4f91a6d4c938398de4e3f924f430853db020b3283c650f14f708ddc954f0" - }, - { - "block_id": "0000019c21ffc672555131a30fa98485f14ebeea", - "extensions": [], - "previous": "0000019b2d1f3f09e2c14326860d5840782fdd84", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205b60a2793d08679b75d3a80695e3bcc5d8697f6d2ebf81885a637dad03a4d14a756ce7f4eac4dd71618ac6c98eeb78e6d0c54867729eb1e9daeebce090227794" - }, - { - "block_id": "0000019d5fd37fc7132aeb00812d8beee7f0de7a", - "extensions": [], - "previous": "0000019c21ffc672555131a30fa98485f14ebeea", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3bc858fcb176034c4b369904f83133ae4a9a47362b86cb8b2d04fec84a8a528f5a431376ccf9766a386a3ae854a996ef6c951c04ab57f394c03cde661aedd5c0" - }, - { - "block_id": "0000019efd15e8d7cc8daf9c8ace72c8c429ad92", - "extensions": [], - "previous": "0000019d5fd37fc7132aeb00812d8beee7f0de7a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5cc81c322db6db9f79e42c084e6b32c223fecc3820ae650fa3946be23512183e631212080731f27576fd00816356c16c059b3e04e6db2bc17ead9bddf3fbe123" - }, - { - "block_id": "0000019feb5cc8457145948fa7447434b4e671ea", - "extensions": [], - "previous": "0000019efd15e8d7cc8daf9c8ace72c8c429ad92", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2043bb15f4e7a99530739958783a8b2d930d01beb76d5561b41a4d132a41d0c9516c09c2773e52e4340162dc00160ceadb7cbe06217c2e6cae0122d43a5bc0dd1f" - }, - { - "block_id": "000001a0571cedb4fc88b67fb88fc4c9b5cf75ad", - "extensions": [], - "previous": "0000019feb5cc8457145948fa7447434b4e671ea", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f07ff0d89912ee46ca4b19661e131a4f0da0d147fd792963e17f2afb6cce1c2953b6929083c7ff775b7d890557f6e17a070954c5b1faafa67de0ecbd07204bf62" - }, - { - "block_id": "000001a17723fcd80dba721a40dc36c5dc9d69aa", - "extensions": [], - "previous": "000001a0571cedb4fc88b67fb88fc4c9b5cf75ad", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2056d46d16551f2d8260a02c2b26cefc942cac90df5fc7b10722ebc5d9f2b2e7d735246aabb56ec613e1c6342ab99b966e2ff7453c800c4b41e21bde209117255d" - }, - { - "block_id": "000001a2b34c6830361710620bdde1bfccbc566b", - "extensions": [], - "previous": "000001a17723fcd80dba721a40dc36c5dc9d69aa", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202f083464ec92cd1333ef41f0ef800e6e864d53ac132964dfc3ab7b0d3482927f31504062a2341d7cb45fd44be313ca13e8100ec2d1ace8bf1479dc18a6d4d95e" - }, - { - "block_id": "000001a3d4f452219395fe7e55ca20e510256f24", - "extensions": [], - "previous": "000001a2b34c6830361710620bdde1bfccbc566b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207b36cad0451e59d2c14c677fa4198a7c2beef071effa3e3e2e7f1e0d4aab80ea7a92c8b638766f45b51c642799e6ff5e4643fdb9e5f22053e360f8492ad44ecb" - }, - { - "block_id": "000001a46342fe73ecf4f518c3a7155972ebc839", - "extensions": [], - "previous": "000001a3d4f452219395fe7e55ca20e510256f24", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f66402cee33c6a5e202c7542736ba78dfdf24d425c0580ee81e43afbd7b46456b286b189edaa48e3fb38b141f06032c46d5942f8b4640a47d610245febec3a3a7" - }, - { - "block_id": "000001a568fd3dc97582092f182a2d7ecfd02e29", - "extensions": [], - "previous": "000001a46342fe73ecf4f518c3a7155972ebc839", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f444d0446c0d78a97a04bca903b80344a62119b31c4077c8a1964366c6790dddb1480a1ff00524e7e259cb6e0825ead378bc47809813808f1084eed47608da6cf" - }, - { - "block_id": "000001a6ac697188702a04e6bc0aab8842390a20", - "extensions": [], - "previous": "000001a568fd3dc97582092f182a2d7ecfd02e29", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2031a1a02165289a637c5227febf2c5173af74746b3df3498931cfef4382331c173c588bdc10f10e330a2d26e5c1ce3a30b5efc5e06d37cd8bc616a1dd4eed6f59" - }, - { - "block_id": "000001a7a1939a7483125cfbc07ca22e404ba8c3", - "extensions": [], - "previous": "000001a6ac697188702a04e6bc0aab8842390a20", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f535b534e3ee9d3f506b8e333e644cad6514560d7ebb52104d2c6dbd3f0634ad05f431316506231be02205ea00aa65879de1e6c2ecd1c1b9e311000c8c9d15dc5" - }, - { - "block_id": "000001a89bc98f70e1a8abf7c399773a85b6d9b8", - "extensions": [], - "previous": "000001a7a1939a7483125cfbc07ca22e404ba8c3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201871b2fb5de1f03077c468e3e89a5207b88da701e61838668a0640d73259f23458a3f0280367cb787b6fa2db020e18c7b32ba0997ff9041396e5ffc87fa830d9" - }, - { - "block_id": "000001a90955db0d8b29427367a7ab0fdd76d8c1", - "extensions": [], - "previous": "000001a89bc98f70e1a8abf7c399773a85b6d9b8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205ab2a22dcfb97387f589c0e5888b83103d106f8c36860c76ee603bf7fae59f505ac2b419d2320824336c82fdff4c81a9c4597322dd1c089f32ea03b93fb899b0" - }, - { - "block_id": "000001aa84be2cdbbfd7a85b46533a6787cfc62d", - "extensions": [], - "previous": "000001a90955db0d8b29427367a7ab0fdd76d8c1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2006853200fe63a4f8851e80fe6307e818264ec1ccea5a8d87d48d68fe4bdab96c311ec4d46f00616dd81860def2c99fc813fa882aa3056ea012b9487e211d4136" - }, - { - "block_id": "000001ab815bb3eb1e4cdc6f0534b5c6512272ae", - "extensions": [], - "previous": "000001aa84be2cdbbfd7a85b46533a6787cfc62d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203384d9b1dcea28d6a948ca4242d6b914acca2a8d83c7d0f1c216ad97e60c78de15162b73341aeebd51773952b78a690939987d34799047cc30ac74e5b69b7c72" - }, - { - "block_id": "000001ac071d837afb5a47df0b12236a7fb3e782", - "extensions": [], - "previous": "000001ab815bb3eb1e4cdc6f0534b5c6512272ae", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f70932c35f01e19ebb4822d8e82c1287d8573c7523a67144627fba36b40b6f01660dacbe0020b3b5a96cff3c1b6be6a206e06a9568dbc62081ee6f00ac83eb874" - }, - { - "block_id": "000001adf4b1966ecf8d80065b1a83654908f3a4", - "extensions": [], - "previous": "000001ac071d837afb5a47df0b12236a7fb3e782", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:26:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f16ee7871b1ce59d60392a5aec7f8ff752a9a1be36c110440772372a05ea4bc2569838344f35ebcbf33aa684f0a763b262f547a3bb4ab39cb1212c35ecbde6644" - }, - { - "block_id": "000001ae67751ece460403af50f2a09d37a4a2c0", - "extensions": [], - "previous": "000001adf4b1966ecf8d80065b1a83654908f3a4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5018576eb01921b919b189e2171734359f4fbd84d86f20f9abdf151a026ea1ac7a1d2fc5d90d53212ed7a29a142dc127e422289d5d40f256a6474220cf0c3269" - }, - { - "block_id": "000001af18a1fff0177c3dd7074bb555c2535e57", - "extensions": [], - "previous": "000001ae67751ece460403af50f2a09d37a4a2c0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202182896f007450a6d8c6e68ada388ebd60197fdd3a5f51b92fbbfbac18f8b1704d120797c5532e7da9a5a4a8745861f54f1e7fb7bd6c31ab3597df557004e9fd" - }, - { - "block_id": "000001b0ff56ace7c988a778481b767f9bd044e6", - "extensions": [], - "previous": "000001af18a1fff0177c3dd7074bb555c2535e57", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f434209cf3908584728a441a21223f838a6fb48749bdfc95a06f48f2e901ff0880c91e01c39770c079aabea26eaa75943dae349ed834b373e303da0c0f1bcef42" - }, - { - "block_id": "000001b1074fcd44c3c042d29b79c4d6d1ad007e", - "extensions": [], - "previous": "000001b0ff56ace7c988a778481b767f9bd044e6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206da336c433f5661dcde9ec7b300c0f622b660eaa795e40efa22baa697c3f3eb762218bb73fb30760e79ab9c2d99cffd9e24fa9e55921b22a527ecf4be08131a1" - }, - { - "block_id": "000001b275ee5e01ac155689ef2e1a828a6fcddb", - "extensions": [], - "previous": "000001b1074fcd44c3c042d29b79c4d6d1ad007e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204eccf85d78e18caafef04d89d1807e979a2fb91a3293ad1828902a215b38d13c06c190b4ab944101516cc4dbf2e80c274e9c459afc185e17fe07bc0e0806c8db" - }, - { - "block_id": "000001b31e34fe22bde72d8ed6bf733397150c48", - "extensions": [], - "previous": "000001b275ee5e01ac155689ef2e1a828a6fcddb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2035ce26f9285368fc5ff3a84ae356db1314caaeddec324fe1c3bd5cc14b0d70e8266082f485fc426b54a58989534a4753b9158e2681ac0f4a7bf484560e4149bf" - }, - { - "block_id": "000001b4624f06508299b04bca0b32d033054a79", - "extensions": [], - "previous": "000001b31e34fe22bde72d8ed6bf733397150c48", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f192339732c763aa662527198489e110c5684418d0a59177d8673edce6fa38e0063b2ba245a92fa43aba2790a2a7fdbff17d9ecb824e5259c9d86e9e4691e1be1" - }, - { - "block_id": "000001b57543a01813e0d27c5c0065190a456d30", - "extensions": [], - "previous": "000001b4624f06508299b04bca0b32d033054a79", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205184166e32a9ed0c7a65f01e507ad3917a0b4db760371d2cfda69524f37284de30f13550a28b02b4e3d866b9500fabb1cb9707cfcc4fae171a5e1c5b238fb4e7" - }, - { - "block_id": "000001b6314a6da6e056a646ba55d23d2be48a64", - "extensions": [], - "previous": "000001b57543a01813e0d27c5c0065190a456d30", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f67a28d14f8c108b47b6adc6afda400bf20bb978dfdcdc0ea11f0a50958fcc537733e6e1d6fc2d6cbd5479d564c600a5454d5f684e5aaac254c3c26e491d36166" - }, - { - "block_id": "000001b7f419c302fa7a0b6d548f109da66ebffc", - "extensions": [], - "previous": "000001b6314a6da6e056a646ba55d23d2be48a64", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20158efd4de780e03c860ec4d68630324cf22c228f34e758c7b88b699ff45d3ab10714eaa96da639432c127693977d61cbbca88a518711afefdef67b6ba048afbf" - }, - { - "block_id": "000001b8a85e517d670a97fdfc6d3c96b809a7af", - "extensions": [], - "previous": "000001b7f419c302fa7a0b6d548f109da66ebffc", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6fb1b1f651359beedc5e958a4afd582fea6f029935ea504d6ded86f0aed2a2ef06b9990e7b5ec32e4c5c59fe03c5ab59bb352c175551dad1b5d3945c3dc76922" - }, - { - "block_id": "000001b9954b97575f44b4956d705fcbaccd19f1", - "extensions": [], - "previous": "000001b8a85e517d670a97fdfc6d3c96b809a7af", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2035da7b945d7b32f135182e4995c1feae8708701249051ed2d860ebceb5ff6e921ce9ef415a9db2efc6014c9fdf2a7eeeed14bc9ba2dcb618d3e8c14bade3a2c5" - }, - { - "block_id": "000001bac4ead249f1733e08d6180540b93e5687", - "extensions": [], - "previous": "000001b9954b97575f44b4956d705fcbaccd19f1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2004797ab5c934a64f728f7a3033b896a4c3b417f3e435dbf8cc6d3e4df396eeae7a51cc952130b03024d8fcd867c17dff84ea004bcded4d5d66a626119168503d" - }, - { - "block_id": "000001bb5340deebded571d7e2f086871d0fc98a", - "extensions": [], - "previous": "000001bac4ead249f1733e08d6180540b93e5687", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7f5e0543e7af91e895ddec64266f5c90cb337572d0b41f4a96fefc596f9490f65037f85318d94b94b9517af4005fe4f0fa3ad71bbbd3447cf7ca3d1f76601bbb" - }, - { - "block_id": "000001bcf554c9a05289295fa720c5566ae1f942", - "extensions": [], - "previous": "000001bb5340deebded571d7e2f086871d0fc98a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206eeed05293f0b688febe29f278bdb1c9f0098a689c22748bc264f0943913bc1c514d8bc92c519a7a6e57cc821d6720d29cbca9d71853f3eeddae3336015c5249" - }, - { - "block_id": "000001bd656f2898668b1bc486b559ff480bd2d5", - "extensions": [], - "previous": "000001bcf554c9a05289295fa720c5566ae1f942", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20589220907e8fd32099b0fa53cf941216a1befd654d81f9f1e969a540e597fae9627da5d0a6e30ed04ea1918fa416510a76e211b6dda3e5c8d6a12437e0cde297" - }, - { - "block_id": "000001bec5864472e19ad33386b5328683188018", - "extensions": [], - "previous": "000001bd656f2898668b1bc486b559ff480bd2d5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2044083f6e51f8dc7c884c638c112144b6a466dcc6ecf9d88e683d1a675ffb4a064c19be2d44604429498629d9ac5a93a9cafb0ae89b663c599ca618ff5a6c1290" - }, - { - "block_id": "000001bff1c3932894acee155643b6f200921300", - "extensions": [], - "previous": "000001bec5864472e19ad33386b5328683188018", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206712301008a5486f2b5e6b8caf86d230f7ba3f1c07597f83dc6004e836496bd454f9938a753efa14ab2af7a4370e2707226e70a22ab76fee0c6231daf4d8d02a" - }, - { - "block_id": "000001c037b727cc16dd734de1aa46a11bcdb162", - "extensions": [], - "previous": "000001bff1c3932894acee155643b6f200921300", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206ae61657cf6ce34bc82b8388ccfb1630eeb346bc6073108637f2f1ac9c4632191a60bcc5ae2d0ca3641a85d4c232016fe958ede2f9dea429a496102e74941348" - }, - { - "block_id": "000001c1de30bf0bd9b277bbddd87bf832efed11", - "extensions": [], - "previous": "000001c037b727cc16dd734de1aa46a11bcdb162", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:27:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200797856742dcc65ec9ca90e2802ae72531514619e35d67b2564070f508616c421d49dd58970deddd059576796cc586fbec73325e787b02c13750730755a5d93b" - }, - { - "block_id": "000001c2040fce9d0e41aa7ed7ceef997981101a", - "extensions": [], - "previous": "000001c1de30bf0bd9b277bbddd87bf832efed11", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7f60c2e3cddaed24d7944f6f28cc4705ca7002e2c99882c7f622ecf66efa68911702be303a5b7df46be267064ec215f4f6289ce84fa45c5090ee77f9bd7f4c51" - }, - { - "block_id": "000001c31136bc64d45bb143898b8699b7f2a82d", - "extensions": [], - "previous": "000001c2040fce9d0e41aa7ed7ceef997981101a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0f05b7f7710824b60220ef9695ea499510084298ad7e5adc24581aecaac9a4712ae0343f229e9ecaba08fda0b21d5aea0dddaab2cbf3d5dd959481b851a43959" - }, - { - "block_id": "000001c4e1d7b3d14070ecc8f0ba0fb9fb177b2e", - "extensions": [], - "previous": "000001c31136bc64d45bb143898b8699b7f2a82d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5be8a66103906f8db1750eaac31de2f4394306a3dc2495a1df306312f6cc8a510d6781ce7d46543aea8afeaeaf4ed964c84435e8312b4b0e984ff28b9a06f481" - }, - { - "block_id": "000001c56a150b3a19d220a44fbbea5a684e6290", - "extensions": [], - "previous": "000001c4e1d7b3d14070ecc8f0ba0fb9fb177b2e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20599b734e86f47f3151acf0b766acdd03cb38e3e941393db6e3c7fb76eb9168897a8b25d862d030267ba07e6235c3d3e71630ebcab41d78080422ed8366ae0ba9" - }, - { - "block_id": "000001c63a478e6500ebcdd159fd32a4673eeec4", - "extensions": [], - "previous": "000001c56a150b3a19d220a44fbbea5a684e6290", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2042d3de442e9eb69aeb1368ffd728efa0d765fa8be17fa3d5ee0c9f8b42232eb0311c523adc714c3a7983216eb4b163a07a6ffaea79f69af597aff3a8eeed8230" - }, - { - "block_id": "000001c71fcabb80b300cc40664ea56db9ff70d3", - "extensions": [], - "previous": "000001c63a478e6500ebcdd159fd32a4673eeec4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204105b66d2aa9a62ad4febd95042b58bcbb75b122960325df10c76a7b158d76c45d4aa31cb84dc1cffbd4acc93356b9ec26254c03e09b4a51d87339d344cde423" - }, - { - "block_id": "000001c8fe77b299441a1fa60cd0993c81ec901e", - "extensions": [], - "previous": "000001c71fcabb80b300cc40664ea56db9ff70d3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2078108d4f85d8996623a2eebe6e1218ea1a9db3dcbd0f17a23ea225315a8eb65471fa2b498caef5b9d5418bc7cad6c5f95f036f2e6378792bcbd2c64d414e7bcf" - }, - { - "block_id": "000001c9c7da4861b8af0437b573c6d10b52ed03", - "extensions": [], - "previous": "000001c8fe77b299441a1fa60cd0993c81ec901e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206bad982eba31d8648c90a06ecceea967e8666b0275d416de60e177d7d12aa7695a4f0777f94c4f21a8a1b6ec447ea3615298f2cbaa6c0e326772bf6f84401e97" - }, - { - "block_id": "000001ca5f643ae9dcb38a780cd01b454cfb13b5", - "extensions": [], - "previous": "000001c9c7da4861b8af0437b573c6d10b52ed03", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2053040073e802430319c68ad9897cd68475cc8db142034a3764aecf4984d5a3805894161ff35acb033474ad2512e181276f491ec7cef27e00bd3a99407516e6cb" - }, - { - "block_id": "000001cb7ef4c4c583285d588f0c62ef1845bdf3", - "extensions": [], - "previous": "000001ca5f643ae9dcb38a780cd01b454cfb13b5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202ed1a5531f92a57ba4b2344101ed5bf10fb6eafcecc29417e94cfee03f75e3f939ed2a5adafdf50745cefecce39b66c4c2041c24b9c5ffb0bb4a4216bf363d7c" - }, - { - "block_id": "000001ccef02174eda53cd0230bf59e495967f9b", - "extensions": [], - "previous": "000001cb7ef4c4c583285d588f0c62ef1845bdf3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2054b2830dcbb3de0c69306217f9594f5a4fa076ec13eb2de4269670a8b4d6612b3cbb03606543b91ad680d298364b8d6312b5e0ae2a84bb51dc6269490d904e07" - }, - { - "block_id": "000001cd97948b316c328cd0f0bfea8b72dcfbe2", - "extensions": [], - "previous": "000001ccef02174eda53cd0230bf59e495967f9b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f53183770ad57cb9aa030ffd8fd8663fc2e95344885e8d61a7e1666d41e9713b61903194b86f11cf36539d3e781709b479c20b79ecf77abecb8d933e92c538e70" - }, - { - "block_id": "000001ce153d9c1e24565ce07fe9b7e6e76f78db", - "extensions": [], - "previous": "000001cd97948b316c328cd0f0bfea8b72dcfbe2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2074281190cd46a6aac192837df6b241f403112e5c727cc26fc08409aa2e4be28b11010eeafd150b0c21d32adb4fc224bbe33e0515143875c4de5a762f38b7ab76" - }, - { - "block_id": "000001cf2cd4a224df48e72cb7380b290971b656", - "extensions": [], - "previous": "000001ce153d9c1e24565ce07fe9b7e6e76f78db", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f12debfbe31cd2538998110915fc4bf242c3c8121a805382975a7c5a8ee8991e9353394f07653cd036d79a60880f9d8b49ea3cd3a2177eec0cd4e042608208ccc" - }, - { - "block_id": "000001d0317bb8fdb38ec9b39addd065ff730895", - "extensions": [], - "previous": "000001cf2cd4a224df48e72cb7380b290971b656", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205d289797ad51876ec068956946f00f7bdbfba5daa56a38dc69f9e90645af1bb86b81a99e97010aee5369f767d8031b3ff2a692772b24572cdb55d78c3f3ee877" - }, - { - "block_id": "000001d12ca918a38ad8fa5fabc8a7326cbc48cd", - "extensions": [], - "previous": "000001d0317bb8fdb38ec9b39addd065ff730895", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2079698ffaa936817dde2fee6db69e7a66679e44fe7525cbcc1e0a641932e4c6411a81bac79927b83ec70c07c2e24433ca434cbc69c674e6d09369498bd1ad52f8" - }, - { - "block_id": "000001d2abf969b9e2f6a799f12c08ef4c2f3705", - "extensions": [], - "previous": "000001d12ca918a38ad8fa5fabc8a7326cbc48cd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2006c9014c522a076829fcc3a69e6b38a9454ac74f77bdae3b8860c337e81507cb7f07fe4e05d65daf9417dcf2cec4bc7a62c3ea0ee4dbd52f758040ce091ebbcc" - }, - { - "block_id": "000001d3593888475f37c9946ca844de45757aa4", - "extensions": [], - "previous": "000001d2abf969b9e2f6a799f12c08ef4c2f3705", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f08df56e6d10bb08c9fc18362e7488e1093cb8889451d7cccb67cd3b633119cc4781bc785ff73d55d80288e3b842e51cb0fa265b213cd7e247855dcff2a102316" - }, - { - "block_id": "000001d43ee4bd0c837df7b1e4c5062f236b5cf6", - "extensions": [], - "previous": "000001d3593888475f37c9946ca844de45757aa4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204980800879013b8996b647ef9fcb3dceb04d9c90bc741420613d2c84a34b381e044355459de2954c910bf4915ad4868938d942c5b385933b67bec4d99f63ff00" - }, - { - "block_id": "000001d559f2ce566aba3ca04c75277d001208a2", - "extensions": [], - "previous": "000001d43ee4bd0c837df7b1e4c5062f236b5cf6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:28:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7746e1e57a3447f4170d08764299e109081b28678c0c3f349ea72422b7e64d22556abd39dfa64aec0238ab5c87479e8f31c7648903a30be7d54b4062e4a49335" - }, - { - "block_id": "000001d60433e28de035ee56a14eb7b01f9c6fb7", - "extensions": [], - "previous": "000001d559f2ce566aba3ca04c75277d001208a2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f24eb0e2408eb5f72b025de8461fab831e81547986695d005392e3999050e2c151d988ea42c522197430afd7c4c5af4d85a25f80b2d964ee616fe26de9b7b4fa6" - }, - { - "block_id": "000001d7ac74499d79f2506c0eefbd77f0845d65", - "extensions": [], - "previous": "000001d60433e28de035ee56a14eb7b01f9c6fb7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f40cbfa2651b0f4188438681377dfdf960ac6ebab75135d468c11a95080caef9f5d68d42e0f58177748405c0c220c555e4bab21872d3cdf9b290c7e37d579f28b" - }, - { - "block_id": "000001d815ae33accb5b4a1dbd303bfecd1d4ea9", - "extensions": [], - "previous": "000001d7ac74499d79f2506c0eefbd77f0845d65", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f172d4fb31326a3cc7d50b71b99785b218c6c8ebe0764e511278c150de3438e071e6134b571a33eaf059b3b92d7ba3c1dc1d7527dbaf389141335ae4eed6ad52c" - }, - { - "block_id": "000001d9e8a67f502ddb10295d7a12f744a3beed", - "extensions": [], - "previous": "000001d815ae33accb5b4a1dbd303bfecd1d4ea9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5c10d58d02d06d901c8c930e221d3fb2b69f50d055b5de8f87a2c1bb0d94721b48b3ab8127231ef2ef783b8094b89a0465765609ab127ca8debd8923f32b69d4" - }, - { - "block_id": "000001da02a9c7e3ecf8040f9f6958a74e04b180", - "extensions": [], - "previous": "000001d9e8a67f502ddb10295d7a12f744a3beed", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3b32e3179475bf17bdf4f0791cb37a9ad7b17c6aa429fbae502980b68a860f48082286928be9ef4791839f4018510a2668316a013ec547f9250e762e34602961" - }, - { - "block_id": "000001db1e7ee5b2a2b8373060214f832210d809", - "extensions": [], - "previous": "000001da02a9c7e3ecf8040f9f6958a74e04b180", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f639bef3f892d263ccdfea55d8d246f46dd67085559cf07030dde63d633315b5d57645e1bb37e5b069b3548d80ba5f9e140d74aa21d01e3e28e8d7a410d67ec3e" - }, - { - "block_id": "000001dcb07a94903e9f98290a151f745d772197", - "extensions": [], - "previous": "000001db1e7ee5b2a2b8373060214f832210d809", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20705b408b167033f70bd1376d0ab61a9074403ff3921126599ae3feb5ca38e2754cf8e70a051d31dc6323e2430d998b16ad0908bb4d406d1ea863304268c5be82" - }, - { - "block_id": "000001dd8910d30d5db3de5f13fcbd099a9057dc", - "extensions": [], - "previous": "000001dcb07a94903e9f98290a151f745d772197", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2b44983378589d6829a4ca1ce5af26d0f0c2958dc10ff5d838e2456dae477a2a160fe20c15306a414a436bbe0e140a1eebff2bf25b5c5df851f16897106e8223" - }, - { - "block_id": "000001de10c49630452f583af17fccca43d4c9e7", - "extensions": [], - "previous": "000001dd8910d30d5db3de5f13fcbd099a9057dc", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0fc64f57c3b47a788273c3c2f23819412d1d906c4c225513163219af7cf9148b5c448470854699f5d023fbec6b94435c26348901826e82fee788e17d9d767388" - }, - { - "block_id": "000001dfd7928c0a02565aba82157957388d0634", - "extensions": [], - "previous": "000001de10c49630452f583af17fccca43d4c9e7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2011446a0092c004cd143b4f2e23baeac729cbaa7e1482ac2b94a0aee8d8af299d274c69f3a170de00a0bbaaacc26fb17da35e753db69126a6e74d33fb05b79e92" - }, - { - "block_id": "000001e001c1451274c03f8592713217b39c97bc", - "extensions": [], - "previous": "000001dfd7928c0a02565aba82157957388d0634", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20481c6c963681da8da0e0777bcb1d55f712004766f8dd39610890d8e9eb3e033947852db9fbd8db7ba0e20835bbcbb6001d02b9512e788f7782ca7ec7c674ba5c" - }, - { - "block_id": "000001e17ef88c2c5c97c76e3fdd3882f8c93ce1", - "extensions": [], - "previous": "000001e001c1451274c03f8592713217b39c97bc", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200d36a63e42e02feaeb8bfd89106fcea088348b229ffa1fe0e972824d4a8543be1cd930e92837e7974b51753ba7af34899df9d32b137af0828f6b4b69de14ff42" - }, - { - "block_id": "000001e29bfbcd93f1da2c3fe6da3196b7538d3d", - "extensions": [], - "previous": "000001e17ef88c2c5c97c76e3fdd3882f8c93ce1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6392d339e7b8c54e2819555189482744f9f0301cd7099726d8a9f6f70236d6b2520e3e9928e14e5ffbb6e673b92b569a9c224faca2f77c7867fed5a2cf5b0486" - }, - { - "block_id": "000001e3470315d56cf5d7907e70e0943c99c30a", - "extensions": [], - "previous": "000001e29bfbcd93f1da2c3fe6da3196b7538d3d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201be488b3947414eb7bbcdcbeb3e74f7f4da5b985183b7dd63ae7900db6b9b5fb15072e7141ff5d6ba657de370051b18d2ef6ceffb3b80bda46750ada2d7546d2" - }, - { - "block_id": "000001e4435905d9282958a4f88c76a667e1951f", - "extensions": [], - "previous": "000001e3470315d56cf5d7907e70e0943c99c30a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7a5ea9bd97765a7bd3bdda651176e65be3a219ddd68709aa3ab62dd6eff1b4b51d52954e8658e8116114667c233b92b290c9bfadb2690913326db17b9f0d1308" - }, - { - "block_id": "000001e5fd85902173a856d552eaeab7287da8fc", - "extensions": [], - "previous": "000001e4435905d9282958a4f88c76a667e1951f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2053fdd3f2010b3af567c7081e700b0b37686f64a002e4e93bc7a4e01a83e752dc4e7f32491b3db70fce53345788309fd423e08ca672bba7a34b93aebff70a8423" - }, - { - "block_id": "000001e6d5c86ac829fc873fd41d1df59c575915", - "extensions": [], - "previous": "000001e5fd85902173a856d552eaeab7287da8fc", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205b76f95248d41f2cea365918b073567d1f273ec174ee8e4235dedcbc54ed60576dd95f96009d0365452510381b992dbd5927d29324e06db3e56689f163d55865" - }, - { - "block_id": "000001e78b0f532fc6771223ca48806d1aff6145", - "extensions": [], - "previous": "000001e6d5c86ac829fc873fd41d1df59c575915", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205753ef2c10809fced9bf122e9fc64d0c6ce511c502368ed9fdcc696eecf962643744e7ac1d2c4dbf17640b8437205e5d491ef0796650b568108e42a49e599d93" - }, - { - "block_id": "000001e8e866265fc701eaab2b5849cc17c96316", - "extensions": [], - "previous": "000001e78b0f532fc6771223ca48806d1aff6145", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20669db2ecd9df54f4e0d82d9bc0cdfc5d14f1f41e35e1f98f6a120feecbca59367d4f113814a745963282608293654e52358b30856fd94f91fe7e2d037e1a1d22" - }, - { - "block_id": "000001e9c138c881602a91e60e6842bc371c396e", - "extensions": [], - "previous": "000001e8e866265fc701eaab2b5849cc17c96316", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:29:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f13760ada1afeaba6a1bfbae0a74c460567168d47c812b216344a46eafe2152085e734408aa311776356095ee15e36a124e8742b29044f78004e73ad9026906ad" - }, - { - "block_id": "000001ea619237e4b64a51ffece5d63ac74a2709", - "extensions": [], - "previous": "000001e9c138c881602a91e60e6842bc371c396e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20578d4295fc8b2f1c46251a20cbd0fda1802bdc58e6b378831ab1d9ade2e8c5da430128a372b1911ddff642023314c39ccdc54d34164ab7e972f40c9969e04d60" - }, - { - "block_id": "000001ebf450bd9262cf046c3a38c46fdc879744", - "extensions": [], - "previous": "000001ea619237e4b64a51ffece5d63ac74a2709", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20383066b48d3e8855113f76941fe7fe44157a49ac224df1a28747a5693bf7d02155af17f3317ff64d6662c594fe60e556e5496ce9d6a78dc2967aa17363faec51" - }, - { - "block_id": "000001ecad6e85d5140c6b86f2d1fecd2f38616d", - "extensions": [], - "previous": "000001ebf450bd9262cf046c3a38c46fdc879744", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2002e13709bcdf1f1f5daa464131566b7b77e219b12ea118b19fab69d404e3bad3421a112ec073f6b9a5a572dfea52062cb70d04cd6c3dce25641c3ff1a36b632e" - }, - { - "block_id": "000001ed70c60c4492948bf8502d51cd7bc739ad", - "extensions": [], - "previous": "000001ecad6e85d5140c6b86f2d1fecd2f38616d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2006eda2897a0e6b4bf8beac5abb20bf268e617bcbd0f321f2b21931b6f3166aa749ee01e0644e315a180d91809f25416b25a1a599f71c2feac1460f0bc7e57ef5" - }, - { - "block_id": "000001ee306254696cbd665a8d8ca8d7671ee44c", - "extensions": [], - "previous": "000001ed70c60c4492948bf8502d51cd7bc739ad", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7a07bb9e6bd846f5182b82b55a1ceccf020a2d07d5fb2cf642f7486ce1d1547f6c814bd3b5d426d902a4392bc0903738249810475146b011ec1b3c4982632d79" - }, - { - "block_id": "000001efbf424b2b1c733af1019d9aea03946305", - "extensions": [], - "previous": "000001ee306254696cbd665a8d8ca8d7671ee44c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f152fde44425fdb37294cafae6dc49dae4476cedbd104cb8dc357f39dbed836cf044eb41acb988cf3457f4426b10eafc45995001e0a6ed2aacd3b429c2b22b0f5" - }, - { - "block_id": "000001f0c6010a191c4e2517eaaf04a993cf3bbd", - "extensions": [], - "previous": "000001efbf424b2b1c733af1019d9aea03946305", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f12c240223d8bfc4972c2e3f597162e10436ad82c908c8dd1a02551e456be24f8257ce3b453cbb7f6dc78d9d6ae3ede57ca23ff22753cf3e7d52eb970032fd012" - }, - { - "block_id": "000001f10ac6bcce1804176ad30802178616e554", - "extensions": [], - "previous": "000001f0c6010a191c4e2517eaaf04a993cf3bbd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f17f43ce0c93ec48a2578768294c6173cadaf712cb89b05abef126249feb7f85043441f7eb045994c9804978cf551020bbfd57d5df2f6abf115ebef7966e60bf6" - }, - { - "block_id": "000001f24aee979b5fd14f4ebf385c63e1044da1", - "extensions": [], - "previous": "000001f10ac6bcce1804176ad30802178616e554", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205c95e7f9d114ea1e8672c82ee1ad98c094088205a9a801b3743aa1ed0e61509b478d45f8fdcdf32f8585f7efde6caa08589bd2ea03b2dbbee245e097afc734e9" - }, - { - "block_id": "000001f3ba5fde34970ada7f24ffc70c4ed3d613", - "extensions": [], - "previous": "000001f24aee979b5fd14f4ebf385c63e1044da1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202a9cf9569818023e5375fe8db7c66ab4b0dd74a804980ee6df63408980e9668972b8085eb5447010e731eb46e460e2d8b4e35798eac6658ef5c22b833c723f46" - }, - { - "block_id": "000001f442c6d65e945ec811d75ead67a59d6b3d", - "extensions": [], - "previous": "000001f3ba5fde34970ada7f24ffc70c4ed3d613", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200e490e45c02cc78aaf958aacdb32c9544bc0c44e479f18ebed6ceb51064fffe63c3495b2c1937b1b181cd88bb0d1f3af0eaad986595983514cbf7ab35f153bc2" - }, - { - "block_id": "000001f517cd43d239d6eefc4ceb7568b96644d0", - "extensions": [], - "previous": "000001f442c6d65e945ec811d75ead67a59d6b3d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5a44261eecc2272d4ae617a2876da61448a920189112fd26f3afe5dc18debc4b327f2e9b3e20368e3a96ff00c8d5bd8e445bdb2542c9ea0e188a62941a29fd6c" - }, - { - "block_id": "000001f608a9a5025199c49d212f893cbdd65f6c", - "extensions": [], - "previous": "000001f517cd43d239d6eefc4ceb7568b96644d0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f11e30503f94180a49df962a70331f47006b632c2c579d939e37a8729ff9c72b764d209cd1f250a9b81735e626ab355dbf23ab0a60f6d76dd8a5e1c70ed7e75e8" - }, - { - "block_id": "000001f75d016e81f9146a588554d8ec5ab3544a", - "extensions": [], - "previous": "000001f608a9a5025199c49d212f893cbdd65f6c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2074cd014e2e41e515cf6c3c9b23d77776e3c967415db19da258ec4b0d07df78f66483af5be7adec100464a488edb0874240f9754775b4b1cc7b167242ec8616fa" - }, - { - "block_id": "000001f81f897f55f6a8222fb273b968e4159ba9", - "extensions": [], - "previous": "000001f75d016e81f9146a588554d8ec5ab3544a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f362e36c70f68ffe14692dab9aa2f1acfb7caa7eb64be957b422d01947e86dad439c94d7e9cd598473e297c9c539998828955e639cdedb5842cbbf04ebdb79bfd" - }, - { - "block_id": "000001f905a687a0e4b12ea5d0fb8d708befb655", - "extensions": [], - "previous": "000001f81f897f55f6a8222fb273b968e4159ba9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0f72d1964257dd4be88c7973a9279253e2d5d0f8d66f08f0692b7238fe9dd8732030c4d634dd98ef66510e0e66c621f3f5d1b2a94904769a94cf3c0f496d22a6" - }, - { - "block_id": "000001fae91d15d1766ca3039ebe36b6bd74db09", - "extensions": [], - "previous": "000001f905a687a0e4b12ea5d0fb8d708befb655", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203c3645e931232d4365c01b40126bcecdf89e92bbefebba893d7ec537f2b95f581a03f20cfde90b000cee4a83db3296abb250ad1dbee0a9d735ece294921cf089" - }, - { - "block_id": "000001fb11ec0b0e6c667c256b5397eb9d41ec69", - "extensions": [], - "previous": "000001fae91d15d1766ca3039ebe36b6bd74db09", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1ec784594e6d493f98c95912a3c939866bdf73f97bfb074404fa5ee403ef65fa44c5bfb43bae6c3a4c711968098ae66a40c2e0600ce6db9402457d56b2f6bc31" - }, - { - "block_id": "000001fc8487d6729be67d0043e004892315eec9", - "extensions": [], - "previous": "000001fb11ec0b0e6c667c256b5397eb9d41ec69", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f46ace60a5d6ea3f3141eb59929004db86c8c222a6f39dc449811314119770a5840eb0a948f6529ec4352dea1bfbe1d93361b2f8fd827a336cb7a5d4ca077d2df" - }, - { - "block_id": "000001fd6c4b750421c74fca7720f5f97c19825b", - "extensions": [], - "previous": "000001fc8487d6729be67d0043e004892315eec9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:30:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2004563d992c0346cb63af61329dc150c99c5c7d99b53e4f0618e46047761e17600b9cc66e600ad86f76a366cb4f8ba4c4159b2d2e7c30b50f1cc7eef831cd7c1c" - }, - { - "block_id": "000001fe4e9b5e541161f7cd0f603f44eefa23af", - "extensions": [], - "previous": "000001fd6c4b750421c74fca7720f5f97c19825b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f77f9781e7d4bb59aa091df6a3d52cfda2a09c122455dcd2389466752b340ab1517822a85968eca97a4a7416025fe7e63beac9c9894af6b1ca5d12001eb60f80b" - }, - { - "block_id": "000001ff5afa87e9ea254fcaf76dbc3940a4a577", - "extensions": [], - "previous": "000001fe4e9b5e541161f7cd0f603f44eefa23af", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6a9ca82b459227de12aa159e7b548c7a758d426a92d6cfc63cedf85c4f36b2f6436e861c251f7c29cf8e15a5751908fb9001cf1c708e89ae36c89d31f6b2a9a5" - }, - { - "block_id": "00000200a03ef346a325091247d9f8903cf91367", - "extensions": [], - "previous": "000001ff5afa87e9ea254fcaf76dbc3940a4a577", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200907daf453f53da4068a4ed2f3eb30f7d58df5b61c5c02b6ddfaaac1e9c7bcc87381cd224b86a632a69871f56c48d66548888cab7aa23890e8a59564428f9fed" - }, - { - "block_id": "000002012592c1ce59e2d41e391c508446cdc93a", - "extensions": [], - "previous": "00000200a03ef346a325091247d9f8903cf91367", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2011b61342c313a933d602099208107506e87032e033a13162bed3cfa4bd64ded949163d98b99f9c3c4d3470f13256c7ec02171a7768e38809ff77e5bda06aecff" - }, - { - "block_id": "000002023d2f8a2e9c9de69b77e4a4a52d9cedb2", - "extensions": [], - "previous": "000002012592c1ce59e2d41e391c508446cdc93a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f603248a5099c37e1d3016d66d4ff3e6f5d83aed410a063d0b1bdb88385b8951747cf41da01cea436753ffafcebbd2177ae144d1320b1727deaae8fd9c6dcf67b" - }, - { - "block_id": "00000203c41b2f04fabe3f2959a65e1d8bf5b674", - "extensions": [], - "previous": "000002023d2f8a2e9c9de69b77e4a4a52d9cedb2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1c364bd32ef1d6bc0a9b3478c653ead036d23b0d7ffbdf1d6963e977ff7f4de66f2b642feefc835df04edab452745472478cdbfc8809ad00ca862d0aaad97459" - }, - { - "block_id": "000002049649ddc33f5eeade4a9d46aad0a0f6e4", - "extensions": [], - "previous": "00000203c41b2f04fabe3f2959a65e1d8bf5b674", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f40e5b5911f0644451961862f135d674ca99c5087bbb9e87ce9b1fcf8948afb26325e3ac006d3fcf10b2cba251fa9b26fb6c4d5f848a330f5135140c7aa5b33f5" - }, - { - "block_id": "0000020531082e2d12d1f25c6f51a32da5a30eb4", - "extensions": [], - "previous": "000002049649ddc33f5eeade4a9d46aad0a0f6e4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206d88c76dd261e88b51ab02199cedf00124a8c0f12bbfbaa24ed8976f2194c03c45dfc7637c42202bcce59f1c8ce6dc6b87fd3e9efede75d2acafd1d9ba423c6a" - }, - { - "block_id": "00000206448a8fadc71d3a9d645afff3a22cc3fe", - "extensions": [], - "previous": "0000020531082e2d12d1f25c6f51a32da5a30eb4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6d55200f6ead4b9baf61aadc4713f9bbf98c62b3022e2c0c817d4288dc8e50924fa666a59df8a9f0de5f0d7c40ecf008dc8eb1668aea250bd511e0a8484136f8" - }, - { - "block_id": "000002077da436f25f14d297dd83333626dd20fb", - "extensions": [], - "previous": "00000206448a8fadc71d3a9d645afff3a22cc3fe", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f33f3cc1d3858346f8e8ee62b4ed581fb76a6328a1ada627fff4468dec9c9f01b42b48a4fb41da2b5ac08d63e28fc3041ed17751dfef7d810c5e5bf51bf5aadf5" - }, - { - "block_id": "000002080abbb21cceaf6ce57826d6e066071970", - "extensions": [], - "previous": "000002077da436f25f14d297dd83333626dd20fb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0d877ff560ca816d6d353cfada0923b2c143b2fc5d60069766d6937dc7682aac55404d341e4f050392527c7eb3072cead6c45713563dfae0f4912701eeb2ad2e" - }, - { - "block_id": "0000020934e252dd6f481d765bbe7c712869fc58", - "extensions": [], - "previous": "000002080abbb21cceaf6ce57826d6e066071970", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204c9ede396088da3c9b798fddd0e8e6227208d8acc5f0731f4f5c6177a8265b3e27aec83a18ff7272756928ea67273acffcde9ab783fc869f7390e9ebae7d7b46" - }, - { - "block_id": "0000020af2625cc26f2d0b83d953e6553fb4c79f", - "extensions": [], - "previous": "0000020934e252dd6f481d765bbe7c712869fc58", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f689b48775d71f8496b665e0e1d68ff2130537d6a07b0eef710c0bf19ae7405b3086e66ec57947876332a175f7fe5a2c0f436b25c7889efe2e6a725335e772ca9" - }, - { - "block_id": "0000020b06ee49fd8553996834803a058dcf3c16", - "extensions": [], - "previous": "0000020af2625cc26f2d0b83d953e6553fb4c79f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2059676e6cc765af0be402a93b82fe0ba5b6c3f9a4850607e22f268c18f8cb873557d22c3ea405fd82dbacd028c1031894eef9010f5624dab72a47742af1501ee1" - }, - { - "block_id": "0000020c7c5aae033b7e2a2c41875da627674f69", - "extensions": [], - "previous": "0000020b06ee49fd8553996834803a058dcf3c16", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200b1c90207dce424ca1931b99eb5bcfbb6e92e3abf10947ab353a91c52a2e25750116f98fcd33f9d3329b611404c1823f6b3209a12dfd1cc2f1a5238efc06751a" - }, - { - "block_id": "0000020d86e68104f0227ead20a21787a40ebd15", - "extensions": [], - "previous": "0000020c7c5aae033b7e2a2c41875da627674f69", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200324bd0db817927df0e0174fe57ef285cff69cacda3aeeb880363500a9f02e3a0783bb4fe8af0166ada5b69034a65094ebf07b0d391ae2a5c4758cdc290882a7" - }, - { - "block_id": "0000020ef2ebe8580ccc91c66748a3e1864f2754", - "extensions": [], - "previous": "0000020d86e68104f0227ead20a21787a40ebd15", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0697ace7d9ba1d6423ff7a710f1d0785edcaa802f4b31d562812833fb7f9d2fc1d127e97235883272677d49960f673be72e55a88c793e09f70ed4a3d7396fab8" - }, - { - "block_id": "0000020f626e60dfb2beeffcb6f7fa2494e0861f", - "extensions": [], - "previous": "0000020ef2ebe8580ccc91c66748a3e1864f2754", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20138fac95d32553e525a6f2b8e5db9fa7d8463e111da90ac9727a055024e2aeda1b7253050db34cc1296b27ad4072ae8197032692d87bac7e9e6c76abc4e9825f" - }, - { - "block_id": "00000210a10503a9da4c61238a1cc63e8bbefd6a", - "extensions": [], - "previous": "0000020f626e60dfb2beeffcb6f7fa2494e0861f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206e22b708a604137b28717f0b2d86b62c7f2b3678d88cc6701e5ed9cddbbdea9845aaa04d034e85ff8822f7965b19a8133a7ef74e03d070b6b51e9868df7753ac" - }, - { - "block_id": "00000211ccd1322631ed1e0bfea671aad6b1f134", - "extensions": [], - "previous": "00000210a10503a9da4c61238a1cc63e8bbefd6a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:31:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2023080ec607d834c47552230b52c6e7f176a6f07f681125e46275d0fcfd02b9fe01fea5f05a82566b47b7a1155b4d915f4ae15409e60e32e298bacb9c7070a662" - }, - { - "block_id": "0000021254f7fefc2d24f134826ef204cd280a7e", - "extensions": [], - "previous": "00000211ccd1322631ed1e0bfea671aad6b1f134", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4c1485f0106ac6336038b9e2e6d2d1d2f125176a54b35b3f616117e5d0bf91c7722170d20f358c1be2f3d35c00b4019e877990ad758e5c1ebdfe33225b89912d" - }, - { - "block_id": "00000213b5c641fe494f557eb9738831b97b7e63", - "extensions": [], - "previous": "0000021254f7fefc2d24f134826ef204cd280a7e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204ad69a25597343e4e0f105cbea671a05f6717cd00132499a3014f16d8e8a1a732e7402abc1911e29a97132e9e3da859e59427fcf3d206d0fda931003cda8bd78" - }, - { - "block_id": "000002146c96ff4da11de4467d9c2389c3de10ce", - "extensions": [], - "previous": "00000213b5c641fe494f557eb9738831b97b7e63", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f45a2abc4931e5a43f0d26d9322db898bd6095da7c7c9041704687caa26f91c1e10e2dfbb0d61bfd7b6c23ff4db6718c863fa4d6e424f4679f77266aebe15b6b5" - }, - { - "block_id": "00000215e0e3deabcc6f435c28a1355c74730fa0", - "extensions": [], - "previous": "000002146c96ff4da11de4467d9c2389c3de10ce", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2051955f5e45dc901208b015b71af3c390cdac6cc43910ffd6e951907eae937fd16e29245fbfe9880e11bfa952dcd7a8494c27a01856101ea0557c801fe57c9edc" - }, - { - "block_id": "0000021670b76b6b8a9609a57646f0a733a6e394", - "extensions": [], - "previous": "00000215e0e3deabcc6f435c28a1355c74730fa0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f55f4563ed16d8c5380de65206829561fbd3bbd3c2e3d5d8ad48bbae3625a3fb41046812b1e24a3f2d1e39dbd3fb290f594c85e7441a203ce12dacf5b1cc8b284" - }, - { - "block_id": "00000217bbc88281ca2c70f8acaa9891052d94ba", - "extensions": [], - "previous": "0000021670b76b6b8a9609a57646f0a733a6e394", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f26332bae0580c4e3c9a7cc39c2580769c436c0b0e7d13db4f8f64cbfc174611a7bee589f58cc81961c2d07cc7fbb08de8358a2978cf65f57b0effb73e9020671" - }, - { - "block_id": "00000218fe49a6c0db82438d26030108dd39a074", - "extensions": [], - "previous": "00000217bbc88281ca2c70f8acaa9891052d94ba", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4ebfc4c040c3477b1009a9d74fafbc808980252bf6ea562d2ca01a22cb2d43be2f61eb590658d460e56ee36ad638e865e5ea7cc12a48449fdadac2cef8ffcc7e" - }, - { - "block_id": "0000021977c5ef7084d350d44e5f9247ef0cc705", - "extensions": [], - "previous": "00000218fe49a6c0db82438d26030108dd39a074", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6633425863700f16f445db4bae9e63c1ad03797c5e91894e3c35b0e1a53e8a00198134b7fc0725eff6c9a0bff88658eeaea74f4ae8c35a2afc45006a318e3d83" - }, - { - "block_id": "0000021a92e6cbe68e229d44d0daccd2638b551e", - "extensions": [], - "previous": "0000021977c5ef7084d350d44e5f9247ef0cc705", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20482c1eeb2cf35c044f33ea929e28d5501183674f211a9b4f28631d39cc50f8065df098970d00295ab416c4064fa6739bc690accdf5d3b38a4341afe42b47cf41" - }, - { - "block_id": "0000021b079cd212123b09cc0824d72ebc3700f0", - "extensions": [], - "previous": "0000021a92e6cbe68e229d44d0daccd2638b551e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2062453bc6e15c9c81ceff535afa895571ffad49fa77df97f398da7448c00499605d78c16d5a36769e89b67fa059f5407bf01b4ddc156740abcee7723299ad53b1" - }, - { - "block_id": "0000021ce4610a79bc3a0eb222d23f20bd30cd5d", - "extensions": [], - "previous": "0000021b079cd212123b09cc0824d72ebc3700f0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2027431e9c0f8ecd76eaa456c5132a4c853d3ad3fafb675c4dadc84b3594636666630e110fdd52ed7b1e4e1014f15ccf793d3ab52f1e3fab827496b876d719605c" - }, - { - "block_id": "0000021d788e76d348850c0bed221a9040f86356", - "extensions": [], - "previous": "0000021ce4610a79bc3a0eb222d23f20bd30cd5d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20019a6e19250b49733c14b69b3dba24bdb582c5381fb1c394e6e56c5ae048c4855414200a9b36da2284fa1ac01c67ba161d7948f18d3386eac35dda7082285a26" - }, - { - "block_id": "0000021e00e324f7386e1394f8b837eeaff18b18", - "extensions": [], - "previous": "0000021d788e76d348850c0bed221a9040f86356", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20565d6823716efa3f5205123a2af13417e7a595727403eed1aca710fd5d51780e0a9211c450963a793f088bf79bb311382e0dd760762ccb8be45c2fd46e369151" - }, - { - "block_id": "0000021ffca953eb1e8121dcc40cd08d40190393", - "extensions": [], - "previous": "0000021e00e324f7386e1394f8b837eeaff18b18", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6ee94830651a32bdf3ae875fc573b4086f6e9c7ed783315b40d402a65602dffb7c00e9c08df607185267862f6af8fe6afbd738aed77de6f780209e55e5ebe7db" - }, - { - "block_id": "0000022075b3832b913c09948b532a1eefc4f7a4", - "extensions": [], - "previous": "0000021ffca953eb1e8121dcc40cd08d40190393", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20026fd602ef15bdb9249afebad05001ddee2e1de2a5910772590deb6c4ea0ece60c87a8bf121adc08a58c928eb291377a6b69e908d3c348d9bb043040ca5260dc" - }, - { - "block_id": "00000221a853002374cbaed5627a281ddd46c30f", - "extensions": [], - "previous": "0000022075b3832b913c09948b532a1eefc4f7a4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206ca6885fe13e0227879d76c2b34c479c4e2f4f40288f1081fae742e7ad666c8e683b012d2c464d18aca9e2c762b1637c2212bfef0197a0ad4a3009f64d6d94df" - }, - { - "block_id": "000002227b6f6b19d2e9152d59655be87ade9de5", - "extensions": [], - "previous": "00000221a853002374cbaed5627a281ddd46c30f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2068776241cd3dae62ee14f557a92da5fa2f5a42918ebfb5bbfd2f1bb568ad338764c9529c031b782a879087db4d04a9d8837ba5114c10cdb210c86063656e1c01" - }, - { - "block_id": "000002236a9719994ab1f3f29472384813055242", - "extensions": [], - "previous": "000002227b6f6b19d2e9152d59655be87ade9de5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20680633ef7dd1316d2bbbc0e9f46edc58d059c9c85bac97ad0515d934b4d7218a119fefca83a1c59654f98abed0c9cea2697d1e93dbe40cb4f36de5f1400f5727" - }, - { - "block_id": "000002245d8fdab1bfdc89b6f268373b9299d4e1", - "extensions": [], - "previous": "000002236a9719994ab1f3f29472384813055242", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20595b1ddd0c2814fbbb5b908f531f6c7389aa12a6b66f5f75667e7d28050ce4c204da4ce7668a380fc2bc8194d83bdc7ff1edc550b4797b803ce9d81f7ea9ada5" - }, - { - "block_id": "00000225f738ffedfda45656f9616303020facf1", - "extensions": [], - "previous": "000002245d8fdab1bfdc89b6f268373b9299d4e1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:32:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2022e2e65b49b6c729e47f30f0dfbc00cbdfda15e295c47ecc52214facb00e794c27b14b086005a74c9289a3b0410f41ecbbe43c3a30092f5df9d46682dd625074" - }, - { - "block_id": "00000226445487e5705a59fe86586147c3ea5e7a", - "extensions": [], - "previous": "00000225f738ffedfda45656f9616303020facf1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7508fc4608766b98504b02f7605dbc90c617d20e329f4831ee12589722eee04273c1c219e05736d1423ca5c55d371d0bb1c669a0d74c76914ee18bd1fca655f0" - }, - { - "block_id": "00000227b94e182e5fb09512ea6fd93c19dd3044", - "extensions": [], - "previous": "00000226445487e5705a59fe86586147c3ea5e7a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207eda799dae557415df199ca79da5048973f72375d2a23a8611d2adc2db8c5a5961bb8483c006991d762a465538b7e5e7b722a919118ba4486fd5029ec2f77bb3" - }, - { - "block_id": "000002283b272ded7a2e0b07e7523ec7ec4a9f10", - "extensions": [], - "previous": "00000227b94e182e5fb09512ea6fd93c19dd3044", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2056f460a35cd74c9bb18cede4bfa1b12ca7fb13e746c15ce371746016d97e177e5d70ba6a3a5d3459a22c10973d9c4178a58cfc8e744d0f3a8e200d94b953788e" - }, - { - "block_id": "00000229743741d64ce1c9152562838afcd56041", - "extensions": [], - "previous": "000002283b272ded7a2e0b07e7523ec7ec4a9f10", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f05a2252cde2c9d74d88372b862563e84442fc2d1a35f25d96f46c0c4aa9cce017e71434275e4ce38d07a29fa8d7b64e20bed1b67d22dfa46575f605bad2c82c0" - }, - { - "block_id": "0000022ad6dceade4d56bc1bbd1a106e537c25bd", - "extensions": [], - "previous": "00000229743741d64ce1c9152562838afcd56041", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f799c04d41220caedfa10062db8f0a03105dbc398d9c976fdd34329d8228cd6933d64b9705a55b8eb2a9282f267dc2c31b48d7631f1312c6486c4c6b7a768d99d" - }, - { - "block_id": "0000022bd4a295e429b8cf03585892e60f106aed", - "extensions": [], - "previous": "0000022ad6dceade4d56bc1bbd1a106e537c25bd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200196e7156a46414ad2fdbf3822baa74087b0b48a4b99f7c4231bbd7dad9478d83471aa43e2ec0cb95e93f01ee81618f76c5e7d24118fbe7b88ef78b545ffff17" - }, - { - "block_id": "0000022cd57fad91187e9b952a8a35b805bcebbf", - "extensions": [], - "previous": "0000022bd4a295e429b8cf03585892e60f106aed", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3c4cdd1c88db0734febe816f3a85667b6df8e1c3ea5fbeed50140173e20b8a502e7e2b1ef0831e211bc7cbe4fcced7743c16847b5790db16cc4b47fc7b4d1137" - }, - { - "block_id": "0000022df6bbcc589443a381d7e56a5cf6b53e06", - "extensions": [], - "previous": "0000022cd57fad91187e9b952a8a35b805bcebbf", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20699aa9a0fe2ebd695343f314695a0a762ef305e48d27ac5c92712ff3a24c7537686c2722a6a07934c9c6b4c75fe073b79159f51188da31ccd9cc219dcfcc93ad" - }, - { - "block_id": "0000022e93cfd0300c6e7f44a479a8cb25332a09", - "extensions": [], - "previous": "0000022df6bbcc589443a381d7e56a5cf6b53e06", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f58df07f4bc861e1dfadcc7cf8e53acf512c3b872d4e4a95ba0d8e9a872c9cca16f328c578f56eecf562140a0145a511408e14affccbf3eefb20dfd0562fcc461" - }, - { - "block_id": "0000022faf6f34fd3d991d7ba3604503493e205d", - "extensions": [], - "previous": "0000022e93cfd0300c6e7f44a479a8cb25332a09", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203f6a175c539e5fb46f5573f1e72d903d884764b4a1360155f0cec398ed0b48ca20f53517b05502e02d103863f1ff7056fe1a8c52a2ae2b55c2da06d83887bf44" - }, - { - "block_id": "00000230c73ecc325b5a282fbdad4db4d10ed39e", - "extensions": [], - "previous": "0000022faf6f34fd3d991d7ba3604503493e205d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207c7290bb38ec467a2c527851c233e6ac6a66feae0bacf570d146a89f24077dfb5cf5cba5a82ca98c4d074caf3711d02885aee6ee3eeacc15cf8da085b771c045" - }, - { - "block_id": "00000231fbdbfd43a0f0a95f1eb0988714eb094c", - "extensions": [], - "previous": "00000230c73ecc325b5a282fbdad4db4d10ed39e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f034c9d4894c6ff4e9270d0c85d1545df5f14e5b2454791b0b58af33a682db6b22865a04cfd84dc55c1de99a50ec8bedeecad4d518167e42480fe25a118652d48" - }, - { - "block_id": "0000023257c8f7eea7ed408daad31e1a144b7c06", - "extensions": [], - "previous": "00000231fbdbfd43a0f0a95f1eb0988714eb094c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20374e6c58c400c568680ba905daf0b06a0b72523bdf5465a0d1fd792935d1364d3e8a95a328067c9dac993307836ff320c116256c940192bc51bdcd832659b921" - }, - { - "block_id": "000002338549cae625ba40eb2c2c58032ce0ecd5", - "extensions": [], - "previous": "0000023257c8f7eea7ed408daad31e1a144b7c06", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1f8c1d21e096062234dfd9cd204c9d35b0c2a57b59935293831c5396750da93c73945d9a87a83cef5a83659c3803c7930e336e3dce032df6986d5a50f5a6f9b1" - }, - { - "block_id": "000002345bc0db7f2ab78ab580c3ea185236757c", - "extensions": [], - "previous": "000002338549cae625ba40eb2c2c58032ce0ecd5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f31b020038ef08cd9b5a4194949d16e64e15425d30c08904f8e47697be3aeef172f8561730db433757d46f820e973c8a4358a7783232019d0773e1271e1b16479" - }, - { - "block_id": "0000023507b1816cd1fd0a695b8ae74afe8cfb77", - "extensions": [], - "previous": "000002345bc0db7f2ab78ab580c3ea185236757c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f64321af408ba8ce57e0d7fa1085de4556311db06575d9b8ad3c5686c2c1fd0076f83cc8382f4aa75e9a06760cfc80ea70b11d52b2329462d9c75e531725efe11" - }, - { - "block_id": "00000236ce3356ceed5222cfbee43c21d18a0a81", - "extensions": [], - "previous": "0000023507b1816cd1fd0a695b8ae74afe8cfb77", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2070110c2875a033860523714b4282270d35ad02db3215ed34ea403f0c241ae318185cecec839356a32b96615e723f4b99d1c4b3955e5e76f848bcb8e0ddf2127d" - }, - { - "block_id": "0000023761134c69d91da8e975ff6e142da6ab4e", - "extensions": [], - "previous": "00000236ce3356ceed5222cfbee43c21d18a0a81", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201fde5c5e25f7fb48dc630323d6f4b44cffd7e8fcc1079d4b98c82eafa75ae5f538f8f08ccff417bae3838348078a2b25fc2512c02519020b2d89bb688d21b9ce" - }, - { - "block_id": "000002389e916da7f51a766da03cb943b1bcac7a", - "extensions": [], - "previous": "0000023761134c69d91da8e975ff6e142da6ab4e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201ff31e27c77d702a4b1cc6b5bde6bfbc996f20c959919992b71f68d4c67ca102350f29c2fc533aec025fc5ad5a496d1a26bd919178a0a354d349c3fc2a559f3d" - }, - { - "block_id": "000002397bfa7ef6f48d9fb12816f1fd4a842d23", - "extensions": [], - "previous": "000002389e916da7f51a766da03cb943b1bcac7a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:33:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2022124a7796390172513a0b9ead7d2419646077c97fcde78c4cf2983e4b9abff639a160543a069ccf75cf70e00e160f5b059f63366ad89fc41d7638c3a216487a" - }, - { - "block_id": "0000023a06af0798a1595788522cb3bb1b7ea6a2", - "extensions": [], - "previous": "000002397bfa7ef6f48d9fb12816f1fd4a842d23", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20290f2689e8856a5ef711244d3a92f1a2ce9391f17ccecef87d0bea966cc087d773d964ceb6ef748c7e105d06fe8749311a1b585ae0ad24b0555adec3ca4bc9a9" - }, - { - "block_id": "0000023bd3f2670cfb3de42e079fcde15a3c6e7f", - "extensions": [], - "previous": "0000023a06af0798a1595788522cb3bb1b7ea6a2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2005b81e044a79e3da3539079a0e99d0df73af0c162f10da24e1d216c62ca37cf020116c509f82f2169399fda47139927a615760d66645afc32a708eb5cf8357a6" - }, - { - "block_id": "0000023c2b2089cd71547923567bc0dcfe82593d", - "extensions": [], - "previous": "0000023bd3f2670cfb3de42e079fcde15a3c6e7f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f274304f2733503ee7ed4cfcc3ba9e7225d39cf578b544a24b1c9ed0b94c61c387bff04dc638751753e8fd60d9f420b81432d59d94b2352de5270b0f6ca07417e" - }, - { - "block_id": "0000023dee67c6f411b6c19f4577cbd2097ab4ac", - "extensions": [], - "previous": "0000023c2b2089cd71547923567bc0dcfe82593d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f650ede90b4f8b70bcf52b1e703dae8e892547cf449630ade06596e4687d6012258e7bc63b2da2fac134ea036d24213b608cc32071f247471de3ac52cdffae585" - }, - { - "block_id": "0000023eed8df45575b9dd07d502de19caf533a2", - "extensions": [], - "previous": "0000023dee67c6f411b6c19f4577cbd2097ab4ac", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206d018e0b1860b048e2b34bc614e59fbd5d29ff821cde0f4baa4abc4404c666d7125df09064f194a61edecf9819ea16acb00dd55acf421752e2a43df012f8705a" - }, - { - "block_id": "0000023f4a64b53727bfd3cb751d54c90b7a03ae", - "extensions": [], - "previous": "0000023eed8df45575b9dd07d502de19caf533a2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207a408a32a7199335c4adf82ed85aabe261a2a75ce7d9ac61ec9059b4f2f847240ec8092eabf30ff29203da12a1ae83b31157fea4d7882d266558649ae8e08f7d" - }, - { - "block_id": "00000240767602ce174fc67ff78ad4357e188224", - "extensions": [], - "previous": "0000023f4a64b53727bfd3cb751d54c90b7a03ae", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201c85ace3109bf3a03e65db4329141b3038f5e6da8897381f15a2e97870ebe38e7f5ce2b37bbae64f87dc76db1ddbf7842186b14b05ff9c2a6569478a97b1f2ca" - }, - { - "block_id": "0000024144a5217d5ea14f0c4cb9f22d8830bb21", - "extensions": [], - "previous": "00000240767602ce174fc67ff78ad4357e188224", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207c1c1a1f7b949db49525207ef5517e4c85c83c9c5921e6e238514ba69bd16c0950e7fad360eafcc53c035beea8b498d21a8f8b5a4852247fe863f7fe5f198af0" - }, - { - "block_id": "000002424567d577276f363a7606b5fcf5f982cc", - "extensions": [], - "previous": "0000024144a5217d5ea14f0c4cb9f22d8830bb21", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204fa0337de2a45e05f3e0c3111003f42e238998783ce6b60bc967a336973feae827e971d7c93e4944cfcadb2c910ba43de6db8beb6db3ce75bb30ddbc868d7c33" - }, - { - "block_id": "00000243074e3eda6f66868baab219e526f9b081", - "extensions": [], - "previous": "000002424567d577276f363a7606b5fcf5f982cc", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6f5c07d444e59d512483dc41e753ff23f7f5444372fc4cd707f1caf0bc0baa03026609b2bfdf3ee10d26a93f3c7c1c06234afb6a2e0cedef3844ff3bf98760a9" - }, - { - "block_id": "00000244851bfbd477e4600c70f42055516b126f", - "extensions": [], - "previous": "00000243074e3eda6f66868baab219e526f9b081", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203da68499216081595ac2bc1e595c9f4a747a2bf3faa8cc058c5e0f6252130b2c12ad5ce06d1a53db6bffea04038c55a692af6676adcc908f34edff9fc6577ffb" - }, - { - "block_id": "00000245f22e96e0b455036049dbac9471ff0d51", - "extensions": [], - "previous": "00000244851bfbd477e4600c70f42055516b126f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205996b3482c2b5512ac29fc081c64ecfbb3cd5fc691c6844086bbbda67dc462a0633b682dff78e5fa469e638281ea074ec57f084eebfb6adb95bc2fcaa4123bf5" - }, - { - "block_id": "000002467a78688cf2bfba40a3b609cd8a6b09e3", - "extensions": [], - "previous": "00000245f22e96e0b455036049dbac9471ff0d51", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203ab14e888dc131c54b654a07e386ba1b7ee6c5b179c94c570510e262230949363f9ce306186ed2003fbc4c4600b534967840af6b0a66bf5db37183d8485d9565" - }, - { - "block_id": "00000247ec9db70702db29cf17e5a88d41c977dd", - "extensions": [], - "previous": "000002467a78688cf2bfba40a3b609cd8a6b09e3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f575e9e369907ffbf7d97a3dd38187ea0586e3b666e3f63476ad1d12e42c7302f71afdb4e24eae83ed7929bd4ead99070a6cda1944c8cd35a7910e70d6ca67caa" - }, - { - "block_id": "000002485e50916329251149c984bb95ce46bab3", - "extensions": [], - "previous": "00000247ec9db70702db29cf17e5a88d41c977dd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2045910b4c8435f2f177e02ab2647f66128fa1de53f4e0daab6d23ca893755811a5cb5f262c1b4c83ea3af49caa8418cd799576f97bfb2c72d8a09779df9d2e8c8" - }, - { - "block_id": "00000249abcbe5bba44794290490e7d1e77f1588", - "extensions": [], - "previous": "000002485e50916329251149c984bb95ce46bab3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0f6c0bf4563e5ab7d920779fc4e933fb4397e80b7b20ce38c8c8a4fc2ebbba16209b76b34b938ade332fc154511158189d0cd48c2a4e59db2d7d298d2a3bfdb5" - }, - { - "block_id": "0000024af9c99ccd2506b27c7b46d7c9223873c8", - "extensions": [], - "previous": "00000249abcbe5bba44794290490e7d1e77f1588", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205444427ab2f52b1a93c1968d87e18c9b772b2e5115618e995af118c9473b7ec2636dc03c667a1a4deff4aa4e72aa0049509a23a0e53d1ab30c549596b076df5d" - }, - { - "block_id": "0000024bb84162923a649e03182095b3e1760bf3", - "extensions": [], - "previous": "0000024af9c99ccd2506b27c7b46d7c9223873c8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1dba0fa54a35634e4f7f148736fbcaf7dc3273518e73cff87b1a3a21d7a545d4509ab4ec8401765212d15716935078234e0e0646307ed0127678e22972a6a30b" - }, - { - "block_id": "0000024c513890f001b4d9de0e2621c501eef776", - "extensions": [], - "previous": "0000024bb84162923a649e03182095b3e1760bf3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206aed6cbcae00507cda88799f6d34a7b4b282c07eb64f5be3a00c073eabb2310b49016e478ac579a64fba6b04075b27bd0e11d076f5316fd8ef4e26004aadd522" - }, - { - "block_id": "0000024d152c2293081ba87a6d5a8ee1bc939088", - "extensions": [], - "previous": "0000024c513890f001b4d9de0e2621c501eef776", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:34:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201245207994dc209af26a982d471094312a14d7fa5d9575b4c095bf064102a6db061c5aae46ba0a17ee6b7db508d4487c15ccf00c07af86225a602f9e37623ddc" - }, - { - "block_id": "0000024eb0fcab7690540f2ac8b709ef9a88c09d", - "extensions": [], - "previous": "0000024d152c2293081ba87a6d5a8ee1bc939088", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f76a706506c483fe69d72f7617c1fd92f3665c62a65f8f7df5059b0b27b5bddb36c9dfa0116518e5461076a73d711e8f14273b0d3b0a5c86b5b34e4e1029a120c" - }, - { - "block_id": "0000024f21c70f8f4d7aeb0b9bd9a87284458545", - "extensions": [], - "previous": "0000024eb0fcab7690540f2ac8b709ef9a88c09d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4596a58150df999fc7f793872d2151ed9880d717033e9663531bbf57292282ff465c37144ab9a969ec67acec2d87058d51f2b11e0d7c68155e63b3733a63ca8e" - }, - { - "block_id": "00000250bee7c0ba374f6778f56625c7936b4eef", - "extensions": [], - "previous": "0000024f21c70f8f4d7aeb0b9bd9a87284458545", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5c105cf7ba858ef352b419973b9276b7778e540c3dec229d7e81720853a7986e68503a38dc310859fa130f70c5b30cc370e3b4f0933172cf3b8de54984c7f52a" - }, - { - "block_id": "00000251816b9b3a65bd5b2ed4a2472976f0e6f5", - "extensions": [], - "previous": "00000250bee7c0ba374f6778f56625c7936b4eef", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203a30f988de1563dd2473c3e546b42a6f43c05598a36ccbe1ad9fb9fff7f1df15042f12e97e444c11adea72c4afb37a1e057ff14c2954bc63a14ec74ba84df103" - }, - { - "block_id": "0000025236996fac09b5bdc72069577b837b7772", - "extensions": [], - "previous": "00000251816b9b3a65bd5b2ed4a2472976f0e6f5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f19917295084ffdc7c2645145eacbdabd8966200fb2337c35e4c68745535e86d12e2259e51b64a2a14d77c15333200f625390d5ba344cfd7a70603f13c72060df" - }, - { - "block_id": "0000025338950168f8e704edf8c32ad805cdd1b3", - "extensions": [], - "previous": "0000025236996fac09b5bdc72069577b837b7772", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f19b0c7b287c7d8a4dc089d01f91aba3b7dffcef4eb2dc0f0d3ef910d1505be4b74551c995dd375d4b0c001b74d261ee7bae8837ffe3248c151747fd769470e1a" - }, - { - "block_id": "00000254f0d759ada28da140050277ef82f19671", - "extensions": [], - "previous": "0000025338950168f8e704edf8c32ad805cdd1b3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1e2bcad3c7849e374d720867ef555769bb2088d879bd5b449ffd896641a0a3796c98dd05b029db0775143acbeab606e8cc6dfd1c8f93b7c68a87950000abbf99" - }, - { - "block_id": "00000255ed67d497cbbcd6face7480f0bbf92679", - "extensions": [], - "previous": "00000254f0d759ada28da140050277ef82f19671", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203bc8b4f42ef5dc614f066f7a5454a27f3209c0fd50522bcd34d08a969e0689a260e76ed9bff392f9216bc98842e7f13fb07567bd94b32f83afc83db06e2c8361" - }, - { - "block_id": "000002564148f7063eb441e8f8a608bac079a256", - "extensions": [], - "previous": "00000255ed67d497cbbcd6face7480f0bbf92679", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2259377e4c4e306b03ab5927157551daf1824b90a0d9b09996dc00345476174a5da501617631fcdaeb9cecdc9d9e0330b521feb0376e948f5e8692bf2962701f" - }, - { - "block_id": "00000257ffb4ea2122ede2ed3840875d09d269e6", - "extensions": [], - "previous": "000002564148f7063eb441e8f8a608bac079a256", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20387aec7eaff4617ef80c42aae049b2203eb42a5b7b6e9f2aecf1f253fc5617d14f6bea4395ba6891d17d5dda92fc4436d05c1d726849e5c19e807ab7ffdf1432" - }, - { - "block_id": "00000258be11b4e46c152359cd67cff844e2be57", - "extensions": [], - "previous": "00000257ffb4ea2122ede2ed3840875d09d269e6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f00963e0929cad34506a800fdfcca529074aaea6bf999d8e930704ef7d75414f130e564497886521452c6bc254f8ad453f2ec7ab0f004a7df593e18bdd141342e" - }, - { - "block_id": "00000259d01d8ff52e970011b4bb09003638c86a", - "extensions": [], - "previous": "00000258be11b4e46c152359cd67cff844e2be57", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207d5f94a48749d5212b0eb388b174b5bdae150c996ff8fc5f0192f8737ffbf1630e70908d621a6fd01305e9fccbdc5079edd6eaf9737b4d8ac4bccaaf7a32b1c6" - }, - { - "block_id": "0000025a7b00495fc4a274b68bd1c9102f30749c", - "extensions": [], - "previous": "00000259d01d8ff52e970011b4bb09003638c86a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2bd4deb9a2c745794f359546c88ac8b098894b357dd4a8c869ba8612d0b278840b414a4f1dcce9980f93f99e60877857640a032a37223e9239f2def269541cdc" - }, - { - "block_id": "0000025b0fb80f06a5a283229ca0705ae1d46c29", - "extensions": [], - "previous": "0000025a7b00495fc4a274b68bd1c9102f30749c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203ec8aba830eacec1074a5df7a7e0f47242ae118906f96f33be850d03dadeb81a516d63f92c3cda3a539b27f9e1855d7214b05a9d36dd5bc7319f2271e08d0dba" - }, - { - "block_id": "0000025c6ccf1b50cd39d25a80e5f9834d1cc6fb", - "extensions": [], - "previous": "0000025b0fb80f06a5a283229ca0705ae1d46c29", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0a97610c5152446509286064bdb29cb01920596bf1e8d6e4ee23a88cc59abc862bb641916b53e2674c111d6a73e6dd5da8fd982df566d740097c6fcd4ed0ab0e" - }, - { - "block_id": "0000025dddd675ef45524a82b006f1625612160a", - "extensions": [], - "previous": "0000025c6ccf1b50cd39d25a80e5f9834d1cc6fb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2015413765f650d5a85f43ddae42da3643d5c97e59e34a8edea823e7eee8b244217548544f8d0033e62b05f9c609bfb165073e345a93061bad163d4e1d43d3104d" - }, - { - "block_id": "0000025eaac17aef44722416e8cab10ce8fe58a9", - "extensions": [], - "previous": "0000025dddd675ef45524a82b006f1625612160a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2037708e76a70f18828e8639bb24be0666a65be5fc0abe53a573ce12ffe5d2c638324a6aed8bb868141dd4908d2f0a624d303d3c9e8e7f67adb9ec8f719b458db0" - }, - { - "block_id": "0000025fbd88db47f4703bce9ffbf78023c9e3b5", - "extensions": [], - "previous": "0000025eaac17aef44722416e8cab10ce8fe58a9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207bdce65ad18e2f8b81fd91113740437cff1cf492c5de818c5e0a975c311c5bf8767df12aa0e3783853a2a8c4f0c46dc38b50cc70c2d62479902ea39880b58482" - }, - { - "block_id": "00000260bb92dc8a7422ad7a3608719fc3f03988", - "extensions": [], - "previous": "0000025fbd88db47f4703bce9ffbf78023c9e3b5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2010db64b60f5d076962d06f8cc5fdf32eb1f9bf751971574c05f68f508d482789191bdf739981c5084298f0ea16f3e6a3dc0674f5d9e40bfa28a0a29a9713af5d" - }, - { - "block_id": "00000261210dcd18486706fae54b8724d2314cb2", - "extensions": [], - "previous": "00000260bb92dc8a7422ad7a3608719fc3f03988", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:35:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f59ca573f214f1ca6a2d589bd5de215c65058b3c886737f18a85672a14f2f1b182eb8900879b8c9772eabe0a6eeb73ecdaffb44ecf5de13320cf2bf758d835dba" - }, - { - "block_id": "00000262c680cf2cb3deb504f9488b83d750443f", - "extensions": [], - "previous": "00000261210dcd18486706fae54b8724d2314cb2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205eb1a200791f8b40080ba4dc81895d0b16353e8038b20a42e1ed596c11b673442f1dc0764a8c3e4635ccddc1850acc68abb0735c1623f5d495b8cbbeeb1207c5" - }, - { - "block_id": "000002633c2e38cb331a21f0ca0eadaeecc7184d", - "extensions": [], - "previous": "00000262c680cf2cb3deb504f9488b83d750443f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7ef69d86a93874886bc52b7a9531e76da9ee739a44caa80242624d71c8bb6a24346a57e8123d489e222ab299dc300d45a0167c0d7ff85b1ce12021cbb80e3791" - }, - { - "block_id": "00000264b709ab1ee36538d7d23b997325587c11", - "extensions": [], - "previous": "000002633c2e38cb331a21f0ca0eadaeecc7184d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0b36739cc26576a315908bdcd50633486329d46de5e255a61eedf25e8237693c1dedd4636af2b6dc6bdd99cbbb09ab6f56187cfbaa94a33302f4d05f2cf75675" - }, - { - "block_id": "0000026528f81c4003116cb6c61ddb309a45ce4a", - "extensions": [], - "previous": "00000264b709ab1ee36538d7d23b997325587c11", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203f08842ce003c8d8d3a3f1e5f97d0b3a5942d57ec8e98e09d1bbbde8c9502d0074d741489ec43f5121cfbaba94664857342fdaaa881f1c4d7943aecb2b507d89" - }, - { - "block_id": "00000266f437878bf52c7b684a6b8e49f5e39d31", - "extensions": [], - "previous": "0000026528f81c4003116cb6c61ddb309a45ce4a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6dc1a5160cec0bd5904c1bbb9830d373c24d9228839f51b207a80f0a061348651442c42d2f5e3aa07765d389546b748da96afd7002569f73e4448dfa17942857" - }, - { - "block_id": "000002674f34c30c207e8143415515cfbabc253f", - "extensions": [], - "previous": "00000266f437878bf52c7b684a6b8e49f5e39d31", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7a657e4c6f054e3017db8a16c06aa1e89e4350d44b35f3fd65c3e0da3d3ccf45388b2c6944d36f202900a94a958eee27183e3fd4c7e7b84f15937eb3bd0f2a0c" - }, - { - "block_id": "00000268d74538bca80e43d9904c76368fd9607c", - "extensions": [], - "previous": "000002674f34c30c207e8143415515cfbabc253f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3715c2e60f61ce5dccb99e70e3c371354a94c4b851d4750bc3287bf938d32f2e1604a348d16101503f0d6cfe9f104ae991e19bb0ac6105913b049fe3200c1307" - }, - { - "block_id": "000002693d24603b522c712113fb27586fe318f4", - "extensions": [], - "previous": "00000268d74538bca80e43d9904c76368fd9607c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20277994a296dbd8be0c8da0d0b7bd4b631f6fde46f538eb8ef0cc47e0931397193bf96a595ff5f91ddf437fc41da5798cf710213e61ce7b6c74e03314aa047465" - }, - { - "block_id": "0000026aa6724994c807db16040acc9f6fcecf4f", - "extensions": [], - "previous": "000002693d24603b522c712113fb27586fe318f4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4db5db4bf469715b7e2eb08fb4cb89020f020589ccbd90e30da4e9c7a0405db17946303dd9be5148818dc1309810dc5415e86b29b3fb266952ea27fd705bfc53" - }, - { - "block_id": "0000026b39aa18f65f34e61e3e7528772f809555", - "extensions": [], - "previous": "0000026aa6724994c807db16040acc9f6fcecf4f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0e2eaa3f7c4b96a778914401bec77be8c5993e7627f4992a4caa37ffd993e3a1459064a1d66b16b4f7825cbef828cb41a91f9cd2977e759801a98c7a3a8596d8" - }, - { - "block_id": "0000026caea0792f6fb7f8367aa81ae0fe20e901", - "extensions": [], - "previous": "0000026b39aa18f65f34e61e3e7528772f809555", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20747255068eca8ba8220fc63a09a9f55dd69c5bbb5aebd43db0fb35314610d19817b71d1014fae6dcce1774ed2ff472d7dd44462e633aa2d00b5c89c954b0ccbb" - }, - { - "block_id": "0000026d0cc86bfa213550d2531251ffd4be0f38", - "extensions": [], - "previous": "0000026caea0792f6fb7f8367aa81ae0fe20e901", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f532b8c5390452a2faba178512b382c9755e860b2156eb179c656503d138dfa76147415125691aa27e929100cecc9584cc7d49abf28a05e9affaaafbf975454c7" - }, - { - "block_id": "0000026e416bf138d05f91c49dea4cb6d814dce8", - "extensions": [], - "previous": "0000026d0cc86bfa213550d2531251ffd4be0f38", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f739f99bff0d7c31a4c9b55fc7fd4de9ca1cf87cf0360adb69a0c89ca0f09d26052f70758dc1242ab3fc2e51edbd6fe74f109b9853a61c99a8d34bad1503a2ddb" - }, - { - "block_id": "0000026f4ba52b4b50e71fc4586f078534ee4a46", - "extensions": [], - "previous": "0000026e416bf138d05f91c49dea4cb6d814dce8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203db6c1bb8c058dbcca590fbe0af1b6ad04567a859df6f710d03cb9b3edd1ca490432a2f740d7ba23bcc7d5f9090ce3c282e8ed10e5b7bddd62c74bebc01087a4" - }, - { - "block_id": "0000027046b5f55d58f98d8197c78ef4254ece24", - "extensions": [], - "previous": "0000026f4ba52b4b50e71fc4586f078534ee4a46", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f319f110c07af6d972459499ab1c7711b920a2fe99c26d24f471e9e50960f86205a61ebd39c17290ef11d30397d41fe69212b48b069f65c9f8656ad71c1a8b7e7" - }, - { - "block_id": "000002713f965156fe90b5a27116bb1f0122e840", - "extensions": [], - "previous": "0000027046b5f55d58f98d8197c78ef4254ece24", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f24fca60e8b474c2b17b8011b28d16df6500a1990bf8de73a79541862f17f3e8d7507780d34fe6fa4b58829a2b95dd054f21c382851f9605c1f22be2b0b2f1112" - }, - { - "block_id": "000002724edb2e3336a96b8bba420b656d805054", - "extensions": [], - "previous": "000002713f965156fe90b5a27116bb1f0122e840", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2067c5c2aaf8522a76e1a45884494127ae25c1dc24e8d2a2942804c5f75a1df9b946ce49162552d03b8e127b9332431b0982783ca818958965c5cc56a204dc58c0" - }, - { - "block_id": "000002734f287eafc4f25a367532c84f49b0ce20", - "extensions": [], - "previous": "000002724edb2e3336a96b8bba420b656d805054", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204dd33208fba35dad89b28255b218068e5ad2964dcab13b51d35490856c6762d62ec0aaed5dc77693726d26cc5d4d72513c1d61515cb5fcfe6a2e6f7b807c8d85" - }, - { - "block_id": "0000027473e92f061f823d3dd7dfbe833f52bcf3", - "extensions": [], - "previous": "000002734f287eafc4f25a367532c84f49b0ce20", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0ba5fafdf897aa5834a5bb31eb0217400b9b925e1e81029ce388cedcb6cac48163562de4c5be2bfa4a2e41e8d771f92f94760b64ee43ff2836bc5a696c01930c" - }, - { - "block_id": "0000027502dc12f1ae5a68f24e38ab1b459a6541", - "extensions": [], - "previous": "0000027473e92f061f823d3dd7dfbe833f52bcf3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:36:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2045d0491211f959870d94c56d4431393fb442465eb81f2f188e29f58e37cfac126e49e7c993b354d97613d7ecf53599c75cb38a4246faab48a7e1ed7ecd2a2e9c" - }, - { - "block_id": "00000276edc60317cb68eca2c39e1096d55be2cb", - "extensions": [], - "previous": "0000027502dc12f1ae5a68f24e38ab1b459a6541", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200f4e79f93f2ddd2c1b392239accd8adaa9bcb21085bede78ce3f1028e4ac4371399660bbc69796bc8081f8a3d8040f279e74d369ac78db291b066ad415a22fc7" - }, - { - "block_id": "0000027716429cebb2d59e66405f1a126ff53ad5", - "extensions": [], - "previous": "00000276edc60317cb68eca2c39e1096d55be2cb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f62bc6b5d55f5fb5001463ec3a99856cda64758c81469964d763713dd8044281c73e3508aa4429a248cdbe5fc9a8a9a43095149819d9684d4b083d5599b529971" - }, - { - "block_id": "00000278f85fcc13dcbc0ba4a8bf7b4500074276", - "extensions": [], - "previous": "0000027716429cebb2d59e66405f1a126ff53ad5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f59d7cc6b2ee6d9a621dd8a6294325f0344fee508c9ee494c082a834ae8d6b9936dce1ba1c82601aeb342643d146bf983f7972ce7e2d2b7963efbb3d15eea4f98" - }, - { - "block_id": "0000027913e115b61308cbfe1d053d7d10bc4495", - "extensions": [], - "previous": "00000278f85fcc13dcbc0ba4a8bf7b4500074276", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206f6ab6e505846bcd9ab6c33d66e04632756126c06123a7aa50d174da1638ea503ccde5f1fc25b6e18e31c2bbe356804d144839ff5df97873b6d9a523921c42f5" - }, - { - "block_id": "0000027a6f385ebddf89f833dd4e301966bfdafe", - "extensions": [], - "previous": "0000027913e115b61308cbfe1d053d7d10bc4495", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201fd1e8ab9cf93ea0fc990708e7f6072bf23ef3e1e4e2c11006a4031c6204b34e731557adc63b2e901ee7c72865e3ca6da3f5b90b3a1988be563956591c3f8387" - }, - { - "block_id": "0000027bd422a83d9a0151fc74d123f26db6672b", - "extensions": [], - "previous": "0000027a6f385ebddf89f833dd4e301966bfdafe", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207a323e8784fbb1c67eb96c7babaf3e936278c2b7e4c456281bb13ea065063afb0238d3e3cc7407aa97cf6cd6e9d2f5cb401d391fc9dd69ec05ce796ddebfb475" - }, - { - "block_id": "0000027cf5b7b9fdd332030fb97e53a148234f2f", - "extensions": [], - "previous": "0000027bd422a83d9a0151fc74d123f26db6672b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4def4166017271c39fda0ab54c4047e1b0f5682bfa9c9578c67397bf83fa0a102ba15701943e079c70e828bcec03e23dbb71cd9b2ab7274fc429182abf95c7a7" - }, - { - "block_id": "0000027d43b7d54f04be677e90cfae1a43e1a456", - "extensions": [], - "previous": "0000027cf5b7b9fdd332030fb97e53a148234f2f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1c32e8c1cc636fc942e978e07c9130243f6a5c891ff88c3906f59c5cffffecb158628aeb58f23362e1e6b1d4b6e0e3f161016b231e0ee08ea5b81ed140507cbc" - }, - { - "block_id": "0000027e66d200bceb27e900c55be8c809b7bd04", - "extensions": [], - "previous": "0000027d43b7d54f04be677e90cfae1a43e1a456", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20196836514b0ce6f437ef1a510877b35cf96f87035db8db10f824611c29590340245561cc327846cd71b7d9397a6600646505a4006c08b3aae815de4c2ea685e2" - }, - { - "block_id": "0000027f542be7d622f3d88ec46ea22f1f6c171b", - "extensions": [], - "previous": "0000027e66d200bceb27e900c55be8c809b7bd04", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2077242f6902f45497236f4b9811fea84081096bd8c5d57ab5a57ea028fef185041713c1e864f2afcbc7a944b0a56d9fb37b6884a415255ab798578797eb01df25" - }, - { - "block_id": "00000280aeb2e2dc9dd9bea915d00097eef7055b", - "extensions": [], - "previous": "0000027f542be7d622f3d88ec46ea22f1f6c171b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f405f4e6d0cad0ff0821cf44a0eef944168518b7c2f1be7c8a503eebde5edb0d30db30ecdb89b6ddd68fca9603386b9f97b13dd61561a4bb90beafd4f69907c53" - }, - { - "block_id": "000002811bc1adb4cf8ac22f3b1ece087c5e1752", - "extensions": [], - "previous": "00000280aeb2e2dc9dd9bea915d00097eef7055b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f05329499b9602418d0fac66d8cd85710c403691a0681712f3675d8387fe223f73d3e8dc41afafa9ac69d85c41e88ce87266ae547926e55f607e211523bd159f2" - }, - { - "block_id": "000002824df4fd3da5a4768bd1fbc3bd1357978e", - "extensions": [], - "previous": "000002811bc1adb4cf8ac22f3b1ece087c5e1752", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20575b5376dbe409c4e2852c9d190f04f21e356f0bbf75e53baa7038803975008f362d9d8ae0124e8c60062abb35a2efae1dd2259418c4a2c1f57d12367d6a595e" - }, - { - "block_id": "00000283cebe098e0decc776e89d5fa68cef7a5a", - "extensions": [], - "previous": "000002824df4fd3da5a4768bd1fbc3bd1357978e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f554b7bbc7b353ae94b713c2b2b82ffe01c6e5498bc8d3770a3b56475d85ad797580039a54377dea0d1ae164269d515c394cc1862deca4f4b2ae4f3d6e0dfc2d5" - }, - { - "block_id": "000002841f053a30f3d265eda7784231cb1a7e67", - "extensions": [], - "previous": "00000283cebe098e0decc776e89d5fa68cef7a5a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20689c10fd18d0d45ab464b62b0614fc792b3fc091e19029f225ddb86b7fe7591f7b637512fd3fea21470a86fc98e23656d2fdaf05f57a08bd5e7e91f7808165e1" - }, - { - "block_id": "00000285385d93b1e93da9f4031daa2b96037d42", - "extensions": [], - "previous": "000002841f053a30f3d265eda7784231cb1a7e67", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4e947ae1ac59238b42acc22877e0a1b9da7e69c93dd43a8f81f6cffa74d2d9101247350c2ac8230ed2cd4508a0e3ca04d74aee7f5075695bcac49fd91e4e8342" - }, - { - "block_id": "000002861835e8245a62c2c4a2e5a046032e0cdd", - "extensions": [], - "previous": "00000285385d93b1e93da9f4031daa2b96037d42", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f238b0681c31d287566a0433e4411c8288e2d33a796d5229b5d8133f9b1b5ad991e7c18052c086524c58ebaa00b543c2aa6c25b5eb213bd01c67cf524b33cfd8c" - }, - { - "block_id": "00000287d2533996fee37af5e8f8765de6e090cd", - "extensions": [], - "previous": "000002861835e8245a62c2c4a2e5a046032e0cdd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2058dad9a820db0ca38a24497b302861ff764f5c8eeb56fa5c6716641b6059910d4261189ada793219a79aef5f6916f1a140df2e87a8734b8d2449757199fca3ee" - }, - { - "block_id": "00000288ff61987d45446ad30d20b2c50342a6f3", - "extensions": [], - "previous": "00000287d2533996fee37af5e8f8765de6e090cd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f091bf0b17fafcd18323748291a48684ab9962b6899685a56a7db22d68056400261bcf12d08f68f5850c4ca3e71c19036f1a5a65443ae2667f79cc816604c39fe" - }, - { - "block_id": "000002894704799fd7eaee5001093bc6db3e5c2a", - "extensions": [], - "previous": "00000288ff61987d45446ad30d20b2c50342a6f3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:37:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1df4634d3728d493f12b1d43d0db80e0188edd1c6622757f40c97d803e02da364d86f344d668f7d300a209e57a305164d3090817efc7a0d851ee4ec36cd6ab4b" - }, - { - "block_id": "0000028ab5181efef3d464741cff2803aaa0f876", - "extensions": [], - "previous": "000002894704799fd7eaee5001093bc6db3e5c2a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2035c09f086649f807ea55ec6e672bddb6823ef4aceed91cc49ad11a014608f67265aeb7665d9543d9fb2aa986ee97e1b483117f56444c5b6275935e411e368159" - }, - { - "block_id": "0000028bcd0ef8464e7faf9205eefc5d2cbfdb7f", - "extensions": [], - "previous": "0000028ab5181efef3d464741cff2803aaa0f876", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20143996f9b9f62873c9c2f03faefb05012751a1dd99bfa3d8cd7e187503a39177473d26b6be4d9c9bf2a67896e217f9173c28a281a4b475ca2e32a9f8c6390421" - }, - { - "block_id": "0000028c7559892399cd3d2c216d8f642a49f8b4", - "extensions": [], - "previous": "0000028bcd0ef8464e7faf9205eefc5d2cbfdb7f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20486746e3b917ac519b170483024fbbfa5453f58a0cbda369f125dd18e9a76af30c08445c0d4150cffb8b24d566c83c23f3d341e7082abad2ec6dbe73273404a3" - }, - { - "block_id": "0000028d939b53caf4b60bc0eb83fbdda34537eb", - "extensions": [], - "previous": "0000028c7559892399cd3d2c216d8f642a49f8b4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2021afe68676d365b9529e3dfd852203984eb14417071375347a3f5f40b51a0b7a09b0dffbee6982599b68b1627b71fe0f8ae6916132954f33e8a9d3ecf813bfd3" - }, - { - "block_id": "0000028ed0e2c55a564402403a4e713c18c1891d", - "extensions": [], - "previous": "0000028d939b53caf4b60bc0eb83fbdda34537eb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f11a4b93c7683f0af937eef15e045b0b0d529cc5f039b4eaa708bb679b6e5be5032d0ca87d823952ffeda85fc88f92c34bb43631f490f5574d103267da65d8e20" - }, - { - "block_id": "0000028ff67f4596c02c63ccf142a07107655ead", - "extensions": [], - "previous": "0000028ed0e2c55a564402403a4e713c18c1891d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0cb6290fe353bfe80ce74263f66ea810df99670988ad5cc4ce4ae537bdda12fc17f7c9f407f74956f197650a0ca6db5a066d7104894f9113fa3495b83664ad40" - }, - { - "block_id": "00000290d9a85b03c2f9f6e67d22715548158cb9", - "extensions": [], - "previous": "0000028ff67f4596c02c63ccf142a07107655ead", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f280615456e8b6b50df764ada350303c321425190814aa202add40af5af97eb931a0173a113acd6b76e9718246492cc79c60df41683edc85ce563d8b6daef78c1" - }, - { - "block_id": "0000029170c4dc7ab5b5e0c8121b763f275d02df", - "extensions": [], - "previous": "00000290d9a85b03c2f9f6e67d22715548158cb9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201489b3c7f65c870385cf5ed9fd1e73f761d4f74dc138d7b7838dc4705cdc47530dbbc16806c067ad6f4a62694de5f48e6478e6e7c18f94cc821bb768620de987" - }, - { - "block_id": "000002922f4ce52eea20a12621cc18c5bad04524", - "extensions": [], - "previous": "0000029170c4dc7ab5b5e0c8121b763f275d02df", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205e3987caee1c239c9c7fcb7aebaddf104e7f9a4c662678320a9858b3a99df67939ae5cea480e13238566ba5502ef2be4536a0429d947074d963c756c48c013df" - }, - { - "block_id": "00000293e66a0c849efcd3dc180692ab7fd5f88c", - "extensions": [], - "previous": "000002922f4ce52eea20a12621cc18c5bad04524", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202cd637e0f3795ae87fd8dc0f9eb9b62336ce6db45c7f379fcbea1b1ac994731e4d819b85e7f5ffbb1c3a4b6536725364b9b72e4160a73e17ba0c28e9dc3ce0df" - }, - { - "block_id": "0000029480db49cd3f2bfa16478454fdd3439d0d", - "extensions": [], - "previous": "00000293e66a0c849efcd3dc180692ab7fd5f88c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200153ef35da2b5685fc73fa96c929e63bdacd1ac2fcaf4c020584c5285b8bf20c462a6acbe75cd5b9366a9c6c4809c0faf4c50ac8cff53cc17aaa32c5683187ce" - }, - { - "block_id": "0000029510b419167bdfcc9a34c8d9b793c22e45", - "extensions": [], - "previous": "0000029480db49cd3f2bfa16478454fdd3439d0d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200216653c40ba26f73f608da734f2c2db9e8abaac3e3344d7ae584cf4439c5ac4135c686cd8a2123e68dcbce087270ce90956a6dff5c95a0086ab25fe777e3095" - }, - { - "block_id": "000002968837b0dd04d485b1c346903b6dd6d3ef", - "extensions": [], - "previous": "0000029510b419167bdfcc9a34c8d9b793c22e45", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f490327af0648d2d4a3a979fe6c15a0832873c35489051148afd8df6b83d170c36de9109fa2ff736b4c886fbde5e8a09097f4999df88c2b2f1a4f77727c20bfec" - }, - { - "block_id": "00000297c47fcebef252e4cffd14a7852f045eea", - "extensions": [], - "previous": "000002968837b0dd04d485b1c346903b6dd6d3ef", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206ad55fd0feaa0c1d434f8cdf231c175df9e1cbb9d7e4f7135606e925e461711b5029be75ed15ff0bf2ad021345ee9ca50ca943a09c7f5100c2f0d6dad32b473f" - }, - { - "block_id": "000002989b8d2cce72a6ab9691653c8aaedb1212", - "extensions": [], - "previous": "00000297c47fcebef252e4cffd14a7852f045eea", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f056898175a36cc0518a6269d680ff91d1f66404cfd9fb86e92db86040407a030685c38473c7f35c16aedadd80f972913af3ca0db2fe592611c8d561953f85143" - }, - { - "block_id": "00000299a71c32024ecd663468b0e66c043d38b5", - "extensions": [], - "previous": "000002989b8d2cce72a6ab9691653c8aaedb1212", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f59361b3c1bc98359cd93bba6d2f7106eee93aee1af60ef683f3842383de1cc8f26290b2ae1079bd6d2fad3856a5cf07551607367549fcced3c63407c71e9c14a" - }, - { - "block_id": "0000029a14f439d53df331b13903128e6e80b915", - "extensions": [], - "previous": "00000299a71c32024ecd663468b0e66c043d38b5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f61e5f2d3c477a5035b3ebaf3e9db178c44fe61c23dea617755e531a208deebd82cd1026477f7ddb596245b1be185d6cfe242cdbb0a6a7796eb3ad077775e4a84" - }, - { - "block_id": "0000029beb122d3f0b3336c748ffc417791f3d7a", - "extensions": [], - "previous": "0000029a14f439d53df331b13903128e6e80b915", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f264f5705d59b7c57a102ac88cd280b7d37bd9e298b8784b82ba6b4409b655a3c1e5e994b48801a27d3cdc5922177dcd88ca10fca81b9f24961801c3a632dc369" - }, - { - "block_id": "0000029c58e29ac5d922d2c4545d98b7488dca61", - "extensions": [], - "previous": "0000029beb122d3f0b3336c748ffc417791f3d7a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f15e12ef6cb9dc1ca246fb5b0751c65e0aad068bea141fcc790e7f08e931bca4962b2b9a6dc8a04a38d651c24b3d0e0a3c6fa7e5edddb9d8e941d18904ccfe060" - }, - { - "block_id": "0000029d34597222605d705af5f82fd98ba80243", - "extensions": [], - "previous": "0000029c58e29ac5d922d2c4545d98b7488dca61", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:38:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5717d4878da2501792cf64089c7261bc5cafa7e864758825613e777273b2481b45a77527e07ea9027c1b309a4097df59a9e33a9da33a3e817f2c2b071b97b866" - }, - { - "block_id": "0000029e5c67663ad468d9083a785fa1ad5c345f", - "extensions": [], - "previous": "0000029d34597222605d705af5f82fd98ba80243", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206d69cf44aa53975abe7023c7feb90a072b788f5a9f15cdb74272a07b1b01d28508e54c064fa2c2b3a591c50f5fd373b7734c8b7169213a0835d0ba1b7697f349" - }, - { - "block_id": "0000029ffc9ed8a775d16dc061e6b56bb7a698da", - "extensions": [], - "previous": "0000029e5c67663ad468d9083a785fa1ad5c345f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f069f89a413598afbfe0a2a39d3315b265f0ebd48c8620d92f3022389bd3403be6696d097bf3e71cb52bf06ada59f83b66e24f2d4505dc3d83f783f583e612d3e" - }, - { - "block_id": "000002a05e73c80baad0fb8d6b91a5e14a3da0a1", - "extensions": [], - "previous": "0000029ffc9ed8a775d16dc061e6b56bb7a698da", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7cdd6d2d50ae8ed91054f171eab2055700b20de385626b14f1b5cb055ee0608837da27791f4c85a0f37acd004b7a1ce56ec19c2b0af92c96b1c123f64a6f8827" - }, - { - "block_id": "000002a1aa3c1cd21dbd3f4b7a554c61e090a841", - "extensions": [], - "previous": "000002a05e73c80baad0fb8d6b91a5e14a3da0a1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206d1811b8026e1b197c2b2784580174ffb546ccb7d4fbb73b4ef1684f49671e4b2ca1ca62f7e189f2c275d9386f121c2d3d459f4729f4c9d43e3f6442e94e86db" - }, - { - "block_id": "000002a2b990a6606c2939bd373d78e207c0771e", - "extensions": [], - "previous": "000002a1aa3c1cd21dbd3f4b7a554c61e090a841", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2032d740b737c58afe355fe6afe15afc550fe33aab376ee086116d673c3522e7633225abb7416663b55b7b11f7370c6630a7dba70562994d2fa6f7d1575841e3ab" - }, - { - "block_id": "000002a31103f4cd3c2540c8e2239590ab45f3b5", - "extensions": [], - "previous": "000002a2b990a6606c2939bd373d78e207c0771e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2201b1a135332156bc343ccc6b8a88e7ed5bc6c034c62113e4ab24829ded78b57b1aa84217e6717f0c0c86fcc372361268611b4ed7a16515057d991dba6afe86" - }, - { - "block_id": "000002a432b3b8bd31a6cacd1797a296f15779d0", - "extensions": [], - "previous": "000002a31103f4cd3c2540c8e2239590ab45f3b5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205af11d33d8d4adf58f0c0dc048f9f01f3f64ea7ecc1493f6c6d9c1dcba4bc20d4c9b0f9047fafb6cbf9f0516b749cc27b0f94e09f509bf80e1eaa62786941084" - }, - { - "block_id": "000002a515f579921dc24ecd952f8931ade04d1b", - "extensions": [], - "previous": "000002a432b3b8bd31a6cacd1797a296f15779d0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f42c20e6dca96c6dd0efa312022b148519538185cd136954a3af9ce5b9053128b6174c04aec71b47f3c605446a14e01edf83759ea27f9e197e6233b4c4037dc97" - }, - { - "block_id": "000002a648f2ab4e42734d1cb9acbb1b56a1919f", - "extensions": [], - "previous": "000002a515f579921dc24ecd952f8931ade04d1b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1179161829d498580bcea771e548ea92d978966acf9ff88baf6baea13eff2e196c9542fe095a209440e9783d9d187c9e1aea0eb9c0568011d6ce8a438b1445d3" - }, - { - "block_id": "000002a75b03487109f48f2889d25ee96961d82b", - "extensions": [], - "previous": "000002a648f2ab4e42734d1cb9acbb1b56a1919f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206353b2345e782bbe314dd2cd2161dc62ec355cbde072f4eb19b7853dd09e0b257676ad901c1472fb4bcc5c58b432076d27692f98f4408a62fafb358ada60d554" - }, - { - "block_id": "000002a8987c30baa6cc2672ff73e0ea83e5b71b", - "extensions": [], - "previous": "000002a75b03487109f48f2889d25ee96961d82b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206cb20414827a183476c6c5d7f9a82280cb6bf510510d287d59afd6cbef654a53352ada2bfb32528b06968b8623716282b9f67b6efa763f6e06553e0fa44dd4bb" - }, - { - "block_id": "000002a9dd486338d7e230fc60c1a3bf1c36a7c0", - "extensions": [], - "previous": "000002a8987c30baa6cc2672ff73e0ea83e5b71b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201070f490533e7610ffe8305a4c1fa898c3e9a617e24a4413d16f11b6a8c762ac295c5d2d15ff7acd0526bd8e7c4d2a5542c3e57661c8ec0e40187c5dba62b890" - }, - { - "block_id": "000002aad9e05cd1b1e39153408627387cef3b07", - "extensions": [], - "previous": "000002a9dd486338d7e230fc60c1a3bf1c36a7c0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20557ea31651df3398337b64156f6b137747019eeef87c4b67cbfe15a68930e3fc56e5f49ef49758bcfec463529f5079f5aaaf3ce41c502db5e0eaa7a4a9ead422" - }, - { - "block_id": "000002ab62d2dc7694679d145494e35adb3058c1", - "extensions": [], - "previous": "000002aad9e05cd1b1e39153408627387cef3b07", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201ca9318ebdce217479f30b395b14c67c1ba0ab237071518449b185ef0ae1f8d84301ffd531ab198351486edde69b6b8218ddbaf03e4415f029039ed1ad18280a" - }, - { - "block_id": "000002ac950a2f77e69bc37296c4564e2be4a3b7", - "extensions": [], - "previous": "000002ab62d2dc7694679d145494e35adb3058c1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20266818a2f77015eafc67cd4694f249d0a8f67ed17e12ca2d71016c37e415deef54ac5d6921ddbf7c8b4da412782e6eff87445228e9072600ee881763457688cd" - }, - { - "block_id": "000002ad6a8557fdd49bf39df985540c11ce5ca7", - "extensions": [], - "previous": "000002ac950a2f77e69bc37296c4564e2be4a3b7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201d23fabe2626bab180fe3da5b030e16690fb9144279a867a059f3126e7d4aa153e5984841c7e2209806e6fec3f11377020d22c0a4f5eaddeb13415e5eb9dc360" - }, - { - "block_id": "000002ae49ee7c616f0f77730705930a6bb17e6d", - "extensions": [], - "previous": "000002ad6a8557fdd49bf39df985540c11ce5ca7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206397a7701f281d6ea82b0d2bd91e12b4412e2e7299e67a2f005fbe5f8ff5a4946523ea2f8992bab04053447dc400bf1d9fcfa91a20a0898928ef74547774430f" - }, - { - "block_id": "000002af8ff8280a9f6dd5a77b3e81d56022bb9b", - "extensions": [], - "previous": "000002ae49ee7c616f0f77730705930a6bb17e6d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205411af18732dd83fd39502cffaa31996a32166dc6bce5b2571c71de31bf59dda7ac1aa4fce15f8feecc7e5b9889e16f774e9ffcd4f593450aacd10921d0ddde0" - }, - { - "block_id": "000002b05511b6380e403cdd5784557347e231a3", - "extensions": [], - "previous": "000002af8ff8280a9f6dd5a77b3e81d56022bb9b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207fb81d2449835d53035290bc01ee4b113c9475e35a2415fd3a2939c067d1a0e679ad71474209cfd46d9458fa78933d178aa50391ea421366238b7bd2caf971e6" - }, - { - "block_id": "000002b105e89e9e7fecc61ed7b13e8782942d72", - "extensions": [], - "previous": "000002b05511b6380e403cdd5784557347e231a3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:39:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2021614b24deed209814e21f27552c24856fa80a5d50aaf562bf7fc567f9c3d6395a44644f00cfb35fcaef02f2c4e157a3789538929586a737c65d65c412feb98e" - }, - { - "block_id": "000002b267aaa347b13858f61a595eabdd85705c", - "extensions": [], - "previous": "000002b105e89e9e7fecc61ed7b13e8782942d72", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201d6c0bc2349cf55e9db4131341297bd29cab241c504c820ecb3e83609960f7374665b196a682ea488db9576af5731ccfbbafaccab8cdbcb6fd38dace96c84bfe" - }, - { - "block_id": "000002b32188d7f65c3b77cc7a253f9a4b0e3fea", - "extensions": [], - "previous": "000002b267aaa347b13858f61a595eabdd85705c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3ea759d7dd4e515a2dd69513b3e3538f6777b09ba30eef9a177237a6d06d05b96a5b983e3ccf3ebc230dfe2718713ecca44338096a6602d91b5872eaf6b94e4d" - }, - { - "block_id": "000002b4da61babd2085413047ede9adf9b03077", - "extensions": [], - "previous": "000002b32188d7f65c3b77cc7a253f9a4b0e3fea", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f77104d12717788240091b6e24b644e5bd17d1d8eee466d9d1d1163103b02d47a50b776b25cb5be487f8d307d3312515322e3703ec2c908e7e2ef7dcc9a38bc7e" - }, - { - "block_id": "000002b5f6952a781128ab99e1cb0ee6c464e931", - "extensions": [], - "previous": "000002b4da61babd2085413047ede9adf9b03077", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206eaa67ec372137b600be9b412634f761e32b0436780adc57a0f1a577a03e692c48b7d8102fbced259e0a39a30da888c08c0dc6434c161a51a91f61a27261c0c8" - }, - { - "block_id": "000002b688c7868cf7a92454cbceba1ae4ccedec", - "extensions": [], - "previous": "000002b5f6952a781128ab99e1cb0ee6c464e931", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f74d6c2b846e175159b476780d7fd5a0fed3fd6c5f18d3517af97861276f37815289652a9db2b117b6f182ea3358c7bd6980c7b0ff7d7135520349cae62937abb" - }, - { - "block_id": "000002b7ff2450cdff933a82583f302ea972fc99", - "extensions": [], - "previous": "000002b688c7868cf7a92454cbceba1ae4ccedec", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f47c156c1d8e672943a14a70aad8ee604699199b79ecc8b659e9e48315c94cf7538411d7a2c155dd2016764541874857b8795a6e3e0b37b200165d41cafc69cda" - }, - { - "block_id": "000002b868bf7d20dd65ddf2ec4c07f982bff0bf", - "extensions": [], - "previous": "000002b7ff2450cdff933a82583f302ea972fc99", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2041313e5f40f7becea7229cc27e0f4a2eda2cfc20b64ea6160f489f0be153e17d23f6096cd907859e0d513d8cb8517dd5d88b654a58917788da1e7bc7449bc4c6" - }, - { - "block_id": "000002b99b49fda8a8111f080c798bc4cf3ed65a", - "extensions": [], - "previous": "000002b868bf7d20dd65ddf2ec4c07f982bff0bf", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f664447b3a6f37c5c638ac872b4366d49c2a0c1bad886cc87b6b9202e35c07eb876f4119c5ce1724a8fbc81b38ba42047307f163769024a33b4ef631d1c5a1e97" - }, - { - "block_id": "000002bac571eb801cb9770277ff848206b10eb1", - "extensions": [], - "previous": "000002b99b49fda8a8111f080c798bc4cf3ed65a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2000b1a1f70963f434fa09381ac8f8dbd0944ed64c501469cd06a3d6961e7640702444acabeebf2154433d7724354f8fdb52a0bcc9096f19857fc690dd0daed680" - }, - { - "block_id": "000002bbc1d94a423cffb94c121f8f4ee2a2d146", - "extensions": [], - "previous": "000002bac571eb801cb9770277ff848206b10eb1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f16661f2d5c3ee2c705fa1906dc17aaeaa3df7e2f63f2933992facb72c71aa4206de63989e2ee95bc6d58401485eb2cee22588dcfd9a92a7027b63b82e1f337af" - }, - { - "block_id": "000002bc8c68a6dc9133cf8fe0e9cea03b07d744", - "extensions": [], - "previous": "000002bbc1d94a423cffb94c121f8f4ee2a2d146", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20565993dcb5b744fad27ac7bac21160f6ccf05f41970a366d08f5d819a825037d4f76f87751e10efc6dc64eeb25281455ee5bc1542b2aaab2264e00bd631fee5c" - }, - { - "block_id": "000002bde21a7c5e5e0c7ec6d11ca1a795c47c52", - "extensions": [], - "previous": "000002bc8c68a6dc9133cf8fe0e9cea03b07d744", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f49aed78a89b25cf7ef00c4590767f722c420b1796a0bcc9cd3dc876e56cc178420fbfa2209f0c7900d04541f26e471416612d24d193e909c2931870e370175df" - }, - { - "block_id": "000002be2acd7fbed1976f2f6681a2d0ba5e4ef9", - "extensions": [], - "previous": "000002bde21a7c5e5e0c7ec6d11ca1a795c47c52", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200c37d1d0fd3cc80380ba6ce06ef9cc97553ee596a999c8e65f2e999b11ef172c40e0135ff2c4e0951780ec4779750716dff0cc7e877a5e4b41cca19d7482d794" - }, - { - "block_id": "000002bf868dfc98dafa068d0fca03decd7ac342", - "extensions": [], - "previous": "000002be2acd7fbed1976f2f6681a2d0ba5e4ef9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201ddf73b2542d275a323d9ccbd02b9e7aa65ee6762c543058ed725cb418c593db2367132f857e9993b217c9c0aa160201fc7e9830372c9c40890ba03ea3eedc60" - }, - { - "block_id": "000002c08e279686f1103aee859553781948f4db", - "extensions": [], - "previous": "000002bf868dfc98dafa068d0fca03decd7ac342", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20195ae1d3af2ec8967c5d78776712ff443ccaef22208bde80eff922ff9c3837b378f6936c29c4c3c1b2420634f974c3d486998b31e924851f5902e135b52fdd2f" - }, - { - "block_id": "000002c1c13cacf70af73c785929da9bc02c8cd7", - "extensions": [], - "previous": "000002c08e279686f1103aee859553781948f4db", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205378129f332a8e9ea202e96b6e74543a8eb1165be459d45615e090ed469aca7935dc938c474fb357213c026267bc50e7cf6c40328fe67a6c9a9334b2e8908335" - }, - { - "block_id": "000002c271bb72eaac8e48b4644a1e7b75b85057", - "extensions": [], - "previous": "000002c1c13cacf70af73c785929da9bc02c8cd7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f59c5301fc038da8fa6cd93487f54d67acf929417c0ac1b58dca46e639ca59000218be5490cac30d065960c96f14d08a1f55a564449b033f26b4768cfa1754bab" - }, - { - "block_id": "000002c3a3f913100537e8a825556b76da391a92", - "extensions": [], - "previous": "000002c271bb72eaac8e48b4644a1e7b75b85057", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f516a99f78671c27166cea3414da7aff6292b3470620327c893eeedd94179ad62725cf484963afebf1bb4b7598fd947383ee08c17c0e78f2bd324908b172bd999" - }, - { - "block_id": "000002c4f575bbc0d5283c2a68c3e04cb13665e5", - "extensions": [], - "previous": "000002c3a3f913100537e8a825556b76da391a92", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4763267b41cdecddadc4b5eb8205a394958abf586df340bed590d2bb4a591b9a1823ff8ddb85e83a876bc0440a51405d5a23f66417d985ce21c04956d3d1e367" - }, - { - "block_id": "000002c5359bf845d58d0b3969705f2b7b524289", - "extensions": [], - "previous": "000002c4f575bbc0d5283c2a68c3e04cb13665e5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:40:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0374901c54c6b5aef46439486e3e60f4147add592ab97b1148564031838ece586ac2fa7975dac8f71a77608ab95ee7d97553e43ad0f2a1190f4bf916d8411770" - }, - { - "block_id": "000002c60635de8312e60f1a56ce14445e10e3eb", - "extensions": [], - "previous": "000002c5359bf845d58d0b3969705f2b7b524289", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202e00d6e3d11709f7007f40dab52842e649465f02048a878ed287e4567cc94eec041cc9e44630ce78e3a8f44d1ce6ccd56a32044068769596efb89f3bb80f134f" - }, - { - "block_id": "000002c7d889e17068760cd7c2b196f05f2304d0", - "extensions": [], - "previous": "000002c60635de8312e60f1a56ce14445e10e3eb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200acc53cffb87b35d89ce46d57a1f10bc4d68ad60ec363773c9676e08b41df4dc459f9a10df0015b155fa590055aae09d5999858047e195c12e5e8a9c0f61096e" - }, - { - "block_id": "000002c8b99949186ab768389c7d6243cd35e259", - "extensions": [], - "previous": "000002c7d889e17068760cd7c2b196f05f2304d0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2c072961736378936f9ae023459339ef31650774619f2e1b90338e69e6d9acb21fee5b3b8b924bb686872d7dcb03032aa9fdb8f9682c8018e1b38e4ad3ab1b31" - }, - { - "block_id": "000002c9838c071236a2d33d081fb81be9f0e71f", - "extensions": [], - "previous": "000002c8b99949186ab768389c7d6243cd35e259", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6ff0af7799dab941a3df192cad505128400835612790b451043b5c7fc6c3a6927904f740e546ad489446d93b133271c7e32846bb1e96c0a36647db4a075f245a" - }, - { - "block_id": "000002ca40c58d5f3a31cc3a80ec5d4287741998", - "extensions": [], - "previous": "000002c9838c071236a2d33d081fb81be9f0e71f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f447157191142f661a3ecc5df254914a9a177cf3d8a97309430664008c693f71138777936e28c4da5c57d6929f006e47df05940470162e42041732b15ab79e805" - }, - { - "block_id": "000002cbf74e772bba7f6e08609ca99e93bf1392", - "extensions": [], - "previous": "000002ca40c58d5f3a31cc3a80ec5d4287741998", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20043c8b375b4abbee74a88b4ef5ac7da373743d49f830391c5a5e29f8b0293cb450e1ff65b32f9fac10ab7ef766c5792e0648e89f838c5bf5a7871dff16da4ddd" - }, - { - "block_id": "000002ccb0307fd2c25b798ddab3469e21e52291", - "extensions": [], - "previous": "000002cbf74e772bba7f6e08609ca99e93bf1392", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f274e9f6ec60f9b5b3718e85b1ab79c0e39d770d977741e415361513c8af0af53459d4b8759a80d83d1869f7d3ec5780aeb3b15ee74fec311945020bcf52df235" - }, - { - "block_id": "000002cdf46c3a6cefdd33746a4cae2b18a1fd7a", - "extensions": [], - "previous": "000002ccb0307fd2c25b798ddab3469e21e52291", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2016f2c9dfff19ef8bf53daf57a6bf15e0b330e5bc2ebaac2221cf7aadd2e5fcfb563cd550282d6db52cc51623fddc05dc590bd161241f2149e256dac4b34f4993" - }, - { - "block_id": "000002ce7384581439c5ad274110bc4c18d392cf", - "extensions": [], - "previous": "000002cdf46c3a6cefdd33746a4cae2b18a1fd7a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20057fd00c253afe7e4bec05dfb3d1ed008e3448f52312a238cc2753330e6a215936b246ab47435bfcde2dcfd26414783bc0a6065aa50203c5315d49bc0d41d196" - }, - { - "block_id": "000002cf3e9e9c9845bfec06814403cedae0001d", - "extensions": [], - "previous": "000002ce7384581439c5ad274110bc4c18d392cf", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20176dc3d8533ae7e90459c150c9fb0373b4bb19fc6eb592f177a194155d6d585b1c904392cf2b4dc9bbcf265f688fb70731fefab89853b05d7e27b13d8e9c21e4" - }, - { - "block_id": "000002d07837df2e2e128463521fe6218ee7f0a3", - "extensions": [], - "previous": "000002cf3e9e9c9845bfec06814403cedae0001d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f67fcff6a04720e38b56848bb3843866b8fe9c9d34d0b1a5a2d3b6f2c2e252d55318925185dd3214ac825b980ec8ecab38029de945e98980707ef8f7905f0d69d" - }, - { - "block_id": "000002d1100d5153914607506771286e920cb2d8", - "extensions": [], - "previous": "000002d07837df2e2e128463521fe6218ee7f0a3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2aaff47c1da8f923a27eed033b9f006c313c6f4e35a421775633c0b7d328adf44fb9243d4537bce0b4f7ccee33a763932cb74525f58d9c78a31932e4f87bfc95" - }, - { - "block_id": "000002d2a963484a9d0344744f8105fff778259b", - "extensions": [], - "previous": "000002d1100d5153914607506771286e920cb2d8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207395fc78a9f7c385f6f41586e687125eae7130ea616526427a1f540fe625bc7d636e8bd6a12a2104ca0c966676a23f321df007cbcb8cae0c5d008ed245063948" - }, - { - "block_id": "000002d30a619d8c08e61e3c9b451159da715b02", - "extensions": [], - "previous": "000002d2a963484a9d0344744f8105fff778259b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2055e37593288caf15782372d354bb6608bd9f1aeae3a9693bbb9446652ea299530c45072567f636fb0887216d22c7fabe0d534bbae3f3e69f6dadcf2f05ae4b0c" - }, - { - "block_id": "000002d4ba9dbb1ee48ca0d98d42dc04299d7576", - "extensions": [], - "previous": "000002d30a619d8c08e61e3c9b451159da715b02", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2043d86afcf023481946bdbf29a6340cd99afa87f27d77e966152cce6df4bd644e27a50ab68f235728457ef22acda6cbf6a4014e6963c2f8be9651f62b7eac6b4a" - }, - { - "block_id": "000002d55bce26ac371a9015d0ce0cf7a061b3d7", - "extensions": [], - "previous": "000002d4ba9dbb1ee48ca0d98d42dc04299d7576", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203e6763f4f7bf6f875e20229937ce865e6fa9ba79451d886105e165f5866da37d018a32f14e7aa44d0deeaa437a18307b628ee09849e49728e37e5bc07ddd2dd3" - }, - { - "block_id": "000002d66cb76a8a7d646627b7c6b71498f5596b", - "extensions": [], - "previous": "000002d55bce26ac371a9015d0ce0cf7a061b3d7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207e549201b97b14d11219d736f3541186811a1e04a110ff44ec2739776da4085750bd5b46bcdd8ca97d857f08745edc3eff03188a45af8af99c417037c66bb51d" - }, - { - "block_id": "000002d7ca94dbb0f106529aff32c5120a0c5f3d", - "extensions": [], - "previous": "000002d66cb76a8a7d646627b7c6b71498f5596b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f49a625ce32f15a701d854ce3620bea4dda228ad770df74ae3970428b92b6b1eb02986437ce8d75e54f436460e2c8e4b1c052965ffe4074439a85dc634b4469a4" - }, - { - "block_id": "000002d81cb8ac1c5fd4b30604f00d6f4f5a68d5", - "extensions": [], - "previous": "000002d7ca94dbb0f106529aff32c5120a0c5f3d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1b8dbf9df79df9b6c67bbe3863ed72e85d6a489e69accae9779425371fb0b3b70867ca6a5513482b350b3eff370f7a661827d09ec556707cc3f24ccf88a48ed8" - }, - { - "block_id": "000002d9b25b97b1d5649c774a7770379b334a13", - "extensions": [], - "previous": "000002d81cb8ac1c5fd4b30604f00d6f4f5a68d5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:41:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204d5bc4d4fe8249fb5d182f6bbda0c6dd193ff4ee4a183f90f55e4e2a904f12521bb554727ef82a7f78e0d4ad6c00f567461fb00dc920b09f8bca9a82281f2f08" - }, - { - "block_id": "000002dabeba3bcc444fdc5cb971aa2a162acc25", - "extensions": [], - "previous": "000002d9b25b97b1d5649c774a7770379b334a13", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f782ef3276a16bfa38a34ee202bead12a5bea26b94c52b2cd5a2b3a6a5b25e17d5fcf1ba7d10cb21dfecf935b81788bf72c71b603f7c8437793ef7904bfe5eb45" - }, - { - "block_id": "000002db28a4666e455ce579fe9063736af4ff81", - "extensions": [], - "previous": "000002dabeba3bcc444fdc5cb971aa2a162acc25", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6140de48cef2392d9a87e92bf9280cf83dabc31a5571698d9c519c2e5a561d30648d8716dd08e646e0b72d6f5470d06f13bf09f0e628e165d3f7c28edcc8d391" - }, - { - "block_id": "000002dc800aa81e2a46d771c181077d145ca788", - "extensions": [], - "previous": "000002db28a4666e455ce579fe9063736af4ff81", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6b443faae2f56dea4095037993794c4d99cf3be0840863d1df61c4eb1407806f76ca9a5687c8451358221443b3c59ae8c04eeb69a11fbc0bfeeb8d0efb2381b0" - }, - { - "block_id": "000002ddecf726c9bf21b8eac9a3759985e95b9f", - "extensions": [], - "previous": "000002dc800aa81e2a46d771c181077d145ca788", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f608c7188dadca1bdb24c4a94fcd07007130b4a7545fb88735a8ee49faa5303c777ec0c573162fabdea41e11b34e10d33b70bb326c9924428885fa531d6b77c67" - }, - { - "block_id": "000002de49febc131ec275dd1b12030966df292b", - "extensions": [], - "previous": "000002ddecf726c9bf21b8eac9a3759985e95b9f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7754ae8ef02aefd7d4da543a440f96a0b7b5634ad7c10767e005a770ebcd10a71b6b65c7bde54d4b15d96193997bf8d1f67434fa7f879f1eb50243d9d7afbbd3" - }, - { - "block_id": "000002df6909db8bdc22e5ff19b3849e4808e7cf", - "extensions": [], - "previous": "000002de49febc131ec275dd1b12030966df292b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2034ab6f83e1c27be6674bd408a0999587855cf4c1fba8558f4f7ca412aba4496874b4909cc55f211b5bb0a539fc089c3fb95a7522a6b9f4c0287faf936f52f2ce" - }, - { - "block_id": "000002e0a9dc0efcb3330ef264ab09d6f163680c", - "extensions": [], - "previous": "000002df6909db8bdc22e5ff19b3849e4808e7cf", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20508efcaf3a3a4d80b115e0d62f4fd4e14bfb314edaaf5020276d63c5db170c1a660005d69f48f58b61d49a2334a9d197dcccffa08b089b1aef4ecd9ecc1788b3" - }, - { - "block_id": "000002e18c5cfaee0333271eed5d739e8271c850", - "extensions": [], - "previous": "000002e0a9dc0efcb3330ef264ab09d6f163680c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20108dadec279fec904dd4a2e5d9504dd13d211642f39726ae3d2ca9ddb52131cb0de7b6408f3461f4299f0186d5b7d6903ebf089da9cc67082e38461121476ae5" - }, - { - "block_id": "000002e228152679f39208132eed86c0bac6be5b", - "extensions": [], - "previous": "000002e18c5cfaee0333271eed5d739e8271c850", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203f0caed9f0a9a15af590b3279ae4260a25f196a8bb97b21b65a3be3f027795997dfcef934749402fed9e9bfa5c6740a9eaaccbeff95b31e0cbb22e8730f9d60c" - }, - { - "block_id": "000002e356b19266b01d5b55c072c2cfc0093850", - "extensions": [], - "previous": "000002e228152679f39208132eed86c0bac6be5b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1eb32de7983e0ed69cae1cfce532afd3164d1919a60d3c9584b695b8271d7abd38e26d4c4c436efcff29da2faba8f06640c0acd7db2355028f20bb045e2ffca8" - }, - { - "block_id": "000002e4180c1b1bb9cd60e8175e163e7bbf628a", - "extensions": [], - "previous": "000002e356b19266b01d5b55c072c2cfc0093850", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0a04f3f7ee102bb3102b51175ca6dd739b6897d544d0e779070afb25659870e209d3f69bc1c4a967ac2420269a67e4ca5581970335be9cc03459927d0026d23f" - }, - { - "block_id": "000002e5d72e3daaba7663f2306d1f2a2d5229e7", - "extensions": [], - "previous": "000002e4180c1b1bb9cd60e8175e163e7bbf628a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f53720248273d7d77aaf0d74ee31324e80e9c6078f299b5d9dc841738fd48671f208905b861891c23c154921fdae81f05d4794febfeb53b56c211b913cfc29caf" - }, - { - "block_id": "000002e628cb896f516f2a6ed29aa53e3b8179f7", - "extensions": [], - "previous": "000002e5d72e3daaba7663f2306d1f2a2d5229e7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f11a6ff40aae5f612a0dccc91333cb8bdceb03518e18d25746f66a1a66be7cff870cbd10d78fc89a8ad7a187d7f4be588c54100b7da6121f3c036e49fec4d21dc" - }, - { - "block_id": "000002e711a32fa86d7ccb08d67cbf37e1d703de", - "extensions": [], - "previous": "000002e628cb896f516f2a6ed29aa53e3b8179f7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f463c692e8cdd7188065d0ce2b37381e3067b0e4de5cb7caac59bd1c0969370b05cfd3279531aaac0ca740d21dddb3975dbfa666c105050ce9d55c68c85806649" - }, - { - "block_id": "000002e87fc0031f70a51ecb221efd6e5145db36", - "extensions": [], - "previous": "000002e711a32fa86d7ccb08d67cbf37e1d703de", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20060fc421c4282dbefb76d0f8b840c2a657b2836283815d0cb1d0398ea9efffd10d53d2d7fc12ae3c01e54dfbe934a244fdcb5e970cea5af083217022f0df53dd" - }, - { - "block_id": "000002e9f13b8ebe0f736de4640b68875de82ee0", - "extensions": [], - "previous": "000002e87fc0031f70a51ecb221efd6e5145db36", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205c3fabb58a558422abb475d87d6abef32fa7b440f01168c3a9744082e773e84551ec690cfad2a4402cbd48dfc4e1ee72f67d9706479f8f2fce34acda3bf38898" - }, - { - "block_id": "000002eac40d21d90f5126c1b39bc35564430eee", - "extensions": [], - "previous": "000002e9f13b8ebe0f736de4640b68875de82ee0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200859eafdf0693151090fc44d48708a76956eecc5d3a6ae9c3efe9d89b4df477161f19d2686c64cdcd9cfaa36ef9f1660f97b19bffe35c1b47d585973766e9b12" - }, - { - "block_id": "000002eba39876e9c1f0d16243300ba7f9217485", - "extensions": [], - "previous": "000002eac40d21d90f5126c1b39bc35564430eee", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3a8ea724d7c3b6e62fc6a744e7099b0aff9eefe004fe7990e60f3fe76b1fc4ae5ae311cc71bf896cf3cd7f414fedbb17b7d525fd8147e3401bedfc0311464c80" - }, - { - "block_id": "000002ec08414036a442e6c769cbc4ff2436a353", - "extensions": [], - "previous": "000002eba39876e9c1f0d16243300ba7f9217485", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203e31da1f388f3a7b5b4dd619e6f61a83fe89c0720abea1b7417d3ab2e30e91ed45ee9b72c31a0c0f329165f3a5676f51d242986fcc1f1950d8086457ace7fc74" - }, - { - "block_id": "000002edb80f768166722fc2e71a34870f96e799", - "extensions": [], - "previous": "000002ec08414036a442e6c769cbc4ff2436a353", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:42:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2043947566790f6edbe69722bb8c298436c72693c0fb396d63f2d487b8fba685bf143087ce45e6ccea2de1ba82895570e8841e0988d840731daaeaeaeefe065643" - }, - { - "block_id": "000002ee0706ad2f65b8c020282c72a1863f5ac5", - "extensions": [], - "previous": "000002edb80f768166722fc2e71a34870f96e799", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2043bf9438a9f3eb14df44a1954942d7f3d8bb1abdb669570c467b8e0368a1ba02230ce10c1d36ee8bc2be7f21b144c4f2c382d188b31eea7552431e71f7fa20d2" - }, - { - "block_id": "000002ef8fb597ef73939e3b5c3b6c43f9a6621a", - "extensions": [], - "previous": "000002ee0706ad2f65b8c020282c72a1863f5ac5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20354f8770a0b026498d7121afd4e95e767620cf9342f087ed11092acea2f9f24f6505e65749b753d09f54e37ca48999b79d775df377cd70b5baa7298847b96453" - }, - { - "block_id": "000002f0a8dcc7964113f32c6908b84cb5d0a2a8", - "extensions": [], - "previous": "000002ef8fb597ef73939e3b5c3b6c43f9a6621a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4c6dc403211c07915d0065d205058a6cfceb157f718d4b5131a90c80924f28e965406bb4d0c61c7bc56ed9ff623d8ac4e6701cc25096fdb16d75f95855d79d53" - }, - { - "block_id": "000002f171c90cf22375109355b5392b1afce85d", - "extensions": [], - "previous": "000002f0a8dcc7964113f32c6908b84cb5d0a2a8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0c4819613439c36d24ef36f42abbe06b814cdfa36ddb35317e94e600383e3fcf6124060c41d86fe5199b26badac3ae6a229ab630ea8f3a0a779c4574d2069605" - }, - { - "block_id": "000002f282275288ffd0ac2610f71bf5e5c6fe7b", - "extensions": [], - "previous": "000002f171c90cf22375109355b5392b1afce85d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20226e2d5dbe5128ae6dd198e9d81397c04037ade347a3c2cd1550ee52217707781e7371acbb281eb9687008f705d837c394202b12ee016efa20d2c02b27c177c7" - }, - { - "block_id": "000002f3331d135db9516c8cc0ed2ed9e4fabd14", - "extensions": [], - "previous": "000002f282275288ffd0ac2610f71bf5e5c6fe7b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f58b87f628b04503b2adb35036800e84d4f9b7dd8dbc3a26222705ba36d52a3381f7c5c328b58a6f1eef251340e3f37edade86558df66cf5a181b320b7c34eb50" - }, - { - "block_id": "000002f4c2a10169024eb6dea6584f3147b53730", - "extensions": [], - "previous": "000002f3331d135db9516c8cc0ed2ed9e4fabd14", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6bcb88a8fb86b9aa0603f8f32ba23bbdbef0716b1bcd5641c6c9f9408c08c88068f7c80ffc8911f855213ecc3af0b3c55b780a59f7dfe87a72e53b8ed6f12a68" - }, - { - "block_id": "000002f5e4f22b657b50184ad6c1ab783f1c9bfb", - "extensions": [], - "previous": "000002f4c2a10169024eb6dea6584f3147b53730", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6d79dd72ed3162677ce355e31cb120795f3189709ee552ee160c48fb74391b6356fb931d6c62bc3bd1594335920307523f5c73fa4078d2bcad5b79bd7f9370a8" - }, - { - "block_id": "000002f6b1f60b08043b0d09e62e8d3da0e558cc", - "extensions": [], - "previous": "000002f5e4f22b657b50184ad6c1ab783f1c9bfb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20543d21f14f06b4b59563933a3526aedd014dea0d4c176824b7c4c6aea05c835f4b76ee0f3f54e085eb086562c43f96f37ea9f639c5a8e2a4df80fddf5c183397" - }, - { - "block_id": "000002f7e4c825e9cd8c7f8fbfa83f85ef427123", - "extensions": [], - "previous": "000002f6b1f60b08043b0d09e62e8d3da0e558cc", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2054d47e933d2f1e8735aa9f1720b4203a5e4bcd8bf0326c2f9f7c8e6468406e776e8be256de97698f3fb81a97f974deae0d70595560ae82d5d5ec473642767ac9" - }, - { - "block_id": "000002f8f0150052861038c0b2da29c7044ecf3a", - "extensions": [], - "previous": "000002f7e4c825e9cd8c7f8fbfa83f85ef427123", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5b604e7030e931a32857a5100610c5f535b74aebca54cdf5e38ed6810e6d15b3357a407b04ccc70abf54a8c491b7437b22e39949e4a04814e3d899a3abb74b94" - }, - { - "block_id": "000002f92c4a29d33ed807df17817c9f85502c56", - "extensions": [], - "previous": "000002f8f0150052861038c0b2da29c7044ecf3a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2030bb02fe80bf4831d72d3c18b2bcd86efdf2276ca6915890d0c6be84806076951b10f405b2809c0cf38616c441737cff9c6bbbeafec47c8a37a658387e415ab6" - }, - { - "block_id": "000002fabd62c5ee4781c4e5f5a6d9b3568ba7f7", - "extensions": [], - "previous": "000002f92c4a29d33ed807df17817c9f85502c56", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4b88fc51849c324aa20d0b81f3e4fa733d8f2e088f7f158208fb7d2efb250bcc5189861b9604441a941b6e1c34e8234c77d2f22eef6977dd5ff8a815c5056712" - }, - { - "block_id": "000002fb54261020d8da26380d29b9f9c15c5650", - "extensions": [], - "previous": "000002fabd62c5ee4781c4e5f5a6d9b3568ba7f7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207cce201b83ff90c4a2088eb0384acb2495b49df81ae50bb61c943d52cab7afa918f6fac460c790a0ac54256704b32efeaec35b3f8bb806cb9f8c65f72448e9a4" - }, - { - "block_id": "000002fc86ec2c3005035923868114280e2650e9", - "extensions": [], - "previous": "000002fb54261020d8da26380d29b9f9c15c5650", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2051933e8a1952447cb396e2b28276f987a2c49820868ba3ebc5e449e71ac786c12364ffe6245eef3ae92dbe8a4a5590c63f006a18c92f857e4e010914d285fcfa" - }, - { - "block_id": "000002fdfa1b2f6b9bc12cb303749c30e0d550b2", - "extensions": [], - "previous": "000002fc86ec2c3005035923868114280e2650e9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1282f6eb949b9b6bb27f0e47522b847f0aa4fae58a1c30d3e7f7b6020d2b08737e935a141b94ec76763b9abc04ba16dca38b18aeb1f701161c55762eb3cd2467" - }, - { - "block_id": "000002fecfa344ed70cb895b142692087e634211", - "extensions": [], - "previous": "000002fdfa1b2f6b9bc12cb303749c30e0d550b2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20775b7f72409e4d24fee40308ed678d1991842efbe12c71187e767be07e3522743767d5eaa88c2750d762001e292cc81297301ec59a4cd3a5ca4946a884794342" - }, - { - "block_id": "000002ff78c5b332bde32dae30a81bb4465899a3", - "extensions": [], - "previous": "000002fecfa344ed70cb895b142692087e634211", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0354bffb714fd76908e0c1efeb22290d92694d50181185a7d911cda635bdb98443157cba925afae25490171a4574eb159d5f7bfa58c102a822212ec239434664" - }, - { - "block_id": "00000300af385970dca2ffc47313bde3c1c32729", - "extensions": [], - "previous": "000002ff78c5b332bde32dae30a81bb4465899a3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f542dc49b550c3559f7d3c3e8ffe44c46ce5c3217c2d78fe2ec76813569546f543d7c1a93186980c04cbdb9700b6db9500896e454dea45a38c4555a678170b0b5" - }, - { - "block_id": "0000030158480240e4ba025950dfe836d9ea870c", - "extensions": [], - "previous": "00000300af385970dca2ffc47313bde3c1c32729", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:43:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2044b56ae487a7f5c094d4b23584c4f785995056357e347bf4c2f486c540466b8f2ec37e055326a432861e51f81a281cf9693c5069ee18cebd260092123462803f" - }, - { - "block_id": "00000302b1c4feb1aa657ab04a7aaa01afa4e6bd", - "extensions": [], - "previous": "0000030158480240e4ba025950dfe836d9ea870c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4557ac7fd11884f030f96ae4261fc0adc0129852923c020a0fc86f8418492bb53fcc24df43e14a05a7a15856d9a74be9529c92a9060112ceb70bc4f5e2ff6586" - }, - { - "block_id": "00000303c342c3c6c1ae315548606c421dac426a", - "extensions": [], - "previous": "00000302b1c4feb1aa657ab04a7aaa01afa4e6bd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f143db73c552751873d42a44c0806d4d067ba38b29079680a7134772c513564882a5acfb781840382777484e93c704b13253c0d9ef9ef6f0f0af2d184ac9e72de" - }, - { - "block_id": "00000304991ab6de66e7092f4aa9412371920f54", - "extensions": [], - "previous": "00000303c342c3c6c1ae315548606c421dac426a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f17bb3d955d369f744ac5b5dc36c7c41344065f5ca68c714a25c68cfc9cf6373d461bc0b46c7425f876fa2543ba1388400d24fe4fdb8fd487dad06a70f41dc2e6" - }, - { - "block_id": "00000305fc4dd8148072edd8301e1c7cd8e60600", - "extensions": [], - "previous": "00000304991ab6de66e7092f4aa9412371920f54", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202b156943f0f009e7d9dfb03b9b27b56a15e38a7e96bef056b7859cc552d106944b45bf59c69674afd0a05fc4f376f228b563b0c845c1d4940f36fce7e6030ffb" - }, - { - "block_id": "00000306fac3b4c64a47901b79944dcaf025ea2b", - "extensions": [], - "previous": "00000305fc4dd8148072edd8301e1c7cd8e60600", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20654437e2378222a2ecbbe5d3308c15d1fa62822ee395229ce64487759ca130074d42ba3685dd643cc4b4c11c6bf1c88ff58f5a6076e558bd961e80be117b1016" - }, - { - "block_id": "00000307c412d50af87b0928d8526233bf2fd325", - "extensions": [], - "previous": "00000306fac3b4c64a47901b79944dcaf025ea2b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20372ccbf0254c90e775dd12f34ae944c877dee4e2969f87cd2aaabdf6c1e6dfdd6b951ecae1ce8d7db3d2039062ef5750d7014efbbdba0dfa3f4202538b947a26" - }, - { - "block_id": "000003089e7485c3a8c072c8ba57398d592f451e", - "extensions": [], - "previous": "00000307c412d50af87b0928d8526233bf2fd325", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6c3534ac47d0e1ae35bbe140d668c079b0f106e7f04d2566e8f5fdf12d4aa08f2e60d93781eca09cbd11674ba75447a086148ae30ce7e8e66042b9cdbbc08fcc" - }, - { - "block_id": "00000309334ed7d7f25602db840f671ebe621b70", - "extensions": [], - "previous": "000003089e7485c3a8c072c8ba57398d592f451e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206b830df1f22e872ac761ef4eaf3028afe9d707700c45a4155dc6cb9cd945d8424a167b0412e94dfaaee6810e1c45dba36f1def8de87efb95cb2f903b9187a82d" - }, - { - "block_id": "0000030a5abdbd31f663bd679678408631c5b385", - "extensions": [], - "previous": "00000309334ed7d7f25602db840f671ebe621b70", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5fecf1279d1d401fb6264645a8bf6fc7531120c65607225dae799a5632eb8afe70c15afe04bb112aac798e93282597b200c4c6bd0299a9f56127043b7784e95f" - }, - { - "block_id": "0000030bbe03bdeb2e8569caafc3c5d802c79038", - "extensions": [], - "previous": "0000030a5abdbd31f663bd679678408631c5b385", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2037ce783df015db87d6431d9288859f32d3a429e509fac2b58ff2e71eb615939a0d14eeb6044af211b95d7fe3b032c95c427bff24eca9a22579d1277989b049a8" - }, - { - "block_id": "0000030cac7de229dcd1ee594bbcc94f3acafdf6", - "extensions": [], - "previous": "0000030bbe03bdeb2e8569caafc3c5d802c79038", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5cc7601e72aa4ee8e698d9f9a91a6e6a76d0d72f28f7c5690e36f644260bb2c71954a7ae5eab01d379229951b35788c904f3f8bb3f2c4e33bfa7abc846fa2c7b" - }, - { - "block_id": "0000030da97a5009c5ea6096eb23345b99d3335c", - "extensions": [], - "previous": "0000030cac7de229dcd1ee594bbcc94f3acafdf6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f495459c85f9d2f26965ad72475444a1f1a39fe40780e40ad33f77153f2408e7f4758726150eca82e96c105ad136cb22d9d155bf06ad5457d334710757c88a64d" - }, - { - "block_id": "0000030e830ef4ac3fe14fe064f26066b1b8e51a", - "extensions": [], - "previous": "0000030da97a5009c5ea6096eb23345b99d3335c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206d4cd35e2fcc2ad345ed8985e46fdecbcfc3dcaa2ad4aa8f8ba1dd9e45e0bd955b9e4c72432d4cf5462c34004a2e5ac77cfd1cca9295ba783c51cf54f899bc4c" - }, - { - "block_id": "0000030f5b0b9b6380031bdf24b4b109fbaa959f", - "extensions": [], - "previous": "0000030e830ef4ac3fe14fe064f26066b1b8e51a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f330b740cc26501e7081249a6ea974c001da568f2b48d1fdba9f3c19d8f101f5f16891b308194ff1c93b2aae7c5fd60e9291981a826727b94117cd67df832f76b" - }, - { - "block_id": "000003103d188ed5a72d9c9fa8218477bf612774", - "extensions": [], - "previous": "0000030f5b0b9b6380031bdf24b4b109fbaa959f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200e9df3c3e152bb181781eccfd74ddc00f9c46ad9cef64f7daf027e3137c9cbf15c9914475558a590314270c4fb0a68ebf18743ca70b4a5505018438659349132" - }, - { - "block_id": "00000311e45c6c2b0f9521297862d0fd44bd2fa7", - "extensions": [], - "previous": "000003103d188ed5a72d9c9fa8218477bf612774", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2021cffbcb8dbb5d885a7320a63e5bb44a2cff54d4c507f0b2fe6c122b5ae7b25d43a03b74f2eb3f3e7f717d706ec7a54edaf09b86ed09f5d1205661f70a500553" - }, - { - "block_id": "000003128942fd608acae34f080e508485cc4dc3", - "extensions": [], - "previous": "00000311e45c6c2b0f9521297862d0fd44bd2fa7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207167fb57d6187bf0a7a66258ed4c26ddc3e2cd0c68f2aca731dab69651760afd5ae0a963d10fdfa3eb4ab23dd9390704312f812e3c986df7ed30f194a48748fe" - }, - { - "block_id": "0000031306a2be03c2dd86c2958aa4777a481017", - "extensions": [], - "previous": "000003128942fd608acae34f080e508485cc4dc3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5a22d254f66819672882fb917d06a00bce3a1a8e82729dcd22f0cf423fac752f5450679ba52be8883e3569dd4d78c9e11b41600fce64b4187508e49430034594" - }, - { - "block_id": "00000314c1f0ea5a729819f310e93c9a07a304ae", - "extensions": [], - "previous": "0000031306a2be03c2dd86c2958aa4777a481017", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4eeba314f7eae26e33a8a826c454ab3cd7e81e39e9a1dc8128b42cc48e333a5802fdbf47a10cd94dc20a197959bf1a3ba48cd741e1b1a4fe8dd666e5c5453c24" - }, - { - "block_id": "00000315478cc8a77ba7bf780e2c05474ed4853d", - "extensions": [], - "previous": "00000314c1f0ea5a729819f310e93c9a07a304ae", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:44:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f336fcf800374d56311d87bc9b708bfcae95adc26ae28bf70b2eeb248041d33724e5e30b1cdbb510c6ef7322a798f441e5d6531906caae3d49b8b64662345213b" - }, - { - "block_id": "00000316ad692560e9b9f3a7a7ab3b178dab03c4", - "extensions": [], - "previous": "00000315478cc8a77ba7bf780e2c05474ed4853d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205dd6b73d63f66bf98f667dd1773b04c531680ea1621369484a92611544b091ef26a45a96f1ce7881717fdaa60ccca1d69d307e215e9285dc503fd9b857f8ae1f" - }, - { - "block_id": "0000031703242d8974a3b2c5c4aadc1d49629adf", - "extensions": [], - "previous": "00000316ad692560e9b9f3a7a7ab3b178dab03c4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3f9fc99c5d55dcd89dd64568c595f1f2cbc5fed743d9fe367a51eabc9a46ee6a5de3f8beddde29600cbce429155bac3b307d0dca205b441b2f549af3015d8ddf" - }, - { - "block_id": "00000318e63f2d83464b8d6a6c43cb3bc0169f44", - "extensions": [], - "previous": "0000031703242d8974a3b2c5c4aadc1d49629adf", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2051a2d826f50be74010dc338c0bc4fc73c73624d5e7d078725dabf973ce9d8ae030331bea753ef8ac5f4dd8469877dd783110000bdf9c2616db17bdcce6557bd6" - }, - { - "block_id": "000003198367db19aca105727bd1dad33cdd5e6c", - "extensions": [], - "previous": "00000318e63f2d83464b8d6a6c43cb3bc0169f44", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6d36b8380e0c1cc725b7c3a457f11a41859a3456fe4197d656b2c4b45e77c6202d0d319c5791f9c51bdc23a0e3ac67c8206a9e9075ace3c6e25b2f7d2766781a" - }, - { - "block_id": "0000031ad56d51efef62f89a0a75d067fede047b", - "extensions": [], - "previous": "000003198367db19aca105727bd1dad33cdd5e6c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f19e14422a520078032c1205ef663b70cf0d3a26fa573e02b323abbb6d7b01c31478748732fc326a5b596ee546166717676bd158695b60fdcb2bbe96092c1c2b7" - }, - { - "block_id": "0000031b962093ddd72c7422149301851b7852b7", - "extensions": [], - "previous": "0000031ad56d51efef62f89a0a75d067fede047b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201946fe0ecb5a05889ff77ab85fcc9902a3ddfe893709f12667958a3a52d8a37c01794e3f88288a2b6a73a51c3aeae2cea5672c1c4f3ff596aebe0550e81f93d1" - }, - { - "block_id": "0000031cfa6f99716d65fc354aa7f674b98ead6a", - "extensions": [], - "previous": "0000031b962093ddd72c7422149301851b7852b7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7ba1c215676044f2456e783fceea4fc16615256b42cd96c5c8996c96734634837902e48f3a4fef8ec75fda38e32a0fb79a492f9a65bad3e61646a17357610b7b" - }, - { - "block_id": "0000031dee65b50d1b2e2cd15a958111355b30a0", - "extensions": [], - "previous": "0000031cfa6f99716d65fc354aa7f674b98ead6a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2060fae636f919bdf149078357246e17968398796137c294d7a47ada52885cf8d2528052331e77d49a483d5749d146b46a563fda59400b43ea7a9ab2f679918225" - }, - { - "block_id": "0000031e32cf8466bbda646772e90e0392f29592", - "extensions": [], - "previous": "0000031dee65b50d1b2e2cd15a958111355b30a0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204df7420e3440d295c3aa03a935f6f834e1d6130122a20487b6cd8011e2ac9c0d592dd3ec30f9ff04396732afd360c5667e9c70ef21bd5fc87af0eb93bb176a8f" - }, - { - "block_id": "0000031f66e98e8b0feacbe4715f48be7efaac02", - "extensions": [], - "previous": "0000031e32cf8466bbda646772e90e0392f29592", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206999ec54652dbfcd8fd5bbe77db70274f33edec292365392f4948a57d00cb2bf57499864bf7967a27ffc7908ecaacbb935430d9303a4beb31f88f68ffd42c218" - }, - { - "block_id": "000003201be0e0eb4107f48e6cfc4f5b6fb58ded", - "extensions": [], - "previous": "0000031f66e98e8b0feacbe4715f48be7efaac02", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20733fc68818f19aa83040b0edcf70c6a2167d744f2e4a148558fb61e6ab33d58944bc70cd0f38f784eddb3ec1367c2cc6281d36b8ff5b8dbb66bef179f9a0fc79" - }, - { - "block_id": "000003215a8d9de4663f3a87ef67ad88b145a0db", - "extensions": [], - "previous": "000003201be0e0eb4107f48e6cfc4f5b6fb58ded", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f27bc4dcf59fec9040a582c13730d002d68cdfa5896080cb75f5fcc9bf11ee3fd4a3c0adead60a0a393ec362c488ee2516c6a15d4830acd9b4617eb07ced9641c" - }, - { - "block_id": "0000032283c051c3864fb83620fb565e087309a9", - "extensions": [], - "previous": "000003215a8d9de4663f3a87ef67ad88b145a0db", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202a0967526e20e8e1719fca865011cf6ee5fadf536c06fc150f8d88873977240c61883316ee7528fe09f885257396427faf19c058d04893f5c9d2f62ca155e26d" - }, - { - "block_id": "000003230a5e9187a992c0dbc55e3dbc870c4bd8", - "extensions": [], - "previous": "0000032283c051c3864fb83620fb565e087309a9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2073ced5bf8d5ce4b63c3266d5e8d0a6806d5a51cf3687043444e8b7001bb906920243e9e3159ee2c3d42b696bc59184362b6f73659e6876c434b002c1cffa788a" - }, - { - "block_id": "000003248f4be5952b2309c1820533806e32b424", - "extensions": [], - "previous": "000003230a5e9187a992c0dbc55e3dbc870c4bd8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5b22e0e5928a8cf6e2adae8c7c53ba1ce44034c361559087e14c58cdacf6dfcd6df475a0f253b5609c05f3247f7f72e66aa629bab04906edfccee6c2dd18308e" - }, - { - "block_id": "000003259d4901a9ca1b995815c2eea0ed51c32c", - "extensions": [], - "previous": "000003248f4be5952b2309c1820533806e32b424", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2017509d018474c7e18cd52a733ac7c52aa41f3fecbbdcd5acd7a39a1e8637187d717e0ab272868d43454ad01589f97bfb1cd55bb8640d80b1ddc2b5ce7135f91f" - }, - { - "block_id": "00000326c3b093503f29dca8728ad695cc84e44c", - "extensions": [], - "previous": "000003259d4901a9ca1b995815c2eea0ed51c32c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20238621131fda4a9243464bdbe5e28cf6318ea772232c29d9c6b7ae8994c9f6f170c6b01ce704e6e49b4618b7c94ac6240d30d4775f5a7b00769fd69f178ae3f4" - }, - { - "block_id": "00000327dfb67ca7f468796beccb412c953f1a40", - "extensions": [], - "previous": "00000326c3b093503f29dca8728ad695cc84e44c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5046b99af1dec3073b421be94e8a74e6253ecac1419abf387c5c33c980107493031e7f6fcc4b7fd40733c871c6fcdb0bc7d7b7e62bcc39f35b813a143bb7309b" - }, - { - "block_id": "000003280ceeedf54b29dda22a8e4d0eed0d6083", - "extensions": [], - "previous": "00000327dfb67ca7f468796beccb412c953f1a40", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20136bdb4a3bafa3ce208d43a7dfecd05ff57df32c95f11a99fe805d727767677d1ad9ef00bfbc90c0d75b97f002b1c3a4fa5c7fa105c04ca69e880421617ed0ea" - }, - { - "block_id": "00000329c62f03c06a3b6d1767950dd3cf12abb2", - "extensions": [], - "previous": "000003280ceeedf54b29dda22a8e4d0eed0d6083", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:45:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204a9a3094eb4cef4807b59455069051dcc9a9931372cd305fb08ffe63add2c2770c3948e438d25d593fe1061c144bcd2bec9b947217a578525babcc3e1e016b7d" - }, - { - "block_id": "0000032ad3297cdf3ae41532b9e282f9c11d6765", - "extensions": [], - "previous": "00000329c62f03c06a3b6d1767950dd3cf12abb2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20422dd5e86059cb5248e26447cc1b6649f5c07f695c84a0fe08b2a62a4bea253d0e0b388ce93e2ec06bf1012d9710fba8d56eaf508b403df33bace57cbf9d402d" - }, - { - "block_id": "0000032bbbeeff94853a356830a08e51933ba824", - "extensions": [], - "previous": "0000032ad3297cdf3ae41532b9e282f9c11d6765", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5856b204b33ea08daed50be6858f4313a2068d865db04178e7602ba944df63895c7c58b62dddd4028ea8ee47107349a3f3d2f9b1bedf0cc1ae2104d43d789da2" - }, - { - "block_id": "0000032c319b70e4c25cb52bb68eb2a2f622d670", - "extensions": [], - "previous": "0000032bbbeeff94853a356830a08e51933ba824", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f52a787acd854cc43291c31be040e11dfd266414992135528a82ecbda5ffd788f1d29af63d94d5ab0fd7001c82455289c34b756b31dce844b86587028616a0b02" - }, - { - "block_id": "0000032da08304a1619769d6b248971fbb86fd55", - "extensions": [], - "previous": "0000032c319b70e4c25cb52bb68eb2a2f622d670", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f47f2c7c4c410ecb9080eafa08e41732c8207c8f4094b18650cdb39d77c914cc65ad7166a10110bf90e3b552fd2e9fbe7aeb99f8e1a10a18cf1187057f466b353" - }, - { - "block_id": "0000032e4de81a136357f163e8f8a71dac45b73d", - "extensions": [], - "previous": "0000032da08304a1619769d6b248971fbb86fd55", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f594e658dac933a0eb92a10e425ece39eea97adb18b2d3da2e36bd13dd3c4ba502780b30b20dc48aa32ab759fbe07945e15b85a3ee6d1633452e5805de91368b0" - }, - { - "block_id": "0000032f977e62049e190183f0424598a23c3a8b", - "extensions": [], - "previous": "0000032e4de81a136357f163e8f8a71dac45b73d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20443d942133e7a08dc8de49c4fa13771833545f1ba783d170875eece659170e8a4fd0baba0e69902edf99efdd1db41bd96fdb43980f8e3d74e8760bcaf33dad16" - }, - { - "block_id": "0000033079264e4e03d83d9ed79cb51b502db4e9", - "extensions": [], - "previous": "0000032f977e62049e190183f0424598a23c3a8b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2023ad986fdaa309ac20f5d524b05f705e3806270fd80350d6b4b36b74c0c3f8b963b09d80226346c9e574705c487f0dc8ac73f58c7e8cea862ffa67d59a179cc5" - }, - { - "block_id": "00000331303ca55e769051156daeb1dd595a5600", - "extensions": [], - "previous": "0000033079264e4e03d83d9ed79cb51b502db4e9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203e6626d950a63e6d3477487cc2b5bc502ff20a89ce913eadf29b61d02ec6877213c35bd702f68f8af06807220b86da8887455d3880e0cd747e6ca8bcae2b1976" - }, - { - "block_id": "0000033202c431b9c486fa0f72c1e8b2266ce887", - "extensions": [], - "previous": "00000331303ca55e769051156daeb1dd595a5600", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206d694f375d4a7a8b915abe74f6cc98b6befe4dfe946edcce6fc5aaad6a43cf6d173e4b2bb0a3e5a9cfe503976d25af6be68c85c19e6b61e648d135d20af7cf17" - }, - { - "block_id": "000003335b7b2143c69163b0ebcd91bff3d35f4f", - "extensions": [], - "previous": "0000033202c431b9c486fa0f72c1e8b2266ce887", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2037167591beecb5af1bc65548ba7290dc8c278bbbe16041a91e40e9e8c4697c543b1187327a9fab94b8ccc2f682e4d3af261c56e613727b350da7c031684d21" - }, - { - "block_id": "00000334281294d4b7de72864c17746694905374", - "extensions": [], - "previous": "000003335b7b2143c69163b0ebcd91bff3d35f4f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2072ab739e690b9209873e56190baa08bee28fc44abb67792738f5c58e07e99061292ada5857dc555aae7c1948511a70046dd4e55127f39b28a9758927dbb02ea9" - }, - { - "block_id": "00000335bdce6a5d483e57a53ad3f0309da91f89", - "extensions": [], - "previous": "00000334281294d4b7de72864c17746694905374", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206fa4194e1a3f93e04e34831561c32fe61c4056a69e498cbf7d38e71c7b24e1a304a1441d90b7996320eed393a81a1447277922c047583a8d40e2bf731cb4ee84" - }, - { - "block_id": "0000033699c405c6582b63ddfe6708d799f7ae00", - "extensions": [], - "previous": "00000335bdce6a5d483e57a53ad3f0309da91f89", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4172607abf9a88f4b4c9383c90cf65ea558f91b33c302aa02ce7075d71a6261b73f98022d992ee8df0ccb680ccaac5ed18f5686115fbadc7e89b204a16d43b77" - }, - { - "block_id": "0000033701291515339dee4d6a7084dcb2998312", - "extensions": [], - "previous": "0000033699c405c6582b63ddfe6708d799f7ae00", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6a26c4b3b0f3e794e972af3d701ccaf8b66212f1f65bcc7e419ad56dca54ea4a556c5452ce091856704dd39aa2864ecc1c90689c351808a6cd6011beb1d38ad8" - }, - { - "block_id": "00000338a320b95594cf31c56b127eb6920f2bd4", - "extensions": [], - "previous": "0000033701291515339dee4d6a7084dcb2998312", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2c9ec9efd132afe3a44d411b4efbe10b468eb9673d07b2587bbe17c6af146e236e822b4b86ecfea326ccefb80caea8270f5393ed6af06fe95c696c14e7f17e22" - }, - { - "block_id": "00000339594e909b3795934d462426432209c707", - "extensions": [], - "previous": "00000338a320b95594cf31c56b127eb6920f2bd4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205ce8922f348448eff085e781222d53c92d310a2963527fbb43b80e839d8172f53be4667d5492c865b1619042d4cf17acf25ef0e93a2a3358e8c60ab8c76c65d1" - }, - { - "block_id": "0000033a93dfea851a2670647c29680319ba1a02", - "extensions": [], - "previous": "00000339594e909b3795934d462426432209c707", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f264a0f5f18658faacdd2c17bf37ca71cf61c24140d05e6bb88711fb0ea9e71b30bd917362797f5ea37e0a7dc33b011dee63a1bb567e87e3f8170260c3c91831a" - }, - { - "block_id": "0000033b8667e3fb5949b5b684ac4dff15047572", - "extensions": [], - "previous": "0000033a93dfea851a2670647c29680319ba1a02", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f759be33f80a770b3c5baee6732e3ece9493a345d7be1ede33d203b9ed49e3e543ea6d97c5b4f0f3ca0b50ebe8c5355d579758c1239645fde1aba71711b88f3db" - }, - { - "block_id": "0000033cba9a31af87961a858f50fb61d73eaf4e", - "extensions": [], - "previous": "0000033b8667e3fb5949b5b684ac4dff15047572", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203c08a237d50c4654299983f9194ae1cbfc4ec6bbae79cd32c55b15b2b73eecf8195067e2a5136e32468baf979a98afebc413d02a51f9224605a4add72e80b227" - }, - { - "block_id": "0000033dfaea30c8b1a662c6dd1c154cc045a75e", - "extensions": [], - "previous": "0000033cba9a31af87961a858f50fb61d73eaf4e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:46:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3a9afe0ca7ec5432345731a769d3757db3233836c487fe2fd919e98265ace5ba1b34a6eb681b40f525bb5d6668014701c5073cde2a81e781fe6ecc6e91ac0e64" - }, - { - "block_id": "0000033ecaa1a6768fea376722e39b7c58afa28a", - "extensions": [], - "previous": "0000033dfaea30c8b1a662c6dd1c154cc045a75e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200373ee2d35633582bc8d39416d0c4cf28872a2372fdeea21600f037422996f0179a7e787723d0eb3d6f79a0d8f24a2d0f5be89c7efa7c9dcf29a7a618d772401" - }, - { - "block_id": "0000033f80a7ea9e9e94cbb363d6d14df9d6de34", - "extensions": [], - "previous": "0000033ecaa1a6768fea376722e39b7c58afa28a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f318f4a3aa43b72713e23584456d6ff970e725dcb7c711ca8be50eb95054b45c87168e932c1e2b568143be0f1a4deee1ed6c409c1225e9d8dd618480fef47e3f6" - }, - { - "block_id": "0000034038d7961eb3a509601ed767e32782400a", - "extensions": [], - "previous": "0000033f80a7ea9e9e94cbb363d6d14df9d6de34", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2074082635e6a0cad76c1c7a1d6e1653245be956063d42b29c1791462d04658ecc253ece60c0419503182f0c432b84e4c69dfda27aaa35ca9fe5592618db804cc7" - }, - { - "block_id": "000003415bc9da76c735bc53dbd6cccf44a6d011", - "extensions": [], - "previous": "0000034038d7961eb3a509601ed767e32782400a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2065cbd1b5edd75b4cc88df828c55a5cbe80c2b6fa7169dd0f1c78507f82a5428d0553aaa306dd27f3f0ac2a921a730adf4ba6a432bcb3bfe8b2825cde027b8f20" - }, - { - "block_id": "00000342f162a3cfb3b3751f01a3b2a553f0f6e1", - "extensions": [], - "previous": "000003415bc9da76c735bc53dbd6cccf44a6d011", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0d52be93d4b8bf9970af808b19fc6c89e5ed20b604f15dddb710cfcf3496d39330ca1bc3c04f99d0cc4a0211f4115a5ca4b14360c729f17b7164eced3a9949df" - }, - { - "block_id": "00000343110e8407f6c60e19eeab52c81dbed445", - "extensions": [], - "previous": "00000342f162a3cfb3b3751f01a3b2a553f0f6e1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6ae1ba8100e0ba651345248046bc190e187ec71e93e41a41b3a213dda8b1487c721c92cef0da2ef974e3e5f5e32035317f1ffd9f83ec12fb9e149105d6219bea" - }, - { - "block_id": "00000344e2667f82042add8bb613e180c74ed5f3", - "extensions": [], - "previous": "00000343110e8407f6c60e19eeab52c81dbed445", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20218fa28289610d7d20ded7d1299b1df7c27bd0a36dd22a7104f0680f76027f9332c9d1f0b6936ab3a25f3ab1df1d879a32a8c49d8530969143df0938074053ab" - }, - { - "block_id": "00000345e4fed452a450605b70377c1c4e739685", - "extensions": [], - "previous": "00000344e2667f82042add8bb613e180c74ed5f3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205156d6766192373de7d911f6e559085dbb07a710a731a4ad4e2446c692c977730b2cb7932f8c82ffe6f3ec0583630596f02949e2c464b2c23e5d1351f170f612" - }, - { - "block_id": "0000034646c9de9e489c5dda504bd253cbcbe623", - "extensions": [], - "previous": "00000345e4fed452a450605b70377c1c4e739685", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f451a0f99aaa7f552411dbcf323fc27cebdb786c613e0aced1deeba4ce576a91e04201984396c3826cf4c5199d32ea7037b89e3c3667c3cf7db8b6508f8c05eb2" - }, - { - "block_id": "00000347c728a6078afbe6133d170a87bad91117", - "extensions": [], - "previous": "0000034646c9de9e489c5dda504bd253cbcbe623", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203e39fd55b9ef02963690db344d01cfb09246dca6d8d8da9746ba49dc6a938a6e36013029b76536037654843083cebc18fddb1271129f9039c0c535878d5fbcb3" - }, - { - "block_id": "0000034878cbc996f594815430046bcfed9da4e6", - "extensions": [], - "previous": "00000347c728a6078afbe6133d170a87bad91117", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207c59671dde1bc7638aad92ad8eb5d3dd3944f498d0608ab1ee3d1ac43c406d02559bf40e72ffce046b15d681ea81be6b00c0de06fd1afc09da8fe1b8ba62cecf" - }, - { - "block_id": "00000349110b071df0e485ab5afe9b5b5e01d0dd", - "extensions": [], - "previous": "0000034878cbc996f594815430046bcfed9da4e6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200ffede30537affcad3bb4c789a3c88682839b11ae4b3a0f31f5d41d483cfc47957f88a482cde994eaa2bf233f98a50df65fb76f8c4b4e29ae9fbebc6da7c5aa9" - }, - { - "block_id": "0000034a1edbd8c865cf2498a5e6443924d8800d", - "extensions": [], - "previous": "00000349110b071df0e485ab5afe9b5b5e01d0dd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207cf5d3f790d006ae0428e613faf24fadbf5d4172f23693fdf787abf194b942850173138e53b5a7bcc2b4a1f6dd84a05ce92c3d38268d816ef7cd998f2fc85216" - }, - { - "block_id": "0000034b9fd293b7bc3fcb27962fcfba06e3b94a", - "extensions": [], - "previous": "0000034a1edbd8c865cf2498a5e6443924d8800d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f718cbfdb54181daee51cb071ef4bdea0e705fabb8ca9b6221c34ae62c6f8e45364f1404e9bcb06f0604b60852f11bf8f023bfae715f6e4493431e1423c6a0e91" - }, - { - "block_id": "0000034cd007a16774c684d253cf83158f68875d", - "extensions": [], - "previous": "0000034b9fd293b7bc3fcb27962fcfba06e3b94a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f51fb8ab2f0958e945416f01b224ff47c2d0eb14e7f6a92984544cc7116ca20d47e91c53321200ce5d6c1f3abfd4f530283c2d7241efc82f4e02759a5d624ad88" - }, - { - "block_id": "0000034dde1355cc6cf760fe2e036a656f5a129a", - "extensions": [], - "previous": "0000034cd007a16774c684d253cf83158f68875d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0ec567a712a7f33951db5d7a4a5401be15a360ea169b92cc7fbc3cb9427540711e784b98b53c5061c4b69d21d01d30030220641c9d033d9ed32257848466ebb6" - }, - { - "block_id": "0000034ed63c3d8ba1fb2cb9f5c5a220af955585", - "extensions": [], - "previous": "0000034dde1355cc6cf760fe2e036a656f5a129a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f56b6c99a59b5c09e83606ed398b772533a5fd5ae8af8d2f8ab3008b9d50af61f692f177c84b3cc3ed2d1c2888511548bb77715ca77facef329627f6fd8fb34d3" - }, - { - "block_id": "0000034f47b93d3abc2740bb4979c2e37569a28e", - "extensions": [], - "previous": "0000034ed63c3d8ba1fb2cb9f5c5a220af955585", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2302b8643384fc41946de75a3b2c3155ed7b4221891751fb6249a292e4d0b14f624fdb05fd90f59c6f13153d2448e60c586f72c21296eb75bc55b32adfb9af9c" - }, - { - "block_id": "00000350197fba8dec6c814412f7f09c0b74bb8a", - "extensions": [], - "previous": "0000034f47b93d3abc2740bb4979c2e37569a28e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6d7cea93f016ee6424e99150d07c7e4a71175412f89789486cbbe29d69456e2026a38876155ce440a4c6765c613a5c515e3c63f174048d8513e2befccffd8762" - }, - { - "block_id": "000003510bbd69720c83865e3a2046a364ba3cd0", - "extensions": [], - "previous": "00000350197fba8dec6c814412f7f09c0b74bb8a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:47:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20571a0d3d2788c3d318c578ec0d0d1447c47f435b9a00c5dd4c5b8e147c49c72358ef203eb3d39465bc3e3ca33f8bcb8f40ec80b55eba696b586b1db2a3b884ae" - }, - { - "block_id": "0000035233133eefa5ebc778e499182c222783a5", - "extensions": [], - "previous": "000003510bbd69720c83865e3a2046a364ba3cd0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f381c4e4fbb89892a6b855542c41c4ea29aff0b121d3191dbe33692a7378005ac770852ae3ea691552924e19010eb9aaedd23b5b76b7b77f436cb98bb11643a3f" - }, - { - "block_id": "0000035384914bd415d67d40d70260f6836be095", - "extensions": [], - "previous": "0000035233133eefa5ebc778e499182c222783a5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f49c0311acfa6151ec320b00164bbe2d6e51432d5b40f40e8830526cca2941c73572b5277f272626b075fa0c3935193d328f0c3393b1119bc1594db702532543c" - }, - { - "block_id": "00000354a2c7feed5957942d25b304c83161ed7a", - "extensions": [], - "previous": "0000035384914bd415d67d40d70260f6836be095", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7b0fb273e263cd9c1a1fc60cbda62f6f918602b967c651641b8d1d94c63552b2748f5271f7cf441c69000878837329c5bf30c8c95c32bced67d9bdac081c4fbd" - }, - { - "block_id": "00000355f4d74f9369b75140275dcdad1b25f2e3", - "extensions": [], - "previous": "00000354a2c7feed5957942d25b304c83161ed7a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206c86c99abc8b067cbf1b2b66f3b24732676976df24f4bfd1517056a5d5876a7d0530feebaa6e15a2552e5a42e2e6ada3a593f50938cf422f15d83eb103438ee9" - }, - { - "block_id": "00000356883ff86a2ae5a7bcef1470a203003b6c", - "extensions": [], - "previous": "00000355f4d74f9369b75140275dcdad1b25f2e3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f03e6091e0174e37cce0081278cdfc54d37b329c4efde1de6947c6690e1e8f2185b2693fc35a25e06b9a2922ac3d7f6070e1f2dc35e8a18fef94b5f4a4dc2cdde" - }, - { - "block_id": "000003574102042cea02eb26b416da28b20c65ea", - "extensions": [], - "previous": "00000356883ff86a2ae5a7bcef1470a203003b6c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0d680127e04c9ba349177bb8773e1aceffc7e2c293ee3bb56f561592206f42571db7f74cd26a325c6d4c0a2d9e45638951fdf3ecdec9f981af7fa0f00330cc05" - }, - { - "block_id": "000003588bf7f820b0a309b9a0710ebb15ad3d76", - "extensions": [], - "previous": "000003574102042cea02eb26b416da28b20c65ea", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f74a22c03c4022c8b4de2242ed3355c8b6eedded4d724ea5633fee596badc13611410b775aca2717a801996e4c90327292574ff2c34a936b1076f6a7b3a481ecd" - }, - { - "block_id": "00000359ce281bab64c02b90c56c3b482605ab53", - "extensions": [], - "previous": "000003588bf7f820b0a309b9a0710ebb15ad3d76", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2001a738ff408ee710285c82d7bd3a7b30da1fdc95101bd8794618a892e1532b217e7345c12b306f59cc13711e7b2a7fcf8c04c26def13c413da9c2f125362f350" - }, - { - "block_id": "0000035a0e0d0246de3062d17b314b5d7a45f7d5", - "extensions": [], - "previous": "00000359ce281bab64c02b90c56c3b482605ab53", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1b1c45cea3e1c6ecda7c82d228467500ce9053a7891730c4fd0c4d376806426850c8c26bdf8ed3414077412d863e4ae735d4db24fb7a5924165f22c9f8f654e3" - }, - { - "block_id": "0000035b670a84c5eb70f639c62fb1320a673dd4", - "extensions": [], - "previous": "0000035a0e0d0246de3062d17b314b5d7a45f7d5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20491ff1ebc2f181a6304ff7f3efe5fc2704fb8599ab7a4d536808b5586fb9e4214498520a2d1e89ba5f0add319c0eee0f7b51119a0d0df1cfddc1ee24b73c6602" - }, - { - "block_id": "0000035c06bc2ff3e35801942f35941fa154aa08", - "extensions": [], - "previous": "0000035b670a84c5eb70f639c62fb1320a673dd4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20494edf8bc77bd3f9bec4a946fc620c034c4455da8b4915b788f395dbd885c4af3275bd350d4f6e098c936dff823d0340f1f462582321fa9f62dcea40e5eae34b" - }, - { - "block_id": "0000035db7ffac069d6ca1ceccdd07c6092fe3fb", - "extensions": [], - "previous": "0000035c06bc2ff3e35801942f35941fa154aa08", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207868364f00547f636bf441f6111aba0c13893321c8c51e82c8d45ba3b832214021601b52f0dbe405e02b6e1ca36ae71871bb27707977ab5bdfd24ce554ba5479" - }, - { - "block_id": "0000035e8da01632abfd6aa54fd6eb567f1fecdb", - "extensions": [], - "previous": "0000035db7ffac069d6ca1ceccdd07c6092fe3fb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206d78626c7b3005506cd6e1021d26467a24b0d7947b79d3a0321a28bc2260ba2f059b3e027603a518c05c8d8e8cb088ed05171f0d6223ba2d82fdc007903086fb" - }, - { - "block_id": "0000035f5e3dc8ac199fbf569879485b5347d61f", - "extensions": [], - "previous": "0000035e8da01632abfd6aa54fd6eb567f1fecdb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4144bfbfacf435a0fbecdda435a9c4c35a9e954add38efa43946c46f6191ef2e0486d06ca3a292a702f1e9b5b38df6de1e2f7bf2b02029e9657e3547300c37c3" - }, - { - "block_id": "00000360076e7cc6ce9f5511b60e5859703458bf", - "extensions": [], - "previous": "0000035f5e3dc8ac199fbf569879485b5347d61f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2052a660a181fc70e72d47a196d5d287cb5fb1f7664987ca657f67190f8b27dd1931c84ae8631846ffd2fffefbef62361da3410070f2bb4a104cfb3e05f6c7ae27" - }, - { - "block_id": "000003613c7bc9fdacc5ddd19be769f4f78959b7", - "extensions": [], - "previous": "00000360076e7cc6ce9f5511b60e5859703458bf", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205ea16338ed0654981ed18241281f34db21ed3025b9425a59a30820b133ee12396c8a27b87d5603c8004bc4fb68ebd90ab82baa0061c00ff5087474fd4b1aee16" - }, - { - "block_id": "00000362e85c4314979f1e74cb011d195814e81e", - "extensions": [], - "previous": "000003613c7bc9fdacc5ddd19be769f4f78959b7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5b4bf1877507518d8f7218e6222afd0aacd6192ba5f2129927e375f2f9e0eff616eb6662e0c9f57307a81aaf1d33c886919fbc46685de6bc07e64efe15df1218" - }, - { - "block_id": "00000363debb45de23695f57048c12fee6048069", - "extensions": [], - "previous": "00000362e85c4314979f1e74cb011d195814e81e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200156285fddf10f52dd57bc8832e13153ca936998835168937a2952ed7257f6835c72eac652ab33047daae3c2e4b12f7ef70ee86348c729127ec713c4d8ac643a" - }, - { - "block_id": "0000036404765d4cc47acba74390ce907712834d", - "extensions": [], - "previous": "00000363debb45de23695f57048c12fee6048069", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200d1b5a98ff76415dce33a31202ca78a83d093f005b96c859fc16059526902b637b2b40e0fe599ec83f8a8498a813f9a593d791e7bbf3a941bca819f357880264" - }, - { - "block_id": "00000365c965b9bd850ae0f5224d1a0d6da37c0f", - "extensions": [], - "previous": "0000036404765d4cc47acba74390ce907712834d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:48:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2015fb5d4123692982748b6dcd0e53d6e05ee4ad24b43058fea0cadde7de849373221fdb4c4efb0d39eb4f9a1b56bb0aa0231669502a81c30346ae56598634c6ed" - }, - { - "block_id": "00000366a4f36c1a567fc37b2906dcc337afece0", - "extensions": [], - "previous": "00000365c965b9bd850ae0f5224d1a0d6da37c0f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20545a93cb3a52e9de9e75054b0f3b76b460871bd2c998756ce3663476e801ac874d41674fb9d2b55ff6eb41d74e751b3d37da532df49a970e4abd921c3d24e539" - }, - { - "block_id": "000003672b86fbb81b798cac66774d0db3ee7f4a", - "extensions": [], - "previous": "00000366a4f36c1a567fc37b2906dcc337afece0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204ee6b9ddc68c2c7104f4d2f74e445265eebe403683234b8c8cb9481dd9f8de9510d09b4b9e3502d9dc3dc125b12daf80de63ac02ba4e54dbfeef13375f0d1b59" - }, - { - "block_id": "00000368fd829ecee9684be5490449b8b0538e75", - "extensions": [], - "previous": "000003672b86fbb81b798cac66774d0db3ee7f4a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2050f6481fc949fe12c40c9549a4f12f94840db982e0cc1e602d7fc64f83b2fec86db6772ebcbfbc786f0184eb939c6e25a3a58910c2c1dad96413f587eb8a1da1" - }, - { - "block_id": "00000369ef94379fd814293a0452fc04f7c78080", - "extensions": [], - "previous": "00000368fd829ecee9684be5490449b8b0538e75", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5069c50a0f30146a377127c6773d20549300bb426eef795c98108fd14baf66826b9cc1abefd0af5af8445efd28c17f157d138812a187789a104b2345d6135733" - }, - { - "block_id": "0000036ad2b2ea50b10d23b157935c9b69f3ffb9", - "extensions": [], - "previous": "00000369ef94379fd814293a0452fc04f7c78080", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202d27a2b698d6c038b24f32f2209b60ee7f355503cbf9b2796554a26e3e100d6218aa7efe952b90e7924a761e963b7786f38191263e8a35f8f936767d229b8487" - }, - { - "block_id": "0000036b994b4d2c852544052de00c5a1aae6d82", - "extensions": [], - "previous": "0000036ad2b2ea50b10d23b157935c9b69f3ffb9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7477471c06f25b22dce5c780c0122a59f92ea19af872d79b4a5ea433c72c066c7f3e2106cf5e6b1dce8411848901e9ecf9ce8755df5343ce08ece7bc290e7073" - }, - { - "block_id": "0000036c499c0f3c78669f52b2ea5c8597b75fb3", - "extensions": [], - "previous": "0000036b994b4d2c852544052de00c5a1aae6d82", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f66509f61e6f57d188fde3a386b2bd954d5502096545820451ee182ac39015482050274be08024968a3142f9254405d6051142946a7a11bd523cc3c80e467b268" - }, - { - "block_id": "0000036d1e816bc84d66abec5ed877dc6d580c4e", - "extensions": [], - "previous": "0000036c499c0f3c78669f52b2ea5c8597b75fb3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2017ca73c04876a3bf34e00a86bc34fa5286040a5f10d60475c8d10efe3331825669f3a7ccb5347631d5b55215325c2912a5d51e47b6982de27a0f509641735f6a" - }, - { - "block_id": "0000036e86b1b456314377855dcee06ba12705ec", - "extensions": [], - "previous": "0000036d1e816bc84d66abec5ed877dc6d580c4e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201f2dc729d6ca547b7efdd80b9acdaa7a3fdb8abada60355a605cca169ff6c58c578eded6dc77ce79868eebb47477a50d48583b77bc22e7c1346f24fd0289cc6f" - }, - { - "block_id": "0000036f7322f7febe231747828db39c9f4cbca7", - "extensions": [], - "previous": "0000036e86b1b456314377855dcee06ba12705ec", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f19e96feee6d395ad1bf2f2ef52d2d3f1b5d2f956c6c92fb977b75d48ba8875af0f9b85ade0c7ef3b068c29dd8540f2abb87df4a7d4953a58c82974ba6315b60d" - }, - { - "block_id": "00000370c63fb469b47fd6664188819b2346bc36", - "extensions": [], - "previous": "0000036f7322f7febe231747828db39c9f4cbca7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203deccf42fa6279e21ec71b5fce9a0ee92ed4e51d51aa55f4dad21b968f94ab991e5fcde1dc4ce07b91ce267808bfce32a39aa1858b2e6b5f5b8fc7965fc5671b" - }, - { - "block_id": "00000371ad06b42fa12ca5747fa1eb4bf21a5ce1", - "extensions": [], - "previous": "00000370c63fb469b47fd6664188819b2346bc36", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2068f56a76ae0b8170eac577624687bfd19d68bcb89f9124313c27e4aebfa79d7d6967a46a3de1916f17c1ce724ebda902671adf627710bad830e1ac9e264e48e8" - }, - { - "block_id": "00000372e876bfa64417e8436ff23ece1b692017", - "extensions": [], - "previous": "00000371ad06b42fa12ca5747fa1eb4bf21a5ce1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205ea220c4db4c4ac5fde7bd3a93398aec12c7016e67b134f9b9d3e4569b0ddb3d04abcec2c2b034d1b11379a527828fa3682adc78c4cdff7c92c30061285a13f5" - }, - { - "block_id": "00000373964123deb087bc36a2153038d037d313", - "extensions": [], - "previous": "00000372e876bfa64417e8436ff23ece1b692017", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f675665b8c189fe2fb149c2db71b55c680dfb51305c8337aa98210756bee2234f04fb1a542035f485d91938af41aa6f3812aaa387ff00685c43dea40556fa47f7" - }, - { - "block_id": "0000037499591b761c7fdd0e2ec539a0f9a3dbd4", - "extensions": [], - "previous": "00000373964123deb087bc36a2153038d037d313", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f465977203a154d572e7b556469946343a8e8d65f5024a08ff8e3d5396ec4c8e079ae6a5f97d472bda00a4a20c09a8514a013d3ba2d373460cb8190a22d7563fd" - }, - { - "block_id": "00000375b503ed22b3ae198367397fb6c9c81704", - "extensions": [], - "previous": "0000037499591b761c7fdd0e2ec539a0f9a3dbd4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f736bd6e61c910e236471b856e8a9ceff9caac98cb3e75939cc1356823f2df24d7a59cae03be2c56439bf38f1ff2735150f214ad012875978edef7c7259ed1ba6" - }, - { - "block_id": "00000376993f88203da707edd0c6ec9188025f0f", - "extensions": [], - "previous": "00000375b503ed22b3ae198367397fb6c9c81704", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204bc4409d89c933d38a094ee56f66e7524910c8a8f1a2ec97697cad17fee4e17e0bf5153c6bad7486ec9da34ad78ee81483a59c6dbb7deb8e0c897b2eb1da1680" - }, - { - "block_id": "000003774a7665abcd42f3fc8636b19c10f12c7c", - "extensions": [], - "previous": "00000376993f88203da707edd0c6ec9188025f0f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206f077df1a6fd4881ad59bc2ce13a4a06a02ca0520fe31826569cb54a7878e614725e26f22170388cd898ed40ad1dc7cf4be50832e367bfe952fd5c567cda3d3e" - }, - { - "block_id": "0000037875b469a25224dc77c0f54e2335aab394", - "extensions": [], - "previous": "000003774a7665abcd42f3fc8636b19c10f12c7c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1a32d95e9e7aa4e478af67ba64800b9ef259852ef5cb6534c55f88ba43df44361bd331588322fe4d49aefbd3b94354f713b346aa45e089fe27c14bc9f7dd1d51" - }, - { - "block_id": "00000379bfb8557a52f651a0cce94f44200361bd", - "extensions": [], - "previous": "0000037875b469a25224dc77c0f54e2335aab394", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:49:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20731abcb4e9954aa6d659b56969bb4c553b489ec2e8adc00a6fa6c2f278dc231c35cfe1e4b2f40f8f6054e34a8ed6bb00a39de6df933800ae183dc9c8deebc897" - }, - { - "block_id": "0000037a279f9d1be8a22c758e1d1617491de056", - "extensions": [], - "previous": "00000379bfb8557a52f651a0cce94f44200361bd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f407c1c891707a332004ea47cadf215ae1d25d19da8acca6d695026ade532334c2469431ff8788f8595bfa7bb770fee2e44df448351e5669ff19806cf3d9cd77f" - }, - { - "block_id": "0000037ba822616b565df557934d4e1b3c3d42c9", - "extensions": [], - "previous": "0000037a279f9d1be8a22c758e1d1617491de056", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f59dbcba787ce57ff82c11d630a195b1f78e24704a4e40a7e6340de00b7b5bc9f555797c0c8988e4138677574c1659c0a4cd965c772e2a895f1c2d866ea0ba8a3" - }, - { - "block_id": "0000037c6c159aa26e84c2adbed0fbea05f92cf4", - "extensions": [], - "previous": "0000037ba822616b565df557934d4e1b3c3d42c9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205503f4dd270e437bf5fabc115d906123e79383e2348fd1ebdad52a1f03ac7d8a2a4764ef8ceb7cd47a491c5c3885b67517b33cc9b29a46d09b3165e86c3179c0" - }, - { - "block_id": "0000037d3e05d8289f57f492e33fcf59432d901e", - "extensions": [], - "previous": "0000037c6c159aa26e84c2adbed0fbea05f92cf4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202c625de53b53fdec812f332a1036c3a8ebb39fde78e637a9267083441d979bbb6e5312cd923d7093ca5a26d8efedfe145893083315910c5b2895b718b0269b08" - }, - { - "block_id": "0000037e61486962907c0691c8adff9016f5359b", - "extensions": [], - "previous": "0000037d3e05d8289f57f492e33fcf59432d901e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20106c6896051c1ef57e2d5cc97adfba01c2f4788214374c6ff421e009c22a3de3329028a18c2cc72bff01dbf4830612561222c10282e77466ba9f08c8b449a78f" - }, - { - "block_id": "0000037f6f319eb84a16b254bd37a9efe3d8aed1", - "extensions": [], - "previous": "0000037e61486962907c0691c8adff9016f5359b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207ed3644f6aca99fb877a94214d500b0b72d8f9d7dfb1adda99063be09a25d94b7df1285710a8d4c4d3da43f0666be937b711c1e7ba7362bec370639988968082" - }, - { - "block_id": "00000380fdae2e48eae0bf50801743bf675435bf", - "extensions": [], - "previous": "0000037f6f319eb84a16b254bd37a9efe3d8aed1", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3b2be8a18e885e22c4d22e9f9d8ceb222b0fedf4f2c89e095191d054fa1a054949a69448a41113d93f15c946a16a6bf742394378b403024934561931742cfd12" - }, - { - "block_id": "00000381a9f6931b8f03d70f4d1165f9d97a13ae", - "extensions": [], - "previous": "00000380fdae2e48eae0bf50801743bf675435bf", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7708b21491cb85c30da8b2f2177a3fbceb7d1b06e9deb2afa287a7b5fb4f6dea67c18921363dd647e994d7cc553495c76404b77aae4a6c5ca1aada9fbc53e1ef" - }, - { - "block_id": "00000382830c20c747323a8f2e232350648add80", - "extensions": [], - "previous": "00000381a9f6931b8f03d70f4d1165f9d97a13ae", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205b8381cb115c6a0130a443c8145324287194e028a1096fd1a009002d6e5fc0b10934bc48b5154d37a39fb8e13deb649aba988f9a918ce0c7254fc137b6862f99" - }, - { - "block_id": "00000383e08a34fc8262277edbf3b4c5c60b5429", - "extensions": [], - "previous": "00000382830c20c747323a8f2e232350648add80", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f13d57991204d191729bf362e563e090724f914e03691a364b42dcb4e41e7f01b3790954cc9b0a0c0cbe7d9c52e5d7380c7ad2723ce52a0075610c063c4e9cc41" - }, - { - "block_id": "00000384c7ac61af37427407275654e8d3fdfbd4", - "extensions": [], - "previous": "00000383e08a34fc8262277edbf3b4c5c60b5429", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200527bd111d9fbe2a8cf8f82f266166c8a7c05236370b53c09eb3b192bcd82a3964c39edd46ed17cddee267e6219bb07de4a1813fc95708ae524bb36a186c08e9" - }, - { - "block_id": "00000385a840f11644e320ab46a5574ecb26ce19", - "extensions": [], - "previous": "00000384c7ac61af37427407275654e8d3fdfbd4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7fe892b9aa6d054b4f96d01fec1c1c92d5e7ef41ac2ebc59dfefc1cb88d73db44e9b2406db647fa3f20f1f3feb0a6b597f880a266bbb13cb320f00e2bb6867d6" - }, - { - "block_id": "000003866b9be67924b9b6fd4157da2817d0d72e", - "extensions": [], - "previous": "00000385a840f11644e320ab46a5574ecb26ce19", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f48640f35f00a6194fcf5e530e923231a2c12ef36c5279b0459a05ba4cfb64a241dab1d0125f0b6928db615716fd45ca1db4f0568399222ae790088c6eabb8583" - }, - { - "block_id": "00000387528dbfe763abc292df17b2c1e5793fbd", - "extensions": [], - "previous": "000003866b9be67924b9b6fd4157da2817d0d72e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f22b4cbf3b243a00c065c0b41cc38383f92da3fd4cfe087cdfa14f828351f7ba8128401c9eb4d463cf7ae8bb8913f40355d9e3335a7bae4dc4a3871ec538bbc59" - }, - { - "block_id": "0000038856b6de705d4aeb1748273137a5a3103e", - "extensions": [], - "previous": "00000387528dbfe763abc292df17b2c1e5793fbd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20696080066a77d6c4b7dfa93495f737969084af7fe6552ab02f4ec365d789eeff4b7c76c0c19404dada097e2abddcc8a65e6fc158c9eee5b221dfdb340099c930" - }, - { - "block_id": "00000389ff750ee482f7e5114e40ee1ea267d78f", - "extensions": [], - "previous": "0000038856b6de705d4aeb1748273137a5a3103e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0fdaa02dbd321666f33b10133a7b4f3a083a01e568d7efe78e7813ef8bbd0fd116b0a7d94b628ac6faf5f81d961cf079e25c92a32ad16a9af346b3437b7ed710" - }, - { - "block_id": "0000038ae47024b9259db5e635ff50fd2474c975", - "extensions": [], - "previous": "00000389ff750ee482f7e5114e40ee1ea267d78f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1d5d68e9756835c16c53f60e62989e38c600a5a9456a2eebb617ca87f5ead7d8720ec4ca428b2872a1973f40b4fb41fbb8f8121e34520f3f11a2aa49b773bc8f" - }, - { - "block_id": "0000038bf3c607d600964d164a579c15cf8f94c3", - "extensions": [], - "previous": "0000038ae47024b9259db5e635ff50fd2474c975", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0e21ccdb766a51affbb9fccfc1126a826d73cb8e6f1cf5bf510acddb11ae18c97905d38f1bc1218fc2325fece7de70f6e230861ac4fcdf64957888212e77b8ab" - }, - { - "block_id": "0000038c316e0b5122c3ab36c55fc94c099d6527", - "extensions": [], - "previous": "0000038bf3c607d600964d164a579c15cf8f94c3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2fd33a26e66c1059cde54e5736dcb54e358ee0486220e6bb47abf55a89b59a7b5693ad1d4732b7332595228c8ef1f7316b44be444bbba07b62900adbe61abda7" - }, - { - "block_id": "0000038d7228f453b21cd4defd328dda98b291d2", - "extensions": [], - "previous": "0000038c316e0b5122c3ab36c55fc94c099d6527", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:50:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20712cbc17f958b93c5cb67cd410d99b9ffaa45c447bdcb8c5f1bda42f608832ee7cc08b42e4500e72863bb7befb418589abb9095a622e84ab70de5c9c0151314e" - }, - { - "block_id": "0000038e9182407885543483c5341f9e12b4767b", - "extensions": [], - "previous": "0000038d7228f453b21cd4defd328dda98b291d2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206a03af30f3060bf5a95cc980452ec1c98d56006ba17772cb5b0f50c4c4005b927963facd8093f723385f0fdd0aa35b94f71c42c07ac14d2653bc48478f780707" - }, - { - "block_id": "0000038fd9f74fef9e2909bcf50c99054a9e43dc", - "extensions": [], - "previous": "0000038e9182407885543483c5341f9e12b4767b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f67b51bff4d8f85a98fc5f7fb3e0b11cbb69411b06c2686eef48fe96e4a7922db4cab21cc7bb58b104fbe54353a6c0c05273c44521a49cc73458450ed888ac777" - }, - { - "block_id": "00000390adf67af4e3005893605d9d6ca290c105", - "extensions": [], - "previous": "0000038fd9f74fef9e2909bcf50c99054a9e43dc", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20073dbe9840486629c8996845ea6f005337e02f84543d80b8bf0b6157335969a37fa2bea2ff6390249079a13e26d2839065a164ee036c7dc4dbd8fcb0aedc33d7" - }, - { - "block_id": "00000391bf13331edd904abe947669700c226003", - "extensions": [], - "previous": "00000390adf67af4e3005893605d9d6ca290c105", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206fbddf6b27a3b38315edeb26be3d589adb745cb7e1a486baba9cfb205077eaa14709f97230766786bc8a7cfe4d131495ae7f37c4e65832aaf90e5803cc1ebf62" - }, - { - "block_id": "000003921fab6b00196b0f1613220c64628ceb6a", - "extensions": [], - "previous": "00000391bf13331edd904abe947669700c226003", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f76e3baadec51e26039a5ed4ee907cde3a9540677c19518a2a663fbca928469744652d963430ca9ef4824acf2a6dd2786db2aa6b6c715393127bc4deebfb13971" - }, - { - "block_id": "0000039356910ea172103bb57f2bf82cfd54ed18", - "extensions": [], - "previous": "000003921fab6b00196b0f1613220c64628ceb6a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203b66eb0b48ff40e4caac0d8c04b81ffbdf9a5045064889b3d1170a9537f05ad17c7336224bcd55a40f920861a07b23378ce1b5922cf3faf3cae41b0995f877ce" - }, - { - "block_id": "0000039421cb058020b811c88d76f9b99d0b7d88", - "extensions": [], - "previous": "0000039356910ea172103bb57f2bf82cfd54ed18", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20474db4a15f934bf95a4d8a8b65684cc36bb2aed61bfe3d0db78ec8b24d8a84f51eef23e64357de9299ab55e35359e379981f6f27fc45bac40582920fd6b4d7b1" - }, - { - "block_id": "000003952cea58247fdb0a62f1affd10364a05bb", - "extensions": [], - "previous": "0000039421cb058020b811c88d76f9b99d0b7d88", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f48f12c69d21d267607ffc24064db66e5fe05366b0c4a1176891184cd6c4e29ab66a1ba1a30a3c14842d2465cab93c958e3c2a1a6d359a3e905a599382f225f30" - }, - { - "block_id": "00000396ca68d818bd9cc9954965fd81fc5d4f0e", - "extensions": [], - "previous": "000003952cea58247fdb0a62f1affd10364a05bb", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f60f7b4ecd6e51ee082bef1abb6cc653d2c022a40614a6f642b3b545cc76bffdf47516b15cfdeb291605bd165db290d2c0315c095d65ba5fda1b2848057cf218a" - }, - { - "block_id": "0000039717230b50eb366c739c82ee39fc1c1189", - "extensions": [], - "previous": "00000396ca68d818bd9cc9954965fd81fc5d4f0e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2cb1350db2e22032b7edb0a8563b410184cc5108527599c04e5494bc563443853f38abee468274cf0e5642ab1d210d872dd597e8486ba1c4b5470add6c5bb7c1" - }, - { - "block_id": "000003981bd6a4cae7563874a39c957db81f2b9e", - "extensions": [], - "previous": "0000039717230b50eb366c739c82ee39fc1c1189", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20399d3878e63ac8955475274fbfd06bcccfcbc171b9360d9f525ca66ec23b5953142ebbf21ea2045364b68c17c0b4a2afeec602705ded9cee8fc989b62c5d2916" - }, - { - "block_id": "00000399e38f41a8a76f39fb6cc5d026e794eb38", - "extensions": [], - "previous": "000003981bd6a4cae7563874a39c957db81f2b9e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207e992be8345b14aa3fe9df6d31c69fae82c752439fa72f4df7d1db29118905cc266c9ef2a1f6a4f73ab25d54abcf004e71a93e280f5dab865cf7de83b89db94e" - }, - { - "block_id": "0000039a7b06d20abb1a0447b686058fac2a2eff", - "extensions": [], - "previous": "00000399e38f41a8a76f39fb6cc5d026e794eb38", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2052ad468a78b0dcddda1dc51342ea622c82883ddb4d82b0d9b406410e8c2b5a13400f2efe28b8863ff7f63e57a43da6afbbffd9c2900ad82b073bf1c2a9d6ed90" - }, - { - "block_id": "0000039ba11224df9029ca5b5bf7a6fc29cdde56", - "extensions": [], - "previous": "0000039a7b06d20abb1a0447b686058fac2a2eff", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20525ec57bdef49041b4b4a8534910dbee2556136165109b43c0e09454f3d3ecf81b3fad1f62c5f5c6fd422a537cd5726a41c2c865dbc3c0d0d019ccb94bfade52" - }, - { - "block_id": "0000039c4fc26eb22706f43f4fcc33d48a01d6ae", - "extensions": [], - "previous": "0000039ba11224df9029ca5b5bf7a6fc29cdde56", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f44b0924db55c8e81d016eead7f699f907d35a99a88b596481728c41ee8df1e845112607e791cc4c6441a708b72695ad10e3b6caeb95696a49565371cd2e03e8e" - }, - { - "block_id": "0000039df84c05c5a97aae884e2dc7f2c0aad43d", - "extensions": [], - "previous": "0000039c4fc26eb22706f43f4fcc33d48a01d6ae", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0862b4165d359f2abaa3613ccbad255ed4c9083c10489d67515746e89ca820f95f2c79fffdd41fd6fdbbc411b7c2510adbb946eedf3c9623d40a704206b188a8" - }, - { - "block_id": "0000039e1d4e3a9b4f0999de32aa0bdc41b838a7", - "extensions": [], - "previous": "0000039df84c05c5a97aae884e2dc7f2c0aad43d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2f32b8fd735417fecbdff1fbdae849b6344b54a5ad1a9a031e1d250ffee981e53dc0e4cdcc0178d44cb3efeba9bf10ff9a2d0308f9576171bcfbbb46714c9ec0" - }, - { - "block_id": "0000039fae527e8b9c2d9ae03225dbb8db701e58", - "extensions": [], - "previous": "0000039e1d4e3a9b4f0999de32aa0bdc41b838a7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4821bb18fb5e672bc7af1dfa68590c11f93911d427d6e165e8cf87e5e9d818890ac8c0eaf1ee1da5d8352ce3ffd9f431f125b3876ad429195723c96442a4080d" - }, - { - "block_id": "000003a0df8d362eca7f0de28300b2db839df222", - "extensions": [], - "previous": "0000039fae527e8b9c2d9ae03225dbb8db701e58", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2034d205645147a5a27cacbc790408e8785fdab404a0246743b652aeb1047b569c0486aa5bbcef73866ce8301892826495748e7fa715f9d7dd5eb499f176921ff4" - }, - { - "block_id": "000003a1a73e6b554f1c4545854f8addd265852f", - "extensions": [], - "previous": "000003a0df8d362eca7f0de28300b2db839df222", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:51:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f76ea084394130c01021d54b604a6e07d38c776f7128e1ed89a3ef9d189ca9716164e07dcfa82850e952813b6784e691417f6a00163b6019115c950c69c8bb36b" - }, - { - "block_id": "000003a20f7aa30464c70e5800c4f3fa3d2bad60", - "extensions": [], - "previous": "000003a1a73e6b554f1c4545854f8addd265852f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3caa78a2579e88ceccade135f81c52637c4c84115fefa808cfbf124f55b593ab631f93da018dbbb4ceec22977275b3edfe70b2208c50cd4511f050a57b4c8a94" - }, - { - "block_id": "000003a3c73e963716d2364d508f60f3ce62d0bf", - "extensions": [], - "previous": "000003a20f7aa30464c70e5800c4f3fa3d2bad60", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201c8298fad7504342cd663bb7c1ca14bc92164feaf22e7079d6943ed623f2975f5223161b75f1803981cc0d5137e857cde8b570b0e7128d79864d90f2d87e0245" - }, - { - "block_id": "000003a4cdeb10ec663031e19bd164045163b6de", - "extensions": [], - "previous": "000003a3c73e963716d2364d508f60f3ce62d0bf", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f200ea5f48216ee65baaad956a5a1effaf628b95e59b8ef95b20f192773309a1a565bc5236df9c726ba604b3d338720344495c6d7e172ece5d560f23dbddfedd1" - }, - { - "block_id": "000003a58f81bd33a993e6365f07e570af44348a", - "extensions": [], - "previous": "000003a4cdeb10ec663031e19bd164045163b6de", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205e66b41b8f4ae64e05483b5a5da725ea0fea68f5c36bb7fa643a264bfdcc19611a4043c5fd31988ed0f555bb898edd834f82aadc70d311ea4375d589c502a338" - }, - { - "block_id": "000003a6da9e7272cde4c9c41827861c2da5cd5e", - "extensions": [], - "previous": "000003a58f81bd33a993e6365f07e570af44348a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200bf3c27afa96561ed1b4aa675209d934c65b62e967f970bdd5fb7ee1240c762a57552bc52802b508054095209b44855c7e6d528f661b31cb6ae8542e2343801c" - }, - { - "block_id": "000003a7bc3f05c1d2e440d2fff5dbba0a272999", - "extensions": [], - "previous": "000003a6da9e7272cde4c9c41827861c2da5cd5e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200a6c62ca4c015b837c238f16ffde2da6b06233e0e2270ae7970a868b82d88de279615eee05a5d51bf39ce9fb081f3aa9b4149f45ac51401d447df8500608cec1" - }, - { - "block_id": "000003a8ae247aa9fcff6203c037344b4cb3405a", - "extensions": [], - "previous": "000003a7bc3f05c1d2e440d2fff5dbba0a272999", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2021535ef81a604ba066c0eb1bee35d3c5fda331d71eed7c128da62814d0128a2f701243350f103555b5d70f00928aa67246b3cf6733bae98dd2379c73270829fb" - }, - { - "block_id": "000003a995f4bbad1cd7c304cb108a566130adb5", - "extensions": [], - "previous": "000003a8ae247aa9fcff6203c037344b4cb3405a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1b143dfa58f297e68d7d8f7cef8b507c162cb0bb3ddee6dfa1bdf68c1c83bc5926d375a18a530d80c40a1b603d59a6396dfc6b28ce0c85598f0ab9818674f417" - }, - { - "block_id": "000003aa01e98ced144e67680ef7e6f0a5f95b85", - "extensions": [], - "previous": "000003a995f4bbad1cd7c304cb108a566130adb5", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2026e93f75401f9cebac902f62a786f10d4f33d0f91e5d6c84566ada68190ebf615c87ff8c218e45c2794ae62d8d83a71e3cdbf4f65529ef1742188b0f3adf2d60" - }, - { - "block_id": "000003abe10ed525b536d532a24a7aad1d52627f", - "extensions": [], - "previous": "000003aa01e98ced144e67680ef7e6f0a5f95b85", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2015d23777ad2fe6d2fb39b7124e37b32cb476d3648316f6efea11f92cf01cc42f34ce957a0aa2ddbdd2badc619f2c4e54c66255b69b03619e3e8769023fa32507" - }, - { - "block_id": "000003ac2d7c82843facc8a34e6b2c2b784fec00", - "extensions": [], - "previous": "000003abe10ed525b536d532a24a7aad1d52627f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20218650a13e572a675f8e9454a4f3e1a98bb20412e9f85f2abf7d91581133a315469960e62fc9f8441901be3d8a6532f1e480a0e3812c7901b65f8a6b130c081c" - }, - { - "block_id": "000003adc1765d2cc47a657f636b5c0e27e36228", - "extensions": [], - "previous": "000003ac2d7c82843facc8a34e6b2c2b784fec00", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2050c42d866a4d650dfc31928549678dccc715fd07d8466117c0d8557c23e3b86c6ff8b3c98e9a75386b4dfb16b6210282a2c2d1a2f58e1295086a29bb7cb53c31" - }, - { - "block_id": "000003aeedd8af7a3e99ae77117d4f624469959a", - "extensions": [], - "previous": "000003adc1765d2cc47a657f636b5c0e27e36228", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206a34f4b0f3d355dff6441ae1a1a418b7403beaa3b55dbce29c1c9299f87619b568998f235cdeb2b03190b69b80f6bfca7a5582a2dee2d1658abffd9014b30a7a" - }, - { - "block_id": "000003af61d2f57ad93ef16f4925c9ef736d3bee", - "extensions": [], - "previous": "000003aeedd8af7a3e99ae77117d4f624469959a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f7a32d46b49c63f7aa45b66830059d5801ba22fa20572a00f43af8817fd2ad3ad63fc65ca0e8a512b5e06626e5d34a616be166e1a716a28a4ad4892729daec738" - }, - { - "block_id": "000003b00b48e58c92d14b25b9bd1e9b7d18bf4d", - "extensions": [], - "previous": "000003af61d2f57ad93ef16f4925c9ef736d3bee", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1bda3b3199c84eb827aae9bac6cdb46b756a91581d0ff1cd054f22cb4b9d07b521d6a3f925c8728887cc36e8825a4ec88d2f2637d652e8914ee129663dfc8e97" - }, - { - "block_id": "000003b1392dae959dec9816252669fcf0d01bc8", - "extensions": [], - "previous": "000003b00b48e58c92d14b25b9bd1e9b7d18bf4d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f141cc1f2f9da91f78d03add6f084ec8842e50a0fe2d801ffa5e49d237a4c668f3108e12e3ecf4cbf82d7c6bcb0382da04c4eec2d93acbbbc720ad568dc2f3832" - }, - { - "block_id": "000003b2508df5edacbce0a6d670d5ec24a954dc", - "extensions": [], - "previous": "000003b1392dae959dec9816252669fcf0d01bc8", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1f9bffddcc28fbb52d62ddaa3dd142e7f113c31e1b6775819b47538e9d957c0f5bd0cd6f2b79cd1485915f4c21e7c6d7cd3c8df5dcbe3ad9049de211e8b722e9" - }, - { - "block_id": "000003b32c4bf541f8cf884f171ef4f494f45b05", - "extensions": [], - "previous": "000003b2508df5edacbce0a6d670d5ec24a954dc", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "205a20a9c8795d1add5f8398b3b516b38af18b8587f224e1ab5254465bf5b5b04034557a533ee2456d7ebdf4cf020c7c43f59af72c3c28fbd8b857fd90463bfd51" - }, - { - "block_id": "000003b456cc307941bdac31cd88a1ad5a456af4", - "extensions": [], - "previous": "000003b32c4bf541f8cf884f171ef4f494f45b05", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2075d25a4004f482f64b486fd07a12fec85cf27efa6e6560fdd6ecc2eb48444cb01c5becf6291f5edea08d22d6cba7a7e26e0d035a67041b3a74fc7a8fd39ea6be" - }, - { - "block_id": "000003b54c376aacc10db6bb6df3f5d88f9efc4a", - "extensions": [], - "previous": "000003b456cc307941bdac31cd88a1ad5a456af4", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:52:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f132cb2a3f34044df8d209eb38481151f9cebb82254ed8533bd9bcd49f3fd14db245e54a37ef1b28ee6a532b47915b57479d124c5e9211d195412389ad7c3db0a" - }, - { - "block_id": "000003b67ac2835b580e39f53f4ab29bccf70bae", - "extensions": [], - "previous": "000003b54c376aacc10db6bb6df3f5d88f9efc4a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1d33ab915c774cf5105fbfc11db0719ef5e81743be1e64158304e39a6734648b491462b4603327db099f52d1984fc3291618b041ae9a4bb7e99757c506f19486" - }, - { - "block_id": "000003b7f646bb9a75342e08b5dc3b3d0c7d0404", - "extensions": [], - "previous": "000003b67ac2835b580e39f53f4ab29bccf70bae", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20199d1c809c5d04b07377ebaa3fdf4c567c0b827f029e6aab1b9baecd8f19cdc40d821e82033112c68b7ab53fc5ab011c786cf70e64e551b5a08dbf8abd70c323" - }, - { - "block_id": "000003b83a106eb18d7aa4cfccb8b4b50222a5dd", - "extensions": [], - "previous": "000003b7f646bb9a75342e08b5dc3b3d0c7d0404", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4213ec62e817f860ea2541098837d69745a22cde3301f36c647f355c2092ee6b6cbf91b0884888e9d14a444670d7d66086db05f906da5697f0a9b7a6a13da401" - }, - { - "block_id": "000003b9b33531975d2720016582223badeb361b", - "extensions": [], - "previous": "000003b83a106eb18d7aa4cfccb8b4b50222a5dd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f68023e73ec3ed9771a5ff1550c0bd8a378320a85fa0096c5d439d30b7d7eb3b4492b69c88fe48cbec272ced162a8720a6851b601b6b0ebf6603e3fb70f986d3d" - }, - { - "block_id": "000003ba6a651a956a49fe073e50fe569f077ad3", - "extensions": [], - "previous": "000003b9b33531975d2720016582223badeb361b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f46e67a09f123e9b5d9e187765336d9cf88909f063be2e47d92459cc49da7a0b46bd5f1f385099b4841a38bc2cff012387af3d202bda32ed4dd342b8096069971" - }, - { - "block_id": "000003bb0abe61267488eb4d91b28052cc3f4539", - "extensions": [], - "previous": "000003ba6a651a956a49fe073e50fe569f077ad3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f19c5545643dbf74b0ae67d2f08831f19a44fe28a400be300d1730943171158712b24d858aa4c32ac533dee19c241f325c630d92c0575375b5b86356a921ece0d" - }, - { - "block_id": "000003bc3b0bf55bcf33e1d188ef780a01263c72", - "extensions": [], - "previous": "000003bb0abe61267488eb4d91b28052cc3f4539", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0fe8765664c9659a3d28e260bdb1acc3964394f1019e9895f6eca9c933d92c7b2f8a1818b77ef3243bed4cbc7a81eff4165e297e1d037af718f13f286b16d17b" - }, - { - "block_id": "000003bda565990464059064394b712b970ed675", - "extensions": [], - "previous": "000003bc3b0bf55bcf33e1d188ef780a01263c72", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20248e013d91cc35e420e908a009af56e27c3b55d191bf1736cbd1342f0bdf437537bc62db2464be75fda05a13b07e90dda8644f05b3e4d2ed753353a1c0bb55da" - }, - { - "block_id": "000003bec7c924132ea71e58247d61bb7b2020b3", - "extensions": [], - "previous": "000003bda565990464059064394b712b970ed675", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f45ea0cbd80830120ecec2b4010474719e2cd1e0e79a7476eabb24ec4a6a3f87c256842193b8f3bf24e954ebd54cf6c3762828977f9027e7571c8dd244740bdc6" - }, - { - "block_id": "000003bfa779e861df10fd8b5ec8af7fa0602789", - "extensions": [], - "previous": "000003bec7c924132ea71e58247d61bb7b2020b3", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3f0af8949ba92c1770de1cb44e0b552690f196b5c28d72c3585239f3fae8a15f5e7d5bb8fec4e6fa5e3e4e274e95b309c6b5eb8041ef5d104002650eecbe5e7d" - }, - { - "block_id": "000003c0f3f0572874d2e1f0e75e4f87c68c7fa6", - "extensions": [], - "previous": "000003bfa779e861df10fd8b5ec8af7fa0602789", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f65ab7871207387e76787d513141e400bf01f3b0f9e50d4b4cb0aae2a2850a09b49831034555a73f469e15f0cbe0352f737f85fc9284e57c278c32885aa0d0660" - }, - { - "block_id": "000003c11832e86450e7b329b94cdab8aca89304", - "extensions": [], - "previous": "000003c0f3f0572874d2e1f0e75e4f87c68c7fa6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f250470577062beba0679c322ba661a0c09376fcfc4d5e2070baa25e6a213977d4a531b37e016b52553a26a01d39db9376722921d9552d84df7d422e55f50f96e" - }, - { - "block_id": "000003c2ff390a6bd4f3f9ee885b7d9577987b85", - "extensions": [], - "previous": "000003c11832e86450e7b329b94cdab8aca89304", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f3d4e1c2896172abd7dd3245e4c513a8cbca04941ebc070ef7ba64ff72061d5d13e299cd27c0bd3c0b622bc7cff74e59419b24740063461b37e373b4f3e34060d" - }, - { - "block_id": "000003c37e26939c8d5321555dc939c733971ab9", - "extensions": [], - "previous": "000003c2ff390a6bd4f3f9ee885b7d9577987b85", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f6621886ea4b6ee94ecb3928e0b3e8c3f6e84638db801a0d44035548f466ec6ef3b1fc8933c662b79f09728aecf8eb0bc9d60ecbc937b5ff7f559016f69ab0a25" - }, - { - "block_id": "000003c48d7373dcfb80b688c41fd83b55353d4c", - "extensions": [], - "previous": "000003c37e26939c8d5321555dc939c733971ab9", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "203d4328acc30281dae21484de77b4f7e2d28693662845da7aa01a4197478426424ae939d1449c3d939a7deafa0a2166fc1320970415a1d2d35d5ba15ca06c20b2" - }, - { - "block_id": "000003c579e0be7f88142a85ad620f82ec52e066", - "extensions": [], - "previous": "000003c48d7373dcfb80b688c41fd83b55353d4c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f38865465a8556ab6c8a8a3281e339b5f38742fa975942f2f0e9e1cfd0d8b1130587aa599b5e58dbaab8481b65946764ef2ac593a7d7b4f80751090900a6f20b5" - }, - { - "block_id": "000003c60e131367bc8548d7b5082107f03582c6", - "extensions": [], - "previous": "000003c579e0be7f88142a85ad620f82ec52e066", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0f3179763ccdeef95104ff16ab7829caa4466089c3d88aa8760df2e9a591223457404107da069e9f5536a5dbf98887217720f4fb8ee26dc9431b99b64c4f8cc4" - }, - { - "block_id": "000003c77930a595c2547e010de4075a213a261a", - "extensions": [], - "previous": "000003c60e131367bc8548d7b5082107f03582c6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20223ed5367d268df76e817a401d0bdffa4fd3b5d198ed9c6a5dc1a4940e3a7bf97c3619e3185a308c53980f3b4c8d39f2d7115931aea635ca46c9ceabf2feaac8" - }, - { - "block_id": "000003c8741fbbbd84da659862600535a0a7d4e7", - "extensions": [], - "previous": "000003c77930a595c2547e010de4075a213a261a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "206414e7d90555a7bbf485f8d87ea0493730ac3d088a368544abe85c3da3d77d274928a1ae78ba2cafd06bc4773ea86b96a4fe6d75b21f761605007b88657d4443" - }, - { - "block_id": "000003c9be55ab03994946089ed850f3e2d8d16f", - "extensions": [], - "previous": "000003c8741fbbbd84da659862600535a0a7d4e7", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:53:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f31565a9c6d7e64b520b444403e09107aae4e3b9e1f03d2b55fa85b769897985c6746a827f855bfb26e3907de9be376023104c981fc6a7609bdc1b424f88e9e8c" - }, - { - "block_id": "000003ca260a51d63be7d9d819b37ccad90a856a", - "extensions": [], - "previous": "000003c9be55ab03994946089ed850f3e2d8d16f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1291a5c89de6e59c7f3aad596388e2a122843e601bd977b2d61c924f3741797154d23f32c21057e337eb23872da80df3b73206c44845820057674b2b2df30f53" - }, - { - "block_id": "000003cb91313040e052a4b573aa7922bc9701d2", - "extensions": [], - "previous": "000003ca260a51d63be7d9d819b37ccad90a856a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2738db2425331a93a792c286e3c737d733a17c13c94fbc96051a61d8690fee706a64a45e2ce1ceb8c91617a348d60e33f5cb44abecf0c923e18b7608c85aeec1" - }, - { - "block_id": "000003cc42eb5ed98d954a3719c6aebf8c32a5ea", - "extensions": [], - "previous": "000003cb91313040e052a4b573aa7922bc9701d2", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1600f27c136d960daa943f89fcb5b6ea7c4ea42bfb08700de5e78775c278076c0a79ca4450ed02bd0e4e76ddae786b4197f0106d1d37626ce92df44d0048ba6c" - }, - { - "block_id": "000003cd24b09de335834831cc4f08f07f7eb9dd", - "extensions": [], - "previous": "000003cc42eb5ed98d954a3719c6aebf8c32a5ea", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2017a8a11f71b176d0a168dde891b5b60287a5987b86d4dc307155854c12a744ab2279b69574bf5469ba9e4735413c50a59d7ed8958518e197dff82ef7cfcfa1af" - }, - { - "block_id": "000003ce6ec61d849254bb01c808030b109d0be6", - "extensions": [], - "previous": "000003cd24b09de335834831cc4f08f07f7eb9dd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "201f7b52e6b25df4cd2e1521bae9fb847636c6eee668ebfebca22d341008a27efe2a34afd27e9af7260c3ca65641e3be93de0dd9d1a8fe55fc0a780ade468931e3" - }, - { - "block_id": "000003cf072cf7f08f4d1e41366f2d13b04fce9b", - "extensions": [], - "previous": "000003ce6ec61d849254bb01c808030b109d0be6", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f5402dcd3e571aec61ca2f4965a01cb13b03bcf88c6c1d9e77854bcb04aadf3650905c60c605ce80e021ec77fc9a63dabea6614ab4e0b99663c3ebd8841e65bd4" - }, - { - "block_id": "000003d057a50916a687f31bc29ea8829952174a", - "extensions": [], - "previous": "000003cf072cf7f08f4d1e41366f2d13b04fce9b", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f64375c3651444ea1df228e7eec91814555597d107cfc0ed99480a32d6c1e192a703113edcf0b628ce8cba141b04eca921b4b74634ea42fd34c0c6d03ba1f7f1a" - }, - { - "block_id": "000003d10d1a67a7e209dc278320f1616860c16a", - "extensions": [], - "previous": "000003d057a50916a687f31bc29ea8829952174a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2683903a7698f8720297f64e7c2875ad4e7585532debf71c01deab1778f90ff21be9beb020f10c47670d995b6783f0dc07af93fa02b16a941a3785cb572bc177" - }, - { - "block_id": "000003d2df83964a7af274051c59db691113c858", - "extensions": [], - "previous": "000003d10d1a67a7e209dc278320f1616860c16a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20368e97557f0a8570293797c8763d6f183e2a2778c2b148158c50a0e3a8bb8ec015e4e1a535de48fbfcf307fc874d40d0acf4fa3bf982aefb68a8c28623333992" - }, - { - "block_id": "000003d3f03e165edbaf139cce86db43155eec59", - "extensions": [], - "previous": "000003d2df83964a7af274051c59db691113c858", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f4561b47889e08b2eff5e6298d6332b845d74cfa06e7151d912ea3cb4792a973a7b8263150ecfc2c2ecd3272bee93faffafb3663b39d793d679d3c1cbea9930d5" - }, - { - "block_id": "000003d45fcb551d87830c62c964a03ac8d27cba", - "extensions": [], - "previous": "000003d3f03e165edbaf139cce86db43155eec59", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2c842fd1d32f2adbfd8f26ed17eb8c14b4b9340b39fa5c60f904441cbe199957349c4d81c40e3107c234ef4ce764aee1b7c38ca506f5400181dcc0b192d8dd43" - }, - { - "block_id": "000003d5004b76eebdcb6f6ee94630c5f5ed48ca", - "extensions": [], - "previous": "000003d45fcb551d87830c62c964a03ac8d27cba", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:33", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0e930acf37bef1e1d09532f254c5dfbc821e013a102f788edeb867b88f5751a77bc739a0db5db4e57cefc5e19216b21bc1a0e13e329aa7811b6b7f3bd92796bf" - }, - { - "block_id": "000003d6da0d3e3be6c914922ca119bfe6e5e119", - "extensions": [], - "previous": "000003d5004b76eebdcb6f6ee94630c5f5ed48ca", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:36", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "202e3754ab05ad552cd9d5c3140f374d80d19140460071daba8f773b766ede02b7614c2d0c1b3784c3edefe78ff36a8a9c7cd877f3513d41870d8d4cd7011a1797" - }, - { - "block_id": "000003d77435e01d323cf968678c1a331594a2a0", - "extensions": [], - "previous": "000003d6da0d3e3be6c914922ca119bfe6e5e119", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:39", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2074c0448d8a5ca8b1b87ada6e7c9c1294168e2bc29d5780ca82c4778458b94f785f428985bf3dc5094a3ce1826de0f95c6560928279e7e3da54e390c61574a594" - }, - { - "block_id": "000003d833780e7e86291741aba5961abaf4332c", - "extensions": [], - "previous": "000003d77435e01d323cf968678c1a331594a2a0", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:42", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20687f3b06333cd5018053bba211929579926a70885b39fa4b6aa9d85edbdf775e292e9d580bc7e13cf54d75c538a61937baf977fd2ed8da09f7033f44ff5f80e3" - }, - { - "block_id": "000003d9b4a7669e55bf857ffe2d4a5fd546f769", - "extensions": [], - "previous": "000003d833780e7e86291741aba5961abaf4332c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:45", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f367acbcdfd7b2a7b5c78a25511da5805c3f3a5a60919f0839196e055d15db517376453be3c3d1788adbb6e64be2b2420cb03ebd4515124caeca33fdef941be99" - }, - { - "block_id": "000003dae0b6e1f81f298f582e012352ee2d2e2e", - "extensions": [], - "previous": "000003d9b4a7669e55bf857ffe2d4a5fd546f769", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:48", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f21d66dd8d46e6d53e73dc81692e693405e01a0ece5c635d378d64283ea9be62944d94a1cb8bfa50055f3cbcd2453912e17f5b4a455ed381e9f3b22cc53981d24" - }, - { - "block_id": "000003db5bcd3ebba037e3e50705ff59ee123a6f", - "extensions": [], - "previous": "000003dae0b6e1f81f298f582e012352ee2d2e2e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:51", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20584b335007d2ee841af65717095d097bbb720ced211d7168859ea37263c6c0960e3d362fe25f49d4f3010686ab60fc88c0f7386373baff6be56b7c48e396c336" - }, - { - "block_id": "000003dc3011a8b3c6d7501a812bcb4ad318c518", - "extensions": [], - "previous": "000003db5bcd3ebba037e3e50705ff59ee123a6f", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:54", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "20236959ddc883db8a277628f78bd25117e65c379c9136ea1ce111dd7c5b78e8192bb0b8f9b1674524018de8af7f609076771de0e010da246d82464512d9dec1fc" - }, - { - "block_id": "000003dd3513c9b6729eac56a08700ad775af4dd", - "extensions": [], - "previous": "000003dc3011a8b3c6d7501a812bcb4ad318c518", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:54:57", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "2001690cdf231fcf1b5dbc7a19e81e0dc8bb5e2e9f52ddce2153569ca53388009069c9876484c58a0286361039811aeea63927cefc2b534a8c274531bd3931b257" - }, - { - "block_id": "000003ded51ea264e3b2d59928106d11471f3111", - "extensions": [], - "previous": "000003dd3513c9b6729eac56a08700ad775af4dd", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f1cef1a07bf0b5528b0382d46916e4a0b439b05d53eee9b16dae096fc05cedc2d29041b61cf8d0be281faf7bad5424d22f92d7a8eb73b2c4db27067540a6a37e7" - }, - { - "block_id": "000003df0ab7a46369806870bbbba5c27fd28b2a", - "extensions": [], - "previous": "000003ded51ea264e3b2d59928106d11471f3111", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:03", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f73f9690ebb4225351c24d78d213967e4fd711170fee5240a0fc0b5222df239341e3f8affafe3c548a72f3a05b8cc6f0f28ed703a32e08b1c0bdf9aed35674b1a" - }, - { - "block_id": "000003e0a4f41b8073bcebd1fec4ea30805983ba", - "extensions": [], - "previous": "000003df0ab7a46369806870bbbba5c27fd28b2a", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:06", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f2a42b8637019359a2320d17f079537f10729014d7a5774b50189a9037e5729cf48013479f338a6e4b8a41a1efeab2f5598fd3ca1064b108e81ca990a318b1860" - }, - { - "block_id": "000003e182bde67b0300a77f052f3db4d999977e", - "extensions": [], - "previous": "000003e0a4f41b8073bcebd1fec4ea30805983ba", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:09", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0a90988555304b6ce92f39ed1ce19c6c328e8d16184702b286541bb2c413c66d06dfb52379fbc3e5e558f2c9ebc4afc5ca346c9cfa3eab0a06b10cec5692cd63" - }, - { - "block_id": "000003e240088184f45e104ce2ae4b6fb98ee566", - "extensions": [], - "previous": "000003e182bde67b0300a77f052f3db4d999977e", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:12", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "200bedb8128afee27245c4782a9693cbbae068ce628ab6fdeb1c4d2766bc2491c936a7d234dcc1bd7df21dc02da3b49592e4d6e19953079886ad6cd7ba32516d60" - }, - { - "block_id": "000003e33bdfd88d15058e5a45246a9249ec4c2d", - "extensions": [], - "previous": "000003e240088184f45e104ce2ae4b6fb98ee566", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:15", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0fa92b7bf0bd3f8cdee9d71c64a51e2814f69ee269e72def237621ef2b28f039403ff341dba01be0b4716ffc631be4d8b0b8f5726714df45a018641c8c5a4326" - }, - { - "block_id": "000003e4dcf6c73d1efabba7b91dc5bb75b6a26c", - "extensions": [], - "previous": "000003e33bdfd88d15058e5a45246a9249ec4c2d", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:18", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0a4fa56e477d42fba80ff4b48b478942356a0d3c719cb5974375bad6810782db221817190752fb5d59fea36adf1b887bc3ca3cd486294d757ba021660a722cb0" - }, - { - "block_id": "000003e5cee172c089d3589f706f04b3757e7d96", - "extensions": [], - "previous": "000003e4dcf6c73d1efabba7b91dc5bb75b6a26c", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:21", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f72b072ef2ad5e4b832e3a19d8fbd5f620650c2c3cc54977493b355154178bb6d265a4a031ce11ba3f91dddacb3c7c1d2d8078a399ac6b902fb48927200d61888" - }, - { - "block_id": "000003e6a2eaccecc0bcdfda9d64605844874227", - "extensions": [], - "previous": "000003e5cee172c089d3589f706f04b3757e7d96", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:24", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f0e2127f690f5cabeb619bf2958615219e6fef2a763d77766975b693ae517f1c26c29cb7d6b9328cbb8d38a25c259277fd8cb2d86f56abc966b98a48775dab47f" - }, - { - "block_id": "000003e7c4fd3221cf407efcf7c1730e2ca54b05", - "extensions": [], - "previous": "000003e6a2eaccecc0bcdfda9d64605844874227", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:27", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "1f557c88c6b17d1b6b51c324deea81cce12d15329a995c75fe826da7abd85cddef188279bf230638f84163bd0e23648be4536e0d7eec1f7617ed3f1e708edefb6b" - }, - { - "block_id": "000003e8b922f4906a45af8e99d86b3511acd7a5", - "extensions": [], - "previous": "000003e7c4fd3221cf407efcf7c1730e2ca54b05", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:55:30", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "207f15578cac20ac0e8af1ebb8f463106b8849577e21cca9fc60da146d1d95df88072dedc6ffb7f7f44a9185bbf9bf8139a5b4285c9f423843720296a44d428856" - } - ] -} diff --git a/hived/tavern/block_api_patterns/get_block_range/one.tavern.yaml b/hived/tavern/block_api_patterns/get_block_range/one.tavern.yaml deleted file mode 100644 index 4a8bbfbf446a7d2ef00cd4472277795661fc63ef..0000000000000000000000000000000000000000 --- a/hived/tavern/block_api_patterns/get_block_range/one.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived block_api.get_block_range - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block_range - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "block_api.get_block_range" - params: {"starting_block_num": 1, "count": 1000} - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "one" - directory: "block_api_patterns/get_block_range" diff --git a/hived/tavern/common.yaml b/hived/tavern/common.yaml deleted file mode 100644 index d491f754945f0419631fe13b4e6df2d61d922f2d..0000000000000000000000000000000000000000 --- a/hived/tavern/common.yaml +++ /dev/null @@ -1,7 +0,0 @@ ---- - name: Common test values - description: Common values for tests - - variables: - service: - url: "{tavern.env_vars.HIVEMIND_URL}" diff --git a/hived/tavern/condenser_api_negative/broadcast_block/too_old.pat.json b/hived/tavern/condenser_api_negative/broadcast_block/too_old.pat.json deleted file mode 100644 index c85e2451ab2316612688b2f7323056d9f88a886a..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/broadcast_block/too_old.pat.json +++ /dev/null @@ -1,37 +0,0 @@ -{ - "code": -32000, - "data": { - "code": 10, - "message": "Assert Exception", - "name": "assert_exception", - "stack": [ - { - "data": { - "head": 48419865, - "item->num": 1, - "max_size": 16 - }, - "format": "item->num > std::max<int64_t>( 0, int64_t(_head->num) - (_max_size) ): attempting to push a block that is too old" - }, - { - "data": {}, - "format": "" - }, - { - "data": { - "new_block": { - "extensions": [], - "previous": "0000000000000000000000000000000000000000", - "timestamp": "1970-01-01T00:00:00", - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "", - "witness_signature": "0000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000" - } - }, - "format": "" - } - ] - }, - "message": "Assert Exception:item->num > std::max<int64_t>( 0, int64_t(_head->num) - (_max_size) ): attempting to push a block that is too old" -} diff --git a/hived/tavern/condenser_api_negative/broadcast_block/too_old.tavern.yaml b/hived/tavern/condenser_api_negative/broadcast_block/too_old.tavern.yaml deleted file mode 100644 index e7fe23de4a992b90005d348b5f6cf7f278cbdc4a..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/broadcast_block/too_old.tavern.yaml +++ /dev/null @@ -1,40 +0,0 @@ ---- - test_name: Hived broadcast_block - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: broadcast_block - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.broadcast_block" - params: [ - { - "previous": "0000000000000000000000000000000000000000", - "timestamp": "1970-01-01T00:00:00", - "witness": "", - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "extensions": [ ], - "witness_signature": "0000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000", - "transactions": [ ] - } - ] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "too_old" - directory: "condenser_api_negative/broadcast_block" - error_response: true - ignore_tags: ['stack'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_negative/broadcast_transaction/missing_posting_authority.pat.json b/hived/tavern/condenser_api_negative/broadcast_transaction/missing_posting_authority.pat.json deleted file mode 100644 index 1d4ba4cb364763a4b5586ae977be200673f18b03..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/broadcast_transaction/missing_posting_authority.pat.json +++ /dev/null @@ -1,182 +0,0 @@ -{ - "code": -32000, - "data": { - "code": 3030000, - "message": "missing required posting authority", - "name": "tx_missing_posting_auth", - "stack": [ - { - "context": { - "file": "transaction_util.hpp", - "hostname": "", - "level": "error", - "line": 58, - "method": "verify_authority", - "timestamp": "2020-11-05T12:51:07" - }, - "data": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM69zfrFGnZtU3gWFWpQJ6GhND1nz7TJsKBTjcWfebS1JzBEweQy", - 1 - ] - ], - "weight_threshold": 1 - }, - "id": "hiveio", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM65PUAPA4yC4RgPtGgsPupxT6yJtMhmT5JHFdsT3uoCbR8WJ25s", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [ - [ - "threespeak", - 1 - ], - [ - "vimm.app", - 1 - ] - ], - "key_auths": [ - [ - "STM6vJmrwaX5TjgTS9dPH8KsArso5m91fVodJvv91j7G765wqcNM9", - 1 - ] - ], - "weight_threshold": 1 - } - }, - "format": "Missing Posting Authority ${id}" - }, - { - "context": { - "file": "transaction_util.hpp", - "hostname": "", - "level": "warn", - "line": 103, - "method": "verify_authority", - "timestamp": "2020-11-05T12:51:07" - }, - "data": { - "auth_containers": [ - { - "type": "vote_operation", - "value": { - "author": "alice", - "permlink": "a-post-by-alice", - "voter": "hiveio", - "weight": 10000 - } - } - ], - "sigs": [] - }, - "format": "" - }, - { - "context": { - "file": "transaction.cpp", - "hostname": "", - "level": "warn", - "line": 232, - "method": "verify_authority", - "timestamp": "2020-11-05T12:51:07" - }, - "data": { - "*this": { - "expiration": "2016-03-24T18:00:21", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "alice", - "permlink": "a-post-by-alice", - "voter": "hiveio", - "weight": 10000 - } - } - ], - "ref_block_num": 0, - "ref_block_prefix": 2181793527, - "signatures": [] - } - }, - "format": "" - }, - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "warn", - "line": 4269, - "method": "_apply_transaction", - "timestamp": "2020-11-05T12:51:07" - }, - "data": { - "trx": { - "expiration": "2016-03-24T18:00:21", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "alice", - "permlink": "a-post-by-alice", - "voter": "hiveio", - "weight": 10000 - } - } - ], - "ref_block_num": 0, - "ref_block_prefix": 2181793527, - "signatures": [] - } - }, - "format": "" - }, - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "warn", - "line": 1176, - "method": "push_transaction", - "timestamp": "2020-11-05T12:51:07" - }, - "data": { - "trx": { - "expiration": "2016-03-24T18:00:21", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "alice", - "permlink": "a-post-by-alice", - "voter": "hiveio", - "weight": 10000 - } - } - ], - "ref_block_num": 0, - "ref_block_prefix": 2181793527, - "signatures": [] - } - }, - "format": "" - } - ] - }, - "message": "missing required posting authority:Missing Posting Authority hiveio" -} diff --git a/hived/tavern/condenser_api_negative/broadcast_transaction/missing_posting_authority.tavern.yaml b/hived/tavern/condenser_api_negative/broadcast_transaction/missing_posting_authority.tavern.yaml deleted file mode 100644 index f003dc51f62b2af9feb687fc64dbf3d0183a8934..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/broadcast_transaction/missing_posting_authority.tavern.yaml +++ /dev/null @@ -1,43 +0,0 @@ ---- - test_name: Hived broadcast_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: broadcast_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.broadcast_transaction" - params: [ - { - "ref_block_prefix": 2181793527, - "expiration":"2016-03-24T18:00:21", - "operations":[ - [ - "vote", - {"voter":"hiveio","author":"alice","permlink":"a-post-by-alice","weight":10000} - ] - ], - "extensions":[], - "signatures":[] - } - ] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "missing_posting_authority" - directory: "condenser_api_negative/broadcast_transaction" - error_response: true - ignore_tags: ['context'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_negative/broadcast_transaction/transaction_tapos_exception.pat.json b/hived/tavern/condenser_api_negative/broadcast_transaction/transaction_tapos_exception.pat.json deleted file mode 100644 index 3cddce545d66eee48bc00c7f858a9e5146f46485..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/broadcast_transaction/transaction_tapos_exception.pat.json +++ /dev/null @@ -1,110 +0,0 @@ -{ - "code": -32000, - "data": { - "code": 4030200, - "message": "transaction tapos exception", - "name": "transaction_tapos_exception", - "stack": [ - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "error", - "line": 4233, - "method": "_apply_transaction", - "timestamp": "2020-11-09T10:59:05" - }, - "data": { - "tapos_block_summary": 3191012121, - "trx.ref_block_prefix": 276291495 - }, - "format": "" - }, - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "warn", - "line": 4269, - "method": "_apply_transaction", - "timestamp": "2020-11-09T10:59:05" - }, - "data": { - "trx": { - "expiration": "2020-11-05T12:58:10", - "extensions": [], - "operations": [ - { - "type": "request_account_recovery_operation", - "value": { - "account_to_recover": "tulpa", - "extensions": [], - "new_owner_authority": { - "account_auths": [], - "key_auths": [ - [ - "STM6wxeXR9kg8uu7vX5LS4HBgKw8sdqHBpzAaacqPwPxYfRx9h5bS", - 1 - ] - ], - "weight_threshold": 1 - }, - "recovery_account": "nalesnik" - } - } - ], - "ref_block_num": 55551, - "ref_block_prefix": 276291495, - "signatures": [ - "204f2dc529f3e884a77ee3e849d0f8c03ff9ec0d9af08894427d15c74eea4dcead50bc2bd23ac188cfb5975acc5a89cb1df1474e42d47d54ac993ed240fdb3f955" - ] - } - }, - "format": "" - }, - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "warn", - "line": 1176, - "method": "push_transaction", - "timestamp": "2020-11-09T10:59:05" - }, - "data": { - "trx": { - "expiration": "2020-11-05T12:58:10", - "extensions": [], - "operations": [ - { - "type": "request_account_recovery_operation", - "value": { - "account_to_recover": "tulpa", - "extensions": [], - "new_owner_authority": { - "account_auths": [], - "key_auths": [ - [ - "STM6wxeXR9kg8uu7vX5LS4HBgKw8sdqHBpzAaacqPwPxYfRx9h5bS", - 1 - ] - ], - "weight_threshold": 1 - }, - "recovery_account": "nalesnik" - } - } - ], - "ref_block_num": 55551, - "ref_block_prefix": 276291495, - "signatures": [ - "204f2dc529f3e884a77ee3e849d0f8c03ff9ec0d9af08894427d15c74eea4dcead50bc2bd23ac188cfb5975acc5a89cb1df1474e42d47d54ac993ed240fdb3f955" - ] - } - }, - "format": "" - } - ] - }, - "message": "transaction tapos exception:" -} diff --git a/hived/tavern/condenser_api_negative/broadcast_transaction/transaction_tapos_exception.tavern.yaml b/hived/tavern/condenser_api_negative/broadcast_transaction/transaction_tapos_exception.tavern.yaml deleted file mode 100644 index 3ef328debca4bf7f38fcf44c9634ca1ed0c8d002..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/broadcast_transaction/transaction_tapos_exception.tavern.yaml +++ /dev/null @@ -1,44 +0,0 @@ ---- - test_name: Hived broadcast_transaction transaction_tapos_exception - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: broadcast_transaction transaction_tapos_exception - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.broadcast_transaction" - params: [ - { 'expiration': '2020-11-05T12:58:10', - 'extensions': [ ], - 'operations': [ [ 'request_account_recovery', - { 'account_to_recover': 'tulpa', - 'extensions': [ ], - 'new_owner_authority': { 'account_auths': [ ], - 'key_auths': [ [ 'STM6wxeXR9kg8uu7vX5LS4HBgKw8sdqHBpzAaacqPwPxYfRx9h5bS', - '1' ] ], - 'weight_threshold': 1 }, - 'recovery_account': 'nalesnik' } ] ], - 'ref_block_num': 55551, - 'ref_block_prefix': 276291495, - 'signatures': [ '204f2dc529f3e884a77ee3e849d0f8c03ff9ec0d9af08894427d15c74eea4dcead50bc2bd23ac188cfb5975acc5a89cb1df1474e42d47d54ac993ed240fdb3f955' ] } - ] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "transaction_tapos_exception" - directory: "condenser_api_negative/broadcast_transaction" - error_response: true - ignore_tags: ['stack'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/missing_posting_authority.pat.json b/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/missing_posting_authority.pat.json deleted file mode 100644 index 1d4ba4cb364763a4b5586ae977be200673f18b03..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/missing_posting_authority.pat.json +++ /dev/null @@ -1,182 +0,0 @@ -{ - "code": -32000, - "data": { - "code": 3030000, - "message": "missing required posting authority", - "name": "tx_missing_posting_auth", - "stack": [ - { - "context": { - "file": "transaction_util.hpp", - "hostname": "", - "level": "error", - "line": 58, - "method": "verify_authority", - "timestamp": "2020-11-05T12:51:07" - }, - "data": { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM69zfrFGnZtU3gWFWpQJ6GhND1nz7TJsKBTjcWfebS1JzBEweQy", - 1 - ] - ], - "weight_threshold": 1 - }, - "id": "hiveio", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM65PUAPA4yC4RgPtGgsPupxT6yJtMhmT5JHFdsT3uoCbR8WJ25s", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [ - [ - "threespeak", - 1 - ], - [ - "vimm.app", - 1 - ] - ], - "key_auths": [ - [ - "STM6vJmrwaX5TjgTS9dPH8KsArso5m91fVodJvv91j7G765wqcNM9", - 1 - ] - ], - "weight_threshold": 1 - } - }, - "format": "Missing Posting Authority ${id}" - }, - { - "context": { - "file": "transaction_util.hpp", - "hostname": "", - "level": "warn", - "line": 103, - "method": "verify_authority", - "timestamp": "2020-11-05T12:51:07" - }, - "data": { - "auth_containers": [ - { - "type": "vote_operation", - "value": { - "author": "alice", - "permlink": "a-post-by-alice", - "voter": "hiveio", - "weight": 10000 - } - } - ], - "sigs": [] - }, - "format": "" - }, - { - "context": { - "file": "transaction.cpp", - "hostname": "", - "level": "warn", - "line": 232, - "method": "verify_authority", - "timestamp": "2020-11-05T12:51:07" - }, - "data": { - "*this": { - "expiration": "2016-03-24T18:00:21", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "alice", - "permlink": "a-post-by-alice", - "voter": "hiveio", - "weight": 10000 - } - } - ], - "ref_block_num": 0, - "ref_block_prefix": 2181793527, - "signatures": [] - } - }, - "format": "" - }, - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "warn", - "line": 4269, - "method": "_apply_transaction", - "timestamp": "2020-11-05T12:51:07" - }, - "data": { - "trx": { - "expiration": "2016-03-24T18:00:21", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "alice", - "permlink": "a-post-by-alice", - "voter": "hiveio", - "weight": 10000 - } - } - ], - "ref_block_num": 0, - "ref_block_prefix": 2181793527, - "signatures": [] - } - }, - "format": "" - }, - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "warn", - "line": 1176, - "method": "push_transaction", - "timestamp": "2020-11-05T12:51:07" - }, - "data": { - "trx": { - "expiration": "2016-03-24T18:00:21", - "extensions": [], - "operations": [ - { - "type": "vote_operation", - "value": { - "author": "alice", - "permlink": "a-post-by-alice", - "voter": "hiveio", - "weight": 10000 - } - } - ], - "ref_block_num": 0, - "ref_block_prefix": 2181793527, - "signatures": [] - } - }, - "format": "" - } - ] - }, - "message": "missing required posting authority:Missing Posting Authority hiveio" -} diff --git a/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/missing_posting_authority.tavern.yaml b/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/missing_posting_authority.tavern.yaml deleted file mode 100644 index 34f6656bfca30a92b8b85126884f7c69cf566c29..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/missing_posting_authority.tavern.yaml +++ /dev/null @@ -1,43 +0,0 @@ ---- - test_name: Hived broadcast_transaction_synchronous - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: broadcast_transaction_synchronous - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.broadcast_transaction_synchronous" - params: [ - { - "ref_block_prefix": 2181793527, - "expiration":"2016-03-24T18:00:21", - "operations":[ - [ - "vote", - {"voter":"hiveio","author":"alice","permlink":"a-post-by-alice","weight":10000} - ] - ], - "extensions":[], - "signatures":[] - } - ] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "missing_posting_authority" - directory: "condenser_api_negative/broadcast_transaction_synchronous" - error_response: true - ignore_tags: ['context'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/transaction_tapos_exception.pat.json b/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/transaction_tapos_exception.pat.json deleted file mode 100644 index 909c7faca113ba019440473c4980eeeb30117247..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/transaction_tapos_exception.pat.json +++ /dev/null @@ -1,110 +0,0 @@ -{ - "code": -32000, - "data": { - "code": 4030200, - "message": "transaction tapos exception", - "name": "transaction_tapos_exception", - "stack": [ - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "error", - "line": 4233, - "method": "_apply_transaction", - "timestamp": "2020-11-09T11:02:09" - }, - "data": { - "tapos_block_summary": 3191012121, - "trx.ref_block_prefix": 276291495 - }, - "format": "" - }, - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "warn", - "line": 4269, - "method": "_apply_transaction", - "timestamp": "2020-11-09T11:02:09" - }, - "data": { - "trx": { - "expiration": "2020-11-05T12:58:10", - "extensions": [], - "operations": [ - { - "type": "request_account_recovery_operation", - "value": { - "account_to_recover": "tulpa", - "extensions": [], - "new_owner_authority": { - "account_auths": [], - "key_auths": [ - [ - "STM6wxeXR9kg8uu7vX5LS4HBgKw8sdqHBpzAaacqPwPxYfRx9h5bS", - 1 - ] - ], - "weight_threshold": 1 - }, - "recovery_account": "nalesnik" - } - } - ], - "ref_block_num": 55551, - "ref_block_prefix": 276291495, - "signatures": [ - "204f2dc529f3e884a77ee3e849d0f8c03ff9ec0d9af08894427d15c74eea4dcead50bc2bd23ac188cfb5975acc5a89cb1df1474e42d47d54ac993ed240fdb3f955" - ] - } - }, - "format": "" - }, - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "warn", - "line": 1176, - "method": "push_transaction", - "timestamp": "2020-11-09T11:02:09" - }, - "data": { - "trx": { - "expiration": "2020-11-05T12:58:10", - "extensions": [], - "operations": [ - { - "type": "request_account_recovery_operation", - "value": { - "account_to_recover": "tulpa", - "extensions": [], - "new_owner_authority": { - "account_auths": [], - "key_auths": [ - [ - "STM6wxeXR9kg8uu7vX5LS4HBgKw8sdqHBpzAaacqPwPxYfRx9h5bS", - 1 - ] - ], - "weight_threshold": 1 - }, - "recovery_account": "nalesnik" - } - } - ], - "ref_block_num": 55551, - "ref_block_prefix": 276291495, - "signatures": [ - "204f2dc529f3e884a77ee3e849d0f8c03ff9ec0d9af08894427d15c74eea4dcead50bc2bd23ac188cfb5975acc5a89cb1df1474e42d47d54ac993ed240fdb3f955" - ] - } - }, - "format": "" - } - ] - }, - "message": "transaction tapos exception:" -} diff --git a/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/transaction_tapos_exception.tavern.yaml b/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/transaction_tapos_exception.tavern.yaml deleted file mode 100644 index af88b0708cd8584b78eab233e3bc42f91440bfef..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/broadcast_transaction_synchronous/transaction_tapos_exception.tavern.yaml +++ /dev/null @@ -1,44 +0,0 @@ ---- - test_name: Hived broadcast_transaction_synchronous - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: broadcast_transaction_synchronous - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.broadcast_transaction_synchronous" - params: [ - { 'expiration': '2020-11-05T12:58:10', - 'extensions': [ ], - 'operations': [ [ 'request_account_recovery', - { 'account_to_recover': 'tulpa', - 'extensions': [ ], - 'new_owner_authority': { 'account_auths': [ ], - 'key_auths': [ [ 'STM6wxeXR9kg8uu7vX5LS4HBgKw8sdqHBpzAaacqPwPxYfRx9h5bS', - '1' ] ], - 'weight_threshold': 1 }, - 'recovery_account': 'nalesnik' } ] ], - 'ref_block_num': 55551, - 'ref_block_prefix': 276291495, - 'signatures': [ '204f2dc529f3e884a77ee3e849d0f8c03ff9ec0d9af08894427d15c74eea4dcead50bc2bd23ac188cfb5975acc5a89cb1df1474e42d47d54ac993ed240fdb3f955' ] } - ] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "transaction_tapos_exception" - directory: "condenser_api_negative/broadcast_transaction_synchronous" - error_response: true - ignore_tags: ['stack'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_negative/get_account_votes/get.pat.json b/hived/tavern/condenser_api_negative/get_account_votes/get.pat.json deleted file mode 100644 index 00b9bf6ee2565efe9ef6a96a9076ca701d537b55..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/get_account_votes/get.pat.json +++ /dev/null @@ -1,23 +0,0 @@ -{ - "code": -32003, - "data": { - "code": 10, - "message": "Assert Exception", - "name": "assert_exception", - "stack": [ - { - "context": { - "file": "condenser_api.cpp", - "hostname": "", - "level": "error", - "line": 776, - "method": "get_account_votes", - "timestamp": "2020-10-28T12:25:32" - }, - "data": {}, - "format": "false: Supported by hivemind" - } - ] - }, - "message": "Assert Exception:false: Supported by hivemind" -} diff --git a/hived/tavern/condenser_api_negative/get_account_votes/get.tavern.yaml b/hived/tavern/condenser_api_negative/get_account_votes/get.tavern.yaml deleted file mode 100644 index a1d9abd4d712dd234a0c989540cee9a10109af6e..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/get_account_votes/get.tavern.yaml +++ /dev/null @@ -1,30 +0,0 @@ ---- - test_name: Hived get_account_votes - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_account_votes - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_votes" - params: [""] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "get" - directory: "condenser_api_negative/get_account_votes" - error_response: true - ignore_tags: ['context'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_negative/get_active_votes/wrong_permlink.pat.json b/hived/tavern/condenser_api_negative/get_active_votes/wrong_permlink.pat.json deleted file mode 100644 index 2af6100b3a435ac7d7445c7379bdae3374fa2e2a..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/get_active_votes/wrong_permlink.pat.json +++ /dev/null @@ -1,42 +0,0 @@ -{ - "code": -32000, - "data": { - "code": 13, - "message": "unknown key", - "name": "N5boost16exception_detail10clone_implINS0_19error_info_injectorISt12out_of_rangeEEEE", - "stack": [ - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "warn", - "line": 828, - "method": "get_comment", - "timestamp": "2020-11-25T13:32:56" - }, - "data": { - "author": 14007, - "permlink": "wrong_permlink", - "what": "unknown key" - }, - "format": "${what}: " - }, - { - "context": { - "file": "database.cpp", - "hostname": "", - "level": "warn", - "line": 838, - "method": "get_comment", - "timestamp": "2020-11-25T13:32:56" - }, - "data": { - "author": "gtg", - "permlink": "wrong_permlink" - }, - "format": "" - } - ] - }, - "message": "unknown key:unknown key: " -} diff --git a/hived/tavern/condenser_api_negative/get_active_votes/wrong_permlink.tavern.yaml b/hived/tavern/condenser_api_negative/get_active_votes/wrong_permlink.tavern.yaml deleted file mode 100644 index 939ed0103ace9592d36fc41caca99a38042e13d3..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/get_active_votes/wrong_permlink.tavern.yaml +++ /dev/null @@ -1,30 +0,0 @@ ---- - test_name: Hived get_active_votes - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_active_votes - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_active_votes" - params: ["gtg","wrong_permlink"] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "wrong_permlink" - directory: "condenser_api_negative/get_active_votes" - error_response: true - ignore_tags: ["timestamp"] \ No newline at end of file diff --git a/hived/tavern/condenser_api_negative/verify_account_authority/gtg.pat.json b/hived/tavern/condenser_api_negative/verify_account_authority/gtg.pat.json deleted file mode 100644 index b98e70dc481128d8cb2b334ac621604c92a4a963..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/verify_account_authority/gtg.pat.json +++ /dev/null @@ -1,109 +0,0 @@ -{ - "code": -32000, - "data": { - "code": 3010000, - "message": "missing required active authority", - "name": "tx_missing_active_auth", - "stack": [ - { - "context": { - "file": "transaction_util.hpp", - "hostname": "", - "level": "error", - "line": 88, - "method": "verify_authority", - "timestamp": "2020-10-30T12:35:56" - }, - "data": { - "auth": { - "account_auths": [], - "key_auths": [ - [ - "STM8NWQYG4BvjGNu8zqqV9fbR7aSCZHcxkVib41PYnpGmPRe6BHVG", - 1 - ] - ], - "weight_threshold": 1 - }, - "id": "gtg", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5RLQ1Jh8Kf56go3xpzoodg4vRsgCeWhANXoEXrYH7bLEwSVyjh", - 1 - ] - ], - "weight_threshold": 1 - } - }, - "format": "Missing Active Authority ${id}" - }, - { - "context": { - "file": "transaction_util.hpp", - "hostname": "", - "level": "warn", - "line": 103, - "method": "verify_authority", - "timestamp": "2020-10-30T12:35:56" - }, - "data": { - "auth_containers": [ - { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "from": "gtg", - "memo": "", - "to": "" - } - } - ], - "sigs": [] - }, - "format": "" - }, - { - "context": { - "file": "transaction.cpp", - "hostname": "", - "level": "warn", - "line": 232, - "method": "verify_authority", - "timestamp": "2020-10-30T12:35:56" - }, - "data": { - "*this": { - "expiration": "1970-01-01T00:00:00", - "extensions": [], - "operations": [ - { - "type": "transfer_operation", - "value": { - "amount": { - "amount": "0", - "nai": "@@000000021", - "precision": 3 - }, - "from": "gtg", - "memo": "", - "to": "" - } - } - ], - "ref_block_num": 0, - "ref_block_prefix": 0, - "signatures": [] - } - }, - "format": "" - } - ] - }, - "message": "missing required active authority:Missing Active Authority gtg" -} diff --git a/hived/tavern/condenser_api_negative/verify_account_authority/gtg.tavern.yaml b/hived/tavern/condenser_api_negative/verify_account_authority/gtg.tavern.yaml deleted file mode 100644 index ddd23574b9bbd4239a98e9978203c01c4a134d7f..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_negative/verify_account_authority/gtg.tavern.yaml +++ /dev/null @@ -1,30 +0,0 @@ ---- - test_name: Hived condenser_api.verify_account_authority Missing Active Authority - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: condenser_api.verify_account_authority Missing Active Authority - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.verify_account_authority" - params: ["gtg", ["STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX"]] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "gtg" - directory: "condenser_api_negative/verify_account_authority" - error_response: true - ignore_tags: ['stack'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/find_proposals/multiple.pat.json b/hived/tavern/condenser_api_patterns/find_proposals/multiple.pat.json deleted file mode 100644 index 1adb481cb88f16bff9f570ff2c414e097d68b542..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/find_proposals/multiple.pat.json +++ /dev/null @@ -1,38 +0,0 @@ -[ - { - "creator": "gtg", - "daily_pay": "240000000.000 HBD", - "end_date": "2029-12-31T23:59:59", - "id": 0, - "permlink": "steemdao", - "proposal_id": 0, - "receiver": "steem.dao", - "start_date": "2019-08-27T00:00:00", - "subject": "Return Proposal", - "total_votes": "44220038566610442" - }, - { - "creator": "jpbliberty", - "daily_pay": "200.000 HBD", - "end_date": "2021-12-31T23:30:00", - "id": 2, - "permlink": "sps-proposal-class-action-lawsuit-against-facebook-google-and-twitter-s-crypto-ad-ban", - "proposal_id": 2, - "receiver": "jpbliberty", - "start_date": "2019-08-27T15:15:00", - "subject": "Class Action Lawsuit vs Facebook Google Crypto Ad Ban", - "total_votes": "3495028030229075" - }, - { - "creator": "demotruk", - "daily_pay": "1000.000 HBD", - "end_date": "2029-08-31T23:59:59", - "id": 21, - "permlink": "reduced-inflation-proposal", - "proposal_id": 21, - "receiver": "null", - "start_date": "2019-08-31T00:00:00", - "subject": "Reduced Inflation Proposal", - "total_votes": "14072390217653764" - } -] diff --git a/hived/tavern/condenser_api_patterns/find_proposals/multiple.tavern.yaml b/hived/tavern/condenser_api_patterns/find_proposals/multiple.tavern.yaml deleted file mode 100644 index 3d81d9724f72f874e2039792d08f4d15826527f2..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/find_proposals/multiple.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api find_proposals multiple - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api find_proposals multiple - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.find_proposals" - params: [[0,2,21,22]] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "multiple" - directory: "condenser_api_patterns/find_proposals" - ignore_tags: ['total_votes'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/find_proposals/zero.pat.json b/hived/tavern/condenser_api_patterns/find_proposals/zero.pat.json deleted file mode 100644 index 4a03d0de331f20db2516c46dc8fbae8c65b2fe36..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/find_proposals/zero.pat.json +++ /dev/null @@ -1,14 +0,0 @@ -[ - { - "creator": "gtg", - "daily_pay": "240000000.000 HBD", - "end_date": "2029-12-31T23:59:59", - "id": 0, - "permlink": "steemdao", - "proposal_id": 0, - "receiver": "steem.dao", - "start_date": "2019-08-27T00:00:00", - "subject": "Return Proposal", - "total_votes": "44220038566610442" - } -] diff --git a/hived/tavern/condenser_api_patterns/find_proposals/zero.tavern.yaml b/hived/tavern/condenser_api_patterns/find_proposals/zero.tavern.yaml deleted file mode 100644 index 64a4853872ebaf112bb55859cb5ae8c43d6c8d05..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/find_proposals/zero.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived account_history_api find_proposals - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api find_proposals - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.find_proposals" - params: [[0]] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "zero" - directory: "condenser_api_patterns/find_proposals" - ignore_tags: ['total_votes'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_account_count/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_count/get.tavern.yaml deleted file mode 100644 index be75135c945d9bb53035dfeb1f2d89c50216a22b..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_count/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_account_count - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_account_count - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_count" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_account_count" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/account_create_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/account_create_operation.pat.json deleted file mode 100644 index f9dec65022eece2cb18e2f78a0089f08c8df4eae..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/account_create_operation.pat.json +++ /dev/null @@ -1,1379 +0,0 @@ -[ - [ - 150, - { - "block": 167504, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM8MMKBm4hCpS2UZ7uq9QgLw9u3pU94cigr2Mc1GkHXK8hUyVF7N", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "0.000 HIVE", - "json_metadata": "", - "memo_key": "STM68dr1xutC2ALapEuscKvsQWCcWAGqbQoqFeztd2ozfz4tL1FNL", - "new_account_name": "bytemaster", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5YoEC3eoFpQ3yfMsjmSacKojnyj7aNG9F9T9dDiT85P2YDPmik", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7B5JNwCp5A1AZkDEdBHrSGggU6XuzevecDL5S5dt4gkLFc6mmy", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-30T12:41:48", - "trx_id": "8ca00b0e2005b29a669f0f8666ee133004fb8894", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 211, - { - "block": 198165, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM5KySdUPQ75KfQ191NUzqNyyVaa8bUCifwWpRPE21ZngqsX7uiE", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM75uzwHGEE2ZmXUHPA1NXUVcVMdBhuurDkASkhEfTDGzmXRWGNB", - "new_account_name": "ben", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM51dx8qTkhgyk18gsiz7sHnjcEpwfMNB61mDC6qupwPkbA1ujKX", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM6RcZsZ99y8ekb9cT4rbB41nYVddt9CsDSi23PwxiDsbwhTt87o", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-31T14:19:42", - "trx_id": "57be922da3d6873ae4c4bf872345ccbbb3318180", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 212, - { - "block": 198182, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM7BGdJqsSGmSLgGZLTvKLbqbFKQfZUpScRwsdMKBvZzgmcZLjbB", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM5YZmGRwYvzxyLXMVYec8HTJS139odv5qffgq7q4npX6k83sprt", - "new_account_name": "michael-a", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM4zoFgsTSBRbhneRfPHcP51YT8yBEfgBspWbpg1H6sxfBpX3Vvc", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM6RkJVu8KHS36V4MgmXSRt8Mq34WUvVXmC3c277udDWpQDFuuwL", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-31T14:20:33", - "trx_id": "1373de6adfdefd5318139587f0550c65ec2edea8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 213, - { - "block": 198188, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM5vmgRGmAvZrwRGjBxeN4okw9t9r3EV4q8BMC73ZC8qSD1YtZwG", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM56GhmKabsqzNTmq51NE1zRqR3S7EgCZgrNEr8MFNh6ysx334Ss", - "new_account_name": "michael-b", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM7sAa7hrjhrgYS9tnGCxc2Gde95eoRdJD85J1qmyHKZNY6Sj9or", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM88VAa29VnEoKyCavfyZM7wtocVr5NpWGaRmXUgFVV9QaUTRcFi", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-31T14:20:51", - "trx_id": "f0a539e32c3ac7c6af3dee43496ecd6dc8fb2a75", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 214, - { - "block": 198194, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM74ErEwsLZerr24skANi6oniQ9hgzQRkfGD6VgKMQhgxDi4Uwjt", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM7xyPHXx7oy58626RHyTkcEu4v4VstGdGqSpvYP8gCMPz1dQymN", - "new_account_name": "val-a", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM7TskrEp9GgC87vxAW3zPXMTz6wzyviZoix5cAQymji67TRcqNx", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5sBqG9J1MtYWDwAP2qhJfb4zsxdidttBo4pbys9UQGtCuPkkde", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-31T14:21:09", - "trx_id": "20873bb9170bc9a67ac4cb6d17be8f95aae7bec6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 215, - { - "block": 198196, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM5GiaHQ6Qkf5rx7enbBwQfRLj7ihuSUSa8oRSmms9f4kC8DaSvS", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM6zW6fr8Uk2F2UeeDWPzeqx52h91oH1TFRYiFu4DUm9AALiHt25", - "new_account_name": "val-b", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5xF5h8fKorGeJXQKBJ7JMGzxNEuikFqDRhxaSSh91MK88wu1UG", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM56KH82jhAYfKKDdoqq2BVUmrHKdyJTjQsCJc7LVDHK5g5Pobh8", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-31T14:21:15", - "trx_id": "16355dbfeb964ae62d2b9040e2b2d7ff94a98770", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 216, - { - "block": 198204, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6f7gNxNfrSLseRf1TrK5rJZtG3B6J4iJVrkJKmo1kmU3DKNnWK", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM7qtpUZfaHwyJk9ZwvXH3EsAxJcYWY4913Vj6xVWkskM8SRV2Ub", - "new_account_name": "dan", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM6LYxj96zdypHYqgDdD6Nyh2NxerN3P1Mp3ddNm7gci63nfrSuZ", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5VNuKafVsvbCeBs8jk77X97SXVAJGkdUpEoAA6GfEALmvYGFYR", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-31T14:21:39", - "trx_id": "1552e4c611631729fdcd8ef2327bb33beb20ba06", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 217, - { - "block": 198206, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM8hiqhFWFSgonq3CCqZWEV4qQEEcnVNCoSkGkagnwEraPnWKaAR", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM5Ccjqmgmpcir4Bn1uNMbiUi8MytuYz3aBTtXineAXu3kHY9ka8", - "new_account_name": "ned", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM6du8wJhCYQCXCWLFXPjT6Xx6FZj1AhXADizzk2t317AXao1cvp", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM83RGb5BZWTqpr8J5gTA9adnYX5aWjQcuvfA4k5zi5qEsNkoHb5", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-31T14:21:45", - "trx_id": "7f08e0025d0ae60aaf099078460a9d024387a44c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 218, - { - "block": 198211, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM8Kx9AC4G5RDH86YKssP8oGgZFaSyCrrtyzi5FoDqhpyTfmoZyx", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM5ttZbyrH434USQkd2cJfy6CNo635y1r4PiT7h2TxadwYoDa5pN", - "new_account_name": "jamesc", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5AWpDh8ZMDSt7EucS9SyLUDFTjeK3srXPTzNFafdcxKLqLN5KY", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7R2YsVRhWumpwr6BETiKC3a6qXyE2XEqTR1sXYwEv6ybkmkv1n", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-31T14:22:00", - "trx_id": "1acbba564ae89a6684c80de51dc553c90f355190", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 429, - { - "block": 433264, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6wcnr3NNZWUyYSSQnHrZ6tGL9nJtRTUV8H4pLzES9zuxPRFk3H", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM5W8FkHwsjqECnUQtJ7jKAjQtCuvk284EuMemNrcVBVbkSoTrFJ", - "new_account_name": "c-cex", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5jsJqAZjTp1NMDsCXaSpAWktrkuwzWbEe8qUEJN4L7BaPyoU3q", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5Y8SE7wGnHdRFkmHqTKnFgnAPJP76NxsTExusig8EcyLEUPr6E", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-08T22:58:45", - "trx_id": "659202237864e3b5525b186a6ac0a8723466b57a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 430, - { - "block": 433268, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM68ypyGPiQiVMKWNPNVhTYimJXc4NQvvDWP7mTGYxBNbdaCcgrD", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM7qnF6qCjZZpwpSnYn6jcC7rvz8m1eaCDAi2cCmyM5rkRm4KDpe", - "new_account_name": "bittrex", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM76ubFCQeqYGJvKhLDeXTvd3mqTTuBTFXnb2qBsSawzqUrLbc4U", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5KPPPvYXEc7pjkEVvugLBfrhEkVNFnBp7PzgMhzWiZnspS147f", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-08T22:58:57", - "trx_id": "6a03027b8bda916d87783aeede4693a27685bea7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 431, - { - "block": 433273, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6S2SvynRRLpuiw2E5zr1LucK3k4zqHL9o1GYweyY27SibTPJCx", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM5oQwU9GLFYLkwrWnna87ZuhE2TWxBtGbU4CugBuaMGEGogEgM5", - "new_account_name": "poloniex", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM8Ujas9YDjjYkqRQdAY9WfCKJwphwQ92Kfw3gviCYNoRbJJr8Nz", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7KhKkjkJ5aWvYV3ZuhryrXLwKGH3pxcbLhKBiWxgjmXiZasSSj", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-08T22:59:12", - "trx_id": "dd0ab5c5f38d28b29989cf4fa7881cfbcd9e42e8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 436, - { - "block": 480176, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6An9oJFZZMm89pDFYxuxHegHWiW8FjwwcmetL9tFU1WkyiBJkF", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM5DrHZc4d4YgcNscTbUuHNMYAYvS2iQEWPgweSL3gKT4Dxe4QRF", - "new_account_name": "bitfinex", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5jNkG1P2w2CWhGti7o4NeRQgKXgnE5yV91dKReWRKJA5FvUUbJ", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5soewi4vettBcnY3u5ozPsG1czEqzQ65KfAdpmdmWnn4PiGnyS", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-10T14:11:30", - "trx_id": "203709dec56fe7c4a51c62819fee72dbdcba46a1", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 437, - { - "block": 480180, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM7A6a8jBKxzwMTPC4H7UzYQfB7Am1Xjk5sJ9vw4Zn7ZNkzNTS1x", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM5DMLZxZ5frg5vjfYNRXtN28vYwh3Yof3SS9KDnF7EsRzp2ku1g", - "new_account_name": "bitstamp", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM7GPsuMBoYvLer59sVzfkqYWVpz5iUr47YewLD9WEmTp1dYsoR2", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7TCVrn1MgnqBGKBgh8g2zrvZsiULHSwUet53gYf6uMX2qUmwMk", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-10T14:11:42", - "trx_id": "420cb26e1792b1609f2c5ee162b1b373dfc82059", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 438, - { - "block": 480182, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM7ySRQe9RsHqAwxqTXyknbQLBZAk9DRcbL3vSoneafkMGytsoUE", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM7xVcAAMCHYnPSZk3pZ3psErzECxoHWinSmBnLKejXKgWKFt8qX", - "new_account_name": "coinbase", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM8NpUozCg6ujajwmkCERJowtMc5nEizknugoktihRRMxcWEbiyN", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7eCKurdPjvLLq1TH8iyBUS8NQ3WrnCfHNVTKQpYySMZc54MhB8", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-10T14:11:48", - "trx_id": "dbe037ae04cba3a996f826b181efe583b7ae5d21", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 439, - { - "block": 480185, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6MQ9WKP5Y2yPn46BeL1paSkucuVhVkhyThfgVK3NWgt3FdeiG9", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM69vnH5ZSRyUEWTU18uiLvqMDuVC8jJgtoxpoL3WwvXSjK4vDcj", - "new_account_name": "btc38", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM7XCbc6TFQRMVuJKuRcZUtZXMytswwqPcmxT11M574qhPKsMtyv", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7VS6d8JmLeTDwH1Ggaep6qUFYCyNX3BeKpUdKDbzadE2jTbdz1", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-10T14:11:57", - "trx_id": "4d2a1fcd8ca4ba049757a661a26439a5cec4805d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 440, - { - "block": 480199, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6Sk4cseiatcyZdGvGhsFJZPmBQ5qCuE4TQ3U8aFd5ZfnrkiYzH", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM7Li39TCCXqoPrBMWXh53iPEX1ebet6MmBUwBSJ2XR3ZpP8eiBr", - "new_account_name": "yunbi", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM65e3BmbCBvSyVUoi3Z6gUAcKDHDAPgcw7jrSsryhCnwdf6FpoG", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM8WWSPKbxfG8yNAJ8zAvKY4M6tiWb3FNvSGASRs5SUefkPmNaih", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-10T14:12:39", - "trx_id": "6f47af162a23fe18a9d722c13315c56f6cd351e6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 441, - { - "block": 480212, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6y6QimG1uK2RvBu2CUm7MpTLkfKkXkZjookEfKoQVMYBW9TWWY", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM7d1SEC31etD3vX25fhKo2QAZ3KTD9GDhD9XgkNgadQTAAeLvgx", - "new_account_name": "kraken", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM55U2FhkdbLxSGUZP7L2z3Chz9h5jWBSLy7hW7iuZbo1tA42K8Y", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM53BBMVexQsXdU8UkyN8iAB8SNMBgga9vem4RgSstiTdfCVNH2e", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-10T14:13:18", - "trx_id": "da82179ad33bbfcd880371b855dc906425ad8d59", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 475, - { - "block": 739855, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6oeAqAsqfs9PAeSJa1bz4ntPDSdyfLeHzM5UxSDBGi244M6UHa", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM6x53WEDDVk3bKU31pNZHuBxvzAS2tSYwjG7rc8qWi1vY5DcY34", - "new_account_name": "stan", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM82NpnGVcrrmDE5gvU2UfNcDa9YwVgFEyjywpLZdg5w9VuG81RU", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7NUmpdSkktoaAw3F6K4wpSrxbe6Tfqxr2Zg3TgL3hKyGhCFeHE", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-19T20:26:00", - "trx_id": "1b84307e978480330b740f964b20c1e2674918ac", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 480, - { - "block": 764820, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM5y61oce9jA9qDZfFBzwQFEUFqcdjGPfDfquMcJjRW1FE4VDQUR", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM7SCGavPLAgT9tic335WDcMDptZRCNM4u7mQQmdBC7p3MRsRyyr", - "new_account_name": "lewrockwell", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5bUk47wFfiYizXwiC9ttRYjsudDs9ZApA78HUehvfEbCSeQ4HD", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7R9LQDCYucWzuiovuakChLNpizx5188rPcbXQ6HvG3NPSrmEEe", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-20T17:33:54", - "trx_id": "bb289475b95eaa893258a11ebbc505d5d58efb3d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 481, - { - "block": 764826, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM69b7F9puDtcLzD2VVQBZiJjHuoMYZ3kMLMUqt66P8QJZQojqM2", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM7ggg2rqPEdUS27dz8anqoDkWPJ7Fco2kUy5yBTgtgtcgoXNAGr", - "new_account_name": "infowars", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM7EzRwyNF4DZ6enZQ3PByS4hVRRJ7T7RTETYZ7PxEZMqSJYCjrk", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7emutNijHeieFC1hB1JpRc1WcUavNBrKHJseW5Ysv5Pk2JxXyM", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-20T17:34:15", - "trx_id": "c3c63f049cb1828e4302e4e3c713dea3c02de635", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 517, - { - "block": 929318, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM5tQG6Kx9ssmCQwftmU57K5UxPnLSZbYC8Vm2eVb562oPmvSrDB", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "100.000 HIVE", - "json_metadata": "", - "memo_key": "STM8SC3JAKgwcXy1A1Umb4pcbnBbTaXBmhHTnYZPX9c3aofErZa1a", - "new_account_name": "bittrex.witness", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM7bwDRd1VYzwC7YnAhqHaiPCZB87k4mnehDxaL1ytitahjkDb9U", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5RRTU1TNGa2qnxhAsRwBiMms734WE6ySAEV3L1vZUMeHj8cXSu", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-26T13:32:18", - "trx_id": "81b2127820396f9a3e9143e135e34a75d6a11f71", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 555, - { - "block": 1041710, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM84LEBJfEUXbUpZjvr5WBZBwYJptm9qpiP7Svx6qY33Sb6RT9Xa", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "10.000 HIVE", - "json_metadata": "", - "memo_key": "STM83MSz1d1pCoDDH1hF5J4uDk8cr9EbPRSGFVa6qEoF43TNYPUTf", - "new_account_name": "steemit.com", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5PrjzZbaR64zDzmSmkL5mhGN79PJFkVCR9wBnoeUxazjBUMn86", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5S4aPJDMKVgzXaDXLCN79sxVcjjGU6LeWUksLm8np3p4PpVgqu", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-30T15:10:36", - "trx_id": "c7c1d6ff09bb5dc7196cd7316ee961bcb315af70", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 557, - { - "block": 1162106, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6x2y7EKu9f4aufRMYM1ZMZ6d4AgtTeGgvmNdd94Ug5wdWcZTts", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "10.000 HIVE", - "json_metadata": "", - "memo_key": "STM5UEmjJAEYxfRVfJ6GwGrsWtQti4SnLGBKyqNL9qKnpVMn6uz7b", - "new_account_name": "coindesk.com", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM7tRimVpL4n4mSNTzBTvxws1JMTpfADa3aPSmpfojyPkcyda25D", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM7biejzixUZgShLQA4T99UE3gg2Ag6Zds2MUF4p7JbLtHkT1bQc", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-05-04T19:49:27", - "trx_id": "2bf3782d561cfa113508169ad553ed770d0b4762", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 558, - { - "block": 1162134, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6joHRHQgSRmcT6pnFL85wjL124MhqT6YmMswzYX9BsNMermvzP", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "10.000 HIVE", - "json_metadata": "", - "memo_key": "STM78WqRbVuzVnNf6rUhYXdA3Dwecc3kXRygrTfBkaA6EefHfYwod", - "new_account_name": "lewrockwell.com", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM7pcHpA75DHhBSqvtYQU27qZS99HtYZDzbNQwHYeCLd4bVjwXXS", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM6XFdwjP7bmxFxS4GHRmFu62sY3RpN6zPV13JyxFAxqwFEcTvz6", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-05-04T19:50:51", - "trx_id": "b059bafbf53db247e9e4ae8f6e90fcb31c7693c9", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 559, - { - "block": 1162164, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM5sgQYbsM9E1DH3QEHuMePCEeQQUmun5eSijksDmHWWe8PniFhz", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "10.000 HIVE", - "json_metadata": "", - "memo_key": "STM6e3fZF4DounACP24BD8b6v2U5LcXpC7Bcvww1XF2Qdoyre8amP", - "new_account_name": "ericpeters", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM6kG7jrPLipT2MCY61Y6Bj15bBGjAK6uA2bUgYKDMV29m9Qfd7L", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM6uKQ7pLbAbQ4CuK9s15wNrvREkd1GJMSHUeydyJ2oeY3Pnwwes", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-05-04T19:52:21", - "trx_id": "aca59338cf99f548c389cfe5ab9cf9b20e3fe055", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 579, - { - "block": 1735438, - "op": [ - "account_create", - { - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6pKNVGdfpUqrNNDZSDM5WtZgSRixB9cxVPzgqwDWB5zX64Ymi4", - 1 - ] - ], - "weight_threshold": 1 - }, - "creator": "steemit", - "fee": "10.000 HIVE", - "json_metadata": "", - "memo_key": "STM4uqeZ8nP6hEu2Sv3rUyVUNdbGzV6djK2wEGqAE1Qe49uhRowum", - "new_account_name": "steemitblog", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5MzR22PLJVoXBvc9B1GA5WZPhWbQLggnBZMseRPeCLqdcWyt6y", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM577PWfStn2GMRqZp6DFqa1QuhEpiqxxcgCA1we3tR2GPDkzXYg", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-05-24T18:54:24", - "trx_id": "7ee9d5cf95aed0871742e94afd7886e25e15ea43", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/account_create_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/account_create_operation.tavern.yaml deleted file mode 100644 index 0cbab853558fbf482925e450a12c802fc1002639..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/account_create_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["steemit",1000,1000,512] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "account_create_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/account_update_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/account_update_operation.pat.json deleted file mode 100644 index e3eb82e7ab71173f7a42a68cfcd8072e557e19f5..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/account_update_operation.pat.json +++ /dev/null @@ -1,235 +0,0 @@ -[ - [ - 139, - { - "block": 2885318, - "op": [ - "account_update", - { - "account": "gtg", - "json_metadata": "", - "memo_key": "STM5F9tCbND6zWPwksy1rEN24WjPiQWSU2vwGgegQVjAcYDe1zTWi", - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM69ZG1hx2rdU2hxQkkmX5MmYkHPCmdNeXg4r6CR7gvKUzYwWPPZ", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T20:54:21", - "trx_id": "28590cbf62ba9f0ec6dd6fe9669852068772ee59", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 140, - { - "block": 2885370, - "op": [ - "account_update", - { - "account": "gtg", - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6AzXNwWRzTWCVTgP4oKQEALTW8HmDuRq1avGWjHH23XBNhux6U", - 1 - ] - ], - "weight_threshold": 1 - }, - "json_metadata": "", - "memo_key": "STM5F9tCbND6zWPwksy1rEN24WjPiQWSU2vwGgegQVjAcYDe1zTWi" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T20:56:57", - "trx_id": "60a00c1016cede7d7924f6b32ba4190252b369b7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 141, - { - "block": 2885375, - "op": [ - "account_update", - { - "account": "gtg", - "json_metadata": "", - "memo_key": "STM78Vaf41p9UUMMJvafLTjMurnnnuAiTqChiT5GBph7VDWahQRsz" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T20:57:12", - "trx_id": "57a8a136ff02b6b89c31b55314c7e3112939be14", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 142, - { - "block": 2885409, - "op": [ - "account_update", - { - "account": "gtg", - "json_metadata": "", - "memo_key": "STM6EY4jgDpLKrJa1RE5y49oUu64BLTrjo4SAso7fxtsaY8Mpzqgx" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T20:58:54", - "trx_id": "3fc900bf7e4ce2f81664359d399ec0c61826f0a0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 143, - { - "block": 2885463, - "op": [ - "account_update", - { - "account": "gtg", - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6AzXNwWRzTWCVTgP4oKQEALTW8HmDuRq1avGWjHH23XBNhux6U", - 1 - ] - ], - "weight_threshold": 1 - }, - "json_metadata": "", - "memo_key": "STM78Vaf41p9UUMMJvafLTjMurnnnuAiTqChiT5GBph7VDWahQRsz", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM5F9tCbND6zWPwksy1rEN24WjPiQWSU2vwGgegQVjAcYDe1zTWi", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM69ZG1hx2rdU2hxQkkmX5MmYkHPCmdNeXg4r6CR7gvKUzYwWPPZ", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T21:01:36", - "trx_id": "59ccc2dd299ada55308a5f4fd9e2735847e92a58", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 355, - { - "block": 3191323, - "op": [ - "account_update", - { - "account": "gtg", - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM6AzXNwWRzTWCVTgP4oKQEALTW8HmDuRq1avGWjHH23XBNhux6U", - 1 - ] - ], - "weight_threshold": 1 - }, - "json_metadata": "", - "memo_key": "STM78Vaf41p9UUMMJvafLTjMurnnnuAiTqChiT5GBph7VDWahQRsz", - "owner": { - "account_auths": [], - "key_auths": [ - [ - "STM6oUcdLthTMc2CDQk5Docc8pB9Zh9s5EQ1wjBZwnMvggbMkRBth", - 1 - ] - ], - "weight_threshold": 1 - }, - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM69ZG1hx2rdU2hxQkkmX5MmYkHPCmdNeXg4r6CR7gvKUzYwWPPZ", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-14T13:29:03", - "trx_id": "8edfc3159e6b8490e86262dc066336f85b730be7", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 377, - { - "block": 3399203, - "op": [ - "account_update", - { - "account": "gtg", - "active": { - "account_auths": [], - "key_auths": [ - [ - "STM4vuEE8F2xyJhwiNCnHxjUVLNXxdFXtVxgghBq5LVLt49zLKLRX", - 1 - ] - ], - "weight_threshold": 1 - }, - "json_metadata": "", - "memo_key": "STM4uD3dfLvbz7Tkd7of4K9VYGnkgrY5BHSQt52vE52CBL5qBfKHN", - "posting": { - "account_auths": [], - "key_auths": [ - [ - "STM5tp5hWbGLL1R3tMVsgYdYxLPyAQFdKoYFbT2hcWUmrU42p1MQC", - 1 - ] - ], - "weight_threshold": 1 - } - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-21T21:48:06", - "trx_id": "5a3a345d3265d40fd4f7ac1906fddec59ac177c3", - "trx_in_block": 1, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/account_update_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/account_update_operation.tavern.yaml deleted file mode 100644 index a185b8055794038e36d9832a1bcdeda230ab0983..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/account_update_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",1000,1000,1024] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "account_update_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/account_witness_proxy_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/account_witness_proxy_operation.pat.json deleted file mode 100644 index d1011f7d11358f1cd503b63bc2c95231d29398fc..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/account_witness_proxy_operation.pat.json +++ /dev/null @@ -1,20 +0,0 @@ -[ - [ - 759, - { - "block": 3924580, - "op": [ - "account_witness_proxy", - { - "account": "geoffrey", - "proxy": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-09T06:52:00", - "trx_id": "481a52b2a98177c699590240ad845c3b354dd77e", - "trx_in_block": 1, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/account_witness_proxy_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/account_witness_proxy_operation.tavern.yaml deleted file mode 100644 index 831624b446e803cbfc01d6979fde31a2408bf2d7..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/account_witness_proxy_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",1000,1000,8192] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "account_witness_proxy_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/account_witness_vote_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/account_witness_vote_operation.pat.json deleted file mode 100644 index 7a3af8766b520ad8ac6c3394667e7bce2777bb4d..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/account_witness_vote_operation.pat.json +++ /dev/null @@ -1,667 +0,0 @@ -[ - [ - 103, - { - "block": 2881334, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T17:35:00", - "trx_id": "1e49a977ca64c7762042f8ef55d8583d66faad66", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 301, - { - "block": 3100789, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "pfunk" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-11T09:40:45", - "trx_id": "7387eac7188e69e610b412074875352b3002945a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 304, - { - "block": 3104291, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "arhag" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-11T12:36:12", - "trx_id": "8540eb01bab6982a2d477f1949cbfedcb335a2da", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 305, - { - "block": 3105480, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "witness.svk" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-11T13:35:54", - "trx_id": "e888067abbcbe74920013a8a441b9eb683ed9aa6", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 306, - { - "block": 3105636, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "smooth.witness" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-11T13:43:42", - "trx_id": "50e85ae81e53ce8793e03f736eb97ea8162c37da", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 328, - { - "block": 3144657, - "op": [ - "account_witness_vote", - { - "account": "anyx", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-12T22:23:33", - "trx_id": "43f186092b37a9ceab49f4f6369a861709523e11", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 404, - { - "block": 3647042, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "liondani" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-30T14:17:06", - "trx_id": "9cc490746a6e3449180a22fc3641bd643708bf67", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 405, - { - "block": 3647231, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "anyx" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-30T14:26:42", - "trx_id": "e1af257db286696d487ee9cf16b3dc29a04a4082", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 406, - { - "block": 3647439, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "roadscape" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-30T14:37:15", - "trx_id": "a8994fd0ba8aa0f91db19445fb2e07bb73dd28ae", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 423, - { - "block": 3701313, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "riverhead" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-01T11:45:42", - "trx_id": "59d382c45ee0b92e770afa05551a912fc2926285", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 459, - { - "block": 3784027, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "complexring" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-04T08:56:48", - "trx_id": "4a85960e6181f411ff5c5409411b472fa652cb16", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 460, - { - "block": 3784059, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "blocktrades" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-04T08:58:24", - "trx_id": "d448cf3bbe37ea308d8905ea580b79ff25b3dc43", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 488, - { - "block": 3820266, - "op": [ - "account_witness_vote", - { - "account": "blocktrades", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-05T15:28:00", - "trx_id": "58ad1534d3bebbd2597aec774859ec9892457fdb", - "trx_in_block": 7, - "virtual_op": 0 - } - ], - [ - 526, - { - "block": 3821908, - "op": [ - "account_witness_vote", - { - "account": "inertia", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-05T16:50:24", - "trx_id": "a7a65ec3e971eeb4a5bf724513e6fbdbbed08982", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 542, - { - "block": 3823310, - "op": [ - "account_witness_vote", - { - "account": "felixxx", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-05T18:00:48", - "trx_id": "09609c5bf3b56f0e33081f671dfe9b027e3767b6", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 579, - { - "block": 3827447, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "chitty" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-05T21:29:00", - "trx_id": "e604c679b75357ae92ce86ce3ed5b7bdcf5f3ef5", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 580, - { - "block": 3827465, - "op": [ - "account_witness_vote", - { - "account": "chitty", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-05T21:29:54", - "trx_id": "0dd584b564b868150d6619f0a212ac62fcfb1198", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 664, - { - "block": 3846204, - "op": [ - "account_witness_vote", - { - "account": "dave-mohican", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-06T13:11:06", - "trx_id": "e2cf917960a80b4c06ccde992c2bd832b2bb0dd7", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 668, - { - "block": 3849814, - "op": [ - "account_witness_vote", - { - "account": "keung0109", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-06T16:12:03", - "trx_id": "ae32b6cc44f73c0be7c423f2e3c1ddcadfbbb509", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 670, - { - "block": 3850577, - "op": [ - "account_witness_vote", - { - "account": "thebluepanda", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-06T16:50:15", - "trx_id": "a29d26cabcfdd985c988d0fb4f09f9665d21ea54", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 678, - { - "block": 3853759, - "op": [ - "account_witness_vote", - { - "account": "fydel", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-06T19:29:45", - "trx_id": "110368b476b60871e9e52df55b642ab0fbb96890", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 679, - { - "block": 3854924, - "op": [ - "account_witness_vote", - { - "account": "heimindanger", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-06T20:28:09", - "trx_id": "53c39c99441fdd23785e16b512ebec26955ae827", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 682, - { - "block": 3857664, - "op": [ - "account_witness_vote", - { - "account": "geoffrey", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-06T22:45:45", - "trx_id": "d9f8c3ebbb04d7652dde97027af657429e2c25f7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 689, - { - "block": 3866830, - "op": [ - "account_witness_vote", - { - "account": "steems", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-07T06:25:21", - "trx_id": "7dc8b63a6a828d7712699c5a79ea0b56063ffa45", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 719, - { - "block": 3892188, - "op": [ - "account_witness_vote", - { - "account": "jesus2", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-08T03:38:51", - "trx_id": "9d0c9228771c188fbaa8dfe86551ef9ab53b6021", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 721, - { - "block": 3893448, - "op": [ - "account_witness_vote", - { - "account": "myheadhurts", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-08T04:42:09", - "trx_id": "211f47c061adbac3f7669ac55df68939e350b2ea", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 733, - { - "block": 3902222, - "op": [ - "account_witness_vote", - { - "account": "spaninv", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-08T12:02:06", - "trx_id": "7005646617a7f33cd49c1b1fb04814ce58e9f803", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 756, - { - "block": 3923321, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "geoffrey" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-09T05:49:03", - "trx_id": "f3103c6232525d7ff40151af618cd8d954c254db", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 774, - { - "block": 3937158, - "op": [ - "account_witness_vote", - { - "account": "dave-hughes", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-09T17:21:09", - "trx_id": "a088f4aa24e994ac3a99556618d9a02fd4dfd22a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 780, - { - "block": 3940856, - "op": [ - "account_witness_vote", - { - "account": "dan", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-09T20:29:30", - "trx_id": "9a2b05e8ae3c5494f65e8a9a1be90c5a6f98b9c6", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 781, - { - "block": 3940858, - "op": [ - "account_witness_vote", - { - "account": "dantheman", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-09T20:29:36", - "trx_id": "66e08a863fd1131d66c770bfe39fac1658c55506", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 782, - { - "block": 3941301, - "op": [ - "account_witness_vote", - { - "account": "dashpaymag", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-09T20:51:48", - "trx_id": "d7e98b40ffc96df685bb8fa4f8a8c79020ef63a0", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 783, - { - "block": 3941303, - "op": [ - "account_witness_vote", - { - "account": "steemed", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-09T20:51:54", - "trx_id": "34e99d1149636597220197a4de7587b461e98eb8", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 899, - { - "block": 3993894, - "op": [ - "account_witness_vote", - { - "account": "pumpkin", - "approve": true, - "witness": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-11T16:48:03", - "trx_id": "d14424769d27d9a1a454c51fcd45baa5850ce7fa", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 984, - { - "block": 4024103, - "op": [ - "account_witness_vote", - { - "account": "gtg", - "approve": true, - "witness": "wackou" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-12T18:07:06", - "trx_id": "c705f03051dbae27787c23b8fefe50bb2ccc0164", - "trx_in_block": 2, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/account_witness_vote_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/account_witness_vote_operation.tavern.yaml deleted file mode 100644 index 4c43f8eeda4fcf615879e416541e439c2ed9d9d9..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/account_witness_vote_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",1000,1000,4096] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "account_witness_vote_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/comment_and_convert_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/comment_and_convert_operation.pat.json deleted file mode 100644 index 4770d1e18551e21bf40c5d0cac43d7921218638a..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/comment_and_convert_operation.pat.json +++ /dev/null @@ -1,431 +0,0 @@ -[ - [ - 66, - { - "block": 2880136, - "op": [ - "comment", - { - "author": "gtg", - "body": "I'm Gandalf.\nThat's not my real name, but even my mom saved my number as ***Gandalf*** in her phone contact list.\nThat counts, right?\nI'm an IT Wizard, system admin, happily married, <strike>owner of a cat</strike>.\nOwned by a cat.\n\n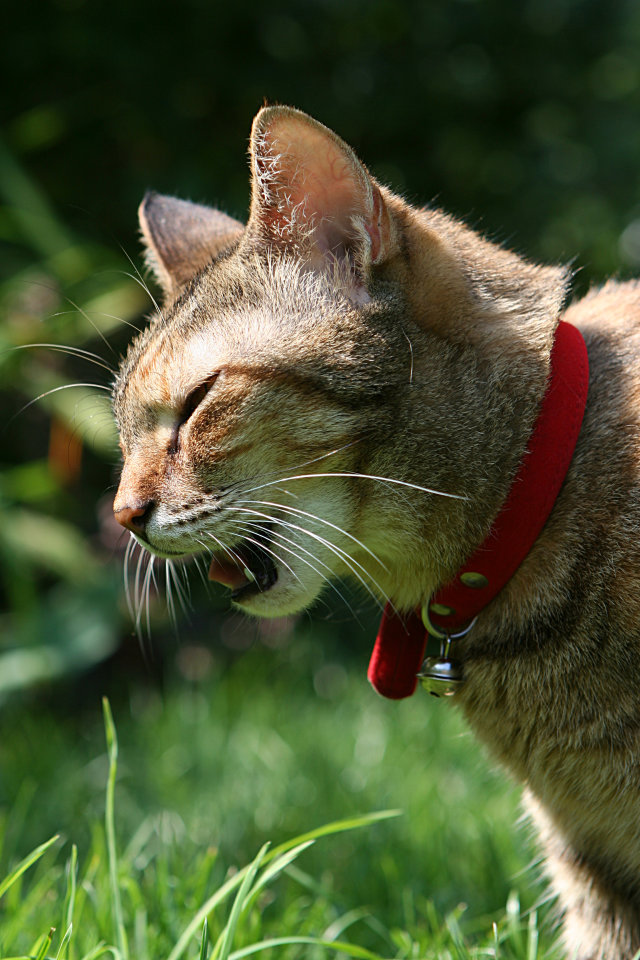\n\n**Nyunya** *(The Cat)* wants to watch everything I do, that's just her way of lending a helping paw. I\u2019m not sure if she is so fascinated by what I do or she wants to make sure I do it right.\n\nWhy am I here? What is my motivation?\nNot much different from that guiding my cat:\nCuriosity and to be a witness (node?) of what is emerging here.\n\nMay the STEEM be with you!", - "json_metadata": "{\"tags\":[\"introduceyourself\",\"cats\"],\"image\":[\"https://grey.house/img/niunia.jpg\"]}", - "parent_author": "", - "parent_permlink": "introduceyourself", - "permlink": "hello-world", - "title": "Hello, World!" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T16:35:03", - "trx_id": "3f99bf13f5e78fcd4120075bea6933339516f188", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 113, - { - "block": 2881669, - "op": [ - "comment", - { - "author": "trogdor", - "body": "Nice, I understand the \"owned by a cat\" sentiment. haha", - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "parent_author": "gtg", - "parent_permlink": "hello-world", - "permlink": "re-gtg-hello-world-20160703t175141501z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T17:51:48", - "trx_id": "86a7bbf1c37601fb1ab5a4b7ff32dc909dd142ec", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 158, - { - "block": 2887536, - "op": [ - "comment", - { - "author": "amartinezque", - "body": "Cats, humans , all we move for instinct and curiosity. Welcome! And your cat also!:P", - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "parent_author": "gtg", - "parent_permlink": "hello-world", - "permlink": "re-gtg-hello-world-20160703t224527020z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T22:45:27", - "trx_id": "bee17bf2245d2b56ccaa71e2e74db943df9ae161", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 182, - { - "block": 2910577, - "op": [ - "comment", - { - "author": "edu-lopov", - "body": "Welcome to Steemit Gandalf!", - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "parent_author": "gtg", - "parent_permlink": "hello-world", - "permlink": "re-gtg-hello-world-20160704t182251522z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-04T18:22:51", - "trx_id": "cac4a9c7f617d140d09053600b36bca7c90524a7", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 188, - { - "block": 2912870, - "op": [ - "convert", - { - "amount": "127.144 HBD", - "owner": "gtg", - "requestid": 1467663446 - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-04T20:18:00", - "trx_id": "8930ea36f61aef6fa7c5c79922cc9892f2d7373b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 189, - { - "block": 2912956, - "op": [ - "convert", - { - "amount": "0.002 HBD", - "owner": "gtg", - "requestid": 1467663726 - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-04T20:22:18", - "trx_id": "804339e4617544b47018e165d7a8ed45ac38f002", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 279, - { - "block": 3072023, - "op": [ - "comment", - { - "author": "gtg", - "body": "Happened to me, my 3rd Jul post https://steemit.com/introduceyourself/@gtg/hello-world \nHit $723.07 at 23:19 UTC+02 ended up ~$256", - "json_metadata": "{\"tags\":[\"catharsis\"],\"links\":[\"https://steemit.com/introduceyourself/@gtg/hello-world\"]}", - "parent_author": "pfunk", - "parent_permlink": "bear-with-me-while-i-bitch-about-losing-usd1000-on-a-post-due-to-the-july-4th-payout-and-fork", - "permlink": "re-pfunk-bear-with-me-while-i-bitch-about-losing-usd1000-on-a-post-due-to-the-july-4th-payout-and-fork-20160710t093728262z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-10T09:37:30", - "trx_id": "722393fcc1264595dd131aa5f12a8e69054ef957", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 286, - { - "block": 3075654, - "op": [ - "comment", - { - "author": "gtg", - "body": "I'm Gandalf.\nThat's not my real name, but even my mom saved my number as ***Gandalf*** in her phone contact list.\nThat counts, right?\nI'm an IT Wizard, system admin, happily married, <strike>owner of a cat</strike>.\nOwned by a cat.\n\n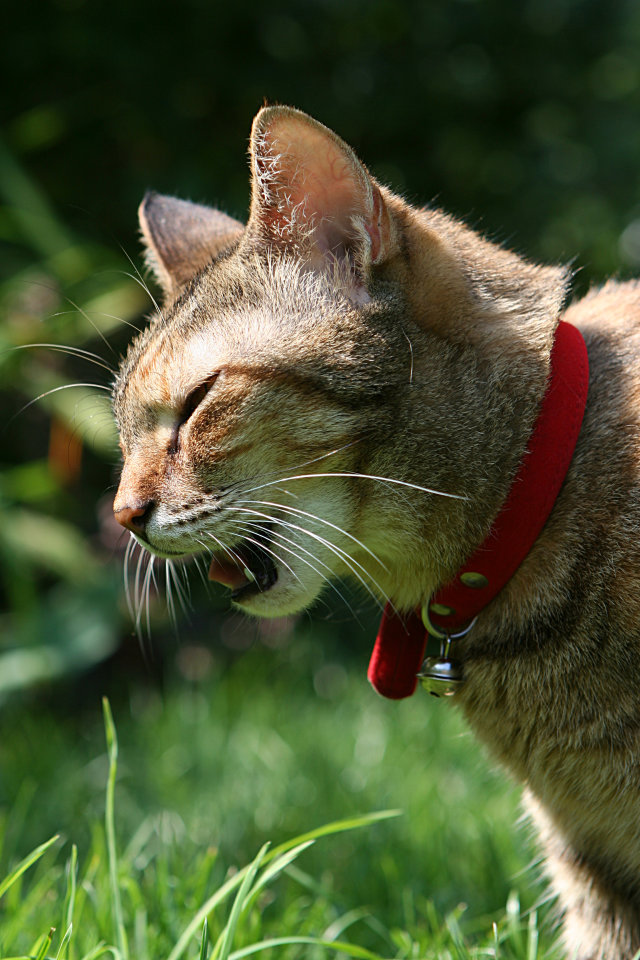\n\n**Nyunya** *(The Cat)* wants to watch everything I do, that's just her way of lending a helping paw. I\u2019m not sure if she is so fascinated by what I do or she wants to make sure I do it right.\n\nWhy am I here? What is my motivation?\nNot much different from that guiding my cat:\nCuriosity and to be a witness (node?) of what is emerging here.\n\nMay the STEEM be with you!", - "json_metadata": "{\"tags\":[\"introduceyourself\",\"cats\"],\"image\":[\"https://grey.house/img/niunia.jpg\"]}", - "parent_author": "", - "parent_permlink": "introduceyourself", - "permlink": "hello-world", - "title": "Hello, World!" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-10T12:39:42", - "trx_id": "a04aa61579b65412036b6e1f79967006b54c508e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 447, - { - "block": 3759799, - "op": [ - "comment", - { - "author": "gtg", - "body": "Zaufanie do delegata si\u0119 liczy. Jego narodowo\u015b\u0107 nie.\nG\u0142osowa\u0107 warto, \u015bwiadomie.\nRozumiem, \u017ce nie ka\u017cdy ma czas i ch\u0119ci, by by\u0107 na bie\u017c\u0105co z tym, co tu si\u0119 dzieje 'od kuchni'. Ja staram si\u0119 przygl\u0105da\u0107 temu uwa\u017cnie i g\u0142osuj\u0119 na tych, kt\u00f3rzy sobie zas\u0142u\u017cyli na to wg mojej skromnej, subiektywnej oceny.\nSam r\u00f3wnie\u017c b\u0119d\u0119 si\u0119 stara\u0142 o wy\u017csz\u0105 pozycj\u0119 na li\u015bcie, ale na razie zbyt wiele innych zaj\u0119\u0107 (zwi\u0105zanych ze steemit)\nAby g\u0142osowa\u0107 tak jak ja mo\u017cna w **cli_wallet** wykona\u0107 polecenie\n`set_voting_proxy \"youraccountname\" \"gtg\" true`\nZr\u00f3b to tylko wtedy je\u015bli jeste\u015b w stanie zaufa\u0107 mi co do moich wybor\u00f3w w sprawie delegat\u00f3w.", - "json_metadata": "{\"tags\":[\"polish\"]}", - "parent_author": "mefisto", - "parent_permlink": "pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness", - "permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-03T12:39:42", - "trx_id": "8325110ddfa8b352975e6fd3bff448c3468efc87", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 450, - { - "block": 3760135, - "op": [ - "comment", - { - "author": "mefisto", - "body": "Kryterium wyboru delegata to osobna sprawa. Co delegat powinien potrafi\u0107?Co robi\u0107? Czy powinien poniek\u0105d s\u0142u\u017cy\u0107 swoim wyborcom?\nJedno zdaje si\u0119 by\u0107 pewne - **powinien by\u0107 aktywnym cz\u0142onkiem spo\u0142eczno\u015bci steemit.** \nJestem niemal pewny, \u017ce jestesmy w stanie wybra\u0107 wskaza\u0107 kilka os\u00f3b, kt\u00f3re mog\u0142yby zbiera\u0107 glosy polskiej spo\u0142eczno\u015bci. Je\u015bli si\u0119 nie myl\u0119, g\u0142os\u00f3w b\u0119dzie wi\u0119cej - je\u015bli system g\u0142osowania na dodatkowych kandydat\u00f3w b\u0119dzie prostszy dla przeci\u0119tnego po\u017ceracza blog\u00f3w... np. pojawi si\u0119 \u0142adny graficzny klient pod windoz\u0119.", - "json_metadata": "{\"tags\":[\"polish\"]}", - "parent_author": "gtg", - "parent_permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "permlink": "re-gtg-re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t125639335z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-03T12:56:33", - "trx_id": "66fb777f2f36b7a3666f236228bddd1eeeeed1ed", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 451, - { - "block": 3760145, - "op": [ - "comment", - { - "author": "mefisto", - "body": "@@ -66,16 +66,17 @@\n otrafi%C4%87?\n+ \n Co robi%C4%87\n", - "json_metadata": "{\"tags\":[\"polish\"]}", - "parent_author": "gtg", - "parent_permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "permlink": "re-gtg-re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t125639335z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-03T12:57:03", - "trx_id": "176074c6fb5f33db4fdf0291c49b7082e9393b33", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 452, - { - "block": 3760160, - "op": [ - "comment", - { - "author": "mefisto", - "body": "@@ -238,17 +238,17 @@\n %C5%BCe jeste\n-s\n+%C5%9B\n my w sta\n@@ -257,16 +257,18 @@\n e wybra%C4%87\n+ /\n wskaza%C4%87\n@@ -331,16 +331,18 @@\n zno%C5%9Bci. \n+%0A%0A\n Je%C5%9Bli si\n", - "json_metadata": "{\"tags\":[\"polish\"]}", - "parent_author": "gtg", - "parent_permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "permlink": "re-gtg-re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t125639335z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-03T12:57:48", - "trx_id": "e6cca453b183babf4de47b155e5fc2fd09adc273", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 453, - { - "block": 3760183, - "op": [ - "comment", - { - "author": "mefisto", - "body": "@@ -220,18 +220,16 @@\n tem \n-niemal pew\n+przekona\n ny, \n", - "json_metadata": "{\"tags\":[\"polish\"]}", - "parent_author": "gtg", - "parent_permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "permlink": "re-gtg-re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t125639335z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-03T12:58:57", - "trx_id": "e1e36b533b9ca71b26e28d81f4191f6f79dc874c", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 474, - { - "block": 3818561, - "op": [ - "comment", - { - "author": "gtg", - "body": "Hello, World!\n\nMy intention is not to make it into top 19. I could name many other candidates (clearly more than 19) with greater commitment to steem(it) who are a lot more appropriate as witnesses, but I'm sure I can be useful as a backup witness.\n\nI introduced myself here:\nhttps://steemit.com/introduceyourself/@gtg/hello-world\nThose of you who use https://steemit.chat know me as **Gandalf**.\nFor some time, I\u2019ve been using my magic powers (mostly those related to security and infrastructure) to improve steemit. For example, I\u2019ve improved our \"Perfect\" Forward Secrecy and made our communication with site more secure.\n\nMy **seed node** (EU based):\n`seed-node = gtg.steem.house:2001`\nYou can also use it to rsync data dir\n`rsync -Pa rsync://gtg.steem.house/witness_node_data_dir .`\n\nMy **witness node** is on a separate data center (an EU-based one as well).\nIf you believe I can be of value to steemit, please vote for me:\n`vote_for_witness YOURACCOUNT gtg true true`\n\n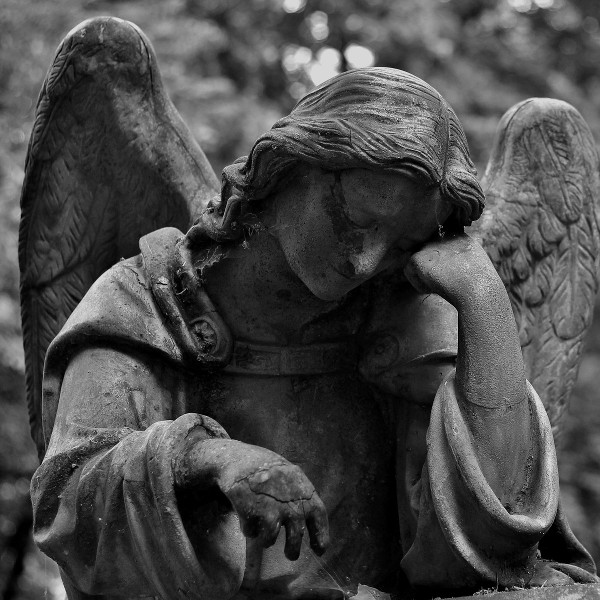", - "json_metadata": "{\"tags\":[\"witness-category\"],\"links\":[\"https://steemit.com/introduceyourself/@gtg/hello-world\"]}", - "parent_author": "", - "parent_permlink": "witness-category", - "permlink": "witness-gtg", - "title": "Witness \"gtg\"" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-05T14:02:24", - "trx_id": "bf5e83b7ddc4efcaf101f5260653a3588e411f36", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 505, - { - "block": 3820615, - "op": [ - "comment", - { - "author": "cire81", - "body": "Thank you for spending your time to make steemit secure. Thanks to people like you we have a much safer environment. Were you paid for your effort on steem?", - "json_metadata": "{\"tags\":[\"witness-category\"]}", - "parent_author": "gtg", - "parent_permlink": "witness-gtg", - "permlink": "re-gtg-witness-gtg-20160805t154528701z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-05T15:45:27", - "trx_id": "47696dcd765db02c2de5827adf4afe65841158eb", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 519, - { - "block": 3821150, - "op": [ - "comment", - { - "author": "iaco", - "body": "As the computer world beyond user interfaces is a magical realm to me, the only way I can get my brain to completely understand it is to just let go and tell my self it's all made of unicorns and runes being cast to make the magic happen :) I thank you for your work:)", - "json_metadata": "{\"tags\":[\"witness-category\"]}", - "parent_author": "gtg", - "parent_permlink": "witness-gtg", - "permlink": "re-gtg-witness-gtg-20160805t161219379z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-05T16:12:21", - "trx_id": "95e2dd24c9aa395d36da098fc8c9589cd7b95c2a", - "trx_in_block": 3, - "virtual_op": 0 - } - ], - [ - 546, - { - "block": 3824218, - "op": [ - "comment", - { - "author": "gtg", - "body": "Dzi\u015b oficjalnie zg\u0142osi\u0142em kandydatur\u0119.\nhttps://steemit.com/witness-category/@gtg/witness-gtg\n> Koncentracja g\u0142os\u00f3w - mo\u017ce z czasem pom\u00f3c wybra\u0107 kogo\u015b z naszych do pierwszej 50-tki.\n\nOd pocz\u0105tku by\u0142em w pierwszej setce i aby g\u0142osowa\u0107 konieczne by\u0142o u\u017cycie `cli_wallet`\nObecnie jestem w pierwszej 50-tce, wi\u0119c mo\u017cna g\u0142osowa\u0107 ju\u017c bezpo\u015brednio ze strony:\nhttps://steemit.com/~witnesses", - "json_metadata": "{\"tags\":[\"polish\"],\"links\":[\"https://steemit.com/witness-category/@gtg/witness-gtg\"]}", - "parent_author": "mefisto", - "parent_permlink": "pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness", - "permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160805t184622691z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-05T18:46:27", - "trx_id": "9c5197bcd87f4207c122ed47e1be9fb2102c0226", - "trx_in_block": 8, - "virtual_op": 0 - } - ], - [ - 874, - { - "block": 3985180, - "op": [ - "comment", - { - "author": "gtg", - "body": "Of course It is truly right. It doesn't manipulate the free market. It says: _\"Hey, better watch out, fake products over there!\"_, so other buyers (upvoters) have choice to follow that advice or not. That's because of that freedom, not against it. Besides, I like cats. :-)", - "json_metadata": "{\"tags\":[\"steemitabuse\"]}", - "parent_author": "heretickitten", - "parent_permlink": "re-cheetah-cheetah-bot-explained-20160811t033141148z", - "permlink": "re-heretickitten-re-cheetah-cheetah-bot-explained-20160811t093103978z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-11T09:31:09", - "trx_id": "f8d0ac4efa8b392935b82eed25678170853e98ff", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 902, - { - "block": 3994822, - "op": [ - "comment", - { - "author": "gtg", - "body": "`overmind`sounds good, but maybe morph it to `overmint`\nWhere `mint`is like in _\u201che made a mint on the stock market\u201d_ and/or like in _\"The United States Mint\"_ + of course like misspelled _\"mind\"_.", - "json_metadata": "{\"tags\":[\"steem\"]}", - "parent_author": "wackou", - "parent_permlink": "btstools-development-bounties", - "permlink": "re-wackou-btstools-development-bounties-20160811t173426180z", - "title": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-11T17:34:33", - "trx_id": "97be0ae220643dc8aff2633140c65ea750f77c41", - "trx_in_block": 3, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/comment_and_convert_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/comment_and_convert_operation.tavern.yaml deleted file mode 100644 index 0ddcb1968c131acdcad59dade5f06d74a5449d07..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/comment_and_convert_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",1000,1000,258] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "comment_and_convert_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/comment_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/comment_operation.pat.json deleted file mode 100644 index a880052f0ad3836eec06233eaac5363d1e1ad6b3..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/comment_operation.pat.json +++ /dev/null @@ -1,25 +0,0 @@ -[ - [ - 66, - { - "block": 2880136, - "op": [ - "comment", - { - "author": "gtg", - "body": "I'm Gandalf.\nThat's not my real name, but even my mom saved my number as ***Gandalf*** in her phone contact list.\nThat counts, right?\nI'm an IT Wizard, system admin, happily married, <strike>owner of a cat</strike>.\nOwned by a cat.\n\n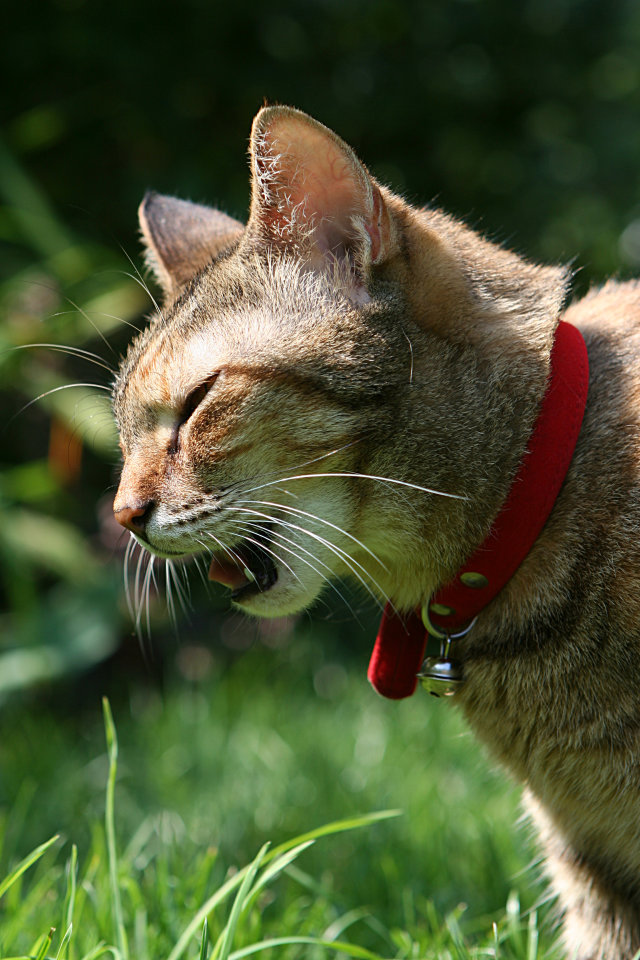\n\n**Nyunya** *(The Cat)* wants to watch everything I do, that's just her way of lending a helping paw. I\u2019m not sure if she is so fascinated by what I do or she wants to make sure I do it right.\n\nWhy am I here? What is my motivation?\nNot much different from that guiding my cat:\nCuriosity and to be a witness (node?) of what is emerging here.\n\nMay the STEEM be with you!", - "json_metadata": "{\"tags\":[\"introduceyourself\",\"cats\"],\"image\":[\"https://grey.house/img/niunia.jpg\"]}", - "parent_author": "", - "parent_permlink": "introduceyourself", - "permlink": "hello-world", - "title": "Hello, World!" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T16:35:03", - "trx_id": "3f99bf13f5e78fcd4120075bea6933339516f188", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/comment_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/comment_operation.tavern.yaml deleted file mode 100644 index 5e7acbdb245768221cf90bd7f82919007e0a0059..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/comment_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",100,100,2] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "comment_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/convert_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/convert_operation.pat.json deleted file mode 100644 index 26793e5ee7057d925b9c74764ace6a9140256884..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/convert_operation.pat.json +++ /dev/null @@ -1,40 +0,0 @@ -[ - [ - 188, - { - "block": 2912870, - "op": [ - "convert", - { - "amount": "127.144 HBD", - "owner": "gtg", - "requestid": 1467663446 - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-04T20:18:00", - "trx_id": "8930ea36f61aef6fa7c5c79922cc9892f2d7373b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 189, - { - "block": 2912956, - "op": [ - "convert", - { - "amount": "0.002 HBD", - "owner": "gtg", - "requestid": 1467663726 - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-04T20:22:18", - "trx_id": "804339e4617544b47018e165d7a8ed45ac38f002", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/convert_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/convert_operation.tavern.yaml deleted file mode 100644 index d7a168e37e8f3eae2fe53a8e7eb09d707dd3b645..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/convert_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",1000,1000,256] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "convert_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/custom_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/custom_operation.pat.json deleted file mode 100644 index fe51488c7066f6687ef680d6bfaa4f7768ef205c..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/custom_operation.pat.json +++ /dev/null @@ -1 +0,0 @@ -[] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/custom_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/custom_operation.tavern.yaml deleted file mode 100644 index 9bfcd882a3a49fd6fddf4247c4f5799b266961a4..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/custom_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest # not found yet, something happened near 3334000 block - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",100,100,32768] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "custom_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/delete_comment_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/delete_comment_operation.pat.json deleted file mode 100644 index c30f171f05b786bf2aa1dbd627adf5e43dcc86a7..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/delete_comment_operation.pat.json +++ /dev/null @@ -1,128 +0,0 @@ -[ - [ - 21, - { - "block": 3166660, - "op": [ - "delete_comment", - { - "author": "murdock1", - "permlink": "test1" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-13T16:49:24", - "trx_id": "7a822d1775bcf9235e6d9b07ed16c973c34636c4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 23, - { - "block": 3166905, - "op": [ - "delete_comment", - { - "author": "murdock1", - "permlink": "test1" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-13T17:01:51", - "trx_id": "d60c1846f0ffd2ee86b86a346c55b7a90fc3197e", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 25, - { - "block": 3167138, - "op": [ - "delete_comment", - { - "author": "murdock1", - "permlink": "test1" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-13T17:13:33", - "trx_id": "e95c4eb02ee1bf63e9280cf1b1a60ccff6679a0d", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 27, - { - "block": 3168032, - "op": [ - "delete_comment", - { - "author": "murdock1", - "permlink": "test1" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-13T17:58:27", - "trx_id": "9aaef150d460677696d46c777badb841af665b2a", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 29, - { - "block": 3168184, - "op": [ - "delete_comment", - { - "author": "murdock1", - "permlink": "test1" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-13T18:06:09", - "trx_id": "0545d66789c78d2a12757bf41dde2798e8a73511", - "trx_in_block": 5, - "virtual_op": 0 - } - ], - [ - 31, - { - "block": 3168448, - "op": [ - "delete_comment", - { - "author": "murdock1", - "permlink": "test1" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-13T18:19:21", - "trx_id": "6a142529d8528f65914853ec30426734a2334398", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 33, - { - "block": 3168585, - "op": [ - "delete_comment", - { - "author": "murdock1", - "permlink": "test1" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-13T18:26:18", - "trx_id": "eae5bfe6ac82f196dc754e6555b678c5ebe32733", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/delete_comment_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/delete_comment_operation.tavern.yaml deleted file mode 100644 index 2be0519c02c6b97b018a63cd00c5f407f422016a..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/delete_comment_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["murdock1",100,100,131072] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "delete_comment_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/feed_publish_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/feed_publish_operation.pat.json deleted file mode 100644 index 6dada4f86c57218141ef848a221af0d02d69d15f..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/feed_publish_operation.pat.json +++ /dev/null @@ -1,65 +0,0 @@ -[ - [ - 472, - { - "block": 3816947, - "op": [ - "feed_publish", - { - "exchange_rate": { - "base": "2.095 HBD", - "quote": "1.000 HIVE" - }, - "publisher": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-05T12:41:30", - "trx_id": "6f276107cc2cae64ba9d0f961262c3f93f1c50c9", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 816, - { - "block": 3956341, - "op": [ - "feed_publish", - { - "exchange_rate": { - "base": "1.868 HBD", - "quote": "1.000 HIVE" - }, - "publisher": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-10T09:26:06", - "trx_id": "ef1c7e808ba7a0d70f95e335c6727375a5706ad4", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 968, - { - "block": 4018570, - "op": [ - "feed_publish", - { - "exchange_rate": { - "base": "1.569 HBD", - "quote": "1.000 HIVE" - }, - "publisher": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-12T13:30:00", - "trx_id": "d32d7b741e2bc311f79a4ae84da3c77433b982a0", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/feed_publish_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/feed_publish_operation.tavern.yaml deleted file mode 100644 index 8908582f62bac94fb8ba1787625fe653613a16a5..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/feed_publish_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",1000,1000,128] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "feed_publish_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/limit_order_cancel_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/limit_order_cancel_operation.pat.json deleted file mode 100644 index e9c34d359d459d30c0d9f800f2ce3549571c46d0..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/limit_order_cancel_operation.pat.json +++ /dev/null @@ -1,38 +0,0 @@ -[ - [ - 698, - { - "block": 3878021, - "op": [ - "limit_order_cancel", - { - "orderid": 1470507044, - "owner": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-07T15:48:00", - "trx_id": "ec9bc6087e2a0147d4065f13f9a4c76fc1bde230", - "trx_in_block": 2, - "virtual_op": 0 - } - ], - [ - 811, - { - "block": 3955064, - "op": [ - "limit_order_cancel", - { - "orderid": 1470646169, - "owner": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-10T08:22:09", - "trx_id": "002eb462739f65940d0ec349aa3ef0fb9f02940c", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/limit_order_cancel_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/limit_order_cancel_operation.tavern.yaml deleted file mode 100644 index a764094ca69d20684c21d4d943b45fc7f7244d50..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/limit_order_cancel_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",1000,1000,64] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "limit_order_cancel_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/limit_order_create_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/limit_order_create_operation.pat.json deleted file mode 100644 index d535529c695b2e4de23c6b4045dae8b0b0461010..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/limit_order_create_operation.pat.json +++ /dev/null @@ -1,112 +0,0 @@ -[ - [ - 605, - { - "block": 2570161, - "op": [ - "limit_order_create", - { - "amount_to_sell": "1.000 HIVE", - "expiration": "2035-10-29T06:32:22", - "fill_or_kill": false, - "min_to_receive": "1.000 HBD", - "orderid": 1, - "owner": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-22T21:18:33", - "trx_id": "3800d567f89bcd11de9fcc3f8db3a0e4b6891940", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 606, - { - "block": 2570178, - "op": [ - "limit_order_create", - { - "amount_to_sell": "1.000 HIVE", - "expiration": "2035-10-29T06:32:22", - "fill_or_kill": false, - "min_to_receive": "2.000 HBD", - "orderid": 2, - "owner": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-22T21:19:24", - "trx_id": "e9ad02c50d1dbb99a152b08e8a085524a5cbb995", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 974, - { - "block": 4429287, - "op": [ - "limit_order_create", - { - "amount_to_sell": "10000.000 HIVE", - "expiration": "2035-10-29T06:32:22", - "fill_or_kill": false, - "min_to_receive": "10200.000 HBD", - "orderid": 5, - "owner": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-26T22:25:30", - "trx_id": "784cecf495f3ee5e38d7dd5addc401ce78026e69", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 980, - { - "block": 4429407, - "op": [ - "limit_order_create", - { - "amount_to_sell": "10000.000 HIVE", - "expiration": "2035-10-29T06:32:22", - "fill_or_kill": false, - "min_to_receive": "9500.000 HBD", - "orderid": 6, - "owner": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-26T22:31:48", - "trx_id": "e3ce02b14cfb7e4de27ef8232f5b4ef3c3783d93", - "trx_in_block": 4, - "virtual_op": 0 - } - ], - [ - 983, - { - "block": 4429419, - "op": [ - "limit_order_create", - { - "amount_to_sell": "100000.000 HIVE", - "expiration": "2035-10-29T06:32:22", - "fill_or_kill": false, - "min_to_receive": "96000.000 HBD", - "orderid": 7, - "owner": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-26T22:32:27", - "trx_id": "b899b9d60a63f8d15d2c7293e48e63e9c4de7ba6", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/limit_order_create_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/limit_order_create_operation.tavern.yaml deleted file mode 100644 index 32552fb452f52996d2a30cd360650dad2496d810..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/limit_order_create_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["steemit",1000,1000,32] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "limit_order_create_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/pow_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/pow_operation.pat.json deleted file mode 100644 index c2aae2165e5c14a870bccb35bf72391422bfae04..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/pow_operation.pat.json +++ /dev/null @@ -1,36 +0,0 @@ -[ - [ - 0, - { - "block": 2794856, - "op": [ - "pow", - { - "block_id": "002aa567ccf390d2cc68306123b0d6fe9dba5cb8", - "nonce": "2194496385481149070", - "props": { - "account_creation_fee": { - "amount": "1", - "nai": "@@000000021", - "precision": 3 - }, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "work": { - "input": "851c610d1495b7019601e2188f1597f4b1804e7b29d0edd5fcbb078da714789d", - "signature": "203e847f9bc84c89cf89c1ac0afb9f5b405a408a46268f16f17bad2c001a0746e22715822421918be92d99ab1b0a9dd249ae3185ffe78bfb7c970cac7d58a7f237", - "work": "00000016a6ea16fb3a956ce4743157b15c49616fdef2a84f6c22224bf05348b1", - "worker": "STM5F9tCbND6zWPwksy1rEN24WjPiQWSU2vwGgegQVjAcYDe1zTWi" - }, - "worker_account": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-30T17:22:18", - "trx_id": "486b5caec9fd094ec21144c67ecef12aa15c8f4f", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/pow_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/pow_operation.tavern.yaml deleted file mode 100644 index ddfda5ac07ea137b139c83efd1a5767891686c0a..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/pow_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",10,10,16384] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "pow_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/report_over_production_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/report_over_production_operation.pat.json deleted file mode 100644 index fe51488c7066f6687ef680d6bfaa4f7768ef205c..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/report_over_production_operation.pat.json +++ /dev/null @@ -1 +0,0 @@ -[] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/report_over_production_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/report_over_production_operation.tavern.yaml deleted file mode 100644 index 1e9ba0ee4e239763eca18ae38ac72d9b68358959..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/report_over_production_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest # not found under 5 mil - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",100,100,32768] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "report_over_production_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/transfer_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/transfer_operation.pat.json deleted file mode 100644 index 3ec558ae896f3c7b64ac2774c745699358702ea2..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/transfer_operation.pat.json +++ /dev/null @@ -1,22 +0,0 @@ -[ - [ - 877, - { - "block": 3985208, - "op": [ - "transfer", - { - "amount": "0.001 HBD", - "from": "vdoh", - "memo": "I believe in Steemit, I believe in You! Good afternoon, I want to invite you to support my post dedicated to my dream and development and good advertizing of community of Steemit! I want to make the balloon on which I am going to visit many large cities and to place the logo and the slogan Steemit on it. Support me and I will support you! URL : https://steemit.com/steem/@vdoh/rise-steemit", - "to": "gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-11T09:32:33", - "trx_id": "6ae67278f73b29b19b7d2eb52f1144752f4441ce", - "trx_in_block": 1, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/transfer_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/transfer_operation.tavern.yaml deleted file mode 100644 index 47c88331c790331de7021f4d3eaec83f0c75bd9e..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/transfer_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",1000,1000,4] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "transfer_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/transfer_to_vesting_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/transfer_to_vesting_operation.pat.json deleted file mode 100644 index 781c685637c1a8f1373a3cfcc5c89f4eb4f85ec5..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/transfer_to_vesting_operation.pat.json +++ /dev/null @@ -1,591 +0,0 @@ -[ - [ - 19, - { - "block": 29833, - "op": [ - "transfer_to_vesting", - { - "amount": "2211.000 HIVE", - "from": "steemit", - "to": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-25T17:28:12", - "trx_id": "7212d7054d2ce95b8c1736390e0c2512bf6cd06a", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 23, - { - "block": 35161, - "op": [ - "transfer_to_vesting", - { - "amount": "100.000 HIVE", - "from": "steemit70", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-25T21:56:03", - "trx_id": "f550ad436b78848a7bd29529efdcbfb350020f9b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 44, - { - "block": 81530, - "op": [ - "transfer_to_vesting", - { - "amount": "1389.000 HIVE", - "from": "steemit", - "to": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-27T12:40:21", - "trx_id": "8f2c02c8eed3409ef0f8e63ce7cac3dd5205378b", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 73, - { - "block": 140313, - "op": [ - "transfer_to_vesting", - { - "amount": "2356.000 HIVE", - "from": "admin", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:53:09", - "trx_id": "ea600f25217ef27cbcb947c4ee2c4b93ec6109ea", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 74, - { - "block": 140322, - "op": [ - "transfer_to_vesting", - { - "amount": "20.000 HIVE", - "from": "steemit78", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:53:36", - "trx_id": "97ef47be42b6c2d2ea51633b25f2e94a1961efac", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 75, - { - "block": 140330, - "op": [ - "transfer_to_vesting", - { - "amount": "41.000 HIVE", - "from": "steemit77", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:54:00", - "trx_id": "d9cd1077e996e7c509af6b8eb6c037baab8d1f28", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 76, - { - "block": 140331, - "op": [ - "transfer_to_vesting", - { - "amount": "41.000 HIVE", - "from": "steemit76", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:54:03", - "trx_id": "6893a5853be7f6f8fdb367ec6485dcc513e15115", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 77, - { - "block": 140351, - "op": [ - "transfer_to_vesting", - { - "amount": "20.000 HIVE", - "from": "steemit75", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:55:03", - "trx_id": "00f69b28125c9b92217d144b9a575a655c13a733", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 78, - { - "block": 140357, - "op": [ - "transfer_to_vesting", - { - "amount": "83.000 HIVE", - "from": "steemit73", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:55:21", - "trx_id": "95d559e25069927c44048ce77d6fdb685ad9e4d8", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 79, - { - "block": 140362, - "op": [ - "transfer_to_vesting", - { - "amount": "168.000 HIVE", - "from": "steemit72", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:55:36", - "trx_id": "5bb71b29f107e590d4580c0ec3300f61fc7435cf", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 80, - { - "block": 140365, - "op": [ - "transfer_to_vesting", - { - "amount": "127.000 HIVE", - "from": "steemit71", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:55:45", - "trx_id": "a83f618dcca0f21c359f2505b899f9cd98f5557c", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 81, - { - "block": 140375, - "op": [ - "transfer_to_vesting", - { - "amount": "90.000 HIVE", - "from": "steemit70", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:56:15", - "trx_id": "f22569f040260ec50b76f21cb8b8baad3faf37f0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 82, - { - "block": 140379, - "op": [ - "transfer_to_vesting", - { - "amount": "106.000 HIVE", - "from": "steemit7", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:56:27", - "trx_id": "33f20cad05e0952f58dea32a0aaa8060c44e8e1d", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 83, - { - "block": 140383, - "op": [ - "transfer_to_vesting", - { - "amount": "275.000 HIVE", - "from": "steemit69", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:56:39", - "trx_id": "31a4b21f848caf0fdbe8ef564778a70f2a9c37fd", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 84, - { - "block": 140390, - "op": [ - "transfer_to_vesting", - { - "amount": "252.000 HIVE", - "from": "steemit68", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:57:00", - "trx_id": "9568d2aabfa0ef938ea5ea4d7e421db2397bf209", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 85, - { - "block": 140399, - "op": [ - "transfer_to_vesting", - { - "amount": "293.000 HIVE", - "from": "steemit67", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:57:27", - "trx_id": "1adcc05b57ccd225ada6251379bf649b95574486", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 86, - { - "block": 140415, - "op": [ - "transfer_to_vesting", - { - "amount": "379.000 HIVE", - "from": "steemit66", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:58:15", - "trx_id": "508316a61ed911cd3302b6ec185ea9a6c93439f2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 87, - { - "block": 140418, - "op": [ - "transfer_to_vesting", - { - "amount": "272.000 HIVE", - "from": "steemit65", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:58:24", - "trx_id": "2849991dae934b73fe0725dea9d2db49d8f0f380", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 88, - { - "block": 140424, - "op": [ - "transfer_to_vesting", - { - "amount": "337.000 HIVE", - "from": "steemit63", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:58:42", - "trx_id": "9194f5594a4380962520f34fa8a98c7a33a74803", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 89, - { - "block": 140428, - "op": [ - "transfer_to_vesting", - { - "amount": "336.000 HIVE", - "from": "steemit62", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:58:54", - "trx_id": "e9336f7a9a51813578e2213d20a2f1adbca63d46", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 90, - { - "block": 140433, - "op": [ - "transfer_to_vesting", - { - "amount": "295.000 HIVE", - "from": "steemit61", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:59:09", - "trx_id": "9733aeb610d3b3f418b90724b4d8f9e5a2cfa64f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 91, - { - "block": 140444, - "op": [ - "transfer_to_vesting", - { - "amount": "2458.000 HIVE", - "from": "steemit", - "to": "" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T13:59:42", - "trx_id": "f4d859c8366ef92adf668b8efc1588d225e2d921", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 92, - { - "block": 140454, - "op": [ - "transfer_to_vesting", - { - "amount": "83.000 HIVE", - "from": "sminer90", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T14:00:12", - "trx_id": "44a5aaed15c326959a6346a40df5b4eaf50fd6ec", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 93, - { - "block": 140456, - "op": [ - "transfer_to_vesting", - { - "amount": "104.000 HIVE", - "from": "sminer9", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T14:00:18", - "trx_id": "50a9557decf4492e618f930ddbe51833b4a8b1db", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 94, - { - "block": 140475, - "op": [ - "transfer_to_vesting", - { - "amount": "357.000 HIVE", - "from": "steemit60", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T14:01:15", - "trx_id": "df923b0e3811a335dbb9fcf8e9360df835ed8e0f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 95, - { - "block": 140479, - "op": [ - "transfer_to_vesting", - { - "amount": "337.000 HIVE", - "from": "steemit6", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T14:01:27", - "trx_id": "2ecb15948efe79175484a165481e4ec184c02391", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 96, - { - "block": 140494, - "op": [ - "transfer_to_vesting", - { - "amount": "421.000 HIVE", - "from": "steemit59", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T14:02:12", - "trx_id": "7f6de53b7200fad93ac8d6ce863ee35ecb6923be", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 97, - { - "block": 140497, - "op": [ - "transfer_to_vesting", - { - "amount": "383.000 HIVE", - "from": "steemit58", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T14:02:21", - "trx_id": "25247615e399b6e8e5ed409452d865313339c2d2", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 98, - { - "block": 140507, - "op": [ - "transfer_to_vesting", - { - "amount": "380.000 HIVE", - "from": "steemit57", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T14:02:51", - "trx_id": "76fbe1da27c21a4c0934e3561a522b7df4964920", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 99, - { - "block": 140510, - "op": [ - "transfer_to_vesting", - { - "amount": "551.000 HIVE", - "from": "steemit56", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T14:03:00", - "trx_id": "e86c4df28c777cd1a1ba9b8da9c9b4ee974f71ec", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 100, - { - "block": 140516, - "op": [ - "transfer_to_vesting", - { - "amount": "510.000 HIVE", - "from": "steemit55", - "to": "steemit" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-29T14:03:18", - "trx_id": "6f1f35eeccc8c0b13608ceba88aa244a3630a672", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/transfer_to_vesting_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/transfer_to_vesting_operation.tavern.yaml deleted file mode 100644 index 66539744464cad752c7fc2af3cb39b5be1a62048..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/transfer_to_vesting_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["steemit",100,100,8] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "transfer_to_vesting_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/vote_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/vote_operation.pat.json deleted file mode 100644 index 50b0baf99e222b0ead163ef0fc9e98aa5dafaf17..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/vote_operation.pat.json +++ /dev/null @@ -1,82 +0,0 @@ -[ - [ - 3, - { - "block": 2797050, - "op": [ - "vote", - { - "author": "pfunk", - "permlink": "guide-maximize-your-mining-hashrate-in-windows-by-mining-steem-in-a-vm", - "voter": "gtg", - "weight": 10000 - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-30T19:12:21", - "trx_id": "e0cd24f9aba3b668b1443786bee3891f644ff23f", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 5, - { - "block": 2797188, - "op": [ - "vote", - { - "author": "pfunk", - "permlink": "security-b-sides-msp-2016-information-security-conference-day-1-opening-keynote-notes-and-recap", - "voter": "gtg", - "weight": 10000 - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-30T19:19:15", - "trx_id": "be93d0b153a5b9a12547180a1d88f2aad1669754", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 7, - { - "block": 2797362, - "op": [ - "vote", - { - "author": "pfunk", - "permlink": "lets-discuss-verification-of-user-accounts-posting-previous-work-to-prevent-impersonation", - "voter": "gtg", - "weight": 10000 - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-30T19:27:57", - "trx_id": "c7a8b59428f96adc3245345bd4fd7c587aad3383", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 9, - { - "block": 2797645, - "op": [ - "vote", - { - "author": "zajac", - "permlink": "hi-steemit-people-lets-waste-some-time-together-", - "voter": "gtg", - "weight": 10000 - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-30T19:42:09", - "trx_id": "f98b305720148c4b37b19c1c7616ad9057e46c32", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/vote_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/vote_operation.tavern.yaml deleted file mode 100644 index 6eeef5a915e7d4548f8abd297a4ba15bb7bee4cb..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/vote_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",10,10,1] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "vote_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/withdraw_vesting_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/withdraw_vesting_operation.pat.json deleted file mode 100644 index 29f48779e2abf5382613a22ad10fc4eb3f9998bc..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/withdraw_vesting_operation.pat.json +++ /dev/null @@ -1,92 +0,0 @@ -[ - [ - 302, - { - "block": 202807, - "op": [ - "withdraw_vesting", - { - "account": "steemit", - "vesting_shares": "200000.000000 VESTS" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-31T18:11:48", - "trx_id": "e50f0a0b877ed47af052db3d78a8ea0114a95695", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 303, - { - "block": 203612, - "op": [ - "withdraw_vesting", - { - "account": "steemit", - "vesting_shares": "260000.000000 VESTS" - } - ], - "op_in_trx": 0, - "timestamp": "2016-03-31T18:52:03", - "trx_id": "243a70091327caa2bd017302181759d2737f4b51", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 387, - { - "block": 377804, - "op": [ - "withdraw_vesting", - { - "account": "steemit", - "vesting_shares": "100000.000000 VESTS" - } - ], - "op_in_trx": 0, - "timestamp": "2016-04-06T22:47:42", - "trx_id": "6def62ca023aba69a406c6bd2bafa9ddaa2f7d85", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 566, - { - "block": 1302668, - "op": [ - "withdraw_vesting", - { - "account": "steemit", - "vesting_shares": "0.000000 VESTS" - } - ], - "op_in_trx": 0, - "timestamp": "2016-05-09T17:17:09", - "trx_id": "0af4b5e8b8eec3b93894f91feec5cd807f324278", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 596, - { - "block": 2287366, - "op": [ - "withdraw_vesting", - { - "account": "steemit", - "vesting_shares": "257910734535.923078 VESTS" - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-13T00:37:21", - "trx_id": "41162d8c246d19ecb21600ddcf23d1fe8b75b7fb", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/withdraw_vesting_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/withdraw_vesting_operation.tavern.yaml deleted file mode 100644 index dac15aa7df229e53bca85cd84d263e7abed2e053..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/withdraw_vesting_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["steemit",1000,1000,16] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "withdraw_vesting_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_history/witness_update_operation.pat.json b/hived/tavern/condenser_api_patterns/get_account_history/witness_update_operation.pat.json deleted file mode 100644 index 34c8897a7c727709510a6466641da0b2c9955153..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/witness_update_operation.pat.json +++ /dev/null @@ -1,137 +0,0 @@ -[ - [ - 114, - { - "block": 2881920, - "op": [ - "witness_update", - { - "block_signing_key": "STM5F9tCbND6zWPwksy1rEN24WjPiQWSU2vwGgegQVjAcYDe1zTWi", - "fee": "0.000 HIVE", - "owner": "gtg", - "props": { - "account_creation_fee": "9.999 HIVE", - "account_subsidy_budget": 797, - "account_subsidy_decay": 347321, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "url": "https://steemit.com/introduceyourself/@gtg/hello-world" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-03T18:04:21", - "trx_id": "d1f352e5d1834cbf166166c4810b752d021f0fc0", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 324, - { - "block": 3134487, - "op": [ - "witness_update", - { - "block_signing_key": "STM776t8h7dXbvM8BYGoLjCr3nYRnmqmvVg9hTrGTn5FQvLkMZKM2", - "fee": "0.000 HIVE", - "owner": "gtg", - "props": { - "account_creation_fee": "9.999 HIVE", - "account_subsidy_budget": 797, - "account_subsidy_decay": 347321, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "url": "https://steemit.com/introduceyourself/@gtg/hello-world" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-12T13:52:36", - "trx_id": "4cee4cbe4e1637e6745bde8ad8eb5c2559ae818b", - "trx_in_block": 6, - "virtual_op": 0 - } - ], - [ - 332, - { - "block": 3153076, - "op": [ - "witness_update", - { - "block_signing_key": "STM776t8h7dXbvM8BYGoLjCr3nYRnmqmvVg9hTrGTn5FQvLkMZKM2", - "fee": "0.000 HIVE", - "owner": "gtg", - "props": { - "account_creation_fee": "5.000 HIVE", - "account_subsidy_budget": 797, - "account_subsidy_decay": 347321, - "hbd_interest_rate": 1000, - "maximum_block_size": 131072 - }, - "url": "https://steemit.com/introduceyourself/@gtg/hello-world" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-13T05:25:36", - "trx_id": "5dda11f0e7df6c556e7d4d6efdf32c3129fce98b", - "trx_in_block": 1, - "virtual_op": 0 - } - ], - [ - 422, - { - "block": 3682342, - "op": [ - "witness_update", - { - "block_signing_key": "STM776t8h7dXbvM8BYGoLjCr3nYRnmqmvVg9hTrGTn5FQvLkMZKM2", - "fee": "0.000 HIVE", - "owner": "gtg", - "props": { - "account_creation_fee": "3.000 HIVE", - "account_subsidy_budget": 797, - "account_subsidy_decay": 347321, - "hbd_interest_rate": 1000, - "maximum_block_size": 65536 - }, - "url": "https://steemit.com/introduceyourself/@gtg/hello-world" - } - ], - "op_in_trx": 0, - "timestamp": "2016-07-31T19:53:18", - "trx_id": "6d409ff427aac442de4475b844cd7033ef79a9ca", - "trx_in_block": 0, - "virtual_op": 0 - } - ], - [ - 918, - { - "block": 3998630, - "op": [ - "witness_update", - { - "block_signing_key": "STM776t8h7dXbvM8BYGoLjCr3nYRnmqmvVg9hTrGTn5FQvLkMZKM2", - "fee": "0.000 HIVE", - "owner": "gtg", - "props": { - "account_creation_fee": "3.000 HIVE", - "account_subsidy_budget": 797, - "account_subsidy_decay": 347321, - "hbd_interest_rate": 1000, - "maximum_block_size": 65536 - }, - "url": "https://steemit.com/witness-category/@gtg/witness-gtg" - } - ], - "op_in_trx": 0, - "timestamp": "2016-08-11T20:50:36", - "trx_id": "90b1c097689f6a556b13f1223f7e4c3a979a402c", - "trx_in_block": 0, - "virtual_op": 0 - } - ] -] diff --git a/hived/tavern/condenser_api_patterns/get_account_history/witness_update_operation.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_history/witness_update_operation.tavern.yaml deleted file mode 100644 index 2161f7e97a9b3a65d6011614b5320462b71a544e..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_history/witness_update_operation.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_transaction - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_transaction - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_history" - params: ["gtg",1000,1000,2048] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "witness_update_operation" - directory: "condenser_api_patterns/get_account_history" diff --git a/hived/tavern/condenser_api_patterns/get_account_references/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_references/get.tavern.yaml deleted file mode 100644 index 1915dfed6d6f35eb25aaaecdafcb1ea372eb3a35..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_references/get.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_account_references - - marks: - - patterntest - - xfail # false: condenser_api::get_account_references --- Needs to be refactored for Hive. - - includes: - - !include ../../common.yaml - - stages: - - name: get_account_references - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_references" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_account_references" diff --git a/hived/tavern/condenser_api_patterns/get_account_reputations/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_account_reputations/get.tavern.yaml deleted file mode 100644 index df3bac03f4a4f44b449bcacaeacedf2114cc23cd..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_account_reputations/get.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_account_reputations - - marks: - - patterntest - - xfail # get_account_references --- Needs to be refactored for Hive - - includes: - - !include ../../common.yaml - - stages: - - name: get_account_reputations - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_account_references" - params: ["account_lower_bound": "", "limit": 1000] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_account_reputations" diff --git a/hived/tavern/condenser_api_patterns/get_accounts/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_accounts/get.tavern.yaml deleted file mode 100644 index 8776f8b034961421e4f010140f312debaa688dcd..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_accounts/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_accounts - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_accounts - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_accounts" - params: [["gtg"]] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_accounts" diff --git a/hived/tavern/condenser_api_patterns/get_accounts/multiple.tavern.yaml b/hived/tavern/condenser_api_patterns/get_accounts/multiple.tavern.yaml deleted file mode 100644 index 707a51d4ccc260883c6b97301bfa996efdac8feb..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_accounts/multiple.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_accounts - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_accounts - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_accounts" - params: [["gtg", "steemit","alice","temp","dan","not.existing.account"]] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "multiple" - directory: "condenser_api_patterns/get_accounts" diff --git a/hived/tavern/condenser_api_patterns/get_active_votes/gtg.tavern.yaml b/hived/tavern/condenser_api_patterns/get_active_votes/gtg.tavern.yaml deleted file mode 100644 index d8edaea87127118245357d9b6a154789fe7c6895..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_active_votes/gtg.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_active_votes - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_active_votes - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_active_votes" - params: ["gtg","heavy-duty-witness-node-infrastructure"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "gtg" - directory: "condenser_api_patterns/get_active_votes" diff --git a/hived/tavern/condenser_api_patterns/get_active_votes/steemit.tavern.yaml b/hived/tavern/condenser_api_patterns/get_active_votes/steemit.tavern.yaml deleted file mode 100644 index b89d13c71032803ebe25d3f262fb4e195866db8f..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_active_votes/steemit.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_active_votes - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_active_votes - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_active_votes" - params: ["steemit","firstpost"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "steemit" - directory: "condenser_api_patterns/get_active_votes" diff --git a/hived/tavern/condenser_api_patterns/get_active_witnesses/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_active_witnesses/get.tavern.yaml deleted file mode 100644 index 209da9df8d0364edb41b4f2e6dcf1ea6abcbcb8a..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_active_witnesses/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_active_witnesses - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_active_witnesses - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_active_witnesses" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_active_witnesses" diff --git a/hived/tavern/condenser_api_patterns/get_block/milion.pat.json b/hived/tavern/condenser_api_patterns/get_block/milion.pat.json deleted file mode 100644 index 78df3bcc10ccb588c9e4a79ae47c3fec20182d5a..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_block/milion.pat.json +++ /dev/null @@ -1,12 +0,0 @@ -{ - "block_id": "000f4240e8f91385f7bff8f5aeebddc9b14e4281", - "extensions": [], - "previous": "000f423f974857674873d93d1909e0eeb7e4916e", - "signing_key": "STM67P2LhV4FCvk2y6sQjNTnp6b1MVTKnftw2mLE2Vxf89Vdn7xYG", - "timestamp": "2016-04-29T04:12:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "abit", - "witness_signature": "1f72bd3f4b06e7dc6b156729f0fd7873163814972eecea9d77cb29bae11d0fea3865c814d11a58e818c2494ce19f4c3d4c3e17eab3b1465ebccb102c52c56472c0" -} diff --git a/hived/tavern/condenser_api_patterns/get_block/milion.tavern.yaml b/hived/tavern/condenser_api_patterns/get_block/milion.tavern.yaml deleted file mode 100644 index d1f4a5a60ec60343b9569994188615f3408cd10c..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_block/milion.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived condenser_api.get_block milion - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block milion - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_block" - params: [1000000] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "milion" - directory: "condenser_api_patterns/get_block" diff --git a/hived/tavern/condenser_api_patterns/get_block/multiple.tavern.yaml b/hived/tavern/condenser_api_patterns/get_block/multiple.tavern.yaml deleted file mode 100644 index 0b54bb551e4085883be0652013eb4df83ff93051..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_block/multiple.tavern.yaml +++ /dev/null @@ -1,68 +0,0 @@ ---- - test_name: Hivemind condenser_api.get_block multiple tests - - marks: - - patterntest - - parametrize: - key: block_id - vals: - - 10 - - 100 - - 1000 - - 10000 - - 200000 - - 5000000 - - 4788663 - - 5119170 - - 5211007 - - 43176948 - - 43177127 - - 43177128 - - 43177933 - - 43177934 - - 43182320 - - 43184379 - - 43186963 - - 44238919 - - 44647676 - - 44976482 - - 45144817 - - 48207066 - - 48332524 - - 48454760 - - 48456274 - - 48456450 - - 48538351 - - 48538352 - - 48538362 - - 48538363 - - 48538364 - - 48539058 - - 48539128 - - 48539125 - - 48539381 - - 48539731 - - includes: - - !include ../../common.yaml - - stages: - - name: get_block multiple tests - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_block" - params: ["{block_id}"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "4788663" - directory: "condenser_api_patterns/get_block" - diff --git a/hived/tavern/condenser_api_patterns/get_block/one.pat.json b/hived/tavern/condenser_api_patterns/get_block/one.pat.json deleted file mode 100644 index 8d0510b5534e7a520e0c86ca107231c37b3a5b7a..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_block/one.pat.json +++ /dev/null @@ -1,12 +0,0 @@ -{ - "block_id": "0000000109833ce528d5bbfb3f6225b39ee10086", - "extensions": [], - "previous": "0000000000000000000000000000000000000000", - "signing_key": "STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX", - "timestamp": "2016-03-24T16:05:00", - "transaction_ids": [], - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "transactions": [], - "witness": "initminer", - "witness_signature": "204f8ad56a8f5cf722a02b035a61b500aa59b9519b2c33c77a80c0a714680a5a5a7a340d909d19996613c5e4ae92146b9add8a7a663eef37d837ef881477313043" -} diff --git a/hived/tavern/condenser_api_patterns/get_block/one.tavern.yaml b/hived/tavern/condenser_api_patterns/get_block/one.tavern.yaml deleted file mode 100644 index e96ea8a218b4fe629bd5e64aba8a9cad59d62a42..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_block/one.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived condenser_api.get_block - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: condenser_api.get_block - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_block" - params: [1] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "one" - directory: "condenser_api_patterns/get_block" diff --git a/hived/tavern/condenser_api_patterns/get_block_header/milion.pat.json b/hived/tavern/condenser_api_patterns/get_block_header/milion.pat.json deleted file mode 100644 index 6f9b1df3978acaef4c757c640626bfcb74af6cd1..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_block_header/milion.pat.json +++ /dev/null @@ -1,7 +0,0 @@ -{ - "extensions": [], - "previous": "000f423f974857674873d93d1909e0eeb7e4916e", - "timestamp": "2016-04-29T04:12:00", - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "witness": "abit" -} diff --git a/hived/tavern/condenser_api_patterns/get_block_header/milion.tavern.yaml b/hived/tavern/condenser_api_patterns/get_block_header/milion.tavern.yaml deleted file mode 100644 index 2dc83999b6d569e8ed16b7519f8a6f4091159eca..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_block_header/milion.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived condenser_api.get_block_header milion - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: block_api.get_block milion - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_block_header" - params: [1000000] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "milion" - directory: "condenser_api_patterns/get_block_header" diff --git a/hived/tavern/condenser_api_patterns/get_block_header/one.pat.json b/hived/tavern/condenser_api_patterns/get_block_header/one.pat.json deleted file mode 100644 index 8184f9d5cf6623414002e0e02e5238be7c8ea852..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_block_header/one.pat.json +++ /dev/null @@ -1,7 +0,0 @@ -{ - "extensions": [], - "previous": "0000000000000000000000000000000000000000", - "timestamp": "2016-03-24T16:05:00", - "transaction_merkle_root": "0000000000000000000000000000000000000000", - "witness": "initminer" -} diff --git a/hived/tavern/condenser_api_patterns/get_block_header/one.tavern.yaml b/hived/tavern/condenser_api_patterns/get_block_header/one.tavern.yaml deleted file mode 100644 index 2b0776a773f8ee7f1c78413d9e0364fe145a8e14..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_block_header/one.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived condenser_api.get_block_header - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: condenser_api.get_block_header - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_block_header" - params: [1] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "one" - directory: "condenser_api_patterns/get_block_header" diff --git a/hived/tavern/condenser_api_patterns/get_blog/gtg.pat.json b/hived/tavern/condenser_api_patterns/get_blog/gtg.pat.json deleted file mode 100644 index d9d8de9b648afa596beff81cec071a2e2d9a8995..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_blog/gtg.pat.json +++ /dev/null @@ -1,8265 +0,0 @@ -[ - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 267328592887, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Interesting. I never get bored.\n> \"_I am also the most boring person you will ever meet._\"\n\nThat's tempting. Can you prove it at SteemFest? :-)", - "body_length": 143, - "cashout_time": "1969-12-31T23:59:59", - "category": "boredom", - "children": 1, - "created": "2016-10-12T08:10:45", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"boredom\"]}", - "last_payout": "2016-11-12T01:29:51", - "last_update": "2016-10-12T08:10:45", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 267328592887, - "parent_author": "thebluepanda", - "parent_permlink": "hail-to-boredom", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-thebluepanda-hail-to-boredom-20161012t081037009z", - "post_id": 1512244, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hail To Boredom", - "title": "", - "total_payout_value": "0.023 HBD", - "url": "/boredom/@thebluepanda/hail-to-boredom#@gtg/re-thebluepanda-hail-to-boredom-20161012t081037009z" - }, - "entry_id": 100, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 318269030693, - "rshares": 0, - "voter": "impactmind" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Lee, welcome to Steemit! :-)", - "body_length": 34, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 1, - "created": "2016-10-12T08:02:18", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"]}", - "last_payout": "2016-11-12T05:54:06", - "last_update": "2016-10-12T08:02:18", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "impactmind", - "parent_permlink": "to-my-fellow-steemit-ers-hello-here-s-my-intro-plus", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-impactmind-to-my-fellow-steemit-ers-hello-here-s-my-intro-plus-20161012t080209911z", - "post_id": 1512222, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "To my fellow steemit...ers? Hello! Here's my intro plus.", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@impactmind/to-my-fellow-steemit-ers-hello-here-s-my-intro-plus#@gtg/re-impactmind-to-my-fellow-steemit-ers-hello-here-s-my-intro-plus-20161012t080209911z" - }, - "entry_id": 99, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Dennis, welcome to Steemit! :-)", - "body_length": 37, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-10-12T06:11:00", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-11-11T23:20:21", - "last_update": "2016-10-12T06:11:00", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "dburklen", - "parent_permlink": "my-introduction", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-dburklen-my-introduction-20161012t061050025z", - "post_id": 1511622, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "My introduction", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@dburklen/my-introduction#@gtg/re-dburklen-my-introduction-20161012t061050025z" - }, - "entry_id": 98, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @shima64, welcome to Steemit! :-)\nProgressive rock and Japan. That makes me think about DP :-)", - "body_length": 101, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 1, - "created": "2016-10-11T21:17:48", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"],\"users\":[\"shima64\"]}", - "last_payout": "2016-11-11T18:04:33", - "last_update": "2016-10-11T21:17:48", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "shima64", - "parent_permlink": "little-about-me-introducing-myself", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-shima64-little-about-me-introducing-myself-20161011t211738141z", - "post_id": 1508174, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Little about me , Introducing myself", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@shima64/little-about-me-introducing-myself#@gtg/re-shima64-little-about-me-introducing-myself-20161011t211738141z" - }, - "entry_id": 97, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Natalya, welcome to Steemit! :-)", - "body_length": 38, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 0, - "created": "2016-10-11T21:13:12", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"]}", - "last_payout": "2016-11-11T19:44:48", - "last_update": "2016-10-11T21:13:12", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "natka2", - "parent_permlink": "let-s-introduce-ourselves-davaite-znakomitsya", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-natka2-let-s-introduce-ourselves-davaite-znakomitsya-20161011t211304815z", - "post_id": 1508143, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "LET'S INTRODUCE OURSELVES!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@natka2/let-s-introduce-ourselves-davaite-znakomitsya#@gtg/re-natka2-let-s-introduce-ourselves-davaite-znakomitsya-20161011t211304815z" - }, - "entry_id": 96, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": -958052066356, - "rshares": 132030407, - "voter": "realmeandi" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @realmeandi, welcome to Steemit! :-)", - "body_length": 42, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 1, - "created": "2016-10-11T21:11:27", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"],\"users\":[\"realmeandi\"]}", - "last_payout": "2016-11-12T02:22:39", - "last_update": "2016-10-11T21:11:27", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 132030407, - "parent_author": "realmeandi", - "parent_permlink": "first-time-here", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-realmeandi-first-time-here-20161011t211118115z", - "post_id": 1508127, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "First time here", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@realmeandi/first-time-here#@gtg/re-realmeandi-first-time-here-20161011t211118115z" - }, - "entry_id": 95, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 387642408233756, - "rshares": 1023984868, - "voter": "future24" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Good luck with SteemFest :-)", - "body_length": 28, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit", - "children": 1, - "created": "2016-10-11T21:09:21", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"steemit\"]}", - "last_payout": "2016-11-11T20:50:15", - "last_update": "2016-10-11T21:09:21", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 1023984868, - "parent_author": "celebr1ty", - "parent_permlink": "the-first-personalized-christmas-toy-to-ned-by-me-handmade", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-celebr1ty-the-first-personalized-christmas-toy-to-ned-by-me-handmade-20161011t210912253z", - "post_id": 1508108, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "The first personalized Christmas toy to @ned by me (handmade)", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/steemit/@celebr1ty/the-first-personalized-christmas-toy-to-ned-by-me-handmade#@gtg/re-celebr1ty-the-first-personalized-christmas-toy-to-ned-by-me-handmade-20161011t210912253z" - }, - "entry_id": 94, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 22316942961150, - "rshares": 3072431146, - "voter": "seisges" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Luis, welcome to Steemit! :-)", - "body_length": 35, - "cashout_time": "1969-12-31T23:59:59", - "category": "art", - "children": 1, - "created": "2016-10-11T20:55:27", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"art\"]}", - "last_payout": "2016-11-12T01:35:27", - "last_update": "2016-10-11T20:55:27", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 3072431146, - "parent_author": "tepuyart", - "parent_permlink": "steemit-llega-a-mi-en-el-momento-perfecto-no-es-casualidad-es-causalidad", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-tepuyart-steemit-llega-a-mi-en-el-momento-perfecto-no-es-casualidad-es-causalidad-20161011t205517014z", - "post_id": 1507971, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Steemit llega a m\u00ed en el momento perfecto, no es casualidad, es causalidad.", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/art/@tepuyart/steemit-llega-a-mi-en-el-momento-perfecto-no-es-casualidad-es-causalidad#@gtg/re-tepuyart-steemit-llega-a-mi-en-el-momento-perfecto-no-es-casualidad-es-causalidad-20161011t205517014z" - }, - "entry_id": 93, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Suzanah, welcome to Steemit, powodzenia! :-)", - "body_length": 50, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 1, - "created": "2016-10-11T09:52:27", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"]}", - "last_payout": "2016-11-10T03:18:39", - "last_update": "2016-10-11T09:52:27", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "knittybynature", - "parent_permlink": "small-town-girl-meets-the-world", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-knittybynature-small-town-girl-meets-the-world-20161011t095223415z", - "post_id": 1502977, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Small town girl meets the world", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@knittybynature/small-town-girl-meets-the-world#@gtg/re-knittybynature-small-town-girl-meets-the-world-20161011t095223415z" - }, - "entry_id": 92, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @avantmidi, welcome to Steemit! :-)\nAdding a picture to your post could make it more appealing.\n( I used my cat for that ;-) )", - "body_length": 132, - "cashout_time": "1969-12-31T23:59:59", - "category": "publishing", - "children": 1, - "created": "2016-10-11T09:35:30", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"publishing\"],\"users\":[\"avantmidi\"]}", - "last_payout": "2016-11-11T09:40:48", - "last_update": "2016-10-11T09:39:30", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "avantmidi", - "parent_permlink": "a-little-more-about-this-goal", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-avantmidi-a-little-more-about-this-goal-20161011t093527210z", - "post_id": 1502888, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "A little more about this Goal", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/publishing/@avantmidi/a-little-more-about-this-goal#@gtg/re-avantmidi-a-little-more-about-this-goal-20161011t093527210z" - }, - "entry_id": 91, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Kate, welcome to Steemit! :-)\nAdding a picture to your post could make it more appealing.\n( I used my cat for that ;-) )", - "body_length": 126, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-10-11T09:24:06", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-11-11T09:24:18", - "last_update": "2016-10-11T09:28:30", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "k8cookie", - "parent_permlink": "hello", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-k8cookie-hello-20161011t092404648z", - "post_id": 1502846, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hello!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@k8cookie/hello#@gtg/re-k8cookie-hello-20161011t092404648z" - }, - "entry_id": 90, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 15792464317401, - "rshares": 10753280407, - "voter": "burnin" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Leonel, welcome to Steemit! :-)", - "body_length": 37, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 0, - "created": "2016-10-11T08:02:09", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"]}", - "last_payout": "2016-11-10T19:46:15", - "last_update": "2016-10-11T08:02:09", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 10753280407, - "parent_author": "burnin", - "parent_permlink": "hi-i-m-burnin-nice-to-meet-you-see-you-at-steemfest", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-burnin-hi-i-m-burnin-nice-to-meet-you-see-you-at-steemfest-20161011t080207493z", - "post_id": 1502462, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hi! I'm @burnin, nice to meet you! See you at Steemfest.", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@burnin/hi-i-m-burnin-nice-to-meet-you-see-you-at-steemfest#@gtg/re-burnin-hi-i-m-burnin-nice-to-meet-you-see-you-at-steemfest-20161011t080207493z" - }, - "entry_id": 89, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Susanne, welcome to Steemit :-)", - "body_length": 37, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 1, - "created": "2016-10-11T07:55:42", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"]}", - "last_payout": "2016-11-10T17:54:36", - "last_update": "2016-10-11T07:55:42", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "avsusanne", - "parent_permlink": "heisann", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-avsusanne-heisann-20161011t075539216z", - "post_id": 1502435, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Heisann!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@avsusanne/heisann#@gtg/re-avsusanne-heisann-20161011t075539216z" - }, - "entry_id": 88, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Thank you for your service! :-)", - "body_length": 31, - "cashout_time": "1969-12-31T23:59:59", - "category": "witness-category", - "children": 1, - "created": "2016-10-10T20:38:33", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"witness-category\"]}", - "last_payout": "2016-11-11T00:08:15", - "last_update": "2016-10-10T20:38:33", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "wackou", - "parent_permlink": "wackou-witness-update-2016-10-10", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-wackou-wackou-witness-update-2016-10-10-20161010t203824861z", - "post_id": 1498398, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Wackou witness update - 2016-10-10", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/witness-category/@wackou/wackou-witness-update-2016-10-10#@gtg/re-wackou-wackou-witness-update-2016-10-10-20161010t203824861z" - }, - "entry_id": 87, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Jenny, welcome to Steemit :-)\nI keep my fingers crossed for [pevo.science](https://pevo.science).", - "body_length": 103, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduction", - "children": 1, - "created": "2016-10-08T21:43:24", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduction\"],\"links\":[\"https://pevo.science\"]}", - "last_payout": "2016-11-09T18:36:57", - "last_update": "2016-10-08T21:43:24", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "muizianer", - "parent_permlink": "hi-everyone-my-introduction-on-steemit", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-muizianer-hi-everyone-my-introduction-on-steemit-20161008t214317480z", - "post_id": 1481293, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hi Everyone - My introduction on Steemit", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduction/@muizianer/hi-everyone-my-introduction-on-steemit#@gtg/re-muizianer-hi-everyone-my-introduction-on-steemit-20161008t214317480z" - }, - "entry_id": 86, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 86851182670227, - "rshares": 11492087906, - "voter": "betamusic" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "I opt for ***Cinnamon***. I think it fits. Also, it's how Raj's dog was called in _\"The Big Bang Theory\"_.\nAnyway, you give him much more than just a name.\nGood work and good luck!", - "body_length": 181, - "cashout_time": "1969-12-31T23:59:59", - "category": "animalrescue", - "children": 1, - "created": "2016-10-06T15:39:00", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"animalrescue\"]}", - "last_payout": "2016-11-06T04:09:24", - "last_update": "2016-10-06T15:39:00", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 11492087906, - "parent_author": "betamusic", - "parent_permlink": "the-incredible-story-of-a-puppy-abandoned", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-betamusic-the-incredible-story-of-a-puppy-abandoned-20161006t153852192z", - "post_id": 1460923, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "The incredible story of a puppy abandoned", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/animalrescue/@betamusic/the-incredible-story-of-a-puppy-abandoned#@gtg/re-betamusic-the-incredible-story-of-a-puppy-abandoned-20161006t153852192z" - }, - "entry_id": 85, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Jim, welcome to Steemit :-)", - "body_length": 33, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 0, - "created": "2016-10-06T06:32:51", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"]}", - "last_payout": "2016-11-06T01:37:09", - "last_update": "2016-10-06T06:32:51", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "dontio", - "parent_permlink": "hello-steemit-i-m-jim-a-new-englander-suburbanite-and-soon-to-be-panamanian-cowboy", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-dontio-hello-steemit-i-m-jim-a-new-englander-suburbanite-and-soon-to-be-panamanian-cowboy-20161006t063245219z", - "post_id": 1457542, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hello Steemit, I'm Jim -- A New Englander Suburbanite and soon-to-be Panamanian Cowboy", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@dontio/hello-steemit-i-m-jim-a-new-englander-suburbanite-and-soon-to-be-panamanian-cowboy#@gtg/re-dontio-hello-steemit-i-m-jim-a-new-englander-suburbanite-and-soon-to-be-panamanian-cowboy-20161006t063245219z" - }, - "entry_id": 84, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @steemvest17,\nGood hint, but please do not abuse #witness-category tag.", - "body_length": 77, - "cashout_time": "1969-12-31T23:59:59", - "category": "life", - "children": 1, - "created": "2016-10-04T16:07:27", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"witness-category\",\"life\"],\"users\":[\"steemvest17\"]}", - "last_payout": "2016-11-04T16:03:36", - "last_update": "2016-10-04T16:07:27", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "steemvest17", - "parent_permlink": "how-to-keep-the-water-hot-for-a-long-time-in-a-stainless-steel-flask", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-steemvest17-how-to-keep-the-water-hot-for-a-long-time-in-a-stainless-steel-flask-20161004t160721542z", - "post_id": 1441797, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "How to Keep the Water Hot for a Long Time in a Stainless Steel Flask", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/life/@steemvest17/how-to-keep-the-water-hot-for-a-long-time-in-a-stainless-steel-flask#@gtg/re-steemvest17-how-to-keep-the-water-hot-for-a-long-time-in-a-stainless-steel-flask-20161004t160721542z" - }, - "entry_id": 83, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Jens, welcome to Steemit :-)\n(Blackbird is awesome!)", - "body_length": 58, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 1, - "created": "2016-10-02T21:54:21", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"]}", - "last_payout": "2016-11-02T21:55:57", - "last_update": "2016-10-02T21:54:21", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "jenskohler", - "parent_permlink": "intro-jens-kohler-old-man", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-jenskohler-intro-jens-kohler-old-man-20161002t215415266z", - "post_id": 1425757, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "INTRO: Jens Kohler, Old Man", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@jenskohler/intro-jens-kohler-old-man#@gtg/re-jenskohler-intro-jens-kohler-old-man-20161002t215415266z" - }, - "entry_id": 82, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 352970428645376, - "rshares": 543179470457, - "voter": "roelandp" - }, - { - "percent": "10000", - "reputation": 334447189554781, - "rshares": 81853469708, - "voter": "timsaid" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Awesome idea, nice story and great initiative!\nRbkvz!", - "body_length": 53, - "cashout_time": "1969-12-31T23:59:59", - "category": "story", - "children": 1, - "created": "2016-10-02T10:26:51", - "curator_payout_value": "0.023 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"story\"]}", - "last_payout": "2016-11-02T00:52:18", - "last_update": "2016-10-02T10:26:51", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 625032940165, - "parent_author": "timsaid", - "parent_permlink": "love-exe-a-steemfest-crypto-love-story-episode-1-or-or-steemfest-ticket-giveaway", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-timsaid-love-exe-a-steemfest-crypto-love-story-episode-1-or-or-steemfest-ticket-giveaway-20161002t102646364z", - "post_id": 1420712, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "love.exe - A SteemFest Crypto Love Story Episode 1 || SteemFest Ticket Giveaway", - "title": "", - "total_payout_value": "0.085 HBD", - "url": "/story/@timsaid/love-exe-a-steemfest-crypto-love-story-episode-1-or-or-steemfest-ticket-giveaway#@gtg/re-timsaid-love-exe-a-steemfest-crypto-love-story-episode-1-or-or-steemfest-ticket-giveaway-20161002t102646364z" - }, - "entry_id": 81, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Felicia, welcome to Steemit :-)\nI somehow missed your introduction post but @thecryptofiend linked this one on #introduceyourself channel at [steemit.chat](https://steemit.chat/channel/introduceyourself)\nNice drawings. Ganbatte ne!", - "body_length": 237, - "cashout_time": "1969-12-31T23:59:59", - "category": "art", - "children": 1, - "created": "2016-10-01T20:29:12", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\",\"art\"],\"users\":[\"thecryptofiend\"],\"links\":[\"https://steemit.chat/channel/introduceyourself\"]}", - "last_payout": "2016-11-02T00:50:09", - "last_update": "2016-10-01T20:29:12", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "scarlet-rain", - "parent_permlink": "my-verification", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-scarlet-rain-my-verification-20161001t202904946z", - "post_id": 1416597, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "My verification", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/art/@scarlet-rain/my-verification#@gtg/re-scarlet-rain-my-verification-20161001t202904946z" - }, - "entry_id": 80, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 156397998488, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "You better don't fall asleep at the office ;-)", - "body_length": 46, - "cashout_time": "1969-12-31T23:59:59", - "category": "nature", - "children": 1, - "created": "2016-09-30T15:45:00", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"nature\"]}", - "last_payout": "2016-10-31T22:59:51", - "last_update": "2016-09-30T15:45:00", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 156397998488, - "parent_author": "skypilot", - "parent_permlink": "my-office-in-the-sky", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-skypilot-my-office-in-the-sky-20160930t154455448z", - "post_id": 1405328, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "My Office in the Sky", - "title": "", - "total_payout_value": "0.023 HBD", - "url": "/nature/@skypilot/my-office-in-the-sky#@gtg/re-skypilot-my-office-in-the-sky-20160930t154455448z" - }, - "entry_id": 79, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Oh, I see. I was curious how come you got from there straight to Warsaw Marathon when I saw your [\u30ef\u30eb\u30b7\u30e3\u30ef\u30de\u30e9\u30bd\u30f3\u51fa\u307e\u3057\u305f\uff01](https://steemit.com/warsaw/@iyuta/i-ran-warsaw-marathon) post :-)", - "body_length": 179, - "cashout_time": "1969-12-31T23:59:59", - "category": "blog", - "children": 1, - "created": "2016-09-30T13:32:36", - "curator_payout_value": "0.000 HBD", - "depth": 3, - "json_metadata": "{\"tags\":[\"blog\"],\"links\":[\"https://steemit.com/warsaw/@iyuta/i-ran-warsaw-marathon\"]}", - "last_payout": "2016-10-31T11:00:00", - "last_update": "2016-09-30T13:32:36", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "iyuta", - "parent_permlink": "re-gtg-re-iyuta-i-might-appear-on-polish-tv-tv-20160930t111647922z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-iyuta-re-gtg-re-iyuta-i-might-appear-on-polish-tv-tv-20160930t133231019z", - "post_id": 1404409, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "I Might Appear On Polish TV? / \u30dd\u30fc\u30e9\u30f3\u30c9\u3067TV\u30c7\u30d3\u30e5\u30fc\u304b\u3082\uff1f", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/blog/@iyuta/i-might-appear-on-polish-tv-tv#@gtg/re-iyuta-re-gtg-re-iyuta-i-might-appear-on-polish-tv-tv-20160930t133231019z" - }, - "entry_id": 78, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 34815477880699, - "rshares": 1003575258, - "voter": "iyuta" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @iyuta, I'm glad you enjoyed your stay in Poland.", - "body_length": 55, - "cashout_time": "1969-12-31T23:59:59", - "category": "blog", - "children": 3, - "created": "2016-09-30T10:59:24", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"blog\"],\"users\":[\"iyuta\"]}", - "last_payout": "2016-10-31T11:00:00", - "last_update": "2016-09-30T10:59:24", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 1003575258, - "parent_author": "iyuta", - "parent_permlink": "i-might-appear-on-polish-tv-tv", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-iyuta-i-might-appear-on-polish-tv-tv-20160930t105919668z", - "post_id": 1403494, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "I Might Appear On Polish TV? / \u30dd\u30fc\u30e9\u30f3\u30c9\u3067TV\u30c7\u30d3\u30e5\u30fc\u304b\u3082\uff1f", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/blog/@iyuta/i-might-appear-on-polish-tv-tv#@gtg/re-iyuta-i-might-appear-on-polish-tv-tv-20160930t105919668z" - }, - "entry_id": 77, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 59936509858616, - "rshares": 51814745073, - "voter": "noisy" - }, - { - "percent": "10000", - "reputation": 14435619141603, - "rshares": 174943688845, - "voter": "repholder" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Not that I would like to destroy your dreams (wake up, @noisy, wake up ;-) ), but We've already checked that with Tesla. Apparently Elon Musk is currently more interested in getting his rocket to Mars, because I wasn't able to get confirmation that it's possible to travel 1200km with S85D in one day without risking my absence on SteemFest. Too much risk that you miss evening drinks ;-) For my route I need 4 stops (80% on supercharger for 30-40minutes each time).\nDoable from Berlin (you only need to stop once) but I would not risk any longer trip. \nAs for crazy, @noisy stuff, come by steam car :-) or at least steam train :-)", - "body_length": 632, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit", - "children": 1, - "created": "2016-09-28T18:36:42", - "curator_payout_value": "0.008 HBD", - "depth": 2, - "json_metadata": "{\"tags\":[\"steemit\"],\"users\":[\"noisy\"]}", - "last_payout": "2016-10-29T21:26:24", - "last_update": "2016-09-28T18:36:42", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 226758433918, - "parent_author": "noisy", - "parent_permlink": "re-serejandmyself-get-your-free-ticket-to-steemfest-inside-20160928t163649993z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-noisy-re-serejandmyself-get-your-free-ticket-to-steemfest-inside-20160928t183636893z", - "post_id": 1387373, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Get Your Free Ticket to Steemfest Inside!", - "title": "", - "total_payout_value": "0.030 HBD", - "url": "/steemit/@serejandmyself/get-your-free-ticket-to-steemfest-inside#@gtg/re-noisy-re-serejandmyself-get-your-free-ticket-to-steemfest-inside-20160928t183636893z" - }, - "entry_id": 76, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Josh, welcome to Steemit! :-)", - "body_length": 35, - "cashout_time": "1969-12-31T23:59:59", - "category": "life", - "children": 0, - "created": "2016-09-28T07:46:54", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"life\"]}", - "last_payout": "2016-10-29T06:39:24", - "last_update": "2016-09-28T07:46:54", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "joshfischbach", - "parent_permlink": "hello-steemers-introduction-post", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-joshfischbach-hello-steemers-introduction-post-20160928t074649292z", - "post_id": 1383116, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hello Steemers! Introduction Post", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/life/@joshfischbach/hello-steemers-introduction-post#@gtg/re-joshfischbach-hello-steemers-introduction-post-20160928t074649292z" - }, - "entry_id": 75, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Isa, welcome to Steemit! :-)\nI know that you are on Steemit for a while, but I found your post just now (while searching for interesting introductions for promotion on [#introduceyourself](https://steemit.chat/channel/introduceyourself) channel at steemit.chat)\nI'll follow you, looking forward for your photographs.\nThere's no way to protect your photos from being stolen, but you could make sure that you would easily prove that original is yours and then ask for compensation, etc. If you have any questions you can contact me directly on [steemit.chat](https://steemit.chat), as [Gandalf](https://steemit.chat/direct/gandalf)", - "body_length": 635, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 1, - "created": "2016-09-27T20:10:57", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"],\"links\":[\"https://steemit.chat/channel/introduceyourself\",\"https://steemit.chat\",\"https://steemit.chat/direct/gandalf\"]}", - "last_payout": "2016-10-28T15:46:27", - "last_update": "2016-09-27T20:10:57", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "isa-za", - "parent_permlink": "hello-good-people-an-other-presentation-for-curious", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-isa-za-hello-good-people-an-other-presentation-for-curious-20160927t201051162z", - "post_id": 1378596, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hello good people ! An other presentation for curious", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@isa-za/hello-good-people-an-other-presentation-for-curious#@gtg/re-isa-za-hello-good-people-an-other-presentation-for-curious-20160927t201051162z" - }, - "entry_id": 74, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 130324293613, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Reply to post with declined payout. How it is affected?", - "body_length": 55, - "cashout_time": "1969-12-31T23:59:59", - "category": "spam", - "children": 1, - "created": "2016-09-27T18:17:18", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"spam\"]}", - "last_payout": "2016-10-28T17:34:03", - "last_update": "2016-09-27T18:17:18", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 130324293613, - "parent_author": "test-safari", - "parent_permlink": "m8vdx-test", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-test-safari-m8vdx-test-20160927t181714536z", - "post_id": 1377626, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Decline Payout test", - "title": "", - "total_payout_value": "0.022 HBD", - "url": "/spam/@test-safari/m8vdx-test#@gtg/re-test-safari-m8vdx-test-20160927t181714536z" - }, - "entry_id": 73, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "> Is my cat awesome and cute?\n\nNo. Two of them are awesome and cute!\n\n> Is there a need for posts about how cute my cat is?\n\nWe already know that but make sure we won't forget.\n\n> Is there life on mars?\n\nWho cares? Cats are awesome and cute!", - "body_length": 241, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit", - "children": 1, - "created": "2016-09-27T07:14:42", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"steemit\"]}", - "last_payout": "2016-10-28T08:23:45", - "last_update": "2016-09-27T07:14:42", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "garethnelsonuk", - "parent_permlink": "my-cat-is-cute-this-is-a-serious-concern-i-promise-you-it-really-is", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-garethnelsonuk-my-cat-is-cute-this-is-a-serious-concern-i-promise-you-it-really-is-20160927t071436295z", - "post_id": 1373228, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "My cat is cute - this is a serious concern (I promise you, it really is)", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/steemit/@garethnelsonuk/my-cat-is-cute-this-is-a-serious-concern-i-promise-you-it-really-is#@gtg/re-garethnelsonuk-my-cat-is-cute-this-is-a-serious-concern-i-promise-you-it-really-is-20160927t071436295z" - }, - "entry_id": 72, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Elena, welcome to Steemit! :-)\nNice introduction, I just reposted it on [#introduceyourself](https://steemit.chat/channel/introduceyourself) channel on [steemit.chat](https://steemit.chat)", - "body_length": 194, - "cashout_time": "1969-12-31T23:59:59", - "category": "life", - "children": 1, - "created": "2016-09-26T15:36:03", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"life\"],\"links\":[\"https://steemit.chat/channel/introduceyourself\",\"https://steemit.chat\"]}", - "last_payout": "2016-10-27T20:42:27", - "last_update": "2016-09-26T15:36:03", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "elena-singer", - "parent_permlink": "is-the-steemit-change-life-for-a-better-as-art-exclusive-interview-with-new-opera-diva-video", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-elena-singer-is-the-steemit-change-life-for-a-better-as-art-exclusive-interview-with-new-opera-diva-video-20160926t153558948z", - "post_id": 1366365, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Does Steemit Change the Life for the Better Similar Ways to Art? Exclusive Interview with New Opera Diva [Video]", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/life/@elena-singer/is-the-steemit-change-life-for-a-better-as-art-exclusive-interview-with-new-opera-diva-video#@gtg/re-elena-singer-is-the-steemit-change-life-for-a-better-as-art-exclusive-interview-with-new-opera-diva-video-20160926t153558948z" - }, - "entry_id": 71, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 282028870728, - "rshares": 54053038, - "voter": "anatworld" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Ann, welcome to Steemit! :-)", - "body_length": 34, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduce", - "children": 1, - "created": "2016-09-25T17:27:21", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduce\"]}", - "last_payout": "2016-10-27T02:53:33", - "last_update": "2016-09-25T17:27:21", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 54053038, - "parent_author": "anatworld", - "parent_permlink": "hi-my-name-is-ann-and-im-16-glad-to-be-here-d", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-anatworld-hi-my-name-is-ann-and-im-16-glad-to-be-here-d-20160925t172717954z", - "post_id": 1357430, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hi! My name is Ann, and im 16. Glad to be here! :D", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduce/@anatworld/hi-my-name-is-ann-and-im-16-glad-to-be-here-d#@gtg/re-anatworld-hi-my-name-is-ann-and-im-16-glad-to-be-here-d-20160925t172717954z" - }, - "entry_id": 70, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Cora, welcome to Steemit :-)", - "body_length": 34, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduction", - "children": 1, - "created": "2016-09-23T21:37:51", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduction\"]}", - "last_payout": "2016-10-25T02:14:30", - "last_update": "2016-09-23T21:37:51", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "corabell99", - "parent_permlink": "hi-i-m-cora-and-i-take-photos-of-people", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-corabell99-hi-i-m-cora-and-i-take-photos-of-people-20160923t213747864z", - "post_id": 1341920, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hi! I'm Cora and I Take Photos of People!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduction/@corabell99/hi-i-m-cora-and-i-take-photos-of-people#@gtg/re-corabell99-hi-i-m-cora-and-i-take-photos-of-people-20160923t213747864z" - }, - "entry_id": 69, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 90708691850244, - "rshares": 33378091443, - "voter": "williambanks" - }, - { - "percent": "10000", - "reputation": 2218615828443, - "rshares": 55513373, - "voter": "badassbarbie" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Dawn, welcome to Steemit :-)", - "body_length": 34, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 1, - "created": "2016-09-23T10:00:36", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-10-24T09:24:42", - "last_update": "2016-09-23T10:00:36", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 33433604816, - "parent_author": "badassbarbie", - "parent_permlink": "a-slow-introduction", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-badassbarbie-a-slow-introduction-20160923t100032871z", - "post_id": 1336222, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "A Slow Introduction...", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@badassbarbie/a-slow-introduction#@gtg/re-badassbarbie-a-slow-introduction-20160923t100032871z" - }, - "entry_id": 68, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 31620973634639, - "rshares": 100665370195, - "voter": "aizensou" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 94958161220, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "I disagree to some extent.\nI agree enough to upvote.", - "body_length": 52, - "cashout_time": "1969-12-31T23:59:59", - "category": "abuse", - "children": 0, - "created": "2016-09-23T09:54:48", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"abuse\"]}", - "last_payout": "2016-10-24T03:31:48", - "last_update": "2016-09-23T09:54:48", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 195623531415, - "parent_author": "kushed", - "parent_permlink": "will-the-real-sock-puppet-please-stand-up", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-kushed-will-the-real-sock-puppet-please-stand-up-20160923t095445401z", - "post_id": 1336191, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Will the real sock puppet please stand up", - "title": "", - "total_payout_value": "0.029 HBD", - "url": "/abuse/@kushed/will-the-real-sock-puppet-please-stand-up#@gtg/re-kushed-will-the-real-sock-puppet-please-stand-up-20160923t095445401z" - }, - "entry_id": 67, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 53180487951407, - "rshares": 248618494135, - "voter": "lovejoy" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 94840305332, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 190067437472, - "rshares": 0, - "voter": "gimme-free" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "No one wants to be told how to behave. Different people like or need different things, so it's very hard to convince one to be more respectful.\nGood will have no good price in a world when only profit matters.\nBut hey, wait:\n\n***Lack of respect, FUD and flame wars are not profitable.***\n\nso\n##### Post Cats, Not Wars! #####", - "body_length": 324, - "cashout_time": "1969-12-31T23:59:59", - "category": "beyondbitcoin", - "children": 1, - "created": "2016-09-23T06:48:06", - "curator_payout_value": "0.010 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"beyondbitcoin\"]}", - "last_payout": "2016-10-24T12:26:36", - "last_update": "2016-09-23T06:48:06", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 343458799467, - "parent_author": "intelliguy", - "parent_permlink": "crypto-respect-all-of-us-need-to-stop-trying-to-drown-each-other-in-public-we-re-hurting-ourselves-here", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-intelliguy-crypto-respect-all-of-us-need-to-stop-trying-to-drown-each-other-in-public-we-re-hurting-ourselves-here-20160923t064804495z", - "post_id": 1335380, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "CryptoRESPECT. All of us need to stop trying to drown each other in public. We're hurting ourselves here!", - "title": "", - "total_payout_value": "0.048 HBD", - "url": "/beyondbitcoin/@intelliguy/crypto-respect-all-of-us-need-to-stop-trying-to-drown-each-other-in-public-we-re-hurting-ourselves-here#@gtg/re-intelliguy-crypto-respect-all-of-us-need-to-stop-trying-to-drown-each-other-in-public-we-re-hurting-ourselves-here-20160923t064804495z" - }, - "entry_id": 66, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 93897518527, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Thank you for your appreciation, @someguy123, but @joseph is right, my low number of missed blocks shouldn't be an only reason to vote for me. I'm doing my best, but `total_missed`is just a number. Reasons of missing blocks are various. Sometimes it might be just a matter of luck or lack of it. I was trying to crash my node too (in the process of testing my infrastructure concept) which would increase that counter, but even then, it would still be in favor of the platform. Witnesses (especially backup) should not hesitate to test new rc versions, etc just because some misses might happen. Also, while considering top19 witnesses, taking into account their % of missed blocks (to total generated) would give a better view about their reliability.\n\nAnyway, such posts have a great value whether we agree with authors point of view or not, because in the end it helps to create awareness of witnesses role and how important it is for Steem.", - "body_length": 944, - "cashout_time": "1969-12-31T23:59:59", - "category": "witness-category", - "children": 0, - "created": "2016-09-22T12:02:00", - "curator_payout_value": "0.000 HBD", - "depth": 2, - "json_metadata": "{\"tags\":[\"witness-category\"],\"users\":[\"someguy123\",\"joseph\"]}", - "last_payout": "2016-10-23T12:07:12", - "last_update": "2016-09-22T12:02:00", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 93897518527, - "parent_author": "joseph", - "parent_permlink": "re-someguy123-it-s-time-to-check-your-witness-votes-20160922t104946159z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-joseph-re-someguy123-it-s-time-to-check-your-witness-votes-20160922t120155708z", - "post_id": 1326578, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "It's time to check your witness votes!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/witness-category/@someguy123/it-s-time-to-check-your-witness-votes#@gtg/re-joseph-re-someguy123-it-s-time-to-check-your-witness-votes-20160922t120155708z" - }, - "entry_id": 65, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Freedom. There's no should. There is nothing wrong in powering down, there is nothing wrong in taking profit from own investment and there is nothing wrong in running operating exchange service (Seriously? I need to defend that? Let's get back on to the right track.)", - "body_length": 267, - "cashout_time": "1969-12-31T23:59:59", - "category": "steem", - "children": 0, - "created": "2016-09-22T08:23:21", - "curator_payout_value": "0.000 HBD", - "depth": 3, - "json_metadata": "{\"tags\":[\"steem\"]}", - "last_payout": "2016-10-23T08:50:57", - "last_update": "2016-09-22T08:23:21", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "transparency", - "parent_permlink": "re-blocktrades-re-transparency-don-t-vote-for-witnesses-your-vote-does-not-matter-20160922t054322996z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-transparency-re-blocktrades-re-transparency-don-t-vote-for-witnesses-your-vote-does-not-matter-20160922t082319044z", - "post_id": 1325275, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Don't \"Vote for Witnesses\", your vote does not matter.", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/steem/@transparency/don-t-vote-for-witnesses-your-vote-does-not-matter#@gtg/re-transparency-re-blocktrades-re-transparency-don-t-vote-for-witnesses-your-vote-does-not-matter-20160922t082319044z" - }, - "entry_id": 64, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 93712296002, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 17498320798649, - "rshares": 36503990407, - "voter": "picokernel" - }, - { - "percent": "10000", - "reputation": 98590241491022, - "rshares": 202030904193, - "voter": "anyx" - }, - { - "percent": "10000", - "reputation": 49113922291, - "rshares": 95543638, - "voter": "plentyoffish" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Because I was mentioned here, as one of those who would go way down in _\"Community Vote\"_ list (i.e. list re-organized in a way that only the number of voters matters, disregarding voters stake) I feel that I need to reply.\n\nAccording to your list I would go down 15 spots. That's a lot, but **you forgot to take the time into account**.\n @steemed is on Steem since beginning, @ned's and @dan's accounts are only one day older. Please compare that time to time I had to gather votes.\n\nI posted my witness application 47 days ago. There are only three users with higher number of votes that registered later to Steem and are placed higher in that classification and one of them made me into top50 (Thank you @anyx) allowing me to get votes from regular users, because other than that only those who were able to user `cli_wallet` could vote for me. Voting for random witness (i.e. outside of top50) is a feature added just recently.\n\nI upvoted this post even if I disagree, because I believe it would help to create awareness of witnesses role and how important it is for Steem. That is exactly what I'm trying to achieve.\n\nAnyone who would like to talk about witnesses related topics can contact me directly on [steemit.chat](https://steemit.chat), as [Gandalf](https://steemit.chat/direct/gandalf) or join [#witness](https://steemit.chat/channel/witness) channel.\n\nAnd by the way: [**Vote for witnesses**](https://steemit.com/~witnesses), your vote **does matter**.", - "body_length": 1466, - "cashout_time": "1969-12-31T23:59:59", - "category": "steem", - "children": 0, - "created": "2016-09-22T08:01:06", - "curator_payout_value": "0.010 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"steem\"],\"users\":[\"steemed\",\"ned\",\"dan\",\"anyx\"],\"links\":[\"https://steemit.chat\",\"https://steemit.chat/direct/gandalf\",\"https://steemit.chat/channel/witness\",\"https://steemit.com/~witnesses\"]}", - "last_payout": "2016-10-23T08:50:57", - "last_update": "2016-09-22T09:44:21", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 332342734240, - "parent_author": "transparency", - "parent_permlink": "don-t-vote-for-witnesses-your-vote-does-not-matter", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-transparency-don-t-vote-for-witnesses-your-vote-does-not-matter-20160922t080102891z", - "post_id": 1325188, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Don't \"Vote for Witnesses\", your vote does not matter.", - "title": "", - "total_payout_value": "0.051 HBD", - "url": "/steem/@transparency/don-t-vote-for-witnesses-your-vote-does-not-matter#@gtg/re-transparency-don-t-vote-for-witnesses-your-vote-does-not-matter-20160922t080102891z" - }, - "entry_id": 63, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 11554252869567, - "rshares": 21149988302, - "voter": "proctologic" - }, - { - "percent": "100", - "reputation": 98590241491022, - "rshares": 4040618083, - "voter": "anyx" - }, - { - "percent": "10000", - "reputation": 140605453893072, - "rshares": 482103747918, - "voter": "jesta" - }, - { - "percent": "10000", - "reputation": 49113922291, - "rshares": 98438900, - "voter": "plentyoffish" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "> The steemit account alone has enough vests to single-handedly make 19 brand new accounts the top 19\n\nThat is the reason that _**\"Decline Voting Rights\"**_ feature was introduced in latest release.\n\n>An account can chose to decline their voting rights after a 30 day delay. This includes voting on content and witnesses. The voting rights cannot be acquired again once they have been declined. This is only to formalize a smart contract between certain accounts and the community that currently only exists as a social contract.", - "body_length": 529, - "cashout_time": "1969-12-31T23:59:59", - "category": "steem", - "children": 0, - "created": "2016-09-22T06:18:24", - "curator_payout_value": "0.024 HBD", - "depth": 3, - "json_metadata": "{\"tags\":[\"steem\"]}", - "last_payout": "2016-10-23T08:50:57", - "last_update": "2016-09-22T06:18:24", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 507392793203, - "parent_author": "picokernel", - "parent_permlink": "re-smooth-re-transparency-don-t-vote-for-witnesses-your-vote-does-not-matter-20160922t045802316z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-picokernel-re-smooth-re-transparency-don-t-vote-for-witnesses-your-vote-does-not-matter-20160922t061821960z", - "post_id": 1324645, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Don't \"Vote for Witnesses\", your vote does not matter.", - "title": "", - "total_payout_value": "0.075 HBD", - "url": "/steem/@transparency/don-t-vote-for-witnesses-your-vote-does-not-matter#@gtg/re-picokernel-re-smooth-re-transparency-don-t-vote-for-witnesses-your-vote-does-not-matter-20160922t061821960z" - }, - "entry_id": 62, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "100", - "reputation": 72155318050858, - "rshares": 375756375, - "voter": "felixxx" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Ingrid, welcome to Steemit! :-)\nThat's interesting, I've never heard about [Miracle Fruit](https://en.wikipedia.org/wiki/Synsepalum_dulcificum) before. Thank you for sharing.", - "body_length": 180, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 1, - "created": "2016-09-21T18:41:09", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"],\"links\":[\"https://en.wikipedia.org/wiki/Synsepalum_dulcificum\"]}", - "last_payout": "2016-10-23T01:26:54", - "last_update": "2016-09-21T18:41:09", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 375756375, - "parent_author": "ingridstrauss", - "parent_permlink": "introducing-myself-how-to-throw-a-party-your-friends-will-never-forget", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-ingridstrauss-introducing-myself-how-to-throw-a-party-your-friends-will-never-forget-20160921t184105613z", - "post_id": 1319577, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Introducing Myself: How To Throw A Party Your Friends Will Never Forget", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@ingridstrauss/introducing-myself-how-to-throw-a-party-your-friends-will-never-forget#@gtg/re-ingridstrauss-introducing-myself-how-to-throw-a-party-your-friends-will-never-forget-20160921t184105613z" - }, - "entry_id": 61, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Here's good post about that subject: [The Tale of the 5 Brothers -- A Voting Power Parable](https://steemit.com/steem-help/@sigmajin/the-tale-of-the-5-brothers-a-voting-power-parable) by @sigmajin. But again: eventually it was **NOT** changed.", - "body_length": 243, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit", - "children": 3, - "created": "2016-09-20T18:29:21", - "curator_payout_value": "0.000 HBD", - "depth": 4, - "json_metadata": "{\"tags\":[\"steemit\"],\"users\":[\"sigmajin\"],\"links\":[\"https://steemit.com/steem-help/@sigmajin/the-tale-of-the-5-brothers-a-voting-power-parable\"]}", - "last_payout": "2016-10-21T18:56:30", - "last_update": "2016-09-20T18:29:21", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "stellabelle", - "parent_permlink": "re-gtg-re-sponge-bob-re-stellabelle-apology-to-mibenkito-honest-whales-investors-witnesses-and-steemit-community-20160920t173554076z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-stellabelle-re-gtg-re-sponge-bob-re-stellabelle-apology-to-mibenkito-honest-whales-investors-witnesses-and-steemit-community-20160920t182918656z", - "post_id": 1309142, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Apology To @mibenkito, Honest Whales, Investors, Witnesses And Steemit Community", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/steemit/@stellabelle/apology-to-mibenkito-honest-whales-investors-witnesses-and-steemit-community#@gtg/re-stellabelle-re-gtg-re-sponge-bob-re-stellabelle-apology-to-mibenkito-honest-whales-investors-witnesses-and-steemit-community-20160920t182918656z" - }, - "entry_id": 60, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 394956111554767, - "rshares": 449189345704, - "voter": "kaylinart" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 92202331826, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 352970428645376, - "rshares": 469951020571, - "voter": "roelandp" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Not true.\n[Steem v0.14.2](https://github.com/steemit/steem/releases/tag/v0.14.2)\n>___Vote Balancing___\n>We have reverted the changes to vote balancing from 0.14.0. Voting power and vote weights are unaffected in this release.\n\nBesides @knircky is right, it was proposal for a target, and a target is different from a maximum.\nAnyway, you can safely vote if you like.\nThat's what is all about and always should be.", - "body_length": 413, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit", - "children": 5, - "created": "2016-09-20T17:31:30", - "curator_payout_value": "0.022 HBD", - "depth": 2, - "json_metadata": "{\"tags\":[\"steemit\"],\"users\":[\"knircky\"],\"links\":[\"https://github.com/steemit/steem/releases/tag/v0.14.2\"]}", - "last_payout": "2016-10-21T18:56:30", - "last_update": "2016-09-20T17:31:30", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 1011342698101, - "parent_author": "sponge-bob", - "parent_permlink": "re-stellabelle-apology-to-mibenkito-honest-whales-investors-witnesses-and-steemit-community-20160920t165737375z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-sponge-bob-re-stellabelle-apology-to-mibenkito-honest-whales-investors-witnesses-and-steemit-community-20160920t173128090z", - "post_id": 1308577, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Apology To @mibenkito, Honest Whales, Investors, Witnesses And Steemit Community", - "title": "", - "total_payout_value": "0.112 HBD", - "url": "/steemit/@stellabelle/apology-to-mibenkito-honest-whales-investors-witnesses-and-steemit-community#@gtg/re-sponge-bob-re-stellabelle-apology-to-mibenkito-honest-whales-investors-witnesses-and-steemit-community-20160920t173128090z" - }, - "entry_id": 59, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 92129129208, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "I disagree with the thesis, but upvoted. \nIt pays to be good. Good writer in this case :-)", - "body_length": 90, - "cashout_time": "1969-12-31T23:59:59", - "category": "business", - "children": 1, - "created": "2016-09-20T15:17:00", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"business\"]}", - "last_payout": "2016-10-21T04:45:21", - "last_update": "2016-09-20T15:17:00", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 92129129208, - "parent_author": "thebluepanda", - "parent_permlink": "it-pays-to-be-a-jerk", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-thebluepanda-it-pays-to-be-a-jerk-20160920t151656985z", - "post_id": 1307304, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "It Pays To Be A Jerk", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/business/@thebluepanda/it-pays-to-be-a-jerk#@gtg/re-thebluepanda-it-pays-to-be-a-jerk-20160920t151656985z" - }, - "entry_id": 58, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Catherine, welcome to Steemit!\nGood luck with your trips. :-)", - "body_length": 67, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 0, - "created": "2016-09-20T06:51:30", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"]}", - "last_payout": "2016-10-21T01:27:42", - "last_update": "2016-09-20T06:51:30", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "wifi", - "parent_permlink": "the-story-of-my-sister", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-wifi-the-story-of-my-sister-20160920t065128739z", - "post_id": 1304262, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "The story of my sister", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@wifi/the-story-of-my-sister#@gtg/re-wifi-the-story-of-my-sister-20160920t065128739z" - }, - "entry_id": 57, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @edmt, welcome to Steemit!\nPlease note, that adding a picture to your post could make it more appealing. ( I used my cat for that ;-) )", - "body_length": 141, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-09-19T21:30:51", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"],\"users\":[\"edmt\"]}", - "last_payout": "2016-10-20T20:31:21", - "last_update": "2016-09-19T21:30:51", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "edmt", - "parent_permlink": "hello-all-new-here-dance-music-raves-and-my-perspective", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-edmt-hello-all-new-here-dance-music-raves-and-my-perspective-20160919t213050556z", - "post_id": 1300525, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hello all, New here! DANCE MUSIC, RAVES, & MY PERSPECTIVE!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@edmt/hello-all-new-here-dance-music-raves-and-my-perspective#@gtg/re-edmt-hello-all-new-here-dance-music-raves-and-my-perspective-20160919t213050556z" - }, - "entry_id": 56, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 91377535755, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 22316942961150, - "rshares": 132462337, - "voter": "seisges" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "What a timing, Heidi :-) Steemit just introduced \"**Reblog**\" feature that you might want to use to present their posts to all your followers.", - "body_length": 142, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit", - "children": 1, - "created": "2016-09-19T19:34:06", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"steemit\"]}", - "last_payout": "2016-10-21T03:47:30", - "last_update": "2016-09-19T19:34:06", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 91509998092, - "parent_author": "heiditravels", - "parent_permlink": "let-s-shed-some-light-on-our-fellow-steemers-nomadnessie-mauinomads-tobydaniel2", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-heiditravels-let-s-shed-some-light-on-our-fellow-steemers-nomadnessie-mauinomads-tobydaniel2-20160919t193404575z", - "post_id": 1299201, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Let's Shed Some Light on Our Fellow Steemers: @nomadnessie @mauinomads @tobydaniel2", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/steemit/@heiditravels/let-s-shed-some-light-on-our-fellow-steemers-nomadnessie-mauinomads-tobydaniel2#@gtg/re-heiditravels-let-s-shed-some-light-on-our-fellow-steemers-nomadnessie-mauinomads-tobydaniel2-20160919t193404575z" - }, - "entry_id": 55, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Brad, welcome to Steemit! :-)", - "body_length": 35, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 1, - "created": "2016-09-19T17:11:51", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-10-20T14:46:18", - "last_update": "2016-09-19T17:11:51", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "bradcostanzo", - "parent_permlink": "intro-insatiable-curiosity-business-bacon-books-and-steem-powered-fingers", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-bradcostanzo-intro-insatiable-curiosity-business-bacon-books-and-steem-powered-fingers-20160919t171147368z", - "post_id": 1297787, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "INTRO: Insatiable Curiosity, Business, Bacon, Books and Steemit Powered Fingers", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@bradcostanzo/intro-insatiable-curiosity-business-bacon-books-and-steem-powered-fingers#@gtg/re-bradcostanzo-intro-insatiable-curiosity-business-bacon-books-and-steem-powered-fingers-20160919t171147368z" - }, - "entry_id": 54, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "1000", - "reputation": 159367821767558, - "rshares": 10651362095, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 326501384824883, - "rshares": 13556914162, - "voter": "quinneaker" - }, - { - "percent": "10000", - "reputation": 247778651437705, - "rshares": 1227437088, - "voter": "saramiller" - }, - { - "percent": "100", - "reputation": 413921132397239, - "rshares": 479134104, - "voter": "gardenofeden" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "@noisy, with all due respect, your comment (and especially flag?!) doesn't make sense and most probably is based on some wrong assumptions that you've made about STEEM and SBD.", - "body_length": 176, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit", - "children": 1, - "created": "2016-09-16T19:44:30", - "curator_payout_value": "0.000 HBD", - "depth": 2, - "json_metadata": "{\"tags\":[\"steemit\"],\"users\":[\"noisy\"]}", - "last_payout": "2016-10-16T10:30:15", - "last_update": "2016-09-16T19:44:30", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 25914847449, - "parent_author": "noisy", - "parent_permlink": "re-gardenofeden-exciting-steemit-announcement-to-build-this-economy-and-support-this-revolutionary-platform-our-online-store-is-now-accepting-20160916t081816003z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-noisy-re-gardenofeden-exciting-steemit-announcement-to-build-this-economy-and-support-this-revolutionary-platform-our-online-store-is-now-accepting-20160916t194428414z", - "post_id": 1269541, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Exciting Steemit Announcement: To Build This Economy and Support This Revolutionary Platform, Our Online Store Is Now Accepting Steem!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/steemit/@gardenofeden/exciting-steemit-announcement-to-build-this-economy-and-support-this-revolutionary-platform-our-online-store-is-now-accepting#@gtg/re-noisy-re-gardenofeden-exciting-steemit-announcement-to-build-this-economy-and-support-this-revolutionary-platform-our-online-store-is-now-accepting-20160916t194428414z" - }, - "entry_id": 53, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 198679831320, - "rshares": 285319616, - "voter": "bentoncbainbridg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Benton, welcome to Steemit! :-)", - "body_length": 37, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-09-16T09:23:21", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-10-17T01:58:24", - "last_update": "2016-09-16T09:23:21", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 285319616, - "parent_author": "bentoncbainbridg", - "parent_permlink": "introducing-myself-benton-c-bainbridge-media-artist-and-tech-dreamer", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-bentoncbainbridg-introducing-myself-benton-c-bainbridge-media-artist-and-tech-dreamer-20160916t092321821z", - "post_id": 1263856, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Introducing myself, Benton C Bainbridge, media artist and tech dreamer", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@bentoncbainbridg/introducing-myself-benton-c-bainbridge-media-artist-and-tech-dreamer#@gtg/re-bentoncbainbridg-introducing-myself-benton-c-bainbridge-media-artist-and-tech-dreamer-20160916t092321821z" - }, - "entry_id": 52, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "1000", - "reputation": 86901300608582, - "rshares": 31602395751, - "voter": "chitty" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Ah, OK, understood.\n\nAssumption is that top witnesses are working hard, thus investing their time / effort / infrastructure costs so it's pretty much comparable with someone who invests (i.e. Powers Up) that much STEEM in Steem platform. In most (all?) cases those are not only efforts related to running witness node. They've done a lot for Steem and they are doing a lot. If they are not, just lets not vote for them. Let's vote for those who do. Also, please note, that in order to keep their whole voting influence (which comes with Steem Power) they can't Power Down, so they need to pay their current expenses from their own very real wallet.\n\nThere are two scenarios:\n1. Steem platform would succeed, become **A LOT** bigger and being a witness will remain fairly paid position\n2. Steem platform would fail, and all those potential Steem Power payouts would be just nice, sentimental, collectible virtual items.\n\nOK. I might sound like devil's advocate. Thing is that I'm curious and I'm paying attention at what they are doing and I'm trying to vote for them accordingly.", - "body_length": 1079, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit", - "children": 0, - "created": "2016-09-15T20:34:00", - "curator_payout_value": "0.000 HBD", - "depth": 5, - "json_metadata": "{\"tags\":[\"steemit\"]}", - "last_payout": "2016-10-16T16:02:54", - "last_update": "2016-09-15T20:34:00", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 31602395751, - "parent_author": "chitty", - "parent_permlink": "re-gtg-re-chitty-re-gtg-re-chitty-a-balanced-voting-system-is-impossible-with-current-witness-s-payouts-20160915t185208490z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-chitty-re-gtg-re-chitty-re-gtg-re-chitty-a-balanced-voting-system-is-impossible-with-current-witness-s-payouts-20160915t203359503z", - "post_id": 1258371, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "A balanced voting system is impossible with current witness\u2019s payouts.", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/steemit/@chitty/a-balanced-voting-system-is-impossible-with-current-witness-s-payouts#@gtg/re-chitty-re-gtg-re-chitty-re-gtg-re-chitty-a-balanced-voting-system-is-impossible-with-current-witness-s-payouts-20160915t203359503z" - }, - "entry_id": 51, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Steem platform is currently very, very small. Witnesses plays very important role and it would get a lot harder when Steem would grow. Every minute they are in \"if\" condition.\nI like current scheme. I think it would make more sense over time.\nOf course, you are right, difference between potential earnings of top19 and backup witnesses is huge but so it is with their responsibilities.\nPlease take a look how many witnesses are really capable of running their nodes effectively.\nI'm not saying that backup witnesses have to set their feed price or take care about keeping their nodes up to date or online at all. They don't have to. That's why they shouldn't be overpaid.\n\nTaking a look at current top 100.\n47 of them (more than a half of backup witnesses) never set their feed price (some of them might not even know how to do that) or have it heavily outdated. 28 are not even able to generate blocks.\n\nYes, running a backup witness is not profitable and shouldn't be.\nYes, I'm a backup witness :-) (to those who do not know that)\n\n[Gandalf](https://steemit.com/@gtg) (\"gtg\")", - "body_length": 1078, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit", - "children": 2, - "created": "2016-09-15T18:43:51", - "curator_payout_value": "0.000 HBD", - "depth": 3, - "json_metadata": "{\"tags\":[\"steemit\"],\"links\":[\"https://steemit.com/@gtg\"]}", - "last_payout": "2016-10-16T16:02:54", - "last_update": "2016-09-15T18:43:51", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "chitty", - "parent_permlink": "re-gtg-re-chitty-a-balanced-voting-system-is-impossible-with-current-witness-s-payouts-20160915t142108544z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-chitty-re-gtg-re-chitty-a-balanced-voting-system-is-impossible-with-current-witness-s-payouts-20160915t184349883z", - "post_id": 1257233, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "A balanced voting system is impossible with current witness\u2019s payouts.", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/steemit/@chitty/a-balanced-voting-system-is-impossible-with-current-witness-s-payouts#@gtg/re-chitty-re-gtg-re-chitty-a-balanced-voting-system-is-impossible-with-current-witness-s-payouts-20160915t184349883z" - }, - "entry_id": 50, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 94312688970406, - "rshares": 1000247174970, - "voter": "liondani" - }, - { - "percent": "1000", - "reputation": 86901300608582, - "rshares": 31585597770, - "voter": "chitty" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 87910520753, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "IMHO, witnesses should - more than others - believe for a long term growth of Steem (as a platform), so Steem Power is appropriate in this case.", - "body_length": 144, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit", - "children": 4, - "created": "2016-09-15T14:15:51", - "curator_payout_value": "0.075 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"steemit\"]}", - "last_payout": "2016-10-16T16:02:54", - "last_update": "2016-09-15T14:15:51", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 1119743293493, - "parent_author": "chitty", - "parent_permlink": "a-balanced-voting-system-is-impossible-with-current-witness-s-payouts", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-chitty-a-balanced-voting-system-is-impossible-with-current-witness-s-payouts-20160915t141551322z", - "post_id": 1254310, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "A balanced voting system is impossible with current witness\u2019s payouts.", - "title": "", - "total_payout_value": "0.242 HBD", - "url": "/steemit/@chitty/a-balanced-voting-system-is-impossible-with-current-witness-s-payouts#@gtg/re-chitty-a-balanced-voting-system-is-impossible-with-current-witness-s-payouts-20160915t141551322z" - }, - "entry_id": 49, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "True, as well as encouraging others to try to do the same :-)", - "body_length": 61, - "cashout_time": "1969-12-31T23:59:59", - "category": "stats", - "children": 0, - "created": "2016-09-15T14:11:09", - "curator_payout_value": "0.000 HBD", - "depth": 5, - "json_metadata": "{\"tags\":[\"stats\"]}", - "last_payout": "2016-10-16T14:02:36", - "last_update": "2016-09-15T14:11:09", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "arcange", - "parent_permlink": "re-gtg-re-arcange-re-gtg-re-arcange-steemsql-com-a-deep-analysis-of-steemians-gratefulness-20160915t132416244z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-arcange-re-gtg-re-arcange-re-gtg-re-arcange-steemsql-com-a-deep-analysis-of-steemians-gratefulness-20160915t141107191z", - "post_id": 1254260, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "[STEEMSQL] A deep analysis of Steemians gratefulness", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/stats/@arcange/steemsql-com-a-deep-analysis-of-steemians-gratefulness#@gtg/re-arcange-re-gtg-re-arcange-re-gtg-re-arcange-steemsql-com-a-deep-analysis-of-steemians-gratefulness-20160915t141107191z" - }, - "entry_id": 48, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 2247042714470, - "rshares": 16249222691, - "voter": "brendio" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "I noticed that just after writing a comment (when went back to continue reading ... ) ___Thank you___ for pointing out. :-)", - "body_length": 123, - "cashout_time": "1969-12-31T23:59:59", - "category": "stats", - "children": 2, - "created": "2016-09-15T13:19:24", - "curator_payout_value": "0.000 HBD", - "depth": 3, - "json_metadata": "{\"tags\":[\"stats\"]}", - "last_payout": "2016-10-16T14:02:36", - "last_update": "2016-09-15T13:19:24", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 16249222691, - "parent_author": "arcange", - "parent_permlink": "re-gtg-re-arcange-steemsql-com-a-deep-analysis-of-steemians-gratefulness-20160915t130619700z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-arcange-re-gtg-re-arcange-steemsql-com-a-deep-analysis-of-steemians-gratefulness-20160915t131924290z", - "post_id": 1253693, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "[STEEMSQL] A deep analysis of Steemians gratefulness", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/stats/@arcange/steemsql-com-a-deep-analysis-of-steemians-gratefulness#@gtg/re-arcange-re-gtg-re-arcange-steemsql-com-a-deep-analysis-of-steemians-gratefulness-20160915t131924290z" - }, - "entry_id": 47, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "@arcange, please do not underestimate ___\"Thank you\"___ while searching for ___\"Thanks\"___ ;-)\n\nThank you,\n[Gandalf](https://steemit.com/@gtg) (\"gtg\")", - "body_length": 150, - "cashout_time": "1969-12-31T23:59:59", - "category": "stats", - "children": 4, - "created": "2016-09-15T13:04:12", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"stats\"],\"users\":[\"arcange\"],\"links\":[\"https://steemit.com/@gtg\"]}", - "last_payout": "2016-10-16T14:02:36", - "last_update": "2016-09-15T13:08:12", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "arcange", - "parent_permlink": "steemsql-com-a-deep-analysis-of-steemians-gratefulness", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-arcange-steemsql-com-a-deep-analysis-of-steemians-gratefulness-20160915t130410195z", - "post_id": 1253559, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "[STEEMSQL] A deep analysis of Steemians gratefulness", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/stats/@arcange/steemsql-com-a-deep-analysis-of-steemians-gratefulness#@gtg/re-arcange-steemsql-com-a-deep-analysis-of-steemians-gratefulness-20160915t130410195z" - }, - "entry_id": 46, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 104734123628606, - "rshares": 1046252244, - "voter": "gamer00" - }, - { - "percent": "10000", - "reputation": 1098231391901, - "rshares": 0, - "voter": "ambyr00" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Jaro, welcome to Steemit! :-)", - "body_length": 35, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 1, - "created": "2016-09-15T07:19:03", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"]}", - "last_payout": "2016-10-16T04:09:03", - "last_update": "2016-09-15T07:19:03", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 1046252244, - "parent_author": "gamer00", - "parent_permlink": "hi-i-m-jaro-and-this-is-my-introductory-verification-post", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-gamer00-hi-i-m-jaro-and-this-is-my-introductory-verification-post-20160915t071902704z", - "post_id": 1251608, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hi! I'm Jaro and this is my introductory verification post!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@gamer00/hi-i-m-jaro-and-this-is-my-introductory-verification-post#@gtg/re-gamer00-hi-i-m-jaro-and-this-is-my-introductory-verification-post-20160915t071902704z" - }, - "entry_id": 45, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Joanna, welcome to Steemit! :-)", - "body_length": 37, - "cashout_time": "1969-12-31T23:59:59", - "category": "introducemyself", - "children": 1, - "created": "2016-09-15T07:14:45", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introducemyself\"]}", - "last_payout": "2016-10-16T01:44:27", - "last_update": "2016-09-15T07:14:45", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "woman-onthe-wing", - "parent_permlink": "introduction-post-gold-at-the-end-of-the-rainbow-on-the-emerald-isle", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-woman-onthe-wing-introduction-post-gold-at-the-end-of-the-rainbow-on-the-emerald-isle-20160915t071444722z", - "post_id": 1251589, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Introduction post - Gold at the end of the rainbow... On the Emerald Isle", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introducemyself/@woman-onthe-wing/introduction-post-gold-at-the-end-of-the-rainbow-on-the-emerald-isle#@gtg/re-woman-onthe-wing-introduction-post-gold-at-the-end-of-the-rainbow-on-the-emerald-isle-20160915t071444722z" - }, - "entry_id": 44, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Eleanor, welcome to steemit! :-)", - "body_length": 38, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-09-07T18:54:48", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-10-08T14:02:03", - "last_update": "2016-09-07T18:54:48", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "eleanorp", - "parent_permlink": "the-best-adventures-start-with-rabbit-holes-eleanor", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-eleanorp-the-best-adventures-start-with-rabbit-holes-eleanor-20160907t185449429z", - "post_id": 1162356, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "The Best Adventures Start With Rabbit Holes... -Eleanor", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@eleanorp/the-best-adventures-start-with-rabbit-holes-eleanor#@gtg/re-eleanorp-the-best-adventures-start-with-rabbit-holes-eleanor-20160907t185449429z" - }, - "entry_id": 43, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Lara, hello Orange, welcome to steemit! :-)", - "body_length": 49, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-09-07T18:48:27", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-10-08T18:23:36", - "last_update": "2016-09-07T18:48:27", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "lara2016", - "parent_permlink": "my-favorite-cat", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-lara2016-my-favorite-cat-20160907t184824412z", - "post_id": 1162277, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "MY FAVORITE CAT!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@lara2016/my-favorite-cat#@gtg/re-lara2016-my-favorite-cat-20160907t184824412z" - }, - "entry_id": 42, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 7603297689867, - "rshares": 327852156, - "voter": "dan-wilson" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @dan-wilson, welcome to steemit!\nDavida and Bowie are so cute :-)", - "body_length": 71, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-09-07T18:41:00", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"],\"users\":[\"dan-wilson\"]}", - "last_payout": "2016-10-08T15:54:54", - "last_update": "2016-09-07T18:41:00", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 327852156, - "parent_author": "dan-wilson", - "parent_permlink": "i-m-an-english-teacher-in-beijing-my-introduction-to-steemit-and-verification", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-dan-wilson-i-m-an-english-teacher-in-beijing-my-introduction-to-steemit-and-verification-20160907t184055495z", - "post_id": 1162192, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "I'm an English teacher in Beijing. My Introduction to Steemit & Verification", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@dan-wilson/i-m-an-english-teacher-in-beijing-my-introduction-to-steemit-and-verification#@gtg/re-dan-wilson-i-m-an-english-teacher-in-beijing-my-introduction-to-steemit-and-verification-20160907t184055495z" - }, - "entry_id": 41, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @cwbrooch, welcome to steemit! :-)\n_\"Cat Lover\"_ sounds like an upvote! ;-)", - "body_length": 81, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-09-04T09:30:09", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"],\"users\":[\"cwbrooch\"]}", - "last_payout": "2016-10-05T01:34:12", - "last_update": "2016-09-04T09:30:09", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "cwbrooch", - "parent_permlink": "teacher-story-teller-cat-lover-about-me", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-cwbrooch-teacher-story-teller-cat-lover-about-me-20160904t093005596z", - "post_id": 1120692, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Teacher. Story teller. Cat Lover. - about me :)", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@cwbrooch/teacher-story-teller-cat-lover-about-me#@gtg/re-cwbrooch-teacher-story-teller-cat-lover-about-me-20160904t093005596z" - }, - "entry_id": 40, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Alex, welcome to steemit! :-)", - "body_length": 35, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-08-31T20:57:24", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-10-01T19:22:30", - "last_update": "2016-08-31T20:57:24", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "amcq", - "parent_permlink": "hello-steemit-i-m-alex-a-new-member-of-the-steemit-team", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-amcq-hello-steemit-i-m-alex-a-new-member-of-the-steemit-team-20160831t205719836z", - "post_id": 1076078, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hello Steemit! I'm Alex, a new member of the Steemit team", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@amcq/hello-steemit-i-m-alex-a-new-member-of-the-steemit-team#@gtg/re-amcq-hello-steemit-i-m-alex-a-new-member-of-the-steemit-team-20160831t205719836z" - }, - "entry_id": 39, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Right, now I remember you, @djm34 from #mining :-) Good luck with getting a bounty!", - "body_length": 83, - "cashout_time": "1969-12-31T23:59:59", - "category": "steem", - "children": 0, - "created": "2016-08-30T18:47:33", - "curator_payout_value": "0.000 HBD", - "depth": 4, - "json_metadata": "{\"tags\":[\"mining\",\"steem\"],\"users\":[\"djm34\"]}", - "last_payout": "2016-09-30T17:29:27", - "last_update": "2016-08-30T18:47:33", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "djm34", - "parent_permlink": "re-gtg-re-djm34-re-picokernel-bounty-community-bounty-for-open-source-gpu-miner-20160830t170611218z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-djm34-re-gtg-re-djm34-re-picokernel-bounty-community-bounty-for-open-source-gpu-miner-20160830t184729782z", - "post_id": 1059888, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "[Bounty] Community bounty for open source GPU miner.", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/steem/@picokernel/bounty-community-bounty-for-open-source-gpu-miner#@gtg/re-djm34-re-gtg-re-djm34-re-picokernel-bounty-community-bounty-for-open-source-gpu-miner-20160830t184729782z" - }, - "entry_id": 38, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 19582509873969, - "rshares": 79431791489, - "voter": "derekareith" - }, - { - "percent": "10000", - "reputation": 17498320798649, - "rshares": 12165573676, - "voter": "picokernel" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "@djm34 if you don't trust @picokernel (no need to) you are free to make your own statement that you would send some amount directly to a winner. Anyway as you can see in comments, @complexring, well known and reputable witness already said to donate his 250 SBD as a part of the bounty.", - "body_length": 286, - "cashout_time": "1969-12-31T23:59:59", - "category": "steem", - "children": 6, - "created": "2016-08-30T16:11:12", - "curator_payout_value": "0.000 HBD", - "depth": 2, - "json_metadata": "{\"tags\":[\"steem\"],\"users\":[\"djm34\",\"picokernel\",\"complexring\"]}", - "last_payout": "2016-09-30T17:29:27", - "last_update": "2016-08-30T16:11:12", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 91597365165, - "parent_author": "djm34", - "parent_permlink": "re-picokernel-bounty-community-bounty-for-open-source-gpu-miner-20160830t155929474z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-djm34-re-picokernel-bounty-community-bounty-for-open-source-gpu-miner-20160830t161109296z", - "post_id": 1057818, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "[Bounty] Community bounty for open source GPU miner.", - "title": "", - "total_payout_value": "0.037 HBD", - "url": "/steem/@picokernel/bounty-community-bounty-for-open-source-gpu-miner#@gtg/re-djm34-re-picokernel-bounty-community-bounty-for-open-source-gpu-miner-20160830t161109296z" - }, - "entry_id": 37, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @emsenn, welcome to steemit! :-)", - "body_length": 38, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 1, - "created": "2016-08-29T21:35:24", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"],\"users\":[\"emsenn\"]}", - "last_payout": "2016-09-29T22:31:27", - "last_update": "2016-08-29T21:35:24", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "emsenn", - "parent_permlink": "introducing-myself-bitcoin-early-adopter-content-producer-and-designer", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-emsenn-introducing-myself-bitcoin-early-adopter-content-producer-and-designer-20160829t213519226z", - "post_id": 1047293, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Introducing Myself: Bitcoin Early Adopter, Content Producer & Designer", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@emsenn/introducing-myself-bitcoin-early-adopter-content-producer-and-designer#@gtg/re-emsenn-introducing-myself-bitcoin-early-adopter-content-producer-and-designer-20160829t213519226z" - }, - "entry_id": 36, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 194260219749, - "rshares": 433291510, - "voter": "chamviet" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Lana, welcome to steemit! :-)", - "body_length": 35, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-08-29T21:24:18", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-09-30T02:28:06", - "last_update": "2016-08-29T21:24:18", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 433291510, - "parent_author": "gusevacvetana", - "parent_permlink": "hello-steemit-verification", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-gusevacvetana-hello-steemit-verification-20160829t212412908z", - "post_id": 1047117, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hello, Steemit!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@gusevacvetana/hello-steemit-verification#@gtg/re-gusevacvetana-hello-steemit-verification-20160829t212412908z" - }, - "entry_id": 35, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @artywah, welcome to steemit! :-)\nHint: Adding a picture to your post could make it more appealing.\n( I used my cat for that ;-) )", - "body_length": 136, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-08-29T19:19:18", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"],\"users\":[\"artywah\"]}", - "last_payout": "2016-09-29T18:28:06", - "last_update": "2016-08-29T19:19:18", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "artywah", - "parent_permlink": "self-proclaimed-geek-and-digital-ancient-in-melbourne-australia", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-artywah-self-proclaimed-geek-and-digital-ancient-in-melbourne-australia-20160829t191915549z", - "post_id": 1045369, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Self-proclaimed geek and digital ancient in Melbourne, Australia", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@artywah/self-proclaimed-geek-and-digital-ancient-in-melbourne-australia#@gtg/re-artywah-self-proclaimed-geek-and-digital-ancient-in-melbourne-australia-20160829t191915549z" - }, - "entry_id": 34, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "We have temperate-continental climate. The average annual temperature within last ten years was 8.8\u00b0C (-1.5\u00b0C in Jan to 18\u00b0C in Jul) and yearly rainfall at 600mm. No lemons, but wonderful cherries! :-)", - "body_length": 201, - "cashout_time": "1969-12-31T23:59:59", - "category": "food", - "children": 1, - "created": "2016-08-29T07:09:06", - "curator_payout_value": "0.000 HBD", - "depth": 3, - "json_metadata": "{\"tags\":[\"food\"]}", - "last_payout": "2016-09-26T08:08:21", - "last_update": "2016-08-29T07:09:06", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "lilmisjenn", - "parent_permlink": "re-gtg-re-lilmisjenn-do-you-have-a-drinking-problem-20160828t114559502z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-lilmisjenn-re-gtg-re-lilmisjenn-do-you-have-a-drinking-problem-20160829t070902349z", - "post_id": 1038181, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Do you have a drinking problem??", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/food/@lilmisjenn/do-you-have-a-drinking-problem#@gtg/re-lilmisjenn-re-gtg-re-lilmisjenn-do-you-have-a-drinking-problem-20160829t070902349z" - }, - "entry_id": 33, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 4848564899135, - "rshares": 225048455, - "voter": "fiona777" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Ekaterina, welcome to steemit! :-)", - "body_length": 40, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-08-27T19:38:03", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-09-27T18:50:42", - "last_update": "2016-08-27T19:38:03", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 225048455, - "parent_author": "fiona777", - "parent_permlink": "the-unshakable-faith-in-happy-future-for-the-disabled-or-how-to-become-independent-in-spite-of-the-limited-possibilities", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-fiona777-the-unshakable-faith-in-happy-future-for-the-disabled-or-how-to-become-independent-in-spite-of-the-limited-possibilities-20160827t193800227z", - "post_id": 1017158, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "The unshakable faith in happy future for the disabled or how to become independent in spite of the limited possibilities", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@fiona777/the-unshakable-faith-in-happy-future-for-the-disabled-or-how-to-become-independent-in-spite-of-the-limited-possibilities#@gtg/re-fiona777-the-unshakable-faith-in-happy-future-for-the-disabled-or-how-to-become-independent-in-spite-of-the-limited-possibilities-20160827t193800227z" - }, - "entry_id": 32, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Please use your **active private key** for a miner, instead your owner key.", - "body_length": 75, - "cashout_time": "1969-12-31T23:59:59", - "category": "mining", - "children": 0, - "created": "2016-08-27T18:39:45", - "curator_payout_value": "0.000 HBD", - "depth": 4, - "json_metadata": "{\"tags\":[\"mining\"]}", - "last_payout": "2016-09-21T13:01:45", - "last_update": "2016-08-27T18:39:45", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "timcliff", - "parent_permlink": "re-gtg-re-timcliff-re-gtg-missing-rewards-while-mining-20160827t155116406z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-timcliff-re-gtg-re-timcliff-re-gtg-missing-rewards-while-mining-20160827t183943508z", - "post_id": 1016368, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Missing rewards while mining - common mistake with keys", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/mining/@gtg/missing-rewards-while-mining#@gtg/re-timcliff-re-gtg-re-timcliff-re-gtg-missing-rewards-while-mining-20160827t183943508z" - }, - "entry_id": 31, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "yes, you can want to use [steemd.com/@youraccount](https://steemd.com) link to check witness details (at the time of generating block your miner become witness)", - "body_length": 160, - "cashout_time": "1969-12-31T23:59:59", - "category": "mining", - "children": 2, - "created": "2016-08-27T15:38:00", - "curator_payout_value": "0.000 HBD", - "depth": 2, - "json_metadata": "{\"tags\":[\"mining\"],\"links\":[\"https://steemd.com\"]}", - "last_payout": "2016-09-21T13:01:45", - "last_update": "2016-08-27T15:38:00", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "timcliff", - "parent_permlink": "re-gtg-missing-rewards-while-mining-20160827t141319137z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-timcliff-re-gtg-missing-rewards-while-mining-20160827t153756820z", - "post_id": 1014083, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Missing rewards while mining - common mistake with keys", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/mining/@gtg/missing-rewards-while-mining#@gtg/re-timcliff-re-gtg-missing-rewards-while-mining-20160827t153756820z" - }, - "entry_id": 30, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "I was 'always' ***Gandalf*** (as I mentioned in my [introduction](https://steemit.com/introduceyourself/@gtg/hello-world)). Unfortunately, on steem that id was already taken, so I used ***gtg*** initials (as in ***G**andalf **t**he **G**rey*).\nOn [steemit.chat](https://steemit.chat) I'm ***Gandalf*** (join us there if you have some spare time :-))\nJust recently, thanks to kindness of @masteryoda and @joseph I was able to finally get @gandalf account on steemit!", - "body_length": 465, - "cashout_time": "1969-12-31T23:59:59", - "category": "technology", - "children": 0, - "created": "2016-08-27T14:59:00", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"technology\"],\"users\":[\"masteryoda\",\"joseph\",\"gandalf\"],\"links\":[\"https://steemit.com/introduceyourself/@gtg/hello-world\"]}", - "last_payout": "2016-09-27T07:33:12", - "last_update": "2016-08-27T14:59:00", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "mynomadicyear", - "parent_permlink": "what-was-your-first-screen-name", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-mynomadicyear-what-was-your-first-screen-name-20160827t145857348z", - "post_id": 1013651, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "What Was Your First Screen Name?", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/technology/@mynomadicyear/what-was-your-first-screen-name#@gtg/re-mynomadicyear-what-was-your-first-screen-name-20160827t145857348z" - }, - "entry_id": 29, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Consider joining [steemit.chat](https://steemit.chat/). You can get there some help on channel #help or promote your posts on #postpromotion related channels. Curate newcomers on #introduceyourself or just have some fun on #general.", - "body_length": 232, - "cashout_time": "1969-12-31T23:59:59", - "category": "aboutme", - "children": 0, - "created": "2016-08-27T12:10:33", - "curator_payout_value": "0.000 HBD", - "depth": 3, - "json_metadata": "{\"tags\":[\"help\",\"postpromotion\",\"introduceyourself\",\"general\",\"aboutme\"],\"links\":[\"https://steemit.chat/\"]}", - "last_payout": "2016-09-26T09:23:57", - "last_update": "2016-08-27T12:10:33", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "eternalmoon", - "parent_permlink": "re-gtg-re-eternalmoon-this-is-my-about-me-entry-enjoy-20160827t041549494z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-eternalmoon-re-gtg-re-eternalmoon-this-is-my-about-me-entry-enjoy-20160827t121030091z", - "post_id": 1011967, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "This is my about me entry (: Enjoy~", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/aboutme/@eternalmoon/this-is-my-about-me-entry-enjoy#@gtg/re-eternalmoon-re-gtg-re-eternalmoon-this-is-my-about-me-entry-enjoy-20160827t121030091z" - }, - "entry_id": 28, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 56150216349, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello @skypilot, welcome to steemit! I had no time to post a comment before, but when you get back to [steemit.chat](https://steemit.chat) you get an idea why ;-)\nI'm looking forward for your posts.\nGood luck!\n\nPS\nI encourage all of you to take a look from time to time at #introduceyourself category. Not at the top stories. Look at those that are earning a $0 or a bit less. Sometimes you might be really surprised about your findings.", - "body_length": 437, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-08-27T11:14:45", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"],\"users\":[\"skypilot\"],\"links\":[\"https://steemit.chat\"]}", - "last_payout": "2016-09-27T01:41:27", - "last_update": "2016-08-27T11:14:45", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 56150216349, - "parent_author": "skypilot", - "parent_permlink": "my-first-real-post-was-a-success-and-i-would-like-to-share-the-story", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-skypilot-my-first-real-post-was-a-success-and-i-would-like-to-share-the-story-20160827t111440904z", - "post_id": 1011532, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "My first real post was a success and I would like to share the story.", - "title": "", - "total_payout_value": "0.028 HBD", - "url": "/introduceyourself/@skypilot/my-first-real-post-was-a-success-and-i-would-like-to-share-the-story#@gtg/re-skypilot-my-first-real-post-was-a-success-and-i-would-like-to-share-the-story-20160827t111440904z" - }, - "entry_id": 27, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 1455868290913, - "rshares": 10058409961, - "voter": "johnerfx" - }, - { - "percent": "10000", - "reputation": 45020858639, - "rshares": 1067633479, - "voter": "johnerminer" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Ania, welcome to steemit! :-)", - "body_length": 35, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 1, - "created": "2016-08-26T18:53:09", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-09-26T21:18:18", - "last_update": "2016-08-26T18:53:09", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 11126043440, - "parent_author": "ania", - "parent_permlink": "hello-world", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-ania-hello-world-20160826t185305711z", - "post_id": 1002047, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hello World!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@ania/hello-world#@gtg/re-ania-hello-world-20160826t185305711z" - }, - "entry_id": 26, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello seasoul, welcome to steemit! :-)", - "body_length": 38, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 1, - "created": "2016-08-26T09:53:24", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-09-25T19:26:21", - "last_update": "2016-08-26T09:53:24", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "seasoul", - "parent_permlink": "aboutme", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-seasoul-aboutme-20160826t095320794z", - "post_id": 995454, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Aboutme", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@seasoul/aboutme#@gtg/re-seasoul-aboutme-20160826t095320794z" - }, - "entry_id": 25, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Samantha, welcome to steemit! :-)\nAdding a picture to your post could make it more appealing.\n( I used my cat for that ;-) )", - "body_length": 130, - "cashout_time": "1969-12-31T23:59:59", - "category": "aboutme", - "children": 2, - "created": "2016-08-26T09:48:39", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"aboutme\"]}", - "last_payout": "2016-09-26T09:23:57", - "last_update": "2016-08-26T09:48:39", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "eternalmoon", - "parent_permlink": "this-is-my-about-me-entry-enjoy", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-eternalmoon-this-is-my-about-me-entry-enjoy-20160826t094835368z", - "post_id": 995412, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "This is my about me entry (: Enjoy~", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/aboutme/@eternalmoon/this-is-my-about-me-entry-enjoy#@gtg/re-eternalmoon-this-is-my-about-me-entry-enjoy-20160826t094835368z" - }, - "entry_id": 24, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Bri, welcome to steemit! :-)\nOut of curiosity: how did you get here?", - "body_length": 74, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 1, - "created": "2016-08-26T09:43:09", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-09-26T08:05:57", - "last_update": "2016-08-26T09:43:09", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "bumblebrii", - "parent_permlink": "about-me", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-bumblebrii-about-me-20160826t094306616z", - "post_id": 995370, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "About me", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@bumblebrii/about-me#@gtg/re-bumblebrii-about-me-20160826t094306616z" - }, - "entry_id": 23, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Daniel, welcome to steemit! :-) Nice introduction and interesting thoughts. Solving (2) is most important IMO, and tied to (1) and (3). Easiest way to test (2) is by trying to convince your non-technical friend to join.", - "body_length": 225, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-08-26T09:35:21", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-09-26T05:41:39", - "last_update": "2016-08-26T09:35:21", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "daniel31oh", - "parent_permlink": "what-in-the-world-have-i-stumbled-upon-a-quick-assessment-and-some-concerns", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-daniel31oh-what-in-the-world-have-i-stumbled-upon-a-quick-assessment-and-some-concerns-20160826t093519227z", - "post_id": 995305, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "What In the World Have I Stumbled Upon?! A Quick Assessment and Some Concerns", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@daniel31oh/what-in-the-world-have-i-stumbled-upon-a-quick-assessment-and-some-concerns#@gtg/re-daniel31oh-what-in-the-world-have-i-stumbled-upon-a-quick-assessment-and-some-concerns-20160826t093519227z" - }, - "entry_id": 22, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 6394420287, - "rshares": 0, - "voter": "indomitable" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello CT Jaynes, welcome to steemit! :-)\nAdding a picture to your post could make it more appealing.\n( I used my cat for that ;-) )", - "body_length": 131, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 1, - "created": "2016-08-26T09:16:06", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-09-26T09:10:03", - "last_update": "2016-08-26T09:16:06", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "indomitable", - "parent_permlink": "hello-i-am-ct-jaynes", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-indomitable-hello-i-am-ct-jaynes-20160826t091604543z", - "post_id": 995154, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hello, I am CT Jaynes.", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@indomitable/hello-i-am-ct-jaynes#@gtg/re-indomitable-hello-i-am-ct-jaynes-20160826t091604543z" - }, - "entry_id": 21, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Diondra, welcome to steemit :-)", - "body_length": 37, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-08-26T09:11:33", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-09-26T06:50:15", - "last_update": "2016-08-26T09:11:33", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "dakini-dion", - "parent_permlink": "let-me-introduce-myself-entering-dakin-dion", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-dakini-dion-let-me-introduce-myself-entering-dakin-dion-20160826t091128267z", - "post_id": 995119, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Let me introduce myself: Entering Dakin-Dion!", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@dakini-dion/let-me-introduce-myself-entering-dakin-dion#@gtg/re-dakini-dion-let-me-introduce-myself-entering-dakin-dion-20160826t091128267z" - }, - "entry_id": 20, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Ksenia, welcome to steemit :-)", - "body_length": 36, - "cashout_time": "1969-12-31T23:59:59", - "category": "travel", - "children": 1, - "created": "2016-08-26T09:08:15", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"travel\"]}", - "last_payout": "2016-09-26T16:08:51", - "last_update": "2016-08-26T09:08:15", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "mynomadicyear", - "parent_permlink": "16-months-out-of-a-suitcase", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-mynomadicyear-16-months-out-of-a-suitcase-20160826t090811525z", - "post_id": 995095, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "16 Months Out Of A Suitcase", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/travel/@mynomadicyear/16-months-out-of-a-suitcase#@gtg/re-mynomadicyear-16-months-out-of-a-suitcase-20160826t090811525z" - }, - "entry_id": 19, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 1871386359293, - "rshares": 1723711669, - "voter": "lilmisjenn" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Just water is fine. Sometimes I add a squeeze of lemon, but I'm not that lucky to get it from own garden, no chance for it to grow in our climate.\n\nWelcome to steemit :-)", - "body_length": 170, - "cashout_time": "1969-12-31T23:59:59", - "category": "food", - "children": 3, - "created": "2016-08-26T08:59:00", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"food\"]}", - "last_payout": "2016-09-26T08:08:21", - "last_update": "2016-08-26T08:59:00", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 1723711669, - "parent_author": "lilmisjenn", - "parent_permlink": "do-you-have-a-drinking-problem", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-lilmisjenn-do-you-have-a-drinking-problem-20160826t085857261z", - "post_id": 995039, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Do you have a drinking problem??", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/food/@lilmisjenn/do-you-have-a-drinking-problem#@gtg/re-lilmisjenn-do-you-have-a-drinking-problem-20160826t085857261z" - }, - "entry_id": 18, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Melissa, welcome to steemit :-)\nAdding a picture to your post could make it more appealing.\n( I used my cat for that ;-) )", - "body_length": 128, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 1, - "created": "2016-08-26T08:39:45", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-09-26T08:42:15", - "last_update": "2016-08-26T08:39:45", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "melissarhiann", - "parent_permlink": "lasting-impression", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-melissarhiann-lasting-impression-20160826t083943073z", - "post_id": 994931, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Lasting Impression", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@melissarhiann/lasting-impression#@gtg/re-melissarhiann-lasting-impression-20160826t083943073z" - }, - "entry_id": 17, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello Zee, welcome to steemit :-)", - "body_length": 33, - "cashout_time": "1969-12-31T23:59:59", - "category": "zee", - "children": 1, - "created": "2016-08-26T08:06:09", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"zee\"]}", - "last_payout": "2016-09-27T01:07:09", - "last_update": "2016-08-26T08:06:09", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "orcish", - "parent_permlink": "my-introduction", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-orcish-my-introduction-20160826t080607987z", - "post_id": 994681, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "My Introduction", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/zee/@orcish/my-introduction#@gtg/re-orcish-my-introduction-20160826t080607987z" - }, - "entry_id": 16, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "That's brave. Welcome (back) to the light side of the Steem! :-)", - "body_length": 64, - "cashout_time": "1969-12-31T23:59:59", - "category": "introduceyourself", - "children": 0, - "created": "2016-08-25T18:51:12", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\"]}", - "last_payout": "2016-09-25T01:23:36", - "last_update": "2016-08-25T18:51:12", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "ltndd1", - "parent_permlink": "re-introducing-myself-the-real-me", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-ltndd1-re-introducing-myself-the-real-me-20160825t185109827z", - "post_id": 985630, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Re-Introducing Myself...The Real Me", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/introduceyourself/@ltndd1/re-introducing-myself-the-real-me#@gtg/re-ltndd1-re-introducing-myself-the-real-me-20160825t185109827z" - }, - "entry_id": 15, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 237638942804685, - "rshares": 347121336514, - "voter": "ash" - }, - { - "percent": "10000", - "reputation": 45020858639, - "rshares": 1015832036, - "voter": "johnerminer" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 48992052363, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 1136133804448921, - "rshares": 23149067557, - "voter": "firepower" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "I am keeping an eye on #introduceyourself channel, as a place where newcomers can promote their introduction posts without being flooded by other random links.\nRules are simple: post link to your introduction, talk with other newcomers. When you are no longer newcomer, curate others, encourage them to enjoy this platform.\n\n_**introduce:**_\n> to present to a particular group of individuals\n> or to the general public for or as if **for the first time** by a formal act\n\nplease, do not abuse this tag or channel\n\n**gandalf** on steemit.chat", - "body_length": 541, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit-chat", - "children": 0, - "created": "2016-08-21T14:30:51", - "curator_payout_value": "0.026 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"introduceyourself\",\"steemit-chat\"]}", - "last_payout": "2016-09-21T01:08:09", - "last_update": "2016-08-21T14:30:51", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 420278288470, - "parent_author": "ash", - "parent_permlink": "steemit-chat-stats-and-rules", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-ash-steemit-chat-stats-and-rules-20160821t143046855z", - "post_id": 920536, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Steemit.chat - Stats & Rules", - "title": "", - "total_payout_value": "0.251 HBD", - "url": "/steemit-chat/@ash/steemit-chat-stats-and-rules#@gtg/re-ash-steemit-chat-stats-and-rules-20160821t143046855z" - }, - "entry_id": 14, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Make sure you are not making common mistake with keys: https://steemit.com/mining/@gtg/missing-rewards-while-mining", - "body_length": 115, - "cashout_time": "1969-12-31T23:59:59", - "category": "steem", - "children": 0, - "created": "2016-08-21T14:00:42", - "curator_payout_value": "0.000 HBD", - "depth": 2, - "json_metadata": "{\"tags\":[\"steem\"],\"links\":[\"https://steemit.com/mining/@gtg/missing-rewards-while-mining\"]}", - "last_payout": "2016-09-18T15:02:36", - "last_update": "2016-08-21T14:00:42", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "bola", - "parent_permlink": "re-milaoz-what-happened-to-the-mining-steem-after-08-15-2016-20160818t144845140z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-bola-re-milaoz-what-happened-to-the-mining-steem-after-08-15-2016-20160821t140040803z", - "post_id": 920218, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "What happened to the Mining STEEM after 08/15/2016 ???", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/steem/@milaoz/what-happened-to-the-mining-steem-after-08-15-2016#@gtg/re-bola-re-milaoz-what-happened-to-the-mining-steem-after-08-15-2016-20160821t140040803z" - }, - "entry_id": 13, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "I encourage miners to visit [Steemit Chat](https://steemit.chat/channel/mining \"#mining\") where you can find help and share your experience.", - "body_length": 140, - "cashout_time": "1969-12-31T23:59:59", - "category": "mining", - "children": 0, - "created": "2016-08-21T13:12:45", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"mining\"],\"links\":[\"https://steemit.chat/channel/mining\"]}", - "last_payout": "2016-09-21T13:01:45", - "last_update": "2016-08-21T13:12:45", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 0, - "parent_author": "gtg", - "parent_permlink": "missing-rewards-while-mining", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-gtg-missing-rewards-while-mining-20160821t131242531z", - "post_id": 919652, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Missing rewards while mining - common mistake with keys", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/mining/@gtg/missing-rewards-while-mining#@gtg/re-gtg-missing-rewards-while-mining-20160821t131242531z" - }, - "entry_id": 12, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 1455868290913, - "rshares": 9975325205, - "voter": "johnerfx" - }, - { - "percent": "10000", - "reputation": 45020858639, - "rshares": 848238359, - "voter": "johnerminer" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 48835908327, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "There's nothing wrong with mining using your _\"steemit.com account\"_. It's **all about your keys** and using **proper miner configuration**. I tried to explain common mistake https://steemit.com/mining/@gtg/missing-rewards-while-mining", - "body_length": 235, - "cashout_time": "1969-12-31T23:59:59", - "category": "mining", - "children": 0, - "created": "2016-08-21T12:33:57", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"mining\"],\"links\":[\"https://steemit.com/mining/@gtg/missing-rewards-while-mining\"]}", - "last_payout": "2016-09-21T10:51:00", - "last_update": "2016-08-21T12:33:57", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 59659471891, - "parent_author": "artakan", - "parent_permlink": "important-info-for-steem-miner-do-not-use-your-steemit-com-account", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-artakan-important-info-for-steem-miner-do-not-use-your-steemit-com-account-20160821t123356072z", - "post_id": 919361, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "[Solved] Important info for STEEM miners, Steemit.com accounts (coming from facebook or reddit) seem to loose the 'Generated blocks'", - "title": "", - "total_payout_value": "0.039 HBD", - "url": "/mining/@artakan/important-info-for-steem-miner-do-not-use-your-steemit-com-account#@gtg/re-artakan-important-info-for-steem-miner-do-not-use-your-steemit-com-account-20160821t123356072z" - }, - "entry_id": 11, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 4402236225514, - "rshares": 93765183, - "voter": "gandalf" - }, - { - "percent": "10000", - "reputation": 11554252869567, - "rshares": 0, - "voter": "proctologic" - }, - { - "percent": "10000", - "reputation": 96813524550, - "rshares": 503120540, - "voter": "paco-steem" - }, - { - "percent": "10000", - "reputation": 377179304234, - "rshares": 5941196935, - "voter": "spaninv" - }, - { - "percent": "10000", - "reputation": 1455868290913, - "rshares": 9975325205, - "voter": "johnerfx" - }, - { - "percent": "10000", - "reputation": 11782050148677, - "rshares": 382763916645, - "voter": "taoteh1221" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 393291314, - "voter": "stiletto" - }, - { - "percent": "10000", - "reputation": 45020858639, - "rshares": 867516503, - "voter": "johnerminer" - }, - { - "percent": "10000", - "reputation": 1240582110360, - "rshares": 2480411371, - "voter": "gary-smith" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 49812626493, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 1800578928408, - "rshares": 7921157988, - "voter": "dasha" - }, - { - "percent": "10000", - "reputation": -14070504625313, - "rshares": 30256483332, - "voter": "r4fken" - }, - { - "percent": "10000", - "reputation": 7952828943, - "rshares": 4368322380, - "voter": "thegoodguy" - }, - { - "percent": "10000", - "reputation": 10929325193, - "rshares": 1775754972, - "voter": "screasey" - }, - { - "percent": "10000", - "reputation": 2351335125519, - "rshares": 14634652647, - "voter": "artakan" - }, - { - "percent": "10000", - "reputation": 513405006906, - "rshares": 539611572, - "voter": "qonq99" - }, - { - "percent": "10000", - "reputation": 4222042361135, - "rshares": 4923096406, - "voter": "fishborne" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1377860390, - "voter": "steeminer" - }, - { - "percent": "10000", - "reputation": 173944750771740, - "rshares": 726277176, - "voter": "spinner" - }, - { - "percent": "10000", - "reputation": 37533992277122, - "rshares": 16175410131, - "voter": "thebluepanda" - }, - { - "percent": "10000", - "reputation": 73710537156, - "rshares": 2362437240, - "voter": "ullikume" - }, - { - "percent": "10000", - "reputation": 9308068985083, - "rshares": 2988929662, - "voter": "mione" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 851733457, - "voter": "steeminion" - }, - { - "percent": "10000", - "reputation": 282028870728, - "rshares": 0, - "voter": "anatworld" - }, - { - "percent": "10000", - "reputation": 70885672379219, - "rshares": 0, - "voter": "pollux.one" - }, - { - "percent": "10000", - "reputation": 147922815034277, - "rshares": 14814536032, - "voter": "sponge-bob" - }, - { - "percent": "10000", - "reputation": 13772130039901, - "rshares": 512292840, - "voter": "kev7000" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 289407830, - "voter": "steeminnow" - }, - { - "percent": "10000", - "reputation": 3715954427739, - "rshares": 11301045003, - "voter": "brains" - }, - { - "percent": "10000", - "reputation": 60253396, - "rshares": 0, - "voter": "byaritzab" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Suppose your miner node found `pow2`, but your `total_missed` count increased, instead of generating a block.\n\nIf this happens, double check your keys used in the `config.ini` file.\n\n```\nwitness = \"minerwitness\"\n\nminer = [\"minerwitness\",\"WIF_ACTIVE_PRIVATE_KEY\"]\nminer = [\"miner1\",\"WIF_ACTIVE_PRIVATE_KEY\"]\nminer = [\"miner2\",\"WIF_ACTIVE_PRIVATE_KEY\"]\nminer = [\"miner3\",\"WIF_ACTIVE_PRIVATE_KEY\"]\n\nmining-threads = 4\n\nprivate-key = WIF_SIGNING_PRIVATE_KEY\n```\n\nUsing keys without paying attention to their roles is a common mistake. @artakan [found out](https://steemit.com/mining/@artakan/important-info-for-steem-miner-do-not-use-your-steemit-com-account \"@artakan - Do not use your steemit.com account for mining\")\nthat issues with missing blocks tend to happen when you are using an account that was created through [steemit.com](https://steemit.com/ \"Blogging is the new mining\") but seems to work for the mined account.\n\nSo erroneous configuration might work for your mined account by pure coincidence. In other words, the same key has been defined for all roles, so: `WIF_ACTIVE_PRIVATE_KEY` is exactly the same as `WIF_SIGNING_PRIVATE_KEY`.\n\n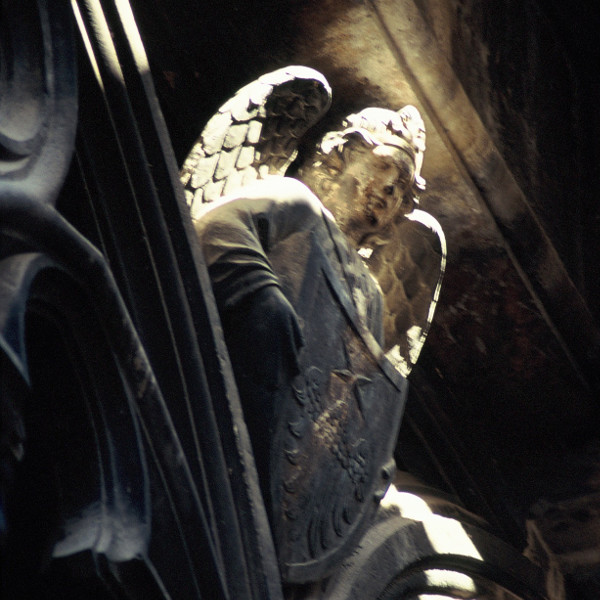\n\nIf you believe this idea is of use and value to Steem, please vote for me as a [witness](https://steemit.com/witness-category/@gtg/witness-gtg \"witness-gtg\")\neither on [Steemit's Witnesses List](https://steemit.com/~witnesses \"Witnesses\") \nor by using your `cli_wallet` command:\n`vote_for_witness \"YOURACCOUNT\" \"gtg\" true true`", - "body_length": 1525, - "cashout_time": "1969-12-31T23:59:59", - "category": "mining", - "children": 7, - "created": "2016-08-21T12:29:18", - "curator_payout_value": "0.079 HBD", - "depth": 0, - "json_metadata": "{\"tags\":[\"mining\",\"steem\",\"steem-mining\"],\"users\":[\"artakan\"],\"links\":[\"https://steemit.com/mining/@artakan/important-info-for-steem-miner-do-not-use-your-steemit-com-account\"]}", - "last_payout": "2016-09-21T13:01:45", - "last_update": "2016-08-21T13:02:30", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 568650179247, - "parent_author": "", - "parent_permlink": "mining", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "missing-rewards-while-mining", - "post_id": 919309, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Missing rewards while mining - common mistake with keys", - "title": "Missing rewards while mining - common mistake with keys", - "total_payout_value": "0.353 HBD", - "url": "/mining/@gtg/missing-rewards-while-mining" - }, - "entry_id": 10, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 7487619572225, - "rshares": 6327963918037, - "voter": "wackou" - }, - { - "percent": "10000", - "reputation": 2750638235966, - "rshares": 19049044601, - "voter": "daowisp" - }, - { - "percent": "10000", - "reputation": 1455868290913, - "rshares": 9559686655, - "voter": "johnerfx" - }, - { - "percent": "10000", - "reputation": 45020858639, - "rshares": 829049571, - "voter": "johnerminer" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 39201460134, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 229959978079725, - "rshares": 19467531428, - "voter": "ausbitbank" - }, - { - "percent": "10000", - "reputation": 140605453893072, - "rshares": 114784244417, - "voter": "jesta" - }, - { - "percent": "10000", - "reputation": 96871175157544, - "rshares": 14083166401, - "voter": "someguy123" - }, - { - "percent": "10000", - "reputation": 17413759953763, - "rshares": 0, - "voter": "yehey" - }, - { - "percent": "10000", - "reputation": -11075113126, - "rshares": 0, - "voter": "masud123" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "It has already been tested in the wild on dedicated servers and VXLAN (however using only a minor block producer, not a full-time witness)\nBig infrastructure providers such as AWS or OVH have plenty usable features that could help (like multi-datacenter private networking), so deploying this won't be hard, but you are not limited to a single infrastructure provider. In more affordable solutions, where you don't have multiple physical network interfaces or multiple outbound routes you can even use OpenVPN, which adds only negligible latency and does its job.", - "body_length": 563, - "cashout_time": "1969-12-31T23:59:59", - "category": "witness-category", - "children": 3, - "created": "2016-08-16T20:18:57", - "curator_payout_value": "2.416 HBD", - "depth": 2, - "json_metadata": "{\"tags\":[\"witness-category\"]}", - "last_payout": "2016-09-16T20:13:00", - "last_update": "2016-08-16T20:18:57", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 6544938101244, - "parent_author": "jesta", - "parent_permlink": "re-gtg-heavy-duty-witness-node-infrastructure-20160816t191107309z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-jesta-re-gtg-heavy-duty-witness-node-infrastructure-20160816t201857176z", - "post_id": 840710, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Heavy duty witness node infrastructure", - "title": "", - "total_payout_value": "7.694 HBD", - "url": "/witness-category/@gtg/heavy-duty-witness-node-infrastructure#@gtg/re-jesta-re-gtg-heavy-duty-witness-node-infrastructure-20160816t201857176z" - }, - "entry_id": 9, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "5500", - "reputation": 240292002602347, - "rshares": 15699644918310, - "voter": "dantheman" - }, - { - "percent": "100", - "reputation": 85602977273, - "rshares": 28854876032, - "voter": "anonymous" - }, - { - "percent": "5000", - "reputation": 8323904861044, - "rshares": 8484864209575, - "voter": "rainman" - }, - { - "percent": "10000", - "reputation": 520836362124341, - "rshares": 37843185317140, - "voter": "blocktrades" - }, - { - "percent": "10000", - "reputation": 43394593255, - "rshares": 2090496972549, - "voter": "steemroller" - }, - { - "percent": "10000", - "reputation": 2110682356890, - "rshares": 74736896239, - "voter": "donaldtrump" - }, - { - "percent": "6600", - "reputation": 57055357664878, - "rshares": 335906168314, - "voter": "modprobe" - }, - { - "percent": "10000", - "reputation": 7487619572225, - "rshares": 6327415081631, - "voter": "wackou" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 33308040478, - "voter": "ihash" - }, - { - "percent": "10000", - "reputation": 358840960832, - "rshares": 1714790303449, - "voter": "rossco99" - }, - { - "percent": "10000", - "reputation": 94312688970406, - "rshares": 912226370971, - "voter": "liondani" - }, - { - "percent": "10000", - "reputation": 82770176676, - "rshares": 2468266694, - "voter": "boy" - }, - { - "percent": "10000", - "reputation": 118819064085695, - "rshares": 1975751593462, - "voter": "xeroc" - }, - { - "percent": "10000", - "reputation": 4712949449, - "rshares": 2995664453, - "voter": "bue-witness" - }, - { - "percent": "10000", - "reputation": 2975000206258, - "rshares": 567206353, - "voter": "bunny" - }, - { - "percent": "100", - "reputation": 62649292215598, - "rshares": 140485443412, - "voter": "complexring" - }, - { - "percent": "10000", - "reputation": 1433162136287, - "rshares": 41823927396, - "voter": "bue" - }, - { - "percent": "10000", - "reputation": 27105921350, - "rshares": 1323151152, - "voter": "mini" - }, - { - "percent": "10000", - "reputation": 41524029136, - "rshares": 170623771, - "voter": "moon" - }, - { - "percent": "10000", - "reputation": 31620973634639, - "rshares": 32232314318, - "voter": "aizensou" - }, - { - "percent": "10000", - "reputation": 1265474677605, - "rshares": 108216927683, - "voter": "bonapartist" - }, - { - "percent": "10000", - "reputation": 4402236225514, - "rshares": 91926650, - "voter": "gandalf" - }, - { - "percent": "10000", - "reputation": 341233778618, - "rshares": 358786763103, - "voter": "boatymcboatface" - }, - { - "percent": "10000", - "reputation": 386160910642701, - "rshares": 297215153038, - "voter": "officialfuzzy" - }, - { - "percent": "10000", - "reputation": 211675309641028, - "rshares": 773689504778, - "voter": "pfunk" - }, - { - "percent": "9700", - "reputation": 87554098144619, - "rshares": 699635263343, - "voter": "cass" - }, - { - "percent": "10000", - "reputation": 11554252869567, - "rshares": 9031234362, - "voter": "proctologic" - }, - { - "percent": "10000", - "reputation": 28222884156, - "rshares": 490844799, - "voter": "healthcare" - }, - { - "percent": "10000", - "reputation": 25973066833, - "rshares": 715096804, - "voter": "daniel.pan" - }, - { - "percent": "10000", - "reputation": 6977247220338, - "rshares": 17603047506, - "voter": "dedriss" - }, - { - "percent": "10000", - "reputation": 86901300608582, - "rshares": 273299516223, - "voter": "chitty" - }, - { - "percent": "10000", - "reputation": 4020329275116, - "rshares": 104430794832, - "voter": "linouxis9" - }, - { - "percent": "10000", - "reputation": 34466536170, - "rshares": 230014747, - "voter": "helen.tan" - }, - { - "percent": "10000", - "reputation": 2750638235966, - "rshares": 19049044601, - "voter": "daowisp" - }, - { - "percent": "10000", - "reputation": 9631183077467, - "rshares": 14623675138, - "voter": "valtr" - }, - { - "percent": "10000", - "reputation": 437808999210623, - "rshares": 116313551950, - "voter": "craig-grant" - }, - { - "percent": "10000", - "reputation": 3828884985898, - "rshares": 60023860440, - "voter": "lovelace" - }, - { - "percent": "10000", - "reputation": 4604092068298, - "rshares": 22731092140, - "voter": "joelinux" - }, - { - "percent": "10000", - "reputation": 1957274366495342, - "rshares": 79387789605, - "voter": "kingscrown" - }, - { - "percent": "10000", - "reputation": 96813524550, - "rshares": 471129748, - "voter": "paco-steem" - }, - { - "percent": "10000", - "reputation": 775562881035, - "rshares": 426310899, - "voter": "ereismatias" - }, - { - "percent": "10000", - "reputation": 377179304234, - "rshares": 5639122835, - "voter": "spaninv" - }, - { - "percent": "10000", - "reputation": -718507041281, - "rshares": 7733788543, - "voter": "nate-atkins" - }, - { - "percent": "10000", - "reputation": 273195968453, - "rshares": 1293680763, - "voter": "karask" - }, - { - "percent": "10000", - "reputation": 4042732628956, - "rshares": 1399620602, - "voter": "coar" - }, - { - "percent": "3301", - "reputation": 8801781140235, - "rshares": 1450957464, - "voter": "murh" - }, - { - "percent": "10000", - "reputation": 3192044915974, - "rshares": 363704119, - "voter": "edtorrez" - }, - { - "percent": "10000", - "reputation": 843234452336, - "rshares": 537523962057, - "voter": "cyber" - }, - { - "percent": "10000", - "reputation": 323603913866384, - "rshares": 14807486260, - "voter": "thecryptofiend" - }, - { - "percent": "10000", - "reputation": 1455868290913, - "rshares": 9767505930, - "voter": "johnerfx" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 368352936, - "voter": "stiletto" - }, - { - "percent": "10000", - "reputation": 78929307854833, - "rshares": 55074207308, - "voter": "juanmiguelsalas" - }, - { - "percent": "10000", - "reputation": 489572657460, - "rshares": 832120526, - "voter": "sunnyray" - }, - { - "percent": "10000", - "reputation": 73173003305, - "rshares": 1021367115, - "voter": "fjccoin" - }, - { - "percent": "10000", - "reputation": 45020858639, - "rshares": 848329794, - "voter": "johnerminer" - }, - { - "percent": "10000", - "reputation": 6945443409938, - "rshares": 44252149522, - "voter": "geoffrey" - }, - { - "percent": "10000", - "reputation": 6433018616216, - "rshares": 84721328962, - "voter": "truthtaco" - }, - { - "percent": "10000", - "reputation": 2457670192450, - "rshares": 636119227, - "voter": "ben99" - }, - { - "percent": "10000", - "reputation": -492606942788, - "rshares": 23438529510, - "voter": "jparty" - }, - { - "percent": "10000", - "reputation": 109424005488868, - "rshares": 688449090, - "voter": "mammasitta" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 39092198167, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 2758500001611, - "rshares": 3985585444, - "voter": "fuck.off" - }, - { - "percent": "10000", - "reputation": 1019011681813, - "rshares": 5429573755, - "voter": "iloveporn" - }, - { - "percent": "10000", - "reputation": 1557874437556, - "rshares": 1940554482, - "voter": "the.bot" - }, - { - "percent": "10000", - "reputation": 2445708956667, - "rshares": 6603022993, - "voter": "johnbradshaw" - }, - { - "percent": "10000", - "reputation": 1691376287481, - "rshares": 4605030334, - "voter": "the.whale" - }, - { - "percent": "10000", - "reputation": 36391001943292, - "rshares": 169187275390, - "voter": "dashpaymag" - }, - { - "percent": "10000", - "reputation": 4335884149720, - "rshares": 261023475510, - "voter": "asmolokalo" - }, - { - "percent": "10000", - "reputation": 352970428645376, - "rshares": 275298167273, - "voter": "roelandp" - }, - { - "percent": "10000", - "reputation": 81983155115382, - "rshares": 2098337565, - "voter": "romanskv" - }, - { - "percent": "10000", - "reputation": 1160886081198, - "rshares": 4363425866, - "voter": "unicornfarts" - }, - { - "percent": "10000", - "reputation": 31794084266065, - "rshares": 6486686814, - "voter": "kakradetome" - }, - { - "percent": "10000", - "reputation": 1136133804448921, - "rshares": 18902778277, - "voter": "firepower" - }, - { - "percent": "10000", - "reputation": 1876603009707, - "rshares": 5644023807, - "voter": "cmtzco" - }, - { - "percent": "10000", - "reputation": 16446258831489, - "rshares": 36679008975, - "voter": "menta" - }, - { - "percent": "10000", - "reputation": 1035952800698, - "rshares": 4605414402, - "voter": "vote" - }, - { - "percent": "10000", - "reputation": 60746824769, - "rshares": 4674772839, - "voter": "manoami" - }, - { - "percent": "10000", - "reputation": 2237331858240, - "rshares": 4848852438, - "voter": "kissmybutt" - }, - { - "percent": "10000", - "reputation": 194260219749, - "rshares": 461256359, - "voter": "chamviet" - }, - { - "percent": "10000", - "reputation": 23452817298, - "rshares": 1128966666, - "voter": "anwar78" - }, - { - "percent": "10000", - "reputation": 9724864408353, - "rshares": 353762811, - "voter": "rxhector" - }, - { - "percent": "10000", - "reputation": 308342072178, - "rshares": 13452861200, - "voter": "bitshares101" - }, - { - "percent": "10000", - "reputation": 229959978079725, - "rshares": 19873104999, - "voter": "ausbitbank" - }, - { - "percent": "10000", - "reputation": 1139583710641, - "rshares": 184112913, - "voter": "ardina" - }, - { - "percent": "10000", - "reputation": 3055657630377, - "rshares": 16336147500, - "voter": "chriscrypto" - }, - { - "percent": "10000", - "reputation": 9074599187627, - "rshares": 4125723797, - "voter": "gikitiki" - }, - { - "percent": "10000", - "reputation": 140605453893072, - "rshares": 119466748741, - "voter": "jesta" - }, - { - "percent": "10000", - "reputation": 7952828943, - "rshares": 3640445804, - "voter": "thegoodguy" - }, - { - "percent": "10000", - "reputation": 19355236534559, - "rshares": 75967203632, - "voter": "paco" - }, - { - "percent": "10000", - "reputation": 213565690794, - "rshares": 19068212733, - "voter": "showmethecoinz" - }, - { - "percent": "10000", - "reputation": 17415198441969, - "rshares": 24373102947, - "voter": "igster" - }, - { - "percent": "10000", - "reputation": 11926659655, - "rshares": 218061986, - "voter": "zoicneo" - }, - { - "percent": "10000", - "reputation": 606835220241, - "rshares": 322673293, - "voter": "dr2073" - }, - { - "percent": "10000", - "reputation": 56007869518919, - "rshares": 12376071844, - "voter": "jpiper20" - }, - { - "percent": "10000", - "reputation": 1145460037045, - "rshares": 252909161, - "voter": "natali22" - }, - { - "percent": "10000", - "reputation": -89927888118, - "rshares": 8322538338, - "voter": "mustafaomar" - }, - { - "percent": "10000", - "reputation": 383758474504, - "rshares": 13618277527, - "voter": "all-of-us" - }, - { - "percent": "10000", - "reputation": 44782427248, - "rshares": 919285482, - "voter": "nika" - }, - { - "percent": "10000", - "reputation": 2923721121912, - "rshares": 6588394536, - "voter": "geronimo" - }, - { - "percent": "10000", - "reputation": 35183938577926, - "rshares": 1901709746, - "voter": "cryptohustlin" - }, - { - "percent": "10000", - "reputation": 879845084613, - "rshares": 2473087864, - "voter": "lostnuggett" - }, - { - "percent": "10000", - "reputation": 102766515024, - "rshares": 31417575434, - "voter": "paquito" - }, - { - "percent": "10000", - "reputation": 272380745, - "rshares": 108742011, - "voter": "sergey22" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 575279737, - "voter": "steeminer" - }, - { - "percent": "10000", - "reputation": 842485513845, - "rshares": 35468156360, - "voter": "fatboy" - }, - { - "percent": "10000", - "reputation": 6225220452973, - "rshares": 2204627326, - "voter": "trisnawati" - }, - { - "percent": "10000", - "reputation": 1277580129, - "rshares": 389417359, - "voter": "vadim" - }, - { - "percent": "10000", - "reputation": 3270661957369, - "rshares": 6721829299, - "voter": "michaeldodridge" - }, - { - "percent": "10000", - "reputation": 36631314624965, - "rshares": 31188038738, - "voter": "celebr1ty" - }, - { - "percent": "10000", - "reputation": 169595925289, - "rshares": 7097922529, - "voter": "lykkeliten" - }, - { - "percent": "10000", - "reputation": -1193908062262, - "rshares": 108632879, - "voter": "steemster1" - }, - { - "percent": "10000", - "reputation": 106575300404, - "rshares": 2854890205, - "voter": "glitterpig" - }, - { - "percent": "10000", - "reputation": 10094642165697, - "rshares": 3046163199, - "voter": "jed78" - }, - { - "percent": "10000", - "reputation": 69073849011, - "rshares": 685101796, - "voter": "metaflute" - }, - { - "percent": "10000", - "reputation": -833837861150, - "rshares": 51143991, - "voter": "sharon" - }, - { - "percent": "10000", - "reputation": 100380231125, - "rshares": 8278623961, - "voter": "rawnetics" - }, - { - "percent": "10000", - "reputation": 37533992277122, - "rshares": 16442949757, - "voter": "thebluepanda" - }, - { - "percent": "10000", - "reputation": 266498838207861, - "rshares": 2339485159, - "voter": "timcliff" - }, - { - "percent": "10000", - "reputation": 3354530245153, - "rshares": 727812194, - "voter": "darknet" - }, - { - "percent": "10000", - "reputation": 44072966049463, - "rshares": 31463628969, - "voter": "mibenkito" - }, - { - "percent": "10000", - "reputation": 3529170665631, - "rshares": 8270953480, - "voter": "trending" - }, - { - "percent": "10000", - "reputation": 9955023637, - "rshares": 66036998, - "voter": "fruityloan" - }, - { - "percent": "10000", - "reputation": 25599522966599, - "rshares": 63377598, - "voter": "dezconocido" - }, - { - "percent": "10000", - "reputation": 64168495356, - "rshares": 64149962, - "voter": "sizil" - }, - { - "percent": "10000", - "reputation": 32682086388813, - "rshares": 27539088793, - "voter": "nonlinearone" - }, - { - "percent": "10000", - "reputation": -1468839175006, - "rshares": 51120963, - "voter": "msjennifer" - }, - { - "percent": "10000", - "reputation": -1343370986030, - "rshares": 50828873, - "voter": "ciao" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 9007787064, - "voter": "thebotkiller" - }, - { - "percent": "10000", - "reputation": 485540, - "rshares": 50521096, - "voter": "steemo" - }, - { - "percent": "10000", - "reputation": 612274223, - "rshares": 18974329448, - "voter": "nixonnox" - }, - { - "percent": "10000", - "reputation": -79575494262, - "rshares": 50390768, - "voter": "steema" - }, - { - "percent": "10000", - "reputation": 6792594761, - "rshares": 165051822, - "voter": "nonamer" - }, - { - "percent": "10000", - "reputation": -291550544386, - "rshares": 52732264, - "voter": "vishal1" - }, - { - "percent": "10000", - "reputation": -894488087252, - "rshares": 50183394, - "voter": "confucius" - }, - { - "percent": "10000", - "reputation": 6355399431384, - "rshares": 1506714481, - "voter": "unrealisback" - }, - { - "percent": "10000", - "reputation": -788377956676, - "rshares": 51153233, - "voter": "jarvis" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50692212, - "voter": "fortuner" - }, - { - "percent": "10000", - "reputation": 17071371161, - "rshares": 225683099, - "voter": "mefisto" - }, - { - "percent": "10000", - "reputation": 83682643470, - "rshares": 106783648, - "voter": "cinderphoenix" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 225874014, - "voter": "jimmytwoshoes" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50684643, - "voter": "johnbyrd" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50682252, - "voter": "thomasaustin" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50673817, - "voter": "widell" - }, - { - "percent": "10000", - "reputation": -49397634, - "rshares": 51613018, - "voter": "vasilii" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 288593312, - "voter": "steeminion" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50311250, - "voter": "revelbrooks" - }, - { - "percent": "10000", - "reputation": 1678817657, - "rshares": 57636462, - "voter": "x5cc7fd68" - }, - { - "percent": "10000", - "reputation": 4690307275921, - "rshares": 52631453, - "voter": "mrlogic" - }, - { - "percent": "10000", - "reputation": 96871175157544, - "rshares": 16295017448, - "voter": "someguy123" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50416427, - "voter": "curpose" - }, - { - "percent": "10000", - "reputation": 34317620219, - "rshares": 60781652, - "voter": "sharonekelly" - }, - { - "percent": "10000", - "reputation": 31646230940, - "rshares": 169640892, - "voter": "mrainp" - }, - { - "percent": "10000", - "reputation": 1442187004, - "rshares": 55791783, - "voter": "lsd123452" - }, - { - "percent": "10000", - "reputation": 202069894760324, - "rshares": 92590152, - "voter": "runridefly" - }, - { - "percent": "10000", - "reputation": 883345, - "rshares": 53142053, - "voter": "fleshtheworld" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 53116957, - "voter": "maximator15" - }, - { - "percent": "10000", - "reputation": 22712560975395, - "rshares": 373265099, - "voter": "ivet" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50588612, - "voter": "troich" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50271139, - "voter": "hitherise" - }, - { - "percent": "10000", - "reputation": 147922815034277, - "rshares": 1418732000, - "voter": "sponge-bob" - }, - { - "percent": "10000", - "reputation": 7402303856, - "rshares": 574697529, - "voter": "grm" - }, - { - "percent": "10000", - "reputation": 10318326138, - "rshares": 3524895174, - "voter": "ericbotticelli" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50710305, - "voter": "prof" - }, - { - "percent": "10000", - "reputation": 28410528693, - "rshares": 55019922, - "voter": "jhonione" - }, - { - "percent": "10000", - "reputation": 450879656589, - "rshares": 126007971, - "voter": "keverw" - }, - { - "percent": "10000", - "reputation": 808429523426, - "rshares": 54052181, - "voter": "topslim" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50054445, - "voter": "coad" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50825587, - "voter": "sofa" - }, - { - "percent": "10000", - "reputation": 7805684953, - "rshares": 52918124, - "voter": "xomtux" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 54693363, - "voter": "steeminnow" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 51488252, - "voter": "efroul" - }, - { - "percent": "10000", - "reputation": -236169662480, - "rshares": 50383632, - "voter": "zzzzzzzzz" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 51446137, - "voter": "asshai" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 54572306, - "voter": "cbdmegami" - }, - { - "percent": "10000", - "reputation": 6483658953, - "rshares": 52397603, - "voter": "doze49" - }, - { - "percent": "10000", - "reputation": 270576347, - "rshares": 50125313, - "voter": "ladyboss" - }, - { - "percent": "10000", - "reputation": 267026468, - "rshares": 53279765, - "voter": "jpitt241" - }, - { - "percent": "10000", - "reputation": 39212869675094, - "rshares": 0, - "voter": "countrygirl" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "dnjsgkr11" - }, - { - "percent": "10000", - "reputation": 27144215734, - "rshares": 0, - "voter": "cptnsolo" - }, - { - "percent": "10000", - "reputation": 17413759953763, - "rshares": 0, - "voter": "yehey" - }, - { - "percent": "10000", - "reputation": 1314618272, - "rshares": 0, - "voter": "b1czu" - }, - { - "percent": "10000", - "reputation": 110205170747082, - "rshares": 0, - "voter": "drakos" - }, - { - "percent": "10000", - "reputation": 3401652000230, - "rshares": 0, - "voter": "andre-verbrick" - }, - { - "percent": "10000", - "reputation": -11075113126, - "rshares": 0, - "voter": "masud123" - }, - { - "percent": "10000", - "reputation": 767526153, - "rshares": 0, - "voter": "jkhoffman" - }, - { - "percent": "10000", - "reputation": 60253396, - "rshares": 0, - "voter": "byaritzab" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "As you know, witness nodes are an essential part of the STEEM universe.\n\nIf I have a witness node with as much as (or as little as) 3% of witness approval, I can choose the right time to shut it down, listen to my favourite music album, walk my cat, and then start it up again, most probably without missing a block.\nA full-time witness, or one of the top19 witnesses, needs to produce blocks 1600 times more frequently.\nMaintaining such a server in the long run is not an easy task.\n\nWitnesses are vulnerable to DDoS attacks. An attacker who knows the network location of your witness node can saturate your uplink to a level that makes you unable to produce blocks on time. Effectively, this means **voting the witness out using a network-level attack**. Finding the IP address of your witness node is easier than you might think. Even if you follow the guidelines for setting up your public seed node on a different machine than the one you used to get your witness running, your witness still needs to connect to other nodes in the network.\nSo what does this mean? Take a look at: http://seeds.quisquis.de/steem.html\n(service provided by cyrano.witness)\n\nDoes any of these IP addresses look familiar?\nThe attacker still doesn't know which of them is yours, right?\n\nAn attack that makes the target IP unavailable for 3 seconds? Not exactly a difficult thing to do, right? So the attacker needs to make a guess by choosing from a set of suspected IP addresses he has obtained. Guessing doesn't cost him much. Neither does a high volume, short period DDoS attack. After that, he can see that your `total_missed` count increases, and he knows he guessed correctly. And then you are out.\n\n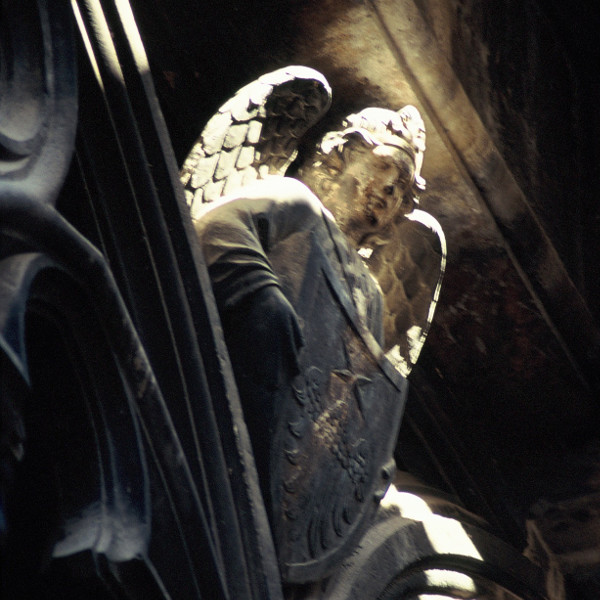\n\n## A concept of infrastructure: ##\n\n2 witness nodes (primary and backup)\n4 dedicated seed nodes\n\nOne witness node has your current signing key and does its job. The other is for failover, backup purposes. For example, whenever you need to upgrade your witness binary, you do that on the backup witness node, then change your signing key, so the backup node and the primary node switch their roles. \n**(Never leave your current signing key on two nodes at the same time!)**\n\nThese 4 seed nodes mentioned here only work on behalf of your witness i.e. they don\u2019t provide seed service on public network interfaces. \n_Your public seed node is not within the scope of this document._\n\nYour witness node connects **ONLY** to your seed nodes using VLAN or another private, low latency network solution of your choice. All other incoming/outgoing connections should be blocked except for the secure shell, preferably limited to access only from your IPs.\n\nEach seed node should be in a separate datacenter, ideally in a different part of the world, never in the same location as any of your (primary/backup) witness nodes. The purpose is to minimize the effects of a successful DDoS attack.\n\n_Please note that setting up all seed nodes in a single data center won\u2019t help. A DDoS attack targeted at that particular network will bring all your nodes down anyway._\n\n```\n +---+\n + +----+ | |\n | | |<-->| B |\n +--+ S1 |<-->| i |\n | | |<-->| g |\n | +----+ | |\n+------+ | | B |\n| +-+ +----+ | a |\n| W(p) | | | |<-->| d |\n| | +--+ S2 |<-->| |\n+------+ | | |<-->| U |\n | +----+ | g |\n VLAN| | l |\n | +----+ | y |\n+------+ | | |<-->| |\n| | +--+ S3 |<-->| I |\n| W(b) | | | |<-->| n |\n| +-+ +----+ | t |\n+------+ | | e |\n | +----+ | r |\n | | |<-->| n |\n +--+ S4 |<-->| e |\n | | |<-->| t |\n + +----+ | |\n +---+\n```\n```\nW(p) - primary witness\nW(b) - backup witness\nS1-4 - seed nodes\n```\nOf course, you need to keep your seed nodes (at least one of them) running all the time, otherwise your witness will not be able to sync the blockchain.\nBut if you do the math, you will see that the odds that all your seed nodes stop working at the same time are much lower than the chances that this happens to your witness node. \n\n\nIf one of your seed nodes falls victim to a DDoS attack, you can, depending on the features offered by your infrastructure provider:\n- do nothing (until most of them are targeted at the same time)\n- change its external interface IP address, routing the old one to a black hole\n- set up a new, additional, temporary seed node somewhere else\n- replace that seed node with a new one in another location\n\nIf you believe this idea is of use and value to Steem, please vote for me as a [witness](https://steemit.com/witness-category/@gtg/witness-gtg \"witness-gtg\")\neither on [Steemit's Witnesses List](https://steemit.com/~witnesses \"Witnesses\") \nor by using your `cli_wallet` command:\n`vote_for_witness \"YOURACCOUNT\" \"gtg\" true true`", - "body_length": 4933, - "cashout_time": "1969-12-31T23:59:59", - "category": "witness-category", - "children": 18, - "created": "2016-08-16T18:11:03", - "curator_payout_value": "243.149 HBD", - "depth": 0, - "json_metadata": "{\"tags\":[\"witness-category\",\"security\",\"steem\",\"steemit\"],\"links\":[\"https://steemit.com/witness-category/@gtg/witness-gtg\"]}", - "last_payout": "2016-09-16T20:13:00", - "last_update": "2016-08-16T18:11:03", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 80964590737004, - "parent_author": "", - "parent_permlink": "witness-category", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "heavy-duty-witness-node-infrastructure", - "post_id": 838525, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Heavy duty witness node infrastructure", - "title": "Heavy duty witness node infrastructure", - "total_payout_value": "745.322 HBD", - "url": "/witness-category/@gtg/heavy-duty-witness-node-infrastructure" - }, - "entry_id": 8, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "6600", - "reputation": 94312688970406, - "rshares": 606815117112, - "voter": "liondani" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 36785360396, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 6552498469686, - "rshares": 4271512211, - "voter": "dennygalindo" - }, - { - "percent": "10000", - "reputation": 37533992277122, - "rshares": 16442858487, - "voter": "thebluepanda" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Oh, thank you for this post, there was a lot of talks about it.\nIt's really not about **$$$**... people won't get rich for just curating. Gamification matters. \nPeople feel great if they successfully find something interesting. People feel great if they can use their Steem Power for others' sake. \nMost concerns are about so called whales and their votes, as it makes them profit even for curating blindly. Minnows on the other hand don't get a lot of profit, but get a lot of fun. Making curation rewards super-linear could solve that problem.", - "body_length": 546, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemit-ideas", - "children": 1, - "created": "2016-08-15T12:33:36", - "curator_payout_value": "0.041 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"steemit-ideas\"]}", - "last_payout": "2016-09-15T07:07:00", - "last_update": "2016-08-15T12:33:36", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 664314848206, - "parent_author": "liondani", - "parent_permlink": "don-t-remove-curation-rewards-just-stop-them-when-the-post-reach-the-trending-page", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-liondani-don-t-remove-curation-rewards-just-stop-them-when-the-post-reach-the-trending-page-20160815t123329115z", - "post_id": 815860, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "DON'T REMOVE Curation Rewards! Just stop them when the post reach the trending page !!!", - "title": "", - "total_payout_value": "0.510 HBD", - "url": "/steemit-ideas/@liondani/don-t-remove-curation-rewards-just-stop-them-when-the-post-reach-the-trending-page#@gtg/re-liondani-don-t-remove-curation-rewards-just-stop-them-when-the-post-reach-the-trending-page-20160815t123329115z" - }, - "entry_id": 7, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 7487619572225, - "rshares": 6301220766886, - "voter": "wackou" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 32376266765, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "`overmind`sounds good, but maybe morph it to `overmint`\nWhere `mint`is like in _\u201che made a mint on the stock market\u201d_ and/or like in _\"The United States Mint\"_ + of course like misspelled _\"mind\"_.", - "body_length": 197, - "cashout_time": "1969-12-31T23:59:59", - "category": "steem", - "children": 0, - "created": "2016-08-11T17:34:33", - "curator_payout_value": "1.567 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"steem\"]}", - "last_payout": "2016-09-09T17:18:09", - "last_update": "2016-08-11T17:34:33", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 6333597033651, - "parent_author": "wackou", - "parent_permlink": "btstools-development-bounties", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-wackou-btstools-development-bounties-20160811t173426180z", - "post_id": 749049, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "bts_tools development bounties!", - "title": "", - "total_payout_value": "4.787 HBD", - "url": "/steem/@wackou/btstools-development-bounties#@gtg/re-wackou-btstools-development-bounties-20160811t173426180z" - }, - "entry_id": 6, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 31446081488, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 549553053579, - "rshares": 30538028685, - "voter": "arcurus" - }, - { - "percent": "10000", - "reputation": 2247042714470, - "rshares": 655057139, - "voter": "brendio" - }, - { - "percent": "10000", - "reputation": 1489048033796, - "rshares": 0, - "voter": "bbkoopsta" - }, - { - "percent": "10000", - "reputation": 23991697734, - "rshares": 0, - "voter": "christophzak" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Of course It is truly right. It doesn't manipulate the free market. It says: _\"Hey, better watch out, fake products over there!\"_, so other buyers (upvoters) have choice to follow that advice or not. That's because of that freedom, not against it. Besides, I like cats. :-)", - "body_length": 273, - "cashout_time": "1969-12-31T23:59:59", - "category": "steemitabuse", - "children": 0, - "created": "2016-08-11T09:31:09", - "curator_payout_value": "0.004 HBD", - "depth": 2, - "json_metadata": "{\"tags\":[\"steemitabuse\"]}", - "last_payout": "2016-09-10T18:15:33", - "last_update": "2016-08-11T09:31:09", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 62639167312, - "parent_author": "heretickitten", - "parent_permlink": "re-cheetah-cheetah-bot-explained-20160811t033141148z", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-heretickitten-re-cheetah-cheetah-bot-explained-20160811t093103978z", - "post_id": 742458, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Cheetah Bot Explained.", - "title": "", - "total_payout_value": "0.040 HBD", - "url": "/steemitabuse/@cheetah/cheetah-bot-explained#@gtg/re-heretickitten-re-cheetah-cheetah-bot-explained-20160811t093103978z" - }, - "entry_id": 5, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 25969877559, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 17071371161, - "rshares": 225647319, - "voter": "mefisto" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Dzi\u015b oficjalnie zg\u0142osi\u0142em kandydatur\u0119.\nhttps://steemit.com/witness-category/@gtg/witness-gtg\n> Koncentracja g\u0142os\u00f3w - mo\u017ce z czasem pom\u00f3c wybra\u0107 kogo\u015b z naszych do pierwszej 50-tki.\n\nOd pocz\u0105tku by\u0142em w pierwszej setce i aby g\u0142osowa\u0107 konieczne by\u0142o u\u017cycie `cli_wallet`\nObecnie jestem w pierwszej 50-tce, wi\u0119c mo\u017cna g\u0142osowa\u0107 ju\u017c bezpo\u015brednio ze strony:\nhttps://steemit.com/~witnesses", - "body_length": 381, - "cashout_time": "1969-12-31T23:59:59", - "category": "polish", - "children": 0, - "created": "2016-08-05T18:46:27", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"polish\"],\"links\":[\"https://steemit.com/witness-category/@gtg/witness-gtg\"]}", - "last_payout": "2016-09-03T03:24:06", - "last_update": "2016-08-05T18:46:27", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 26195524878, - "parent_author": "mefisto", - "parent_permlink": "pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160805t184622691z", - "post_id": 631648, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "[PL] Czy polska spo\u0142eczno\u015b\u0107 powinna mie\u0107 swojego delegata (witness) ?", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/polish/@mefisto/pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness#@gtg/re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160805t184622691z" - }, - "entry_id": 4, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 10004142276726, - "rshares": 7498426175709, - "voter": "steempty" - }, - { - "percent": "10000", - "reputation": 520836362124341, - "rshares": 42312727614219, - "voter": "blocktrades" - }, - { - "percent": "10000", - "reputation": 608057298751, - "rshares": 305178325808, - "voter": "justin" - }, - { - "percent": "10000", - "reputation": 138490649, - "rshares": 869557685770, - "voter": "silver" - }, - { - "percent": "10000", - "reputation": 94312688970406, - "rshares": 0, - "voter": "liondani" - }, - { - "percent": "10000", - "reputation": 27406317697121, - "rshares": 5114872713665, - "voter": "roadscape" - }, - { - "percent": "10000", - "reputation": 62649292215598, - "rshares": 2289539684123, - "voter": "complexring" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 2180802207, - "voter": "chloe" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 2185117237, - "voter": "jen" - }, - { - "percent": "10000", - "reputation": 8921856669928, - "rshares": 14176153154, - "voter": "danknugs" - }, - { - "percent": "10000", - "reputation": 29872431292097, - "rshares": 198373663832, - "voter": "steemservices" - }, - { - "percent": "10000", - "reputation": 31620973634639, - "rshares": 30116948361, - "voter": "aizensou" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 46091054, - "voter": "kelly" - }, - { - "percent": "10000", - "reputation": 1241362310762, - "rshares": 6428425796, - "voter": "bentley" - }, - { - "percent": "10000", - "reputation": 4402236225514, - "rshares": 91926650, - "voter": "gandalf" - }, - { - "percent": "10000", - "reputation": 341233778618, - "rshares": 345893040115, - "voter": "boatymcboatface" - }, - { - "percent": "10000", - "reputation": 11554252869567, - "rshares": 27177508174, - "voter": "proctologic" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 316897956, - "voter": "sophia" - }, - { - "percent": "10000", - "reputation": 6434093816548, - "rshares": 21914098976, - "voter": "hcf27" - }, - { - "percent": "10000", - "reputation": 2403329501298, - "rshares": 7368210672, - "voter": "modeprator" - }, - { - "percent": "10000", - "reputation": 14648354466714, - "rshares": 103782757471, - "voter": "edgeland" - }, - { - "percent": "10000", - "reputation": 70083642919654, - "rshares": 785540122070, - "voter": "steemship" - }, - { - "percent": "10000", - "reputation": 377179304234, - "rshares": 5786912627, - "voter": "spaninv" - }, - { - "percent": "10000", - "reputation": 12049210200013, - "rshares": 196850398821, - "voter": "pal" - }, - { - "percent": "3301", - "reputation": 8801781140235, - "rshares": 2792608078, - "voter": "murh" - }, - { - "percent": "10000", - "reputation": 5102472394558, - "rshares": 98699236, - "voter": "itay" - }, - { - "percent": "10000", - "reputation": 1455868290913, - "rshares": 9975325205, - "voter": "johnerfx" - }, - { - "percent": "10000", - "reputation": 11782050148677, - "rshares": 206512826638, - "voter": "taoteh1221" - }, - { - "percent": "10000", - "reputation": 78929307854833, - "rshares": 57136530202, - "voter": "juanmiguelsalas" - }, - { - "percent": "10000", - "reputation": -455410726389, - "rshares": 9128119179, - "voter": "somedude" - }, - { - "percent": "10000", - "reputation": 4083609920412, - "rshares": 0, - "voter": "ola-haukland" - }, - { - "percent": "10000", - "reputation": 394956111554767, - "rshares": 203906072916, - "voter": "kaylinart" - }, - { - "percent": "10000", - "reputation": 45020858639, - "rshares": 867610016, - "voter": "johnerminer" - }, - { - "percent": "10000", - "reputation": 6945443409938, - "rshares": 32485842972, - "voter": "geoffrey" - }, - { - "percent": "10000", - "reputation": 1139112169460, - "rshares": 0, - "voter": "kwonjs77" - }, - { - "percent": "10000", - "reputation": 1240582110360, - "rshares": 2480411371, - "voter": "gary-smith" - }, - { - "percent": "10000", - "reputation": -492606942788, - "rshares": 23267718250, - "voter": "jparty" - }, - { - "percent": "10000", - "reputation": 109424005488868, - "rshares": 1953019618, - "voter": "mammasitta" - }, - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 25911121779, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 561848944506, - "rshares": 4679981480, - "voter": "madwallace" - }, - { - "percent": "10000", - "reputation": 62121500090796, - "rshares": 39716978937, - "voter": "lauralemons" - }, - { - "percent": "10000", - "reputation": 194260219749, - "rshares": 461256359, - "voter": "chamviet" - }, - { - "percent": "10000", - "reputation": -14070504625313, - "rshares": 15413814887, - "voter": "r4fken" - }, - { - "percent": "10000", - "reputation": 2447276760686, - "rshares": 6283643209, - "voter": "endaksi1" - }, - { - "percent": "10000", - "reputation": 17498320798649, - "rshares": 16250564070, - "voter": "picokernel" - }, - { - "percent": "100", - "reputation": 116503940714958, - "rshares": 702264837, - "voter": "furion" - }, - { - "percent": "10000", - "reputation": 98590241491022, - "rshares": 80421874395, - "voter": "anyx" - }, - { - "percent": "10000", - "reputation": 4454717561447, - "rshares": 2241994263, - "voter": "egjoshslim" - }, - { - "percent": "10000", - "reputation": 19355236534559, - "rshares": 120632910259, - "voter": "paco" - }, - { - "percent": "10000", - "reputation": 37145059769006, - "rshares": 6621475753, - "voter": "senseiteekay" - }, - { - "percent": "10000", - "reputation": 122134768146, - "rshares": 226092177, - "voter": "jesse5th" - }, - { - "percent": "10000", - "reputation": 273611816138333, - "rshares": 23810445079, - "voter": "inertia" - }, - { - "percent": "10000", - "reputation": 171730563, - "rshares": 210829632, - "voter": "glassice" - }, - { - "percent": "10000", - "reputation": -424880418803, - "rshares": 203070157, - "voter": "lenar79" - }, - { - "percent": "10000", - "reputation": 549553053579, - "rshares": 15277675835, - "voter": "arcurus" - }, - { - "percent": "10000", - "reputation": 11027870280, - "rshares": 0, - "voter": "smospider" - }, - { - "percent": "10000", - "reputation": 22729726767685, - "rshares": 101769596658, - "voter": "johnsmith" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 330264489, - "voter": "steeminer" - }, - { - "percent": "10000", - "reputation": 99228490804, - "rshares": 55123516, - "voter": "cire81" - }, - { - "percent": "10000", - "reputation": 36631314624965, - "rshares": 20346345482, - "voter": "celebr1ty" - }, - { - "percent": "10000", - "reputation": 5762427931179, - "rshares": 116522786, - "voter": "kibela" - }, - { - "percent": "10000", - "reputation": 120735429296211, - "rshares": 4195953733, - "voter": "sulev" - }, - { - "percent": "10000", - "reputation": 106575300404, - "rshares": 1863529002, - "voter": "glitterpig" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 88147854, - "voter": "alrx6918" - }, - { - "percent": "10000", - "reputation": -2405411059, - "rshares": 156246825, - "voter": "vitz81" - }, - { - "percent": "10000", - "reputation": 100380231125, - "rshares": 7842906910, - "voter": "rawnetics" - }, - { - "percent": "10000", - "reputation": -5843262501385, - "rshares": 2110595726, - "voter": "naturalista" - }, - { - "percent": "10000", - "reputation": 1601368865949, - "rshares": 47302483, - "voter": "persianqueen" - }, - { - "percent": "10000", - "reputation": 48401633178775, - "rshares": 309993436467, - "voter": "glitterfart" - }, - { - "percent": "10000", - "reputation": -487137935046, - "rshares": 102759457, - "voter": "caitlinm" - }, - { - "percent": "10000", - "reputation": 28008551303674, - "rshares": 71928410, - "voter": "jellenmark" - }, - { - "percent": "10000", - "reputation": 175988457163, - "rshares": 151302584, - "voter": "agussudaryanto" - }, - { - "percent": "10000", - "reputation": -1343370986030, - "rshares": 12663424, - "voter": "ciao" - }, - { - "percent": "10000", - "reputation": 35191397417, - "rshares": 519527818, - "voter": "iaco" - }, - { - "percent": "10000", - "reputation": 485540, - "rshares": 14786662, - "voter": "steemo" - }, - { - "percent": "10000", - "reputation": 612274223, - "rshares": 1568749518, - "voter": "nixonnox" - }, - { - "percent": "10000", - "reputation": 503316919452805, - "rshares": 3195610059, - "voter": "hanshotfirst" - }, - { - "percent": "10000", - "reputation": -79575494262, - "rshares": 2458086, - "voter": "steema" - }, - { - "percent": "10000", - "reputation": -894488087252, - "rshares": 12950553, - "voter": "confucius" - }, - { - "percent": "10000", - "reputation": 358319354936, - "rshares": 911816960, - "voter": "bledarus" - }, - { - "percent": "10", - "reputation": -711516507127, - "rshares": 1251336, - "voter": "softpunk" - }, - { - "percent": "10000", - "reputation": -788377956676, - "rshares": 3742919, - "voter": "jarvis" - }, - { - "percent": "10000", - "reputation": 31215012571, - "rshares": 37069491, - "voter": "thecurator" - }, - { - "percent": "10000", - "reputation": 17071371161, - "rshares": 229846452, - "voter": "mefisto" - }, - { - "percent": "10000", - "reputation": 83682643470, - "rshares": 104558988, - "voter": "cinderphoenix" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 98540677, - "voter": "steeminion" - }, - { - "percent": "10000", - "reputation": 5861882, - "rshares": 57959648, - "voter": "piezolit" - }, - { - "percent": "10000", - "reputation": 9708508, - "rshares": 8108416, - "voter": "brucy" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 35603456, - "voter": "shukerona" - }, - { - "percent": "10000", - "reputation": 13043111, - "rshares": 56023735, - "voter": "leticiapink" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 295195987, - "voter": "steeminnow" - }, - { - "percent": "10000", - "reputation": 1146430807136, - "rshares": 0, - "voter": "apocalypse612" - }, - { - "percent": "10000", - "reputation": 11384534489, - "rshares": 0, - "voter": "spiritplay" - }, - { - "percent": "0", - "reputation": 135393506, - "rshares": 0, - "voter": "chicmagnet" - }, - { - "percent": "10000", - "reputation": 2120107899278, - "rshares": 0, - "voter": "jamzed" - }, - { - "percent": "10000", - "reputation": 3836479042999, - "rshares": 0, - "voter": "rouer66" - }, - { - "percent": "10000", - "reputation": 52235871881, - "rshares": 0, - "voter": "technotricks" - }, - { - "percent": "10000", - "reputation": 24803810555151, - "rshares": 0, - "voter": "blacktranquility" - }, - { - "percent": "10000", - "reputation": 189925704820, - "rshares": 0, - "voter": "envy112" - }, - { - "percent": "10000", - "reputation": 34318553443285, - "rshares": 0, - "voter": "madlenfox" - }, - { - "percent": "10000", - "reputation": 2625878277965, - "rshares": 0, - "voter": "vandalizmrecordz" - }, - { - "percent": "10000", - "reputation": 3296992202, - "rshares": 0, - "voter": "omahadealer" - }, - { - "percent": "10000", - "reputation": 110896908209, - "rshares": 0, - "voter": "camilojjj" - }, - { - "percent": "10000", - "reputation": 21630385026, - "rshares": 0, - "voter": "buzman" - }, - { - "percent": "10000", - "reputation": 3213735751, - "rshares": 0, - "voter": "aloush" - }, - { - "percent": "10000", - "reputation": 400935635953211, - "rshares": 0, - "voter": "andrianna" - }, - { - "percent": "10000", - "reputation": 65050507116, - "rshares": 0, - "voter": "jamesweller" - }, - { - "percent": "10000", - "reputation": 1252187262452, - "rshares": 0, - "voter": "semantralist" - }, - { - "percent": "10000", - "reputation": 398219179319, - "rshares": 0, - "voter": "contact95" - }, - { - "percent": "10000", - "reputation": 8688164, - "rshares": 0, - "voter": "piotrdab" - }, - { - "percent": "10000", - "reputation": 1422632093238, - "rshares": 0, - "voter": "lanceman" - }, - { - "percent": "10000", - "reputation": 1432603806298, - "rshares": 0, - "voter": "martwykotek" - }, - { - "percent": "10000", - "reputation": 44818356660, - "rshares": 0, - "voter": "rakibfiha" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "dnjsgkr11" - }, - { - "percent": "100", - "reputation": 1226754203051, - "rshares": 0, - "voter": "palejack" - }, - { - "percent": "10000", - "reputation": 83305054375, - "rshares": 0, - "voter": "animalbenefits" - }, - { - "percent": "10000", - "reputation": 4901712891, - "rshares": 0, - "voter": "benoit" - }, - { - "percent": "10000", - "reputation": 7280759792, - "rshares": 0, - "voter": "cryptodc" - }, - { - "percent": "10000", - "reputation": 110636848734, - "rshares": 0, - "voter": "danlocks.com" - }, - { - "percent": "10000", - "reputation": 9757554074387, - "rshares": 0, - "voter": "bleedpoet" - }, - { - "percent": "10000", - "reputation": 82366823186, - "rshares": 0, - "voter": "yanching" - }, - { - "percent": "10000", - "reputation": 460266953754, - "rshares": 0, - "voter": "carolynseymour" - }, - { - "percent": "10000", - "reputation": 12214914624, - "rshares": 0, - "voter": "orbitalqq" - }, - { - "percent": "10000", - "reputation": 840282966, - "rshares": 0, - "voter": "allenjack" - }, - { - "percent": "10000", - "reputation": 10852430072, - "rshares": 0, - "voter": "drcryptocoach" - }, - { - "percent": "10000", - "reputation": 57913191723, - "rshares": 0, - "voter": "mila7272" - }, - { - "percent": "10000", - "reputation": 3381778816658, - "rshares": 0, - "voter": "aditor" - }, - { - "percent": "10000", - "reputation": 3741527076026, - "rshares": 0, - "voter": "jackjohanneshemp" - }, - { - "percent": "10000", - "reputation": 139612597, - "rshares": 0, - "voter": "necada" - }, - { - "percent": "10000", - "reputation": 8961603094, - "rshares": 0, - "voter": "rodimusprime" - }, - { - "percent": "10000", - "reputation": 33446684, - "rshares": 0, - "voter": "jumbolove" - }, - { - "percent": "10000", - "reputation": 279218179107, - "rshares": 0, - "voter": "tiandao" - }, - { - "percent": "10000", - "reputation": 985171153288, - "rshares": 0, - "voter": "bronxb17" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "augustyn" - }, - { - "percent": "10000", - "reputation": 425127733548, - "rshares": 0, - "voter": "ciag" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "nemethx" - }, - { - "percent": "10000", - "reputation": 8923971358917, - "rshares": 0, - "voter": "irfanullah" - }, - { - "percent": "10000", - "reputation": 137533089168, - "rshares": 0, - "voter": "yusuf2829" - }, - { - "percent": "10000", - "reputation": 259855282540, - "rshares": 0, - "voter": "mero" - }, - { - "percent": "10000", - "reputation": 2272046398, - "rshares": 0, - "voter": "ggd3yydze" - }, - { - "percent": "10000", - "reputation": 5130188464, - "rshares": 0, - "voter": "kbh2017" - }, - { - "percent": "10000", - "reputation": 418242269128, - "rshares": 0, - "voter": "dobro88888888" - }, - { - "percent": "10000", - "reputation": 13881685593, - "rshares": 0, - "voter": "mikelspickles" - }, - { - "percent": "10000", - "reputation": 4054569358897, - "rshares": 0, - "voter": "lacremo-it" - }, - { - "percent": "10000", - "reputation": 1078193881, - "rshares": 0, - "voter": "intutkri2" - }, - { - "percent": "10000", - "reputation": 11467025924, - "rshares": 0, - "voter": "boban" - }, - { - "percent": "10000", - "reputation": 3025495096, - "rshares": 0, - "voter": "growthwithconnie" - }, - { - "percent": "10000", - "reputation": 61314926322, - "rshares": 0, - "voter": "shrivastava.sud" - }, - { - "percent": "10000", - "reputation": 145637556, - "rshares": 0, - "voter": "sunnybank007" - }, - { - "percent": "10000", - "reputation": 1609978261257, - "rshares": 0, - "voter": "kingjan" - }, - { - "percent": "10000", - "reputation": 91277590629, - "rshares": 0, - "voter": "syehazis" - }, - { - "percent": "10000", - "reputation": 977892141891, - "rshares": 0, - "voter": "swisssteembeat" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "directcoin" - }, - { - "percent": "10000", - "reputation": 330828174442, - "rshares": 0, - "voter": "teukuarmia" - }, - { - "percent": "10000", - "reputation": 415075815, - "rshares": 0, - "voter": "kanumary" - }, - { - "percent": "10000", - "reputation": 84464116993, - "rshares": 0, - "voter": "nururrahman" - }, - { - "percent": "10000", - "reputation": 1420329207, - "rshares": 0, - "voter": "hkjn" - }, - { - "percent": "10000", - "reputation": 5051477584, - "rshares": 0, - "voter": "siyulaters" - }, - { - "percent": "10000", - "reputation": 170482814, - "rshares": 0, - "voter": "archdroid" - }, - { - "percent": "10000", - "reputation": 364839806113, - "rshares": 0, - "voter": "edsinestragos" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "socratestar" - }, - { - "percent": "10000", - "reputation": 7077816439, - "rshares": 0, - "voter": "tekendra" - }, - { - "percent": "10000", - "reputation": 6884826572914, - "rshares": 0, - "voter": "enriqueig" - }, - { - "percent": "10000", - "reputation": 1030829174880, - "rshares": 0, - "voter": "victhor" - }, - { - "percent": "10000", - "reputation": 5561644222257, - "rshares": 0, - "voter": "jahangirbalti" - }, - { - "percent": "10000", - "reputation": 1367335236, - "rshares": 0, - "voter": "supremacia3" - }, - { - "percent": "10000", - "reputation": 28627806298, - "rshares": 0, - "voter": "wanispwnz" - }, - { - "percent": "10000", - "reputation": 381110239767, - "rshares": 0, - "voter": "gigpen" - }, - { - "percent": "10000", - "reputation": 1727391164, - "rshares": 0, - "voter": "vovimcalcifer" - }, - { - "percent": "10000", - "reputation": 11412324126, - "rshares": 0, - "voter": "ashikyeamin" - }, - { - "percent": "10000", - "reputation": 39353604, - "rshares": 0, - "voter": "bmf" - }, - { - "percent": "10000", - "reputation": 6397576292475, - "rshares": 0, - "voter": "thirdeye7" - }, - { - "percent": "10000", - "reputation": 27263952970, - "rshares": 0, - "voter": "brentian" - }, - { - "percent": "10000", - "reputation": 30509582168, - "rshares": 0, - "voter": "dat81" - }, - { - "percent": "10000", - "reputation": 32192089, - "rshares": 0, - "voter": "hardeepsinh" - }, - { - "percent": "10000", - "reputation": 2298002796, - "rshares": 0, - "voter": "sudhansu" - }, - { - "percent": "10000", - "reputation": 243085212, - "rshares": 0, - "voter": "stoyanyankov" - }, - { - "percent": "10000", - "reputation": 53986679, - "rshares": 0, - "voter": "iferyaya" - }, - { - "percent": "10000", - "reputation": 11908482237, - "rshares": 0, - "voter": "crowdie" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "jazzuly" - }, - { - "percent": "10000", - "reputation": 6827060529, - "rshares": 0, - "voter": "shushan1986" - }, - { - "percent": "10000", - "reputation": 122177855397, - "rshares": 0, - "voter": "ivrmakers" - }, - { - "percent": "-10000", - "reputation": 6166814, - "rshares": 0, - "voter": "sitio77" - }, - { - "percent": "10000", - "reputation": 289674123991, - "rshares": 0, - "voter": "olayar" - }, - { - "percent": "10000", - "reputation": -65245820138, - "rshares": 0, - "voter": "mosabhelal" - }, - { - "percent": "10000", - "reputation": 485993160, - "rshares": 0, - "voter": "antigono00" - }, - { - "percent": "10000", - "reputation": 283484167, - "rshares": 0, - "voter": "messmokdad" - }, - { - "percent": "10000", - "reputation": 1676884917531, - "rshares": 0, - "voter": "woodermax" - }, - { - "percent": "10000", - "reputation": -3916132001, - "rshares": 0, - "voter": "soleiltravel" - }, - { - "percent": "10000", - "reputation": -141528509999, - "rshares": 0, - "voter": "dollarbo-y" - }, - { - "percent": "10000", - "reputation": 80829565229, - "rshares": 0, - "voter": "danulinka" - }, - { - "percent": "10000", - "reputation": 845405976, - "rshares": 0, - "voter": "buy4balance" - }, - { - "percent": "10000", - "reputation": 91634664573, - "rshares": 0, - "voter": "fernana" - }, - { - "percent": "10000", - "reputation": 11273825894, - "rshares": 0, - "voter": "abbasmhd" - }, - { - "percent": "10000", - "reputation": 120383126, - "rshares": 0, - "voter": "rozaidimor" - }, - { - "percent": "10000", - "reputation": -5775212, - "rshares": 0, - "voter": "elanur" - }, - { - "percent": "10000", - "reputation": 49213065, - "rshares": 0, - "voter": "ahmedelbasha" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "smartemmy" - }, - { - "percent": "10000", - "reputation": 17772407, - "rshares": 0, - "voter": "mohamedkamal" - }, - { - "percent": "10000", - "reputation": 8320716127, - "rshares": 0, - "voter": "setio" - }, - { - "percent": "10000", - "reputation": 32068582, - "rshares": 0, - "voter": "pedro.simoes" - }, - { - "percent": "10000", - "reputation": 12147546785578, - "rshares": 0, - "voter": "braini" - }, - { - "percent": "10000", - "reputation": 4609840939796, - "rshares": 0, - "voter": "wholeself-in" - }, - { - "percent": "10000", - "reputation": 10208259268, - "rshares": 0, - "voter": "hesselholt" - }, - { - "percent": "10000", - "reputation": 1202422239, - "rshares": 0, - "voter": "arabo" - }, - { - "percent": "10000", - "reputation": -18668708, - "rshares": 0, - "voter": "astarte89" - }, - { - "percent": "10000", - "reputation": -148167670889, - "rshares": 0, - "voter": "inzouikroft" - }, - { - "percent": "10000", - "reputation": 394626784, - "rshares": 0, - "voter": "samuellucky" - }, - { - "percent": "10000", - "reputation": -862591467153, - "rshares": 0, - "voter": "h00pla85" - }, - { - "percent": "10000", - "reputation": 13757045542796, - "rshares": 0, - "voter": "tonygreene113" - }, - { - "percent": "10000", - "reputation": 157756045103, - "rshares": 0, - "voter": "benjy87" - }, - { - "percent": "10000", - "reputation": 10772384915, - "rshares": 0, - "voter": "antonyjoseph" - }, - { - "percent": "10000", - "reputation": 3857954524, - "rshares": 0, - "voter": "nikunjo" - }, - { - "percent": "10000", - "reputation": 242173734555, - "rshares": 0, - "voter": "emilnashar" - }, - { - "percent": "10000", - "reputation": 5824508534, - "rshares": 0, - "voter": "jhaypenones" - }, - { - "percent": "10000", - "reputation": -98824940708, - "rshares": 0, - "voter": "samuraiz" - }, - { - "percent": "10000", - "reputation": 777012168, - "rshares": 0, - "voter": "ajax27" - }, - { - "percent": "10000", - "reputation": 4294306473, - "rshares": 0, - "voter": "spinvibes" - }, - { - "percent": "10000", - "reputation": 453462354, - "rshares": 0, - "voter": "farhan05" - }, - { - "percent": "10000", - "reputation": 8043686101, - "rshares": 0, - "voter": "cikamatko" - }, - { - "percent": "10000", - "reputation": 463762412, - "rshares": 0, - "voter": "vetonmisiri" - }, - { - "percent": "10000", - "reputation": 5949684536, - "rshares": 0, - "voter": "socialwealth" - }, - { - "percent": "10000", - "reputation": 34921636505, - "rshares": 0, - "voter": "ecodata" - }, - { - "percent": "10000", - "reputation": 322064194546, - "rshares": 0, - "voter": "ronaldsteemit89" - }, - { - "percent": "10000", - "reputation": 719582687, - "rshares": 0, - "voter": "mattracer" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "retro1" - }, - { - "percent": "10000", - "reputation": 633826445, - "rshares": 0, - "voter": "asnous" - }, - { - "percent": "10000", - "reputation": 1513312554, - "rshares": 0, - "voter": "goldensteemit" - }, - { - "percent": "10000", - "reputation": 1291083960, - "rshares": 0, - "voter": "alkalize" - }, - { - "percent": "10000", - "reputation": 616507792, - "rshares": 0, - "voter": "ayushmanantal" - }, - { - "percent": "10000", - "reputation": 624612666345342, - "rshares": 0, - "voter": "dobartim" - }, - { - "percent": "10000", - "reputation": 40634383118, - "rshares": 0, - "voter": "moemyint" - }, - { - "percent": "10000", - "reputation": 5657058454, - "rshares": 0, - "voter": "shaunicierockart" - }, - { - "percent": "10000", - "reputation": 13675771, - "rshares": 0, - "voter": "saib0t" - }, - { - "percent": "10000", - "reputation": 44159667270901, - "rshares": 0, - "voter": "jasonmunapasee" - }, - { - "percent": "10000", - "reputation": 59386723823, - "rshares": 0, - "voter": "abouche.youness" - }, - { - "percent": "10000", - "reputation": 1985754615509, - "rshares": 0, - "voter": "physalis" - }, - { - "percent": "10000", - "reputation": 2990474565, - "rshares": 0, - "voter": "clementgirardweb" - }, - { - "percent": "10000", - "reputation": 301826001124, - "rshares": 0, - "voter": "feiyu93" - }, - { - "percent": "10000", - "reputation": 19440951884, - "rshares": 0, - "voter": "semisam" - }, - { - "percent": "10000", - "reputation": 1291269538, - "rshares": 0, - "voter": "dadabosade" - }, - { - "percent": "10000", - "reputation": 1853476474, - "rshares": 0, - "voter": "erfan007" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "aladodo" - }, - { - "percent": "10000", - "reputation": 61462627, - "rshares": 0, - "voter": "sekou" - }, - { - "percent": "10000", - "reputation": 234745476838, - "rshares": 0, - "voter": "ygriffiny" - }, - { - "percent": "10000", - "reputation": 595510022, - "rshares": 0, - "voter": "stufrost" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "jorji7" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "dg258" - }, - { - "percent": "10000", - "reputation": 16221007213, - "rshares": 0, - "voter": "bleizer" - }, - { - "percent": "10000", - "reputation": 488788732, - "rshares": 0, - "voter": "habibruis" - }, - { - "percent": "10000", - "reputation": 22802717702, - "rshares": 0, - "voter": "xrysam" - }, - { - "percent": "10000", - "reputation": 35997314, - "rshares": 0, - "voter": "shivangijain" - }, - { - "percent": "10000", - "reputation": 49742155476, - "rshares": 0, - "voter": "eversmile" - }, - { - "percent": "10000", - "reputation": 760705145, - "rshares": 0, - "voter": "wyvern8888" - }, - { - "percent": "10000", - "reputation": 33644429822, - "rshares": 0, - "voter": "diegoarroyook" - }, - { - "percent": "10000", - "reputation": 18134615, - "rshares": 0, - "voter": "subways" - }, - { - "percent": "10000", - "reputation": 432962879632, - "rshares": 0, - "voter": "indraperdanaok" - }, - { - "percent": "10000", - "reputation": 315364496896, - "rshares": 0, - "voter": "romiferns" - }, - { - "percent": "10000", - "reputation": 766351103387, - "rshares": 0, - "voter": "snarf" - }, - { - "percent": "10000", - "reputation": 36336534705, - "rshares": 0, - "voter": "pariyadi" - }, - { - "percent": "10000", - "reputation": 239373373651, - "rshares": 0, - "voter": "cryptonull" - }, - { - "percent": "0", - "reputation": 0, - "rshares": 0, - "voter": "jasonchappell" - }, - { - "percent": "10000", - "reputation": 238233259858, - "rshares": 0, - "voter": "zomboy" - }, - { - "percent": "10000", - "reputation": 30628550723, - "rshares": 0, - "voter": "irishgirlcrypto" - }, - { - "percent": "10000", - "reputation": 1516794756, - "rshares": 0, - "voter": "saurabhkujur" - }, - { - "percent": "10000", - "reputation": 1863553064, - "rshares": 0, - "voter": "atcexperts" - }, - { - "percent": "10000", - "reputation": 4654344805960, - "rshares": 0, - "voter": "habercitr" - }, - { - "percent": "10000", - "reputation": 809145435731, - "rshares": 0, - "voter": "shanto24" - }, - { - "percent": "10000", - "reputation": 666854601861, - "rshares": 0, - "voter": "engineeringsteem" - }, - { - "percent": "10000", - "reputation": 159615238, - "rshares": 0, - "voter": "neel0199" - }, - { - "percent": "10000", - "reputation": 386955447, - "rshares": 0, - "voter": "frankyguti" - }, - { - "percent": "10000", - "reputation": 1571178856, - "rshares": 0, - "voter": "steemingallofit" - }, - { - "percent": "10000", - "reputation": 67727393070, - "rshares": 0, - "voter": "nicksongarcia" - }, - { - "percent": "10000", - "reputation": 12760317004, - "rshares": 0, - "voter": "rafiul010" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "sergeyl" - }, - { - "percent": "10000", - "reputation": 19306159645, - "rshares": 0, - "voter": "dogeo" - }, - { - "percent": "10000", - "reputation": 20446878654, - "rshares": 0, - "voter": "bitcurrencies" - }, - { - "percent": "10000", - "reputation": 171875081, - "rshares": 0, - "voter": "blaximus" - }, - { - "percent": "10000", - "reputation": 196949334821, - "rshares": 0, - "voter": "fahimgazi" - }, - { - "percent": "10000", - "reputation": 8443607152, - "rshares": 0, - "voter": "ahmadraza89" - }, - { - "percent": "10000", - "reputation": 3029811482, - "rshares": 0, - "voter": "brianr702" - }, - { - "percent": "10000", - "reputation": 21407022349, - "rshares": 0, - "voter": "animefanrd" - }, - { - "percent": "10000", - "reputation": 17805816, - "rshares": 0, - "voter": "matiascec" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "josefto" - }, - { - "percent": "10000", - "reputation": 17153740581, - "rshares": 0, - "voter": "lucadema" - }, - { - "percent": "10000", - "reputation": 34809979848873, - "rshares": 0, - "voter": "yakubenko" - }, - { - "percent": "10000", - "reputation": 99062501783, - "rshares": 0, - "voter": "arcrypto" - }, - { - "percent": "10000", - "reputation": 276485595715, - "rshares": 0, - "voter": "m3mt" - }, - { - "percent": "10000", - "reputation": 1111016822, - "rshares": 0, - "voter": "laurentmounif" - }, - { - "percent": "10000", - "reputation": 4259609998610, - "rshares": 0, - "voter": "virus95" - }, - { - "percent": "0", - "reputation": 32366306714, - "rshares": 0, - "voter": "earnxtreme" - }, - { - "percent": "10000", - "reputation": 10898561, - "rshares": 0, - "voter": "massi274" - }, - { - "percent": "10000", - "reputation": 187465986902, - "rshares": 0, - "voter": "eldean" - }, - { - "percent": "10000", - "reputation": -4911455320, - "rshares": 0, - "voter": "reysmiled" - }, - { - "percent": "10000", - "reputation": 705586109336, - "rshares": 0, - "voter": "vianney" - }, - { - "percent": "10000", - "reputation": 553596837, - "rshares": 0, - "voter": "webukrnet" - }, - { - "percent": "10000", - "reputation": 45315317186, - "rshares": 0, - "voter": "raiculetzz" - }, - { - "percent": "10000", - "reputation": 769130566351, - "rshares": 0, - "voter": "lordantoni" - }, - { - "percent": "10000", - "reputation": 329420886, - "rshares": 0, - "voter": "skyzohkey" - }, - { - "percent": "10000", - "reputation": 33069820017, - "rshares": 0, - "voter": "norod" - }, - { - "percent": "10000", - "reputation": 3344298322, - "rshares": 0, - "voter": "tinystream" - }, - { - "percent": "10000", - "reputation": 2850103351, - "rshares": 0, - "voter": "hachevln" - }, - { - "percent": "10000", - "reputation": 27795438, - "rshares": 0, - "voter": "xedon" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "sushicat" - }, - { - "percent": "10000", - "reputation": 3747495510, - "rshares": 0, - "voter": "jesusvmorales" - }, - { - "percent": "10000", - "reputation": 42018202, - "rshares": 0, - "voter": "roadix" - }, - { - "percent": "10000", - "reputation": 2197232078, - "rshares": 0, - "voter": "mememetics" - }, - { - "percent": "10000", - "reputation": 3656282141, - "rshares": 0, - "voter": "hood-news-review" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "levon377" - }, - { - "percent": "10000", - "reputation": 902622842150, - "rshares": 0, - "voter": "sabinaschiavon" - }, - { - "percent": "10000", - "reputation": 3467655996, - "rshares": 0, - "voter": "rajdolla" - }, - { - "percent": "10000", - "reputation": -320223217, - "rshares": 0, - "voter": "musaka" - }, - { - "percent": "10000", - "reputation": 9457125, - "rshares": 0, - "voter": "fl0ri4n" - }, - { - "percent": "10000", - "reputation": 1993071336164, - "rshares": 0, - "voter": "allisgood" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "afolayemi1" - }, - { - "percent": "10000", - "reputation": 105517947488, - "rshares": 0, - "voter": "kaanhan" - }, - { - "percent": "10000", - "reputation": 1589868354, - "rshares": 0, - "voter": "sfjawad" - }, - { - "percent": "10000", - "reputation": 91642253902, - "rshares": 0, - "voter": "wovenone" - }, - { - "percent": "10000", - "reputation": 19837973739, - "rshares": 0, - "voter": "hashkeks" - }, - { - "percent": "10000", - "reputation": 11199825912, - "rshares": 0, - "voter": "amirullah99" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "luka2609" - }, - { - "percent": "10000", - "reputation": 247470791, - "rshares": 0, - "voter": "draco1" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "kekzdozer" - }, - { - "percent": "10000", - "reputation": 490205504, - "rshares": 0, - "voter": "wohugb" - }, - { - "percent": "10000", - "reputation": 85025833, - "rshares": 0, - "voter": "celiadiz" - }, - { - "percent": "10000", - "reputation": 4942684759, - "rshares": 0, - "voter": "reynoriega" - }, - { - "percent": "10000", - "reputation": 157058223278, - "rshares": 0, - "voter": "jnmtrust" - }, - { - "percent": "10000", - "reputation": 43676564, - "rshares": 0, - "voter": "slyster12" - }, - { - "percent": "10000", - "reputation": 118699355010, - "rshares": 0, - "voter": "dani123" - }, - { - "percent": "10000", - "reputation": 1172386369087, - "rshares": 0, - "voter": "johnskotts" - }, - { - "percent": "10000", - "reputation": 20908184788, - "rshares": 0, - "voter": "jenesis725" - }, - { - "percent": "10000", - "reputation": 2748695148, - "rshares": 0, - "voter": "syahrulgeudong" - }, - { - "percent": "10000", - "reputation": 4489817172, - "rshares": 0, - "voter": "demarco2016" - }, - { - "percent": "10000", - "reputation": 6517226246902, - "rshares": 0, - "voter": "f-steemit" - }, - { - "percent": "10000", - "reputation": 9609600379, - "rshares": 0, - "voter": "muhammadasrill" - }, - { - "percent": "10000", - "reputation": 302783386, - "rshares": 0, - "voter": "happyreaper23" - }, - { - "percent": "10000", - "reputation": 409900794135, - "rshares": 0, - "voter": "carony" - }, - { - "percent": "10000", - "reputation": 7799119516, - "rshares": 0, - "voter": "steemnarrow" - }, - { - "percent": "10000", - "reputation": 125877134519, - "rshares": 0, - "voter": "gratamaster" - }, - { - "percent": "10000", - "reputation": 137671090105, - "rshares": 0, - "voter": "kriptoqraf" - }, - { - "percent": "10000", - "reputation": 396350450662, - "rshares": 0, - "voter": "zbordom" - }, - { - "percent": "0", - "reputation": -711037006, - "rshares": 0, - "voter": "warisman01" - }, - { - "percent": "10000", - "reputation": 8302086906, - "rshares": 0, - "voter": "daveyz" - }, - { - "percent": "10000", - "reputation": 55716404, - "rshares": 0, - "voter": "zeeintrouble" - }, - { - "percent": "10000", - "reputation": 1908329824249, - "rshares": 0, - "voter": "sonsory" - }, - { - "percent": "10000", - "reputation": 10919912893, - "rshares": 0, - "voter": "urannium" - }, - { - "percent": "10000", - "reputation": 264310312, - "rshares": 0, - "voter": "deviasyifak" - }, - { - "percent": "10000", - "reputation": 2112514001, - "rshares": 0, - "voter": "luigi777" - }, - { - "percent": "10000", - "reputation": 608768469, - "rshares": 0, - "voter": "cogitoergosumary" - }, - { - "percent": "10000", - "reputation": -1012194420500, - "rshares": 0, - "voter": "a-a-10" - }, - { - "percent": "10000", - "reputation": 5681120186658, - "rshares": 0, - "voter": "josegaldame" - }, - { - "percent": "10000", - "reputation": 2853104844, - "rshares": 0, - "voter": "lydia2018" - }, - { - "percent": "10000", - "reputation": 33561949138, - "rshares": 0, - "voter": "korolyx" - }, - { - "percent": "10000", - "reputation": 84258748, - "rshares": 0, - "voter": "blaqtryb" - }, - { - "percent": "10000", - "reputation": 923078064152, - "rshares": 0, - "voter": "who-knock" - }, - { - "percent": "10000", - "reputation": 19396535882312, - "rshares": 0, - "voter": "montanacellist" - }, - { - "percent": "10000", - "reputation": 5584751, - "rshares": 0, - "voter": "rosario07" - }, - { - "percent": "10000", - "reputation": 13122254511361, - "rshares": 0, - "voter": "cryptoosid" - }, - { - "percent": "10000", - "reputation": 1092592468, - "rshares": 0, - "voter": "thewinner" - }, - { - "percent": "10000", - "reputation": 1021933925318, - "rshares": 0, - "voter": "joaoprobst" - }, - { - "percent": "10000", - "reputation": 1089632001372, - "rshares": 0, - "voter": "yum-yum" - }, - { - "percent": "10000", - "reputation": 1213857176, - "rshares": 0, - "voter": "cocosarayhenry" - }, - { - "percent": "10000", - "reputation": 39363204, - "rshares": 0, - "voter": "gabycapo19" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "crypto-rn" - }, - { - "percent": "10000", - "reputation": 64752023188, - "rshares": 0, - "voter": "victoralf" - }, - { - "percent": "10000", - "reputation": 45150432632, - "rshares": 0, - "voter": "imamhidayat" - }, - { - "percent": "10000", - "reputation": -56545764675, - "rshares": 0, - "voter": "keuxal" - }, - { - "percent": "10000", - "reputation": 28327487790, - "rshares": 0, - "voter": "dragos18" - }, - { - "percent": "10000", - "reputation": -51078362, - "rshares": 0, - "voter": "mareqqq" - }, - { - "percent": "10000", - "reputation": 27946911293, - "rshares": 0, - "voter": "kyler2018" - }, - { - "percent": "10000", - "reputation": 7274318398, - "rshares": 0, - "voter": "nikossal" - }, - { - "percent": "10000", - "reputation": 4075238550, - "rshares": 0, - "voter": "sgtalienjr" - }, - { - "percent": "10000", - "reputation": 643340785882, - "rshares": 0, - "voter": "serfer307" - }, - { - "percent": "10000", - "reputation": 1137208219, - "rshares": 0, - "voter": "freddyfdg2" - }, - { - "percent": "10000", - "reputation": 390381235110, - "rshares": 0, - "voter": "impulse-moses" - }, - { - "percent": "10000", - "reputation": 24614626235, - "rshares": 0, - "voter": "mahesh8668" - }, - { - "percent": "10000", - "reputation": 1088904842, - "rshares": 0, - "voter": "sandy0526" - }, - { - "percent": "10000", - "reputation": 2375318762, - "rshares": 0, - "voter": "hendrysono" - }, - { - "percent": "10000", - "reputation": 6167203131, - "rshares": 0, - "voter": "kaycrown" - }, - { - "percent": "10000", - "reputation": 3562900762977, - "rshares": 0, - "voter": "informatica" - }, - { - "percent": "10000", - "reputation": 319480738, - "rshares": 0, - "voter": "carlosng18" - }, - { - "percent": "10000", - "reputation": 15040238400, - "rshares": 0, - "voter": "curiouscouple22" - }, - { - "percent": "10000", - "reputation": 1044861254, - "rshares": 0, - "voter": "arjunyadav" - }, - { - "percent": "10000", - "reputation": 5081861583378, - "rshares": 0, - "voter": "brightvibes" - }, - { - "percent": "10000", - "reputation": 10089019706, - "rshares": 0, - "voter": "ramirez-art" - }, - { - "percent": "10000", - "reputation": 5082319971, - "rshares": 0, - "voter": "songcaoxian" - }, - { - "percent": "10000", - "reputation": 3002171996, - "rshares": 0, - "voter": "skourav" - }, - { - "percent": "10000", - "reputation": 101880592, - "rshares": 0, - "voter": "zhansultan" - }, - { - "percent": "-10000", - "reputation": 94731362, - "rshares": 0, - "voter": "luisito2508" - }, - { - "percent": "10000", - "reputation": 383566465, - "rshares": 0, - "voter": "zeusobotic" - }, - { - "percent": "10000", - "reputation": 2091304434, - "rshares": 0, - "voter": "marcos1961" - }, - { - "percent": "10000", - "reputation": 123946064, - "rshares": 0, - "voter": "sergejspi" - }, - { - "percent": "10000", - "reputation": 789303216252, - "rshares": 0, - "voter": "magoo57" - }, - { - "percent": "10000", - "reputation": 2541515, - "rshares": 0, - "voter": "yeuls" - }, - { - "percent": "10000", - "reputation": 416718956, - "rshares": 0, - "voter": "alexamaestre" - }, - { - "percent": "10000", - "reputation": 179983689531, - "rshares": 0, - "voter": "kanika-44" - }, - { - "percent": "10000", - "reputation": 364305100, - "rshares": 0, - "voter": "massielcarolina" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "rajboy13" - }, - { - "percent": "10000", - "reputation": 635014329, - "rshares": 0, - "voter": "franklin777" - }, - { - "percent": "10000", - "reputation": 9556000, - "rshares": 0, - "voter": "irfanismail1" - }, - { - "percent": "10000", - "reputation": 227794512382, - "rshares": 0, - "voter": "kamtasia" - }, - { - "percent": "10000", - "reputation": 204297994, - "rshares": 0, - "voter": "rameshmech222" - }, - { - "percent": "10000", - "reputation": 2901771, - "rshares": 0, - "voter": "giddiik" - }, - { - "percent": "10000", - "reputation": 477431438966, - "rshares": 0, - "voter": "dreamfirefly" - }, - { - "percent": "10000", - "reputation": 601220867, - "rshares": 0, - "voter": "deepanshu3236" - }, - { - "percent": "10000", - "reputation": 68724150801, - "rshares": 0, - "voter": "kissormeetei" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "megatronxd" - }, - { - "percent": "10000", - "reputation": 1545446819, - "rshares": 0, - "voter": "lovecharles2004" - }, - { - "percent": "10000", - "reputation": 153738794015, - "rshares": 0, - "voter": "foridulislam21" - }, - { - "percent": "10000", - "reputation": 794089776, - "rshares": 0, - "voter": "videosfactory" - }, - { - "percent": "10000", - "reputation": 1622399486565, - "rshares": 0, - "voter": "semtroneum" - }, - { - "percent": "10000", - "reputation": 758723464, - "rshares": 0, - "voter": "mehangi" - }, - { - "percent": "10000", - "reputation": 8749970696, - "rshares": 0, - "voter": "mik1" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "bobbyjoe23" - }, - { - "percent": "10000", - "reputation": 506340573, - "rshares": 0, - "voter": "ashraf471" - }, - { - "percent": "10000", - "reputation": 35387161267, - "rshares": 0, - "voter": "seolana" - }, - { - "percent": "10000", - "reputation": 603325004098, - "rshares": 0, - "voter": "eccles" - }, - { - "percent": "10000", - "reputation": 3041836202, - "rshares": 0, - "voter": "emprendedor10" - }, - { - "percent": "10000", - "reputation": 118637561941, - "rshares": 0, - "voter": "grayheaven" - }, - { - "percent": "10000", - "reputation": 5930253366, - "rshares": 0, - "voter": "talwindersingh91" - }, - { - "percent": "10000", - "reputation": 35101477131, - "rshares": 0, - "voter": "jingsaiy" - }, - { - "percent": "10000", - "reputation": 2693573458412, - "rshares": 0, - "voter": "carbodexkim" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "noname-iznogood" - }, - { - "percent": "10000", - "reputation": 1272026, - "rshares": 0, - "voter": "jvasquez1192" - }, - { - "percent": "10000", - "reputation": 6589465534, - "rshares": 0, - "voter": "barradalagoa.tur" - }, - { - "percent": "10000", - "reputation": 4839553883, - "rshares": 0, - "voter": "ericaobrogo25" - }, - { - "percent": "10000", - "reputation": 198700434, - "rshares": 0, - "voter": "sarakhan00" - }, - { - "percent": "10000", - "reputation": 223788343, - "rshares": 0, - "voter": "shankz29" - }, - { - "percent": "10000", - "reputation": 507413085, - "rshares": 0, - "voter": "imjustbad" - }, - { - "percent": "10000", - "reputation": 3601593891, - "rshares": 0, - "voter": "ratizon" - }, - { - "percent": "10000", - "reputation": 1349459182, - "rshares": 0, - "voter": "luialejavencar" - }, - { - "percent": "10000", - "reputation": 4802252298, - "rshares": 0, - "voter": "orshanec" - }, - { - "percent": "10000", - "reputation": 38259757, - "rshares": 0, - "voter": "steemjasmyn" - }, - { - "percent": "10000", - "reputation": 242938700, - "rshares": 0, - "voter": "ritband" - }, - { - "percent": "10000", - "reputation": 86111896644, - "rshares": 0, - "voter": "yetzysavir" - }, - { - "percent": "10000", - "reputation": 33498757, - "rshares": 0, - "voter": "dannywtf6" - }, - { - "percent": "10000", - "reputation": 4045632622, - "rshares": 0, - "voter": "vnhh" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "plmorin" - }, - { - "percent": "10000", - "reputation": 2367797, - "rshares": 0, - "voter": "rameez693" - }, - { - "percent": "10000", - "reputation": 54460615, - "rshares": 0, - "voter": "sikandarhussain" - }, - { - "percent": "10000", - "reputation": 109649668569655, - "rshares": 0, - "voter": "julianhorack" - }, - { - "percent": "10000", - "reputation": -637041519266, - "rshares": 0, - "voter": "mib-coin-usa" - }, - { - "percent": "10000", - "reputation": 317059690, - "rshares": 0, - "voter": "nana404" - }, - { - "percent": "10000", - "reputation": 618923595, - "rshares": 0, - "voter": "lana1916" - }, - { - "percent": "10000", - "reputation": 90101841475, - "rshares": 0, - "voter": "niosper" - }, - { - "percent": "10000", - "reputation": 309697668, - "rshares": 0, - "voter": "tokiblack" - }, - { - "percent": "10000", - "reputation": 216020035070, - "rshares": 0, - "voter": "agolasol" - }, - { - "percent": "10000", - "reputation": 11210087305887, - "rshares": 0, - "voter": "krazzytrukker" - }, - { - "percent": "10000", - "reputation": 24808694, - "rshares": 0, - "voter": "hamza563" - }, - { - "percent": "10000", - "reputation": 197291, - "rshares": 0, - "voter": "oubinxx" - }, - { - "percent": "10000", - "reputation": 90198657212, - "rshares": 0, - "voter": "steemzvibe" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "yasbitoshi" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "elenadeav" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "moisesdario" - }, - { - "percent": "10000", - "reputation": 68848765, - "rshares": 0, - "voter": "alisingh" - }, - { - "percent": "10000", - "reputation": 26537238412, - "rshares": 0, - "voter": "bhojpuri" - }, - { - "percent": "0", - "reputation": 870848080, - "rshares": 0, - "voter": "caduvaca" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "makcbv" - }, - { - "percent": "10000", - "reputation": 206851823620, - "rshares": 0, - "voter": "reckoner.dev" - }, - { - "percent": "10000", - "reputation": 4633675, - "rshares": 0, - "voter": "cazing" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Hello, World!\n\nMy intention is not to make it into top 19. I could name many other candidates (clearly more than 19) with greater commitment to steem(it) who are a lot more appropriate as witnesses, but I'm sure I can be useful as a backup witness.\n\nI introduced myself here:\nhttps://steemit.com/introduceyourself/@gtg/hello-world\nThose of you who use https://steemit.chat know me as **Gandalf**.\nFor some time, I\u2019ve been using my magic powers (mostly those related to security and infrastructure) to improve steemit. For example, I\u2019ve improved our \"Perfect\" Forward Secrecy and made our communication with site more secure.\n\nMy **seed node** (EU based):\n`seed-node = gtg.steem.house:2001`\nYou can also use it to rsync data dir\n`rsync -Pa rsync://gtg.steem.house/witness_node_data_dir .`\n\nMy **witness node** is on a separate data center (an EU-based one as well).\nIf you believe I can be of value to steemit, please vote for me:\n`vote_for_witness YOURACCOUNT gtg true true`\n\n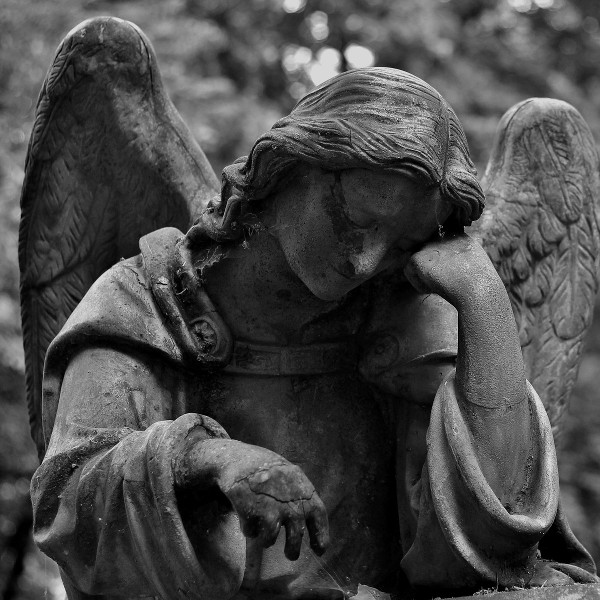", - "body_length": 1023, - "cashout_time": "1969-12-31T23:59:59", - "category": "witness-category", - "children": 231, - "created": "2016-08-05T14:02:24", - "curator_payout_value": "231.879 HBD", - "depth": 0, - "json_metadata": "{\"tags\":[\"witness-category\"],\"links\":[\"https://steemit.com/introduceyourself/@gtg/hello-world\"]}", - "last_payout": "2016-09-05T05:17:57", - "last_update": "2016-08-05T14:02:24", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 61504610477823, - "parent_author": "", - "parent_permlink": "witness-category", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "witness-gtg", - "post_id": 624896, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Witness \"gtg\"", - "title": "Witness \"gtg\"", - "total_payout_value": "703.088 HBD", - "url": "/witness-category/@gtg/witness-gtg" - }, - "entry_id": 3, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 25398689181, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Zaufanie do delegata si\u0119 liczy. Jego narodowo\u015b\u0107 nie.\nG\u0142osowa\u0107 warto, \u015bwiadomie.\nRozumiem, \u017ce nie ka\u017cdy ma czas i ch\u0119ci, by by\u0107 na bie\u017c\u0105co z tym, co tu si\u0119 dzieje 'od kuchni'. Ja staram si\u0119 przygl\u0105da\u0107 temu uwa\u017cnie i g\u0142osuj\u0119 na tych, kt\u00f3rzy sobie zas\u0142u\u017cyli na to wg mojej skromnej, subiektywnej oceny.\nSam r\u00f3wnie\u017c b\u0119d\u0119 si\u0119 stara\u0142 o wy\u017csz\u0105 pozycj\u0119 na li\u015bcie, ale na razie zbyt wiele innych zaj\u0119\u0107 (zwi\u0105zanych ze steemit)\nAby g\u0142osowa\u0107 tak jak ja mo\u017cna w **cli_wallet** wykona\u0107 polecenie\n`set_voting_proxy \"youraccountname\" \"gtg\" true`\nZr\u00f3b to tylko wtedy je\u015bli jeste\u015b w stanie zaufa\u0107 mi co do moich wybor\u00f3w w sprawie delegat\u00f3w.", - "body_length": 622, - "cashout_time": "1969-12-31T23:59:59", - "category": "polish", - "children": 1, - "created": "2016-08-03T12:39:42", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"polish\"]}", - "last_payout": "2016-09-03T03:24:06", - "last_update": "2016-08-03T12:39:42", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 25398689181, - "parent_author": "mefisto", - "parent_permlink": "pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z", - "post_id": 579859, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "[PL] Czy polska spo\u0142eczno\u015b\u0107 powinna mie\u0107 swojego delegata (witness) ?", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/polish/@mefisto/pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness#@gtg/re-mefisto-pl-czy-polska-spolecznosc-powinna-miec-swojego-delegata-witness-20160803t123936611z" - }, - "entry_id": 2, - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "blog": "gtg", - "comment": { - "active_votes": [ - { - "percent": "10000", - "reputation": 159367821767558, - "rshares": 14835621194, - "voter": "gtg" - } - ], - "author": "gtg", - "author_reputation": 159367821767558, - "beneficiaries": [], - "body": "Happened to me, my 3rd Jul post https://steemit.com/introduceyourself/@gtg/hello-world \nHit $723.07 at 23:19 UTC+02 ended up ~$256", - "body_length": 130, - "cashout_time": "1969-12-31T23:59:59", - "category": "catharsis", - "children": 0, - "created": "2016-07-10T09:37:30", - "curator_payout_value": "0.000 HBD", - "depth": 1, - "json_metadata": "{\"tags\":[\"catharsis\"],\"links\":[\"https://steemit.com/introduceyourself/@gtg/hello-world\"]}", - "last_payout": "2016-08-24T21:48:48", - "last_update": "2016-07-10T09:37:30", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 14835621194, - "parent_author": "pfunk", - "parent_permlink": "bear-with-me-while-i-bitch-about-losing-usd1000-on-a-post-due-to-the-july-4th-payout-and-fork", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "re-pfunk-bear-with-me-while-i-bitch-about-losing-usd1000-on-a-post-due-to-the-july-4th-payout-and-fork-20160710t093728262z", - "post_id": 72863, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Bear with me while I bitch about losing $1000 on a post due to the July 4th payout and fork", - "title": "", - "total_payout_value": "0.000 HBD", - "url": "/catharsis/@pfunk/bear-with-me-while-i-bitch-about-losing-usd1000-on-a-post-due-to-the-july-4th-payout-and-fork#@gtg/re-pfunk-bear-with-me-while-i-bitch-about-losing-usd1000-on-a-post-due-to-the-july-4th-payout-and-fork-20160710t093728262z" - }, - "entry_id": 1, - "reblogged_on": "1970-01-01T00:00:00" - } -] diff --git a/hived/tavern/condenser_api_patterns/get_blog/gtg.tavern.yaml b/hived/tavern/condenser_api_patterns/get_blog/gtg.tavern.yaml deleted file mode 100644 index a61cb9b28e5bb54aff833add6960bdd5949516b8..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_blog/gtg.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_blog - - marks: - - patterntest - - xfail #follow_api_plugin not enabled. - - includes: - - !include ../../common.yaml - - stages: - - name: get_blog - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_blog" - params: ["gtg", 100, 100] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "gtg" - directory: "condenser_api_patterns/get_blog" diff --git a/hived/tavern/condenser_api_patterns/get_blog/steemit.pat.json b/hived/tavern/condenser_api_patterns/get_blog/steemit.pat.json deleted file mode 100644 index 3f46182e0727370314ba3d2f8a539801bcd499c5..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_blog/steemit.pat.json +++ /dev/null @@ -1,3447 +0,0 @@ -[ - { - "blog": "steemit", - "comment": { - "active_votes": [ - { - "percent": "100", - "reputation": 240292002602347, - "rshares": 375241, - "voter": "dantheman" - }, - { - "percent": "10000", - "reputation": 190869539046, - "rshares": 886132, - "voter": "mr11acdee" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 5100, - "voter": "steemit78" - }, - { - "percent": "10000", - "reputation": 85602977273, - "rshares": 1259167, - "voter": "anonymous" - }, - { - "percent": "10000", - "reputation": 159370, - "rshares": 318519, - "voter": "hello" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 153384, - "voter": "world" - }, - { - "percent": "0", - "reputation": 94449026656258, - "rshares": -936400, - "voter": "ned" - }, - { - "percent": "10000", - "reputation": 18646364, - "rshares": 59412, - "voter": "fufubar1" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 14997, - "voter": "anonymous1" - }, - { - "percent": "100", - "reputation": 1993860928264, - "rshares": 1441, - "voter": "red" - }, - { - "percent": "10000", - "reputation": 94312688970406, - "rshares": 551390835500, - "voter": "liondani" - }, - { - "percent": "10000", - "reputation": 27406317697121, - "rshares": 82748, - "voter": "roadscape" - }, - { - "percent": "100", - "reputation": 118819064085695, - "rshares": 10772, - "voter": "xeroc" - }, - { - "percent": "10000", - "reputation": 32771884740822, - "rshares": 7685088000, - "voter": "markopaasila" - }, - { - "percent": "10000", - "reputation": 11388588016470, - "rshares": 454510724, - "voter": "tshering-tamang" - }, - { - "percent": "10000", - "reputation": -1240421481231, - "rshares": 681946946, - "voter": "romangelsi" - }, - { - "percent": "10000", - "reputation": 4753753353368, - "rshares": 504895891, - "voter": "dedmatvey" - }, - { - "percent": "10000", - "reputation": 4604092068298, - "rshares": 498863058, - "voter": "joelinux" - }, - { - "percent": "0", - "reputation": 1007246031801, - "rshares": 9590417, - "voter": "piranhax" - }, - { - "percent": "10000", - "reputation": -681981461850, - "rshares": 473258270, - "voter": "ned-reddit-login" - }, - { - "percent": "3600", - "reputation": 1043419862504, - "rshares": 0, - "voter": "fernando-sanz" - }, - { - "percent": "10000", - "reputation": 1185517433922, - "rshares": 425903066, - "voter": "gekko" - }, - { - "percent": "10000", - "reputation": -505427556815, - "rshares": 381805870, - "voter": "gilang-ramadhan" - }, - { - "percent": "10000", - "reputation": 14430883217, - "rshares": 392459059, - "voter": "kamvreto" - }, - { - "percent": "10000", - "reputation": 1591969083452385, - "rshares": 422984262, - "voter": "acidyo" - }, - { - "percent": "10000", - "reputation": 3148075991236, - "rshares": 47179379651, - "voter": "tosch" - }, - { - "percent": "10000", - "reputation": 283933253441837, - "rshares": 7831667988, - "voter": "klye" - }, - { - "percent": "10000", - "reputation": 4042732628956, - "rshares": 1019950749, - "voter": "coar" - }, - { - "percent": "1509", - "reputation": 8801781140235, - "rshares": 1746058458, - "voter": "murh" - }, - { - "percent": "100", - "reputation": 24151212829722, - "rshares": 0, - "voter": "ashe-oro" - }, - { - "percent": "10000", - "reputation": 4291149730479, - "rshares": 22639073725, - "voter": "drinkzya" - }, - { - "percent": "10000", - "reputation": 26408240002629, - "rshares": 0, - "voter": "hien-tran" - }, - { - "percent": "10000", - "reputation": -12409054499907, - "rshares": 0, - "voter": "noganoo" - }, - { - "percent": "10000", - "reputation": 1013326481658, - "rshares": 742566481, - "voter": "patrick-g" - }, - { - "percent": "10000", - "reputation": 2457670192450, - "rshares": 40624969, - "voter": "ben99" - }, - { - "percent": "10000", - "reputation": 5852240682118, - "rshares": 0, - "voter": "tskeene" - }, - { - "percent": "10000", - "reputation": 1835960725851, - "rshares": 1742755097, - "voter": "sunshinecrypto" - }, - { - "percent": "10000", - "reputation": 352970428645376, - "rshares": 0, - "voter": "roelandp" - }, - { - "percent": "10000", - "reputation": 60543564391215, - "rshares": 0, - "voter": "stealthtrader" - }, - { - "percent": "10000", - "reputation": 356176599126, - "rshares": 108855472, - "voter": "kingtylervvs" - }, - { - "percent": "10000", - "reputation": 17498320798649, - "rshares": 0, - "voter": "picokernel" - }, - { - "percent": "10000", - "reputation": 229959978079725, - "rshares": 0, - "voter": "ausbitbank" - }, - { - "percent": "10000", - "reputation": 18714483502974, - "rshares": 485112237, - "voter": "marinabogumil" - }, - { - "percent": "10000", - "reputation": 7005072232924, - "rshares": 0, - "voter": "sebastien" - }, - { - "percent": "10000", - "reputation": 987629623608, - "rshares": 917398502, - "voter": "decrypt" - }, - { - "percent": "10000", - "reputation": 37145059769006, - "rshares": 5067187498, - "voter": "senseiteekay" - }, - { - "percent": "10000", - "reputation": 196800352745, - "rshares": 5154897955, - "voter": "r33drum" - }, - { - "percent": "10000", - "reputation": 378844291332, - "rshares": 5033902237, - "voter": "cryptosi" - }, - { - "percent": "10000", - "reputation": 56189611335832, - "rshares": 1037079223, - "voter": "condra" - }, - { - "percent": "10000", - "reputation": 1844972282153, - "rshares": 233032838, - "voter": "jearson" - }, - { - "percent": "10000", - "reputation": 123261195963, - "rshares": 240809500, - "voter": "tritium" - }, - { - "percent": "10000", - "reputation": 373877166913597, - "rshares": 123321995, - "voter": "allmonitors" - }, - { - "percent": "10000", - "reputation": 29008237643, - "rshares": 226074637, - "voter": "artjedi" - }, - { - "percent": "10000", - "reputation": 11311191021706, - "rshares": 931542394, - "voter": "anduweb" - }, - { - "percent": "10000", - "reputation": 273611816138333, - "rshares": 2292983350, - "voter": "inertia" - }, - { - "percent": "10000", - "reputation": 3703778977425, - "rshares": 128561059, - "voter": "maximkichev" - }, - { - "percent": "10000", - "reputation": 113908011659, - "rshares": 183438273, - "voter": "a9inchcock" - }, - { - "percent": "10000", - "reputation": 1795616951424, - "rshares": 266262926, - "voter": "desmonid" - }, - { - "percent": "10000", - "reputation": 1210922323731, - "rshares": 71498008, - "voter": "madhatting" - }, - { - "percent": "10000", - "reputation": 4957775761011, - "rshares": 23726644841, - "voter": "ubg" - }, - { - "percent": "10000", - "reputation": 15057444088214, - "rshares": 3741408303, - "voter": "royaltiffany" - }, - { - "percent": "10000", - "reputation": 4858418486, - "rshares": 131577259, - "voter": "gribgo" - }, - { - "percent": "10000", - "reputation": 977136623294522, - "rshares": 12371398765, - "voter": "deanliu" - }, - { - "percent": "10000", - "reputation": 2110857461943, - "rshares": 28907874049, - "voter": "orm" - }, - { - "percent": "10000", - "reputation": 513405006906, - "rshares": 528988007, - "voter": "qonq99" - }, - { - "percent": "10000", - "reputation": 9853253860, - "rshares": 129537329, - "voter": "rd7783" - }, - { - "percent": "10000", - "reputation": 100161586091, - "rshares": 615020728, - "voter": "slava" - }, - { - "percent": "10000", - "reputation": 5788213054794, - "rshares": 0, - "voter": "flyboyzombie" - }, - { - "percent": "1", - "reputation": -2135533532384, - "rshares": 0, - "voter": "social" - }, - { - "percent": "10000", - "reputation": 281653583054, - "rshares": 100102503, - "voter": "sictransitgloria" - }, - { - "percent": "10000", - "reputation": 1405302097754, - "rshares": 95219365, - "voter": "curator" - }, - { - "percent": "10000", - "reputation": -385936503026, - "rshares": 232295871, - "voter": "dubovoy" - }, - { - "percent": "10000", - "reputation": 35204206, - "rshares": 0, - "voter": "solos" - }, - { - "percent": "10000", - "reputation": 3895922975, - "rshares": 0, - "voter": "alvintang" - }, - { - "percent": "10000", - "reputation": 5095600502, - "rshares": 96945805, - "voter": "creatorgalaxy" - }, - { - "percent": "10000", - "reputation": 3804816886, - "rshares": 99813231, - "voter": "trigonice29" - }, - { - "percent": "10000", - "reputation": 3133295975341, - "rshares": 0, - "voter": "blysards" - }, - { - "percent": "10000", - "reputation": 76132392265, - "rshares": 0, - "voter": "nick.kharchenko" - }, - { - "percent": "10000", - "reputation": 35524530484330, - "rshares": 3721016208, - "voter": "uwe69" - }, - { - "percent": "10000", - "reputation": 3240369658, - "rshares": 1279854, - "voter": "nigmat" - }, - { - "percent": "10000", - "reputation": 352641672569442, - "rshares": 13974353753, - "voter": "magicmonk" - }, - { - "percent": "10000", - "reputation": 4742036843307, - "rshares": 4968585456, - "voter": "satoshifpv" - }, - { - "percent": "10000", - "reputation": -5843262501385, - "rshares": 1660613178, - "voter": "naturalista" - }, - { - "percent": "10000", - "reputation": 6121114155708, - "rshares": 10847083143, - "voter": "metrox" - }, - { - "percent": "10000", - "reputation": 28131790010, - "rshares": 18340928, - "voter": "bestmalik" - }, - { - "percent": "10000", - "reputation": 169634730, - "rshares": 59563315, - "voter": "kolyan31" - }, - { - "percent": "10000", - "reputation": 6332027618857, - "rshares": 710989138, - "voter": "romancs" - }, - { - "percent": "10000", - "reputation": 241302356483, - "rshares": 59366614, - "voter": "luke490" - }, - { - "percent": "10000", - "reputation": 137324193, - "rshares": 58762473, - "voter": "bro66" - }, - { - "percent": "10000", - "reputation": 387642408233756, - "rshares": 201822591, - "voter": "future24" - }, - { - "percent": "10000", - "reputation": 2228947083088, - "rshares": 58623688, - "voter": "mythras" - }, - { - "percent": "10000", - "reputation": -1158370380860, - "rshares": 56536509, - "voter": "imarealboy777" - }, - { - "percent": "0", - "reputation": -29653420748873, - "rshares": 0, - "voter": "matrixdweller" - }, - { - "percent": "10000", - "reputation": -862242552844, - "rshares": 48299362, - "voter": "smartguylabcoat" - }, - { - "percent": "10000", - "reputation": 34436412607, - "rshares": 59157099, - "voter": "mabiturm" - }, - { - "percent": "10000", - "reputation": -1327285945383, - "rshares": 48283979, - "voter": "captainamerica" - }, - { - "percent": "10000", - "reputation": 469950374409, - "rshares": 54761612, - "voter": "edbriv" - }, - { - "percent": "10000", - "reputation": 61353484752754, - "rshares": 0, - "voter": "anarchyhasnogods" - }, - { - "percent": "10000", - "reputation": 1228277364481, - "rshares": 865125771, - "voter": "rittr" - }, - { - "percent": "10000", - "reputation": 136837946011250, - "rshares": 0, - "voter": "tumutanzi" - }, - { - "percent": "10000", - "reputation": 416108237939, - "rshares": 92614447, - "voter": "jelloducky" - }, - { - "percent": "10000", - "reputation": 21641668306, - "rshares": 52740989, - "voter": "tcstix" - }, - { - "percent": "10000", - "reputation": 143728394, - "rshares": 49467477, - "voter": "friedwater" - }, - { - "percent": "10000", - "reputation": -32027729845, - "rshares": 57366185, - "voter": "denisdiaz" - }, - { - "percent": "10000", - "reputation": 1957696033438, - "rshares": 0, - "voter": "gbonikz" - }, - { - "percent": "10000", - "reputation": 20197423275926, - "rshares": 132070449, - "voter": "loganarchy" - }, - { - "percent": "10000", - "reputation": -5027605173, - "rshares": 48811442, - "voter": "love-spirit-nerd" - }, - { - "percent": "10000", - "reputation": 38373343517335, - "rshares": 0, - "voter": "darkflame" - }, - { - "percent": "10000", - "reputation": 28694344106492, - "rshares": 0, - "voter": "sneak" - }, - { - "percent": "10000", - "reputation": 48954175480649, - "rshares": 0, - "voter": "jacobcards" - }, - { - "percent": "10000", - "reputation": 6900034272, - "rshares": 51549585, - "voter": "dikanevn" - }, - { - "percent": "10000", - "reputation": 121015180119970, - "rshares": 0, - "voter": "shieha" - }, - { - "percent": "10000", - "reputation": 189415327585, - "rshares": 54017869, - "voter": "zelious" - }, - { - "percent": "0", - "reputation": 7439666530016, - "rshares": 0, - "voter": "allyouneedtoknow" - }, - { - "percent": "10000", - "reputation": 280674585514224, - "rshares": 0, - "voter": "justyy" - }, - { - "percent": "10000", - "reputation": 171005551503977, - "rshares": 50129944, - "voter": "freebornangel" - }, - { - "percent": "10000", - "reputation": 35747770521, - "rshares": 53196086, - "voter": "f1111111" - }, - { - "percent": "10000", - "reputation": 29087925814502, - "rshares": 0, - "voter": "anomaly" - }, - { - "percent": "10000", - "reputation": 182276949970503, - "rshares": 52394017140, - "voter": "jack8831" - }, - { - "percent": "10000", - "reputation": 56377206283, - "rshares": 0, - "voter": "buckland" - }, - { - "percent": "10", - "reputation": -948627542088, - "rshares": 0, - "voter": "guest123" - }, - { - "percent": "10000", - "reputation": 559165550719, - "rshares": 0, - "voter": "syahhiran" - }, - { - "percent": "10000", - "reputation": 1256588330819, - "rshares": 0, - "voter": "rarcntv" - }, - { - "percent": "10000", - "reputation": 8202257082095, - "rshares": 0, - "voter": "nataleeoliver" - }, - { - "percent": "10000", - "reputation": 374029964227311, - "rshares": 0, - "voter": "goldmatters" - }, - { - "percent": "10000", - "reputation": 104734123628606, - "rshares": 0, - "voter": "gamer00" - }, - { - "percent": "10000", - "reputation": 48134259536775, - "rshares": 0, - "voter": "curiesea" - }, - { - "percent": "10000", - "reputation": 338501754215, - "rshares": 0, - "voter": "missmarzipan" - }, - { - "percent": "3000", - "reputation": 4741271817501, - "rshares": 0, - "voter": "thejohalfiles" - }, - { - "percent": "10000", - "reputation": 9325804713964, - "rshares": 0, - "voter": "steemwart" - }, - { - "percent": "10000", - "reputation": 16372175688451, - "rshares": 0, - "voter": "mapesa" - }, - { - "percent": "10000", - "reputation": 16302945972, - "rshares": 0, - "voter": "max-max" - }, - { - "percent": "10000", - "reputation": 4189940271139, - "rshares": 0, - "voter": "joshuaatiemo" - }, - { - "percent": "10000", - "reputation": 47533070564928, - "rshares": 0, - "voter": "jumaidafajar" - }, - { - "percent": "10000", - "reputation": 419129457, - "rshares": 0, - "voter": "leolina1" - }, - { - "percent": "10000", - "reputation": 10786227734650, - "rshares": 0, - "voter": "rebatesteem" - }, - { - "percent": "10000", - "reputation": 23963417769759, - "rshares": 0, - "voter": "aidancloquell" - }, - { - "percent": "10000", - "reputation": 8108634791, - "rshares": 0, - "voter": "rm802" - }, - { - "percent": "10000", - "reputation": 19259306626624, - "rshares": 0, - "voter": "krasotka" - }, - { - "percent": "10000", - "reputation": 5123476713510, - "rshares": 0, - "voter": "alamyrjunior" - }, - { - "percent": "10000", - "reputation": 2968937121056, - "rshares": 0, - "voter": "networker5" - }, - { - "percent": "10000", - "reputation": 803492751996, - "rshares": 0, - "voter": "evdoggformayor" - }, - { - "percent": "10000", - "reputation": 19610705040132, - "rshares": 0, - "voter": "askari" - }, - { - "percent": "10000", - "reputation": 5038471003192, - "rshares": 0, - "voter": "blockrush" - }, - { - "percent": "10000", - "reputation": 44575515286240, - "rshares": 0, - "voter": "barvon" - }, - { - "percent": "10000", - "reputation": 19787083619454, - "rshares": 0, - "voter": "fajarsdq" - }, - { - "percent": "10000", - "reputation": 4243903288898, - "rshares": 0, - "voter": "juandemarte" - }, - { - "percent": "10000", - "reputation": 10704737102960, - "rshares": 0, - "voter": "lazarescu.irinel" - }, - { - "percent": "10000", - "reputation": 14660082985165, - "rshares": 0, - "voter": "thedeplorable1" - }, - { - "percent": "10000", - "reputation": 398504517684857, - "rshares": 0, - "voter": "kalemandra" - }, - { - "percent": "5000", - "reputation": 32583772981101, - "rshares": 0, - "voter": "ades" - }, - { - "percent": "10000", - "reputation": 11856803019184, - "rshares": 0, - "voter": "rizkiavonna" - }, - { - "percent": "10000", - "reputation": 8807709091999, - "rshares": 0, - "voter": "tohamy7" - }, - { - "percent": "10000", - "reputation": 12325904700970, - "rshares": 0, - "voter": "justinashby" - }, - { - "percent": "10000", - "reputation": 65535006526149, - "rshares": 0, - "voter": "thereikiforest" - }, - { - "percent": "10000", - "reputation": 1990050986, - "rshares": 0, - "voter": "serendipitie" - }, - { - "percent": "10000", - "reputation": 8241421646010, - "rshares": 0, - "voter": "anwarabdullah" - }, - { - "percent": "10000", - "reputation": 3968803698350, - "rshares": 0, - "voter": "rocketbeee" - }, - { - "percent": "10000", - "reputation": 214940431491515, - "rshares": 0, - "voter": "ackza" - }, - { - "percent": "10000", - "reputation": 471040634648, - "rshares": 0, - "voter": "nilim" - }, - { - "percent": "10000", - "reputation": 872253711, - "rshares": 0, - "voter": "renatrazumov" - }, - { - "percent": "10000", - "reputation": 1163438797757, - "rshares": 0, - "voter": "jelkasmi" - }, - { - "percent": "10000", - "reputation": 794094672611, - "rshares": 0, - "voter": "dimitrya123" - }, - { - "percent": "10000", - "reputation": 4850148191446, - "rshares": 0, - "voter": "crawfish37" - }, - { - "percent": "10000", - "reputation": 48845341788290, - "rshares": 0, - "voter": "laodr" - }, - { - "percent": "10000", - "reputation": 43833051603, - "rshares": 0, - "voter": "lkisaid" - }, - { - "percent": "10000", - "reputation": 2920705765790, - "rshares": 0, - "voter": "mikev" - }, - { - "percent": "10000", - "reputation": 16606765863, - "rshares": 0, - "voter": "hakan0356" - }, - { - "percent": "10000", - "reputation": 646119505336, - "rshares": 0, - "voter": "tasartcraft" - }, - { - "percent": "10000", - "reputation": 190033440499, - "rshares": 0, - "voter": "urmokas" - }, - { - "percent": "10000", - "reputation": 45652148998, - "rshares": 0, - "voter": "michael-fagundes" - }, - { - "percent": "0", - "reputation": 4386202992, - "rshares": 0, - "voter": "kalamur" - }, - { - "percent": "0", - "reputation": 409566886382, - "rshares": 0, - "voter": "abhinavsharma" - }, - { - "percent": "10000", - "reputation": 62721105887, - "rshares": 0, - "voter": "contentguy" - }, - { - "percent": "10000", - "reputation": 22746586322771, - "rshares": 0, - "voter": "globocop" - }, - { - "percent": "10000", - "reputation": 29870130383591, - "rshares": 0, - "voter": "hafizul" - }, - { - "percent": "10000", - "reputation": 215512501868, - "rshares": 0, - "voter": "hothelp1by1" - }, - { - "percent": "10000", - "reputation": 14801286947008, - "rshares": 0, - "voter": "vannfrik" - }, - { - "percent": "10000", - "reputation": 5975764175706, - "rshares": 0, - "voter": "manuel78" - }, - { - "percent": "10000", - "reputation": 1330728109592, - "rshares": 0, - "voter": "brado" - }, - { - "percent": "10000", - "reputation": 18181019075, - "rshares": 0, - "voter": "strateg" - }, - { - "percent": "0", - "reputation": 7196763893, - "rshares": 0, - "voter": "quinsmacqueen" - }, - { - "percent": "10000", - "reputation": 1092260798193, - "rshares": 0, - "voter": "brucebrownftw" - }, - { - "percent": "10000", - "reputation": 512087953369, - "rshares": 0, - "voter": "mrstaf" - }, - { - "percent": "10000", - "reputation": 769439802936, - "rshares": 0, - "voter": "avvah" - }, - { - "percent": "100", - "reputation": 833020053165265, - "rshares": 0, - "voter": "stackin" - }, - { - "percent": "10000", - "reputation": 765950713270, - "rshares": 0, - "voter": "laloelectrix" - }, - { - "percent": "10000", - "reputation": 298990380256, - "rshares": 0, - "voter": "heejaekim" - }, - { - "percent": "10000", - "reputation": 11555767777330, - "rshares": 0, - "voter": "thethreehugs" - }, - { - "percent": "10000", - "reputation": 333248577305, - "rshares": 0, - "voter": "otitrader" - }, - { - "percent": "10000", - "reputation": 518563532954882, - "rshares": 0, - "voter": "clixmoney" - }, - { - "percent": "10000", - "reputation": 622097727947, - "rshares": 0, - "voter": "tryword" - }, - { - "percent": "10000", - "reputation": -634870987521, - "rshares": 0, - "voter": "dioneaguiar" - }, - { - "percent": "10000", - "reputation": 679438518, - "rshares": 0, - "voter": "yann.moalic" - }, - { - "percent": "10000", - "reputation": 35047304553654, - "rshares": 0, - "voter": "shaunf" - }, - { - "percent": "10000", - "reputation": 788462040366, - "rshares": 0, - "voter": "cleemit" - }, - { - "percent": "10000", - "reputation": 671261891680, - "rshares": 0, - "voter": "deeluvli1" - }, - { - "percent": "10000", - "reputation": 78049643501, - "rshares": 0, - "voter": "noval" - }, - { - "percent": "10000", - "reputation": 71053914235, - "rshares": 0, - "voter": "pixzelplethora" - }, - { - "percent": "10000", - "reputation": 11535312131, - "rshares": 0, - "voter": "smart3dweb" - }, - { - "percent": "10000", - "reputation": 2521246416524, - "rshares": 0, - "voter": "joey-cryptoboy" - }, - { - "percent": "10000", - "reputation": 367893852, - "rshares": 0, - "voter": "mateorite" - }, - { - "percent": "10000", - "reputation": 5888846795, - "rshares": 0, - "voter": "somaflaco" - }, - { - "percent": "10000", - "reputation": 2152941530718, - "rshares": 0, - "voter": "enki74" - }, - { - "percent": "10000", - "reputation": 110560203334, - "rshares": 0, - "voter": "jungleebitcoin" - }, - { - "percent": "10000", - "reputation": -69319092879, - "rshares": 0, - "voter": "correctdrop" - }, - { - "percent": "10000", - "reputation": 2058050404764, - "rshares": 0, - "voter": "clintjunior" - }, - { - "percent": "10000", - "reputation": 951539942306, - "rshares": 0, - "voter": "tediursa24" - }, - { - "percent": "10000", - "reputation": 62289248493193, - "rshares": 0, - "voter": "mxzn" - }, - { - "percent": "10000", - "reputation": 1030198306829, - "rshares": 0, - "voter": "hgmsilvergold" - }, - { - "percent": "10000", - "reputation": 1049729257309, - "rshares": 0, - "voter": "antares007" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "tridenspoon333" - }, - { - "percent": "10000", - "reputation": 6633483148, - "rshares": 0, - "voter": "vipek1996" - }, - { - "percent": "10000", - "reputation": 59521008461, - "rshares": 0, - "voter": "prima-nia" - }, - { - "percent": "10000", - "reputation": 51310500722, - "rshares": 0, - "voter": "redris" - }, - { - "percent": "10000", - "reputation": 1162151678669, - "rshares": 0, - "voter": "savetheanimals" - }, - { - "percent": "10000", - "reputation": 63411784193, - "rshares": 0, - "voter": "tarunmewara" - }, - { - "percent": "-10000", - "reputation": 110711587046355, - "rshares": 0, - "voter": "overkillcoin" - }, - { - "percent": "10000", - "reputation": 228450255458, - "rshares": 0, - "voter": "tuakanamorgan" - }, - { - "percent": "10000", - "reputation": 69653104045391, - "rshares": 0, - "voter": "anacristinasilva" - }, - { - "percent": "10000", - "reputation": 20798619412, - "rshares": 0, - "voter": "filmonaut" - }, - { - "percent": "-100", - "reputation": 3899813800176, - "rshares": 0, - "voter": "firstamendment" - }, - { - "percent": "10000", - "reputation": 68635880449, - "rshares": 0, - "voter": "timcrypto" - }, - { - "percent": "10000", - "reputation": 20302398026168, - "rshares": 0, - "voter": "cryptotem" - }, - { - "percent": "10000", - "reputation": 697958030131, - "rshares": 0, - "voter": "mikej" - }, - { - "percent": "10000", - "reputation": 47552691317, - "rshares": 0, - "voter": "hwrs" - }, - { - "percent": "10000", - "reputation": 1877811371, - "rshares": 0, - "voter": "sinai770judea" - }, - { - "percent": "10000", - "reputation": 33832199316, - "rshares": 0, - "voter": "tuneralliance" - }, - { - "percent": "10000", - "reputation": 2837751128, - "rshares": 0, - "voter": "zeji" - }, - { - "percent": "10000", - "reputation": 17435334887, - "rshares": 0, - "voter": "ricoalfianda" - }, - { - "percent": "10000", - "reputation": 2001092299106, - "rshares": 0, - "voter": "satfit" - }, - { - "percent": "10000", - "reputation": 10806312348321, - "rshares": 0, - "voter": "machhour" - }, - { - "percent": "10000", - "reputation": 3405952535, - "rshares": 0, - "voter": "bitgenio" - }, - { - "percent": "10000", - "reputation": 12976028341, - "rshares": 0, - "voter": "ahmedmansi" - }, - { - "percent": "10000", - "reputation": 4101813308863, - "rshares": 0, - "voter": "theoccultcorner" - }, - { - "percent": "10000", - "reputation": 284852594097573, - "rshares": 0, - "voter": "reseller" - }, - { - "percent": "10000", - "reputation": 65572893861, - "rshares": 0, - "voter": "ainsleyjo1952" - }, - { - "percent": "10000", - "reputation": -26425603909, - "rshares": 0, - "voter": "novi" - }, - { - "percent": "10000", - "reputation": 6247941147391, - "rshares": 0, - "voter": "androsform" - }, - { - "percent": "10000", - "reputation": 4409923662602, - "rshares": 0, - "voter": "jooyoung" - }, - { - "percent": "10000", - "reputation": 418242269128, - "rshares": 0, - "voter": "dobro88888888" - }, - { - "percent": "10000", - "reputation": 278893346456, - "rshares": 0, - "voter": "ghaaspur" - }, - { - "percent": "10000", - "reputation": 1464464526853, - "rshares": 0, - "voter": "xodyd2da" - }, - { - "percent": "10000", - "reputation": 184717254436, - "rshares": 0, - "voter": "trisun" - }, - { - "percent": "-10000", - "reputation": 17362709009463, - "rshares": 0, - "voter": "tngflx" - }, - { - "percent": "10000", - "reputation": 303115312, - "rshares": 0, - "voter": "karaban" - }, - { - "percent": "10000", - "reputation": 1884358880088, - "rshares": 0, - "voter": "empath" - }, - { - "percent": "0", - "reputation": 0, - "rshares": 0, - "voter": "pcbildrnoob" - }, - { - "percent": "10000", - "reputation": 181401000658, - "rshares": 0, - "voter": "joecaffeine" - }, - { - "percent": "10000", - "reputation": 7029528119, - "rshares": 0, - "voter": "laolballs" - }, - { - "percent": "10000", - "reputation": 2498676417, - "rshares": 0, - "voter": "braamsteyn7777" - }, - { - "percent": "10000", - "reputation": 1343359566195, - "rshares": 0, - "voter": "udibekwe" - }, - { - "percent": "10000", - "reputation": 331933685755, - "rshares": 0, - "voter": "jackolanternbob" - }, - { - "percent": "10000", - "reputation": 273201532417, - "rshares": 0, - "voter": "rondoncr" - }, - { - "percent": "10000", - "reputation": 104746597592, - "rshares": 0, - "voter": "doubledeeyt" - }, - { - "percent": "10000", - "reputation": 9745075470775, - "rshares": 0, - "voter": "joshvel" - }, - { - "percent": "10000", - "reputation": 20289419458, - "rshares": 0, - "voter": "lembach3d" - }, - { - "percent": "10000", - "reputation": 72792921653, - "rshares": 0, - "voter": "vulturestkn" - }, - { - "percent": "10000", - "reputation": -11092548376, - "rshares": 0, - "voter": "darknessprincess" - }, - { - "percent": "10000", - "reputation": 20447544397525, - "rshares": 0, - "voter": "misrori" - }, - { - "percent": "10000", - "reputation": 463841238681, - "rshares": 0, - "voter": "afrikanprince" - }, - { - "percent": "10000", - "reputation": 104517401250678, - "rshares": 0, - "voter": "jjprac" - }, - { - "percent": "10000", - "reputation": 3091902701938, - "rshares": 0, - "voter": "ayuwandira" - }, - { - "percent": "10000", - "reputation": 8898324586, - "rshares": 0, - "voter": "ilicoin" - }, - { - "percent": "10000", - "reputation": 28866926271333, - "rshares": 0, - "voter": "pkvlogs" - }, - { - "percent": "10000", - "reputation": 251946106284, - "rshares": 0, - "voter": "bitstudio" - }, - { - "percent": "-1000", - "reputation": 1980506790459, - "rshares": 0, - "voter": "taintedblood" - }, - { - "percent": "10000", - "reputation": 682770422710, - "rshares": 0, - "voter": "epsicktick" - }, - { - "percent": "10000", - "reputation": 153975992, - "rshares": 0, - "voter": "csggene3" - }, - { - "percent": "10000", - "reputation": 40104351499, - "rshares": 0, - "voter": "tothemoonin2017" - }, - { - "percent": "10000", - "reputation": 92327401387, - "rshares": 0, - "voter": "geek4geek" - }, - { - "percent": "2500", - "reputation": 26703458140145, - "rshares": 0, - "voter": "kharrazi" - }, - { - "percent": "10000", - "reputation": 926393237238, - "rshares": 0, - "voter": "zufrizal" - }, - { - "percent": "10000", - "reputation": 255497876975, - "rshares": 0, - "voter": "academix87" - }, - { - "percent": "500", - "reputation": 19153420107615, - "rshares": 0, - "voter": "planetenamek" - }, - { - "percent": "10000", - "reputation": 16306785801, - "rshares": 0, - "voter": "muliaeko" - }, - { - "percent": "10000", - "reputation": 231215767703, - "rshares": 0, - "voter": "boyjack" - }, - { - "percent": "10000", - "reputation": 439350325, - "rshares": 0, - "voter": "jatniel" - }, - { - "percent": "10000", - "reputation": 967027557509, - "rshares": 0, - "voter": "juanangel40bcn" - }, - { - "percent": "10000", - "reputation": 26077885556, - "rshares": 0, - "voter": "jodywrites" - }, - { - "percent": "10000", - "reputation": 810135688569, - "rshares": 0, - "voter": "permatek" - }, - { - "percent": "10000", - "reputation": 780337768524, - "rshares": 0, - "voter": "cyberspace" - }, - { - "percent": "10000", - "reputation": 679018914115, - "rshares": 0, - "voter": "fareehasheharyar" - }, - { - "percent": "10000", - "reputation": 47525279939, - "rshares": 0, - "voter": "saynie" - }, - { - "percent": "10000", - "reputation": 713624891901, - "rshares": 0, - "voter": "plainoldme" - }, - { - "percent": "10000", - "reputation": 235305836617, - "rshares": 0, - "voter": "razaqbarry" - }, - { - "percent": "10000", - "reputation": 1209731503393, - "rshares": 0, - "voter": "antoniokarteli" - }, - { - "percent": "10000", - "reputation": 8248374211806, - "rshares": 0, - "voter": "dream.trip" - }, - { - "percent": "10000", - "reputation": 3411826005, - "rshares": 0, - "voter": "reinhardbaust" - }, - { - "percent": "10000", - "reputation": 438679075638, - "rshares": 0, - "voter": "newpioneer" - }, - { - "percent": "10000", - "reputation": 6994381548, - "rshares": 0, - "voter": "melvinbonner" - }, - { - "percent": "10000", - "reputation": -43699775080, - "rshares": 0, - "voter": "zulfahmi2141" - }, - { - "percent": "10000", - "reputation": 171438113871, - "rshares": 0, - "voter": "maninjapan1989" - }, - { - "percent": "10000", - "reputation": 3240603415894, - "rshares": 0, - "voter": "andravasko" - }, - { - "percent": "10000", - "reputation": 17563933807813, - "rshares": 0, - "voter": "gilma" - }, - { - "percent": "10000", - "reputation": 48066228162, - "rshares": 0, - "voter": "tom74" - }, - { - "percent": "10000", - "reputation": 1256083697451, - "rshares": 0, - "voter": "josephfugata" - }, - { - "percent": "10000", - "reputation": 4526614988, - "rshares": 0, - "voter": "bitcointauji" - }, - { - "percent": "10000", - "reputation": 4230071570826, - "rshares": 0, - "voter": "gray00" - }, - { - "percent": "10000", - "reputation": -14848685118, - "rshares": 0, - "voter": "divyang101" - }, - { - "percent": "10000", - "reputation": 48110734587913, - "rshares": 0, - "voter": "bahagia-arbi" - }, - { - "percent": "10000", - "reputation": 4877222464, - "rshares": 0, - "voter": "bhim" - }, - { - "percent": "10000", - "reputation": 5585491362, - "rshares": 0, - "voter": "bickell" - }, - { - "percent": "10000", - "reputation": 48474751589, - "rshares": 0, - "voter": "tfpostman" - }, - { - "percent": "10000", - "reputation": -21112938149, - "rshares": 0, - "voter": "anujkumar" - }, - { - "percent": "5100", - "reputation": 140482604041851, - "rshares": 0, - "voter": "fbslo" - }, - { - "percent": "10000", - "reputation": 20091098175, - "rshares": 0, - "voter": "renijuliani" - }, - { - "percent": "10000", - "reputation": 8320716127, - "rshares": 0, - "voter": "setio" - }, - { - "percent": "10000", - "reputation": 15427543916099, - "rshares": 0, - "voter": "mooncryption" - }, - { - "percent": "10000", - "reputation": 2434330471487, - "rshares": 0, - "voter": "shintamonica" - }, - { - "percent": "10000", - "reputation": 3916912866701, - "rshares": 0, - "voter": "caratzky" - }, - { - "percent": "10000", - "reputation": 10808960235, - "rshares": 0, - "voter": "komrad" - }, - { - "percent": "10000", - "reputation": 253303445, - "rshares": 0, - "voter": "therivernile" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "deep.gohil" - }, - { - "percent": "10000", - "reputation": 1706636906846, - "rshares": 0, - "voter": "thomasduder" - }, - { - "percent": "10000", - "reputation": 93431455759, - "rshares": 0, - "voter": "sensistar" - }, - { - "percent": "10000", - "reputation": 105915849604, - "rshares": 0, - "voter": "abdelone" - }, - { - "percent": "10000", - "reputation": 13504938371, - "rshares": 0, - "voter": "gegec" - }, - { - "percent": "10000", - "reputation": 8475559512816, - "rshares": 0, - "voter": "ambmicheal" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "rincewind" - }, - { - "percent": "10000", - "reputation": 22668422039138, - "rshares": 0, - "voter": "alishannoor" - }, - { - "percent": "10000", - "reputation": 31250011559, - "rshares": 0, - "voter": "ahhjoeinhk" - }, - { - "percent": "10000", - "reputation": 22161276554, - "rshares": 0, - "voter": "kalhiade" - }, - { - "percent": "10000", - "reputation": 26067838103, - "rshares": 0, - "voter": "faridrizkia" - }, - { - "percent": "10000", - "reputation": 489008294936, - "rshares": 0, - "voter": "teamslovenia" - }, - { - "percent": "10000", - "reputation": 86080771148846, - "rshares": 0, - "voter": "xiaoshancun" - }, - { - "percent": "10000", - "reputation": 18812216005, - "rshares": 0, - "voter": "mikewebb274" - }, - { - "percent": "10000", - "reputation": 16886517518, - "rshares": 0, - "voter": "andrath" - }, - { - "percent": "10000", - "reputation": 5010654057, - "rshares": 0, - "voter": "torosan" - }, - { - "percent": "10000", - "reputation": 69430737377176, - "rshares": 0, - "voter": "ghayas" - }, - { - "percent": "10000", - "reputation": 48988494748523, - "rshares": 0, - "voter": "krevasilis" - }, - { - "percent": "100", - "reputation": 3541438533225, - "rshares": 0, - "voter": "koinbot" - }, - { - "percent": "10000", - "reputation": 118599105990, - "rshares": 0, - "voter": "synergy-now" - }, - { - "percent": "10000", - "reputation": 1046602731199, - "rshares": 0, - "voter": "steemblogs" - }, - { - "percent": "10000", - "reputation": 2042790257, - "rshares": 0, - "voter": "tujuhpelita" - }, - { - "percent": "10000", - "reputation": 4224359059633, - "rshares": 0, - "voter": "palani" - }, - { - "percent": "10000", - "reputation": 2505286236282, - "rshares": 0, - "voter": "as-i-see-it" - }, - { - "percent": "10000", - "reputation": 1338831844783, - "rshares": 0, - "voter": "heyeshuang" - }, - { - "percent": "10000", - "reputation": 12195267435725, - "rshares": 0, - "voter": "arslan786" - }, - { - "percent": "10000", - "reputation": 192390896224, - "rshares": 0, - "voter": "cgf117" - }, - { - "percent": "10000", - "reputation": 153970191181, - "rshares": 0, - "voter": "amazingtech100" - }, - { - "percent": "0", - "reputation": 7500168684837, - "rshares": 0, - "voter": "dreamm" - }, - { - "percent": "10000", - "reputation": -4012528688, - "rshares": 0, - "voter": "sweetssssj" - }, - { - "percent": "10000", - "reputation": 2017956520, - "rshares": 0, - "voter": "ceikdo" - }, - { - "percent": "10000", - "reputation": 484759901457, - "rshares": 0, - "voter": "sunsquall" - }, - { - "percent": "10000", - "reputation": 7998705639, - "rshares": 0, - "voter": "muhammadilyas93" - }, - { - "percent": "10000", - "reputation": 17253145691864, - "rshares": 0, - "voter": "cre47iv3" - }, - { - "percent": "10000", - "reputation": 517045098554, - "rshares": 0, - "voter": "joao-cacador" - }, - { - "percent": "3554", - "reputation": 77468752355, - "rshares": 0, - "voter": "jamsphonna" - }, - { - "percent": "-1000", - "reputation": 38277242620416, - "rshares": 0, - "voter": "abbak7" - }, - { - "percent": "10000", - "reputation": 148461146192759, - "rshares": 0, - "voter": "thelifeofjord" - }, - { - "percent": "10000", - "reputation": 146985742512, - "rshares": 0, - "voter": "utpoldebnath" - }, - { - "percent": "10000", - "reputation": 1245938181729, - "rshares": 0, - "voter": "sagorkhan" - }, - { - "percent": "10000", - "reputation": 2662968641, - "rshares": 0, - "voter": "shivpremi" - }, - { - "percent": "100", - "reputation": 19827334573854, - "rshares": 0, - "voter": "shabbirahmad" - }, - { - "percent": "10000", - "reputation": 973118905183, - "rshares": 0, - "voter": "ibeljr" - }, - { - "percent": "10000", - "reputation": 104339117366, - "rshares": 0, - "voter": "umelard" - }, - { - "percent": "10000", - "reputation": 7224362399, - "rshares": 0, - "voter": "stijndehaan" - }, - { - "percent": "10000", - "reputation": 876649496170, - "rshares": 0, - "voter": "iamericmorrison" - }, - { - "percent": "10000", - "reputation": 25932272878, - "rshares": 0, - "voter": "shiningstar" - }, - { - "percent": "1", - "reputation": 0, - "rshares": 0, - "voter": "steemprojects1" - }, - { - "percent": "5", - "reputation": 9266029, - "rshares": 0, - "voter": "steemprojects2" - }, - { - "percent": "10000", - "reputation": 65386511489, - "rshares": 0, - "voter": "stuvi" - }, - { - "percent": "10000", - "reputation": 11082705516, - "rshares": 0, - "voter": "zia161" - }, - { - "percent": "10000", - "reputation": 141349312842, - "rshares": 0, - "voter": "arslanq" - }, - { - "percent": "10000", - "reputation": 32936031613, - "rshares": 0, - "voter": "kevca16" - }, - { - "percent": "10000", - "reputation": 5869816453, - "rshares": 0, - "voter": "mcreg" - }, - { - "percent": "10000", - "reputation": 9096688109, - "rshares": 0, - "voter": "waheebisb" - }, - { - "percent": "10000", - "reputation": 171248220741, - "rshares": 0, - "voter": "rizaokur" - }, - { - "percent": "10000", - "reputation": 2133047242401, - "rshares": 0, - "voter": "seanstein" - }, - { - "percent": "10000", - "reputation": 434406231937, - "rshares": 0, - "voter": "piszozo" - }, - { - "percent": "10000", - "reputation": 986078901143, - "rshares": 0, - "voter": "vicmariki" - }, - { - "percent": "500", - "reputation": 827618875719, - "rshares": 0, - "voter": "contribution" - }, - { - "percent": "10000", - "reputation": 46928324502, - "rshares": 0, - "voter": "oep" - }, - { - "percent": "10000", - "reputation": 49540970, - "rshares": 0, - "voter": "akshitgrover" - }, - { - "percent": "10000", - "reputation": 409917207, - "rshares": 0, - "voter": "jackson12" - }, - { - "percent": "10000", - "reputation": 138377365150, - "rshares": 0, - "voter": "dtldesign" - }, - { - "percent": "10000", - "reputation": 3025934704561, - "rshares": 0, - "voter": "h4ck3rm1k3st33m" - }, - { - "percent": "10000", - "reputation": 514137420082, - "rshares": 0, - "voter": "smokeasare165" - }, - { - "percent": "10000", - "reputation": 1455841681924, - "rshares": 0, - "voter": "tinoschloegl" - }, - { - "percent": "10000", - "reputation": 1047625818, - "rshares": 0, - "voter": "liftu" - }, - { - "percent": "10000", - "reputation": 434522490493, - "rshares": 0, - "voter": "atul8888" - }, - { - "percent": "10000", - "reputation": 299446985155, - "rshares": 0, - "voter": "goroshkodo" - }, - { - "percent": "10000", - "reputation": 27330270603, - "rshares": 0, - "voter": "tarmizislow" - }, - { - "percent": "10000", - "reputation": 2026188411, - "rshares": 0, - "voter": "nurmasyithah" - }, - { - "percent": "10000", - "reputation": 18518523717, - "rshares": 0, - "voter": "ritikagupta" - }, - { - "percent": "10000", - "reputation": 22655162330, - "rshares": 0, - "voter": "anniemohler" - }, - { - "percent": "10000", - "reputation": 6151477307370, - "rshares": 0, - "voter": "olaivart" - }, - { - "percent": "10000", - "reputation": 226477842496, - "rshares": 0, - "voter": "thedrewshow" - }, - { - "percent": "-10000", - "reputation": 0, - "rshares": 0, - "voter": "alpha27" - }, - { - "percent": "10000", - "reputation": 4015949422, - "rshares": 0, - "voter": "adventuretours" - }, - { - "percent": "10000", - "reputation": 2380429434957, - "rshares": 0, - "voter": "advexon" - }, - { - "percent": "10000", - "reputation": 6428962492377, - "rshares": 0, - "voter": "cloudconnect" - }, - { - "percent": "10000", - "reputation": 1902528118381, - "rshares": 0, - "voter": "ontheverge" - }, - { - "percent": "10000", - "reputation": 4317696913, - "rshares": 0, - "voter": "celsomichida" - }, - { - "percent": "10000", - "reputation": 3759225279, - "rshares": 0, - "voter": "cannan" - }, - { - "percent": "10000", - "reputation": 1523709091079, - "rshares": 0, - "voter": "cloudbuster" - }, - { - "percent": "10000", - "reputation": 162480613274, - "rshares": 0, - "voter": "adrienoor" - }, - { - "percent": "10000", - "reputation": -563072732957, - "rshares": 0, - "voter": "hussnain" - }, - { - "percent": "10000", - "reputation": 1467929911, - "rshares": 0, - "voter": "shanloth" - }, - { - "percent": "10000", - "reputation": 9015395877, - "rshares": 0, - "voter": "samirnyaupane" - }, - { - "percent": "10000", - "reputation": 10694759666, - "rshares": 0, - "voter": "slackeramericana" - }, - { - "percent": "10000", - "reputation": 3120106738222, - "rshares": 0, - "voter": "thabiggdogg" - }, - { - "percent": "10000", - "reputation": 385781825940, - "rshares": 0, - "voter": "gilnambatac" - }, - { - "percent": "10000", - "reputation": 7962135395, - "rshares": 0, - "voter": "withgraham" - }, - { - "percent": "10000", - "reputation": 26222775057, - "rshares": 0, - "voter": "sephirot" - }, - { - "percent": "10000", - "reputation": 66854446738, - "rshares": 0, - "voter": "coffeeman" - }, - { - "percent": "10000", - "reputation": 41501057693, - "rshares": 0, - "voter": "stefunniy" - }, - { - "percent": "10000", - "reputation": 7789392208, - "rshares": 0, - "voter": "ghanexs" - }, - { - "percent": "10000", - "reputation": 1911567894442, - "rshares": 0, - "voter": "razipelangi" - }, - { - "percent": "10000", - "reputation": 565179863478, - "rshares": 0, - "voter": "dreamdiary" - }, - { - "percent": "10000", - "reputation": 3923276788526, - "rshares": 0, - "voter": "crypto4euro" - }, - { - "percent": "10000", - "reputation": 13170123102, - "rshares": 0, - "voter": "rtsampa" - }, - { - "percent": "10000", - "reputation": -753792225902, - "rshares": 0, - "voter": "mahi2raj" - }, - { - "percent": "10000", - "reputation": -635713021405, - "rshares": 0, - "voter": "danyflores" - }, - { - "percent": "10000", - "reputation": 177948427330, - "rshares": 0, - "voter": "oraclefrequency" - }, - { - "percent": "10000", - "reputation": 26337803094, - "rshares": 0, - "voter": "kartikohri1712" - }, - { - "percent": "10000", - "reputation": 275419252617, - "rshares": 0, - "voter": "cryptoaltcoin" - }, - { - "percent": "10000", - "reputation": 279405739464, - "rshares": 0, - "voter": "einarscorner" - }, - { - "percent": "10000", - "reputation": 718488845398, - "rshares": 0, - "voter": "dudithedoctor" - }, - { - "percent": "100", - "reputation": 343541613992585, - "rshares": 0, - "voter": "dirapa" - }, - { - "percent": "10000", - "reputation": 406981083, - "rshares": 0, - "voter": "arfouche" - }, - { - "percent": "10000", - "reputation": 425592459004, - "rshares": 0, - "voter": "kristenbruce" - }, - { - "percent": "10000", - "reputation": 3013400348, - "rshares": 0, - "voter": "okclear" - }, - { - "percent": "10000", - "reputation": 103041707769806, - "rshares": 0, - "voter": "mysearchisover" - }, - { - "percent": "10000", - "reputation": 30569702809, - "rshares": 0, - "voter": "dropd" - }, - { - "percent": "10000", - "reputation": 16046862173, - "rshares": 0, - "voter": "rulilesmana" - }, - { - "percent": "10000", - "reputation": 794249759, - "rshares": 0, - "voter": "cryptomaniac6" - }, - { - "percent": "10000", - "reputation": 119507852, - "rshares": 0, - "voter": "kim3ra" - }, - { - "percent": "10000", - "reputation": 26898775193091, - "rshares": 0, - "voter": "movement19" - }, - { - "percent": "10000", - "reputation": 367997243259, - "rshares": 0, - "voter": "aacr07" - }, - { - "percent": "10000", - "reputation": 347931912166, - "rshares": 0, - "voter": "layra" - }, - { - "percent": "10000", - "reputation": 7443820021, - "rshares": 0, - "voter": "vardhanbtc" - }, - { - "percent": "10000", - "reputation": 64251297055, - "rshares": 0, - "voter": "azeemprime" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "dbnx" - }, - { - "percent": "10000", - "reputation": 7922356058897, - "rshares": 0, - "voter": "shredz7" - }, - { - "percent": "10000", - "reputation": 94969491, - "rshares": 0, - "voter": "bitstreamgains" - }, - { - "percent": "10000", - "reputation": -19669107258, - "rshares": 0, - "voter": "hendrimaca" - }, - { - "percent": "10000", - "reputation": 1495750112002, - "rshares": 0, - "voter": "aan01" - }, - { - "percent": "10000", - "reputation": 250338481362, - "rshares": 0, - "voter": "successmindset" - }, - { - "percent": "10000", - "reputation": 583567123463302, - "rshares": 0, - "voter": "hatu" - }, - { - "percent": "10000", - "reputation": 6911078301, - "rshares": 0, - "voter": "khabirulhafiz" - }, - { - "percent": "10000", - "reputation": -726440779532, - "rshares": 0, - "voter": "yady" - }, - { - "percent": "10000", - "reputation": -1578132601, - "rshares": 0, - "voter": "samsonjura1" - }, - { - "percent": "10000", - "reputation": 71411614402, - "rshares": 0, - "voter": "ambitiouslife" - }, - { - "percent": "10000", - "reputation": 1392741879, - "rshares": 0, - "voter": "whyse" - }, - { - "percent": "10000", - "reputation": 3874452762, - "rshares": 0, - "voter": "skyflow" - }, - { - "percent": "10000", - "reputation": 739229350399, - "rshares": 0, - "voter": "kimtoma" - }, - { - "percent": "10000", - "reputation": 1748289895691, - "rshares": 0, - "voter": "asherunderwood" - }, - { - "percent": "10000", - "reputation": 38452448274, - "rshares": 0, - "voter": "lorden" - }, - { - "percent": "10000", - "reputation": 73325782068, - "rshares": 0, - "voter": "juicyvegandwarf" - }, - { - "percent": "10000", - "reputation": -149922805, - "rshares": 0, - "voter": "ushan007" - }, - { - "percent": "10000", - "reputation": 2662993712, - "rshares": 0, - "voter": "ihsan6837" - }, - { - "percent": "10000", - "reputation": 2316420258, - "rshares": 0, - "voter": "suryarose" - }, - { - "percent": "10000", - "reputation": 24621699124, - "rshares": 0, - "voter": "sol7142" - }, - { - "percent": "10000", - "reputation": 23722141431, - "rshares": 0, - "voter": "fikrialoy" - }, - { - "percent": "10000", - "reputation": -166535280, - "rshares": 0, - "voter": "mr-lahey" - }, - { - "percent": "10000", - "reputation": 11981081855, - "rshares": 0, - "voter": "mahsabmirza" - }, - { - "percent": "1000", - "reputation": 4557349567330, - "rshares": 0, - "voter": "pwangdu" - }, - { - "percent": "10000", - "reputation": 2002379332, - "rshares": 0, - "voter": "salda" - }, - { - "percent": "10000", - "reputation": 157642435260, - "rshares": 0, - "voter": "muzzlealem" - }, - { - "percent": "10000", - "reputation": 2218652752433, - "rshares": 0, - "voter": "pakjos" - }, - { - "percent": "10000", - "reputation": 28204184034, - "rshares": 0, - "voter": "mhdfadhal" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "dannykastner" - }, - { - "percent": "10000", - "reputation": 66912417582, - "rshares": 0, - "voter": "ibul11" - }, - { - "percent": "10000", - "reputation": 665118181066, - "rshares": 0, - "voter": "nigtroy" - }, - { - "percent": "10000", - "reputation": 11349258518, - "rshares": 0, - "voter": "uripsurya" - }, - { - "percent": "10000", - "reputation": 53385326, - "rshares": 0, - "voter": "jazman.zhens" - }, - { - "percent": "10000", - "reputation": 47266392264, - "rshares": 0, - "voter": "intervote" - }, - { - "percent": "10000", - "reputation": 1242404669, - "rshares": 0, - "voter": "mahend" - }, - { - "percent": "10000", - "reputation": 695858497, - "rshares": 0, - "voter": "anandasungkar" - }, - { - "percent": "10000", - "reputation": 1370538027, - "rshares": 0, - "voter": "homeless.global" - }, - { - "percent": "10000", - "reputation": 1731294599, - "rshares": 0, - "voter": "intansteemityes" - }, - { - "percent": "10000", - "reputation": 43971275379, - "rshares": 0, - "voter": "perminus-gaita" - }, - { - "percent": "10000", - "reputation": 14890860265, - "rshares": 0, - "voter": "phost" - }, - { - "percent": "10000", - "reputation": 2221096395269, - "rshares": 0, - "voter": "debart" - }, - { - "percent": "10000", - "reputation": 139124119367, - "rshares": 0, - "voter": "blurrydude" - }, - { - "percent": "10000", - "reputation": 27178121277927, - "rshares": 0, - "voter": "tsnaks" - }, - { - "percent": "10000", - "reputation": 11437207523627, - "rshares": 0, - "voter": "legendchew" - }, - { - "percent": "100", - "reputation": 180887712001, - "rshares": 0, - "voter": "cryptonegocios" - }, - { - "percent": "10000", - "reputation": 101139191212, - "rshares": 0, - "voter": "alexcozzy" - }, - { - "percent": "10000", - "reputation": 42167305, - "rshares": 0, - "voter": "joe.ster" - }, - { - "percent": "10000", - "reputation": 2959195565, - "rshares": 0, - "voter": "pandu13" - }, - { - "percent": "10000", - "reputation": 2413876943, - "rshares": 0, - "voter": "sasakhan" - }, - { - "percent": "10000", - "reputation": 270169809, - "rshares": 0, - "voter": "faisalyus" - }, - { - "percent": "10000", - "reputation": 20397543141918, - "rshares": 0, - "voter": "sawyn" - }, - { - "percent": "10000", - "reputation": 135832376, - "rshares": 0, - "voter": "diegocedenno" - }, - { - "percent": "10000", - "reputation": 3390855600076, - "rshares": 0, - "voter": "calebotamus" - }, - { - "percent": "10000", - "reputation": 16375701, - "rshares": 0, - "voter": "lsanneh78128" - }, - { - "percent": "10000", - "reputation": 99196394608, - "rshares": 0, - "voter": "nazaruddin885" - }, - { - "percent": "10000", - "reputation": 6004774856238, - "rshares": 0, - "voter": "chaseburnett" - }, - { - "percent": "10000", - "reputation": 1304920063150, - "rshares": 0, - "voter": "sisirhasan" - }, - { - "percent": "10000", - "reputation": 139033616025, - "rshares": 0, - "voter": "donyanyo" - }, - { - "percent": "10000", - "reputation": 675965273965, - "rshares": 0, - "voter": "oregontravel" - }, - { - "percent": "-10000", - "reputation": 27409393057658, - "rshares": 0, - "voter": "luegenbaron" - }, - { - "percent": "10000", - "reputation": 1242095462, - "rshares": 0, - "voter": "edkrassenstein" - }, - { - "percent": "10000", - "reputation": -8656090342, - "rshares": 0, - "voter": "munawirawin" - }, - { - "percent": "10000", - "reputation": 52015121371, - "rshares": 0, - "voter": "salgetra" - }, - { - "percent": "-10000", - "reputation": 35525970129140, - "rshares": 0, - "voter": "zaxan" - }, - { - "percent": "10000", - "reputation": 12448009637, - "rshares": 0, - "voter": "rollings" - }, - { - "percent": "10000", - "reputation": 56810129135, - "rshares": 0, - "voter": "kyle07" - }, - { - "percent": "10000", - "reputation": -56633199769, - "rshares": 0, - "voter": "pchanger" - }, - { - "percent": "10000", - "reputation": 2220689763292, - "rshares": 0, - "voter": "uncleboy" - }, - { - "percent": "10000", - "reputation": 5944210218, - "rshares": 0, - "voter": "alfhi" - }, - { - "percent": "0", - "reputation": 93270469, - "rshares": 0, - "voter": "htetmyathtut" - }, - { - "percent": "10000", - "reputation": 1535119282990, - "rshares": 0, - "voter": "trisolaran" - }, - { - "percent": "10000", - "reputation": 9054698465381, - "rshares": 0, - "voter": "arafs" - }, - { - "percent": "10000", - "reputation": 250332631092, - "rshares": 0, - "voter": "abysoyjoy" - }, - { - "percent": "10000", - "reputation": 2482350646, - "rshares": 0, - "voter": "stylo419" - }, - { - "percent": "10000", - "reputation": 25427769512752, - "rshares": 0, - "voter": "cookntell" - }, - { - "percent": "10000", - "reputation": 2640884245, - "rshares": 0, - "voter": "rakkarage" - }, - { - "percent": "10000", - "reputation": 319261957505, - "rshares": 0, - "voter": "grasozauru" - }, - { - "percent": "10000", - "reputation": 83917984354, - "rshares": 0, - "voter": "norabx" - }, - { - "percent": "10000", - "reputation": 10639158327880, - "rshares": 0, - "voter": "joyvancouver" - }, - { - "percent": "10000", - "reputation": 55079152062, - "rshares": 0, - "voter": "antchatz" - }, - { - "percent": "10000", - "reputation": 25167381987, - "rshares": 0, - "voter": "tutchpa" - }, - { - "percent": "-10000", - "reputation": 53337322068101, - "rshares": 0, - "voter": "cosmophobia" - }, - { - "percent": "6600", - "reputation": 38003370, - "rshares": 0, - "voter": "rajaji" - }, - { - "percent": "10000", - "reputation": 60057862, - "rshares": 0, - "voter": "liqquid" - }, - { - "percent": "10000", - "reputation": 11764005733, - "rshares": 0, - "voter": "aceh-post" - }, - { - "percent": "10000", - "reputation": 76704499, - "rshares": 0, - "voter": "dannanares" - }, - { - "percent": "10000", - "reputation": 338705120185, - "rshares": 0, - "voter": "husana" - }, - { - "percent": "10000", - "reputation": 145628145437, - "rshares": 0, - "voter": "davidmichael" - }, - { - "percent": "10000", - "reputation": 249434026, - "rshares": 0, - "voter": "wprpn" - }, - { - "percent": "10000", - "reputation": 488997421, - "rshares": 0, - "voter": "datuparulas17" - }, - { - "percent": "10000", - "reputation": 28432163, - "rshares": 0, - "voter": "trie" - }, - { - "percent": "-10000", - "reputation": -74205049136, - "rshares": 0, - "voter": "chirstonawba" - }, - { - "percent": "10000", - "reputation": 1427580552, - "rshares": 0, - "voter": "sulaiman86" - }, - { - "percent": "10000", - "reputation": 4717350663803, - "rshares": 0, - "voter": "electronicsworld" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "engrravijain" - }, - { - "percent": "10000", - "reputation": 32836973991, - "rshares": 0, - "voter": "alfredolopez1980" - }, - { - "percent": "10000", - "reputation": 842214722281, - "rshares": 0, - "voter": "gustavomonraz" - }, - { - "percent": "10000", - "reputation": 10653163880, - "rshares": 0, - "voter": "tahoorsaleem" - }, - { - "percent": "10000", - "reputation": 758939022885, - "rshares": 0, - "voter": "starfinger13" - }, - { - "percent": "10000", - "reputation": 397930184267, - "rshares": 0, - "voter": "conscalisthenics" - }, - { - "percent": "10000", - "reputation": 719725316, - "rshares": 0, - "voter": "syd44723" - }, - { - "percent": "10000", - "reputation": 4455302617675, - "rshares": 0, - "voter": "sutter" - }, - { - "percent": "10000", - "reputation": 4264322585, - "rshares": 0, - "voter": "samsonite18654" - }, - { - "percent": "10000", - "reputation": 3068512069, - "rshares": 0, - "voter": "iqra.naz" - }, - { - "percent": "10000", - "reputation": 4540320429, - "rshares": 0, - "voter": "drezz" - }, - { - "percent": "10000", - "reputation": -15883346236, - "rshares": 0, - "voter": "cutelace" - }, - { - "percent": "10000", - "reputation": -30938089449, - "rshares": 0, - "voter": "chirstonawba5" - }, - { - "percent": "8500", - "reputation": 281899083684, - "rshares": 0, - "voter": "devkapoor423" - }, - { - "percent": "10000", - "reputation": 30199308052561, - "rshares": 0, - "voter": "blanchy" - }, - { - "percent": "10000", - "reputation": 15551598, - "rshares": 0, - "voter": "rakeshban357" - }, - { - "percent": "10000", - "reputation": 187417680256, - "rshares": 0, - "voter": "douglasjames" - }, - { - "percent": "10000", - "reputation": 152403944888, - "rshares": 0, - "voter": "michaelabbas" - }, - { - "percent": "10000", - "reputation": 718164361629, - "rshares": 0, - "voter": "gonewithwind" - }, - { - "percent": "10000", - "reputation": 4968746857731, - "rshares": 0, - "voter": "snow.owl" - }, - { - "percent": "10000", - "reputation": 71132023, - "rshares": 0, - "voter": "tonthatthienvu" - }, - { - "percent": "10000", - "reputation": 510851312, - "rshares": 0, - "voter": "strangeworldnews" - }, - { - "percent": "10000", - "reputation": 4525252411, - "rshares": 0, - "voter": "dogra" - }, - { - "percent": "10000", - "reputation": 20782653433, - "rshares": 0, - "voter": "umairx97" - }, - { - "percent": "10000", - "reputation": -22287440682, - "rshares": 0, - "voter": "coolpeopleifb" - }, - { - "percent": "10000", - "reputation": 292028647126, - "rshares": 0, - "voter": "gabu01" - }, - { - "percent": "10000", - "reputation": 1135378766699, - "rshares": 0, - "voter": "magicalbot" - }, - { - "percent": "10000", - "reputation": 790439385274, - "rshares": 0, - "voter": "f21steem" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "hoobit" - }, - { - "percent": "10000", - "reputation": 2699259380, - "rshares": 0, - "voter": "ivancraigcaine" - }, - { - "percent": "10000", - "reputation": 5570673069, - "rshares": 0, - "voter": "kranko" - }, - { - "percent": "10000", - "reputation": 2037695796, - "rshares": 0, - "voter": "successinwork" - }, - { - "percent": "0", - "reputation": 4245375856, - "rshares": 0, - "voter": "abfelix96" - }, - { - "percent": "0", - "reputation": 7200884, - "rshares": 0, - "voter": "maldonadog" - }, - { - "percent": "-10000", - "reputation": 152903685005, - "rshares": 0, - "voter": "drakeler" - }, - { - "percent": "10000", - "reputation": 1069695515, - "rshares": 0, - "voter": "cyrex88" - }, - { - "percent": "10000", - "reputation": 218299278813, - "rshares": 0, - "voter": "rikyu" - }, - { - "percent": "10000", - "reputation": 8359148, - "rshares": 0, - "voter": "krimimimi" - }, - { - "percent": "10000", - "reputation": 208431577, - "rshares": 0, - "voter": "johannav" - } - ], - "author": "steemit", - "author_reputation": 12944616889, - "beneficiaries": [], - "body": "Steemit is a social media platform where anyone can earn STEEM points by posting. The more people who like a post, the more STEEM the poster earns. Anyone can sell their STEEM for cash or vest it to boost their voting power.", - "body_length": 224, - "cashout_time": "1969-12-31T23:59:59", - "category": "meta", - "children": 430, - "created": "2016-03-30T18:30:18", - "curator_payout_value": "0.756 HBD", - "depth": 0, - "json_metadata": "", - "last_payout": "2016-08-24T19:59:42", - "last_update": "2016-03-30T18:30:18", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 830053779138, - "parent_author": "", - "parent_permlink": "meta", - "pending_payout_value": "0.000 HBD", - "percent_hbd": 10000, - "permlink": "firstpost", - "post_id": 1, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Welcome to Steem!", - "title": "Welcome to Steem!", - "total_payout_value": "0.942 HBD", - "url": "/meta/@steemit/firstpost" - }, - "entry_id": 0, - "reblogged_on": "1970-01-01T00:00:00" - } -] diff --git a/hived/tavern/condenser_api_patterns/get_blog/steemit.tavern.yaml b/hived/tavern/condenser_api_patterns/get_blog/steemit.tavern.yaml deleted file mode 100644 index 17121033f38621596573296ff87d5ae6641f7a0f..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_blog/steemit.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_blog - - marks: - - patterntest - - xfail #follow_api_plugin not enabled. - - includes: - - !include ../../common.yaml - - stages: - - name: get_blog - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_blog" - params: ["steemit", 0, 1] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "steemit" - directory: "condenser_api_patterns/get_blog" diff --git a/hived/tavern/condenser_api_patterns/get_blog_authors/gtg.tavern.yaml b/hived/tavern/condenser_api_patterns/get_blog_authors/gtg.tavern.yaml deleted file mode 100644 index 7ed6e2ccb5ae62d547c6c5884ddb3eaa03cfb778..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_blog_authors/gtg.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_blog_authors - - marks: - - patterntest - - xfail # _follow_api: follow_api_plugin not enabled. - - includes: - - !include ../../common.yaml - - stages: - - name: get_blog_authors - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_blog_authors" - params: ["gtg"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "gtg" - directory: "condenser_api_patterns/get_blog_authors" diff --git a/hived/tavern/condenser_api_patterns/get_blog_authors/steemit.tavern.yaml b/hived/tavern/condenser_api_patterns/get_blog_authors/steemit.tavern.yaml deleted file mode 100644 index 1063acf3bf7b368419a85874f595946f7e7e02a2..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_blog_authors/steemit.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_blog_authors - - marks: - - patterntest - - xfail # _follow_api: follow_api_plugin not enabled. - - includes: - - !include ../../common.yaml - - stages: - - name: get_blog_authors - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_blog_authors" - params: ["steemit"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "steemit" - directory: "condenser_api_patterns/get_blog_authors" diff --git a/hived/tavern/condenser_api_patterns/get_blog_entries/gtg.pat.json b/hived/tavern/condenser_api_patterns/get_blog_entries/gtg.pat.json deleted file mode 100644 index ecfb50cbf08dc634663a5f9997a594051d885e4e..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_blog_entries/gtg.pat.json +++ /dev/null @@ -1,702 +0,0 @@ -[ - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5584, - "permlink": "qieua6", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5583, - "permlink": "qid9xy", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5582, - "permlink": "qia4s9", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5581, - "permlink": "qi6x5u", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5580, - "permlink": "qi6x48", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5579, - "permlink": "qhzwfw", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5578, - "permlink": "qhzw8c", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5577, - "permlink": "qhxms4", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5576, - "permlink": "qhs2sm", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5575, - "permlink": "qhqqhn", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5574, - "permlink": "qhqlzb", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5573, - "permlink": "qhq7wc", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5572, - "permlink": "qhp4at", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5571, - "permlink": "qhe3p8", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5570, - "permlink": "qhe0d3", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5569, - "permlink": "qhdzh0", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5568, - "permlink": "qhdk6s", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5567, - "permlink": "qhb9i0", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5566, - "permlink": "qhb8wo", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5565, - "permlink": "qhb8l2", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5564, - "permlink": "qhb8dv", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5563, - "permlink": "qhb87b", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5562, - "permlink": "qh7r1m", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5561, - "permlink": "qh7n7n", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5560, - "permlink": "qh66nn", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5559, - "permlink": "qh5e7p", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5558, - "permlink": "qh5dx8", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5557, - "permlink": "qh4v8b", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5556, - "permlink": "qh4s5o", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5555, - "permlink": "qh4rlv", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5554, - "permlink": "qgycts", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5553, - "permlink": "qgx95x", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5552, - "permlink": "qgwvyz", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5551, - "permlink": "qgsskb", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5550, - "permlink": "qgsofj", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5549, - "permlink": "qgotgk", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5548, - "permlink": "qgo7ut", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5547, - "permlink": "qgnv1v", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5546, - "permlink": "qgn1ed", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5545, - "permlink": "qgn15x", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5544, - "permlink": "qgmapk", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5543, - "permlink": "qgm763", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5542, - "permlink": "how-to-survive-eclipse-cheat-sheet", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5541, - "permlink": "qgikd9", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5540, - "permlink": "qghpwi", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5539, - "permlink": "qghojj", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5538, - "permlink": "qghmw1", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5537, - "permlink": "qghe2z", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5536, - "permlink": "qggrkw", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5535, - "permlink": "qgepw5", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5534, - "permlink": "qgekq5", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5533, - "permlink": "qgeh21", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5532, - "permlink": "qga3dk", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5531, - "permlink": "qg93jh", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5530, - "permlink": "qg92ge", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5529, - "permlink": "qg5kdx", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5528, - "permlink": "qg1lia", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5527, - "permlink": "qftncv", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5526, - "permlink": "qfpw7j", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5525, - "permlink": "qfo0nt", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5524, - "permlink": "qfnvrg", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5523, - "permlink": "qfnpv5", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5522, - "permlink": "qflv63", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5521, - "permlink": "qflumv", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5520, - "permlink": "qfk1sa", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5519, - "permlink": "qfji1u", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5518, - "permlink": "qfj6c0", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5517, - "permlink": "qfj5vw", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5516, - "permlink": "qfj3z9", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5515, - "permlink": "qfj3vn", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5514, - "permlink": "qfiuqx", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5513, - "permlink": "witness-update-release-candidate-for-eclipse-is-out", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5512, - "permlink": "qfiq6k", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5511, - "permlink": "qfglzu", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5510, - "permlink": "qfgesa", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5509, - "permlink": "qfgc9u", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5508, - "permlink": "qffpa7", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5507, - "permlink": "qff17c", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5506, - "permlink": "qfbg1m", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5505, - "permlink": "qf9556", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5504, - "permlink": "qf7mif", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5503, - "permlink": "qf3gxr", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5502, - "permlink": "qf2mas", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5501, - "permlink": "qf1yj1", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5500, - "permlink": "qesisk", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5499, - "permlink": "qepccm", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5498, - "permlink": "qep8xr", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5497, - "permlink": "qenkwy", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5496, - "permlink": "qejqiu", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5495, - "permlink": "qeiwmk", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5494, - "permlink": "qeiw01", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5493, - "permlink": "qeh4ox", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5492, - "permlink": "qeh3o6", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5491, - "permlink": "qefqia", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5490, - "permlink": "qefn67", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5489, - "permlink": "qecrgw", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5488, - "permlink": "qe97gy", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5487, - "permlink": "qe91gt", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5486, - "permlink": "qe6o6s", - "reblogged_on": "1970-01-01T00:00:00" - }, - { - "author": "gtg", - "blog": "gtg", - "entry_id": 5485, - "permlink": "qe60gw", - "reblogged_on": "1970-01-01T00:00:00" - } -] diff --git a/hived/tavern/condenser_api_patterns/get_blog_entries/gtg.tavern.yaml b/hived/tavern/condenser_api_patterns/get_blog_entries/gtg.tavern.yaml deleted file mode 100644 index 71d8a04b95ea67125535faded9f0c35b98a2c2ce..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_blog_entries/gtg.tavern.yaml +++ /dev/null @@ -1,30 +0,0 @@ ---- - test_name: Hived get_blog_entries - - marks: - - patterntest - - xfail # some pat parameters disappear - - includes: - - !include ../../common.yaml - - stages: - - name: get_blog_entries - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_blog_entries" - params: ["gtg",0,100] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "gtg" - directory: "condenser_api_patterns/get_blog_entries" - ignore_tags: ['entry_id','permlink','reblogged_on'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_blog_entries/steemit.pat.json b/hived/tavern/condenser_api_patterns/get_blog_entries/steemit.pat.json deleted file mode 100644 index f12769acbbeb89ee2e12869058dec6af5cc3b633..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_blog_entries/steemit.pat.json +++ /dev/null @@ -1,9 +0,0 @@ -[ - { - "author": "steemit", - "blog": "steemit", - "entry_id": 0, - "permlink": "firstpost", - "reblogged_on": "1970-01-01T00:00:00" - } -] diff --git a/hived/tavern/condenser_api_patterns/get_blog_entries/steemit.tavern.yaml b/hived/tavern/condenser_api_patterns/get_blog_entries/steemit.tavern.yaml deleted file mode 100644 index e3cf74326b65f50f674580f1262ba0fac932e378..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_blog_entries/steemit.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_blog_entries - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_blog_authors - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_blog_entries" - params: ["steemit",0,100] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "steemit" - directory: "condenser_api_patterns/get_blog_entries" diff --git a/hived/tavern/condenser_api_patterns/get_chain_properties/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_chain_properties/get.tavern.yaml deleted file mode 100644 index 5875160a192f153f169b58a8d2abd8839bac212b..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_chain_properties/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_chain_properties - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_chain_properties - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_chain_properties" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_chain_properties" diff --git a/hived/tavern/condenser_api_patterns/get_comment_discussions_by_payout/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_comment_discussions_by_payout/get.tavern.yaml deleted file mode 100644 index fe71edaf15b50134c1eddb34ac24f215ddcec1be..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_comment_discussions_by_payout/get.tavern.yaml +++ /dev/null @@ -1,33 +0,0 @@ ---- - test_name: Hived get_comment_discussions_by_payout - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_comment_discussions_by_payout - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_comment_discussions_by_payout" - params: [ - { - "tag": "hive", - "limit": 10, - } - ] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_comment_discussions_by_payout" diff --git a/hived/tavern/condenser_api_patterns/get_config/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_config/get.tavern.yaml deleted file mode 100644 index 9fb802fce6f273ef80da53481b5618ab31014b6e..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_config/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_config - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_config - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_config" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_config" diff --git a/hived/tavern/condenser_api_patterns/get_conversion_requests/get.pat.json b/hived/tavern/condenser_api_patterns/get_conversion_requests/get.pat.json deleted file mode 100644 index fe51488c7066f6687ef680d6bfaa4f7768ef205c..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_conversion_requests/get.pat.json +++ /dev/null @@ -1 +0,0 @@ -[] diff --git a/hived/tavern/condenser_api_patterns/get_conversion_requests/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_conversion_requests/get.tavern.yaml deleted file mode 100644 index 3876cc47ef28efc5bf1b72f5a5a19a8a329c6388..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_conversion_requests/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_conversion_requests - - marks: - - patterntest # as conversions requests change over time, it tests only if API is called - - includes: - - !include ../../common.yaml - - stages: - - name: get_conversion_requests - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_conversion_requests" - params: [144423] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_conversion_requests" diff --git a/hived/tavern/condenser_api_patterns/get_current_median_history_price/get.pat.json b/hived/tavern/condenser_api_patterns/get_current_median_history_price/get.pat.json deleted file mode 100644 index a14ae4ceb5f440ff9dcfc7cea583864fbbf83d6e..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_current_median_history_price/get.pat.json +++ /dev/null @@ -1,4 +0,0 @@ -{ - "base": "0.137 HBD", - "quote": "1.000 HIVE" -} diff --git a/hived/tavern/condenser_api_patterns/get_current_median_history_price/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_current_median_history_price/get.tavern.yaml deleted file mode 100644 index 99e94040a347a9f489115964d963fd230bc0485e..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_current_median_history_price/get.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_current_median_history_price - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_current_median_history_price - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_current_median_history_price" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_current_median_history_price" - ignore_tags: ["base","quote"] \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_discussions_by_active/steem.tavern.yaml b/hived/tavern/condenser_api_patterns/get_discussions_by_active/steem.tavern.yaml deleted file mode 100644 index 9c7e4679be02314b6207ffbadec22d283345cbf2..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_discussions_by_active/steem.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_discussions_by_active - - marks: - - patterntest - - xfail # _tags_api: tags_api_plugin not enabled. - - includes: - - !include ../../common.yaml - - stages: - - name: get_discussions_by_active - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_discussions_by_active" - params: ["steem"] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "steem" - directory: "condenser_api_patterns/get_discussions_by_active" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_discussions_by_cashout/steem.tavern.yaml b/hived/tavern/condenser_api_patterns/get_discussions_by_cashout/steem.tavern.yaml deleted file mode 100644 index 8c909fbc81203c8a0be7f56939d5bf732d8bfb4f..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_discussions_by_cashout/steem.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_discussions_by_cashout - - marks: - - patterntest - - xfail # _tags_api: tags_api_plugin not enabled. - - includes: - - !include ../../common.yaml - - stages: - - name: get_discussions_by_cashout - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_discussions_by_cashout" - params: ["steem"] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "steem" - directory: "condenser_api_patterns/get_discussions_by_cashout" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_discussions_by_children/steem.tavern.yaml b/hived/tavern/condenser_api_patterns/get_discussions_by_children/steem.tavern.yaml deleted file mode 100644 index 17e70e3e1e48cc3241a4a540be19438312303c95..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_discussions_by_children/steem.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_discussions_by_children - - marks: - - patterntest - - xfail # _tags_api: tags_api_plugin not enabled. - - includes: - - !include ../../common.yaml - - stages: - - name: get_discussions_by_children - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_discussions_by_children" - params: ["steem"] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "steem" - directory: "condenser_api_patterns/get_discussions_by_children" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_discussions_by_votes/steem.tavern.yaml b/hived/tavern/condenser_api_patterns/get_discussions_by_votes/steem.tavern.yaml deleted file mode 100644 index c979896a0c08ecd1fd9d065f55184095ac169360..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_discussions_by_votes/steem.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_discussions_by_votes - - marks: - - patterntest - - xfail # _tags_api: tags_api_plugin not enabled. - - includes: - - !include ../../common.yaml - - stages: - - name: get_discussions_by_votes - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_discussions_by_votes" - params: ["steem"] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "steem" - directory: "condenser_api_patterns/get_discussions_by_votes" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_dynamic_global_properties/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_dynamic_global_properties/get.tavern.yaml deleted file mode 100644 index e2de453e570157784e19be6d9f6638fdc01be758..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_dynamic_global_properties/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_dynamic_global_properties - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_dynamic_global_properties - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_dynamic_global_properties" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_dynamic_global_properties" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_escrow/addicttolife.pat.json b/hived/tavern/condenser_api_patterns/get_escrow/addicttolife.pat.json deleted file mode 100644 index 0d2c291cba51a91ffa7ea39380eda3ba3514fae7..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_escrow/addicttolife.pat.json +++ /dev/null @@ -1,15 +0,0 @@ -{ - "agent": "ongame", - "agent_approved": true, - "disputed": false, - "escrow_expiration": "2018-11-24T17:31:26", - "escrow_id": 1, - "from": "addicttolife", - "hbd_balance": "0.000 HBD", - "hive_balance": "4.832 HIVE", - "id": 158, - "pending_fee": "0.000 HIVE", - "ratification_deadline": "2018-11-23T17:31:26", - "to": "fundition.help", - "to_approved": true -} diff --git a/hived/tavern/condenser_api_patterns/get_escrow/addicttolife.tavern.yaml b/hived/tavern/condenser_api_patterns/get_escrow/addicttolife.tavern.yaml deleted file mode 100644 index eb3c89b8ac7480574e5e9e72fe4724807e5267bb..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_escrow/addicttolife.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_escrow addicttolife - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_escrow - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_escrow" - params: ["addicttolife", 1] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "addicttolife" - directory: "condenser_api_patterns/get_escrow" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_escrow/blervin.pat.json b/hived/tavern/condenser_api_patterns/get_escrow/blervin.pat.json deleted file mode 100644 index 501a572f7968bbc2f3804d7b6d2dddba9c9c8237..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_escrow/blervin.pat.json +++ /dev/null @@ -1,15 +0,0 @@ -{ - "agent": "monstersescrow", - "agent_approved": true, - "disputed": false, - "escrow_expiration": "2018-06-21T04:58:32", - "escrow_id": 4, - "from": "blervin", - "hbd_balance": "0.001 HBD", - "hive_balance": "0.000 HIVE", - "id": 89, - "pending_fee": "0.000 HBD", - "ratification_deadline": "2018-06-21T03:58:32", - "to": "duplibot", - "to_approved": true -} diff --git a/hived/tavern/condenser_api_patterns/get_escrow/blervin.tavern.yaml b/hived/tavern/condenser_api_patterns/get_escrow/blervin.tavern.yaml deleted file mode 100644 index 270cf48fa36942f9462c1323f1ec7d72e01976ba..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_escrow/blervin.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_escrow blervin - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_escrow - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_escrow" - params: ["blervin", 4] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "blervin" - directory: "condenser_api_patterns/get_escrow" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_escrow/duplibot.pat.json b/hived/tavern/condenser_api_patterns/get_escrow/duplibot.pat.json deleted file mode 100644 index 3f096a6b16b760b3de6bbe59e59c37a300b302a7..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_escrow/duplibot.pat.json +++ /dev/null @@ -1,15 +0,0 @@ -{ - "agent": "monstersescrow", - "agent_approved": true, - "disputed": false, - "escrow_expiration": "2018-06-26T20:25:45", - "escrow_id": 13, - "from": "duplibot", - "hbd_balance": "0.010 HBD", - "hive_balance": "0.000 HIVE", - "id": 104, - "pending_fee": "0.000 HBD", - "ratification_deadline": "2018-06-26T19:25:45", - "to": "blervin", - "to_approved": true -} diff --git a/hived/tavern/condenser_api_patterns/get_escrow/duplibot.tavern.yaml b/hived/tavern/condenser_api_patterns/get_escrow/duplibot.tavern.yaml deleted file mode 100644 index ea93c693d031648572bf9e4b8ba89f20679e6cb4..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_escrow/duplibot.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_escrow duplibot - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_escrow - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_escrow" - params: ["duplibot", 13] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "duplibot" - directory: "condenser_api_patterns/get_escrow" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_expiring_vesting_delegations/duplibot.tavern.yaml b/hived/tavern/condenser_api_patterns/get_expiring_vesting_delegations/duplibot.tavern.yaml deleted file mode 100644 index 48fc98572b5ecb5b1bae653ba915a6c002beac7d..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_expiring_vesting_delegations/duplibot.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_expiring_vesting_delegations duplibot - - marks: - - patterntest # test only if there is result - hard to test real data as expiring delegations change over time - - includes: - - !include ../../common.yaml - - stages: - - name: get_expiring_vesting_delegations - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_expiring_vesting_delegations" - params: ["duplibot", "1970-01-01T00:00:00"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "duplibot" - directory: "condenser_api_patterns/get_expiring_vesting_delegations" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_feed/duplibot.tavern.yaml b/hived/tavern/condenser_api_patterns/get_feed/duplibot.tavern.yaml deleted file mode 100644 index 3fa742fbf8bb30357dcdffa6e1fc7d56da625028..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_feed/duplibot.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_feed duplibot - - marks: - - patterntest - - xfail # follow_api_plugin not enabled. - - includes: - - !include ../../common.yaml - - stages: - - name: get_feed - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_feed" - params: ["duplibot", 13] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "duplibot" - directory: "condenser_api_patterns/get_feed" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_feed_entries/duplibot.tavern.yaml b/hived/tavern/condenser_api_patterns/get_feed_entries/duplibot.tavern.yaml deleted file mode 100644 index a89147cec1995773e671be03474485bea8422763..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_feed_entries/duplibot.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_feed_entries duplibot - - marks: - - patterntest - - xfail # follow_api_plugin not enabled. - includes: - - !include ../../common.yaml - - stages: - - name: get_feed_entries - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_feed_entries" - params: ["duplibot", 13] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "duplibot" - directory: "condenser_api_patterns/get_feed_entries" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_feed_history/get.pat.json b/hived/tavern/condenser_api_patterns/get_feed_history/get.pat.json deleted file mode 100644 index 7562d14d41995112d719bbd72ddced073d478dfb..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_feed_history/get.pat.json +++ /dev/null @@ -1,345 +0,0 @@ -{ - "current_median_history": { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - "id": 0, - "price_history": [ - { - "base": "0.142 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.142 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.142 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.142 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.142 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.140 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.140 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.142 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.141 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.142 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.141 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.141 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.141 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.138 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.137 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.136 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.137 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.137 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.137 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.137 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.137 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.137 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.137 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.137 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.137 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.136 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.135 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.135 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.135 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.135 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.135 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.136 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.135 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.135 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.136 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.135 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.134 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.131 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.131 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.130 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.130 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.129 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.128 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.127 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.126 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.126 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.126 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.126 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.125 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.125 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.124 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.124 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.124 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.124 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.124 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.122 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.122 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.123 HBD", - "quote": "1.000 HIVE" - }, - { - "base": "0.122 HBD", - "quote": "1.000 HIVE" - } - ] -} diff --git a/hived/tavern/condenser_api_patterns/get_feed_history/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_feed_history/get.tavern.yaml deleted file mode 100644 index 5faccc5f9b3e177eb357f716ea39ab007836835b..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_feed_history/get.tavern.yaml +++ /dev/null @@ -1,29 +0,0 @@ ---- - test_name: Hived get_feed_history duplibot - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_feed_history - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_feed_history" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_feed_history" - ignore_tags: ['base','quote'] \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_hardfork_version/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_hardfork_version/get.tavern.yaml deleted file mode 100644 index 5770f40048db171fa250b605e273ea9f459fd121..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_hardfork_version/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_hardfork_version - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_hardfork_version - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_hardfork_version" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_hardfork_version" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_key_references/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_key_references/get.tavern.yaml deleted file mode 100644 index 94fb5bc1d08b215baca5100ae5ea16017184cb1c..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_key_references/get.tavern.yaml +++ /dev/null @@ -1,33 +0,0 @@ ---- - test_name: Hived get_key_references - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_key_references - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_key_references" - params: [[ - "STM5tp5hWbGLL1R3tMVsgYdYxLPyAQFdKoYFbT2hcWUmrU42p1MQC", - "STM7sw22HqsXbz7D2CmJfmMwt9rimtk518dRzsR1f8Cgw52dQR1pR", - "STM5ya7Y8XKBRuWL2uL8NsSnB691cCzca87Ho9MXaPZ1ZYdGaJBBc", - "STM8epVc3pAcL44kvfvntsQGWVAyeQmFKC9nFdbPFYW1fCEg4sM14" - ]] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_key_references" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_market_history/15.pat.json b/hived/tavern/condenser_api_patterns/get_market_history/15.pat.json deleted file mode 100644 index 923952aad87c886447f2b05173439fe0530e2e89..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history/15.pat.json +++ /dev/null @@ -1,6481 +0,0 @@ -[ - { - "hive": { - "close": 8589, - "high": 8589, - "low": 8589, - "open": 8589, - "volume": 8589 - }, - "id": 2760978, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-11-05T11:11:30", - "seconds": 15 - }, - { - "hive": { - "close": 4957, - "high": 1229, - "low": 4957, - "open": 1229, - "volume": 12312 - }, - "id": 2760981, - "non_hive": { - "close": 567, - "high": 142, - "low": 567, - "open": 142, - "volume": 1413 - }, - "open": "2020-11-05T11:12:45", - "seconds": 15 - }, - { - "hive": { - "close": 17168, - "high": 17168, - "low": 17168, - "open": 17168, - "volume": 17168 - }, - "id": 2760983, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T11:13:15", - "seconds": 15 - }, - { - "hive": { - "close": 17, - "high": 17, - "low": 17, - "open": 17, - "volume": 17 - }, - "id": 2760985, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-11-05T11:14:45", - "seconds": 15 - }, - { - "hive": { - "close": 17339, - "high": 17339, - "low": 17339, - "open": 17339, - "volume": 17339 - }, - "id": 2760987, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T11:30:00", - "seconds": 15 - }, - { - "hive": { - "close": 60, - "high": 60, - "low": 60, - "open": 60, - "volume": 60 - }, - "id": 2760990, - "non_hive": { - "close": 7, - "high": 7, - "low": 7, - "open": 7, - "volume": 7 - }, - "open": "2020-11-05T12:03:15", - "seconds": 15 - }, - { - "hive": { - "close": 23625, - "high": 23625, - "low": 23625, - "open": 23625, - "volume": 23625 - }, - "id": 2760994, - "non_hive": { - "close": 2725, - "high": 2725, - "low": 2725, - "open": 2725, - "volume": 2725 - }, - "open": "2020-11-05T12:07:00", - "seconds": 15 - }, - { - "hive": { - "close": 667, - "high": 667, - "low": 667, - "open": 667, - "volume": 667 - }, - "id": 2760997, - "non_hive": { - "close": 77, - "high": 77, - "low": 77, - "open": 77, - "volume": 77 - }, - "open": "2020-11-05T12:09:45", - "seconds": 15 - }, - { - "hive": { - "close": 69, - "high": 69, - "low": 69, - "open": 69, - "volume": 69 - }, - "id": 2760999, - "non_hive": { - "close": 8, - "high": 8, - "low": 8, - "open": 8, - "volume": 8 - }, - "open": "2020-11-05T12:15:45", - "seconds": 15 - }, - { - "hive": { - "close": 52, - "high": 52, - "low": 52, - "open": 52, - "volume": 52 - }, - "id": 2761002, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-05T12:17:30", - "seconds": 15 - }, - { - "hive": { - "close": 9087, - "high": 3286, - "low": 9087, - "open": 3286, - "volume": 12373 - }, - "id": 2761004, - "non_hive": { - "close": 1048, - "high": 379, - "low": 1048, - "open": 379, - "volume": 1427 - }, - "open": "2020-11-05T12:22:30", - "seconds": 15 - }, - { - "hive": { - "close": 4804, - "high": 4804, - "low": 4804, - "open": 4804, - "volume": 4804 - }, - "id": 2761007, - "non_hive": { - "close": 554, - "high": 554, - "low": 554, - "open": 554, - "volume": 554 - }, - "open": "2020-11-05T12:38:15", - "seconds": 15 - }, - { - "hive": { - "close": 312, - "high": 312, - "low": 312, - "open": 312, - "volume": 312 - }, - "id": 2761010, - "non_hive": { - "close": 36, - "high": 36, - "low": 36, - "open": 36, - "volume": 36 - }, - "open": "2020-11-05T12:58:15", - "seconds": 15 - }, - { - "hive": { - "close": 75290, - "high": 75290, - "low": 6023, - "open": 17798, - "volume": 99111 - }, - "id": 2761013, - "non_hive": { - "close": 8684, - "high": 8684, - "low": 694, - "open": 2052, - "volume": 11430 - }, - "open": "2020-11-05T13:33:00", - "seconds": 15 - }, - { - "hive": { - "close": 6085, - "high": 6085, - "low": 297, - "open": 297, - "volume": 12460 - }, - "id": 2761017, - "non_hive": { - "close": 705, - "high": 705, - "low": 34, - "open": 34, - "volume": 1440 - }, - "open": "2020-11-05T13:35:30", - "seconds": 15 - }, - { - "hive": { - "close": 16801, - "high": 16801, - "low": 16801, - "open": 16801, - "volume": 16801 - }, - "id": 2761020, - "non_hive": { - "close": 1956, - "high": 1956, - "low": 1956, - "open": 1956, - "volume": 1956 - }, - "open": "2020-11-05T13:43:15", - "seconds": 15 - }, - { - "hive": { - "close": 240, - "high": 240, - "low": 370, - "open": 370, - "volume": 610 - }, - "id": 2761023, - "non_hive": { - "close": 28, - "high": 28, - "low": 43, - "open": 43, - "volume": 71 - }, - "open": "2020-11-05T13:47:45", - "seconds": 15 - }, - { - "hive": { - "close": 50671, - "high": 50671, - "low": 50671, - "open": 50671, - "volume": 50671 - }, - "id": 2761026, - "non_hive": { - "close": 5902, - "high": 5902, - "low": 5902, - "open": 5902, - "volume": 5902 - }, - "open": "2020-11-05T13:48:30", - "seconds": 15 - }, - { - "hive": { - "close": 487948, - "high": 13263, - "low": 487948, - "open": 13263, - "volume": 501211 - }, - "id": 2761028, - "non_hive": { - "close": 56835, - "high": 1545, - "low": 56835, - "open": 1545, - "volume": 58380 - }, - "open": "2020-11-05T13:53:15", - "seconds": 15 - }, - { - "hive": { - "close": 404960, - "high": 50989, - "low": 404960, - "open": 50989, - "volume": 455949 - }, - "id": 2761031, - "non_hive": { - "close": 47169, - "high": 5940, - "low": 47169, - "open": 5940, - "volume": 53109 - }, - "open": "2020-11-05T13:56:00", - "seconds": 15 - }, - { - "hive": { - "close": 11000, - "high": 11000, - "low": 11000, - "open": 11000, - "volume": 11000 - }, - "id": 2761034, - "non_hive": { - "close": 1280, - "high": 1280, - "low": 1280, - "open": 1280, - "volume": 1280 - }, - "open": "2020-11-05T13:56:15", - "seconds": 15 - }, - { - "hive": { - "close": 738, - "high": 738, - "low": 738, - "open": 738, - "volume": 738 - }, - "id": 2761035, - "non_hive": { - "close": 86, - "high": 86, - "low": 86, - "open": 86, - "volume": 86 - }, - "open": "2020-11-05T13:58:45", - "seconds": 15 - }, - { - "hive": { - "close": 8151, - "high": 8151, - "low": 8151, - "open": 8151, - "volume": 8151 - }, - "id": 2761037, - "non_hive": { - "close": 949, - "high": 949, - "low": 949, - "open": 949, - "volume": 949 - }, - "open": "2020-11-05T13:59:45", - "seconds": 15 - }, - { - "hive": { - "close": 8282, - "high": 8282, - "low": 8282, - "open": 8282, - "volume": 8282 - }, - "id": 2761039, - "non_hive": { - "close": 964, - "high": 964, - "low": 964, - "open": 964, - "volume": 964 - }, - "open": "2020-11-05T14:00:15", - "seconds": 15 - }, - { - "hive": { - "close": 231, - "high": 231, - "low": 231, - "open": 231, - "volume": 231 - }, - "id": 2761043, - "non_hive": { - "close": 27, - "high": 27, - "low": 27, - "open": 27, - "volume": 27 - }, - "open": "2020-11-05T14:00:45", - "seconds": 15 - }, - { - "hive": { - "close": 17191, - "high": 17191, - "low": 17191, - "open": 17191, - "volume": 17191 - }, - "id": 2761044, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T14:01:15", - "seconds": 15 - }, - { - "hive": { - "close": 17191, - "high": 17191, - "low": 17191, - "open": 17191, - "volume": 17191 - }, - "id": 2761046, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T14:02:00", - "seconds": 15 - }, - { - "hive": { - "close": 17191, - "high": 17191, - "low": 17191, - "open": 17191, - "volume": 17191 - }, - "id": 2761048, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T14:02:15", - "seconds": 15 - }, - { - "hive": { - "close": 86, - "high": 1855, - "low": 86, - "open": 1855, - "volume": 1941 - }, - "id": 2761049, - "non_hive": { - "close": 10, - "high": 216, - "low": 10, - "open": 216, - "volume": 226 - }, - "open": "2020-11-05T14:04:00", - "seconds": 15 - }, - { - "hive": { - "close": 249, - "high": 249, - "low": 249, - "open": 249, - "volume": 249 - }, - "id": 2761051, - "non_hive": { - "close": 29, - "high": 29, - "low": 29, - "open": 29, - "volume": 29 - }, - "open": "2020-11-05T14:06:00", - "seconds": 15 - }, - { - "hive": { - "close": 15120, - "high": 15120, - "low": 15120, - "open": 15120, - "volume": 15120 - }, - "id": 2761054, - "non_hive": { - "close": 1760, - "high": 1760, - "low": 1760, - "open": 1760, - "volume": 1760 - }, - "open": "2020-11-05T14:07:00", - "seconds": 15 - }, - { - "hive": { - "close": 6010, - "high": 6010, - "low": 6010, - "open": 6010, - "volume": 6010 - }, - "id": 2761056, - "non_hive": { - "close": 700, - "high": 700, - "low": 700, - "open": 700, - "volume": 700 - }, - "open": "2020-11-05T14:18:30", - "seconds": 15 - }, - { - "hive": { - "close": 309759, - "high": 17241, - "low": 309759, - "open": 17241, - "volume": 327000 - }, - "id": 2761059, - "non_hive": { - "close": 35932, - "high": 2000, - "low": 35932, - "open": 2000, - "volume": 37932 - }, - "open": "2020-11-05T14:19:45", - "seconds": 15 - }, - { - "hive": { - "close": 4295, - "high": 4295, - "low": 4295, - "open": 4295, - "volume": 4295 - }, - "id": 2761061, - "non_hive": { - "close": 500, - "high": 500, - "low": 500, - "open": 500, - "volume": 500 - }, - "open": "2020-11-05T14:21:45", - "seconds": 15 - }, - { - "hive": { - "close": 17239, - "high": 17239, - "low": 17239, - "open": 17239, - "volume": 17239 - }, - "id": 2761064, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:24:45", - "seconds": 15 - }, - { - "hive": { - "close": 11828, - "high": 11828, - "low": 11828, - "open": 11828, - "volume": 11828 - }, - "id": 2761066, - "non_hive": { - "close": 1377, - "high": 1377, - "low": 1377, - "open": 1377, - "volume": 1377 - }, - "open": "2020-11-05T14:25:00", - "seconds": 15 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2761069, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:25:30", - "seconds": 15 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2761070, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:26:15", - "seconds": 15 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2761072, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:26:45", - "seconds": 15 - }, - { - "hive": { - "close": 17242, - "high": 17242, - "low": 17242, - "open": 17242, - "volume": 17242 - }, - "id": 2761073, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:27:15", - "seconds": 15 - }, - { - "hive": { - "close": 112070, - "high": 17239, - "low": 112070, - "open": 17239, - "volume": 129309 - }, - "id": 2761075, - "non_hive": { - "close": 13000, - "high": 2000, - "low": 13000, - "open": 2000, - "volume": 15000 - }, - "open": "2020-11-05T14:27:45", - "seconds": 15 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2761076, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:28:15", - "seconds": 15 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2761078, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:28:45", - "seconds": 15 - }, - { - "hive": { - "close": 9741, - "high": 9741, - "low": 9741, - "open": 9741, - "volume": 9741 - }, - "id": 2761079, - "non_hive": { - "close": 1130, - "high": 1130, - "low": 1130, - "open": 1130, - "volume": 1130 - }, - "open": "2020-11-05T14:29:15", - "seconds": 15 - }, - { - "hive": { - "close": 17180, - "high": 17180, - "low": 17180, - "open": 17180, - "volume": 34360 - }, - "id": 2761081, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-05T14:30:30", - "seconds": 15 - }, - { - "hive": { - "close": 196815, - "high": 196815, - "low": 17181, - "open": 17181, - "volume": 213996 - }, - "id": 2761084, - "non_hive": { - "close": 22912, - "high": 22912, - "low": 2000, - "open": 2000, - "volume": 24912 - }, - "open": "2020-11-05T14:30:45", - "seconds": 15 - }, - { - "hive": { - "close": 10012, - "high": 10012, - "low": 10012, - "open": 10012, - "volume": 10012 - }, - "id": 2761085, - "non_hive": { - "close": 1165, - "high": 1165, - "low": 1165, - "open": 1165, - "volume": 1165 - }, - "open": "2020-11-05T14:31:30", - "seconds": 15 - }, - { - "hive": { - "close": 233801, - "high": 10042, - "low": 233801, - "open": 10042, - "volume": 243843 - }, - "id": 2761087, - "non_hive": { - "close": 27121, - "high": 1165, - "low": 27121, - "open": 1165, - "volume": 28286 - }, - "open": "2020-11-05T14:31:45", - "seconds": 15 - }, - { - "hive": { - "close": 85811, - "high": 85811, - "low": 17181, - "open": 17181, - "volume": 102992 - }, - "id": 2761088, - "non_hive": { - "close": 9989, - "high": 9989, - "low": 1999, - "open": 1999, - "volume": 11988 - }, - "open": "2020-11-05T14:33:15", - "seconds": 15 - }, - { - "hive": { - "close": 17232, - "high": 17232, - "low": 17232, - "open": 17232, - "volume": 17232 - }, - "id": 2761090, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T14:34:00", - "seconds": 15 - }, - { - "hive": { - "close": 3643, - "high": 3643, - "low": 17171, - "open": 17171, - "volume": 77015 - }, - "id": 2761092, - "non_hive": { - "close": 428, - "high": 428, - "low": 1999, - "open": 1999, - "volume": 8975 - }, - "open": "2020-11-05T14:36:00", - "seconds": 15 - }, - { - "hive": { - "close": 84994, - "high": 84994, - "low": 84994, - "open": 84994, - "volume": 84994 - }, - "id": 2761095, - "non_hive": { - "close": 9985, - "high": 9985, - "low": 9985, - "open": 9985, - "volume": 9985 - }, - "open": "2020-11-05T14:36:45", - "seconds": 15 - }, - { - "hive": { - "close": 1984, - "high": 1984, - "low": 1984, - "open": 1984, - "volume": 1984 - }, - "id": 2761096, - "non_hive": { - "close": 230, - "high": 230, - "low": 230, - "open": 230, - "volume": 230 - }, - "open": "2020-11-05T14:38:30", - "seconds": 15 - }, - { - "hive": { - "close": 7250, - "high": 7250, - "low": 2392, - "open": 2392, - "volume": 9642 - }, - "id": 2761098, - "non_hive": { - "close": 854, - "high": 854, - "low": 281, - "open": 281, - "volume": 1135 - }, - "open": "2020-11-05T14:39:00", - "seconds": 15 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2761100, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:40:00", - "seconds": 15 - }, - { - "hive": { - "close": 9722, - "high": 9722, - "low": 9722, - "open": 9722, - "volume": 9722 - }, - "id": 2761103, - "non_hive": { - "close": 1145, - "high": 1145, - "low": 1145, - "open": 1145, - "volume": 1145 - }, - "open": "2020-11-05T14:43:30", - "seconds": 15 - }, - { - "hive": { - "close": 44999, - "high": 8328, - "low": 44999, - "open": 8328, - "volume": 53327 - }, - "id": 2761105, - "non_hive": { - "close": 5300, - "high": 981, - "low": 5300, - "open": 981, - "volume": 6281 - }, - "open": "2020-11-05T14:43:45", - "seconds": 15 - }, - { - "hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 6000 - }, - "id": 2761106, - "non_hive": { - "close": 698, - "high": 698, - "low": 698, - "open": 698, - "volume": 698 - }, - "open": "2020-11-05T14:45:00", - "seconds": 15 - }, - { - "hive": { - "close": 16981, - "high": 16981, - "low": 14949, - "open": 51, - "volume": 31981 - }, - "id": 2761109, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1734, - "open": 6, - "volume": 3739 - }, - "open": "2020-11-05T14:45:30", - "seconds": 15 - }, - { - "hive": { - "close": 229759, - "high": 229759, - "low": 229759, - "open": 229759, - "volume": 229759 - }, - "id": 2761110, - "non_hive": { - "close": 27061, - "high": 27061, - "low": 27061, - "open": 27061, - "volume": 27061 - }, - "open": "2020-11-05T14:45:45", - "seconds": 15 - }, - { - "hive": { - "close": 127010, - "high": 127010, - "low": 16981, - "open": 16981, - "volume": 143991 - }, - "id": 2761111, - "non_hive": { - "close": 14959, - "high": 14959, - "low": 1999, - "open": 1999, - "volume": 16958 - }, - "open": "2020-11-05T14:46:00", - "seconds": 15 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2761113, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:47:15", - "seconds": 15 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2761115, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:47:45", - "seconds": 15 - }, - { - "hive": { - "close": 13276, - "high": 13276, - "low": 13276, - "open": 13276, - "volume": 13276 - }, - "id": 2761116, - "non_hive": { - "close": 1558, - "high": 1558, - "low": 1558, - "open": 1558, - "volume": 1558 - }, - "open": "2020-11-05T14:50:15", - "seconds": 15 - }, - { - "hive": { - "close": 13000, - "high": 13000, - "low": 13000, - "open": 13000, - "volume": 13000 - }, - "id": 2761119, - "non_hive": { - "close": 1508, - "high": 1508, - "low": 1508, - "open": 1508, - "volume": 1508 - }, - "open": "2020-11-05T14:51:15", - "seconds": 15 - }, - { - "hive": { - "close": 1681, - "high": 1681, - "low": 3758, - "open": 3758, - "volume": 11469 - }, - "id": 2761121, - "non_hive": { - "close": 198, - "high": 198, - "low": 441, - "open": 441, - "volume": 1347 - }, - "open": "2020-11-05T14:53:15", - "seconds": 15 - }, - { - "hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 6000 - }, - "id": 2761123, - "non_hive": { - "close": 698, - "high": 698, - "low": 698, - "open": 698, - "volume": 698 - }, - "open": "2020-11-05T14:55:30", - "seconds": 15 - }, - { - "hive": { - "close": 4480, - "high": 4480, - "low": 4480, - "open": 4480, - "volume": 4480 - }, - "id": 2761126, - "non_hive": { - "close": 521, - "high": 521, - "low": 521, - "open": 521, - "volume": 521 - }, - "open": "2020-11-05T14:56:00", - "seconds": 15 - }, - { - "hive": { - "close": 28717, - "high": 28717, - "low": 28717, - "open": 28717, - "volume": 28717 - }, - "id": 2761128, - "non_hive": { - "close": 3370, - "high": 3370, - "low": 3370, - "open": 3370, - "volume": 3370 - }, - "open": "2020-11-05T14:58:00", - "seconds": 15 - }, - { - "hive": { - "close": 43623, - "high": 43623, - "low": 18311, - "open": 18311, - "volume": 84998 - }, - "id": 2761130, - "non_hive": { - "close": 5138, - "high": 5138, - "low": 2148, - "open": 2148, - "volume": 9993 - }, - "open": "2020-11-05T14:58:15", - "seconds": 15 - }, - { - "hive": { - "close": 17191, - "high": 51, - "low": 17191, - "open": 51, - "volume": 17242 - }, - "id": 2761131, - "non_hive": { - "close": 1994, - "high": 6, - "low": 1994, - "open": 6, - "volume": 2000 - }, - "open": "2020-11-05T15:00:00", - "seconds": 15 - }, - { - "hive": { - "close": 7505, - "high": 7505, - "low": 7505, - "open": 7505, - "volume": 7505 - }, - "id": 2761135, - "non_hive": { - "close": 884, - "high": 884, - "low": 884, - "open": 884, - "volume": 884 - }, - "open": "2020-11-05T15:00:15", - "seconds": 15 - }, - { - "hive": { - "close": 50, - "high": 50, - "low": 50, - "open": 50, - "volume": 50 - }, - "id": 2761136, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-05T15:00:45", - "seconds": 15 - }, - { - "hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 6000 - }, - "id": 2761137, - "non_hive": { - "close": 698, - "high": 698, - "low": 698, - "open": 698, - "volume": 698 - }, - "open": "2020-11-05T15:03:30", - "seconds": 15 - }, - { - "hive": { - "close": 7500, - "high": 7500, - "low": 7500, - "open": 7500, - "volume": 7500 - }, - "id": 2761139, - "non_hive": { - "close": 883, - "high": 883, - "low": 883, - "open": 883, - "volume": 883 - }, - "open": "2020-11-05T15:04:00", - "seconds": 15 - }, - { - "hive": { - "close": 28926, - "high": 28926, - "low": 6031, - "open": 6031, - "volume": 34957 - }, - "id": 2761141, - "non_hive": { - "close": 3407, - "high": 3407, - "low": 710, - "open": 710, - "volume": 4117 - }, - "open": "2020-11-05T15:04:15", - "seconds": 15 - }, - { - "hive": { - "close": 178017, - "high": 178017, - "low": 16981, - "open": 16981, - "volume": 194998 - }, - "id": 2761142, - "non_hive": { - "close": 20964, - "high": 20964, - "low": 1999, - "open": 1999, - "volume": 22963 - }, - "open": "2020-11-05T15:04:45", - "seconds": 15 - }, - { - "hive": { - "close": 2588, - "high": 51, - "low": 2588, - "open": 51, - "volume": 17242 - }, - "id": 2761143, - "non_hive": { - "close": 300, - "high": 6, - "low": 300, - "open": 6, - "volume": 2006 - }, - "open": "2020-11-05T15:05:15", - "seconds": 15 - }, - { - "hive": { - "close": 17172, - "high": 17172, - "low": 17172, - "open": 17172, - "volume": 17172 - }, - "id": 2761146, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T15:07:15", - "seconds": 15 - }, - { - "hive": { - "close": 5884, - "high": 5116, - "low": 5884, - "open": 5116, - "volume": 11000 - }, - "id": 2761148, - "non_hive": { - "close": 685, - "high": 596, - "low": 685, - "open": 596, - "volume": 1281 - }, - "open": "2020-11-05T15:08:30", - "seconds": 15 - }, - { - "hive": { - "close": 163, - "high": 163, - "low": 163, - "open": 163, - "volume": 163 - }, - "id": 2761150, - "non_hive": { - "close": 19, - "high": 19, - "low": 19, - "open": 19, - "volume": 19 - }, - "open": "2020-11-05T15:14:00", - "seconds": 15 - }, - { - "hive": { - "close": 21610, - "high": 21610, - "low": 21610, - "open": 21610, - "volume": 21610 - }, - "id": 2761153, - "non_hive": { - "close": 2536, - "high": 2536, - "low": 2536, - "open": 2536, - "volume": 2536 - }, - "open": "2020-11-05T15:14:30", - "seconds": 15 - }, - { - "hive": { - "close": 10481, - "high": 10481, - "low": 74514, - "open": 74514, - "volume": 84995 - }, - "id": 2761154, - "non_hive": { - "close": 1230, - "high": 1230, - "low": 8744, - "open": 8744, - "volume": 9974 - }, - "open": "2020-11-05T15:14:45", - "seconds": 15 - }, - { - "hive": { - "close": 10000, - "high": 10000, - "low": 10000, - "open": 10000, - "volume": 10000 - }, - "id": 2761155, - "non_hive": { - "close": 1160, - "high": 1160, - "low": 1160, - "open": 1160, - "volume": 1160 - }, - "open": "2020-11-05T15:16:30", - "seconds": 15 - }, - { - "hive": { - "close": 42, - "high": 42, - "low": 2000, - "open": 2000, - "volume": 2042 - }, - "id": 2761158, - "non_hive": { - "close": 5, - "high": 5, - "low": 234, - "open": 234, - "volume": 239 - }, - "open": "2020-11-05T15:18:00", - "seconds": 15 - }, - { - "hive": { - "close": 758127, - "high": 758127, - "low": 6511, - "open": 6511, - "volume": 1000157 - }, - "id": 2761160, - "non_hive": { - "close": 89735, - "high": 89735, - "low": 764, - "open": 764, - "volume": 118364 - }, - "open": "2020-11-05T15:23:45", - "seconds": 15 - }, - { - "hive": { - "close": 2925, - "high": 4075, - "low": 2925, - "open": 4075, - "volume": 7000 - }, - "id": 2761163, - "non_hive": { - "close": 341, - "high": 482, - "low": 341, - "open": 482, - "volume": 823 - }, - "open": "2020-11-05T15:29:00", - "seconds": 15 - }, - { - "hive": { - "close": 5740, - "high": 4260, - "low": 5740, - "open": 4260, - "volume": 10000 - }, - "id": 2761166, - "non_hive": { - "close": 670, - "high": 498, - "low": 670, - "open": 498, - "volume": 1168 - }, - "open": "2020-11-05T15:29:45", - "seconds": 15 - }, - { - "hive": { - "close": 5718, - "high": 282, - "low": 5718, - "open": 282, - "volume": 6000 - }, - "id": 2761167, - "non_hive": { - "close": 665, - "high": 33, - "low": 665, - "open": 33, - "volume": 698 - }, - "open": "2020-11-05T15:32:00", - "seconds": 15 - }, - { - "hive": { - "close": 562, - "high": 562, - "low": 562, - "open": 562, - "volume": 562 - }, - "id": 2761170, - "non_hive": { - "close": 66, - "high": 66, - "low": 66, - "open": 66, - "volume": 66 - }, - "open": "2020-11-05T15:35:45", - "seconds": 15 - }, - { - "hive": { - "close": 2599, - "high": 566, - "low": 2599, - "open": 566, - "volume": 3500 - }, - "id": 2761173, - "non_hive": { - "close": 301, - "high": 66, - "low": 301, - "open": 66, - "volume": 406 - }, - "open": "2020-11-05T15:37:45", - "seconds": 15 - }, - { - "hive": { - "close": 9190, - "high": 810, - "low": 9190, - "open": 810, - "volume": 10000 - }, - "id": 2761175, - "non_hive": { - "close": 1065, - "high": 94, - "low": 1065, - "open": 94, - "volume": 1159 - }, - "open": "2020-11-05T15:44:00", - "seconds": 15 - }, - { - "hive": { - "close": 16472, - "high": 16472, - "low": 16472, - "open": 16472, - "volume": 16472 - }, - "id": 2761178, - "non_hive": { - "close": 1933, - "high": 1933, - "low": 1933, - "open": 1933, - "volume": 1933 - }, - "open": "2020-11-05T15:49:45", - "seconds": 15 - }, - { - "hive": { - "close": 5674, - "high": 5674, - "low": 5674, - "open": 5674, - "volume": 5674 - }, - "id": 2761181, - "non_hive": { - "close": 666, - "high": 666, - "low": 666, - "open": 666, - "volume": 666 - }, - "open": "2020-11-05T15:50:15", - "seconds": 15 - }, - { - "hive": { - "close": 1704, - "high": 1704, - "low": 1704, - "open": 1704, - "volume": 1704 - }, - "id": 2761184, - "non_hive": { - "close": 200, - "high": 200, - "low": 200, - "open": 200, - "volume": 200 - }, - "open": "2020-11-05T15:55:30", - "seconds": 15 - }, - { - "hive": { - "close": 4295, - "high": 4295, - "low": 4295, - "open": 4295, - "volume": 4295 - }, - "id": 2761187, - "non_hive": { - "close": 504, - "high": 504, - "low": 504, - "open": 504, - "volume": 504 - }, - "open": "2020-11-05T15:58:45", - "seconds": 15 - }, - { - "hive": { - "close": 8505, - "high": 8505, - "low": 17045, - "open": 17045, - "volume": 25550 - }, - "id": 2761189, - "non_hive": { - "close": 998, - "high": 998, - "low": 1999, - "open": 1999, - "volume": 2997 - }, - "open": "2020-11-05T16:00:15", - "seconds": 15 - }, - { - "hive": { - "close": 14631, - "high": 14631, - "low": 14631, - "open": 14631, - "volume": 14631 - }, - "id": 2761193, - "non_hive": { - "close": 1716, - "high": 1716, - "low": 1716, - "open": 1716, - "volume": 1716 - }, - "open": "2020-11-05T16:03:45", - "seconds": 15 - }, - { - "hive": { - "close": 2414, - "high": 2414, - "low": 2414, - "open": 2414, - "volume": 2414 - }, - "id": 2761195, - "non_hive": { - "close": 283, - "high": 283, - "low": 283, - "open": 283, - "volume": 283 - }, - "open": "2020-11-05T16:26:15", - "seconds": 15 - }, - { - "hive": { - "close": 31072, - "high": 31072, - "low": 31072, - "open": 31072, - "volume": 31072 - }, - "id": 2761198, - "non_hive": { - "close": 3646, - "high": 3646, - "low": 3646, - "open": 3646, - "volume": 3646 - }, - "open": "2020-11-05T16:26:30", - "seconds": 15 - }, - { - "hive": { - "close": 366, - "high": 366, - "low": 250, - "open": 250, - "volume": 616 - }, - "id": 2761199, - "non_hive": { - "close": 43, - "high": 43, - "low": 29, - "open": 29, - "volume": 72 - }, - "open": "2020-11-05T16:27:00", - "seconds": 15 - }, - { - "hive": { - "close": 503950, - "high": 503950, - "low": 503950, - "open": 503950, - "volume": 503950 - }, - "id": 2761201, - "non_hive": { - "close": 59102, - "high": 59102, - "low": 59102, - "open": 59102, - "volume": 59102 - }, - "open": "2020-11-05T16:27:45", - "seconds": 15 - }, - { - "hive": { - "close": 320032, - "high": 24356, - "low": 320032, - "open": 24356, - "volume": 344388 - }, - "id": 2761202, - "non_hive": { - "close": 37532, - "high": 2858, - "low": 37532, - "open": 2858, - "volume": 40390 - }, - "open": "2020-11-05T16:32:30", - "seconds": 15 - }, - { - "hive": { - "close": 18203, - "high": 707, - "low": 18203, - "open": 707, - "volume": 36003 - }, - "id": 2761205, - "non_hive": { - "close": 2129, - "high": 83, - "low": 2129, - "open": 83, - "volume": 4212 - }, - "open": "2020-11-05T16:34:45", - "seconds": 15 - }, - { - "hive": { - "close": 50000, - "high": 50000, - "low": 50000, - "open": 50000, - "volume": 50000 - }, - "id": 2761207, - "non_hive": { - "close": 5850, - "high": 5850, - "low": 5850, - "open": 5850, - "volume": 5850 - }, - "open": "2020-11-05T16:35:00", - "seconds": 15 - }, - { - "hive": { - "close": 20, - "high": 20, - "low": 20, - "open": 20, - "volume": 20 - }, - "id": 2761210, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-11-05T16:35:15", - "seconds": 15 - }, - { - "hive": { - "close": 1116, - "high": 1116, - "low": 1116, - "open": 1116, - "volume": 1116 - }, - "id": 2761211, - "non_hive": { - "close": 131, - "high": 131, - "low": 131, - "open": 131, - "volume": 131 - }, - "open": "2020-11-05T16:39:45", - "seconds": 15 - }, - { - "hive": { - "close": 9996, - "high": 9996, - "low": 9996, - "open": 9996, - "volume": 9996 - }, - "id": 2761213, - "non_hive": { - "close": 1173, - "high": 1173, - "low": 1173, - "open": 1173, - "volume": 1173 - }, - "open": "2020-11-05T16:41:30", - "seconds": 15 - }, - { - "hive": { - "close": 22047, - "high": 22047, - "low": 22047, - "open": 22047, - "volume": 22047 - }, - "id": 2761216, - "non_hive": { - "close": 2587, - "high": 2587, - "low": 2587, - "open": 2587, - "volume": 2587 - }, - "open": "2020-11-05T16:42:00", - "seconds": 15 - }, - { - "hive": { - "close": 5664, - "high": 5664, - "low": 16841, - "open": 16841, - "volume": 22505 - }, - "id": 2761218, - "non_hive": { - "close": 665, - "high": 665, - "low": 1976, - "open": 1976, - "volume": 2641 - }, - "open": "2020-11-05T16:46:15", - "seconds": 15 - }, - { - "hive": { - "close": 436931, - "high": 104838, - "low": 436931, - "open": 104838, - "volume": 558558 - }, - "id": 2761221, - "non_hive": { - "close": 51121, - "high": 12359, - "low": 51121, - "open": 12359, - "volume": 65450 - }, - "open": "2020-11-05T16:47:00", - "seconds": 15 - }, - { - "hive": { - "close": 2242, - "high": 2242, - "low": 2242, - "open": 2242, - "volume": 2242 - }, - "id": 2761223, - "non_hive": { - "close": 262, - "high": 262, - "low": 262, - "open": 262, - "volume": 262 - }, - "open": "2020-11-05T16:47:30", - "seconds": 15 - }, - { - "hive": { - "close": 69657, - "high": 69657, - "low": 14867, - "open": 14867, - "volume": 84524 - }, - "id": 2761224, - "non_hive": { - "close": 8143, - "high": 8143, - "low": 1737, - "open": 1737, - "volume": 9880 - }, - "open": "2020-11-05T16:54:45", - "seconds": 15 - }, - { - "hive": { - "close": 15920, - "high": 15920, - "low": 15920, - "open": 15920, - "volume": 15920 - }, - "id": 2761227, - "non_hive": { - "close": 1860, - "high": 1860, - "low": 1860, - "open": 1860, - "volume": 1860 - }, - "open": "2020-11-05T17:00:15", - "seconds": 15 - }, - { - "hive": { - "close": 141440, - "high": 141440, - "low": 17118, - "open": 17118, - "volume": 158558 - }, - "id": 2761231, - "non_hive": { - "close": 16525, - "high": 16525, - "low": 1999, - "open": 1999, - "volume": 18524 - }, - "open": "2020-11-05T17:04:00", - "seconds": 15 - }, - { - "hive": { - "close": 57135, - "high": 57135, - "low": 57135, - "open": 57135, - "volume": 57135 - }, - "id": 2761233, - "non_hive": { - "close": 6672, - "high": 6672, - "low": 6672, - "open": 6672, - "volume": 6672 - }, - "open": "2020-11-05T17:07:00", - "seconds": 15 - }, - { - "hive": { - "close": 14922, - "high": 14922, - "low": 14922, - "open": 14922, - "volume": 14922 - }, - "id": 2761236, - "non_hive": { - "close": 1760, - "high": 1760, - "low": 1760, - "open": 1760, - "volume": 1760 - }, - "open": "2020-11-05T17:16:00", - "seconds": 15 - }, - { - "hive": { - "close": 3510, - "high": 3510, - "low": 3510, - "open": 3510, - "volume": 3510 - }, - "id": 2761239, - "non_hive": { - "close": 414, - "high": 414, - "low": 414, - "open": 414, - "volume": 414 - }, - "open": "2020-11-05T17:17:45", - "seconds": 15 - }, - { - "hive": { - "close": 13440, - "high": 13440, - "low": 13440, - "open": 13440, - "volume": 13440 - }, - "id": 2761241, - "non_hive": { - "close": 1585, - "high": 1585, - "low": 1585, - "open": 1585, - "volume": 1585 - }, - "open": "2020-11-05T17:20:45", - "seconds": 15 - }, - { - "hive": { - "close": 1288, - "high": 1288, - "low": 1288, - "open": 1288, - "volume": 1288 - }, - "id": 2761244, - "non_hive": { - "close": 152, - "high": 152, - "low": 152, - "open": 152, - "volume": 152 - }, - "open": "2020-11-05T17:22:30", - "seconds": 15 - }, - { - "hive": { - "close": 19603, - "high": 19603, - "low": 19603, - "open": 19603, - "volume": 19603 - }, - "id": 2761246, - "non_hive": { - "close": 2312, - "high": 2312, - "low": 2312, - "open": 2312, - "volume": 2312 - }, - "open": "2020-11-05T17:35:00", - "seconds": 15 - }, - { - "hive": { - "close": 40463, - "high": 40463, - "low": 40463, - "open": 40463, - "volume": 40463 - }, - "id": 2761249, - "non_hive": { - "close": 4768, - "high": 4768, - "low": 4768, - "open": 4768, - "volume": 4768 - }, - "open": "2020-11-05T17:41:30", - "seconds": 15 - }, - { - "hive": { - "close": 12897, - "high": 12897, - "low": 12897, - "open": 12897, - "volume": 12897 - }, - "id": 2761252, - "non_hive": { - "close": 1519, - "high": 1519, - "low": 1519, - "open": 1519, - "volume": 1519 - }, - "open": "2020-11-05T17:47:30", - "seconds": 15 - }, - { - "hive": { - "close": 4076, - "high": 4076, - "low": 4076, - "open": 4076, - "volume": 4076 - }, - "id": 2761255, - "non_hive": { - "close": 480, - "high": 480, - "low": 480, - "open": 480, - "volume": 480 - }, - "open": "2020-11-05T17:52:30", - "seconds": 15 - }, - { - "hive": { - "close": 14087, - "high": 14087, - "low": 14087, - "open": 14087, - "volume": 14087 - }, - "id": 2761258, - "non_hive": { - "close": 1660, - "high": 1660, - "low": 1660, - "open": 1660, - "volume": 1660 - }, - "open": "2020-11-05T17:53:30", - "seconds": 15 - }, - { - "hive": { - "close": 712, - "high": 712, - "low": 712, - "open": 712, - "volume": 712 - }, - "id": 2761260, - "non_hive": { - "close": 84, - "high": 84, - "low": 84, - "open": 84, - "volume": 84 - }, - "open": "2020-11-05T17:58:15", - "seconds": 15 - }, - { - "hive": { - "close": 1807, - "high": 1807, - "low": 1807, - "open": 1807, - "volume": 1807 - }, - "id": 2761263, - "non_hive": { - "close": 213, - "high": 213, - "low": 213, - "open": 213, - "volume": 213 - }, - "open": "2020-11-05T18:16:45", - "seconds": 15 - }, - { - "hive": { - "close": 37628, - "high": 37628, - "low": 37628, - "open": 37628, - "volume": 37628 - }, - "id": 2761267, - "non_hive": { - "close": 4434, - "high": 4434, - "low": 4434, - "open": 4434, - "volume": 4434 - }, - "open": "2020-11-05T18:17:30", - "seconds": 15 - }, - { - "hive": { - "close": 10234, - "high": 10234, - "low": 10234, - "open": 10234, - "volume": 10234 - }, - "id": 2761269, - "non_hive": { - "close": 1206, - "high": 1206, - "low": 1206, - "open": 1206, - "volume": 1206 - }, - "open": "2020-11-05T18:23:30", - "seconds": 15 - }, - { - "hive": { - "close": 3988, - "high": 3988, - "low": 3988, - "open": 3988, - "volume": 3988 - }, - "id": 2761272, - "non_hive": { - "close": 470, - "high": 470, - "low": 470, - "open": 470, - "volume": 470 - }, - "open": "2020-11-05T18:28:15", - "seconds": 15 - }, - { - "hive": { - "close": 2172, - "high": 2172, - "low": 2172, - "open": 2172, - "volume": 2172 - }, - "id": 2761275, - "non_hive": { - "close": 256, - "high": 256, - "low": 256, - "open": 256, - "volume": 256 - }, - "open": "2020-11-05T18:33:00", - "seconds": 15 - }, - { - "hive": { - "close": 128, - "high": 1349, - "low": 128, - "open": 5058, - "volume": 22320 - }, - "id": 2761278, - "non_hive": { - "close": 15, - "high": 159, - "low": 15, - "open": 596, - "volume": 2629 - }, - "open": "2020-11-05T18:39:15", - "seconds": 15 - }, - { - "hive": { - "close": 16066, - "high": 16066, - "low": 16066, - "open": 16066, - "volume": 16066 - }, - "id": 2761281, - "non_hive": { - "close": 1874, - "high": 1874, - "low": 1874, - "open": 1874, - "volume": 1874 - }, - "open": "2020-11-05T18:46:30", - "seconds": 15 - }, - { - "hive": { - "close": 4758, - "high": 4758, - "low": 4758, - "open": 4758, - "volume": 4758 - }, - "id": 2761284, - "non_hive": { - "close": 555, - "high": 555, - "low": 555, - "open": 555, - "volume": 555 - }, - "open": "2020-11-05T18:53:15", - "seconds": 15 - }, - { - "hive": { - "close": 9935, - "high": 9935, - "low": 74, - "open": 74, - "volume": 10009 - }, - "id": 2761287, - "non_hive": { - "close": 1159, - "high": 1159, - "low": 8, - "open": 8, - "volume": 1167 - }, - "open": "2020-11-05T18:55:45", - "seconds": 15 - }, - { - "hive": { - "close": 33630, - "high": 33630, - "low": 33630, - "open": 33630, - "volume": 33630 - }, - "id": 2761290, - "non_hive": { - "close": 3923, - "high": 3923, - "low": 3923, - "open": 3923, - "volume": 3923 - }, - "open": "2020-11-05T18:58:45", - "seconds": 15 - }, - { - "hive": { - "close": 8130, - "high": 8130, - "low": 8130, - "open": 8130, - "volume": 8130 - }, - "id": 2761292, - "non_hive": { - "close": 957, - "high": 957, - "low": 957, - "open": 957, - "volume": 957 - }, - "open": "2020-11-05T19:25:30", - "seconds": 15 - }, - { - "hive": { - "close": 8853, - "high": 8853, - "low": 8853, - "open": 8853, - "volume": 8853 - }, - "id": 2761296, - "non_hive": { - "close": 1042, - "high": 1042, - "low": 1042, - "open": 1042, - "volume": 1042 - }, - "open": "2020-11-05T19:29:30", - "seconds": 15 - }, - { - "hive": { - "close": 34546, - "high": 34546, - "low": 4043, - "open": 4043, - "volume": 38589 - }, - "id": 2761298, - "non_hive": { - "close": 4073, - "high": 4073, - "low": 476, - "open": 476, - "volume": 4549 - }, - "open": "2020-11-05T19:30:30", - "seconds": 15 - }, - { - "hive": { - "close": 2985, - "high": 2985, - "low": 2985, - "open": 2985, - "volume": 2985 - }, - "id": 2761301, - "non_hive": { - "close": 352, - "high": 352, - "low": 352, - "open": 352, - "volume": 352 - }, - "open": "2020-11-05T19:32:30", - "seconds": 15 - }, - { - "hive": { - "close": 2298, - "high": 2298, - "low": 10419, - "open": 10419, - "volume": 12717 - }, - "id": 2761303, - "non_hive": { - "close": 271, - "high": 271, - "low": 1227, - "open": 1227, - "volume": 1498 - }, - "open": "2020-11-05T19:35:30", - "seconds": 15 - }, - { - "hive": { - "close": 15369, - "high": 15369, - "low": 15369, - "open": 15369, - "volume": 15369 - }, - "id": 2761306, - "non_hive": { - "close": 1812, - "high": 1812, - "low": 1812, - "open": 1812, - "volume": 1812 - }, - "open": "2020-11-05T19:38:15", - "seconds": 15 - }, - { - "hive": { - "close": 5827, - "high": 5827, - "low": 5827, - "open": 5827, - "volume": 5827 - }, - "id": 2761308, - "non_hive": { - "close": 687, - "high": 687, - "low": 687, - "open": 687, - "volume": 687 - }, - "open": "2020-11-05T19:39:00", - "seconds": 15 - }, - { - "hive": { - "close": 1696, - "high": 1696, - "low": 1696, - "open": 1696, - "volume": 1696 - }, - "id": 2761310, - "non_hive": { - "close": 200, - "high": 200, - "low": 200, - "open": 200, - "volume": 200 - }, - "open": "2020-11-05T19:43:45", - "seconds": 15 - }, - { - "hive": { - "close": 30678, - "high": 30678, - "low": 30678, - "open": 30678, - "volume": 30678 - }, - "id": 2761313, - "non_hive": { - "close": 3617, - "high": 3617, - "low": 3617, - "open": 3617, - "volume": 3617 - }, - "open": "2020-11-05T19:45:00", - "seconds": 15 - }, - { - "hive": { - "close": 1696, - "high": 1696, - "low": 1696, - "open": 1696, - "volume": 1696 - }, - "id": 2761316, - "non_hive": { - "close": 200, - "high": 200, - "low": 200, - "open": 200, - "volume": 200 - }, - "open": "2020-11-05T19:51:30", - "seconds": 15 - }, - { - "hive": { - "close": 3104, - "high": 3104, - "low": 3104, - "open": 3104, - "volume": 3104 - }, - "id": 2761319, - "non_hive": { - "close": 366, - "high": 366, - "low": 366, - "open": 366, - "volume": 366 - }, - "open": "2020-11-05T19:52:00", - "seconds": 15 - }, - { - "hive": { - "close": 6463, - "high": 6463, - "low": 6463, - "open": 6463, - "volume": 6463 - }, - "id": 2761321, - "non_hive": { - "close": 762, - "high": 762, - "low": 762, - "open": 762, - "volume": 762 - }, - "open": "2020-11-05T19:59:30", - "seconds": 15 - }, - { - "hive": { - "close": 27260, - "high": 27260, - "low": 27260, - "open": 27260, - "volume": 27260 - }, - "id": 2761324, - "non_hive": { - "close": 3214, - "high": 3214, - "low": 3214, - "open": 3214, - "volume": 3214 - }, - "open": "2020-11-05T20:05:15", - "seconds": 15 - }, - { - "hive": { - "close": 9007, - "high": 9007, - "low": 9007, - "open": 9007, - "volume": 9007 - }, - "id": 2761328, - "non_hive": { - "close": 1062, - "high": 1062, - "low": 1062, - "open": 1062, - "volume": 1062 - }, - "open": "2020-11-05T20:11:15", - "seconds": 15 - }, - { - "hive": { - "close": 1413828, - "high": 1413828, - "low": 1413828, - "open": 1413828, - "volume": 1413828 - }, - "id": 2761331, - "non_hive": { - "close": 166689, - "high": 166689, - "low": 166689, - "open": 166689, - "volume": 166689 - }, - "open": "2020-11-05T20:12:15", - "seconds": 15 - }, - { - "hive": { - "close": 4005, - "high": 4005, - "low": 4005, - "open": 4005, - "volume": 4005 - }, - "id": 2761333, - "non_hive": { - "close": 472, - "high": 472, - "low": 472, - "open": 472, - "volume": 472 - }, - "open": "2020-11-05T20:13:15", - "seconds": 15 - }, - { - "hive": { - "close": 89932, - "high": 6755, - "low": 89932, - "open": 6755, - "volume": 100000 - }, - "id": 2761335, - "non_hive": { - "close": 10497, - "high": 796, - "low": 10497, - "open": 796, - "volume": 11683 - }, - "open": "2020-11-05T20:40:30", - "seconds": 15 - }, - { - "hive": { - "close": 81, - "high": 81, - "low": 81, - "open": 81, - "volume": 81 - }, - "id": 2761338, - "non_hive": { - "close": 9, - "high": 9, - "low": 9, - "open": 9, - "volume": 9 - }, - "open": "2020-11-05T20:49:45", - "seconds": 15 - }, - { - "hive": { - "close": 19085, - "high": 19085, - "low": 19085, - "open": 19085, - "volume": 19085 - }, - "id": 2761341, - "non_hive": { - "close": 2246, - "high": 2246, - "low": 2246, - "open": 2246, - "volume": 2246 - }, - "open": "2020-11-05T21:01:15", - "seconds": 15 - }, - { - "hive": { - "close": 17003, - "high": 17003, - "low": 356218, - "open": 3782, - "volume": 377003 - }, - "id": 2761345, - "non_hive": { - "close": 2001, - "high": 2001, - "low": 41582, - "open": 445, - "volume": 44028 - }, - "open": "2020-11-05T21:02:00", - "seconds": 15 - }, - { - "hive": { - "close": 117431, - "high": 2569, - "low": 117431, - "open": 2569, - "volume": 120000 - }, - "id": 2761347, - "non_hive": { - "close": 13707, - "high": 300, - "low": 13707, - "open": 300, - "volume": 14007 - }, - "open": "2020-11-05T21:02:15", - "seconds": 15 - }, - { - "hive": { - "close": 17103, - "high": 17103, - "low": 17103, - "open": 17103, - "volume": 17103 - }, - "id": 2761348, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:03:00", - "seconds": 15 - }, - { - "hive": { - "close": 17103, - "high": 17103, - "low": 17103, - "open": 17103, - "volume": 17103 - }, - "id": 2761350, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:03:30", - "seconds": 15 - }, - { - "hive": { - "close": 17103, - "high": 17103, - "low": 17103, - "open": 17103, - "volume": 17103 - }, - "id": 2761351, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:04:00", - "seconds": 15 - }, - { - "hive": { - "close": 17046, - "high": 17046, - "low": 17046, - "open": 17046, - "volume": 17046 - }, - "id": 2761353, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:04:30", - "seconds": 15 - }, - { - "hive": { - "close": 17046, - "high": 17046, - "low": 17046, - "open": 17046, - "volume": 17046 - }, - "id": 2761354, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:04:45", - "seconds": 15 - }, - { - "hive": { - "close": 17046, - "high": 17046, - "low": 17046, - "open": 17046, - "volume": 17046 - }, - "id": 2761355, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:05:00", - "seconds": 15 - }, - { - "hive": { - "close": 17046, - "high": 17046, - "low": 17046, - "open": 17046, - "volume": 17046 - }, - "id": 2761358, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:05:15", - "seconds": 15 - }, - { - "hive": { - "close": 16995, - "high": 16995, - "low": 16995, - "open": 16995, - "volume": 16995 - }, - "id": 2761359, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T21:07:45", - "seconds": 15 - }, - { - "hive": { - "close": 4011, - "high": 4011, - "low": 4011, - "open": 4011, - "volume": 4011 - }, - "id": 2761361, - "non_hive": { - "close": 472, - "high": 472, - "low": 472, - "open": 472, - "volume": 472 - }, - "open": "2020-11-05T21:09:30", - "seconds": 15 - }, - { - "hive": { - "close": 4444, - "high": 4444, - "low": 4444, - "open": 4444, - "volume": 4444 - }, - "id": 2761363, - "non_hive": { - "close": 522, - "high": 522, - "low": 522, - "open": 522, - "volume": 522 - }, - "open": "2020-11-05T21:09:45", - "seconds": 15 - }, - { - "hive": { - "close": 8459, - "high": 8459, - "low": 8459, - "open": 8459, - "volume": 8459 - }, - "id": 2761364, - "non_hive": { - "close": 995, - "high": 995, - "low": 995, - "open": 995, - "volume": 995 - }, - "open": "2020-11-05T21:12:45", - "seconds": 15 - }, - { - "hive": { - "close": 8484, - "high": 8484, - "low": 8484, - "open": 8484, - "volume": 8484 - }, - "id": 2761367, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-11-05T21:16:00", - "seconds": 15 - }, - { - "hive": { - "close": 17046, - "high": 17046, - "low": 17046, - "open": 17046, - "volume": 17046 - }, - "id": 2761370, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:19:15", - "seconds": 15 - }, - { - "hive": { - "close": 17046, - "high": 17046, - "low": 17046, - "open": 17046, - "volume": 17046 - }, - "id": 2761372, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:19:45", - "seconds": 15 - }, - { - "hive": { - "close": 17046, - "high": 17046, - "low": 17046, - "open": 17046, - "volume": 17046 - }, - "id": 2761373, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:20:00", - "seconds": 15 - }, - { - "hive": { - "close": 1234, - "high": 1234, - "low": 1234, - "open": 1234, - "volume": 1234 - }, - "id": 2761376, - "non_hive": { - "close": 145, - "high": 145, - "low": 145, - "open": 145, - "volume": 145 - }, - "open": "2020-11-05T21:20:30", - "seconds": 15 - }, - { - "hive": { - "close": 52, - "high": 52, - "low": 52, - "open": 52, - "volume": 52 - }, - "id": 2761377, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-05T21:23:15", - "seconds": 15 - }, - { - "hive": { - "close": 12699, - "high": 12301, - "low": 12699, - "open": 12301, - "volume": 25000 - }, - "id": 2761379, - "non_hive": { - "close": 1493, - "high": 1447, - "low": 1493, - "open": 1447, - "volume": 2940 - }, - "open": "2020-11-05T21:24:15", - "seconds": 15 - }, - { - "hive": { - "close": 5987, - "high": 161, - "low": 5987, - "open": 161, - "volume": 13845 - }, - "id": 2761381, - "non_hive": { - "close": 700, - "high": 19, - "low": 700, - "open": 19, - "volume": 1622 - }, - "open": "2020-11-05T21:27:00", - "seconds": 15 - }, - { - "hive": { - "close": 838, - "high": 838, - "low": 16949, - "open": 16949, - "volume": 17787 - }, - "id": 2761384, - "non_hive": { - "close": 99, - "high": 99, - "low": 1999, - "open": 1999, - "volume": 2098 - }, - "open": "2020-11-05T21:28:00", - "seconds": 15 - }, - { - "hive": { - "close": 4152, - "high": 4152, - "low": 4152, - "open": 4152, - "volume": 4152 - }, - "id": 2761386, - "non_hive": { - "close": 490, - "high": 490, - "low": 490, - "open": 490, - "volume": 490 - }, - "open": "2020-11-05T21:28:45", - "seconds": 15 - }, - { - "hive": { - "close": 1901, - "high": 17100, - "low": 1901, - "open": 17100, - "volume": 19001 - }, - "id": 2761387, - "non_hive": { - "close": 222, - "high": 2000, - "low": 222, - "open": 2000, - "volume": 2222 - }, - "open": "2020-11-05T21:31:15", - "seconds": 15 - }, - { - "hive": { - "close": 17101, - "high": 17101, - "low": 17101, - "open": 17101, - "volume": 17101 - }, - "id": 2761390, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T21:31:30", - "seconds": 15 - }, - { - "hive": { - "close": 755, - "high": 755, - "low": 755, - "open": 755, - "volume": 755 - }, - "id": 2761391, - "non_hive": { - "close": 89, - "high": 89, - "low": 89, - "open": 89, - "volume": 89 - }, - "open": "2020-11-05T21:34:30", - "seconds": 15 - }, - { - "hive": { - "close": 2020, - "high": 2020, - "low": 2020, - "open": 2020, - "volume": 2020 - }, - "id": 2761393, - "non_hive": { - "close": 238, - "high": 238, - "low": 238, - "open": 238, - "volume": 238 - }, - "open": "2020-11-05T21:35:45", - "seconds": 15 - }, - { - "hive": { - "close": 8501, - "high": 8501, - "low": 8501, - "open": 8501, - "volume": 8501 - }, - "id": 2761396, - "non_hive": { - "close": 1001, - "high": 1001, - "low": 1001, - "open": 1001, - "volume": 1001 - }, - "open": "2020-11-05T21:46:00", - "seconds": 15 - }, - { - "hive": { - "close": 9364, - "high": 9364, - "low": 9364, - "open": 9364, - "volume": 9364 - }, - "id": 2761399, - "non_hive": { - "close": 1095, - "high": 1095, - "low": 1095, - "open": 1095, - "volume": 1095 - }, - "open": "2020-11-05T21:46:30", - "seconds": 15 - }, - { - "hive": { - "close": 6021, - "high": 6021, - "low": 6021, - "open": 6021, - "volume": 6021 - }, - "id": 2761400, - "non_hive": { - "close": 704, - "high": 704, - "low": 704, - "open": 704, - "volume": 704 - }, - "open": "2020-11-05T21:47:45", - "seconds": 15 - }, - { - "hive": { - "close": 34415, - "high": 77, - "low": 34415, - "open": 77, - "volume": 123000 - }, - "id": 2761402, - "non_hive": { - "close": 4014, - "high": 9, - "low": 4014, - "open": 9, - "volume": 14348 - }, - "open": "2020-11-05T21:49:00", - "seconds": 15 - }, - { - "hive": { - "close": 601, - "high": 601, - "low": 8477, - "open": 8477, - "volume": 111990 - }, - "id": 2761404, - "non_hive": { - "close": 71, - "high": 71, - "low": 998, - "open": 998, - "volume": 13209 - }, - "open": "2020-11-05T21:52:00", - "seconds": 15 - }, - { - "hive": { - "close": 409, - "high": 409, - "low": 409, - "open": 409, - "volume": 409 - }, - "id": 2761407, - "non_hive": { - "close": 48, - "high": 48, - "low": 48, - "open": 48, - "volume": 48 - }, - "open": "2020-11-05T21:54:15", - "seconds": 15 - }, - { - "hive": { - "close": 6002, - "high": 6002, - "low": 6002, - "open": 6002, - "volume": 6002 - }, - "id": 2761409, - "non_hive": { - "close": 708, - "high": 708, - "low": 708, - "open": 708, - "volume": 708 - }, - "open": "2020-11-05T21:55:00", - "seconds": 15 - }, - { - "hive": { - "close": 113016, - "high": 113016, - "low": 113016, - "open": 113016, - "volume": 113016 - }, - "id": 2761412, - "non_hive": { - "close": 13336, - "high": 13336, - "low": 13336, - "open": 13336, - "volume": 13336 - }, - "open": "2020-11-05T21:55:30", - "seconds": 15 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2761413, - "non_hive": { - "close": 117, - "high": 117, - "low": 117, - "open": 117, - "volume": 117 - }, - "open": "2020-11-05T21:59:15", - "seconds": 15 - }, - { - "hive": { - "close": 3476, - "high": 3476, - "low": 3476, - "open": 3476, - "volume": 3476 - }, - "id": 2761415, - "non_hive": { - "close": 410, - "high": 410, - "low": 410, - "open": 410, - "volume": 410 - }, - "open": "2020-11-05T22:00:45", - "seconds": 15 - }, - { - "hive": { - "close": 13474, - "high": 13474, - "low": 13474, - "open": 13474, - "volume": 13474 - }, - "id": 2761419, - "non_hive": { - "close": 1589, - "high": 1589, - "low": 1589, - "open": 1589, - "volume": 1589 - }, - "open": "2020-11-05T22:01:45", - "seconds": 15 - }, - { - "hive": { - "close": 15143, - "high": 992, - "low": 15143, - "open": 992, - "volume": 16135 - }, - "id": 2761421, - "non_hive": { - "close": 1786, - "high": 117, - "low": 1786, - "open": 117, - "volume": 1903 - }, - "open": "2020-11-05T22:03:45", - "seconds": 15 - }, - { - "hive": { - "close": 16958, - "high": 16958, - "low": 16958, - "open": 16958, - "volume": 16958 - }, - "id": 2761423, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T22:04:30", - "seconds": 15 - }, - { - "hive": { - "close": 16958, - "high": 16958, - "low": 16958, - "open": 16958, - "volume": 16958 - }, - "id": 2761425, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T22:04:45", - "seconds": 15 - }, - { - "hive": { - "close": 16958, - "high": 16958, - "low": 16958, - "open": 16958, - "volume": 16958 - }, - "id": 2761426, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T22:05:00", - "seconds": 15 - }, - { - "hive": { - "close": 16958, - "high": 16958, - "low": 16958, - "open": 16958, - "volume": 16958 - }, - "id": 2761429, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T22:05:30", - "seconds": 15 - }, - { - "hive": { - "close": 16958, - "high": 16958, - "low": 16958, - "open": 16958, - "volume": 16958 - }, - "id": 2761430, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T22:05:45", - "seconds": 15 - }, - { - "hive": { - "close": 16958, - "high": 16958, - "low": 16958, - "open": 16958, - "volume": 16958 - }, - "id": 2761431, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T22:06:15", - "seconds": 15 - }, - { - "hive": { - "close": 31966, - "high": 31966, - "low": 11040, - "open": 11040, - "volume": 43006 - }, - "id": 2761433, - "non_hive": { - "close": 3770, - "high": 3770, - "low": 1302, - "open": 1302, - "volume": 5072 - }, - "open": "2020-11-05T22:06:30", - "seconds": 15 - }, - { - "hive": { - "close": 84268, - "high": 84268, - "low": 84268, - "open": 84268, - "volume": 84268 - }, - "id": 2761434, - "non_hive": { - "close": 9973, - "high": 9973, - "low": 9973, - "open": 9973, - "volume": 9973 - }, - "open": "2020-11-05T22:18:30", - "seconds": 15 - }, - { - "hive": { - "close": 30432, - "high": 30432, - "low": 30432, - "open": 30432, - "volume": 30432 - }, - "id": 2761437, - "non_hive": { - "close": 3602, - "high": 3602, - "low": 3602, - "open": 3602, - "volume": 3602 - }, - "open": "2020-11-05T22:22:15", - "seconds": 15 - }, - { - "hive": { - "close": 32652, - "high": 32652, - "low": 32652, - "open": 32652, - "volume": 32652 - }, - "id": 2761440, - "non_hive": { - "close": 3864, - "high": 3864, - "low": 3864, - "open": 3864, - "volume": 3864 - }, - "open": "2020-11-05T22:23:00", - "seconds": 15 - }, - { - "hive": { - "close": 253202, - "high": 253202, - "low": 253202, - "open": 253202, - "volume": 253202 - }, - "id": 2761442, - "non_hive": { - "close": 29969, - "high": 29969, - "low": 29969, - "open": 29969, - "volume": 29969 - }, - "open": "2020-11-05T22:36:30", - "seconds": 15 - }, - { - "hive": { - "close": 382734, - "high": 25347, - "low": 382734, - "open": 25347, - "volume": 408081 - }, - "id": 2761445, - "non_hive": { - "close": 45296, - "high": 3000, - "low": 45296, - "open": 3000, - "volume": 48296 - }, - "open": "2020-11-05T22:40:30", - "seconds": 15 - }, - { - "hive": { - "close": 441631, - "high": 441631, - "low": 441631, - "open": 441631, - "volume": 441631 - }, - "id": 2761448, - "non_hive": { - "close": 52266, - "high": 52266, - "low": 52266, - "open": 52266, - "volume": 52266 - }, - "open": "2020-11-05T22:45:30", - "seconds": 15 - }, - { - "hive": { - "close": 20867, - "high": 20867, - "low": 20867, - "open": 20867, - "volume": 20867 - }, - "id": 2761451, - "non_hive": { - "close": 2470, - "high": 2470, - "low": 2470, - "open": 2470, - "volume": 2470 - }, - "open": "2020-11-05T22:54:30", - "seconds": 15 - }, - { - "hive": { - "close": 4004, - "high": 4004, - "low": 4004, - "open": 4004, - "volume": 4004 - }, - "id": 2761454, - "non_hive": { - "close": 474, - "high": 474, - "low": 474, - "open": 474, - "volume": 474 - }, - "open": "2020-11-05T23:02:15", - "seconds": 15 - }, - { - "hive": { - "close": 149865, - "high": 149865, - "low": 217002, - "open": 217002, - "volume": 1043809 - }, - "id": 2761458, - "non_hive": { - "close": 17804, - "high": 17804, - "low": 25685, - "open": 25685, - "volume": 123639 - }, - "open": "2020-11-05T23:03:00", - "seconds": 15 - }, - { - "hive": { - "close": 336, - "high": 336, - "low": 336, - "open": 336, - "volume": 336 - }, - "id": 2761460, - "non_hive": { - "close": 40, - "high": 40, - "low": 40, - "open": 40, - "volume": 40 - }, - "open": "2020-11-05T23:06:00", - "seconds": 15 - }, - { - "hive": { - "close": 64225, - "high": 1979, - "low": 64225, - "open": 1979, - "volume": 83102 - }, - "id": 2761463, - "non_hive": { - "close": 7601, - "high": 235, - "low": 7601, - "open": 235, - "volume": 9836 - }, - "open": "2020-11-05T23:32:45", - "seconds": 15 - }, - { - "hive": { - "close": 499819, - "high": 175181, - "low": 499819, - "open": 175181, - "volume": 675000 - }, - "id": 2761466, - "non_hive": { - "close": 58728, - "high": 20584, - "low": 58728, - "open": 20584, - "volume": 79312 - }, - "open": "2020-11-05T23:33:15", - "seconds": 15 - }, - { - "hive": { - "close": 17020, - "high": 17020, - "low": 17020, - "open": 17020, - "volume": 17020 - }, - "id": 2761468, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T23:34:00", - "seconds": 15 - }, - { - "hive": { - "close": 17020, - "high": 17020, - "low": 17020, - "open": 17020, - "volume": 17020 - }, - "id": 2761470, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T23:34:15", - "seconds": 15 - }, - { - "hive": { - "close": 17020, - "high": 17020, - "low": 17020, - "open": 17020, - "volume": 17020 - }, - "id": 2761471, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T23:34:45", - "seconds": 15 - }, - { - "hive": { - "close": 17020, - "high": 17020, - "low": 17020, - "open": 17020, - "volume": 17020 - }, - "id": 2761472, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T23:35:30", - "seconds": 15 - }, - { - "hive": { - "close": 17020, - "high": 17020, - "low": 17020, - "open": 17020, - "volume": 17020 - }, - "id": 2761475, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T23:36:00", - "seconds": 15 - }, - { - "hive": { - "close": 16992, - "high": 16992, - "low": 16992, - "open": 16992, - "volume": 16992 - }, - "id": 2761477, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T23:36:30", - "seconds": 15 - }, - { - "hive": { - "close": 16992, - "high": 16992, - "low": 16992, - "open": 16992, - "volume": 16992 - }, - "id": 2761478, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T23:37:00", - "seconds": 15 - }, - { - "hive": { - "close": 15102, - "high": 2492, - "low": 15102, - "open": 2492, - "volume": 27288 - }, - "id": 2761480, - "non_hive": { - "close": 1767, - "high": 293, - "low": 1767, - "open": 293, - "volume": 3199 - }, - "open": "2020-11-05T23:41:45", - "seconds": 15 - }, - { - "hive": { - "close": 245, - "high": 245, - "low": 72712, - "open": 72712, - "volume": 72957 - }, - "id": 2761483, - "non_hive": { - "close": 29, - "high": 29, - "low": 8507, - "open": 8507, - "volume": 8536 - }, - "open": "2020-11-05T23:42:30", - "seconds": 15 - }, - { - "hive": { - "close": 14007, - "high": 14007, - "low": 14007, - "open": 14007, - "volume": 14007 - }, - "id": 2761485, - "non_hive": { - "close": 1656, - "high": 1656, - "low": 1656, - "open": 1656, - "volume": 1656 - }, - "open": "2020-11-05T23:45:15", - "seconds": 15 - }, - { - "hive": { - "close": 7686, - "high": 14141, - "low": 7686, - "open": 14141, - "volume": 21827 - }, - "id": 2761488, - "non_hive": { - "close": 900, - "high": 1656, - "low": 900, - "open": 1656, - "volume": 2556 - }, - "open": "2020-11-05T23:45:45", - "seconds": 15 - }, - { - "hive": { - "close": 7791, - "high": 7791, - "low": 2902, - "open": 2902, - "volume": 10693 - }, - "id": 2761489, - "non_hive": { - "close": 921, - "high": 921, - "low": 343, - "open": 343, - "volume": 1264 - }, - "open": "2020-11-05T23:47:30", - "seconds": 15 - }, - { - "hive": { - "close": 27076, - "high": 27076, - "low": 27076, - "open": 27076, - "volume": 27076 - }, - "id": 2761491, - "non_hive": { - "close": 3201, - "high": 3201, - "low": 3201, - "open": 3201, - "volume": 3201 - }, - "open": "2020-11-05T23:47:45", - "seconds": 15 - }, - { - "hive": { - "close": 6829, - "high": 2580, - "low": 6829, - "open": 2580, - "volume": 12580 - }, - "id": 2761492, - "non_hive": { - "close": 800, - "high": 305, - "low": 800, - "open": 305, - "volume": 1477 - }, - "open": "2020-11-05T23:52:45", - "seconds": 15 - }, - { - "hive": { - "close": 960262, - "high": 964, - "low": 960262, - "open": 964, - "volume": 961226 - }, - "id": 2761495, - "non_hive": { - "close": 112351, - "high": 113, - "low": 112351, - "open": 113, - "volume": 112464 - }, - "open": "2020-11-05T23:53:45", - "seconds": 15 - }, - { - "hive": { - "close": 15597, - "high": 15597, - "low": 15597, - "open": 15597, - "volume": 15597 - }, - "id": 2761497, - "non_hive": { - "close": 1844, - "high": 1844, - "low": 1844, - "open": 1844, - "volume": 1844 - }, - "open": "2020-11-05T23:55:00", - "seconds": 15 - }, - { - "hive": { - "close": 507, - "high": 507, - "low": 507, - "open": 507, - "volume": 507 - }, - "id": 2761500, - "non_hive": { - "close": 60, - "high": 60, - "low": 60, - "open": 60, - "volume": 60 - }, - "open": "2020-11-05T23:56:45", - "seconds": 15 - }, - { - "hive": { - "close": 1129101, - "high": 16271, - "low": 1129101, - "open": 16271, - "volume": 2000000 - }, - "id": 2761502, - "non_hive": { - "close": 132105, - "high": 1904, - "low": 132105, - "open": 1904, - "volume": 234009 - }, - "open": "2020-11-06T00:02:00", - "seconds": 15 - }, - { - "hive": { - "close": 10953, - "high": 10953, - "low": 10953, - "open": 10953, - "volume": 10953 - }, - "id": 2761507, - "non_hive": { - "close": 1295, - "high": 1295, - "low": 1295, - "open": 1295, - "volume": 1295 - }, - "open": "2020-11-06T00:04:15", - "seconds": 15 - }, - { - "hive": { - "close": 1394556, - "high": 11067, - "low": 1394556, - "open": 11067, - "volume": 1405623 - }, - "id": 2761509, - "non_hive": { - "close": 163164, - "high": 1295, - "low": 163164, - "open": 1295, - "volume": 164459 - }, - "open": "2020-11-06T00:05:15", - "seconds": 15 - }, - { - "hive": { - "close": 1500000, - "high": 1500000, - "low": 1500000, - "open": 1500000, - "volume": 1500000 - }, - "id": 2761512, - "non_hive": { - "close": 175501, - "high": 175501, - "low": 175501, - "open": 175501, - "volume": 175501 - }, - "open": "2020-11-06T00:05:45", - "seconds": 15 - }, - { - "hive": { - "close": 110000, - "high": 110000, - "low": 110000, - "open": 110000, - "volume": 110000 - }, - "id": 2761513, - "non_hive": { - "close": 12870, - "high": 12870, - "low": 12870, - "open": 12870, - "volume": 12870 - }, - "open": "2020-11-06T00:09:30", - "seconds": 15 - }, - { - "hive": { - "close": 11481, - "high": 11481, - "low": 11481, - "open": 11481, - "volume": 11481 - }, - "id": 2761515, - "non_hive": { - "close": 1353, - "high": 1353, - "low": 1353, - "open": 1353, - "volume": 1353 - }, - "open": "2020-11-06T00:15:00", - "seconds": 15 - }, - { - "hive": { - "close": 2994, - "high": 2994, - "low": 5483, - "open": 5483, - "volume": 8477 - }, - "id": 2761518, - "non_hive": { - "close": 353, - "high": 353, - "low": 646, - "open": 646, - "volume": 999 - }, - "open": "2020-11-06T00:16:30", - "seconds": 15 - }, - { - "hive": { - "close": 267642, - "high": 76, - "low": 267642, - "open": 17084, - "volume": 284802 - }, - "id": 2761520, - "non_hive": { - "close": 31314, - "high": 9, - "low": 31314, - "open": 1999, - "volume": 33322 - }, - "open": "2020-11-06T00:17:00", - "seconds": 15 - }, - { - "hive": { - "close": 3767, - "high": 3767, - "low": 3767, - "open": 3767, - "volume": 3767 - }, - "id": 2761522, - "non_hive": { - "close": 444, - "high": 444, - "low": 444, - "open": 444, - "volume": 444 - }, - "open": "2020-11-06T00:20:15", - "seconds": 15 - }, - { - "hive": { - "close": 132527, - "high": 132527, - "low": 17021, - "open": 17021, - "volume": 149548 - }, - "id": 2761525, - "non_hive": { - "close": 15572, - "high": 15572, - "low": 1999, - "open": 1999, - "volume": 17571 - }, - "open": "2020-11-06T00:28:00", - "seconds": 15 - }, - { - "hive": { - "close": 323953, - "high": 3015, - "low": 323953, - "open": 3015, - "volume": 344060 - }, - "id": 2761528, - "non_hive": { - "close": 37906, - "high": 353, - "low": 37906, - "open": 353, - "volume": 40259 - }, - "open": "2020-11-06T00:40:30", - "seconds": 15 - }, - { - "hive": { - "close": 124826, - "high": 3785, - "low": 124826, - "open": 3785, - "volume": 128611 - }, - "id": 2761531, - "non_hive": { - "close": 14604, - "high": 443, - "low": 14604, - "open": 443, - "volume": 15047 - }, - "open": "2020-11-06T00:41:00", - "seconds": 15 - }, - { - "hive": { - "close": 17022, - "high": 17022, - "low": 17022, - "open": 17022, - "volume": 17022 - }, - "id": 2761533, - "non_hive": { - "close": 1991, - "high": 1991, - "low": 1991, - "open": 1991, - "volume": 1991 - }, - "open": "2020-11-06T00:50:30", - "seconds": 15 - }, - { - "hive": { - "close": 33000, - "high": 33000, - "low": 33000, - "open": 33000, - "volume": 33000 - }, - "id": 2761536, - "non_hive": { - "close": 3861, - "high": 3861, - "low": 3861, - "open": 3861, - "volume": 3861 - }, - "open": "2020-11-06T00:55:45", - "seconds": 15 - }, - { - "hive": { - "close": 1584, - "high": 1584, - "low": 1584, - "open": 1584, - "volume": 1584 - }, - "id": 2761539, - "non_hive": { - "close": 186, - "high": 186, - "low": 186, - "open": 186, - "volume": 186 - }, - "open": "2020-11-06T01:09:00", - "seconds": 15 - }, - { - "hive": { - "close": 16891, - "high": 16891, - "low": 16891, - "open": 16891, - "volume": 16891 - }, - "id": 2761543, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-06T01:13:45", - "seconds": 15 - }, - { - "hive": { - "close": 8227, - "high": 8227, - "low": 5971, - "open": 5971, - "volume": 14198 - }, - "id": 2761546, - "non_hive": { - "close": 977, - "high": 977, - "low": 707, - "open": 707, - "volume": 1684 - }, - "open": "2020-11-06T01:14:30", - "seconds": 15 - }, - { - "hive": { - "close": 1044, - "high": 1044, - "low": 1044, - "open": 1044, - "volume": 1044 - }, - "id": 2761548, - "non_hive": { - "close": 124, - "high": 124, - "low": 124, - "open": 124, - "volume": 124 - }, - "open": "2020-11-06T01:25:30", - "seconds": 15 - }, - { - "hive": { - "close": 16837, - "high": 16837, - "low": 16837, - "open": 16837, - "volume": 16837 - }, - "id": 2761551, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-06T01:28:30", - "seconds": 15 - }, - { - "hive": { - "close": 918, - "high": 918, - "low": 918, - "open": 918, - "volume": 918 - }, - "id": 2761553, - "non_hive": { - "close": 109, - "high": 109, - "low": 109, - "open": 109, - "volume": 109 - }, - "open": "2020-11-06T01:28:45", - "seconds": 15 - }, - { - "hive": { - "close": 7657, - "high": 7657, - "low": 7657, - "open": 7657, - "volume": 7657 - }, - "id": 2761554, - "non_hive": { - "close": 909, - "high": 909, - "low": 909, - "open": 909, - "volume": 909 - }, - "open": "2020-11-06T01:37:30", - "seconds": 15 - }, - { - "hive": { - "close": 8415, - "high": 8415, - "low": 8415, - "open": 8415, - "volume": 8415 - }, - "id": 2761557, - "non_hive": { - "close": 999, - "high": 999, - "low": 999, - "open": 999, - "volume": 999 - }, - "open": "2020-11-06T01:38:45", - "seconds": 15 - }, - { - "hive": { - "close": 16806, - "high": 16806, - "low": 16806, - "open": 16806, - "volume": 16806 - }, - "id": 2761559, - "non_hive": { - "close": 1995, - "high": 1995, - "low": 1995, - "open": 1995, - "volume": 1995 - }, - "open": "2020-11-06T01:40:45", - "seconds": 15 - }, - { - "hive": { - "close": 1382746, - "high": 17010, - "low": 1382746, - "open": 17010, - "volume": 1427743 - }, - "id": 2761562, - "non_hive": { - "close": 161782, - "high": 2000, - "low": 161782, - "open": 2000, - "volume": 167070 - }, - "open": "2020-11-06T01:44:00", - "seconds": 15 - }, - { - "hive": { - "close": 4698, - "high": 4698, - "low": 4698, - "open": 4698, - "volume": 4698 - }, - "id": 2761564, - "non_hive": { - "close": 556, - "high": 556, - "low": 556, - "open": 556, - "volume": 556 - }, - "open": "2020-11-06T01:50:45", - "seconds": 15 - }, - { - "hive": { - "close": 665313, - "high": 665313, - "low": 12193, - "open": 12193, - "volume": 858763 - }, - "id": 2761567, - "non_hive": { - "close": 79030, - "high": 79030, - "low": 1443, - "open": 1443, - "volume": 102001 - }, - "open": "2020-11-06T02:01:30", - "seconds": 15 - }, - { - "hive": { - "close": 150994, - "high": 150994, - "low": 150994, - "open": 150994, - "volume": 150994 - }, - "id": 2761571, - "non_hive": { - "close": 17936, - "high": 17936, - "low": 17936, - "open": 17936, - "volume": 17936 - }, - "open": "2020-11-06T02:01:45", - "seconds": 15 - }, - { - "hive": { - "close": 2188343, - "high": 2188343, - "low": 435231, - "open": 435231, - "volume": 2623574 - }, - "id": 2761572, - "non_hive": { - "close": 259975, - "high": 259975, - "low": 51700, - "open": 51700, - "volume": 311675 - }, - "open": "2020-11-06T02:10:15", - "seconds": 15 - }, - { - "hive": { - "close": 2421718, - "high": 2421718, - "low": 2421718, - "open": 2421718, - "volume": 2421718 - }, - "id": 2761575, - "non_hive": { - "close": 287700, - "high": 287700, - "low": 287700, - "open": 287700, - "volume": 287700 - }, - "open": "2020-11-06T02:12:15", - "seconds": 15 - }, - { - "hive": { - "close": 819546, - "high": 819546, - "low": 1992688, - "open": 1992688, - "volume": 2812234 - }, - "id": 2761577, - "non_hive": { - "close": 97444, - "high": 97444, - "low": 236731, - "open": 236731, - "volume": 334175 - }, - "open": "2020-11-06T02:15:30", - "seconds": 15 - }, - { - "hive": { - "close": 1054483, - "high": 1054483, - "low": 1054483, - "open": 1054483, - "volume": 1054483 - }, - "id": 2761580, - "non_hive": { - "close": 125378, - "high": 125378, - "low": 125378, - "open": 125378, - "volume": 125378 - }, - "open": "2020-11-06T02:18:30", - "seconds": 15 - }, - { - "hive": { - "close": 1614096, - "high": 1614096, - "low": 1614096, - "open": 1614096, - "volume": 1614096 - }, - "id": 2761582, - "non_hive": { - "close": 191916, - "high": 191916, - "low": 191916, - "open": 191916, - "volume": 191916 - }, - "open": "2020-11-06T02:24:00", - "seconds": 15 - }, - { - "hive": { - "close": 1202431, - "high": 1202431, - "low": 1202431, - "open": 1202431, - "volume": 1202431 - }, - "id": 2761585, - "non_hive": { - "close": 142969, - "high": 142969, - "low": 142969, - "open": 142969, - "volume": 142969 - }, - "open": "2020-11-06T02:26:30", - "seconds": 15 - }, - { - "hive": { - "close": 11278, - "high": 11278, - "low": 11278, - "open": 11278, - "volume": 11278 - }, - "id": 2761588, - "non_hive": { - "close": 1341, - "high": 1341, - "low": 1341, - "open": 1341, - "volume": 1341 - }, - "open": "2020-11-06T02:32:15", - "seconds": 15 - }, - { - "hive": { - "close": 20227, - "high": 20227, - "low": 20227, - "open": 20227, - "volume": 20227 - }, - "id": 2761591, - "non_hive": { - "close": 2405, - "high": 2405, - "low": 2405, - "open": 2405, - "volume": 2405 - }, - "open": "2020-11-06T02:35:45", - "seconds": 15 - }, - { - "hive": { - "close": 3411, - "high": 40875, - "low": 3411, - "open": 40875, - "volume": 44286 - }, - "id": 2761594, - "non_hive": { - "close": 405, - "high": 4860, - "low": 405, - "open": 4860, - "volume": 5265 - }, - "open": "2020-11-06T02:40:15", - "seconds": 15 - }, - { - "hive": { - "close": 1055208, - "high": 1055208, - "low": 16821, - "open": 16821, - "volume": 3173848 - }, - "id": 2761597, - "non_hive": { - "close": 125939, - "high": 125939, - "low": 1999, - "open": 1999, - "volume": 377950 - }, - "open": "2020-11-06T02:46:15", - "seconds": 15 - }, - { - "hive": { - "close": 1191, - "high": 1191, - "low": 1191, - "open": 1191, - "volume": 1191 - }, - "id": 2761600, - "non_hive": { - "close": 142, - "high": 142, - "low": 142, - "open": 142, - "volume": 142 - }, - "open": "2020-11-06T03:16:15", - "seconds": 15 - }, - { - "hive": { - "close": 10000, - "high": 10000, - "low": 10000, - "open": 10000, - "volume": 10000 - }, - "id": 2761604, - "non_hive": { - "close": 1180, - "high": 1180, - "low": 1180, - "open": 1180, - "volume": 1180 - }, - "open": "2020-11-06T03:18:00", - "seconds": 15 - }, - { - "hive": { - "close": 6001, - "high": 6001, - "low": 6001, - "open": 6001, - "volume": 6001 - }, - "id": 2761606, - "non_hive": { - "close": 716, - "high": 716, - "low": 716, - "open": 716, - "volume": 716 - }, - "open": "2020-11-06T03:36:45", - "seconds": 15 - }, - { - "hive": { - "close": 265, - "high": 265, - "low": 265, - "open": 265, - "volume": 265 - }, - "id": 2761609, - "non_hive": { - "close": 31, - "high": 31, - "low": 31, - "open": 31, - "volume": 31 - }, - "open": "2020-11-06T03:38:00", - "seconds": 15 - }, - { - "hive": { - "close": 10755, - "high": 10755, - "low": 10755, - "open": 10755, - "volume": 10755 - }, - "id": 2761611, - "non_hive": { - "close": 1283, - "high": 1283, - "low": 1283, - "open": 1283, - "volume": 1283 - }, - "open": "2020-11-06T03:43:30", - "seconds": 15 - }, - { - "hive": { - "close": 2228, - "high": 2228, - "low": 2228, - "open": 2228, - "volume": 2228 - }, - "id": 2761614, - "non_hive": { - "close": 266, - "high": 266, - "low": 266, - "open": 266, - "volume": 266 - }, - "open": "2020-11-06T04:29:45", - "seconds": 15 - }, - { - "hive": { - "close": 9994, - "high": 9994, - "low": 9994, - "open": 9994, - "volume": 9994 - }, - "id": 2761618, - "non_hive": { - "close": 1192, - "high": 1192, - "low": 1192, - "open": 1192, - "volume": 1192 - }, - "open": "2020-11-06T04:43:45", - "seconds": 15 - }, - { - "hive": { - "close": 88024, - "high": 88024, - "low": 3645, - "open": 3645, - "volume": 91669 - }, - "id": 2761621, - "non_hive": { - "close": 10554, - "high": 10554, - "low": 435, - "open": 435, - "volume": 10989 - }, - "open": "2020-11-06T04:46:30", - "seconds": 15 - }, - { - "hive": { - "close": 2645, - "high": 2645, - "low": 2645, - "open": 2645, - "volume": 2645 - }, - "id": 2761624, - "non_hive": { - "close": 317, - "high": 317, - "low": 317, - "open": 317, - "volume": 317 - }, - "open": "2020-11-06T04:47:30", - "seconds": 15 - }, - { - "hive": { - "close": 14036, - "high": 14036, - "low": 14036, - "open": 14036, - "volume": 14036 - }, - "id": 2761626, - "non_hive": { - "close": 1682, - "high": 1682, - "low": 1682, - "open": 1682, - "volume": 1682 - }, - "open": "2020-11-06T04:51:45", - "seconds": 15 - }, - { - "hive": { - "close": 1612, - "high": 1612, - "low": 1612, - "open": 1612, - "volume": 1612 - }, - "id": 2761629, - "non_hive": { - "close": 193, - "high": 193, - "low": 193, - "open": 193, - "volume": 193 - }, - "open": "2020-11-06T04:52:30", - "seconds": 15 - }, - { - "hive": { - "close": 425, - "high": 425, - "low": 425, - "open": 425, - "volume": 425 - }, - "id": 2761631, - "non_hive": { - "close": 51, - "high": 51, - "low": 51, - "open": 51, - "volume": 51 - }, - "open": "2020-11-06T04:59:15", - "seconds": 15 - }, - { - "hive": { - "close": 8179, - "high": 8179, - "low": 8179, - "open": 8179, - "volume": 8179 - }, - "id": 2761634, - "non_hive": { - "close": 980, - "high": 980, - "low": 980, - "open": 980, - "volume": 980 - }, - "open": "2020-11-06T05:02:00", - "seconds": 15 - }, - { - "hive": { - "close": 44894, - "high": 44894, - "low": 44894, - "open": 44894, - "volume": 44894 - }, - "id": 2761638, - "non_hive": { - "close": 5380, - "high": 5380, - "low": 5380, - "open": 5380, - "volume": 5380 - }, - "open": "2020-11-06T05:07:45", - "seconds": 15 - }, - { - "hive": { - "close": 83237, - "high": 16763, - "low": 83237, - "open": 16763, - "volume": 100000 - }, - "id": 2761641, - "non_hive": { - "close": 9930, - "high": 2000, - "low": 9930, - "open": 2000, - "volume": 11930 - }, - "open": "2020-11-06T05:10:15", - "seconds": 15 - }, - { - "hive": { - "close": 16763, - "high": 16763, - "low": 16763, - "open": 16763, - "volume": 16763 - }, - "id": 2761644, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-06T05:15:00", - "seconds": 15 - }, - { - "hive": { - "close": 64651, - "high": 64651, - "low": 64651, - "open": 64651, - "volume": 64651 - }, - "id": 2761647, - "non_hive": { - "close": 7713, - "high": 7713, - "low": 7713, - "open": 7713, - "volume": 7713 - }, - "open": "2020-11-06T05:16:15", - "seconds": 15 - }, - { - "hive": { - "close": 1701, - "high": 16886, - "low": 1701, - "open": 16886, - "volume": 18587 - }, - "id": 2761649, - "non_hive": { - "close": 201, - "high": 2000, - "low": 201, - "open": 2000, - "volume": 2201 - }, - "open": "2020-11-06T05:19:45", - "seconds": 15 - }, - { - "hive": { - "close": 16312, - "high": 16312, - "low": 16312, - "open": 16312, - "volume": 16312 - }, - "id": 2761651, - "non_hive": { - "close": 1945, - "high": 1945, - "low": 1945, - "open": 1945, - "volume": 1945 - }, - "open": "2020-11-06T05:46:15", - "seconds": 15 - }, - { - "hive": { - "close": 4221, - "high": 4221, - "low": 16886, - "open": 16886, - "volume": 21107 - }, - "id": 2761654, - "non_hive": { - "close": 500, - "high": 500, - "low": 2000, - "open": 2000, - "volume": 2500 - }, - "open": "2020-11-06T05:50:15", - "seconds": 15 - }, - { - "hive": { - "close": 1802, - "high": 1802, - "low": 1802, - "open": 1802, - "volume": 1802 - }, - "id": 2761657, - "non_hive": { - "close": 213, - "high": 213, - "low": 213, - "open": 213, - "volume": 213 - }, - "open": "2020-11-06T06:06:30", - "seconds": 15 - }, - { - "hive": { - "close": 158993, - "high": 158993, - "low": 3765, - "open": 3765, - "volume": 956681 - }, - "id": 2761661, - "non_hive": { - "close": 18952, - "high": 18952, - "low": 445, - "open": 445, - "volume": 113998 - }, - "open": "2020-11-06T06:06:45", - "seconds": 15 - }, - { - "hive": { - "close": 11255, - "high": 11255, - "low": 11255, - "open": 11255, - "volume": 11255 - }, - "id": 2761662, - "non_hive": { - "close": 1341, - "high": 1341, - "low": 1341, - "open": 1341, - "volume": 1341 - }, - "open": "2020-11-06T06:21:00", - "seconds": 15 - }, - { - "hive": { - "close": 5524, - "high": 5524, - "low": 5524, - "open": 5524, - "volume": 5524 - }, - "id": 2761665, - "non_hive": { - "close": 658, - "high": 658, - "low": 658, - "open": 658, - "volume": 658 - }, - "open": "2020-11-06T06:24:00", - "seconds": 15 - }, - { - "hive": { - "close": 10201, - "high": 10201, - "low": 10201, - "open": 10201, - "volume": 10201 - }, - "id": 2761667, - "non_hive": { - "close": 1216, - "high": 1216, - "low": 1216, - "open": 1216, - "volume": 1216 - }, - "open": "2020-11-06T06:24:30", - "seconds": 15 - }, - { - "hive": { - "close": 5151, - "high": 5151, - "low": 5151, - "open": 5151, - "volume": 5151 - }, - "id": 2761668, - "non_hive": { - "close": 614, - "high": 614, - "low": 614, - "open": 614, - "volume": 614 - }, - "open": "2020-11-06T06:49:15", - "seconds": 15 - }, - { - "hive": { - "close": 208994, - "high": 208994, - "low": 208994, - "open": 208994, - "volume": 208994 - }, - "id": 2761671, - "non_hive": { - "close": 24912, - "high": 24912, - "low": 24912, - "open": 24912, - "volume": 24912 - }, - "open": "2020-11-06T06:49:30", - "seconds": 15 - }, - { - "hive": { - "close": 208994, - "high": 208994, - "low": 208994, - "open": 208994, - "volume": 208994 - }, - "id": 2761672, - "non_hive": { - "close": 24912, - "high": 24912, - "low": 24912, - "open": 24912, - "volume": 24912 - }, - "open": "2020-11-06T06:54:30", - "seconds": 15 - }, - { - "hive": { - "close": 402, - "high": 2047, - "low": 402, - "open": 2047, - "volume": 2449 - }, - "id": 2761675, - "non_hive": { - "close": 47, - "high": 244, - "low": 47, - "open": 244, - "volume": 291 - }, - "open": "2020-11-06T06:54:45", - "seconds": 15 - }, - { - "hive": { - "close": 150998, - "high": 150998, - "low": 150998, - "open": 150998, - "volume": 150998 - }, - "id": 2761676, - "non_hive": { - "close": 17999, - "high": 17999, - "low": 17999, - "open": 17999, - "volume": 17999 - }, - "open": "2020-11-06T06:56:00", - "seconds": 15 - }, - { - "hive": { - "close": 4874, - "high": 4874, - "low": 4874, - "open": 4874, - "volume": 4874 - }, - "id": 2761679, - "non_hive": { - "close": 581, - "high": 581, - "low": 581, - "open": 581, - "volume": 581 - }, - "open": "2020-11-06T06:56:45", - "seconds": 15 - }, - { - "hive": { - "close": 13775, - "high": 13775, - "low": 13775, - "open": 13775, - "volume": 13775 - }, - "id": 2761680, - "non_hive": { - "close": 1642, - "high": 1642, - "low": 1642, - "open": 1642, - "volume": 1642 - }, - "open": "2020-11-06T06:59:30", - "seconds": 15 - }, - { - "hive": { - "close": 980, - "high": 980, - "low": 980, - "open": 980, - "volume": 980 - }, - "id": 2761682, - "non_hive": { - "close": 116, - "high": 116, - "low": 116, - "open": 116, - "volume": 116 - }, - "open": "2020-11-06T06:59:45", - "seconds": 15 - }, - { - "hive": { - "close": 91996, - "high": 91996, - "low": 91996, - "open": 91996, - "volume": 91996 - }, - "id": 2761683, - "non_hive": { - "close": 10966, - "high": 10966, - "low": 10966, - "open": 10966, - "volume": 10966 - }, - "open": "2020-11-06T07:00:00", - "seconds": 15 - }, - { - "hive": { - "close": 1459, - "high": 1459, - "low": 1459, - "open": 1459, - "volume": 1459 - }, - "id": 2761687, - "non_hive": { - "close": 174, - "high": 174, - "low": 174, - "open": 174, - "volume": 174 - }, - "open": "2020-11-06T07:01:30", - "seconds": 15 - }, - { - "hive": { - "close": 2626, - "high": 2357, - "low": 2249, - "open": 2357, - "volume": 7232 - }, - "id": 2761689, - "non_hive": { - "close": 313, - "high": 281, - "low": 268, - "open": 281, - "volume": 862 - }, - "open": "2020-11-06T07:05:00", - "seconds": 15 - }, - { - "hive": { - "close": 16779, - "high": 16779, - "low": 16779, - "open": 16779, - "volume": 16779 - }, - "id": 2761692, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-06T07:08:15", - "seconds": 15 - }, - { - "hive": { - "close": 84297, - "high": 84297, - "low": 84297, - "open": 84297, - "volume": 84297 - }, - "id": 2761694, - "non_hive": { - "close": 10043, - "high": 10043, - "low": 10043, - "open": 10043, - "volume": 10043 - }, - "open": "2020-11-06T07:09:00", - "seconds": 15 - }, - { - "hive": { - "close": 38, - "high": 38, - "low": 38, - "open": 38, - "volume": 38 - }, - "id": 2761696, - "non_hive": { - "close": 4, - "high": 4, - "low": 4, - "open": 4, - "volume": 4 - }, - "open": "2020-11-06T07:09:45", - "seconds": 15 - }, - { - "hive": { - "close": 16807, - "high": 16807, - "low": 16807, - "open": 16807, - "volume": 16807 - }, - "id": 2761697, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-06T07:11:15", - "seconds": 15 - }, - { - "hive": { - "close": 16807, - "high": 16807, - "low": 16807, - "open": 16807, - "volume": 16807 - }, - "id": 2761700, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-06T07:11:30", - "seconds": 15 - }, - { - "hive": { - "close": 14493, - "high": 14493, - "low": 14493, - "open": 14493, - "volume": 14493 - }, - "id": 2761701, - "non_hive": { - "close": 1724, - "high": 1724, - "low": 1724, - "open": 1724, - "volume": 1724 - }, - "open": "2020-11-06T07:12:15", - "seconds": 15 - }, - { - "hive": { - "close": 5926, - "high": 5926, - "low": 5926, - "open": 5926, - "volume": 5926 - }, - "id": 2761703, - "non_hive": { - "close": 705, - "high": 705, - "low": 705, - "open": 705, - "volume": 705 - }, - "open": "2020-11-06T07:18:00", - "seconds": 15 - }, - { - "hive": { - "close": 18464, - "high": 18464, - "low": 18464, - "open": 18464, - "volume": 18464 - }, - "id": 2761706, - "non_hive": { - "close": 2201, - "high": 2201, - "low": 2201, - "open": 2201, - "volume": 2201 - }, - "open": "2020-11-06T07:41:45", - "seconds": 15 - }, - { - "hive": { - "close": 1704, - "high": 1704, - "low": 1704, - "open": 1704, - "volume": 1704 - }, - "id": 2761709, - "non_hive": { - "close": 203, - "high": 203, - "low": 203, - "open": 203, - "volume": 203 - }, - "open": "2020-11-06T07:42:15", - "seconds": 15 - }, - { - "hive": { - "close": 1166, - "high": 1166, - "low": 1166, - "open": 1166, - "volume": 1166 - }, - "id": 2761711, - "non_hive": { - "close": 139, - "high": 139, - "low": 139, - "open": 139, - "volume": 139 - }, - "open": "2020-11-06T07:54:15", - "seconds": 15 - }, - { - "hive": { - "close": 463201, - "high": 463201, - "low": 463201, - "open": 463201, - "volume": 463201 - }, - "id": 2761714, - "non_hive": { - "close": 55181, - "high": 55181, - "low": 55181, - "open": 55181, - "volume": 55181 - }, - "open": "2020-11-06T07:58:00", - "seconds": 15 - }, - { - "hive": { - "close": 1107, - "high": 1107, - "low": 1107, - "open": 1107, - "volume": 1107 - }, - "id": 2761717, - "non_hive": { - "close": 132, - "high": 132, - "low": 132, - "open": 132, - "volume": 132 - }, - "open": "2020-11-06T08:06:45", - "seconds": 15 - }, - { - "hive": { - "close": 1000000, - "high": 1000000, - "low": 1000000, - "open": 1000000, - "volume": 1000000 - }, - "id": 2761721, - "non_hive": { - "close": 119130, - "high": 119130, - "low": 119130, - "open": 119130, - "volume": 119130 - }, - "open": "2020-11-06T08:09:30", - "seconds": 15 - }, - { - "hive": { - "close": 4289, - "high": 4289, - "low": 4289, - "open": 4289, - "volume": 4289 - }, - "id": 2761723, - "non_hive": { - "close": 511, - "high": 511, - "low": 511, - "open": 511, - "volume": 511 - }, - "open": "2020-11-06T08:19:30", - "seconds": 15 - }, - { - "hive": { - "close": 8271, - "high": 8271, - "low": 8271, - "open": 8271, - "volume": 8271 - }, - "id": 2761726, - "non_hive": { - "close": 986, - "high": 986, - "low": 986, - "open": 986, - "volume": 986 - }, - "open": "2020-11-06T08:20:00", - "seconds": 15 - }, - { - "hive": { - "close": 33559, - "high": 11485, - "low": 33559, - "open": 11485, - "volume": 47872 - }, - "id": 2761729, - "non_hive": { - "close": 3997, - "high": 1369, - "low": 3997, - "open": 1369, - "volume": 5703 - }, - "open": "2020-11-06T08:21:15", - "seconds": 15 - }, - { - "hive": { - "close": 31061, - "high": 31061, - "low": 31061, - "open": 31061, - "volume": 31061 - }, - "id": 2761731, - "non_hive": { - "close": 3700, - "high": 3700, - "low": 3700, - "open": 3700, - "volume": 3700 - }, - "open": "2020-11-06T08:23:45", - "seconds": 15 - }, - { - "hive": { - "close": 8624, - "high": 8624, - "low": 8624, - "open": 8624, - "volume": 8624 - }, - "id": 2761733, - "non_hive": { - "close": 1028, - "high": 1028, - "low": 1028, - "open": 1028, - "volume": 1028 - }, - "open": "2020-11-06T08:28:45", - "seconds": 15 - }, - { - "hive": { - "close": 4437, - "high": 4437, - "low": 4437, - "open": 4437, - "volume": 4437 - }, - "id": 2761736, - "non_hive": { - "close": 529, - "high": 529, - "low": 529, - "open": 529, - "volume": 529 - }, - "open": "2020-11-06T08:35:15", - "seconds": 15 - }, - { - "hive": { - "close": 18983, - "high": 11024, - "low": 18983, - "open": 11024, - "volume": 44570 - }, - "id": 2761739, - "non_hive": { - "close": 2239, - "high": 1314, - "low": 2239, - "open": 1314, - "volume": 5288 - }, - "open": "2020-11-06T08:36:45", - "seconds": 15 - }, - { - "hive": { - "close": 62919, - "high": 62919, - "low": 62919, - "open": 62919, - "volume": 62919 - }, - "id": 2761741, - "non_hive": { - "close": 7500, - "high": 7500, - "low": 7500, - "open": 7500, - "volume": 7500 - }, - "open": "2020-11-06T08:40:30", - "seconds": 15 - }, - { - "hive": { - "close": 18983, - "high": 18983, - "low": 18983, - "open": 18983, - "volume": 18983 - }, - "id": 2761744, - "non_hive": { - "close": 2261, - "high": 2261, - "low": 2261, - "open": 2261, - "volume": 2261 - }, - "open": "2020-11-06T08:47:00", - "seconds": 15 - }, - { - "hive": { - "close": 318, - "high": 318, - "low": 318, - "open": 318, - "volume": 318 - }, - "id": 2761747, - "non_hive": { - "close": 38, - "high": 38, - "low": 38, - "open": 38, - "volume": 38 - }, - "open": "2020-11-06T08:51:30", - "seconds": 15 - }, - { - "hive": { - "close": 4974, - "high": 4974, - "low": 4974, - "open": 4974, - "volume": 4974 - }, - "id": 2761750, - "non_hive": { - "close": 593, - "high": 593, - "low": 593, - "open": 593, - "volume": 593 - }, - "open": "2020-11-06T09:05:15", - "seconds": 15 - }, - { - "hive": { - "close": 125839, - "high": 125839, - "low": 125839, - "open": 125839, - "volume": 125839 - }, - "id": 2761754, - "non_hive": { - "close": 15000, - "high": 15000, - "low": 15000, - "open": 15000, - "volume": 15000 - }, - "open": "2020-11-06T09:13:00", - "seconds": 15 - }, - { - "hive": { - "close": 19857, - "high": 19857, - "low": 19857, - "open": 19857, - "volume": 19857 - }, - "id": 2761757, - "non_hive": { - "close": 2367, - "high": 2367, - "low": 2367, - "open": 2367, - "volume": 2367 - }, - "open": "2020-11-06T09:26:30", - "seconds": 15 - }, - { - "hive": { - "close": 4289, - "high": 4289, - "low": 4289, - "open": 4289, - "volume": 4289 - }, - "id": 2761760, - "non_hive": { - "close": 511, - "high": 511, - "low": 511, - "open": 511, - "volume": 511 - }, - "open": "2020-11-06T09:27:00", - "seconds": 15 - }, - { - "hive": { - "close": 2365, - "high": 2365, - "low": 2365, - "open": 2365, - "volume": 2365 - }, - "id": 2761762, - "non_hive": { - "close": 282, - "high": 282, - "low": 282, - "open": 282, - "volume": 282 - }, - "open": "2020-11-06T09:31:15", - "seconds": 15 - }, - { - "hive": { - "close": 2810, - "high": 2810, - "low": 2810, - "open": 2810, - "volume": 2810 - }, - "id": 2761765, - "non_hive": { - "close": 335, - "high": 335, - "low": 335, - "open": 335, - "volume": 335 - }, - "open": "2020-11-06T09:52:45", - "seconds": 15 - }, - { - "hive": { - "close": 7902, - "high": 7902, - "low": 7902, - "open": 7902, - "volume": 7902 - }, - "id": 2761768, - "non_hive": { - "close": 942, - "high": 942, - "low": 942, - "open": 942, - "volume": 942 - }, - "open": "2020-11-06T10:25:00", - "seconds": 15 - }, - { - "hive": { - "close": 4250, - "high": 4250, - "low": 4250, - "open": 4250, - "volume": 4250 - }, - "id": 2761772, - "non_hive": { - "close": 506, - "high": 506, - "low": 506, - "open": 506, - "volume": 506 - }, - "open": "2020-11-06T10:34:15", - "seconds": 15 - }, - { - "hive": { - "close": 64001, - "high": 64001, - "low": 64001, - "open": 64001, - "volume": 64001 - }, - "id": 2761775, - "non_hive": { - "close": 7629, - "high": 7629, - "low": 7629, - "open": 7629, - "volume": 7629 - }, - "open": "2020-11-06T10:47:15", - "seconds": 15 - }, - { - "hive": { - "close": 218, - "high": 218, - "low": 218, - "open": 218, - "volume": 218 - }, - "id": 2761778, - "non_hive": { - "close": 26, - "high": 26, - "low": 26, - "open": 26, - "volume": 26 - }, - "open": "2020-11-06T10:49:15", - "seconds": 15 - }, - { - "hive": { - "close": 485620, - "high": 485620, - "low": 485620, - "open": 485620, - "volume": 485620 - }, - "id": 2761780, - "non_hive": { - "close": 57885, - "high": 57885, - "low": 57885, - "open": 57885, - "volume": 57885 - }, - "open": "2020-11-06T10:57:15", - "seconds": 15 - }, - { - "hive": { - "close": 1000000, - "high": 1000000, - "low": 5881, - "open": 5881, - "volume": 1135074 - }, - "id": 2761783, - "non_hive": { - "close": 119924, - "high": 119924, - "low": 702, - "open": 702, - "volume": 136116 - }, - "open": "2020-11-06T10:58:30", - "seconds": 15 - }, - { - "hive": { - "close": 5862, - "high": 5862, - "low": 5862, - "open": 5862, - "volume": 5862 - }, - "id": 2761785, - "non_hive": { - "close": 703, - "high": 703, - "low": 703, - "open": 703, - "volume": 703 - }, - "open": "2020-11-06T11:08:00", - "seconds": 15 - }, - { - "hive": { - "close": 5792, - "high": 5792, - "low": 5829, - "open": 5829, - "volume": 11621 - }, - "id": 2761789, - "non_hive": { - "close": 700, - "high": 700, - "low": 702, - "open": 702, - "volume": 1402 - }, - "open": "2020-11-06T11:08:30", - "seconds": 15 - }, - { - "hive": { - "close": 821582, - "high": 821582, - "low": 5753, - "open": 5753, - "volume": 893289 - }, - "id": 2761790, - "non_hive": { - "close": 102697, - "high": 102697, - "low": 698, - "open": 698, - "volume": 111571 - }, - "open": "2020-11-06T11:09:15", - "seconds": 15 - } -] diff --git a/hived/tavern/condenser_api_patterns/get_market_history/15.tavern.yaml b/hived/tavern/condenser_api_patterns/get_market_history/15.tavern.yaml deleted file mode 100644 index 49cbf92e5e62bdb8206b1879f20779f3c36d4cf4..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history/15.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_market_history - - marks: - - patterntest # this works only for new data from one day before now - - includes: - - !include ../../common.yaml - - stages: - - name: get_market_history - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_market_history" - params: [15,"2016-04-03T00:00:00","2031-11-01T00:00:00"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "15" - directory: "condenser_api_patterns/get_market_history" diff --git a/hived/tavern/condenser_api_patterns/get_market_history/300.pat.json b/hived/tavern/condenser_api_patterns/get_market_history/300.pat.json deleted file mode 100644 index 7cff6af378aaa855bf849c5aa2560f41dbe203b0..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history/300.pat.json +++ /dev/null @@ -1,55387 +0,0 @@ -[ - { - "hive": { - "close": 181, - "high": 37583, - "low": 181, - "open": 12422, - "volume": 79784 - }, - "id": 2748611, - "non_hive": { - "close": 29, - "high": 6051, - "low": 29, - "open": 1999, - "volume": 12842 - }, - "open": "2020-10-17T11:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1979, - "high": 1979, - "low": 1979, - "open": 1979, - "volume": 1979 - }, - "id": 2748617, - "non_hive": { - "close": 315, - "high": 315, - "low": 315, - "open": 315, - "volume": 315 - }, - "open": "2020-10-17T11:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2134, - "high": 2134, - "low": 458, - "open": 31323, - "volume": 33915 - }, - "id": 2748620, - "non_hive": { - "close": 340, - "high": 340, - "low": 72, - "open": 4990, - "volume": 5402 - }, - "open": "2020-10-17T11:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 22491, - "high": 7894, - "low": 22491, - "open": 4180, - "volume": 234806 - }, - "id": 2748625, - "non_hive": { - "close": 3577, - "high": 1271, - "low": 3577, - "open": 666, - "volume": 37397 - }, - "open": "2020-10-17T11:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 692, - "high": 692, - "low": 692, - "open": 692, - "volume": 692 - }, - "id": 2748631, - "non_hive": { - "close": 110, - "high": 110, - "low": 110, - "open": 110, - "volume": 110 - }, - "open": "2020-10-17T11:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12689, - "high": 12689, - "low": 12689, - "open": 12689, - "volume": 12689 - }, - "id": 2748634, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-17T11:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 4130, - "high": 4130, - "low": 4130, - "open": 4130, - "volume": 4130 - }, - "id": 2748637, - "non_hive": { - "close": 651, - "high": 651, - "low": 651, - "open": 651, - "volume": 651 - }, - "open": "2020-10-17T11:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 3768, - "high": 3768, - "low": 3768, - "open": 3768, - "volume": 3768 - }, - "id": 2748640, - "non_hive": { - "close": 594, - "high": 594, - "low": 594, - "open": 594, - "volume": 594 - }, - "open": "2020-10-17T12:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 82, - "high": 82, - "low": 82, - "open": 82, - "volume": 82 - }, - "id": 2748644, - "non_hive": { - "close": 13, - "high": 13, - "low": 13, - "open": 13, - "volume": 13 - }, - "open": "2020-10-17T12:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 31817, - "high": 9516, - "low": 13629, - "open": 9516, - "volume": 54962 - }, - "id": 2748647, - "non_hive": { - "close": 5015, - "high": 1500, - "low": 2148, - "open": 1500, - "volume": 8663 - }, - "open": "2020-10-17T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 613, - "high": 8727, - "low": 613, - "open": 8727, - "volume": 19800 - }, - "id": 2748652, - "non_hive": { - "close": 96, - "high": 1400, - "low": 96, - "open": 1400, - "volume": 3174 - }, - "open": "2020-10-17T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 70132, - "high": 70132, - "low": 12460, - "open": 12460, - "volume": 242089 - }, - "id": 2748656, - "non_hive": { - "close": 11281, - "high": 11281, - "low": 1999, - "open": 1999, - "volume": 38911 - }, - "open": "2020-10-17T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12104, - "high": 12104, - "low": 7686, - "open": 7686, - "volume": 19790 - }, - "id": 2748659, - "non_hive": { - "close": 1947, - "high": 1947, - "low": 1233, - "open": 1233, - "volume": 3180 - }, - "open": "2020-10-17T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 16036, - "high": 52451, - "low": 6174, - "open": 6155, - "volume": 80816 - }, - "id": 2748665, - "non_hive": { - "close": 2600, - "high": 8509, - "low": 1001, - "open": 998, - "volume": 13108 - }, - "open": "2020-10-17T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 23492, - "high": 23492, - "low": 7410, - "open": 7944, - "volume": 38846 - }, - "id": 2748671, - "non_hive": { - "close": 3811, - "high": 3811, - "low": 1189, - "open": 1275, - "volume": 6275 - }, - "open": "2020-10-17T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 8622, - "high": 8622, - "low": 8622, - "open": 8622, - "volume": 8622 - }, - "id": 2748676, - "non_hive": { - "close": 1398, - "high": 1398, - "low": 1398, - "open": 1398, - "volume": 1398 - }, - "open": "2020-10-17T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 844, - "high": 844, - "low": 3707, - "open": 3707, - "volume": 4551 - }, - "id": 2748679, - "non_hive": { - "close": 137, - "high": 137, - "low": 601, - "open": 601, - "volume": 738 - }, - "open": "2020-10-17T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2044, - "high": 17956, - "low": 2044, - "open": 17956, - "volume": 20000 - }, - "id": 2748684, - "non_hive": { - "close": 328, - "high": 2883, - "low": 328, - "open": 2883, - "volume": 3211 - }, - "open": "2020-10-17T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2242, - "high": 2242, - "low": 2242, - "open": 2242, - "volume": 2242 - }, - "id": 2748687, - "non_hive": { - "close": 360, - "high": 360, - "low": 360, - "open": 360, - "volume": 360 - }, - "open": "2020-10-17T15:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 10020, - "high": 10020, - "low": 10020, - "open": 10020, - "volume": 10020 - }, - "id": 2748690, - "non_hive": { - "close": 1609, - "high": 1609, - "low": 1609, - "open": 1609, - "volume": 1609 - }, - "open": "2020-10-17T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 18271, - "high": 18271, - "low": 18271, - "open": 18271, - "volume": 18271 - }, - "id": 2748693, - "non_hive": { - "close": 2933, - "high": 2933, - "low": 2933, - "open": 2933, - "volume": 2933 - }, - "open": "2020-10-17T15:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1325, - "high": 1325, - "low": 1325, - "open": 1325, - "volume": 1325 - }, - "id": 2748696, - "non_hive": { - "close": 214, - "high": 214, - "low": 214, - "open": 214, - "volume": 214 - }, - "open": "2020-10-17T15:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 8944, - "high": 8944, - "low": 8944, - "open": 8944, - "volume": 8944 - }, - "id": 2748699, - "non_hive": { - "close": 1450, - "high": 1450, - "low": 1450, - "open": 1450, - "volume": 1450 - }, - "open": "2020-10-17T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 67, - "high": 67, - "low": 6111, - "open": 2810, - "volume": 8988 - }, - "id": 2748702, - "non_hive": { - "close": 11, - "high": 11, - "low": 989, - "open": 455, - "volume": 1455 - }, - "open": "2020-10-17T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2426, - "high": 12030, - "low": 18760, - "open": 303, - "volume": 154037 - }, - "id": 2748708, - "non_hive": { - "close": 393, - "high": 1949, - "low": 3031, - "open": 49, - "volume": 24941 - }, - "open": "2020-10-17T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 24911, - "high": 1235, - "low": 24911, - "open": 1235, - "volume": 32621 - }, - "id": 2748716, - "non_hive": { - "close": 3999, - "high": 200, - "low": 3999, - "open": 200, - "volume": 5245 - }, - "open": "2020-10-17T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 18932, - "high": 12455, - "low": 18932, - "open": 12455, - "volume": 31387 - }, - "id": 2748721, - "non_hive": { - "close": 3039, - "high": 2000, - "low": 3039, - "open": 2000, - "volume": 5039 - }, - "open": "2020-10-17T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1601, - "high": 12454, - "low": 1601, - "open": 12455, - "volume": 106020 - }, - "id": 2748724, - "non_hive": { - "close": 257, - "high": 2000, - "low": 257, - "open": 2000, - "volume": 17024 - }, - "open": "2020-10-17T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 177, - "high": 177, - "low": 6345, - "open": 12649, - "volume": 288127 - }, - "id": 2748729, - "non_hive": { - "close": 28, - "high": 28, - "low": 1000, - "open": 2000, - "volume": 45443 - }, - "open": "2020-10-17T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12471, - "high": 4295, - "low": 3390, - "open": 3390, - "volume": 20156 - }, - "id": 2748732, - "non_hive": { - "close": 1991, - "high": 693, - "low": 532, - "open": 532, - "volume": 3216 - }, - "open": "2020-10-17T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 9229, - "high": 9229, - "low": 9229, - "open": 9229, - "volume": 9229 - }, - "id": 2748739, - "non_hive": { - "close": 1472, - "high": 1472, - "low": 1472, - "open": 1472, - "volume": 1472 - }, - "open": "2020-10-17T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 16569, - "high": 16569, - "low": 6754, - "open": 2475, - "volume": 65349 - }, - "id": 2748742, - "non_hive": { - "close": 2675, - "high": 2675, - "low": 1077, - "open": 395, - "volume": 10463 - }, - "open": "2020-10-17T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 5536, - "high": 5536, - "low": 5536, - "open": 5536, - "volume": 5536 - }, - "id": 2748747, - "non_hive": { - "close": 893, - "high": 893, - "low": 893, - "open": 893, - "volume": 893 - }, - "open": "2020-10-17T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 43, - "high": 43, - "low": 6001, - "open": 6001, - "volume": 6044 - }, - "id": 2748750, - "non_hive": { - "close": 7, - "high": 7, - "low": 968, - "open": 968, - "volume": 975 - }, - "open": "2020-10-17T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 2444, - "high": 2444, - "low": 2444, - "open": 2444, - "volume": 2444 - }, - "id": 2748755, - "non_hive": { - "close": 390, - "high": 390, - "low": 390, - "open": 390, - "volume": 390 - }, - "open": "2020-10-17T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 112418, - "high": 112418, - "low": 12679, - "open": 12679, - "volume": 164020 - }, - "id": 2748759, - "non_hive": { - "close": 18138, - "high": 18138, - "low": 1999, - "open": 1999, - "volume": 26282 - }, - "open": "2020-10-17T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 26384, - "high": 26384, - "low": 9747, - "open": 9747, - "volume": 36131 - }, - "id": 2748762, - "non_hive": { - "close": 4257, - "high": 4257, - "low": 1572, - "open": 1572, - "volume": 5829 - }, - "open": "2020-10-17T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 9436, - "high": 9436, - "low": 9436, - "open": 9436, - "volume": 9436 - }, - "id": 2748765, - "non_hive": { - "close": 1521, - "high": 1521, - "low": 1521, - "open": 1521, - "volume": 1521 - }, - "open": "2020-10-17T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 27274, - "high": 27274, - "low": 27274, - "open": 27274, - "volume": 27274 - }, - "id": 2748768, - "non_hive": { - "close": 4399, - "high": 4399, - "low": 4399, - "open": 4399, - "volume": 4399 - }, - "open": "2020-10-17T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 31368, - "high": 31368, - "low": 31368, - "open": 31368, - "volume": 31368 - }, - "id": 2748771, - "non_hive": { - "close": 5080, - "high": 5080, - "low": 5080, - "open": 5080, - "volume": 5080 - }, - "open": "2020-10-17T19:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 8336, - "high": 53804, - "low": 8336, - "open": 31509, - "volume": 203583 - }, - "id": 2748775, - "non_hive": { - "close": 1308, - "high": 8715, - "low": 1308, - "open": 5103, - "volume": 32512 - }, - "open": "2020-10-17T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 5358, - "high": 6, - "low": 7251, - "open": 12742, - "volume": 203282 - }, - "id": 2748783, - "non_hive": { - "close": 858, - "high": 1, - "low": 1137, - "open": 1999, - "volume": 32495 - }, - "open": "2020-10-17T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 9213, - "high": 9213, - "low": 9213, - "open": 9213, - "volume": 9213 - }, - "id": 2748791, - "non_hive": { - "close": 1473, - "high": 1473, - "low": 1473, - "open": 1473, - "volume": 1473 - }, - "open": "2020-10-17T19:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 8450, - "high": 8450, - "low": 8450, - "open": 8450, - "volume": 8450 - }, - "id": 2748794, - "non_hive": { - "close": 1351, - "high": 1351, - "low": 1351, - "open": 1351, - "volume": 1351 - }, - "open": "2020-10-17T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1982, - "high": 1982, - "low": 1982, - "open": 1982, - "volume": 1982 - }, - "id": 2748797, - "non_hive": { - "close": 317, - "high": 317, - "low": 317, - "open": 317, - "volume": 317 - }, - "open": "2020-10-17T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 19184, - "high": 19184, - "low": 19184, - "open": 19184, - "volume": 19184 - }, - "id": 2748800, - "non_hive": { - "close": 3067, - "high": 3067, - "low": 3067, - "open": 3067, - "volume": 3067 - }, - "open": "2020-10-17T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1900, - "high": 1900, - "low": 1900, - "open": 1900, - "volume": 1900 - }, - "id": 2748803, - "non_hive": { - "close": 300, - "high": 300, - "low": 300, - "open": 300, - "volume": 300 - }, - "open": "2020-10-17T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 20101, - "high": 20101, - "low": 9082, - "open": 9082, - "volume": 41649 - }, - "id": 2748806, - "non_hive": { - "close": 3221, - "high": 3221, - "low": 1436, - "open": 1436, - "volume": 6630 - }, - "open": "2020-10-17T20:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 9312, - "high": 9312, - "low": 9312, - "open": 9312, - "volume": 9312 - }, - "id": 2748813, - "non_hive": { - "close": 1492, - "high": 1492, - "low": 1492, - "open": 1492, - "volume": 1492 - }, - "open": "2020-10-17T20:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 17742, - "high": 4418, - "low": 17742, - "open": 4418, - "volume": 22160 - }, - "id": 2748816, - "non_hive": { - "close": 2843, - "high": 708, - "low": 2843, - "open": 708, - "volume": 3551 - }, - "open": "2020-10-17T20:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 365128, - "high": 365128, - "low": 31436, - "open": 31436, - "volume": 1077106 - }, - "id": 2748821, - "non_hive": { - "close": 59504, - "high": 59504, - "low": 5041, - "open": 5041, - "volume": 174801 - }, - "open": "2020-10-17T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 20507, - "high": 20507, - "low": 20507, - "open": 20507, - "volume": 20507 - }, - "id": 2748826, - "non_hive": { - "close": 3342, - "high": 3342, - "low": 3342, - "open": 3342, - "volume": 3342 - }, - "open": "2020-10-17T20:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1454, - "high": 2095, - "low": 1454, - "open": 2095, - "volume": 3549 - }, - "id": 2748829, - "non_hive": { - "close": 230, - "high": 332, - "low": 230, - "open": 332, - "volume": 562 - }, - "open": "2020-10-17T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 7518, - "high": 7518, - "low": 7518, - "open": 7518, - "volume": 7518 - }, - "id": 2748832, - "non_hive": { - "close": 1188, - "high": 1188, - "low": 1188, - "open": 1188, - "volume": 1188 - }, - "open": "2020-10-17T20:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 75905, - "high": 75905, - "low": 7524, - "open": 7524, - "volume": 83429 - }, - "id": 2748835, - "non_hive": { - "close": 12000, - "high": 12000, - "low": 1189, - "open": 1189, - "volume": 13189 - }, - "open": "2020-10-17T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 7524, - "high": 7524, - "low": 7524, - "open": 7524, - "volume": 7524 - }, - "id": 2748841, - "non_hive": { - "close": 1189, - "high": 1189, - "low": 1189, - "open": 1189, - "volume": 1189 - }, - "open": "2020-10-17T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 671, - "high": 7073, - "low": 671, - "open": 32477, - "volume": 202459 - }, - "id": 2748844, - "non_hive": { - "close": 106, - "high": 1118, - "low": 106, - "open": 5133, - "volume": 31995 - }, - "open": "2020-10-17T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6320, - "high": 6320, - "low": 6320, - "open": 6320, - "volume": 6320 - }, - "id": 2748847, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-10-17T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 55832, - "high": 55832, - "low": 7505, - "open": 7505, - "volume": 63337 - }, - "id": 2748850, - "non_hive": { - "close": 8816, - "high": 8816, - "low": 1185, - "open": 1185, - "volume": 10001 - }, - "open": "2020-10-17T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 11063, - "high": 11063, - "low": 11063, - "open": 11063, - "volume": 11063 - }, - "id": 2748853, - "non_hive": { - "close": 1747, - "high": 1747, - "low": 1747, - "open": 1747, - "volume": 1747 - }, - "open": "2020-10-17T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6334, - "high": 6334, - "low": 6334, - "open": 6334, - "volume": 6334 - }, - "id": 2748856, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-17T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 9578, - "high": 9578, - "low": 7567, - "open": 7567, - "volume": 28197 - }, - "id": 2748859, - "non_hive": { - "close": 1561, - "high": 1561, - "low": 1198, - "open": 1198, - "volume": 4512 - }, - "open": "2020-10-17T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 11027, - "high": 6135, - "low": 11027, - "open": 5012, - "volume": 22174 - }, - "id": 2748862, - "non_hive": { - "close": 1763, - "high": 999, - "low": 1763, - "open": 816, - "volume": 3578 - }, - "open": "2020-10-17T22:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 7696, - "high": 7696, - "low": 7696, - "open": 7696, - "volume": 7696 - }, - "id": 2748869, - "non_hive": { - "close": 1230, - "high": 1230, - "low": 1230, - "open": 1230, - "volume": 1230 - }, - "open": "2020-10-17T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 7696, - "high": 10018, - "low": 7696, - "open": 10018, - "volume": 17714 - }, - "id": 2748872, - "non_hive": { - "close": 1230, - "high": 1602, - "low": 1230, - "open": 1602, - "volume": 2832 - }, - "open": "2020-10-17T22:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7696, - "high": 7696, - "low": 7696, - "open": 7696, - "volume": 7696 - }, - "id": 2748876, - "non_hive": { - "close": 1230, - "high": 1230, - "low": 1230, - "open": 1230, - "volume": 1230 - }, - "open": "2020-10-17T22:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 23090, - "high": 32608, - "low": 23090, - "open": 32608, - "volume": 55698 - }, - "id": 2748879, - "non_hive": { - "close": 3692, - "high": 5214, - "low": 3692, - "open": 5214, - "volume": 8906 - }, - "open": "2020-10-17T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 21547, - "high": 21547, - "low": 1302, - "open": 7694, - "volume": 30543 - }, - "id": 2748882, - "non_hive": { - "close": 3445, - "high": 3445, - "low": 208, - "open": 1230, - "volume": 4883 - }, - "open": "2020-10-17T22:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 11497, - "high": 5992, - "low": 11497, - "open": 5992, - "volume": 24759 - }, - "id": 2748887, - "non_hive": { - "close": 1837, - "high": 958, - "low": 1837, - "open": 958, - "volume": 3957 - }, - "open": "2020-10-17T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 195993, - "high": 6, - "low": 58978, - "open": 6, - "volume": 706921 - }, - "id": 2748894, - "non_hive": { - "close": 32339, - "high": 1, - "low": 9428, - "open": 1, - "volume": 115872 - }, - "open": "2020-10-17T23:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 945, - "high": 945, - "low": 945, - "open": 945, - "volume": 945 - }, - "id": 2748899, - "non_hive": { - "close": 156, - "high": 156, - "low": 156, - "open": 156, - "volume": 156 - }, - "open": "2020-10-17T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 25455, - "high": 25455, - "low": 25455, - "open": 25455, - "volume": 25455 - }, - "id": 2748902, - "non_hive": { - "close": 4200, - "high": 4200, - "low": 4200, - "open": 4200, - "volume": 4200 - }, - "open": "2020-10-17T23:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 20000, - "high": 20000, - "low": 20000, - "open": 20000, - "volume": 20000 - }, - "id": 2748905, - "non_hive": { - "close": 3300, - "high": 3300, - "low": 3300, - "open": 3300, - "volume": 3300 - }, - "open": "2020-10-17T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 15419, - "high": 15419, - "low": 31552, - "open": 31552, - "volume": 46971 - }, - "id": 2748908, - "non_hive": { - "close": 2539, - "high": 2539, - "low": 5195, - "open": 5195, - "volume": 7734 - }, - "open": "2020-10-18T00:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 428533, - "high": 428533, - "low": 31552, - "open": 31552, - "volume": 460085 - }, - "id": 2748914, - "non_hive": { - "close": 70708, - "high": 70708, - "low": 5206, - "open": 5206, - "volume": 75914 - }, - "open": "2020-10-18T00:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1674, - "high": 1674, - "low": 1674, - "open": 1674, - "volume": 1674 - }, - "id": 2748917, - "non_hive": { - "close": 276, - "high": 276, - "low": 276, - "open": 276, - "volume": 276 - }, - "open": "2020-10-18T00:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 96910, - "high": 96910, - "low": 96910, - "open": 96910, - "volume": 96910 - }, - "id": 2748920, - "non_hive": { - "close": 15990, - "high": 15990, - "low": 15990, - "open": 15990, - "volume": 15990 - }, - "open": "2020-10-18T00:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 2698, - "high": 2698, - "low": 2698, - "open": 2698, - "volume": 2698 - }, - "id": 2748923, - "non_hive": { - "close": 445, - "high": 445, - "low": 445, - "open": 445, - "volume": 445 - }, - "open": "2020-10-18T01:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 9903, - "high": 9903, - "low": 9903, - "open": 9903, - "volume": 9903 - }, - "id": 2748927, - "non_hive": { - "close": 1634, - "high": 1634, - "low": 1634, - "open": 1634, - "volume": 1634 - }, - "open": "2020-10-18T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 2370, - "high": 2370, - "low": 2370, - "open": 2370, - "volume": 2370 - }, - "id": 2748930, - "non_hive": { - "close": 391, - "high": 391, - "low": 391, - "open": 391, - "volume": 391 - }, - "open": "2020-10-18T01:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 15184, - "high": 15184, - "low": 15184, - "open": 15184, - "volume": 15184 - }, - "id": 2748933, - "non_hive": { - "close": 2505, - "high": 2505, - "low": 2505, - "open": 2505, - "volume": 2505 - }, - "open": "2020-10-18T01:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 5406, - "high": 5406, - "low": 31415, - "open": 31415, - "volume": 36821 - }, - "id": 2748936, - "non_hive": { - "close": 883, - "high": 883, - "low": 5131, - "open": 5131, - "volume": 6014 - }, - "open": "2020-10-18T02:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 31483, - "high": 31483, - "low": 31483, - "open": 31483, - "volume": 31483 - }, - "id": 2748942, - "non_hive": { - "close": 5142, - "high": 5142, - "low": 5142, - "open": 5142, - "volume": 5142 - }, - "open": "2020-10-18T02:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 9166, - "high": 9166, - "low": 9166, - "open": 9166, - "volume": 9166 - }, - "id": 2748945, - "non_hive": { - "close": 1512, - "high": 1512, - "low": 1512, - "open": 1512, - "volume": 1512 - }, - "open": "2020-10-18T02:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 19055, - "high": 12495, - "low": 19055, - "open": 12495, - "volume": 31550 - }, - "id": 2748948, - "non_hive": { - "close": 3049, - "high": 2000, - "low": 3049, - "open": 2000, - "volume": 5049 - }, - "open": "2020-10-18T02:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 121246, - "high": 5234, - "low": 335, - "open": 12160, - "volume": 158030 - }, - "id": 2748951, - "non_hive": { - "close": 19945, - "high": 861, - "low": 55, - "open": 1999, - "volume": 25994 - }, - "open": "2020-10-18T02:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12491, - "high": 12491, - "low": 12491, - "open": 12491, - "volume": 24982 - }, - "id": 2748957, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-10-18T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 115909, - "high": 16105, - "low": 12158, - "open": 12158, - "volume": 521686 - }, - "id": 2748963, - "non_hive": { - "close": 19067, - "high": 2657, - "low": 1999, - "open": 1999, - "volume": 85821 - }, - "open": "2020-10-18T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 18950, - "high": 31671, - "low": 18950, - "open": 666, - "volume": 63703 - }, - "id": 2748971, - "non_hive": { - "close": 3052, - "high": 5183, - "low": 3052, - "open": 108, - "volume": 10343 - }, - "open": "2020-10-18T03:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12216, - "high": 12216, - "low": 12221, - "open": 12221, - "volume": 55431 - }, - "id": 2748976, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 1999, - "open": 1999, - "volume": 9072 - }, - "open": "2020-10-18T03:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 10594, - "high": 10594, - "low": 32358, - "open": 12216, - "volume": 101175 - }, - "id": 2748981, - "non_hive": { - "close": 1748, - "high": 1748, - "low": 5212, - "open": 2000, - "volume": 16547 - }, - "open": "2020-10-18T03:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 19922, - "high": 12413, - "low": 19922, - "open": 12413, - "volume": 32335 - }, - "id": 2748988, - "non_hive": { - "close": 3209, - "high": 2000, - "low": 3209, - "open": 2000, - "volume": 5209 - }, - "open": "2020-10-18T03:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 18975, - "high": 12413, - "low": 18975, - "open": 12413, - "volume": 31388 - }, - "id": 2748991, - "non_hive": { - "close": 3057, - "high": 2000, - "low": 3057, - "open": 2000, - "volume": 5057 - }, - "open": "2020-10-18T03:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 13683, - "high": 12408, - "low": 13683, - "open": 12408, - "volume": 31365 - }, - "id": 2748994, - "non_hive": { - "close": 2205, - "high": 2000, - "low": 2205, - "open": 2000, - "volume": 5055 - }, - "open": "2020-10-18T04:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 18957, - "high": 12408, - "low": 18957, - "open": 12408, - "volume": 31365 - }, - "id": 2748998, - "non_hive": { - "close": 3055, - "high": 2000, - "low": 3055, - "open": 2000, - "volume": 5055 - }, - "open": "2020-10-18T04:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 31414, - "high": 31414, - "low": 31414, - "open": 31414, - "volume": 31414 - }, - "id": 2749001, - "non_hive": { - "close": 5062, - "high": 5062, - "low": 5062, - "open": 5062, - "volume": 5062 - }, - "open": "2020-10-18T04:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 5382, - "high": 12407, - "low": 5382, - "open": 12407, - "volume": 31414 - }, - "id": 2749004, - "non_hive": { - "close": 867, - "high": 2000, - "low": 867, - "open": 2000, - "volume": 5063 - }, - "open": "2020-10-18T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 8688, - "high": 9877, - "low": 8688, - "open": 9877, - "volume": 31421 - }, - "id": 2749007, - "non_hive": { - "close": 1400, - "high": 1592, - "low": 1400, - "open": 1592, - "volume": 5064 - }, - "open": "2020-10-18T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 31444, - "high": 31444, - "low": 31444, - "open": 31444, - "volume": 31444 - }, - "id": 2749010, - "non_hive": { - "close": 5067, - "high": 5067, - "low": 5067, - "open": 5067, - "volume": 5067 - }, - "open": "2020-10-18T04:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6018, - "high": 25452, - "low": 6018, - "open": 25452, - "volume": 31470 - }, - "id": 2749013, - "non_hive": { - "close": 969, - "high": 4102, - "low": 969, - "open": 4102, - "volume": 5071 - }, - "open": "2020-10-18T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 543, - "high": 30927, - "low": 543, - "open": 30927, - "volume": 31470 - }, - "id": 2749016, - "non_hive": { - "close": 87, - "high": 5000, - "low": 87, - "open": 5000, - "volume": 5087 - }, - "open": "2020-10-18T04:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 93943, - "high": 93943, - "low": 93943, - "open": 93943, - "volume": 93943 - }, - "id": 2749019, - "non_hive": { - "close": 15135, - "high": 15135, - "low": 15135, - "open": 15135, - "volume": 15135 - }, - "open": "2020-10-18T04:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 18410, - "high": 621, - "low": 18410, - "open": 621, - "volume": 31454 - }, - "id": 2749022, - "non_hive": { - "close": 2947, - "high": 100, - "low": 2947, - "open": 100, - "volume": 5047 - }, - "open": "2020-10-18T04:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 31477, - "high": 31477, - "low": 31477, - "open": 31477, - "volume": 31477 - }, - "id": 2749025, - "non_hive": { - "close": 5039, - "high": 5039, - "low": 5039, - "open": 5039, - "volume": 5039 - }, - "open": "2020-10-18T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12162, - "high": 12162, - "low": 12162, - "open": 12162, - "volume": 12162 - }, - "id": 2749029, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-18T05:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 839, - "high": 839, - "low": 8640, - "open": 8640, - "volume": 9479 - }, - "id": 2749032, - "non_hive": { - "close": 138, - "high": 138, - "low": 1421, - "open": 1421, - "volume": 1559 - }, - "open": "2020-10-18T05:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 11231, - "high": 11231, - "low": 11231, - "open": 11231, - "volume": 11231 - }, - "id": 2749037, - "non_hive": { - "close": 1827, - "high": 1827, - "low": 1827, - "open": 1827, - "volume": 1827 - }, - "open": "2020-10-18T05:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 12289, - "high": 12289, - "low": 12289, - "open": 12289, - "volume": 12289 - }, - "id": 2749040, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-18T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 571, - "high": 571, - "low": 571, - "open": 571, - "volume": 571 - }, - "id": 2749043, - "non_hive": { - "close": 93, - "high": 93, - "low": 93, - "open": 93, - "volume": 93 - }, - "open": "2020-10-18T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 5322, - "high": 5322, - "low": 12421, - "open": 12421, - "volume": 17743 - }, - "id": 2749046, - "non_hive": { - "close": 857, - "high": 857, - "low": 1999, - "open": 1999, - "volume": 2856 - }, - "open": "2020-10-18T06:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12227, - "high": 12227, - "low": 63287, - "open": 63287, - "volume": 137864 - }, - "id": 2749050, - "non_hive": { - "close": 1969, - "high": 1969, - "low": 10190, - "open": 10190, - "volume": 22199 - }, - "open": "2020-10-18T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 2806, - "high": 2806, - "low": 12553, - "open": 15522, - "volume": 46843 - }, - "id": 2749056, - "non_hive": { - "close": 452, - "high": 452, - "low": 1999, - "open": 2498, - "volume": 7492 - }, - "open": "2020-10-18T06:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2435, - "high": 2435, - "low": 51, - "open": 12645, - "volume": 53486 - }, - "id": 2749061, - "non_hive": { - "close": 388, - "high": 388, - "low": 8, - "open": 2000, - "volume": 8482 - }, - "open": "2020-10-18T06:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 29456, - "high": 2563, - "low": 29456, - "open": 2563, - "volume": 32019 - }, - "id": 2749069, - "non_hive": { - "close": 4657, - "high": 408, - "low": 4657, - "open": 408, - "volume": 5065 - }, - "open": "2020-10-18T06:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 27804, - "high": 12647, - "low": 27804, - "open": 12647, - "volume": 40451 - }, - "id": 2749072, - "non_hive": { - "close": 4396, - "high": 2000, - "low": 4396, - "open": 2000, - "volume": 6396 - }, - "open": "2020-10-18T06:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 8002, - "high": 8002, - "low": 12553, - "open": 12553, - "volume": 49604 - }, - "id": 2749075, - "non_hive": { - "close": 1288, - "high": 1288, - "low": 1999, - "open": 1999, - "volume": 7915 - }, - "open": "2020-10-18T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2682, - "high": 2682, - "low": 4418, - "open": 4418, - "volume": 7100 - }, - "id": 2749083, - "non_hive": { - "close": 432, - "high": 432, - "low": 711, - "open": 711, - "volume": 1143 - }, - "open": "2020-10-18T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 19248, - "high": 12550, - "low": 19248, - "open": 12550, - "volume": 31798 - }, - "id": 2749086, - "non_hive": { - "close": 3067, - "high": 2000, - "low": 3067, - "open": 2000, - "volume": 5067 - }, - "open": "2020-10-18T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 10370, - "high": 10370, - "low": 19249, - "open": 12549, - "volume": 54588 - }, - "id": 2749089, - "non_hive": { - "close": 1670, - "high": 1670, - "low": 3067, - "open": 2000, - "volume": 8736 - }, - "open": "2020-10-18T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 161, - "high": 161, - "low": 12420, - "open": 12420, - "volume": 12581 - }, - "id": 2749096, - "non_hive": { - "close": 26, - "high": 26, - "low": 1999, - "open": 1999, - "volume": 2025 - }, - "open": "2020-10-18T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 7002, - "high": 7002, - "low": 7002, - "open": 7002, - "volume": 7002 - }, - "id": 2749099, - "non_hive": { - "close": 1127, - "high": 1127, - "low": 1127, - "open": 1127, - "volume": 1127 - }, - "open": "2020-10-18T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 25101, - "high": 25101, - "low": 5418, - "open": 5418, - "volume": 65194 - }, - "id": 2749102, - "non_hive": { - "close": 4085, - "high": 4085, - "low": 872, - "open": 872, - "volume": 10540 - }, - "open": "2020-10-18T07:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 5087, - "high": 5087, - "low": 11594, - "open": 11594, - "volume": 16681 - }, - "id": 2749105, - "non_hive": { - "close": 828, - "high": 828, - "low": 1886, - "open": 1886, - "volume": 2714 - }, - "open": "2020-10-18T08:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 391, - "high": 391, - "low": 391, - "open": 391, - "volume": 391 - }, - "id": 2749109, - "non_hive": { - "close": 63, - "high": 63, - "low": 63, - "open": 63, - "volume": 63 - }, - "open": "2020-10-18T08:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 12201, - "high": 938, - "low": 12201, - "open": 938, - "volume": 13139 - }, - "id": 2749112, - "non_hive": { - "close": 2003, - "high": 154, - "low": 2003, - "open": 154, - "volume": 2157 - }, - "open": "2020-10-18T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 23879, - "high": 23879, - "low": 23879, - "open": 23879, - "volume": 23879 - }, - "id": 2749117, - "non_hive": { - "close": 3920, - "high": 3920, - "low": 3920, - "open": 3920, - "volume": 3920 - }, - "open": "2020-10-18T08:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11587, - "high": 14784, - "low": 11587, - "open": 14784, - "volume": 26371 - }, - "id": 2749120, - "non_hive": { - "close": 1902, - "high": 2427, - "low": 1902, - "open": 2427, - "volume": 4329 - }, - "open": "2020-10-18T08:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12268, - "high": 12268, - "low": 12269, - "open": 12269, - "volume": 36805 - }, - "id": 2749125, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 6000 - }, - "open": "2020-10-18T09:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12267, - "high": 12267, - "low": 12267, - "open": 12267, - "volume": 24534 - }, - "id": 2749133, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-10-18T09:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 73699, - "high": 678, - "low": 73699, - "open": 678, - "volume": 74377 - }, - "id": 2749137, - "non_hive": { - "close": 12013, - "high": 111, - "low": 12013, - "open": 111, - "volume": 12124 - }, - "open": "2020-10-18T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2461, - "high": 2461, - "low": 2461, - "open": 2461, - "volume": 2461 - }, - "id": 2749142, - "non_hive": { - "close": 401, - "high": 401, - "low": 401, - "open": 401, - "volume": 401 - }, - "open": "2020-10-18T09:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1919, - "high": 15418, - "low": 1919, - "open": 15418, - "volume": 17669 - }, - "id": 2749145, - "non_hive": { - "close": 312, - "high": 2508, - "low": 312, - "open": 2508, - "volume": 2874 - }, - "open": "2020-10-18T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 4106, - "high": 4106, - "low": 4106, - "open": 4106, - "volume": 4106 - }, - "id": 2749148, - "non_hive": { - "close": 669, - "high": 669, - "low": 669, - "open": 669, - "volume": 669 - }, - "open": "2020-10-18T09:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 19226, - "high": 2644, - "low": 19226, - "open": 8164, - "volume": 42532 - }, - "id": 2749151, - "non_hive": { - "close": 3076, - "high": 431, - "low": 3076, - "open": 1330, - "volume": 6837 - }, - "open": "2020-10-18T09:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 2184, - "high": 2184, - "low": 2184, - "open": 2184, - "volume": 2184 - }, - "id": 2749156, - "non_hive": { - "close": 349, - "high": 349, - "low": 349, - "open": 349, - "volume": 349 - }, - "open": "2020-10-18T10:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12273, - "high": 12273, - "low": 12273, - "open": 12273, - "volume": 12273 - }, - "id": 2749160, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-18T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 24547, - "high": 24547, - "low": 24547, - "open": 24547, - "volume": 24547 - }, - "id": 2749163, - "non_hive": { - "close": 4001, - "high": 4001, - "low": 4001, - "open": 4001, - "volume": 4001 - }, - "open": "2020-10-18T10:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 63327, - "high": 63327, - "low": 63327, - "open": 63327, - "volume": 63327 - }, - "id": 2749166, - "non_hive": { - "close": 10321, - "high": 10321, - "low": 10321, - "open": 10321, - "volume": 10321 - }, - "open": "2020-10-18T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 27288, - "high": 5442, - "low": 27288, - "open": 5442, - "volume": 63327 - }, - "id": 2749169, - "non_hive": { - "close": 4447, - "high": 887, - "low": 4447, - "open": 887, - "volume": 10321 - }, - "open": "2020-10-18T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 37615, - "high": 37615, - "low": 37615, - "open": 37615, - "volume": 37615 - }, - "id": 2749172, - "non_hive": { - "close": 6131, - "high": 6131, - "low": 6131, - "open": 6131, - "volume": 6131 - }, - "open": "2020-10-18T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 17105, - "high": 17105, - "low": 17105, - "open": 17105, - "volume": 17105 - }, - "id": 2749175, - "non_hive": { - "close": 2788, - "high": 2788, - "low": 2788, - "open": 2788, - "volume": 2788 - }, - "open": "2020-10-18T10:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 2289, - "high": 13491, - "low": 2289, - "open": 13491, - "volume": 99097 - }, - "id": 2749178, - "non_hive": { - "close": 373, - "high": 2199, - "low": 373, - "open": 2199, - "volume": 16152 - }, - "open": "2020-10-18T10:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 16743, - "high": 16743, - "low": 16743, - "open": 16743, - "volume": 16743 - }, - "id": 2749181, - "non_hive": { - "close": 2729, - "high": 2729, - "low": 2729, - "open": 2729, - "volume": 2729 - }, - "open": "2020-10-18T10:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12271, - "high": 12271, - "low": 12271, - "open": 12271, - "volume": 12271 - }, - "id": 2749184, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-18T11:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2546, - "high": 2546, - "low": 12271, - "open": 12271, - "volume": 14817 - }, - "id": 2749188, - "non_hive": { - "close": 415, - "high": 415, - "low": 1999, - "open": 1999, - "volume": 2414 - }, - "open": "2020-10-18T11:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13444, - "high": 13444, - "low": 13444, - "open": 13444, - "volume": 13444 - }, - "id": 2749191, - "non_hive": { - "close": 2191, - "high": 2191, - "low": 2191, - "open": 2191, - "volume": 2191 - }, - "open": "2020-10-18T11:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 50307, - "high": 50307, - "low": 50307, - "open": 50307, - "volume": 50307 - }, - "id": 2749194, - "non_hive": { - "close": 8200, - "high": 8200, - "low": 8200, - "open": 8200, - "volume": 8200 - }, - "open": "2020-10-18T11:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 125000, - "high": 37288, - "low": 125000, - "open": 37288, - "volume": 202088 - }, - "id": 2749197, - "non_hive": { - "close": 20363, - "high": 6078, - "low": 20363, - "open": 6078, - "volume": 32928 - }, - "open": "2020-10-18T12:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 24, - "high": 24, - "low": 24, - "open": 24, - "volume": 24 - }, - "id": 2749203, - "non_hive": { - "close": 4, - "high": 4, - "low": 4, - "open": 4, - "volume": 4 - }, - "open": "2020-10-18T12:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6651, - "high": 6651, - "low": 6651, - "open": 6651, - "volume": 6651 - }, - "id": 2749206, - "non_hive": { - "close": 1083, - "high": 1083, - "low": 1083, - "open": 1083, - "volume": 1083 - }, - "open": "2020-10-18T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3065, - "high": 19217, - "low": 3065, - "open": 19217, - "volume": 22282 - }, - "id": 2749209, - "non_hive": { - "close": 499, - "high": 3129, - "low": 499, - "open": 3129, - "volume": 3628 - }, - "open": "2020-10-18T12:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12266, - "high": 12266, - "low": 1426, - "open": 10859, - "volume": 85981 - }, - "id": 2749214, - "non_hive": { - "close": 1997, - "high": 1997, - "low": 232, - "open": 1767, - "volume": 13997 - }, - "open": "2020-10-18T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 53383, - "high": 53383, - "low": 7976, - "open": 7976, - "volume": 61359 - }, - "id": 2749221, - "non_hive": { - "close": 8691, - "high": 8691, - "low": 1298, - "open": 1298, - "volume": 9989 - }, - "open": "2020-10-18T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6002, - "high": 6002, - "low": 6002, - "open": 6002, - "volume": 6002 - }, - "id": 2749224, - "non_hive": { - "close": 977, - "high": 977, - "low": 977, - "open": 977, - "volume": 977 - }, - "open": "2020-10-18T13:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 7450, - "high": 7450, - "low": 36461, - "open": 36461, - "volume": 43911 - }, - "id": 2749228, - "non_hive": { - "close": 1213, - "high": 1213, - "low": 5936, - "open": 5936, - "volume": 7149 - }, - "open": "2020-10-18T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2303, - "high": 2303, - "low": 2303, - "open": 2303, - "volume": 2303 - }, - "id": 2749231, - "non_hive": { - "close": 375, - "high": 375, - "low": 375, - "open": 375, - "volume": 375 - }, - "open": "2020-10-18T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 92406, - "high": 92406, - "low": 92406, - "open": 92406, - "volume": 92406 - }, - "id": 2749234, - "non_hive": { - "close": 15044, - "high": 15044, - "low": 15044, - "open": 15044, - "volume": 15044 - }, - "open": "2020-10-18T13:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 15503, - "high": 15503, - "low": 47219, - "open": 47219, - "volume": 62722 - }, - "id": 2749237, - "non_hive": { - "close": 2527, - "high": 2527, - "low": 7687, - "open": 7687, - "volume": 10214 - }, - "open": "2020-10-18T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 21382, - "high": 2896, - "low": 21382, - "open": 2896, - "volume": 28647 - }, - "id": 2749243, - "non_hive": { - "close": 3481, - "high": 472, - "low": 3481, - "open": 472, - "volume": 4665 - }, - "open": "2020-10-18T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 116300, - "high": 116300, - "low": 25746, - "open": 25746, - "volume": 142046 - }, - "id": 2749246, - "non_hive": { - "close": 18957, - "high": 18957, - "low": 4191, - "open": 4191, - "volume": 23148 - }, - "open": "2020-10-18T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2743, - "high": 6257, - "low": 2743, - "open": 8898, - "volume": 17898 - }, - "id": 2749249, - "non_hive": { - "close": 446, - "high": 1020, - "low": 446, - "open": 1450, - "volume": 2916 - }, - "open": "2020-10-18T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 6142, - "high": 6142, - "low": 6142, - "open": 6142, - "volume": 6142 - }, - "id": 2749254, - "non_hive": { - "close": 1001, - "high": 1001, - "low": 1001, - "open": 1001, - "volume": 1001 - }, - "open": "2020-10-18T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 4790, - "high": 4790, - "low": 4790, - "open": 4790, - "volume": 4790 - }, - "id": 2749257, - "non_hive": { - "close": 780, - "high": 780, - "low": 780, - "open": 780, - "volume": 780 - }, - "open": "2020-10-18T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 8879, - "high": 8879, - "low": 8879, - "open": 8879, - "volume": 8879 - }, - "id": 2749260, - "non_hive": { - "close": 1445, - "high": 1445, - "low": 1445, - "open": 1445, - "volume": 1445 - }, - "open": "2020-10-18T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 2457, - "high": 350, - "low": 2457, - "open": 3193, - "volume": 6000 - }, - "id": 2749263, - "non_hive": { - "close": 399, - "high": 57, - "low": 399, - "open": 520, - "volume": 976 - }, - "open": "2020-10-18T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 271840, - "high": 6981, - "low": 8898, - "open": 6981, - "volume": 287719 - }, - "id": 2749266, - "non_hive": { - "close": 44310, - "high": 1138, - "low": 1450, - "open": 1138, - "volume": 46898 - }, - "open": "2020-10-18T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6135, - "high": 6135, - "low": 6135, - "open": 6135, - "volume": 6135 - }, - "id": 2749272, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-18T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 12488, - "high": 30, - "low": 12488, - "open": 815, - "volume": 364868 - }, - "id": 2749275, - "non_hive": { - "close": 2000, - "high": 5, - "low": 2000, - "open": 133, - "volume": 58775 - }, - "open": "2020-10-18T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2799, - "high": 2799, - "low": 1488, - "open": 1839, - "volume": 239793 - }, - "id": 2749281, - "non_hive": { - "close": 456, - "high": 456, - "low": 238, - "open": 296, - "volume": 38456 - }, - "open": "2020-10-18T15:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12491, - "high": 6139, - "low": 12491, - "open": 6139, - "volume": 18630 - }, - "id": 2749288, - "non_hive": { - "close": 2000, - "high": 1000, - "low": 2000, - "open": 1000, - "volume": 3000 - }, - "open": "2020-10-18T15:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2749293, - "non_hive": { - "close": 162, - "high": 162, - "low": 162, - "open": 162, - "volume": 162 - }, - "open": "2020-10-18T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 4003, - "high": 4003, - "low": 4003, - "open": 4003, - "volume": 4003 - }, - "id": 2749296, - "non_hive": { - "close": 651, - "high": 651, - "low": 651, - "open": 651, - "volume": 651 - }, - "open": "2020-10-18T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 28017, - "high": 20071, - "low": 28017, - "open": 20071, - "volume": 100224 - }, - "id": 2749300, - "non_hive": { - "close": 4554, - "high": 3264, - "low": 4554, - "open": 3264, - "volume": 16293 - }, - "open": "2020-10-18T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 66993, - "high": 66993, - "low": 12403, - "open": 12403, - "volume": 289543 - }, - "id": 2749303, - "non_hive": { - "close": 10895, - "high": 10895, - "low": 1999, - "open": 1999, - "volume": 47026 - }, - "open": "2020-10-18T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12298, - "high": 12298, - "low": 12298, - "open": 12298, - "volume": 12298 - }, - "id": 2749307, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-18T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 33696, - "high": 33696, - "low": 33696, - "open": 33696, - "volume": 33696 - }, - "id": 2749310, - "non_hive": { - "close": 5480, - "high": 5480, - "low": 5480, - "open": 5480, - "volume": 5480 - }, - "open": "2020-10-18T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 10000, - "high": 8332, - "low": 1770, - "open": 8332, - "volume": 97939 - }, - "id": 2749313, - "non_hive": { - "close": 1602, - "high": 1355, - "low": 283, - "open": 1355, - "volume": 15892 - }, - "open": "2020-10-18T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12344, - "high": 695, - "low": 12344, - "open": 12308, - "volume": 94000 - }, - "id": 2749321, - "non_hive": { - "close": 2000, - "high": 113, - "low": 2000, - "open": 1999, - "volume": 15258 - }, - "open": "2020-10-18T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 12344, - "high": 12344, - "low": 12344, - "open": 12344, - "volume": 12344 - }, - "id": 2749331, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-18T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 12421, - "high": 10284, - "low": 83543, - "open": 10284, - "volume": 143511 - }, - "id": 2749334, - "non_hive": { - "close": 2000, - "high": 1670, - "low": 13450, - "open": 1670, - "volume": 23120 - }, - "open": "2020-10-18T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 86335, - "high": 86335, - "low": 2737, - "open": 2737, - "volume": 89072 - }, - "id": 2749344, - "non_hive": { - "close": 14005, - "high": 14005, - "low": 440, - "open": 440, - "volume": 14445 - }, - "open": "2020-10-18T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4974, - "high": 4974, - "low": 100000, - "open": 12329, - "volume": 761242 - }, - "id": 2749349, - "non_hive": { - "close": 807, - "high": 807, - "low": 16018, - "open": 1999, - "volume": 122292 - }, - "open": "2020-10-18T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2025, - "high": 3620, - "low": 2025, - "open": 12421, - "volume": 112329 - }, - "id": 2749364, - "non_hive": { - "close": 326, - "high": 587, - "low": 326, - "open": 2000, - "volume": 18169 - }, - "open": "2020-10-18T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 10277, - "high": 2001, - "low": 10345, - "open": 2001, - "volume": 24624 - }, - "id": 2749374, - "non_hive": { - "close": 1664, - "high": 324, - "low": 1675, - "open": 324, - "volume": 3987 - }, - "open": "2020-10-18T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6, - "high": 6, - "low": 68, - "open": 68, - "volume": 74 - }, - "id": 2749378, - "non_hive": { - "close": 1, - "high": 1, - "low": 11, - "open": 11, - "volume": 12 - }, - "open": "2020-10-18T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 370, - "high": 370, - "low": 370, - "open": 370, - "volume": 370 - }, - "id": 2749381, - "non_hive": { - "close": 60, - "high": 60, - "low": 60, - "open": 60, - "volume": 60 - }, - "open": "2020-10-18T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 58286, - "high": 7971, - "low": 58286, - "open": 10396, - "volume": 108367 - }, - "id": 2749384, - "non_hive": { - "close": 9336, - "high": 1291, - "low": 9336, - "open": 1674, - "volume": 17407 - }, - "open": "2020-10-18T19:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2377, - "high": 12421, - "low": 2377, - "open": 12421, - "volume": 32373 - }, - "id": 2749392, - "non_hive": { - "close": 380, - "high": 2000, - "low": 380, - "open": 2000, - "volume": 5202 - }, - "open": "2020-10-18T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 4260, - "high": 28113, - "low": 4260, - "open": 12335, - "volume": 44708 - }, - "id": 2749395, - "non_hive": { - "close": 689, - "high": 4558, - "low": 689, - "open": 1999, - "volume": 7246 - }, - "open": "2020-10-18T19:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12342, - "high": 23429, - "low": 12342, - "open": 87645, - "volume": 149796 - }, - "id": 2749400, - "non_hive": { - "close": 1999, - "high": 3797, - "low": 1999, - "open": 14203, - "volume": 24274 - }, - "open": "2020-10-18T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 246969, - "high": 246969, - "low": 246969, - "open": 246969, - "volume": 246969 - }, - "id": 2749407, - "non_hive": { - "close": 40001, - "high": 40001, - "low": 40001, - "open": 40001, - "volume": 40001 - }, - "open": "2020-10-18T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 53350, - "high": 53350, - "low": 53350, - "open": 53350, - "volume": 53350 - }, - "id": 2749410, - "non_hive": { - "close": 8641, - "high": 8641, - "low": 8641, - "open": 8641, - "volume": 8641 - }, - "open": "2020-10-18T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1921, - "high": 3550, - "low": 3731, - "open": 3550, - "volume": 9202 - }, - "id": 2749413, - "non_hive": { - "close": 311, - "high": 575, - "low": 604, - "open": 575, - "volume": 1490 - }, - "open": "2020-10-18T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 3000, - "high": 3000, - "low": 12357, - "open": 12357, - "volume": 15357 - }, - "id": 2749418, - "non_hive": { - "close": 486, - "high": 486, - "low": 1999, - "open": 1999, - "volume": 2485 - }, - "open": "2020-10-18T20:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1569, - "high": 12178, - "low": 1569, - "open": 12178, - "volume": 13747 - }, - "id": 2749424, - "non_hive": { - "close": 253, - "high": 1970, - "low": 253, - "open": 1970, - "volume": 2223 - }, - "open": "2020-10-18T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 3813, - "high": 12401, - "low": 3813, - "open": 12401, - "volume": 16214 - }, - "id": 2749428, - "non_hive": { - "close": 614, - "high": 2000, - "low": 614, - "open": 2000, - "volume": 2614 - }, - "open": "2020-10-18T20:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 5915, - "high": 61742, - "low": 12365, - "open": 48964, - "volume": 128986 - }, - "id": 2749431, - "non_hive": { - "close": 958, - "high": 10000, - "low": 1999, - "open": 7920, - "volume": 20877 - }, - "open": "2020-10-18T20:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 12348, - "high": 12348, - "low": 49163, - "open": 12413, - "volume": 73924 - }, - "id": 2749439, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 7920, - "open": 2000, - "volume": 11919 - }, - "open": "2020-10-18T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7205, - "high": 7205, - "low": 7205, - "open": 7205, - "volume": 7205 - }, - "id": 2749444, - "non_hive": { - "close": 1167, - "high": 1167, - "low": 1167, - "open": 1167, - "volume": 1167 - }, - "open": "2020-10-18T20:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 61716, - "high": 61716, - "low": 32653, - "open": 32653, - "volume": 152655 - }, - "id": 2749447, - "non_hive": { - "close": 9996, - "high": 9996, - "low": 5287, - "open": 5287, - "volume": 24723 - }, - "open": "2020-10-18T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7446, - "high": 7446, - "low": 7446, - "open": 7446, - "volume": 7446 - }, - "id": 2749452, - "non_hive": { - "close": 1206, - "high": 1206, - "low": 1206, - "open": 1206, - "volume": 1206 - }, - "open": "2020-10-18T20:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 19022, - "high": 36736, - "low": 19022, - "open": 12830, - "volume": 81008 - }, - "id": 2749455, - "non_hive": { - "close": 3062, - "high": 5950, - "low": 3062, - "open": 2078, - "volume": 13090 - }, - "open": "2020-10-18T20:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 173, - "high": 12348, - "low": 173, - "open": 12348, - "volume": 12521 - }, - "id": 2749461, - "non_hive": { - "close": 28, - "high": 1999, - "low": 28, - "open": 1999, - "volume": 2027 - }, - "open": "2020-10-18T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 80, - "high": 80, - "low": 80, - "open": 80, - "volume": 80 - }, - "id": 2749467, - "non_hive": { - "close": 13, - "high": 13, - "low": 13, - "open": 13, - "volume": 13 - }, - "open": "2020-10-18T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6175, - "high": 6175, - "low": 3557, - "open": 3557, - "volume": 9732 - }, - "id": 2749470, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 576, - "open": 576, - "volume": 1576 - }, - "open": "2020-10-18T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 61727, - "high": 12419, - "low": 61727, - "open": 12419, - "volume": 160386 - }, - "id": 2749475, - "non_hive": { - "close": 9938, - "high": 2000, - "low": 9938, - "open": 2000, - "volume": 25824 - }, - "open": "2020-10-18T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 23160, - "high": 25827, - "low": 1997, - "open": 12419, - "volume": 444837 - }, - "id": 2749478, - "non_hive": { - "close": 3751, - "high": 4183, - "low": 319, - "open": 1998, - "volume": 71339 - }, - "open": "2020-10-18T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 5600, - "high": 5600, - "low": 5600, - "open": 5600, - "volume": 5600 - }, - "id": 2749487, - "non_hive": { - "close": 907, - "high": 907, - "low": 907, - "open": 907, - "volume": 907 - }, - "open": "2020-10-18T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 7699, - "high": 7699, - "low": 7699, - "open": 7699, - "volume": 7699 - }, - "id": 2749491, - "non_hive": { - "close": 1247, - "high": 1247, - "low": 1247, - "open": 1247, - "volume": 1247 - }, - "open": "2020-10-18T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 49887, - "high": 49887, - "low": 49887, - "open": 49887, - "volume": 49887 - }, - "id": 2749494, - "non_hive": { - "close": 8080, - "high": 8080, - "low": 8080, - "open": 8080, - "volume": 8080 - }, - "open": "2020-10-18T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 5050, - "high": 5050, - "low": 543559, - "open": 14287, - "volume": 562896 - }, - "id": 2749497, - "non_hive": { - "close": 818, - "high": 818, - "low": 88037, - "open": 2314, - "volume": 91169 - }, - "open": "2020-10-18T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 56234, - "high": 27784, - "low": 56234, - "open": 27784, - "volume": 197711 - }, - "id": 2749502, - "non_hive": { - "close": 8998, - "high": 4500, - "low": 8998, - "open": 4500, - "volume": 31864 - }, - "open": "2020-10-18T23:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2268, - "high": 2268, - "low": 2268, - "open": 2268, - "volume": 2268 - }, - "id": 2749505, - "non_hive": { - "close": 363, - "high": 363, - "low": 363, - "open": 363, - "volume": 363 - }, - "open": "2020-10-18T23:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 53746, - "high": 53746, - "low": 10022, - "open": 10022, - "volume": 63768 - }, - "id": 2749508, - "non_hive": { - "close": 8705, - "high": 8705, - "low": 1603, - "open": 1603, - "volume": 10308 - }, - "open": "2020-10-18T23:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 3704, - "high": 3704, - "low": 3704, - "open": 3704, - "volume": 3704 - }, - "id": 2749511, - "non_hive": { - "close": 600, - "high": 600, - "low": 600, - "open": 600, - "volume": 600 - }, - "open": "2020-10-18T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 9423, - "high": 9423, - "low": 9423, - "open": 9423, - "volume": 9423 - }, - "id": 2749514, - "non_hive": { - "close": 1526, - "high": 1526, - "low": 1526, - "open": 1526, - "volume": 1526 - }, - "open": "2020-10-19T00:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 14707, - "high": 23426, - "low": 14707, - "open": 23426, - "volume": 47400 - }, - "id": 2749519, - "non_hive": { - "close": 2382, - "high": 3800, - "low": 2382, - "open": 3800, - "volume": 7683 - }, - "open": "2020-10-19T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12375, - "high": 25809, - "low": 232668, - "open": 25809, - "volume": 320466 - }, - "id": 2749525, - "non_hive": { - "close": 2000, - "high": 4180, - "low": 37227, - "open": 4180, - "volume": 51407 - }, - "open": "2020-10-19T00:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 12398, - "high": 12398, - "low": 12413, - "open": 12413, - "volume": 37209 - }, - "id": 2749537, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 6000 - }, - "open": "2020-10-19T00:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 210, - "high": 210, - "low": 210, - "open": 210, - "volume": 210 - }, - "id": 2749543, - "non_hive": { - "close": 34, - "high": 34, - "low": 34, - "open": 34, - "volume": 34 - }, - "open": "2020-10-19T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 715, - "high": 715, - "low": 715, - "open": 715, - "volume": 715 - }, - "id": 2749546, - "non_hive": { - "close": 116, - "high": 116, - "low": 116, - "open": 116, - "volume": 116 - }, - "open": "2020-10-19T00:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 37263, - "high": 6169, - "low": 37263, - "open": 6169, - "volume": 44150 - }, - "id": 2749549, - "non_hive": { - "close": 6010, - "high": 1000, - "low": 6010, - "open": 1000, - "volume": 7126 - }, - "open": "2020-10-19T00:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 117, - "high": 117, - "low": 50565, - "open": 50565, - "volume": 50682 - }, - "id": 2749556, - "non_hive": { - "close": 19, - "high": 19, - "low": 8091, - "open": 8091, - "volume": 8110 - }, - "open": "2020-10-19T01:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6463, - "high": 197488, - "low": 6463, - "open": 197488, - "volume": 203951 - }, - "id": 2749561, - "non_hive": { - "close": 1034, - "high": 31602, - "low": 1034, - "open": 31602, - "volume": 32636 - }, - "open": "2020-10-19T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 4302, - "high": 4302, - "low": 4302, - "open": 4302, - "volume": 4302 - }, - "id": 2749565, - "non_hive": { - "close": 697, - "high": 697, - "low": 697, - "open": 697, - "volume": 697 - }, - "open": "2020-10-19T01:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1833, - "high": 1833, - "low": 1833, - "open": 1833, - "volume": 1833 - }, - "id": 2749568, - "non_hive": { - "close": 297, - "high": 297, - "low": 297, - "open": 297, - "volume": 297 - }, - "open": "2020-10-19T02:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1703, - "high": 1703, - "low": 1703, - "open": 1703, - "volume": 1703 - }, - "id": 2749572, - "non_hive": { - "close": 276, - "high": 276, - "low": 276, - "open": 276, - "volume": 276 - }, - "open": "2020-10-19T02:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 45647, - "high": 45647, - "low": 4501, - "open": 4501, - "volume": 50148 - }, - "id": 2749575, - "non_hive": { - "close": 7399, - "high": 7399, - "low": 729, - "open": 729, - "volume": 8128 - }, - "open": "2020-10-19T02:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 3387, - "high": 23894, - "low": 3387, - "open": 23894, - "volume": 27281 - }, - "id": 2749578, - "non_hive": { - "close": 549, - "high": 3873, - "low": 549, - "open": 3873, - "volume": 4422 - }, - "open": "2020-10-19T03:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 4991, - "high": 7113, - "low": 4991, - "open": 7113, - "volume": 12104 - }, - "id": 2749584, - "non_hive": { - "close": 809, - "high": 1153, - "low": 809, - "open": 1153, - "volume": 1962 - }, - "open": "2020-10-19T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2905, - "high": 2905, - "low": 2905, - "open": 2905, - "volume": 2905 - }, - "id": 2749589, - "non_hive": { - "close": 471, - "high": 471, - "low": 471, - "open": 471, - "volume": 471 - }, - "open": "2020-10-19T03:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2506, - "high": 1321, - "low": 2506, - "open": 1321, - "volume": 10000 - }, - "id": 2749592, - "non_hive": { - "close": 405, - "high": 214, - "low": 405, - "open": 214, - "volume": 1619 - }, - "open": "2020-10-19T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 37, - "high": 37, - "low": 37, - "open": 37, - "volume": 37 - }, - "id": 2749595, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-10-19T03:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12313, - "high": 12313, - "low": 12026, - "open": 12026, - "volume": 24339 - }, - "id": 2749598, - "non_hive": { - "close": 1997, - "high": 1997, - "low": 1949, - "open": 1949, - "volume": 3946 - }, - "open": "2020-10-19T03:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 6, - "high": 6, - "low": 60137, - "open": 60137, - "volume": 60143 - }, - "id": 2749601, - "non_hive": { - "close": 1, - "high": 1, - "low": 9753, - "open": 9753, - "volume": 9754 - }, - "open": "2020-10-19T03:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 9249, - "high": 17695, - "low": 9249, - "open": 17695, - "volume": 26944 - }, - "id": 2749604, - "non_hive": { - "close": 1500, - "high": 2870, - "low": 1500, - "open": 2870, - "volume": 4370 - }, - "open": "2020-10-19T04:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 16463, - "high": 16463, - "low": 16463, - "open": 16463, - "volume": 16463 - }, - "id": 2749608, - "non_hive": { - "close": 2670, - "high": 2670, - "low": 2670, - "open": 2670, - "volume": 2670 - }, - "open": "2020-10-19T04:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 11574, - "high": 11574, - "low": 11574, - "open": 11574, - "volume": 11574 - }, - "id": 2749611, - "non_hive": { - "close": 1877, - "high": 1877, - "low": 1877, - "open": 1877, - "volume": 1877 - }, - "open": "2020-10-19T04:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 2034, - "high": 530, - "low": 2034, - "open": 530, - "volume": 2564 - }, - "id": 2749614, - "non_hive": { - "close": 329, - "high": 86, - "low": 329, - "open": 86, - "volume": 415 - }, - "open": "2020-10-19T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 113424, - "high": 456, - "low": 113424, - "open": 456, - "volume": 127000 - }, - "id": 2749617, - "non_hive": { - "close": 17947, - "high": 74, - "low": 17947, - "open": 74, - "volume": 20099 - }, - "open": "2020-10-19T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 12332, - "high": 12332, - "low": 12332, - "open": 12332, - "volume": 12332 - }, - "id": 2749620, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-19T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1421, - "high": 1421, - "low": 1421, - "open": 1421, - "volume": 1421 - }, - "id": 2749623, - "non_hive": { - "close": 230, - "high": 230, - "low": 230, - "open": 230, - "volume": 230 - }, - "open": "2020-10-19T05:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 4298, - "high": 18499, - "low": 4298, - "open": 18499, - "volume": 22797 - }, - "id": 2749627, - "non_hive": { - "close": 697, - "high": 3000, - "low": 697, - "open": 3000, - "volume": 3697 - }, - "open": "2020-10-19T05:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 15425, - "high": 31202, - "low": 15425, - "open": 31202, - "volume": 46627 - }, - "id": 2749632, - "non_hive": { - "close": 2501, - "high": 5060, - "low": 2501, - "open": 5060, - "volume": 7561 - }, - "open": "2020-10-19T05:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 19985, - "high": 12496, - "low": 19985, - "open": 12496, - "volume": 32481 - }, - "id": 2749635, - "non_hive": { - "close": 3198, - "high": 2000, - "low": 3198, - "open": 2000, - "volume": 5198 - }, - "open": "2020-10-19T05:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 27938, - "high": 2005, - "low": 27938, - "open": 2005, - "volume": 34486 - }, - "id": 2749638, - "non_hive": { - "close": 4471, - "high": 325, - "low": 4471, - "open": 325, - "volume": 5532 - }, - "open": "2020-10-19T05:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 20413, - "high": 3235, - "low": 20413, - "open": 12342, - "volume": 48486 - }, - "id": 2749642, - "non_hive": { - "close": 3266, - "high": 524, - "low": 3266, - "open": 1999, - "volume": 7789 - }, - "open": "2020-10-19T05:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2537, - "high": 5297, - "low": 2537, - "open": 5297, - "volume": 32909 - }, - "id": 2749648, - "non_hive": { - "close": 406, - "high": 848, - "low": 406, - "open": 848, - "volume": 5267 - }, - "open": "2020-10-19T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 32702, - "high": 32702, - "low": 32702, - "open": 32702, - "volume": 32702 - }, - "id": 2749651, - "non_hive": { - "close": 5233, - "high": 5233, - "low": 5233, - "open": 5233, - "volume": 5233 - }, - "open": "2020-10-19T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13515, - "high": 13515, - "low": 13515, - "open": 13515, - "volume": 13515 - }, - "id": 2749654, - "non_hive": { - "close": 2163, - "high": 2163, - "low": 2163, - "open": 2163, - "volume": 2163 - }, - "open": "2020-10-19T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 17690, - "high": 1634, - "low": 17690, - "open": 1634, - "volume": 34328 - }, - "id": 2749657, - "non_hive": { - "close": 2799, - "high": 259, - "low": 2799, - "open": 259, - "volume": 5435 - }, - "open": "2020-10-19T06:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 17553, - "high": 17553, - "low": 14938, - "open": 17756, - "volume": 50247 - }, - "id": 2749663, - "non_hive": { - "close": 2780, - "high": 2780, - "low": 2363, - "open": 2810, - "volume": 7953 - }, - "open": "2020-10-19T06:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 8713, - "high": 8713, - "low": 8713, - "open": 8713, - "volume": 8713 - }, - "id": 2749668, - "non_hive": { - "close": 1411, - "high": 1411, - "low": 1411, - "open": 1411, - "volume": 1411 - }, - "open": "2020-10-19T06:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 444, - "high": 444, - "low": 6294, - "open": 8873, - "volume": 36045 - }, - "id": 2749671, - "non_hive": { - "close": 72, - "high": 72, - "low": 998, - "open": 1411, - "volume": 5730 - }, - "open": "2020-10-19T07:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 376, - "high": 376, - "low": 376, - "open": 376, - "volume": 376 - }, - "id": 2749677, - "non_hive": { - "close": 61, - "high": 61, - "low": 61, - "open": 61, - "volume": 61 - }, - "open": "2020-10-19T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 27000, - "high": 27000, - "low": 27000, - "open": 27000, - "volume": 27000 - }, - "id": 2749680, - "non_hive": { - "close": 4372, - "high": 4372, - "low": 4372, - "open": 4372, - "volume": 4372 - }, - "open": "2020-10-19T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2811, - "high": 2811, - "low": 2811, - "open": 2811, - "volume": 2811 - }, - "id": 2749683, - "non_hive": { - "close": 455, - "high": 455, - "low": 455, - "open": 455, - "volume": 455 - }, - "open": "2020-10-19T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 65647, - "high": 65647, - "low": 103380, - "open": 103380, - "volume": 169027 - }, - "id": 2749686, - "non_hive": { - "close": 10631, - "high": 10631, - "low": 16741, - "open": 16741, - "volume": 27372 - }, - "open": "2020-10-19T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3607, - "high": 172, - "low": 491, - "open": 172, - "volume": 11607 - }, - "id": 2749690, - "non_hive": { - "close": 584, - "high": 28, - "low": 79, - "open": 28, - "volume": 1879 - }, - "open": "2020-10-19T07:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 2520, - "high": 2520, - "low": 2520, - "open": 2520, - "volume": 2520 - }, - "id": 2749696, - "non_hive": { - "close": 408, - "high": 408, - "low": 408, - "open": 408, - "volume": 408 - }, - "open": "2020-10-19T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 62300, - "high": 35168, - "low": 62300, - "open": 35168, - "volume": 97468 - }, - "id": 2749699, - "non_hive": { - "close": 9968, - "high": 5627, - "low": 9968, - "open": 5627, - "volume": 15595 - }, - "open": "2020-10-19T07:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 38223, - "high": 3700, - "low": 38223, - "open": 3700, - "volume": 98581 - }, - "id": 2749702, - "non_hive": { - "close": 6061, - "high": 588, - "low": 6061, - "open": 588, - "volume": 15649 - }, - "open": "2020-10-19T07:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12484, - "high": 12484, - "low": 12484, - "open": 12484, - "volume": 12484 - }, - "id": 2749708, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-19T08:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 14138, - "high": 14138, - "low": 14138, - "open": 14138, - "volume": 14138 - }, - "id": 2749712, - "non_hive": { - "close": 2265, - "high": 2265, - "low": 2265, - "open": 2265, - "volume": 2265 - }, - "open": "2020-10-19T08:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6343, - "high": 6343, - "low": 6343, - "open": 6343, - "volume": 6343 - }, - "id": 2749715, - "non_hive": { - "close": 1006, - "high": 1006, - "low": 1006, - "open": 1006, - "volume": 1006 - }, - "open": "2020-10-19T08:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 100000, - "high": 100000, - "low": 11291, - "open": 11291, - "volume": 111291 - }, - "id": 2749718, - "non_hive": { - "close": 16020, - "high": 16020, - "low": 1790, - "open": 1790, - "volume": 17810 - }, - "open": "2020-10-19T08:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 2010, - "high": 14936, - "low": 2010, - "open": 14936, - "volume": 16946 - }, - "id": 2749721, - "non_hive": { - "close": 322, - "high": 2393, - "low": 322, - "open": 2393, - "volume": 2715 - }, - "open": "2020-10-19T09:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 4744, - "high": 12510, - "low": 4744, - "open": 12510, - "volume": 17254 - }, - "id": 2749725, - "non_hive": { - "close": 757, - "high": 1999, - "low": 757, - "open": 1999, - "volume": 2756 - }, - "open": "2020-10-19T09:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6942, - "high": 6942, - "low": 6942, - "open": 6942, - "volume": 6942 - }, - "id": 2749728, - "non_hive": { - "close": 1112, - "high": 1112, - "low": 1112, - "open": 1112, - "volume": 1112 - }, - "open": "2020-10-19T09:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 15617, - "high": 15617, - "low": 5538, - "open": 5538, - "volume": 52791 - }, - "id": 2749731, - "non_hive": { - "close": 2503, - "high": 2503, - "low": 887, - "open": 887, - "volume": 8460 - }, - "open": "2020-10-19T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 42016, - "high": 755, - "low": 330, - "open": 755, - "volume": 99902 - }, - "id": 2749734, - "non_hive": { - "close": 6713, - "high": 121, - "low": 52, - "open": 121, - "volume": 15984 - }, - "open": "2020-10-19T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 15772, - "high": 15772, - "low": 15772, - "open": 15772, - "volume": 15772 - }, - "id": 2749739, - "non_hive": { - "close": 2525, - "high": 2525, - "low": 2525, - "open": 2525, - "volume": 2525 - }, - "open": "2020-10-19T10:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 2710, - "high": 53231, - "low": 2710, - "open": 110084, - "volume": 210084 - }, - "id": 2749742, - "non_hive": { - "close": 429, - "high": 8527, - "low": 429, - "open": 17634, - "volume": 33616 - }, - "open": "2020-10-19T10:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 63765, - "high": 63765, - "low": 63765, - "open": 63765, - "volume": 63765 - }, - "id": 2749747, - "non_hive": { - "close": 10214, - "high": 10214, - "low": 10214, - "open": 10214, - "volume": 10214 - }, - "open": "2020-10-19T11:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 3864, - "high": 3864, - "low": 12486, - "open": 12486, - "volume": 16350 - }, - "id": 2749751, - "non_hive": { - "close": 619, - "high": 619, - "low": 1999, - "open": 1999, - "volume": 2618 - }, - "open": "2020-10-19T11:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 7257, - "high": 7257, - "low": 7257, - "open": 7257, - "volume": 7257 - }, - "id": 2749754, - "non_hive": { - "close": 1162, - "high": 1162, - "low": 1162, - "open": 1162, - "volume": 1162 - }, - "open": "2020-10-19T11:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 9252, - "high": 9252, - "low": 5229, - "open": 5229, - "volume": 23471 - }, - "id": 2749757, - "non_hive": { - "close": 1482, - "high": 1482, - "low": 837, - "open": 837, - "volume": 3759 - }, - "open": "2020-10-19T11:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 299, - "high": 299, - "low": 14698, - "open": 14698, - "volume": 14997 - }, - "id": 2749762, - "non_hive": { - "close": 48, - "high": 48, - "low": 2353, - "open": 2353, - "volume": 2401 - }, - "open": "2020-10-19T11:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 5346, - "high": 5346, - "low": 5346, - "open": 5346, - "volume": 5346 - }, - "id": 2749767, - "non_hive": { - "close": 856, - "high": 856, - "low": 856, - "open": 856, - "volume": 856 - }, - "open": "2020-10-19T11:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 2905, - "high": 2905, - "low": 2905, - "open": 2905, - "volume": 2905 - }, - "id": 2749770, - "non_hive": { - "close": 465, - "high": 465, - "low": 465, - "open": 465, - "volume": 465 - }, - "open": "2020-10-19T11:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 10514, - "high": 106, - "low": 10514, - "open": 136664, - "volume": 280658 - }, - "id": 2749773, - "non_hive": { - "close": 1683, - "high": 17, - "low": 1683, - "open": 21890, - "volume": 44951 - }, - "open": "2020-10-19T12:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 39826, - "high": 174, - "low": 39826, - "open": 174, - "volume": 40000 - }, - "id": 2749780, - "non_hive": { - "close": 6374, - "high": 28, - "low": 6374, - "open": 28, - "volume": 6402 - }, - "open": "2020-10-19T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 11917, - "high": 11917, - "low": 11917, - "open": 11917, - "volume": 11917 - }, - "id": 2749783, - "non_hive": { - "close": 1910, - "high": 1910, - "low": 1910, - "open": 1910, - "volume": 1910 - }, - "open": "2020-10-19T12:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 13095, - "high": 13095, - "low": 1581, - "open": 1581, - "volume": 14676 - }, - "id": 2749786, - "non_hive": { - "close": 2120, - "high": 2120, - "low": 253, - "open": 253, - "volume": 2373 - }, - "open": "2020-10-19T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 456, - "high": 456, - "low": 24698, - "open": 24698, - "volume": 25154 - }, - "id": 2749791, - "non_hive": { - "close": 74, - "high": 74, - "low": 4000, - "open": 4000, - "volume": 4074 - }, - "open": "2020-10-19T12:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 24, - "high": 24, - "low": 51320, - "open": 22048, - "volume": 139642 - }, - "id": 2749796, - "non_hive": { - "close": 4, - "high": 4, - "low": 8311, - "open": 3571, - "volume": 22616 - }, - "open": "2020-10-19T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12251, - "high": 4054, - "low": 12251, - "open": 4054, - "volume": 22054 - }, - "id": 2749803, - "non_hive": { - "close": 1960, - "high": 656, - "low": 1960, - "open": 656, - "volume": 3546 - }, - "open": "2020-10-19T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 66201, - "high": 66201, - "low": 8302, - "open": 8302, - "volume": 101835 - }, - "id": 2749809, - "non_hive": { - "close": 10722, - "high": 10722, - "low": 1343, - "open": 1343, - "volume": 16488 - }, - "open": "2020-10-19T13:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3319, - "high": 3319, - "low": 3319, - "open": 3319, - "volume": 3319 - }, - "id": 2749814, - "non_hive": { - "close": 537, - "high": 537, - "low": 537, - "open": 537, - "volume": 537 - }, - "open": "2020-10-19T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 40342, - "high": 40342, - "low": 9037, - "open": 9037, - "volume": 49379 - }, - "id": 2749817, - "non_hive": { - "close": 6530, - "high": 6530, - "low": 1462, - "open": 1462, - "volume": 7992 - }, - "open": "2020-10-19T14:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2459, - "high": 2459, - "low": 2459, - "open": 2459, - "volume": 2459 - }, - "id": 2749821, - "non_hive": { - "close": 398, - "high": 398, - "low": 398, - "open": 398, - "volume": 398 - }, - "open": "2020-10-19T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 37070, - "high": 37070, - "low": 37070, - "open": 37070, - "volume": 37070 - }, - "id": 2749824, - "non_hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 6000 - }, - "open": "2020-10-19T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 38584, - "high": 38584, - "low": 38584, - "open": 38584, - "volume": 38584 - }, - "id": 2749827, - "non_hive": { - "close": 6245, - "high": 6245, - "low": 6245, - "open": 6245, - "volume": 6245 - }, - "open": "2020-10-19T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 30402, - "high": 30402, - "low": 30402, - "open": 30402, - "volume": 30402 - }, - "id": 2749830, - "non_hive": { - "close": 4921, - "high": 4921, - "low": 4921, - "open": 4921, - "volume": 4921 - }, - "open": "2020-10-19T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12665, - "high": 12665, - "low": 12665, - "open": 12665, - "volume": 12665 - }, - "id": 2749833, - "non_hive": { - "close": 2050, - "high": 2050, - "low": 2050, - "open": 2050, - "volume": 2050 - }, - "open": "2020-10-19T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1975, - "high": 23767, - "low": 1975, - "open": 23767, - "volume": 153377 - }, - "id": 2749836, - "non_hive": { - "close": 319, - "high": 3847, - "low": 319, - "open": 3847, - "volume": 24824 - }, - "open": "2020-10-19T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 5000, - "high": 1050, - "low": 5506, - "open": 1050, - "volume": 99583 - }, - "id": 2749841, - "non_hive": { - "close": 809, - "high": 170, - "low": 881, - "open": 170, - "volume": 15963 - }, - "open": "2020-10-19T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1583, - "high": 37079, - "low": 1583, - "open": 12356, - "volume": 51018 - }, - "id": 2749849, - "non_hive": { - "close": 256, - "high": 6001, - "low": 256, - "open": 1999, - "volume": 8256 - }, - "open": "2020-10-19T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 123782, - "high": 123782, - "low": 123782, - "open": 123782, - "volume": 123782 - }, - "id": 2749857, - "non_hive": { - "close": 20000, - "high": 20000, - "low": 20000, - "open": 20000, - "volume": 20000 - }, - "open": "2020-10-19T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6038, - "high": 174, - "low": 6038, - "open": 174, - "volume": 6344 - }, - "id": 2749860, - "non_hive": { - "close": 957, - "high": 28, - "low": 957, - "open": 28, - "volume": 1006 - }, - "open": "2020-10-19T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 12356, - "high": 12356, - "low": 12356, - "open": 12356, - "volume": 12356 - }, - "id": 2749863, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-19T15:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 11489, - "high": 12356, - "low": 11489, - "open": 12356, - "volume": 23845 - }, - "id": 2749866, - "non_hive": { - "close": 1840, - "high": 1999, - "low": 1840, - "open": 1999, - "volume": 3839 - }, - "open": "2020-10-19T15:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 14473, - "high": 14473, - "low": 14473, - "open": 14473, - "volume": 14473 - }, - "id": 2749871, - "non_hive": { - "close": 2316, - "high": 2316, - "low": 2316, - "open": 2316, - "volume": 2316 - }, - "open": "2020-10-19T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 71837, - "high": 71837, - "low": 12492, - "open": 12492, - "volume": 84329 - }, - "id": 2749874, - "non_hive": { - "close": 11501, - "high": 11501, - "low": 1999, - "open": 1999, - "volume": 13500 - }, - "open": "2020-10-19T15:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 17505, - "high": 6796, - "low": 553, - "open": 9124, - "volume": 41845 - }, - "id": 2749877, - "non_hive": { - "close": 2801, - "high": 1088, - "low": 88, - "open": 1460, - "volume": 6696 - }, - "open": "2020-10-19T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 53153, - "high": 53153, - "low": 53153, - "open": 53153, - "volume": 53153 - }, - "id": 2749882, - "non_hive": { - "close": 8505, - "high": 8505, - "low": 8505, - "open": 8505, - "volume": 8505 - }, - "open": "2020-10-19T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 14813, - "high": 10237, - "low": 2263, - "open": 10237, - "volume": 27313 - }, - "id": 2749885, - "non_hive": { - "close": 2370, - "high": 1638, - "low": 362, - "open": 1638, - "volume": 4370 - }, - "open": "2020-10-19T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 18124, - "high": 18124, - "low": 18124, - "open": 18124, - "volume": 18124 - }, - "id": 2749892, - "non_hive": { - "close": 2900, - "high": 2900, - "low": 2900, - "open": 2900, - "volume": 2900 - }, - "open": "2020-10-19T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 4582, - "high": 4582, - "low": 4582, - "open": 4582, - "volume": 4582 - }, - "id": 2749895, - "non_hive": { - "close": 733, - "high": 733, - "low": 733, - "open": 733, - "volume": 733 - }, - "open": "2020-10-19T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 35000, - "high": 35000, - "low": 8264, - "open": 8264, - "volume": 43264 - }, - "id": 2749898, - "non_hive": { - "close": 5599, - "high": 5599, - "low": 1322, - "open": 1322, - "volume": 6921 - }, - "open": "2020-10-19T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 122998, - "high": 162, - "low": 122998, - "open": 162, - "volume": 125879 - }, - "id": 2749903, - "non_hive": { - "close": 19676, - "high": 26, - "low": 19676, - "open": 26, - "volume": 20137 - }, - "open": "2020-10-19T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 237, - "high": 237, - "low": 26275, - "open": 26275, - "volume": 26512 - }, - "id": 2749909, - "non_hive": { - "close": 38, - "high": 38, - "low": 4203, - "open": 4203, - "volume": 4241 - }, - "open": "2020-10-19T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6249, - "high": 6249, - "low": 6249, - "open": 6249, - "volume": 6249 - }, - "id": 2749913, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-19T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 8405, - "high": 8405, - "low": 8405, - "open": 8405, - "volume": 8405 - }, - "id": 2749917, - "non_hive": { - "close": 1345, - "high": 1345, - "low": 1345, - "open": 1345, - "volume": 1345 - }, - "open": "2020-10-19T17:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 93, - "high": 93, - "low": 93, - "open": 93, - "volume": 93 - }, - "id": 2749920, - "non_hive": { - "close": 15, - "high": 15, - "low": 15, - "open": 15, - "volume": 15 - }, - "open": "2020-10-19T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 2523, - "high": 2523, - "low": 2523, - "open": 2523, - "volume": 2523 - }, - "id": 2749923, - "non_hive": { - "close": 404, - "high": 404, - "low": 404, - "open": 404, - "volume": 404 - }, - "open": "2020-10-19T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 100319, - "high": 39251, - "low": 100319, - "open": 39251, - "volume": 505514 - }, - "id": 2749926, - "non_hive": { - "close": 16051, - "high": 6284, - "low": 16051, - "open": 6284, - "volume": 80890 - }, - "open": "2020-10-19T17:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 11912, - "high": 5938, - "low": 11912, - "open": 5938, - "volume": 1147565 - }, - "id": 2749929, - "non_hive": { - "close": 1885, - "high": 950, - "low": 1885, - "open": 950, - "volume": 182103 - }, - "open": "2020-10-19T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 27873, - "high": 2699, - "low": 27873, - "open": 2699, - "volume": 30572 - }, - "id": 2749937, - "non_hive": { - "close": 4460, - "high": 432, - "low": 4460, - "open": 432, - "volume": 4892 - }, - "open": "2020-10-19T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13916, - "high": 13916, - "low": 13916, - "open": 13916, - "volume": 13916 - }, - "id": 2749940, - "non_hive": { - "close": 2228, - "high": 2228, - "low": 2228, - "open": 2228, - "volume": 2228 - }, - "open": "2020-10-19T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6421, - "high": 6421, - "low": 6421, - "open": 6421, - "volume": 6421 - }, - "id": 2749943, - "non_hive": { - "close": 1028, - "high": 1028, - "low": 1028, - "open": 1028, - "volume": 1028 - }, - "open": "2020-10-19T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 6381, - "high": 2699, - "low": 611, - "open": 2699, - "volume": 15802 - }, - "id": 2749946, - "non_hive": { - "close": 1021, - "high": 432, - "low": 97, - "open": 432, - "volume": 2528 - }, - "open": "2020-10-19T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 8198, - "high": 8198, - "low": 8198, - "open": 8198, - "volume": 8198 - }, - "id": 2749951, - "non_hive": { - "close": 1312, - "high": 1312, - "low": 1312, - "open": 1312, - "volume": 1312 - }, - "open": "2020-10-19T18:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 14585, - "high": 14585, - "low": 14585, - "open": 14585, - "volume": 14585 - }, - "id": 2749954, - "non_hive": { - "close": 2335, - "high": 2335, - "low": 2335, - "open": 2335, - "volume": 2335 - }, - "open": "2020-10-19T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11581, - "high": 943, - "low": 11581, - "open": 4797, - "volume": 17321 - }, - "id": 2749957, - "non_hive": { - "close": 1854, - "high": 151, - "low": 1854, - "open": 768, - "volume": 2773 - }, - "open": "2020-10-19T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 18357, - "high": 18357, - "low": 1643, - "open": 1643, - "volume": 20000 - }, - "id": 2749964, - "non_hive": { - "close": 2939, - "high": 2939, - "low": 263, - "open": 263, - "volume": 3202 - }, - "open": "2020-10-19T19:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 14366, - "high": 14366, - "low": 14366, - "open": 14366, - "volume": 14366 - }, - "id": 2749967, - "non_hive": { - "close": 2300, - "high": 2300, - "low": 2300, - "open": 2300, - "volume": 2300 - }, - "open": "2020-10-19T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 22298, - "high": 22298, - "low": 3555, - "open": 3555, - "volume": 25853 - }, - "id": 2749970, - "non_hive": { - "close": 3570, - "high": 3570, - "low": 569, - "open": 569, - "volume": 4139 - }, - "open": "2020-10-19T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 19326, - "high": 1061, - "low": 19326, - "open": 6250, - "volume": 90065 - }, - "id": 2749975, - "non_hive": { - "close": 3072, - "high": 170, - "low": 3072, - "open": 1000, - "volume": 14354 - }, - "open": "2020-10-19T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12493, - "high": 12493, - "low": 12493, - "open": 12493, - "volume": 12493 - }, - "id": 2749985, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-19T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 41131, - "high": 41131, - "low": 41131, - "open": 41131, - "volume": 41131 - }, - "id": 2749988, - "non_hive": { - "close": 6584, - "high": 6584, - "low": 6584, - "open": 6584, - "volume": 6584 - }, - "open": "2020-10-19T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6222, - "high": 106184, - "low": 6222, - "open": 106184, - "volume": 144950 - }, - "id": 2749991, - "non_hive": { - "close": 996, - "high": 17000, - "low": 996, - "open": 17000, - "volume": 23206 - }, - "open": "2020-10-19T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 56237, - "high": 12535, - "low": 56237, - "open": 33471, - "volume": 329978 - }, - "id": 2749997, - "non_hive": { - "close": 8998, - "high": 2007, - "low": 8998, - "open": 5358, - "volume": 52818 - }, - "open": "2020-10-19T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 61046, - "high": 61046, - "low": 31586, - "open": 31586, - "volume": 124218 - }, - "id": 2750002, - "non_hive": { - "close": 9878, - "high": 9878, - "low": 5061, - "open": 5061, - "volume": 20000 - }, - "open": "2020-10-19T20:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16377, - "high": 16377, - "low": 343, - "open": 343, - "volume": 20250 - }, - "id": 2750005, - "non_hive": { - "close": 2650, - "high": 2650, - "low": 55, - "open": 55, - "volume": 3276 - }, - "open": "2020-10-19T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 458, - "high": 18542, - "low": 458, - "open": 18542, - "volume": 19000 - }, - "id": 2750008, - "non_hive": { - "close": 73, - "high": 3000, - "low": 73, - "open": 3000, - "volume": 3073 - }, - "open": "2020-10-19T20:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 12051, - "high": 12051, - "low": 458, - "open": 458, - "volume": 12509 - }, - "id": 2750011, - "non_hive": { - "close": 1950, - "high": 1950, - "low": 74, - "open": 74, - "volume": 2024 - }, - "open": "2020-10-19T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 3936, - "high": 3936, - "low": 3936, - "open": 3936, - "volume": 3936 - }, - "id": 2750015, - "non_hive": { - "close": 637, - "high": 637, - "low": 637, - "open": 637, - "volume": 637 - }, - "open": "2020-10-19T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 468, - "high": 4753, - "low": 468, - "open": 4753, - "volume": 25345 - }, - "id": 2750018, - "non_hive": { - "close": 75, - "high": 769, - "low": 75, - "open": 769, - "volume": 4071 - }, - "open": "2020-10-19T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2707, - "high": 2707, - "low": 2707, - "open": 2707, - "volume": 2707 - }, - "id": 2750025, - "non_hive": { - "close": 438, - "high": 438, - "low": 438, - "open": 438, - "volume": 438 - }, - "open": "2020-10-19T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 29629, - "high": 29629, - "low": 9309, - "open": 9309, - "volume": 38938 - }, - "id": 2750028, - "non_hive": { - "close": 4794, - "high": 4794, - "low": 1506, - "open": 1506, - "volume": 6300 - }, - "open": "2020-10-19T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 18544, - "high": 24403, - "low": 18544, - "open": 24403, - "volume": 42947 - }, - "id": 2750032, - "non_hive": { - "close": 3000, - "high": 3948, - "low": 3000, - "open": 3948, - "volume": 6948 - }, - "open": "2020-10-19T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 11769, - "high": 11769, - "low": 11769, - "open": 11769, - "volume": 11769 - }, - "id": 2750035, - "non_hive": { - "close": 1904, - "high": 1904, - "low": 1904, - "open": 1904, - "volume": 1904 - }, - "open": "2020-10-19T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 284, - "high": 284, - "low": 287, - "open": 1632, - "volume": 141507 - }, - "id": 2750038, - "non_hive": { - "close": 46, - "high": 46, - "low": 45, - "open": 264, - "volume": 22510 - }, - "open": "2020-10-19T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 9524, - "high": 12577, - "low": 9524, - "open": 12577, - "volume": 1632954 - }, - "id": 2750048, - "non_hive": { - "close": 1457, - "high": 2000, - "low": 1457, - "open": 2000, - "volume": 254521 - }, - "open": "2020-10-19T22:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 36287, - "high": 36287, - "low": 12364, - "open": 12364, - "volume": 48651 - }, - "id": 2750055, - "non_hive": { - "close": 5870, - "high": 5870, - "low": 1999, - "open": 1999, - "volume": 7869 - }, - "open": "2020-10-19T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 104498, - "high": 12998, - "low": 104498, - "open": 12998, - "volume": 198867 - }, - "id": 2750059, - "non_hive": { - "close": 15991, - "high": 2000, - "low": 15991, - "open": 2000, - "volume": 30510 - }, - "open": "2020-10-19T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4088, - "high": 4088, - "low": 4088, - "open": 4088, - "volume": 4088 - }, - "id": 2750065, - "non_hive": { - "close": 658, - "high": 658, - "low": 658, - "open": 658, - "volume": 658 - }, - "open": "2020-10-19T23:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 529, - "high": 529, - "low": 529, - "open": 529, - "volume": 529 - }, - "id": 2750068, - "non_hive": { - "close": 85, - "high": 85, - "low": 85, - "open": 85, - "volume": 85 - }, - "open": "2020-10-19T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 31827, - "high": 31827, - "low": 12502, - "open": 12502, - "volume": 44329 - }, - "id": 2750071, - "non_hive": { - "close": 5091, - "high": 5091, - "low": 1999, - "open": 1999, - "volume": 7090 - }, - "open": "2020-10-20T00:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4364, - "high": 37, - "low": 18988, - "open": 12839, - "volume": 49855 - }, - "id": 2750077, - "non_hive": { - "close": 698, - "high": 6, - "low": 3037, - "open": 2054, - "volume": 7975 - }, - "open": "2020-10-20T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 132, - "high": 132, - "low": 132, - "open": 132, - "volume": 132 - }, - "id": 2750083, - "non_hive": { - "close": 21, - "high": 21, - "low": 21, - "open": 21, - "volume": 21 - }, - "open": "2020-10-20T00:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 21671, - "high": 21671, - "low": 12628, - "open": 12628, - "volume": 34299 - }, - "id": 2750086, - "non_hive": { - "close": 3432, - "high": 3432, - "low": 1999, - "open": 1999, - "volume": 5431 - }, - "open": "2020-10-20T00:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 537, - "high": 537, - "low": 537, - "open": 537, - "volume": 537 - }, - "id": 2750089, - "non_hive": { - "close": 85, - "high": 85, - "low": 85, - "open": 85, - "volume": 85 - }, - "open": "2020-10-20T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 7000, - "high": 7000, - "low": 12974, - "open": 12974, - "volume": 45922 - }, - "id": 2750092, - "non_hive": { - "close": 1082, - "high": 1082, - "low": 2000, - "open": 2000, - "volume": 7082 - }, - "open": "2020-10-20T00:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 7258, - "high": 7258, - "low": 7258, - "open": 7258, - "volume": 7258 - }, - "id": 2750100, - "non_hive": { - "close": 1148, - "high": 1148, - "low": 1148, - "open": 1148, - "volume": 1148 - }, - "open": "2020-10-20T01:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 105326, - "high": 11667, - "low": 105326, - "open": 11667, - "volume": 128962 - }, - "id": 2750104, - "non_hive": { - "close": 16234, - "high": 1844, - "low": 16234, - "open": 1844, - "volume": 19923 - }, - "open": "2020-10-20T01:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 14471, - "high": 5346, - "low": 14471, - "open": 5346, - "volume": 101179 - }, - "id": 2750109, - "non_hive": { - "close": 2287, - "high": 845, - "low": 2287, - "open": 845, - "volume": 15991 - }, - "open": "2020-10-20T01:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 2391, - "high": 2391, - "low": 2391, - "open": 2391, - "volume": 2391 - }, - "id": 2750114, - "non_hive": { - "close": 378, - "high": 378, - "low": 378, - "open": 378, - "volume": 378 - }, - "open": "2020-10-20T02:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 34261, - "high": 34261, - "low": 50477, - "open": 7989, - "volume": 356147 - }, - "id": 2750118, - "non_hive": { - "close": 5426, - "high": 5426, - "low": 7723, - "open": 1223, - "volume": 54829 - }, - "open": "2020-10-20T02:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12484, - "high": 10593, - "low": 61407, - "open": 10593, - "volume": 349876 - }, - "id": 2750124, - "non_hive": { - "close": 1910, - "high": 1621, - "low": 9395, - "open": 1621, - "volume": 53531 - }, - "open": "2020-10-20T02:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 10702, - "high": 10702, - "low": 10702, - "open": 10702, - "volume": 10702 - }, - "id": 2750130, - "non_hive": { - "close": 1694, - "high": 1694, - "low": 1694, - "open": 1694, - "volume": 1694 - }, - "open": "2020-10-20T02:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6917, - "high": 10845, - "low": 6917, - "open": 10845, - "volume": 17762 - }, - "id": 2750133, - "non_hive": { - "close": 1092, - "high": 1713, - "low": 1092, - "open": 1713, - "volume": 2805 - }, - "open": "2020-10-20T02:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 633, - "high": 6, - "low": 44, - "open": 6, - "volume": 1785 - }, - "id": 2750138, - "non_hive": { - "close": 100, - "high": 1, - "low": 6, - "open": 1, - "volume": 281 - }, - "open": "2020-10-20T02:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 10159, - "high": 10159, - "low": 10159, - "open": 10159, - "volume": 10159 - }, - "id": 2750144, - "non_hive": { - "close": 1604, - "high": 1604, - "low": 1604, - "open": 1604, - "volume": 1604 - }, - "open": "2020-10-20T03:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 10234, - "high": 10234, - "low": 13071, - "open": 13071, - "volume": 23305 - }, - "id": 2750148, - "non_hive": { - "close": 1566, - "high": 1566, - "low": 2000, - "open": 2000, - "volume": 3566 - }, - "open": "2020-10-20T03:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 5152, - "high": 5152, - "low": 12739, - "open": 12739, - "volume": 17891 - }, - "id": 2750153, - "non_hive": { - "close": 809, - "high": 809, - "low": 1999, - "open": 1999, - "volume": 2808 - }, - "open": "2020-10-20T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 65518, - "high": 65518, - "low": 65518, - "open": 65518, - "volume": 65518 - }, - "id": 2750156, - "non_hive": { - "close": 10281, - "high": 10281, - "low": 10281, - "open": 10281, - "volume": 10281 - }, - "open": "2020-10-20T03:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 15171, - "high": 13064, - "low": 15171, - "open": 13064, - "volume": 28235 - }, - "id": 2750159, - "non_hive": { - "close": 2321, - "high": 1999, - "low": 2321, - "open": 1999, - "volume": 4320 - }, - "open": "2020-10-20T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 61934, - "high": 61934, - "low": 12746, - "open": 12746, - "volume": 74680 - }, - "id": 2750164, - "non_hive": { - "close": 9718, - "high": 9718, - "low": 1999, - "open": 1999, - "volume": 11717 - }, - "open": "2020-10-20T03:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 95182, - "high": 95182, - "low": 12746, - "open": 12746, - "volume": 107928 - }, - "id": 2750167, - "non_hive": { - "close": 14935, - "high": 14935, - "low": 1999, - "open": 1999, - "volume": 16934 - }, - "open": "2020-10-20T03:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 726, - "high": 726, - "low": 12746, - "open": 12746, - "volume": 13472 - }, - "id": 2750170, - "non_hive": { - "close": 114, - "high": 114, - "low": 1999, - "open": 1999, - "volume": 2113 - }, - "open": "2020-10-20T03:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 12747, - "high": 12747, - "low": 12747, - "open": 12747, - "volume": 12747 - }, - "id": 2750173, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-20T04:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 10070, - "high": 10070, - "low": 10070, - "open": 10070, - "volume": 10070 - }, - "id": 2750177, - "non_hive": { - "close": 1580, - "high": 1580, - "low": 1580, - "open": 1580, - "volume": 1580 - }, - "open": "2020-10-20T05:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 23939, - "high": 23939, - "low": 23939, - "open": 23939, - "volume": 23939 - }, - "id": 2750181, - "non_hive": { - "close": 3756, - "high": 3756, - "low": 3756, - "open": 3756, - "volume": 3756 - }, - "open": "2020-10-20T05:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 22416, - "high": 22416, - "low": 22416, - "open": 22416, - "volume": 22416 - }, - "id": 2750184, - "non_hive": { - "close": 3517, - "high": 3517, - "low": 3517, - "open": 3517, - "volume": 3517 - }, - "open": "2020-10-20T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 20460, - "high": 19364, - "low": 20460, - "open": 19364, - "volume": 93141 - }, - "id": 2750187, - "non_hive": { - "close": 3208, - "high": 3038, - "low": 3208, - "open": 3038, - "volume": 14609 - }, - "open": "2020-10-20T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 11263, - "high": 11263, - "low": 11263, - "open": 11263, - "volume": 11263 - }, - "id": 2750190, - "non_hive": { - "close": 1766, - "high": 1766, - "low": 1766, - "open": 1766, - "volume": 1766 - }, - "open": "2020-10-20T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 976, - "high": 31986, - "low": 976, - "open": 31986, - "volume": 64121 - }, - "id": 2750194, - "non_hive": { - "close": 153, - "high": 5015, - "low": 153, - "open": 5015, - "volume": 10053 - }, - "open": "2020-10-20T06:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6106, - "high": 50995, - "low": 12747, - "open": 12747, - "volume": 69848 - }, - "id": 2750199, - "non_hive": { - "close": 958, - "high": 8001, - "low": 1999, - "open": 1999, - "volume": 10958 - }, - "open": "2020-10-20T07:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6323, - "high": 23570, - "low": 12748, - "open": 23570, - "volume": 53768 - }, - "id": 2750205, - "non_hive": { - "close": 992, - "high": 3698, - "low": 1999, - "open": 3698, - "volume": 8434 - }, - "open": "2020-10-20T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 832, - "high": 22168, - "low": 832, - "open": 22168, - "volume": 23000 - }, - "id": 2750210, - "non_hive": { - "close": 130, - "high": 3476, - "low": 130, - "open": 3476, - "volume": 3606 - }, - "open": "2020-10-20T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 766, - "high": 19234, - "low": 766, - "open": 19234, - "volume": 20000 - }, - "id": 2750213, - "non_hive": { - "close": 120, - "high": 3016, - "low": 120, - "open": 3016, - "volume": 3136 - }, - "open": "2020-10-20T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1632, - "high": 1632, - "low": 1632, - "open": 1632, - "volume": 1632 - }, - "id": 2750216, - "non_hive": { - "close": 256, - "high": 256, - "low": 256, - "open": 256, - "volume": 256 - }, - "open": "2020-10-20T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6378, - "high": 6, - "low": 6378, - "open": 6, - "volume": 6390 - }, - "id": 2750219, - "non_hive": { - "close": 1000, - "high": 1, - "low": 1000, - "open": 1, - "volume": 1002 - }, - "open": "2020-10-20T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 268, - "high": 63, - "low": 268, - "open": 63, - "volume": 1051 - }, - "id": 2750224, - "non_hive": { - "close": 42, - "high": 10, - "low": 42, - "open": 10, - "volume": 165 - }, - "open": "2020-10-20T07:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1258, - "high": 779, - "low": 1258, - "open": 779, - "volume": 2037 - }, - "id": 2750227, - "non_hive": { - "close": 197, - "high": 122, - "low": 197, - "open": 122, - "volume": 319 - }, - "open": "2020-10-20T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 15479, - "high": 13, - "low": 15479, - "open": 13, - "volume": 54067 - }, - "id": 2750230, - "non_hive": { - "close": 2372, - "high": 2, - "low": 2372, - "open": 2, - "volume": 8289 - }, - "open": "2020-10-20T07:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 12838, - "high": 182961, - "low": 177, - "open": 471, - "volume": 235002 - }, - "id": 2750237, - "non_hive": { - "close": 2000, - "high": 28703, - "low": 27, - "open": 72, - "volume": 36801 - }, - "open": "2020-10-20T08:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12833, - "high": 307, - "low": 12526, - "open": 307, - "volume": 25973 - }, - "id": 2750247, - "non_hive": { - "close": 2000, - "high": 48, - "low": 1952, - "open": 48, - "volume": 4048 - }, - "open": "2020-10-20T08:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 51022, - "high": 51022, - "low": 35403, - "open": 35403, - "volume": 86425 - }, - "id": 2750253, - "non_hive": { - "close": 8000, - "high": 8000, - "low": 5551, - "open": 5551, - "volume": 13551 - }, - "open": "2020-10-20T08:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6087, - "high": 44918, - "low": 12756, - "open": 12756, - "volume": 63761 - }, - "id": 2750258, - "non_hive": { - "close": 954, - "high": 7043, - "low": 1999, - "open": 1999, - "volume": 9996 - }, - "open": "2020-10-20T08:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 9420, - "high": 9420, - "low": 5114, - "open": 12845, - "volume": 35111 - }, - "id": 2750263, - "non_hive": { - "close": 1476, - "high": 1476, - "low": 796, - "open": 2000, - "volume": 5476 - }, - "open": "2020-10-20T08:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 34634, - "high": 15366, - "low": 34634, - "open": 15366, - "volume": 50000 - }, - "id": 2750269, - "non_hive": { - "close": 5298, - "high": 2351, - "low": 5298, - "open": 2351, - "volume": 7649 - }, - "open": "2020-10-20T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 4500, - "high": 7635, - "low": 4500, - "open": 12765, - "volume": 28245 - }, - "id": 2750272, - "non_hive": { - "close": 704, - "high": 1197, - "low": 704, - "open": 1999, - "volume": 4424 - }, - "open": "2020-10-20T08:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1034, - "high": 1034, - "low": 1034, - "open": 1034, - "volume": 1034 - }, - "id": 2750277, - "non_hive": { - "close": 162, - "high": 162, - "low": 162, - "open": 162, - "volume": 162 - }, - "open": "2020-10-20T08:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 11000, - "high": 30747, - "low": 11000, - "open": 30747, - "volume": 41747 - }, - "id": 2750280, - "non_hive": { - "close": 1723, - "high": 4817, - "low": 1723, - "open": 4817, - "volume": 6540 - }, - "open": "2020-10-20T09:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 57448, - "high": 57448, - "low": 57448, - "open": 57448, - "volume": 57448 - }, - "id": 2750286, - "non_hive": { - "close": 9000, - "high": 9000, - "low": 9000, - "open": 9000, - "volume": 9000 - }, - "open": "2020-10-20T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 4762, - "high": 12, - "low": 4762, - "open": 12, - "volume": 22973 - }, - "id": 2750289, - "non_hive": { - "close": 746, - "high": 2, - "low": 746, - "open": 2, - "volume": 3599 - }, - "open": "2020-10-20T09:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 10950, - "high": 10950, - "low": 10950, - "open": 10950, - "volume": 10950 - }, - "id": 2750292, - "non_hive": { - "close": 1715, - "high": 1715, - "low": 1715, - "open": 1715, - "volume": 1715 - }, - "open": "2020-10-20T09:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1814, - "high": 1814, - "low": 1814, - "open": 1814, - "volume": 1814 - }, - "id": 2750295, - "non_hive": { - "close": 284, - "high": 284, - "low": 284, - "open": 284, - "volume": 284 - }, - "open": "2020-10-20T09:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 40979, - "high": 40979, - "low": 40979, - "open": 40979, - "volume": 40979 - }, - "id": 2750298, - "non_hive": { - "close": 6421, - "high": 6421, - "low": 6421, - "open": 6421, - "volume": 6421 - }, - "open": "2020-10-20T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 59231, - "high": 134, - "low": 59231, - "open": 88351, - "volume": 398350 - }, - "id": 2750301, - "non_hive": { - "close": 9121, - "high": 21, - "low": 9121, - "open": 13837, - "volume": 62196 - }, - "open": "2020-10-20T10:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 14627, - "high": 14627, - "low": 447, - "open": 447, - "volume": 15074 - }, - "id": 2750310, - "non_hive": { - "close": 2291, - "high": 2291, - "low": 70, - "open": 70, - "volume": 2361 - }, - "open": "2020-10-20T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 7963, - "high": 7963, - "low": 7963, - "open": 7963, - "volume": 7963 - }, - "id": 2750315, - "non_hive": { - "close": 1226, - "high": 1226, - "low": 1226, - "open": 1226, - "volume": 1226 - }, - "open": "2020-10-20T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12182, - "high": 12182, - "low": 12182, - "open": 12182, - "volume": 12182 - }, - "id": 2750318, - "non_hive": { - "close": 1908, - "high": 1908, - "low": 1908, - "open": 1908, - "volume": 1908 - }, - "open": "2020-10-20T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 44802, - "high": 5025, - "low": 44802, - "open": 5025, - "volume": 49827 - }, - "id": 2750321, - "non_hive": { - "close": 6899, - "high": 774, - "low": 6899, - "open": 774, - "volume": 7673 - }, - "open": "2020-10-20T10:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 2038, - "high": 2038, - "low": 2038, - "open": 2038, - "volume": 2038 - }, - "id": 2750324, - "non_hive": { - "close": 319, - "high": 319, - "low": 319, - "open": 319, - "volume": 319 - }, - "open": "2020-10-20T10:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 3875, - "high": 6864, - "low": 3875, - "open": 6864, - "volume": 10739 - }, - "id": 2750327, - "non_hive": { - "close": 606, - "high": 1074, - "low": 606, - "open": 1074, - "volume": 1680 - }, - "open": "2020-10-20T11:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 201302, - "high": 1127494, - "low": 201302, - "open": 12777, - "volume": 1404200 - }, - "id": 2750333, - "non_hive": { - "close": 31000, - "high": 176746, - "low": 31000, - "open": 1999, - "volume": 219516 - }, - "open": "2020-10-20T11:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 31718, - "high": 8522, - "low": 12759, - "open": 12759, - "volume": 91281 - }, - "id": 2750338, - "non_hive": { - "close": 4971, - "high": 1336, - "low": 1999, - "open": 1999, - "volume": 14307 - }, - "open": "2020-10-20T11:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 19897, - "high": 6940, - "low": 19897, - "open": 6940, - "volume": 96940 - }, - "id": 2750344, - "non_hive": { - "close": 3118, - "high": 1088, - "low": 3118, - "open": 1088, - "volume": 15195 - }, - "open": "2020-10-20T11:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3484, - "high": 210, - "low": 1072, - "open": 25518, - "volume": 30768 - }, - "id": 2750349, - "non_hive": { - "close": 546, - "high": 33, - "low": 168, - "open": 4000, - "volume": 4823 - }, - "open": "2020-10-20T11:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 2627, - "high": 2627, - "low": 2627, - "open": 2627, - "volume": 2627 - }, - "id": 2750354, - "non_hive": { - "close": 404, - "high": 404, - "low": 404, - "open": 404, - "volume": 404 - }, - "open": "2020-10-20T12:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 69831, - "high": 69831, - "low": 69831, - "open": 69831, - "volume": 69831 - }, - "id": 2750358, - "non_hive": { - "close": 10938, - "high": 10938, - "low": 10938, - "open": 10938, - "volume": 10938 - }, - "open": "2020-10-20T12:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1869, - "high": 1869, - "low": 1869, - "open": 1869, - "volume": 1869 - }, - "id": 2750361, - "non_hive": { - "close": 293, - "high": 293, - "low": 293, - "open": 293, - "volume": 293 - }, - "open": "2020-10-20T12:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 13547, - "high": 13547, - "low": 13547, - "open": 13547, - "volume": 13547 - }, - "id": 2750364, - "non_hive": { - "close": 2123, - "high": 2123, - "low": 2123, - "open": 2123, - "volume": 2123 - }, - "open": "2020-10-20T12:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7145, - "high": 10945, - "low": 7145, - "open": 10945, - "volume": 30018 - }, - "id": 2750367, - "non_hive": { - "close": 1118, - "high": 1715, - "low": 1118, - "open": 1715, - "volume": 4702 - }, - "open": "2020-10-20T12:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 94081, - "high": 94081, - "low": 12763, - "open": 12763, - "volume": 153003 - }, - "id": 2750370, - "non_hive": { - "close": 14744, - "high": 14744, - "low": 1999, - "open": 1999, - "volume": 23976 - }, - "open": "2020-10-20T12:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 80824, - "high": 191, - "low": 80824, - "open": 191, - "volume": 94000 - }, - "id": 2750373, - "non_hive": { - "close": 12447, - "high": 30, - "low": 12447, - "open": 30, - "volume": 14477 - }, - "open": "2020-10-20T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 27903, - "high": 1003, - "low": 27903, - "open": 1003, - "volume": 52118 - }, - "id": 2750376, - "non_hive": { - "close": 4367, - "high": 157, - "low": 4367, - "open": 157, - "volume": 8157 - }, - "open": "2020-10-20T12:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 2233, - "high": 741, - "low": 2233, - "open": 741, - "volume": 65047 - }, - "id": 2750381, - "non_hive": { - "close": 347, - "high": 116, - "low": 347, - "open": 116, - "volume": 10116 - }, - "open": "2020-10-20T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 613, - "high": 51064, - "low": 613, - "open": 12780, - "volume": 77435 - }, - "id": 2750391, - "non_hive": { - "close": 94, - "high": 7991, - "low": 94, - "open": 1999, - "volume": 12083 - }, - "open": "2020-10-20T13:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2021, - "high": 926, - "low": 2021, - "open": 12781, - "volume": 28707 - }, - "id": 2750397, - "non_hive": { - "close": 311, - "high": 145, - "low": 311, - "open": 1999, - "volume": 4454 - }, - "open": "2020-10-20T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 11316, - "high": 15464, - "low": 11316, - "open": 207, - "volume": 47313 - }, - "id": 2750402, - "non_hive": { - "close": 1722, - "high": 2420, - "low": 1722, - "open": 32, - "volume": 7333 - }, - "open": "2020-10-20T13:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1704, - "high": 1704, - "low": 1704, - "open": 1704, - "volume": 1704 - }, - "id": 2750409, - "non_hive": { - "close": 259, - "high": 259, - "low": 259, - "open": 259, - "volume": 259 - }, - "open": "2020-10-20T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 9612, - "high": 118, - "low": 9612, - "open": 118, - "volume": 15730 - }, - "id": 2750412, - "non_hive": { - "close": 1462, - "high": 18, - "low": 1462, - "open": 18, - "volume": 2393 - }, - "open": "2020-10-20T13:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 9531, - "high": 9531, - "low": 9531, - "open": 9531, - "volume": 9531 - }, - "id": 2750415, - "non_hive": { - "close": 1490, - "high": 1490, - "low": 1490, - "open": 1490, - "volume": 1490 - }, - "open": "2020-10-20T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1893, - "high": 1893, - "low": 1893, - "open": 1893, - "volume": 1893 - }, - "id": 2750418, - "non_hive": { - "close": 295, - "high": 295, - "low": 295, - "open": 295, - "volume": 295 - }, - "open": "2020-10-20T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13452, - "high": 527, - "low": 10973, - "open": 540, - "volume": 28000 - }, - "id": 2750421, - "non_hive": { - "close": 2085, - "high": 82, - "low": 1700, - "open": 84, - "volume": 4340 - }, - "open": "2020-10-20T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 43232, - "high": 43232, - "low": 12833, - "open": 12833, - "volume": 69065 - }, - "id": 2750429, - "non_hive": { - "close": 6739, - "high": 6739, - "low": 1999, - "open": 1999, - "volume": 10764 - }, - "open": "2020-10-20T14:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 490, - "high": 861, - "low": 3703, - "open": 861, - "volume": 5054 - }, - "id": 2750432, - "non_hive": { - "close": 76, - "high": 134, - "low": 574, - "open": 134, - "volume": 784 - }, - "open": "2020-10-20T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 8700, - "high": 8700, - "low": 8700, - "open": 8700, - "volume": 8700 - }, - "id": 2750438, - "non_hive": { - "close": 1348, - "high": 1348, - "low": 1348, - "open": 1348, - "volume": 1348 - }, - "open": "2020-10-20T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 299749, - "high": 2800, - "low": 299749, - "open": 4203, - "volume": 399152 - }, - "id": 2750441, - "non_hive": { - "close": 45651, - "high": 434, - "low": 45651, - "open": 651, - "volume": 60809 - }, - "open": "2020-10-20T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13219, - "high": 13219, - "low": 12903, - "open": 12903, - "volume": 26122 - }, - "id": 2750446, - "non_hive": { - "close": 2049, - "high": 2049, - "low": 1999, - "open": 1999, - "volume": 4048 - }, - "open": "2020-10-20T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 497030, - "high": 497030, - "low": 497030, - "open": 497030, - "volume": 497030 - }, - "id": 2750449, - "non_hive": { - "close": 77039, - "high": 77039, - "low": 77039, - "open": 77039, - "volume": 77039 - }, - "open": "2020-10-20T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 54187, - "high": 54187, - "low": 54187, - "open": 54187, - "volume": 54187 - }, - "id": 2750452, - "non_hive": { - "close": 8399, - "high": 8399, - "low": 8399, - "open": 8399, - "volume": 8399 - }, - "open": "2020-10-20T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 3247, - "high": 3247, - "low": 3247, - "open": 3247, - "volume": 3247 - }, - "id": 2750455, - "non_hive": { - "close": 503, - "high": 503, - "low": 503, - "open": 503, - "volume": 503 - }, - "open": "2020-10-20T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 37356, - "high": 37356, - "low": 12911, - "open": 12911, - "volume": 50267 - }, - "id": 2750458, - "non_hive": { - "close": 5787, - "high": 5787, - "low": 1999, - "open": 1999, - "volume": 7786 - }, - "open": "2020-10-20T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 12704, - "high": 3195, - "low": 8398, - "open": 12911, - "volume": 37208 - }, - "id": 2750461, - "non_hive": { - "close": 1967, - "high": 495, - "low": 1300, - "open": 1999, - "volume": 5761 - }, - "open": "2020-10-20T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6765, - "high": 742, - "low": 207, - "open": 207, - "volume": 8340 - }, - "id": 2750468, - "non_hive": { - "close": 1048, - "high": 115, - "low": 32, - "open": 32, - "volume": 1292 - }, - "open": "2020-10-20T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 264, - "high": 264, - "low": 265498, - "open": 265498, - "volume": 265762 - }, - "id": 2750477, - "non_hive": { - "close": 41, - "high": 41, - "low": 41107, - "open": 41107, - "volume": 41148 - }, - "open": "2020-10-20T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6471, - "high": 6471, - "low": 6471, - "open": 6471, - "volume": 6471 - }, - "id": 2750482, - "non_hive": { - "close": 1002, - "high": 1002, - "low": 1002, - "open": 1002, - "volume": 1002 - }, - "open": "2020-10-20T15:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1833, - "high": 516, - "low": 11952, - "open": 11952, - "volume": 33164 - }, - "id": 2750485, - "non_hive": { - "close": 284, - "high": 80, - "low": 1850, - "open": 1850, - "volume": 5134 - }, - "open": "2020-10-20T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6716, - "high": 246, - "low": 6716, - "open": 246, - "volume": 6962 - }, - "id": 2750491, - "non_hive": { - "close": 1022, - "high": 38, - "low": 1022, - "open": 38, - "volume": 1060 - }, - "open": "2020-10-20T15:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 4522, - "high": 16149, - "low": 4522, - "open": 16149, - "volume": 20671 - }, - "id": 2750494, - "non_hive": { - "close": 700, - "high": 2500, - "low": 700, - "open": 2500, - "volume": 3200 - }, - "open": "2020-10-20T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 91767, - "high": 91767, - "low": 91767, - "open": 91767, - "volume": 91767 - }, - "id": 2750498, - "non_hive": { - "close": 14207, - "high": 14207, - "low": 14207, - "open": 14207, - "volume": 14207 - }, - "open": "2020-10-20T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12919, - "high": 12919, - "low": 12919, - "open": 12919, - "volume": 51676 - }, - "id": 2750501, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 7996 - }, - "open": "2020-10-20T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1823, - "high": 1823, - "low": 1823, - "open": 1823, - "volume": 1823 - }, - "id": 2750509, - "non_hive": { - "close": 282, - "high": 282, - "low": 282, - "open": 282, - "volume": 282 - }, - "open": "2020-10-20T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12546, - "high": 12986, - "low": 12546, - "open": 12986, - "volume": 329067 - }, - "id": 2750512, - "non_hive": { - "close": 1906, - "high": 2000, - "low": 1906, - "open": 2000, - "volume": 50351 - }, - "open": "2020-10-20T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 12986, - "high": 32913, - "low": 2362, - "open": 32913, - "volume": 1213043 - }, - "id": 2750515, - "non_hive": { - "close": 1972, - "high": 5000, - "low": 354, - "open": 5000, - "volume": 182079 - }, - "open": "2020-10-20T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 72, - "high": 72, - "low": 72, - "open": 72, - "volume": 72 - }, - "id": 2750520, - "non_hive": { - "close": 11, - "high": 11, - "low": 11, - "open": 11, - "volume": 11 - }, - "open": "2020-10-20T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1389, - "high": 4134, - "low": 1389, - "open": 4134, - "volume": 5576 - }, - "id": 2750523, - "non_hive": { - "close": 208, - "high": 628, - "low": 208, - "open": 628, - "volume": 844 - }, - "open": "2020-10-20T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 193814, - "high": 193814, - "low": 193814, - "open": 193814, - "volume": 193814 - }, - "id": 2750526, - "non_hive": { - "close": 30000, - "high": 30000, - "low": 30000, - "open": 30000, - "volume": 30000 - }, - "open": "2020-10-20T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1389, - "high": 1389, - "low": 1389, - "open": 1389, - "volume": 1389 - }, - "id": 2750530, - "non_hive": { - "close": 214, - "high": 214, - "low": 214, - "open": 214, - "volume": 214 - }, - "open": "2020-10-20T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12981, - "high": 6, - "low": 12968, - "open": 12973, - "volume": 129741 - }, - "id": 2750533, - "non_hive": { - "close": 2000, - "high": 1, - "low": 1997, - "open": 2000, - "volume": 19998 - }, - "open": "2020-10-20T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 9, - "high": 13872, - "low": 9, - "open": 7969, - "volume": 52759 - }, - "id": 2750549, - "non_hive": { - "close": 1, - "high": 2147, - "low": 1, - "open": 1228, - "volume": 8146 - }, - "open": "2020-10-20T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 3589, - "high": 3589, - "low": 3589, - "open": 3589, - "volume": 3589 - }, - "id": 2750556, - "non_hive": { - "close": 552, - "high": 552, - "low": 552, - "open": 552, - "volume": 552 - }, - "open": "2020-10-20T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 7033, - "high": 7033, - "low": 7033, - "open": 7033, - "volume": 7033 - }, - "id": 2750559, - "non_hive": { - "close": 1088, - "high": 1088, - "low": 1088, - "open": 1088, - "volume": 1088 - }, - "open": "2020-10-20T17:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 60000, - "high": 6, - "low": 60000, - "open": 49346, - "volume": 116488 - }, - "id": 2750562, - "non_hive": { - "close": 9180, - "high": 1, - "low": 9180, - "open": 7633, - "volume": 17906 - }, - "open": "2020-10-20T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 5388, - "high": 5388, - "low": 5388, - "open": 5388, - "volume": 5388 - }, - "id": 2750566, - "non_hive": { - "close": 824, - "high": 824, - "low": 824, - "open": 824, - "volume": 824 - }, - "open": "2020-10-20T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1530, - "high": 1530, - "low": 1530, - "open": 1530, - "volume": 1530 - }, - "id": 2750569, - "non_hive": { - "close": 234, - "high": 234, - "low": 234, - "open": 234, - "volume": 234 - }, - "open": "2020-10-20T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 7684, - "high": 346, - "low": 6154, - "open": 6154, - "volume": 54892 - }, - "id": 2750572, - "non_hive": { - "close": 1175, - "high": 53, - "low": 941, - "open": 941, - "volume": 8396 - }, - "open": "2020-10-20T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 8559, - "high": 13224, - "low": 8559, - "open": 13224, - "volume": 37000 - }, - "id": 2750578, - "non_hive": { - "close": 1283, - "high": 1999, - "low": 1283, - "open": 1999, - "volume": 5568 - }, - "open": "2020-10-20T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 181, - "high": 12925, - "low": 181, - "open": 12925, - "volume": 13106 - }, - "id": 2750581, - "non_hive": { - "close": 27, - "high": 1999, - "low": 27, - "open": 1999, - "volume": 2026 - }, - "open": "2020-10-20T19:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2880, - "high": 6, - "low": 2880, - "open": 6, - "volume": 15949 - }, - "id": 2750587, - "non_hive": { - "close": 440, - "high": 1, - "low": 440, - "open": 1, - "volume": 2440 - }, - "open": "2020-10-20T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13043, - "high": 6583, - "low": 13043, - "open": 6583, - "volume": 19626 - }, - "id": 2750594, - "non_hive": { - "close": 1997, - "high": 1008, - "low": 1997, - "open": 1008, - "volume": 3005 - }, - "open": "2020-10-20T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6988, - "high": 6988, - "low": 6988, - "open": 6988, - "volume": 6988 - }, - "id": 2750599, - "non_hive": { - "close": 1070, - "high": 1070, - "low": 1070, - "open": 1070, - "volume": 1070 - }, - "open": "2020-10-20T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 4064, - "high": 13645, - "low": 4064, - "open": 13645, - "volume": 17709 - }, - "id": 2750602, - "non_hive": { - "close": 622, - "high": 2089, - "low": 622, - "open": 2089, - "volume": 2711 - }, - "open": "2020-10-20T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 5405, - "high": 5405, - "low": 13200, - "open": 13200, - "volume": 18605 - }, - "id": 2750605, - "non_hive": { - "close": 819, - "high": 819, - "low": 1999, - "open": 1999, - "volume": 2818 - }, - "open": "2020-10-20T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 10381, - "high": 10381, - "low": 10381, - "open": 10381, - "volume": 10381 - }, - "id": 2750608, - "non_hive": { - "close": 1572, - "high": 1572, - "low": 1572, - "open": 1572, - "volume": 1572 - }, - "open": "2020-10-20T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 19606, - "high": 19606, - "low": 19606, - "open": 19606, - "volume": 19606 - }, - "id": 2750612, - "non_hive": { - "close": 3000, - "high": 3000, - "low": 3000, - "open": 3000, - "volume": 3000 - }, - "open": "2020-10-20T20:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 19, - "high": 19, - "low": 19, - "open": 19, - "volume": 19 - }, - "id": 2750615, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 3, - "volume": 3 - }, - "open": "2020-10-20T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 11429, - "high": 11429, - "low": 11429, - "open": 11429, - "volume": 11429 - }, - "id": 2750618, - "non_hive": { - "close": 1749, - "high": 1749, - "low": 1749, - "open": 1749, - "volume": 1749 - }, - "open": "2020-10-20T20:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 27624, - "high": 27624, - "low": 1615, - "open": 1615, - "volume": 67923 - }, - "id": 2750621, - "non_hive": { - "close": 4279, - "high": 4279, - "low": 247, - "open": 247, - "volume": 10448 - }, - "open": "2020-10-20T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 3269, - "high": 41089, - "low": 3269, - "open": 12911, - "volume": 201128 - }, - "id": 2750625, - "non_hive": { - "close": 495, - "high": 6362, - "low": 495, - "open": 1999, - "volume": 30657 - }, - "open": "2020-10-20T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 5882, - "high": 5882, - "low": 609, - "open": 13072, - "volume": 64846 - }, - "id": 2750632, - "non_hive": { - "close": 907, - "high": 907, - "low": 93, - "open": 1999, - "volume": 9943 - }, - "open": "2020-10-20T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 11613, - "high": 11613, - "low": 11613, - "open": 11613, - "volume": 11613 - }, - "id": 2750640, - "non_hive": { - "close": 1776, - "high": 1776, - "low": 1776, - "open": 1776, - "volume": 1776 - }, - "open": "2020-10-20T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 2638, - "high": 2638, - "low": 2638, - "open": 2638, - "volume": 2638 - }, - "id": 2750643, - "non_hive": { - "close": 403, - "high": 403, - "low": 403, - "open": 403, - "volume": 403 - }, - "open": "2020-10-20T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2639, - "high": 2639, - "low": 2639, - "open": 2639, - "volume": 2639 - }, - "id": 2750646, - "non_hive": { - "close": 403, - "high": 403, - "low": 403, - "open": 403, - "volume": 403 - }, - "open": "2020-10-20T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13093, - "high": 13093, - "low": 13093, - "open": 13093, - "volume": 13093 - }, - "id": 2750649, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-20T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 100, - "high": 100, - "low": 100, - "open": 100, - "volume": 100 - }, - "id": 2750652, - "non_hive": { - "close": 15, - "high": 15, - "low": 15, - "open": 15, - "volume": 15 - }, - "open": "2020-10-20T21:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 8821, - "high": 8821, - "low": 8821, - "open": 8821, - "volume": 8821 - }, - "id": 2750655, - "non_hive": { - "close": 1348, - "high": 1348, - "low": 1348, - "open": 1348, - "volume": 1348 - }, - "open": "2020-10-20T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 4261, - "high": 4261, - "low": 4261, - "open": 4261, - "volume": 4261 - }, - "id": 2750658, - "non_hive": { - "close": 651, - "high": 651, - "low": 651, - "open": 651, - "volume": 651 - }, - "open": "2020-10-20T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13332, - "high": 18000, - "low": 13332, - "open": 13082, - "volume": 61414 - }, - "id": 2750661, - "non_hive": { - "close": 2000, - "high": 2752, - "low": 2000, - "open": 1999, - "volume": 9350 - }, - "open": "2020-10-20T22:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 5206, - "high": 5206, - "low": 5206, - "open": 5206, - "volume": 5206 - }, - "id": 2750666, - "non_hive": { - "close": 781, - "high": 781, - "low": 781, - "open": 781, - "volume": 781 - }, - "open": "2020-10-20T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 12005, - "high": 12005, - "low": 1000, - "open": 1000, - "volume": 18871 - }, - "id": 2750669, - "non_hive": { - "close": 1801, - "high": 1801, - "low": 150, - "open": 150, - "volume": 2831 - }, - "open": "2020-10-20T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 9592, - "high": 16111, - "low": 9592, - "open": 16111, - "volume": 25703 - }, - "id": 2750674, - "non_hive": { - "close": 1439, - "high": 2417, - "low": 1439, - "open": 2417, - "volume": 3856 - }, - "open": "2020-10-20T22:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1501, - "high": 1501, - "low": 1501, - "open": 1501, - "volume": 1501 - }, - "id": 2750678, - "non_hive": { - "close": 225, - "high": 225, - "low": 225, - "open": 225, - "volume": 225 - }, - "open": "2020-10-20T22:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2533, - "high": 2533, - "low": 2533, - "open": 2533, - "volume": 2533 - }, - "id": 2750681, - "non_hive": { - "close": 380, - "high": 380, - "low": 380, - "open": 380, - "volume": 380 - }, - "open": "2020-10-20T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 474, - "high": 9905, - "low": 474, - "open": 9905, - "volume": 10379 - }, - "id": 2750684, - "non_hive": { - "close": 71, - "high": 1486, - "low": 71, - "open": 1486, - "volume": 1557 - }, - "open": "2020-10-20T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 10452, - "high": 10452, - "low": 21831, - "open": 21831, - "volume": 32283 - }, - "id": 2750689, - "non_hive": { - "close": 1568, - "high": 1568, - "low": 3275, - "open": 3275, - "volume": 4843 - }, - "open": "2020-10-20T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 54240, - "high": 54240, - "low": 54240, - "open": 54240, - "volume": 54240 - }, - "id": 2750694, - "non_hive": { - "close": 8136, - "high": 8136, - "low": 8136, - "open": 8136, - "volume": 8136 - }, - "open": "2020-10-20T22:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 446, - "high": 446, - "low": 446, - "open": 446, - "volume": 446 - }, - "id": 2750697, - "non_hive": { - "close": 67, - "high": 67, - "low": 67, - "open": 67, - "volume": 67 - }, - "open": "2020-10-20T23:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 17324, - "high": 53, - "low": 17324, - "open": 53, - "volume": 17377 - }, - "id": 2750701, - "non_hive": { - "close": 2599, - "high": 8, - "low": 2599, - "open": 8, - "volume": 2607 - }, - "open": "2020-10-20T23:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1353, - "high": 1353, - "low": 20751, - "open": 20751, - "volume": 22104 - }, - "id": 2750706, - "non_hive": { - "close": 203, - "high": 203, - "low": 3113, - "open": 3113, - "volume": 3316 - }, - "open": "2020-10-20T23:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 8056, - "high": 91944, - "low": 8056, - "open": 91944, - "volume": 100000 - }, - "id": 2750711, - "non_hive": { - "close": 1208, - "high": 13793, - "low": 1208, - "open": 13793, - "volume": 15001 - }, - "open": "2020-10-20T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 13333, - "high": 11946, - "low": 13333, - "open": 11946, - "volume": 45278 - }, - "id": 2750714, - "non_hive": { - "close": 2000, - "high": 1792, - "low": 2000, - "open": 1792, - "volume": 6792 - }, - "open": "2020-10-20T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 2006, - "high": 2006, - "low": 2006, - "open": 2006, - "volume": 2006 - }, - "id": 2750717, - "non_hive": { - "close": 301, - "high": 301, - "low": 301, - "open": 301, - "volume": 301 - }, - "open": "2020-10-21T00:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1866, - "high": 1866, - "low": 1866, - "open": 1866, - "volume": 1866 - }, - "id": 2750722, - "non_hive": { - "close": 280, - "high": 280, - "low": 280, - "open": 280, - "volume": 280 - }, - "open": "2020-10-21T00:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 37072, - "high": 37072, - "low": 37072, - "open": 37072, - "volume": 37072 - }, - "id": 2750725, - "non_hive": { - "close": 5561, - "high": 5561, - "low": 5561, - "open": 5561, - "volume": 5561 - }, - "open": "2020-10-21T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 29417, - "high": 29417, - "low": 19709, - "open": 19709, - "volume": 49126 - }, - "id": 2750728, - "non_hive": { - "close": 4413, - "high": 4413, - "low": 2956, - "open": 2956, - "volume": 7369 - }, - "open": "2020-10-21T00:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 6652, - "high": 6652, - "low": 6652, - "open": 6652, - "volume": 6652 - }, - "id": 2750731, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-10-21T00:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11880, - "high": 11880, - "low": 11880, - "open": 11880, - "volume": 11880 - }, - "id": 2750734, - "non_hive": { - "close": 1782, - "high": 1782, - "low": 1782, - "open": 1782, - "volume": 1782 - }, - "open": "2020-10-21T01:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 7462, - "high": 7462, - "low": 7462, - "open": 7462, - "volume": 7462 - }, - "id": 2750738, - "non_hive": { - "close": 1119, - "high": 1119, - "low": 1119, - "open": 1119, - "volume": 1119 - }, - "open": "2020-10-21T01:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 25144, - "high": 25144, - "low": 25144, - "open": 25144, - "volume": 25144 - }, - "id": 2750741, - "non_hive": { - "close": 3772, - "high": 3772, - "low": 3772, - "open": 3772, - "volume": 3772 - }, - "open": "2020-10-21T02:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 94991, - "high": 8005, - "low": 94991, - "open": 8005, - "volume": 102996 - }, - "id": 2750745, - "non_hive": { - "close": 14250, - "high": 1201, - "low": 14250, - "open": 1201, - "volume": 15451 - }, - "open": "2020-10-21T02:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 9459, - "high": 9459, - "low": 9459, - "open": 9459, - "volume": 9459 - }, - "id": 2750749, - "non_hive": { - "close": 1419, - "high": 1419, - "low": 1419, - "open": 1419, - "volume": 1419 - }, - "open": "2020-10-21T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 27537, - "high": 27537, - "low": 27537, - "open": 27537, - "volume": 27537 - }, - "id": 2750752, - "non_hive": { - "close": 4131, - "high": 4131, - "low": 4131, - "open": 4131, - "volume": 4131 - }, - "open": "2020-10-21T03:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 3488, - "high": 10512, - "low": 3488, - "open": 10512, - "volume": 14000 - }, - "id": 2750756, - "non_hive": { - "close": 523, - "high": 1577, - "low": 523, - "open": 1577, - "volume": 2100 - }, - "open": "2020-10-21T03:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 18064, - "high": 18064, - "low": 268013, - "open": 268013, - "volume": 484410 - }, - "id": 2750759, - "non_hive": { - "close": 2710, - "high": 2710, - "low": 40205, - "open": 40205, - "volume": 72668 - }, - "open": "2020-10-21T03:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6652, - "high": 6652, - "low": 6652, - "open": 6652, - "volume": 6652 - }, - "id": 2750764, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-10-21T04:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 8000, - "high": 8000, - "low": 8000, - "open": 8000, - "volume": 8000 - }, - "id": 2750768, - "non_hive": { - "close": 1200, - "high": 1200, - "low": 1200, - "open": 1200, - "volume": 1200 - }, - "open": "2020-10-21T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 41769, - "high": 29190, - "low": 41769, - "open": 29190, - "volume": 70959 - }, - "id": 2750771, - "non_hive": { - "close": 6266, - "high": 4379, - "low": 6266, - "open": 4379, - "volume": 10645 - }, - "open": "2020-10-21T04:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 16049, - "high": 16049, - "low": 3065, - "open": 9565, - "volume": 249807 - }, - "id": 2750774, - "non_hive": { - "close": 2452, - "high": 2452, - "low": 459, - "open": 1435, - "volume": 37517 - }, - "open": "2020-10-21T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 137456, - "high": 137456, - "low": 1951, - "open": 1951, - "volume": 139407 - }, - "id": 2750779, - "non_hive": { - "close": 21000, - "high": 21000, - "low": 298, - "open": 298, - "volume": 21298 - }, - "open": "2020-10-21T04:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11005, - "high": 11005, - "low": 11005, - "open": 11005, - "volume": 11005 - }, - "id": 2750784, - "non_hive": { - "close": 1682, - "high": 1682, - "low": 1682, - "open": 1682, - "volume": 1682 - }, - "open": "2020-10-21T05:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 7596, - "high": 46719, - "low": 7596, - "open": 46719, - "volume": 54315 - }, - "id": 2750788, - "non_hive": { - "close": 1160, - "high": 7140, - "low": 1160, - "open": 7140, - "volume": 8300 - }, - "open": "2020-10-21T05:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2075, - "high": 2075, - "low": 2075, - "open": 2075, - "volume": 2075 - }, - "id": 2750791, - "non_hive": { - "close": 317, - "high": 317, - "low": 317, - "open": 317, - "volume": 317 - }, - "open": "2020-10-21T05:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 98, - "high": 98, - "low": 7876, - "open": 7876, - "volume": 7974 - }, - "id": 2750794, - "non_hive": { - "close": 15, - "high": 15, - "low": 1204, - "open": 1204, - "volume": 1219 - }, - "open": "2020-10-21T05:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2887, - "high": 2887, - "low": 2887, - "open": 2887, - "volume": 2887 - }, - "id": 2750797, - "non_hive": { - "close": 436, - "high": 436, - "low": 436, - "open": 436, - "volume": 436 - }, - "open": "2020-10-21T05:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 23653, - "high": 23653, - "low": 23653, - "open": 23653, - "volume": 23653 - }, - "id": 2750800, - "non_hive": { - "close": 3579, - "high": 3579, - "low": 3579, - "open": 3579, - "volume": 3579 - }, - "open": "2020-10-21T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 26192, - "high": 26192, - "low": 6827, - "open": 6827, - "volume": 33019 - }, - "id": 2750803, - "non_hive": { - "close": 3963, - "high": 3963, - "low": 1032, - "open": 1032, - "volume": 4995 - }, - "open": "2020-10-21T06:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 65364, - "high": 65364, - "low": 65364, - "open": 65364, - "volume": 65364 - }, - "id": 2750807, - "non_hive": { - "close": 9890, - "high": 9890, - "low": 9890, - "open": 9890, - "volume": 9890 - }, - "open": "2020-10-21T06:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2512, - "high": 2512, - "low": 2512, - "open": 2512, - "volume": 2512 - }, - "id": 2750810, - "non_hive": { - "close": 380, - "high": 380, - "low": 380, - "open": 380, - "volume": 380 - }, - "open": "2020-10-21T06:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 66488, - "high": 66488, - "low": 66488, - "open": 66488, - "volume": 66488 - }, - "id": 2750813, - "non_hive": { - "close": 10060, - "high": 10060, - "low": 10060, - "open": 10060, - "volume": 10060 - }, - "open": "2020-10-21T06:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 2513, - "high": 15281, - "low": 2513, - "open": 15281, - "volume": 23155 - }, - "id": 2750816, - "non_hive": { - "close": 380, - "high": 2312, - "low": 380, - "open": 2312, - "volume": 3503 - }, - "open": "2020-10-21T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 25293, - "high": 25293, - "low": 18303, - "open": 18303, - "volume": 43596 - }, - "id": 2750819, - "non_hive": { - "close": 3827, - "high": 3827, - "low": 2769, - "open": 2769, - "volume": 6596 - }, - "open": "2020-10-21T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 10143, - "high": 10143, - "low": 10143, - "open": 10143, - "volume": 10143 - }, - "id": 2750823, - "non_hive": { - "close": 1551, - "high": 1551, - "low": 1551, - "open": 1551, - "volume": 1551 - }, - "open": "2020-10-21T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 16010, - "high": 16010, - "low": 16010, - "open": 16010, - "volume": 16010 - }, - "id": 2750826, - "non_hive": { - "close": 2448, - "high": 2448, - "low": 2448, - "open": 2448, - "volume": 2448 - }, - "open": "2020-10-21T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13230, - "high": 13215, - "low": 66458, - "open": 13215, - "volume": 404791 - }, - "id": 2750829, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 10046, - "open": 2000, - "volume": 61236 - }, - "open": "2020-10-21T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 7823, - "high": 13192, - "low": 7823, - "open": 13192, - "volume": 47399 - }, - "id": 2750838, - "non_hive": { - "close": 1186, - "high": 2000, - "low": 1186, - "open": 2000, - "volume": 7186 - }, - "open": "2020-10-21T07:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 667, - "high": 667, - "low": 15000, - "open": 47810, - "volume": 63477 - }, - "id": 2750845, - "non_hive": { - "close": 102, - "high": 102, - "low": 2292, - "open": 7306, - "volume": 9700 - }, - "open": "2020-10-21T07:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 14581, - "high": 14581, - "low": 43154, - "open": 43154, - "volume": 57735 - }, - "id": 2750851, - "non_hive": { - "close": 2231, - "high": 2231, - "low": 6598, - "open": 6598, - "volume": 8829 - }, - "open": "2020-10-21T07:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 32661, - "high": 32661, - "low": 32661, - "open": 32661, - "volume": 32661 - }, - "id": 2750854, - "non_hive": { - "close": 4994, - "high": 4994, - "low": 4994, - "open": 4994, - "volume": 4994 - }, - "open": "2020-10-21T08:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 20576, - "high": 20576, - "low": 20576, - "open": 20576, - "volume": 20576 - }, - "id": 2750858, - "non_hive": { - "close": 3146, - "high": 3146, - "low": 3146, - "open": 3146, - "volume": 3146 - }, - "open": "2020-10-21T08:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 42456, - "high": 42456, - "low": 13072, - "open": 13072, - "volume": 73868 - }, - "id": 2750861, - "non_hive": { - "close": 6536, - "high": 6536, - "low": 1999, - "open": 1999, - "volume": 11341 - }, - "open": "2020-10-21T09:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 7142, - "high": 7142, - "low": 7142, - "open": 7142, - "volume": 7142 - }, - "id": 2750865, - "non_hive": { - "close": 1099, - "high": 1099, - "low": 1099, - "open": 1099, - "volume": 1099 - }, - "open": "2020-10-21T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 24126, - "high": 106101, - "low": 24126, - "open": 106101, - "volume": 142251 - }, - "id": 2750868, - "non_hive": { - "close": 3712, - "high": 16325, - "low": 3712, - "open": 16325, - "volume": 21887 - }, - "open": "2020-10-21T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 674, - "high": 442, - "low": 674, - "open": 442, - "volume": 5411 - }, - "id": 2750872, - "non_hive": { - "close": 103, - "high": 68, - "low": 103, - "open": 68, - "volume": 831 - }, - "open": "2020-10-21T10:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 116, - "high": 13642, - "low": 116, - "open": 12999, - "volume": 27620 - }, - "id": 2750875, - "non_hive": { - "close": 17, - "high": 2099, - "low": 17, - "open": 1999, - "volume": 4247 - }, - "open": "2020-10-21T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 163, - "high": 830, - "low": 163, - "open": 830, - "volume": 993 - }, - "id": 2750878, - "non_hive": { - "close": 24, - "high": 127, - "low": 24, - "open": 127, - "volume": 151 - }, - "open": "2020-10-21T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 20997, - "high": 20997, - "low": 13079, - "open": 13079, - "volume": 55073 - }, - "id": 2750881, - "non_hive": { - "close": 3210, - "high": 3210, - "low": 1999, - "open": 1999, - "volume": 8419 - }, - "open": "2020-10-21T10:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6544, - "high": 3800, - "low": 6544, - "open": 3800, - "volume": 10344 - }, - "id": 2750887, - "non_hive": { - "close": 1000, - "high": 581, - "low": 1000, - "open": 581, - "volume": 1581 - }, - "open": "2020-10-21T10:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 26, - "high": 26, - "low": 1734, - "open": 6538, - "volume": 37663 - }, - "id": 2750891, - "non_hive": { - "close": 4, - "high": 4, - "low": 264, - "open": 999, - "volume": 5754 - }, - "open": "2020-10-21T10:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 2996, - "high": 124, - "low": 8638, - "open": 124, - "volume": 11954 - }, - "id": 2750900, - "non_hive": { - "close": 453, - "high": 19, - "low": 1306, - "open": 19, - "volume": 1808 - }, - "open": "2020-10-21T10:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13008, - "high": 13008, - "low": 11257, - "open": 13227, - "volume": 113008 - }, - "id": 2750906, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1688, - "open": 2000, - "volume": 17102 - }, - "open": "2020-10-21T10:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 23034, - "high": 3258, - "low": 23034, - "open": 3258, - "volume": 39623 - }, - "id": 2750911, - "non_hive": { - "close": 3455, - "high": 501, - "low": 3455, - "open": 501, - "volume": 5956 - }, - "open": "2020-10-21T11:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 8596, - "high": 8596, - "low": 8596, - "open": 8596, - "volume": 8596 - }, - "id": 2750917, - "non_hive": { - "close": 1321, - "high": 1321, - "low": 1321, - "open": 1321, - "volume": 1321 - }, - "open": "2020-10-21T11:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13131, - "high": 13131, - "low": 13131, - "open": 13131, - "volume": 13131 - }, - "id": 2750920, - "non_hive": { - "close": 2017, - "high": 2017, - "low": 2017, - "open": 2017, - "volume": 2017 - }, - "open": "2020-10-21T11:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13021, - "high": 13021, - "low": 13021, - "open": 13021, - "volume": 13021 - }, - "id": 2750923, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-21T11:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 9311, - "high": 482, - "low": 9311, - "open": 482, - "volume": 10829 - }, - "id": 2750926, - "non_hive": { - "close": 1429, - "high": 74, - "low": 1429, - "open": 74, - "volume": 1662 - }, - "open": "2020-10-21T11:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 9171, - "high": 9171, - "low": 9171, - "open": 9171, - "volume": 9171 - }, - "id": 2750929, - "non_hive": { - "close": 1408, - "high": 1408, - "low": 1408, - "open": 1408, - "volume": 1408 - }, - "open": "2020-10-21T12:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 5589, - "high": 5589, - "low": 5589, - "open": 5589, - "volume": 5589 - }, - "id": 2750933, - "non_hive": { - "close": 858, - "high": 858, - "low": 858, - "open": 858, - "volume": 858 - }, - "open": "2020-10-21T12:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 12402, - "high": 735, - "low": 13235, - "open": 3342, - "volume": 69419 - }, - "id": 2750936, - "non_hive": { - "close": 1876, - "high": 113, - "low": 2000, - "open": 513, - "volume": 10502 - }, - "open": "2020-10-21T12:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 356585, - "high": 356585, - "low": 238, - "open": 238, - "volume": 356823 - }, - "id": 2750949, - "non_hive": { - "close": 54200, - "high": 54200, - "low": 36, - "open": 36, - "volume": 54236 - }, - "open": "2020-10-21T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 24909, - "high": 24909, - "low": 1000, - "open": 1000, - "volume": 25909 - }, - "id": 2750954, - "non_hive": { - "close": 3786, - "high": 3786, - "low": 151, - "open": 151, - "volume": 3937 - }, - "open": "2020-10-21T12:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 4049, - "high": 4049, - "low": 1277, - "open": 12284, - "volume": 30630 - }, - "id": 2750959, - "non_hive": { - "close": 622, - "high": 622, - "low": 196, - "open": 1886, - "volume": 4703 - }, - "open": "2020-10-21T12:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 33096, - "high": 3580, - "low": 5384, - "open": 5384, - "volume": 55080 - }, - "id": 2750966, - "non_hive": { - "close": 5084, - "high": 550, - "low": 813, - "open": 813, - "volume": 8446 - }, - "open": "2020-10-21T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 47375, - "high": 47375, - "low": 42940, - "open": 42940, - "volume": 183987 - }, - "id": 2750974, - "non_hive": { - "close": 7283, - "high": 7283, - "low": 6596, - "open": 6596, - "volume": 28277 - }, - "open": "2020-10-21T13:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 34300, - "high": 34300, - "low": 13010, - "open": 13010, - "volume": 47310 - }, - "id": 2750980, - "non_hive": { - "close": 5273, - "high": 5273, - "low": 1999, - "open": 1999, - "volume": 7272 - }, - "open": "2020-10-21T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1453756, - "high": 6, - "low": 1453756, - "open": 6, - "volume": 1508111 - }, - "id": 2750983, - "non_hive": { - "close": 219517, - "high": 1, - "low": 219517, - "open": 1, - "volume": 227815 - }, - "open": "2020-10-21T13:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 4668, - "high": 4668, - "low": 13060, - "open": 13060, - "volume": 17728 - }, - "id": 2750988, - "non_hive": { - "close": 715, - "high": 715, - "low": 1999, - "open": 1999, - "volume": 2714 - }, - "open": "2020-10-21T13:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 416908, - "high": 416908, - "low": 4221, - "open": 4221, - "volume": 421129 - }, - "id": 2750991, - "non_hive": { - "close": 64057, - "high": 64057, - "low": 646, - "open": 646, - "volume": 64703 - }, - "open": "2020-10-21T13:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 6000 - }, - "id": 2750996, - "non_hive": { - "close": 922, - "high": 922, - "low": 922, - "open": 922, - "volume": 922 - }, - "open": "2020-10-21T13:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6781, - "high": 6781, - "low": 216, - "open": 25714, - "volume": 187722 - }, - "id": 2750999, - "non_hive": { - "close": 1042, - "high": 1042, - "low": 33, - "open": 3951, - "volume": 28835 - }, - "open": "2020-10-21T13:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6206, - "high": 6206, - "low": 6206, - "open": 6206, - "volume": 6206 - }, - "id": 2751009, - "non_hive": { - "close": 953, - "high": 953, - "low": 953, - "open": 953, - "volume": 953 - }, - "open": "2020-10-21T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 37243, - "high": 37243, - "low": 37243, - "open": 37243, - "volume": 37243 - }, - "id": 2751013, - "non_hive": { - "close": 5719, - "high": 5719, - "low": 5719, - "open": 5719, - "volume": 5719 - }, - "open": "2020-10-21T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 12796, - "high": 37657, - "low": 1830, - "open": 1830, - "volume": 159094 - }, - "id": 2751016, - "non_hive": { - "close": 1965, - "high": 5786, - "low": 281, - "open": 281, - "volume": 24434 - }, - "open": "2020-10-21T14:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3273, - "high": 3273, - "low": 13017, - "open": 13017, - "volume": 16290 - }, - "id": 2751021, - "non_hive": { - "close": 503, - "high": 503, - "low": 1999, - "open": 1999, - "volume": 2502 - }, - "open": "2020-10-21T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 24350, - "high": 24350, - "low": 24350, - "open": 24350, - "volume": 24350 - }, - "id": 2751024, - "non_hive": { - "close": 3739, - "high": 3739, - "low": 3739, - "open": 3739, - "volume": 3739 - }, - "open": "2020-10-21T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12991, - "high": 12991, - "low": 10127, - "open": 10127, - "volume": 36136 - }, - "id": 2751027, - "non_hive": { - "close": 1996, - "high": 1996, - "low": 1555, - "open": 1555, - "volume": 5550 - }, - "open": "2020-10-21T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7529, - "high": 7529, - "low": 7529, - "open": 7529, - "volume": 7529 - }, - "id": 2751032, - "non_hive": { - "close": 1156, - "high": 1156, - "low": 1156, - "open": 1156, - "volume": 1156 - }, - "open": "2020-10-21T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1010, - "high": 1010, - "low": 1010, - "open": 1010, - "volume": 1010 - }, - "id": 2751035, - "non_hive": { - "close": 155, - "high": 155, - "low": 155, - "open": 155, - "volume": 155 - }, - "open": "2020-10-21T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 8550, - "high": 8550, - "low": 8550, - "open": 8550, - "volume": 8550 - }, - "id": 2751038, - "non_hive": { - "close": 1312, - "high": 1312, - "low": 1312, - "open": 1312, - "volume": 1312 - }, - "open": "2020-10-21T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 5736, - "high": 37690, - "low": 5736, - "open": 37690, - "volume": 43426 - }, - "id": 2751041, - "non_hive": { - "close": 880, - "high": 5783, - "low": 880, - "open": 5783, - "volume": 6663 - }, - "open": "2020-10-21T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 54076, - "high": 574, - "low": 54076, - "open": 574, - "volume": 54650 - }, - "id": 2751047, - "non_hive": { - "close": 8207, - "high": 88, - "low": 8207, - "open": 88, - "volume": 8295 - }, - "open": "2020-10-21T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3191, - "high": 16809, - "low": 3191, - "open": 16809, - "volume": 20000 - }, - "id": 2751052, - "non_hive": { - "close": 484, - "high": 2551, - "low": 484, - "open": 2551, - "volume": 3035 - }, - "open": "2020-10-21T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1568, - "high": 1568, - "low": 12072, - "open": 12072, - "volume": 13640 - }, - "id": 2751055, - "non_hive": { - "close": 238, - "high": 238, - "low": 1832, - "open": 1832, - "volume": 2070 - }, - "open": "2020-10-21T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 34337, - "high": 17342, - "low": 34337, - "open": 17342, - "volume": 173342 - }, - "id": 2751058, - "non_hive": { - "close": 5202, - "high": 2632, - "low": 5202, - "open": 2632, - "volume": 26269 - }, - "open": "2020-10-21T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 24954, - "high": 1953, - "low": 24954, - "open": 1953, - "volume": 40152 - }, - "id": 2751062, - "non_hive": { - "close": 3767, - "high": 296, - "low": 3767, - "open": 296, - "volume": 6063 - }, - "open": "2020-10-21T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 8862, - "high": 6984, - "low": 8862, - "open": 13178, - "volume": 29024 - }, - "id": 2751067, - "non_hive": { - "close": 1344, - "high": 1060, - "low": 1344, - "open": 1999, - "volume": 4403 - }, - "open": "2020-10-21T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 16060, - "high": 1739, - "low": 3200, - "open": 1739, - "volume": 25331 - }, - "id": 2751071, - "non_hive": { - "close": 2465, - "high": 267, - "low": 491, - "open": 267, - "volume": 3888 - }, - "open": "2020-10-21T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2880, - "high": 2880, - "low": 10951, - "open": 3467, - "volume": 297443 - }, - "id": 2751079, - "non_hive": { - "close": 442, - "high": 442, - "low": 1680, - "open": 532, - "volume": 45641 - }, - "open": "2020-10-21T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 13244, - "high": 13244, - "low": 13244, - "open": 13244, - "volume": 13244 - }, - "id": 2751087, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-21T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 27071, - "high": 27071, - "low": 210, - "open": 13034, - "volume": 262291 - }, - "id": 2751090, - "non_hive": { - "close": 4154, - "high": 4154, - "low": 32, - "open": 1999, - "volume": 40246 - }, - "open": "2020-10-21T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 35440, - "high": 325, - "low": 35440, - "open": 75004, - "volume": 319984 - }, - "id": 2751095, - "non_hive": { - "close": 5438, - "high": 50, - "low": 5438, - "open": 11509, - "volume": 49100 - }, - "open": "2020-10-21T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 91624, - "high": 91624, - "low": 91624, - "open": 91624, - "volume": 91624 - }, - "id": 2751103, - "non_hive": { - "close": 14059, - "high": 14059, - "low": 14059, - "open": 14059, - "volume": 14059 - }, - "open": "2020-10-21T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 26074, - "high": 26074, - "low": 26074, - "open": 26074, - "volume": 26074 - }, - "id": 2751106, - "non_hive": { - "close": 4001, - "high": 4001, - "low": 4001, - "open": 4001, - "volume": 4001 - }, - "open": "2020-10-21T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 95634, - "high": 95634, - "low": 95634, - "open": 95634, - "volume": 95634 - }, - "id": 2751110, - "non_hive": { - "close": 14673, - "high": 14673, - "low": 14673, - "open": 14673, - "volume": 14673 - }, - "open": "2020-10-21T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 52143, - "high": 52143, - "low": 1004, - "open": 25068, - "volume": 78215 - }, - "id": 2751113, - "non_hive": { - "close": 8000, - "high": 8000, - "low": 154, - "open": 3846, - "volume": 12000 - }, - "open": "2020-10-21T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 28815, - "high": 104, - "low": 28815, - "open": 104, - "volume": 28919 - }, - "id": 2751118, - "non_hive": { - "close": 4421, - "high": 16, - "low": 4421, - "open": 16, - "volume": 4437 - }, - "open": "2020-10-21T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 104289, - "high": 104289, - "low": 23224, - "open": 23224, - "volume": 177080 - }, - "id": 2751121, - "non_hive": { - "close": 16001, - "high": 16001, - "low": 3563, - "open": 3563, - "volume": 27169 - }, - "open": "2020-10-21T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 29564, - "high": 1003, - "low": 621614, - "open": 126893, - "volume": 779074 - }, - "id": 2751126, - "non_hive": { - "close": 4536, - "high": 154, - "low": 95373, - "open": 19469, - "volume": 119532 - }, - "open": "2020-10-21T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 93100, - "high": 13036, - "low": 93100, - "open": 13036, - "volume": 366447 - }, - "id": 2751134, - "non_hive": { - "close": 14059, - "high": 2000, - "low": 14059, - "open": 2000, - "volume": 55382 - }, - "open": "2020-10-21T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 105732, - "high": 105732, - "low": 2747, - "open": 2323, - "volume": 210164 - }, - "id": 2751139, - "non_hive": { - "close": 16208, - "high": 16208, - "low": 420, - "open": 356, - "volume": 32209 - }, - "open": "2020-10-21T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 134715, - "high": 134715, - "low": 134715, - "open": 134715, - "volume": 134715 - }, - "id": 2751150, - "non_hive": { - "close": 20651, - "high": 20651, - "low": 20651, - "open": 20651, - "volume": 20651 - }, - "open": "2020-10-21T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1644, - "high": 51405, - "low": 1644, - "open": 51405, - "volume": 75379 - }, - "id": 2751153, - "non_hive": { - "close": 252, - "high": 7880, - "low": 252, - "open": 7880, - "volume": 11555 - }, - "open": "2020-10-21T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 9415, - "high": 9415, - "low": 9415, - "open": 9415, - "volume": 9415 - }, - "id": 2751160, - "non_hive": { - "close": 1441, - "high": 1441, - "low": 1441, - "open": 1441, - "volume": 1441 - }, - "open": "2020-10-21T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 61412, - "high": 11971, - "low": 20457, - "open": 20457, - "volume": 94036 - }, - "id": 2751163, - "non_hive": { - "close": 9408, - "high": 1834, - "low": 3131, - "open": 3131, - "volume": 14403 - }, - "open": "2020-10-21T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 19564, - "high": 19564, - "low": 19564, - "open": 19564, - "volume": 19564 - }, - "id": 2751168, - "non_hive": { - "close": 2997, - "high": 2997, - "low": 2997, - "open": 2997, - "volume": 2997 - }, - "open": "2020-10-21T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 27788, - "high": 27788, - "low": 10360, - "open": 10360, - "volume": 38148 - }, - "id": 2751172, - "non_hive": { - "close": 4257, - "high": 4257, - "low": 1587, - "open": 1587, - "volume": 5844 - }, - "open": "2020-10-21T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2591, - "high": 2591, - "low": 225410, - "open": 225410, - "volume": 228001 - }, - "id": 2751176, - "non_hive": { - "close": 397, - "high": 397, - "low": 34530, - "open": 34530, - "volume": 34927 - }, - "open": "2020-10-21T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 24000, - "high": 24000, - "low": 24000, - "open": 24000, - "volume": 24000 - }, - "id": 2751180, - "non_hive": { - "close": 3676, - "high": 3676, - "low": 3676, - "open": 3676, - "volume": 3676 - }, - "open": "2020-10-21T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 34799, - "high": 1925, - "low": 34799, - "open": 1925, - "volume": 36724 - }, - "id": 2751183, - "non_hive": { - "close": 5331, - "high": 295, - "low": 5331, - "open": 295, - "volume": 5626 - }, - "open": "2020-10-21T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 143432, - "high": 143432, - "low": 143432, - "open": 143432, - "volume": 143432 - }, - "id": 2751188, - "non_hive": { - "close": 22000, - "high": 22000, - "low": 22000, - "open": 22000, - "volume": 22000 - }, - "open": "2020-10-21T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 379, - "high": 379, - "low": 379, - "open": 379, - "volume": 379 - }, - "id": 2751191, - "non_hive": { - "close": 58, - "high": 58, - "low": 58, - "open": 58, - "volume": 58 - }, - "open": "2020-10-21T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 67075, - "high": 67075, - "low": 67075, - "open": 67075, - "volume": 67075 - }, - "id": 2751194, - "non_hive": { - "close": 10283, - "high": 10283, - "low": 10283, - "open": 10283, - "volume": 10283 - }, - "open": "2020-10-21T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 104081, - "high": 104081, - "low": 104081, - "open": 104081, - "volume": 104081 - }, - "id": 2751198, - "non_hive": { - "close": 15956, - "high": 15956, - "low": 15956, - "open": 15956, - "volume": 15956 - }, - "open": "2020-10-21T20:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13047, - "high": 13047, - "low": 13047, - "open": 13047, - "volume": 13047 - }, - "id": 2751201, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-21T20:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1540, - "high": 13243, - "low": 1540, - "open": 13243, - "volume": 23028 - }, - "id": 2751204, - "non_hive": { - "close": 232, - "high": 2000, - "low": 232, - "open": 2000, - "volume": 3477 - }, - "open": "2020-10-21T20:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 234, - "high": 234, - "low": 13046, - "open": 13046, - "volume": 13280 - }, - "id": 2751207, - "non_hive": { - "close": 36, - "high": 36, - "low": 1999, - "open": 1999, - "volume": 2035 - }, - "open": "2020-10-21T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 17128, - "high": 660797, - "low": 197, - "open": 197, - "volume": 708842 - }, - "id": 2751212, - "non_hive": { - "close": 2625, - "high": 101302, - "low": 30, - "open": 30, - "volume": 108666 - }, - "open": "2020-10-21T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 150000, - "high": 16096, - "low": 150000, - "open": 16096, - "volume": 166096 - }, - "id": 2751220, - "non_hive": { - "close": 22995, - "high": 2468, - "low": 22995, - "open": 2468, - "volume": 25463 - }, - "open": "2020-10-21T20:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 19811, - "high": 48479, - "low": 19811, - "open": 48479, - "volume": 133279 - }, - "id": 2751225, - "non_hive": { - "close": 3037, - "high": 7433, - "low": 3037, - "open": 7433, - "volume": 20434 - }, - "open": "2020-10-21T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 303994, - "high": 2276, - "low": 303994, - "open": 2276, - "volume": 306270 - }, - "id": 2751231, - "non_hive": { - "close": 46510, - "high": 349, - "low": 46510, - "open": 349, - "volume": 46859 - }, - "open": "2020-10-21T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 32578, - "high": 32578, - "low": 32578, - "open": 32578, - "volume": 32578 - }, - "id": 2751236, - "non_hive": { - "close": 4995, - "high": 4995, - "low": 4995, - "open": 4995, - "volume": 4995 - }, - "open": "2020-10-21T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6952, - "high": 6952, - "low": 6952, - "open": 6952, - "volume": 6952 - }, - "id": 2751239, - "non_hive": { - "close": 1066, - "high": 1066, - "low": 1066, - "open": 1066, - "volume": 1066 - }, - "open": "2020-10-21T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3879, - "high": 3879, - "low": 3879, - "open": 3879, - "volume": 3879 - }, - "id": 2751242, - "non_hive": { - "close": 595, - "high": 595, - "low": 595, - "open": 595, - "volume": 595 - }, - "open": "2020-10-21T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 4170, - "high": 2125, - "low": 4170, - "open": 2125, - "volume": 24155 - }, - "id": 2751245, - "non_hive": { - "close": 635, - "high": 325, - "low": 635, - "open": 325, - "volume": 3680 - }, - "open": "2020-10-21T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6575, - "high": 6575, - "low": 6575, - "open": 6575, - "volume": 6575 - }, - "id": 2751248, - "non_hive": { - "close": 999, - "high": 999, - "low": 999, - "open": 999, - "volume": 999 - }, - "open": "2020-10-21T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 13242, - "high": 1500, - "low": 13242, - "open": 1500, - "volume": 54468 - }, - "id": 2751251, - "non_hive": { - "close": 2000, - "high": 228, - "low": 2000, - "open": 228, - "volume": 8228 - }, - "open": "2020-10-21T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6736, - "high": 6508, - "low": 6736, - "open": 82105, - "volume": 148321 - }, - "id": 2751260, - "non_hive": { - "close": 1017, - "high": 983, - "low": 1017, - "open": 12399, - "volume": 22399 - }, - "open": "2020-10-21T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 11309, - "high": 11309, - "low": 13244, - "open": 13244, - "volume": 24553 - }, - "id": 2751270, - "non_hive": { - "close": 1718, - "high": 1718, - "low": 2011, - "open": 2011, - "volume": 3729 - }, - "open": "2020-10-21T22:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 53, - "high": 9145, - "low": 53, - "open": 9145, - "volume": 48133 - }, - "id": 2751274, - "non_hive": { - "close": 8, - "high": 1381, - "low": 8, - "open": 1381, - "volume": 7266 - }, - "open": "2020-10-21T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 12719, - "high": 13, - "low": 12719, - "open": 3312, - "volume": 290268 - }, - "id": 2751279, - "non_hive": { - "close": 1909, - "high": 2, - "low": 1909, - "open": 501, - "volume": 43576 - }, - "open": "2020-10-21T22:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 594, - "high": 594, - "low": 594, - "open": 594, - "volume": 594 - }, - "id": 2751284, - "non_hive": { - "close": 89, - "high": 89, - "low": 89, - "open": 89, - "volume": 89 - }, - "open": "2020-10-21T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 17565, - "high": 19395, - "low": 17565, - "open": 19395, - "volume": 37000 - }, - "id": 2751287, - "non_hive": { - "close": 2634, - "high": 2911, - "low": 2634, - "open": 2911, - "volume": 5551 - }, - "open": "2020-10-21T22:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 494, - "high": 494, - "low": 494, - "open": 494, - "volume": 494 - }, - "id": 2751290, - "non_hive": { - "close": 75, - "high": 75, - "low": 75, - "open": 75, - "volume": 75 - }, - "open": "2020-10-21T23:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 223, - "high": 223, - "low": 12673, - "open": 499, - "volume": 38851 - }, - "id": 2751294, - "non_hive": { - "close": 34, - "high": 34, - "low": 1900, - "open": 75, - "volume": 5827 - }, - "open": "2020-10-21T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 64139, - "high": 64139, - "low": 225, - "open": 1245, - "volume": 180881 - }, - "id": 2751299, - "non_hive": { - "close": 9749, - "high": 9749, - "low": 34, - "open": 189, - "volume": 27488 - }, - "open": "2020-10-21T23:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 15917, - "high": 15917, - "low": 13160, - "open": 13160, - "volume": 29077 - }, - "id": 2751304, - "non_hive": { - "close": 2419, - "high": 2419, - "low": 1999, - "open": 1999, - "volume": 4418 - }, - "open": "2020-10-21T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 72807, - "high": 13871, - "low": 72807, - "open": 13871, - "volume": 100000 - }, - "id": 2751307, - "non_hive": { - "close": 10935, - "high": 2108, - "low": 10935, - "open": 2108, - "volume": 15067 - }, - "open": "2020-10-22T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6003, - "high": 6003, - "low": 6003, - "open": 6003, - "volume": 6003 - }, - "id": 2751312, - "non_hive": { - "close": 912, - "high": 912, - "low": 912, - "open": 912, - "volume": 912 - }, - "open": "2020-10-22T01:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 15057, - "high": 16301, - "low": 3037, - "open": 16301, - "volume": 71302 - }, - "id": 2751316, - "non_hive": { - "close": 2261, - "high": 2476, - "low": 456, - "open": 2476, - "volume": 10737 - }, - "open": "2020-10-22T01:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11480, - "high": 11480, - "low": 11480, - "open": 11480, - "volume": 11480 - }, - "id": 2751324, - "non_hive": { - "close": 1744, - "high": 1744, - "low": 1744, - "open": 1744, - "volume": 1744 - }, - "open": "2020-10-22T01:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 4353, - "high": 4353, - "low": 2137, - "open": 2137, - "volume": 19805 - }, - "id": 2751327, - "non_hive": { - "close": 654, - "high": 654, - "low": 320, - "open": 320, - "volume": 2974 - }, - "open": "2020-10-22T02:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3503, - "high": 6, - "low": 3503, - "open": 6, - "volume": 3509 - }, - "id": 2751334, - "non_hive": { - "close": 526, - "high": 1, - "low": 526, - "open": 1, - "volume": 527 - }, - "open": "2020-10-22T02:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 148, - "high": 6, - "low": 148, - "open": 6, - "volume": 421 - }, - "id": 2751337, - "non_hive": { - "close": 22, - "high": 1, - "low": 22, - "open": 1, - "volume": 63 - }, - "open": "2020-10-22T02:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 25127, - "high": 6, - "low": 46709, - "open": 6, - "volume": 73521 - }, - "id": 2751342, - "non_hive": { - "close": 3819, - "high": 1, - "low": 6958, - "open": 1, - "volume": 11033 - }, - "open": "2020-10-22T02:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6281, - "high": 6281, - "low": 6281, - "open": 6281, - "volume": 6281 - }, - "id": 2751345, - "non_hive": { - "close": 945, - "high": 945, - "low": 945, - "open": 945, - "volume": 945 - }, - "open": "2020-10-22T03:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 93, - "high": 93, - "low": 93, - "open": 93, - "volume": 93 - }, - "id": 2751349, - "non_hive": { - "close": 14, - "high": 14, - "low": 14, - "open": 14, - "volume": 14 - }, - "open": "2020-10-22T04:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1240338, - "high": 1240338, - "low": 13159, - "open": 13159, - "volume": 1311456 - }, - "id": 2751353, - "non_hive": { - "close": 188529, - "high": 188529, - "low": 1999, - "open": 1999, - "volume": 199337 - }, - "open": "2020-10-22T04:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1456579, - "high": 1456579, - "low": 13158, - "open": 13158, - "volume": 2165260 - }, - "id": 2751356, - "non_hive": { - "close": 221400, - "high": 221400, - "low": 1999, - "open": 1999, - "volume": 329117 - }, - "open": "2020-10-22T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13158, - "high": 13158, - "low": 13158, - "open": 13158, - "volume": 13158 - }, - "id": 2751359, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-22T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 703954, - "high": 703954, - "low": 703954, - "open": 703954, - "volume": 703954 - }, - "id": 2751363, - "non_hive": { - "close": 107001, - "high": 107001, - "low": 107001, - "open": 107001, - "volume": 107001 - }, - "open": "2020-10-22T05:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 19300, - "high": 19300, - "low": 280, - "open": 280, - "volume": 32652 - }, - "id": 2751366, - "non_hive": { - "close": 2953, - "high": 2953, - "low": 42, - "open": 42, - "volume": 4994 - }, - "open": "2020-10-22T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2751369, - "non_hive": { - "close": 153, - "high": 153, - "low": 153, - "open": 153, - "volume": 153 - }, - "open": "2020-10-22T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13072, - "high": 13072, - "low": 13072, - "open": 13072, - "volume": 13072 - }, - "id": 2751372, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-22T06:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 527843, - "high": 527843, - "low": 527843, - "open": 527843, - "volume": 527843 - }, - "id": 2751376, - "non_hive": { - "close": 80760, - "high": 80760, - "low": 80760, - "open": 80760, - "volume": 80760 - }, - "open": "2020-10-22T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3395, - "high": 10646, - "low": 3395, - "open": 10646, - "volume": 14041 - }, - "id": 2751379, - "non_hive": { - "close": 519, - "high": 1628, - "low": 519, - "open": 1628, - "volume": 2147 - }, - "open": "2020-10-22T06:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6542, - "high": 6542, - "low": 9684, - "open": 9684, - "volume": 16226 - }, - "id": 2751383, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1480, - "open": 1480, - "volume": 2480 - }, - "open": "2020-10-22T06:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 98188, - "high": 98188, - "low": 98188, - "open": 98188, - "volume": 98188 - }, - "id": 2751388, - "non_hive": { - "close": 15007, - "high": 15007, - "low": 15007, - "open": 15007, - "volume": 15007 - }, - "open": "2020-10-22T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 419, - "high": 674, - "low": 419, - "open": 674, - "volume": 1093 - }, - "id": 2751392, - "non_hive": { - "close": 64, - "high": 103, - "low": 64, - "open": 103, - "volume": 167 - }, - "open": "2020-10-22T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13093, - "high": 15744, - "low": 13093, - "open": 15744, - "volume": 28837 - }, - "id": 2751395, - "non_hive": { - "close": 1999, - "high": 2405, - "low": 1999, - "open": 2405, - "volume": 4404 - }, - "open": "2020-10-22T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13289, - "high": 4220, - "low": 13289, - "open": 4220, - "volume": 17509 - }, - "id": 2751399, - "non_hive": { - "close": 2031, - "high": 645, - "low": 2031, - "open": 645, - "volume": 2676 - }, - "open": "2020-10-22T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 7786, - "high": 7786, - "low": 7786, - "open": 7786, - "volume": 7786 - }, - "id": 2751404, - "non_hive": { - "close": 1188, - "high": 1188, - "low": 1188, - "open": 1188, - "volume": 1188 - }, - "open": "2020-10-22T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 16081, - "high": 16081, - "low": 16081, - "open": 16081, - "volume": 16081 - }, - "id": 2751407, - "non_hive": { - "close": 2459, - "high": 2459, - "low": 2459, - "open": 2459, - "volume": 2459 - }, - "open": "2020-10-22T07:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 22738, - "high": 22738, - "low": 15440, - "open": 15440, - "volume": 38178 - }, - "id": 2751410, - "non_hive": { - "close": 3477, - "high": 3477, - "low": 2361, - "open": 2361, - "volume": 5838 - }, - "open": "2020-10-22T07:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 80399, - "high": 9273, - "low": 80399, - "open": 9273, - "volume": 89672 - }, - "id": 2751415, - "non_hive": { - "close": 12277, - "high": 1416, - "low": 12277, - "open": 1416, - "volume": 13693 - }, - "open": "2020-10-22T08:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 769337, - "high": 769337, - "low": 268993, - "open": 54335, - "volume": 3690961 - }, - "id": 2751420, - "non_hive": { - "close": 118368, - "high": 118368, - "low": 41075, - "open": 8297, - "volume": 565903 - }, - "open": "2020-10-22T08:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 4143, - "high": 4143, - "low": 4143, - "open": 4143, - "volume": 4143 - }, - "id": 2751425, - "non_hive": { - "close": 648, - "high": 648, - "low": 648, - "open": 648, - "volume": 648 - }, - "open": "2020-10-22T09:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 113091, - "high": 173912, - "low": 113091, - "open": 7626, - "volume": 307699 - }, - "id": 2751429, - "non_hive": { - "close": 17302, - "high": 27095, - "low": 17302, - "open": 1188, - "volume": 47585 - }, - "open": "2020-10-22T09:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1330, - "high": 1330, - "low": 1330, - "open": 1330, - "volume": 1330 - }, - "id": 2751434, - "non_hive": { - "close": 207, - "high": 207, - "low": 207, - "open": 207, - "volume": 207 - }, - "open": "2020-10-22T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 3959, - "high": 3959, - "low": 3959, - "open": 3959, - "volume": 3959 - }, - "id": 2751437, - "non_hive": { - "close": 606, - "high": 606, - "low": 606, - "open": 606, - "volume": 606 - }, - "open": "2020-10-22T09:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 12903, - "high": 2694, - "low": 12903, - "open": 2694, - "volume": 41403 - }, - "id": 2751440, - "non_hive": { - "close": 2000, - "high": 420, - "low": 2000, - "open": 420, - "volume": 6420 - }, - "open": "2020-10-22T10:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12903, - "high": 12903, - "low": 12903, - "open": 12903, - "volume": 38709 - }, - "id": 2751450, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 6000 - }, - "open": "2020-10-22T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 3865, - "high": 16661, - "low": 3865, - "open": 12847, - "volume": 294060 - }, - "id": 2751456, - "non_hive": { - "close": 599, - "high": 2599, - "low": 599, - "open": 1999, - "volume": 45751 - }, - "open": "2020-10-22T10:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 27172, - "high": 27172, - "low": 77917, - "open": 12984, - "volume": 120637 - }, - "id": 2751464, - "non_hive": { - "close": 4228, - "high": 4228, - "low": 12000, - "open": 2000, - "volume": 18626 - }, - "open": "2020-10-22T10:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1331, - "high": 1331, - "low": 1331, - "open": 1331, - "volume": 1331 - }, - "id": 2751469, - "non_hive": { - "close": 207, - "high": 207, - "low": 207, - "open": 207, - "volume": 207 - }, - "open": "2020-10-22T10:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 258, - "high": 4049, - "low": 258, - "open": 4049, - "volume": 4307 - }, - "id": 2751472, - "non_hive": { - "close": 40, - "high": 628, - "low": 40, - "open": 628, - "volume": 668 - }, - "open": "2020-10-22T11:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 269767, - "high": 269767, - "low": 269767, - "open": 269767, - "volume": 269767 - }, - "id": 2751476, - "non_hive": { - "close": 41814, - "high": 41814, - "low": 41814, - "open": 41814, - "volume": 41814 - }, - "open": "2020-10-22T11:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2942, - "high": 2942, - "low": 2942, - "open": 2942, - "volume": 2942 - }, - "id": 2751479, - "non_hive": { - "close": 456, - "high": 456, - "low": 456, - "open": 456, - "volume": 456 - }, - "open": "2020-10-22T11:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 11076, - "high": 11076, - "low": 11076, - "open": 11076, - "volume": 11076 - }, - "id": 2751482, - "non_hive": { - "close": 1715, - "high": 1715, - "low": 1715, - "open": 1715, - "volume": 1715 - }, - "open": "2020-10-22T11:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 2439, - "high": 4689, - "low": 2439, - "open": 2674, - "volume": 116201 - }, - "id": 2751485, - "non_hive": { - "close": 375, - "high": 726, - "low": 375, - "open": 414, - "volume": 17987 - }, - "open": "2020-10-22T11:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6454, - "high": 6454, - "low": 6454, - "open": 6454, - "volume": 6454 - }, - "id": 2751493, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-22T11:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1464, - "high": 1464, - "low": 1464, - "open": 1464, - "volume": 1464 - }, - "id": 2751496, - "non_hive": { - "close": 227, - "high": 227, - "low": 227, - "open": 227, - "volume": 227 - }, - "open": "2020-10-22T12:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 23048, - "high": 5052, - "low": 23048, - "open": 5052, - "volume": 100000 - }, - "id": 2751500, - "non_hive": { - "close": 3549, - "high": 783, - "low": 3549, - "open": 783, - "volume": 15409 - }, - "open": "2020-10-22T12:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 11224, - "high": 11224, - "low": 32528, - "open": 34675, - "volume": 911224 - }, - "id": 2751503, - "non_hive": { - "close": 1738, - "high": 1738, - "low": 4890, - "open": 5340, - "volume": 138976 - }, - "open": "2020-10-22T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 4318, - "high": 4318, - "low": 1686, - "open": 1686, - "volume": 6004 - }, - "id": 2751509, - "non_hive": { - "close": 669, - "high": 669, - "low": 261, - "open": 261, - "volume": 930 - }, - "open": "2020-10-22T12:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12916, - "high": 12916, - "low": 4269, - "open": 731, - "volume": 17916 - }, - "id": 2751512, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 640, - "open": 110, - "volume": 2749 - }, - "open": "2020-10-22T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 2792, - "high": 2208, - "low": 2792, - "open": 2208, - "volume": 5000 - }, - "id": 2751516, - "non_hive": { - "close": 432, - "high": 342, - "low": 432, - "open": 342, - "volume": 774 - }, - "open": "2020-10-22T12:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 10675, - "high": 18721, - "low": 10675, - "open": 18721, - "volume": 73955 - }, - "id": 2751519, - "non_hive": { - "close": 1653, - "high": 2899, - "low": 1653, - "open": 2899, - "volume": 11452 - }, - "open": "2020-10-22T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 36519, - "high": 36519, - "low": 325, - "open": 6707, - "volume": 56460 - }, - "id": 2751525, - "non_hive": { - "close": 5658, - "high": 5658, - "low": 50, - "open": 1038, - "volume": 8745 - }, - "open": "2020-10-22T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 65516, - "high": 65516, - "low": 5812, - "open": 7097, - "volume": 123872 - }, - "id": 2751531, - "non_hive": { - "close": 10151, - "high": 10151, - "low": 900, - "open": 1099, - "volume": 19191 - }, - "open": "2020-10-22T13:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 13323, - "high": 13323, - "low": 13323, - "open": 13323, - "volume": 13323 - }, - "id": 2751536, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-22T13:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 11345, - "high": 11345, - "low": 11345, - "open": 11345, - "volume": 11345 - }, - "id": 2751539, - "non_hive": { - "close": 1703, - "high": 1703, - "low": 1703, - "open": 1703, - "volume": 1703 - }, - "open": "2020-10-22T13:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 113173, - "high": 113173, - "low": 6150, - "open": 6150, - "volume": 139323 - }, - "id": 2751542, - "non_hive": { - "close": 17535, - "high": 17535, - "low": 923, - "open": 923, - "volume": 21556 - }, - "open": "2020-10-22T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6061, - "high": 3939, - "low": 6061, - "open": 3939, - "volume": 10000 - }, - "id": 2751546, - "non_hive": { - "close": 938, - "high": 610, - "low": 938, - "open": 610, - "volume": 1548 - }, - "open": "2020-10-22T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 8582, - "high": 8582, - "low": 8582, - "open": 8582, - "volume": 8582 - }, - "id": 2751549, - "non_hive": { - "close": 1329, - "high": 1329, - "low": 1329, - "open": 1329, - "volume": 1329 - }, - "open": "2020-10-22T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 10434, - "high": 10434, - "low": 10434, - "open": 10434, - "volume": 10434 - }, - "id": 2751552, - "non_hive": { - "close": 1616, - "high": 1616, - "low": 1616, - "open": 1616, - "volume": 1616 - }, - "open": "2020-10-22T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 6, - "high": 6, - "low": 2474, - "open": 2474, - "volume": 15394 - }, - "id": 2751555, - "non_hive": { - "close": 1, - "high": 1, - "low": 383, - "open": 383, - "volume": 2384 - }, - "open": "2020-10-22T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 5101, - "high": 5101, - "low": 5101, - "open": 5101, - "volume": 5101 - }, - "id": 2751560, - "non_hive": { - "close": 790, - "high": 790, - "low": 790, - "open": 790, - "volume": 790 - }, - "open": "2020-10-22T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 141139, - "high": 25, - "low": 141139, - "open": 4998, - "volume": 305023 - }, - "id": 2751563, - "non_hive": { - "close": 21034, - "high": 4, - "low": 21034, - "open": 774, - "volume": 45706 - }, - "open": "2020-10-22T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 4703, - "high": 4703, - "low": 4703, - "open": 4703, - "volume": 4703 - }, - "id": 2751569, - "non_hive": { - "close": 701, - "high": 701, - "low": 701, - "open": 701, - "volume": 701 - }, - "open": "2020-10-22T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1006, - "high": 1006, - "low": 1006, - "open": 1006, - "volume": 1006 - }, - "id": 2751572, - "non_hive": { - "close": 150, - "high": 150, - "low": 150, - "open": 150, - "volume": 150 - }, - "open": "2020-10-22T15:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 4250, - "high": 15750, - "low": 4250, - "open": 15750, - "volume": 20000 - }, - "id": 2751575, - "non_hive": { - "close": 633, - "high": 2347, - "low": 633, - "open": 2347, - "volume": 2980 - }, - "open": "2020-10-22T15:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 24702, - "high": 24702, - "low": 24702, - "open": 24702, - "volume": 24702 - }, - "id": 2751578, - "non_hive": { - "close": 3681, - "high": 3681, - "low": 3681, - "open": 3681, - "volume": 3681 - }, - "open": "2020-10-22T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 181, - "high": 181, - "low": 181, - "open": 181, - "volume": 181 - }, - "id": 2751581, - "non_hive": { - "close": 28, - "high": 28, - "low": 28, - "open": 28, - "volume": 28 - }, - "open": "2020-10-22T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 136, - "high": 136, - "low": 136, - "open": 136, - "volume": 136 - }, - "id": 2751584, - "non_hive": { - "close": 21, - "high": 21, - "low": 21, - "open": 21, - "volume": 21 - }, - "open": "2020-10-22T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 91, - "high": 91, - "low": 91, - "open": 91, - "volume": 91 - }, - "id": 2751588, - "non_hive": { - "close": 14, - "high": 14, - "low": 14, - "open": 14, - "volume": 14 - }, - "open": "2020-10-22T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13008, - "high": 13008, - "low": 13008, - "open": 13008, - "volume": 13008 - }, - "id": 2751591, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-22T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1704, - "high": 1704, - "low": 1704, - "open": 1704, - "volume": 1704 - }, - "id": 2751594, - "non_hive": { - "close": 262, - "high": 262, - "low": 262, - "open": 262, - "volume": 262 - }, - "open": "2020-10-22T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 11304, - "high": 11304, - "low": 11304, - "open": 11304, - "volume": 11304 - }, - "id": 2751597, - "non_hive": { - "close": 1737, - "high": 1737, - "low": 1737, - "open": 1737, - "volume": 1737 - }, - "open": "2020-10-22T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13008, - "high": 13008, - "low": 13008, - "open": 13008, - "volume": 13008 - }, - "id": 2751600, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-22T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 8000, - "high": 8000, - "low": 13008, - "open": 13008, - "volume": 21008 - }, - "id": 2751603, - "non_hive": { - "close": 1230, - "high": 1230, - "low": 1999, - "open": 1999, - "volume": 3229 - }, - "open": "2020-10-22T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 34098, - "high": 34098, - "low": 560, - "open": 4463, - "volume": 82471 - }, - "id": 2751606, - "non_hive": { - "close": 5278, - "high": 5278, - "low": 86, - "open": 690, - "volume": 12758 - }, - "open": "2020-10-22T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 7272, - "high": 7272, - "low": 85605, - "open": 85605, - "volume": 92877 - }, - "id": 2751611, - "non_hive": { - "close": 1126, - "high": 1126, - "low": 13254, - "open": 13254, - "volume": 14380 - }, - "open": "2020-10-22T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 182, - "high": 7314, - "low": 182, - "open": 7314, - "volume": 7496 - }, - "id": 2751617, - "non_hive": { - "close": 28, - "high": 1132, - "low": 28, - "open": 1132, - "volume": 1160 - }, - "open": "2020-10-22T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 96559, - "high": 96559, - "low": 96559, - "open": 96559, - "volume": 96559 - }, - "id": 2751620, - "non_hive": { - "close": 14950, - "high": 14950, - "low": 14950, - "open": 14950, - "volume": 14950 - }, - "open": "2020-10-22T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12936, - "high": 1086, - "low": 12936, - "open": 1086, - "volume": 27000 - }, - "id": 2751623, - "non_hive": { - "close": 1993, - "high": 168, - "low": 1993, - "open": 168, - "volume": 4161 - }, - "open": "2020-10-22T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11690, - "high": 11690, - "low": 11690, - "open": 11690, - "volume": 11690 - }, - "id": 2751626, - "non_hive": { - "close": 1809, - "high": 1809, - "low": 1809, - "open": 1809, - "volume": 1809 - }, - "open": "2020-10-22T17:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 5457, - "high": 5457, - "low": 1228, - "open": 1228, - "volume": 6685 - }, - "id": 2751629, - "non_hive": { - "close": 845, - "high": 845, - "low": 190, - "open": 190, - "volume": 1035 - }, - "open": "2020-10-22T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6445, - "high": 6445, - "low": 6445, - "open": 6445, - "volume": 6445 - }, - "id": 2751633, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-10-22T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 16005, - "high": 8984, - "low": 60005, - "open": 8984, - "volume": 84994 - }, - "id": 2751636, - "non_hive": { - "close": 2478, - "high": 1391, - "low": 9290, - "open": 1391, - "volume": 13159 - }, - "open": "2020-10-22T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13093, - "high": 13093, - "low": 13093, - "open": 13093, - "volume": 13093 - }, - "id": 2751643, - "non_hive": { - "close": 2027, - "high": 2027, - "low": 2027, - "open": 2027, - "volume": 2027 - }, - "open": "2020-10-22T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 310, - "high": 310, - "low": 310, - "open": 310, - "volume": 310 - }, - "id": 2751646, - "non_hive": { - "close": 48, - "high": 48, - "low": 48, - "open": 48, - "volume": 48 - }, - "open": "2020-10-22T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 8494, - "high": 8494, - "low": 8494, - "open": 8494, - "volume": 8494 - }, - "id": 2751649, - "non_hive": { - "close": 1315, - "high": 1315, - "low": 1315, - "open": 1315, - "volume": 1315 - }, - "open": "2020-10-22T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 15004, - "high": 15004, - "low": 15004, - "open": 15004, - "volume": 15004 - }, - "id": 2751652, - "non_hive": { - "close": 2323, - "high": 2323, - "low": 2323, - "open": 2323, - "volume": 2323 - }, - "open": "2020-10-22T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6452, - "high": 6452, - "low": 6452, - "open": 6452, - "volume": 6452 - }, - "id": 2751655, - "non_hive": { - "close": 999, - "high": 999, - "low": 999, - "open": 999, - "volume": 999 - }, - "open": "2020-10-22T19:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 19383, - "high": 19383, - "low": 25842, - "open": 25842, - "volume": 45225 - }, - "id": 2751659, - "non_hive": { - "close": 3001, - "high": 3001, - "low": 4001, - "open": 4001, - "volume": 7002 - }, - "open": "2020-10-22T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 28917, - "high": 28917, - "low": 28917, - "open": 28917, - "volume": 28917 - }, - "id": 2751664, - "non_hive": { - "close": 4477, - "high": 4477, - "low": 4477, - "open": 4477, - "volume": 4477 - }, - "open": "2020-10-22T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 400, - "high": 400, - "low": 620, - "open": 620, - "volume": 80150 - }, - "id": 2751667, - "non_hive": { - "close": 62, - "high": 62, - "low": 96, - "open": 96, - "volume": 12412 - }, - "open": "2020-10-22T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 400, - "high": 400, - "low": 400, - "open": 400, - "volume": 400 - }, - "id": 2751672, - "non_hive": { - "close": 62, - "high": 62, - "low": 62, - "open": 62, - "volume": 62 - }, - "open": "2020-10-22T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11087, - "high": 11087, - "low": 11087, - "open": 11087, - "volume": 11087 - }, - "id": 2751675, - "non_hive": { - "close": 1717, - "high": 1717, - "low": 1717, - "open": 1717, - "volume": 1717 - }, - "open": "2020-10-22T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 665, - "high": 665, - "low": 17705, - "open": 17705, - "volume": 18370 - }, - "id": 2751678, - "non_hive": { - "close": 103, - "high": 103, - "low": 2741, - "open": 2741, - "volume": 2844 - }, - "open": "2020-10-22T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 52, - "high": 200, - "low": 52, - "open": 200, - "volume": 71985 - }, - "id": 2751684, - "non_hive": { - "close": 8, - "high": 31, - "low": 8, - "open": 31, - "volume": 11130 - }, - "open": "2020-10-22T20:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4546, - "high": 74799, - "low": 4546, - "open": 74799, - "volume": 80475 - }, - "id": 2751689, - "non_hive": { - "close": 700, - "high": 11519, - "low": 700, - "open": 11519, - "volume": 12393 - }, - "open": "2020-10-22T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 46, - "high": 9010, - "low": 46, - "open": 9010, - "volume": 9056 - }, - "id": 2751693, - "non_hive": { - "close": 7, - "high": 1387, - "low": 7, - "open": 1387, - "volume": 1394 - }, - "open": "2020-10-22T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 46518, - "high": 10852, - "low": 228, - "open": 228, - "volume": 57598 - }, - "id": 2751696, - "non_hive": { - "close": 7141, - "high": 1666, - "low": 35, - "open": 35, - "volume": 8842 - }, - "open": "2020-10-22T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 13128, - "high": 13128, - "low": 13128, - "open": 13128, - "volume": 166966 - }, - "id": 2751703, - "non_hive": { - "close": 1998, - "high": 2000, - "low": 1998, - "open": 2000, - "volume": 25419 - }, - "open": "2020-10-22T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 11458, - "high": 13139, - "low": 11458, - "open": 13139, - "volume": 128476 - }, - "id": 2751708, - "non_hive": { - "close": 1742, - "high": 2000, - "low": 1742, - "open": 2000, - "volume": 19547 - }, - "open": "2020-10-22T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11458, - "high": 11458, - "low": 11458, - "open": 11458, - "volume": 11458 - }, - "id": 2751714, - "non_hive": { - "close": 1758, - "high": 1758, - "low": 1758, - "open": 1758, - "volume": 1758 - }, - "open": "2020-10-22T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 8243, - "high": 8243, - "low": 8243, - "open": 8243, - "volume": 8243 - }, - "id": 2751718, - "non_hive": { - "close": 1265, - "high": 1265, - "low": 1265, - "open": 1265, - "volume": 1265 - }, - "open": "2020-10-22T22:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 19551, - "high": 1036, - "low": 12000, - "open": 12000, - "volume": 32587 - }, - "id": 2751721, - "non_hive": { - "close": 3000, - "high": 159, - "low": 1841, - "open": 1841, - "volume": 5000 - }, - "open": "2020-10-22T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 3138, - "high": 47862, - "low": 19855, - "open": 13145, - "volume": 105036 - }, - "id": 2751726, - "non_hive": { - "close": 481, - "high": 7342, - "low": 3020, - "open": 2000, - "volume": 16069 - }, - "open": "2020-10-22T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 35541, - "high": 1571, - "low": 12771, - "open": 1571, - "volume": 84579 - }, - "id": 2751734, - "non_hive": { - "close": 5452, - "high": 241, - "low": 1940, - "open": 241, - "volume": 12926 - }, - "open": "2020-10-22T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 13012, - "high": 91170, - "low": 343, - "open": 13043, - "volume": 180182 - }, - "id": 2751742, - "non_hive": { - "close": 1999, - "high": 14022, - "low": 52, - "open": 2000, - "volume": 27673 - }, - "open": "2020-10-22T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 375, - "high": 25192, - "low": 375, - "open": 13012, - "volume": 51579 - }, - "id": 2751749, - "non_hive": { - "close": 57, - "high": 3872, - "low": 57, - "open": 1999, - "volume": 7926 - }, - "open": "2020-10-22T23:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2500, - "high": 339, - "low": 13148, - "open": 339, - "volume": 79656 - }, - "id": 2751753, - "non_hive": { - "close": 383, - "high": 52, - "low": 2000, - "open": 52, - "volume": 12134 - }, - "open": "2020-10-22T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 13055, - "high": 6, - "low": 47, - "open": 13121, - "volume": 171662 - }, - "id": 2751765, - "non_hive": { - "close": 1999, - "high": 1, - "low": 7, - "open": 2000, - "volume": 26240 - }, - "open": "2020-10-22T23:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 10606, - "high": 6, - "low": 10606, - "open": 203116, - "volume": 550670 - }, - "id": 2751782, - "non_hive": { - "close": 1624, - "high": 1, - "low": 1624, - "open": 31117, - "volume": 84391 - }, - "open": "2020-10-22T23:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13037, - "high": 6, - "low": 13037, - "open": 12996, - "volume": 150856 - }, - "id": 2751793, - "non_hive": { - "close": 1999, - "high": 1, - "low": 1999, - "open": 1999, - "volume": 23178 - }, - "open": "2020-10-22T23:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 13054, - "high": 13054, - "low": 13054, - "open": 13054, - "volume": 13054 - }, - "id": 2751800, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-22T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 78, - "high": 78, - "low": 2486, - "open": 2486, - "volume": 2564 - }, - "id": 2751803, - "non_hive": { - "close": 12, - "high": 12, - "low": 381, - "open": 381, - "volume": 393 - }, - "open": "2020-10-22T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 12 - }, - "id": 2751808, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 1, - "volume": 2 - }, - "open": "2020-10-22T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1137, - "high": 1131, - "low": 1137, - "open": 1131, - "volume": 2268 - }, - "id": 2751811, - "non_hive": { - "close": 174, - "high": 174, - "low": 174, - "open": 174, - "volume": 348 - }, - "open": "2020-10-22T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 216, - "high": 216, - "low": 216, - "open": 216, - "volume": 216 - }, - "id": 2751816, - "non_hive": { - "close": 33, - "high": 33, - "low": 33, - "open": 33, - "volume": 33 - }, - "open": "2020-10-23T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 20000, - "high": 20000, - "low": 13004, - "open": 13004, - "volume": 33004 - }, - "id": 2751821, - "non_hive": { - "close": 3076, - "high": 3076, - "low": 1999, - "open": 1999, - "volume": 5075 - }, - "open": "2020-10-23T00:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13011, - "high": 15498, - "low": 13011, - "open": 36268, - "volume": 64777 - }, - "id": 2751824, - "non_hive": { - "close": 2000, - "high": 2385, - "low": 2000, - "open": 5581, - "volume": 9966 - }, - "open": "2020-10-23T00:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 4267, - "high": 4267, - "low": 13012, - "open": 13012, - "volume": 30291 - }, - "id": 2751829, - "non_hive": { - "close": 656, - "high": 656, - "low": 2000, - "open": 2000, - "volume": 4656 - }, - "open": "2020-10-23T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12255, - "high": 12255, - "low": 12255, - "open": 12255, - "volume": 12255 - }, - "id": 2751834, - "non_hive": { - "close": 1884, - "high": 1884, - "low": 1884, - "open": 1884, - "volume": 1884 - }, - "open": "2020-10-23T00:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 19431, - "high": 13157, - "low": 19431, - "open": 13157, - "volume": 85000 - }, - "id": 2751837, - "non_hive": { - "close": 2953, - "high": 2000, - "low": 2953, - "open": 2000, - "volume": 12919 - }, - "open": "2020-10-23T00:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 475, - "high": 475, - "low": 475, - "open": 475, - "volume": 475 - }, - "id": 2751840, - "non_hive": { - "close": 73, - "high": 73, - "low": 73, - "open": 73, - "volume": 73 - }, - "open": "2020-10-23T01:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 3652, - "high": 3652, - "low": 3652, - "open": 3652, - "volume": 3652 - }, - "id": 2751844, - "non_hive": { - "close": 561, - "high": 561, - "low": 561, - "open": 561, - "volume": 561 - }, - "open": "2020-10-23T01:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 14000, - "high": 14000, - "low": 14000, - "open": 14000, - "volume": 14000 - }, - "id": 2751847, - "non_hive": { - "close": 2150, - "high": 2150, - "low": 2150, - "open": 2150, - "volume": 2150 - }, - "open": "2020-10-23T01:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 702, - "high": 702, - "low": 9362, - "open": 35000, - "volume": 45064 - }, - "id": 2751850, - "non_hive": { - "close": 108, - "high": 108, - "low": 1438, - "open": 5376, - "volume": 6922 - }, - "open": "2020-10-23T02:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2355, - "high": 2355, - "low": 13014, - "open": 13014, - "volume": 15369 - }, - "id": 2751856, - "non_hive": { - "close": 362, - "high": 362, - "low": 1999, - "open": 1999, - "volume": 2361 - }, - "open": "2020-10-23T02:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13014, - "high": 13014, - "low": 13014, - "open": 13014, - "volume": 13014 - }, - "id": 2751859, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-23T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 262451, - "high": 262451, - "low": 262451, - "open": 262451, - "volume": 262451 - }, - "id": 2751862, - "non_hive": { - "close": 40313, - "high": 40313, - "low": 40313, - "open": 40313, - "volume": 40313 - }, - "open": "2020-10-23T02:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13, - "high": 13, - "low": 134684, - "open": 134684, - "volume": 134697 - }, - "id": 2751865, - "non_hive": { - "close": 2, - "high": 2, - "low": 20688, - "open": 20688, - "volume": 20690 - }, - "open": "2020-10-23T03:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 130000, - "high": 130000, - "low": 67790, - "open": 67790, - "volume": 284489 - }, - "id": 2751869, - "non_hive": { - "close": 20005, - "high": 20005, - "low": 10412, - "open": 10412, - "volume": 43751 - }, - "open": "2020-10-23T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 96468, - "high": 96468, - "low": 96468, - "open": 96468, - "volume": 96468 - }, - "id": 2751872, - "non_hive": { - "close": 14856, - "high": 14856, - "low": 14856, - "open": 14856, - "volume": 14856 - }, - "open": "2020-10-23T03:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 928067, - "high": 928067, - "low": 1000000, - "open": 1000000, - "volume": 1928067 - }, - "id": 2751875, - "non_hive": { - "close": 142922, - "high": 142922, - "low": 153999, - "open": 153999, - "volume": 296921 - }, - "open": "2020-10-23T03:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 999990, - "high": 999990, - "low": 999990, - "open": 999990, - "volume": 999990 - }, - "id": 2751880, - "non_hive": { - "close": 154863, - "high": 154863, - "low": 154863, - "open": 154863, - "volume": 154863 - }, - "open": "2020-10-23T04:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 999990, - "high": 7000000, - "low": 999990, - "open": 7000000, - "volume": 7999990 - }, - "id": 2751884, - "non_hive": { - "close": 154863, - "high": 1084054, - "low": 154863, - "open": 1084054, - "volume": 1238917 - }, - "open": "2020-10-23T04:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1380000, - "high": 1380000, - "low": 2709, - "open": 2709, - "volume": 2451109 - }, - "id": 2751889, - "non_hive": { - "close": 213713, - "high": 213713, - "low": 419, - "open": 419, - "volume": 379588 - }, - "open": "2020-10-23T04:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 99996, - "high": 99996, - "low": 100000, - "open": 100000, - "volume": 199996 - }, - "id": 2751896, - "non_hive": { - "close": 15486, - "high": 15486, - "low": 15486, - "open": 15486, - "volume": 30972 - }, - "open": "2020-10-23T04:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 10002, - "high": 10002, - "low": 9901, - "open": 10099, - "volume": 30002 - }, - "id": 2751901, - "non_hive": { - "close": 1549, - "high": 1549, - "low": 1533, - "open": 1564, - "volume": 4646 - }, - "open": "2020-10-23T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 76447, - "high": 2072, - "low": 76447, - "open": 2072, - "volume": 773427 - }, - "id": 2751906, - "non_hive": { - "close": 11622, - "high": 321, - "low": 11622, - "open": 321, - "volume": 117651 - }, - "open": "2020-10-23T04:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1517, - "high": 1517, - "low": 12916, - "open": 12916, - "volume": 14433 - }, - "id": 2751911, - "non_hive": { - "close": 235, - "high": 235, - "low": 1999, - "open": 1999, - "volume": 2234 - }, - "open": "2020-10-23T05:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 12916, - "high": 12916, - "low": 12916, - "open": 12916, - "volume": 12916 - }, - "id": 2751915, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-23T05:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 91, - "high": 16540, - "low": 91, - "open": 12916, - "volume": 42463 - }, - "id": 2751918, - "non_hive": { - "close": 13, - "high": 2560, - "low": 13, - "open": 1999, - "volume": 6571 - }, - "open": "2020-10-23T05:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12916, - "high": 12916, - "low": 12916, - "open": 12916, - "volume": 25832 - }, - "id": 2751925, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-10-23T05:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1924, - "high": 1930, - "low": 4711, - "open": 4711, - "volume": 75635 - }, - "id": 2751930, - "non_hive": { - "close": 298, - "high": 299, - "low": 729, - "open": 729, - "volume": 11712 - }, - "open": "2020-10-23T05:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 91, - "high": 91, - "low": 91, - "open": 91, - "volume": 91 - }, - "id": 2751937, - "non_hive": { - "close": 14, - "high": 14, - "low": 14, - "open": 14, - "volume": 14 - }, - "open": "2020-10-23T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 10622, - "high": 10622, - "low": 10622, - "open": 10622, - "volume": 10622 - }, - "id": 2751940, - "non_hive": { - "close": 1645, - "high": 1645, - "low": 1645, - "open": 1645, - "volume": 1645 - }, - "open": "2020-10-23T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 15807, - "high": 15807, - "low": 15807, - "open": 15807, - "volume": 15807 - }, - "id": 2751943, - "non_hive": { - "close": 2448, - "high": 2448, - "low": 2448, - "open": 2448, - "volume": 2448 - }, - "open": "2020-10-23T06:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 26003, - "high": 26003, - "low": 26003, - "open": 26003, - "volume": 26003 - }, - "id": 2751947, - "non_hive": { - "close": 4027, - "high": 4027, - "low": 4027, - "open": 4027, - "volume": 4027 - }, - "open": "2020-10-23T06:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2085, - "high": 2085, - "low": 2085, - "open": 2085, - "volume": 2085 - }, - "id": 2751950, - "non_hive": { - "close": 323, - "high": 323, - "low": 323, - "open": 323, - "volume": 323 - }, - "open": "2020-10-23T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3493, - "high": 3493, - "low": 3493, - "open": 3493, - "volume": 3493 - }, - "id": 2751953, - "non_hive": { - "close": 541, - "high": 541, - "low": 541, - "open": 541, - "volume": 541 - }, - "open": "2020-10-23T06:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6808, - "high": 13154, - "low": 6808, - "open": 13154, - "volume": 21751 - }, - "id": 2751956, - "non_hive": { - "close": 1054, - "high": 2037, - "low": 1054, - "open": 2037, - "volume": 3368 - }, - "open": "2020-10-23T06:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 2060, - "high": 2060, - "low": 50217, - "open": 41228, - "volume": 125980 - }, - "id": 2751960, - "non_hive": { - "close": 319, - "high": 319, - "low": 7684, - "open": 6382, - "volume": 19393 - }, - "open": "2020-10-23T06:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 65, - "high": 7830, - "low": 65, - "open": 7830, - "volume": 7895 - }, - "id": 2751964, - "non_hive": { - "close": 10, - "high": 1212, - "low": 10, - "open": 1212, - "volume": 1222 - }, - "open": "2020-10-23T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 75043, - "high": 75043, - "low": 170, - "open": 20609, - "volume": 285850 - }, - "id": 2751967, - "non_hive": { - "close": 11616, - "high": 11616, - "low": 26, - "open": 3190, - "volume": 44246 - }, - "open": "2020-10-23T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 27702, - "high": 27702, - "low": 27702, - "open": 27702, - "volume": 27702 - }, - "id": 2751976, - "non_hive": { - "close": 4288, - "high": 4288, - "low": 4288, - "open": 4288, - "volume": 4288 - }, - "open": "2020-10-23T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 103, - "high": 103, - "low": 103, - "open": 103, - "volume": 103 - }, - "id": 2751979, - "non_hive": { - "close": 16, - "high": 16, - "low": 16, - "open": 16, - "volume": 16 - }, - "open": "2020-10-23T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13282, - "high": 13282, - "low": 31010, - "open": 31010, - "volume": 44292 - }, - "id": 2751982, - "non_hive": { - "close": 2056, - "high": 2056, - "low": 4800, - "open": 4800, - "volume": 6856 - }, - "open": "2020-10-23T07:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 12, - "high": 12, - "low": 1603, - "open": 12882, - "volume": 39564 - }, - "id": 2751985, - "non_hive": { - "close": 2, - "high": 2, - "low": 248, - "open": 1994, - "volume": 6124 - }, - "open": "2020-10-23T07:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 103930, - "high": 13070, - "low": 103930, - "open": 13070, - "volume": 463891 - }, - "id": 2751991, - "non_hive": { - "close": 15901, - "high": 2000, - "low": 15901, - "open": 2000, - "volume": 70977 - }, - "open": "2020-10-23T08:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 13148, - "high": 38783, - "low": 13148, - "open": 75000, - "volume": 188162 - }, - "id": 2751997, - "non_hive": { - "close": 1996, - "high": 6000, - "low": 1996, - "open": 11475, - "volume": 28817 - }, - "open": "2020-10-23T08:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2748, - "high": 162, - "low": 2748, - "open": 162, - "volume": 18562 - }, - "id": 2752003, - "non_hive": { - "close": 412, - "high": 25, - "low": 412, - "open": 25, - "volume": 2814 - }, - "open": "2020-10-23T08:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1315, - "high": 18685, - "low": 1315, - "open": 18685, - "volume": 20000 - }, - "id": 2752006, - "non_hive": { - "close": 202, - "high": 2876, - "low": 202, - "open": 2876, - "volume": 3078 - }, - "open": "2020-10-23T08:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 81074, - "high": 9862, - "low": 81074, - "open": 9862, - "volume": 124541 - }, - "id": 2752009, - "non_hive": { - "close": 12169, - "high": 1523, - "low": 12169, - "open": 1523, - "volume": 18739 - }, - "open": "2020-10-23T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 11228, - "high": 19968, - "low": 11228, - "open": 18035, - "volume": 49231 - }, - "id": 2752014, - "non_hive": { - "close": 1730, - "high": 3078, - "low": 1730, - "open": 2780, - "volume": 7588 - }, - "open": "2020-10-23T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 75000, - "high": 75000, - "low": 1747, - "open": 1747, - "volume": 76747 - }, - "id": 2752020, - "non_hive": { - "close": 11554, - "high": 11554, - "low": 269, - "open": 269, - "volume": 11823 - }, - "open": "2020-10-23T09:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 2004, - "high": 2004, - "low": 2004, - "open": 2004, - "volume": 2004 - }, - "id": 2752024, - "non_hive": { - "close": 309, - "high": 309, - "low": 309, - "open": 309, - "volume": 309 - }, - "open": "2020-10-23T09:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 765, - "high": 765, - "low": 44000, - "open": 44000, - "volume": 44765 - }, - "id": 2752027, - "non_hive": { - "close": 118, - "high": 118, - "low": 6778, - "open": 6778, - "volume": 6896 - }, - "open": "2020-10-23T09:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 25703, - "high": 25703, - "low": 12210, - "open": 12210, - "volume": 37913 - }, - "id": 2752031, - "non_hive": { - "close": 3962, - "high": 3962, - "low": 1881, - "open": 1881, - "volume": 5843 - }, - "open": "2020-10-23T09:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1246, - "high": 1246, - "low": 1246, - "open": 1246, - "volume": 1246 - }, - "id": 2752034, - "non_hive": { - "close": 192, - "high": 192, - "low": 192, - "open": 192, - "volume": 192 - }, - "open": "2020-10-23T09:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 11729, - "high": 11729, - "low": 11729, - "open": 11729, - "volume": 11729 - }, - "id": 2752037, - "non_hive": { - "close": 1807, - "high": 1807, - "low": 1807, - "open": 1807, - "volume": 1807 - }, - "open": "2020-10-23T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 80, - "high": 1420, - "low": 80, - "open": 1420, - "volume": 1500 - }, - "id": 2752041, - "non_hive": { - "close": 12, - "high": 219, - "low": 12, - "open": 219, - "volume": 231 - }, - "open": "2020-10-23T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2459, - "high": 2459, - "low": 2459, - "open": 2459, - "volume": 2459 - }, - "id": 2752046, - "non_hive": { - "close": 379, - "high": 379, - "low": 379, - "open": 379, - "volume": 379 - }, - "open": "2020-10-23T10:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1486, - "high": 9811, - "low": 1486, - "open": 9811, - "volume": 11297 - }, - "id": 2752049, - "non_hive": { - "close": 229, - "high": 1512, - "low": 229, - "open": 1512, - "volume": 1741 - }, - "open": "2020-10-23T10:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 901, - "high": 901, - "low": 901, - "open": 901, - "volume": 901 - }, - "id": 2752052, - "non_hive": { - "close": 139, - "high": 139, - "low": 139, - "open": 139, - "volume": 139 - }, - "open": "2020-10-23T10:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 9968, - "high": 18688, - "low": 9968, - "open": 18688, - "volume": 28656 - }, - "id": 2752055, - "non_hive": { - "close": 1535, - "high": 2880, - "low": 1535, - "open": 2880, - "volume": 4415 - }, - "open": "2020-10-23T11:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 76443, - "high": 76443, - "low": 76443, - "open": 76443, - "volume": 76443 - }, - "id": 2752060, - "non_hive": { - "close": 11781, - "high": 11781, - "low": 11781, - "open": 11781, - "volume": 11781 - }, - "open": "2020-10-23T11:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 65329, - "high": 65329, - "low": 5400, - "open": 5400, - "volume": 70729 - }, - "id": 2752063, - "non_hive": { - "close": 10070, - "high": 10070, - "low": 832, - "open": 832, - "volume": 10902 - }, - "open": "2020-10-23T11:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 233, - "high": 233, - "low": 233, - "open": 233, - "volume": 233 - }, - "id": 2752066, - "non_hive": { - "close": 36, - "high": 36, - "low": 36, - "open": 36, - "volume": 36 - }, - "open": "2020-10-23T11:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1265, - "high": 13735, - "low": 1265, - "open": 13735, - "volume": 15000 - }, - "id": 2752069, - "non_hive": { - "close": 194, - "high": 2116, - "low": 194, - "open": 2116, - "volume": 2310 - }, - "open": "2020-10-23T11:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 642, - "high": 642, - "low": 642, - "open": 642, - "volume": 642 - }, - "id": 2752072, - "non_hive": { - "close": 99, - "high": 99, - "low": 99, - "open": 99, - "volume": 99 - }, - "open": "2020-10-23T11:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 3996, - "high": 39, - "low": 3996, - "open": 39, - "volume": 334483 - }, - "id": 2752075, - "non_hive": { - "close": 614, - "high": 6, - "low": 614, - "open": 6, - "volume": 51457 - }, - "open": "2020-10-23T11:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 129874, - "high": 129874, - "low": 87152, - "open": 87152, - "volume": 3238556 - }, - "id": 2752083, - "non_hive": { - "close": 20113, - "high": 20113, - "low": 13407, - "open": 13407, - "volume": 500993 - }, - "open": "2020-10-23T12:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 11700, - "high": 11700, - "low": 11700, - "open": 11700, - "volume": 11700 - }, - "id": 2752087, - "non_hive": { - "close": 1812, - "high": 1812, - "low": 1812, - "open": 1812, - "volume": 1812 - }, - "open": "2020-10-23T12:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 13071, - "high": 3002, - "low": 13071, - "open": 13001, - "volume": 48044 - }, - "id": 2752090, - "non_hive": { - "close": 2000, - "high": 462, - "low": 2000, - "open": 2000, - "volume": 7381 - }, - "open": "2020-10-23T12:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 8995, - "high": 36207, - "low": 18, - "open": 13054, - "volume": 114499 - }, - "id": 2752097, - "non_hive": { - "close": 1379, - "high": 5598, - "low": 2, - "open": 2000, - "volume": 17604 - }, - "open": "2020-10-23T12:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 12936, - "high": 12936, - "low": 12936, - "open": 12936, - "volume": 12936 - }, - "id": 2752111, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-23T12:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 671, - "high": 671, - "low": 671, - "open": 671, - "volume": 671 - }, - "id": 2752114, - "non_hive": { - "close": 103, - "high": 103, - "low": 103, - "open": 103, - "volume": 103 - }, - "open": "2020-10-23T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 12883, - "high": 12883, - "low": 2601, - "open": 2601, - "volume": 15484 - }, - "id": 2752117, - "non_hive": { - "close": 1993, - "high": 1993, - "low": 402, - "open": 402, - "volume": 2395 - }, - "open": "2020-10-23T13:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12929, - "high": 12929, - "low": 12929, - "open": 12929, - "volume": 12929 - }, - "id": 2752121, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-23T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 9315, - "high": 9315, - "low": 9315, - "open": 9315, - "volume": 9315 - }, - "id": 2752124, - "non_hive": { - "close": 1441, - "high": 1441, - "low": 1441, - "open": 1441, - "volume": 1441 - }, - "open": "2020-10-23T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 86802, - "high": 86802, - "low": 86802, - "open": 86802, - "volume": 86802 - }, - "id": 2752127, - "non_hive": { - "close": 13427, - "high": 13427, - "low": 13427, - "open": 13427, - "volume": 13427 - }, - "open": "2020-10-23T13:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 22000, - "high": 402851, - "low": 22000, - "open": 402851, - "volume": 573547 - }, - "id": 2752130, - "non_hive": { - "close": 3401, - "high": 62319, - "low": 3401, - "open": 62319, - "volume": 88721 - }, - "open": "2020-10-23T13:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1132, - "high": 194, - "low": 1132, - "open": 194, - "volume": 1727 - }, - "id": 2752135, - "non_hive": { - "close": 175, - "high": 30, - "low": 175, - "open": 30, - "volume": 267 - }, - "open": "2020-10-23T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 13035, - "high": 653, - "low": 13035, - "open": 4000, - "volume": 40308 - }, - "id": 2752142, - "non_hive": { - "close": 2000, - "high": 101, - "low": 2000, - "open": 617, - "volume": 6200 - }, - "open": "2020-10-23T13:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 136522, - "high": 9965, - "low": 136522, - "open": 13035, - "volume": 246915 - }, - "id": 2752151, - "non_hive": { - "close": 20943, - "high": 1529, - "low": 20943, - "open": 2000, - "volume": 37879 - }, - "open": "2020-10-23T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 7, - "high": 601, - "low": 7, - "open": 16003, - "volume": 20608 - }, - "id": 2752156, - "non_hive": { - "close": 1, - "high": 93, - "low": 1, - "open": 2474, - "volume": 3185 - }, - "open": "2020-10-23T14:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "id": 2752162, - "non_hive": { - "close": 773, - "high": 773, - "low": 773, - "open": 773, - "volume": 773 - }, - "open": "2020-10-23T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12946, - "high": 7527, - "low": 12946, - "open": 7527, - "volume": 25340 - }, - "id": 2752165, - "non_hive": { - "close": 1999, - "high": 1163, - "low": 1999, - "open": 1163, - "volume": 3914 - }, - "open": "2020-10-23T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12996, - "high": 12996, - "low": 12996, - "open": 12996, - "volume": 12996 - }, - "id": 2752170, - "non_hive": { - "close": 2008, - "high": 2008, - "low": 2008, - "open": 2008, - "volume": 2008 - }, - "open": "2020-10-23T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 12299, - "high": 12299, - "low": 12299, - "open": 12299, - "volume": 12299 - }, - "id": 2752173, - "non_hive": { - "close": 1901, - "high": 1901, - "low": 1901, - "open": 1901, - "volume": 1901 - }, - "open": "2020-10-23T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12337, - "high": 12337, - "low": 12337, - "open": 12337, - "volume": 12337 - }, - "id": 2752177, - "non_hive": { - "close": 1907, - "high": 1907, - "low": 1907, - "open": 1907, - "volume": 1907 - }, - "open": "2020-10-23T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 201932, - "high": 51, - "low": 201932, - "open": 12952, - "volume": 254001 - }, - "id": 2752180, - "non_hive": { - "close": 30976, - "high": 8, - "low": 30976, - "open": 2000, - "volume": 38978 - }, - "open": "2020-10-23T15:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 83421, - "high": 5031, - "low": 83421, - "open": 5031, - "volume": 115000 - }, - "id": 2752185, - "non_hive": { - "close": 12680, - "high": 772, - "low": 12680, - "open": 772, - "volume": 17524 - }, - "open": "2020-10-23T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 15540, - "high": 2040, - "low": 15540, - "open": 2040, - "volume": 17580 - }, - "id": 2752190, - "non_hive": { - "close": 2391, - "high": 314, - "low": 2391, - "open": 314, - "volume": 2705 - }, - "open": "2020-10-23T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13009, - "high": 13001, - "low": 13009, - "open": 13001, - "volume": 33054 - }, - "id": 2752195, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 5081 - }, - "open": "2020-10-23T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 173352, - "high": 173352, - "low": 12992, - "open": 12992, - "volume": 186344 - }, - "id": 2752202, - "non_hive": { - "close": 26682, - "high": 26682, - "low": 1999, - "open": 1999, - "volume": 28681 - }, - "open": "2020-10-23T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6503, - "high": 6503, - "low": 6503, - "open": 6503, - "volume": 6503 - }, - "id": 2752206, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-23T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 97, - "high": 97, - "low": 6503, - "open": 6502, - "volume": 13102 - }, - "id": 2752209, - "non_hive": { - "close": 15, - "high": 15, - "low": 1000, - "open": 1000, - "volume": 2015 - }, - "open": "2020-10-23T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 17738, - "high": 2105, - "low": 17738, - "open": 13157, - "volume": 33000 - }, - "id": 2752215, - "non_hive": { - "close": 2696, - "high": 320, - "low": 2696, - "open": 2000, - "volume": 5016 - }, - "open": "2020-10-23T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 27520, - "high": 4561, - "low": 27520, - "open": 13028, - "volume": 163021 - }, - "id": 2752220, - "non_hive": { - "close": 4148, - "high": 700, - "low": 4148, - "open": 1999, - "volume": 24934 - }, - "open": "2020-10-23T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 34, - "high": 3168, - "low": 34, - "open": 3168, - "volume": 3202 - }, - "id": 2752225, - "non_hive": { - "close": 5, - "high": 486, - "low": 5, - "open": 486, - "volume": 491 - }, - "open": "2020-10-23T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 6503, - "high": 6503, - "low": 13048, - "open": 7782, - "volume": 27333 - }, - "id": 2752228, - "non_hive": { - "close": 997, - "high": 997, - "low": 1999, - "open": 1193, - "volume": 4189 - }, - "open": "2020-10-23T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 4018, - "high": 13156, - "low": 4018, - "open": 13156, - "volume": 30330 - }, - "id": 2752234, - "non_hive": { - "close": 610, - "high": 2000, - "low": 610, - "open": 2000, - "volume": 4610 - }, - "open": "2020-10-23T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 13157, - "high": 13157, - "low": 13157, - "open": 13157, - "volume": 13157 - }, - "id": 2752240, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-23T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13154, - "high": 16162, - "low": 13154, - "open": 13152, - "volume": 114793 - }, - "id": 2752243, - "non_hive": { - "close": 1999, - "high": 2470, - "low": 1999, - "open": 2000, - "volume": 17462 - }, - "open": "2020-10-23T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13154, - "high": 171, - "low": 13154, - "open": 13154, - "volume": 26479 - }, - "id": 2752255, - "non_hive": { - "close": 1999, - "high": 26, - "low": 1999, - "open": 1999, - "volume": 4024 - }, - "open": "2020-10-23T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 313, - "high": 18701, - "low": 313, - "open": 18701, - "volume": 48900 - }, - "id": 2752262, - "non_hive": { - "close": 47, - "high": 2863, - "low": 47, - "open": 2863, - "volume": 7485 - }, - "open": "2020-10-23T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1791, - "high": 130, - "low": 1791, - "open": 130, - "volume": 1921 - }, - "id": 2752267, - "non_hive": { - "close": 274, - "high": 20, - "low": 274, - "open": 20, - "volume": 294 - }, - "open": "2020-10-23T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 832, - "high": 832, - "low": 832, - "open": 832, - "volume": 832 - }, - "id": 2752272, - "non_hive": { - "close": 127, - "high": 127, - "low": 127, - "open": 127, - "volume": 127 - }, - "open": "2020-10-23T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13156, - "high": 14997, - "low": 13156, - "open": 14997, - "volume": 28153 - }, - "id": 2752275, - "non_hive": { - "close": 2000, - "high": 2295, - "low": 2000, - "open": 2295, - "volume": 4295 - }, - "open": "2020-10-23T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 3104, - "high": 3104, - "low": 3104, - "open": 3104, - "volume": 3104 - }, - "id": 2752281, - "non_hive": { - "close": 475, - "high": 475, - "low": 475, - "open": 475, - "volume": 475 - }, - "open": "2020-10-23T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 12, - "high": 21205, - "low": 12, - "open": 21205, - "volume": 23145 - }, - "id": 2752284, - "non_hive": { - "close": 1, - "high": 3244, - "low": 1, - "open": 3244, - "volume": 3539 - }, - "open": "2020-10-23T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 7539, - "high": 7539, - "low": 7539, - "open": 7539, - "volume": 7539 - }, - "id": 2752289, - "non_hive": { - "close": 1153, - "high": 1153, - "low": 1153, - "open": 1153, - "volume": 1153 - }, - "open": "2020-10-23T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4101, - "high": 13123, - "low": 4101, - "open": 13123, - "volume": 30347 - }, - "id": 2752292, - "non_hive": { - "close": 624, - "high": 1999, - "low": 624, - "open": 1999, - "volume": 4622 - }, - "open": "2020-10-23T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 189458, - "high": 189458, - "low": 189458, - "open": 189458, - "volume": 189458 - }, - "id": 2752298, - "non_hive": { - "close": 29000, - "high": 29000, - "low": 29000, - "open": 29000, - "volume": 29000 - }, - "open": "2020-10-23T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6494, - "high": 6494, - "low": 6494, - "open": 6494, - "volume": 6494 - }, - "id": 2752301, - "non_hive": { - "close": 993, - "high": 993, - "low": 993, - "open": 993, - "volume": 993 - }, - "open": "2020-10-23T18:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 500, - "high": 13239, - "low": 80, - "open": 13239, - "volume": 246040 - }, - "id": 2752304, - "non_hive": { - "close": 75, - "high": 2000, - "low": 12, - "open": 2000, - "volume": 37029 - }, - "open": "2020-10-23T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13332, - "high": 17820, - "low": 13332, - "open": 4915, - "volume": 73581 - }, - "id": 2752307, - "non_hive": { - "close": 2000, - "high": 2724, - "low": 2000, - "open": 751, - "volume": 11209 - }, - "open": "2020-10-23T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 15180, - "high": 13330, - "low": 67205, - "open": 13332, - "volume": 127204 - }, - "id": 2752315, - "non_hive": { - "close": 2277, - "high": 2000, - "low": 10080, - "open": 2000, - "volume": 19081 - }, - "open": "2020-10-23T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 23, - "high": 2968, - "low": 23, - "open": 2968, - "volume": 2991 - }, - "id": 2752320, - "non_hive": { - "close": 3, - "high": 453, - "low": 3, - "open": 453, - "volume": 456 - }, - "open": "2020-10-23T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13093, - "high": 13093, - "low": 13093, - "open": 13093, - "volume": 13093 - }, - "id": 2752323, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-23T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1768, - "high": 216148, - "low": 1768, - "open": 216148, - "volume": 217916 - }, - "id": 2752326, - "non_hive": { - "close": 265, - "high": 33001, - "low": 265, - "open": 33001, - "volume": 33266 - }, - "open": "2020-10-23T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 17518, - "high": 17518, - "low": 17518, - "open": 17518, - "volume": 17518 - }, - "id": 2752329, - "non_hive": { - "close": 2676, - "high": 2676, - "low": 2676, - "open": 2676, - "volume": 2676 - }, - "open": "2020-10-23T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 323374, - "high": 32729, - "low": 323374, - "open": 32729, - "volume": 387257 - }, - "id": 2752332, - "non_hive": { - "close": 48510, - "high": 5000, - "low": 48510, - "open": 5000, - "volume": 58186 - }, - "open": "2020-10-23T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 231919, - "high": 231919, - "low": 13102, - "open": 13102, - "volume": 265021 - }, - "id": 2752338, - "non_hive": { - "close": 35416, - "high": 35416, - "low": 1999, - "open": 1999, - "volume": 40468 - }, - "open": "2020-10-23T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13098, - "high": 726, - "low": 13098, - "open": 13097, - "volume": 26921 - }, - "id": 2752341, - "non_hive": { - "close": 1999, - "high": 111, - "low": 1999, - "open": 1999, - "volume": 4109 - }, - "open": "2020-10-23T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 928, - "high": 3936, - "low": 928, - "open": 3936, - "volume": 55030 - }, - "id": 2752346, - "non_hive": { - "close": 140, - "high": 601, - "low": 140, - "open": 601, - "volume": 8363 - }, - "open": "2020-10-23T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 82155, - "high": 82155, - "low": 82155, - "open": 82155, - "volume": 82155 - }, - "id": 2752354, - "non_hive": { - "close": 12482, - "high": 12482, - "low": 12482, - "open": 12482, - "volume": 12482 - }, - "open": "2020-10-23T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 66313, - "high": 66313, - "low": 34687, - "open": 34687, - "volume": 101000 - }, - "id": 2752357, - "non_hive": { - "close": 10075, - "high": 10075, - "low": 5270, - "open": 5270, - "volume": 15345 - }, - "open": "2020-10-23T20:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6551, - "high": 6551, - "low": 6551, - "open": 6551, - "volume": 6551 - }, - "id": 2752361, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-23T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 14515, - "high": 14515, - "low": 14515, - "open": 14515, - "volume": 14515 - }, - "id": 2752365, - "non_hive": { - "close": 2212, - "high": 2212, - "low": 2212, - "open": 2212, - "volume": 2212 - }, - "open": "2020-10-23T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1902, - "high": 1902, - "low": 10000, - "open": 10000, - "volume": 11902 - }, - "id": 2752368, - "non_hive": { - "close": 290, - "high": 290, - "low": 1524, - "open": 1524, - "volume": 1814 - }, - "open": "2020-10-23T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6, - "high": 6, - "low": 5279, - "open": 13, - "volume": 5298 - }, - "id": 2752373, - "non_hive": { - "close": 1, - "high": 1, - "low": 805, - "open": 2, - "volume": 808 - }, - "open": "2020-10-23T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 9707, - "high": 9707, - "low": 9707, - "open": 9707, - "volume": 9707 - }, - "id": 2752379, - "non_hive": { - "close": 1481, - "high": 1481, - "low": 1481, - "open": 1481, - "volume": 1481 - }, - "open": "2020-10-23T22:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 33971, - "high": 33971, - "low": 33971, - "open": 33971, - "volume": 33971 - }, - "id": 2752383, - "non_hive": { - "close": 5186, - "high": 5186, - "low": 5186, - "open": 5186, - "volume": 5186 - }, - "open": "2020-10-23T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 10237, - "high": 3521, - "low": 10237, - "open": 3521, - "volume": 13758 - }, - "id": 2752386, - "non_hive": { - "close": 1561, - "high": 537, - "low": 1561, - "open": 537, - "volume": 2098 - }, - "open": "2020-10-23T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 10860, - "high": 10860, - "low": 10860, - "open": 10860, - "volume": 10860 - }, - "id": 2752389, - "non_hive": { - "close": 1658, - "high": 1658, - "low": 1658, - "open": 1658, - "volume": 1658 - }, - "open": "2020-10-23T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 712, - "high": 712, - "low": 306659, - "open": 306659, - "volume": 1017716 - }, - "id": 2752393, - "non_hive": { - "close": 109, - "high": 109, - "low": 46814, - "open": 46814, - "volume": 155481 - }, - "open": "2020-10-23T23:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 11047, - "high": 11047, - "low": 11047, - "open": 11047, - "volume": 11047 - }, - "id": 2752397, - "non_hive": { - "close": 1689, - "high": 1689, - "low": 1689, - "open": 1689, - "volume": 1689 - }, - "open": "2020-10-23T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 16692, - "high": 16692, - "low": 16692, - "open": 16692, - "volume": 16692 - }, - "id": 2752400, - "non_hive": { - "close": 2552, - "high": 2552, - "low": 2552, - "open": 2552, - "volume": 2552 - }, - "open": "2020-10-23T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 14452, - "high": 73915, - "low": 14452, - "open": 73915, - "volume": 999000 - }, - "id": 2752403, - "non_hive": { - "close": 2183, - "high": 11300, - "low": 2183, - "open": 11300, - "volume": 151957 - }, - "open": "2020-10-23T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6024, - "high": 6024, - "low": 6024, - "open": 6024, - "volume": 6024 - }, - "id": 2752406, - "non_hive": { - "close": 921, - "high": 921, - "low": 921, - "open": 921, - "volume": 921 - }, - "open": "2020-10-24T00:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 19001, - "high": 19001, - "low": 19001, - "open": 19001, - "volume": 19001 - }, - "id": 2752411, - "non_hive": { - "close": 2905, - "high": 2905, - "low": 2905, - "open": 2905, - "volume": 2905 - }, - "open": "2020-10-24T00:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6000, - "high": 30003, - "low": 6000, - "open": 30003, - "volume": 36003 - }, - "id": 2752414, - "non_hive": { - "close": 917, - "high": 4587, - "low": 917, - "open": 4587, - "volume": 5504 - }, - "open": "2020-10-24T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12500, - "high": 12500, - "low": 12500, - "open": 12500, - "volume": 12500 - }, - "id": 2752419, - "non_hive": { - "close": 1911, - "high": 1911, - "low": 1911, - "open": 1911, - "volume": 1911 - }, - "open": "2020-10-24T00:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 9942, - "high": 9942, - "low": 9942, - "open": 9942, - "volume": 9942 - }, - "id": 2752422, - "non_hive": { - "close": 1520, - "high": 1520, - "low": 1520, - "open": 1520, - "volume": 1520 - }, - "open": "2020-10-24T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 13082, - "high": 18969, - "low": 183, - "open": 26629, - "volume": 433976 - }, - "id": 2752426, - "non_hive": { - "close": 1999, - "high": 2900, - "low": 27, - "open": 4071, - "volume": 65535 - }, - "open": "2020-10-24T01:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 46729, - "high": 46729, - "low": 46729, - "open": 46729, - "volume": 46729 - }, - "id": 2752432, - "non_hive": { - "close": 7143, - "high": 7143, - "low": 7143, - "open": 7143, - "volume": 7143 - }, - "open": "2020-10-24T01:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 37924, - "high": 37924, - "low": 37924, - "open": 37924, - "volume": 37924 - }, - "id": 2752435, - "non_hive": { - "close": 5797, - "high": 5797, - "low": 5797, - "open": 5797, - "volume": 5797 - }, - "open": "2020-10-24T02:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 78, - "high": 12507, - "low": 78, - "open": 12507, - "volume": 19722 - }, - "id": 2752439, - "non_hive": { - "close": 11, - "high": 1912, - "low": 11, - "open": 1912, - "volume": 3014 - }, - "open": "2020-10-24T02:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 3604, - "high": 3604, - "low": 3604, - "open": 3604, - "volume": 3604 - }, - "id": 2752444, - "non_hive": { - "close": 551, - "high": 551, - "low": 551, - "open": 551, - "volume": 551 - }, - "open": "2020-10-24T02:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 10001, - "high": 1000, - "low": 220, - "open": 3920, - "volume": 34797 - }, - "id": 2752447, - "non_hive": { - "close": 1529, - "high": 153, - "low": 33, - "open": 599, - "volume": 5319 - }, - "open": "2020-10-24T03:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12165, - "high": 13, - "low": 12165, - "open": 20002, - "volume": 192394 - }, - "id": 2752456, - "non_hive": { - "close": 1858, - "high": 2, - "low": 1858, - "open": 3058, - "volume": 29405 - }, - "open": "2020-10-24T03:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 5745, - "high": 929, - "low": 12, - "open": 929, - "volume": 6838 - }, - "id": 2752470, - "non_hive": { - "close": 878, - "high": 142, - "low": 1, - "open": 142, - "volume": 1044 - }, - "open": "2020-10-24T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13237, - "high": 13237, - "low": 13281, - "open": 13281, - "volume": 39785 - }, - "id": 2752475, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 6000 - }, - "open": "2020-10-24T03:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13207, - "high": 13102, - "low": 13237, - "open": 13237, - "volume": 39546 - }, - "id": 2752481, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 6000 - }, - "open": "2020-10-24T03:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 3004, - "high": 3004, - "low": 3004, - "open": 3004, - "volume": 3004 - }, - "id": 2752487, - "non_hive": { - "close": 459, - "high": 459, - "low": 459, - "open": 459, - "volume": 459 - }, - "open": "2020-10-24T03:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 2683, - "high": 8145, - "low": 2683, - "open": 8145, - "volume": 24005 - }, - "id": 2752490, - "non_hive": { - "close": 407, - "high": 1244, - "low": 407, - "open": 1244, - "volume": 3651 - }, - "open": "2020-10-24T03:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 115273, - "high": 115273, - "low": 13088, - "open": 13088, - "volume": 128361 - }, - "id": 2752493, - "non_hive": { - "close": 17613, - "high": 17613, - "low": 1999, - "open": 1999, - "volume": 19612 - }, - "open": "2020-10-24T03:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 18000, - "high": 71725, - "low": 15547, - "open": 71725, - "volume": 118000 - }, - "id": 2752496, - "non_hive": { - "close": 2749, - "high": 10959, - "low": 2374, - "open": 10959, - "volume": 18026 - }, - "open": "2020-10-24T04:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 13177, - "high": 569, - "low": 13177, - "open": 5891, - "volume": 21621 - }, - "id": 2752502, - "non_hive": { - "close": 2000, - "high": 87, - "low": 2000, - "open": 900, - "volume": 3290 - }, - "open": "2020-10-24T04:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 13138, - "high": 13138, - "low": 13177, - "open": 13177, - "volume": 52635 - }, - "id": 2752508, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 8000 - }, - "open": "2020-10-24T04:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6862, - "high": 6862, - "low": 447, - "open": 447, - "volume": 14503 - }, - "id": 2752516, - "non_hive": { - "close": 1049, - "high": 1049, - "low": 68, - "open": 68, - "volume": 2216 - }, - "open": "2020-10-24T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 5792, - "high": 9282, - "low": 5792, - "open": 9282, - "volume": 21000 - }, - "id": 2752521, - "non_hive": { - "close": 880, - "high": 1413, - "low": 880, - "open": 1413, - "volume": 3195 - }, - "open": "2020-10-24T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 344, - "high": 29131, - "low": 344, - "open": 29131, - "volume": 29475 - }, - "id": 2752524, - "non_hive": { - "close": 51, - "high": 4450, - "low": 51, - "open": 4450, - "volume": 4501 - }, - "open": "2020-10-24T04:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6265, - "high": 6265, - "low": 6265, - "open": 6265, - "volume": 6265 - }, - "id": 2752527, - "non_hive": { - "close": 957, - "high": 957, - "low": 957, - "open": 957, - "volume": 957 - }, - "open": "2020-10-24T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2533, - "high": 247794, - "low": 2533, - "open": 6823, - "volume": 277522 - }, - "id": 2752531, - "non_hive": { - "close": 379, - "high": 37847, - "low": 379, - "open": 1042, - "volume": 42379 - }, - "open": "2020-10-24T05:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 527, - "high": 527, - "low": 527, - "open": 527, - "volume": 527 - }, - "id": 2752537, - "non_hive": { - "close": 80, - "high": 80, - "low": 80, - "open": 80, - "volume": 80 - }, - "open": "2020-10-24T05:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 13093, - "high": 13276, - "low": 13093, - "open": 13276, - "volume": 128822 - }, - "id": 2752540, - "non_hive": { - "close": 1964, - "high": 2028, - "low": 1964, - "open": 2028, - "volume": 19364 - }, - "open": "2020-10-24T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 22813, - "high": 22813, - "low": 22813, - "open": 22813, - "volume": 22813 - }, - "id": 2752543, - "non_hive": { - "close": 3422, - "high": 3422, - "low": 3422, - "open": 3422, - "volume": 3422 - }, - "open": "2020-10-24T06:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 129599, - "high": 9805, - "low": 129599, - "open": 9805, - "volume": 139404 - }, - "id": 2752547, - "non_hive": { - "close": 19441, - "high": 1471, - "low": 19441, - "open": 1471, - "volume": 20912 - }, - "open": "2020-10-24T06:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 9783, - "high": 9783, - "low": 9783, - "open": 9783, - "volume": 9783 - }, - "id": 2752550, - "non_hive": { - "close": 1494, - "high": 1494, - "low": 1494, - "open": 1494, - "volume": 1494 - }, - "open": "2020-10-24T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4139, - "high": 4139, - "low": 4139, - "open": 4139, - "volume": 4139 - }, - "id": 2752553, - "non_hive": { - "close": 632, - "high": 632, - "low": 632, - "open": 632, - "volume": 632 - }, - "open": "2020-10-24T06:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 123956, - "high": 123956, - "low": 13086, - "open": 13085, - "volume": 150127 - }, - "id": 2752556, - "non_hive": { - "close": 18945, - "high": 18945, - "low": 1999, - "open": 1999, - "volume": 22943 - }, - "open": "2020-10-24T06:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 11449, - "high": 11449, - "low": 11449, - "open": 11449, - "volume": 11449 - }, - "id": 2752561, - "non_hive": { - "close": 1749, - "high": 1749, - "low": 1749, - "open": 1749, - "volume": 1749 - }, - "open": "2020-10-24T06:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 14420, - "high": 14420, - "low": 1637, - "open": 1637, - "volume": 16057 - }, - "id": 2752564, - "non_hive": { - "close": 2204, - "high": 2204, - "low": 250, - "open": 250, - "volume": 2454 - }, - "open": "2020-10-24T06:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 47800, - "high": 13328, - "low": 47800, - "open": 13328, - "volume": 161752 - }, - "id": 2752567, - "non_hive": { - "close": 7128, - "high": 2000, - "low": 7128, - "open": 2000, - "volume": 24221 - }, - "open": "2020-10-24T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 52, - "high": 52, - "low": 52, - "open": 52, - "volume": 52 - }, - "id": 2752570, - "non_hive": { - "close": 8, - "high": 8, - "low": 8, - "open": 8, - "volume": 8 - }, - "open": "2020-10-24T07:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 5219, - "high": 5219, - "low": 7842, - "open": 13418, - "volume": 151283 - }, - "id": 2752574, - "non_hive": { - "close": 797, - "high": 797, - "low": 1160, - "open": 2000, - "volume": 22634 - }, - "open": "2020-10-24T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 7069, - "high": 7069, - "low": 7069, - "open": 7069, - "volume": 7069 - }, - "id": 2752582, - "non_hive": { - "close": 1079, - "high": 1079, - "low": 1079, - "open": 1079, - "volume": 1079 - }, - "open": "2020-10-24T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 9974, - "high": 13519, - "low": 9974, - "open": 13519, - "volume": 29287 - }, - "id": 2752585, - "non_hive": { - "close": 1473, - "high": 2000, - "low": 1473, - "open": 2000, - "volume": 4330 - }, - "open": "2020-10-24T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2995, - "high": 2995, - "low": 31835, - "open": 13534, - "volume": 119191 - }, - "id": 2752588, - "non_hive": { - "close": 457, - "high": 457, - "low": 4618, - "open": 2000, - "volume": 17452 - }, - "open": "2020-10-24T07:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 12680, - "high": 12680, - "low": 3065, - "open": 3065, - "volume": 15745 - }, - "id": 2752593, - "non_hive": { - "close": 1932, - "high": 1932, - "low": 467, - "open": 467, - "volume": 2399 - }, - "open": "2020-10-24T08:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 7030, - "high": 14643, - "low": 7030, - "open": 14643, - "volume": 21673 - }, - "id": 2752599, - "non_hive": { - "close": 1071, - "high": 2231, - "low": 1071, - "open": 2231, - "volume": 3302 - }, - "open": "2020-10-24T08:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6228, - "high": 6228, - "low": 20000, - "open": 20000, - "volume": 26228 - }, - "id": 2752602, - "non_hive": { - "close": 949, - "high": 949, - "low": 3046, - "open": 3046, - "volume": 3995 - }, - "open": "2020-10-24T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 75500, - "high": 11665, - "low": 75500, - "open": 6891, - "volume": 165091 - }, - "id": 2752605, - "non_hive": { - "close": 10965, - "high": 1778, - "low": 10965, - "open": 1050, - "volume": 24315 - }, - "open": "2020-10-24T08:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 14683, - "high": 14683, - "low": 13126, - "open": 13126, - "volume": 27809 - }, - "id": 2752609, - "non_hive": { - "close": 2237, - "high": 2237, - "low": 1999, - "open": 1999, - "volume": 4236 - }, - "open": "2020-10-24T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 111, - "high": 15680, - "low": 111, - "open": 15680, - "volume": 15791 - }, - "id": 2752613, - "non_hive": { - "close": 16, - "high": 2387, - "low": 16, - "open": 2387, - "volume": 2403 - }, - "open": "2020-10-24T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 9, - "high": 1188, - "low": 9, - "open": 1188, - "volume": 1197 - }, - "id": 2752616, - "non_hive": { - "close": 1, - "high": 181, - "low": 1, - "open": 181, - "volume": 182 - }, - "open": "2020-10-24T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 108199, - "high": 108199, - "low": 108199, - "open": 108199, - "volume": 108199 - }, - "id": 2752620, - "non_hive": { - "close": 16488, - "high": 16488, - "low": 16488, - "open": 16488, - "volume": 16488 - }, - "open": "2020-10-24T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6566, - "high": 6566, - "low": 6566, - "open": 6566, - "volume": 6566 - }, - "id": 2752623, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-24T10:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 802, - "high": 105681, - "low": 802, - "open": 105681, - "volume": 106483 - }, - "id": 2752626, - "non_hive": { - "close": 120, - "high": 16103, - "low": 120, - "open": 16103, - "volume": 16223 - }, - "open": "2020-10-24T10:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 401432, - "high": 13121, - "low": 401432, - "open": 13121, - "volume": 414553 - }, - "id": 2752629, - "non_hive": { - "close": 61158, - "high": 1999, - "low": 61158, - "open": 1999, - "volume": 63157 - }, - "open": "2020-10-24T10:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 9838, - "high": 3279, - "low": 9838, - "open": 3279, - "volume": 46692 - }, - "id": 2752634, - "non_hive": { - "close": 1498, - "high": 500, - "low": 1498, - "open": 500, - "volume": 7112 - }, - "open": "2020-10-24T10:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 3302, - "high": 13167, - "low": 3302, - "open": 13167, - "volume": 29636 - }, - "id": 2752640, - "non_hive": { - "close": 501, - "high": 1999, - "low": 501, - "open": 1999, - "volume": 4499 - }, - "open": "2020-10-24T11:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 7946, - "high": 7946, - "low": 7946, - "open": 7946, - "volume": 7946 - }, - "id": 2752647, - "non_hive": { - "close": 1206, - "high": 1206, - "low": 1206, - "open": 1206, - "volume": 1206 - }, - "open": "2020-10-24T11:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 87582, - "high": 87582, - "low": 87582, - "open": 87582, - "volume": 87582 - }, - "id": 2752650, - "non_hive": { - "close": 13286, - "high": 13286, - "low": 13286, - "open": 13286, - "volume": 13286 - }, - "open": "2020-10-24T11:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 10732, - "high": 79600, - "low": 9862, - "open": 79600, - "volume": 111783 - }, - "id": 2752653, - "non_hive": { - "close": 1628, - "high": 12075, - "low": 1496, - "open": 12075, - "volume": 16957 - }, - "open": "2020-10-24T11:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 42857, - "high": 1503, - "low": 42857, - "open": 1503, - "volume": 44360 - }, - "id": 2752657, - "non_hive": { - "close": 6501, - "high": 228, - "low": 6501, - "open": 228, - "volume": 6729 - }, - "open": "2020-10-24T11:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 118995, - "high": 13183, - "low": 118995, - "open": 13183, - "volume": 248896 - }, - "id": 2752662, - "non_hive": { - "close": 17992, - "high": 2000, - "low": 17992, - "open": 2000, - "volume": 37665 - }, - "open": "2020-10-24T12:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1124, - "high": 1124, - "low": 13183, - "open": 13183, - "volume": 14307 - }, - "id": 2752666, - "non_hive": { - "close": 170, - "high": 170, - "low": 1993, - "open": 1993, - "volume": 2163 - }, - "open": "2020-10-24T12:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 84189, - "high": 84189, - "low": 4102, - "open": 4102, - "volume": 88291 - }, - "id": 2752669, - "non_hive": { - "close": 12838, - "high": 12838, - "low": 620, - "open": 620, - "volume": 13458 - }, - "open": "2020-10-24T13:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 570, - "high": 570, - "low": 570, - "open": 570, - "volume": 570 - }, - "id": 2752673, - "non_hive": { - "close": 87, - "high": 87, - "low": 87, - "open": 87, - "volume": 87 - }, - "open": "2020-10-24T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13227, - "high": 13227, - "low": 13227, - "open": 13227, - "volume": 26454 - }, - "id": 2752676, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-10-24T13:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4433, - "high": 8795, - "low": 4433, - "open": 13227, - "volume": 105817 - }, - "id": 2752680, - "non_hive": { - "close": 670, - "high": 1330, - "low": 670, - "open": 2000, - "volume": 16000 - }, - "open": "2020-10-24T13:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 35017, - "high": 945, - "low": 483985, - "open": 13228, - "volume": 602871 - }, - "id": 2752694, - "non_hive": { - "close": 5268, - "high": 144, - "low": 72811, - "open": 2014, - "volume": 90787 - }, - "open": "2020-10-24T13:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 35017, - "high": 35017, - "low": 13142, - "open": 13142, - "volume": 48159 - }, - "id": 2752703, - "non_hive": { - "close": 5329, - "high": 5329, - "low": 1999, - "open": 1999, - "volume": 7328 - }, - "open": "2020-10-24T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 5515, - "high": 5515, - "low": 5515, - "open": 5515, - "volume": 5515 - }, - "id": 2752708, - "non_hive": { - "close": 839, - "high": 839, - "low": 839, - "open": 839, - "volume": 839 - }, - "open": "2020-10-24T14:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 157, - "high": 157, - "low": 157, - "open": 157, - "volume": 157 - }, - "id": 2752711, - "non_hive": { - "close": 24, - "high": 24, - "low": 24, - "open": 24, - "volume": 24 - }, - "open": "2020-10-24T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 73939, - "high": 73939, - "low": 12959, - "open": 12959, - "volume": 86898 - }, - "id": 2752714, - "non_hive": { - "close": 11275, - "high": 11275, - "low": 1975, - "open": 1975, - "volume": 13250 - }, - "open": "2020-10-24T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 10000, - "high": 10000, - "low": 10000, - "open": 10000, - "volume": 10000 - }, - "id": 2752718, - "non_hive": { - "close": 1525, - "high": 1525, - "low": 1525, - "open": 1525, - "volume": 1525 - }, - "open": "2020-10-24T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 2105, - "high": 2105, - "low": 2105, - "open": 2105, - "volume": 2105 - }, - "id": 2752721, - "non_hive": { - "close": 321, - "high": 321, - "low": 321, - "open": 321, - "volume": 321 - }, - "open": "2020-10-24T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 162994, - "high": 77998, - "low": 162994, - "open": 77998, - "volume": 240992 - }, - "id": 2752724, - "non_hive": { - "close": 24855, - "high": 11894, - "low": 24855, - "open": 11894, - "volume": 36749 - }, - "open": "2020-10-24T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 347996, - "high": 36973, - "low": 347996, - "open": 36973, - "volume": 384969 - }, - "id": 2752729, - "non_hive": { - "close": 53065, - "high": 5638, - "low": 53065, - "open": 5638, - "volume": 58703 - }, - "open": "2020-10-24T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 260061, - "high": 260061, - "low": 260061, - "open": 260061, - "volume": 260061 - }, - "id": 2752734, - "non_hive": { - "close": 39656, - "high": 39656, - "low": 39656, - "open": 39656, - "volume": 39656 - }, - "open": "2020-10-24T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 7803, - "high": 7803, - "low": 7803, - "open": 7803, - "volume": 7803 - }, - "id": 2752737, - "non_hive": { - "close": 1190, - "high": 1190, - "low": 1190, - "open": 1190, - "volume": 1190 - }, - "open": "2020-10-24T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12000, - "high": 12000, - "low": 12000, - "open": 12000, - "volume": 12000 - }, - "id": 2752740, - "non_hive": { - "close": 1830, - "high": 1830, - "low": 1830, - "open": 1830, - "volume": 1830 - }, - "open": "2020-10-24T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 12171, - "high": 12171, - "low": 12171, - "open": 12171, - "volume": 12171 - }, - "id": 2752743, - "non_hive": { - "close": 1856, - "high": 1856, - "low": 1856, - "open": 1856, - "volume": 1856 - }, - "open": "2020-10-24T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13158, - "high": 18277, - "low": 13158, - "open": 18277, - "volume": 208148 - }, - "id": 2752746, - "non_hive": { - "close": 1999, - "high": 2787, - "low": 1999, - "open": 2787, - "volume": 31726 - }, - "open": "2020-10-24T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 4164, - "high": 13158, - "low": 4164, - "open": 13158, - "volume": 17322 - }, - "id": 2752760, - "non_hive": { - "close": 632, - "high": 1999, - "low": 632, - "open": 1999, - "volume": 2631 - }, - "open": "2020-10-24T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 380, - "high": 380, - "low": 46653, - "open": 46653, - "volume": 47033 - }, - "id": 2752764, - "non_hive": { - "close": 58, - "high": 58, - "low": 7114, - "open": 7114, - "volume": 7172 - }, - "open": "2020-10-24T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 32795, - "high": 32795, - "low": 1548, - "open": 6558, - "volume": 51781 - }, - "id": 2752769, - "non_hive": { - "close": 5001, - "high": 5001, - "low": 236, - "open": 1000, - "volume": 7896 - }, - "open": "2020-10-24T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 984, - "high": 984, - "low": 984, - "open": 984, - "volume": 984 - }, - "id": 2752774, - "non_hive": { - "close": 150, - "high": 150, - "low": 150, - "open": 150, - "volume": 150 - }, - "open": "2020-10-24T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6544, - "high": 6544, - "low": 6544, - "open": 6544, - "volume": 6544 - }, - "id": 2752777, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-10-24T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 13195, - "high": 13195, - "low": 13195, - "open": 13195, - "volume": 13195 - }, - "id": 2752780, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-24T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 19701, - "high": 13195, - "low": 19701, - "open": 13195, - "volume": 86805 - }, - "id": 2752783, - "non_hive": { - "close": 2985, - "high": 2000, - "low": 2985, - "open": 2000, - "volume": 13155 - }, - "open": "2020-10-24T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 9607, - "high": 31608, - "low": 3801, - "open": 13116, - "volume": 106262 - }, - "id": 2752786, - "non_hive": { - "close": 1465, - "high": 4820, - "low": 579, - "open": 1999, - "volume": 16201 - }, - "open": "2020-10-24T17:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6408, - "high": 45806, - "low": 6408, - "open": 45806, - "volume": 54648 - }, - "id": 2752795, - "non_hive": { - "close": 971, - "high": 6985, - "low": 971, - "open": 6985, - "volume": 8327 - }, - "open": "2020-10-24T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 13196, - "high": 13158, - "low": 3211, - "open": 6789, - "volume": 49551 - }, - "id": 2752801, - "non_hive": { - "close": 2000, - "high": 1999, - "low": 486, - "open": 1029, - "volume": 7514 - }, - "open": "2020-10-24T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 8877, - "high": 4176, - "low": 7618, - "open": 13196, - "volume": 118688 - }, - "id": 2752809, - "non_hive": { - "close": 1345, - "high": 633, - "low": 1154, - "open": 2000, - "volume": 17985 - }, - "open": "2020-10-24T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 997, - "high": 997, - "low": 997, - "open": 997, - "volume": 997 - }, - "id": 2752818, - "non_hive": { - "close": 152, - "high": 152, - "low": 152, - "open": 152, - "volume": 152 - }, - "open": "2020-10-24T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 997, - "high": 997, - "low": 997, - "open": 997, - "volume": 997 - }, - "id": 2752821, - "non_hive": { - "close": 152, - "high": 152, - "low": 152, - "open": 152, - "volume": 152 - }, - "open": "2020-10-24T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 322, - "high": 322, - "low": 322, - "open": 322, - "volume": 322 - }, - "id": 2752824, - "non_hive": { - "close": 49, - "high": 49, - "low": 49, - "open": 49, - "volume": 49 - }, - "open": "2020-10-24T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 21550, - "high": 19136, - "low": 32807, - "open": 6850, - "volume": 113151 - }, - "id": 2752827, - "non_hive": { - "close": 3286, - "high": 2918, - "low": 5000, - "open": 1044, - "volume": 17250 - }, - "open": "2020-10-24T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11234, - "high": 11234, - "low": 9186, - "open": 9186, - "volume": 24422 - }, - "id": 2752835, - "non_hive": { - "close": 1713, - "high": 1713, - "low": 1400, - "open": 1400, - "volume": 3723 - }, - "open": "2020-10-24T19:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12717, - "high": 786, - "low": 126700, - "open": 126700, - "volume": 140203 - }, - "id": 2752843, - "non_hive": { - "close": 1939, - "high": 120, - "low": 19309, - "open": 19309, - "volume": 21368 - }, - "open": "2020-10-24T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 8782, - "high": 8782, - "low": 8782, - "open": 8782, - "volume": 8782 - }, - "id": 2752849, - "non_hive": { - "close": 1339, - "high": 1339, - "low": 1339, - "open": 1339, - "volume": 1339 - }, - "open": "2020-10-24T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 21510, - "high": 21542, - "low": 21510, - "open": 21542, - "volume": 194876 - }, - "id": 2752852, - "non_hive": { - "close": 3278, - "high": 3285, - "low": 3278, - "open": 3285, - "volume": 29714 - }, - "open": "2020-10-24T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 32783, - "high": 32783, - "low": 32783, - "open": 32783, - "volume": 32783 - }, - "id": 2752861, - "non_hive": { - "close": 4999, - "high": 4999, - "low": 4999, - "open": 4999, - "volume": 4999 - }, - "open": "2020-10-24T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 158476, - "high": 158476, - "low": 158476, - "open": 158476, - "volume": 158476 - }, - "id": 2752864, - "non_hive": { - "close": 24166, - "high": 24166, - "low": 24166, - "open": 24166, - "volume": 24166 - }, - "open": "2020-10-24T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 15004, - "high": 15004, - "low": 15004, - "open": 15004, - "volume": 15004 - }, - "id": 2752867, - "non_hive": { - "close": 2288, - "high": 2288, - "low": 2288, - "open": 2288, - "volume": 2288 - }, - "open": "2020-10-24T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 33621, - "high": 33621, - "low": 33621, - "open": 33621, - "volume": 33621 - }, - "id": 2752871, - "non_hive": { - "close": 5127, - "high": 5127, - "low": 5127, - "open": 5127, - "volume": 5127 - }, - "open": "2020-10-24T20:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 7909, - "high": 7909, - "low": 32867, - "open": 19930, - "volume": 296117 - }, - "id": 2752874, - "non_hive": { - "close": 1206, - "high": 1206, - "low": 4999, - "open": 3039, - "volume": 45110 - }, - "open": "2020-10-24T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7065, - "high": 7065, - "low": 7065, - "open": 7065, - "volume": 7065 - }, - "id": 2752879, - "non_hive": { - "close": 1077, - "high": 1077, - "low": 1077, - "open": 1077, - "volume": 1077 - }, - "open": "2020-10-24T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 4684, - "high": 4684, - "low": 4684, - "open": 4684, - "volume": 4684 - }, - "id": 2752882, - "non_hive": { - "close": 714, - "high": 714, - "low": 714, - "open": 714, - "volume": 714 - }, - "open": "2020-10-24T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 7082, - "high": 7082, - "low": 7082, - "open": 7082, - "volume": 7082 - }, - "id": 2752885, - "non_hive": { - "close": 1077, - "high": 1077, - "low": 1077, - "open": 1077, - "volume": 1077 - }, - "open": "2020-10-24T20:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13177, - "high": 671, - "low": 13803, - "open": 671, - "volume": 80375 - }, - "id": 2752888, - "non_hive": { - "close": 2000, - "high": 102, - "low": 2091, - "open": 102, - "volume": 12195 - }, - "open": "2020-10-24T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 3912, - "high": 1161, - "low": 13177, - "open": 1161, - "volume": 31427 - }, - "id": 2752896, - "non_hive": { - "close": 594, - "high": 177, - "low": 2000, - "open": 177, - "volume": 4771 - }, - "open": "2020-10-24T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 10269, - "high": 2996, - "low": 10269, - "open": 2996, - "volume": 53730 - }, - "id": 2752904, - "non_hive": { - "close": 1550, - "high": 454, - "low": 1550, - "open": 454, - "volume": 8135 - }, - "open": "2020-10-24T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 37449, - "high": 37449, - "low": 13120, - "open": 13120, - "volume": 50569 - }, - "id": 2752909, - "non_hive": { - "close": 5709, - "high": 5709, - "low": 1999, - "open": 1999, - "volume": 7708 - }, - "open": "2020-10-24T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 10453, - "high": 10453, - "low": 13123, - "open": 13123, - "volume": 23576 - }, - "id": 2752912, - "non_hive": { - "close": 1593, - "high": 1593, - "low": 1999, - "open": 1999, - "volume": 3592 - }, - "open": "2020-10-24T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13124, - "high": 13124, - "low": 13124, - "open": 13124, - "volume": 13124 - }, - "id": 2752915, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-24T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6566, - "high": 6566, - "low": 6566, - "open": 6566, - "volume": 6566 - }, - "id": 2752918, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-24T21:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 13117, - "high": 13117, - "low": 13117, - "open": 13117, - "volume": 13117 - }, - "id": 2752921, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-24T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 3005, - "high": 2387, - "low": 334, - "open": 9886, - "volume": 15612 - }, - "id": 2752924, - "non_hive": { - "close": 458, - "high": 364, - "low": 50, - "open": 1507, - "volume": 2379 - }, - "open": "2020-10-24T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 8543, - "high": 8543, - "low": 8543, - "open": 8543, - "volume": 8543 - }, - "id": 2752929, - "non_hive": { - "close": 1302, - "high": 1302, - "low": 1302, - "open": 1302, - "volume": 1302 - }, - "open": "2020-10-24T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 146606, - "high": 146606, - "low": 1569, - "open": 1569, - "volume": 200005 - }, - "id": 2752932, - "non_hive": { - "close": 22356, - "high": 22356, - "low": 239, - "open": 239, - "volume": 30498 - }, - "open": "2020-10-24T22:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 26455, - "high": 26455, - "low": 13116, - "open": 13116, - "volume": 39571 - }, - "id": 2752936, - "non_hive": { - "close": 4032, - "high": 4032, - "low": 1999, - "open": 1999, - "volume": 6031 - }, - "open": "2020-10-24T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 19684, - "high": 19684, - "low": 19684, - "open": 19684, - "volume": 19684 - }, - "id": 2752941, - "non_hive": { - "close": 3000, - "high": 3000, - "low": 3000, - "open": 3000, - "volume": 3000 - }, - "open": "2020-10-24T22:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 164, - "high": 190, - "low": 164, - "open": 190, - "volume": 354 - }, - "id": 2752944, - "non_hive": { - "close": 25, - "high": 29, - "low": 25, - "open": 29, - "volume": 54 - }, - "open": "2020-10-24T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 27558, - "high": 28568, - "low": 27558, - "open": 28568, - "volume": 69708 - }, - "id": 2752949, - "non_hive": { - "close": 4200, - "high": 4354, - "low": 4200, - "open": 4354, - "volume": 10624 - }, - "open": "2020-10-24T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 16681, - "high": 13319, - "low": 16681, - "open": 13319, - "volume": 30000 - }, - "id": 2752953, - "non_hive": { - "close": 2504, - "high": 2000, - "low": 2504, - "open": 2000, - "volume": 4504 - }, - "open": "2020-10-24T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13036, - "high": 136319, - "low": 13119, - "open": 13119, - "volume": 958284 - }, - "id": 2752956, - "non_hive": { - "close": 1999, - "high": 20914, - "low": 1999, - "open": 1999, - "volume": 146773 - }, - "open": "2020-10-24T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3094, - "high": 3098, - "low": 3103, - "open": 3103, - "volume": 12395 - }, - "id": 2752961, - "non_hive": { - "close": 474, - "high": 475, - "low": 475, - "open": 475, - "volume": 1899 - }, - "open": "2020-10-24T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 6275, - "high": 2587, - "low": 6275, - "open": 3089, - "volume": 24968 - }, - "id": 2752968, - "non_hive": { - "close": 962, - "high": 397, - "low": 962, - "open": 474, - "volume": 3830 - }, - "open": "2020-10-24T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 6497, - "high": 6497, - "low": 38171, - "open": 35940, - "volume": 442023 - }, - "id": 2752973, - "non_hive": { - "close": 998, - "high": 998, - "low": 5853, - "open": 5515, - "volume": 67804 - }, - "open": "2020-10-24T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1674, - "high": 1674, - "low": 1674, - "open": 1674, - "volume": 1674 - }, - "id": 2752981, - "non_hive": { - "close": 256, - "high": 256, - "low": 256, - "open": 256, - "volume": 256 - }, - "open": "2020-10-24T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 5987, - "high": 5987, - "low": 5987, - "open": 5987, - "volume": 5987 - }, - "id": 2752984, - "non_hive": { - "close": 923, - "high": 923, - "low": 923, - "open": 923, - "volume": 923 - }, - "open": "2020-10-25T00:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6379, - "high": 280, - "low": 6099, - "open": 6379, - "volume": 31902 - }, - "id": 2752989, - "non_hive": { - "close": 978, - "high": 43, - "low": 935, - "open": 978, - "volume": 4891 - }, - "open": "2020-10-25T00:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 9232, - "high": 9232, - "low": 6365, - "open": 6365, - "volume": 15597 - }, - "id": 2752998, - "non_hive": { - "close": 1421, - "high": 1421, - "low": 977, - "open": 977, - "volume": 2398 - }, - "open": "2020-10-25T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 77602, - "high": 77602, - "low": 3756, - "open": 3756, - "volume": 94039 - }, - "id": 2753003, - "non_hive": { - "close": 11985, - "high": 11985, - "low": 578, - "open": 578, - "volume": 14518 - }, - "open": "2020-10-25T01:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 10, - "high": 10, - "low": 10, - "open": 10, - "volume": 10 - }, - "id": 2753007, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 1, - "volume": 1 - }, - "open": "2020-10-25T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 92178, - "high": 92178, - "low": 3706, - "open": 9244, - "volume": 105128 - }, - "id": 2753010, - "non_hive": { - "close": 14236, - "high": 14236, - "low": 572, - "open": 1427, - "volume": 16235 - }, - "open": "2020-10-25T01:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12547, - "high": 129506, - "low": 12950, - "open": 12950, - "volume": 155003 - }, - "id": 2753015, - "non_hive": { - "close": 1937, - "high": 20001, - "low": 1999, - "open": 1999, - "volume": 23937 - }, - "open": "2020-10-25T01:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3004, - "high": 3004, - "low": 3004, - "open": 3004, - "volume": 3004 - }, - "id": 2753019, - "non_hive": { - "close": 464, - "high": 464, - "low": 464, - "open": 464, - "volume": 464 - }, - "open": "2020-10-25T01:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 49741, - "high": 49741, - "low": 6874, - "open": 6874, - "volume": 56615 - }, - "id": 2753022, - "non_hive": { - "close": 7682, - "high": 7682, - "low": 1061, - "open": 1061, - "volume": 8743 - }, - "open": "2020-10-25T01:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13022, - "high": 4752, - "low": 13022, - "open": 20000, - "volume": 38428 - }, - "id": 2753027, - "non_hive": { - "close": 2000, - "high": 734, - "low": 2000, - "open": 3088, - "volume": 5923 - }, - "open": "2020-10-25T01:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13008, - "high": 13008, - "low": 16, - "open": 13008, - "volume": 26032 - }, - "id": 2753033, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2, - "open": 2000, - "volume": 4002 - }, - "open": "2020-10-25T02:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 13008, - "high": 13008, - "low": 13039, - "open": 2031, - "volume": 41117 - }, - "id": 2753039, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 312, - "volume": 6312 - }, - "open": "2020-10-25T02:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 21976, - "high": 12994, - "low": 21976, - "open": 13008, - "volume": 205197 - }, - "id": 2753045, - "non_hive": { - "close": 3370, - "high": 2000, - "low": 3370, - "open": 2000, - "volume": 31495 - }, - "open": "2020-10-25T02:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 12780, - "high": 1095, - "low": 12780, - "open": 1095, - "volume": 36000 - }, - "id": 2753054, - "non_hive": { - "close": 1948, - "high": 168, - "low": 1948, - "open": 168, - "volume": 5505 - }, - "open": "2020-10-25T02:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 6407, - "high": 1226, - "low": 6407, - "open": 1226, - "volume": 7633 - }, - "id": 2753059, - "non_hive": { - "close": 977, - "high": 187, - "low": 977, - "open": 187, - "volume": 1164 - }, - "open": "2020-10-25T02:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 152695, - "high": 6421, - "low": 152695, - "open": 6421, - "volume": 165552 - }, - "id": 2753062, - "non_hive": { - "close": 23115, - "high": 975, - "low": 23115, - "open": 975, - "volume": 25065 - }, - "open": "2020-10-25T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 49999, - "high": 49999, - "low": 49999, - "open": 49999, - "volume": 49999 - }, - "id": 2753065, - "non_hive": { - "close": 7568, - "high": 7568, - "low": 7568, - "open": 7568, - "volume": 7568 - }, - "open": "2020-10-25T04:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 98956, - "high": 488115, - "low": 98956, - "open": 488115, - "volume": 600000 - }, - "id": 2753069, - "non_hive": { - "close": 14862, - "high": 73886, - "low": 14862, - "open": 73886, - "volume": 90697 - }, - "open": "2020-10-25T04:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 899, - "high": 899, - "low": 899, - "open": 899, - "volume": 899 - }, - "id": 2753072, - "non_hive": { - "close": 136, - "high": 136, - "low": 136, - "open": 136, - "volume": 136 - }, - "open": "2020-10-25T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 14, - "high": 1419, - "low": 14, - "open": 12316, - "volume": 32979 - }, - "id": 2753075, - "non_hive": { - "close": 2, - "high": 217, - "low": 2, - "open": 1863, - "volume": 5003 - }, - "open": "2020-10-25T05:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6436, - "high": 6436, - "low": 6436, - "open": 6436, - "volume": 6436 - }, - "id": 2753081, - "non_hive": { - "close": 975, - "high": 975, - "low": 975, - "open": 975, - "volume": 975 - }, - "open": "2020-10-25T05:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 10217, - "high": 10217, - "low": 1887, - "open": 11256, - "volume": 43998 - }, - "id": 2753084, - "non_hive": { - "close": 1567, - "high": 1567, - "low": 287, - "open": 1712, - "volume": 6712 - }, - "open": "2020-10-25T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 19569, - "high": 19569, - "low": 13042, - "open": 13042, - "volume": 32611 - }, - "id": 2753089, - "non_hive": { - "close": 3001, - "high": 3001, - "low": 1999, - "open": 1999, - "volume": 5000 - }, - "open": "2020-10-25T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 2995, - "high": 2995, - "low": 2995, - "open": 2995, - "volume": 2995 - }, - "id": 2753092, - "non_hive": { - "close": 459, - "high": 459, - "low": 459, - "open": 459, - "volume": 459 - }, - "open": "2020-10-25T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 7080, - "high": 7080, - "low": 7080, - "open": 7080, - "volume": 7080 - }, - "id": 2753095, - "non_hive": { - "close": 1075, - "high": 1075, - "low": 1075, - "open": 1075, - "volume": 1075 - }, - "open": "2020-10-25T06:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 8079, - "high": 8079, - "low": 8079, - "open": 8079, - "volume": 8079 - }, - "id": 2753099, - "non_hive": { - "close": 1238, - "high": 1238, - "low": 1238, - "open": 1238, - "volume": 1238 - }, - "open": "2020-10-25T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 260994, - "high": 260994, - "low": 13063, - "open": 13063, - "volume": 274057 - }, - "id": 2753102, - "non_hive": { - "close": 39958, - "high": 39958, - "low": 1999, - "open": 1999, - "volume": 41957 - }, - "open": "2020-10-25T06:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16201, - "high": 16201, - "low": 16201, - "open": 16201, - "volume": 16201 - }, - "id": 2753105, - "non_hive": { - "close": 2484, - "high": 2484, - "low": 2484, - "open": 2484, - "volume": 2484 - }, - "open": "2020-10-25T06:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 31965, - "high": 31965, - "low": 31965, - "open": 31965, - "volume": 31965 - }, - "id": 2753108, - "non_hive": { - "close": 4901, - "high": 4901, - "low": 4901, - "open": 4901, - "volume": 4901 - }, - "open": "2020-10-25T07:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6421, - "high": 2772, - "low": 6421, - "open": 2772, - "volume": 37089 - }, - "id": 2753112, - "non_hive": { - "close": 975, - "high": 425, - "low": 975, - "open": 425, - "volume": 5675 - }, - "open": "2020-10-25T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 11281, - "high": 13116, - "low": 89, - "open": 13116, - "volume": 76124 - }, - "id": 2753115, - "non_hive": { - "close": 1703, - "high": 2000, - "low": 13, - "open": 2000, - "volume": 11560 - }, - "open": "2020-10-25T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 14316, - "high": 14316, - "low": 14316, - "open": 14316, - "volume": 14316 - }, - "id": 2753123, - "non_hive": { - "close": 2180, - "high": 2180, - "low": 2180, - "open": 2180, - "volume": 2180 - }, - "open": "2020-10-25T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1189, - "high": 1189, - "low": 13134, - "open": 13134, - "volume": 14323 - }, - "id": 2753126, - "non_hive": { - "close": 181, - "high": 181, - "low": 1999, - "open": 1999, - "volume": 2180 - }, - "open": "2020-10-25T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 492, - "high": 492, - "low": 11945, - "open": 11945, - "volume": 44739 - }, - "id": 2753131, - "non_hive": { - "close": 75, - "high": 75, - "low": 1818, - "open": 1818, - "volume": 6812 - }, - "open": "2020-10-25T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13105, - "high": 13105, - "low": 13105, - "open": 13105, - "volume": 13105 - }, - "id": 2753136, - "non_hive": { - "close": 1996, - "high": 1996, - "low": 1996, - "open": 1996, - "volume": 1996 - }, - "open": "2020-10-25T07:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 13141, - "high": 6565, - "low": 13141, - "open": 6565, - "volume": 51749 - }, - "id": 2753139, - "non_hive": { - "close": 1999, - "high": 1000, - "low": 1999, - "open": 1000, - "volume": 7874 - }, - "open": "2020-10-25T07:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 18112, - "high": 18112, - "low": 13141, - "open": 13141, - "volume": 31253 - }, - "id": 2753146, - "non_hive": { - "close": 2757, - "high": 2757, - "low": 1999, - "open": 1999, - "volume": 4756 - }, - "open": "2020-10-25T07:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 184, - "high": 184, - "low": 10994, - "open": 13146, - "volume": 35313 - }, - "id": 2753151, - "non_hive": { - "close": 28, - "high": 28, - "low": 1671, - "open": 1999, - "volume": 5369 - }, - "open": "2020-10-25T08:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 13242, - "high": 185, - "low": 13244, - "open": 185, - "volume": 26671 - }, - "id": 2753161, - "non_hive": { - "close": 2000, - "high": 28, - "low": 2000, - "open": 28, - "volume": 4028 - }, - "open": "2020-10-25T08:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 33703, - "high": 33703, - "low": 13429, - "open": 13429, - "volume": 60264 - }, - "id": 2753166, - "non_hive": { - "close": 5133, - "high": 5133, - "low": 2028, - "open": 2028, - "volume": 9160 - }, - "open": "2020-10-25T08:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 47956, - "high": 6552, - "low": 47956, - "open": 13132, - "volume": 67640 - }, - "id": 2753171, - "non_hive": { - "close": 7300, - "high": 998, - "low": 7300, - "open": 1999, - "volume": 10297 - }, - "open": "2020-10-25T08:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 3439, - "high": 3439, - "low": 3439, - "open": 3439, - "volume": 3439 - }, - "id": 2753175, - "non_hive": { - "close": 522, - "high": 522, - "low": 522, - "open": 522, - "volume": 522 - }, - "open": "2020-10-25T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 7490, - "high": 10263, - "low": 7490, - "open": 10263, - "volume": 223636 - }, - "id": 2753178, - "non_hive": { - "close": 1126, - "high": 1563, - "low": 1126, - "open": 1563, - "volume": 33783 - }, - "open": "2020-10-25T08:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6408, - "high": 13211, - "low": 6408, - "open": 13211, - "volume": 19619 - }, - "id": 2753184, - "non_hive": { - "close": 970, - "high": 2000, - "low": 970, - "open": 2000, - "volume": 2970 - }, - "open": "2020-10-25T09:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6408, - "high": 8778, - "low": 2211, - "open": 13186, - "volume": 205390 - }, - "id": 2753188, - "non_hive": { - "close": 974, - "high": 1337, - "low": 332, - "open": 1983, - "volume": 30977 - }, - "open": "2020-10-25T09:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 45018, - "high": 748, - "low": 45018, - "open": 2456, - "volume": 175748 - }, - "id": 2753199, - "non_hive": { - "close": 6761, - "high": 114, - "low": 6761, - "open": 374, - "volume": 26406 - }, - "open": "2020-10-25T09:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 13223, - "high": 16614, - "low": 13223, - "open": 16614, - "volume": 29837 - }, - "id": 2753205, - "non_hive": { - "close": 1999, - "high": 2513, - "low": 1999, - "open": 2513, - "volume": 4512 - }, - "open": "2020-10-25T09:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 629, - "high": 629, - "low": 629, - "open": 629, - "volume": 629 - }, - "id": 2753210, - "non_hive": { - "close": 95, - "high": 95, - "low": 95, - "open": 95, - "volume": 95 - }, - "open": "2020-10-25T09:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 12395, - "high": 12395, - "low": 12395, - "open": 12395, - "volume": 12395 - }, - "id": 2753213, - "non_hive": { - "close": 1875, - "high": 1875, - "low": 1875, - "open": 1875, - "volume": 1875 - }, - "open": "2020-10-25T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 4595, - "high": 186, - "low": 4595, - "open": 186, - "volume": 100000 - }, - "id": 2753216, - "non_hive": { - "close": 689, - "high": 28, - "low": 689, - "open": 28, - "volume": 15019 - }, - "open": "2020-10-25T10:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 531, - "high": 531, - "low": 13246, - "open": 13246, - "volume": 33058 - }, - "id": 2753220, - "non_hive": { - "close": 81, - "high": 81, - "low": 1999, - "open": 1999, - "volume": 5000 - }, - "open": "2020-10-25T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 19704, - "high": 19704, - "low": 13132, - "open": 13132, - "volume": 32836 - }, - "id": 2753223, - "non_hive": { - "close": 3001, - "high": 3001, - "low": 1999, - "open": 1999, - "volume": 5000 - }, - "open": "2020-10-25T10:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6457, - "high": 13, - "low": 6457, - "open": 13244, - "volume": 72574 - }, - "id": 2753226, - "non_hive": { - "close": 975, - "high": 2, - "low": 975, - "open": 2000, - "volume": 10977 - }, - "open": "2020-10-25T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 13245, - "high": 13245, - "low": 13245, - "open": 13245, - "volume": 39735 - }, - "id": 2753238, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 5997 - }, - "open": "2020-10-25T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 8874, - "high": 8874, - "low": 8874, - "open": 8874, - "volume": 8874 - }, - "id": 2753244, - "non_hive": { - "close": 1347, - "high": 1347, - "low": 1347, - "open": 1347, - "volume": 1347 - }, - "open": "2020-10-25T10:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1633, - "high": 1633, - "low": 4296, - "open": 4296, - "volume": 5929 - }, - "id": 2753247, - "non_hive": { - "close": 248, - "high": 248, - "low": 652, - "open": 652, - "volume": 900 - }, - "open": "2020-10-25T10:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 164631, - "high": 164631, - "low": 3336, - "open": 13737, - "volume": 214631 - }, - "id": 2753250, - "non_hive": { - "close": 25000, - "high": 25000, - "low": 506, - "open": 2086, - "volume": 32592 - }, - "open": "2020-10-25T11:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 114214, - "high": 114214, - "low": 256982, - "open": 256982, - "volume": 521196 - }, - "id": 2753256, - "non_hive": { - "close": 17344, - "high": 17344, - "low": 39023, - "open": 39023, - "volume": 79145 - }, - "open": "2020-10-25T11:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 34975, - "high": 3253, - "low": 3135, - "open": 25407, - "volume": 66770 - }, - "id": 2753263, - "non_hive": { - "close": 5311, - "high": 494, - "low": 476, - "open": 3858, - "volume": 10139 - }, - "open": "2020-10-25T11:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6586, - "high": 6586, - "low": 6586, - "open": 6586, - "volume": 6586 - }, - "id": 2753270, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-25T11:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 754, - "high": 2246, - "low": 754, - "open": 2246, - "volume": 3000 - }, - "id": 2753273, - "non_hive": { - "close": 114, - "high": 341, - "low": 114, - "open": 341, - "volume": 455 - }, - "open": "2020-10-25T12:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 500, - "high": 500, - "low": 500, - "open": 500, - "volume": 500 - }, - "id": 2753277, - "non_hive": { - "close": 76, - "high": 76, - "low": 76, - "open": 76, - "volume": 76 - }, - "open": "2020-10-25T12:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1883, - "high": 1883, - "low": 1883, - "open": 1883, - "volume": 1883 - }, - "id": 2753280, - "non_hive": { - "close": 286, - "high": 286, - "low": 286, - "open": 286, - "volume": 286 - }, - "open": "2020-10-25T12:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 322, - "high": 322, - "low": 322, - "open": 322, - "volume": 322 - }, - "id": 2753283, - "non_hive": { - "close": 49, - "high": 49, - "low": 49, - "open": 49, - "volume": 49 - }, - "open": "2020-10-25T12:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 15755, - "high": 9331, - "low": 15755, - "open": 9331, - "volume": 59331 - }, - "id": 2753286, - "non_hive": { - "close": 2392, - "high": 1417, - "low": 2392, - "open": 1417, - "volume": 9009 - }, - "open": "2020-10-25T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3464, - "high": 3464, - "low": 13224, - "open": 13224, - "volume": 16688 - }, - "id": 2753292, - "non_hive": { - "close": 526, - "high": 526, - "low": 2008, - "open": 2008, - "volume": 2534 - }, - "open": "2020-10-25T12:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 671, - "high": 671, - "low": 671, - "open": 671, - "volume": 671 - }, - "id": 2753297, - "non_hive": { - "close": 102, - "high": 102, - "low": 102, - "open": 102, - "volume": 102 - }, - "open": "2020-10-25T13:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 3826, - "high": 3826, - "low": 3826, - "open": 3826, - "volume": 3826 - }, - "id": 2753301, - "non_hive": { - "close": 581, - "high": 581, - "low": 581, - "open": 581, - "volume": 581 - }, - "open": "2020-10-25T13:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 210, - "high": 210, - "low": 210, - "open": 210, - "volume": 210 - }, - "id": 2753304, - "non_hive": { - "close": 32, - "high": 32, - "low": 32, - "open": 32, - "volume": 32 - }, - "open": "2020-10-25T13:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 19738, - "high": 19738, - "low": 19738, - "open": 19738, - "volume": 19738 - }, - "id": 2753307, - "non_hive": { - "close": 2997, - "high": 2997, - "low": 2997, - "open": 2997, - "volume": 2997 - }, - "open": "2020-10-25T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 4781, - "high": 4781, - "low": 2209, - "open": 2209, - "volume": 6990 - }, - "id": 2753310, - "non_hive": { - "close": 726, - "high": 726, - "low": 335, - "open": 335, - "volume": 1061 - }, - "open": "2020-10-25T13:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 691, - "high": 691, - "low": 691, - "open": 691, - "volume": 691 - }, - "id": 2753313, - "non_hive": { - "close": 105, - "high": 105, - "low": 105, - "open": 105, - "volume": 105 - }, - "open": "2020-10-25T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 29905, - "high": 13, - "low": 29905, - "open": 27164, - "volume": 100000 - }, - "id": 2753317, - "non_hive": { - "close": 4540, - "high": 2, - "low": 4540, - "open": 4137, - "volume": 15197 - }, - "open": "2020-10-25T14:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 16920, - "high": 16920, - "low": 16920, - "open": 16920, - "volume": 16920 - }, - "id": 2753320, - "non_hive": { - "close": 2577, - "high": 2577, - "low": 2577, - "open": 2577, - "volume": 2577 - }, - "open": "2020-10-25T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12528, - "high": 12528, - "low": 12528, - "open": 12528, - "volume": 12528 - }, - "id": 2753323, - "non_hive": { - "close": 1908, - "high": 1908, - "low": 1908, - "open": 1908, - "volume": 1908 - }, - "open": "2020-10-25T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 551, - "high": 551, - "low": 2428, - "open": 2428, - "volume": 2979 - }, - "id": 2753326, - "non_hive": { - "close": 84, - "high": 84, - "low": 369, - "open": 369, - "volume": 453 - }, - "open": "2020-10-25T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 186653, - "high": 186653, - "low": 5842, - "open": 5842, - "volume": 287488 - }, - "id": 2753329, - "non_hive": { - "close": 28523, - "high": 28523, - "low": 890, - "open": 890, - "volume": 43928 - }, - "open": "2020-10-25T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 2925, - "high": 2925, - "low": 2925, - "open": 2925, - "volume": 2925 - }, - "id": 2753334, - "non_hive": { - "close": 447, - "high": 447, - "low": 447, - "open": 447, - "volume": 447 - }, - "open": "2020-10-25T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 26615, - "high": 26615, - "low": 26615, - "open": 26615, - "volume": 26615 - }, - "id": 2753338, - "non_hive": { - "close": 4067, - "high": 4067, - "low": 4067, - "open": 4067, - "volume": 4067 - }, - "open": "2020-10-25T15:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 4954, - "high": 13068, - "low": 4954, - "open": 13068, - "volume": 38466 - }, - "id": 2753341, - "non_hive": { - "close": 757, - "high": 1997, - "low": 757, - "open": 1997, - "volume": 5878 - }, - "open": "2020-10-25T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1695, - "high": 1695, - "low": 1695, - "open": 1695, - "volume": 1695 - }, - "id": 2753344, - "non_hive": { - "close": 259, - "high": 259, - "low": 259, - "open": 259, - "volume": 259 - }, - "open": "2020-10-25T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13168, - "high": 39712, - "low": 13168, - "open": 13783, - "volume": 87050 - }, - "id": 2753347, - "non_hive": { - "close": 2000, - "high": 6068, - "low": 2000, - "open": 2106, - "volume": 13277 - }, - "open": "2020-10-25T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13166, - "high": 45818, - "low": 72, - "open": 13171, - "volume": 183425 - }, - "id": 2753355, - "non_hive": { - "close": 2000, - "high": 7001, - "low": 10, - "open": 2000, - "volume": 27903 - }, - "open": "2020-10-25T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12849, - "high": 1912, - "low": 13166, - "open": 13166, - "volume": 54231 - }, - "id": 2753368, - "non_hive": { - "close": 1954, - "high": 292, - "low": 2000, - "open": 2000, - "volume": 8246 - }, - "open": "2020-10-25T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 13090, - "high": 13090, - "low": 5311, - "open": 5311, - "volume": 18401 - }, - "id": 2753376, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 811, - "open": 811, - "volume": 2810 - }, - "open": "2020-10-25T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13160, - "high": 7475, - "low": 13160, - "open": 7475, - "volume": 20635 - }, - "id": 2753380, - "non_hive": { - "close": 2009, - "high": 1142, - "low": 2009, - "open": 1142, - "volume": 3151 - }, - "open": "2020-10-25T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 491, - "high": 491, - "low": 13160, - "open": 13160, - "volume": 283651 - }, - "id": 2753385, - "non_hive": { - "close": 75, - "high": 75, - "low": 2009, - "open": 2009, - "volume": 43316 - }, - "open": "2020-10-25T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 13159, - "high": 13159, - "low": 13159, - "open": 13159, - "volume": 13159 - }, - "id": 2753392, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-25T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 8776, - "high": 5574, - "low": 8776, - "open": 5574, - "volume": 247457 - }, - "id": 2753395, - "non_hive": { - "close": 1317, - "high": 846, - "low": 1317, - "open": 846, - "volume": 37332 - }, - "open": "2020-10-25T17:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 198, - "high": 514, - "low": 198, - "open": 514, - "volume": 712 - }, - "id": 2753401, - "non_hive": { - "close": 30, - "high": 78, - "low": 30, - "open": 78, - "volume": 108 - }, - "open": "2020-10-25T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 11773, - "high": 16878, - "low": 11773, - "open": 16878, - "volume": 99990 - }, - "id": 2753404, - "non_hive": { - "close": 1764, - "high": 2553, - "low": 1764, - "open": 2553, - "volume": 15018 - }, - "open": "2020-10-25T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 46, - "high": 46, - "low": 46, - "open": 46, - "volume": 46 - }, - "id": 2753409, - "non_hive": { - "close": 7, - "high": 7, - "low": 7, - "open": 7, - "volume": 7 - }, - "open": "2020-10-25T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 14050, - "high": 138, - "low": 14050, - "open": 23000, - "volume": 100000 - }, - "id": 2753412, - "non_hive": { - "close": 2104, - "high": 21, - "low": 2104, - "open": 3479, - "volume": 15020 - }, - "open": "2020-10-25T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 36293, - "high": 44423, - "low": 13266, - "open": 44423, - "volume": 113266 - }, - "id": 2753416, - "non_hive": { - "close": 5477, - "high": 6704, - "low": 1999, - "open": 6704, - "volume": 17090 - }, - "open": "2020-10-25T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1534, - "high": 1534, - "low": 6444, - "open": 6444, - "volume": 13695 - }, - "id": 2753423, - "non_hive": { - "close": 233, - "high": 233, - "low": 973, - "open": 973, - "volume": 2071 - }, - "open": "2020-10-25T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 20226, - "high": 3093, - "low": 20226, - "open": 13170, - "volume": 67740 - }, - "id": 2753426, - "non_hive": { - "close": 3056, - "high": 471, - "low": 3056, - "open": 1999, - "volume": 10266 - }, - "open": "2020-10-25T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 133587, - "high": 133587, - "low": 13136, - "open": 13136, - "volume": 2559834 - }, - "id": 2753433, - "non_hive": { - "close": 20688, - "high": 20688, - "low": 1999, - "open": 1999, - "volume": 392432 - }, - "open": "2020-10-25T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 13083, - "high": 6229, - "low": 3771, - "open": 6229, - "volume": 23083 - }, - "id": 2753442, - "non_hive": { - "close": 2000, - "high": 964, - "low": 576, - "open": 964, - "volume": 3540 - }, - "open": "2020-10-25T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 22345, - "high": 6418, - "low": 22345, - "open": 6418, - "volume": 76917 - }, - "id": 2753447, - "non_hive": { - "close": 3385, - "high": 991, - "low": 3385, - "open": 991, - "volume": 11704 - }, - "open": "2020-10-25T18:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 500000, - "high": 1713, - "low": 86801, - "open": 13197, - "volume": 1014743 - }, - "id": 2753453, - "non_hive": { - "close": 75765, - "high": 263, - "low": 13151, - "open": 2000, - "volume": 153788 - }, - "open": "2020-10-25T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 10185, - "high": 10185, - "low": 10185, - "open": 10185, - "volume": 10185 - }, - "id": 2753462, - "non_hive": { - "close": 1556, - "high": 1556, - "low": 1556, - "open": 1556, - "volume": 1556 - }, - "open": "2020-10-25T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 8487, - "high": 8487, - "low": 2900, - "open": 2900, - "volume": 116796 - }, - "id": 2753465, - "non_hive": { - "close": 1310, - "high": 1310, - "low": 443, - "open": 443, - "volume": 17881 - }, - "open": "2020-10-25T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 55525, - "high": 19448, - "low": 55525, - "open": 12961, - "volume": 107409 - }, - "id": 2753473, - "non_hive": { - "close": 8485, - "high": 3001, - "low": 8485, - "open": 1999, - "volume": 16462 - }, - "open": "2020-10-25T19:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 19110, - "high": 6484, - "low": 19110, - "open": 6484, - "volume": 51000 - }, - "id": 2753478, - "non_hive": { - "close": 2920, - "high": 1000, - "low": 2920, - "open": 1000, - "volume": 7803 - }, - "open": "2020-10-25T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 35735, - "high": 35735, - "low": 6414, - "open": 530, - "volume": 91165 - }, - "id": 2753481, - "non_hive": { - "close": 5500, - "high": 5500, - "low": 973, - "open": 81, - "volume": 13960 - }, - "open": "2020-10-25T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6820, - "high": 6820, - "low": 13085, - "open": 13085, - "volume": 32649 - }, - "id": 2753485, - "non_hive": { - "close": 1049, - "high": 1049, - "low": 1999, - "open": 1999, - "volume": 5000 - }, - "open": "2020-10-25T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 7624, - "high": 6325, - "low": 7624, - "open": 13004, - "volume": 43158 - }, - "id": 2753488, - "non_hive": { - "close": 1166, - "high": 974, - "low": 1166, - "open": 1999, - "volume": 6631 - }, - "open": "2020-10-25T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 8078, - "high": 8078, - "low": 5448, - "open": 5448, - "volume": 13526 - }, - "id": 2753495, - "non_hive": { - "close": 1236, - "high": 1236, - "low": 833, - "open": 833, - "volume": 2069 - }, - "open": "2020-10-25T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 13080, - "high": 13, - "low": 61802, - "open": 13589, - "volume": 251664 - }, - "id": 2753498, - "non_hive": { - "close": 1999, - "high": 2, - "low": 9365, - "open": 2078, - "volume": 38192 - }, - "open": "2020-10-25T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 13122, - "high": 22622, - "low": 15, - "open": 2996, - "volume": 81614 - }, - "id": 2753505, - "non_hive": { - "close": 1999, - "high": 3459, - "low": 2, - "open": 458, - "volume": 12455 - }, - "open": "2020-10-25T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6553, - "high": 13, - "low": 13128, - "open": 74999, - "volume": 175872 - }, - "id": 2753516, - "non_hive": { - "close": 1002, - "high": 2, - "low": 1999, - "open": 11425, - "volume": 26801 - }, - "open": "2020-10-25T20:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 55402, - "high": 13170, - "low": 55402, - "open": 13170, - "volume": 74993 - }, - "id": 2753525, - "non_hive": { - "close": 8395, - "high": 2000, - "low": 8395, - "open": 2000, - "volume": 11370 - }, - "open": "2020-10-25T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 13092, - "high": 13248, - "low": 13092, - "open": 13085, - "volume": 65304 - }, - "id": 2753528, - "non_hive": { - "close": 1999, - "high": 2027, - "low": 1999, - "open": 1999, - "volume": 9979 - }, - "open": "2020-10-25T20:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 19652, - "high": 19652, - "low": 13100, - "open": 13100, - "volume": 93961 - }, - "id": 2753535, - "non_hive": { - "close": 3002, - "high": 3002, - "low": 1999, - "open": 1999, - "volume": 14343 - }, - "open": "2020-10-25T20:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 437, - "high": 13123, - "low": 437, - "open": 13123, - "volume": 26683 - }, - "id": 2753542, - "non_hive": { - "close": 66, - "high": 1999, - "low": 66, - "open": 1999, - "volume": 4064 - }, - "open": "2020-10-25T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 716, - "high": 5373, - "low": 716, - "open": 5373, - "volume": 19259 - }, - "id": 2753549, - "non_hive": { - "close": 108, - "high": 822, - "low": 108, - "open": 822, - "volume": 2930 - }, - "open": "2020-10-25T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7075, - "high": 13112, - "low": 7075, - "open": 13112, - "volume": 20187 - }, - "id": 2753554, - "non_hive": { - "close": 1079, - "high": 2000, - "low": 1079, - "open": 2000, - "volume": 3079 - }, - "open": "2020-10-25T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 7078, - "high": 7078, - "low": 7078, - "open": 7078, - "volume": 7078 - }, - "id": 2753557, - "non_hive": { - "close": 1079, - "high": 1079, - "low": 1079, - "open": 1079, - "volume": 1079 - }, - "open": "2020-10-25T20:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 74996, - "high": 931, - "low": 7663, - "open": 6036, - "volume": 165031 - }, - "id": 2753560, - "non_hive": { - "close": 11426, - "high": 142, - "low": 1167, - "open": 920, - "volume": 25143 - }, - "open": "2020-10-25T20:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1405, - "high": 1405, - "low": 13125, - "open": 13125, - "volume": 37777 - }, - "id": 2753572, - "non_hive": { - "close": 215, - "high": 215, - "low": 2000, - "open": 2000, - "volume": 5770 - }, - "open": "2020-10-25T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 3178, - "high": 3178, - "low": 68705, - "open": 13168, - "volume": 153178 - }, - "id": 2753580, - "non_hive": { - "close": 486, - "high": 486, - "low": 10411, - "open": 2000, - "volume": 23227 - }, - "open": "2020-10-25T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 13475, - "high": 13475, - "low": 13475, - "open": 13475, - "volume": 13475 - }, - "id": 2753586, - "non_hive": { - "close": 2055, - "high": 2055, - "low": 2055, - "open": 2055, - "volume": 2055 - }, - "open": "2020-10-25T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 3400, - "high": 13177, - "low": 3400, - "open": 13177, - "volume": 499999 - }, - "id": 2753589, - "non_hive": { - "close": 513, - "high": 2000, - "low": 513, - "open": 2000, - "volume": 75776 - }, - "open": "2020-10-25T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 3352, - "high": 3352, - "low": 3352, - "open": 3352, - "volume": 3352 - }, - "id": 2753594, - "non_hive": { - "close": 511, - "high": 511, - "low": 511, - "open": 511, - "volume": 511 - }, - "open": "2020-10-25T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 7892, - "high": 7892, - "low": 7892, - "open": 7892, - "volume": 7892 - }, - "id": 2753597, - "non_hive": { - "close": 1203, - "high": 1203, - "low": 1203, - "open": 1203, - "volume": 1203 - }, - "open": "2020-10-25T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 11006, - "high": 11006, - "low": 11006, - "open": 11006, - "volume": 11006 - }, - "id": 2753600, - "non_hive": { - "close": 1661, - "high": 1661, - "low": 1661, - "open": 1661, - "volume": 1661 - }, - "open": "2020-10-25T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 2896, - "high": 8088, - "low": 2896, - "open": 8088, - "volume": 10984 - }, - "id": 2753603, - "non_hive": { - "close": 441, - "high": 1232, - "low": 441, - "open": 1232, - "volume": 1673 - }, - "open": "2020-10-25T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12644, - "high": 12644, - "low": 12644, - "open": 12644, - "volume": 12644 - }, - "id": 2753606, - "non_hive": { - "close": 1926, - "high": 1926, - "low": 1926, - "open": 1926, - "volume": 1926 - }, - "open": "2020-10-25T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 13372, - "high": 13372, - "low": 13133, - "open": 13133, - "volume": 26505 - }, - "id": 2753610, - "non_hive": { - "close": 2037, - "high": 2037, - "low": 1999, - "open": 1999, - "volume": 4036 - }, - "open": "2020-10-25T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 19698, - "high": 19698, - "low": 19698, - "open": 19698, - "volume": 39396 - }, - "id": 2753613, - "non_hive": { - "close": 3000, - "high": 3000, - "low": 3000, - "open": 3000, - "volume": 6000 - }, - "open": "2020-10-25T22:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 13221, - "high": 13221, - "low": 13236, - "open": 13235, - "volume": 52926 - }, - "id": 2753617, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 8000 - }, - "open": "2020-10-25T22:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 3017, - "high": 3017, - "low": 13221, - "open": 13221, - "volume": 16238 - }, - "id": 2753623, - "non_hive": { - "close": 457, - "high": 457, - "low": 2000, - "open": 2000, - "volume": 2457 - }, - "open": "2020-10-25T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 126287, - "high": 126287, - "low": 126287, - "open": 126287, - "volume": 126287 - }, - "id": 2753627, - "non_hive": { - "close": 19237, - "high": 19237, - "low": 19237, - "open": 19237, - "volume": 19237 - }, - "open": "2020-10-25T22:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 13194, - "high": 90344, - "low": 1120, - "open": 13123, - "volume": 512576 - }, - "id": 2753630, - "non_hive": { - "close": 1999, - "high": 13822, - "low": 169, - "open": 1999, - "volume": 78372 - }, - "open": "2020-10-25T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 14801, - "high": 14801, - "low": 14801, - "open": 14801, - "volume": 14801 - }, - "id": 2753636, - "non_hive": { - "close": 2264, - "high": 2264, - "low": 2264, - "open": 2264, - "volume": 2264 - }, - "open": "2020-10-25T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 7706, - "high": 17104, - "low": 7706, - "open": 17104, - "volume": 24810 - }, - "id": 2753640, - "non_hive": { - "close": 1178, - "high": 2616, - "low": 1178, - "open": 2616, - "volume": 3794 - }, - "open": "2020-10-25T23:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 5464, - "high": 6421, - "low": 5464, - "open": 6421, - "volume": 11918 - }, - "id": 2753645, - "non_hive": { - "close": 827, - "high": 975, - "low": 827, - "open": 975, - "volume": 1807 - }, - "open": "2020-10-25T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 5997, - "high": 976, - "low": 5997, - "open": 976, - "volume": 27149 - }, - "id": 2753648, - "non_hive": { - "close": 905, - "high": 148, - "low": 905, - "open": 148, - "volume": 4101 - }, - "open": "2020-10-25T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 5487, - "high": 5487, - "low": 13127, - "open": 13127, - "volume": 18614 - }, - "id": 2753653, - "non_hive": { - "close": 836, - "high": 836, - "low": 1999, - "open": 1999, - "volume": 2835 - }, - "open": "2020-10-26T00:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 75000, - "high": 75000, - "low": 75000, - "open": 75000, - "volume": 75000 - }, - "id": 2753658, - "non_hive": { - "close": 11426, - "high": 11426, - "low": 11426, - "open": 11426, - "volume": 11426 - }, - "open": "2020-10-26T00:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 22000, - "high": 22000, - "low": 22000, - "open": 22000, - "volume": 22000 - }, - "id": 2753661, - "non_hive": { - "close": 3351, - "high": 3351, - "low": 3351, - "open": 3351, - "volume": 3351 - }, - "open": "2020-10-26T00:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 52, - "high": 52, - "low": 52, - "open": 52, - "volume": 52 - }, - "id": 2753664, - "non_hive": { - "close": 8, - "high": 8, - "low": 8, - "open": 8, - "volume": 8 - }, - "open": "2020-10-26T00:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 23812, - "high": 23812, - "low": 13159, - "open": 13159, - "volume": 36971 - }, - "id": 2753667, - "non_hive": { - "close": 3619, - "high": 3619, - "low": 1999, - "open": 1999, - "volume": 5618 - }, - "open": "2020-10-26T00:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 12067, - "high": 7836, - "low": 13161, - "open": 7836, - "volume": 33064 - }, - "id": 2753670, - "non_hive": { - "close": 1833, - "high": 1191, - "low": 1999, - "open": 1191, - "volume": 5023 - }, - "open": "2020-10-26T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 44707, - "high": 44707, - "low": 5293, - "open": 7866, - "volume": 57866 - }, - "id": 2753675, - "non_hive": { - "close": 6792, - "high": 6792, - "low": 804, - "open": 1195, - "volume": 8791 - }, - "open": "2020-10-26T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 5711, - "high": 5711, - "low": 1122, - "open": 1122, - "volume": 6833 - }, - "id": 2753680, - "non_hive": { - "close": 868, - "high": 868, - "low": 170, - "open": 170, - "volume": 1038 - }, - "open": "2020-10-26T00:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 657, - "high": 657, - "low": 123000, - "open": 13208, - "volume": 136865 - }, - "id": 2753685, - "non_hive": { - "close": 100, - "high": 100, - "low": 18623, - "open": 2000, - "volume": 20723 - }, - "open": "2020-10-26T01:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 99, - "high": 99, - "low": 99, - "open": 99, - "volume": 99 - }, - "id": 2753691, - "non_hive": { - "close": 14, - "high": 14, - "low": 14, - "open": 14, - "volume": 14 - }, - "open": "2020-10-26T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 28835, - "high": 28835, - "low": 28835, - "open": 28835, - "volume": 28835 - }, - "id": 2753694, - "non_hive": { - "close": 4380, - "high": 4380, - "low": 4380, - "open": 4380, - "volume": 4380 - }, - "open": "2020-10-26T01:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6584, - "high": 6584, - "low": 60753, - "open": 997, - "volume": 81581 - }, - "id": 2753697, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 9171, - "open": 151, - "volume": 12322 - }, - "open": "2020-10-26T01:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 271160, - "high": 271160, - "low": 74999, - "open": 60753, - "volume": 406912 - }, - "id": 2753701, - "non_hive": { - "close": 41184, - "high": 41184, - "low": 11390, - "open": 9227, - "volume": 61801 - }, - "open": "2020-10-26T02:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 9534, - "high": 9534, - "low": 55731, - "open": 5540, - "volume": 87534 - }, - "id": 2753707, - "non_hive": { - "close": 1448, - "high": 1448, - "low": 8410, - "open": 841, - "volume": 13224 - }, - "open": "2020-10-26T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 9959, - "high": 3902, - "low": 9959, - "open": 13251, - "volume": 100000 - }, - "id": 2753713, - "non_hive": { - "close": 1502, - "high": 589, - "low": 1502, - "open": 2000, - "volume": 15091 - }, - "open": "2020-10-26T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 70320, - "high": 70320, - "low": 3628, - "open": 3628, - "volume": 73948 - }, - "id": 2753722, - "non_hive": { - "close": 10685, - "high": 10685, - "low": 551, - "open": 551, - "volume": 11236 - }, - "open": "2020-10-26T03:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7480, - "high": 100002, - "low": 7480, - "open": 100002, - "volume": 107482 - }, - "id": 2753725, - "non_hive": { - "close": 1136, - "high": 15195, - "low": 1136, - "open": 15195, - "volume": 16331 - }, - "open": "2020-10-26T03:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 9249, - "high": 20003, - "low": 9249, - "open": 20003, - "volume": 29252 - }, - "id": 2753730, - "non_hive": { - "close": 1404, - "high": 3038, - "low": 1404, - "open": 3038, - "volume": 4442 - }, - "open": "2020-10-26T03:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 16923, - "high": 3584, - "low": 16923, - "open": 3584, - "volume": 69562 - }, - "id": 2753735, - "non_hive": { - "close": 2553, - "high": 544, - "low": 2553, - "open": 544, - "volume": 10531 - }, - "open": "2020-10-26T03:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 26, - "high": 26, - "low": 26, - "open": 26, - "volume": 26 - }, - "id": 2753740, - "non_hive": { - "close": 4, - "high": 4, - "low": 4, - "open": 4, - "volume": 4 - }, - "open": "2020-10-26T04:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 19, - "high": 19, - "low": 19, - "open": 19, - "volume": 19 - }, - "id": 2753744, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 3, - "volume": 3 - }, - "open": "2020-10-26T04:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 752, - "high": 33631, - "low": 752, - "open": 33631, - "volume": 34383 - }, - "id": 2753747, - "non_hive": { - "close": 114, - "high": 5100, - "low": 114, - "open": 5100, - "volume": 5214 - }, - "open": "2020-10-26T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7300, - "high": 13193, - "low": 7300, - "open": 13193, - "volume": 75194 - }, - "id": 2753750, - "non_hive": { - "close": 1105, - "high": 1999, - "low": 1105, - "open": 1999, - "volume": 11389 - }, - "open": "2020-10-26T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 9121, - "high": 9121, - "low": 9121, - "open": 9121, - "volume": 9121 - }, - "id": 2753762, - "non_hive": { - "close": 1384, - "high": 1384, - "low": 1384, - "open": 1384, - "volume": 1384 - }, - "open": "2020-10-26T04:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 9670, - "high": 9670, - "low": 9670, - "open": 9670, - "volume": 9670 - }, - "id": 2753765, - "non_hive": { - "close": 1467, - "high": 1467, - "low": 1467, - "open": 1467, - "volume": 1467 - }, - "open": "2020-10-26T04:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 410276, - "high": 13, - "low": 410276, - "open": 1700, - "volume": 701700 - }, - "id": 2753768, - "non_hive": { - "close": 61236, - "high": 2, - "low": 61236, - "open": 256, - "volume": 104990 - }, - "open": "2020-10-26T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 7, - "high": 317, - "low": 7, - "open": 317, - "volume": 324 - }, - "id": 2753776, - "non_hive": { - "close": 1, - "high": 48, - "low": 1, - "open": 48, - "volume": 49 - }, - "open": "2020-10-26T05:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6177, - "high": 6177, - "low": 13258, - "open": 13258, - "volume": 25885 - }, - "id": 2753779, - "non_hive": { - "close": 935, - "high": 935, - "low": 1999, - "open": 1999, - "volume": 3907 - }, - "open": "2020-10-26T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 252, - "high": 252, - "low": 252, - "open": 252, - "volume": 252 - }, - "id": 2753782, - "non_hive": { - "close": 38, - "high": 38, - "low": 38, - "open": 38, - "volume": 38 - }, - "open": "2020-10-26T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 277, - "high": 277, - "low": 277, - "open": 277, - "volume": 277 - }, - "id": 2753785, - "non_hive": { - "close": 42, - "high": 42, - "low": 42, - "open": 42, - "volume": 42 - }, - "open": "2020-10-26T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13056, - "high": 13056, - "low": 13056, - "open": 13056, - "volume": 13056 - }, - "id": 2753788, - "non_hive": { - "close": 1978, - "high": 1978, - "low": 1978, - "open": 1978, - "volume": 1978 - }, - "open": "2020-10-26T06:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6000, - "high": 14618, - "low": 6000, - "open": 14618, - "volume": 20618 - }, - "id": 2753792, - "non_hive": { - "close": 909, - "high": 2216, - "low": 909, - "open": 2216, - "volume": 3125 - }, - "open": "2020-10-26T06:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 217681, - "high": 217681, - "low": 217681, - "open": 217681, - "volume": 217681 - }, - "id": 2753796, - "non_hive": { - "close": 33000, - "high": 33000, - "low": 33000, - "open": 33000, - "volume": 33000 - }, - "open": "2020-10-26T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 16372, - "high": 16372, - "low": 16372, - "open": 16372, - "volume": 16372 - }, - "id": 2753799, - "non_hive": { - "close": 2482, - "high": 2482, - "low": 2482, - "open": 2482, - "volume": 2482 - }, - "open": "2020-10-26T06:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 9862, - "high": 9862, - "low": 9862, - "open": 9862, - "volume": 9862 - }, - "id": 2753802, - "non_hive": { - "close": 1495, - "high": 1495, - "low": 1495, - "open": 1495, - "volume": 1495 - }, - "open": "2020-10-26T06:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 4294, - "high": 593, - "low": 158, - "open": 23842, - "volume": 29118 - }, - "id": 2753805, - "non_hive": { - "close": 646, - "high": 90, - "low": 23, - "open": 3614, - "volume": 4408 - }, - "open": "2020-10-26T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 4294, - "high": 4294, - "low": 4294, - "open": 4294, - "volume": 4294 - }, - "id": 2753812, - "non_hive": { - "close": 650, - "high": 650, - "low": 650, - "open": 650, - "volume": 650 - }, - "open": "2020-10-26T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 28101, - "high": 28101, - "low": 28101, - "open": 28101, - "volume": 28101 - }, - "id": 2753815, - "non_hive": { - "close": 4257, - "high": 4257, - "low": 4257, - "open": 4257, - "volume": 4257 - }, - "open": "2020-10-26T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 10000, - "high": 23857, - "low": 10000, - "open": 23857, - "volume": 39948 - }, - "id": 2753818, - "non_hive": { - "close": 1513, - "high": 3614, - "low": 1513, - "open": 3614, - "volume": 6049 - }, - "open": "2020-10-26T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 4394, - "high": 15606, - "low": 4394, - "open": 15606, - "volume": 20000 - }, - "id": 2753823, - "non_hive": { - "close": 665, - "high": 2364, - "low": 665, - "open": 2364, - "volume": 3029 - }, - "open": "2020-10-26T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 178, - "high": 178, - "low": 2192, - "open": 2192, - "volume": 2370 - }, - "id": 2753826, - "non_hive": { - "close": 27, - "high": 27, - "low": 332, - "open": 332, - "volume": 359 - }, - "open": "2020-10-26T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 19771, - "high": 19771, - "low": 19771, - "open": 19771, - "volume": 19771 - }, - "id": 2753829, - "non_hive": { - "close": 2997, - "high": 2997, - "low": 2997, - "open": 2997, - "volume": 2997 - }, - "open": "2020-10-26T08:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 73, - "high": 1546, - "low": 5711, - "open": 2385, - "volume": 69834 - }, - "id": 2753833, - "non_hive": { - "close": 11, - "high": 234, - "low": 855, - "open": 359, - "volume": 10517 - }, - "open": "2020-10-26T08:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16722, - "high": 16722, - "low": 16722, - "open": 16722, - "volume": 16722 - }, - "id": 2753840, - "non_hive": { - "close": 2525, - "high": 2525, - "low": 2525, - "open": 2525, - "volume": 2525 - }, - "open": "2020-10-26T08:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 100000, - "high": 2085, - "low": 4883, - "open": 4883, - "volume": 106968 - }, - "id": 2753843, - "non_hive": { - "close": 15150, - "high": 316, - "low": 739, - "open": 739, - "volume": 16205 - }, - "open": "2020-10-26T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 18968, - "high": 18968, - "low": 18968, - "open": 18968, - "volume": 18968 - }, - "id": 2753848, - "non_hive": { - "close": 2874, - "high": 2874, - "low": 2874, - "open": 2874, - "volume": 2874 - }, - "open": "2020-10-26T08:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6444, - "high": 24052, - "low": 6501, - "open": 24052, - "volume": 59544 - }, - "id": 2753851, - "non_hive": { - "close": 975, - "high": 3644, - "low": 970, - "open": 3644, - "volume": 8983 - }, - "open": "2020-10-26T09:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1394, - "high": 158, - "low": 1394, - "open": 158, - "volume": 1552 - }, - "id": 2753857, - "non_hive": { - "close": 211, - "high": 24, - "low": 211, - "open": 24, - "volume": 235 - }, - "open": "2020-10-26T09:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 317404, - "high": 317404, - "low": 4898, - "open": 13258, - "volume": 341996 - }, - "id": 2753860, - "non_hive": { - "close": 48097, - "high": 48097, - "low": 738, - "open": 1999, - "volume": 51809 - }, - "open": "2020-10-26T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6480, - "high": 26503, - "low": 6480, - "open": 26503, - "volume": 52743 - }, - "id": 2753863, - "non_hive": { - "close": 971, - "high": 4016, - "low": 971, - "open": 4016, - "volume": 7961 - }, - "open": "2020-10-26T09:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3003, - "high": 3003, - "low": 3003, - "open": 3003, - "volume": 3003 - }, - "id": 2753866, - "non_hive": { - "close": 455, - "high": 455, - "low": 455, - "open": 455, - "volume": 455 - }, - "open": "2020-10-26T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 1770, - "high": 1770, - "low": 13215, - "open": 13215, - "volume": 14985 - }, - "id": 2753869, - "non_hive": { - "close": 268, - "high": 268, - "low": 1999, - "open": 1999, - "volume": 2267 - }, - "open": "2020-10-26T09:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6585, - "high": 13, - "low": 6585, - "open": 17228, - "volume": 534449 - }, - "id": 2753872, - "non_hive": { - "close": 967, - "high": 2, - "low": 967, - "open": 2606, - "volume": 79124 - }, - "open": "2020-10-26T09:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 6601, - "high": 6925, - "low": 6601, - "open": 6510, - "volume": 67534 - }, - "id": 2753877, - "non_hive": { - "close": 967, - "high": 1031, - "low": 967, - "open": 969, - "volume": 9955 - }, - "open": "2020-10-26T09:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6503, - "high": 13378, - "low": 6525, - "open": 6525, - "volume": 46346 - }, - "id": 2753882, - "non_hive": { - "close": 968, - "high": 1999, - "low": 968, - "open": 968, - "volume": 6905 - }, - "open": "2020-10-26T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6596, - "high": 71379, - "low": 7, - "open": 7, - "volume": 151652 - }, - "id": 2753890, - "non_hive": { - "close": 969, - "high": 10757, - "low": 1, - "open": 1, - "volume": 22656 - }, - "open": "2020-10-26T10:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6596, - "high": 333, - "low": 6596, - "open": 333, - "volume": 13525 - }, - "id": 2753898, - "non_hive": { - "close": 969, - "high": 49, - "low": 969, - "open": 49, - "volume": 1987 - }, - "open": "2020-10-26T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 80, - "high": 80, - "low": 24921, - "open": 24921, - "volume": 25001 - }, - "id": 2753905, - "non_hive": { - "close": 12, - "high": 12, - "low": 3660, - "open": 3660, - "volume": 3672 - }, - "open": "2020-10-26T10:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 14, - "high": 20155, - "low": 14, - "open": 13481, - "volume": 40095 - }, - "id": 2753908, - "non_hive": { - "close": 2, - "high": 3000, - "low": 2, - "open": 1999, - "volume": 5957 - }, - "open": "2020-10-26T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 58, - "high": 7428, - "low": 58, - "open": 6510, - "volume": 33436 - }, - "id": 2753913, - "non_hive": { - "close": 8, - "high": 1117, - "low": 8, - "open": 969, - "volume": 5009 - }, - "open": "2020-10-26T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 9673, - "high": 9673, - "low": 9673, - "open": 9673, - "volume": 9673 - }, - "id": 2753918, - "non_hive": { - "close": 1457, - "high": 1457, - "low": 1457, - "open": 1457, - "volume": 1457 - }, - "open": "2020-10-26T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6450, - "high": 6450, - "low": 6450, - "open": 6450, - "volume": 6450 - }, - "id": 2753921, - "non_hive": { - "close": 973, - "high": 973, - "low": 973, - "open": 973, - "volume": 973 - }, - "open": "2020-10-26T10:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1552, - "high": 1552, - "low": 1552, - "open": 1552, - "volume": 1552 - }, - "id": 2753924, - "non_hive": { - "close": 235, - "high": 235, - "low": 235, - "open": 235, - "volume": 235 - }, - "open": "2020-10-26T11:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 46639, - "high": 18706, - "low": 46639, - "open": 18706, - "volume": 75000 - }, - "id": 2753928, - "non_hive": { - "close": 7035, - "high": 2831, - "low": 7035, - "open": 2831, - "volume": 11323 - }, - "open": "2020-10-26T11:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 64902, - "high": 64902, - "low": 104, - "open": 104, - "volume": 65006 - }, - "id": 2753931, - "non_hive": { - "close": 9838, - "high": 9838, - "low": 15, - "open": 15, - "volume": 9853 - }, - "open": "2020-10-26T11:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 17287, - "high": 33163, - "low": 17287, - "open": 6429, - "volume": 68429 - }, - "id": 2753934, - "non_hive": { - "close": 2616, - "high": 5027, - "low": 2616, - "open": 973, - "volume": 10364 - }, - "open": "2020-10-26T11:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 60103, - "high": 6525, - "low": 60103, - "open": 6525, - "volume": 75000 - }, - "id": 2753938, - "non_hive": { - "close": 9066, - "high": 989, - "low": 9066, - "open": 989, - "volume": 11322 - }, - "open": "2020-10-26T11:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13662, - "high": 13662, - "low": 13662, - "open": 13662, - "volume": 13662 - }, - "id": 2753941, - "non_hive": { - "close": 2071, - "high": 2071, - "low": 2071, - "open": 2071, - "volume": 2071 - }, - "open": "2020-10-26T11:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 12646, - "high": 4961, - "low": 12646, - "open": 4961, - "volume": 59370 - }, - "id": 2753944, - "non_hive": { - "close": 1907, - "high": 752, - "low": 1907, - "open": 752, - "volume": 8980 - }, - "open": "2020-10-26T11:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 19818, - "high": 19818, - "low": 13194, - "open": 13194, - "volume": 33012 - }, - "id": 2753947, - "non_hive": { - "close": 3004, - "high": 3004, - "low": 1999, - "open": 1999, - "volume": 5003 - }, - "open": "2020-10-26T12:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 23140, - "high": 13, - "low": 23140, - "open": 16720, - "volume": 74995 - }, - "id": 2753951, - "non_hive": { - "close": 3490, - "high": 2, - "low": 3490, - "open": 2529, - "volume": 11321 - }, - "open": "2020-10-26T12:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13210, - "high": 13210, - "low": 13210, - "open": 13210, - "volume": 13210 - }, - "id": 2753954, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-26T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 31109, - "high": 31109, - "low": 31109, - "open": 31109, - "volume": 31109 - }, - "id": 2753957, - "non_hive": { - "close": 4710, - "high": 4710, - "low": 4710, - "open": 4710, - "volume": 4710 - }, - "open": "2020-10-26T12:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 50756, - "high": 13244, - "low": 50756, - "open": 13244, - "volume": 64000 - }, - "id": 2753960, - "non_hive": { - "close": 7664, - "high": 2000, - "low": 7664, - "open": 2000, - "volume": 9664 - }, - "open": "2020-10-26T12:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 6, - "high": 6, - "low": 13202, - "open": 13202, - "volume": 13208 - }, - "id": 2753963, - "non_hive": { - "close": 1, - "high": 1, - "low": 1999, - "open": 1999, - "volume": 2000 - }, - "open": "2020-10-26T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 74994, - "high": 74994, - "low": 74994, - "open": 74994, - "volume": 74994 - }, - "id": 2753966, - "non_hive": { - "close": 11360, - "high": 11360, - "low": 11360, - "open": 11360, - "volume": 11360 - }, - "open": "2020-10-26T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 52809, - "high": 68064, - "low": 52809, - "open": 68064, - "volume": 120873 - }, - "id": 2753969, - "non_hive": { - "close": 8000, - "high": 10311, - "low": 8000, - "open": 10311, - "volume": 18311 - }, - "open": "2020-10-26T13:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2753973, - "non_hive": { - "close": 151, - "high": 151, - "low": 151, - "open": 151, - "volume": 151 - }, - "open": "2020-10-26T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2753976, - "non_hive": { - "close": 151, - "high": 151, - "low": 151, - "open": 151, - "volume": 151 - }, - "open": "2020-10-26T13:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6133, - "high": 22640, - "low": 6133, - "open": 10727, - "volume": 291590 - }, - "id": 2753979, - "non_hive": { - "close": 922, - "high": 3430, - "low": 922, - "open": 1624, - "volume": 44033 - }, - "open": "2020-10-26T13:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 20050, - "high": 20050, - "low": 500000, - "open": 13194, - "volume": 4333291 - }, - "id": 2753990, - "non_hive": { - "close": 3053, - "high": 3053, - "low": 75499, - "open": 1999, - "volume": 658445 - }, - "open": "2020-10-26T13:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 13244, - "high": 164, - "low": 13244, - "open": 6436, - "volume": 70381 - }, - "id": 2754000, - "non_hive": { - "close": 2000, - "high": 25, - "low": 2000, - "open": 975, - "volume": 10678 - }, - "open": "2020-10-26T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 65104, - "high": 13175, - "low": 65104, - "open": 13175, - "volume": 492550 - }, - "id": 2754009, - "non_hive": { - "close": 9700, - "high": 1999, - "low": 9700, - "open": 1999, - "volume": 74237 - }, - "open": "2020-10-26T13:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 422088, - "high": 36615, - "low": 6642, - "open": 36615, - "volume": 543569 - }, - "id": 2754023, - "non_hive": { - "close": 63702, - "high": 5526, - "low": 999, - "open": 5526, - "volume": 82026 - }, - "open": "2020-10-26T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 7000, - "high": 7000, - "low": 7000, - "open": 7000, - "volume": 7000 - }, - "id": 2754030, - "non_hive": { - "close": 1056, - "high": 1056, - "low": 1056, - "open": 1056, - "volume": 1056 - }, - "open": "2020-10-26T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6252, - "high": 6252, - "low": 6252, - "open": 6252, - "volume": 6252 - }, - "id": 2754034, - "non_hive": { - "close": 943, - "high": 943, - "low": 943, - "open": 943, - "volume": 943 - }, - "open": "2020-10-26T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 2604, - "high": 377, - "low": 2604, - "open": 377, - "volume": 2981 - }, - "id": 2754037, - "non_hive": { - "close": 393, - "high": 57, - "low": 393, - "open": 57, - "volume": 450 - }, - "open": "2020-10-26T14:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 7006, - "high": 2991, - "low": 7006, - "open": 2991, - "volume": 9997 - }, - "id": 2754042, - "non_hive": { - "close": 1054, - "high": 450, - "low": 1054, - "open": 450, - "volume": 1504 - }, - "open": "2020-10-26T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 15767, - "high": 15767, - "low": 3292, - "open": 3292, - "volume": 19059 - }, - "id": 2754046, - "non_hive": { - "close": 2373, - "high": 2373, - "low": 495, - "open": 495, - "volume": 2868 - }, - "open": "2020-10-26T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2754049, - "non_hive": { - "close": 149, - "high": 149, - "low": 149, - "open": 149, - "volume": 149 - }, - "open": "2020-10-26T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 11865, - "high": 13385, - "low": 11865, - "open": 13386, - "volume": 125250 - }, - "id": 2754052, - "non_hive": { - "close": 1772, - "high": 2000, - "low": 1772, - "open": 2000, - "volume": 18711 - }, - "open": "2020-10-26T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 70848, - "high": 13385, - "low": 70848, - "open": 13385, - "volume": 84233 - }, - "id": 2754057, - "non_hive": { - "close": 10585, - "high": 2000, - "low": 10585, - "open": 2000, - "volume": 12585 - }, - "open": "2020-10-26T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 9846, - "high": 9846, - "low": 13297, - "open": 13297, - "volume": 23143 - }, - "id": 2754060, - "non_hive": { - "close": 1481, - "high": 1481, - "low": 1999, - "open": 1999, - "volume": 3480 - }, - "open": "2020-10-26T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 61682, - "high": 61682, - "low": 320, - "open": 12977, - "volume": 74979 - }, - "id": 2754063, - "non_hive": { - "close": 9278, - "high": 9278, - "low": 48, - "open": 1951, - "volume": 11277 - }, - "open": "2020-10-26T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13297, - "high": 13297, - "low": 13297, - "open": 13297, - "volume": 13297 - }, - "id": 2754068, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-26T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13310, - "high": 9958, - "low": 13310, - "open": 9958, - "volume": 27812 - }, - "id": 2754071, - "non_hive": { - "close": 1999, - "high": 1497, - "low": 1999, - "open": 1497, - "volume": 4179 - }, - "open": "2020-10-26T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 19090, - "high": 19090, - "low": 19090, - "open": 19090, - "volume": 19090 - }, - "id": 2754077, - "non_hive": { - "close": 2870, - "high": 2870, - "low": 2870, - "open": 2870, - "volume": 2870 - }, - "open": "2020-10-26T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6175, - "high": 6175, - "low": 13310, - "open": 13310, - "volume": 19971 - }, - "id": 2754080, - "non_hive": { - "close": 928, - "high": 928, - "low": 1999, - "open": 1999, - "volume": 3000 - }, - "open": "2020-10-26T15:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 15443, - "high": 15443, - "low": 15086, - "open": 15086, - "volume": 30529 - }, - "id": 2754084, - "non_hive": { - "close": 2323, - "high": 2323, - "low": 2269, - "open": 2269, - "volume": 4592 - }, - "open": "2020-10-26T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 8234, - "high": 22014, - "low": 22320, - "open": 22014, - "volume": 259987 - }, - "id": 2754089, - "non_hive": { - "close": 1230, - "high": 3302, - "low": 3334, - "open": 3302, - "volume": 38853 - }, - "open": "2020-10-26T15:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6487, - "high": 6487, - "low": 3346, - "open": 13389, - "volume": 80096 - }, - "id": 2754093, - "non_hive": { - "close": 973, - "high": 973, - "low": 495, - "open": 2000, - "volume": 11945 - }, - "open": "2020-10-26T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 24485, - "high": 24485, - "low": 5399, - "open": 1094, - "volume": 80978 - }, - "id": 2754098, - "non_hive": { - "close": 3679, - "high": 3679, - "low": 809, - "open": 164, - "volume": 12164 - }, - "open": "2020-10-26T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 17397, - "high": 17397, - "low": 20000, - "open": 20000, - "volume": 43875 - }, - "id": 2754104, - "non_hive": { - "close": 2617, - "high": 2617, - "low": 3005, - "open": 3005, - "volume": 6596 - }, - "open": "2020-10-26T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 20000, - "high": 20000, - "low": 20000, - "open": 20000, - "volume": 20000 - }, - "id": 2754110, - "non_hive": { - "close": 3006, - "high": 3006, - "low": 3006, - "open": 3006, - "volume": 3006 - }, - "open": "2020-10-26T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6478, - "high": 638, - "low": 2282, - "open": 638, - "volume": 65565 - }, - "id": 2754113, - "non_hive": { - "close": 974, - "high": 96, - "low": 337, - "open": 96, - "volume": 9777 - }, - "open": "2020-10-26T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 36930, - "high": 36930, - "low": 36930, - "open": 36930, - "volume": 36930 - }, - "id": 2754118, - "non_hive": { - "close": 5555, - "high": 5555, - "low": 5555, - "open": 5555, - "volume": 5555 - }, - "open": "2020-10-26T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 74, - "high": 74, - "low": 74, - "open": 74, - "volume": 74 - }, - "id": 2754121, - "non_hive": { - "close": 11, - "high": 11, - "low": 11, - "open": 11, - "volume": 11 - }, - "open": "2020-10-26T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 38761, - "high": 38761, - "low": 38761, - "open": 38761, - "volume": 38761 - }, - "id": 2754124, - "non_hive": { - "close": 5830, - "high": 5830, - "low": 5830, - "open": 5830, - "volume": 5830 - }, - "open": "2020-10-26T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 3311, - "high": 3311, - "low": 37332, - "open": 37332, - "volume": 40643 - }, - "id": 2754127, - "non_hive": { - "close": 498, - "high": 498, - "low": 5615, - "open": 5615, - "volume": 6113 - }, - "open": "2020-10-26T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 4950, - "high": 38712, - "low": 4950, - "open": 38712, - "volume": 43662 - }, - "id": 2754132, - "non_hive": { - "close": 737, - "high": 5823, - "low": 737, - "open": 5823, - "volume": 6560 - }, - "open": "2020-10-26T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 4950, - "high": 5803, - "low": 5005, - "open": 5803, - "volume": 15758 - }, - "id": 2754136, - "non_hive": { - "close": 744, - "high": 873, - "low": 752, - "open": 873, - "volume": 2369 - }, - "open": "2020-10-26T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 744, - "high": 744, - "low": 9712, - "open": 9712, - "volume": 10456 - }, - "id": 2754142, - "non_hive": { - "close": 112, - "high": 112, - "low": 1461, - "open": 1461, - "volume": 1573 - }, - "open": "2020-10-26T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 13294, - "high": 13294, - "low": 76056, - "open": 76056, - "volume": 89350 - }, - "id": 2754148, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 11440, - "open": 11440, - "volume": 13440 - }, - "open": "2020-10-26T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 20125, - "high": 12738, - "low": 20125, - "open": 13411, - "volume": 1141808 - }, - "id": 2754152, - "non_hive": { - "close": 3000, - "high": 1921, - "low": 3000, - "open": 2000, - "volume": 170285 - }, - "open": "2020-10-26T17:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 9224, - "high": 259, - "low": 9224, - "open": 259, - "volume": 9483 - }, - "id": 2754159, - "non_hive": { - "close": 1387, - "high": 39, - "low": 1387, - "open": 39, - "volume": 1426 - }, - "open": "2020-10-26T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6337, - "high": 6337, - "low": 20000, - "open": 20000, - "volume": 26337 - }, - "id": 2754164, - "non_hive": { - "close": 953, - "high": 953, - "low": 3007, - "open": 3007, - "volume": 3960 - }, - "open": "2020-10-26T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 117198, - "high": 117198, - "low": 6993, - "open": 33543, - "volume": 171030 - }, - "id": 2754169, - "non_hive": { - "close": 17623, - "high": 17623, - "low": 1048, - "open": 5043, - "volume": 25713 - }, - "open": "2020-10-26T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6993, - "high": 19598, - "low": 321, - "open": 13301, - "volume": 71586 - }, - "id": 2754176, - "non_hive": { - "close": 1048, - "high": 2947, - "low": 48, - "open": 1999, - "volume": 10753 - }, - "open": "2020-10-26T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1647, - "high": 5346, - "low": 1647, - "open": 5346, - "volume": 14010 - }, - "id": 2754189, - "non_hive": { - "close": 246, - "high": 804, - "low": 246, - "open": 804, - "volume": 2099 - }, - "open": "2020-10-26T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 132937, - "high": 6326, - "low": 667, - "open": 6326, - "volume": 146923 - }, - "id": 2754194, - "non_hive": { - "close": 19923, - "high": 949, - "low": 99, - "open": 949, - "volume": 22019 - }, - "open": "2020-10-26T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 7034, - "high": 1920, - "low": 7034, - "open": 1920, - "volume": 9436 - }, - "id": 2754201, - "non_hive": { - "close": 1048, - "high": 287, - "low": 1048, - "open": 287, - "volume": 1407 - }, - "open": "2020-10-26T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 3436, - "high": 33, - "low": 650, - "open": 6384, - "volume": 122105 - }, - "id": 2754206, - "non_hive": { - "close": 515, - "high": 5, - "low": 96, - "open": 952, - "volume": 18202 - }, - "open": "2020-10-26T17:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 7051, - "high": 7051, - "low": 7051, - "open": 7051, - "volume": 7051 - }, - "id": 2754214, - "non_hive": { - "close": 1049, - "high": 1049, - "low": 1049, - "open": 1049, - "volume": 1049 - }, - "open": "2020-10-26T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 5559, - "high": 6392, - "low": 659, - "open": 6392, - "volume": 12610 - }, - "id": 2754218, - "non_hive": { - "close": 827, - "high": 951, - "low": 98, - "open": 951, - "volume": 1876 - }, - "open": "2020-10-26T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6816, - "high": 6816, - "low": 1492, - "open": 1492, - "volume": 8308 - }, - "id": 2754223, - "non_hive": { - "close": 1015, - "high": 1015, - "low": 221, - "open": 221, - "volume": 1236 - }, - "open": "2020-10-26T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2028, - "high": 2028, - "low": 2028, - "open": 2028, - "volume": 2028 - }, - "id": 2754226, - "non_hive": { - "close": 302, - "high": 302, - "low": 302, - "open": 302, - "volume": 302 - }, - "open": "2020-10-26T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 5819, - "high": 7011, - "low": 5819, - "open": 7011, - "volume": 800000 - }, - "id": 2754229, - "non_hive": { - "close": 857, - "high": 1044, - "low": 857, - "open": 1044, - "volume": 118335 - }, - "open": "2020-10-26T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7051, - "high": 7051, - "low": 13514, - "open": 324, - "volume": 27633 - }, - "id": 2754233, - "non_hive": { - "close": 1047, - "high": 1047, - "low": 1999, - "open": 48, - "volume": 4092 - }, - "open": "2020-10-26T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6970, - "high": 9389, - "low": 142, - "open": 9389, - "volume": 1086677 - }, - "id": 2754238, - "non_hive": { - "close": 1035, - "high": 1398, - "low": 21, - "open": 1398, - "volume": 161364 - }, - "open": "2020-10-26T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6481, - "high": 753, - "low": 6481, - "open": 753, - "volume": 33030 - }, - "id": 2754246, - "non_hive": { - "close": 951, - "high": 111, - "low": 951, - "open": 111, - "volume": 4854 - }, - "open": "2020-10-26T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1576, - "high": 91800, - "low": 1079, - "open": 69176, - "volume": 163739 - }, - "id": 2754249, - "non_hive": { - "close": 231, - "high": 13670, - "low": 158, - "open": 10301, - "volume": 24376 - }, - "open": "2020-10-26T18:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 950, - "high": 950, - "low": 477, - "open": 13469, - "volume": 49427 - }, - "id": 2754254, - "non_hive": { - "close": 141, - "high": 141, - "low": 70, - "open": 1999, - "volume": 7335 - }, - "open": "2020-10-26T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 8004, - "high": 8004, - "low": 8004, - "open": 8004, - "volume": 8004 - }, - "id": 2754261, - "non_hive": { - "close": 1188, - "high": 1188, - "low": 1188, - "open": 1188, - "volume": 1188 - }, - "open": "2020-10-26T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13426, - "high": 1288, - "low": 21, - "open": 4515, - "volume": 33039 - }, - "id": 2754264, - "non_hive": { - "close": 1999, - "high": 192, - "low": 3, - "open": 670, - "volume": 4915 - }, - "open": "2020-10-26T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 39944, - "high": 30204, - "low": 39944, - "open": 30204, - "volume": 400000 - }, - "id": 2754273, - "non_hive": { - "close": 5654, - "high": 4500, - "low": 5654, - "open": 4500, - "volume": 57983 - }, - "open": "2020-10-26T19:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 38712, - "high": 38712, - "low": 13664, - "open": 13664, - "volume": 87801 - }, - "id": 2754276, - "non_hive": { - "close": 5765, - "high": 5765, - "low": 1999, - "open": 1999, - "volume": 12987 - }, - "open": "2020-10-26T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 167, - "high": 237, - "low": 167, - "open": 237, - "volume": 22320 - }, - "id": 2754279, - "non_hive": { - "close": 24, - "high": 35, - "low": 24, - "open": 35, - "volume": 3277 - }, - "open": "2020-10-26T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 12966, - "high": 12966, - "low": 17656, - "open": 17656, - "volume": 30622 - }, - "id": 2754285, - "non_hive": { - "close": 1897, - "high": 1897, - "low": 2583, - "open": 2583, - "volume": 4480 - }, - "open": "2020-10-26T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 7119, - "high": 7119, - "low": 698, - "open": 698, - "volume": 7817 - }, - "id": 2754290, - "non_hive": { - "close": 1042, - "high": 1042, - "low": 102, - "open": 102, - "volume": 1144 - }, - "open": "2020-10-26T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1026, - "high": 5831, - "low": 7102, - "open": 7102, - "volume": 584977 - }, - "id": 2754294, - "non_hive": { - "close": 154, - "high": 880, - "low": 1043, - "open": 1043, - "volume": 87522 - }, - "open": "2020-10-26T20:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 69854, - "high": 69854, - "low": 1146, - "open": 1146, - "volume": 71000 - }, - "id": 2754302, - "non_hive": { - "close": 10541, - "high": 10541, - "low": 172, - "open": 172, - "volume": 10713 - }, - "open": "2020-10-26T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 4702, - "high": 4702, - "low": 2430, - "open": 7074, - "volume": 27776 - }, - "id": 2754305, - "non_hive": { - "close": 705, - "high": 705, - "low": 358, - "open": 1046, - "volume": 4109 - }, - "open": "2020-10-26T20:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 16358, - "high": 58259, - "low": 7000, - "open": 7000, - "volume": 97462 - }, - "id": 2754310, - "non_hive": { - "close": 2468, - "high": 8790, - "low": 1050, - "open": 1050, - "volume": 14695 - }, - "open": "2020-10-26T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12175, - "high": 12175, - "low": 12175, - "open": 12175, - "volume": 12175 - }, - "id": 2754316, - "non_hive": { - "close": 1837, - "high": 1837, - "low": 1837, - "open": 1837, - "volume": 1837 - }, - "open": "2020-10-26T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 3260, - "high": 3260, - "low": 12899, - "open": 12899, - "volume": 16159 - }, - "id": 2754319, - "non_hive": { - "close": 492, - "high": 492, - "low": 1946, - "open": 1946, - "volume": 2438 - }, - "open": "2020-10-26T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1716, - "high": 1716, - "low": 14845, - "open": 14845, - "volume": 16561 - }, - "id": 2754325, - "non_hive": { - "close": 259, - "high": 259, - "low": 2240, - "open": 2240, - "volume": 2499 - }, - "open": "2020-10-26T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6670, - "high": 13338, - "low": 6670, - "open": 13338, - "volume": 20008 - }, - "id": 2754330, - "non_hive": { - "close": 1000, - "high": 2000, - "low": 1000, - "open": 2000, - "volume": 3000 - }, - "open": "2020-10-26T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 18745, - "high": 18745, - "low": 13255, - "open": 13255, - "volume": 32000 - }, - "id": 2754335, - "non_hive": { - "close": 2827, - "high": 2827, - "low": 1999, - "open": 1999, - "volume": 4826 - }, - "open": "2020-10-26T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2300, - "high": 2300, - "low": 2300, - "open": 2300, - "volume": 2300 - }, - "id": 2754340, - "non_hive": { - "close": 347, - "high": 347, - "low": 347, - "open": 347, - "volume": 347 - }, - "open": "2020-10-26T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 9536, - "high": 9536, - "low": 74994, - "open": 74994, - "volume": 539638 - }, - "id": 2754343, - "non_hive": { - "close": 1439, - "high": 1439, - "low": 11309, - "open": 11309, - "volume": 81384 - }, - "open": "2020-10-26T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7630, - "high": 37881, - "low": 6670, - "open": 37881, - "volume": 717201 - }, - "id": 2754352, - "non_hive": { - "close": 1144, - "high": 5713, - "low": 1000, - "open": 5713, - "volume": 107600 - }, - "open": "2020-10-26T21:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 7235, - "high": 2387, - "low": 7235, - "open": 2387, - "volume": 163775 - }, - "id": 2754357, - "non_hive": { - "close": 1039, - "high": 358, - "low": 1039, - "open": 358, - "volume": 23994 - }, - "open": "2020-10-26T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 75996, - "high": 68997, - "low": 75996, - "open": 68997, - "volume": 229631 - }, - "id": 2754364, - "non_hive": { - "close": 10913, - "high": 9908, - "low": 10913, - "open": 9908, - "volume": 32975 - }, - "open": "2020-10-26T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 9916, - "high": 9916, - "low": 9916, - "open": 9916, - "volume": 9916 - }, - "id": 2754370, - "non_hive": { - "close": 1424, - "high": 1424, - "low": 1424, - "open": 1424, - "volume": 1424 - }, - "open": "2020-10-26T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 138997, - "high": 82994, - "low": 138997, - "open": 82994, - "volume": 221991 - }, - "id": 2754374, - "non_hive": { - "close": 19960, - "high": 11918, - "low": 19960, - "open": 11918, - "volume": 31878 - }, - "open": "2020-10-26T22:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13471, - "high": 13471, - "low": 13471, - "open": 13471, - "volume": 13471 - }, - "id": 2754378, - "non_hive": { - "close": 1946, - "high": 1946, - "low": 1946, - "open": 1946, - "volume": 1946 - }, - "open": "2020-10-26T22:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7117, - "high": 47, - "low": 7218, - "open": 7218, - "volume": 28764 - }, - "id": 2754381, - "non_hive": { - "close": 1038, - "high": 7, - "low": 1043, - "open": 1043, - "volume": 4176 - }, - "open": "2020-10-26T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 609994, - "high": 609994, - "low": 7147, - "open": 7147, - "volume": 757642 - }, - "id": 2754386, - "non_hive": { - "close": 91102, - "high": 91102, - "low": 1047, - "open": 1047, - "volume": 113074 - }, - "open": "2020-10-26T23:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13372, - "high": 13372, - "low": 13372, - "open": 13372, - "volume": 13372 - }, - "id": 2754390, - "non_hive": { - "close": 1997, - "high": 1997, - "low": 1997, - "open": 1997, - "volume": 1997 - }, - "open": "2020-10-26T23:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 301535, - "high": 301535, - "low": 301535, - "open": 301535, - "volume": 301535 - }, - "id": 2754393, - "non_hive": { - "close": 45030, - "high": 45030, - "low": 45030, - "open": 45030, - "volume": 45030 - }, - "open": "2020-10-26T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 41980, - "high": 13715, - "low": 41980, - "open": 13715, - "volume": 76374 - }, - "id": 2754396, - "non_hive": { - "close": 6186, - "high": 2048, - "low": 6186, - "open": 2048, - "volume": 11292 - }, - "open": "2020-10-26T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 10074, - "high": 13375, - "low": 10074, - "open": 13423, - "volume": 215477 - }, - "id": 2754399, - "non_hive": { - "close": 1472, - "high": 1993, - "low": 1472, - "open": 1999, - "volume": 31883 - }, - "open": "2020-10-26T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 7060, - "high": 7060, - "low": 7112, - "open": 7112, - "volume": 39432 - }, - "id": 2754406, - "non_hive": { - "close": 1051, - "high": 1051, - "low": 1049, - "open": 1049, - "volume": 5847 - }, - "open": "2020-10-27T00:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 14638, - "high": 92419, - "low": 2469, - "open": 75000, - "volume": 184526 - }, - "id": 2754411, - "non_hive": { - "close": 2185, - "high": 13801, - "low": 368, - "open": 11199, - "volume": 27553 - }, - "open": "2020-10-27T00:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 7102, - "high": 7102, - "low": 7102, - "open": 7102, - "volume": 7102 - }, - "id": 2754417, - "non_hive": { - "close": 1059, - "high": 1059, - "low": 1059, - "open": 1059, - "volume": 1059 - }, - "open": "2020-10-27T00:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4095, - "high": 4095, - "low": 4095, - "open": 4095, - "volume": 4095 - }, - "id": 2754420, - "non_hive": { - "close": 611, - "high": 611, - "low": 611, - "open": 611, - "volume": 611 - }, - "open": "2020-10-27T00:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 75000, - "high": 75000, - "low": 75000, - "open": 75000, - "volume": 75000 - }, - "id": 2754423, - "non_hive": { - "close": 11199, - "high": 11199, - "low": 11199, - "open": 11199, - "volume": 11199 - }, - "open": "2020-10-27T01:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 5363, - "high": 5363, - "low": 555820, - "open": 555820, - "volume": 561183 - }, - "id": 2754427, - "non_hive": { - "close": 801, - "high": 801, - "low": 83000, - "open": 83000, - "volume": 83801 - }, - "open": "2020-10-27T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 13433, - "high": 10125, - "low": 13433, - "open": 10125, - "volume": 50424 - }, - "id": 2754432, - "non_hive": { - "close": 2000, - "high": 1512, - "low": 2000, - "open": 1512, - "volume": 7512 - }, - "open": "2020-10-27T01:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1221, - "high": 5587, - "low": 7847, - "open": 13433, - "volume": 53827 - }, - "id": 2754440, - "non_hive": { - "close": 182, - "high": 834, - "low": 1168, - "open": 2000, - "volume": 8021 - }, - "open": "2020-10-27T01:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6709, - "high": 6709, - "low": 6709, - "open": 6709, - "volume": 6709 - }, - "id": 2754448, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-27T01:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 74999, - "high": 74999, - "low": 400, - "open": 400, - "volume": 75399 - }, - "id": 2754451, - "non_hive": { - "close": 11161, - "high": 11161, - "low": 59, - "open": 59, - "volume": 11220 - }, - "open": "2020-10-27T01:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 6817, - "high": 30821, - "low": 6817, - "open": 13401, - "volume": 72401 - }, - "id": 2754454, - "non_hive": { - "close": 997, - "high": 4600, - "low": 997, - "open": 1999, - "volume": 10744 - }, - "open": "2020-10-27T02:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 5484, - "high": 5484, - "low": 13560, - "open": 13560, - "volume": 19044 - }, - "id": 2754460, - "non_hive": { - "close": 809, - "high": 809, - "low": 1999, - "open": 1999, - "volume": 2808 - }, - "open": "2020-10-27T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1628, - "high": 1628, - "low": 1628, - "open": 1628, - "volume": 1628 - }, - "id": 2754463, - "non_hive": { - "close": 240, - "high": 240, - "low": 240, - "open": 240, - "volume": 240 - }, - "open": "2020-10-27T03:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 22723, - "high": 13556, - "low": 22723, - "open": 13556, - "volume": 68000 - }, - "id": 2754467, - "non_hive": { - "close": 3325, - "high": 2000, - "low": 3325, - "open": 2000, - "volume": 10004 - }, - "open": "2020-10-27T03:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 2543, - "high": 2543, - "low": 79021, - "open": 13649, - "volume": 102543 - }, - "id": 2754470, - "non_hive": { - "close": 375, - "high": 375, - "low": 11538, - "open": 1998, - "volume": 14984 - }, - "open": "2020-10-27T03:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 68, - "high": 47, - "low": 2453, - "open": 11017, - "volume": 990083 - }, - "id": 2754475, - "non_hive": { - "close": 10, - "high": 7, - "low": 358, - "open": 1624, - "volume": 145016 - }, - "open": "2020-10-27T03:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 13656, - "high": 11999, - "low": 13656, - "open": 11999, - "volume": 32791 - }, - "id": 2754485, - "non_hive": { - "close": 1999, - "high": 1763, - "low": 1999, - "open": 1763, - "volume": 4810 - }, - "open": "2020-10-27T03:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 17994, - "high": 17994, - "low": 17994, - "open": 17994, - "volume": 17994 - }, - "id": 2754488, - "non_hive": { - "close": 2634, - "high": 2634, - "low": 2634, - "open": 2634, - "volume": 2634 - }, - "open": "2020-10-27T04:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 205316, - "high": 6, - "low": 205316, - "open": 7160, - "volume": 500000 - }, - "id": 2754492, - "non_hive": { - "close": 29769, - "high": 1, - "low": 29769, - "open": 1048, - "volume": 72627 - }, - "open": "2020-10-27T04:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 11703, - "high": 11703, - "low": 49180, - "open": 11000, - "volume": 111703 - }, - "id": 2754495, - "non_hive": { - "close": 1702, - "high": 1702, - "low": 7087, - "open": 1595, - "volume": 16148 - }, - "open": "2020-10-27T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 989, - "high": 989, - "low": 13794, - "open": 13794, - "volume": 22011 - }, - "id": 2754500, - "non_hive": { - "close": 144, - "high": 144, - "low": 1999, - "open": 1999, - "volume": 3191 - }, - "open": "2020-10-27T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13227, - "high": 13227, - "low": 13227, - "open": 13227, - "volume": 13227 - }, - "id": 2754503, - "non_hive": { - "close": 1917, - "high": 1917, - "low": 1917, - "open": 1917, - "volume": 1917 - }, - "open": "2020-10-27T04:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 55716, - "high": 13877, - "low": 55716, - "open": 13877, - "volume": 69593 - }, - "id": 2754506, - "non_hive": { - "close": 8029, - "high": 2000, - "low": 8029, - "open": 2000, - "volume": 10029 - }, - "open": "2020-10-27T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 10432, - "high": 10432, - "low": 10432, - "open": 10432, - "volume": 10432 - }, - "id": 2754509, - "non_hive": { - "close": 1500, - "high": 1500, - "low": 1500, - "open": 1500, - "volume": 1500 - }, - "open": "2020-10-27T04:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 9295, - "high": 9295, - "low": 9295, - "open": 9295, - "volume": 9295 - }, - "id": 2754512, - "non_hive": { - "close": 1347, - "high": 1347, - "low": 1347, - "open": 1347, - "volume": 1347 - }, - "open": "2020-10-27T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 4014, - "high": 202503, - "low": 4014, - "open": 50000, - "volume": 300000 - }, - "id": 2754516, - "non_hive": { - "close": 577, - "high": 29340, - "low": 577, - "open": 7244, - "volume": 43461 - }, - "open": "2020-10-27T05:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 40000, - "high": 40000, - "low": 40000, - "open": 40000, - "volume": 40000 - }, - "id": 2754521, - "non_hive": { - "close": 5797, - "high": 5797, - "low": 5797, - "open": 5797, - "volume": 5797 - }, - "open": "2020-10-27T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 282, - "high": 282, - "low": 282, - "open": 282, - "volume": 282 - }, - "id": 2754524, - "non_hive": { - "close": 41, - "high": 41, - "low": 41, - "open": 41, - "volume": 41 - }, - "open": "2020-10-27T06:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13802, - "high": 3905, - "low": 13802, - "open": 23315, - "volume": 61655 - }, - "id": 2754528, - "non_hive": { - "close": 1999, - "high": 566, - "low": 1999, - "open": 3379, - "volume": 8933 - }, - "open": "2020-10-27T06:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6470, - "high": 6470, - "low": 6470, - "open": 6470, - "volume": 6470 - }, - "id": 2754534, - "non_hive": { - "close": 937, - "high": 937, - "low": 937, - "open": 937, - "volume": 937 - }, - "open": "2020-10-27T06:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 22012, - "high": 7673, - "low": 3359, - "open": 13812, - "volume": 143471 - }, - "id": 2754537, - "non_hive": { - "close": 3187, - "high": 1111, - "low": 486, - "open": 1999, - "volume": 20771 - }, - "open": "2020-10-27T06:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 86347, - "high": 86347, - "low": 86347, - "open": 86347, - "volume": 86347 - }, - "id": 2754543, - "non_hive": { - "close": 12500, - "high": 12500, - "low": 12500, - "open": 12500, - "volume": 12500 - }, - "open": "2020-10-27T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 849, - "high": 849, - "low": 849, - "open": 849, - "volume": 849 - }, - "id": 2754546, - "non_hive": { - "close": 123, - "high": 123, - "low": 123, - "open": 123, - "volume": 123 - }, - "open": "2020-10-27T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 53709, - "high": 46291, - "low": 53709, - "open": 46291, - "volume": 100000 - }, - "id": 2754550, - "non_hive": { - "close": 7734, - "high": 6689, - "low": 7734, - "open": 6689, - "volume": 14423 - }, - "open": "2020-10-27T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13830, - "high": 13830, - "low": 19, - "open": 10000, - "volume": 200498 - }, - "id": 2754553, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 2, - "open": 1440, - "volume": 28879 - }, - "open": "2020-10-27T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 85, - "high": 11310, - "low": 85, - "open": 11310, - "volume": 111218 - }, - "id": 2754562, - "non_hive": { - "close": 12, - "high": 1635, - "low": 12, - "open": 1635, - "volume": 16021 - }, - "open": "2020-10-27T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7799, - "high": 6, - "low": 7799, - "open": 13645, - "volume": 21450 - }, - "id": 2754573, - "non_hive": { - "close": 1119, - "high": 1, - "low": 1119, - "open": 1962, - "volume": 3082 - }, - "open": "2020-10-27T07:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6461, - "high": 2254, - "low": 41471, - "open": 2254, - "volume": 50186 - }, - "id": 2754578, - "non_hive": { - "close": 934, - "high": 326, - "low": 5995, - "open": 326, - "volume": 7255 - }, - "open": "2020-10-27T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 50000, - "high": 50000, - "low": 50000, - "open": 50000, - "volume": 50000 - }, - "id": 2754583, - "non_hive": { - "close": 7199, - "high": 7199, - "low": 7199, - "open": 7199, - "volume": 7199 - }, - "open": "2020-10-27T07:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 17981, - "high": 17981, - "low": 13813, - "open": 13813, - "volume": 31794 - }, - "id": 2754586, - "non_hive": { - "close": 2603, - "high": 2603, - "low": 1999, - "open": 1999, - "volume": 4602 - }, - "open": "2020-10-27T07:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13864, - "high": 19973, - "low": 13864, - "open": 19973, - "volume": 33837 - }, - "id": 2754589, - "non_hive": { - "close": 1999, - "high": 2880, - "low": 1999, - "open": 2880, - "volume": 4879 - }, - "open": "2020-10-27T08:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1506, - "high": 1506, - "low": 1506, - "open": 1506, - "volume": 1506 - }, - "id": 2754593, - "non_hive": { - "close": 217, - "high": 217, - "low": 217, - "open": 217, - "volume": 217 - }, - "open": "2020-10-27T08:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13879, - "high": 4892, - "low": 13879, - "open": 4892, - "volume": 145638 - }, - "id": 2754596, - "non_hive": { - "close": 1999, - "high": 705, - "low": 1999, - "open": 705, - "volume": 20985 - }, - "open": "2020-10-27T08:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13879, - "high": 13879, - "low": 2236, - "open": 13879, - "volume": 57800 - }, - "id": 2754605, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 321, - "open": 1999, - "volume": 8318 - }, - "open": "2020-10-27T08:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 36570, - "high": 23789, - "low": 211, - "open": 23789, - "volume": 60570 - }, - "id": 2754610, - "non_hive": { - "close": 5266, - "high": 3444, - "low": 30, - "open": 3444, - "volume": 8740 - }, - "open": "2020-10-27T08:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 4989, - "high": 4989, - "low": 4989, - "open": 4989, - "volume": 4989 - }, - "id": 2754615, - "non_hive": { - "close": 720, - "high": 720, - "low": 720, - "open": 720, - "volume": 720 - }, - "open": "2020-10-27T08:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 75986, - "high": 75986, - "low": 75986, - "open": 75986, - "volume": 75986 - }, - "id": 2754618, - "non_hive": { - "close": 10967, - "high": 10967, - "low": 10967, - "open": 10967, - "volume": 10967 - }, - "open": "2020-10-27T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 38920, - "high": 38920, - "low": 14, - "open": 14, - "volume": 39322 - }, - "id": 2754621, - "non_hive": { - "close": 5635, - "high": 5635, - "low": 2, - "open": 2, - "volume": 5693 - }, - "open": "2020-10-27T08:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 58204, - "high": 58204, - "low": 58204, - "open": 58204, - "volume": 58204 - }, - "id": 2754624, - "non_hive": { - "close": 8427, - "high": 8427, - "low": 8427, - "open": 8427, - "volume": 8427 - }, - "open": "2020-10-27T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 11445, - "high": 16390, - "low": 11445, - "open": 16390, - "volume": 47865 - }, - "id": 2754628, - "non_hive": { - "close": 1657, - "high": 2373, - "low": 1657, - "open": 2373, - "volume": 6930 - }, - "open": "2020-10-27T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 207008, - "high": 207008, - "low": 207008, - "open": 207008, - "volume": 207008 - }, - "id": 2754631, - "non_hive": { - "close": 29970, - "high": 29970, - "low": 29970, - "open": 29970, - "volume": 29970 - }, - "open": "2020-10-27T09:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 64989, - "high": 64989, - "low": 14, - "open": 14, - "volume": 65003 - }, - "id": 2754634, - "non_hive": { - "close": 9410, - "high": 9410, - "low": 2, - "open": 2, - "volume": 9412 - }, - "open": "2020-10-27T09:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 4435, - "high": 16604, - "low": 6694, - "open": 6694, - "volume": 39427 - }, - "id": 2754637, - "non_hive": { - "close": 642, - "high": 2404, - "low": 969, - "open": 969, - "volume": 5708 - }, - "open": "2020-10-27T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 5430, - "high": 5430, - "low": 5430, - "open": 5430, - "volume": 5430 - }, - "id": 2754641, - "non_hive": { - "close": 786, - "high": 786, - "low": 786, - "open": 786, - "volume": 786 - }, - "open": "2020-10-27T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 353, - "high": 353, - "low": 353, - "open": 353, - "volume": 353 - }, - "id": 2754645, - "non_hive": { - "close": 51, - "high": 51, - "low": 51, - "open": 51, - "volume": 51 - }, - "open": "2020-10-27T10:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6075, - "high": 13925, - "low": 6075, - "open": 13925, - "volume": 20000 - }, - "id": 2754648, - "non_hive": { - "close": 872, - "high": 2000, - "low": 872, - "open": 2000, - "volume": 2872 - }, - "open": "2020-10-27T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 2678, - "high": 13925, - "low": 2678, - "open": 13925, - "volume": 33530 - }, - "id": 2754651, - "non_hive": { - "close": 384, - "high": 2000, - "low": 384, - "open": 2000, - "volume": 4815 - }, - "open": "2020-10-27T10:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 559619, - "high": 2929, - "low": 6628, - "open": 6628, - "volume": 582994 - }, - "id": 2754654, - "non_hive": { - "close": 81001, - "high": 424, - "low": 954, - "open": 954, - "volume": 84378 - }, - "open": "2020-10-27T10:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 12484, - "high": 1623, - "low": 12484, - "open": 114030, - "volume": 593159 - }, - "id": 2754659, - "non_hive": { - "close": 1806, - "high": 235, - "low": 1806, - "open": 16505, - "volume": 85861 - }, - "open": "2020-10-27T10:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 55713, - "high": 13926, - "low": 55713, - "open": 13926, - "volume": 69639 - }, - "id": 2754668, - "non_hive": { - "close": 8000, - "high": 2000, - "low": 8000, - "open": 2000, - "volume": 10000 - }, - "open": "2020-10-27T10:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6498, - "high": 6498, - "low": 6498, - "open": 6498, - "volume": 6498 - }, - "id": 2754671, - "non_hive": { - "close": 937, - "high": 937, - "low": 937, - "open": 937, - "volume": 937 - }, - "open": "2020-10-27T11:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13867, - "high": 2974, - "low": 6498, - "open": 6498, - "volume": 43196 - }, - "id": 2754675, - "non_hive": { - "close": 2000, - "high": 429, - "low": 937, - "open": 937, - "volume": 6230 - }, - "open": "2020-10-27T11:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 9365, - "high": 2339, - "low": 9365, - "open": 13927, - "volume": 25631 - }, - "id": 2754680, - "non_hive": { - "close": 1344, - "high": 336, - "low": 1344, - "open": 2000, - "volume": 3680 - }, - "open": "2020-10-27T11:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13, - "high": 13, - "low": 15225, - "open": 15225, - "volume": 15238 - }, - "id": 2754683, - "non_hive": { - "close": 2, - "high": 2, - "low": 2200, - "open": 2200, - "volume": 2202 - }, - "open": "2020-10-27T11:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 17338, - "high": 17338, - "low": 17338, - "open": 17338, - "volume": 17338 - }, - "id": 2754688, - "non_hive": { - "close": 2500, - "high": 2500, - "low": 2500, - "open": 2500, - "volume": 2500 - }, - "open": "2020-10-27T11:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 4560, - "high": 104, - "low": 4560, - "open": 104, - "volume": 22878 - }, - "id": 2754691, - "non_hive": { - "close": 654, - "high": 15, - "low": 654, - "open": 15, - "volume": 3285 - }, - "open": "2020-10-27T12:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 10806, - "high": 3194, - "low": 10806, - "open": 3194, - "volume": 14000 - }, - "id": 2754697, - "non_hive": { - "close": 1550, - "high": 460, - "low": 1550, - "open": 460, - "volume": 2010 - }, - "open": "2020-10-27T12:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 271, - "high": 271, - "low": 271, - "open": 271, - "volume": 271 - }, - "id": 2754700, - "non_hive": { - "close": 39, - "high": 39, - "low": 39, - "open": 39, - "volume": 39 - }, - "open": "2020-10-27T12:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 719113, - "high": 719113, - "low": 60273, - "open": 60273, - "volume": 779386 - }, - "id": 2754703, - "non_hive": { - "close": 104117, - "high": 104117, - "low": 8726, - "open": 8726, - "volume": 112843 - }, - "open": "2020-10-27T12:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 691, - "high": 691, - "low": 13838, - "open": 13838, - "volume": 14529 - }, - "id": 2754706, - "non_hive": { - "close": 100, - "high": 100, - "low": 1999, - "open": 1999, - "volume": 2099 - }, - "open": "2020-10-27T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16523, - "high": 2575, - "low": 16523, - "open": 20747, - "volume": 74481 - }, - "id": 2754709, - "non_hive": { - "close": 2386, - "high": 372, - "low": 2386, - "open": 2997, - "volume": 10758 - }, - "open": "2020-10-27T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 65079, - "high": 65079, - "low": 13849, - "open": 13849, - "volume": 82973 - }, - "id": 2754715, - "non_hive": { - "close": 9417, - "high": 9417, - "low": 1999, - "open": 1999, - "volume": 12000 - }, - "open": "2020-10-27T12:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 55606, - "high": 9101, - "low": 6635, - "open": 13822, - "volume": 98987 - }, - "id": 2754718, - "non_hive": { - "close": 8042, - "high": 1317, - "low": 959, - "open": 1999, - "volume": 14316 - }, - "open": "2020-10-27T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 175000, - "high": 175000, - "low": 13823, - "open": 13823, - "volume": 188823 - }, - "id": 2754725, - "non_hive": { - "close": 25320, - "high": 25320, - "low": 1999, - "open": 1999, - "volume": 27319 - }, - "open": "2020-10-27T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 14051, - "high": 2640, - "low": 24973, - "open": 24973, - "volume": 41664 - }, - "id": 2754730, - "non_hive": { - "close": 2033, - "high": 382, - "low": 3613, - "open": 3613, - "volume": 6028 - }, - "open": "2020-10-27T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 973, - "high": 25241, - "low": 973, - "open": 25241, - "volume": 32318 - }, - "id": 2754735, - "non_hive": { - "close": 140, - "high": 3652, - "low": 140, - "open": 3652, - "volume": 4675 - }, - "open": "2020-10-27T13:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 2412, - "high": 2412, - "low": 2412, - "open": 2412, - "volume": 2412 - }, - "id": 2754740, - "non_hive": { - "close": 349, - "high": 349, - "low": 349, - "open": 349, - "volume": 349 - }, - "open": "2020-10-27T13:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 1500, - "high": 41209, - "low": 1500, - "open": 41209, - "volume": 42709 - }, - "id": 2754743, - "non_hive": { - "close": 217, - "high": 5962, - "low": 217, - "open": 5962, - "volume": 6179 - }, - "open": "2020-10-27T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 436964, - "high": 436964, - "low": 29638, - "open": 29638, - "volume": 466602 - }, - "id": 2754746, - "non_hive": { - "close": 63228, - "high": 63228, - "low": 4287, - "open": 4287, - "volume": 67515 - }, - "open": "2020-10-27T13:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13124, - "high": 13124, - "low": 13124, - "open": 13124, - "volume": 13124 - }, - "id": 2754749, - "non_hive": { - "close": 1899, - "high": 1899, - "low": 1899, - "open": 1899, - "volume": 1899 - }, - "open": "2020-10-27T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 56704, - "high": 56704, - "low": 56704, - "open": 56704, - "volume": 56704 - }, - "id": 2754753, - "non_hive": { - "close": 8205, - "high": 8205, - "low": 8205, - "open": 8205, - "volume": 8205 - }, - "open": "2020-10-27T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 4146, - "high": 4146, - "low": 6663, - "open": 6663, - "volume": 10809 - }, - "id": 2754756, - "non_hive": { - "close": 600, - "high": 600, - "low": 964, - "open": 964, - "volume": 1564 - }, - "open": "2020-10-27T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 2131, - "high": 6911, - "low": 2131, - "open": 6911, - "volume": 34427 - }, - "id": 2754759, - "non_hive": { - "close": 308, - "high": 1000, - "low": 308, - "open": 1000, - "volume": 4979 - }, - "open": "2020-10-27T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 7005, - "high": 7005, - "low": 7005, - "open": 7005, - "volume": 7005 - }, - "id": 2754764, - "non_hive": { - "close": 1013, - "high": 1013, - "low": 1013, - "open": 1013, - "volume": 1013 - }, - "open": "2020-10-27T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6654, - "high": 8000, - "low": 6654, - "open": 8000, - "volume": 35483 - }, - "id": 2754767, - "non_hive": { - "close": 962, - "high": 1157, - "low": 962, - "open": 1157, - "volume": 5131 - }, - "open": "2020-10-27T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 14003, - "high": 14003, - "low": 14003, - "open": 14003, - "volume": 14003 - }, - "id": 2754773, - "non_hive": { - "close": 2025, - "high": 2025, - "low": 2025, - "open": 2025, - "volume": 2025 - }, - "open": "2020-10-27T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 5691, - "high": 5691, - "low": 5691, - "open": 5691, - "volume": 5691 - }, - "id": 2754777, - "non_hive": { - "close": 823, - "high": 823, - "low": 823, - "open": 823, - "volume": 823 - }, - "open": "2020-10-27T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 83, - "high": 262, - "low": 83, - "open": 18582, - "volume": 40290 - }, - "id": 2754780, - "non_hive": { - "close": 12, - "high": 38, - "low": 12, - "open": 2687, - "volume": 5826 - }, - "open": "2020-10-27T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6577, - "high": 470, - "low": 533, - "open": 533, - "volume": 7580 - }, - "id": 2754785, - "non_hive": { - "close": 951, - "high": 68, - "low": 77, - "open": 77, - "volume": 1096 - }, - "open": "2020-10-27T15:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1473, - "high": 1189, - "low": 1473, - "open": 1189, - "volume": 2662 - }, - "id": 2754790, - "non_hive": { - "close": 213, - "high": 172, - "low": 213, - "open": 172, - "volume": 385 - }, - "open": "2020-10-27T15:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 4214, - "high": 4214, - "low": 4214, - "open": 4214, - "volume": 4214 - }, - "id": 2754795, - "non_hive": { - "close": 609, - "high": 609, - "low": 609, - "open": 609, - "volume": 609 - }, - "open": "2020-10-27T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 103385, - "high": 103385, - "low": 50001, - "open": 50001, - "volume": 153386 - }, - "id": 2754798, - "non_hive": { - "close": 14950, - "high": 14950, - "low": 7228, - "open": 7228, - "volume": 22178 - }, - "open": "2020-10-27T15:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 23128, - "high": 145, - "low": 23128, - "open": 10126, - "volume": 61097 - }, - "id": 2754801, - "non_hive": { - "close": 3318, - "high": 21, - "low": 3318, - "open": 1463, - "volume": 8789 - }, - "open": "2020-10-27T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 75000, - "high": 75000, - "low": 6619, - "open": 6619, - "volume": 81619 - }, - "id": 2754806, - "non_hive": { - "close": 10817, - "high": 10817, - "low": 954, - "open": 954, - "volume": 11771 - }, - "open": "2020-10-27T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 75000, - "high": 75000, - "low": 75000, - "open": 75000, - "volume": 75000 - }, - "id": 2754812, - "non_hive": { - "close": 10817, - "high": 10817, - "low": 10817, - "open": 10817, - "volume": 10817 - }, - "open": "2020-10-27T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6612, - "high": 30666, - "low": 6612, - "open": 30666, - "volume": 58080 - }, - "id": 2754815, - "non_hive": { - "close": 953, - "high": 4423, - "low": 953, - "open": 4423, - "volume": 8375 - }, - "open": "2020-10-27T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2157, - "high": 2157, - "low": 2157, - "open": 2157, - "volume": 2157 - }, - "id": 2754821, - "non_hive": { - "close": 311, - "high": 311, - "low": 311, - "open": 311, - "volume": 311 - }, - "open": "2020-10-27T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 26635, - "high": 26635, - "low": 26635, - "open": 26635, - "volume": 26635 - }, - "id": 2754824, - "non_hive": { - "close": 3838, - "high": 3838, - "low": 3838, - "open": 3838, - "volume": 3838 - }, - "open": "2020-10-27T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 15309, - "high": 4441, - "low": 13027, - "open": 29023, - "volume": 114380 - }, - "id": 2754827, - "non_hive": { - "close": 2206, - "high": 640, - "low": 1869, - "open": 4182, - "volume": 16469 - }, - "open": "2020-10-27T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 20854, - "high": 11005, - "low": 20854, - "open": 11005, - "volume": 31859 - }, - "id": 2754835, - "non_hive": { - "close": 3007, - "high": 1587, - "low": 3007, - "open": 1587, - "volume": 4594 - }, - "open": "2020-10-27T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 5377, - "high": 5377, - "low": 5377, - "open": 5377, - "volume": 5377 - }, - "id": 2754840, - "non_hive": { - "close": 774, - "high": 774, - "low": 774, - "open": 774, - "volume": 774 - }, - "open": "2020-10-27T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 60526, - "high": 14474, - "low": 60526, - "open": 14474, - "volume": 75000 - }, - "id": 2754843, - "non_hive": { - "close": 8685, - "high": 2087, - "low": 8685, - "open": 2087, - "volume": 10772 - }, - "open": "2020-10-27T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 47357, - "high": 27185, - "low": 47357, - "open": 61000, - "volume": 168563 - }, - "id": 2754846, - "non_hive": { - "close": 6776, - "high": 3920, - "low": 6776, - "open": 8783, - "volume": 24220 - }, - "open": "2020-10-27T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 42027, - "high": 13973, - "low": 42027, - "open": 13974, - "volume": 181500 - }, - "id": 2754853, - "non_hive": { - "close": 6014, - "high": 2000, - "low": 6014, - "open": 2000, - "volume": 25974 - }, - "open": "2020-10-27T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13870, - "high": 82248, - "low": 36428, - "open": 13870, - "volume": 284539 - }, - "id": 2754858, - "non_hive": { - "close": 1999, - "high": 11860, - "low": 5212, - "open": 1999, - "volume": 40956 - }, - "open": "2020-10-27T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6976, - "high": 6976, - "low": 5378, - "open": 5378, - "volume": 612354 - }, - "id": 2754866, - "non_hive": { - "close": 1006, - "high": 1006, - "low": 775, - "open": 775, - "volume": 88299 - }, - "open": "2020-10-27T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 60981, - "high": 34, - "low": 60981, - "open": 34, - "volume": 75000 - }, - "id": 2754873, - "non_hive": { - "close": 8720, - "high": 5, - "low": 8720, - "open": 5, - "volume": 10725 - }, - "open": "2020-10-27T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 36681, - "high": 5559, - "low": 36681, - "open": 5559, - "volume": 155559 - }, - "id": 2754876, - "non_hive": { - "close": 5245, - "high": 801, - "low": 5245, - "open": 801, - "volume": 22251 - }, - "open": "2020-10-27T17:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 69403, - "high": 3143, - "low": 13908, - "open": 13908, - "volume": 89771 - }, - "id": 2754882, - "non_hive": { - "close": 10000, - "high": 453, - "low": 1999, - "open": 1999, - "volume": 12929 - }, - "open": "2020-10-27T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 29386, - "high": 4889, - "low": 29386, - "open": 4889, - "volume": 223000 - }, - "id": 2754887, - "non_hive": { - "close": 4190, - "high": 704, - "low": 4190, - "open": 704, - "volume": 31880 - }, - "open": "2020-10-27T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 12603, - "high": 382, - "low": 13900, - "open": 2787, - "volume": 49702 - }, - "id": 2754893, - "non_hive": { - "close": 1813, - "high": 55, - "low": 1999, - "open": 401, - "volume": 7150 - }, - "open": "2020-10-27T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 23042, - "high": 23042, - "low": 207, - "open": 33259, - "volume": 119402 - }, - "id": 2754902, - "non_hive": { - "close": 3320, - "high": 3320, - "low": 29, - "open": 4784, - "volume": 17130 - }, - "open": "2020-10-27T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7802, - "high": 3619, - "low": 22884, - "open": 13985, - "volume": 78421 - }, - "id": 2754909, - "non_hive": { - "close": 1123, - "high": 521, - "low": 3263, - "open": 2000, - "volume": 11207 - }, - "open": "2020-10-27T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 329848, - "high": 329848, - "low": 46750, - "open": 2468, - "volume": 551615 - }, - "id": 2754915, - "non_hive": { - "close": 47526, - "high": 47526, - "low": 6666, - "open": 355, - "volume": 79384 - }, - "open": "2020-10-27T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 37853, - "high": 37853, - "low": 80243, - "open": 80243, - "volume": 118096 - }, - "id": 2754923, - "non_hive": { - "close": 5454, - "high": 5454, - "low": 11561, - "open": 11561, - "volume": 17015 - }, - "open": "2020-10-27T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 60875, - "high": 16248, - "low": 177877, - "open": 16248, - "volume": 332000 - }, - "id": 2754928, - "non_hive": { - "close": 8618, - "high": 2341, - "low": 25180, - "open": 2341, - "volume": 47130 - }, - "open": "2020-10-27T17:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1263, - "high": 1263, - "low": 1263, - "open": 1263, - "volume": 1263 - }, - "id": 2754934, - "non_hive": { - "close": 181, - "high": 181, - "low": 181, - "open": 181, - "volume": 181 - }, - "open": "2020-10-27T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 5080, - "high": 5080, - "low": 53752, - "open": 5276, - "volume": 80080 - }, - "id": 2754938, - "non_hive": { - "close": 726, - "high": 726, - "low": 7610, - "open": 754, - "volume": 11354 - }, - "open": "2020-10-27T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 60874, - "high": 14126, - "low": 60874, - "open": 14126, - "volume": 75000 - }, - "id": 2754943, - "non_hive": { - "close": 8618, - "high": 2000, - "low": 8618, - "open": 2000, - "volume": 10618 - }, - "open": "2020-10-27T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 20820, - "high": 330, - "low": 20820, - "open": 330, - "volume": 170820 - }, - "id": 2754946, - "non_hive": { - "close": 2947, - "high": 47, - "low": 2947, - "open": 47, - "volume": 24181 - }, - "open": "2020-10-27T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 4551, - "high": 4551, - "low": 4551, - "open": 4551, - "volume": 4551 - }, - "id": 2754952, - "non_hive": { - "close": 650, - "high": 650, - "low": 650, - "open": 650, - "volume": 650 - }, - "open": "2020-10-27T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 18491, - "high": 8163, - "low": 14003, - "open": 14003, - "volume": 81266 - }, - "id": 2754955, - "non_hive": { - "close": 2641, - "high": 1166, - "low": 1999, - "open": 1999, - "volume": 11606 - }, - "open": "2020-10-27T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 16007, - "high": 5203, - "low": 16007, - "open": 5203, - "volume": 21210 - }, - "id": 2754961, - "non_hive": { - "close": 2265, - "high": 743, - "low": 2265, - "open": 743, - "volume": 3008 - }, - "open": "2020-10-27T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 14004, - "high": 14004, - "low": 14004, - "open": 14004, - "volume": 14004 - }, - "id": 2754964, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-27T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 90779, - "high": 2430, - "low": 90779, - "open": 2430, - "volume": 150414 - }, - "id": 2754967, - "non_hive": { - "close": 12850, - "high": 347, - "low": 12850, - "open": 347, - "volume": 21339 - }, - "open": "2020-10-27T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 290472, - "high": 49, - "low": 14004, - "open": 14004, - "volume": 406093 - }, - "id": 2754970, - "non_hive": { - "close": 41487, - "high": 7, - "low": 1999, - "open": 1999, - "volume": 57999 - }, - "open": "2020-10-27T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 60000, - "high": 60000, - "low": 75000, - "open": 75000, - "volume": 135000 - }, - "id": 2754976, - "non_hive": { - "close": 8569, - "high": 8569, - "low": 10711, - "open": 10711, - "volume": 19280 - }, - "open": "2020-10-27T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 75000, - "high": 75000, - "low": 75000, - "open": 75000, - "volume": 75000 - }, - "id": 2754981, - "non_hive": { - "close": 10711, - "high": 10711, - "low": 10711, - "open": 10711, - "volume": 10711 - }, - "open": "2020-10-27T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 14, - "high": 14, - "low": 14, - "open": 14, - "volume": 14 - }, - "id": 2754984, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-10-27T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 63000, - "high": 63000, - "low": 63000, - "open": 63000, - "volume": 63000 - }, - "id": 2754987, - "non_hive": { - "close": 8998, - "high": 8998, - "low": 8998, - "open": 8998, - "volume": 8998 - }, - "open": "2020-10-27T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12140, - "high": 12140, - "low": 40454, - "open": 40454, - "volume": 52594 - }, - "id": 2754990, - "non_hive": { - "close": 1734, - "high": 1734, - "low": 5778, - "open": 5778, - "volume": 7512 - }, - "open": "2020-10-27T20:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 25819, - "high": 2044, - "low": 25819, - "open": 2044, - "volume": 76603 - }, - "id": 2754996, - "non_hive": { - "close": 3685, - "high": 292, - "low": 3685, - "open": 292, - "volume": 10938 - }, - "open": "2020-10-27T20:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 477000, - "high": 18004, - "low": 477000, - "open": 18004, - "volume": 495004 - }, - "id": 2755003, - "non_hive": { - "close": 68087, - "high": 2570, - "low": 68087, - "open": 2570, - "volume": 70657 - }, - "open": "2020-10-27T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 5478, - "high": 952, - "low": 989, - "open": 952, - "volume": 23049 - }, - "id": 2755006, - "non_hive": { - "close": 782, - "high": 136, - "low": 140, - "open": 136, - "volume": 3288 - }, - "open": "2020-10-27T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 14011, - "high": 14011, - "low": 14011, - "open": 14011, - "volume": 14011 - }, - "id": 2755013, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-27T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 11006, - "high": 11006, - "low": 11006, - "open": 11006, - "volume": 11006 - }, - "id": 2755016, - "non_hive": { - "close": 1571, - "high": 1571, - "low": 1571, - "open": 1571, - "volume": 1571 - }, - "open": "2020-10-27T20:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 12905, - "high": 6158, - "low": 12905, - "open": 6158, - "volume": 57000 - }, - "id": 2755019, - "non_hive": { - "close": 1826, - "high": 879, - "low": 1826, - "open": 879, - "volume": 8120 - }, - "open": "2020-10-27T20:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 59000, - "high": 59000, - "low": 59000, - "open": 59000, - "volume": 59000 - }, - "id": 2755022, - "non_hive": { - "close": 8417, - "high": 8417, - "low": 8417, - "open": 8417, - "volume": 8417 - }, - "open": "2020-10-27T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1485, - "high": 1485, - "low": 1485, - "open": 1485, - "volume": 1485 - }, - "id": 2755026, - "non_hive": { - "close": 212, - "high": 212, - "low": 212, - "open": 212, - "volume": 212 - }, - "open": "2020-10-27T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 5699, - "high": 5699, - "low": 37027, - "open": 37027, - "volume": 42726 - }, - "id": 2755029, - "non_hive": { - "close": 815, - "high": 815, - "low": 5285, - "open": 5285, - "volume": 6100 - }, - "open": "2020-10-27T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 202584, - "high": 202584, - "low": 40980, - "open": 4378, - "volume": 382689 - }, - "id": 2755032, - "non_hive": { - "close": 29211, - "high": 29211, - "low": 5824, - "open": 626, - "volume": 54871 - }, - "open": "2020-10-27T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 31888, - "high": 13985, - "low": 31888, - "open": 13985, - "volume": 75000 - }, - "id": 2755039, - "non_hive": { - "close": 4514, - "high": 2000, - "low": 4514, - "open": 2000, - "volume": 10660 - }, - "open": "2020-10-27T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 60873, - "high": 3773, - "low": 28505, - "open": 7282, - "volume": 144282 - }, - "id": 2755042, - "non_hive": { - "close": 8617, - "high": 543, - "low": 4035, - "open": 1047, - "volume": 20450 - }, - "open": "2020-10-27T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 21, - "high": 7118, - "low": 21, - "open": 196, - "volume": 89447 - }, - "id": 2755048, - "non_hive": { - "close": 2, - "high": 1020, - "low": 2, - "open": 28, - "volume": 12688 - }, - "open": "2020-10-27T22:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 472121, - "high": 942, - "low": 55016, - "open": 12625, - "volume": 780167 - }, - "id": 2755056, - "non_hive": { - "close": 66830, - "high": 135, - "low": 7787, - "open": 1809, - "volume": 110461 - }, - "open": "2020-10-27T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 7138, - "high": 7138, - "low": 13987, - "open": 13987, - "volume": 35715 - }, - "id": 2755063, - "non_hive": { - "close": 1028, - "high": 1028, - "low": 1999, - "open": 1999, - "volume": 5116 - }, - "open": "2020-10-27T22:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7315, - "high": 13978, - "low": 7315, - "open": 13978, - "volume": 21293 - }, - "id": 2755066, - "non_hive": { - "close": 1046, - "high": 1999, - "low": 1046, - "open": 1999, - "volume": 3045 - }, - "open": "2020-10-27T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 5914, - "high": 5914, - "low": 7340, - "open": 7340, - "volume": 27204 - }, - "id": 2755069, - "non_hive": { - "close": 848, - "high": 848, - "low": 1045, - "open": 1045, - "volume": 3892 - }, - "open": "2020-10-27T22:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 12279, - "high": 12279, - "low": 7333, - "open": 20990, - "volume": 54641 - }, - "id": 2755074, - "non_hive": { - "close": 1755, - "high": 1755, - "low": 1044, - "open": 3000, - "volume": 7798 - }, - "open": "2020-10-27T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 75000, - "high": 75000, - "low": 75000, - "open": 75000, - "volume": 75000 - }, - "id": 2755081, - "non_hive": { - "close": 10649, - "high": 10649, - "low": 10649, - "open": 10649, - "volume": 10649 - }, - "open": "2020-10-27T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 726164, - "high": 726164, - "low": 126, - "open": 1368, - "volume": 1651822 - }, - "id": 2755084, - "non_hive": { - "close": 104710, - "high": 104710, - "low": 18, - "open": 196, - "volume": 238062 - }, - "open": "2020-10-27T22:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 14027, - "high": 14027, - "low": 14027, - "open": 14027, - "volume": 14027 - }, - "id": 2755087, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-27T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 11448, - "high": 11448, - "low": 26662, - "open": 22803, - "volume": 86448 - }, - "id": 2755091, - "non_hive": { - "close": 1636, - "high": 1636, - "low": 3774, - "open": 3238, - "volume": 12271 - }, - "open": "2020-10-27T23:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 75000, - "high": 15069, - "low": 45931, - "open": 15069, - "volume": 136000 - }, - "id": 2755096, - "non_hive": { - "close": 10616, - "high": 2133, - "low": 6501, - "open": 2133, - "volume": 19250 - }, - "open": "2020-10-27T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 66000, - "high": 7308, - "low": 14004, - "open": 14004, - "volume": 173312 - }, - "id": 2755101, - "non_hive": { - "close": 9437, - "high": 1045, - "low": 1999, - "open": 1999, - "volume": 24776 - }, - "open": "2020-10-27T23:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 49492, - "high": 14027, - "low": 49492, - "open": 14027, - "volume": 75000 - }, - "id": 2755108, - "non_hive": { - "close": 7028, - "high": 1999, - "low": 7028, - "open": 1999, - "volume": 10663 - }, - "open": "2020-10-27T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 75000, - "high": 75000, - "low": 75000, - "open": 75000, - "volume": 150000 - }, - "id": 2755111, - "non_hive": { - "close": 10651, - "high": 10651, - "low": 10650, - "open": 10650, - "volume": 21301 - }, - "open": "2020-10-27T23:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 75000, - "high": 75000, - "low": 75000, - "open": 75000, - "volume": 75000 - }, - "id": 2755116, - "non_hive": { - "close": 10651, - "high": 10651, - "low": 10651, - "open": 10651, - "volume": 10651 - }, - "open": "2020-10-27T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 670667, - "high": 12667, - "low": 670667, - "open": 12667, - "volume": 1000000 - }, - "id": 2755119, - "non_hive": { - "close": 94772, - "high": 1815, - "low": 94772, - "open": 1815, - "volume": 141545 - }, - "open": "2020-10-27T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 4741, - "high": 4741, - "low": 14048, - "open": 14048, - "volume": 18789 - }, - "id": 2755122, - "non_hive": { - "close": 675, - "high": 675, - "low": 1999, - "open": 1999, - "volume": 2674 - }, - "open": "2020-10-28T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 74995, - "high": 74995, - "low": 74995, - "open": 74995, - "volume": 74995 - }, - "id": 2755129, - "non_hive": { - "close": 10676, - "high": 10676, - "low": 10676, - "open": 10676, - "volume": 10676 - }, - "open": "2020-10-28T00:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7363, - "high": 30371, - "low": 7363, - "open": 30371, - "volume": 62084 - }, - "id": 2755132, - "non_hive": { - "close": 1040, - "high": 4324, - "low": 1040, - "open": 4324, - "volume": 8829 - }, - "open": "2020-10-28T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 11216, - "high": 11216, - "low": 2585, - "open": 2585, - "volume": 13801 - }, - "id": 2755135, - "non_hive": { - "close": 1603, - "high": 1603, - "low": 368, - "open": 368, - "volume": 1971 - }, - "open": "2020-10-28T00:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 6113, - "high": 6113, - "low": 6113, - "open": 6113, - "volume": 6113 - }, - "id": 2755138, - "non_hive": { - "close": 870, - "high": 870, - "low": 870, - "open": 870, - "volume": 870 - }, - "open": "2020-10-28T00:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 7935, - "high": 7935, - "low": 7935, - "open": 7935, - "volume": 7935 - }, - "id": 2755141, - "non_hive": { - "close": 1129, - "high": 1129, - "low": 1129, - "open": 1129, - "volume": 1129 - }, - "open": "2020-10-28T01:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 344, - "high": 344, - "low": 344, - "open": 344, - "volume": 344 - }, - "id": 2755145, - "non_hive": { - "close": 49, - "high": 49, - "low": 49, - "open": 49, - "volume": 49 - }, - "open": "2020-10-28T01:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 5175, - "high": 5175, - "low": 6982, - "open": 6982, - "volume": 19458 - }, - "id": 2755148, - "non_hive": { - "close": 742, - "high": 742, - "low": 994, - "open": 994, - "volume": 2780 - }, - "open": "2020-10-28T02:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 7271, - "high": 7271, - "low": 2107, - "open": 2107, - "volume": 23267 - }, - "id": 2755152, - "non_hive": { - "close": 1047, - "high": 1047, - "low": 302, - "open": 302, - "volume": 3348 - }, - "open": "2020-10-28T02:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4424, - "high": 4424, - "low": 4424, - "open": 4424, - "volume": 4424 - }, - "id": 2755155, - "non_hive": { - "close": 638, - "high": 638, - "low": 638, - "open": 638, - "volume": 638 - }, - "open": "2020-10-28T02:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13191, - "high": 13191, - "low": 13191, - "open": 13191, - "volume": 13191 - }, - "id": 2755158, - "non_hive": { - "close": 1901, - "high": 1901, - "low": 1901, - "open": 1901, - "volume": 1901 - }, - "open": "2020-10-28T02:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13978, - "high": 43393, - "low": 13978, - "open": 43393, - "volume": 63572 - }, - "id": 2755161, - "non_hive": { - "close": 1998, - "high": 6239, - "low": 1998, - "open": 6239, - "volume": 9124 - }, - "open": "2020-10-28T02:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 22656, - "high": 22656, - "low": 5475, - "open": 5475, - "volume": 28131 - }, - "id": 2755164, - "non_hive": { - "close": 3265, - "high": 3265, - "low": 789, - "open": 789, - "volume": 4054 - }, - "open": "2020-10-28T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1551, - "high": 1551, - "low": 1551, - "open": 1551, - "volume": 1551 - }, - "id": 2755167, - "non_hive": { - "close": 223, - "high": 223, - "low": 223, - "open": 223, - "volume": 223 - }, - "open": "2020-10-28T02:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 10869, - "high": 118, - "low": 598, - "open": 2775, - "volume": 215058 - }, - "id": 2755170, - "non_hive": { - "close": 1545, - "high": 17, - "low": 85, - "open": 399, - "volume": 30631 - }, - "open": "2020-10-28T02:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13958, - "high": 13958, - "low": 13958, - "open": 13958, - "volume": 13958 - }, - "id": 2755182, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-28T03:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6340, - "high": 6340, - "low": 6340, - "open": 6340, - "volume": 6340 - }, - "id": 2755186, - "non_hive": { - "close": 909, - "high": 909, - "low": 909, - "open": 909, - "volume": 909 - }, - "open": "2020-10-28T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2755189, - "non_hive": { - "close": 143, - "high": 143, - "low": 143, - "open": 143, - "volume": 143 - }, - "open": "2020-10-28T03:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 227, - "high": 1800, - "low": 227, - "open": 942, - "volume": 26339 - }, - "id": 2755192, - "non_hive": { - "close": 32, - "high": 259, - "low": 32, - "open": 135, - "volume": 3788 - }, - "open": "2020-10-28T03:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1216, - "high": 3084, - "low": 33871, - "open": 13896, - "volume": 92888 - }, - "id": 2755199, - "non_hive": { - "close": 175, - "high": 444, - "low": 4855, - "open": 1999, - "volume": 13326 - }, - "open": "2020-10-28T03:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 74999, - "high": 74999, - "low": 74999, - "open": 74999, - "volume": 74999 - }, - "id": 2755206, - "non_hive": { - "close": 10792, - "high": 10792, - "low": 10792, - "open": 10792, - "volume": 10792 - }, - "open": "2020-10-28T04:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 12004, - "high": 6441, - "low": 12004, - "open": 15000, - "volume": 59599 - }, - "id": 2755210, - "non_hive": { - "close": 1726, - "high": 927, - "low": 1726, - "open": 2158, - "volume": 8574 - }, - "open": "2020-10-28T04:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 146, - "high": 146, - "low": 13908, - "open": 13908, - "volume": 14054 - }, - "id": 2755215, - "non_hive": { - "close": 21, - "high": 21, - "low": 1999, - "open": 1999, - "volume": 2020 - }, - "open": "2020-10-28T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 13950, - "high": 13950, - "low": 13950, - "open": 13950, - "volume": 13950 - }, - "id": 2755220, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-28T04:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 69579, - "high": 70003, - "low": 69579, - "open": 70003, - "volume": 141593 - }, - "id": 2755223, - "non_hive": { - "close": 9998, - "high": 10065, - "low": 9998, - "open": 10065, - "volume": 20352 - }, - "open": "2020-10-28T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 61034, - "high": 13, - "low": 61034, - "open": 13, - "volume": 74996 - }, - "id": 2755229, - "non_hive": { - "close": 8750, - "high": 2, - "low": 8750, - "open": 2, - "volume": 10752 - }, - "open": "2020-10-28T05:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1499, - "high": 13, - "low": 137, - "open": 137, - "volume": 29518 - }, - "id": 2755232, - "non_hive": { - "close": 214, - "high": 2, - "low": 19, - "open": 19, - "volume": 4230 - }, - "open": "2020-10-28T05:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 20355, - "high": 1583, - "low": 20355, - "open": 1583, - "volume": 35893 - }, - "id": 2755237, - "non_hive": { - "close": 2916, - "high": 227, - "low": 2916, - "open": 227, - "volume": 5143 - }, - "open": "2020-10-28T05:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 69580, - "high": 34773, - "low": 69580, - "open": 34773, - "volume": 104353 - }, - "id": 2755240, - "non_hive": { - "close": 10000, - "high": 5000, - "low": 10000, - "open": 5000, - "volume": 15000 - }, - "open": "2020-10-28T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 10484, - "high": 1359, - "low": 10484, - "open": 1359, - "volume": 62203 - }, - "id": 2755243, - "non_hive": { - "close": 1502, - "high": 195, - "low": 1502, - "open": 195, - "volume": 8914 - }, - "open": "2020-10-28T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13962, - "high": 13962, - "low": 13962, - "open": 13962, - "volume": 55848 - }, - "id": 2755246, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 7996 - }, - "open": "2020-10-28T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 92858, - "high": 14, - "low": 92858, - "open": 14046, - "volume": 1669544 - }, - "id": 2755253, - "non_hive": { - "close": 13000, - "high": 2, - "low": 13000, - "open": 2000, - "volume": 234632 - }, - "open": "2020-10-28T06:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2367, - "high": 7435, - "low": 7455, - "open": 7435, - "volume": 84481 - }, - "id": 2755257, - "non_hive": { - "close": 331, - "high": 1041, - "low": 1039, - "open": 1041, - "volume": 11820 - }, - "open": "2020-10-28T06:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2755267, - "non_hive": { - "close": 140, - "high": 140, - "low": 140, - "open": 140, - "volume": 140 - }, - "open": "2020-10-28T06:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 5264, - "high": 5264, - "low": 5264, - "open": 5264, - "volume": 5264 - }, - "id": 2755270, - "non_hive": { - "close": 737, - "high": 737, - "low": 737, - "open": 737, - "volume": 737 - }, - "open": "2020-10-28T06:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 18366, - "high": 18366, - "low": 18366, - "open": 18366, - "volume": 18366 - }, - "id": 2755273, - "non_hive": { - "close": 2571, - "high": 2571, - "low": 2571, - "open": 2571, - "volume": 2571 - }, - "open": "2020-10-28T06:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 17651, - "high": 17651, - "low": 17651, - "open": 17651, - "volume": 17651 - }, - "id": 2755276, - "non_hive": { - "close": 2471, - "high": 2471, - "low": 2471, - "open": 2471, - "volume": 2471 - }, - "open": "2020-10-28T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 42, - "high": 42, - "low": 271, - "open": 271, - "volume": 313 - }, - "id": 2755280, - "non_hive": { - "close": 6, - "high": 6, - "low": 38, - "open": 38, - "volume": 44 - }, - "open": "2020-10-28T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 16651, - "high": 16651, - "low": 38446, - "open": 38446, - "volume": 55097 - }, - "id": 2755285, - "non_hive": { - "close": 2331, - "high": 2331, - "low": 5382, - "open": 5382, - "volume": 7713 - }, - "open": "2020-10-28T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 1057, - "high": 1057, - "low": 1057, - "open": 1057, - "volume": 1057 - }, - "id": 2755290, - "non_hive": { - "close": 148, - "high": 148, - "low": 148, - "open": 148, - "volume": 148 - }, - "open": "2020-10-28T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 7475, - "high": 15837, - "low": 7475, - "open": 15837, - "volume": 294553 - }, - "id": 2755293, - "non_hive": { - "close": 1039, - "high": 2217, - "low": 1039, - "open": 2217, - "volume": 41005 - }, - "open": "2020-10-28T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 58150, - "high": 58150, - "low": 14380, - "open": 14380, - "volume": 72530 - }, - "id": 2755296, - "non_hive": { - "close": 8077, - "high": 8077, - "low": 1997, - "open": 1997, - "volume": 10074 - }, - "open": "2020-10-28T07:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 13283, - "high": 13283, - "low": 13283, - "open": 13283, - "volume": 13283 - }, - "id": 2755299, - "non_hive": { - "close": 1845, - "high": 1845, - "low": 1845, - "open": 1845, - "volume": 1845 - }, - "open": "2020-10-28T08:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 21216, - "high": 21216, - "low": 21216, - "open": 21216, - "volume": 21216 - }, - "id": 2755303, - "non_hive": { - "close": 2947, - "high": 2947, - "low": 2947, - "open": 2947, - "volume": 2947 - }, - "open": "2020-10-28T08:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 36004, - "high": 6076, - "low": 36004, - "open": 6076, - "volume": 42080 - }, - "id": 2755306, - "non_hive": { - "close": 5001, - "high": 844, - "low": 5001, - "open": 844, - "volume": 5845 - }, - "open": "2020-10-28T10:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 21116, - "high": 21605, - "low": 21116, - "open": 21605, - "volume": 42721 - }, - "id": 2755312, - "non_hive": { - "close": 2933, - "high": 3001, - "low": 2933, - "open": 3001, - "volume": 5934 - }, - "open": "2020-10-28T10:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 298077, - "high": 298077, - "low": 237216, - "open": 237216, - "volume": 714444 - }, - "id": 2755317, - "non_hive": { - "close": 41933, - "high": 41933, - "low": 32949, - "open": 32949, - "volume": 100000 - }, - "open": "2020-10-28T10:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 61236, - "high": 61236, - "low": 61236, - "open": 61236, - "volume": 61236 - }, - "id": 2755320, - "non_hive": { - "close": 8613, - "high": 8613, - "low": 8613, - "open": 8613, - "volume": 8613 - }, - "open": "2020-10-28T10:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 14, - "high": 14, - "low": 14, - "open": 14, - "volume": 14 - }, - "id": 2755323, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-10-28T10:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 199, - "high": 199, - "low": 199, - "open": 199, - "volume": 199 - }, - "id": 2755326, - "non_hive": { - "close": 28, - "high": 28, - "low": 28, - "open": 28, - "volume": 28 - }, - "open": "2020-10-28T11:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 118, - "high": 14610, - "low": 96, - "open": 14610, - "volume": 32342 - }, - "id": 2755330, - "non_hive": { - "close": 16, - "high": 2060, - "low": 13, - "open": 2060, - "volume": 4559 - }, - "open": "2020-10-28T11:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 24510, - "high": 24510, - "low": 214, - "open": 214, - "volume": 68725 - }, - "id": 2755334, - "non_hive": { - "close": 3520, - "high": 3520, - "low": 30, - "open": 30, - "volume": 9806 - }, - "open": "2020-10-28T11:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 330654, - "high": 4349, - "low": 330654, - "open": 4349, - "volume": 451995 - }, - "id": 2755337, - "non_hive": { - "close": 46492, - "high": 620, - "low": 46492, - "open": 620, - "volume": 63680 - }, - "open": "2020-10-28T12:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 20006, - "high": 20006, - "low": 20006, - "open": 20006, - "volume": 20006 - }, - "id": 2755341, - "non_hive": { - "close": 2813, - "high": 2813, - "low": 2813, - "open": 2813, - "volume": 2813 - }, - "open": "2020-10-28T12:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3897, - "high": 6600, - "low": 3897, - "open": 6600, - "volume": 10497 - }, - "id": 2755344, - "non_hive": { - "close": 547, - "high": 928, - "low": 547, - "open": 928, - "volume": 1475 - }, - "open": "2020-10-28T12:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 234, - "high": 234, - "low": 3001, - "open": 3001, - "volume": 3235 - }, - "id": 2755349, - "non_hive": { - "close": 33, - "high": 33, - "low": 422, - "open": 422, - "volume": 455 - }, - "open": "2020-10-28T12:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 18154, - "high": 81846, - "low": 18154, - "open": 81846, - "volume": 100000 - }, - "id": 2755354, - "non_hive": { - "close": 2552, - "high": 11508, - "low": 2552, - "open": 11508, - "volume": 14060 - }, - "open": "2020-10-28T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 71429, - "high": 4786, - "low": 71429, - "open": 12069, - "volume": 135020 - }, - "id": 2755358, - "non_hive": { - "close": 10000, - "high": 673, - "low": 10000, - "open": 1697, - "volume": 18936 - }, - "open": "2020-10-28T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 270, - "high": 270, - "low": 270, - "open": 270, - "volume": 270 - }, - "id": 2755361, - "non_hive": { - "close": 38, - "high": 38, - "low": 38, - "open": 38, - "volume": 38 - }, - "open": "2020-10-28T13:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13082, - "high": 13082, - "low": 13082, - "open": 13082, - "volume": 13082 - }, - "id": 2755364, - "non_hive": { - "close": 1839, - "high": 1839, - "low": 1839, - "open": 1839, - "volume": 1839 - }, - "open": "2020-10-28T13:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 14, - "high": 14, - "low": 14, - "open": 14, - "volume": 14 - }, - "id": 2755367, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-10-28T13:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2893, - "high": 7, - "low": 2893, - "open": 12944, - "volume": 146521 - }, - "id": 2755370, - "non_hive": { - "close": 405, - "high": 1, - "low": 405, - "open": 1820, - "volume": 20593 - }, - "open": "2020-10-28T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 7436, - "high": 2306, - "low": 14285, - "open": 11391, - "volume": 218399 - }, - "id": 2755381, - "non_hive": { - "close": 1041, - "high": 323, - "low": 1999, - "open": 1595, - "volume": 30576 - }, - "open": "2020-10-28T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 7455, - "high": 7455, - "low": 7455, - "open": 7455, - "volume": 7455 - }, - "id": 2755395, - "non_hive": { - "close": 1039, - "high": 1039, - "low": 1039, - "open": 1039, - "volume": 1039 - }, - "open": "2020-10-28T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 700192, - "high": 700192, - "low": 14290, - "open": 7003, - "volume": 721485 - }, - "id": 2755398, - "non_hive": { - "close": 98001, - "high": 98001, - "low": 1999, - "open": 980, - "volume": 100980 - }, - "open": "2020-10-28T14:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 14378, - "high": 14378, - "low": 14378, - "open": 14378, - "volume": 14378 - }, - "id": 2755403, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-28T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 5268, - "high": 5268, - "low": 14315, - "open": 14315, - "volume": 19583 - }, - "id": 2755406, - "non_hive": { - "close": 736, - "high": 736, - "low": 1999, - "open": 1999, - "volume": 2735 - }, - "open": "2020-10-28T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 41196, - "high": 41196, - "low": 14289, - "open": 14289, - "volume": 55485 - }, - "id": 2755409, - "non_hive": { - "close": 5766, - "high": 5766, - "low": 1999, - "open": 1999, - "volume": 7765 - }, - "open": "2020-10-28T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 14378, - "high": 73378, - "low": 456, - "open": 73378, - "volume": 111801 - }, - "id": 2755412, - "non_hive": { - "close": 2000, - "high": 10270, - "low": 63, - "open": 10270, - "volume": 15633 - }, - "open": "2020-10-28T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1372, - "high": 1372, - "low": 1372, - "open": 1372, - "volume": 1372 - }, - "id": 2755418, - "non_hive": { - "close": 191, - "high": 191, - "low": 191, - "open": 191, - "volume": 191 - }, - "open": "2020-10-28T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 10474, - "high": 1158, - "low": 10474, - "open": 1158, - "volume": 92537 - }, - "id": 2755421, - "non_hive": { - "close": 1464, - "high": 162, - "low": 1464, - "open": 162, - "volume": 12940 - }, - "open": "2020-10-28T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 14574, - "high": 14574, - "low": 14574, - "open": 14574, - "volume": 14574 - }, - "id": 2755427, - "non_hive": { - "close": 2037, - "high": 2037, - "low": 2037, - "open": 2037, - "volume": 2037 - }, - "open": "2020-10-28T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 28589, - "high": 28589, - "low": 28589, - "open": 28589, - "volume": 28589 - }, - "id": 2755430, - "non_hive": { - "close": 4000, - "high": 4000, - "low": 4000, - "open": 4000, - "volume": 4000 - }, - "open": "2020-10-28T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 77736, - "high": 77736, - "low": 14305, - "open": 70663, - "volume": 852087 - }, - "id": 2755433, - "non_hive": { - "close": 10929, - "high": 10929, - "low": 1999, - "open": 9880, - "volume": 119319 - }, - "open": "2020-10-28T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 2582, - "high": 2582, - "low": 2582, - "open": 2582, - "volume": 2582 - }, - "id": 2755444, - "non_hive": { - "close": 363, - "high": 363, - "low": 363, - "open": 363, - "volume": 363 - }, - "open": "2020-10-28T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 156617, - "high": 156617, - "low": 22264, - "open": 22264, - "volume": 265937 - }, - "id": 2755447, - "non_hive": { - "close": 22021, - "high": 22021, - "low": 3130, - "open": 3130, - "volume": 37391 - }, - "open": "2020-10-28T15:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 711212, - "high": 2809, - "low": 4202, - "open": 53283, - "volume": 814021 - }, - "id": 2755450, - "non_hive": { - "close": 100000, - "high": 395, - "low": 590, - "open": 7491, - "volume": 114453 - }, - "open": "2020-10-28T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 30000, - "high": 17716, - "low": 30000, - "open": 100000, - "volume": 147716 - }, - "id": 2755455, - "non_hive": { - "close": 4217, - "high": 2491, - "low": 4217, - "open": 14059, - "volume": 20767 - }, - "open": "2020-10-28T15:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 5648, - "high": 7369, - "low": 1195, - "open": 1195, - "volume": 14212 - }, - "id": 2755460, - "non_hive": { - "close": 794, - "high": 1036, - "low": 167, - "open": 167, - "volume": 1997 - }, - "open": "2020-10-28T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 341, - "high": 341, - "low": 12000, - "open": 346636, - "volume": 734318 - }, - "id": 2755465, - "non_hive": { - "close": 48, - "high": 48, - "low": 1686, - "open": 48730, - "volume": 103231 - }, - "open": "2020-10-28T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1316, - "high": 1316, - "low": 3583, - "open": 13417, - "volume": 18316 - }, - "id": 2755472, - "non_hive": { - "close": 185, - "high": 185, - "low": 503, - "open": 1885, - "volume": 2573 - }, - "open": "2020-10-28T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 42, - "high": 42, - "low": 7700, - "open": 7700, - "volume": 7742 - }, - "id": 2755477, - "non_hive": { - "close": 6, - "high": 6, - "low": 1080, - "open": 1080, - "volume": 1086 - }, - "open": "2020-10-28T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 2249, - "high": 2249, - "low": 5598, - "open": 5598, - "volume": 7847 - }, - "id": 2755482, - "non_hive": { - "close": 316, - "high": 316, - "low": 785, - "open": 785, - "volume": 1101 - }, - "open": "2020-10-28T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 211585, - "high": 211585, - "low": 14000, - "open": 26550, - "volume": 752135 - }, - "id": 2755485, - "non_hive": { - "close": 29750, - "high": 29750, - "low": 1966, - "open": 3730, - "volume": 105696 - }, - "open": "2020-10-28T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 939, - "high": 16103, - "low": 939, - "open": 16103, - "volume": 37842 - }, - "id": 2755492, - "non_hive": { - "close": 132, - "high": 2264, - "low": 132, - "open": 2264, - "volume": 5320 - }, - "open": "2020-10-28T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 5657, - "high": 284, - "low": 1288, - "open": 284, - "volume": 10786 - }, - "id": 2755497, - "non_hive": { - "close": 795, - "high": 40, - "low": 181, - "open": 40, - "volume": 1516 - }, - "open": "2020-10-28T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 393754, - "high": 491, - "low": 393754, - "open": 35025, - "volume": 547025 - }, - "id": 2755503, - "non_hive": { - "close": 54877, - "high": 69, - "low": 54877, - "open": 4922, - "volume": 76286 - }, - "open": "2020-10-28T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 7402, - "high": 7402, - "low": 7402, - "open": 7402, - "volume": 7402 - }, - "id": 2755508, - "non_hive": { - "close": 1039, - "high": 1039, - "low": 1039, - "open": 1039, - "volume": 1039 - }, - "open": "2020-10-28T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 142395, - "high": 142395, - "low": 11598, - "open": 11598, - "volume": 153993 - }, - "id": 2755511, - "non_hive": { - "close": 20000, - "high": 20000, - "low": 1628, - "open": 1628, - "volume": 21628 - }, - "open": "2020-10-28T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 25945, - "high": 25945, - "low": 25945, - "open": 25945, - "volume": 25945 - }, - "id": 2755517, - "non_hive": { - "close": 3644, - "high": 3644, - "low": 3644, - "open": 3644, - "volume": 3644 - }, - "open": "2020-10-28T17:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 8176, - "high": 8176, - "low": 8176, - "open": 8176, - "volume": 8176 - }, - "id": 2755520, - "non_hive": { - "close": 1148, - "high": 1148, - "low": 1148, - "open": 1148, - "volume": 1148 - }, - "open": "2020-10-28T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 9131, - "high": 9131, - "low": 3536, - "open": 6464, - "volume": 19131 - }, - "id": 2755523, - "non_hive": { - "close": 1282, - "high": 1282, - "low": 496, - "open": 907, - "volume": 2685 - }, - "open": "2020-10-28T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 363, - "high": 363, - "low": 95166, - "open": 95166, - "volume": 153527 - }, - "id": 2755528, - "non_hive": { - "close": 51, - "high": 51, - "low": 13361, - "open": 13361, - "volume": 21555 - }, - "open": "2020-10-28T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 442, - "high": 442, - "low": 442, - "open": 442, - "volume": 442 - }, - "id": 2755535, - "non_hive": { - "close": 62, - "high": 62, - "low": 62, - "open": 62, - "volume": 62 - }, - "open": "2020-10-28T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 19772, - "high": 19772, - "low": 19772, - "open": 19772, - "volume": 19772 - }, - "id": 2755538, - "non_hive": { - "close": 2770, - "high": 2770, - "low": 2770, - "open": 2770, - "volume": 2770 - }, - "open": "2020-10-28T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 857, - "high": 63792, - "low": 857, - "open": 63792, - "volume": 64649 - }, - "id": 2755541, - "non_hive": { - "close": 120, - "high": 8937, - "low": 120, - "open": 8937, - "volume": 9057 - }, - "open": "2020-10-28T17:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 5685, - "high": 14325, - "low": 5685, - "open": 14325, - "volume": 999000 - }, - "id": 2755544, - "non_hive": { - "close": 789, - "high": 2000, - "low": 789, - "open": 2000, - "volume": 138899 - }, - "open": "2020-10-28T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2850, - "high": 86, - "low": 2850, - "open": 86, - "volume": 227803 - }, - "id": 2755548, - "non_hive": { - "close": 395, - "high": 12, - "low": 395, - "open": 12, - "volume": 31663 - }, - "open": "2020-10-28T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 14390, - "high": 41828, - "low": 14390, - "open": 41828, - "volume": 63034 - }, - "id": 2755556, - "non_hive": { - "close": 1998, - "high": 5812, - "low": 1998, - "open": 5812, - "volume": 8757 - }, - "open": "2020-10-28T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 11303, - "high": 14, - "low": 7327, - "open": 1756, - "volume": 94055 - }, - "id": 2755559, - "non_hive": { - "close": 1576, - "high": 2, - "low": 1014, - "open": 245, - "volume": 13070 - }, - "open": "2020-10-28T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 4439, - "high": 173, - "low": 4439, - "open": 173, - "volume": 4641 - }, - "id": 2755568, - "non_hive": { - "close": 612, - "high": 24, - "low": 612, - "open": 24, - "volume": 640 - }, - "open": "2020-10-28T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7540, - "high": 5759, - "low": 7540, - "open": 14351, - "volume": 118971 - }, - "id": 2755571, - "non_hive": { - "close": 1032, - "high": 806, - "low": 1032, - "open": 1999, - "volume": 16520 - }, - "open": "2020-10-28T18:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 463532, - "high": 21751, - "low": 66536, - "open": 21751, - "volume": 719705 - }, - "id": 2755577, - "non_hive": { - "close": 63890, - "high": 3000, - "low": 9170, - "open": 3000, - "volume": 99201 - }, - "open": "2020-10-28T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 61157, - "high": 61157, - "low": 943, - "open": 23302, - "volume": 176910 - }, - "id": 2755584, - "non_hive": { - "close": 8566, - "high": 8566, - "low": 130, - "open": 3214, - "volume": 24628 - }, - "open": "2020-10-28T19:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 84760, - "high": 90086, - "low": 9182, - "open": 9182, - "volume": 441557 - }, - "id": 2755593, - "non_hive": { - "close": 11872, - "high": 12618, - "low": 1286, - "open": 1286, - "volume": 61847 - }, - "open": "2020-10-28T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 75028, - "high": 3055, - "low": 20900, - "open": 83061, - "volume": 529981 - }, - "id": 2755604, - "non_hive": { - "close": 10511, - "high": 428, - "low": 2927, - "open": 11634, - "volume": 74241 - }, - "open": "2020-10-28T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 67783, - "high": 72037, - "low": 73515, - "open": 73515, - "volume": 353098 - }, - "id": 2755618, - "non_hive": { - "close": 9496, - "high": 10092, - "low": 10299, - "open": 10299, - "volume": 49467 - }, - "open": "2020-10-28T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 59879, - "high": 26239, - "low": 38913, - "open": 66419, - "volume": 378689 - }, - "id": 2755629, - "non_hive": { - "close": 8407, - "high": 3684, - "low": 5451, - "open": 9305, - "volume": 53099 - }, - "open": "2020-10-28T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 5984, - "high": 5984, - "low": 55603, - "open": 55603, - "volume": 90998 - }, - "id": 2755641, - "non_hive": { - "close": 853, - "high": 853, - "low": 7806, - "open": 7806, - "volume": 12826 - }, - "open": "2020-10-28T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 55430, - "high": 14146, - "low": 14146, - "open": 14146, - "volume": 147669 - }, - "id": 2755648, - "non_hive": { - "close": 7836, - "high": 2000, - "low": 1999, - "open": 2000, - "volume": 20875 - }, - "open": "2020-10-28T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 14146, - "high": 23449, - "low": 14146, - "open": 7326, - "volume": 101505 - }, - "id": 2755654, - "non_hive": { - "close": 2000, - "high": 3343, - "low": 2000, - "open": 1043, - "volume": 14386 - }, - "open": "2020-10-28T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 274, - "high": 274, - "low": 274, - "open": 274, - "volume": 274 - }, - "id": 2755666, - "non_hive": { - "close": 39, - "high": 39, - "low": 39, - "open": 39, - "volume": 39 - }, - "open": "2020-10-28T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 51607, - "high": 2446, - "low": 819, - "open": 2446, - "volume": 1376120 - }, - "id": 2755670, - "non_hive": { - "close": 7225, - "high": 346, - "low": 114, - "open": 346, - "volume": 192773 - }, - "open": "2020-10-28T20:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 28643, - "high": 48557, - "low": 108, - "open": 108, - "volume": 287826 - }, - "id": 2755681, - "non_hive": { - "close": 4010, - "high": 6798, - "low": 15, - "open": 15, - "volume": 40286 - }, - "open": "2020-10-28T20:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 506148, - "high": 45686, - "low": 506148, - "open": 45686, - "volume": 1906050 - }, - "id": 2755698, - "non_hive": { - "close": 70547, - "high": 6396, - "low": 70547, - "open": 6396, - "volume": 266521 - }, - "open": "2020-10-28T20:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 8318, - "high": 8318, - "low": 8318, - "open": 8318, - "volume": 8318 - }, - "id": 2755705, - "non_hive": { - "close": 1164, - "high": 1164, - "low": 1164, - "open": 1164, - "volume": 1164 - }, - "open": "2020-10-28T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 14349, - "high": 14349, - "low": 14349, - "open": 14349, - "volume": 14349 - }, - "id": 2755708, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-28T20:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 14293, - "high": 164029, - "low": 14293, - "open": 164029, - "volume": 223117 - }, - "id": 2755711, - "non_hive": { - "close": 1999, - "high": 22952, - "low": 1999, - "open": 22952, - "volume": 31219 - }, - "open": "2020-10-28T20:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 3592, - "high": 3592, - "low": 3592, - "open": 3592, - "volume": 3592 - }, - "id": 2755716, - "non_hive": { - "close": 503, - "high": 503, - "low": 503, - "open": 503, - "volume": 503 - }, - "open": "2020-10-28T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 8572, - "high": 22008, - "low": 8572, - "open": 22008, - "volume": 54981 - }, - "id": 2755720, - "non_hive": { - "close": 1200, - "high": 3081, - "low": 1200, - "open": 3081, - "volume": 7697 - }, - "open": "2020-10-28T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 7143, - "high": 7143, - "low": 7143, - "open": 7143, - "volume": 7143 - }, - "id": 2755723, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-28T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3517, - "high": 28574, - "low": 3517, - "open": 28574, - "volume": 34070 - }, - "id": 2755726, - "non_hive": { - "close": 492, - "high": 4000, - "low": 492, - "open": 4000, - "volume": 4769 - }, - "open": "2020-10-28T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 5006, - "high": 5006, - "low": 5006, - "open": 5006, - "volume": 5006 - }, - "id": 2755729, - "non_hive": { - "close": 700, - "high": 700, - "low": 700, - "open": 700, - "volume": 700 - }, - "open": "2020-10-28T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1001, - "high": 1001, - "low": 1001, - "open": 1001, - "volume": 1001 - }, - "id": 2755732, - "non_hive": { - "close": 140, - "high": 140, - "low": 140, - "open": 140, - "volume": 140 - }, - "open": "2020-10-28T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 52839, - "high": 52839, - "low": 79, - "open": 79, - "volume": 52918 - }, - "id": 2755735, - "non_hive": { - "close": 7397, - "high": 7397, - "low": 11, - "open": 11, - "volume": 7408 - }, - "open": "2020-10-28T21:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 587, - "high": 2650, - "low": 12833, - "open": 43, - "volume": 330381 - }, - "id": 2755738, - "non_hive": { - "close": 82, - "high": 371, - "low": 1790, - "open": 6, - "volume": 46095 - }, - "open": "2020-10-28T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 7542, - "high": 6882, - "low": 7542, - "open": 6882, - "volume": 204470 - }, - "id": 2755745, - "non_hive": { - "close": 1037, - "high": 960, - "low": 1037, - "open": 960, - "volume": 28248 - }, - "open": "2020-10-28T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 72, - "high": 72, - "low": 72, - "open": 72, - "volume": 72 - }, - "id": 2755748, - "non_hive": { - "close": 10, - "high": 10, - "low": 10, - "open": 10, - "volume": 10 - }, - "open": "2020-10-28T22:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 548754, - "high": 21, - "low": 219363, - "open": 21, - "volume": 1234544 - }, - "id": 2755752, - "non_hive": { - "close": 74191, - "high": 3, - "low": 29657, - "open": 3, - "volume": 167279 - }, - "open": "2020-10-28T22:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 166430, - "high": 569, - "low": 166430, - "open": 14412, - "volume": 319735 - }, - "id": 2755759, - "non_hive": { - "close": 22490, - "high": 79, - "low": 22490, - "open": 1999, - "volume": 43265 - }, - "open": "2020-10-28T22:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 831822, - "high": 21635, - "low": 831822, - "open": 21635, - "volume": 868256 - }, - "id": 2755765, - "non_hive": { - "close": 112408, - "high": 3000, - "low": 112408, - "open": 3000, - "volume": 117408 - }, - "open": "2020-10-28T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 8642, - "high": 6342, - "low": 8642, - "open": 6342, - "volume": 30662 - }, - "id": 2755768, - "non_hive": { - "close": 1175, - "high": 874, - "low": 1175, - "open": 874, - "volume": 4203 - }, - "open": "2020-10-28T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 547821, - "high": 547821, - "low": 2869, - "open": 2869, - "volume": 702505 - }, - "id": 2755776, - "non_hive": { - "close": 76370, - "high": 76370, - "low": 392, - "open": 392, - "volume": 97788 - }, - "open": "2020-10-28T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 14496, - "high": 14496, - "low": 14402, - "open": 14402, - "volume": 36358 - }, - "id": 2755779, - "non_hive": { - "close": 2022, - "high": 2022, - "low": 1999, - "open": 1999, - "volume": 5057 - }, - "open": "2020-10-28T22:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 2932, - "high": 2932, - "low": 14056, - "open": 14400, - "volume": 45727 - }, - "id": 2755784, - "non_hive": { - "close": 409, - "high": 409, - "low": 1952, - "open": 2000, - "volume": 6360 - }, - "open": "2020-10-28T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 27067, - "high": 3716, - "low": 27067, - "open": 3716, - "volume": 30783 - }, - "id": 2755790, - "non_hive": { - "close": 3773, - "high": 518, - "low": 3773, - "open": 518, - "volume": 4291 - }, - "open": "2020-10-28T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13632, - "high": 13632, - "low": 13632, - "open": 13632, - "volume": 13632 - }, - "id": 2755793, - "non_hive": { - "close": 1899, - "high": 1899, - "low": 1899, - "open": 1899, - "volume": 1899 - }, - "open": "2020-10-28T23:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 7672, - "high": 7672, - "low": 7672, - "open": 7672, - "volume": 7672 - }, - "id": 2755796, - "non_hive": { - "close": 1057, - "high": 1057, - "low": 1057, - "open": 1057, - "volume": 1057 - }, - "open": "2020-10-28T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 464998, - "high": 11924, - "low": 464998, - "open": 14350, - "volume": 1251496 - }, - "id": 2755799, - "non_hive": { - "close": 63239, - "high": 1662, - "low": 63239, - "open": 1999, - "volume": 170655 - }, - "open": "2020-10-28T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 25106, - "high": 985, - "low": 25106, - "open": 985, - "volume": 43056 - }, - "id": 2755810, - "non_hive": { - "close": 3391, - "high": 134, - "low": 3391, - "open": 134, - "volume": 5826 - }, - "open": "2020-10-28T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12559, - "high": 49076, - "low": 14545, - "open": 14545, - "volume": 117147 - }, - "id": 2755813, - "non_hive": { - "close": 1737, - "high": 6844, - "low": 1999, - "open": 1999, - "volume": 16247 - }, - "open": "2020-10-29T00:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 97898, - "high": 97898, - "low": 1895, - "open": 1895, - "volume": 99793 - }, - "id": 2755820, - "non_hive": { - "close": 13540, - "high": 13540, - "low": 262, - "open": 262, - "volume": 13802 - }, - "open": "2020-10-29T00:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 118615, - "high": 14, - "low": 118615, - "open": 209, - "volume": 257134 - }, - "id": 2755824, - "non_hive": { - "close": 16013, - "high": 2, - "low": 16013, - "open": 29, - "volume": 34800 - }, - "open": "2020-10-29T00:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6976, - "high": 6976, - "low": 822067, - "open": 14621, - "volume": 1006976 - }, - "id": 2755831, - "non_hive": { - "close": 951, - "high": 951, - "low": 110979, - "open": 1993, - "volume": 135975 - }, - "open": "2020-10-29T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2984, - "high": 2984, - "low": 7689, - "open": 7689, - "volume": 10673 - }, - "id": 2755836, - "non_hive": { - "close": 407, - "high": 407, - "low": 1048, - "open": 1048, - "volume": 1455 - }, - "open": "2020-10-29T00:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 5244, - "high": 14756, - "low": 5244, - "open": 14756, - "volume": 20000 - }, - "id": 2755839, - "non_hive": { - "close": 710, - "high": 2000, - "low": 710, - "open": 2000, - "volume": 2710 - }, - "open": "2020-10-29T00:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1389, - "high": 1389, - "low": 1389, - "open": 1389, - "volume": 1389 - }, - "id": 2755842, - "non_hive": { - "close": 188, - "high": 188, - "low": 188, - "open": 188, - "volume": 188 - }, - "open": "2020-10-29T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 8099, - "high": 8099, - "low": 8099, - "open": 8099, - "volume": 8099 - }, - "id": 2755846, - "non_hive": { - "close": 1104, - "high": 1104, - "low": 1104, - "open": 1104, - "volume": 1104 - }, - "open": "2020-10-29T01:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 7661, - "high": 6185, - "low": 7661, - "open": 6185, - "volume": 44424 - }, - "id": 2755849, - "non_hive": { - "close": 1037, - "high": 843, - "low": 1037, - "open": 843, - "volume": 6026 - }, - "open": "2020-10-29T02:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 43, - "high": 6232, - "low": 43, - "open": 4576, - "volume": 18398 - }, - "id": 2755853, - "non_hive": { - "close": 5, - "high": 857, - "low": 5, - "open": 624, - "volume": 2519 - }, - "open": "2020-10-29T02:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 20058, - "high": 9159, - "low": 20058, - "open": 9159, - "volume": 1945090 - }, - "id": 2755858, - "non_hive": { - "close": 2707, - "high": 1254, - "low": 2707, - "open": 1254, - "volume": 262901 - }, - "open": "2020-10-29T02:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1355, - "high": 1355, - "low": 1355, - "open": 1355, - "volume": 1355 - }, - "id": 2755862, - "non_hive": { - "close": 184, - "high": 184, - "low": 184, - "open": 184, - "volume": 184 - }, - "open": "2020-10-29T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 7723, - "high": 7723, - "low": 7723, - "open": 7723, - "volume": 7723 - }, - "id": 2755866, - "non_hive": { - "close": 1052, - "high": 1052, - "low": 1052, - "open": 1052, - "volume": 1052 - }, - "open": "2020-10-29T03:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2062, - "high": 2831, - "low": 2062, - "open": 2831, - "volume": 7549 - }, - "id": 2755869, - "non_hive": { - "close": 281, - "high": 386, - "low": 281, - "open": 386, - "volume": 1029 - }, - "open": "2020-10-29T03:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1267, - "high": 1267, - "low": 1267, - "open": 1267, - "volume": 1267 - }, - "id": 2755872, - "non_hive": { - "close": 172, - "high": 172, - "low": 172, - "open": 172, - "volume": 172 - }, - "open": "2020-10-29T05:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1257, - "high": 1257, - "low": 1257, - "open": 1257, - "volume": 1257 - }, - "id": 2755876, - "non_hive": { - "close": 171, - "high": 171, - "low": 171, - "open": 171, - "volume": 171 - }, - "open": "2020-10-29T05:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 9243, - "high": 9243, - "low": 9243, - "open": 9243, - "volume": 9243 - }, - "id": 2755879, - "non_hive": { - "close": 1260, - "high": 1260, - "low": 1260, - "open": 1260, - "volume": 1260 - }, - "open": "2020-10-29T05:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 5422, - "high": 5422, - "low": 5422, - "open": 5422, - "volume": 5422 - }, - "id": 2755882, - "non_hive": { - "close": 739, - "high": 739, - "low": 739, - "open": 739, - "volume": 739 - }, - "open": "2020-10-29T06:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 8082, - "high": 2347, - "low": 8082, - "open": 1929, - "volume": 12358 - }, - "id": 2755886, - "non_hive": { - "close": 1101, - "high": 320, - "low": 1101, - "open": 263, - "volume": 1684 - }, - "open": "2020-10-29T06:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 3708, - "high": 163754, - "low": 3708, - "open": 163754, - "volume": 200000 - }, - "id": 2755889, - "non_hive": { - "close": 500, - "high": 22332, - "low": 500, - "open": 22332, - "volume": 27267 - }, - "open": "2020-10-29T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 7560, - "high": 7560, - "low": 7560, - "open": 7560, - "volume": 7560 - }, - "id": 2755894, - "non_hive": { - "close": 1031, - "high": 1031, - "low": 1031, - "open": 1031, - "volume": 1031 - }, - "open": "2020-10-29T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 4824, - "high": 4824, - "low": 4824, - "open": 4824, - "volume": 4824 - }, - "id": 2755898, - "non_hive": { - "close": 660, - "high": 660, - "low": 660, - "open": 660, - "volume": 660 - }, - "open": "2020-10-29T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2923, - "high": 2923, - "low": 2923, - "open": 2923, - "volume": 2923 - }, - "id": 2755901, - "non_hive": { - "close": 400, - "high": 400, - "low": 400, - "open": 400, - "volume": 400 - }, - "open": "2020-10-29T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 3158, - "high": 14660, - "low": 3158, - "open": 14660, - "volume": 47918 - }, - "id": 2755904, - "non_hive": { - "close": 430, - "high": 2000, - "low": 430, - "open": 2000, - "volume": 6536 - }, - "open": "2020-10-29T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 20022, - "high": 20022, - "low": 20022, - "open": 20022, - "volume": 20022 - }, - "id": 2755909, - "non_hive": { - "close": 2731, - "high": 2731, - "low": 2731, - "open": 2731, - "volume": 2731 - }, - "open": "2020-10-29T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 8702, - "high": 8702, - "low": 8702, - "open": 8702, - "volume": 8702 - }, - "id": 2755912, - "non_hive": { - "close": 1187, - "high": 1187, - "low": 1187, - "open": 1187, - "volume": 1187 - }, - "open": "2020-10-29T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6725, - "high": 11130, - "low": 6725, - "open": 11130, - "volume": 36150 - }, - "id": 2755915, - "non_hive": { - "close": 916, - "high": 1518, - "low": 916, - "open": 1518, - "volume": 4929 - }, - "open": "2020-10-29T07:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 5652, - "high": 5652, - "low": 14663, - "open": 14663, - "volume": 20315 - }, - "id": 2755918, - "non_hive": { - "close": 771, - "high": 771, - "low": 1999, - "open": 1999, - "volume": 2770 - }, - "open": "2020-10-29T08:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 407, - "high": 7328, - "low": 407, - "open": 7328, - "volume": 7735 - }, - "id": 2755922, - "non_hive": { - "close": 55, - "high": 998, - "low": 55, - "open": 998, - "volume": 1053 - }, - "open": "2020-10-29T08:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 444000, - "high": 14362, - "low": 444000, - "open": 14362, - "volume": 516089 - }, - "id": 2755927, - "non_hive": { - "close": 59940, - "high": 1945, - "low": 59940, - "open": 1945, - "volume": 69697 - }, - "open": "2020-10-29T08:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 21725, - "high": 21725, - "low": 21725, - "open": 21725, - "volume": 21725 - }, - "id": 2755932, - "non_hive": { - "close": 2933, - "high": 2933, - "low": 2933, - "open": 2933, - "volume": 2933 - }, - "open": "2020-10-29T08:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 59029, - "high": 59029, - "low": 59029, - "open": 59029, - "volume": 59029 - }, - "id": 2755935, - "non_hive": { - "close": 7969, - "high": 7969, - "low": 7969, - "open": 7969, - "volume": 7969 - }, - "open": "2020-10-29T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 39444, - "high": 39444, - "low": 39444, - "open": 39444, - "volume": 39444 - }, - "id": 2755938, - "non_hive": { - "close": 5325, - "high": 5325, - "low": 5325, - "open": 5325, - "volume": 5325 - }, - "open": "2020-10-29T08:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 41488, - "low": 1000, - "open": 41488, - "volume": 42488 - }, - "id": 2755941, - "non_hive": { - "close": 134, - "high": 5601, - "low": 134, - "open": 5601, - "volume": 5735 - }, - "open": "2020-10-29T09:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 10525, - "high": 10525, - "low": 39444, - "open": 39444, - "volume": 49969 - }, - "id": 2755947, - "non_hive": { - "close": 1421, - "high": 1421, - "low": 5325, - "open": 5325, - "volume": 6746 - }, - "open": "2020-10-29T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2158, - "high": 14118, - "low": 2158, - "open": 14118, - "volume": 99499 - }, - "id": 2755952, - "non_hive": { - "close": 290, - "high": 1906, - "low": 290, - "open": 1906, - "volume": 13430 - }, - "open": "2020-10-29T09:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 503, - "high": 503, - "low": 5273, - "open": 5273, - "volume": 5776 - }, - "id": 2755959, - "non_hive": { - "close": 68, - "high": 68, - "low": 710, - "open": 710, - "volume": 778 - }, - "open": "2020-10-29T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 39445, - "high": 4274, - "low": 39445, - "open": 4274, - "volume": 43719 - }, - "id": 2755963, - "non_hive": { - "close": 5325, - "high": 577, - "low": 5325, - "open": 577, - "volume": 5902 - }, - "open": "2020-10-29T09:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 15982, - "high": 10254, - "low": 1559, - "open": 10254, - "volume": 188404 - }, - "id": 2755968, - "non_hive": { - "close": 2157, - "high": 1384, - "low": 210, - "open": 1384, - "volume": 25395 - }, - "open": "2020-10-29T09:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 2800, - "high": 40038, - "low": 2800, - "open": 40038, - "volume": 43187 - }, - "id": 2755976, - "non_hive": { - "close": 377, - "high": 5392, - "low": 377, - "open": 5392, - "volume": 5816 - }, - "open": "2020-10-29T09:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 14873, - "high": 40058, - "low": 14873, - "open": 40058, - "volume": 97995 - }, - "id": 2755980, - "non_hive": { - "close": 1999, - "high": 5393, - "low": 1999, - "open": 5393, - "volume": 13182 - }, - "open": "2020-10-29T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 15498, - "high": 8809, - "low": 15498, - "open": 8809, - "volume": 24307 - }, - "id": 2755989, - "non_hive": { - "close": 2090, - "high": 1188, - "low": 2090, - "open": 1188, - "volume": 3278 - }, - "open": "2020-10-29T10:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2922, - "high": 2922, - "low": 2922, - "open": 2922, - "volume": 2922 - }, - "id": 2755995, - "non_hive": { - "close": 394, - "high": 394, - "low": 394, - "open": 394, - "volume": 394 - }, - "open": "2020-10-29T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 2327, - "high": 2327, - "low": 1090, - "open": 1090, - "volume": 3417 - }, - "id": 2755998, - "non_hive": { - "close": 314, - "high": 314, - "low": 147, - "open": 147, - "volume": 461 - }, - "open": "2020-10-29T10:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 5212, - "high": 5212, - "low": 5212, - "open": 5212, - "volume": 5212 - }, - "id": 2756001, - "non_hive": { - "close": 703, - "high": 703, - "low": 703, - "open": 703, - "volume": 703 - }, - "open": "2020-10-29T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "id": 2756004, - "non_hive": { - "close": 268, - "high": 268, - "low": 268, - "open": 268, - "volume": 268 - }, - "open": "2020-10-29T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3944, - "high": 3944, - "low": 3944, - "open": 3944, - "volume": 3944 - }, - "id": 2756007, - "non_hive": { - "close": 532, - "high": 532, - "low": 532, - "open": 532, - "volume": 532 - }, - "open": "2020-10-29T10:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 37074, - "high": 36629, - "low": 37074, - "open": 36629, - "volume": 73703 - }, - "id": 2756010, - "non_hive": { - "close": 5000, - "high": 4940, - "low": 5000, - "open": 4940, - "volume": 9940 - }, - "open": "2020-10-29T11:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 20916, - "high": 20916, - "low": 20916, - "open": 20916, - "volume": 20916 - }, - "id": 2756014, - "non_hive": { - "close": 2821, - "high": 2821, - "low": 2821, - "open": 2821, - "volume": 2821 - }, - "open": "2020-10-29T11:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 11300, - "high": 11300, - "low": 11300, - "open": 11300, - "volume": 11300 - }, - "id": 2756017, - "non_hive": { - "close": 1519, - "high": 1519, - "low": 1519, - "open": 1519, - "volume": 1519 - }, - "open": "2020-10-29T11:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 107131, - "high": 107131, - "low": 70886, - "open": 70886, - "volume": 178017 - }, - "id": 2756020, - "non_hive": { - "close": 14452, - "high": 14452, - "low": 9562, - "open": 9562, - "volume": 24014 - }, - "open": "2020-10-29T11:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 5000, - "high": 741292, - "low": 5000, - "open": 741292, - "volume": 746292 - }, - "id": 2756023, - "non_hive": { - "close": 674, - "high": 100000, - "low": 674, - "open": 100000, - "volume": 100674 - }, - "open": "2020-10-29T11:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 14932, - "high": 33988, - "low": 14932, - "open": 33988, - "volume": 85989 - }, - "id": 2756028, - "non_hive": { - "close": 2000, - "high": 4585, - "low": 2000, - "open": 4585, - "volume": 11571 - }, - "open": "2020-10-29T12:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6458, - "high": 6458, - "low": 6458, - "open": 6458, - "volume": 6458 - }, - "id": 2756035, - "non_hive": { - "close": 864, - "high": 864, - "low": 864, - "open": 864, - "volume": 864 - }, - "open": "2020-10-29T12:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 92760, - "high": 92760, - "low": 92760, - "open": 92760, - "volume": 92760 - }, - "id": 2756038, - "non_hive": { - "close": 12424, - "high": 12424, - "low": 12424, - "open": 12424, - "volume": 12424 - }, - "open": "2020-10-29T12:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 39593, - "high": 39593, - "low": 39593, - "open": 39593, - "volume": 39593 - }, - "id": 2756041, - "non_hive": { - "close": 5303, - "high": 5303, - "low": 5303, - "open": 5303, - "volume": 5303 - }, - "open": "2020-10-29T12:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 853, - "high": 7473, - "low": 853, - "open": 7473, - "volume": 8326 - }, - "id": 2756044, - "non_hive": { - "close": 114, - "high": 1001, - "low": 114, - "open": 1001, - "volume": 1115 - }, - "open": "2020-10-29T12:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 43353, - "high": 43353, - "low": 14870, - "open": 14870, - "volume": 58223 - }, - "id": 2756048, - "non_hive": { - "close": 5831, - "high": 5831, - "low": 1999, - "open": 1999, - "volume": 7830 - }, - "open": "2020-10-29T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 468, - "high": 468, - "low": 468, - "open": 468, - "volume": 468 - }, - "id": 2756051, - "non_hive": { - "close": 63, - "high": 63, - "low": 63, - "open": 63, - "volume": 63 - }, - "open": "2020-10-29T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 8854, - "high": 8854, - "low": 8854, - "open": 8854, - "volume": 8854 - }, - "id": 2756055, - "non_hive": { - "close": 1190, - "high": 1190, - "low": 1190, - "open": 1190, - "volume": 1190 - }, - "open": "2020-10-29T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 4758, - "high": 4758, - "low": 4758, - "open": 4758, - "volume": 4758 - }, - "id": 2756058, - "non_hive": { - "close": 640, - "high": 640, - "low": 640, - "open": 640, - "volume": 640 - }, - "open": "2020-10-29T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 215738, - "high": 215738, - "low": 14820, - "open": 14820, - "volume": 296570 - }, - "id": 2756061, - "non_hive": { - "close": 29103, - "high": 29103, - "low": 1993, - "open": 1993, - "volume": 40000 - }, - "open": "2020-10-29T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 918785, - "high": 918785, - "low": 118342, - "open": 9925, - "volume": 1047052 - }, - "id": 2756064, - "non_hive": { - "close": 124036, - "high": 124036, - "low": 15964, - "open": 1339, - "volume": 141339 - }, - "open": "2020-10-29T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 9186, - "high": 9104, - "low": 9186, - "open": 9104, - "volume": 36595 - }, - "id": 2756070, - "non_hive": { - "close": 1240, - "high": 1229, - "low": 1240, - "open": 1229, - "volume": 4940 - }, - "open": "2020-10-29T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 5303, - "high": 5303, - "low": 5303, - "open": 5303, - "volume": 5303 - }, - "id": 2756075, - "non_hive": { - "close": 716, - "high": 716, - "low": 716, - "open": 716, - "volume": 716 - }, - "open": "2020-10-29T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 88855, - "high": 15000, - "low": 88855, - "open": 15000, - "volume": 103855 - }, - "id": 2756078, - "non_hive": { - "close": 11995, - "high": 2025, - "low": 11995, - "open": 2025, - "volume": 14020 - }, - "open": "2020-10-29T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 464330, - "high": 464330, - "low": 464330, - "open": 464330, - "volume": 464330 - }, - "id": 2756083, - "non_hive": { - "close": 62684, - "high": 62684, - "low": 62684, - "open": 62684, - "volume": 62684 - }, - "open": "2020-10-29T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 14813, - "high": 14813, - "low": 14813, - "open": 14813, - "volume": 14813 - }, - "id": 2756086, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-29T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12162, - "high": 14813, - "low": 12162, - "open": 14813, - "volume": 26975 - }, - "id": 2756089, - "non_hive": { - "close": 1642, - "high": 2000, - "low": 1642, - "open": 2000, - "volume": 3642 - }, - "open": "2020-10-29T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 14707, - "high": 14707, - "low": 14707, - "open": 14707, - "volume": 14707 - }, - "id": 2756093, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-29T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3193, - "high": 3193, - "low": 273, - "open": 14708, - "volume": 99109 - }, - "id": 2756096, - "non_hive": { - "close": 434, - "high": 434, - "low": 37, - "open": 1999, - "volume": 13470 - }, - "open": "2020-10-29T15:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1020, - "high": 317, - "low": 1020, - "open": 317, - "volume": 17985 - }, - "id": 2756102, - "non_hive": { - "close": 138, - "high": 43, - "low": 138, - "open": 43, - "volume": 2434 - }, - "open": "2020-10-29T15:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 14154, - "high": 16822, - "low": 14154, - "open": 16822, - "volume": 33786 - }, - "id": 2756105, - "non_hive": { - "close": 1914, - "high": 2275, - "low": 1914, - "open": 2275, - "volume": 4569 - }, - "open": "2020-10-29T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 7804, - "high": 7804, - "low": 7804, - "open": 7804, - "volume": 7804 - }, - "id": 2756108, - "non_hive": { - "close": 1055, - "high": 1055, - "low": 1055, - "open": 1055, - "volume": 1055 - }, - "open": "2020-10-29T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 2589, - "high": 2589, - "low": 1376, - "open": 1376, - "volume": 3965 - }, - "id": 2756111, - "non_hive": { - "close": 350, - "high": 350, - "low": 186, - "open": 186, - "volume": 536 - }, - "open": "2020-10-29T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 15366, - "high": 30546, - "low": 15366, - "open": 30546, - "volume": 1500771 - }, - "id": 2756117, - "non_hive": { - "close": 2058, - "high": 4129, - "low": 2058, - "open": 4129, - "volume": 201406 - }, - "open": "2020-10-29T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 11820, - "high": 11820, - "low": 1445, - "open": 1445, - "volume": 13265 - }, - "id": 2756122, - "non_hive": { - "close": 1596, - "high": 1596, - "low": 195, - "open": 195, - "volume": 1791 - }, - "open": "2020-10-29T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 148, - "high": 148, - "low": 234, - "open": 11664, - "volume": 26943 - }, - "id": 2756126, - "non_hive": { - "close": 20, - "high": 20, - "low": 31, - "open": 1575, - "volume": 3626 - }, - "open": "2020-10-29T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2000, - "high": 573, - "low": 2000, - "open": 14330, - "volume": 24330 - }, - "id": 2756131, - "non_hive": { - "close": 267, - "high": 77, - "low": 267, - "open": 1923, - "volume": 3262 - }, - "open": "2020-10-29T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 2837, - "high": 2837, - "low": 2837, - "open": 2837, - "volume": 2837 - }, - "id": 2756138, - "non_hive": { - "close": 383, - "high": 383, - "low": 383, - "open": 383, - "volume": 383 - }, - "open": "2020-10-29T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 139609, - "high": 139609, - "low": 139609, - "open": 139609, - "volume": 139609 - }, - "id": 2756141, - "non_hive": { - "close": 18860, - "high": 18860, - "low": 18860, - "open": 18860, - "volume": 18860 - }, - "open": "2020-10-29T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 8409, - "high": 14815, - "low": 2250, - "open": 14815, - "volume": 93028 - }, - "id": 2756144, - "non_hive": { - "close": 1134, - "high": 1999, - "low": 299, - "open": 1999, - "volume": 12498 - }, - "open": "2020-10-29T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11000, - "high": 11000, - "low": 6416, - "open": 6416, - "volume": 17416 - }, - "id": 2756152, - "non_hive": { - "close": 1484, - "high": 1484, - "low": 865, - "open": 865, - "volume": 2349 - }, - "open": "2020-10-29T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12615, - "high": 12615, - "low": 12615, - "open": 12615, - "volume": 12615 - }, - "id": 2756156, - "non_hive": { - "close": 1705, - "high": 1705, - "low": 1705, - "open": 1705, - "volume": 1705 - }, - "open": "2020-10-29T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 15692, - "high": 15692, - "low": 3344, - "open": 3344, - "volume": 19036 - }, - "id": 2756159, - "non_hive": { - "close": 2122, - "high": 2122, - "low": 452, - "open": 452, - "volume": 2574 - }, - "open": "2020-10-29T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 10005, - "high": 576, - "low": 32944, - "open": 7661, - "volume": 53345 - }, - "id": 2756162, - "non_hive": { - "close": 1353, - "high": 78, - "low": 4455, - "open": 1036, - "volume": 7214 - }, - "open": "2020-10-29T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 7142, - "high": 7142, - "low": 7142, - "open": 7142, - "volume": 7142 - }, - "id": 2756169, - "non_hive": { - "close": 965, - "high": 965, - "low": 965, - "open": 965, - "volume": 965 - }, - "open": "2020-10-29T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1294, - "high": 1294, - "low": 1294, - "open": 1294, - "volume": 1294 - }, - "id": 2756172, - "non_hive": { - "close": 175, - "high": 175, - "low": 175, - "open": 175, - "volume": 175 - }, - "open": "2020-10-29T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 20890, - "high": 20890, - "low": 20890, - "open": 20890, - "volume": 20890 - }, - "id": 2756175, - "non_hive": { - "close": 2823, - "high": 2823, - "low": 2823, - "open": 2823, - "volume": 2823 - }, - "open": "2020-10-29T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 4002, - "high": 4002, - "low": 4002, - "open": 4002, - "volume": 4002 - }, - "id": 2756179, - "non_hive": { - "close": 541, - "high": 541, - "low": 541, - "open": 541, - "volume": 541 - }, - "open": "2020-10-29T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 47, - "high": 37593, - "low": 47, - "open": 53706, - "volume": 184390 - }, - "id": 2756182, - "non_hive": { - "close": 6, - "high": 5083, - "low": 6, - "open": 7260, - "volume": 24926 - }, - "open": "2020-10-29T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 14496, - "high": 853, - "low": 14646, - "open": 853, - "volume": 57990 - }, - "id": 2756190, - "non_hive": { - "close": 1943, - "high": 115, - "low": 1962, - "open": 115, - "volume": 7774 - }, - "open": "2020-10-29T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 6822, - "high": 6822, - "low": 14799, - "open": 14799, - "volume": 21621 - }, - "id": 2756195, - "non_hive": { - "close": 922, - "high": 922, - "low": 1999, - "open": 1999, - "volume": 2921 - }, - "open": "2020-10-29T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 111550, - "high": 96377, - "low": 14616, - "open": 14616, - "volume": 228989 - }, - "id": 2756198, - "non_hive": { - "close": 15057, - "high": 13009, - "low": 1972, - "open": 1972, - "volume": 30908 - }, - "open": "2020-10-29T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 810, - "high": 810, - "low": 810, - "open": 810, - "volume": 810 - }, - "id": 2756203, - "non_hive": { - "close": 108, - "high": 108, - "low": 108, - "open": 108, - "volume": 108 - }, - "open": "2020-10-29T19:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2127, - "high": 2127, - "low": 2127, - "open": 2127, - "volume": 2127 - }, - "id": 2756207, - "non_hive": { - "close": 285, - "high": 285, - "low": 285, - "open": 285, - "volume": 285 - }, - "open": "2020-10-29T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 37178, - "high": 37178, - "low": 37178, - "open": 37178, - "volume": 37178 - }, - "id": 2756210, - "non_hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "open": "2020-10-29T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12385, - "high": 12385, - "low": 12385, - "open": 12385, - "volume": 12385 - }, - "id": 2756213, - "non_hive": { - "close": 1660, - "high": 1660, - "low": 1660, - "open": 1660, - "volume": 1660 - }, - "open": "2020-10-29T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2179, - "high": 12631, - "low": 9592, - "open": 410, - "volume": 24812 - }, - "id": 2756216, - "non_hive": { - "close": 294, - "high": 1705, - "low": 1285, - "open": 55, - "volume": 3339 - }, - "open": "2020-10-29T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 72289, - "high": 2711, - "low": 72289, - "open": 2711, - "volume": 75000 - }, - "id": 2756222, - "non_hive": { - "close": 9757, - "high": 366, - "low": 9757, - "open": 366, - "volume": 10123 - }, - "open": "2020-10-29T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 4650, - "high": 4650, - "low": 4650, - "open": 4650, - "volume": 4650 - }, - "id": 2756225, - "non_hive": { - "close": 628, - "high": 628, - "low": 628, - "open": 628, - "volume": 628 - }, - "open": "2020-10-29T20:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 3969, - "high": 14728, - "low": 3969, - "open": 14728, - "volume": 18697 - }, - "id": 2756229, - "non_hive": { - "close": 536, - "high": 1989, - "low": 536, - "open": 1989, - "volume": 2525 - }, - "open": "2020-10-29T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 3295, - "high": 3295, - "low": 3295, - "open": 3295, - "volume": 3295 - }, - "id": 2756234, - "non_hive": { - "close": 445, - "high": 445, - "low": 445, - "open": 445, - "volume": 445 - }, - "open": "2020-10-29T20:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 277714, - "high": 277714, - "low": 6803, - "open": 2904, - "volume": 305439 - }, - "id": 2756237, - "non_hive": { - "close": 37504, - "high": 37504, - "low": 911, - "open": 392, - "volume": 41229 - }, - "open": "2020-10-29T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 597, - "high": 597, - "low": 597, - "open": 597, - "volume": 597 - }, - "id": 2756241, - "non_hive": { - "close": 80, - "high": 80, - "low": 80, - "open": 80, - "volume": 80 - }, - "open": "2020-10-29T20:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 23665, - "high": 14924, - "low": 23665, - "open": 14924, - "volume": 38589 - }, - "id": 2756244, - "non_hive": { - "close": 3171, - "high": 2000, - "low": 3171, - "open": 2000, - "volume": 5171 - }, - "open": "2020-10-29T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 19586, - "high": 19586, - "low": 19586, - "open": 19586, - "volume": 19586 - }, - "id": 2756247, - "non_hive": { - "close": 2645, - "high": 2645, - "low": 2645, - "open": 2645, - "volume": 2645 - }, - "open": "2020-10-29T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 55907, - "high": 7123, - "low": 55907, - "open": 7123, - "volume": 63030 - }, - "id": 2756250, - "non_hive": { - "close": 7550, - "high": 962, - "low": 7550, - "open": 962, - "volume": 8512 - }, - "open": "2020-10-29T20:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 3000, - "high": 3000, - "low": 3000, - "open": 3000, - "volume": 3000 - }, - "id": 2756255, - "non_hive": { - "close": 405, - "high": 405, - "low": 405, - "open": 405, - "volume": 405 - }, - "open": "2020-10-29T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 16616, - "high": 4013, - "low": 59246, - "open": 59246, - "volume": 79875 - }, - "id": 2756259, - "non_hive": { - "close": 2244, - "high": 542, - "low": 8001, - "open": 8001, - "volume": 10787 - }, - "open": "2020-10-29T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2858, - "high": 2858, - "low": 5454, - "open": 200007, - "volume": 213865 - }, - "id": 2756266, - "non_hive": { - "close": 386, - "high": 386, - "low": 736, - "open": 27010, - "volume": 28881 - }, - "open": "2020-10-29T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 50000, - "high": 5524, - "low": 50000, - "open": 5524, - "volume": 55524 - }, - "id": 2756273, - "non_hive": { - "close": 6752, - "high": 746, - "low": 6752, - "open": 746, - "volume": 7498 - }, - "open": "2020-10-29T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 19720, - "high": 3376, - "low": 19720, - "open": 3376, - "volume": 63883 - }, - "id": 2756278, - "non_hive": { - "close": 2663, - "high": 456, - "low": 2663, - "open": 456, - "volume": 8627 - }, - "open": "2020-10-29T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 148109, - "high": 148109, - "low": 8657, - "open": 8657, - "volume": 156766 - }, - "id": 2756281, - "non_hive": { - "close": 20000, - "high": 20000, - "low": 1169, - "open": 1169, - "volume": 21169 - }, - "open": "2020-10-29T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 15558, - "high": 7390, - "low": 15558, - "open": 7390, - "volume": 26258 - }, - "id": 2756287, - "non_hive": { - "close": 2101, - "high": 998, - "low": 2101, - "open": 998, - "volume": 3546 - }, - "open": "2020-10-29T22:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 525, - "high": 525, - "low": 525, - "open": 525, - "volume": 525 - }, - "id": 2756294, - "non_hive": { - "close": 71, - "high": 71, - "low": 71, - "open": 71, - "volume": 71 - }, - "open": "2020-10-29T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 797134, - "high": 797134, - "low": 10348, - "open": 67279, - "volume": 874761 - }, - "id": 2756297, - "non_hive": { - "close": 107645, - "high": 107645, - "low": 1397, - "open": 9085, - "volume": 118127 - }, - "open": "2020-10-29T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 96, - "high": 96, - "low": 34406, - "open": 34406, - "volume": 34502 - }, - "id": 2756304, - "non_hive": { - "close": 13, - "high": 13, - "low": 4646, - "open": 4646, - "volume": 4659 - }, - "open": "2020-10-29T23:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 52491, - "high": 11871, - "low": 52491, - "open": 11871, - "volume": 83966 - }, - "id": 2756310, - "non_hive": { - "close": 7085, - "high": 1603, - "low": 7085, - "open": 1603, - "volume": 11335 - }, - "open": "2020-10-29T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 118493, - "high": 118493, - "low": 118493, - "open": 118493, - "volume": 118493 - }, - "id": 2756317, - "non_hive": { - "close": 16000, - "high": 16000, - "low": 16000, - "open": 16000, - "volume": 16000 - }, - "open": "2020-10-29T23:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 14754, - "high": 14754, - "low": 14754, - "open": 14754, - "volume": 14754 - }, - "id": 2756320, - "non_hive": { - "close": 1992, - "high": 1992, - "low": 1992, - "open": 1992, - "volume": 1992 - }, - "open": "2020-10-29T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 19998, - "high": 19998, - "low": 19998, - "open": 19998, - "volume": 19998 - }, - "id": 2756323, - "non_hive": { - "close": 2700, - "high": 2700, - "low": 2700, - "open": 2700, - "volume": 2700 - }, - "open": "2020-10-29T23:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 1622, - "high": 1622, - "low": 437, - "open": 437, - "volume": 2059 - }, - "id": 2756326, - "non_hive": { - "close": 219, - "high": 219, - "low": 59, - "open": 59, - "volume": 278 - }, - "open": "2020-10-29T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1684, - "high": 1684, - "low": 1684, - "open": 1684, - "volume": 1684 - }, - "id": 2756330, - "non_hive": { - "close": 227, - "high": 227, - "low": 227, - "open": 227, - "volume": 227 - }, - "open": "2020-10-29T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 4377, - "high": 4377, - "low": 4377, - "open": 4377, - "volume": 4377 - }, - "id": 2756333, - "non_hive": { - "close": 591, - "high": 591, - "low": 591, - "open": 591, - "volume": 591 - }, - "open": "2020-10-29T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 741, - "high": 563, - "low": 741, - "open": 563, - "volume": 4401 - }, - "id": 2756336, - "non_hive": { - "close": 100, - "high": 76, - "low": 100, - "open": 76, - "volume": 594 - }, - "open": "2020-10-30T00:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 22247, - "high": 22247, - "low": 14821, - "open": 14821, - "volume": 37068 - }, - "id": 2756341, - "non_hive": { - "close": 3002, - "high": 3002, - "low": 1999, - "open": 1999, - "volume": 5001 - }, - "open": "2020-10-30T00:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1700, - "high": 10034, - "low": 8285, - "open": 156, - "volume": 78555 - }, - "id": 2756344, - "non_hive": { - "close": 229, - "high": 1354, - "low": 1095, - "open": 21, - "volume": 10501 - }, - "open": "2020-10-30T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 115719, - "high": 7, - "low": 115719, - "open": 103, - "volume": 115829 - }, - "id": 2756351, - "non_hive": { - "close": 15534, - "high": 1, - "low": 15534, - "open": 14, - "volume": 15549 - }, - "open": "2020-10-30T00:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 629, - "high": 629, - "low": 33000, - "open": 33000, - "volume": 108381 - }, - "id": 2756358, - "non_hive": { - "close": 85, - "high": 85, - "low": 4430, - "open": 4430, - "volume": 14605 - }, - "open": "2020-10-30T00:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 268, - "high": 37040, - "low": 268, - "open": 37040, - "volume": 37308 - }, - "id": 2756363, - "non_hive": { - "close": 36, - "high": 5000, - "low": 36, - "open": 5000, - "volume": 5036 - }, - "open": "2020-10-30T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 3314, - "high": 3314, - "low": 3314, - "open": 3314, - "volume": 3314 - }, - "id": 2756369, - "non_hive": { - "close": 446, - "high": 446, - "low": 446, - "open": 446, - "volume": 446 - }, - "open": "2020-10-30T01:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 292, - "high": 37898, - "low": 292, - "open": 37898, - "volume": 38190 - }, - "id": 2756372, - "non_hive": { - "close": 38, - "high": 5099, - "low": 38, - "open": 5099, - "volume": 5137 - }, - "open": "2020-10-30T01:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 61, - "high": 61, - "low": 61, - "open": 61, - "volume": 61 - }, - "id": 2756375, - "non_hive": { - "close": 8, - "high": 8, - "low": 8, - "open": 8, - "volume": 8 - }, - "open": "2020-10-30T01:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 27050, - "high": 38152, - "low": 10000, - "open": 38152, - "volume": 75202 - }, - "id": 2756378, - "non_hive": { - "close": 3651, - "high": 5150, - "low": 1349, - "open": 5150, - "volume": 10150 - }, - "open": "2020-10-30T01:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 33721, - "high": 33721, - "low": 3328, - "open": 3328, - "volume": 37049 - }, - "id": 2756383, - "non_hive": { - "close": 4552, - "high": 4552, - "low": 448, - "open": 448, - "volume": 5000 - }, - "open": "2020-10-30T01:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 11324, - "high": 11324, - "low": 11324, - "open": 11324, - "volume": 11324 - }, - "id": 2756386, - "non_hive": { - "close": 1513, - "high": 1513, - "low": 1513, - "open": 1513, - "volume": 1513 - }, - "open": "2020-10-30T02:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 5221, - "high": 5221, - "low": 8239, - "open": 8239, - "volume": 13460 - }, - "id": 2756390, - "non_hive": { - "close": 705, - "high": 705, - "low": 1112, - "open": 1112, - "volume": 1817 - }, - "open": "2020-10-30T02:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 2140, - "high": 2140, - "low": 11487, - "open": 11487, - "volume": 13627 - }, - "id": 2756393, - "non_hive": { - "close": 289, - "high": 289, - "low": 1551, - "open": 1551, - "volume": 1840 - }, - "open": "2020-10-30T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 13896, - "high": 13896, - "low": 13896, - "open": 13896, - "volume": 13896 - }, - "id": 2756398, - "non_hive": { - "close": 1872, - "high": 1872, - "low": 1872, - "open": 1872, - "volume": 1872 - }, - "open": "2020-10-30T02:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 15815, - "high": 15815, - "low": 15815, - "open": 15815, - "volume": 15815 - }, - "id": 2756401, - "non_hive": { - "close": 2135, - "high": 2135, - "low": 2135, - "open": 2135, - "volume": 2135 - }, - "open": "2020-10-30T03:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1563, - "high": 1563, - "low": 1563, - "open": 1563, - "volume": 1563 - }, - "id": 2756405, - "non_hive": { - "close": 211, - "high": 211, - "low": 211, - "open": 211, - "volume": 211 - }, - "open": "2020-10-30T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 72225, - "high": 72225, - "low": 1792, - "open": 1792, - "volume": 74017 - }, - "id": 2756408, - "non_hive": { - "close": 9748, - "high": 9748, - "low": 241, - "open": 241, - "volume": 9989 - }, - "open": "2020-10-30T03:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 6000 - }, - "id": 2756411, - "non_hive": { - "close": 809, - "high": 809, - "low": 809, - "open": 809, - "volume": 809 - }, - "open": "2020-10-30T03:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 2414, - "high": 2414, - "low": 2414, - "open": 2414, - "volume": 2414 - }, - "id": 2756414, - "non_hive": { - "close": 326, - "high": 326, - "low": 326, - "open": 326, - "volume": 326 - }, - "open": "2020-10-30T04:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 47007, - "high": 47007, - "low": 47007, - "open": 47007, - "volume": 47007 - }, - "id": 2756418, - "non_hive": { - "close": 6346, - "high": 6346, - "low": 6346, - "open": 6346, - "volume": 6346 - }, - "open": "2020-10-30T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6289, - "high": 711, - "low": 5000, - "open": 5000, - "volume": 12000 - }, - "id": 2756421, - "non_hive": { - "close": 848, - "high": 96, - "low": 674, - "open": 674, - "volume": 1618 - }, - "open": "2020-10-30T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 14616, - "high": 185, - "low": 14616, - "open": 1014, - "volume": 1146829 - }, - "id": 2756426, - "non_hive": { - "close": 1900, - "high": 25, - "low": 1900, - "open": 137, - "volume": 150164 - }, - "open": "2020-10-30T04:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 246, - "high": 11492, - "low": 246, - "open": 11492, - "volume": 11738 - }, - "id": 2756431, - "non_hive": { - "close": 32, - "high": 1550, - "low": 32, - "open": 1550, - "volume": 1582 - }, - "open": "2020-10-30T04:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1799, - "high": 1799, - "low": 4028, - "open": 14822, - "volume": 20649 - }, - "id": 2756436, - "non_hive": { - "close": 243, - "high": 243, - "low": 543, - "open": 1999, - "volume": 2785 - }, - "open": "2020-10-30T04:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 622, - "high": 622, - "low": 386, - "open": 761, - "volume": 19196 - }, - "id": 2756441, - "non_hive": { - "close": 84, - "high": 84, - "low": 50, - "open": 102, - "volume": 2538 - }, - "open": "2020-10-30T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 289, - "high": 289, - "low": 289, - "open": 289, - "volume": 289 - }, - "id": 2756447, - "non_hive": { - "close": 39, - "high": 39, - "low": 39, - "open": 39, - "volume": 39 - }, - "open": "2020-10-30T05:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1400, - "high": 12603, - "low": 1400, - "open": 12603, - "volume": 14003 - }, - "id": 2756450, - "non_hive": { - "close": 182, - "high": 1700, - "low": 182, - "open": 1700, - "volume": 1882 - }, - "open": "2020-10-30T05:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 14828, - "high": 14828, - "low": 14828, - "open": 14828, - "volume": 14828 - }, - "id": 2756455, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-30T05:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 13346, - "high": 26586, - "low": 13346, - "open": 26586, - "volume": 66000 - }, - "id": 2756458, - "non_hive": { - "close": 1735, - "high": 3586, - "low": 1735, - "open": 3586, - "volume": 8837 - }, - "open": "2020-10-30T05:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 7416, - "high": 43109, - "low": 7416, - "open": 43109, - "volume": 50525 - }, - "id": 2756461, - "non_hive": { - "close": 1000, - "high": 5813, - "low": 1000, - "open": 5813, - "volume": 6813 - }, - "open": "2020-10-30T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 376, - "high": 17231, - "low": 376, - "open": 17231, - "volume": 17607 - }, - "id": 2756464, - "non_hive": { - "close": 49, - "high": 2321, - "low": 49, - "open": 2321, - "volume": 2370 - }, - "open": "2020-10-30T06:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 11514, - "high": 11514, - "low": 11514, - "open": 11514, - "volume": 11514 - }, - "id": 2756468, - "non_hive": { - "close": 1551, - "high": 1551, - "low": 1551, - "open": 1551, - "volume": 1551 - }, - "open": "2020-10-30T06:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 41638, - "high": 41638, - "low": 41638, - "open": 41638, - "volume": 41638 - }, - "id": 2756471, - "non_hive": { - "close": 5612, - "high": 5612, - "low": 5612, - "open": 5612, - "volume": 5612 - }, - "open": "2020-10-30T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 459, - "high": 4304, - "low": 459, - "open": 2643, - "volume": 42853 - }, - "id": 2756474, - "non_hive": { - "close": 60, - "high": 580, - "low": 60, - "open": 355, - "volume": 5770 - }, - "open": "2020-10-30T06:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 459, - "high": 459, - "low": 459, - "open": 459, - "volume": 459 - }, - "id": 2756479, - "non_hive": { - "close": 61, - "high": 61, - "low": 61, - "open": 61, - "volume": 61 - }, - "open": "2020-10-30T06:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 86563, - "high": 25741, - "low": 86563, - "open": 25741, - "volume": 112304 - }, - "id": 2756482, - "non_hive": { - "close": 11662, - "high": 3468, - "low": 11662, - "open": 3468, - "volume": 15130 - }, - "open": "2020-10-30T06:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 22312, - "high": 22312, - "low": 14962, - "open": 13316, - "volume": 125434 - }, - "id": 2756487, - "non_hive": { - "close": 3006, - "high": 3006, - "low": 1960, - "open": 1794, - "volume": 16731 - }, - "open": "2020-10-30T06:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 160, - "high": 12225, - "low": 160, - "open": 12225, - "volume": 19745 - }, - "id": 2756493, - "non_hive": { - "close": 21, - "high": 1647, - "low": 20, - "open": 1647, - "volume": 2658 - }, - "open": "2020-10-30T06:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 7788, - "high": 7788, - "low": 7788, - "open": 7788, - "volume": 7788 - }, - "id": 2756500, - "non_hive": { - "close": 1049, - "high": 1049, - "low": 1049, - "open": 1049, - "volume": 1049 - }, - "open": "2020-10-30T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 89, - "high": 89, - "low": 10684, - "open": 9659, - "volume": 80748 - }, - "id": 2756503, - "non_hive": { - "close": 12, - "high": 12, - "low": 1438, - "open": 1301, - "volume": 10875 - }, - "open": "2020-10-30T07:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 3022, - "high": 3022, - "low": 3022, - "open": 3022, - "volume": 3022 - }, - "id": 2756510, - "non_hive": { - "close": 407, - "high": 407, - "low": 407, - "open": 407, - "volume": 407 - }, - "open": "2020-10-30T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 112, - "high": 112, - "low": 112, - "open": 112, - "volume": 112 - }, - "id": 2756513, - "non_hive": { - "close": 15, - "high": 15, - "low": 15, - "open": 15, - "volume": 15 - }, - "open": "2020-10-30T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 15196, - "high": 15196, - "low": 71, - "open": 15265, - "volume": 30532 - }, - "id": 2756516, - "non_hive": { - "close": 1991, - "high": 1991, - "low": 9, - "open": 2000, - "volume": 4000 - }, - "open": "2020-10-30T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 3047, - "high": 2103, - "low": 102112, - "open": 2103, - "volume": 571929 - }, - "id": 2756521, - "non_hive": { - "close": 410, - "high": 283, - "low": 13172, - "open": 283, - "volume": 74304 - }, - "open": "2020-10-30T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 56163, - "high": 3947, - "low": 56163, - "open": 3947, - "volume": 78947 - }, - "id": 2756528, - "non_hive": { - "close": 7093, - "high": 531, - "low": 7093, - "open": 531, - "volume": 10054 - }, - "open": "2020-10-30T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 19153, - "high": 15832, - "low": 7867, - "open": 15832, - "volume": 209000 - }, - "id": 2756533, - "non_hive": { - "close": 2394, - "high": 2000, - "low": 983, - "open": 2000, - "volume": 26195 - }, - "open": "2020-10-30T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 37243, - "high": 37243, - "low": 14869, - "open": 14869, - "volume": 52112 - }, - "id": 2756540, - "non_hive": { - "close": 5009, - "high": 5009, - "low": 1999, - "open": 1999, - "volume": 7008 - }, - "open": "2020-10-30T07:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 17809, - "high": 17809, - "low": 17809, - "open": 17809, - "volume": 17809 - }, - "id": 2756543, - "non_hive": { - "close": 2390, - "high": 2390, - "low": 2390, - "open": 2390, - "volume": 2390 - }, - "open": "2020-10-30T07:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 21162, - "high": 21162, - "low": 21162, - "open": 21162, - "volume": 21162 - }, - "id": 2756546, - "non_hive": { - "close": 2840, - "high": 2840, - "low": 2840, - "open": 2840, - "volume": 2840 - }, - "open": "2020-10-30T07:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 701, - "high": 14895, - "low": 701, - "open": 14895, - "volume": 30506 - }, - "id": 2756549, - "non_hive": { - "close": 94, - "high": 1999, - "low": 94, - "open": 1999, - "volume": 4094 - }, - "open": "2020-10-30T07:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 15, - "high": 15, - "low": 15, - "open": 15, - "volume": 15 - }, - "id": 2756553, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-10-30T08:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 4337, - "high": 9321, - "low": 193, - "open": 9321, - "volume": 38325 - }, - "id": 2756557, - "non_hive": { - "close": 582, - "high": 1251, - "low": 25, - "open": 1251, - "volume": 5141 - }, - "open": "2020-10-30T08:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 26942, - "high": 26942, - "low": 14926, - "open": 14926, - "volume": 41868 - }, - "id": 2756563, - "non_hive": { - "close": 3609, - "high": 3609, - "low": 1999, - "open": 1999, - "volume": 5608 - }, - "open": "2020-10-30T08:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 7184, - "high": 7184, - "low": 7184, - "open": 7184, - "volume": 7184 - }, - "id": 2756566, - "non_hive": { - "close": 962, - "high": 962, - "low": 962, - "open": 962, - "volume": 962 - }, - "open": "2020-10-30T08:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 19281, - "high": 15993, - "low": 19281, - "open": 15993, - "volume": 65000 - }, - "id": 2756569, - "non_hive": { - "close": 2410, - "high": 2000, - "low": 2410, - "open": 2000, - "volume": 8127 - }, - "open": "2020-10-30T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 49785, - "high": 15999, - "low": 49785, - "open": 15999, - "volume": 358340 - }, - "id": 2756572, - "non_hive": { - "close": 5974, - "high": 2000, - "low": 5974, - "open": 2000, - "volume": 44468 - }, - "open": "2020-10-30T08:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 815, - "high": 11008, - "low": 815, - "open": 15266, - "volume": 27089 - }, - "id": 2756575, - "non_hive": { - "close": 108, - "high": 1470, - "low": 108, - "open": 2038, - "volume": 3616 - }, - "open": "2020-10-30T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1415, - "high": 9585, - "low": 1415, - "open": 9585, - "volume": 11000 - }, - "id": 2756581, - "non_hive": { - "close": 188, - "high": 1280, - "low": 188, - "open": 1280, - "volume": 1468 - }, - "open": "2020-10-30T09:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 944, - "high": 106119, - "low": 944, - "open": 106119, - "volume": 119734 - }, - "id": 2756584, - "non_hive": { - "close": 125, - "high": 14196, - "low": 125, - "open": 14196, - "volume": 16016 - }, - "open": "2020-10-30T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6435, - "high": 2789, - "low": 6435, - "open": 2789, - "volume": 9224 - }, - "id": 2756589, - "non_hive": { - "close": 859, - "high": 373, - "low": 859, - "open": 373, - "volume": 1232 - }, - "open": "2020-10-30T09:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 14654, - "high": 14654, - "low": 14654, - "open": 14654, - "volume": 14654 - }, - "id": 2756592, - "non_hive": { - "close": 1958, - "high": 1958, - "low": 1958, - "open": 1958, - "volume": 1958 - }, - "open": "2020-10-30T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 42356, - "high": 26796, - "low": 42356, - "open": 26796, - "volume": 73190 - }, - "id": 2756596, - "non_hive": { - "close": 5086, - "high": 3575, - "low": 5086, - "open": 3575, - "volume": 9199 - }, - "open": "2020-10-30T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 21000, - "high": 21000, - "low": 21665, - "open": 21665, - "volume": 42665 - }, - "id": 2756601, - "non_hive": { - "close": 2730, - "high": 2730, - "low": 2814, - "open": 2814, - "volume": 5544 - }, - "open": "2020-10-30T10:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 569, - "high": 569, - "low": 9653, - "open": 347, - "volume": 25963 - }, - "id": 2756606, - "non_hive": { - "close": 74, - "high": 74, - "low": 1159, - "open": 45, - "volume": 3277 - }, - "open": "2020-10-30T10:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 62800, - "high": 17207, - "low": 4644, - "open": 15422, - "volume": 108629 - }, - "id": 2756610, - "non_hive": { - "close": 8139, - "high": 2231, - "low": 601, - "open": 1999, - "volume": 14079 - }, - "open": "2020-10-30T11:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 42928, - "high": 42928, - "low": 42928, - "open": 42928, - "volume": 42928 - }, - "id": 2756617, - "non_hive": { - "close": 5561, - "high": 5561, - "low": 5561, - "open": 5561, - "volume": 5561 - }, - "open": "2020-10-30T11:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3103, - "high": 3103, - "low": 3103, - "open": 3103, - "volume": 3103 - }, - "id": 2756620, - "non_hive": { - "close": 402, - "high": 402, - "low": 402, - "open": 402, - "volume": 402 - }, - "open": "2020-10-30T11:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 11518, - "high": 16721, - "low": 11518, - "open": 16721, - "volume": 28239 - }, - "id": 2756623, - "non_hive": { - "close": 1492, - "high": 2166, - "low": 1492, - "open": 2166, - "volume": 3658 - }, - "open": "2020-10-30T11:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1389, - "high": 1389, - "low": 60460, - "open": 60460, - "volume": 61849 - }, - "id": 2756626, - "non_hive": { - "close": 180, - "high": 180, - "low": 7831, - "open": 7831, - "volume": 8011 - }, - "open": "2020-10-30T11:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 86003, - "high": 86003, - "low": 86003, - "open": 86003, - "volume": 86003 - }, - "id": 2756631, - "non_hive": { - "close": 11132, - "high": 11132, - "low": 11132, - "open": 11132, - "volume": 11132 - }, - "open": "2020-10-30T12:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 42232, - "high": 42232, - "low": 42232, - "open": 42232, - "volume": 42232 - }, - "id": 2756635, - "non_hive": { - "close": 5466, - "high": 5466, - "low": 5466, - "open": 5466, - "volume": 5466 - }, - "open": "2020-10-30T12:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1343, - "high": 22047, - "low": 1343, - "open": 22047, - "volume": 23390 - }, - "id": 2756638, - "non_hive": { - "close": 167, - "high": 2818, - "low": 167, - "open": 2818, - "volume": 2985 - }, - "open": "2020-10-30T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 24187, - "high": 24187, - "low": 24187, - "open": 24187, - "volume": 24187 - }, - "id": 2756641, - "non_hive": { - "close": 3094, - "high": 3094, - "low": 3094, - "open": 3094, - "volume": 3094 - }, - "open": "2020-10-30T13:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 27846, - "high": 27846, - "low": 27846, - "open": 27846, - "volume": 27846 - }, - "id": 2756645, - "non_hive": { - "close": 3562, - "high": 3562, - "low": 3562, - "open": 3562, - "volume": 3562 - }, - "open": "2020-10-30T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 368, - "high": 120, - "low": 120, - "open": 120, - "volume": 488 - }, - "id": 2756648, - "non_hive": { - "close": 46, - "high": 15, - "low": 15, - "open": 15, - "volume": 61 - }, - "open": "2020-10-30T13:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3007, - "high": 3007, - "low": 3007, - "open": 3007, - "volume": 3007 - }, - "id": 2756651, - "non_hive": { - "close": 384, - "high": 384, - "low": 384, - "open": 384, - "volume": 384 - }, - "open": "2020-10-30T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 9885, - "high": 9885, - "low": 1256, - "open": 53354, - "volume": 65318 - }, - "id": 2756654, - "non_hive": { - "close": 1264, - "high": 1264, - "low": 160, - "open": 6812, - "volume": 8341 - }, - "open": "2020-10-30T13:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 493, - "high": 493, - "low": 4000, - "open": 4000, - "volume": 4493 - }, - "id": 2756661, - "non_hive": { - "close": 63, - "high": 63, - "low": 510, - "open": 510, - "volume": 573 - }, - "open": "2020-10-30T13:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 282, - "high": 282, - "low": 282, - "open": 282, - "volume": 282 - }, - "id": 2756665, - "non_hive": { - "close": 36, - "high": 36, - "low": 36, - "open": 36, - "volume": 36 - }, - "open": "2020-10-30T13:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 4811, - "high": 4811, - "low": 4811, - "open": 4811, - "volume": 4811 - }, - "id": 2756668, - "non_hive": { - "close": 614, - "high": 614, - "low": 614, - "open": 614, - "volume": 614 - }, - "open": "2020-10-30T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 46411, - "high": 46411, - "low": 5804, - "open": 5804, - "volume": 52215 - }, - "id": 2756671, - "non_hive": { - "close": 5937, - "high": 5937, - "low": 742, - "open": 742, - "volume": 6679 - }, - "open": "2020-10-30T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 2019, - "high": 2958, - "low": 2019, - "open": 2958, - "volume": 4977 - }, - "id": 2756675, - "non_hive": { - "close": 258, - "high": 378, - "low": 258, - "open": 378, - "volume": 636 - }, - "open": "2020-10-30T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 360, - "high": 360, - "low": 360, - "open": 360, - "volume": 360 - }, - "id": 2756678, - "non_hive": { - "close": 46, - "high": 46, - "low": 46, - "open": 46, - "volume": 46 - }, - "open": "2020-10-30T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 16934, - "high": 16934, - "low": 16934, - "open": 16934, - "volume": 16934 - }, - "id": 2756681, - "non_hive": { - "close": 2162, - "high": 2162, - "low": 2162, - "open": 2162, - "volume": 2162 - }, - "open": "2020-10-30T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16084, - "high": 16084, - "low": 5807, - "open": 5807, - "volume": 21891 - }, - "id": 2756684, - "non_hive": { - "close": 2058, - "high": 2058, - "low": 742, - "open": 742, - "volume": 2800 - }, - "open": "2020-10-30T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 500322, - "high": 500322, - "low": 500322, - "open": 500322, - "volume": 500322 - }, - "id": 2756689, - "non_hive": { - "close": 64000, - "high": 64000, - "low": 64000, - "open": 64000, - "volume": 64000 - }, - "open": "2020-10-30T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1025, - "high": 1025, - "low": 1025, - "open": 1025, - "volume": 1025 - }, - "id": 2756692, - "non_hive": { - "close": 131, - "high": 131, - "low": 131, - "open": 131, - "volume": 131 - }, - "open": "2020-10-30T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 17219, - "high": 2348, - "low": 17219, - "open": 2348, - "volume": 19567 - }, - "id": 2756695, - "non_hive": { - "close": 2200, - "high": 300, - "low": 2200, - "open": 300, - "volume": 2500 - }, - "open": "2020-10-30T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1748, - "high": 62, - "low": 1748, - "open": 5790, - "volume": 7600 - }, - "id": 2756698, - "non_hive": { - "close": 223, - "high": 8, - "low": 223, - "open": 739, - "volume": 970 - }, - "open": "2020-10-30T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 9252, - "high": 9252, - "low": 9252, - "open": 9252, - "volume": 9252 - }, - "id": 2756704, - "non_hive": { - "close": 1180, - "high": 1180, - "low": 1180, - "open": 1180, - "volume": 1180 - }, - "open": "2020-10-30T15:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 78, - "high": 78, - "low": 6891, - "open": 6891, - "volume": 6969 - }, - "id": 2756707, - "non_hive": { - "close": 10, - "high": 10, - "low": 879, - "open": 879, - "volume": 889 - }, - "open": "2020-10-30T15:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "id": 2756710, - "non_hive": { - "close": 640, - "high": 640, - "low": 640, - "open": 640, - "volume": 640 - }, - "open": "2020-10-30T15:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 16001, - "high": 16001, - "low": 16001, - "open": 16001, - "volume": 16001 - }, - "id": 2756713, - "non_hive": { - "close": 2048, - "high": 2048, - "low": 2048, - "open": 2048, - "volume": 2048 - }, - "open": "2020-10-30T15:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 10072, - "high": 3915, - "low": 10072, - "open": 3915, - "volume": 47190 - }, - "id": 2756716, - "non_hive": { - "close": 1241, - "high": 501, - "low": 1241, - "open": 501, - "volume": 5933 - }, - "open": "2020-10-30T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 8782, - "high": 8782, - "low": 8782, - "open": 8782, - "volume": 8782 - }, - "id": 2756719, - "non_hive": { - "close": 1124, - "high": 1124, - "low": 1124, - "open": 1124, - "volume": 1124 - }, - "open": "2020-10-30T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 2469, - "high": 2469, - "low": 2469, - "open": 2469, - "volume": 2469 - }, - "id": 2756722, - "non_hive": { - "close": 316, - "high": 316, - "low": 316, - "open": 316, - "volume": 316 - }, - "open": "2020-10-30T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 84258, - "high": 492, - "low": 52148, - "open": 492, - "volume": 154697 - }, - "id": 2756725, - "non_hive": { - "close": 10704, - "high": 63, - "low": 6523, - "open": 63, - "volume": 19553 - }, - "open": "2020-10-30T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 310016, - "high": 310016, - "low": 310016, - "open": 310016, - "volume": 310016 - }, - "id": 2756735, - "non_hive": { - "close": 39372, - "high": 39372, - "low": 39372, - "open": 39372, - "volume": 39372 - }, - "open": "2020-10-30T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 806451, - "high": 15748, - "low": 806451, - "open": 15748, - "volume": 2162524 - }, - "id": 2756738, - "non_hive": { - "close": 99999, - "high": 2000, - "low": 99999, - "open": 2000, - "volume": 270900 - }, - "open": "2020-10-30T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 9748, - "high": 34252, - "low": 5418, - "open": 5418, - "volume": 65167 - }, - "id": 2756746, - "non_hive": { - "close": 1237, - "high": 4348, - "low": 680, - "open": 680, - "volume": 8264 - }, - "open": "2020-10-30T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 31125, - "high": 1875, - "low": 31125, - "open": 1875, - "volume": 33000 - }, - "id": 2756752, - "non_hive": { - "close": 3909, - "high": 238, - "low": 3909, - "open": 238, - "volume": 4147 - }, - "open": "2020-10-30T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 87259, - "high": 221, - "low": 15741, - "open": 221, - "volume": 103221 - }, - "id": 2756755, - "non_hive": { - "close": 11026, - "high": 28, - "low": 1989, - "open": 28, - "volume": 13043 - }, - "open": "2020-10-30T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1354, - "high": 1354, - "low": 1354, - "open": 1354, - "volume": 1354 - }, - "id": 2756762, - "non_hive": { - "close": 171, - "high": 171, - "low": 171, - "open": 171, - "volume": 171 - }, - "open": "2020-10-30T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 23217, - "high": 4609, - "low": 23217, - "open": 15922, - "volume": 44748 - }, - "id": 2756765, - "non_hive": { - "close": 2878, - "high": 579, - "low": 2878, - "open": 2000, - "volume": 5582 - }, - "open": "2020-10-30T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1250, - "high": 1250, - "low": 1250, - "open": 1250, - "volume": 1250 - }, - "id": 2756768, - "non_hive": { - "close": 153, - "high": 153, - "low": 153, - "open": 153, - "volume": 153 - }, - "open": "2020-10-30T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 14849, - "high": 14849, - "low": 14849, - "open": 14849, - "volume": 14849 - }, - "id": 2756771, - "non_hive": { - "close": 1867, - "high": 1867, - "low": 1867, - "open": 1867, - "volume": 1867 - }, - "open": "2020-10-30T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 101, - "high": 15999, - "low": 101, - "open": 15999, - "volume": 16100 - }, - "id": 2756775, - "non_hive": { - "close": 12, - "high": 2000, - "low": 12, - "open": 2000, - "volume": 2012 - }, - "open": "2020-10-30T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 61114, - "high": 61114, - "low": 6555, - "open": 6555, - "volume": 83457 - }, - "id": 2756778, - "non_hive": { - "close": 7742, - "high": 7742, - "low": 821, - "open": 821, - "volume": 10562 - }, - "open": "2020-10-30T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 27043, - "high": 4336, - "low": 2332, - "open": 4336, - "volume": 33711 - }, - "id": 2756783, - "non_hive": { - "close": 3424, - "high": 549, - "low": 295, - "open": 549, - "volume": 4268 - }, - "open": "2020-10-30T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 379, - "high": 379, - "low": 379, - "open": 379, - "volume": 379 - }, - "id": 2756788, - "non_hive": { - "close": 48, - "high": 48, - "low": 48, - "open": 48, - "volume": 48 - }, - "open": "2020-10-30T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 53066, - "high": 15999, - "low": 53066, - "open": 15999, - "volume": 69065 - }, - "id": 2756791, - "non_hive": { - "close": 6633, - "high": 2000, - "low": 6633, - "open": 2000, - "volume": 8633 - }, - "open": "2020-10-30T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 15998, - "high": 15998, - "low": 15998, - "open": 15998, - "volume": 15998 - }, - "id": 2756794, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-30T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 5222, - "high": 230, - "low": 15998, - "open": 15998, - "volume": 81581 - }, - "id": 2756797, - "non_hive": { - "close": 657, - "high": 29, - "low": 2000, - "open": 2000, - "volume": 10256 - }, - "open": "2020-10-30T17:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 9507, - "high": 9507, - "low": 14480, - "open": 15906, - "volume": 57311 - }, - "id": 2756808, - "non_hive": { - "close": 1196, - "high": 1196, - "low": 1810, - "open": 2001, - "volume": 7196 - }, - "open": "2020-10-30T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 38504, - "high": 2337, - "low": 15907, - "open": 15430, - "volume": 180733 - }, - "id": 2756818, - "non_hive": { - "close": 4911, - "high": 299, - "low": 1999, - "open": 1940, - "volume": 22836 - }, - "open": "2020-10-30T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 11368, - "high": 11368, - "low": 1521, - "open": 1521, - "volume": 12889 - }, - "id": 2756827, - "non_hive": { - "close": 1450, - "high": 1450, - "low": 193, - "open": 193, - "volume": 1643 - }, - "open": "2020-10-30T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1011, - "high": 1011, - "low": 2802, - "open": 20072, - "volume": 27829 - }, - "id": 2756830, - "non_hive": { - "close": 129, - "high": 129, - "low": 357, - "open": 2560, - "volume": 3549 - }, - "open": "2020-10-30T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 79120, - "high": 1522, - "low": 79120, - "open": 1522, - "volume": 205163 - }, - "id": 2756837, - "non_hive": { - "close": 9731, - "high": 194, - "low": 9731, - "open": 194, - "volume": 25473 - }, - "open": "2020-10-30T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3493, - "high": 3493, - "low": 3493, - "open": 3493, - "volume": 3493 - }, - "id": 2756842, - "non_hive": { - "close": 445, - "high": 445, - "low": 445, - "open": 445, - "volume": 445 - }, - "open": "2020-10-30T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 232, - "high": 232, - "low": 232, - "open": 232, - "volume": 232 - }, - "id": 2756845, - "non_hive": { - "close": 29, - "high": 29, - "low": 29, - "open": 29, - "volume": 29 - }, - "open": "2020-10-30T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 855, - "high": 12088, - "low": 855, - "open": 12088, - "volume": 12943 - }, - "id": 2756848, - "non_hive": { - "close": 107, - "high": 1513, - "low": 107, - "open": 1513, - "volume": 1620 - }, - "open": "2020-10-30T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1395, - "high": 1090, - "low": 1395, - "open": 1090, - "volume": 2485 - }, - "id": 2756851, - "non_hive": { - "close": 173, - "high": 136, - "low": 173, - "open": 136, - "volume": 309 - }, - "open": "2020-10-30T18:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 65, - "high": 32, - "low": 65, - "open": 44649, - "volume": 55097 - }, - "id": 2756856, - "non_hive": { - "close": 7, - "high": 4, - "low": 7, - "open": 5567, - "volume": 6868 - }, - "open": "2020-10-30T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 9913, - "high": 9913, - "low": 3968, - "open": 3968, - "volume": 13881 - }, - "id": 2756860, - "non_hive": { - "close": 1262, - "high": 1262, - "low": 505, - "open": 505, - "volume": 1767 - }, - "open": "2020-10-30T19:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 10696, - "high": 10696, - "low": 3707, - "open": 1420, - "volume": 83744 - }, - "id": 2756866, - "non_hive": { - "close": 1368, - "high": 1368, - "low": 472, - "open": 181, - "volume": 10701 - }, - "open": "2020-10-30T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 73501, - "high": 73501, - "low": 73501, - "open": 73501, - "volume": 73501 - }, - "id": 2756875, - "non_hive": { - "close": 9400, - "high": 9400, - "low": 9400, - "open": 9400, - "volume": 9400 - }, - "open": "2020-10-30T19:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12074, - "high": 23459, - "low": 12074, - "open": 23459, - "volume": 35533 - }, - "id": 2756878, - "non_hive": { - "close": 1544, - "high": 3000, - "low": 1544, - "open": 3000, - "volume": 4544 - }, - "open": "2020-10-30T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 39100, - "high": 39100, - "low": 39100, - "open": 39100, - "volume": 39100 - }, - "id": 2756881, - "non_hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "open": "2020-10-30T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 39103, - "high": 39103, - "low": 39103, - "open": 39103, - "volume": 39103 - }, - "id": 2756884, - "non_hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "open": "2020-10-30T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 39100, - "high": 4175, - "low": 19036, - "open": 19036, - "volume": 64993 - }, - "id": 2756887, - "non_hive": { - "close": 5000, - "high": 534, - "low": 2434, - "open": 2434, - "volume": 8311 - }, - "open": "2020-10-30T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6407, - "high": 6407, - "low": 6407, - "open": 6407, - "volume": 6407 - }, - "id": 2756894, - "non_hive": { - "close": 818, - "high": 818, - "low": 818, - "open": 818, - "volume": 818 - }, - "open": "2020-10-30T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 149282, - "high": 149282, - "low": 1167, - "open": 1167, - "volume": 150449 - }, - "id": 2756897, - "non_hive": { - "close": 19060, - "high": 19060, - "low": 149, - "open": 149, - "volume": 19209 - }, - "open": "2020-10-30T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 8288, - "high": 8288, - "low": 8288, - "open": 8288, - "volume": 8288 - }, - "id": 2756901, - "non_hive": { - "close": 1060, - "high": 1060, - "low": 1060, - "open": 1060, - "volume": 1060 - }, - "open": "2020-10-30T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 115001, - "high": 15999, - "low": 115001, - "open": 15999, - "volume": 131000 - }, - "id": 2756905, - "non_hive": { - "close": 14375, - "high": 2000, - "low": 14375, - "open": 2000, - "volume": 16375 - }, - "open": "2020-10-30T20:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 15638, - "high": 15638, - "low": 15638, - "open": 15638, - "volume": 15638 - }, - "id": 2756908, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-30T20:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 582140, - "high": 582140, - "low": 74998, - "open": 74998, - "volume": 657138 - }, - "id": 2756911, - "non_hive": { - "close": 74415, - "high": 74415, - "low": 9586, - "open": 9586, - "volume": 84001 - }, - "open": "2020-10-30T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 26984, - "high": 861, - "low": 26984, - "open": 861, - "volume": 44752 - }, - "id": 2756914, - "non_hive": { - "close": 3373, - "high": 110, - "low": 3373, - "open": 110, - "volume": 5599 - }, - "open": "2020-10-30T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 23344, - "high": 23344, - "low": 23344, - "open": 23344, - "volume": 23344 - }, - "id": 2756917, - "non_hive": { - "close": 2984, - "high": 2984, - "low": 2984, - "open": 2984, - "volume": 2984 - }, - "open": "2020-10-30T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 6762, - "high": 6762, - "low": 6762, - "open": 6762, - "volume": 6762 - }, - "id": 2756921, - "non_hive": { - "close": 864, - "high": 864, - "low": 864, - "open": 864, - "volume": 864 - }, - "open": "2020-10-30T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 9598, - "high": 1104, - "low": 9598, - "open": 1104, - "volume": 10702 - }, - "id": 2756924, - "non_hive": { - "close": 1225, - "high": 141, - "low": 1225, - "open": 141, - "volume": 1366 - }, - "open": "2020-10-30T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 25646, - "high": 25646, - "low": 9631, - "open": 9631, - "volume": 35277 - }, - "id": 2756929, - "non_hive": { - "close": 3278, - "high": 3278, - "low": 1231, - "open": 1231, - "volume": 4509 - }, - "open": "2020-10-30T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 27078, - "high": 27078, - "low": 27078, - "open": 27078, - "volume": 27078 - }, - "id": 2756933, - "non_hive": { - "close": 3461, - "high": 3461, - "low": 3461, - "open": 3461, - "volume": 3461 - }, - "open": "2020-10-30T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 9514, - "high": 9514, - "low": 9514, - "open": 9514, - "volume": 9514 - }, - "id": 2756936, - "non_hive": { - "close": 1216, - "high": 1216, - "low": 1216, - "open": 1216, - "volume": 1216 - }, - "open": "2020-10-30T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 7824, - "high": 7824, - "low": 7824, - "open": 7824, - "volume": 7824 - }, - "id": 2756940, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-30T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1573, - "high": 7011, - "low": 1573, - "open": 7011, - "volume": 8584 - }, - "id": 2756943, - "non_hive": { - "close": 201, - "high": 896, - "low": 201, - "open": 896, - "volume": 1097 - }, - "open": "2020-10-30T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 15053, - "high": 15053, - "low": 15053, - "open": 15053, - "volume": 15053 - }, - "id": 2756946, - "non_hive": { - "close": 1924, - "high": 1924, - "low": 1924, - "open": 1924, - "volume": 1924 - }, - "open": "2020-10-30T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 23473, - "high": 23473, - "low": 23473, - "open": 23473, - "volume": 23473 - }, - "id": 2756949, - "non_hive": { - "close": 3000, - "high": 3000, - "low": 3000, - "open": 3000, - "volume": 3000 - }, - "open": "2020-10-30T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 221555, - "high": 221555, - "low": 79724, - "open": 79724, - "volume": 604940 - }, - "id": 2756953, - "non_hive": { - "close": 28317, - "high": 28317, - "low": 10189, - "open": 10189, - "volume": 77316 - }, - "open": "2020-10-30T23:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 1682, - "high": 1682, - "low": 12800, - "open": 12800, - "volume": 14482 - }, - "id": 2756956, - "non_hive": { - "close": 215, - "high": 215, - "low": 1636, - "open": 1636, - "volume": 1851 - }, - "open": "2020-10-30T23:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6077, - "high": 6077, - "low": 6077, - "open": 6077, - "volume": 6077 - }, - "id": 2756961, - "non_hive": { - "close": 776, - "high": 776, - "low": 776, - "open": 776, - "volume": 776 - }, - "open": "2020-10-30T23:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 26409, - "high": 26409, - "low": 26409, - "open": 26409, - "volume": 26409 - }, - "id": 2756964, - "non_hive": { - "close": 3372, - "high": 3372, - "low": 3372, - "open": 3372, - "volume": 3372 - }, - "open": "2020-10-30T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 4032, - "high": 52252, - "low": 4032, - "open": 52252, - "volume": 81252 - }, - "id": 2756967, - "non_hive": { - "close": 514, - "high": 6672, - "low": 514, - "open": 6672, - "volume": 10374 - }, - "open": "2020-10-31T00:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 399739, - "high": 791, - "low": 399739, - "open": 791, - "volume": 1224000 - }, - "id": 2756974, - "non_hive": { - "close": 49967, - "high": 101, - "low": 49967, - "open": 101, - "volume": 153092 - }, - "open": "2020-10-31T00:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 807970, - "high": 264, - "low": 807970, - "open": 264, - "volume": 845543 - }, - "id": 2756977, - "non_hive": { - "close": 99379, - "high": 33, - "low": 99379, - "open": 33, - "volume": 104002 - }, - "open": "2020-10-31T00:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 62585, - "high": 62585, - "low": 15664, - "open": 15664, - "volume": 78249 - }, - "id": 2756980, - "non_hive": { - "close": 7991, - "high": 7991, - "low": 1999, - "open": 1999, - "volume": 9990 - }, - "open": "2020-10-31T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 39165, - "high": 20001, - "low": 15665, - "open": 15665, - "volume": 74831 - }, - "id": 2756983, - "non_hive": { - "close": 5001, - "high": 2554, - "low": 1999, - "open": 1999, - "volume": 9554 - }, - "open": "2020-10-31T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1235, - "high": 22344, - "low": 1235, - "open": 22344, - "volume": 23579 - }, - "id": 2756990, - "non_hive": { - "close": 157, - "high": 2853, - "low": 157, - "open": 2853, - "volume": 3010 - }, - "open": "2020-10-31T00:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 5173, - "high": 8455, - "low": 5173, - "open": 8455, - "volume": 13628 - }, - "id": 2756995, - "non_hive": { - "close": 660, - "high": 1079, - "low": 660, - "open": 1079, - "volume": 1739 - }, - "open": "2020-10-31T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 913, - "high": 913, - "low": 913, - "open": 913, - "volume": 913 - }, - "id": 2756999, - "non_hive": { - "close": 116, - "high": 116, - "low": 116, - "open": 116, - "volume": 116 - }, - "open": "2020-10-31T01:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 28800, - "high": 28800, - "low": 28800, - "open": 28800, - "volume": 28800 - }, - "id": 2757002, - "non_hive": { - "close": 3677, - "high": 3677, - "low": 3677, - "open": 3677, - "volume": 3677 - }, - "open": "2020-10-31T01:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 3267, - "high": 20208, - "low": 3267, - "open": 20208, - "volume": 67299 - }, - "id": 2757005, - "non_hive": { - "close": 414, - "high": 2580, - "low": 414, - "open": 2580, - "volume": 8579 - }, - "open": "2020-10-31T01:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 722, - "high": 23, - "low": 722, - "open": 8000, - "volume": 8745 - }, - "id": 2757008, - "non_hive": { - "close": 91, - "high": 3, - "low": 91, - "open": 1021, - "volume": 1115 - }, - "open": "2020-10-31T02:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 70582, - "high": 70582, - "low": 7744, - "open": 7744, - "volume": 78326 - }, - "id": 2757014, - "non_hive": { - "close": 9012, - "high": 9012, - "low": 988, - "open": 988, - "volume": 10000 - }, - "open": "2020-10-31T02:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 685, - "high": 685, - "low": 685, - "open": 685, - "volume": 685 - }, - "id": 2757017, - "non_hive": { - "close": 86, - "high": 86, - "low": 86, - "open": 86, - "volume": 86 - }, - "open": "2020-10-31T02:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7, - "high": 7, - "low": 7, - "open": 7, - "volume": 7 - }, - "id": 2757020, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 1, - "volume": 1 - }, - "open": "2020-10-31T02:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 11721, - "high": 16258, - "low": 11721, - "open": 16258, - "volume": 27979 - }, - "id": 2757023, - "non_hive": { - "close": 1441, - "high": 2000, - "low": 1441, - "open": 2000, - "volume": 3441 - }, - "open": "2020-10-31T02:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 351, - "high": 351, - "low": 15960, - "open": 15960, - "volume": 16311 - }, - "id": 2757026, - "non_hive": { - "close": 44, - "high": 44, - "low": 1999, - "open": 1999, - "volume": 2043 - }, - "open": "2020-10-31T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 92770, - "high": 92770, - "low": 298, - "open": 298, - "volume": 240805 - }, - "id": 2757029, - "non_hive": { - "close": 11845, - "high": 11845, - "low": 37, - "open": 37, - "volume": 30639 - }, - "open": "2020-10-31T02:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 16360, - "high": 250, - "low": 44326, - "open": 117237, - "volume": 208589 - }, - "id": 2757033, - "non_hive": { - "close": 2087, - "high": 32, - "low": 5448, - "open": 14968, - "volume": 26274 - }, - "open": "2020-10-31T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 21494, - "high": 10120, - "low": 21494, - "open": 10120, - "volume": 74452 - }, - "id": 2757039, - "non_hive": { - "close": 2742, - "high": 1292, - "low": 2742, - "open": 1292, - "volume": 9503 - }, - "open": "2020-10-31T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 81874, - "high": 124698, - "low": 81874, - "open": 124698, - "volume": 206572 - }, - "id": 2757044, - "non_hive": { - "close": 10452, - "high": 15919, - "low": 10452, - "open": 15919, - "volume": 26371 - }, - "open": "2020-10-31T03:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 18751, - "high": 18751, - "low": 18751, - "open": 18751, - "volume": 18751 - }, - "id": 2757047, - "non_hive": { - "close": 2394, - "high": 2394, - "low": 2394, - "open": 2394, - "volume": 2394 - }, - "open": "2020-10-31T03:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 58284, - "high": 15, - "low": 58284, - "open": 15455, - "volume": 207910 - }, - "id": 2757050, - "non_hive": { - "close": 7164, - "high": 2, - "low": 7164, - "open": 1973, - "volume": 26189 - }, - "open": "2020-10-31T03:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 12490, - "high": 12490, - "low": 12490, - "open": 12490, - "volume": 12490 - }, - "id": 2757055, - "non_hive": { - "close": 1594, - "high": 1594, - "low": 1594, - "open": 1594, - "volume": 1594 - }, - "open": "2020-10-31T03:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 28030, - "high": 28030, - "low": 28030, - "open": 28030, - "volume": 28030 - }, - "id": 2757058, - "non_hive": { - "close": 3577, - "high": 3577, - "low": 3577, - "open": 3577, - "volume": 3577 - }, - "open": "2020-10-31T04:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 15676, - "high": 15676, - "low": 15676, - "open": 15676, - "volume": 15676 - }, - "id": 2757062, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-31T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 27562, - "high": 27562, - "low": 27562, - "open": 27562, - "volume": 27562 - }, - "id": 2757065, - "non_hive": { - "close": 3517, - "high": 3517, - "low": 3517, - "open": 3517, - "volume": 3517 - }, - "open": "2020-10-31T04:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 23346, - "high": 23346, - "low": 4372, - "open": 4372, - "volume": 27718 - }, - "id": 2757068, - "non_hive": { - "close": 2979, - "high": 2979, - "low": 557, - "open": 557, - "volume": 3536 - }, - "open": "2020-10-31T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2335, - "high": 2335, - "low": 4993, - "open": 4993, - "volume": 24107 - }, - "id": 2757071, - "non_hive": { - "close": 298, - "high": 298, - "low": 636, - "open": 636, - "volume": 3075 - }, - "open": "2020-10-31T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 100, - "high": 100, - "low": 100, - "open": 100, - "volume": 100 - }, - "id": 2757077, - "non_hive": { - "close": 12, - "high": 12, - "low": 12, - "open": 12, - "volume": 12 - }, - "open": "2020-10-31T06:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 191502, - "high": 191502, - "low": 191502, - "open": 191502, - "volume": 191502 - }, - "id": 2757081, - "non_hive": { - "close": 24438, - "high": 24438, - "low": 24438, - "open": 24438, - "volume": 24438 - }, - "open": "2020-10-31T06:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 2022, - "high": 16519, - "low": 2022, - "open": 16519, - "volume": 65000 - }, - "id": 2757084, - "non_hive": { - "close": 257, - "high": 2108, - "low": 257, - "open": 2108, - "volume": 8292 - }, - "open": "2020-10-31T06:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 3916, - "high": 3916, - "low": 3916, - "open": 3916, - "volume": 3916 - }, - "id": 2757087, - "non_hive": { - "close": 500, - "high": 500, - "low": 500, - "open": 500, - "volume": 500 - }, - "open": "2020-10-31T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 3493, - "high": 3493, - "low": 3493, - "open": 3493, - "volume": 3493 - }, - "id": 2757090, - "non_hive": { - "close": 446, - "high": 446, - "low": 446, - "open": 446, - "volume": 446 - }, - "open": "2020-10-31T07:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 98692, - "high": 98692, - "low": 98692, - "open": 98692, - "volume": 98692 - }, - "id": 2757094, - "non_hive": { - "close": 12601, - "high": 12601, - "low": 12601, - "open": 12601, - "volume": 12601 - }, - "open": "2020-10-31T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3917, - "high": 3917, - "low": 3917, - "open": 3917, - "volume": 3917 - }, - "id": 2757097, - "non_hive": { - "close": 500, - "high": 500, - "low": 500, - "open": 500, - "volume": 500 - }, - "open": "2020-10-31T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 18446, - "high": 9098, - "low": 18446, - "open": 9098, - "volume": 35658 - }, - "id": 2757100, - "non_hive": { - "close": 2267, - "high": 1161, - "low": 2267, - "open": 1161, - "volume": 4463 - }, - "open": "2020-10-31T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16339, - "high": 16268, - "low": 16339, - "open": 16268, - "volume": 45532 - }, - "id": 2757105, - "non_hive": { - "close": 2008, - "high": 2000, - "low": 2008, - "open": 2000, - "volume": 5597 - }, - "open": "2020-10-31T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 10729, - "high": 10729, - "low": 10729, - "open": 10729, - "volume": 10729 - }, - "id": 2757108, - "non_hive": { - "close": 1318, - "high": 1318, - "low": 1318, - "open": 1318, - "volume": 1318 - }, - "open": "2020-10-31T07:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 156879, - "high": 156879, - "low": 156879, - "open": 156879, - "volume": 156879 - }, - "id": 2757111, - "non_hive": { - "close": 20000, - "high": 20000, - "low": 20000, - "open": 20000, - "volume": 20000 - }, - "open": "2020-10-31T08:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1491, - "high": 4078, - "low": 1491, - "open": 4078, - "volume": 5569 - }, - "id": 2757115, - "non_hive": { - "close": 190, - "high": 520, - "low": 190, - "open": 520, - "volume": 710 - }, - "open": "2020-10-31T08:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 2062, - "high": 2062, - "low": 6224, - "open": 9464, - "volume": 17750 - }, - "id": 2757119, - "non_hive": { - "close": 263, - "high": 263, - "low": 793, - "open": 1206, - "volume": 2262 - }, - "open": "2020-10-31T08:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 38, - "high": 4547, - "low": 38, - "open": 4547, - "volume": 4585 - }, - "id": 2757123, - "non_hive": { - "close": 4, - "high": 569, - "low": 4, - "open": 569, - "volume": 573 - }, - "open": "2020-10-31T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 12255, - "high": 12255, - "low": 12255, - "open": 12255, - "volume": 12255 - }, - "id": 2757127, - "non_hive": { - "close": 1532, - "high": 1532, - "low": 1532, - "open": 1532, - "volume": 1532 - }, - "open": "2020-10-31T09:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 963, - "high": 98775, - "low": 963, - "open": 74993, - "volume": 174731 - }, - "id": 2757130, - "non_hive": { - "close": 118, - "high": 12348, - "low": 118, - "open": 9374, - "volume": 21840 - }, - "open": "2020-10-31T09:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1054, - "high": 1054, - "low": 1054, - "open": 1054, - "volume": 1054 - }, - "id": 2757133, - "non_hive": { - "close": 132, - "high": 132, - "low": 132, - "open": 132, - "volume": 132 - }, - "open": "2020-10-31T09:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 26581, - "high": 496, - "low": 26581, - "open": 496, - "volume": 426581 - }, - "id": 2757136, - "non_hive": { - "close": 3216, - "high": 61, - "low": 3216, - "open": 61, - "volume": 52113 - }, - "open": "2020-10-31T09:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 5308, - "high": 16392, - "low": 5308, - "open": 16392, - "volume": 21700 - }, - "id": 2757139, - "non_hive": { - "close": 647, - "high": 2000, - "low": 647, - "open": 2000, - "volume": 2647 - }, - "open": "2020-10-31T09:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1709, - "high": 1709, - "low": 403, - "open": 24180, - "volume": 26292 - }, - "id": 2757142, - "non_hive": { - "close": 214, - "high": 214, - "low": 50, - "open": 3027, - "volume": 3291 - }, - "open": "2020-10-31T09:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 110233, - "high": 1461, - "low": 110233, - "open": 1461, - "volume": 155818 - }, - "id": 2757147, - "non_hive": { - "close": 13448, - "high": 183, - "low": 13448, - "open": 183, - "volume": 19025 - }, - "open": "2020-10-31T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 65544, - "high": 6037, - "low": 65544, - "open": 15967, - "volume": 225408 - }, - "id": 2757152, - "non_hive": { - "close": 7865, - "high": 756, - "low": 7865, - "open": 1999, - "volume": 27308 - }, - "open": "2020-10-31T10:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 41966, - "high": 2525, - "low": 14535, - "open": 2525, - "volume": 119483 - }, - "id": 2757159, - "non_hive": { - "close": 4994, - "high": 316, - "low": 1729, - "open": 316, - "volume": 14294 - }, - "open": "2020-10-31T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 10928, - "high": 10928, - "low": 15978, - "open": 15978, - "volume": 26906 - }, - "id": 2757164, - "non_hive": { - "close": 1368, - "high": 1368, - "low": 1999, - "open": 1999, - "volume": 3367 - }, - "open": "2020-10-31T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 16386, - "high": 16386, - "low": 16386, - "open": 16386, - "volume": 16386 - }, - "id": 2757169, - "non_hive": { - "close": 2050, - "high": 2050, - "low": 2050, - "open": 2050, - "volume": 2050 - }, - "open": "2020-10-31T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 79759, - "high": 15002, - "low": 79759, - "open": 15002, - "volume": 94761 - }, - "id": 2757172, - "non_hive": { - "close": 9978, - "high": 1877, - "low": 9978, - "open": 1877, - "volume": 11855 - }, - "open": "2020-10-31T10:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 34033, - "high": 175, - "low": 34033, - "open": 175, - "volume": 74998 - }, - "id": 2757175, - "non_hive": { - "close": 3934, - "high": 22, - "low": 3934, - "open": 22, - "volume": 8771 - }, - "open": "2020-10-31T10:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 5705, - "high": 5705, - "low": 5705, - "open": 5705, - "volume": 5705 - }, - "id": 2757178, - "non_hive": { - "close": 713, - "high": 713, - "low": 713, - "open": 713, - "volume": 713 - }, - "open": "2020-10-31T11:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 8909, - "high": 13992, - "low": 16018, - "open": 16018, - "volume": 38919 - }, - "id": 2757182, - "non_hive": { - "close": 1112, - "high": 1747, - "low": 1999, - "open": 1999, - "volume": 4858 - }, - "open": "2020-10-31T11:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 20526, - "high": 20526, - "low": 16018, - "open": 16018, - "volume": 36544 - }, - "id": 2757186, - "non_hive": { - "close": 2563, - "high": 2563, - "low": 1999, - "open": 1999, - "volume": 4562 - }, - "open": "2020-10-31T11:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 10000, - "high": 10000, - "low": 10000, - "open": 10000, - "volume": 10000 - }, - "id": 2757189, - "non_hive": { - "close": 1181, - "high": 1181, - "low": 1181, - "open": 1181, - "volume": 1181 - }, - "open": "2020-10-31T11:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 8807, - "high": 8807, - "low": 3656, - "open": 3656, - "volume": 12463 - }, - "id": 2757192, - "non_hive": { - "close": 1100, - "high": 1100, - "low": 456, - "open": 456, - "volume": 1556 - }, - "open": "2020-10-31T12:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 22317, - "high": 30233, - "low": 22317, - "open": 16001, - "volume": 131725 - }, - "id": 2757198, - "non_hive": { - "close": 2788, - "high": 3778, - "low": 2788, - "open": 1999, - "volume": 16459 - }, - "open": "2020-10-31T12:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 13000, - "high": 13000, - "low": 13000, - "open": 13000, - "volume": 13000 - }, - "id": 2757204, - "non_hive": { - "close": 1623, - "high": 1623, - "low": 1623, - "open": 1623, - "volume": 1623 - }, - "open": "2020-10-31T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 4213, - "high": 4213, - "low": 4213, - "open": 4213, - "volume": 4213 - }, - "id": 2757207, - "non_hive": { - "close": 526, - "high": 526, - "low": 526, - "open": 526, - "volume": 526 - }, - "open": "2020-10-31T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 216, - "high": 216, - "low": 216, - "open": 216, - "volume": 216 - }, - "id": 2757211, - "non_hive": { - "close": 27, - "high": 27, - "low": 27, - "open": 27, - "volume": 27 - }, - "open": "2020-10-31T13:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 33712, - "high": 33712, - "low": 33712, - "open": 33712, - "volume": 33712 - }, - "id": 2757214, - "non_hive": { - "close": 4214, - "high": 4214, - "low": 4214, - "open": 4214, - "volume": 4214 - }, - "open": "2020-10-31T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 3742, - "high": 5905, - "low": 3742, - "open": 5905, - "volume": 21522 - }, - "id": 2757217, - "non_hive": { - "close": 467, - "high": 738, - "low": 467, - "open": 738, - "volume": 2687 - }, - "open": "2020-10-31T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 7002, - "high": 7002, - "low": 56126, - "open": 56126, - "volume": 221135 - }, - "id": 2757220, - "non_hive": { - "close": 875, - "high": 875, - "low": 7000, - "open": 7000, - "volume": 27619 - }, - "open": "2020-10-31T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 12372, - "high": 12500, - "low": 12372, - "open": 12500, - "volume": 24872 - }, - "id": 2757228, - "non_hive": { - "close": 1546, - "high": 1562, - "low": 1546, - "open": 1562, - "volume": 3108 - }, - "open": "2020-10-31T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 44951, - "high": 14085, - "low": 6800, - "open": 14085, - "volume": 234422 - }, - "id": 2757232, - "non_hive": { - "close": 5574, - "high": 1760, - "low": 818, - "open": 1760, - "volume": 29065 - }, - "open": "2020-10-31T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 91676, - "high": 91676, - "low": 12322, - "open": 12322, - "volume": 103998 - }, - "id": 2757240, - "non_hive": { - "close": 11454, - "high": 11454, - "low": 1539, - "open": 1539, - "volume": 12993 - }, - "open": "2020-10-31T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 96995, - "high": 96995, - "low": 96995, - "open": 96995, - "volume": 96995 - }, - "id": 2757243, - "non_hive": { - "close": 11979, - "high": 11979, - "low": 11979, - "open": 11979, - "volume": 11979 - }, - "open": "2020-10-31T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 510446, - "high": 200996, - "low": 1825, - "open": 194000, - "volume": 1047869 - }, - "id": 2757246, - "non_hive": { - "close": 63337, - "high": 24940, - "low": 225, - "open": 23959, - "volume": 129892 - }, - "open": "2020-10-31T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 4561, - "high": 4561, - "low": 4561, - "open": 4561, - "volume": 4561 - }, - "id": 2757256, - "non_hive": { - "close": 570, - "high": 570, - "low": 570, - "open": 570, - "volume": 570 - }, - "open": "2020-10-31T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 9179, - "high": 9179, - "low": 9179, - "open": 9179, - "volume": 9179 - }, - "id": 2757260, - "non_hive": { - "close": 1147, - "high": 1147, - "low": 1147, - "open": 1147, - "volume": 1147 - }, - "open": "2020-10-31T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 111996, - "high": 111996, - "low": 35921, - "open": 35921, - "volume": 503982 - }, - "id": 2757263, - "non_hive": { - "close": 13999, - "high": 13999, - "low": 4489, - "open": 4489, - "volume": 62993 - }, - "open": "2020-10-31T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 199992, - "high": 199992, - "low": 199992, - "open": 199992, - "volume": 199992 - }, - "id": 2757270, - "non_hive": { - "close": 24998, - "high": 24998, - "low": 24998, - "open": 24998, - "volume": 24998 - }, - "open": "2020-10-31T15:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 29251, - "high": 29251, - "low": 29251, - "open": 29251, - "volume": 29251 - }, - "id": 2757273, - "non_hive": { - "close": 3743, - "high": 3743, - "low": 3743, - "open": 3743, - "volume": 3743 - }, - "open": "2020-10-31T15:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 56712, - "high": 56712, - "low": 13325, - "open": 12996, - "volume": 83033 - }, - "id": 2757276, - "non_hive": { - "close": 7258, - "high": 7258, - "low": 1705, - "open": 1663, - "volume": 10626 - }, - "open": "2020-10-31T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 19730, - "high": 19730, - "low": 19730, - "open": 19730, - "volume": 19730 - }, - "id": 2757281, - "non_hive": { - "close": 2525, - "high": 2525, - "low": 2525, - "open": 2525, - "volume": 2525 - }, - "open": "2020-10-31T15:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 11564, - "high": 11564, - "low": 11564, - "open": 11564, - "volume": 11564 - }, - "id": 2757284, - "non_hive": { - "close": 1480, - "high": 1480, - "low": 1480, - "open": 1480, - "volume": 1480 - }, - "open": "2020-10-31T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 906, - "high": 906, - "low": 6945, - "open": 55, - "volume": 7906 - }, - "id": 2757287, - "non_hive": { - "close": 116, - "high": 116, - "low": 883, - "open": 7, - "volume": 1006 - }, - "open": "2020-10-31T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 106298, - "high": 106298, - "low": 13146, - "open": 13146, - "volume": 119444 - }, - "id": 2757292, - "non_hive": { - "close": 13585, - "high": 13585, - "low": 1680, - "open": 1680, - "volume": 15265 - }, - "open": "2020-10-31T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 70, - "high": 70, - "low": 70, - "open": 70, - "volume": 70 - }, - "id": 2757298, - "non_hive": { - "close": 9, - "high": 9, - "low": 9, - "open": 9, - "volume": 9 - }, - "open": "2020-10-31T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 44577, - "high": 44577, - "low": 44577, - "open": 44577, - "volume": 44577 - }, - "id": 2757301, - "non_hive": { - "close": 5692, - "high": 5692, - "low": 5692, - "open": 5692, - "volume": 5692 - }, - "open": "2020-10-31T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 20454, - "high": 15934, - "low": 20454, - "open": 15934, - "volume": 36388 - }, - "id": 2757304, - "non_hive": { - "close": 2567, - "high": 2000, - "low": 2567, - "open": 2000, - "volume": 4567 - }, - "open": "2020-10-31T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 18176, - "high": 1059, - "low": 18176, - "open": 1059, - "volume": 19235 - }, - "id": 2757307, - "non_hive": { - "close": 2317, - "high": 135, - "low": 2317, - "open": 135, - "volume": 2452 - }, - "open": "2020-10-31T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1489, - "high": 44831, - "low": 1489, - "open": 44831, - "volume": 50590 - }, - "id": 2757310, - "non_hive": { - "close": 189, - "high": 5712, - "low": 189, - "open": 5712, - "volume": 6443 - }, - "open": "2020-10-31T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 200991, - "high": 2653, - "low": 200991, - "open": 2653, - "volume": 699143 - }, - "id": 2757315, - "non_hive": { - "close": 24937, - "high": 333, - "low": 24937, - "open": 333, - "volume": 86761 - }, - "open": "2020-10-31T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 4944, - "high": 4944, - "low": 16120, - "open": 16120, - "volume": 337804 - }, - "id": 2757325, - "non_hive": { - "close": 628, - "high": 628, - "low": 1999, - "open": 1999, - "volume": 41924 - }, - "open": "2020-10-31T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 10800, - "high": 10800, - "low": 10800, - "open": 10800, - "volume": 10800 - }, - "id": 2757331, - "non_hive": { - "close": 1371, - "high": 1371, - "low": 1371, - "open": 1371, - "volume": 1371 - }, - "open": "2020-10-31T17:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 17066, - "high": 2197, - "low": 12000, - "open": 12190, - "volume": 43453 - }, - "id": 2757334, - "non_hive": { - "close": 2166, - "high": 279, - "low": 1523, - "open": 1548, - "volume": 5516 - }, - "open": "2020-10-31T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 20447, - "high": 20447, - "low": 20447, - "open": 20447, - "volume": 20447 - }, - "id": 2757341, - "non_hive": { - "close": 2595, - "high": 2595, - "low": 2595, - "open": 2595, - "volume": 2595 - }, - "open": "2020-10-31T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 410, - "high": 410, - "low": 410, - "open": 410, - "volume": 410 - }, - "id": 2757344, - "non_hive": { - "close": 52, - "high": 52, - "low": 52, - "open": 52, - "volume": 52 - }, - "open": "2020-10-31T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 23891, - "high": 23891, - "low": 23891, - "open": 23891, - "volume": 23891 - }, - "id": 2757347, - "non_hive": { - "close": 3032, - "high": 3032, - "low": 3032, - "open": 3032, - "volume": 3032 - }, - "open": "2020-10-31T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1079, - "high": 1079, - "low": 1079, - "open": 1079, - "volume": 1079 - }, - "id": 2757350, - "non_hive": { - "close": 137, - "high": 137, - "low": 137, - "open": 137, - "volume": 137 - }, - "open": "2020-10-31T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 19439, - "high": 275, - "low": 19439, - "open": 103593, - "volume": 123307 - }, - "id": 2757353, - "non_hive": { - "close": 2467, - "high": 35, - "low": 2467, - "open": 13147, - "volume": 15649 - }, - "open": "2020-10-31T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 4439, - "high": 1001, - "low": 8730, - "open": 1001, - "volume": 23239 - }, - "id": 2757358, - "non_hive": { - "close": 563, - "high": 127, - "low": 1086, - "open": 127, - "volume": 2926 - }, - "open": "2020-10-31T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13043, - "high": 6292, - "low": 13043, - "open": 6292, - "volume": 31919 - }, - "id": 2757366, - "non_hive": { - "close": 1654, - "high": 798, - "low": 1654, - "open": 798, - "volume": 4048 - }, - "open": "2020-10-31T17:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1057, - "high": 1057, - "low": 1057, - "open": 1057, - "volume": 1057 - }, - "id": 2757373, - "non_hive": { - "close": 134, - "high": 134, - "low": 134, - "open": 134, - "volume": 134 - }, - "open": "2020-10-31T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2840, - "high": 128000, - "low": 7166, - "open": 128000, - "volume": 145172 - }, - "id": 2757377, - "non_hive": { - "close": 355, - "high": 16000, - "low": 891, - "open": 16000, - "volume": 18141 - }, - "open": "2020-10-31T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 13961, - "high": 686, - "low": 977, - "open": 143993, - "volume": 378922 - }, - "id": 2757383, - "non_hive": { - "close": 1745, - "high": 87, - "low": 122, - "open": 17999, - "volume": 47366 - }, - "open": "2020-10-31T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 119998, - "high": 184, - "low": 119998, - "open": 38035, - "volume": 580996 - }, - "id": 2757392, - "non_hive": { - "close": 14888, - "high": 23, - "low": 14888, - "open": 4754, - "volume": 72147 - }, - "open": "2020-10-31T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 200986, - "high": 200994, - "low": 4990, - "open": 200994, - "volume": 534966 - }, - "id": 2757397, - "non_hive": { - "close": 24936, - "high": 24937, - "low": 619, - "open": 24937, - "volume": 66372 - }, - "open": "2020-10-31T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 41963, - "high": 41963, - "low": 54059, - "open": 54059, - "volume": 96022 - }, - "id": 2757405, - "non_hive": { - "close": 5245, - "high": 5245, - "low": 6706, - "open": 6706, - "volume": 11951 - }, - "open": "2020-10-31T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 120000, - "high": 184, - "low": 6041, - "open": 6041, - "volume": 328337 - }, - "id": 2757408, - "non_hive": { - "close": 15000, - "high": 23, - "low": 749, - "open": 749, - "volume": 41036 - }, - "open": "2020-10-31T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1144, - "high": 1144, - "low": 1144, - "open": 1144, - "volume": 1144 - }, - "id": 2757417, - "non_hive": { - "close": 145, - "high": 145, - "low": 145, - "open": 145, - "volume": 145 - }, - "open": "2020-10-31T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 44266, - "high": 44266, - "low": 7000, - "open": 7000, - "volume": 51266 - }, - "id": 2757420, - "non_hive": { - "close": 5607, - "high": 5607, - "low": 886, - "open": 886, - "volume": 6493 - }, - "open": "2020-10-31T18:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 200000, - "high": 200000, - "low": 200000, - "open": 200000, - "volume": 200000 - }, - "id": 2757423, - "non_hive": { - "close": 25000, - "high": 25000, - "low": 25000, - "open": 25000, - "volume": 25000 - }, - "open": "2020-10-31T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 76009, - "high": 15999, - "low": 76009, - "open": 72000, - "volume": 500000 - }, - "id": 2757426, - "non_hive": { - "close": 9501, - "high": 2000, - "low": 9501, - "open": 9000, - "volume": 62500 - }, - "open": "2020-10-31T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 56009, - "high": 15999, - "low": 56009, - "open": 15999, - "volume": 100000 - }, - "id": 2757435, - "non_hive": { - "close": 7001, - "high": 2000, - "low": 7001, - "open": 2000, - "volume": 12500 - }, - "open": "2020-10-31T19:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 50687, - "high": 50687, - "low": 15771, - "open": 15771, - "volume": 66458 - }, - "id": 2757439, - "non_hive": { - "close": 6428, - "high": 6428, - "low": 1999, - "open": 1999, - "volume": 8427 - }, - "open": "2020-10-31T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 7156, - "high": 12859, - "low": 50399, - "open": 12859, - "volume": 3220015 - }, - "id": 2757442, - "non_hive": { - "close": 907, - "high": 1630, - "low": 6299, - "open": 1630, - "volume": 402622 - }, - "open": "2020-10-31T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 151992, - "high": 15999, - "low": 151992, - "open": 15999, - "volume": 10365504 - }, - "id": 2757453, - "non_hive": { - "close": 18923, - "high": 2000, - "low": 18923, - "open": 2000, - "volume": 1295581 - }, - "open": "2020-10-31T19:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 5322, - "high": 5322, - "low": 15772, - "open": 15772, - "volume": 21094 - }, - "id": 2757459, - "non_hive": { - "close": 675, - "high": 675, - "low": 1999, - "open": 1999, - "volume": 2674 - }, - "open": "2020-10-31T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 71993, - "high": 72000, - "low": 7599999, - "open": 16119, - "volume": 7991210 - }, - "id": 2757462, - "non_hive": { - "close": 8999, - "high": 9000, - "low": 927283, - "open": 2000, - "volume": 975944 - }, - "open": "2020-10-31T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12000, - "high": 14003, - "low": 12000, - "open": 14003, - "volume": 26003 - }, - "id": 2757470, - "non_hive": { - "close": 1499, - "high": 1750, - "low": 1499, - "open": 1750, - "volume": 3249 - }, - "open": "2020-10-31T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 111997, - "high": 70325, - "low": 111997, - "open": 70325, - "volume": 182322 - }, - "id": 2757474, - "non_hive": { - "close": 13989, - "high": 8784, - "low": 13989, - "open": 8784, - "volume": 22773 - }, - "open": "2020-10-31T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7308, - "high": 16128, - "low": 16129, - "open": 16128, - "volume": 327760 - }, - "id": 2757479, - "non_hive": { - "close": 906, - "high": 2000, - "low": 1999, - "open": 2000, - "volume": 40637 - }, - "open": "2020-10-31T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 95999, - "high": 95999, - "low": 95999, - "open": 95999, - "volume": 95999 - }, - "id": 2757491, - "non_hive": { - "close": 11998, - "high": 11998, - "low": 11998, - "open": 11998, - "volume": 11998 - }, - "open": "2020-10-31T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 184398, - "high": 184398, - "low": 15599, - "open": 15599, - "volume": 199997 - }, - "id": 2757494, - "non_hive": { - "close": 23046, - "high": 23046, - "low": 1949, - "open": 1949, - "volume": 24995 - }, - "open": "2020-10-31T20:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 15606, - "high": 16048, - "low": 2000, - "open": 2000, - "volume": 33654 - }, - "id": 2757498, - "non_hive": { - "close": 1977, - "high": 2033, - "low": 250, - "open": 250, - "volume": 4260 - }, - "open": "2020-10-31T20:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 8002, - "high": 8002, - "low": 8002, - "open": 8002, - "volume": 8002 - }, - "id": 2757503, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-10-31T20:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 8629, - "high": 15999, - "low": 8629, - "open": 15999, - "volume": 48016 - }, - "id": 2757506, - "non_hive": { - "close": 1078, - "high": 2000, - "low": 1078, - "open": 2000, - "volume": 6001 - }, - "open": "2020-10-31T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2001, - "high": 2001, - "low": 2001, - "open": 2001, - "volume": 2001 - }, - "id": 2757510, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 250 - }, - "open": "2020-10-31T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 24101, - "high": 15999, - "low": 24101, - "open": 15999, - "volume": 175000 - }, - "id": 2757513, - "non_hive": { - "close": 2988, - "high": 2000, - "low": 2988, - "open": 2000, - "volume": 21736 - }, - "open": "2020-10-31T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 6162, - "high": 6162, - "low": 15874, - "open": 15874, - "volume": 22036 - }, - "id": 2757518, - "non_hive": { - "close": 776, - "high": 776, - "low": 1999, - "open": 1999, - "volume": 2775 - }, - "open": "2020-10-31T20:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 5000, - "open": 5000, - "volume": 15712 - }, - "id": 2757523, - "non_hive": { - "close": 126, - "high": 126, - "low": 620, - "open": 620, - "volume": 1969 - }, - "open": "2020-10-31T20:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1015, - "high": 1015, - "low": 1015, - "open": 1015, - "volume": 1015 - }, - "id": 2757527, - "non_hive": { - "close": 126, - "high": 126, - "low": 126, - "open": 126, - "volume": 126 - }, - "open": "2020-10-31T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 161758, - "high": 16115, - "low": 161758, - "open": 17986, - "volume": 345766 - }, - "id": 2757531, - "non_hive": { - "close": 20058, - "high": 2000, - "low": 20058, - "open": 2232, - "volume": 42893 - }, - "open": "2020-10-31T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 4125, - "high": 784, - "low": 16117, - "open": 16117, - "volume": 53260 - }, - "id": 2757538, - "non_hive": { - "close": 512, - "high": 98, - "low": 2000, - "open": 2000, - "volume": 6610 - }, - "open": "2020-10-31T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 7479, - "high": 7479, - "low": 7479, - "open": 7479, - "volume": 14958 - }, - "id": 2757548, - "non_hive": { - "close": 928, - "high": 930, - "low": 928, - "open": 930, - "volume": 1858 - }, - "open": "2020-10-31T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 10000, - "high": 10000, - "low": 581, - "open": 581, - "volume": 10581 - }, - "id": 2757553, - "non_hive": { - "close": 1249, - "high": 1249, - "low": 72, - "open": 72, - "volume": 1321 - }, - "open": "2020-10-31T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 4779, - "high": 4779, - "low": 5221, - "open": 5221, - "volume": 10000 - }, - "id": 2757557, - "non_hive": { - "close": 597, - "high": 597, - "low": 652, - "open": 652, - "volume": 1249 - }, - "open": "2020-10-31T21:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2932, - "high": 76004, - "low": 8011, - "open": 8011, - "volume": 102960 - }, - "id": 2757561, - "non_hive": { - "close": 366, - "high": 9493, - "low": 1000, - "open": 1000, - "volume": 12858 - }, - "open": "2020-10-31T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 16152, - "high": 16152, - "low": 16152, - "open": 16152, - "volume": 16152 - }, - "id": 2757568, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-10-31T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 2165, - "high": 15332, - "low": 2165, - "open": 15332, - "volume": 17497 - }, - "id": 2757571, - "non_hive": { - "close": 268, - "high": 1900, - "low": 268, - "open": 1900, - "volume": 2168 - }, - "open": "2020-10-31T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 297, - "high": 297, - "low": 297, - "open": 297, - "volume": 297 - }, - "id": 2757575, - "non_hive": { - "close": 37, - "high": 37, - "low": 37, - "open": 37, - "volume": 37 - }, - "open": "2020-10-31T22:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1683, - "high": 13317, - "low": 1683, - "open": 13317, - "volume": 22631 - }, - "id": 2757579, - "non_hive": { - "close": 202, - "high": 1623, - "low": 202, - "open": 1623, - "volume": 2755 - }, - "open": "2020-10-31T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 6000 - }, - "id": 2757584, - "non_hive": { - "close": 747, - "high": 747, - "low": 747, - "open": 747, - "volume": 747 - }, - "open": "2020-10-31T22:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 45423, - "high": 45423, - "low": 353, - "open": 16005, - "volume": 101605 - }, - "id": 2757587, - "non_hive": { - "close": 5749, - "high": 5749, - "low": 44, - "open": 1999, - "volume": 12793 - }, - "open": "2020-10-31T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 39613, - "high": 39613, - "low": 39613, - "open": 39613, - "volume": 39613 - }, - "id": 2757592, - "non_hive": { - "close": 5001, - "high": 5001, - "low": 5001, - "open": 5001, - "volume": 5001 - }, - "open": "2020-10-31T22:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 59747, - "high": 59747, - "low": 59747, - "open": 59747, - "volume": 59747 - }, - "id": 2757595, - "non_hive": { - "close": 7539, - "high": 7539, - "low": 7539, - "open": 7539, - "volume": 7539 - }, - "open": "2020-10-31T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2872, - "high": 2872, - "low": 37147, - "open": 16524, - "volume": 56543 - }, - "id": 2757598, - "non_hive": { - "close": 362, - "high": 362, - "low": 4495, - "open": 2000, - "volume": 6857 - }, - "open": "2020-10-31T22:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 65195, - "high": 65195, - "low": 15861, - "open": 15861, - "volume": 1023678 - }, - "id": 2757603, - "non_hive": { - "close": 8240, - "high": 8240, - "low": 1999, - "open": 1999, - "volume": 129105 - }, - "open": "2020-10-31T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 23828, - "high": 23828, - "low": 23828, - "open": 23828, - "volume": 23828 - }, - "id": 2757607, - "non_hive": { - "close": 3000, - "high": 3000, - "low": 3000, - "open": 3000, - "volume": 3000 - }, - "open": "2020-10-31T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 8500, - "high": 8500, - "low": 8500, - "open": 8500, - "volume": 8500 - }, - "id": 2757610, - "non_hive": { - "close": 1045, - "high": 1045, - "low": 1045, - "open": 1045, - "volume": 1045 - }, - "open": "2020-10-31T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1509, - "high": 16067, - "low": 1509, - "open": 16067, - "volume": 65777 - }, - "id": 2757613, - "non_hive": { - "close": 187, - "high": 1999, - "low": 187, - "open": 1999, - "volume": 8183 - }, - "open": "2020-10-31T23:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 40002, - "high": 40002, - "low": 40002, - "open": 40002, - "volume": 40002 - }, - "id": 2757621, - "non_hive": { - "close": 5040, - "high": 5040, - "low": 5040, - "open": 5040, - "volume": 5040 - }, - "open": "2020-10-31T23:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 15999, - "high": 15999, - "low": 15999, - "open": 15999, - "volume": 47997 - }, - "id": 2757624, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 6000 - }, - "open": "2020-10-31T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 15998, - "high": 548, - "low": 126002, - "open": 548, - "volume": 158546 - }, - "id": 2757629, - "non_hive": { - "close": 2000, - "high": 69, - "low": 15750, - "open": 69, - "volume": 19819 - }, - "open": "2020-10-31T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 16000, - "high": 15998, - "low": 16000, - "open": 15998, - "volume": 56501 - }, - "id": 2757635, - "non_hive": { - "close": 1999, - "high": 2000, - "low": 1999, - "open": 2000, - "volume": 7061 - }, - "open": "2020-10-31T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 16257, - "high": 16007, - "low": 16257, - "open": 14597, - "volume": 1508560 - }, - "id": 2757641, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1810, - "volume": 186935 - }, - "open": "2020-10-31T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 266758, - "high": 15702, - "low": 16018, - "open": 15702, - "volume": 594112 - }, - "id": 2757652, - "non_hive": { - "close": 33313, - "high": 1961, - "low": 1999, - "open": 1961, - "volume": 74185 - }, - "open": "2020-11-01T00:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 14590, - "high": 14590, - "low": 14590, - "open": 14590, - "volume": 14590 - }, - "id": 2757659, - "non_hive": { - "close": 1822, - "high": 1822, - "low": 1822, - "open": 1822, - "volume": 1822 - }, - "open": "2020-11-01T00:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 10529, - "high": 10529, - "low": 1305, - "open": 1305, - "volume": 11834 - }, - "id": 2757662, - "non_hive": { - "close": 1326, - "high": 1326, - "low": 163, - "open": 163, - "volume": 1489 - }, - "open": "2020-11-01T00:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 94997, - "high": 94997, - "low": 5345, - "open": 5345, - "volume": 100342 - }, - "id": 2757667, - "non_hive": { - "close": 11969, - "high": 11969, - "low": 673, - "open": 673, - "volume": 12642 - }, - "open": "2020-11-01T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 4993, - "high": 4993, - "low": 4993, - "open": 4993, - "volume": 4993 - }, - "id": 2757672, - "non_hive": { - "close": 629, - "high": 629, - "low": 629, - "open": 629, - "volume": 629 - }, - "open": "2020-11-01T00:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16593, - "high": 16593, - "low": 16593, - "open": 16593, - "volume": 16593 - }, - "id": 2757675, - "non_hive": { - "close": 2097, - "high": 2097, - "low": 2097, - "open": 2097, - "volume": 2097 - }, - "open": "2020-11-01T00:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 20000, - "high": 3301, - "low": 20000, - "open": 3301, - "volume": 23301 - }, - "id": 2757678, - "non_hive": { - "close": 2526, - "high": 417, - "low": 2526, - "open": 417, - "volume": 2943 - }, - "open": "2020-11-01T00:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11860, - "high": 11860, - "low": 11860, - "open": 11860, - "volume": 11860 - }, - "id": 2757683, - "non_hive": { - "close": 1498, - "high": 1498, - "low": 1498, - "open": 1498, - "volume": 1498 - }, - "open": "2020-11-01T00:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1507, - "high": 79877, - "low": 1507, - "open": 79877, - "volume": 81384 - }, - "id": 2757686, - "non_hive": { - "close": 190, - "high": 10088, - "low": 190, - "open": 10088, - "volume": 10278 - }, - "open": "2020-11-01T01:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 15819, - "high": 7909, - "low": 14278, - "open": 7909, - "volume": 106039 - }, - "id": 2757691, - "non_hive": { - "close": 1998, - "high": 999, - "low": 1797, - "open": 999, - "volume": 13384 - }, - "open": "2020-11-01T01:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 23729, - "high": 23729, - "low": 23729, - "open": 23729, - "volume": 23729 - }, - "id": 2757698, - "non_hive": { - "close": 2997, - "high": 2997, - "low": 2997, - "open": 2997, - "volume": 2997 - }, - "open": "2020-11-01T01:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 10665, - "high": 10665, - "low": 10665, - "open": 10665, - "volume": 10665 - }, - "id": 2757701, - "non_hive": { - "close": 1347, - "high": 1347, - "low": 1347, - "open": 1347, - "volume": 1347 - }, - "open": "2020-11-01T01:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1266837, - "high": 1266837, - "low": 1266837, - "open": 1266837, - "volume": 1266837 - }, - "id": 2757704, - "non_hive": { - "close": 160001, - "high": 160001, - "low": 160001, - "open": 160001, - "volume": 160001 - }, - "open": "2020-11-01T01:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1920, - "high": 1920, - "low": 1920, - "open": 1920, - "volume": 1920 - }, - "id": 2757707, - "non_hive": { - "close": 242, - "high": 242, - "low": 242, - "open": 242, - "volume": 242 - }, - "open": "2020-11-01T01:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 74557, - "high": 5657, - "low": 29611, - "open": 29611, - "volume": 309825 - }, - "id": 2757710, - "non_hive": { - "close": 9436, - "high": 716, - "low": 3742, - "open": 3742, - "volume": 39174 - }, - "open": "2020-11-01T02:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 7867, - "high": 7867, - "low": 119786, - "open": 119786, - "volume": 828859 - }, - "id": 2757718, - "non_hive": { - "close": 1007, - "high": 1007, - "low": 15160, - "open": 15160, - "volume": 105906 - }, - "open": "2020-11-01T02:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 24916, - "high": 24916, - "low": 24916, - "open": 24916, - "volume": 24916 - }, - "id": 2757723, - "non_hive": { - "close": 3189, - "high": 3189, - "low": 3189, - "open": 3189, - "volume": 3189 - }, - "open": "2020-11-01T02:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 3439, - "high": 179, - "low": 3439, - "open": 179, - "volume": 9635 - }, - "id": 2757726, - "non_hive": { - "close": 440, - "high": 23, - "low": 440, - "open": 23, - "volume": 1233 - }, - "open": "2020-11-01T02:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6872, - "high": 6872, - "low": 11979, - "open": 15801, - "volume": 513073 - }, - "id": 2757729, - "non_hive": { - "close": 879, - "high": 879, - "low": 1512, - "open": 2000, - "volume": 64821 - }, - "open": "2020-11-01T02:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6000, - "high": 6962, - "low": 6000, - "open": 15844, - "volume": 53533 - }, - "id": 2757736, - "non_hive": { - "close": 757, - "high": 879, - "low": 757, - "open": 2000, - "volume": 6757 - }, - "open": "2020-11-01T02:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 160922, - "high": 182, - "low": 160922, - "open": 966, - "volume": 446467 - }, - "id": 2757744, - "non_hive": { - "close": 20116, - "high": 23, - "low": 20116, - "open": 122, - "volume": 55832 - }, - "open": "2020-11-01T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 11077, - "high": 6926, - "low": 11077, - "open": 15998, - "volume": 1049999 - }, - "id": 2757747, - "non_hive": { - "close": 1384, - "high": 866, - "low": 1384, - "open": 2000, - "volume": 131257 - }, - "open": "2020-11-01T02:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 430000, - "high": 430000, - "low": 430000, - "open": 430000, - "volume": 430000 - }, - "id": 2757754, - "non_hive": { - "close": 53752, - "high": 53752, - "low": 53752, - "open": 53752, - "volume": 53752 - }, - "open": "2020-11-01T02:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 4943, - "high": 4943, - "low": 10255, - "open": 8935, - "volume": 24133 - }, - "id": 2757757, - "non_hive": { - "close": 632, - "high": 632, - "low": 1261, - "open": 1117, - "volume": 3010 - }, - "open": "2020-11-01T03:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 298444, - "high": 298444, - "low": 15649, - "open": 15649, - "volume": 468688 - }, - "id": 2757763, - "non_hive": { - "close": 38171, - "high": 38171, - "low": 1999, - "open": 1999, - "volume": 59940 - }, - "open": "2020-11-01T04:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 47, - "high": 9489, - "low": 47, - "open": 9489, - "volume": 9536 - }, - "id": 2757769, - "non_hive": { - "close": 6, - "high": 1212, - "low": 6, - "open": 1212, - "volume": 1218 - }, - "open": "2020-11-01T04:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1330, - "high": 1330, - "low": 16127, - "open": 16127, - "volume": 22592 - }, - "id": 2757773, - "non_hive": { - "close": 170, - "high": 170, - "low": 2000, - "open": 2000, - "volume": 2807 - }, - "open": "2020-11-01T04:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 320824, - "high": 7145, - "low": 320824, - "open": 7145, - "volume": 467919 - }, - "id": 2757779, - "non_hive": { - "close": 39461, - "high": 886, - "low": 39461, - "open": 886, - "volume": 57561 - }, - "open": "2020-11-01T04:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 86100, - "high": 86100, - "low": 15663, - "open": 15663, - "volume": 114763 - }, - "id": 2757786, - "non_hive": { - "close": 11011, - "high": 11011, - "low": 1999, - "open": 1999, - "volume": 14670 - }, - "open": "2020-11-01T04:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 12205, - "high": 12205, - "low": 12205, - "open": 12205, - "volume": 12205 - }, - "id": 2757789, - "non_hive": { - "close": 1560, - "high": 1560, - "low": 1560, - "open": 1560, - "volume": 1560 - }, - "open": "2020-11-01T05:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 5593, - "high": 5593, - "low": 15639, - "open": 15639, - "volume": 36871 - }, - "id": 2757793, - "non_hive": { - "close": 715, - "high": 715, - "low": 1999, - "open": 1999, - "volume": 4713 - }, - "open": "2020-11-01T05:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 117, - "high": 117, - "low": 34914, - "open": 86, - "volume": 35117 - }, - "id": 2757800, - "non_hive": { - "close": 15, - "high": 15, - "low": 4462, - "open": 11, - "volume": 4488 - }, - "open": "2020-11-01T05:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 66, - "high": 3137, - "low": 66, - "open": 3137, - "volume": 3203 - }, - "id": 2757805, - "non_hive": { - "close": 8, - "high": 400, - "low": 8, - "open": 400, - "volume": 408 - }, - "open": "2020-11-01T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 338069, - "high": 338069, - "low": 15677, - "open": 15677, - "volume": 390672 - }, - "id": 2757808, - "non_hive": { - "close": 43240, - "high": 43240, - "low": 1999, - "open": 1999, - "volume": 49950 - }, - "open": "2020-11-01T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 39483, - "high": 15872, - "low": 39483, - "open": 15872, - "volume": 55355 - }, - "id": 2757811, - "non_hive": { - "close": 4974, - "high": 2000, - "low": 4974, - "open": 2000, - "volume": 6974 - }, - "open": "2020-11-01T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 15000, - "high": 9103, - "low": 385, - "open": 9103, - "volume": 117303 - }, - "id": 2757815, - "non_hive": { - "close": 1890, - "high": 1163, - "low": 48, - "open": 1163, - "volume": 14899 - }, - "open": "2020-11-01T06:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7128, - "high": 872, - "low": 7128, - "open": 872, - "volume": 8000 - }, - "id": 2757827, - "non_hive": { - "close": 898, - "high": 110, - "low": 898, - "open": 110, - "volume": 1008 - }, - "open": "2020-11-01T06:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 15748, - "high": 15748, - "low": 15748, - "open": 15748, - "volume": 15748 - }, - "id": 2757830, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-01T06:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 79606, - "high": 79606, - "low": 1970, - "open": 1970, - "volume": 81576 - }, - "id": 2757833, - "non_hive": { - "close": 10110, - "high": 10110, - "low": 250, - "open": 250, - "volume": 10360 - }, - "open": "2020-11-01T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 4181, - "high": 556, - "low": 4181, - "open": 54000, - "volume": 414537 - }, - "id": 2757836, - "non_hive": { - "close": 503, - "high": 71, - "low": 503, - "open": 6858, - "volume": 52573 - }, - "open": "2020-11-01T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 179395, - "high": 179395, - "low": 12000, - "open": 12000, - "volume": 207395 - }, - "id": 2757843, - "non_hive": { - "close": 22420, - "high": 22420, - "low": 1498, - "open": 1498, - "volume": 25917 - }, - "open": "2020-11-01T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 10743, - "high": 10743, - "low": 15748, - "open": 15748, - "volume": 235887 - }, - "id": 2757846, - "non_hive": { - "close": 1374, - "high": 1374, - "low": 1999, - "open": 1999, - "volume": 29969 - }, - "open": "2020-11-01T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 16121, - "high": 347, - "low": 16121, - "open": 347, - "volume": 16468 - }, - "id": 2757849, - "non_hive": { - "close": 2000, - "high": 44, - "low": 2000, - "open": 44, - "volume": 2044 - }, - "open": "2020-11-01T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 75349, - "high": 75349, - "low": 4796, - "open": 16121, - "volume": 1293988 - }, - "id": 2757854, - "non_hive": { - "close": 9349, - "high": 9349, - "low": 594, - "open": 2000, - "volume": 160467 - }, - "open": "2020-11-01T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 133886, - "high": 16112, - "low": 133886, - "open": 16112, - "volume": 149998 - }, - "id": 2757868, - "non_hive": { - "close": 16610, - "high": 1999, - "low": 16610, - "open": 1999, - "volume": 18609 - }, - "open": "2020-11-01T07:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7929, - "high": 7929, - "low": 16011, - "open": 16011, - "volume": 623940 - }, - "id": 2757871, - "non_hive": { - "close": 990, - "high": 990, - "low": 1999, - "open": 1999, - "volume": 77901 - }, - "open": "2020-11-01T07:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13128, - "high": 17370, - "low": 13128, - "open": 17370, - "volume": 30498 - }, - "id": 2757878, - "non_hive": { - "close": 1637, - "high": 2167, - "low": 1637, - "open": 2167, - "volume": 3804 - }, - "open": "2020-11-01T08:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 16164, - "high": 10571, - "low": 22, - "open": 25378, - "volume": 86922 - }, - "id": 2757883, - "non_hive": { - "close": 1999, - "high": 1308, - "low": 2, - "open": 3140, - "volume": 10751 - }, - "open": "2020-11-01T08:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 8219, - "high": 8219, - "low": 8219, - "open": 8219, - "volume": 8219 - }, - "id": 2757892, - "non_hive": { - "close": 1017, - "high": 1017, - "low": 1017, - "open": 1017, - "volume": 1017 - }, - "open": "2020-11-01T08:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6468, - "high": 11072, - "low": 6468, - "open": 11072, - "volume": 17540 - }, - "id": 2757895, - "non_hive": { - "close": 800, - "high": 1370, - "low": 800, - "open": 1370, - "volume": 2170 - }, - "open": "2020-11-01T08:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 4238, - "high": 9485, - "low": 4238, - "open": 9485, - "volume": 13723 - }, - "id": 2757900, - "non_hive": { - "close": 524, - "high": 1173, - "low": 524, - "open": 1173, - "volume": 1697 - }, - "open": "2020-11-01T08:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 16024, - "high": 16024, - "low": 13457, - "open": 13457, - "volume": 39191 - }, - "id": 2757903, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 1665, - "open": 1665, - "volume": 4867 - }, - "open": "2020-11-01T09:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 16152, - "high": 16152, - "low": 16152, - "open": 16152, - "volume": 50251 - }, - "id": 2757908, - "non_hive": { - "close": 1999, - "high": 2000, - "low": 1999, - "open": 2000, - "volume": 6221 - }, - "open": "2020-11-01T09:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 2996, - "high": 2996, - "low": 16000, - "open": 16000, - "volume": 18996 - }, - "id": 2757912, - "non_hive": { - "close": 374, - "high": 374, - "low": 1997, - "open": 1997, - "volume": 2371 - }, - "open": "2020-11-01T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 95258, - "high": 95258, - "low": 95258, - "open": 95258, - "volume": 95258 - }, - "id": 2757917, - "non_hive": { - "close": 11890, - "high": 11890, - "low": 11890, - "open": 11890, - "volume": 11890 - }, - "open": "2020-11-01T09:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 8582, - "high": 8582, - "low": 8582, - "open": 8582, - "volume": 8582 - }, - "id": 2757920, - "non_hive": { - "close": 1071, - "high": 1071, - "low": 1071, - "open": 1071, - "volume": 1071 - }, - "open": "2020-11-01T09:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 64286, - "high": 64286, - "low": 64286, - "open": 64286, - "volume": 64286 - }, - "id": 2757923, - "non_hive": { - "close": 8022, - "high": 8022, - "low": 8022, - "open": 8022, - "volume": 8022 - }, - "open": "2020-11-01T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 76046, - "high": 76046, - "low": 16129, - "open": 16129, - "volume": 200427 - }, - "id": 2757926, - "non_hive": { - "close": 9492, - "high": 9492, - "low": 2013, - "open": 2013, - "volume": 25016 - }, - "open": "2020-11-01T09:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 16122, - "high": 9446, - "low": 16122, - "open": 9446, - "volume": 51746 - }, - "id": 2757931, - "non_hive": { - "close": 1999, - "high": 1179, - "low": 1999, - "open": 1179, - "volume": 6433 - }, - "open": "2020-11-01T09:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 297888, - "high": 297888, - "low": 297888, - "open": 297888, - "volume": 297888 - }, - "id": 2757936, - "non_hive": { - "close": 36954, - "high": 36954, - "low": 36954, - "open": 36954, - "volume": 36954 - }, - "open": "2020-11-01T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 156875, - "high": 156875, - "low": 156875, - "open": 156875, - "volume": 156875 - }, - "id": 2757939, - "non_hive": { - "close": 19578, - "high": 19578, - "low": 19578, - "open": 19578, - "volume": 19578 - }, - "open": "2020-11-01T10:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 304, - "high": 304, - "low": 304, - "open": 304, - "volume": 304 - }, - "id": 2757943, - "non_hive": { - "close": 38, - "high": 38, - "low": 38, - "open": 38, - "volume": 38 - }, - "open": "2020-11-01T10:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1442, - "high": 1442, - "low": 1000, - "open": 1000, - "volume": 2442 - }, - "id": 2757946, - "non_hive": { - "close": 180, - "high": 180, - "low": 124, - "open": 124, - "volume": 304 - }, - "open": "2020-11-01T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 3398, - "high": 3398, - "low": 3398, - "open": 3398, - "volume": 3398 - }, - "id": 2757951, - "non_hive": { - "close": 424, - "high": 424, - "low": 424, - "open": 424, - "volume": 424 - }, - "open": "2020-11-01T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 80146, - "high": 80146, - "low": 80146, - "open": 80146, - "volume": 80146 - }, - "id": 2757954, - "non_hive": { - "close": 10000, - "high": 10000, - "low": 10000, - "open": 10000, - "volume": 10000 - }, - "open": "2020-11-01T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 8020, - "high": 8020, - "low": 8020, - "open": 8020, - "volume": 8020 - }, - "id": 2757957, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-11-01T10:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 200000, - "high": 200000, - "low": 405211, - "open": 405211, - "volume": 605211 - }, - "id": 2757960, - "non_hive": { - "close": 24940, - "high": 24940, - "low": 50529, - "open": 50529, - "volume": 75469 - }, - "open": "2020-11-01T11:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 487, - "high": 74282, - "low": 487, - "open": 56232, - "volume": 250128 - }, - "id": 2757964, - "non_hive": { - "close": 60, - "high": 9270, - "low": 60, - "open": 7012, - "volume": 31204 - }, - "open": "2020-11-01T11:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 5101, - "high": 5101, - "low": 5101, - "open": 5101, - "volume": 5101 - }, - "id": 2757970, - "non_hive": { - "close": 636, - "high": 636, - "low": 636, - "open": 636, - "volume": 636 - }, - "open": "2020-11-01T11:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1482, - "high": 1482, - "low": 1482, - "open": 1482, - "volume": 1482 - }, - "id": 2757973, - "non_hive": { - "close": 185, - "high": 185, - "low": 185, - "open": 185, - "volume": 185 - }, - "open": "2020-11-01T11:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2847, - "high": 11670, - "low": 2847, - "open": 11670, - "volume": 25346 - }, - "id": 2757976, - "non_hive": { - "close": 355, - "high": 1456, - "low": 355, - "open": 1456, - "volume": 3162 - }, - "open": "2020-11-01T11:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 24664, - "high": 16193, - "low": 24664, - "open": 16193, - "volume": 40857 - }, - "id": 2757981, - "non_hive": { - "close": 3046, - "high": 2000, - "low": 3046, - "open": 2000, - "volume": 5046 - }, - "open": "2020-11-01T11:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 176595, - "high": 176595, - "low": 24664, - "open": 1999, - "volume": 219451 - }, - "id": 2757984, - "non_hive": { - "close": 22000, - "high": 22000, - "low": 3046, - "open": 249, - "volume": 27295 - }, - "open": "2020-11-01T11:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 25424, - "high": 16193, - "low": 25424, - "open": 16193, - "volume": 41617 - }, - "id": 2757990, - "non_hive": { - "close": 3139, - "high": 2000, - "low": 3139, - "open": 2000, - "volume": 5139 - }, - "open": "2020-11-01T11:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 25424, - "high": 16193, - "low": 25424, - "open": 16193, - "volume": 41617 - }, - "id": 2757993, - "non_hive": { - "close": 3139, - "high": 2000, - "low": 3139, - "open": 2000, - "volume": 5139 - }, - "open": "2020-11-01T12:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 47422, - "high": 47422, - "low": 47422, - "open": 47422, - "volume": 47422 - }, - "id": 2757997, - "non_hive": { - "close": 5900, - "high": 5900, - "low": 5900, - "open": 5900, - "volume": 5900 - }, - "open": "2020-11-01T12:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 24535, - "high": 52761, - "low": 24535, - "open": 52761, - "volume": 105507 - }, - "id": 2758000, - "non_hive": { - "close": 3050, - "high": 6559, - "low": 3050, - "open": 6559, - "volume": 13116 - }, - "open": "2020-11-01T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16004, - "high": 16000, - "low": 16004, - "open": 16000, - "volume": 48007 - }, - "id": 2758003, - "non_hive": { - "close": 1988, - "high": 1988, - "low": 1988, - "open": 1988, - "volume": 5964 - }, - "open": "2020-11-01T12:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 5746, - "high": 97191, - "low": 5000, - "open": 89, - "volume": 127703 - }, - "id": 2758009, - "non_hive": { - "close": 714, - "high": 12077, - "low": 617, - "open": 11, - "volume": 15863 - }, - "open": "2020-11-01T12:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 3806, - "high": 3806, - "low": 13665, - "open": 13665, - "volume": 17471 - }, - "id": 2758016, - "non_hive": { - "close": 473, - "high": 473, - "low": 1698, - "open": 1698, - "volume": 2171 - }, - "open": "2020-11-01T12:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 42888, - "high": 42888, - "low": 35581, - "open": 35581, - "volume": 78469 - }, - "id": 2758019, - "non_hive": { - "close": 5329, - "high": 5329, - "low": 4421, - "open": 4421, - "volume": 9750 - }, - "open": "2020-11-01T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 4236, - "high": 4236, - "low": 4236, - "open": 4236, - "volume": 4236 - }, - "id": 2758024, - "non_hive": { - "close": 526, - "high": 526, - "low": 526, - "open": 526, - "volume": 526 - }, - "open": "2020-11-01T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 482, - "high": 30000, - "low": 482, - "open": 30000, - "volume": 30482 - }, - "id": 2758028, - "non_hive": { - "close": 59, - "high": 3728, - "low": 59, - "open": 3728, - "volume": 3787 - }, - "open": "2020-11-01T13:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 26153, - "high": 73180, - "low": 94493, - "open": 94493, - "volume": 215168 - }, - "id": 2758031, - "non_hive": { - "close": 3251, - "high": 9097, - "low": 11746, - "open": 11746, - "volume": 26747 - }, - "open": "2020-11-01T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 19224, - "high": 19224, - "low": 37053, - "open": 37053, - "volume": 56277 - }, - "id": 2758036, - "non_hive": { - "close": 2391, - "high": 2391, - "low": 4608, - "open": 4608, - "volume": 6999 - }, - "open": "2020-11-01T13:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 74557, - "high": 74557, - "low": 74557, - "open": 74557, - "volume": 74557 - }, - "id": 2758041, - "non_hive": { - "close": 9273, - "high": 9273, - "low": 9273, - "open": 9273, - "volume": 9273 - }, - "open": "2020-11-01T13:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 24538, - "high": 24538, - "low": 24538, - "open": 24538, - "volume": 24538 - }, - "id": 2758044, - "non_hive": { - "close": 3052, - "high": 3052, - "low": 3052, - "open": 3052, - "volume": 3052 - }, - "open": "2020-11-01T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 201006, - "high": 201006, - "low": 201006, - "open": 201006, - "volume": 201006 - }, - "id": 2758047, - "non_hive": { - "close": 25000, - "high": 25000, - "low": 25000, - "open": 25000, - "volume": 25000 - }, - "open": "2020-11-01T13:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 10323, - "high": 43200, - "low": 10323, - "open": 43200, - "volume": 71382 - }, - "id": 2758050, - "non_hive": { - "close": 1274, - "high": 5373, - "low": 1274, - "open": 5373, - "volume": 8868 - }, - "open": "2020-11-01T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 189, - "high": 811, - "low": 189, - "open": 10323, - "volume": 117820 - }, - "id": 2758055, - "non_hive": { - "close": 23, - "high": 101, - "low": 23, - "open": 1283, - "volume": 14654 - }, - "open": "2020-11-01T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 500000, - "high": 184888, - "low": 5705, - "open": 184888, - "volume": 694597 - }, - "id": 2758063, - "non_hive": { - "close": 61761, - "high": 23000, - "low": 704, - "open": 23000, - "volume": 85963 - }, - "open": "2020-11-01T14:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 307162, - "high": 8110, - "low": 307162, - "open": 1890, - "volume": 510000 - }, - "id": 2758071, - "non_hive": { - "close": 37934, - "high": 1009, - "low": 37934, - "open": 235, - "volume": 62995 - }, - "open": "2020-11-01T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 16080, - "high": 16080, - "low": 16080, - "open": 16080, - "volume": 16080 - }, - "id": 2758076, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-01T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 232, - "high": 104397, - "low": 232, - "open": 104397, - "volume": 118551 - }, - "id": 2758079, - "non_hive": { - "close": 28, - "high": 12987, - "low": 28, - "open": 12987, - "volume": 14746 - }, - "open": "2020-11-01T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16626, - "high": 16626, - "low": 16626, - "open": 16626, - "volume": 16626 - }, - "id": 2758084, - "non_hive": { - "close": 2068, - "high": 2068, - "low": 2068, - "open": 2068, - "volume": 2068 - }, - "open": "2020-11-01T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 8, - "high": 8, - "low": 8, - "open": 8, - "volume": 8 - }, - "id": 2758087, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 1, - "volume": 1 - }, - "open": "2020-11-01T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 217, - "high": 217, - "low": 217, - "open": 217, - "volume": 217 - }, - "id": 2758090, - "non_hive": { - "close": 27, - "high": 27, - "low": 27, - "open": 27, - "volume": 27 - }, - "open": "2020-11-01T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 24176, - "high": 24176, - "low": 24176, - "open": 24176, - "volume": 24176 - }, - "id": 2758093, - "non_hive": { - "close": 3007, - "high": 3007, - "low": 3007, - "open": 3007, - "volume": 3007 - }, - "open": "2020-11-01T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 70273, - "high": 70273, - "low": 70112, - "open": 70112, - "volume": 140385 - }, - "id": 2758097, - "non_hive": { - "close": 8742, - "high": 8742, - "low": 8721, - "open": 8721, - "volume": 17463 - }, - "open": "2020-11-01T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3470, - "high": 3470, - "low": 3470, - "open": 3470, - "volume": 3470 - }, - "id": 2758101, - "non_hive": { - "close": 431, - "high": 431, - "low": 431, - "open": 431, - "volume": 431 - }, - "open": "2020-11-01T15:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 16085, - "high": 16085, - "low": 170000, - "open": 170000, - "volume": 186085 - }, - "id": 2758104, - "non_hive": { - "close": 2001, - "high": 2001, - "low": 21148, - "open": 21148, - "volume": 23149 - }, - "open": "2020-11-01T15:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 417, - "high": 417, - "low": 41656, - "open": 41656, - "volume": 42073 - }, - "id": 2758109, - "non_hive": { - "close": 52, - "high": 52, - "low": 5182, - "open": 5182, - "volume": 5234 - }, - "open": "2020-11-01T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 37979, - "high": 12021, - "low": 19044, - "open": 24324, - "volume": 160818 - }, - "id": 2758112, - "non_hive": { - "close": 4723, - "high": 1500, - "low": 2368, - "open": 3031, - "volume": 20015 - }, - "open": "2020-11-01T15:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 64278, - "high": 1226, - "low": 64278, - "open": 1226, - "volume": 65504 - }, - "id": 2758119, - "non_hive": { - "close": 8021, - "high": 153, - "low": 8021, - "open": 153, - "volume": 8174 - }, - "open": "2020-11-01T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 2547, - "high": 40, - "low": 2547, - "open": 78343, - "volume": 258468 - }, - "id": 2758124, - "non_hive": { - "close": 316, - "high": 5, - "low": 316, - "open": 9776, - "volume": 32207 - }, - "open": "2020-11-01T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 3920, - "high": 16080, - "low": 3920, - "open": 16080, - "volume": 20000 - }, - "id": 2758140, - "non_hive": { - "close": 487, - "high": 2000, - "low": 487, - "open": 2000, - "volume": 2487 - }, - "open": "2020-11-01T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1862, - "high": 80, - "low": 1862, - "open": 10967, - "volume": 30967 - }, - "id": 2758143, - "non_hive": { - "close": 231, - "high": 10, - "low": 231, - "open": 1368, - "volume": 3855 - }, - "open": "2020-11-01T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 696, - "high": 11289, - "low": 696, - "open": 11289, - "volume": 20000 - }, - "id": 2758151, - "non_hive": { - "close": 86, - "high": 1408, - "low": 86, - "open": 1408, - "volume": 2491 - }, - "open": "2020-11-01T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 44211, - "high": 44211, - "low": 5060, - "open": 5060, - "volume": 49271 - }, - "id": 2758154, - "non_hive": { - "close": 5517, - "high": 5517, - "low": 631, - "open": 631, - "volume": 6148 - }, - "open": "2020-11-01T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 16082, - "high": 5617, - "low": 16082, - "open": 16027, - "volume": 97856 - }, - "id": 2758157, - "non_hive": { - "close": 2000, - "high": 701, - "low": 2000, - "open": 1999, - "volume": 12200 - }, - "open": "2020-11-01T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6127, - "high": 6127, - "low": 6127, - "open": 6127, - "volume": 6127 - }, - "id": 2758163, - "non_hive": { - "close": 762, - "high": 762, - "low": 762, - "open": 762, - "volume": 762 - }, - "open": "2020-11-01T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2405, - "high": 3176, - "low": 2405, - "open": 3176, - "volume": 8838 - }, - "id": 2758166, - "non_hive": { - "close": 299, - "high": 395, - "low": 299, - "open": 395, - "volume": 1099 - }, - "open": "2020-11-01T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 72400, - "high": 72400, - "low": 72400, - "open": 72400, - "volume": 72400 - }, - "id": 2758169, - "non_hive": { - "close": 9001, - "high": 9001, - "low": 9001, - "open": 9001, - "volume": 9001 - }, - "open": "2020-11-01T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 264, - "high": 264, - "low": 178762, - "open": 178762, - "volume": 441842 - }, - "id": 2758173, - "non_hive": { - "close": 33, - "high": 33, - "low": 22224, - "open": 22224, - "volume": 55024 - }, - "open": "2020-11-01T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 4417, - "high": 4417, - "low": 4417, - "open": 4417, - "volume": 4417 - }, - "id": 2758179, - "non_hive": { - "close": 551, - "high": 551, - "low": 551, - "open": 551, - "volume": 551 - }, - "open": "2020-11-01T17:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 665, - "high": 665, - "low": 665, - "open": 665, - "volume": 665 - }, - "id": 2758182, - "non_hive": { - "close": 83, - "high": 83, - "low": 83, - "open": 83, - "volume": 83 - }, - "open": "2020-11-01T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 89, - "high": 650, - "low": 89, - "open": 650, - "volume": 2365 - }, - "id": 2758185, - "non_hive": { - "close": 11, - "high": 81, - "low": 11, - "open": 81, - "volume": 294 - }, - "open": "2020-11-01T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 19000, - "high": 19000, - "low": 9324, - "open": 16193, - "volume": 144351 - }, - "id": 2758192, - "non_hive": { - "close": 2371, - "high": 2371, - "low": 1151, - "open": 2000, - "volume": 17871 - }, - "open": "2020-11-01T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 16467, - "high": 16467, - "low": 16467, - "open": 16467, - "volume": 16467 - }, - "id": 2758199, - "non_hive": { - "close": 2055, - "high": 2055, - "low": 2055, - "open": 2055, - "volume": 2055 - }, - "open": "2020-11-01T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 36260, - "high": 36260, - "low": 36260, - "open": 36260, - "volume": 36260 - }, - "id": 2758202, - "non_hive": { - "close": 4525, - "high": 4525, - "low": 4525, - "open": 4525, - "volume": 4525 - }, - "open": "2020-11-01T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12004, - "high": 46934, - "low": 12004, - "open": 46934, - "volume": 58938 - }, - "id": 2758205, - "non_hive": { - "close": 1498, - "high": 5857, - "low": 1498, - "open": 5857, - "volume": 7355 - }, - "open": "2020-11-01T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 560929, - "high": 168, - "low": 8188, - "open": 30726, - "volume": 804446 - }, - "id": 2758210, - "non_hive": { - "close": 70001, - "high": 21, - "low": 1021, - "open": 3834, - "volume": 100388 - }, - "open": "2020-11-01T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 192636, - "high": 496, - "low": 13504, - "open": 36000, - "volume": 299335 - }, - "id": 2758217, - "non_hive": { - "close": 24040, - "high": 62, - "low": 1684, - "open": 4492, - "volume": 37354 - }, - "open": "2020-11-01T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 24039, - "high": 24039, - "low": 24039, - "open": 24039, - "volume": 24039 - }, - "id": 2758229, - "non_hive": { - "close": 3000, - "high": 3000, - "low": 3000, - "open": 3000, - "volume": 3000 - }, - "open": "2020-11-01T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 104, - "high": 8503, - "low": 104, - "open": 8503, - "volume": 10104 - }, - "id": 2758232, - "non_hive": { - "close": 12, - "high": 1061, - "low": 12, - "open": 1061, - "volume": 1259 - }, - "open": "2020-11-01T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 32358, - "high": 32358, - "low": 32358, - "open": 32358, - "volume": 32358 - }, - "id": 2758237, - "non_hive": { - "close": 4038, - "high": 4038, - "low": 4038, - "open": 4038, - "volume": 4038 - }, - "open": "2020-11-01T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 37759, - "high": 9479, - "low": 37759, - "open": 9479, - "volume": 47238 - }, - "id": 2758240, - "non_hive": { - "close": 4712, - "high": 1183, - "low": 4712, - "open": 1183, - "volume": 5895 - }, - "open": "2020-11-01T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 8767, - "high": 8767, - "low": 5762, - "open": 5762, - "volume": 14529 - }, - "id": 2758245, - "non_hive": { - "close": 1094, - "high": 1094, - "low": 719, - "open": 719, - "volume": 1813 - }, - "open": "2020-11-01T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 10609, - "high": 464, - "low": 10609, - "open": 464, - "volume": 92771 - }, - "id": 2758250, - "non_hive": { - "close": 1310, - "high": 58, - "low": 1310, - "open": 58, - "volume": 11539 - }, - "open": "2020-11-01T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 837, - "high": 144846, - "low": 22, - "open": 16186, - "volume": 647717 - }, - "id": 2758256, - "non_hive": { - "close": 103, - "high": 17919, - "low": 2, - "open": 2000, - "volume": 80077 - }, - "open": "2020-11-01T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 19188, - "high": 647, - "low": 19188, - "open": 647, - "volume": 154298 - }, - "id": 2758263, - "non_hive": { - "close": 2306, - "high": 80, - "low": 2306, - "open": 80, - "volume": 18934 - }, - "open": "2020-11-01T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 4220, - "high": 4220, - "low": 16076, - "open": 16076, - "volume": 20296 - }, - "id": 2758269, - "non_hive": { - "close": 526, - "high": 526, - "low": 1999, - "open": 1999, - "volume": 2525 - }, - "open": "2020-11-01T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 18000 - }, - "id": 2758275, - "non_hive": { - "close": 748, - "high": 748, - "low": 748, - "open": 748, - "volume": 2244 - }, - "open": "2020-11-01T19:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2000, - "high": 850, - "low": 2000, - "open": 27484, - "volume": 47880 - }, - "id": 2758281, - "non_hive": { - "close": 249, - "high": 106, - "low": 249, - "open": 3426, - "volume": 5968 - }, - "open": "2020-11-01T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1971, - "high": 257, - "low": 1971, - "open": 257, - "volume": 16334 - }, - "id": 2758284, - "non_hive": { - "close": 242, - "high": 32, - "low": 242, - "open": 32, - "volume": 2029 - }, - "open": "2020-11-01T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2101, - "high": 2101, - "low": 1497, - "open": 1497, - "volume": 3598 - }, - "id": 2758288, - "non_hive": { - "close": 262, - "high": 262, - "low": 186, - "open": 186, - "volume": 448 - }, - "open": "2020-11-01T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 16104, - "high": 16104, - "low": 16104, - "open": 16104, - "volume": 16104 - }, - "id": 2758291, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-01T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 16132, - "high": 16129, - "low": 16132, - "open": 16129, - "volume": 80648 - }, - "id": 2758294, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 9995 - }, - "open": "2020-11-01T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 16127, - "high": 16127, - "low": 21657, - "open": 3935, - "volume": 124536 - }, - "id": 2758303, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2685, - "open": 488, - "volume": 15442 - }, - "open": "2020-11-01T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 16133, - "high": 11762, - "low": 16133, - "open": 16126, - "volume": 108637 - }, - "id": 2758312, - "non_hive": { - "close": 1999, - "high": 1463, - "low": 1999, - "open": 2000, - "volume": 13476 - }, - "open": "2020-11-01T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 16135, - "high": 16135, - "low": 16135, - "open": 16135, - "volume": 80675 - }, - "id": 2758324, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 9995 - }, - "open": "2020-11-01T20:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 16194, - "high": 16194, - "low": 16194, - "open": 16194, - "volume": 16194 - }, - "id": 2758334, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-01T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 16194, - "high": 695, - "low": 16194, - "open": 695, - "volume": 49277 - }, - "id": 2758337, - "non_hive": { - "close": 2000, - "high": 86, - "low": 2000, - "open": 86, - "volume": 6086 - }, - "open": "2020-11-01T20:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 16201, - "high": 16194, - "low": 16201, - "open": 16194, - "volume": 41521 - }, - "id": 2758344, - "non_hive": { - "close": 1999, - "high": 2000, - "low": 1999, - "open": 2000, - "volume": 5126 - }, - "open": "2020-11-01T20:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 9175, - "high": 6900, - "low": 9175, - "open": 6900, - "volume": 16075 - }, - "id": 2758348, - "non_hive": { - "close": 1128, - "high": 851, - "low": 1128, - "open": 851, - "volume": 1979 - }, - "open": "2020-11-01T20:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 8302, - "high": 8302, - "low": 3741, - "open": 16259, - "volume": 255273 - }, - "id": 2758353, - "non_hive": { - "close": 1036, - "high": 1036, - "low": 460, - "open": 2000, - "volume": 31717 - }, - "open": "2020-11-01T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 42923, - "high": 42923, - "low": 11000, - "open": 16260, - "volume": 91146 - }, - "id": 2758360, - "non_hive": { - "close": 5353, - "high": 5353, - "low": 1352, - "open": 1999, - "volume": 11281 - }, - "open": "2020-11-01T20:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 81, - "high": 81, - "low": 81, - "open": 81, - "volume": 81 - }, - "id": 2758369, - "non_hive": { - "close": 10, - "high": 10, - "low": 10, - "open": 10, - "volume": 10 - }, - "open": "2020-11-01T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 44130, - "high": 44130, - "low": 16044, - "open": 16044, - "volume": 60174 - }, - "id": 2758372, - "non_hive": { - "close": 5501, - "high": 5501, - "low": 1999, - "open": 1999, - "volume": 7500 - }, - "open": "2020-11-01T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 29396, - "high": 26024, - "low": 29396, - "open": 5001, - "volume": 60421 - }, - "id": 2758375, - "non_hive": { - "close": 3660, - "high": 3242, - "low": 3660, - "open": 623, - "volume": 7525 - }, - "open": "2020-11-01T20:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 21182, - "high": 21182, - "low": 16041, - "open": 16041, - "volume": 37223 - }, - "id": 2758380, - "non_hive": { - "close": 2641, - "high": 2641, - "low": 1999, - "open": 1999, - "volume": 4640 - }, - "open": "2020-11-01T20:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 17403, - "high": 17403, - "low": 17403, - "open": 17403, - "volume": 17403 - }, - "id": 2758383, - "non_hive": { - "close": 2140, - "high": 2140, - "low": 2140, - "open": 2140, - "volume": 2140 - }, - "open": "2020-11-01T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 16259, - "high": 4381, - "low": 16259, - "open": 4381, - "volume": 20640 - }, - "id": 2758387, - "non_hive": { - "close": 2000, - "high": 546, - "low": 2000, - "open": 546, - "volume": 2546 - }, - "open": "2020-11-01T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 32046, - "high": 2910, - "low": 16259, - "open": 2910, - "volume": 51215 - }, - "id": 2758392, - "non_hive": { - "close": 3942, - "high": 358, - "low": 1999, - "open": 358, - "volume": 6299 - }, - "open": "2020-11-01T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 9701, - "high": 9701, - "low": 9701, - "open": 9701, - "volume": 9701 - }, - "id": 2758397, - "non_hive": { - "close": 1193, - "high": 1193, - "low": 1193, - "open": 1193, - "volume": 1193 - }, - "open": "2020-11-01T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 7602, - "high": 7602, - "low": 2855, - "open": 2855, - "volume": 10457 - }, - "id": 2758400, - "non_hive": { - "close": 935, - "high": 935, - "low": 351, - "open": 351, - "volume": 1286 - }, - "open": "2020-11-01T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 5173, - "high": 31513, - "low": 5173, - "open": 31513, - "volume": 41704 - }, - "id": 2758403, - "non_hive": { - "close": 636, - "high": 3875, - "low": 636, - "open": 3875, - "volume": 5128 - }, - "open": "2020-11-01T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6699, - "high": 6699, - "low": 6699, - "open": 6699, - "volume": 6699 - }, - "id": 2758406, - "non_hive": { - "close": 824, - "high": 824, - "low": 824, - "open": 824, - "volume": 824 - }, - "open": "2020-11-01T21:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2170, - "high": 2170, - "low": 2170, - "open": 2170, - "volume": 2170 - }, - "id": 2758409, - "non_hive": { - "close": 267, - "high": 267, - "low": 267, - "open": 267, - "volume": 267 - }, - "open": "2020-11-01T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 21267, - "high": 21267, - "low": 21267, - "open": 21267, - "volume": 21267 - }, - "id": 2758412, - "non_hive": { - "close": 2616, - "high": 2616, - "low": 2616, - "open": 2616, - "volume": 2616 - }, - "open": "2020-11-01T22:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 41688, - "high": 41688, - "low": 41688, - "open": 41688, - "volume": 41688 - }, - "id": 2758416, - "non_hive": { - "close": 5128, - "high": 5128, - "low": 5128, - "open": 5128, - "volume": 5128 - }, - "open": "2020-11-01T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 3276, - "high": 3276, - "low": 3276, - "open": 3276, - "volume": 3276 - }, - "id": 2758419, - "non_hive": { - "close": 403, - "high": 403, - "low": 403, - "open": 403, - "volume": 403 - }, - "open": "2020-11-01T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 32000, - "high": 32000, - "low": 32000, - "open": 32000, - "volume": 32000 - }, - "id": 2758422, - "non_hive": { - "close": 3936, - "high": 3936, - "low": 3936, - "open": 3936, - "volume": 3936 - }, - "open": "2020-11-01T22:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 8097, - "high": 8097, - "low": 8097, - "open": 8097, - "volume": 8097 - }, - "id": 2758425, - "non_hive": { - "close": 995, - "high": 995, - "low": 995, - "open": 995, - "volume": 995 - }, - "open": "2020-11-01T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 7503, - "high": 7503, - "low": 544822, - "open": 544822, - "volume": 552325 - }, - "id": 2758429, - "non_hive": { - "close": 923, - "high": 923, - "low": 67017, - "open": 67017, - "volume": 67940 - }, - "open": "2020-11-01T23:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6105, - "high": 6105, - "low": 6105, - "open": 6105, - "volume": 6105 - }, - "id": 2758434, - "non_hive": { - "close": 751, - "high": 751, - "low": 751, - "open": 751, - "volume": 751 - }, - "open": "2020-11-01T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 5405, - "high": 5405, - "low": 24124, - "open": 24124, - "volume": 122032 - }, - "id": 2758437, - "non_hive": { - "close": 674, - "high": 674, - "low": 2967, - "open": 2967, - "volume": 15174 - }, - "open": "2020-11-01T23:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 22330, - "high": 22330, - "low": 22330, - "open": 22330, - "volume": 22330 - }, - "id": 2758442, - "non_hive": { - "close": 2784, - "high": 2784, - "low": 2784, - "open": 2784, - "volume": 2784 - }, - "open": "2020-11-01T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4677, - "high": 175, - "low": 4677, - "open": 175, - "volume": 4852 - }, - "id": 2758445, - "non_hive": { - "close": 575, - "high": 22, - "low": 575, - "open": 22, - "volume": 597 - }, - "open": "2020-11-01T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 3952, - "high": 3952, - "low": 4677, - "open": 4677, - "volume": 8629 - }, - "id": 2758448, - "non_hive": { - "close": 498, - "high": 498, - "low": 589, - "open": 589, - "volume": 1087 - }, - "open": "2020-11-01T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 28000, - "high": 28000, - "low": 28000, - "open": 28000, - "volume": 28000 - }, - "id": 2758451, - "non_hive": { - "close": 3527, - "high": 3527, - "low": 3527, - "open": 3527, - "volume": 3527 - }, - "open": "2020-11-01T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 56526, - "high": 56526, - "low": 56526, - "open": 56526, - "volume": 56526 - }, - "id": 2758454, - "non_hive": { - "close": 7178, - "high": 7178, - "low": 7178, - "open": 7178, - "volume": 7178 - }, - "open": "2020-11-02T00:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 944, - "high": 944, - "low": 944, - "open": 944, - "volume": 944 - }, - "id": 2758459, - "non_hive": { - "close": 119, - "high": 119, - "low": 119, - "open": 119, - "volume": 119 - }, - "open": "2020-11-02T00:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13405, - "high": 13405, - "low": 13405, - "open": 13405, - "volume": 13405 - }, - "id": 2758462, - "non_hive": { - "close": 1701, - "high": 1701, - "low": 1701, - "open": 1701, - "volume": 1701 - }, - "open": "2020-11-02T00:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 6012, - "high": 23815, - "low": 16172, - "open": 23815, - "volume": 136492 - }, - "id": 2758465, - "non_hive": { - "close": 749, - "high": 3022, - "low": 2000, - "open": 3022, - "volume": 17022 - }, - "open": "2020-11-02T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 16119, - "high": 69025, - "low": 16039, - "open": 15875, - "volume": 3168937 - }, - "id": 2758478, - "non_hive": { - "close": 2000, - "high": 8727, - "low": 1990, - "open": 1999, - "volume": 400044 - }, - "open": "2020-11-02T00:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 637051, - "high": 30000, - "low": 16119, - "open": 15819, - "volume": 4923582 - }, - "id": 2758494, - "non_hive": { - "close": 80543, - "high": 3793, - "low": 2000, - "open": 1999, - "volume": 622442 - }, - "open": "2020-11-02T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 814881, - "high": 196992, - "low": 15405, - "open": 196992, - "volume": 1348102 - }, - "id": 2758505, - "non_hive": { - "close": 102777, - "high": 24906, - "low": 1942, - "open": 24906, - "volume": 170123 - }, - "open": "2020-11-02T00:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 16193, - "high": 7, - "low": 1167, - "open": 1647, - "volume": 344961 - }, - "id": 2758516, - "non_hive": { - "close": 2000, - "high": 1, - "low": 143, - "open": 207, - "volume": 43307 - }, - "open": "2020-11-02T00:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 95, - "high": 87, - "low": 16193, - "open": 16193, - "volume": 64741 - }, - "id": 2758525, - "non_hive": { - "close": 12, - "high": 11, - "low": 2000, - "open": 2000, - "volume": 8023 - }, - "open": "2020-11-02T00:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 103994, - "high": 103994, - "low": 103994, - "open": 103994, - "volume": 103994 - }, - "id": 2758536, - "non_hive": { - "close": 12998, - "high": 12998, - "low": 12998, - "open": 12998, - "volume": 12998 - }, - "open": "2020-11-02T01:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 16223, - "high": 63362, - "low": 16223, - "open": 15861, - "volume": 95446 - }, - "id": 2758540, - "non_hive": { - "close": 1999, - "high": 7990, - "low": 1999, - "open": 1999, - "volume": 11988 - }, - "open": "2020-11-02T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 43380, - "high": 43380, - "low": 43380, - "open": 43380, - "volume": 43380 - }, - "id": 2758545, - "non_hive": { - "close": 5461, - "high": 5461, - "low": 5461, - "open": 5461, - "volume": 5461 - }, - "open": "2020-11-02T01:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 11263, - "high": 8663, - "low": 11263, - "open": 8663, - "volume": 19926 - }, - "id": 2758548, - "non_hive": { - "close": 1418, - "high": 1091, - "low": 1418, - "open": 1091, - "volume": 2509 - }, - "open": "2020-11-02T01:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 14948, - "high": 14948, - "low": 15886, - "open": 15886, - "volume": 30834 - }, - "id": 2758552, - "non_hive": { - "close": 1882, - "high": 1882, - "low": 1999, - "open": 1999, - "volume": 3881 - }, - "open": "2020-11-02T01:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 134111, - "high": 134111, - "low": 15886, - "open": 15886, - "volume": 149997 - }, - "id": 2758555, - "non_hive": { - "close": 16884, - "high": 16884, - "low": 1999, - "open": 1999, - "volume": 18883 - }, - "open": "2020-11-02T01:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 173773, - "high": 173773, - "low": 15886, - "open": 15886, - "volume": 198001 - }, - "id": 2758558, - "non_hive": { - "close": 21895, - "high": 21895, - "low": 1999, - "open": 1999, - "volume": 24944 - }, - "open": "2020-11-02T01:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 158140, - "high": 155904, - "low": 15873, - "open": 15873, - "volume": 372026 - }, - "id": 2758561, - "non_hive": { - "close": 19939, - "high": 19658, - "low": 1999, - "open": 1999, - "volume": 46902 - }, - "open": "2020-11-02T01:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 200991, - "high": 159992, - "low": 302274, - "open": 159992, - "volume": 711023 - }, - "id": 2758566, - "non_hive": { - "close": 24923, - "high": 19927, - "low": 37482, - "open": 19927, - "volume": 88255 - }, - "open": "2020-11-02T01:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 16588, - "high": 16588, - "low": 15862, - "open": 15862, - "volume": 32450 - }, - "id": 2758571, - "non_hive": { - "close": 2091, - "high": 2091, - "low": 1999, - "open": 1999, - "volume": 4090 - }, - "open": "2020-11-02T02:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13000, - "high": 13000, - "low": 13000, - "open": 13000, - "volume": 13000 - }, - "id": 2758575, - "non_hive": { - "close": 1638, - "high": 1638, - "low": 1638, - "open": 1638, - "volume": 1638 - }, - "open": "2020-11-02T02:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 198, - "high": 198, - "low": 198, - "open": 198, - "volume": 198 - }, - "id": 2758578, - "non_hive": { - "close": 25, - "high": 25, - "low": 25, - "open": 25, - "volume": 25 - }, - "open": "2020-11-02T02:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 80, - "high": 2595, - "low": 80, - "open": 2595, - "volume": 7055 - }, - "id": 2758581, - "non_hive": { - "close": 10, - "high": 327, - "low": 10, - "open": 327, - "volume": 888 - }, - "open": "2020-11-02T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 34165, - "high": 16174, - "low": 34165, - "open": 16174, - "volume": 66514 - }, - "id": 2758587, - "non_hive": { - "close": 4224, - "high": 2000, - "low": 4224, - "open": 2000, - "volume": 8224 - }, - "open": "2020-11-02T03:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 16174, - "high": 17666, - "low": 16174, - "open": 17666, - "volume": 33840 - }, - "id": 2758590, - "non_hive": { - "close": 2000, - "high": 2207, - "low": 2000, - "open": 2207, - "volume": 4207 - }, - "open": "2020-11-02T03:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 8757, - "high": 8757, - "low": 16143, - "open": 16143, - "volume": 57186 - }, - "id": 2758595, - "non_hive": { - "close": 1085, - "high": 1085, - "low": 2000, - "open": 2000, - "volume": 7085 - }, - "open": "2020-11-02T03:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 107857, - "high": 145213, - "low": 107857, - "open": 145213, - "volume": 253070 - }, - "id": 2758604, - "non_hive": { - "close": 13326, - "high": 17942, - "low": 13326, - "open": 17942, - "volume": 31268 - }, - "open": "2020-11-02T03:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1143078, - "high": 1143078, - "low": 16000, - "open": 16000, - "volume": 1478731 - }, - "id": 2758607, - "non_hive": { - "close": 142884, - "high": 142884, - "low": 1999, - "open": 1999, - "volume": 184835 - }, - "open": "2020-11-02T04:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 14568, - "high": 14568, - "low": 1000, - "open": 1000, - "volume": 15568 - }, - "id": 2758611, - "non_hive": { - "close": 1835, - "high": 1835, - "low": 125, - "open": 125, - "volume": 1960 - }, - "open": "2020-11-02T04:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4612, - "high": 4612, - "low": 1302, - "open": 1302, - "volume": 5914 - }, - "id": 2758615, - "non_hive": { - "close": 581, - "high": 581, - "low": 164, - "open": 164, - "volume": 745 - }, - "open": "2020-11-02T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7923, - "high": 7923, - "low": 7923, - "open": 7923, - "volume": 7923 - }, - "id": 2758620, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-11-02T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 9276, - "high": 9276, - "low": 3335, - "open": 3335, - "volume": 12611 - }, - "id": 2758623, - "non_hive": { - "close": 1169, - "high": 1169, - "low": 420, - "open": 420, - "volume": 1589 - }, - "open": "2020-11-02T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 64341, - "high": 64341, - "low": 15668, - "open": 15668, - "volume": 95677 - }, - "id": 2758626, - "non_hive": { - "close": 8213, - "high": 8213, - "low": 1999, - "open": 1999, - "volume": 12211 - }, - "open": "2020-11-02T04:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1128, - "high": 1128, - "low": 1128, - "open": 1128, - "volume": 1128 - }, - "id": 2758631, - "non_hive": { - "close": 144, - "high": 144, - "low": 144, - "open": 144, - "volume": 144 - }, - "open": "2020-11-02T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 5432, - "high": 5432, - "low": 5432, - "open": 5432, - "volume": 5432 - }, - "id": 2758635, - "non_hive": { - "close": 693, - "high": 693, - "low": 693, - "open": 693, - "volume": 693 - }, - "open": "2020-11-02T05:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 3051, - "high": 1098, - "low": 3051, - "open": 1098, - "volume": 4149 - }, - "id": 2758638, - "non_hive": { - "close": 389, - "high": 140, - "low": 389, - "open": 140, - "volume": 529 - }, - "open": "2020-11-02T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 793837, - "high": 2454, - "low": 793837, - "open": 2454, - "volume": 812167 - }, - "id": 2758641, - "non_hive": { - "close": 100000, - "high": 310, - "low": 100000, - "open": 310, - "volume": 102310 - }, - "open": "2020-11-02T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1717248, - "high": 31500, - "low": 1717248, - "open": 31500, - "volume": 1825738 - }, - "id": 2758646, - "non_hive": { - "close": 214656, - "high": 3968, - "low": 214656, - "open": 3968, - "volume": 228258 - }, - "open": "2020-11-02T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 15079, - "high": 16185, - "low": 15079, - "open": 16186, - "volume": 233592 - }, - "id": 2758653, - "non_hive": { - "close": 1863, - "high": 2000, - "low": 1863, - "open": 2000, - "volume": 28863 - }, - "open": "2020-11-02T06:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 4552, - "high": 4552, - "low": 16000, - "open": 16000, - "volume": 20552 - }, - "id": 2758659, - "non_hive": { - "close": 569, - "high": 569, - "low": 1999, - "open": 1999, - "volume": 2568 - }, - "open": "2020-11-02T06:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 802, - "high": 802, - "low": 802, - "open": 802, - "volume": 802 - }, - "id": 2758662, - "non_hive": { - "close": 100, - "high": 100, - "low": 100, - "open": 100, - "volume": 100 - }, - "open": "2020-11-02T06:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6602, - "high": 6602, - "low": 6602, - "open": 6602, - "volume": 6602 - }, - "id": 2758665, - "non_hive": { - "close": 815, - "high": 815, - "low": 815, - "open": 815, - "volume": 815 - }, - "open": "2020-11-02T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 835913, - "high": 835913, - "low": 153991, - "open": 153991, - "volume": 989904 - }, - "id": 2758669, - "non_hive": { - "close": 103180, - "high": 103180, - "low": 18995, - "open": 18995, - "volume": 122175 - }, - "open": "2020-11-02T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 305, - "high": 305, - "low": 1727, - "open": 16203, - "volume": 3476579 - }, - "id": 2758674, - "non_hive": { - "close": 39, - "high": 39, - "low": 213, - "open": 1999, - "volume": 439942 - }, - "open": "2020-11-02T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 193405, - "high": 239169, - "low": 5096, - "open": 5096, - "volume": 1816858 - }, - "id": 2758685, - "non_hive": { - "close": 24689, - "high": 30531, - "low": 649, - "open": 649, - "volume": 231921 - }, - "open": "2020-11-02T07:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 619682, - "high": 194995, - "low": 619682, - "open": 194995, - "volume": 1009672 - }, - "id": 2758692, - "non_hive": { - "close": 79105, - "high": 24892, - "low": 79105, - "open": 24892, - "volume": 128889 - }, - "open": "2020-11-02T07:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 204, - "high": 11210, - "low": 204, - "open": 11210, - "volume": 152421 - }, - "id": 2758698, - "non_hive": { - "close": 26, - "high": 1431, - "low": 26, - "open": 1431, - "volume": 19457 - }, - "open": "2020-11-02T08:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 15599, - "high": 15599, - "low": 15599, - "open": 15599, - "volume": 15599 - }, - "id": 2758704, - "non_hive": { - "close": 1981, - "high": 1981, - "low": 1981, - "open": 1981, - "volume": 1981 - }, - "open": "2020-11-02T08:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2614, - "high": 2614, - "low": 37390, - "open": 37390, - "volume": 40004 - }, - "id": 2758707, - "non_hive": { - "close": 332, - "high": 332, - "low": 4748, - "open": 4748, - "volume": 5080 - }, - "open": "2020-11-02T08:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3000, - "high": 3000, - "low": 3000, - "open": 3000, - "volume": 3000 - }, - "id": 2758710, - "non_hive": { - "close": 380, - "high": 380, - "low": 380, - "open": 380, - "volume": 380 - }, - "open": "2020-11-02T08:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 3395, - "high": 63, - "low": 3395, - "open": 63, - "volume": 3458 - }, - "id": 2758713, - "non_hive": { - "close": 431, - "high": 8, - "low": 431, - "open": 8, - "volume": 439 - }, - "open": "2020-11-02T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 10, - "high": 2501, - "low": 10, - "open": 2501, - "volume": 6832 - }, - "id": 2758716, - "non_hive": { - "close": 1, - "high": 312, - "low": 1, - "open": 312, - "volume": 852 - }, - "open": "2020-11-02T08:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 983872, - "high": 16128, - "low": 983872, - "open": 16128, - "volume": 1000000 - }, - "id": 2758721, - "non_hive": { - "close": 122000, - "high": 2000, - "low": 122000, - "open": 2000, - "volume": 124000 - }, - "open": "2020-11-02T09:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 44873, - "high": 3506, - "low": 44873, - "open": 15760, - "volume": 118206 - }, - "id": 2758725, - "non_hive": { - "close": 5564, - "high": 445, - "low": 5564, - "open": 1999, - "volume": 14822 - }, - "open": "2020-11-02T09:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 8082, - "high": 8082, - "low": 1792, - "open": 1792, - "volume": 9874 - }, - "id": 2758732, - "non_hive": { - "close": 1024, - "high": 1024, - "low": 227, - "open": 227, - "volume": 1251 - }, - "open": "2020-11-02T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 15777, - "high": 15777, - "low": 15777, - "open": 15777, - "volume": 15777 - }, - "id": 2758737, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-02T09:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 350, - "high": 350, - "low": 350, - "open": 350, - "volume": 350 - }, - "id": 2758740, - "non_hive": { - "close": 44, - "high": 44, - "low": 44, - "open": 44, - "volume": 44 - }, - "open": "2020-11-02T10:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2565, - "high": 2565, - "low": 100430, - "open": 100430, - "volume": 102995 - }, - "id": 2758744, - "non_hive": { - "close": 327, - "high": 327, - "low": 12801, - "open": 12801, - "volume": 13128 - }, - "open": "2020-11-02T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 31342, - "high": 31342, - "low": 31342, - "open": 31342, - "volume": 31342 - }, - "id": 2758749, - "non_hive": { - "close": 3995, - "high": 3995, - "low": 3995, - "open": 3995, - "volume": 3995 - }, - "open": "2020-11-02T10:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 5189, - "high": 5189, - "low": 5189, - "open": 5189, - "volume": 5189 - }, - "id": 2758752, - "non_hive": { - "close": 661, - "high": 661, - "low": 661, - "open": 661, - "volume": 661 - }, - "open": "2020-11-02T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 8675, - "high": 8675, - "low": 8675, - "open": 8675, - "volume": 8675 - }, - "id": 2758755, - "non_hive": { - "close": 1105, - "high": 1105, - "low": 1105, - "open": 1105, - "volume": 1105 - }, - "open": "2020-11-02T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 17868, - "high": 17868, - "low": 44873, - "open": 44873, - "volume": 62741 - }, - "id": 2758758, - "non_hive": { - "close": 2276, - "high": 2276, - "low": 5715, - "open": 5715, - "volume": 7991 - }, - "open": "2020-11-02T10:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 14485, - "high": 86, - "low": 14485, - "open": 123000, - "volume": 137571 - }, - "id": 2758761, - "non_hive": { - "close": 1845, - "high": 11, - "low": 1845, - "open": 15667, - "volume": 17523 - }, - "open": "2020-11-02T10:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13807, - "high": 557, - "low": 13807, - "open": 7851, - "volume": 39695 - }, - "id": 2758766, - "non_hive": { - "close": 1735, - "high": 71, - "low": 1735, - "open": 1000, - "volume": 5006 - }, - "open": "2020-11-02T11:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 127, - "high": 15783, - "low": 15909, - "open": 15909, - "volume": 31819 - }, - "id": 2758772, - "non_hive": { - "close": 16, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4016 - }, - "open": "2020-11-02T11:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 15702, - "high": 15702, - "low": 1647, - "open": 15783, - "volume": 84010 - }, - "id": 2758777, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 207, - "open": 2000, - "volume": 10652 - }, - "open": "2020-11-02T11:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 4917, - "high": 4917, - "low": 4917, - "open": 4917, - "volume": 4917 - }, - "id": 2758788, - "non_hive": { - "close": 626, - "high": 626, - "low": 626, - "open": 626, - "volume": 626 - }, - "open": "2020-11-02T11:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 7918, - "high": 7918, - "low": 7918, - "open": 7918, - "volume": 7918 - }, - "id": 2758791, - "non_hive": { - "close": 1008, - "high": 1008, - "low": 1008, - "open": 1008, - "volume": 1008 - }, - "open": "2020-11-02T11:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 283, - "high": 35357, - "low": 283, - "open": 15711, - "volume": 67062 - }, - "id": 2758794, - "non_hive": { - "close": 36, - "high": 4501, - "low": 36, - "open": 1999, - "volume": 8535 - }, - "open": "2020-11-02T11:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6193, - "high": 17407, - "low": 6193, - "open": 17407, - "volume": 23600 - }, - "id": 2758801, - "non_hive": { - "close": 787, - "high": 2213, - "low": 787, - "open": 2213, - "volume": 3000 - }, - "open": "2020-11-02T12:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 91161, - "high": 91161, - "low": 91161, - "open": 91161, - "volume": 91161 - }, - "id": 2758805, - "non_hive": { - "close": 11590, - "high": 11590, - "low": 11590, - "open": 11590, - "volume": 11590 - }, - "open": "2020-11-02T12:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 78, - "high": 133, - "low": 78, - "open": 23597, - "volume": 348808 - }, - "id": 2758808, - "non_hive": { - "close": 9, - "high": 17, - "low": 9, - "open": 3000, - "volume": 44345 - }, - "open": "2020-11-02T12:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1054, - "high": 251, - "low": 1054, - "open": 251, - "volume": 1305 - }, - "id": 2758816, - "non_hive": { - "close": 134, - "high": 32, - "low": 134, - "open": 32, - "volume": 166 - }, - "open": "2020-11-02T12:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 39355, - "high": 39355, - "low": 14169, - "open": 14169, - "volume": 53524 - }, - "id": 2758821, - "non_hive": { - "close": 5000, - "high": 5000, - "low": 1800, - "open": 1800, - "volume": 6800 - }, - "open": "2020-11-02T12:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 15742, - "high": 15742, - "low": 7856, - "open": 7878, - "volume": 31476 - }, - "id": 2758826, - "non_hive": { - "close": 2001, - "high": 2001, - "low": 998, - "open": 1001, - "volume": 4000 - }, - "open": "2020-11-02T12:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 11640, - "high": 527, - "low": 11640, - "open": 11567, - "volume": 6384657 - }, - "id": 2758833, - "non_hive": { - "close": 1463, - "high": 67, - "low": 1463, - "open": 1469, - "volume": 802519 - }, - "open": "2020-11-02T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 220, - "high": 220, - "low": 220, - "open": 220, - "volume": 220 - }, - "id": 2758840, - "non_hive": { - "close": 28, - "high": 28, - "low": 28, - "open": 28, - "volume": 28 - }, - "open": "2020-11-02T13:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 10333, - "high": 10333, - "low": 15873, - "open": 15873, - "volume": 26206 - }, - "id": 2758844, - "non_hive": { - "close": 1302, - "high": 1302, - "low": 1999, - "open": 1999, - "volume": 3301 - }, - "open": "2020-11-02T13:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 8767, - "high": 8767, - "low": 8767, - "open": 8767, - "volume": 8767 - }, - "id": 2758847, - "non_hive": { - "close": 1104, - "high": 1104, - "low": 1104, - "open": 1104, - "volume": 1104 - }, - "open": "2020-11-02T13:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 199992, - "high": 15999, - "low": 48, - "open": 15999, - "volume": 242943 - }, - "id": 2758850, - "non_hive": { - "close": 24999, - "high": 2000, - "low": 6, - "open": 2000, - "volume": 30368 - }, - "open": "2020-11-02T13:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 13670, - "high": 143992, - "low": 16000, - "open": 16000, - "volume": 173662 - }, - "id": 2758853, - "non_hive": { - "close": 1708, - "high": 17999, - "low": 1999, - "open": 1999, - "volume": 21706 - }, - "open": "2020-11-02T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 12608, - "high": 12608, - "low": 2330, - "open": 2330, - "volume": 14938 - }, - "id": 2758858, - "non_hive": { - "close": 1576, - "high": 1576, - "low": 291, - "open": 291, - "volume": 1867 - }, - "open": "2020-11-02T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 928, - "high": 1000, - "low": 6998, - "open": 1000, - "volume": 187759 - }, - "id": 2758862, - "non_hive": { - "close": 116, - "high": 125, - "low": 874, - "open": 125, - "volume": 23468 - }, - "open": "2020-11-02T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 832, - "high": 832, - "low": 832, - "open": 832, - "volume": 832 - }, - "id": 2758876, - "non_hive": { - "close": 104, - "high": 104, - "low": 104, - "open": 104, - "volume": 104 - }, - "open": "2020-11-02T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6722, - "high": 6722, - "low": 6722, - "open": 6722, - "volume": 6722 - }, - "id": 2758879, - "non_hive": { - "close": 837, - "high": 837, - "low": 837, - "open": 837, - "volume": 837 - }, - "open": "2020-11-02T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3344, - "high": 3344, - "low": 6722, - "open": 6722, - "volume": 10066 - }, - "id": 2758882, - "non_hive": { - "close": 418, - "high": 418, - "low": 840, - "open": 840, - "volume": 1258 - }, - "open": "2020-11-02T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 1808, - "high": 260768, - "low": 260768, - "open": 260768, - "volume": 262576 - }, - "id": 2758885, - "non_hive": { - "close": 226, - "high": 32596, - "low": 32596, - "open": 32596, - "volume": 32822 - }, - "open": "2020-11-02T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 809544, - "high": 16, - "low": 809544, - "open": 16, - "volume": 1177949 - }, - "id": 2758890, - "non_hive": { - "close": 100000, - "high": 2, - "low": 100000, - "open": 2, - "volume": 145840 - }, - "open": "2020-11-02T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 17098, - "high": 63920, - "low": 10373, - "open": 10373, - "volume": 396337 - }, - "id": 2758899, - "non_hive": { - "close": 2102, - "high": 7990, - "low": 1275, - "open": 1275, - "volume": 49385 - }, - "open": "2020-11-02T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 13057, - "high": 13057, - "low": 11097, - "open": 11097, - "volume": 24154 - }, - "id": 2758907, - "non_hive": { - "close": 1630, - "high": 1630, - "low": 1364, - "open": 1364, - "volume": 2994 - }, - "open": "2020-11-02T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 10620, - "high": 6486, - "low": 10620, - "open": 2957, - "volume": 20063 - }, - "id": 2758912, - "non_hive": { - "close": 1306, - "high": 810, - "low": 1306, - "open": 369, - "volume": 2485 - }, - "open": "2020-11-02T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 265665, - "high": 265665, - "low": 11596, - "open": 100104, - "volume": 532053 - }, - "id": 2758920, - "non_hive": { - "close": 33180, - "high": 33180, - "low": 1447, - "open": 12500, - "volume": 66446 - }, - "open": "2020-11-02T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 16128, - "high": 16128, - "low": 16128, - "open": 16128, - "volume": 72610 - }, - "id": 2758924, - "non_hive": { - "close": 1999, - "high": 2000, - "low": 1999, - "open": 2000, - "volume": 9003 - }, - "open": "2020-11-02T15:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 21178, - "high": 21178, - "low": 21178, - "open": 21178, - "volume": 21178 - }, - "id": 2758931, - "non_hive": { - "close": 2626, - "high": 2626, - "low": 2626, - "open": 2626, - "volume": 2626 - }, - "open": "2020-11-02T15:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 3028, - "high": 3028, - "low": 3028, - "open": 3028, - "volume": 3028 - }, - "id": 2758934, - "non_hive": { - "close": 378, - "high": 378, - "low": 378, - "open": 378, - "volume": 378 - }, - "open": "2020-11-02T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 25370, - "high": 2130, - "low": 16127, - "open": 2130, - "volume": 64962 - }, - "id": 2758937, - "non_hive": { - "close": 3146, - "high": 265, - "low": 1999, - "open": 265, - "volume": 8056 - }, - "open": "2020-11-02T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 12817, - "high": 16141, - "low": 12817, - "open": 16141, - "volume": 542425 - }, - "id": 2758941, - "non_hive": { - "close": 1575, - "high": 2000, - "low": 1575, - "open": 2000, - "volume": 66950 - }, - "open": "2020-11-02T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 13127, - "high": 5811, - "low": 15196, - "open": 1073, - "volume": 185604 - }, - "id": 2758946, - "non_hive": { - "close": 1637, - "high": 725, - "low": 1868, - "open": 133, - "volume": 23056 - }, - "open": "2020-11-02T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6203, - "high": 1002, - "low": 5192, - "open": 13000, - "volume": 51756 - }, - "id": 2758960, - "non_hive": { - "close": 764, - "high": 125, - "low": 638, - "open": 1614, - "volume": 6408 - }, - "open": "2020-11-02T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 7925, - "high": 7007, - "low": 7925, - "open": 7007, - "volume": 14932 - }, - "id": 2758968, - "non_hive": { - "close": 976, - "high": 863, - "low": 976, - "open": 863, - "volume": 1839 - }, - "open": "2020-11-02T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1915, - "high": 1915, - "low": 1915, - "open": 1915, - "volume": 1915 - }, - "id": 2758973, - "non_hive": { - "close": 235, - "high": 235, - "low": 235, - "open": 235, - "volume": 235 - }, - "open": "2020-11-02T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 32480, - "high": 162, - "low": 32480, - "open": 2940, - "volume": 60193 - }, - "id": 2758976, - "non_hive": { - "close": 3967, - "high": 20, - "low": 3967, - "open": 362, - "volume": 7373 - }, - "open": "2020-11-02T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 5320, - "high": 5793, - "low": 13006, - "open": 13006, - "volume": 56217 - }, - "id": 2758980, - "non_hive": { - "close": 663, - "high": 722, - "low": 1601, - "open": 1601, - "volume": 6984 - }, - "open": "2020-11-02T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 11906, - "high": 11906, - "low": 11906, - "open": 11906, - "volume": 11906 - }, - "id": 2758987, - "non_hive": { - "close": 1483, - "high": 1483, - "low": 1483, - "open": 1483, - "volume": 1483 - }, - "open": "2020-11-02T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1819, - "high": 1819, - "low": 1887, - "open": 1887, - "volume": 3706 - }, - "id": 2758990, - "non_hive": { - "close": 227, - "high": 227, - "low": 235, - "open": 235, - "volume": 462 - }, - "open": "2020-11-02T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 368, - "high": 368, - "low": 368, - "open": 368, - "volume": 368 - }, - "id": 2758993, - "non_hive": { - "close": 46, - "high": 46, - "low": 46, - "open": 46, - "volume": 46 - }, - "open": "2020-11-02T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 618, - "high": 618, - "low": 618, - "open": 618, - "volume": 618 - }, - "id": 2758996, - "non_hive": { - "close": 77, - "high": 77, - "low": 77, - "open": 77, - "volume": 77 - }, - "open": "2020-11-02T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 7585, - "high": 16, - "low": 7585, - "open": 16054, - "volume": 67261 - }, - "id": 2758999, - "non_hive": { - "close": 933, - "high": 2, - "low": 933, - "open": 1999, - "volume": 8322 - }, - "open": "2020-11-02T17:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 30910, - "high": 16040, - "low": 46510, - "open": 16040, - "volume": 231788 - }, - "id": 2759008, - "non_hive": { - "close": 3775, - "high": 1999, - "low": 5680, - "open": 1999, - "volume": 28388 - }, - "open": "2020-11-02T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 2228, - "high": 2228, - "low": 57002, - "open": 4093, - "volume": 189185 - }, - "id": 2759015, - "non_hive": { - "close": 277, - "high": 277, - "low": 6954, - "open": 500, - "volume": 23091 - }, - "open": "2020-11-02T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 11343, - "high": 11343, - "low": 3363, - "open": 16637, - "volume": 45194 - }, - "id": 2759021, - "non_hive": { - "close": 1411, - "high": 1411, - "low": 404, - "open": 2000, - "volume": 5537 - }, - "open": "2020-11-02T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 4405, - "high": 4405, - "low": 4405, - "open": 4405, - "volume": 4405 - }, - "id": 2759026, - "non_hive": { - "close": 548, - "high": 548, - "low": 548, - "open": 548, - "volume": 548 - }, - "open": "2020-11-02T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 74871, - "high": 74871, - "low": 74871, - "open": 74871, - "volume": 74871 - }, - "id": 2759029, - "non_hive": { - "close": 9245, - "high": 9245, - "low": 9245, - "open": 9245, - "volume": 9245 - }, - "open": "2020-11-02T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 16420, - "high": 3242, - "low": 16420, - "open": 3242, - "volume": 19662 - }, - "id": 2759032, - "non_hive": { - "close": 2036, - "high": 402, - "low": 2036, - "open": 402, - "volume": 2438 - }, - "open": "2020-11-02T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 572, - "high": 572, - "low": 572, - "open": 572, - "volume": 572 - }, - "id": 2759037, - "non_hive": { - "close": 71, - "high": 71, - "low": 71, - "open": 71, - "volume": 71 - }, - "open": "2020-11-02T18:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 961, - "high": 961, - "low": 115994, - "open": 16581, - "volume": 133536 - }, - "id": 2759041, - "non_hive": { - "close": 116, - "high": 116, - "low": 13991, - "open": 2000, - "volume": 16107 - }, - "open": "2020-11-02T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 333426, - "high": 48951, - "low": 2464, - "open": 2464, - "volume": 417258 - }, - "id": 2759047, - "non_hive": { - "close": 40981, - "high": 6063, - "low": 297, - "open": 297, - "volume": 51339 - }, - "open": "2020-11-02T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 34845, - "high": 34845, - "low": 1406, - "open": 16325, - "volume": 117695 - }, - "id": 2759052, - "non_hive": { - "close": 4282, - "high": 4282, - "low": 169, - "open": 2000, - "volume": 14450 - }, - "open": "2020-11-02T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 699, - "high": 52273, - "low": 699, - "open": 52273, - "volume": 52972 - }, - "id": 2759062, - "non_hive": { - "close": 84, - "high": 6420, - "low": 84, - "open": 6420, - "volume": 6504 - }, - "open": "2020-11-02T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 173, - "high": 19695, - "low": 173, - "open": 19695, - "volume": 19868 - }, - "id": 2759065, - "non_hive": { - "close": 20, - "high": 2419, - "low": 20, - "open": 2419, - "volume": 2439 - }, - "open": "2020-11-02T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 11577, - "high": 11577, - "low": 11577, - "open": 11577, - "volume": 11577 - }, - "id": 2759068, - "non_hive": { - "close": 1411, - "high": 1411, - "low": 1411, - "open": 1411, - "volume": 1411 - }, - "open": "2020-11-02T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 8165, - "high": 8165, - "low": 8165, - "open": 8165, - "volume": 8165 - }, - "id": 2759071, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-11-02T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 304, - "high": 36842, - "low": 304, - "open": 8158, - "volume": 45304 - }, - "id": 2759074, - "non_hive": { - "close": 37, - "high": 4512, - "low": 37, - "open": 999, - "volume": 5548 - }, - "open": "2020-11-02T18:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 11063, - "high": 11063, - "low": 11063, - "open": 11063, - "volume": 11063 - }, - "id": 2759079, - "non_hive": { - "close": 1359, - "high": 1359, - "low": 1359, - "open": 1359, - "volume": 1359 - }, - "open": "2020-11-02T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6716, - "high": 6716, - "low": 6716, - "open": 6716, - "volume": 6716 - }, - "id": 2759082, - "non_hive": { - "close": 825, - "high": 825, - "low": 825, - "open": 825, - "volume": 825 - }, - "open": "2020-11-02T19:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 8851, - "high": 8851, - "low": 8851, - "open": 8851, - "volume": 8851 - }, - "id": 2759086, - "non_hive": { - "close": 1087, - "high": 1087, - "low": 1087, - "open": 1087, - "volume": 1087 - }, - "open": "2020-11-02T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 30536, - "high": 1680, - "low": 30536, - "open": 1680, - "volume": 802506 - }, - "id": 2759089, - "non_hive": { - "close": 3683, - "high": 206, - "low": 3683, - "open": 206, - "volume": 96805 - }, - "open": "2020-11-02T19:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 4735, - "high": 4735, - "low": 4735, - "open": 4735, - "volume": 4735 - }, - "id": 2759092, - "non_hive": { - "close": 570, - "high": 570, - "low": 570, - "open": 570, - "volume": 570 - }, - "open": "2020-11-02T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1135, - "high": 1135, - "low": 1135, - "open": 1135, - "volume": 1135 - }, - "id": 2759095, - "non_hive": { - "close": 137, - "high": 137, - "low": 137, - "open": 137, - "volume": 137 - }, - "open": "2020-11-02T20:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 8274, - "high": 8274, - "low": 8274, - "open": 8274, - "volume": 8274 - }, - "id": 2759099, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-11-02T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "id": 2759102, - "non_hive": { - "close": 603, - "high": 603, - "low": 603, - "open": 603, - "volume": 603 - }, - "open": "2020-11-02T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 2103, - "high": 11831, - "low": 2103, - "open": 11831, - "volume": 13934 - }, - "id": 2759105, - "non_hive": { - "close": 253, - "high": 1427, - "low": 253, - "open": 1427, - "volume": 1680 - }, - "open": "2020-11-02T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 63294, - "high": 63294, - "low": 63294, - "open": 63294, - "volume": 63294 - }, - "id": 2759110, - "non_hive": { - "close": 7634, - "high": 7634, - "low": 7634, - "open": 7634, - "volume": 7634 - }, - "open": "2020-11-02T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 8274, - "high": 8274, - "low": 8274, - "open": 8274, - "volume": 8274 - }, - "id": 2759113, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-11-02T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 3017, - "high": 3017, - "low": 31345, - "open": 31345, - "volume": 34362 - }, - "id": 2759117, - "non_hive": { - "close": 364, - "high": 364, - "low": 3780, - "open": 3780, - "volume": 4144 - }, - "open": "2020-11-02T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 39127, - "high": 39127, - "low": 39127, - "open": 39127, - "volume": 39127 - }, - "id": 2759122, - "non_hive": { - "close": 4719, - "high": 4719, - "low": 4719, - "open": 4719, - "volume": 4719 - }, - "open": "2020-11-02T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 30148, - "high": 9625, - "low": 30148, - "open": 9625, - "volume": 107446 - }, - "id": 2759125, - "non_hive": { - "close": 3636, - "high": 1161, - "low": 3636, - "open": 1161, - "volume": 12959 - }, - "open": "2020-11-02T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 50, - "high": 4553, - "low": 50, - "open": 4553, - "volume": 4603 - }, - "id": 2759130, - "non_hive": { - "close": 6, - "high": 557, - "low": 6, - "open": 557, - "volume": 563 - }, - "open": "2020-11-02T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 18025, - "high": 18025, - "low": 18025, - "open": 18025, - "volume": 18025 - }, - "id": 2759133, - "non_hive": { - "close": 2205, - "high": 2205, - "low": 2205, - "open": 2205, - "volume": 2205 - }, - "open": "2020-11-02T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1095, - "high": 16582, - "low": 1095, - "open": 16582, - "volume": 61119 - }, - "id": 2759136, - "non_hive": { - "close": 132, - "high": 2000, - "low": 132, - "open": 2000, - "volume": 7370 - }, - "open": "2020-11-02T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 16488, - "high": 16488, - "low": 16488, - "open": 16488, - "volume": 16488 - }, - "id": 2759139, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-02T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 12062, - "high": 5096, - "low": 12062, - "open": 5096, - "volume": 57093 - }, - "id": 2759143, - "non_hive": { - "close": 1461, - "high": 618, - "low": 1461, - "open": 618, - "volume": 6920 - }, - "open": "2020-11-02T22:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 3786, - "high": 3786, - "low": 12762, - "open": 12762, - "volume": 16548 - }, - "id": 2759149, - "non_hive": { - "close": 459, - "high": 459, - "low": 1541, - "open": 1541, - "volume": 2000 - }, - "open": "2020-11-02T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 24534, - "high": 14536, - "low": 15061, - "open": 14536, - "volume": 85995 - }, - "id": 2759152, - "non_hive": { - "close": 3000, - "high": 1778, - "low": 1841, - "open": 1778, - "volume": 10516 - }, - "open": "2020-11-02T22:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 22295, - "high": 9156, - "low": 22295, - "open": 9156, - "volume": 84063 - }, - "id": 2759159, - "non_hive": { - "close": 2679, - "high": 1115, - "low": 2679, - "open": 1115, - "volume": 10119 - }, - "open": "2020-11-02T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1035, - "high": 1035, - "low": 1035, - "open": 1035, - "volume": 1035 - }, - "id": 2759164, - "non_hive": { - "close": 126, - "high": 126, - "low": 126, - "open": 126, - "volume": 126 - }, - "open": "2020-11-02T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1144, - "high": 155169, - "low": 1144, - "open": 49786, - "volume": 1149345 - }, - "id": 2759167, - "non_hive": { - "close": 139, - "high": 18968, - "low": 139, - "open": 6055, - "volume": 139938 - }, - "open": "2020-11-02T22:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 22901, - "high": 5046, - "low": 22901, - "open": 35111, - "volume": 63058 - }, - "id": 2759172, - "non_hive": { - "close": 2800, - "high": 617, - "low": 2800, - "open": 4293, - "volume": 7710 - }, - "open": "2020-11-02T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 8740, - "high": 8740, - "low": 9436, - "open": 9436, - "volume": 18176 - }, - "id": 2759177, - "non_hive": { - "close": 1068, - "high": 1068, - "low": 1147, - "open": 1147, - "volume": 2215 - }, - "open": "2020-11-02T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 180, - "high": 180, - "low": 180, - "open": 180, - "volume": 180 - }, - "id": 2759182, - "non_hive": { - "close": 22, - "high": 22, - "low": 22, - "open": 22, - "volume": 22 - }, - "open": "2020-11-02T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 516, - "high": 516, - "low": 516, - "open": 516, - "volume": 516 - }, - "id": 2759185, - "non_hive": { - "close": 63, - "high": 63, - "low": 63, - "open": 63, - "volume": 63 - }, - "open": "2020-11-02T23:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 14093, - "high": 14093, - "low": 14093, - "open": 14093, - "volume": 14093 - }, - "id": 2759188, - "non_hive": { - "close": 1723, - "high": 1723, - "low": 1723, - "open": 1723, - "volume": 1723 - }, - "open": "2020-11-02T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 766780, - "high": 43793, - "low": 766780, - "open": 43793, - "volume": 1058656 - }, - "id": 2759191, - "non_hive": { - "close": 92403, - "high": 5354, - "low": 92403, - "open": 5354, - "volume": 127922 - }, - "open": "2020-11-02T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 10001, - "high": 10001, - "low": 10001, - "open": 10001, - "volume": 10001 - }, - "id": 2759196, - "non_hive": { - "close": 1205, - "high": 1205, - "low": 1205, - "open": 1205, - "volume": 1205 - }, - "open": "2020-11-02T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 32, - "high": 4952, - "low": 32, - "open": 4952, - "volume": 4984 - }, - "id": 2759199, - "non_hive": { - "close": 3, - "high": 605, - "low": 3, - "open": 605, - "volume": 608 - }, - "open": "2020-11-03T00:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12000, - "high": 12000, - "low": 1718, - "open": 1718, - "volume": 13718 - }, - "id": 2759204, - "non_hive": { - "close": 1465, - "high": 1465, - "low": 209, - "open": 209, - "volume": 1674 - }, - "open": "2020-11-03T00:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 46, - "high": 5537, - "low": 46, - "open": 5537, - "volume": 5583 - }, - "id": 2759207, - "non_hive": { - "close": 5, - "high": 676, - "low": 5, - "open": 676, - "volume": 681 - }, - "open": "2020-11-03T00:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 8175, - "high": 8175, - "low": 8175, - "open": 8175, - "volume": 8175 - }, - "id": 2759210, - "non_hive": { - "close": 999, - "high": 999, - "low": 999, - "open": 999, - "volume": 999 - }, - "open": "2020-11-03T01:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 5972, - "high": 5972, - "low": 5972, - "open": 5972, - "volume": 5972 - }, - "id": 2759214, - "non_hive": { - "close": 729, - "high": 729, - "low": 729, - "open": 729, - "volume": 729 - }, - "open": "2020-11-03T01:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 8283, - "high": 14666, - "low": 8283, - "open": 10296, - "volume": 33245 - }, - "id": 2759217, - "non_hive": { - "close": 1012, - "high": 1792, - "low": 1012, - "open": 1258, - "volume": 4062 - }, - "open": "2020-11-03T02:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 971527, - "high": 16560, - "low": 1478, - "open": 16560, - "volume": 1410076 - }, - "id": 2759221, - "non_hive": { - "close": 117069, - "high": 2000, - "low": 177, - "open": 2000, - "volume": 169901 - }, - "open": "2020-11-03T02:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 139, - "high": 139, - "low": 139, - "open": 139, - "volume": 139 - }, - "id": 2759228, - "non_hive": { - "close": 17, - "high": 17, - "low": 17, - "open": 17, - "volume": 17 - }, - "open": "2020-11-03T02:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 50, - "high": 16665, - "low": 18525, - "open": 16665, - "volume": 35240 - }, - "id": 2759231, - "non_hive": { - "close": 6, - "high": 2000, - "low": 2223, - "open": 2000, - "volume": 4229 - }, - "open": "2020-11-03T02:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 2175, - "high": 2175, - "low": 2175, - "open": 2175, - "volume": 2175 - }, - "id": 2759234, - "non_hive": { - "close": 261, - "high": 261, - "low": 261, - "open": 261, - "volume": 261 - }, - "open": "2020-11-03T02:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 36908, - "high": 408, - "low": 36908, - "open": 408, - "volume": 37316 - }, - "id": 2759237, - "non_hive": { - "close": 4429, - "high": 49, - "low": 4429, - "open": 49, - "volume": 4478 - }, - "open": "2020-11-03T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 32742, - "high": 32742, - "low": 32742, - "open": 32742, - "volume": 32742 - }, - "id": 2759242, - "non_hive": { - "close": 3929, - "high": 3929, - "low": 3929, - "open": 3929, - "volume": 3929 - }, - "open": "2020-11-03T03:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 13, - "high": 659, - "low": 13, - "open": 659, - "volume": 672 - }, - "id": 2759246, - "non_hive": { - "close": 1, - "high": 79, - "low": 1, - "open": 79, - "volume": 80 - }, - "open": "2020-11-03T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 300, - "high": 300, - "low": 300, - "open": 300, - "volume": 300 - }, - "id": 2759249, - "non_hive": { - "close": 36, - "high": 36, - "low": 36, - "open": 36, - "volume": 36 - }, - "open": "2020-11-03T03:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 48642, - "high": 48642, - "low": 48642, - "open": 48642, - "volume": 48642 - }, - "id": 2759252, - "non_hive": { - "close": 5803, - "high": 5803, - "low": 5803, - "open": 5803, - "volume": 5803 - }, - "open": "2020-11-03T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 6627, - "high": 16806, - "low": 7746, - "open": 16806, - "volume": 47985 - }, - "id": 2759255, - "non_hive": { - "close": 788, - "high": 2000, - "low": 921, - "open": 2000, - "volume": 5709 - }, - "open": "2020-11-03T03:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 30103, - "high": 2445, - "low": 30103, - "open": 2445, - "volume": 32548 - }, - "id": 2759263, - "non_hive": { - "close": 3582, - "high": 291, - "low": 3582, - "open": 291, - "volume": 3873 - }, - "open": "2020-11-03T03:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1858, - "high": 923, - "low": 1858, - "open": 923, - "volume": 5890 - }, - "id": 2759266, - "non_hive": { - "close": 221, - "high": 110, - "low": 221, - "open": 110, - "volume": 701 - }, - "open": "2020-11-03T03:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 17650, - "high": 17650, - "low": 17650, - "open": 17650, - "volume": 17650 - }, - "id": 2759270, - "non_hive": { - "close": 2096, - "high": 2096, - "low": 2096, - "open": 2096, - "volume": 2096 - }, - "open": "2020-11-03T03:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 277, - "high": 24040, - "low": 277, - "open": 24040, - "volume": 24317 - }, - "id": 2759273, - "non_hive": { - "close": 32, - "high": 2856, - "low": 32, - "open": 2856, - "volume": 2888 - }, - "open": "2020-11-03T04:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1095, - "high": 1095, - "low": 1095, - "open": 1095, - "volume": 1095 - }, - "id": 2759277, - "non_hive": { - "close": 130, - "high": 130, - "low": 130, - "open": 130, - "volume": 130 - }, - "open": "2020-11-03T04:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 6968, - "high": 8, - "low": 6968, - "open": 11729, - "volume": 18705 - }, - "id": 2759280, - "non_hive": { - "close": 822, - "high": 1, - "low": 822, - "open": 1384, - "volume": 2207 - }, - "open": "2020-11-03T04:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 11699, - "high": 11699, - "low": 11699, - "open": 11699, - "volume": 11699 - }, - "id": 2759285, - "non_hive": { - "close": 1402, - "high": 1402, - "low": 1402, - "open": 1402, - "volume": 1402 - }, - "open": "2020-11-03T04:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 20000, - "high": 20000, - "low": 20000, - "open": 20000, - "volume": 20000 - }, - "id": 2759288, - "non_hive": { - "close": 2396, - "high": 2396, - "low": 2396, - "open": 2396, - "volume": 2396 - }, - "open": "2020-11-03T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 893, - "high": 893, - "low": 893, - "open": 893, - "volume": 893 - }, - "id": 2759292, - "non_hive": { - "close": 107, - "high": 107, - "low": 107, - "open": 107, - "volume": 107 - }, - "open": "2020-11-03T05:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 16695, - "high": 16695, - "low": 16695, - "open": 16695, - "volume": 16695 - }, - "id": 2759295, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T05:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 14350, - "high": 14350, - "low": 14350, - "open": 14350, - "volume": 14350 - }, - "id": 2759298, - "non_hive": { - "close": 1719, - "high": 1719, - "low": 1719, - "open": 1719, - "volume": 1719 - }, - "open": "2020-11-03T05:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 11770, - "high": 83518, - "low": 11770, - "open": 83518, - "volume": 112090 - }, - "id": 2759301, - "non_hive": { - "close": 1401, - "high": 10000, - "low": 1401, - "open": 10000, - "volume": 13401 - }, - "open": "2020-11-03T05:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 18000, - "high": 14000, - "low": 905, - "open": 905, - "volume": 64797 - }, - "id": 2759307, - "non_hive": { - "close": 2143, - "high": 1667, - "low": 107, - "open": 107, - "volume": 7713 - }, - "open": "2020-11-03T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 48, - "high": 20099, - "low": 48, - "open": 20099, - "volume": 20147 - }, - "id": 2759315, - "non_hive": { - "close": 5, - "high": 2394, - "low": 5, - "open": 2394, - "volume": 2399 - }, - "open": "2020-11-03T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 174, - "high": 10507, - "low": 174, - "open": 10507, - "volume": 10681 - }, - "id": 2759318, - "non_hive": { - "close": 20, - "high": 1249, - "low": 20, - "open": 1249, - "volume": 1269 - }, - "open": "2020-11-03T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 11677, - "high": 11677, - "low": 11677, - "open": 11677, - "volume": 11677 - }, - "id": 2759322, - "non_hive": { - "close": 1398, - "high": 1398, - "low": 1398, - "open": 1398, - "volume": 1398 - }, - "open": "2020-11-03T06:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 694, - "high": 309, - "low": 694, - "open": 16697, - "volume": 50089 - }, - "id": 2759325, - "non_hive": { - "close": 83, - "high": 37, - "low": 83, - "open": 1999, - "volume": 5997 - }, - "open": "2020-11-03T06:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 425, - "high": 8, - "low": 149, - "open": 30002, - "volume": 68592 - }, - "id": 2759333, - "non_hive": { - "close": 51, - "high": 1, - "low": 17, - "open": 3594, - "volume": 8214 - }, - "open": "2020-11-03T06:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 10266, - "high": 10266, - "low": 10266, - "open": 10266, - "volume": 10266 - }, - "id": 2759342, - "non_hive": { - "close": 1229, - "high": 1229, - "low": 1229, - "open": 1229, - "volume": 1229 - }, - "open": "2020-11-03T07:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1773, - "high": 1773, - "low": 1773, - "open": 1773, - "volume": 1773 - }, - "id": 2759346, - "non_hive": { - "close": 212, - "high": 212, - "low": 212, - "open": 212, - "volume": 212 - }, - "open": "2020-11-03T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 7853, - "high": 367, - "low": 1202, - "open": 18846, - "volume": 28268 - }, - "id": 2759349, - "non_hive": { - "close": 940, - "high": 44, - "low": 143, - "open": 2257, - "volume": 3384 - }, - "open": "2020-11-03T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 25227, - "high": 25227, - "low": 12000, - "open": 12000, - "volume": 37227 - }, - "id": 2759356, - "non_hive": { - "close": 3022, - "high": 3022, - "low": 1437, - "open": 1437, - "volume": 4459 - }, - "open": "2020-11-03T07:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 51, - "high": 30379, - "low": 51, - "open": 30379, - "volume": 482845 - }, - "id": 2759361, - "non_hive": { - "close": 6, - "high": 3639, - "low": 6, - "open": 3639, - "volume": 57023 - }, - "open": "2020-11-03T07:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 9609, - "high": 9609, - "low": 9609, - "open": 9609, - "volume": 9609 - }, - "id": 2759367, - "non_hive": { - "close": 1133, - "high": 1133, - "low": 1133, - "open": 1133, - "volume": 1133 - }, - "open": "2020-11-03T07:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 23, - "high": 24073, - "low": 23, - "open": 7329, - "volume": 42114 - }, - "id": 2759370, - "non_hive": { - "close": 2, - "high": 2839, - "low": 2, - "open": 864, - "volume": 4965 - }, - "open": "2020-11-03T07:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1832, - "high": 1832, - "low": 10978, - "open": 10978, - "volume": 12810 - }, - "id": 2759378, - "non_hive": { - "close": 216, - "high": 216, - "low": 1294, - "open": 1294, - "volume": 1510 - }, - "open": "2020-11-03T08:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3683, - "high": 5566, - "low": 3683, - "open": 5566, - "volume": 9249 - }, - "id": 2759384, - "non_hive": { - "close": 434, - "high": 656, - "low": 434, - "open": 656, - "volume": 1090 - }, - "open": "2020-11-03T08:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1737, - "high": 1737, - "low": 11967, - "open": 11967, - "volume": 36321 - }, - "id": 2759387, - "non_hive": { - "close": 205, - "high": 205, - "low": 1410, - "open": 1410, - "volume": 4281 - }, - "open": "2020-11-03T08:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 246333, - "high": 246333, - "low": 14000, - "open": 14000, - "volume": 260333 - }, - "id": 2759392, - "non_hive": { - "close": 29056, - "high": 29056, - "low": 1651, - "open": 1651, - "volume": 30707 - }, - "open": "2020-11-03T08:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 128959, - "high": 128959, - "low": 128959, - "open": 128959, - "volume": 128959 - }, - "id": 2759395, - "non_hive": { - "close": 15212, - "high": 15212, - "low": 15212, - "open": 15212, - "volume": 15212 - }, - "open": "2020-11-03T09:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 15107, - "high": 15107, - "low": 15107, - "open": 15107, - "volume": 15107 - }, - "id": 2759399, - "non_hive": { - "close": 1782, - "high": 1782, - "low": 1782, - "open": 1782, - "volume": 1782 - }, - "open": "2020-11-03T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3611, - "high": 3611, - "low": 2391, - "open": 2391, - "volume": 6002 - }, - "id": 2759402, - "non_hive": { - "close": 426, - "high": 426, - "low": 282, - "open": 282, - "volume": 708 - }, - "open": "2020-11-03T09:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 16810, - "high": 16810, - "low": 16810, - "open": 16810, - "volume": 16810 - }, - "id": 2759405, - "non_hive": { - "close": 1983, - "high": 1983, - "low": 1983, - "open": 1983, - "volume": 1983 - }, - "open": "2020-11-03T09:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 7809, - "high": 1430, - "low": 7809, - "open": 343, - "volume": 9922 - }, - "id": 2759408, - "non_hive": { - "close": 913, - "high": 171, - "low": 913, - "open": 41, - "volume": 1165 - }, - "open": "2020-11-03T09:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1004, - "high": 1004, - "low": 1004, - "open": 1004, - "volume": 1004 - }, - "id": 2759413, - "non_hive": { - "close": 120, - "high": 120, - "low": 120, - "open": 120, - "volume": 120 - }, - "open": "2020-11-03T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 449151, - "high": 449151, - "low": 6828, - "open": 6828, - "volume": 455979 - }, - "id": 2759416, - "non_hive": { - "close": 53700, - "high": 53700, - "low": 816, - "open": 816, - "volume": 54516 - }, - "open": "2020-11-03T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 9, - "high": 219128, - "low": 9, - "open": 219128, - "volume": 887921 - }, - "id": 2759422, - "non_hive": { - "close": 1, - "high": 26199, - "low": 1, - "open": 26199, - "volume": 106127 - }, - "open": "2020-11-03T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 111, - "high": 888, - "low": 111, - "open": 888, - "volume": 999 - }, - "id": 2759426, - "non_hive": { - "close": 13, - "high": 106, - "low": 13, - "open": 106, - "volume": 119 - }, - "open": "2020-11-03T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 16, - "high": 1104, - "low": 16, - "open": 1104, - "volume": 1120 - }, - "id": 2759430, - "non_hive": { - "close": 1, - "high": 132, - "low": 1, - "open": 132, - "volume": 133 - }, - "open": "2020-11-03T10:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3600, - "high": 3600, - "low": 3600, - "open": 3600, - "volume": 3600 - }, - "id": 2759433, - "non_hive": { - "close": 430, - "high": 430, - "low": 430, - "open": 430, - "volume": 430 - }, - "open": "2020-11-03T11:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 73115, - "high": 9796, - "low": 73115, - "open": 1623, - "volume": 101623 - }, - "id": 2759437, - "non_hive": { - "close": 8556, - "high": 1170, - "low": 8556, - "open": 193, - "volume": 11919 - }, - "open": "2020-11-03T11:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 24046, - "high": 24046, - "low": 24046, - "open": 24046, - "volume": 24046 - }, - "id": 2759442, - "non_hive": { - "close": 2813, - "high": 2813, - "low": 2813, - "open": 2813, - "volume": 2813 - }, - "open": "2020-11-03T11:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 64968, - "high": 25082, - "low": 17091, - "open": 17091, - "volume": 258654 - }, - "id": 2759445, - "non_hive": { - "close": 7668, - "high": 2995, - "low": 2000, - "open": 2000, - "volume": 30652 - }, - "open": "2020-11-03T11:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 100000, - "high": 100000, - "low": 100000, - "open": 100000, - "volume": 100000 - }, - "id": 2759460, - "non_hive": { - "close": 11802, - "high": 11802, - "low": 11802, - "open": 11802, - "volume": 11802 - }, - "open": "2020-11-03T11:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 516, - "high": 32503, - "low": 516, - "open": 32503, - "volume": 33019 - }, - "id": 2759463, - "non_hive": { - "close": 60, - "high": 3879, - "low": 60, - "open": 3879, - "volume": 3939 - }, - "open": "2020-11-03T11:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 4650, - "high": 37926, - "low": 4650, - "open": 37926, - "volume": 42576 - }, - "id": 2759466, - "non_hive": { - "close": 555, - "high": 4527, - "low": 555, - "open": 4527, - "volume": 5082 - }, - "open": "2020-11-03T12:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 671, - "high": 50276, - "low": 671, - "open": 50276, - "volume": 50947 - }, - "id": 2759470, - "non_hive": { - "close": 80, - "high": 6000, - "low": 80, - "open": 6000, - "volume": 6080 - }, - "open": "2020-11-03T12:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 57424, - "high": 57424, - "low": 57424, - "open": 57424, - "volume": 57424 - }, - "id": 2759475, - "non_hive": { - "close": 6852, - "high": 6852, - "low": 6852, - "open": 6852, - "volume": 6852 - }, - "open": "2020-11-03T12:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7035, - "high": 14715, - "low": 7035, - "open": 14715, - "volume": 88794 - }, - "id": 2759478, - "non_hive": { - "close": 839, - "high": 1756, - "low": 839, - "open": 1756, - "volume": 10591 - }, - "open": "2020-11-03T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 2249, - "high": 8490, - "low": 8934, - "open": 8490, - "volume": 20755 - }, - "id": 2759489, - "non_hive": { - "close": 268, - "high": 1013, - "low": 1064, - "open": 1013, - "volume": 2474 - }, - "open": "2020-11-03T12:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 71, - "high": 12097, - "low": 71, - "open": 12097, - "volume": 12168 - }, - "id": 2759494, - "non_hive": { - "close": 8, - "high": 1443, - "low": 8, - "open": 1443, - "volume": 1451 - }, - "open": "2020-11-03T12:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 277, - "high": 519, - "low": 80, - "open": 4662, - "volume": 17918 - }, - "id": 2759499, - "non_hive": { - "close": 33, - "high": 62, - "low": 9, - "open": 556, - "volume": 2137 - }, - "open": "2020-11-03T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 159, - "high": 10141, - "low": 159, - "open": 10141, - "volume": 10300 - }, - "id": 2759506, - "non_hive": { - "close": 18, - "high": 1210, - "low": 18, - "open": 1210, - "volume": 1228 - }, - "open": "2020-11-03T13:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 10002, - "high": 10002, - "low": 10002, - "open": 10002, - "volume": 10002 - }, - "id": 2759510, - "non_hive": { - "close": 1194, - "high": 1194, - "low": 1194, - "open": 1194, - "volume": 1194 - }, - "open": "2020-11-03T13:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 3093, - "high": 3093, - "low": 3093, - "open": 3093, - "volume": 3093 - }, - "id": 2759513, - "non_hive": { - "close": 365, - "high": 365, - "low": 365, - "open": 365, - "volume": 365 - }, - "open": "2020-11-03T13:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 25088, - "high": 25088, - "low": 16751, - "open": 16751, - "volume": 41839 - }, - "id": 2759516, - "non_hive": { - "close": 2995, - "high": 2995, - "low": 1999, - "open": 1999, - "volume": 4994 - }, - "open": "2020-11-03T13:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 14045, - "high": 14045, - "low": 14045, - "open": 14045, - "volume": 14045 - }, - "id": 2759519, - "non_hive": { - "close": 1676, - "high": 1676, - "low": 1676, - "open": 1676, - "volume": 1676 - }, - "open": "2020-11-03T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 428642, - "high": 16943, - "low": 428642, - "open": 16943, - "volume": 590528 - }, - "id": 2759522, - "non_hive": { - "close": 50578, - "high": 2000, - "low": 50578, - "open": 2000, - "volume": 69685 - }, - "open": "2020-11-03T13:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 251, - "high": 251, - "low": 251, - "open": 251, - "volume": 251 - }, - "id": 2759526, - "non_hive": { - "close": 30, - "high": 30, - "low": 30, - "open": 30, - "volume": 30 - }, - "open": "2020-11-03T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 8, - "high": 8, - "low": 16692, - "open": 16692, - "volume": 16700 - }, - "id": 2759530, - "non_hive": { - "close": 1, - "high": 1, - "low": 1992, - "open": 1992, - "volume": 1993 - }, - "open": "2020-11-03T14:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 49723, - "high": 49723, - "low": 49723, - "open": 49723, - "volume": 49723 - }, - "id": 2759533, - "non_hive": { - "close": 5935, - "high": 5935, - "low": 5935, - "open": 5935, - "volume": 5935 - }, - "open": "2020-11-03T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 500550, - "high": 209461, - "low": 500550, - "open": 209461, - "volume": 710011 - }, - "id": 2759536, - "non_hive": { - "close": 59740, - "high": 24999, - "low": 59740, - "open": 24999, - "volume": 84739 - }, - "open": "2020-11-03T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 25, - "high": 25, - "low": 207926, - "open": 207926, - "volume": 207951 - }, - "id": 2759539, - "non_hive": { - "close": 3, - "high": 3, - "low": 24818, - "open": 24818, - "volume": 24821 - }, - "open": "2020-11-03T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 42376, - "high": 93, - "low": 42376, - "open": 93, - "volume": 42469 - }, - "id": 2759544, - "non_hive": { - "close": 4957, - "high": 11, - "low": 4957, - "open": 11, - "volume": 4968 - }, - "open": "2020-11-03T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 9894, - "high": 8368, - "low": 9894, - "open": 8368, - "volume": 35276 - }, - "id": 2759549, - "non_hive": { - "close": 1181, - "high": 999, - "low": 1181, - "open": 999, - "volume": 4211 - }, - "open": "2020-11-03T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 972, - "high": 972, - "low": 972, - "open": 972, - "volume": 972 - }, - "id": 2759554, - "non_hive": { - "close": 116, - "high": 116, - "low": 116, - "open": 116, - "volume": 116 - }, - "open": "2020-11-03T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 133016, - "high": 62449, - "low": 133016, - "open": 164724, - "volume": 690972 - }, - "id": 2759557, - "non_hive": { - "close": 15695, - "high": 7457, - "low": 15695, - "open": 19653, - "volume": 82293 - }, - "open": "2020-11-03T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 6661, - "high": 6661, - "low": 220606, - "open": 16945, - "volume": 244212 - }, - "id": 2759565, - "non_hive": { - "close": 795, - "high": 795, - "low": 26035, - "open": 2000, - "volume": 28830 - }, - "open": "2020-11-03T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1091, - "high": 234467, - "low": 135751, - "open": 16945, - "volume": 1252324 - }, - "id": 2759569, - "non_hive": { - "close": 130, - "high": 27970, - "low": 16018, - "open": 2000, - "volume": 148117 - }, - "open": "2020-11-03T15:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 378585, - "high": 16824, - "low": 378585, - "open": 1915, - "volume": 829520 - }, - "id": 2759574, - "non_hive": { - "close": 44673, - "high": 2000, - "low": 44673, - "open": 227, - "volume": 97957 - }, - "open": "2020-11-03T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 19105, - "high": 167, - "low": 19105, - "open": 167, - "volume": 25657 - }, - "id": 2759579, - "non_hive": { - "close": 2280, - "high": 20, - "low": 2280, - "open": 20, - "volume": 3062 - }, - "open": "2020-11-03T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 80547, - "high": 54450, - "low": 80547, - "open": 16750, - "volume": 211200 - }, - "id": 2759584, - "non_hive": { - "close": 9471, - "high": 6501, - "low": 9471, - "open": 1999, - "volume": 24965 - }, - "open": "2020-11-03T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 51754, - "high": 9503, - "low": 51754, - "open": 16829, - "volume": 156408 - }, - "id": 2759589, - "non_hive": { - "close": 6085, - "high": 1134, - "low": 6085, - "open": 2000, - "volume": 18527 - }, - "open": "2020-11-03T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 154520, - "high": 9642, - "low": 154520, - "open": 9642, - "volume": 164162 - }, - "id": 2759602, - "non_hive": { - "close": 18170, - "high": 1134, - "low": 18170, - "open": 1134, - "volume": 19304 - }, - "open": "2020-11-03T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 156, - "high": 10174, - "low": 156, - "open": 16756, - "volume": 27086 - }, - "id": 2759605, - "non_hive": { - "close": 18, - "high": 1214, - "low": 18, - "open": 1999, - "volume": 3231 - }, - "open": "2020-11-03T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 16998, - "high": 16998, - "low": 16998, - "open": 16998, - "volume": 16998 - }, - "id": 2759610, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 17007, - "high": 17007, - "low": 17007, - "open": 17007, - "volume": 17007 - }, - "id": 2759613, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7654, - "high": 12722, - "low": 7654, - "open": 12722, - "volume": 20376 - }, - "id": 2759616, - "non_hive": { - "close": 900, - "high": 1496, - "low": 900, - "open": 1496, - "volume": 2396 - }, - "open": "2020-11-03T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 18418, - "high": 18418, - "low": 18418, - "open": 18418, - "volume": 18418 - }, - "id": 2759619, - "non_hive": { - "close": 2164, - "high": 2164, - "low": 2164, - "open": 2164, - "volume": 2164 - }, - "open": "2020-11-03T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 10583, - "high": 62299, - "low": 10583, - "open": 62299, - "volume": 72882 - }, - "id": 2759622, - "non_hive": { - "close": 1244, - "high": 7326, - "low": 1244, - "open": 7326, - "volume": 8570 - }, - "open": "2020-11-03T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 17015, - "high": 17015, - "low": 17015, - "open": 17015, - "volume": 51045 - }, - "id": 2759625, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 5997 - }, - "open": "2020-11-03T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 800830, - "high": 17015, - "low": 800830, - "open": 17015, - "volume": 840173 - }, - "id": 2759631, - "non_hive": { - "close": 93697, - "high": 1999, - "low": 93697, - "open": 1999, - "volume": 98310 - }, - "open": "2020-11-03T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 75893, - "high": 16718, - "low": 75893, - "open": 17094, - "volume": 193936 - }, - "id": 2759636, - "non_hive": { - "close": 8839, - "high": 1956, - "low": 8839, - "open": 1999, - "volume": 22606 - }, - "open": "2020-11-03T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12907, - "high": 12907, - "low": 12907, - "open": 12907, - "volume": 12907 - }, - "id": 2759642, - "non_hive": { - "close": 1517, - "high": 1517, - "low": 1517, - "open": 1517, - "volume": 1517 - }, - "open": "2020-11-03T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 17091, - "high": 17091, - "low": 17091, - "open": 17091, - "volume": 17091 - }, - "id": 2759645, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T17:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 17089, - "high": 12926, - "low": 4162, - "open": 17090, - "volume": 81229 - }, - "id": 2759648, - "non_hive": { - "close": 2000, - "high": 1519, - "low": 487, - "open": 2000, - "volume": 9513 - }, - "open": "2020-11-03T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 17094, - "high": 17094, - "low": 9741, - "open": 17094, - "volume": 95211 - }, - "id": 2759658, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1134, - "open": 1999, - "volume": 11129 - }, - "open": "2020-11-03T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 9783, - "high": 9783, - "low": 17094, - "open": 17094, - "volume": 61063 - }, - "id": 2759667, - "non_hive": { - "close": 1150, - "high": 1150, - "low": 2000, - "open": 2000, - "volume": 7150 - }, - "open": "2020-11-03T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 17089, - "high": 17089, - "low": 17089, - "open": 17089, - "volume": 17089 - }, - "id": 2759675, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 17093, - "high": 17093, - "low": 31130, - "open": 17090, - "volume": 82480 - }, - "id": 2759678, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 3626, - "open": 1999, - "volume": 9625 - }, - "open": "2020-11-03T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 70517, - "high": 9776, - "low": 111991, - "open": 9776, - "volume": 278366 - }, - "id": 2759685, - "non_hive": { - "close": 8180, - "high": 1144, - "low": 12991, - "open": 1144, - "volume": 32342 - }, - "open": "2020-11-03T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 129310, - "high": 129310, - "low": 129310, - "open": 129310, - "volume": 129310 - }, - "id": 2759692, - "non_hive": { - "close": 15000, - "high": 15000, - "low": 15000, - "open": 15000, - "volume": 15000 - }, - "open": "2020-11-03T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 9, - "high": 1973100, - "low": 9, - "open": 1973100, - "volume": 1973109 - }, - "id": 2759695, - "non_hive": { - "close": 1, - "high": 228879, - "low": 1, - "open": 228879, - "volume": 228880 - }, - "open": "2020-11-03T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 30432, - "high": 10747, - "low": 6846, - "open": 10747, - "volume": 58198 - }, - "id": 2759701, - "non_hive": { - "close": 3576, - "high": 1263, - "low": 804, - "open": 1263, - "volume": 6838 - }, - "open": "2020-11-03T18:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 20800, - "high": 20800, - "low": 20800, - "open": 20800, - "volume": 20800 - }, - "id": 2759707, - "non_hive": { - "close": 2443, - "high": 2443, - "low": 2443, - "open": 2443, - "volume": 2443 - }, - "open": "2020-11-03T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 49312, - "high": 49312, - "low": 1584, - "open": 1584, - "volume": 67903 - }, - "id": 2759710, - "non_hive": { - "close": 5799, - "high": 5799, - "low": 185, - "open": 185, - "volume": 7983 - }, - "open": "2020-11-03T18:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1796, - "high": 9414, - "low": 1796, - "open": 9414, - "volume": 11210 - }, - "id": 2759715, - "non_hive": { - "close": 211, - "high": 1106, - "low": 211, - "open": 1106, - "volume": 1317 - }, - "open": "2020-11-03T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 17036, - "high": 93753, - "low": 17036, - "open": 5806, - "volume": 133631 - }, - "id": 2759721, - "non_hive": { - "close": 1999, - "high": 11014, - "low": 1999, - "open": 682, - "volume": 15694 - }, - "open": "2020-11-03T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3345, - "high": 3345, - "low": 3345, - "open": 3345, - "volume": 3345 - }, - "id": 2759729, - "non_hive": { - "close": 393, - "high": 393, - "low": 393, - "open": 393, - "volume": 393 - }, - "open": "2020-11-03T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 17025, - "high": 22396, - "low": 17025, - "open": 22396, - "volume": 39421 - }, - "id": 2759732, - "non_hive": { - "close": 1999, - "high": 2631, - "low": 1999, - "open": 2631, - "volume": 4630 - }, - "open": "2020-11-03T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 866, - "high": 2925, - "low": 866, - "open": 17016, - "volume": 33807 - }, - "id": 2759737, - "non_hive": { - "close": 101, - "high": 344, - "low": 101, - "open": 1999, - "volume": 3972 - }, - "open": "2020-11-03T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 5136, - "high": 5136, - "low": 5136, - "open": 5136, - "volume": 5136 - }, - "id": 2759742, - "non_hive": { - "close": 604, - "high": 604, - "low": 604, - "open": 604, - "volume": 604 - }, - "open": "2020-11-03T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 88941, - "high": 88941, - "low": 88941, - "open": 88941, - "volume": 88941 - }, - "id": 2759745, - "non_hive": { - "close": 10459, - "high": 10459, - "low": 10459, - "open": 10459, - "volume": 10459 - }, - "open": "2020-11-03T20:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 16998, - "high": 16998, - "low": 16998, - "open": 16998, - "volume": 67992 - }, - "id": 2759749, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 8000 - }, - "open": "2020-11-03T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 42169, - "high": 42169, - "low": 42169, - "open": 42169, - "volume": 42169 - }, - "id": 2759757, - "non_hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "open": "2020-11-03T20:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 16907, - "high": 42243, - "low": 368, - "open": 42243, - "volume": 73863 - }, - "id": 2759760, - "non_hive": { - "close": 1999, - "high": 5000, - "low": 43, - "open": 5000, - "volume": 8739 - }, - "open": "2020-11-03T20:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12, - "high": 16909, - "low": 12, - "open": 16909, - "volume": 35380 - }, - "id": 2759769, - "non_hive": { - "close": 1, - "high": 1999, - "low": 1, - "open": 1999, - "volume": 4180 - }, - "open": "2020-11-03T20:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16633, - "high": 16633, - "low": 16633, - "open": 16633, - "volume": 16633 - }, - "id": 2759776, - "non_hive": { - "close": 1967, - "high": 1967, - "low": 1967, - "open": 1967, - "volume": 1967 - }, - "open": "2020-11-03T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 21649, - "high": 21649, - "low": 3305, - "open": 3305, - "volume": 25198 - }, - "id": 2759779, - "non_hive": { - "close": 2584, - "high": 2584, - "low": 392, - "open": 392, - "volume": 3005 - }, - "open": "2020-11-03T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12284, - "high": 12284, - "low": 12284, - "open": 12284, - "volume": 12284 - }, - "id": 2759784, - "non_hive": { - "close": 1466, - "high": 1466, - "low": 1466, - "open": 1466, - "volume": 1466 - }, - "open": "2020-11-03T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 24078, - "high": 24078, - "low": 24078, - "open": 24078, - "volume": 24078 - }, - "id": 2759787, - "non_hive": { - "close": 2865, - "high": 2865, - "low": 2865, - "open": 2865, - "volume": 2865 - }, - "open": "2020-11-03T20:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 57958, - "high": 57958, - "low": 57958, - "open": 57958, - "volume": 57958 - }, - "id": 2759790, - "non_hive": { - "close": 6897, - "high": 6897, - "low": 6897, - "open": 6897, - "volume": 6897 - }, - "open": "2020-11-03T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 10226, - "high": 10226, - "low": 10226, - "open": 10226, - "volume": 10226 - }, - "id": 2759794, - "non_hive": { - "close": 1221, - "high": 1221, - "low": 1221, - "open": 1221, - "volume": 1221 - }, - "open": "2020-11-03T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 41566, - "high": 11164, - "low": 41566, - "open": 11164, - "volume": 52730 - }, - "id": 2759797, - "non_hive": { - "close": 4963, - "high": 1333, - "low": 4963, - "open": 1333, - "volume": 6296 - }, - "open": "2020-11-03T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 16784, - "high": 20100, - "low": 140, - "open": 17069, - "volume": 67820 - }, - "id": 2759802, - "non_hive": { - "close": 2004, - "high": 2400, - "low": 16, - "open": 2038, - "volume": 8097 - }, - "open": "2020-11-03T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 16807, - "high": 16805, - "low": 16807, - "open": 16805, - "volume": 53612 - }, - "id": 2759809, - "non_hive": { - "close": 1999, - "high": 2000, - "low": 1999, - "open": 2000, - "volume": 6379 - }, - "open": "2020-11-03T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 176303, - "high": 50, - "low": 9469, - "open": 16805, - "volume": 250053 - }, - "id": 2759814, - "non_hive": { - "close": 20980, - "high": 6, - "low": 1126, - "open": 2000, - "volume": 29755 - }, - "open": "2020-11-03T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 15756, - "high": 3292, - "low": 15756, - "open": 3292, - "volume": 19048 - }, - "id": 2759823, - "non_hive": { - "close": 1875, - "high": 393, - "low": 1875, - "open": 393, - "volume": 2268 - }, - "open": "2020-11-03T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 20209, - "high": 16805, - "low": 20, - "open": 16805, - "volume": 129797 - }, - "id": 2759826, - "non_hive": { - "close": 2404, - "high": 2000, - "low": 2, - "open": 2000, - "volume": 15440 - }, - "open": "2020-11-03T21:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 26143, - "high": 1294, - "low": 1305, - "open": 1294, - "volume": 44452 - }, - "id": 2759836, - "non_hive": { - "close": 3110, - "high": 154, - "low": 153, - "open": 154, - "volume": 5286 - }, - "open": "2020-11-03T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 50445, - "high": 50445, - "low": 50445, - "open": 50445, - "volume": 50445 - }, - "id": 2759846, - "non_hive": { - "close": 6001, - "high": 6001, - "low": 6001, - "open": 6001, - "volume": 6001 - }, - "open": "2020-11-03T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 146621, - "high": 14379, - "low": 146621, - "open": 14379, - "volume": 161000 - }, - "id": 2759849, - "non_hive": { - "close": 17301, - "high": 1711, - "low": 17301, - "open": 1711, - "volume": 19012 - }, - "open": "2020-11-03T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 61712, - "high": 16996, - "low": 61712, - "open": 16996, - "volume": 78708 - }, - "id": 2759853, - "non_hive": { - "close": 7261, - "high": 2000, - "low": 7261, - "open": 2000, - "volume": 9261 - }, - "open": "2020-11-03T22:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 621, - "high": 16891, - "low": 115, - "open": 16808, - "volume": 92160 - }, - "id": 2759856, - "non_hive": { - "close": 73, - "high": 2010, - "low": 13, - "open": 1999, - "volume": 10960 - }, - "open": "2020-11-03T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 8404, - "high": 266555, - "low": 924, - "open": 924, - "volume": 1104110 - }, - "id": 2759862, - "non_hive": { - "close": 1000, - "high": 31720, - "low": 109, - "open": 109, - "volume": 131382 - }, - "open": "2020-11-03T22:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 24112, - "high": 16808, - "low": 8474, - "open": 8474, - "volume": 70476 - }, - "id": 2759867, - "non_hive": { - "close": 2869, - "high": 2000, - "low": 1000, - "open": 1000, - "volume": 8377 - }, - "open": "2020-11-03T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 4252, - "high": 99008, - "low": 4252, - "open": 16999, - "volume": 341413 - }, - "id": 2759877, - "non_hive": { - "close": 500, - "high": 11782, - "low": 500, - "open": 2000, - "volume": 40415 - }, - "open": "2020-11-03T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 2126, - "high": 176419, - "low": 2126, - "open": 4252, - "volume": 189133 - }, - "id": 2759888, - "non_hive": { - "close": 252, - "high": 20953, - "low": 250, - "open": 505, - "volume": 22460 - }, - "open": "2020-11-03T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 4252, - "high": 6327, - "low": 2126, - "open": 6327, - "volume": 14831 - }, - "id": 2759897, - "non_hive": { - "close": 500, - "high": 750, - "low": 250, - "open": 750, - "volume": 1752 - }, - "open": "2020-11-03T22:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 546, - "high": 546, - "low": 2126, - "open": 2126, - "volume": 13252 - }, - "id": 2759906, - "non_hive": { - "close": 65, - "high": 65, - "low": 250, - "open": 250, - "volume": 1569 - }, - "open": "2020-11-03T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 4252 - }, - "id": 2759915, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 500 - }, - "open": "2020-11-03T23:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 4252 - }, - "id": 2759920, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 500 - }, - "open": "2020-11-03T23:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 851, - "high": 851, - "low": 851, - "open": 851, - "volume": 851 - }, - "id": 2759925, - "non_hive": { - "close": 100, - "high": 100, - "low": 100, - "open": 100, - "volume": 100 - }, - "open": "2020-11-03T23:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 447, - "high": 7028, - "low": 447, - "open": 12601, - "volume": 24291 - }, - "id": 2759928, - "non_hive": { - "close": 53, - "high": 836, - "low": 53, - "open": 1498, - "volume": 2888 - }, - "open": "2020-11-03T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 5162, - "high": 5162, - "low": 5162, - "open": 5162, - "volume": 5162 - }, - "id": 2759934, - "non_hive": { - "close": 614, - "high": 614, - "low": 614, - "open": 614, - "volume": 614 - }, - "open": "2020-11-03T23:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759937, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 250 - }, - "open": "2020-11-03T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 6375, - "high": 6375, - "low": 6375, - "open": 6375, - "volume": 6375 - }, - "id": 2759940, - "non_hive": { - "close": 750, - "high": 750, - "low": 750, - "open": 750, - "volume": 750 - }, - "open": "2020-11-03T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1757, - "high": 1757, - "low": 1757, - "open": 1757, - "volume": 1757 - }, - "id": 2759943, - "non_hive": { - "close": 209, - "high": 209, - "low": 209, - "open": 209, - "volume": 209 - }, - "open": "2020-11-03T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 850, - "high": 3371, - "low": 6375, - "open": 3371, - "volume": 13996 - }, - "id": 2759946, - "non_hive": { - "close": 100, - "high": 401, - "low": 750, - "open": 401, - "volume": 1651 - }, - "open": "2020-11-03T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 10178, - "high": 10178, - "low": 21765, - "open": 16997, - "volume": 286023 - }, - "id": 2759955, - "non_hive": { - "close": 1210, - "high": 1210, - "low": 2559, - "open": 2000, - "volume": 33663 - }, - "open": "2020-11-04T00:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 340, - "high": 850, - "low": 850, - "open": 850, - "volume": 3740 - }, - "id": 2759963, - "non_hive": { - "close": 40, - "high": 100, - "low": 100, - "open": 100, - "volume": 440 - }, - "open": "2020-11-04T00:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2712730, - "high": 504, - "low": 2712730, - "open": 504, - "volume": 8000000 - }, - "id": 2759966, - "non_hive": { - "close": 314947, - "high": 60, - "low": 314947, - "open": 60, - "volume": 929103 - }, - "open": "2020-11-04T00:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 455, - "high": 455, - "low": 455, - "open": 455, - "volume": 455 - }, - "id": 2759971, - "non_hive": { - "close": 54, - "high": 54, - "low": 54, - "open": 54, - "volume": 54 - }, - "open": "2020-11-04T00:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 88, - "high": 765, - "low": 88, - "open": 11004, - "volume": 264630 - }, - "id": 2759974, - "non_hive": { - "close": 10, - "high": 91, - "low": 10, - "open": 1308, - "volume": 31459 - }, - "open": "2020-11-04T00:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 598, - "high": 16220, - "low": 55, - "open": 3635, - "volume": 37326 - }, - "id": 2759982, - "non_hive": { - "close": 71, - "high": 1928, - "low": 6, - "open": 432, - "volume": 4436 - }, - "open": "2020-11-04T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 542, - "high": 35208, - "low": 542, - "open": 35208, - "volume": 35750 - }, - "id": 2759991, - "non_hive": { - "close": 62, - "high": 4185, - "low": 62, - "open": 4185, - "volume": 4247 - }, - "open": "2020-11-04T00:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 683, - "high": 683, - "low": 1600, - "open": 1600, - "volume": 19819 - }, - "id": 2759994, - "non_hive": { - "close": 81, - "high": 81, - "low": 187, - "open": 187, - "volume": 2337 - }, - "open": "2020-11-04T01:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3155, - "high": 3155, - "low": 3155, - "open": 3155, - "volume": 3155 - }, - "id": 2760000, - "non_hive": { - "close": 374, - "high": 374, - "low": 374, - "open": 374, - "volume": 374 - }, - "open": "2020-11-04T01:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 3787, - "high": 3787, - "low": 4647, - "open": 4647, - "volume": 8434 - }, - "id": 2760003, - "non_hive": { - "close": 449, - "high": 449, - "low": 550, - "open": 550, - "volume": 999 - }, - "open": "2020-11-04T01:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 96324, - "high": 96324, - "low": 6058, - "open": 6058, - "volume": 1240019 - }, - "id": 2760006, - "non_hive": { - "close": 11455, - "high": 11455, - "low": 718, - "open": 718, - "volume": 147461 - }, - "open": "2020-11-04T01:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 29042, - "high": 17221, - "low": 28138, - "open": 17221, - "volume": 181743 - }, - "id": 2760010, - "non_hive": { - "close": 3372, - "high": 2000, - "low": 3267, - "open": 2000, - "volume": 21104 - }, - "open": "2020-11-04T01:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1455, - "high": 1455, - "low": 1455, - "open": 1455, - "volume": 1455 - }, - "id": 2760018, - "non_hive": { - "close": 173, - "high": 173, - "low": 173, - "open": 173, - "volume": 173 - }, - "open": "2020-11-04T01:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1564, - "high": 1564, - "low": 1564, - "open": 1564, - "volume": 1564 - }, - "id": 2760021, - "non_hive": { - "close": 186, - "high": 186, - "low": 186, - "open": 186, - "volume": 186 - }, - "open": "2020-11-04T01:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 78665, - "high": 13967, - "low": 253022, - "open": 13967, - "volume": 345654 - }, - "id": 2760024, - "non_hive": { - "close": 9135, - "high": 1622, - "low": 29382, - "open": 1622, - "volume": 40139 - }, - "open": "2020-11-04T02:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 71570, - "high": 71570, - "low": 71570, - "open": 71570, - "volume": 71570 - }, - "id": 2760031, - "non_hive": { - "close": 8311, - "high": 8311, - "low": 8311, - "open": 8311, - "volume": 8311 - }, - "open": "2020-11-04T02:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 12417, - "high": 12417, - "low": 12417, - "open": 12417, - "volume": 12417 - }, - "id": 2760034, - "non_hive": { - "close": 1442, - "high": 1442, - "low": 1442, - "open": 1442, - "volume": 1442 - }, - "open": "2020-11-04T02:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 5270, - "high": 5270, - "low": 5270, - "open": 5270, - "volume": 5270 - }, - "id": 2760037, - "non_hive": { - "close": 612, - "high": 612, - "low": 612, - "open": 612, - "volume": 612 - }, - "open": "2020-11-04T02:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 111, - "high": 111, - "low": 111, - "open": 111, - "volume": 111 - }, - "id": 2760040, - "non_hive": { - "close": 13, - "high": 13, - "low": 13, - "open": 13, - "volume": 13 - }, - "open": "2020-11-04T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 8456, - "high": 8456, - "low": 8456, - "open": 8456, - "volume": 8456 - }, - "id": 2760043, - "non_hive": { - "close": 982, - "high": 982, - "low": 982, - "open": 982, - "volume": 982 - }, - "open": "2020-11-04T03:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 77503, - "high": 77503, - "low": 77503, - "open": 77503, - "volume": 77503 - }, - "id": 2760047, - "non_hive": { - "close": 9000, - "high": 9000, - "low": 9000, - "open": 9000, - "volume": 9000 - }, - "open": "2020-11-04T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 23922, - "high": 23922, - "low": 4366, - "open": 4366, - "volume": 28288 - }, - "id": 2760050, - "non_hive": { - "close": 2778, - "high": 2778, - "low": 507, - "open": 507, - "volume": 3285 - }, - "open": "2020-11-04T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 20564, - "high": 20564, - "low": 20564, - "open": 20564, - "volume": 20564 - }, - "id": 2760055, - "non_hive": { - "close": 2388, - "high": 2388, - "low": 2388, - "open": 2388, - "volume": 2388 - }, - "open": "2020-11-04T03:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 55768, - "high": 55768, - "low": 70472, - "open": 18851, - "volume": 145091 - }, - "id": 2760058, - "non_hive": { - "close": 6476, - "high": 6476, - "low": 8182, - "open": 2189, - "volume": 16847 - }, - "open": "2020-11-04T03:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 40482, - "high": 40482, - "low": 40482, - "open": 40482, - "volume": 40482 - }, - "id": 2760063, - "non_hive": { - "close": 4700, - "high": 4700, - "low": 4700, - "open": 4700, - "volume": 4700 - }, - "open": "2020-11-04T03:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 17395, - "high": 17395, - "low": 17395, - "open": 17395, - "volume": 17395 - }, - "id": 2760066, - "non_hive": { - "close": 2020, - "high": 2020, - "low": 2020, - "open": 2020, - "volume": 2020 - }, - "open": "2020-11-04T04:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 21614, - "high": 21614, - "low": 21614, - "open": 21614, - "volume": 21614 - }, - "id": 2760070, - "non_hive": { - "close": 2510, - "high": 2510, - "low": 2510, - "open": 2510, - "volume": 2510 - }, - "open": "2020-11-04T04:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 9989, - "high": 9989, - "low": 9989, - "open": 9989, - "volume": 9989 - }, - "id": 2760073, - "non_hive": { - "close": 1160, - "high": 1160, - "low": 1160, - "open": 1160, - "volume": 1160 - }, - "open": "2020-11-04T04:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 49721, - "high": 750279, - "low": 49721, - "open": 750279, - "volume": 800000 - }, - "id": 2760076, - "non_hive": { - "close": 5772, - "high": 87118, - "low": 5772, - "open": 87118, - "volume": 92890 - }, - "open": "2020-11-04T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 25850, - "high": 25850, - "low": 25850, - "open": 25850, - "volume": 25850 - }, - "id": 2760079, - "non_hive": { - "close": 3001, - "high": 3001, - "low": 3001, - "open": 3001, - "volume": 3001 - }, - "open": "2020-11-04T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 12262, - "high": 12262, - "low": 12262, - "open": 12262, - "volume": 12262 - }, - "id": 2760082, - "non_hive": { - "close": 1423, - "high": 1423, - "low": 1423, - "open": 1423, - "volume": 1423 - }, - "open": "2020-11-04T04:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 23288, - "high": 23288, - "low": 23288, - "open": 23288, - "volume": 23288 - }, - "id": 2760085, - "non_hive": { - "close": 2704, - "high": 2704, - "low": 2704, - "open": 2704, - "volume": 2704 - }, - "open": "2020-11-04T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 3814, - "high": 3814, - "low": 3814, - "open": 3814, - "volume": 3814 - }, - "id": 2760088, - "non_hive": { - "close": 442, - "high": 442, - "low": 442, - "open": 442, - "volume": 442 - }, - "open": "2020-11-04T05:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 567585, - "high": 567585, - "low": 4850, - "open": 4850, - "volume": 756969 - }, - "id": 2760092, - "non_hive": { - "close": 67449, - "high": 67449, - "low": 563, - "open": 563, - "volume": 89480 - }, - "open": "2020-11-04T05:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 14422, - "high": 16661, - "low": 324, - "open": 16830, - "volume": 48237 - }, - "id": 2760095, - "non_hive": { - "close": 1713, - "high": 1979, - "low": 37, - "open": 1999, - "volume": 5728 - }, - "open": "2020-11-04T06:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13960, - "high": 13960, - "low": 2408, - "open": 2408, - "volume": 16368 - }, - "id": 2760102, - "non_hive": { - "close": 1659, - "high": 1659, - "low": 286, - "open": 286, - "volume": 1945 - }, - "open": "2020-11-04T06:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7282, - "high": 7282, - "low": 7282, - "open": 7282, - "volume": 7282 - }, - "id": 2760105, - "non_hive": { - "close": 865, - "high": 865, - "low": 865, - "open": 865, - "volume": 865 - }, - "open": "2020-11-04T06:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3845, - "high": 3845, - "low": 67, - "open": 4362, - "volume": 15978 - }, - "id": 2760108, - "non_hive": { - "close": 457, - "high": 457, - "low": 7, - "open": 518, - "volume": 1897 - }, - "open": "2020-11-04T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 791, - "high": 791, - "low": 791, - "open": 791, - "volume": 791 - }, - "id": 2760113, - "non_hive": { - "close": 94, - "high": 94, - "low": 94, - "open": 94, - "volume": 94 - }, - "open": "2020-11-04T07:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 307876, - "high": 22393, - "low": 307876, - "open": 22393, - "volume": 2022393 - }, - "id": 2760117, - "non_hive": { - "close": 35097, - "high": 2661, - "low": 35097, - "open": 2661, - "volume": 231985 - }, - "open": "2020-11-04T07:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 3124, - "high": 3124, - "low": 3124, - "open": 3124, - "volume": 3124 - }, - "id": 2760124, - "non_hive": { - "close": 369, - "high": 369, - "low": 369, - "open": 369, - "volume": 369 - }, - "open": "2020-11-04T07:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 16931, - "high": 16931, - "low": 15299, - "open": 15299, - "volume": 32230 - }, - "id": 2760127, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1806, - "open": 1806, - "volume": 3805 - }, - "open": "2020-11-04T08:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 708, - "high": 12932, - "low": 708, - "open": 12932, - "volume": 40012 - }, - "id": 2760133, - "non_hive": { - "close": 80, - "high": 1528, - "low": 80, - "open": 1528, - "volume": 4721 - }, - "open": "2020-11-04T08:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 6003, - "high": 6003, - "low": 17000, - "open": 17000, - "volume": 23003 - }, - "id": 2760138, - "non_hive": { - "close": 709, - "high": 709, - "low": 2007, - "open": 2007, - "volume": 2716 - }, - "open": "2020-11-04T08:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 507, - "high": 372, - "low": 507, - "open": 10923, - "volume": 43199 - }, - "id": 2760143, - "non_hive": { - "close": 57, - "high": 44, - "low": 57, - "open": 1290, - "volume": 5101 - }, - "open": "2020-11-04T08:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 4244, - "high": 6692, - "low": 16946, - "open": 6692, - "volume": 27882 - }, - "id": 2760146, - "non_hive": { - "close": 501, - "high": 790, - "low": 1999, - "open": 790, - "volume": 3290 - }, - "open": "2020-11-04T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 194, - "high": 194, - "low": 486, - "open": 486, - "volume": 680 - }, - "id": 2760151, - "non_hive": { - "close": 23, - "high": 23, - "low": 57, - "open": 57, - "volume": 80 - }, - "open": "2020-11-04T08:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 5085, - "high": 5085, - "low": 5085, - "open": 5085, - "volume": 5085 - }, - "id": 2760156, - "non_hive": { - "close": 600, - "high": 600, - "low": 600, - "open": 600, - "volume": 600 - }, - "open": "2020-11-04T09:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 14742, - "high": 305, - "low": 14742, - "open": 305, - "volume": 15047 - }, - "id": 2760160, - "non_hive": { - "close": 1740, - "high": 36, - "low": 1740, - "open": 36, - "volume": 1776 - }, - "open": "2020-11-04T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 11511, - "high": 8489, - "low": 11511, - "open": 8489, - "volume": 20000 - }, - "id": 2760165, - "non_hive": { - "close": 1350, - "high": 1000, - "low": 1350, - "open": 1000, - "volume": 2350 - }, - "open": "2020-11-04T09:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 25, - "high": 25, - "low": 89412, - "open": 89412, - "volume": 89437 - }, - "id": 2760168, - "non_hive": { - "close": 3, - "high": 3, - "low": 10550, - "open": 10550, - "volume": 10553 - }, - "open": "2020-11-04T09:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 60944, - "high": 60944, - "low": 60944, - "open": 60944, - "volume": 60944 - }, - "id": 2760171, - "non_hive": { - "close": 7189, - "high": 7189, - "low": 7189, - "open": 7189, - "volume": 7189 - }, - "open": "2020-11-04T09:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 11953, - "high": 17503, - "low": 11953, - "open": 17503, - "volume": 51929 - }, - "id": 2760174, - "non_hive": { - "close": 1362, - "high": 2000, - "low": 1362, - "open": 2000, - "volume": 5926 - }, - "open": "2020-11-04T10:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 2458, - "high": 17542, - "low": 2458, - "open": 17542, - "volume": 20000 - }, - "id": 2760178, - "non_hive": { - "close": 280, - "high": 2000, - "low": 280, - "open": 2000, - "volume": 2280 - }, - "open": "2020-11-04T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2698, - "high": 2698, - "low": 2698, - "open": 2698, - "volume": 2698 - }, - "id": 2760181, - "non_hive": { - "close": 313, - "high": 313, - "low": 313, - "open": 313, - "volume": 313 - }, - "open": "2020-11-04T10:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 8694, - "high": 8694, - "low": 8694, - "open": 8694, - "volume": 8694 - }, - "id": 2760184, - "non_hive": { - "close": 1008, - "high": 1008, - "low": 1008, - "open": 1008, - "volume": 1008 - }, - "open": "2020-11-04T10:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 9090, - "high": 9090, - "low": 9090, - "open": 9090, - "volume": 9090 - }, - "id": 2760187, - "non_hive": { - "close": 1054, - "high": 1054, - "low": 1054, - "open": 1054, - "volume": 1054 - }, - "open": "2020-11-04T10:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 85873, - "high": 85873, - "low": 85873, - "open": 85873, - "volume": 85873 - }, - "id": 2760190, - "non_hive": { - "close": 9960, - "high": 9960, - "low": 9960, - "open": 9960, - "volume": 9960 - }, - "open": "2020-11-04T10:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 2664, - "high": 2664, - "low": 14563, - "open": 14563, - "volume": 17227 - }, - "id": 2760193, - "non_hive": { - "close": 309, - "high": 309, - "low": 1689, - "open": 1689, - "volume": 1998 - }, - "open": "2020-11-04T11:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 62873, - "high": 62873, - "low": 62873, - "open": 62873, - "volume": 62873 - }, - "id": 2760197, - "non_hive": { - "close": 7292, - "high": 7292, - "low": 7292, - "open": 7292, - "volume": 7292 - }, - "open": "2020-11-04T11:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 105, - "high": 22762, - "low": 105, - "open": 22762, - "volume": 31007 - }, - "id": 2760200, - "non_hive": { - "close": 11, - "high": 2640, - "low": 11, - "open": 2640, - "volume": 3595 - }, - "open": "2020-11-04T11:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 5006, - "high": 5006, - "low": 5006, - "open": 5006, - "volume": 5006 - }, - "id": 2760203, - "non_hive": { - "close": 580, - "high": 580, - "low": 580, - "open": 580, - "volume": 580 - }, - "open": "2020-11-04T11:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 5331, - "high": 16738, - "low": 185, - "open": 510, - "volume": 40306 - }, - "id": 2760206, - "non_hive": { - "close": 607, - "high": 1941, - "low": 21, - "open": 59, - "volume": 4628 - }, - "open": "2020-11-04T11:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 163, - "high": 163, - "low": 18870, - "open": 18870, - "volume": 19033 - }, - "id": 2760214, - "non_hive": { - "close": 19, - "high": 19, - "low": 2151, - "open": 2151, - "volume": 2170 - }, - "open": "2020-11-04T11:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 56, - "high": 3543, - "low": 56, - "open": 1472, - "volume": 104181 - }, - "id": 2760217, - "non_hive": { - "close": 6, - "high": 411, - "low": 6, - "open": 169, - "volume": 11982 - }, - "open": "2020-11-04T11:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 5864, - "high": 24136, - "low": 49, - "open": 3121, - "volume": 122674 - }, - "id": 2760227, - "non_hive": { - "close": 680, - "high": 2800, - "low": 5, - "open": 362, - "volume": 14230 - }, - "open": "2020-11-04T12:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1474, - "high": 948, - "low": 15, - "open": 948, - "volume": 5463 - }, - "id": 2760234, - "non_hive": { - "close": 168, - "high": 110, - "low": 1, - "open": 110, - "volume": 624 - }, - "open": "2020-11-04T12:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 1489, - "high": 1489, - "low": 1489, - "open": 1489, - "volume": 1489 - }, - "id": 2760239, - "non_hive": { - "close": 172, - "high": 172, - "low": 172, - "open": 172, - "volume": 172 - }, - "open": "2020-11-04T12:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 15688, - "high": 17312, - "low": 15688, - "open": 17312, - "volume": 33000 - }, - "id": 2760242, - "non_hive": { - "close": 1812, - "high": 2000, - "low": 1812, - "open": 2000, - "volume": 3812 - }, - "open": "2020-11-04T12:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 135, - "high": 6771, - "low": 135, - "open": 16985, - "volume": 60828 - }, - "id": 2760245, - "non_hive": { - "close": 15, - "high": 797, - "low": 15, - "open": 1999, - "volume": 7058 - }, - "open": "2020-11-04T12:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 2261, - "high": 2261, - "low": 2261, - "open": 2261, - "volume": 2261 - }, - "id": 2760250, - "non_hive": { - "close": 266, - "high": 266, - "low": 266, - "open": 266, - "volume": 266 - }, - "open": "2020-11-04T12:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 133145, - "high": 133145, - "low": 133145, - "open": 133145, - "volume": 133145 - }, - "id": 2760253, - "non_hive": { - "close": 15655, - "high": 15655, - "low": 15655, - "open": 15655, - "volume": 15655 - }, - "open": "2020-11-04T13:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 17767, - "high": 139760, - "low": 17767, - "open": 12196, - "volume": 169723 - }, - "id": 2760257, - "non_hive": { - "close": 2089, - "high": 16433, - "low": 2089, - "open": 1434, - "volume": 19956 - }, - "open": "2020-11-04T13:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 59, - "high": 59, - "low": 59, - "open": 59, - "volume": 59 - }, - "id": 2760262, - "non_hive": { - "close": 7, - "high": 7, - "low": 7, - "open": 7, - "volume": 7 - }, - "open": "2020-11-04T13:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 238, - "high": 238, - "low": 238, - "open": 238, - "volume": 238 - }, - "id": 2760265, - "non_hive": { - "close": 28, - "high": 28, - "low": 28, - "open": 28, - "volume": 28 - }, - "open": "2020-11-04T13:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 5592, - "high": 536, - "low": 5592, - "open": 536, - "volume": 6128 - }, - "id": 2760268, - "non_hive": { - "close": 657, - "high": 63, - "low": 657, - "open": 63, - "volume": 720 - }, - "open": "2020-11-04T13:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 78811, - "high": 78811, - "low": 9244, - "open": 9244, - "volume": 88055 - }, - "id": 2760273, - "non_hive": { - "close": 9264, - "high": 9264, - "low": 1086, - "open": 1086, - "volume": 10350 - }, - "open": "2020-11-04T13:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 11799, - "high": 11799, - "low": 11799, - "open": 11799, - "volume": 11799 - }, - "id": 2760276, - "non_hive": { - "close": 1387, - "high": 1387, - "low": 1387, - "open": 1387, - "volume": 1387 - }, - "open": "2020-11-04T13:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 30549, - "high": 30549, - "low": 30549, - "open": 30549, - "volume": 30549 - }, - "id": 2760279, - "non_hive": { - "close": 3591, - "high": 3591, - "low": 3591, - "open": 3591, - "volume": 3591 - }, - "open": "2020-11-04T13:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 8958, - "high": 8958, - "low": 8958, - "open": 8958, - "volume": 8958 - }, - "id": 2760282, - "non_hive": { - "close": 1053, - "high": 1053, - "low": 1053, - "open": 1053, - "volume": 1053 - }, - "open": "2020-11-04T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 631, - "high": 9584, - "low": 631, - "open": 9584, - "volume": 42996 - }, - "id": 2760285, - "non_hive": { - "close": 72, - "high": 1126, - "low": 72, - "open": 1126, - "volume": 5049 - }, - "open": "2020-11-04T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 62992, - "high": 62992, - "low": 62992, - "open": 62992, - "volume": 62992 - }, - "id": 2760289, - "non_hive": { - "close": 7400, - "high": 7400, - "low": 7400, - "open": 7400, - "volume": 7400 - }, - "open": "2020-11-04T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 8571, - "high": 8571, - "low": 631, - "open": 631, - "volume": 9202 - }, - "id": 2760293, - "non_hive": { - "close": 1007, - "high": 1007, - "low": 74, - "open": 74, - "volume": 1081 - }, - "open": "2020-11-04T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 17315, - "high": 17315, - "low": 2863, - "open": 17391, - "volume": 37569 - }, - "id": 2760296, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 329, - "open": 2000, - "volume": 4329 - }, - "open": "2020-11-04T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 41883, - "high": 41883, - "low": 17038, - "open": 17038, - "volume": 71382 - }, - "id": 2760301, - "non_hive": { - "close": 4914, - "high": 4914, - "low": 1999, - "open": 1999, - "volume": 8375 - }, - "open": "2020-11-04T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 17315, - "high": 2362, - "low": 17315, - "open": 2362, - "volume": 532527 - }, - "id": 2760306, - "non_hive": { - "close": 2000, - "high": 277, - "low": 2000, - "open": 277, - "volume": 61765 - }, - "open": "2020-11-04T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 155358, - "high": 17389, - "low": 155358, - "open": 17389, - "volume": 172747 - }, - "id": 2760316, - "non_hive": { - "close": 17867, - "high": 2000, - "low": 17867, - "open": 2000, - "volume": 19867 - }, - "open": "2020-11-04T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 10582, - "high": 10582, - "low": 10582, - "open": 10582, - "volume": 10582 - }, - "id": 2760319, - "non_hive": { - "close": 1227, - "high": 1227, - "low": 1227, - "open": 1227, - "volume": 1227 - }, - "open": "2020-11-04T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 11431, - "high": 1086, - "low": 5573, - "open": 1086, - "volume": 18090 - }, - "id": 2760322, - "non_hive": { - "close": 1326, - "high": 126, - "low": 646, - "open": 126, - "volume": 2098 - }, - "open": "2020-11-04T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 8617, - "high": 8624, - "low": 8617, - "open": 8624, - "volume": 17241 - }, - "id": 2760327, - "non_hive": { - "close": 999, - "high": 1000, - "low": 999, - "open": 1000, - "volume": 1999 - }, - "open": "2020-11-04T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 67272, - "high": 8526, - "low": 100, - "open": 100, - "volume": 86045 - }, - "id": 2760332, - "non_hive": { - "close": 7800, - "high": 989, - "low": 11, - "open": 11, - "volume": 9977 - }, - "open": "2020-11-04T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 656, - "high": 656, - "low": 656, - "open": 656, - "volume": 656 - }, - "id": 2760338, - "non_hive": { - "close": 76, - "high": 76, - "low": 76, - "open": 76, - "volume": 76 - }, - "open": "2020-11-04T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2760341, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-04T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 32352, - "high": 129, - "low": 32352, - "open": 17242, - "volume": 236818 - }, - "id": 2760344, - "non_hive": { - "close": 3720, - "high": 15, - "low": 3720, - "open": 2000, - "volume": 27252 - }, - "open": "2020-11-04T15:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 8633, - "high": 8633, - "low": 8633, - "open": 8633, - "volume": 8633 - }, - "id": 2760347, - "non_hive": { - "close": 1001, - "high": 1001, - "low": 1001, - "open": 1001, - "volume": 1001 - }, - "open": "2020-11-04T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 10007, - "high": 1000, - "low": 17390, - "open": 1000, - "volume": 28397 - }, - "id": 2760351, - "non_hive": { - "close": 1151, - "high": 116, - "low": 2000, - "open": 116, - "volume": 3267 - }, - "open": "2020-11-04T16:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 12209, - "high": 1486, - "low": 11993, - "open": 1486, - "volume": 25688 - }, - "id": 2760358, - "non_hive": { - "close": 1404, - "high": 171, - "low": 1379, - "open": 171, - "volume": 2954 - }, - "open": "2020-11-04T16:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 13981, - "high": 13981, - "low": 13981, - "open": 13981, - "volume": 13981 - }, - "id": 2760365, - "non_hive": { - "close": 1608, - "high": 1608, - "low": 1608, - "open": 1608, - "volume": 1608 - }, - "open": "2020-11-04T16:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 5124, - "high": 10869, - "low": 5124, - "open": 10869, - "volume": 15993 - }, - "id": 2760368, - "non_hive": { - "close": 589, - "high": 1250, - "low": 589, - "open": 1250, - "volume": 1839 - }, - "open": "2020-11-04T16:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 12221, - "high": 17563, - "low": 12221, - "open": 17000, - "volume": 48384 - }, - "id": 2760371, - "non_hive": { - "close": 1393, - "high": 2020, - "low": 1393, - "open": 1954, - "volume": 5550 - }, - "open": "2020-11-04T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 103, - "high": 11875, - "low": 103, - "open": 5323, - "volume": 196010 - }, - "id": 2760376, - "non_hive": { - "close": 11, - "high": 1365, - "low": 11, - "open": 607, - "volume": 22307 - }, - "open": "2020-11-04T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 661, - "high": 58764, - "low": 661, - "open": 8803, - "volume": 68228 - }, - "id": 2760382, - "non_hive": { - "close": 74, - "high": 6755, - "low": 74, - "open": 1011, - "volume": 7840 - }, - "open": "2020-11-04T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 3399, - "high": 3399, - "low": 17390, - "open": 17390, - "volume": 20789 - }, - "id": 2760386, - "non_hive": { - "close": 391, - "high": 391, - "low": 1999, - "open": 1999, - "volume": 2390 - }, - "open": "2020-11-04T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 17244, - "high": 73096, - "low": 468, - "open": 66599, - "volume": 193401 - }, - "id": 2760391, - "non_hive": { - "close": 1999, - "high": 8479, - "low": 53, - "open": 7659, - "volume": 22363 - }, - "open": "2020-11-04T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1771, - "high": 1771, - "low": 1771, - "open": 1771, - "volume": 1771 - }, - "id": 2760398, - "non_hive": { - "close": 205, - "high": 205, - "low": 205, - "open": 205, - "volume": 205 - }, - "open": "2020-11-04T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 870, - "high": 870, - "low": 870, - "open": 870, - "volume": 870 - }, - "id": 2760401, - "non_hive": { - "close": 101, - "high": 101, - "low": 101, - "open": 101, - "volume": 101 - }, - "open": "2020-11-04T16:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 215, - "high": 215, - "low": 215, - "open": 215, - "volume": 215 - }, - "id": 2760404, - "non_hive": { - "close": 25, - "high": 25, - "low": 25, - "open": 25, - "volume": 25 - }, - "open": "2020-11-04T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1629, - "high": 1629, - "low": 1629, - "open": 1629, - "volume": 1629 - }, - "id": 2760408, - "non_hive": { - "close": 189, - "high": 189, - "low": 189, - "open": 189, - "volume": 189 - }, - "open": "2020-11-04T17:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1783, - "high": 1783, - "low": 1783, - "open": 1783, - "volume": 1783 - }, - "id": 2760411, - "non_hive": { - "close": 206, - "high": 206, - "low": 206, - "open": 206, - "volume": 206 - }, - "open": "2020-11-04T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 37768, - "high": 13276, - "low": 37768, - "open": 13276, - "volume": 51044 - }, - "id": 2760414, - "non_hive": { - "close": 4381, - "high": 1540, - "low": 4381, - "open": 1540, - "volume": 5921 - }, - "open": "2020-11-04T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1844, - "high": 1844, - "low": 12776, - "open": 12776, - "volume": 14620 - }, - "id": 2760419, - "non_hive": { - "close": 214, - "high": 214, - "low": 1482, - "open": 1482, - "volume": 1696 - }, - "open": "2020-11-04T17:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 51, - "high": 51, - "low": 51, - "open": 51, - "volume": 51 - }, - "id": 2760424, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-04T17:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3536, - "high": 3492, - "low": 3536, - "open": 3492, - "volume": 7028 - }, - "id": 2760427, - "non_hive": { - "close": 410, - "high": 405, - "low": 410, - "open": 405, - "volume": 815 - }, - "open": "2020-11-04T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 20, - "high": 1596, - "low": 20, - "open": 1596, - "volume": 1616 - }, - "id": 2760430, - "non_hive": { - "close": 2, - "high": 185, - "low": 2, - "open": 185, - "volume": 187 - }, - "open": "2020-11-04T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 552, - "high": 58831, - "low": 207, - "open": 207, - "volume": 59590 - }, - "id": 2760433, - "non_hive": { - "close": 64, - "high": 6821, - "low": 24, - "open": 24, - "volume": 6909 - }, - "open": "2020-11-04T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 68415, - "high": 17628, - "low": 68415, - "open": 17628, - "volume": 721681 - }, - "id": 2760436, - "non_hive": { - "close": 7724, - "high": 2000, - "low": 7724, - "open": 2000, - "volume": 81534 - }, - "open": "2020-11-04T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 159986, - "high": 159986, - "low": 2814, - "open": 2814, - "volume": 180057 - }, - "id": 2760441, - "non_hive": { - "close": 18542, - "high": 18542, - "low": 325, - "open": 325, - "volume": 20866 - }, - "open": "2020-11-04T18:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 224, - "high": 224, - "low": 3286, - "open": 3286, - "volume": 20654 - }, - "id": 2760447, - "non_hive": { - "close": 26, - "high": 26, - "low": 379, - "open": 379, - "volume": 2386 - }, - "open": "2020-11-04T18:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 20, - "high": 20, - "low": 20, - "open": 20, - "volume": 20 - }, - "id": 2760450, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-11-04T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 104, - "high": 104, - "low": 104, - "open": 104, - "volume": 104 - }, - "id": 2760453, - "non_hive": { - "close": 12, - "high": 12, - "low": 12, - "open": 12, - "volume": 12 - }, - "open": "2020-11-04T19:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 3764, - "high": 3764, - "low": 3764, - "open": 3764, - "volume": 3764 - }, - "id": 2760457, - "non_hive": { - "close": 435, - "high": 435, - "low": 435, - "open": 435, - "volume": 435 - }, - "open": "2020-11-04T19:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3034, - "high": 649, - "low": 3034, - "open": 649, - "volume": 5971 - }, - "id": 2760460, - "non_hive": { - "close": 350, - "high": 75, - "low": 350, - "open": 75, - "volume": 689 - }, - "open": "2020-11-04T19:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 6346, - "high": 6346, - "low": 6346, - "open": 6346, - "volume": 6346 - }, - "id": 2760465, - "non_hive": { - "close": 732, - "high": 732, - "low": 732, - "open": 732, - "volume": 732 - }, - "open": "2020-11-04T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2948, - "high": 2948, - "low": 2948, - "open": 2948, - "volume": 2948 - }, - "id": 2760468, - "non_hive": { - "close": 339, - "high": 339, - "low": 339, - "open": 339, - "volume": 339 - }, - "open": "2020-11-04T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 220839, - "high": 220839, - "low": 14000, - "open": 14000, - "volume": 234839 - }, - "id": 2760471, - "non_hive": { - "close": 25580, - "high": 25580, - "low": 1617, - "open": 1617, - "volume": 27197 - }, - "open": "2020-11-04T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 100, - "high": 2003, - "low": 100, - "open": 2003, - "volume": 2103 - }, - "id": 2760474, - "non_hive": { - "close": 11, - "high": 232, - "low": 11, - "open": 232, - "volume": 243 - }, - "open": "2020-11-04T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 100, - "high": 5882, - "low": 100, - "open": 5882, - "volume": 5982 - }, - "id": 2760478, - "non_hive": { - "close": 11, - "high": 681, - "low": 11, - "open": 681, - "volume": 692 - }, - "open": "2020-11-04T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 426400, - "high": 426400, - "low": 4620, - "open": 4620, - "volume": 431020 - }, - "id": 2760483, - "non_hive": { - "close": 49365, - "high": 49365, - "low": 533, - "open": 533, - "volume": 49898 - }, - "open": "2020-11-04T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 4466, - "high": 4466, - "low": 57, - "open": 4382, - "volume": 85767 - }, - "id": 2760486, - "non_hive": { - "close": 517, - "high": 517, - "low": 6, - "open": 507, - "volume": 9927 - }, - "open": "2020-11-04T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 15593, - "high": 9528, - "low": 15593, - "open": 8768, - "volume": 33889 - }, - "id": 2760493, - "non_hive": { - "close": 1805, - "high": 1103, - "low": 1805, - "open": 1015, - "volume": 3923 - }, - "open": "2020-11-04T20:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1037, - "high": 137992, - "low": 1037, - "open": 137992, - "volume": 139029 - }, - "id": 2760500, - "non_hive": { - "close": 120, - "high": 15972, - "low": 120, - "open": 15972, - "volume": 16092 - }, - "open": "2020-11-04T20:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 4183, - "high": 4183, - "low": 4183, - "open": 4183, - "volume": 4183 - }, - "id": 2760505, - "non_hive": { - "close": 484, - "high": 484, - "low": 484, - "open": 484, - "volume": 484 - }, - "open": "2020-11-04T20:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 53, - "high": 5696, - "low": 53, - "open": 5696, - "volume": 5749 - }, - "id": 2760508, - "non_hive": { - "close": 6, - "high": 659, - "low": 6, - "open": 659, - "volume": 665 - }, - "open": "2020-11-04T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 29120, - "high": 29120, - "low": 29120, - "open": 29120, - "volume": 29120 - }, - "id": 2760511, - "non_hive": { - "close": 3369, - "high": 3369, - "low": 3369, - "open": 3369, - "volume": 3369 - }, - "open": "2020-11-04T20:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 3854, - "high": 3854, - "low": 3854, - "open": 3854, - "volume": 3854 - }, - "id": 2760514, - "non_hive": { - "close": 447, - "high": 447, - "low": 447, - "open": 447, - "volume": 447 - }, - "open": "2020-11-04T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 4251, - "high": 4251, - "low": 5262, - "open": 5262, - "volume": 9513 - }, - "id": 2760518, - "non_hive": { - "close": 493, - "high": 493, - "low": 610, - "open": 610, - "volume": 1103 - }, - "open": "2020-11-04T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 8862, - "high": 8862, - "low": 8862, - "open": 8862, - "volume": 8862 - }, - "id": 2760521, - "non_hive": { - "close": 1010, - "high": 1010, - "low": 1010, - "open": 1010, - "volume": 1010 - }, - "open": "2020-11-04T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 621, - "high": 621, - "low": 621, - "open": 621, - "volume": 621 - }, - "id": 2760524, - "non_hive": { - "close": 72, - "high": 72, - "low": 72, - "open": 72, - "volume": 72 - }, - "open": "2020-11-04T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 1501, - "high": 1501, - "low": 1501, - "open": 1501, - "volume": 1501 - }, - "id": 2760527, - "non_hive": { - "close": 174, - "high": 174, - "low": 174, - "open": 174, - "volume": 174 - }, - "open": "2020-11-04T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 587, - "high": 5229, - "low": 587, - "open": 5229, - "volume": 9398 - }, - "id": 2760530, - "non_hive": { - "close": 68, - "high": 606, - "low": 68, - "open": 606, - "volume": 1089 - }, - "open": "2020-11-04T21:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 11896, - "high": 104, - "low": 11896, - "open": 104, - "volume": 12000 - }, - "id": 2760535, - "non_hive": { - "close": 1356, - "high": 12, - "low": 1356, - "open": 12, - "volume": 1368 - }, - "open": "2020-11-04T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 2200, - "high": 2200, - "low": 13460, - "open": 13460, - "volume": 15660 - }, - "id": 2760538, - "non_hive": { - "close": 253, - "high": 253, - "low": 1547, - "open": 1547, - "volume": 1800 - }, - "open": "2020-11-04T22:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 12290, - "high": 12290, - "low": 12290, - "open": 12290, - "volume": 12290 - }, - "id": 2760544, - "non_hive": { - "close": 1425, - "high": 1425, - "low": 1425, - "open": 1425, - "volume": 1425 - }, - "open": "2020-11-04T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 36284, - "high": 36284, - "low": 5215, - "open": 5215, - "volume": 102994 - }, - "id": 2760547, - "non_hive": { - "close": 4208, - "high": 4208, - "low": 604, - "open": 604, - "volume": 11942 - }, - "open": "2020-11-04T22:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 17390, - "high": 25894, - "low": 8738, - "open": 25894, - "volume": 69412 - }, - "id": 2760550, - "non_hive": { - "close": 1999, - "high": 3000, - "low": 996, - "open": 3000, - "volume": 7995 - }, - "open": "2020-11-04T22:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 1179, - "high": 1179, - "low": 1179, - "open": 1179, - "volume": 1179 - }, - "id": 2760555, - "non_hive": { - "close": 136, - "high": 136, - "low": 136, - "open": 136, - "volume": 136 - }, - "open": "2020-11-04T22:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 432543, - "high": 432543, - "low": 9046, - "open": 9046, - "volume": 441589 - }, - "id": 2760558, - "non_hive": { - "close": 49950, - "high": 49950, - "low": 1043, - "open": 1043, - "volume": 50993 - }, - "open": "2020-11-04T22:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 948941, - "high": 948941, - "low": 17390, - "open": 17390, - "volume": 1442391 - }, - "id": 2760563, - "non_hive": { - "close": 109593, - "high": 109593, - "low": 2000, - "open": 2000, - "volume": 166496 - }, - "open": "2020-11-04T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 3905, - "high": 3905, - "low": 637356, - "open": 129266, - "volume": 770527 - }, - "id": 2760568, - "non_hive": { - "close": 453, - "high": 453, - "low": 73608, - "open": 14929, - "volume": 88990 - }, - "open": "2020-11-04T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 507782, - "high": 672, - "low": 507782, - "open": 14092, - "volume": 943145 - }, - "id": 2760573, - "non_hive": { - "close": 58395, - "high": 78, - "low": 58395, - "open": 1634, - "volume": 108493 - }, - "open": "2020-11-04T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 58446, - "high": 58446, - "low": 17391, - "open": 17391, - "volume": 158357 - }, - "id": 2760579, - "non_hive": { - "close": 6779, - "high": 6779, - "low": 1999, - "open": 1999, - "volume": 18277 - }, - "open": "2020-11-04T22:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 6547, - "high": 6547, - "low": 2562, - "open": 2562, - "volume": 9109 - }, - "id": 2760582, - "non_hive": { - "close": 759, - "high": 759, - "low": 297, - "open": 297, - "volume": 1056 - }, - "open": "2020-11-04T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 34141, - "high": 34141, - "low": 53481, - "open": 53481, - "volume": 128086 - }, - "id": 2760587, - "non_hive": { - "close": 3958, - "high": 3958, - "low": 6200, - "open": 6200, - "volume": 14849 - }, - "open": "2020-11-04T23:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 25998, - "high": 25998, - "low": 25998, - "open": 25998, - "volume": 25998 - }, - "id": 2760594, - "non_hive": { - "close": 3014, - "high": 3014, - "low": 3014, - "open": 3014, - "volume": 3014 - }, - "open": "2020-11-04T23:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 14552, - "high": 32571, - "low": 5232, - "open": 32571, - "volume": 52355 - }, - "id": 2760597, - "non_hive": { - "close": 1687, - "high": 3776, - "low": 606, - "open": 3776, - "volume": 6069 - }, - "open": "2020-11-04T23:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 517, - "high": 517, - "low": 20000, - "open": 20000, - "volume": 173042 - }, - "id": 2760604, - "non_hive": { - "close": 60, - "high": 60, - "low": 2318, - "open": 2318, - "volume": 20060 - }, - "open": "2020-11-04T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 3080, - "high": 3080, - "low": 10942, - "open": 10942, - "volume": 14022 - }, - "id": 2760608, - "non_hive": { - "close": 357, - "high": 357, - "low": 1250, - "open": 1250, - "volume": 1607 - }, - "open": "2020-11-04T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 27735, - "high": 27735, - "low": 7862, - "open": 7862, - "volume": 35597 - }, - "id": 2760613, - "non_hive": { - "close": 3215, - "high": 3215, - "low": 911, - "open": 911, - "volume": 4126 - }, - "open": "2020-11-04T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 20912, - "high": 20912, - "low": 20912, - "open": 20912, - "volume": 20912 - }, - "id": 2760616, - "non_hive": { - "close": 2424, - "high": 2424, - "low": 2424, - "open": 2424, - "volume": 2424 - }, - "open": "2020-11-04T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 10904, - "high": 10904, - "low": 10904, - "open": 10904, - "volume": 10904 - }, - "id": 2760619, - "non_hive": { - "close": 1264, - "high": 1264, - "low": 1264, - "open": 1264, - "volume": 1264 - }, - "open": "2020-11-05T00:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 145566, - "high": 59509, - "low": 433, - "open": 141408, - "volume": 501350 - }, - "id": 2760624, - "non_hive": { - "close": 16627, - "high": 6898, - "low": 49, - "open": 16391, - "volume": 57607 - }, - "open": "2020-11-05T00:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2074, - "high": 2074, - "low": 6569, - "open": 6569, - "volume": 8643 - }, - "id": 2760631, - "non_hive": { - "close": 240, - "high": 240, - "low": 759, - "open": 759, - "volume": 999 - }, - "open": "2020-11-05T00:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 36229, - "high": 26611, - "low": 36229, - "open": 26611, - "volume": 62840 - }, - "id": 2760634, - "non_hive": { - "close": 4200, - "high": 3085, - "low": 4200, - "open": 3085, - "volume": 7285 - }, - "open": "2020-11-05T00:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 73874, - "high": 73874, - "low": 73874, - "open": 73874, - "volume": 73874 - }, - "id": 2760639, - "non_hive": { - "close": 8564, - "high": 8564, - "low": 8564, - "open": 8564, - "volume": 8564 - }, - "open": "2020-11-05T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 7514, - "high": 7514, - "low": 19092, - "open": 78, - "volume": 58347 - }, - "id": 2760642, - "non_hive": { - "close": 870, - "high": 870, - "low": 2180, - "open": 9, - "volume": 6676 - }, - "open": "2020-11-05T00:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 310, - "high": 33451, - "low": 310, - "open": 9752, - "volume": 43513 - }, - "id": 2760646, - "non_hive": { - "close": 35, - "high": 3873, - "low": 35, - "open": 1129, - "volume": 5037 - }, - "open": "2020-11-05T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 266349, - "high": 266349, - "low": 8852, - "open": 8852, - "volume": 275201 - }, - "id": 2760650, - "non_hive": { - "close": 30874, - "high": 30874, - "low": 1026, - "open": 1026, - "volume": 31900 - }, - "open": "2020-11-05T00:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 19714, - "high": 19714, - "low": 5610, - "open": 5610, - "volume": 25324 - }, - "id": 2760653, - "non_hive": { - "close": 2285, - "high": 2285, - "low": 647, - "open": 647, - "volume": 2932 - }, - "open": "2020-11-05T01:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 1877, - "high": 1877, - "low": 1877, - "open": 1877, - "volume": 1877 - }, - "id": 2760657, - "non_hive": { - "close": 217, - "high": 217, - "low": 217, - "open": 217, - "volume": 217 - }, - "open": "2020-11-05T01:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3002, - "high": 3002, - "low": 3002, - "open": 3002, - "volume": 3002 - }, - "id": 2760660, - "non_hive": { - "close": 348, - "high": 348, - "low": 348, - "open": 348, - "volume": 348 - }, - "open": "2020-11-05T01:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 13080, - "high": 13080, - "low": 13080, - "open": 13080, - "volume": 13080 - }, - "id": 2760663, - "non_hive": { - "close": 1516, - "high": 1516, - "low": 1516, - "open": 1516, - "volume": 1516 - }, - "open": "2020-11-05T01:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 5626, - "high": 5626, - "low": 5626, - "open": 5626, - "volume": 5626 - }, - "id": 2760666, - "non_hive": { - "close": 652, - "high": 652, - "low": 652, - "open": 652, - "volume": 652 - }, - "open": "2020-11-05T02:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 164164, - "high": 164164, - "low": 164164, - "open": 164164, - "volume": 164164 - }, - "id": 2760670, - "non_hive": { - "close": 19027, - "high": 19027, - "low": 19027, - "open": 19027, - "volume": 19027 - }, - "open": "2020-11-05T02:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3295, - "high": 3295, - "low": 3295, - "open": 3295, - "volume": 3295 - }, - "id": 2760673, - "non_hive": { - "close": 382, - "high": 382, - "low": 382, - "open": 382, - "volume": 382 - }, - "open": "2020-11-05T02:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1231, - "high": 1231, - "low": 1231, - "open": 1231, - "volume": 1231 - }, - "id": 2760676, - "non_hive": { - "close": 142, - "high": 142, - "low": 142, - "open": 142, - "volume": 142 - }, - "open": "2020-11-05T02:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1682, - "high": 1682, - "low": 1682, - "open": 1682, - "volume": 1682 - }, - "id": 2760679, - "non_hive": { - "close": 195, - "high": 195, - "low": 195, - "open": 195, - "volume": 195 - }, - "open": "2020-11-05T02:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 5220, - "high": 5220, - "low": 5220, - "open": 5220, - "volume": 5220 - }, - "id": 2760682, - "non_hive": { - "close": 604, - "high": 604, - "low": 604, - "open": 604, - "volume": 604 - }, - "open": "2020-11-05T02:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 18722, - "high": 18722, - "low": 18722, - "open": 18722, - "volume": 18722 - }, - "id": 2760685, - "non_hive": { - "close": 2170, - "high": 2170, - "low": 2170, - "open": 2170, - "volume": 2170 - }, - "open": "2020-11-05T03:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 2769, - "high": 2769, - "low": 2769, - "open": 2769, - "volume": 2769 - }, - "id": 2760689, - "non_hive": { - "close": 321, - "high": 321, - "low": 321, - "open": 321, - "volume": 321 - }, - "open": "2020-11-05T03:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2760692, - "non_hive": { - "close": 115, - "high": 115, - "low": 115, - "open": 115, - "volume": 115 - }, - "open": "2020-11-05T03:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 56970, - "high": 56970, - "low": 56970, - "open": 56970, - "volume": 56970 - }, - "id": 2760695, - "non_hive": { - "close": 6603, - "high": 6603, - "low": 6603, - "open": 6603, - "volume": 6603 - }, - "open": "2020-11-05T03:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 551, - "high": 4616, - "low": 551, - "open": 673, - "volume": 5840 - }, - "id": 2760698, - "non_hive": { - "close": 63, - "high": 535, - "low": 63, - "open": 78, - "volume": 676 - }, - "open": "2020-11-05T03:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 114546, - "high": 2727, - "low": 114546, - "open": 2727, - "volume": 117273 - }, - "id": 2760701, - "non_hive": { - "close": 13272, - "high": 316, - "low": 13272, - "open": 316, - "volume": 13588 - }, - "open": "2020-11-05T03:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1018, - "high": 1018, - "low": 1018, - "open": 1018, - "volume": 1018 - }, - "id": 2760706, - "non_hive": { - "close": 118, - "high": 118, - "low": 118, - "open": 118, - "volume": 118 - }, - "open": "2020-11-05T04:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 52, - "high": 54112, - "low": 52, - "open": 54112, - "volume": 54164 - }, - "id": 2760710, - "non_hive": { - "close": 6, - "high": 6244, - "low": 6, - "open": 6244, - "volume": 6250 - }, - "open": "2020-11-05T04:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 253525, - "high": 253525, - "low": 65386, - "open": 65386, - "volume": 1192149 - }, - "id": 2760713, - "non_hive": { - "close": 29375, - "high": 29375, - "low": 7545, - "open": 7545, - "volume": 138087 - }, - "open": "2020-11-05T04:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 260, - "high": 27170, - "low": 260, - "open": 27170, - "volume": 27586 - }, - "id": 2760716, - "non_hive": { - "close": 29, - "high": 3148, - "low": 29, - "open": 3148, - "volume": 3195 - }, - "open": "2020-11-05T04:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 14000, - "high": 14000, - "low": 14000, - "open": 14000, - "volume": 14000 - }, - "id": 2760719, - "non_hive": { - "close": 1597, - "high": 1597, - "low": 1597, - "open": 1597, - "volume": 1597 - }, - "open": "2020-11-05T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 8, - "high": 8, - "low": 7267, - "open": 3532, - "volume": 1607851 - }, - "id": 2760722, - "non_hive": { - "close": 1, - "high": 1, - "low": 829, - "open": 403, - "volume": 186264 - }, - "open": "2020-11-05T04:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 12261, - "high": 12261, - "low": 17580, - "open": 17580, - "volume": 134374 - }, - "id": 2760730, - "non_hive": { - "close": 1413, - "high": 1413, - "low": 2000, - "open": 2000, - "volume": 15413 - }, - "open": "2020-11-05T04:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 59, - "high": 59, - "low": 59, - "open": 59, - "volume": 59 - }, - "id": 2760744, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-05T04:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 6315, - "high": 6315, - "low": 17256, - "open": 17256, - "volume": 34390 - }, - "id": 2760747, - "non_hive": { - "close": 732, - "high": 732, - "low": 1999, - "open": 1999, - "volume": 3985 - }, - "open": "2020-11-05T04:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 5533, - "high": 5533, - "low": 5533, - "open": 5533, - "volume": 5533 - }, - "id": 2760754, - "non_hive": { - "close": 640, - "high": 640, - "low": 640, - "open": 640, - "volume": 640 - }, - "open": "2020-11-05T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 12407, - "high": 12407, - "low": 12407, - "open": 12407, - "volume": 12407 - }, - "id": 2760758, - "non_hive": { - "close": 1438, - "high": 1438, - "low": 1438, - "open": 1438, - "volume": 1438 - }, - "open": "2020-11-05T05:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 7911, - "high": 7911, - "low": 7911, - "open": 7911, - "volume": 7911 - }, - "id": 2760761, - "non_hive": { - "close": 917, - "high": 917, - "low": 917, - "open": 917, - "volume": 917 - }, - "open": "2020-11-05T05:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 11181, - "high": 11181, - "low": 11181, - "open": 11181, - "volume": 11181 - }, - "id": 2760764, - "non_hive": { - "close": 1296, - "high": 1296, - "low": 1296, - "open": 1296, - "volume": 1296 - }, - "open": "2020-11-05T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6445, - "high": 6445, - "low": 6445, - "open": 6445, - "volume": 6445 - }, - "id": 2760767, - "non_hive": { - "close": 746, - "high": 746, - "low": 746, - "open": 746, - "volume": 746 - }, - "open": "2020-11-05T05:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 10327, - "high": 10327, - "low": 10327, - "open": 10327, - "volume": 10327 - }, - "id": 2760770, - "non_hive": { - "close": 1197, - "high": 1197, - "low": 1197, - "open": 1197, - "volume": 1197 - }, - "open": "2020-11-05T06:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 51, - "high": 51, - "low": 5851, - "open": 5851, - "volume": 351269 - }, - "id": 2760774, - "non_hive": { - "close": 6, - "high": 6, - "low": 678, - "open": 678, - "volume": 40712 - }, - "open": "2020-11-05T06:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 9903, - "high": 154993, - "low": 9903, - "open": 154993, - "volume": 164896 - }, - "id": 2760781, - "non_hive": { - "close": 1148, - "high": 17968, - "low": 1148, - "open": 17968, - "volume": 19116 - }, - "open": "2020-11-05T06:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 13544, - "high": 13544, - "low": 13544, - "open": 13544, - "volume": 13544 - }, - "id": 2760786, - "non_hive": { - "close": 1570, - "high": 1570, - "low": 1570, - "open": 1570, - "volume": 1570 - }, - "open": "2020-11-05T06:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 3332, - "high": 6635, - "low": 28341, - "open": 6635, - "volume": 61546 - }, - "id": 2760789, - "non_hive": { - "close": 386, - "high": 769, - "low": 3283, - "open": 769, - "volume": 7130 - }, - "open": "2020-11-05T06:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 6076, - "high": 1467, - "low": 615, - "open": 1467, - "volume": 14226 - }, - "id": 2760794, - "non_hive": { - "close": 704, - "high": 170, - "low": 71, - "open": 170, - "volume": 1648 - }, - "open": "2020-11-05T06:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 6064, - "high": 4330, - "low": 1753, - "open": 6076, - "volume": 32232 - }, - "id": 2760800, - "non_hive": { - "close": 703, - "high": 502, - "low": 203, - "open": 704, - "volume": 3736 - }, - "open": "2020-11-05T06:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 3451, - "high": 65519, - "low": 3451, - "open": 65519, - "volume": 90684 - }, - "id": 2760810, - "non_hive": { - "close": 400, - "high": 7595, - "low": 400, - "open": 7595, - "volume": 10512 - }, - "open": "2020-11-05T06:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6050, - "high": 6050, - "low": 6050, - "open": 6050, - "volume": 6050 - }, - "id": 2760816, - "non_hive": { - "close": 701, - "high": 701, - "low": 701, - "open": 701, - "volume": 701 - }, - "open": "2020-11-05T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13106, - "high": 17253, - "low": 6040, - "open": 17253, - "volume": 36399 - }, - "id": 2760819, - "non_hive": { - "close": 1518, - "high": 1999, - "low": 699, - "open": 1999, - "volume": 4216 - }, - "open": "2020-11-05T07:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 79325, - "high": 2286, - "low": 79325, - "open": 2286, - "volume": 81611 - }, - "id": 2760827, - "non_hive": { - "close": 9191, - "high": 265, - "low": 9191, - "open": 265, - "volume": 9456 - }, - "open": "2020-11-05T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 17321, - "high": 17321, - "low": 341690, - "open": 341690, - "volume": 359011 - }, - "id": 2760832, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 39450, - "open": 39450, - "volume": 41450 - }, - "open": "2020-11-05T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3949, - "high": 4979, - "low": 3949, - "open": 17321, - "volume": 789407 - }, - "id": 2760837, - "non_hive": { - "close": 455, - "high": 577, - "low": 455, - "open": 2000, - "volume": 91143 - }, - "open": "2020-11-05T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 146750, - "high": 51889, - "low": 146750, - "open": 17256, - "volume": 225895 - }, - "id": 2760847, - "non_hive": { - "close": 17000, - "high": 6015, - "low": 17000, - "open": 1999, - "volume": 26173 - }, - "open": "2020-11-05T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 9453, - "high": 17271, - "low": 9453, - "open": 17271, - "volume": 113079 - }, - "id": 2760852, - "non_hive": { - "close": 1094, - "high": 1999, - "low": 1094, - "open": 1999, - "volume": 13088 - }, - "open": "2020-11-05T07:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 6185, - "high": 6185, - "low": 6185, - "open": 6185, - "volume": 6185 - }, - "id": 2760864, - "non_hive": { - "close": 713, - "high": 713, - "low": 713, - "open": 713, - "volume": 713 - }, - "open": "2020-11-05T07:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 17343, - "high": 11160, - "low": 17343, - "open": 11160, - "volume": 32362 - }, - "id": 2760867, - "non_hive": { - "close": 1999, - "high": 1287, - "low": 1999, - "open": 1287, - "volume": 3731 - }, - "open": "2020-11-05T07:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 239320, - "high": 239320, - "low": 88840, - "open": 88840, - "volume": 328160 - }, - "id": 2760874, - "non_hive": { - "close": 27742, - "high": 27742, - "low": 10245, - "open": 10245, - "volume": 37987 - }, - "open": "2020-11-05T08:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 17003, - "high": 17003, - "low": 17003, - "open": 17003, - "volume": 17003 - }, - "id": 2760878, - "non_hive": { - "close": 1971, - "high": 1971, - "low": 1971, - "open": 1971, - "volume": 1971 - }, - "open": "2020-11-05T08:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 3879, - "high": 3879, - "low": 3879, - "open": 3879, - "volume": 3879 - }, - "id": 2760881, - "non_hive": { - "close": 448, - "high": 448, - "low": 448, - "open": 448, - "volume": 448 - }, - "open": "2020-11-05T08:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 14656, - "high": 14656, - "low": 14656, - "open": 14656, - "volume": 14656 - }, - "id": 2760884, - "non_hive": { - "close": 1699, - "high": 1699, - "low": 1699, - "open": 1699, - "volume": 1699 - }, - "open": "2020-11-05T08:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 13276, - "high": 13276, - "low": 13276, - "open": 13276, - "volume": 13276 - }, - "id": 2760887, - "non_hive": { - "close": 1539, - "high": 1539, - "low": 1539, - "open": 1539, - "volume": 1539 - }, - "open": "2020-11-05T09:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 8658, - "high": 17313, - "low": 8663, - "open": 17313, - "volume": 69260 - }, - "id": 2760891, - "non_hive": { - "close": 1000, - "high": 2000, - "low": 1000, - "open": 2000, - "volume": 8000 - }, - "open": "2020-11-05T09:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 821430, - "high": 821430, - "low": 17253, - "open": 17253, - "volume": 4017999 - }, - "id": 2760899, - "non_hive": { - "close": 96518, - "high": 96518, - "low": 1999, - "open": 1999, - "volume": 470040 - }, - "open": "2020-11-05T09:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 72362, - "high": 72362, - "low": 17024, - "open": 17024, - "volume": 89386 - }, - "id": 2760902, - "non_hive": { - "close": 8497, - "high": 8497, - "low": 1999, - "open": 1999, - "volume": 10496 - }, - "open": "2020-11-05T09:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 17180, - "high": 255, - "low": 17180, - "open": 255, - "volume": 17435 - }, - "id": 2760907, - "non_hive": { - "close": 2000, - "high": 30, - "low": 2000, - "open": 30, - "volume": 2030 - }, - "open": "2020-11-05T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 77, - "high": 77, - "low": 6048, - "open": 6048, - "volume": 6125 - }, - "id": 2760912, - "non_hive": { - "close": 9, - "high": 9, - "low": 704, - "open": 704, - "volume": 713 - }, - "open": "2020-11-05T09:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 17245, - "high": 17245, - "low": 7808, - "open": 7808, - "volume": 25053 - }, - "id": 2760917, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 904, - "open": 904, - "volume": 2904 - }, - "open": "2020-11-05T09:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 17182, - "high": 17182, - "low": 134139, - "open": 17240, - "volume": 2513514 - }, - "id": 2760922, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 15560, - "open": 2000, - "volume": 291574 - }, - "open": "2020-11-05T09:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1486, - "high": 1486, - "low": 567060, - "open": 567060, - "volume": 568546 - }, - "id": 2760936, - "non_hive": { - "close": 173, - "high": 173, - "low": 66007, - "open": 66007, - "volume": 66180 - }, - "open": "2020-11-05T09:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 44935, - "high": 44935, - "low": 17527, - "open": 95273, - "volume": 157735 - }, - "id": 2760941, - "non_hive": { - "close": 5231, - "high": 5231, - "low": 2040, - "open": 11090, - "volume": 18361 - }, - "open": "2020-11-05T09:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 250286, - "high": 8908, - "low": 10604, - "open": 8908, - "volume": 1402468 - }, - "id": 2760945, - "non_hive": { - "close": 29069, - "high": 1046, - "low": 1231, - "open": 1046, - "volume": 162903 - }, - "open": "2020-11-05T10:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 8, - "high": 8, - "low": 619, - "open": 619, - "volume": 627 - }, - "id": 2760955, - "non_hive": { - "close": 1, - "high": 1, - "low": 71, - "open": 71, - "volume": 72 - }, - "open": "2020-11-05T10:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 374, - "high": 374, - "low": 3698, - "open": 3698, - "volume": 4072 - }, - "id": 2760958, - "non_hive": { - "close": 44, - "high": 44, - "low": 434, - "open": 434, - "volume": 478 - }, - "open": "2020-11-05T10:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 7746, - "high": 4121, - "low": 7746, - "open": 4121, - "volume": 11867 - }, - "id": 2760963, - "non_hive": { - "close": 900, - "high": 479, - "low": 900, - "open": 479, - "volume": 1379 - }, - "open": "2020-11-05T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 71856, - "high": 71856, - "low": 71856, - "open": 71856, - "volume": 71856 - }, - "id": 2760966, - "non_hive": { - "close": 8433, - "high": 8433, - "low": 8433, - "open": 8433, - "volume": 8433 - }, - "open": "2020-11-05T10:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 7769, - "high": 7769, - "low": 7769, - "open": 7769, - "volume": 7769 - }, - "id": 2760969, - "non_hive": { - "close": 901, - "high": 901, - "low": 901, - "open": 901, - "volume": 901 - }, - "open": "2020-11-05T11:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 4878, - "high": 2525, - "low": 4878, - "open": 2525, - "volume": 87048 - }, - "id": 2760973, - "non_hive": { - "close": 563, - "high": 296, - "low": 563, - "open": 296, - "volume": 10060 - }, - "open": "2020-11-05T11:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 17, - "high": 17, - "low": 4957, - "open": 8589, - "volume": 38086 - }, - "id": 2760980, - "non_hive": { - "close": 2, - "high": 2, - "low": 567, - "open": 1000, - "volume": 4414 - }, - "open": "2020-11-05T11:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 17339, - "high": 17339, - "low": 17339, - "open": 17339, - "volume": 17339 - }, - "id": 2760989, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T11:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 60, - "high": 60, - "low": 60, - "open": 60, - "volume": 60 - }, - "id": 2760992, - "non_hive": { - "close": 7, - "high": 7, - "low": 7, - "open": 7, - "volume": 7 - }, - "open": "2020-11-05T12:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 667, - "high": 667, - "low": 23625, - "open": 23625, - "volume": 24292 - }, - "id": 2760996, - "non_hive": { - "close": 77, - "high": 77, - "low": 2725, - "open": 2725, - "volume": 2802 - }, - "open": "2020-11-05T12:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 52, - "high": 69, - "low": 52, - "open": 69, - "volume": 121 - }, - "id": 2761001, - "non_hive": { - "close": 6, - "high": 8, - "low": 6, - "open": 8, - "volume": 14 - }, - "open": "2020-11-05T12:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 9087, - "high": 3286, - "low": 9087, - "open": 3286, - "volume": 12373 - }, - "id": 2761006, - "non_hive": { - "close": 1048, - "high": 379, - "low": 1048, - "open": 379, - "volume": 1427 - }, - "open": "2020-11-05T12:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4804, - "high": 4804, - "low": 4804, - "open": 4804, - "volume": 4804 - }, - "id": 2761009, - "non_hive": { - "close": 554, - "high": 554, - "low": 554, - "open": 554, - "volume": 554 - }, - "open": "2020-11-05T12:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 312, - "high": 312, - "low": 312, - "open": 312, - "volume": 312 - }, - "id": 2761012, - "non_hive": { - "close": 36, - "high": 36, - "low": 36, - "open": 36, - "volume": 36 - }, - "open": "2020-11-05T12:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 75290, - "high": 75290, - "low": 6023, - "open": 17798, - "volume": 99111 - }, - "id": 2761015, - "non_hive": { - "close": 8684, - "high": 8684, - "low": 694, - "open": 2052, - "volume": 11430 - }, - "open": "2020-11-05T13:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 6085, - "high": 6085, - "low": 297, - "open": 297, - "volume": 12460 - }, - "id": 2761019, - "non_hive": { - "close": 705, - "high": 705, - "low": 34, - "open": 34, - "volume": 1440 - }, - "open": "2020-11-05T13:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 16801, - "high": 16801, - "low": 16801, - "open": 16801, - "volume": 16801 - }, - "id": 2761022, - "non_hive": { - "close": 1956, - "high": 1956, - "low": 1956, - "open": 1956, - "volume": 1956 - }, - "open": "2020-11-05T13:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 50671, - "high": 240, - "low": 370, - "open": 370, - "volume": 51281 - }, - "id": 2761025, - "non_hive": { - "close": 5902, - "high": 28, - "low": 43, - "open": 43, - "volume": 5973 - }, - "open": "2020-11-05T13:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 487948, - "high": 13263, - "low": 487948, - "open": 13263, - "volume": 501211 - }, - "id": 2761030, - "non_hive": { - "close": 56835, - "high": 1545, - "low": 56835, - "open": 1545, - "volume": 58380 - }, - "open": "2020-11-05T13:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 8151, - "high": 738, - "low": 11000, - "open": 50989, - "volume": 475838 - }, - "id": 2761033, - "non_hive": { - "close": 949, - "high": 86, - "low": 1280, - "open": 5940, - "volume": 55424 - }, - "open": "2020-11-05T13:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 86, - "high": 231, - "low": 86, - "open": 8282, - "volume": 62027 - }, - "id": 2761041, - "non_hive": { - "close": 10, - "high": 27, - "low": 10, - "open": 964, - "volume": 7214 - }, - "open": "2020-11-05T14:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 15120, - "high": 249, - "low": 15120, - "open": 249, - "volume": 15369 - }, - "id": 2761053, - "non_hive": { - "close": 1760, - "high": 29, - "low": 1760, - "open": 29, - "volume": 1789 - }, - "open": "2020-11-05T14:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 309759, - "high": 6010, - "low": 309759, - "open": 6010, - "volume": 333010 - }, - "id": 2761058, - "non_hive": { - "close": 35932, - "high": 700, - "low": 35932, - "open": 700, - "volume": 38632 - }, - "open": "2020-11-05T14:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 17239, - "high": 4295, - "low": 17239, - "open": 4295, - "volume": 21534 - }, - "id": 2761063, - "non_hive": { - "close": 2000, - "high": 500, - "low": 2000, - "open": 500, - "volume": 2500 - }, - "open": "2020-11-05T14:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 9741, - "high": 11828, - "low": 17242, - "open": 11828, - "volume": 254325 - }, - "id": 2761068, - "non_hive": { - "close": 1130, - "high": 1377, - "low": 2000, - "open": 1377, - "volume": 29507 - }, - "open": "2020-11-05T14:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 17232, - "high": 17180, - "low": 233801, - "open": 17180, - "volume": 622435 - }, - "id": 2761083, - "non_hive": { - "close": 1999, - "high": 2000, - "low": 27121, - "open": 2000, - "volume": 72350 - }, - "open": "2020-11-05T14:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 7250, - "high": 7250, - "low": 1984, - "open": 17171, - "volume": 173635 - }, - "id": 2761094, - "non_hive": { - "close": 854, - "high": 854, - "low": 230, - "open": 1999, - "volume": 20325 - }, - "open": "2020-11-05T14:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 44999, - "high": 8328, - "low": 17241, - "open": 17241, - "volume": 80290 - }, - "id": 2761102, - "non_hive": { - "close": 5300, - "high": 981, - "low": 2000, - "open": 2000, - "volume": 9426 - }, - "open": "2020-11-05T14:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 17241, - "high": 229759, - "low": 14949, - "open": 6000, - "volume": 446213 - }, - "id": 2761108, - "non_hive": { - "close": 2000, - "high": 27061, - "low": 1734, - "open": 698, - "volume": 52456 - }, - "open": "2020-11-05T14:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1681, - "high": 1681, - "low": 13000, - "open": 13276, - "volume": 37745 - }, - "id": 2761118, - "non_hive": { - "close": 198, - "high": 198, - "low": 1508, - "open": 1558, - "volume": 4413 - }, - "open": "2020-11-05T14:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 43623, - "high": 43623, - "low": 4480, - "open": 6000, - "volume": 124195 - }, - "id": 2761125, - "non_hive": { - "close": 5138, - "high": 5138, - "low": 521, - "open": 698, - "volume": 14582 - }, - "open": "2020-11-05T14:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 178017, - "high": 50, - "low": 17191, - "open": 51, - "volume": 268252 - }, - "id": 2761133, - "non_hive": { - "close": 20964, - "high": 6, - "low": 1994, - "open": 6, - "volume": 31551 - }, - "open": "2020-11-05T15:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 5884, - "high": 51, - "low": 2588, - "open": 51, - "volume": 45414 - }, - "id": 2761145, - "non_hive": { - "close": 685, - "high": 6, - "low": 300, - "open": 6, - "volume": 5287 - }, - "open": "2020-11-05T15:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 10481, - "high": 10481, - "low": 163, - "open": 163, - "volume": 106768 - }, - "id": 2761152, - "non_hive": { - "close": 1230, - "high": 1230, - "low": 19, - "open": 19, - "volume": 12529 - }, - "open": "2020-11-05T15:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 42, - "high": 42, - "low": 10000, - "open": 10000, - "volume": 12042 - }, - "id": 2761157, - "non_hive": { - "close": 5, - "high": 5, - "low": 1160, - "open": 1160, - "volume": 1399 - }, - "open": "2020-11-05T15:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 758127, - "high": 758127, - "low": 6511, - "open": 6511, - "volume": 1000157 - }, - "id": 2761162, - "non_hive": { - "close": 89735, - "high": 89735, - "low": 764, - "open": 764, - "volume": 118364 - }, - "open": "2020-11-05T15:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 5740, - "high": 4075, - "low": 2925, - "open": 4075, - "volume": 17000 - }, - "id": 2761165, - "non_hive": { - "close": 670, - "high": 482, - "low": 341, - "open": 482, - "volume": 1991 - }, - "open": "2020-11-05T15:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 5718, - "high": 282, - "low": 5718, - "open": 282, - "volume": 6000 - }, - "id": 2761169, - "non_hive": { - "close": 665, - "high": 33, - "low": 665, - "open": 33, - "volume": 698 - }, - "open": "2020-11-05T15:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 2599, - "high": 562, - "low": 2599, - "open": 562, - "volume": 4062 - }, - "id": 2761172, - "non_hive": { - "close": 301, - "high": 66, - "low": 301, - "open": 66, - "volume": 472 - }, - "open": "2020-11-05T15:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 9190, - "high": 810, - "low": 9190, - "open": 810, - "volume": 10000 - }, - "id": 2761177, - "non_hive": { - "close": 1065, - "high": 94, - "low": 1065, - "open": 94, - "volume": 1159 - }, - "open": "2020-11-05T15:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 16472, - "high": 16472, - "low": 16472, - "open": 16472, - "volume": 16472 - }, - "id": 2761180, - "non_hive": { - "close": 1933, - "high": 1933, - "low": 1933, - "open": 1933, - "volume": 1933 - }, - "open": "2020-11-05T15:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 5674, - "high": 5674, - "low": 5674, - "open": 5674, - "volume": 5674 - }, - "id": 2761183, - "non_hive": { - "close": 666, - "high": 666, - "low": 666, - "open": 666, - "volume": 666 - }, - "open": "2020-11-05T15:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 4295, - "high": 1704, - "low": 4295, - "open": 1704, - "volume": 5999 - }, - "id": 2761186, - "non_hive": { - "close": 504, - "high": 200, - "low": 504, - "open": 200, - "volume": 704 - }, - "open": "2020-11-05T15:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 14631, - "high": 8505, - "low": 17045, - "open": 17045, - "volume": 40181 - }, - "id": 2761191, - "non_hive": { - "close": 1716, - "high": 998, - "low": 1999, - "open": 1999, - "volume": 4713 - }, - "open": "2020-11-05T16:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 503950, - "high": 366, - "low": 250, - "open": 2414, - "volume": 538052 - }, - "id": 2761197, - "non_hive": { - "close": 59102, - "high": 43, - "low": 29, - "open": 283, - "volume": 63103 - }, - "open": "2020-11-05T16:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 18203, - "high": 707, - "low": 18203, - "open": 24356, - "volume": 380391 - }, - "id": 2761204, - "non_hive": { - "close": 2129, - "high": 83, - "low": 2129, - "open": 2858, - "volume": 44602 - }, - "open": "2020-11-05T16:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 1116, - "high": 1116, - "low": 20, - "open": 50000, - "volume": 51136 - }, - "id": 2761209, - "non_hive": { - "close": 131, - "high": 131, - "low": 2, - "open": 5850, - "volume": 5983 - }, - "open": "2020-11-05T16:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 22047, - "high": 9996, - "low": 22047, - "open": 9996, - "volume": 32043 - }, - "id": 2761215, - "non_hive": { - "close": 2587, - "high": 1173, - "low": 2587, - "open": 1173, - "volume": 3760 - }, - "open": "2020-11-05T16:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2242, - "high": 104838, - "low": 2242, - "open": 16841, - "volume": 583305 - }, - "id": 2761220, - "non_hive": { - "close": 262, - "high": 12359, - "low": 262, - "open": 1976, - "volume": 68353 - }, - "open": "2020-11-05T16:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 69657, - "high": 69657, - "low": 14867, - "open": 14867, - "volume": 84524 - }, - "id": 2761226, - "non_hive": { - "close": 8143, - "high": 8143, - "low": 1737, - "open": 1737, - "volume": 9880 - }, - "open": "2020-11-05T16:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 141440, - "high": 15920, - "low": 17118, - "open": 15920, - "volume": 174478 - }, - "id": 2761229, - "non_hive": { - "close": 16525, - "high": 1860, - "low": 1999, - "open": 1860, - "volume": 20384 - }, - "open": "2020-11-05T17:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 57135, - "high": 57135, - "low": 57135, - "open": 57135, - "volume": 57135 - }, - "id": 2761235, - "non_hive": { - "close": 6672, - "high": 6672, - "low": 6672, - "open": 6672, - "volume": 6672 - }, - "open": "2020-11-05T17:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 3510, - "high": 3510, - "low": 14922, - "open": 14922, - "volume": 18432 - }, - "id": 2761238, - "non_hive": { - "close": 414, - "high": 414, - "low": 1760, - "open": 1760, - "volume": 2174 - }, - "open": "2020-11-05T17:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1288, - "high": 1288, - "low": 13440, - "open": 13440, - "volume": 14728 - }, - "id": 2761243, - "non_hive": { - "close": 152, - "high": 152, - "low": 1585, - "open": 1585, - "volume": 1737 - }, - "open": "2020-11-05T17:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 19603, - "high": 19603, - "low": 19603, - "open": 19603, - "volume": 19603 - }, - "id": 2761248, - "non_hive": { - "close": 2312, - "high": 2312, - "low": 2312, - "open": 2312, - "volume": 2312 - }, - "open": "2020-11-05T17:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 40463, - "high": 40463, - "low": 40463, - "open": 40463, - "volume": 40463 - }, - "id": 2761251, - "non_hive": { - "close": 4768, - "high": 4768, - "low": 4768, - "open": 4768, - "volume": 4768 - }, - "open": "2020-11-05T17:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 12897, - "high": 12897, - "low": 12897, - "open": 12897, - "volume": 12897 - }, - "id": 2761254, - "non_hive": { - "close": 1519, - "high": 1519, - "low": 1519, - "open": 1519, - "volume": 1519 - }, - "open": "2020-11-05T17:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 14087, - "high": 14087, - "low": 4076, - "open": 4076, - "volume": 18163 - }, - "id": 2761257, - "non_hive": { - "close": 1660, - "high": 1660, - "low": 480, - "open": 480, - "volume": 2140 - }, - "open": "2020-11-05T17:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 712, - "high": 712, - "low": 712, - "open": 712, - "volume": 712 - }, - "id": 2761262, - "non_hive": { - "close": 84, - "high": 84, - "low": 84, - "open": 84, - "volume": 84 - }, - "open": "2020-11-05T17:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 37628, - "high": 1807, - "low": 37628, - "open": 1807, - "volume": 39435 - }, - "id": 2761265, - "non_hive": { - "close": 4434, - "high": 213, - "low": 4434, - "open": 213, - "volume": 4647 - }, - "open": "2020-11-05T18:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 10234, - "high": 10234, - "low": 10234, - "open": 10234, - "volume": 10234 - }, - "id": 2761271, - "non_hive": { - "close": 1206, - "high": 1206, - "low": 1206, - "open": 1206, - "volume": 1206 - }, - "open": "2020-11-05T18:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 3988, - "high": 3988, - "low": 3988, - "open": 3988, - "volume": 3988 - }, - "id": 2761274, - "non_hive": { - "close": 470, - "high": 470, - "low": 470, - "open": 470, - "volume": 470 - }, - "open": "2020-11-05T18:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2172, - "high": 2172, - "low": 2172, - "open": 2172, - "volume": 2172 - }, - "id": 2761277, - "non_hive": { - "close": 256, - "high": 256, - "low": 256, - "open": 256, - "volume": 256 - }, - "open": "2020-11-05T18:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 128, - "high": 1349, - "low": 128, - "open": 5058, - "volume": 22320 - }, - "id": 2761280, - "non_hive": { - "close": 15, - "high": 159, - "low": 15, - "open": 596, - "volume": 2629 - }, - "open": "2020-11-05T18:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 16066, - "high": 16066, - "low": 16066, - "open": 16066, - "volume": 16066 - }, - "id": 2761283, - "non_hive": { - "close": 1874, - "high": 1874, - "low": 1874, - "open": 1874, - "volume": 1874 - }, - "open": "2020-11-05T18:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 4758, - "high": 4758, - "low": 4758, - "open": 4758, - "volume": 4758 - }, - "id": 2761286, - "non_hive": { - "close": 555, - "high": 555, - "low": 555, - "open": 555, - "volume": 555 - }, - "open": "2020-11-05T18:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 33630, - "high": 9935, - "low": 74, - "open": 74, - "volume": 43639 - }, - "id": 2761289, - "non_hive": { - "close": 3923, - "high": 1159, - "low": 8, - "open": 8, - "volume": 5090 - }, - "open": "2020-11-05T18:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 8853, - "high": 8130, - "low": 8853, - "open": 8130, - "volume": 16983 - }, - "id": 2761294, - "non_hive": { - "close": 1042, - "high": 957, - "low": 1042, - "open": 957, - "volume": 1999 - }, - "open": "2020-11-05T19:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2985, - "high": 2985, - "low": 4043, - "open": 4043, - "volume": 41574 - }, - "id": 2761300, - "non_hive": { - "close": 352, - "high": 352, - "low": 476, - "open": 476, - "volume": 4901 - }, - "open": "2020-11-05T19:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 5827, - "high": 2298, - "low": 10419, - "open": 10419, - "volume": 33913 - }, - "id": 2761305, - "non_hive": { - "close": 687, - "high": 271, - "low": 1227, - "open": 1227, - "volume": 3997 - }, - "open": "2020-11-05T19:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1696, - "high": 1696, - "low": 1696, - "open": 1696, - "volume": 1696 - }, - "id": 2761312, - "non_hive": { - "close": 200, - "high": 200, - "low": 200, - "open": 200, - "volume": 200 - }, - "open": "2020-11-05T19:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 30678, - "high": 30678, - "low": 30678, - "open": 30678, - "volume": 30678 - }, - "id": 2761315, - "non_hive": { - "close": 3617, - "high": 3617, - "low": 3617, - "open": 3617, - "volume": 3617 - }, - "open": "2020-11-05T19:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 3104, - "high": 1696, - "low": 3104, - "open": 1696, - "volume": 4800 - }, - "id": 2761318, - "non_hive": { - "close": 366, - "high": 200, - "low": 366, - "open": 200, - "volume": 566 - }, - "open": "2020-11-05T19:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 6463, - "high": 6463, - "low": 6463, - "open": 6463, - "volume": 6463 - }, - "id": 2761323, - "non_hive": { - "close": 762, - "high": 762, - "low": 762, - "open": 762, - "volume": 762 - }, - "open": "2020-11-05T19:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 27260, - "high": 27260, - "low": 27260, - "open": 27260, - "volume": 27260 - }, - "id": 2761326, - "non_hive": { - "close": 3214, - "high": 3214, - "low": 3214, - "open": 3214, - "volume": 3214 - }, - "open": "2020-11-05T20:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 4005, - "high": 9007, - "low": 4005, - "open": 9007, - "volume": 1426840 - }, - "id": 2761330, - "non_hive": { - "close": 472, - "high": 1062, - "low": 472, - "open": 1062, - "volume": 168223 - }, - "open": "2020-11-05T20:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 89932, - "high": 6755, - "low": 89932, - "open": 6755, - "volume": 100000 - }, - "id": 2761337, - "non_hive": { - "close": 10497, - "high": 796, - "low": 10497, - "open": 796, - "volume": 11683 - }, - "open": "2020-11-05T20:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 81, - "high": 81, - "low": 81, - "open": 81, - "volume": 81 - }, - "id": 2761340, - "non_hive": { - "close": 9, - "high": 9, - "low": 9, - "open": 9, - "volume": 9 - }, - "open": "2020-11-05T20:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 17046, - "high": 17003, - "low": 117431, - "open": 19085, - "volume": 601489 - }, - "id": 2761343, - "non_hive": { - "close": 2000, - "high": 2001, - "low": 13707, - "open": 2246, - "volume": 70281 - }, - "open": "2020-11-05T21:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 4444, - "high": 4011, - "low": 17046, - "open": 17046, - "volume": 59542 - }, - "id": 2761357, - "non_hive": { - "close": 522, - "high": 472, - "low": 2000, - "open": 2000, - "volume": 6993 - }, - "open": "2020-11-05T21:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 8459, - "high": 8459, - "low": 8459, - "open": 8459, - "volume": 8459 - }, - "id": 2761366, - "non_hive": { - "close": 995, - "high": 995, - "low": 995, - "open": 995, - "volume": 995 - }, - "open": "2020-11-05T21:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 17046, - "high": 8484, - "low": 17046, - "open": 8484, - "volume": 42576 - }, - "id": 2761369, - "non_hive": { - "close": 2000, - "high": 998, - "low": 2000, - "open": 998, - "volume": 4998 - }, - "open": "2020-11-05T21:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 12699, - "high": 12301, - "low": 52, - "open": 17046, - "volume": 43332 - }, - "id": 2761375, - "non_hive": { - "close": 1493, - "high": 1447, - "low": 6, - "open": 2000, - "volume": 5091 - }, - "open": "2020-11-05T21:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 4152, - "high": 838, - "low": 5987, - "open": 161, - "volume": 35784 - }, - "id": 2761383, - "non_hive": { - "close": 490, - "high": 99, - "low": 700, - "open": 19, - "volume": 4210 - }, - "open": "2020-11-05T21:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 755, - "high": 755, - "low": 1901, - "open": 17100, - "volume": 36857 - }, - "id": 2761389, - "non_hive": { - "close": 89, - "high": 89, - "low": 222, - "open": 2000, - "volume": 4311 - }, - "open": "2020-11-05T21:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 2020, - "high": 2020, - "low": 2020, - "open": 2020, - "volume": 2020 - }, - "id": 2761395, - "non_hive": { - "close": 238, - "high": 238, - "low": 238, - "open": 238, - "volume": 238 - }, - "open": "2020-11-05T21:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 34415, - "high": 8501, - "low": 34415, - "open": 8501, - "volume": 146886 - }, - "id": 2761398, - "non_hive": { - "close": 4014, - "high": 1001, - "low": 4014, - "open": 1001, - "volume": 17148 - }, - "open": "2020-11-05T21:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 409, - "high": 601, - "low": 409, - "open": 8477, - "volume": 112399 - }, - "id": 2761406, - "non_hive": { - "close": 48, - "high": 71, - "low": 48, - "open": 998, - "volume": 13257 - }, - "open": "2020-11-05T21:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000, - "high": 113016, - "low": 1000, - "open": 6002, - "volume": 120018 - }, - "id": 2761411, - "non_hive": { - "close": 117, - "high": 13336, - "low": 117, - "open": 708, - "volume": 14161 - }, - "open": "2020-11-05T21:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 16958, - "high": 3476, - "low": 16958, - "open": 3476, - "volume": 67001 - }, - "id": 2761417, - "non_hive": { - "close": 1999, - "high": 410, - "low": 1999, - "open": 410, - "volume": 7900 - }, - "open": "2020-11-05T22:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 31966, - "high": 31966, - "low": 16958, - "open": 16958, - "volume": 110838 - }, - "id": 2761428, - "non_hive": { - "close": 3770, - "high": 3770, - "low": 1999, - "open": 1999, - "volume": 13068 - }, - "open": "2020-11-05T22:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 84268, - "high": 84268, - "low": 84268, - "open": 84268, - "volume": 84268 - }, - "id": 2761436, - "non_hive": { - "close": 9973, - "high": 9973, - "low": 9973, - "open": 9973, - "volume": 9973 - }, - "open": "2020-11-05T22:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 32652, - "high": 30432, - "low": 32652, - "open": 30432, - "volume": 63084 - }, - "id": 2761439, - "non_hive": { - "close": 3864, - "high": 3602, - "low": 3864, - "open": 3602, - "volume": 7466 - }, - "open": "2020-11-05T22:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 253202, - "high": 253202, - "low": 253202, - "open": 253202, - "volume": 253202 - }, - "id": 2761444, - "non_hive": { - "close": 29969, - "high": 29969, - "low": 29969, - "open": 29969, - "volume": 29969 - }, - "open": "2020-11-05T22:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 382734, - "high": 25347, - "low": 382734, - "open": 25347, - "volume": 408081 - }, - "id": 2761447, - "non_hive": { - "close": 45296, - "high": 3000, - "low": 45296, - "open": 3000, - "volume": 48296 - }, - "open": "2020-11-05T22:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 441631, - "high": 441631, - "low": 441631, - "open": 441631, - "volume": 441631 - }, - "id": 2761450, - "non_hive": { - "close": 52266, - "high": 52266, - "low": 52266, - "open": 52266, - "volume": 52266 - }, - "open": "2020-11-05T22:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 20867, - "high": 20867, - "low": 20867, - "open": 20867, - "volume": 20867 - }, - "id": 2761453, - "non_hive": { - "close": 2470, - "high": 2470, - "low": 2470, - "open": 2470, - "volume": 2470 - }, - "open": "2020-11-05T22:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 149865, - "high": 149865, - "low": 217002, - "open": 4004, - "volume": 1047813 - }, - "id": 2761456, - "non_hive": { - "close": 17804, - "high": 17804, - "low": 25685, - "open": 474, - "volume": 124113 - }, - "open": "2020-11-05T23:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 336, - "high": 336, - "low": 336, - "open": 336, - "volume": 336 - }, - "id": 2761462, - "non_hive": { - "close": 40, - "high": 40, - "low": 40, - "open": 40, - "volume": 40 - }, - "open": "2020-11-05T23:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 17020, - "high": 1979, - "low": 499819, - "open": 1979, - "volume": 809162 - }, - "id": 2761465, - "non_hive": { - "close": 2000, - "high": 235, - "low": 58728, - "open": 235, - "volume": 95148 - }, - "open": "2020-11-05T23:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 16992, - "high": 16992, - "low": 17020, - "open": 17020, - "volume": 68024 - }, - "id": 2761474, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 8000 - }, - "open": "2020-11-05T23:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 245, - "high": 245, - "low": 72712, - "open": 2492, - "volume": 100245 - }, - "id": 2761482, - "non_hive": { - "close": 29, - "high": 29, - "low": 8507, - "open": 293, - "volume": 11735 - }, - "open": "2020-11-05T23:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 27076, - "high": 14007, - "low": 7686, - "open": 14007, - "volume": 73603 - }, - "id": 2761487, - "non_hive": { - "close": 3201, - "high": 1656, - "low": 900, - "open": 1656, - "volume": 8677 - }, - "open": "2020-11-05T23:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 960262, - "high": 2580, - "low": 960262, - "open": 2580, - "volume": 973806 - }, - "id": 2761494, - "non_hive": { - "close": 112351, - "high": 305, - "low": 112351, - "open": 305, - "volume": 113941 - }, - "open": "2020-11-05T23:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 507, - "high": 507, - "low": 15597, - "open": 15597, - "volume": 16104 - }, - "id": 2761499, - "non_hive": { - "close": 60, - "high": 60, - "low": 1844, - "open": 1844, - "volume": 1904 - }, - "open": "2020-11-05T23:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 10953, - "high": 10953, - "low": 1129101, - "open": 16271, - "volume": 2010953 - }, - "id": 2761504, - "non_hive": { - "close": 1295, - "high": 1295, - "low": 132105, - "open": 1904, - "volume": 235304 - }, - "open": "2020-11-06T00:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 110000, - "high": 11067, - "low": 110000, - "open": 11067, - "volume": 3015623 - }, - "id": 2761511, - "non_hive": { - "close": 12870, - "high": 1295, - "low": 12870, - "open": 1295, - "volume": 352830 - }, - "open": "2020-11-06T00:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 267642, - "high": 76, - "low": 267642, - "open": 11481, - "volume": 304760 - }, - "id": 2761517, - "non_hive": { - "close": 31314, - "high": 9, - "low": 31314, - "open": 1353, - "volume": 35674 - }, - "open": "2020-11-06T00:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 3767, - "high": 3767, - "low": 3767, - "open": 3767, - "volume": 3767 - }, - "id": 2761524, - "non_hive": { - "close": 444, - "high": 444, - "low": 444, - "open": 444, - "volume": 444 - }, - "open": "2020-11-06T00:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 132527, - "high": 132527, - "low": 17021, - "open": 17021, - "volume": 149548 - }, - "id": 2761527, - "non_hive": { - "close": 15572, - "high": 15572, - "low": 1999, - "open": 1999, - "volume": 17571 - }, - "open": "2020-11-06T00:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 124826, - "high": 3015, - "low": 124826, - "open": 3015, - "volume": 472671 - }, - "id": 2761530, - "non_hive": { - "close": 14604, - "high": 353, - "low": 14604, - "open": 353, - "volume": 55306 - }, - "open": "2020-11-06T00:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 17022, - "high": 17022, - "low": 17022, - "open": 17022, - "volume": 17022 - }, - "id": 2761535, - "non_hive": { - "close": 1991, - "high": 1991, - "low": 1991, - "open": 1991, - "volume": 1991 - }, - "open": "2020-11-06T00:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 33000, - "high": 33000, - "low": 33000, - "open": 33000, - "volume": 33000 - }, - "id": 2761538, - "non_hive": { - "close": 3861, - "high": 3861, - "low": 3861, - "open": 3861, - "volume": 3861 - }, - "open": "2020-11-06T00:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1584, - "high": 1584, - "low": 1584, - "open": 1584, - "volume": 1584 - }, - "id": 2761541, - "non_hive": { - "close": 186, - "high": 186, - "low": 186, - "open": 186, - "volume": 186 - }, - "open": "2020-11-06T01:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 8227, - "high": 8227, - "low": 16891, - "open": 16891, - "volume": 31089 - }, - "id": 2761545, - "non_hive": { - "close": 977, - "high": 977, - "low": 1999, - "open": 1999, - "volume": 3683 - }, - "open": "2020-11-06T01:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 918, - "high": 1044, - "low": 16837, - "open": 1044, - "volume": 18799 - }, - "id": 2761550, - "non_hive": { - "close": 109, - "high": 124, - "low": 1999, - "open": 124, - "volume": 2232 - }, - "open": "2020-11-06T01:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 8415, - "high": 8415, - "low": 7657, - "open": 7657, - "volume": 16072 - }, - "id": 2761556, - "non_hive": { - "close": 999, - "high": 999, - "low": 909, - "open": 909, - "volume": 1908 - }, - "open": "2020-11-06T01:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 1382746, - "high": 16806, - "low": 1382746, - "open": 16806, - "volume": 1444549 - }, - "id": 2761561, - "non_hive": { - "close": 161782, - "high": 1995, - "low": 161782, - "open": 1995, - "volume": 169065 - }, - "open": "2020-11-06T01:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 4698, - "high": 4698, - "low": 4698, - "open": 4698, - "volume": 4698 - }, - "id": 2761566, - "non_hive": { - "close": 556, - "high": 556, - "low": 556, - "open": 556, - "volume": 556 - }, - "open": "2020-11-06T01:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 150994, - "high": 665313, - "low": 12193, - "open": 12193, - "volume": 1009757 - }, - "id": 2761569, - "non_hive": { - "close": 17936, - "high": 79030, - "low": 1443, - "open": 1443, - "volume": 119937 - }, - "open": "2020-11-06T02:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 2421718, - "high": 2421718, - "low": 435231, - "open": 435231, - "volume": 5045292 - }, - "id": 2761574, - "non_hive": { - "close": 287700, - "high": 287700, - "low": 51700, - "open": 51700, - "volume": 599375 - }, - "open": "2020-11-06T02:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1054483, - "high": 819546, - "low": 1992688, - "open": 1992688, - "volume": 3866717 - }, - "id": 2761579, - "non_hive": { - "close": 125378, - "high": 97444, - "low": 236731, - "open": 236731, - "volume": 459553 - }, - "open": "2020-11-06T02:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1614096, - "high": 1614096, - "low": 1614096, - "open": 1614096, - "volume": 1614096 - }, - "id": 2761584, - "non_hive": { - "close": 191916, - "high": 191916, - "low": 191916, - "open": 191916, - "volume": 191916 - }, - "open": "2020-11-06T02:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 1202431, - "high": 1202431, - "low": 1202431, - "open": 1202431, - "volume": 1202431 - }, - "id": 2761587, - "non_hive": { - "close": 142969, - "high": 142969, - "low": 142969, - "open": 142969, - "volume": 142969 - }, - "open": "2020-11-06T02:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 11278, - "high": 11278, - "low": 11278, - "open": 11278, - "volume": 11278 - }, - "id": 2761590, - "non_hive": { - "close": 1341, - "high": 1341, - "low": 1341, - "open": 1341, - "volume": 1341 - }, - "open": "2020-11-06T02:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 20227, - "high": 20227, - "low": 20227, - "open": 20227, - "volume": 20227 - }, - "id": 2761593, - "non_hive": { - "close": 2405, - "high": 2405, - "low": 2405, - "open": 2405, - "volume": 2405 - }, - "open": "2020-11-06T02:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 3411, - "high": 40875, - "low": 3411, - "open": 40875, - "volume": 44286 - }, - "id": 2761596, - "non_hive": { - "close": 405, - "high": 4860, - "low": 405, - "open": 4860, - "volume": 5265 - }, - "open": "2020-11-06T02:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1055208, - "high": 1055208, - "low": 16821, - "open": 16821, - "volume": 3173848 - }, - "id": 2761599, - "non_hive": { - "close": 125939, - "high": 125939, - "low": 1999, - "open": 1999, - "volume": 377950 - }, - "open": "2020-11-06T02:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 10000, - "high": 1191, - "low": 10000, - "open": 1191, - "volume": 11191 - }, - "id": 2761602, - "non_hive": { - "close": 1180, - "high": 142, - "low": 1180, - "open": 142, - "volume": 1322 - }, - "open": "2020-11-06T03:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 265, - "high": 6001, - "low": 265, - "open": 6001, - "volume": 6266 - }, - "id": 2761608, - "non_hive": { - "close": 31, - "high": 716, - "low": 31, - "open": 716, - "volume": 747 - }, - "open": "2020-11-06T03:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 10755, - "high": 10755, - "low": 10755, - "open": 10755, - "volume": 10755 - }, - "id": 2761613, - "non_hive": { - "close": 1283, - "high": 1283, - "low": 1283, - "open": 1283, - "volume": 1283 - }, - "open": "2020-11-06T03:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2228, - "high": 2228, - "low": 2228, - "open": 2228, - "volume": 2228 - }, - "id": 2761616, - "non_hive": { - "close": 266, - "high": 266, - "low": 266, - "open": 266, - "volume": 266 - }, - "open": "2020-11-06T04:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 9994, - "high": 9994, - "low": 9994, - "open": 9994, - "volume": 9994 - }, - "id": 2761620, - "non_hive": { - "close": 1192, - "high": 1192, - "low": 1192, - "open": 1192, - "volume": 1192 - }, - "open": "2020-11-06T04:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 2645, - "high": 88024, - "low": 3645, - "open": 3645, - "volume": 94314 - }, - "id": 2761623, - "non_hive": { - "close": 317, - "high": 10554, - "low": 435, - "open": 435, - "volume": 11306 - }, - "open": "2020-11-06T04:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1612, - "high": 14036, - "low": 1612, - "open": 14036, - "volume": 15648 - }, - "id": 2761628, - "non_hive": { - "close": 193, - "high": 1682, - "low": 193, - "open": 1682, - "volume": 1875 - }, - "open": "2020-11-06T04:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 425, - "high": 425, - "low": 425, - "open": 425, - "volume": 425 - }, - "id": 2761633, - "non_hive": { - "close": 51, - "high": 51, - "low": 51, - "open": 51, - "volume": 51 - }, - "open": "2020-11-06T04:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 8179, - "high": 8179, - "low": 8179, - "open": 8179, - "volume": 8179 - }, - "id": 2761636, - "non_hive": { - "close": 980, - "high": 980, - "low": 980, - "open": 980, - "volume": 980 - }, - "open": "2020-11-06T05:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 44894, - "high": 44894, - "low": 44894, - "open": 44894, - "volume": 44894 - }, - "id": 2761640, - "non_hive": { - "close": 5380, - "high": 5380, - "low": 5380, - "open": 5380, - "volume": 5380 - }, - "open": "2020-11-06T05:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 83237, - "high": 16763, - "low": 83237, - "open": 16763, - "volume": 100000 - }, - "id": 2761643, - "non_hive": { - "close": 9930, - "high": 2000, - "low": 9930, - "open": 2000, - "volume": 11930 - }, - "open": "2020-11-06T05:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 1701, - "high": 16763, - "low": 1701, - "open": 16763, - "volume": 100001 - }, - "id": 2761646, - "non_hive": { - "close": 201, - "high": 2000, - "low": 201, - "open": 2000, - "volume": 11914 - }, - "open": "2020-11-06T05:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 16312, - "high": 16312, - "low": 16312, - "open": 16312, - "volume": 16312 - }, - "id": 2761653, - "non_hive": { - "close": 1945, - "high": 1945, - "low": 1945, - "open": 1945, - "volume": 1945 - }, - "open": "2020-11-06T05:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 4221, - "high": 4221, - "low": 16886, - "open": 16886, - "volume": 21107 - }, - "id": 2761656, - "non_hive": { - "close": 500, - "high": 500, - "low": 2000, - "open": 2000, - "volume": 2500 - }, - "open": "2020-11-06T05:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 158993, - "high": 158993, - "low": 3765, - "open": 1802, - "volume": 958483 - }, - "id": 2761659, - "non_hive": { - "close": 18952, - "high": 18952, - "low": 445, - "open": 213, - "volume": 114211 - }, - "open": "2020-11-06T06:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 10201, - "high": 10201, - "low": 5524, - "open": 11255, - "volume": 26980 - }, - "id": 2761664, - "non_hive": { - "close": 1216, - "high": 1216, - "low": 658, - "open": 1341, - "volume": 3215 - }, - "open": "2020-11-06T06:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 208994, - "high": 5151, - "low": 208994, - "open": 5151, - "volume": 214145 - }, - "id": 2761670, - "non_hive": { - "close": 24912, - "high": 614, - "low": 24912, - "open": 614, - "volume": 25526 - }, - "open": "2020-11-06T06:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 402, - "high": 208994, - "low": 402, - "open": 208994, - "volume": 211443 - }, - "id": 2761674, - "non_hive": { - "close": 47, - "high": 24912, - "low": 47, - "open": 24912, - "volume": 25203 - }, - "open": "2020-11-06T06:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 980, - "high": 4874, - "low": 980, - "open": 150998, - "volume": 170627 - }, - "id": 2761678, - "non_hive": { - "close": 116, - "high": 581, - "low": 116, - "open": 17999, - "volume": 20338 - }, - "open": "2020-11-06T06:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1459, - "high": 1459, - "low": 91996, - "open": 91996, - "volume": 93455 - }, - "id": 2761685, - "non_hive": { - "close": 174, - "high": 174, - "low": 10966, - "open": 10966, - "volume": 11140 - }, - "open": "2020-11-06T07:00:00", - "seconds": 300 - }, - { - "hive": { - "close": 38, - "high": 2357, - "low": 38, - "open": 2357, - "volume": 108346 - }, - "id": 2761691, - "non_hive": { - "close": 4, - "high": 281, - "low": 4, - "open": 281, - "volume": 12908 - }, - "open": "2020-11-06T07:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 14493, - "high": 14493, - "low": 16807, - "open": 16807, - "volume": 48107 - }, - "id": 2761699, - "non_hive": { - "close": 1724, - "high": 1724, - "low": 1999, - "open": 1999, - "volume": 5722 - }, - "open": "2020-11-06T07:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 5926, - "high": 5926, - "low": 5926, - "open": 5926, - "volume": 5926 - }, - "id": 2761705, - "non_hive": { - "close": 705, - "high": 705, - "low": 705, - "open": 705, - "volume": 705 - }, - "open": "2020-11-06T07:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 1704, - "high": 18464, - "low": 1704, - "open": 18464, - "volume": 20168 - }, - "id": 2761708, - "non_hive": { - "close": 203, - "high": 2201, - "low": 203, - "open": 2201, - "volume": 2404 - }, - "open": "2020-11-06T07:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 1166, - "high": 1166, - "low": 1166, - "open": 1166, - "volume": 1166 - }, - "id": 2761713, - "non_hive": { - "close": 139, - "high": 139, - "low": 139, - "open": 139, - "volume": 139 - }, - "open": "2020-11-06T07:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 463201, - "high": 463201, - "low": 463201, - "open": 463201, - "volume": 463201 - }, - "id": 2761716, - "non_hive": { - "close": 55181, - "high": 55181, - "low": 55181, - "open": 55181, - "volume": 55181 - }, - "open": "2020-11-06T07:55:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000000, - "high": 1107, - "low": 1000000, - "open": 1107, - "volume": 1001107 - }, - "id": 2761719, - "non_hive": { - "close": 119130, - "high": 132, - "low": 119130, - "open": 132, - "volume": 119262 - }, - "open": "2020-11-06T08:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 4289, - "high": 4289, - "low": 4289, - "open": 4289, - "volume": 4289 - }, - "id": 2761725, - "non_hive": { - "close": 511, - "high": 511, - "low": 511, - "open": 511, - "volume": 511 - }, - "open": "2020-11-06T08:15:00", - "seconds": 300 - }, - { - "hive": { - "close": 31061, - "high": 8271, - "low": 33559, - "open": 8271, - "volume": 87204 - }, - "id": 2761728, - "non_hive": { - "close": 3700, - "high": 986, - "low": 3997, - "open": 986, - "volume": 10389 - }, - "open": "2020-11-06T08:20:00", - "seconds": 300 - }, - { - "hive": { - "close": 8624, - "high": 8624, - "low": 8624, - "open": 8624, - "volume": 8624 - }, - "id": 2761735, - "non_hive": { - "close": 1028, - "high": 1028, - "low": 1028, - "open": 1028, - "volume": 1028 - }, - "open": "2020-11-06T08:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 18983, - "high": 4437, - "low": 18983, - "open": 4437, - "volume": 49007 - }, - "id": 2761738, - "non_hive": { - "close": 2239, - "high": 529, - "low": 2239, - "open": 529, - "volume": 5817 - }, - "open": "2020-11-06T08:35:00", - "seconds": 300 - }, - { - "hive": { - "close": 62919, - "high": 62919, - "low": 62919, - "open": 62919, - "volume": 62919 - }, - "id": 2761743, - "non_hive": { - "close": 7500, - "high": 7500, - "low": 7500, - "open": 7500, - "volume": 7500 - }, - "open": "2020-11-06T08:40:00", - "seconds": 300 - }, - { - "hive": { - "close": 18983, - "high": 18983, - "low": 18983, - "open": 18983, - "volume": 18983 - }, - "id": 2761746, - "non_hive": { - "close": 2261, - "high": 2261, - "low": 2261, - "open": 2261, - "volume": 2261 - }, - "open": "2020-11-06T08:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 318, - "high": 318, - "low": 318, - "open": 318, - "volume": 318 - }, - "id": 2761749, - "non_hive": { - "close": 38, - "high": 38, - "low": 38, - "open": 38, - "volume": 38 - }, - "open": "2020-11-06T08:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 4974, - "high": 4974, - "low": 4974, - "open": 4974, - "volume": 4974 - }, - "id": 2761752, - "non_hive": { - "close": 593, - "high": 593, - "low": 593, - "open": 593, - "volume": 593 - }, - "open": "2020-11-06T09:05:00", - "seconds": 300 - }, - { - "hive": { - "close": 125839, - "high": 125839, - "low": 125839, - "open": 125839, - "volume": 125839 - }, - "id": 2761756, - "non_hive": { - "close": 15000, - "high": 15000, - "low": 15000, - "open": 15000, - "volume": 15000 - }, - "open": "2020-11-06T09:10:00", - "seconds": 300 - }, - { - "hive": { - "close": 4289, - "high": 19857, - "low": 4289, - "open": 19857, - "volume": 24146 - }, - "id": 2761759, - "non_hive": { - "close": 511, - "high": 2367, - "low": 511, - "open": 2367, - "volume": 2878 - }, - "open": "2020-11-06T09:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 2365, - "high": 2365, - "low": 2365, - "open": 2365, - "volume": 2365 - }, - "id": 2761764, - "non_hive": { - "close": 282, - "high": 282, - "low": 282, - "open": 282, - "volume": 282 - }, - "open": "2020-11-06T09:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 2810, - "high": 2810, - "low": 2810, - "open": 2810, - "volume": 2810 - }, - "id": 2761767, - "non_hive": { - "close": 335, - "high": 335, - "low": 335, - "open": 335, - "volume": 335 - }, - "open": "2020-11-06T09:50:00", - "seconds": 300 - }, - { - "hive": { - "close": 7902, - "high": 7902, - "low": 7902, - "open": 7902, - "volume": 7902 - }, - "id": 2761770, - "non_hive": { - "close": 942, - "high": 942, - "low": 942, - "open": 942, - "volume": 942 - }, - "open": "2020-11-06T10:25:00", - "seconds": 300 - }, - { - "hive": { - "close": 4250, - "high": 4250, - "low": 4250, - "open": 4250, - "volume": 4250 - }, - "id": 2761774, - "non_hive": { - "close": 506, - "high": 506, - "low": 506, - "open": 506, - "volume": 506 - }, - "open": "2020-11-06T10:30:00", - "seconds": 300 - }, - { - "hive": { - "close": 218, - "high": 218, - "low": 64001, - "open": 64001, - "volume": 64219 - }, - "id": 2761777, - "non_hive": { - "close": 26, - "high": 26, - "low": 7629, - "open": 7629, - "volume": 7655 - }, - "open": "2020-11-06T10:45:00", - "seconds": 300 - }, - { - "hive": { - "close": 1000000, - "high": 1000000, - "low": 485620, - "open": 485620, - "volume": 1620694 - }, - "id": 2761782, - "non_hive": { - "close": 119924, - "high": 119924, - "low": 57885, - "open": 57885, - "volume": 194001 - }, - "open": "2020-11-06T10:55:00", - "seconds": 300 - } -] diff --git a/hived/tavern/condenser_api_patterns/get_market_history/300.tavern.yaml b/hived/tavern/condenser_api_patterns/get_market_history/300.tavern.yaml deleted file mode 100644 index 3e87d6b9bf5d89810b920b2d894fee653726c709..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history/300.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_market_history - - marks: - - patterntest # this works only for new data from less than month before now - - includes: - - !include ../../common.yaml - - stages: - - name: get_market_history - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_market_history" - params: [300,"2016-04-03T00:00:00","2031-10-20T00:00:00"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "300" - directory: "condenser_api_patterns/get_market_history" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_market_history/3600.pat.json b/hived/tavern/condenser_api_patterns/get_market_history/3600.pat.json deleted file mode 100644 index 9a654b355567b3f2cba71bf35395444605bd3a9c..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history/3600.pat.json +++ /dev/null @@ -1,108207 +0,0 @@ -[ - { - "hive": { - "close": 687, - "high": 385246, - "low": 13, - "open": 31368, - "volume": 2710547 - }, - "id": 2556899, - "non_hive": { - "close": 164, - "high": 96270, - "low": 3, - "open": 7655, - "volume": 663223 - }, - "open": "2020-03-11T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1167, - "high": 36, - "low": 2957, - "open": 2780, - "volume": 6129638 - }, - "id": 2556948, - "non_hive": { - "close": 291, - "high": 9, - "low": 689, - "open": 689, - "volume": 1506969 - }, - "open": "2020-03-11T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6857, - "high": 36, - "low": 13, - "open": 2515, - "volume": 6835373 - }, - "id": 2557021, - "non_hive": { - "close": 1687, - "high": 9, - "low": 3, - "open": 626, - "volume": 1678779 - }, - "open": "2020-03-11T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 349260, - "high": 349260, - "low": 292600, - "open": 307000, - "volume": 20562150 - }, - "id": 2557075, - "non_hive": { - "close": 89416, - "high": 89416, - "low": 70838, - "open": 75324, - "volume": 5133050 - }, - "open": "2020-03-11T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 291019, - "high": 2308, - "low": 400000, - "open": 94237, - "volume": 3022577 - }, - "id": 2557184, - "non_hive": { - "close": 72754, - "high": 592, - "low": 98880, - "open": 24126, - "volume": 763063 - }, - "open": "2020-03-11T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 108680, - "high": 108680, - "low": 6, - "open": 102703, - "volume": 6092168 - }, - "id": 2557251, - "non_hive": { - "close": 27889, - "high": 27889, - "low": 1, - "open": 26130, - "volume": 1554208 - }, - "open": "2020-03-11T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8, - "high": 274, - "low": 11, - "open": 2806, - "volume": 4571291 - }, - "id": 2557325, - "non_hive": { - "close": 2, - "high": 71, - "low": 2, - "open": 714, - "volume": 1170374 - }, - "open": "2020-03-11T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8227, - "high": 202, - "low": 12, - "open": 5000, - "volume": 3209253 - }, - "id": 2557391, - "non_hive": { - "close": 2074, - "high": 52, - "low": 3, - "open": 1265, - "volume": 820476 - }, - "open": "2020-03-11T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12079, - "high": 134, - "low": 97, - "open": 16876, - "volume": 4425984 - }, - "id": 2557458, - "non_hive": { - "close": 3078, - "high": 35, - "low": 24, - "open": 4286, - "volume": 1129625 - }, - "open": "2020-03-11T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9590, - "high": 7, - "low": 12, - "open": 400000, - "volume": 855258 - }, - "id": 2557523, - "non_hive": { - "close": 2421, - "high": 2, - "low": 3, - "open": 101016, - "volume": 216319 - }, - "open": "2020-03-11T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3581912, - "high": 3, - "low": 20029, - "open": 70751, - "volume": 14265814 - }, - "id": 2557577, - "non_hive": { - "close": 903000, - "high": 1, - "low": 5047, - "open": 17861, - "volume": 3602543 - }, - "open": "2020-03-11T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1344, - "high": 3, - "low": 10, - "open": 183639, - "volume": 12100925 - }, - "id": 2557646, - "non_hive": { - "close": 344, - "high": 1, - "low": 2, - "open": 46282, - "volume": 3049600 - }, - "open": "2020-03-11T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 432, - "high": 1154, - "low": 44, - "open": 84582, - "volume": 2373993 - }, - "id": 2557705, - "non_hive": { - "close": 110, - "high": 296, - "low": 11, - "open": 21520, - "volume": 603405 - }, - "open": "2020-03-11T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 425, - "high": 680, - "low": 12, - "open": 162754, - "volume": 4362850 - }, - "id": 2557759, - "non_hive": { - "close": 108, - "high": 173, - "low": 3, - "open": 41033, - "volume": 1097937 - }, - "open": "2020-03-12T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 516, - "high": 70, - "low": 198758, - "open": 5115, - "volume": 4349812 - }, - "id": 2557803, - "non_hive": { - "close": 131, - "high": 18, - "low": 49227, - "open": 1300, - "volume": 1088108 - }, - "open": "2020-03-12T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6489, - "high": 229, - "low": 48, - "open": 1344, - "volume": 1863646 - }, - "id": 2557872, - "non_hive": { - "close": 1584, - "high": 57, - "low": 11, - "open": 334, - "volume": 458356 - }, - "open": "2020-03-12T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 735, - "high": 28, - "low": 2985, - "open": 246, - "volume": 602559 - }, - "id": 2557922, - "non_hive": { - "close": 182, - "high": 7, - "low": 727, - "open": 61, - "volume": 147013 - }, - "open": "2020-03-12T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21240, - "high": 40, - "low": 67, - "open": 492, - "volume": 3082053 - }, - "id": 2557961, - "non_hive": { - "close": 5173, - "high": 10, - "low": 16, - "open": 122, - "volume": 750979 - }, - "open": "2020-03-12T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6, - "high": 20, - "low": 6, - "open": 4907, - "volume": 1269089 - }, - "id": 2558010, - "non_hive": { - "close": 1, - "high": 5, - "low": 1, - "open": 1195, - "volume": 303392 - }, - "open": "2020-03-12T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 58, - "high": 16, - "low": 13, - "open": 144, - "volume": 1161014 - }, - "id": 2558053, - "non_hive": { - "close": 14, - "high": 4, - "low": 3, - "open": 35, - "volume": 271156 - }, - "open": "2020-03-12T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4012, - "high": 58, - "low": 24674, - "open": 19270, - "volume": 2507906 - }, - "id": 2558101, - "non_hive": { - "close": 954, - "high": 14, - "low": 5483, - "open": 4492, - "volume": 573504 - }, - "open": "2020-03-12T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11297, - "high": 16, - "low": 4517, - "open": 4292, - "volume": 714822 - }, - "id": 2558160, - "non_hive": { - "close": 2520, - "high": 4, - "low": 1004, - "open": 954, - "volume": 163547 - }, - "open": "2020-03-12T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1277, - "high": 497, - "low": 2000, - "open": 2000, - "volume": 2695252 - }, - "id": 2558204, - "non_hive": { - "close": 300, - "high": 118, - "low": 449, - "open": 449, - "volume": 628896 - }, - "open": "2020-03-12T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 93382, - "high": 8, - "low": 78359, - "open": 9868, - "volume": 978649 - }, - "id": 2558268, - "non_hive": { - "close": 22212, - "high": 2, - "low": 17636, - "open": 2278, - "volume": 227883 - }, - "open": "2020-03-12T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2354, - "high": 29, - "low": 91565, - "open": 67, - "volume": 3449769 - }, - "id": 2558310, - "non_hive": { - "close": 546, - "high": 7, - "low": 20144, - "open": 16, - "volume": 779638 - }, - "open": "2020-03-12T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 121, - "high": 370, - "low": 27, - "open": 5792, - "volume": 1109861 - }, - "id": 2558377, - "non_hive": { - "close": 27, - "high": 86, - "low": 5, - "open": 1300, - "volume": 239378 - }, - "open": "2020-03-12T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1088, - "high": 177342, - "low": 10, - "open": 221056, - "volume": 1988296 - }, - "id": 2558436, - "non_hive": { - "close": 233, - "high": 39503, - "low": 2, - "open": 46670, - "volume": 430393 - }, - "open": "2020-03-12T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49978, - "high": 125165, - "low": 2000, - "open": 1585, - "volume": 1322491 - }, - "id": 2558504, - "non_hive": { - "close": 11155, - "high": 28011, - "low": 431, - "open": 353, - "volume": 293290 - }, - "open": "2020-03-12T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14004, - "high": 144, - "low": 13614, - "open": 362, - "volume": 1346402 - }, - "id": 2558563, - "non_hive": { - "close": 3151, - "high": 34, - "low": 2975, - "open": 81, - "volume": 302311 - }, - "open": "2020-03-12T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8699, - "high": 17, - "low": 80, - "open": 9786, - "volume": 837446 - }, - "id": 2558638, - "non_hive": { - "close": 1945, - "high": 4, - "low": 17, - "open": 2202, - "volume": 185516 - }, - "open": "2020-03-12T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1974, - "high": 1014, - "low": 36, - "open": 46, - "volume": 3165021 - }, - "id": 2558707, - "non_hive": { - "close": 429, - "high": 228, - "low": 7, - "open": 10, - "volume": 676644 - }, - "open": "2020-03-12T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2277, - "high": 4, - "low": 10, - "open": 5858, - "volume": 7258656 - }, - "id": 2558770, - "non_hive": { - "close": 462, - "high": 1, - "low": 2, - "open": 1296, - "volume": 1558257 - }, - "open": "2020-03-12T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9733, - "high": 31, - "low": 335, - "open": 31, - "volume": 7472111 - }, - "id": 2558853, - "non_hive": { - "close": 2177, - "high": 7, - "low": 67, - "open": 7, - "volume": 1567814 - }, - "open": "2020-03-12T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1003, - "high": 21688, - "low": 10, - "open": 590, - "volume": 4179909 - }, - "id": 2558935, - "non_hive": { - "close": 217, - "high": 4854, - "low": 2, - "open": 132, - "volume": 880062 - }, - "open": "2020-03-12T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9905, - "high": 120, - "low": 5, - "open": 7672, - "volume": 1374306 - }, - "id": 2559007, - "non_hive": { - "close": 2133, - "high": 26, - "low": 1, - "open": 1658, - "volume": 286014 - }, - "open": "2020-03-12T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16386, - "high": 4656, - "low": 40, - "open": 33498, - "volume": 2955300 - }, - "id": 2559065, - "non_hive": { - "close": 3278, - "high": 1000, - "low": 8, - "open": 6804, - "volume": 610653 - }, - "open": "2020-03-12T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28510, - "high": 9995, - "low": 40, - "open": 1139, - "volume": 2836472 - }, - "id": 2559149, - "non_hive": { - "close": 5867, - "high": 2135, - "low": 8, - "open": 241, - "volume": 577108 - }, - "open": "2020-03-12T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 83, - "low": 331013, - "open": 164497, - "volume": 7029151 - }, - "id": 2559225, - "non_hive": { - "close": 19, - "high": 17, - "low": 60000, - "open": 32927, - "volume": 1371515 - }, - "open": "2020-03-13T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42483, - "high": 768, - "low": 400000, - "open": 170, - "volume": 5679250 - }, - "id": 2559301, - "non_hive": { - "close": 8284, - "high": 162, - "low": 73244, - "open": 34, - "volume": 1077760 - }, - "open": "2020-03-13T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25363, - "high": 15560, - "low": 28, - "open": 42483, - "volume": 5197331 - }, - "id": 2559356, - "non_hive": { - "close": 4818, - "high": 3111, - "low": 5, - "open": 8284, - "volume": 984013 - }, - "open": "2020-03-13T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39635, - "high": 1257, - "low": 7641, - "open": 20001, - "volume": 2318284 - }, - "id": 2559435, - "non_hive": { - "close": 7844, - "high": 249, - "low": 1415, - "open": 3834, - "volume": 446214 - }, - "open": "2020-03-13T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49773, - "high": 5855, - "low": 90000, - "open": 22360, - "volume": 997510 - }, - "id": 2559492, - "non_hive": { - "close": 10090, - "high": 1229, - "low": 16794, - "open": 4427, - "volume": 197219 - }, - "open": "2020-03-13T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 110, - "high": 78, - "low": 322766, - "open": 299200, - "volume": 1720481 - }, - "id": 2559529, - "non_hive": { - "close": 22, - "high": 16, - "low": 60000, - "open": 59840, - "volume": 326303 - }, - "open": "2020-03-13T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59210, - "high": 25, - "low": 29, - "open": 15448, - "volume": 1413864 - }, - "id": 2559578, - "non_hive": { - "close": 11605, - "high": 5, - "low": 5, - "open": 2935, - "volume": 275237 - }, - "open": "2020-03-13T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 452, - "high": 452, - "low": 207, - "open": 207, - "volume": 2214538 - }, - "id": 2559639, - "non_hive": { - "close": 95, - "high": 95, - "low": 40, - "open": 40, - "volume": 450266 - }, - "open": "2020-03-13T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5000, - "high": 4768, - "low": 18045, - "open": 24911, - "volume": 610462 - }, - "id": 2559700, - "non_hive": { - "close": 1035, - "high": 1000, - "low": 3624, - "open": 5004, - "volume": 123786 - }, - "open": "2020-03-13T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3142, - "high": 48, - "low": 1741, - "open": 5277, - "volume": 6496657 - }, - "id": 2559741, - "non_hive": { - "close": 647, - "high": 10, - "low": 326, - "open": 1060, - "volume": 1315045 - }, - "open": "2020-03-13T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3756, - "high": 3756, - "low": 400000, - "open": 49, - "volume": 5146085 - }, - "id": 2559823, - "non_hive": { - "close": 777, - "high": 777, - "low": 78320, - "open": 10, - "volume": 1014566 - }, - "open": "2020-03-13T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 80926, - "high": 33, - "low": 1500, - "open": 245047, - "volume": 3348928 - }, - "id": 2559875, - "non_hive": { - "close": 16670, - "high": 7, - "low": 300, - "open": 49499, - "volume": 688295 - }, - "open": "2020-03-13T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28356, - "high": 19, - "low": 10, - "open": 218, - "volume": 4399012 - }, - "id": 2559929, - "non_hive": { - "close": 5896, - "high": 4, - "low": 2, - "open": 45, - "volume": 905925 - }, - "open": "2020-03-13T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1046, - "high": 52, - "low": 1601, - "open": 842, - "volume": 2143995 - }, - "id": 2559986, - "non_hive": { - "close": 215, - "high": 11, - "low": 320, - "open": 175, - "volume": 433480 - }, - "open": "2020-03-13T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 300, - "high": 68, - "low": 337, - "open": 299271, - "volume": 837452 - }, - "id": 2560041, - "non_hive": { - "close": 60, - "high": 14, - "low": 67, - "open": 60000, - "volume": 169293 - }, - "open": "2020-03-13T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 298698, - "high": 518, - "low": 33, - "open": 3566, - "volume": 2379101 - }, - "id": 2560104, - "non_hive": { - "close": 59845, - "high": 106, - "low": 6, - "open": 724, - "volume": 480445 - }, - "open": "2020-03-13T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3092, - "high": 3092, - "low": 20163, - "open": 17621, - "volume": 6962862 - }, - "id": 2560165, - "non_hive": { - "close": 644, - "high": 644, - "low": 3952, - "open": 3594, - "volume": 1427469 - }, - "open": "2020-03-13T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 73255, - "high": 3756, - "low": 26, - "open": 2732, - "volume": 1849783 - }, - "id": 2560245, - "non_hive": { - "close": 14683, - "high": 785, - "low": 5, - "open": 569, - "volume": 373576 - }, - "open": "2020-03-13T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 121560, - "high": 355, - "low": 14, - "open": 160336, - "volume": 6865228 - }, - "id": 2560322, - "non_hive": { - "close": 24171, - "high": 72, - "low": 2, - "open": 32137, - "volume": 1359561 - }, - "open": "2020-03-13T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10372, - "high": 5, - "low": 10, - "open": 30012, - "volume": 5032681 - }, - "id": 2560381, - "non_hive": { - "close": 2061, - "high": 1, - "low": 1, - "open": 6000, - "volume": 996405 - }, - "open": "2020-03-13T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 158579, - "high": 158579, - "low": 6, - "open": 100661, - "volume": 1838955 - }, - "id": 2560455, - "non_hive": { - "close": 31596, - "high": 31596, - "low": 1, - "open": 20000, - "volume": 364778 - }, - "open": "2020-03-13T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1921, - "high": 36506, - "low": 5465, - "open": 452, - "volume": 12979264 - }, - "id": 2560521, - "non_hive": { - "close": 396, - "high": 7604, - "low": 1074, - "open": 90, - "volume": 2618205 - }, - "open": "2020-03-13T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 102809, - "high": 26905, - "low": 36, - "open": 2464, - "volume": 1770107 - }, - "id": 2560605, - "non_hive": { - "close": 20843, - "high": 5590, - "low": 7, - "open": 508, - "volume": 360320 - }, - "open": "2020-03-13T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 400000, - "high": 39, - "low": 45, - "open": 400000, - "volume": 3670205 - }, - "id": 2560657, - "non_hive": { - "close": 80724, - "high": 8, - "low": 9, - "open": 80994, - "volume": 742169 - }, - "open": "2020-03-13T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30295, - "high": 1089, - "low": 15, - "open": 2011, - "volume": 2977105 - }, - "id": 2560709, - "non_hive": { - "close": 6116, - "high": 220, - "low": 3, - "open": 406, - "volume": 599763 - }, - "open": "2020-03-14T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12099, - "high": 1386, - "low": 25000, - "open": 400000, - "volume": 1377612 - }, - "id": 2560760, - "non_hive": { - "close": 2438, - "high": 280, - "low": 5000, - "open": 80085, - "volume": 275827 - }, - "open": "2020-03-14T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1984, - "high": 543, - "low": 3773, - "open": 182479, - "volume": 11452258 - }, - "id": 2560793, - "non_hive": { - "close": 400, - "high": 110, - "low": 754, - "open": 36769, - "volume": 2310177 - }, - "open": "2020-03-14T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50637, - "high": 9223, - "low": 14, - "open": 535, - "volume": 16690206 - }, - "id": 2560840, - "non_hive": { - "close": 9370, - "high": 1876, - "low": 2, - "open": 108, - "volume": 3247017 - }, - "open": "2020-03-14T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1086, - "high": 135, - "low": 49744, - "open": 4533, - "volume": 771465 - }, - "id": 2560952, - "non_hive": { - "close": 215, - "high": 27, - "low": 9210, - "open": 861, - "volume": 149331 - }, - "open": "2020-03-14T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24760, - "high": 619, - "low": 550, - "open": 10151, - "volume": 12625423 - }, - "id": 2560994, - "non_hive": { - "close": 4600, - "high": 120, - "low": 99, - "open": 1956, - "volume": 2286097 - }, - "open": "2020-03-14T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1986, - "high": 2066, - "low": 3178, - "open": 962, - "volume": 2674318 - }, - "id": 2561039, - "non_hive": { - "close": 387, - "high": 405, - "low": 572, - "open": 178, - "volume": 496746 - }, - "open": "2020-03-14T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4295, - "high": 51, - "low": 4295, - "open": 13646, - "volume": 2678327 - }, - "id": 2561100, - "non_hive": { - "close": 799, - "high": 10, - "low": 799, - "open": 2657, - "volume": 508474 - }, - "open": "2020-03-14T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 800000, - "high": 651, - "low": 165, - "open": 651, - "volume": 6509214 - }, - "id": 2561142, - "non_hive": { - "close": 145608, - "high": 127, - "low": 30, - "open": 127, - "volume": 1200619 - }, - "open": "2020-03-14T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53494, - "high": 5, - "low": 789, - "open": 3326, - "volume": 5933740 - }, - "id": 2561184, - "non_hive": { - "close": 9764, - "high": 1, - "low": 143, - "open": 627, - "volume": 1093241 - }, - "open": "2020-03-14T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 306036, - "high": 26, - "low": 800000, - "open": 800000, - "volume": 15801671 - }, - "id": 2561273, - "non_hive": { - "close": 51415, - "high": 5, - "low": 128159, - "open": 146024, - "volume": 2845982 - }, - "open": "2020-03-14T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 800000, - "high": 20, - "low": 50, - "open": 800000, - "volume": 7260926 - }, - "id": 2561335, - "non_hive": { - "close": 146400, - "high": 4, - "low": 8, - "open": 143279, - "volume": 1342043 - }, - "open": "2020-03-14T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 85899, - "high": 79, - "low": 5710, - "open": 800000, - "volume": 8530757 - }, - "id": 2561409, - "non_hive": { - "close": 16203, - "high": 15, - "low": 1034, - "open": 144960, - "volume": 1579809 - }, - "open": "2020-03-14T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13605, - "high": 20, - "low": 11, - "open": 1668, - "volume": 12936829 - }, - "id": 2561469, - "non_hive": { - "close": 2624, - "high": 4, - "low": 2, - "open": 314, - "volume": 2452686 - }, - "open": "2020-03-14T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4273, - "high": 15, - "low": 8, - "open": 323352, - "volume": 2215103 - }, - "id": 2561542, - "non_hive": { - "close": 821, - "high": 3, - "low": 1, - "open": 60000, - "volume": 417581 - }, - "open": "2020-03-14T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 112, - "high": 10, - "low": 500000, - "open": 2899, - "volume": 3751216 - }, - "id": 2561609, - "non_hive": { - "close": 22, - "high": 2, - "low": 94678, - "open": 557, - "volume": 718200 - }, - "open": "2020-03-14T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 260760, - "high": 45, - "low": 260760, - "open": 52831, - "volume": 8411533 - }, - "id": 2561668, - "non_hive": { - "close": 49846, - "high": 9, - "low": 49846, - "open": 10103, - "volume": 1614863 - }, - "open": "2020-03-14T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 79257, - "high": 10, - "low": 489, - "open": 10, - "volume": 3855886 - }, - "id": 2561715, - "non_hive": { - "close": 14369, - "high": 2, - "low": 88, - "open": 2, - "volume": 720939 - }, - "open": "2020-03-14T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43, - "high": 1508, - "low": 13, - "open": 11653, - "volume": 3750088 - }, - "id": 2561774, - "non_hive": { - "close": 8, - "high": 286, - "low": 2, - "open": 2210, - "volume": 686197 - }, - "open": "2020-03-14T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 96365, - "high": 21, - "low": 1000, - "open": 87442, - "volume": 5806899 - }, - "id": 2561834, - "non_hive": { - "close": 17924, - "high": 4, - "low": 181, - "open": 15897, - "volume": 1063993 - }, - "open": "2020-03-14T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 5, - "low": 400000, - "open": 400000, - "volume": 4388151 - }, - "id": 2561898, - "non_hive": { - "close": 1, - "high": 1, - "low": 72960, - "open": 72960, - "volume": 821570 - }, - "open": "2020-03-14T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 5, - "low": 22, - "open": 500000, - "volume": 3990912 - }, - "id": 2561958, - "non_hive": { - "close": 1, - "high": 1, - "low": 4, - "open": 92550, - "volume": 737985 - }, - "open": "2020-03-14T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30000, - "high": 5, - "low": 52, - "open": 40880, - "volume": 3509050 - }, - "id": 2562019, - "non_hive": { - "close": 5520, - "high": 1, - "low": 9, - "open": 7751, - "volume": 645044 - }, - "open": "2020-03-14T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 5, - "low": 44, - "open": 18740, - "volume": 2023815 - }, - "id": 2562077, - "non_hive": { - "close": 1, - "high": 1, - "low": 8, - "open": 3448, - "volume": 376643 - }, - "open": "2020-03-14T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 328159, - "high": 7204, - "low": 25, - "open": 20342, - "volume": 4464532 - }, - "id": 2562131, - "non_hive": { - "close": 60000, - "high": 1366, - "low": 4, - "open": 3719, - "volume": 829309 - }, - "open": "2020-03-15T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9037, - "high": 5, - "low": 100, - "open": 97228, - "volume": 1839809 - }, - "id": 2562191, - "non_hive": { - "close": 1690, - "high": 1, - "low": 18, - "open": 17777, - "volume": 337029 - }, - "open": "2020-03-15T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2246, - "high": 5, - "low": 68, - "open": 149, - "volume": 1768229 - }, - "id": 2562233, - "non_hive": { - "close": 420, - "high": 1, - "low": 12, - "open": 28, - "volume": 327778 - }, - "open": "2020-03-15T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36716, - "high": 5, - "low": 100266, - "open": 2658, - "volume": 3920559 - }, - "id": 2562288, - "non_hive": { - "close": 6794, - "high": 1, - "low": 18354, - "open": 497, - "volume": 726890 - }, - "open": "2020-03-15T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 146823, - "high": 69, - "low": 10000, - "open": 256081, - "volume": 1813526 - }, - "id": 2562342, - "non_hive": { - "close": 27179, - "high": 13, - "low": 1851, - "open": 47433, - "volume": 336475 - }, - "open": "2020-03-15T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 158753, - "low": 70805, - "open": 70805, - "volume": 2962560 - }, - "id": 2562379, - "non_hive": { - "close": 111090, - "high": 29591, - "low": 13106, - "open": 13106, - "volume": 549931 - }, - "open": "2020-03-15T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4946, - "high": 118, - "low": 11, - "open": 17933, - "volume": 2731736 - }, - "id": 2562421, - "non_hive": { - "close": 919, - "high": 22, - "low": 2, - "open": 3332, - "volume": 506257 - }, - "open": "2020-03-15T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16, - "high": 5, - "low": 134, - "open": 21, - "volume": 3511413 - }, - "id": 2562480, - "non_hive": { - "close": 3, - "high": 1, - "low": 24, - "open": 4, - "volume": 647512 - }, - "open": "2020-03-15T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2779, - "high": 5, - "low": 1000, - "open": 19477, - "volume": 2829930 - }, - "id": 2562524, - "non_hive": { - "close": 508, - "high": 1, - "low": 182, - "open": 3606, - "volume": 518504 - }, - "open": "2020-03-15T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14932, - "high": 21, - "low": 75, - "open": 21, - "volume": 17148363 - }, - "id": 2562575, - "non_hive": { - "close": 2745, - "high": 4, - "low": 13, - "open": 4, - "volume": 3133786 - }, - "open": "2020-03-15T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 800000, - "high": 10, - "low": 1182, - "open": 81944, - "volume": 2970530 - }, - "id": 2562632, - "non_hive": { - "close": 144560, - "high": 2, - "low": 212, - "open": 14821, - "volume": 542047 - }, - "open": "2020-03-15T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12059, - "high": 95374, - "low": 1255, - "open": 331079, - "volume": 1396976 - }, - "id": 2562679, - "non_hive": { - "close": 2211, - "high": 17528, - "low": 227, - "open": 60000, - "volume": 254480 - }, - "open": "2020-03-15T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2621, - "high": 43, - "low": 330193, - "open": 43, - "volume": 10740476 - }, - "id": 2562718, - "non_hive": { - "close": 485, - "high": 8, - "low": 59806, - "open": 8, - "volume": 1972642 - }, - "open": "2020-03-15T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24482, - "high": 16, - "low": 303, - "open": 64942, - "volume": 526139 - }, - "id": 2562780, - "non_hive": { - "close": 4529, - "high": 3, - "low": 55, - "open": 11901, - "volume": 96863 - }, - "open": "2020-03-15T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 102, - "high": 5, - "low": 25, - "open": 3923, - "volume": 3784318 - }, - "id": 2562830, - "non_hive": { - "close": 19, - "high": 1, - "low": 4, - "open": 719, - "volume": 698836 - }, - "open": "2020-03-15T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 526, - "high": 5, - "low": 526, - "open": 573, - "volume": 11428528 - }, - "id": 2562885, - "non_hive": { - "close": 96, - "high": 1, - "low": 96, - "open": 106, - "volume": 2134818 - }, - "open": "2020-03-15T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 315, - "high": 10, - "low": 15, - "open": 11158, - "volume": 12806355 - }, - "id": 2562949, - "non_hive": { - "close": 57, - "high": 2, - "low": 2, - "open": 2046, - "volume": 2355367 - }, - "open": "2020-03-15T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2800000, - "high": 413104, - "low": 72, - "open": 800000, - "volume": 11746666 - }, - "id": 2563013, - "non_hive": { - "close": 520800, - "high": 77173, - "low": 13, - "open": 145840, - "volume": 2165709 - }, - "open": "2020-03-15T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23076, - "high": 21, - "low": 68, - "open": 325181, - "volume": 20969869 - }, - "id": 2563069, - "non_hive": { - "close": 4296, - "high": 4, - "low": 12, - "open": 60000, - "volume": 3854666 - }, - "open": "2020-03-15T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 94878, - "high": 54087, - "low": 11, - "open": 480, - "volume": 4999979 - }, - "id": 2563136, - "non_hive": { - "close": 17277, - "high": 9996, - "low": 2, - "open": 88, - "volume": 912993 - }, - "open": "2020-03-15T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38148, - "high": 173317, - "low": 10, - "open": 85103, - "volume": 14623921 - }, - "id": 2563178, - "non_hive": { - "close": 6943, - "high": 32416, - "low": 1, - "open": 15568, - "volume": 2715792 - }, - "open": "2020-03-15T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35191, - "high": 76, - "low": 26, - "open": 33161, - "volume": 2288357 - }, - "id": 2563230, - "non_hive": { - "close": 6408, - "high": 14, - "low": 4, - "open": 6035, - "volume": 417476 - }, - "open": "2020-03-15T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33401, - "high": 359, - "low": 734, - "open": 359, - "volume": 2394395 - }, - "id": 2563274, - "non_hive": { - "close": 6127, - "high": 66, - "low": 133, - "open": 66, - "volume": 437983 - }, - "open": "2020-03-15T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22050, - "high": 22050, - "low": 1872, - "open": 55191, - "volume": 2591161 - }, - "id": 2563313, - "non_hive": { - "close": 4049, - "high": 4049, - "low": 340, - "open": 10050, - "volume": 473933 - }, - "open": "2020-03-15T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 974, - "high": 40035, - "low": 1743, - "open": 1743, - "volume": 4286914 - }, - "id": 2563354, - "non_hive": { - "close": 182, - "high": 7488, - "low": 317, - "open": 317, - "volume": 796758 - }, - "open": "2020-03-16T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 189497, - "high": 5, - "low": 8, - "open": 461, - "volume": 2209253 - }, - "id": 2563400, - "non_hive": { - "close": 34604, - "high": 1, - "low": 1, - "open": 86, - "volume": 405408 - }, - "open": "2020-03-16T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40243, - "high": 125, - "low": 611, - "open": 125, - "volume": 826984 - }, - "id": 2563439, - "non_hive": { - "close": 7330, - "high": 23, - "low": 111, - "open": 23, - "volume": 150677 - }, - "open": "2020-03-16T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13423, - "high": 113090, - "low": 233, - "open": 405523, - "volume": 2009446 - }, - "id": 2563469, - "non_hive": { - "close": 2458, - "high": 20749, - "low": 42, - "open": 73868, - "volume": 366930 - }, - "open": "2020-03-16T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 140, - "high": 91, - "low": 25, - "open": 26015, - "volume": 10011050 - }, - "id": 2563502, - "non_hive": { - "close": 26, - "high": 17, - "low": 4, - "open": 4751, - "volume": 1847883 - }, - "open": "2020-03-16T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 75390, - "high": 2925, - "low": 714, - "open": 7656, - "volume": 8562892 - }, - "id": 2563560, - "non_hive": { - "close": 13707, - "high": 541, - "low": 129, - "open": 1399, - "volume": 1558882 - }, - "open": "2020-03-16T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 339, - "high": 199517, - "low": 74, - "open": 5473, - "volume": 7820005 - }, - "id": 2563587, - "non_hive": { - "close": 62, - "high": 36911, - "low": 13, - "open": 1000, - "volume": 1439925 - }, - "open": "2020-03-16T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 207, - "high": 30526, - "low": 1552, - "open": 640, - "volume": 8667111 - }, - "id": 2563634, - "non_hive": { - "close": 38, - "high": 5641, - "low": 282, - "open": 118, - "volume": 1579021 - }, - "open": "2020-03-16T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 146628, - "high": 448, - "low": 26, - "open": 5200, - "volume": 1258476 - }, - "id": 2563677, - "non_hive": { - "close": 26635, - "high": 82, - "low": 4, - "open": 945, - "volume": 228685 - }, - "open": "2020-03-16T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5480, - "high": 54, - "low": 1000, - "open": 22655, - "volume": 498788 - }, - "id": 2563709, - "non_hive": { - "close": 1000, - "high": 10, - "low": 181, - "open": 4115, - "volume": 90682 - }, - "open": "2020-03-16T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 381, - "high": 142, - "low": 128, - "open": 49324, - "volume": 5260976 - }, - "id": 2563746, - "non_hive": { - "close": 68, - "high": 26, - "low": 22, - "open": 9000, - "volume": 941320 - }, - "open": "2020-03-16T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111, - "high": 1088, - "low": 11, - "open": 343057, - "volume": 1577985 - }, - "id": 2563795, - "non_hive": { - "close": 20, - "high": 197, - "low": 1, - "open": 61943, - "volume": 283491 - }, - "open": "2020-03-16T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1104, - "high": 36170, - "low": 1104, - "open": 11079, - "volume": 304916 - }, - "id": 2563833, - "non_hive": { - "close": 197, - "high": 6533, - "low": 197, - "open": 2000, - "volume": 54608 - }, - "open": "2020-03-16T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 22, - "low": 100, - "open": 22, - "volume": 993236 - }, - "id": 2563854, - "non_hive": { - "close": 17, - "high": 4, - "low": 17, - "open": 4, - "volume": 176658 - }, - "open": "2020-03-16T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 109221, - "high": 31118, - "low": 86403, - "open": 11019, - "volume": 964668 - }, - "id": 2563906, - "non_hive": { - "close": 19381, - "high": 5667, - "low": 15257, - "open": 1947, - "volume": 170995 - }, - "open": "2020-03-16T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299, - "high": 10316, - "low": 6, - "open": 20656, - "volume": 1079786 - }, - "id": 2563927, - "non_hive": { - "close": 53, - "high": 1878, - "low": 1, - "open": 3711, - "volume": 192978 - }, - "open": "2020-03-16T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 5, - "low": 11, - "open": 43829, - "volume": 2557062 - }, - "id": 2563970, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 7785, - "volume": 446627 - }, - "open": "2020-03-16T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74917, - "high": 5, - "low": 158974, - "open": 17375, - "volume": 6843815 - }, - "id": 2564008, - "non_hive": { - "close": 13415, - "high": 1, - "low": 27260, - "open": 3096, - "volume": 1190510 - }, - "open": "2020-03-16T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49141, - "high": 15292, - "low": 51, - "open": 3591, - "volume": 8235251 - }, - "id": 2564086, - "non_hive": { - "close": 8418, - "high": 2744, - "low": 8, - "open": 643, - "volume": 1434065 - }, - "open": "2020-03-16T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6141, - "high": 370, - "low": 120, - "open": 5003, - "volume": 965567 - }, - "id": 2564146, - "non_hive": { - "close": 1052, - "high": 65, - "low": 20, - "open": 878, - "volume": 165902 - }, - "open": "2020-03-16T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2567, - "high": 314, - "low": 89, - "open": 20462, - "volume": 493428 - }, - "id": 2564186, - "non_hive": { - "close": 439, - "high": 55, - "low": 15, - "open": 3505, - "volume": 85853 - }, - "open": "2020-03-16T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2000, - "high": 97, - "low": 18, - "open": 10254, - "volume": 915790 - }, - "id": 2564233, - "non_hive": { - "close": 342, - "high": 17, - "low": 3, - "open": 1757, - "volume": 158260 - }, - "open": "2020-03-16T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46, - "high": 750, - "low": 12, - "open": 240530, - "volume": 1982474 - }, - "id": 2564273, - "non_hive": { - "close": 8, - "high": 131, - "low": 2, - "open": 41246, - "volume": 340868 - }, - "open": "2020-03-16T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 5, - "low": 24, - "open": 126110, - "volume": 1090450 - }, - "id": 2564312, - "non_hive": { - "close": 4, - "high": 1, - "low": 4, - "open": 21596, - "volume": 186740 - }, - "open": "2020-03-16T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 247539, - "high": 5, - "low": 607, - "open": 6578, - "volume": 5450770 - }, - "id": 2564347, - "non_hive": { - "close": 42824, - "high": 1, - "low": 103, - "open": 1125, - "volume": 933469 - }, - "open": "2020-03-17T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 69, - "high": 178, - "low": 8824, - "open": 178, - "volume": 604090 - }, - "id": 2564404, - "non_hive": { - "close": 12, - "high": 31, - "low": 1500, - "open": 31, - "volume": 103533 - }, - "open": "2020-03-17T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 318770, - "high": 1561, - "low": 18, - "open": 5939, - "volume": 3385081 - }, - "id": 2564428, - "non_hive": { - "close": 56167, - "high": 278, - "low": 3, - "open": 1033, - "volume": 589829 - }, - "open": "2020-03-17T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30000, - "high": 20767, - "low": 800000, - "open": 342672, - "volume": 4813096 - }, - "id": 2564480, - "non_hive": { - "close": 5220, - "high": 3695, - "low": 136000, - "open": 60000, - "volume": 830621 - }, - "open": "2020-03-17T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 584796, - "high": 12970, - "low": 12, - "open": 800000, - "volume": 3343367 - }, - "id": 2564509, - "non_hive": { - "close": 100000, - "high": 2268, - "low": 2, - "open": 136088, - "volume": 569736 - }, - "open": "2020-03-17T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 16, - "low": 20000, - "open": 20000, - "volume": 7549495 - }, - "id": 2564536, - "non_hive": { - "close": 1735, - "high": 3, - "low": 3402, - "open": 3402, - "volume": 1333054 - }, - "open": "2020-03-17T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26, - "high": 463, - "low": 9, - "open": 40287, - "volume": 3407990 - }, - "id": 2564572, - "non_hive": { - "close": 4, - "high": 82, - "low": 1, - "open": 7010, - "volume": 591932 - }, - "open": "2020-03-17T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 800000, - "high": 20698, - "low": 200, - "open": 200, - "volume": 2265626 - }, - "id": 2564659, - "non_hive": { - "close": 138400, - "high": 3655, - "low": 34, - "open": 34, - "volume": 392278 - }, - "open": "2020-03-17T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 403870, - "high": 1298716, - "low": 25, - "open": 800000, - "volume": 17308655 - }, - "id": 2564699, - "non_hive": { - "close": 71500, - "high": 231171, - "low": 4, - "open": 138408, - "volume": 3059739 - }, - "open": "2020-03-17T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 235, - "high": 1202, - "low": 235, - "open": 6056, - "volume": 4069846 - }, - "id": 2564737, - "non_hive": { - "close": 40, - "high": 214, - "low": 40, - "open": 1078, - "volume": 713249 - }, - "open": "2020-03-17T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 438174, - "high": 42143, - "low": 12, - "open": 170054, - "volume": 848953 - }, - "id": 2564776, - "non_hive": { - "close": 76135, - "high": 7488, - "low": 2, - "open": 29514, - "volume": 147634 - }, - "open": "2020-03-17T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29507, - "high": 6915, - "low": 396, - "open": 550000, - "volume": 1179325 - }, - "id": 2564805, - "non_hive": { - "close": 5129, - "high": 1223, - "low": 68, - "open": 95676, - "volume": 205343 - }, - "open": "2020-03-17T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 765830, - "high": 194694, - "low": 127, - "open": 37733, - "volume": 2909609 - }, - "id": 2564830, - "non_hive": { - "close": 133637, - "high": 34461, - "low": 22, - "open": 6650, - "volume": 510143 - }, - "open": "2020-03-17T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 11, - "low": 112, - "open": 113, - "volume": 1857686 - }, - "id": 2564875, - "non_hive": { - "close": 2, - "high": 2, - "low": 19, - "open": 20, - "volume": 323753 - }, - "open": "2020-03-17T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 181, - "low": 60, - "open": 339467, - "volume": 2484832 - }, - "id": 2564919, - "non_hive": { - "close": 1760, - "high": 32, - "low": 10, - "open": 59712, - "volume": 435544 - }, - "open": "2020-03-17T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14263, - "high": 42483, - "low": 78, - "open": 10261, - "volume": 451688 - }, - "id": 2564961, - "non_hive": { - "close": 2488, - "high": 7488, - "low": 13, - "open": 1792, - "volume": 78791 - }, - "open": "2020-03-17T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39, - "high": 804634, - "low": 40, - "open": 21708, - "volume": 11495260 - }, - "id": 2564990, - "non_hive": { - "close": 6, - "high": 142903, - "low": 6, - "open": 3783, - "volume": 2029542 - }, - "open": "2020-03-17T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 405, - "high": 71884, - "low": 270, - "open": 79560, - "volume": 5191045 - }, - "id": 2565080, - "non_hive": { - "close": 70, - "high": 12753, - "low": 46, - "open": 13969, - "volume": 906109 - }, - "open": "2020-03-17T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21387, - "high": 5, - "low": 114, - "open": 342383, - "volume": 13733856 - }, - "id": 2565132, - "non_hive": { - "close": 3783, - "high": 1, - "low": 19, - "open": 60401, - "volume": 2418229 - }, - "open": "2020-03-17T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7989, - "high": 11, - "low": 123, - "open": 800000, - "volume": 5531969 - }, - "id": 2565174, - "non_hive": { - "close": 1390, - "high": 2, - "low": 21, - "open": 139084, - "volume": 964618 - }, - "open": "2020-03-17T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 700968, - "high": 405, - "low": 18, - "open": 343416, - "volume": 5609137 - }, - "id": 2565228, - "non_hive": { - "close": 122691, - "high": 72, - "low": 3, - "open": 60000, - "volume": 980526 - }, - "open": "2020-03-17T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 235210, - "low": 72, - "open": 15539, - "volume": 5993884 - }, - "id": 2565279, - "non_hive": { - "close": 175, - "high": 42479, - "low": 12, - "open": 2735, - "volume": 1050699 - }, - "open": "2020-03-17T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59317, - "high": 5575, - "low": 6, - "open": 341312, - "volume": 1917126 - }, - "id": 2565324, - "non_hive": { - "close": 10319, - "high": 986, - "low": 1, - "open": 60000, - "volume": 336500 - }, - "open": "2020-03-17T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 154, - "high": 154, - "low": 41, - "open": 27272, - "volume": 673634 - }, - "id": 2565359, - "non_hive": { - "close": 27, - "high": 27, - "low": 7, - "open": 4742, - "volume": 117137 - }, - "open": "2020-03-17T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 919, - "high": 39922, - "low": 5151, - "open": 190, - "volume": 7243766 - }, - "id": 2565389, - "non_hive": { - "close": 160, - "high": 7210, - "low": 891, - "open": 33, - "volume": 1278908 - }, - "open": "2020-03-18T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32887, - "high": 32439, - "low": 130, - "open": 800000, - "volume": 4457714 - }, - "id": 2565443, - "non_hive": { - "close": 5696, - "high": 5696, - "low": 22, - "open": 138480, - "volume": 772600 - }, - "open": "2020-03-18T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 483208, - "high": 3523, - "low": 12, - "open": 115883, - "volume": 4161167 - }, - "id": 2565478, - "non_hive": { - "close": 83595, - "high": 615, - "low": 2, - "open": 20224, - "volume": 721476 - }, - "open": "2020-03-18T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 62, - "high": 62, - "low": 800000, - "open": 800000, - "volume": 5705915 - }, - "id": 2565515, - "non_hive": { - "close": 11, - "high": 11, - "low": 138376, - "open": 138376, - "volume": 992164 - }, - "open": "2020-03-18T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 491811, - "high": 905470, - "low": 122, - "open": 58304, - "volume": 5776441 - }, - "id": 2565549, - "non_hive": { - "close": 88821, - "high": 163528, - "low": 21, - "open": 10288, - "volume": 1027654 - }, - "open": "2020-03-18T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19529, - "high": 1658558, - "low": 308, - "open": 45, - "volume": 4861387 - }, - "id": 2565593, - "non_hive": { - "close": 3452, - "high": 300000, - "low": 54, - "open": 8, - "volume": 871729 - }, - "open": "2020-03-18T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40, - "high": 5, - "low": 11, - "open": 112330, - "volume": 7294688 - }, - "id": 2565635, - "non_hive": { - "close": 7, - "high": 1, - "low": 1, - "open": 19855, - "volume": 1281426 - }, - "open": "2020-03-18T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19840, - "high": 13149, - "low": 157, - "open": 800000, - "volume": 2637822 - }, - "id": 2565685, - "non_hive": { - "close": 3492, - "high": 2331, - "low": 27, - "open": 139333, - "volume": 459581 - }, - "open": "2020-03-18T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10044, - "high": 22, - "low": 12, - "open": 25585, - "volume": 897088 - }, - "id": 2565718, - "non_hive": { - "close": 1749, - "high": 4, - "low": 2, - "open": 4479, - "volume": 156799 - }, - "open": "2020-03-18T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20107, - "high": 74, - "low": 242, - "open": 32891, - "volume": 1005427 - }, - "id": 2565754, - "non_hive": { - "close": 3502, - "high": 13, - "low": 42, - "open": 5727, - "volume": 175220 - }, - "open": "2020-03-18T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74, - "high": 11, - "low": 74, - "open": 344593, - "volume": 1732780 - }, - "id": 2565788, - "non_hive": { - "close": 12, - "high": 2, - "low": 12, - "open": 60000, - "volume": 302328 - }, - "open": "2020-03-18T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2364, - "high": 2364, - "low": 1110, - "open": 27269, - "volume": 3690009 - }, - "id": 2565825, - "non_hive": { - "close": 415, - "high": 415, - "low": 193, - "open": 4749, - "volume": 644688 - }, - "open": "2020-03-18T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2808, - "high": 516, - "low": 15, - "open": 444, - "volume": 5576943 - }, - "id": 2565858, - "non_hive": { - "close": 500, - "high": 92, - "low": 2, - "open": 78, - "volume": 983652 - }, - "open": "2020-03-18T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17494, - "high": 268621, - "low": 200, - "open": 27178, - "volume": 44356871 - }, - "id": 2565904, - "non_hive": { - "close": 3963, - "high": 61509, - "low": 35, - "open": 4804, - "volume": 9367465 - }, - "open": "2020-03-18T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36323, - "high": 28, - "low": 79646, - "open": 450, - "volume": 29730066 - }, - "id": 2566018, - "non_hive": { - "close": 8673, - "high": 7, - "low": 16725, - "open": 102, - "volume": 6990271 - }, - "open": "2020-03-18T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 271479, - "high": 2917040, - "low": 9, - "open": 1257862, - "volume": 19926189 - }, - "id": 2566144, - "non_hive": { - "close": 62593, - "high": 714675, - "low": 2, - "open": 300000, - "volume": 4747238 - }, - "open": "2020-03-18T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 240, - "high": 2876, - "low": 100, - "open": 80203, - "volume": 6009755 - }, - "id": 2566239, - "non_hive": { - "close": 56, - "high": 674, - "low": 21, - "open": 18581, - "volume": 1332593 - }, - "open": "2020-03-18T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8613, - "high": 157, - "low": 90, - "open": 400000, - "volume": 9247425 - }, - "id": 2566292, - "non_hive": { - "close": 1895, - "high": 37, - "low": 19, - "open": 86440, - "volume": 2101169 - }, - "open": "2020-03-18T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 275206, - "high": 4000000, - "low": 45572, - "open": 275108, - "volume": 16889114 - }, - "id": 2566351, - "non_hive": { - "close": 63297, - "high": 939600, - "low": 8878, - "open": 60000, - "volume": 3767234 - }, - "open": "2020-03-18T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 316, - "high": 94392, - "low": 432, - "open": 44259, - "volume": 26996235 - }, - "id": 2566426, - "non_hive": { - "close": 68, - "high": 23314, - "low": 92, - "open": 10223, - "volume": 6349400 - }, - "open": "2020-03-18T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7072, - "high": 2463679, - "low": 10, - "open": 725000, - "volume": 10084281 - }, - "id": 2566516, - "non_hive": { - "close": 1727, - "high": 603503, - "low": 2, - "open": 156963, - "volume": 2365311 - }, - "open": "2020-03-18T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1028, - "high": 578, - "low": 116, - "open": 1250000, - "volume": 24104953 - }, - "id": 2566571, - "non_hive": { - "close": 261, - "high": 147, - "low": 25, - "open": 306203, - "volume": 5908246 - }, - "open": "2020-03-18T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40821, - "high": 3, - "low": 14, - "open": 18092, - "volume": 5933760 - }, - "id": 2566646, - "non_hive": { - "close": 9718, - "high": 1, - "low": 3, - "open": 4595, - "volume": 1418314 - }, - "open": "2020-03-18T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 83780, - "high": 19, - "low": 1000, - "open": 18497, - "volume": 21757969 - }, - "id": 2566707, - "non_hive": { - "close": 21866, - "high": 5, - "low": 238, - "open": 4640, - "volume": 5534294 - }, - "open": "2020-03-18T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5000, - "high": 3, - "low": 4, - "open": 4799, - "volume": 25006184 - }, - "id": 2566797, - "non_hive": { - "close": 1368, - "high": 1, - "low": 1, - "open": 1238, - "volume": 6781550 - }, - "open": "2020-03-19T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 207845, - "high": 3, - "low": 207845, - "open": 3, - "volume": 6484235 - }, - "id": 2566878, - "non_hive": { - "close": 47243, - "high": 1, - "low": 47243, - "open": 1, - "volume": 1649497 - }, - "open": "2020-03-19T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100000, - "high": 3, - "low": 202199, - "open": 37001, - "volume": 3678178 - }, - "id": 2566961, - "non_hive": { - "close": 23500, - "high": 1, - "low": 44119, - "open": 10277, - "volume": 851537 - }, - "open": "2020-03-19T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28573, - "high": 28573, - "low": 6689, - "open": 8000, - "volume": 5946289 - }, - "id": 2567064, - "non_hive": { - "close": 7911, - "high": 7911, - "low": 1539, - "open": 1863, - "volume": 1522381 - }, - "open": "2020-03-19T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 108186, - "high": 3, - "low": 550, - "open": 4000, - "volume": 2881369 - }, - "id": 2567157, - "non_hive": { - "close": 29589, - "high": 1, - "low": 132, - "open": 1004, - "volume": 750004 - }, - "open": "2020-03-19T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 544, - "high": 56253, - "low": 205561, - "open": 7146, - "volume": 7234769 - }, - "id": 2567233, - "non_hive": { - "close": 152, - "high": 16795, - "low": 51821, - "open": 1936, - "volume": 1978508 - }, - "open": "2020-03-19T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1324, - "high": 3, - "low": 227756, - "open": 6761, - "volume": 3195270 - }, - "id": 2567315, - "non_hive": { - "close": 415, - "high": 1, - "low": 60000, - "open": 1900, - "volume": 938553 - }, - "open": "2020-03-19T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 751753, - "high": 31, - "low": 23, - "open": 8948, - "volume": 5055536 - }, - "id": 2567394, - "non_hive": { - "close": 235775, - "high": 10, - "low": 6, - "open": 2752, - "volume": 1519229 - }, - "open": "2020-03-19T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35360, - "high": 3628, - "low": 224509, - "open": 67, - "volume": 5709964 - }, - "id": 2567475, - "non_hive": { - "close": 10616, - "high": 1270, - "low": 60000, - "open": 21, - "volume": 1836326 - }, - "open": "2020-03-19T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20530, - "high": 2, - "low": 380000, - "open": 1430, - "volume": 2326633 - }, - "id": 2567563, - "non_hive": { - "close": 6970, - "high": 1, - "low": 114380, - "open": 500, - "volume": 734631 - }, - "open": "2020-03-19T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 169026, - "high": 38, - "low": 10, - "open": 212700, - "volume": 1833643 - }, - "id": 2567619, - "non_hive": { - "close": 51432, - "high": 13, - "low": 3, - "open": 65512, - "volume": 567372 - }, - "open": "2020-03-19T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7026, - "high": 1546, - "low": 9998, - "open": 73161, - "volume": 1667991 - }, - "id": 2567670, - "non_hive": { - "close": 2317, - "high": 521, - "low": 2808, - "open": 22490, - "volume": 503124 - }, - "open": "2020-03-19T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1353, - "high": 4859, - "low": 5650, - "open": 8243, - "volume": 1044474 - }, - "id": 2567743, - "non_hive": { - "close": 437, - "high": 1586, - "low": 1586, - "open": 2317, - "volume": 310253 - }, - "open": "2020-03-19T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 177897, - "high": 5004, - "low": 526, - "open": 99716, - "volume": 3396059 - }, - "id": 2567793, - "non_hive": { - "close": 48848, - "high": 1575, - "low": 139, - "open": 29915, - "volume": 962257 - }, - "open": "2020-03-19T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 398, - "high": 87, - "low": 13, - "open": 10437, - "volume": 898161 - }, - "id": 2567869, - "non_hive": { - "close": 108, - "high": 24, - "low": 3, - "open": 2866, - "volume": 236589 - }, - "open": "2020-03-19T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 279, - "high": 7, - "low": 800000, - "open": 3660, - "volume": 5575440 - }, - "id": 2567931, - "non_hive": { - "close": 69, - "high": 2, - "low": 197840, - "open": 912, - "volume": 1412232 - }, - "open": "2020-03-19T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 61548, - "high": 61548, - "low": 247349, - "open": 93635, - "volume": 2916105 - }, - "id": 2568012, - "non_hive": { - "close": 16291, - "high": 16291, - "low": 60000, - "open": 23465, - "volume": 740406 - }, - "open": "2020-03-19T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3542, - "high": 19159, - "low": 10, - "open": 61657, - "volume": 3103726 - }, - "id": 2568080, - "non_hive": { - "close": 946, - "high": 6131, - "low": 2, - "open": 15291, - "volume": 865736 - }, - "open": "2020-03-19T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 209650, - "high": 3, - "low": 708, - "open": 3624, - "volume": 2068544 - }, - "id": 2568152, - "non_hive": { - "close": 55015, - "high": 1, - "low": 181, - "open": 968, - "volume": 532638 - }, - "open": "2020-03-19T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 227634, - "high": 3, - "low": 429, - "open": 91896, - "volume": 1155155 - }, - "id": 2568191, - "non_hive": { - "close": 58408, - "high": 1, - "low": 110, - "open": 24439, - "volume": 305815 - }, - "open": "2020-03-19T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 3, - "low": 12, - "open": 28, - "volume": 4288592 - }, - "id": 2568243, - "non_hive": { - "close": 1, - "high": 1, - "low": 3, - "open": 8, - "volume": 1207274 - }, - "open": "2020-03-19T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 3, - "low": 1806, - "open": 3, - "volume": 625324 - }, - "id": 2568316, - "non_hive": { - "close": 4, - "high": 1, - "low": 477, - "open": 1, - "volume": 171687 - }, - "open": "2020-03-19T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11388, - "high": 3, - "low": 8, - "open": 18, - "volume": 2289107 - }, - "id": 2568386, - "non_hive": { - "close": 3141, - "high": 1, - "low": 2, - "open": 5, - "volume": 610672 - }, - "open": "2020-03-19T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9081, - "high": 6, - "low": 27, - "open": 366461, - "volume": 1163223 - }, - "id": 2568459, - "non_hive": { - "close": 2604, - "high": 2, - "low": 7, - "open": 96836, - "volume": 311686 - }, - "open": "2020-03-19T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 87275, - "high": 3, - "low": 4, - "open": 12146, - "volume": 498622 - }, - "id": 2568505, - "non_hive": { - "close": 22553, - "high": 1, - "low": 1, - "open": 3141, - "volume": 130947 - }, - "open": "2020-03-20T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 165425, - "high": 39, - "low": 165425, - "open": 68, - "volume": 2192377 - }, - "id": 2568546, - "non_hive": { - "close": 42315, - "high": 11, - "low": 42315, - "open": 19, - "volume": 572165 - }, - "open": "2020-03-20T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 3, - "low": 131, - "open": 396, - "volume": 2673005 - }, - "id": 2568583, - "non_hive": { - "close": 3, - "high": 1, - "low": 30, - "open": 105, - "volume": 664272 - }, - "open": "2020-03-20T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 227757, - "high": 3, - "low": 570000, - "open": 94737, - "volume": 2645192 - }, - "id": 2568666, - "non_hive": { - "close": 60000, - "high": 1, - "low": 133437, - "open": 23024, - "volume": 658352 - }, - "open": "2020-03-20T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 106151, - "high": 3, - "low": 550000, - "open": 5684, - "volume": 1576266 - }, - "id": 2568725, - "non_hive": { - "close": 27587, - "high": 1, - "low": 131230, - "open": 1501, - "volume": 392525 - }, - "open": "2020-03-20T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 200000, - "high": 7, - "low": 500000, - "open": 203000, - "volume": 1708661 - }, - "id": 2568771, - "non_hive": { - "close": 52160, - "high": 2, - "low": 120500, - "open": 49532, - "volume": 436879 - }, - "open": "2020-03-20T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 175111, - "high": 3, - "low": 103, - "open": 25378, - "volume": 2404756 - }, - "id": 2568802, - "non_hive": { - "close": 49381, - "high": 1, - "low": 27, - "open": 7183, - "volume": 644201 - }, - "open": "2020-03-20T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 3, - "low": 100, - "open": 71869, - "volume": 3429468 - }, - "id": 2568866, - "non_hive": { - "close": 1, - "high": 1, - "low": 27, - "open": 20252, - "volume": 982428 - }, - "open": "2020-03-20T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4770, - "high": 3, - "low": 10, - "open": 89731, - "volume": 6674145 - }, - "id": 2568939, - "non_hive": { - "close": 1193, - "high": 1, - "low": 2, - "open": 25313, - "volume": 1776553 - }, - "open": "2020-03-20T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 154067, - "high": 169291, - "low": 800000, - "open": 47131, - "volume": 9756571 - }, - "id": 2569005, - "non_hive": { - "close": 43909, - "high": 48381, - "low": 192880, - "open": 12678, - "volume": 2612085 - }, - "open": "2020-03-20T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 37927, - "low": 100, - "open": 191, - "volume": 4184442 - }, - "id": 2569101, - "non_hive": { - "close": 24, - "high": 10923, - "low": 24, - "open": 54, - "volume": 1120034 - }, - "open": "2020-03-20T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 250000, - "high": 3, - "low": 10, - "open": 68862, - "volume": 3393700 - }, - "id": 2569148, - "non_hive": { - "close": 61150, - "high": 1, - "low": 2, - "open": 18386, - "volume": 865079 - }, - "open": "2020-03-20T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 219496, - "high": 18745, - "low": 98870, - "open": 68155, - "volume": 6342442 - }, - "id": 2569214, - "non_hive": { - "close": 61459, - "high": 5254, - "low": 24915, - "open": 18400, - "volume": 1729578 - }, - "open": "2020-03-20T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50800, - "high": 26562, - "low": 369, - "open": 354, - "volume": 2936529 - }, - "id": 2569285, - "non_hive": { - "close": 13789, - "high": 7464, - "low": 100, - "open": 99, - "volume": 804799 - }, - "open": "2020-03-20T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 338, - "high": 338, - "low": 338, - "open": 338, - "volume": 338 - }, - "id": 2569330, - "non_hive": { - "close": 95, - "high": 95, - "low": 95, - "open": 95, - "volume": 95 - }, - "open": "2020-03-20T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 3, - "low": 37, - "open": 3, - "volume": 176 - }, - "id": 2569334, - "non_hive": { - "close": 7, - "high": 1, - "low": 10, - "open": 1, - "volume": 50 - }, - "open": "2020-03-21T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 3, - "low": 8, - "open": 3, - "volume": 1021 - }, - "id": 2569352, - "non_hive": { - "close": 1, - "high": 1, - "low": 2, - "open": 1, - "volume": 277 - }, - "open": "2020-03-21T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 640, - "high": 640, - "low": 640, - "open": 640, - "volume": 640 - }, - "id": 2569367, - "non_hive": { - "close": 173, - "high": 173, - "low": 173, - "open": 173, - "volume": 173 - }, - "open": "2020-03-21T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 982, - "high": 982, - "low": 982, - "open": 982, - "volume": 982 - }, - "id": 2569371, - "non_hive": { - "close": 266, - "high": 266, - "low": 266, - "open": 266, - "volume": 266 - }, - "open": "2020-03-21T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74, - "high": 74, - "low": 1303, - "open": 1303, - "volume": 1377 - }, - "id": 2569375, - "non_hive": { - "close": 20, - "high": 20, - "low": 352, - "open": 352, - "volume": 372 - }, - "open": "2020-03-21T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1303, - "high": 1303, - "low": 1303, - "open": 1303, - "volume": 1303 - }, - "id": 2569382, - "non_hive": { - "close": 352, - "high": 352, - "low": 352, - "open": 352, - "volume": 352 - }, - "open": "2020-03-21T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 7, - "low": 7, - "open": 7, - "volume": 7 - }, - "id": 2569386, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-03-21T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46, - "high": 3, - "low": 23, - "open": 1336, - "volume": 1408 - }, - "id": 2569390, - "non_hive": { - "close": 13, - "high": 1, - "low": 6, - "open": 361, - "volume": 381 - }, - "open": "2020-03-21T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5999, - "high": 5999, - "low": 5999, - "open": 5999, - "volume": 5999 - }, - "id": 2569401, - "non_hive": { - "close": 1625, - "high": 1625, - "low": 1625, - "open": 1625, - "volume": 1625 - }, - "open": "2020-03-21T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 708, - "high": 708, - "low": 708, - "open": 708, - "volume": 708 - }, - "id": 2569405, - "non_hive": { - "close": 199, - "high": 199, - "low": 199, - "open": 199, - "volume": 199 - }, - "open": "2020-03-21T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2569409, - "non_hive": { - "close": 270, - "high": 270, - "low": 270, - "open": 270, - "volume": 270 - }, - "open": "2020-03-21T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30, - "high": 30, - "low": 30, - "open": 30, - "volume": 30 - }, - "id": 2569413, - "non_hive": { - "close": 8, - "high": 8, - "low": 8, - "open": 8, - "volume": 8 - }, - "open": "2020-03-21T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 3, - "low": 248, - "open": 248, - "volume": 251 - }, - "id": 2569417, - "non_hive": { - "close": 1, - "high": 1, - "low": 67, - "open": 67, - "volume": 68 - }, - "open": "2020-03-21T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 78, - "high": 447, - "low": 78, - "open": 447, - "volume": 525 - }, - "id": 2569424, - "non_hive": { - "close": 21, - "high": 121, - "low": 21, - "open": 121, - "volume": 142 - }, - "open": "2020-03-21T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 108, - "high": 108, - "low": 108, - "open": 108, - "volume": 108 - }, - "id": 2569431, - "non_hive": { - "close": 29, - "high": 29, - "low": 29, - "open": 29, - "volume": 29 - }, - "open": "2020-03-21T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10676, - "high": 10676, - "low": 4, - "open": 45, - "volume": 10725 - }, - "id": 2569435, - "non_hive": { - "close": 3000, - "high": 3000, - "low": 1, - "open": 12, - "volume": 3013 - }, - "open": "2020-03-22T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28037, - "high": 18560, - "low": 28037, - "open": 18560, - "volume": 154000 - }, - "id": 2569445, - "non_hive": { - "close": 7569, - "high": 5028, - "low": 7569, - "open": 5028, - "volume": 41596 - }, - "open": "2020-03-22T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49, - "high": 49, - "low": 1487, - "open": 1487, - "volume": 1536 - }, - "id": 2569449, - "non_hive": { - "close": 14, - "high": 14, - "low": 418, - "open": 418, - "volume": 432 - }, - "open": "2020-03-22T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42032, - "high": 42032, - "low": 42032, - "open": 42032, - "volume": 42032 - }, - "id": 2569455, - "non_hive": { - "close": 11348, - "high": 11348, - "low": 11348, - "open": 11348, - "volume": 11348 - }, - "open": "2020-03-22T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 101, - "high": 101, - "low": 101, - "open": 101, - "volume": 101 - }, - "id": 2569459, - "non_hive": { - "close": 27, - "high": 27, - "low": 27, - "open": 27, - "volume": 27 - }, - "open": "2020-03-22T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 12, - "low": 12, - "open": 12, - "volume": 12 - }, - "id": 2569463, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 3, - "volume": 3 - }, - "open": "2020-03-22T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30, - "high": 30, - "low": 30, - "open": 30, - "volume": 30 - }, - "id": 2569467, - "non_hive": { - "close": 8, - "high": 8, - "low": 8, - "open": 8, - "volume": 8 - }, - "open": "2020-03-22T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 319, - "high": 319, - "low": 12, - "open": 12, - "volume": 54698 - }, - "id": 2569471, - "non_hive": { - "close": 91, - "high": 91, - "low": 3, - "open": 3, - "volume": 15540 - }, - "open": "2020-03-22T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6671, - "high": 6671, - "low": 6671, - "open": 6671, - "volume": 6671 - }, - "id": 2569481, - "non_hive": { - "close": 1900, - "high": 1900, - "low": 1900, - "open": 1900, - "volume": 1900 - }, - "open": "2020-03-22T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 63, - "high": 35, - "low": 3311, - "open": 35, - "volume": 3514 - }, - "id": 2569485, - "non_hive": { - "close": 18, - "high": 10, - "low": 943, - "open": 10, - "volume": 1001 - }, - "open": "2020-03-22T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 3, - "low": 2377, - "open": 207, - "volume": 5960 - }, - "id": 2569495, - "non_hive": { - "close": 1, - "high": 1, - "low": 677, - "open": 59, - "volume": 1698 - }, - "open": "2020-03-22T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 278, - "high": 278, - "low": 15, - "open": 15, - "volume": 293 - }, - "id": 2569510, - "non_hive": { - "close": 75, - "high": 75, - "low": 4, - "open": 4, - "volume": 79 - }, - "open": "2020-03-22T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 12, - "low": 12, - "open": 12, - "volume": 12 - }, - "id": 2569517, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 3, - "volume": 3 - }, - "open": "2020-03-22T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 186, - "high": 186, - "low": 186, - "open": 186, - "volume": 186 - }, - "id": 2569521, - "non_hive": { - "close": 50, - "high": 50, - "low": 50, - "open": 50, - "volume": 50 - }, - "open": "2020-03-22T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 442, - "high": 442, - "low": 442, - "open": 442, - "volume": 442 - }, - "id": 2569525, - "non_hive": { - "close": 119, - "high": 119, - "low": 119, - "open": 119, - "volume": 119 - }, - "open": "2020-03-22T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 115, - "high": 9964, - "low": 115, - "open": 9964, - "volume": 10079 - }, - "id": 2569529, - "non_hive": { - "close": 31, - "high": 2838, - "low": 31, - "open": 2838, - "volume": 2869 - }, - "open": "2020-03-22T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 45, - "low": 4, - "open": 45, - "volume": 49 - }, - "id": 2569536, - "non_hive": { - "close": 1, - "high": 12, - "low": 1, - "open": 12, - "volume": 13 - }, - "open": "2020-03-23T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3806, - "high": 3806, - "low": 12, - "open": 12, - "volume": 4093 - }, - "id": 2569544, - "non_hive": { - "close": 1084, - "high": 1084, - "low": 3, - "open": 3, - "volume": 1161 - }, - "open": "2020-03-23T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 102, - "high": 10, - "low": 102, - "open": 10, - "volume": 112 - }, - "id": 2569554, - "non_hive": { - "close": 29, - "high": 3, - "low": 29, - "open": 3, - "volume": 32 - }, - "open": "2020-03-23T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 11, - "low": 11, - "open": 11, - "volume": 11 - }, - "id": 2569561, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 3, - "volume": 3 - }, - "open": "2020-03-23T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32, - "high": 32, - "low": 32, - "open": 32, - "volume": 32 - }, - "id": 2569565, - "non_hive": { - "close": 9, - "high": 9, - "low": 9, - "open": 9, - "volume": 9 - }, - "open": "2020-03-23T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3546, - "high": 203, - "low": 3546, - "open": 7285, - "volume": 11034 - }, - "id": 2569569, - "non_hive": { - "close": 1010, - "high": 58, - "low": 1010, - "open": 2075, - "volume": 3143 - }, - "open": "2020-03-23T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 11, - "low": 11, - "open": 11, - "volume": 11 - }, - "id": 2569576, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 3, - "volume": 3 - }, - "open": "2020-03-23T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2991, - "high": 2991, - "low": 2991, - "open": 2991, - "volume": 2991 - }, - "id": 2569580, - "non_hive": { - "close": 852, - "high": 852, - "low": 852, - "open": 852, - "volume": 852 - }, - "open": "2020-03-23T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1938, - "high": 4199, - "low": 4199, - "open": 4199, - "volume": 6137 - }, - "id": 2569584, - "non_hive": { - "close": 552, - "high": 1196, - "low": 1196, - "open": 1196, - "volume": 1748 - }, - "open": "2020-03-23T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 73, - "high": 73, - "low": 5193, - "open": 5193, - "volume": 5266 - }, - "id": 2569591, - "non_hive": { - "close": 21, - "high": 21, - "low": 1478, - "open": 1478, - "volume": 1499 - }, - "open": "2020-03-23T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 278, - "high": 116, - "low": 77, - "open": 193, - "volume": 5387 - }, - "id": 2569598, - "non_hive": { - "close": 80, - "high": 35, - "low": 22, - "open": 56, - "volume": 1606 - }, - "open": "2020-03-23T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 14, - "low": 14, - "open": 14, - "volume": 14 - }, - "id": 2569608, - "non_hive": { - "close": 4, - "high": 4, - "low": 4, - "open": 4, - "volume": 4 - }, - "open": "2020-03-23T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 434, - "high": 434, - "low": 434, - "open": 434, - "volume": 434 - }, - "id": 2569612, - "non_hive": { - "close": 125, - "high": 125, - "low": 125, - "open": 125, - "volume": 125 - }, - "open": "2020-03-23T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111, - "high": 8590, - "low": 111, - "open": 8590, - "volume": 8701 - }, - "id": 2569616, - "non_hive": { - "close": 32, - "high": 2577, - "low": 32, - "open": 2577, - "volume": 2609 - }, - "open": "2020-03-23T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4326, - "high": 4326, - "low": 4, - "open": 46, - "volume": 4376 - }, - "id": 2569623, - "non_hive": { - "close": 1298, - "high": 1298, - "low": 1, - "open": 13, - "volume": 1312 - }, - "open": "2020-03-24T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 11, - "low": 11, - "open": 11, - "volume": 11 - }, - "id": 2569633, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 3, - "volume": 3 - }, - "open": "2020-03-24T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2576, - "high": 2576, - "low": 4220, - "open": 4220, - "volume": 13349 - }, - "id": 2569637, - "non_hive": { - "close": 773, - "high": 773, - "low": 1266, - "open": 1266, - "volume": 4005 - }, - "open": "2020-03-24T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20, - "high": 456, - "low": 20, - "open": 456, - "volume": 476 - }, - "id": 2569649, - "non_hive": { - "close": 6, - "high": 137, - "low": 6, - "open": 137, - "volume": 143 - }, - "open": "2020-03-24T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9791, - "high": 10728, - "low": 9791, - "open": 10728, - "volume": 238111 - }, - "id": 2569656, - "non_hive": { - "close": 2488, - "high": 3095, - "low": 2488, - "open": 3095, - "volume": 62763 - }, - "open": "2020-03-24T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 116331, - "high": 1174, - "low": 116331, - "open": 1174, - "volume": 181226 - }, - "id": 2569661, - "non_hive": { - "close": 29547, - "high": 352, - "low": 29547, - "open": 352, - "volume": 46095 - }, - "open": "2020-03-24T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 99, - "high": 99, - "low": 99, - "open": 99, - "volume": 99 - }, - "id": 2569665, - "non_hive": { - "close": 25, - "high": 25, - "low": 25, - "open": 25, - "volume": 25 - }, - "open": "2020-03-24T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 12, - "low": 12, - "open": 12, - "volume": 12 - }, - "id": 2569669, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 3, - "volume": 3 - }, - "open": "2020-03-24T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28, - "high": 28, - "low": 28, - "open": 28, - "volume": 28 - }, - "id": 2569673, - "non_hive": { - "close": 7, - "high": 7, - "low": 7, - "open": 7, - "volume": 7 - }, - "open": "2020-03-24T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3636, - "high": 3636, - "low": 3636, - "open": 3636, - "volume": 3636 - }, - "id": 2569677, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-03-24T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 158845, - "high": 158845, - "low": 100000, - "open": 100000, - "volume": 258845 - }, - "id": 2569681, - "non_hive": { - "close": 40346, - "high": 40346, - "low": 25399, - "open": 25399, - "volume": 65745 - }, - "open": "2020-03-24T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 3937, - "low": 12, - "open": 3937, - "volume": 3949 - }, - "id": 2569687, - "non_hive": { - "close": 3, - "high": 1000, - "low": 3, - "open": 1000, - "volume": 1003 - }, - "open": "2020-03-24T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1019, - "high": 1019, - "low": 12, - "open": 12, - "volume": 1276 - }, - "id": 2569694, - "non_hive": { - "close": 259, - "high": 259, - "low": 3, - "open": 3, - "volume": 324 - }, - "open": "2020-03-24T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20177, - "high": 20177, - "low": 20177, - "open": 20177, - "volume": 20177 - }, - "id": 2569704, - "non_hive": { - "close": 5125, - "high": 5125, - "low": 5125, - "open": 5125, - "volume": 5125 - }, - "open": "2020-03-24T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3937, - "high": 3937, - "low": 12, - "open": 12, - "volume": 3949 - }, - "id": 2569708, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 3, - "open": 3, - "volume": 1003 - }, - "open": "2020-03-24T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 232, - "high": 232, - "low": 232, - "open": 232, - "volume": 232 - }, - "id": 2569715, - "non_hive": { - "close": 59, - "high": 59, - "low": 59, - "open": 59, - "volume": 59 - }, - "open": "2020-03-24T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3086, - "high": 3086, - "low": 402, - "open": 402, - "volume": 3488 - }, - "id": 2569719, - "non_hive": { - "close": 784, - "high": 784, - "low": 102, - "open": 102, - "volume": 886 - }, - "open": "2020-03-24T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 103, - "high": 83, - "low": 103, - "open": 83, - "volume": 186 - }, - "id": 2569726, - "non_hive": { - "close": 26, - "high": 21, - "low": 26, - "open": 21, - "volume": 47 - }, - "open": "2020-03-24T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 78181, - "high": 1055, - "low": 78181, - "open": 40, - "volume": 144068 - }, - "id": 2569733, - "non_hive": { - "close": 18797, - "high": 268, - "low": 18797, - "open": 10, - "volume": 35220 - }, - "open": "2020-03-25T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 109, - "high": 109, - "low": 109, - "open": 109, - "volume": 109 - }, - "id": 2569744, - "non_hive": { - "close": 26, - "high": 26, - "low": 26, - "open": 26, - "volume": 26 - }, - "open": "2020-03-25T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9, - "high": 9, - "low": 9, - "open": 9, - "volume": 9 - }, - "id": 2569748, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-03-25T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 147751, - "high": 48747, - "low": 69199, - "open": 69199, - "volume": 265697 - }, - "id": 2569752, - "non_hive": { - "close": 39476, - "high": 13093, - "low": 17576, - "open": 17576, - "volume": 70145 - }, - "open": "2020-03-25T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1393, - "high": 1393, - "low": 92, - "open": 1000, - "volume": 1934733 - }, - "id": 2569759, - "non_hive": { - "close": 418, - "high": 418, - "low": 22, - "open": 267, - "volume": 499295 - }, - "open": "2020-03-25T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33346, - "high": 100000, - "low": 33346, - "open": 100000, - "volume": 133346 - }, - "id": 2569784, - "non_hive": { - "close": 10002, - "high": 29997, - "low": 10002, - "open": 29997, - "volume": 39999 - }, - "open": "2020-03-25T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3649, - "high": 3, - "low": 3649, - "open": 3, - "volume": 24057 - }, - "id": 2569791, - "non_hive": { - "close": 1003, - "high": 1, - "low": 1003, - "open": 1, - "volume": 6615 - }, - "open": "2020-03-25T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1006, - "high": 1006, - "low": 11, - "open": 11, - "volume": 1017 - }, - "id": 2569798, - "non_hive": { - "close": 302, - "high": 302, - "low": 3, - "open": 3, - "volume": 305 - }, - "open": "2020-03-25T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 241, - "high": 4887, - "low": 11, - "open": 4887, - "volume": 5139 - }, - "id": 2569805, - "non_hive": { - "close": 66, - "high": 1466, - "low": 3, - "open": 1466, - "volume": 1535 - }, - "open": "2020-03-25T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42558, - "high": 123804, - "low": 42558, - "open": 11, - "volume": 166373 - }, - "id": 2569815, - "non_hive": { - "close": 10232, - "high": 34046, - "low": 10232, - "open": 3, - "volume": 44281 - }, - "open": "2020-03-25T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2501, - "high": 2501, - "low": 2501, - "open": 2501, - "volume": 2501 - }, - "id": 2569822, - "non_hive": { - "close": 601, - "high": 601, - "low": 601, - "open": 601, - "volume": 601 - }, - "open": "2020-03-25T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30, - "high": 30, - "low": 13216, - "open": 13216, - "volume": 13246 - }, - "id": 2569826, - "non_hive": { - "close": 9, - "high": 9, - "low": 3928, - "open": 3928, - "volume": 3937 - }, - "open": "2020-03-25T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4510, - "high": 3, - "low": 5333, - "open": 17486, - "volume": 1738220 - }, - "id": 2569833, - "non_hive": { - "close": 1343, - "high": 1, - "low": 1279, - "open": 5197, - "volume": 445862 - }, - "open": "2020-03-25T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1477, - "high": 1477, - "low": 41, - "open": 41, - "volume": 14100 - }, - "id": 2569866, - "non_hive": { - "close": 440, - "high": 440, - "low": 12, - "open": 12, - "volume": 4198 - }, - "open": "2020-03-26T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2037, - "high": 772, - "low": 2037, - "open": 14875, - "volume": 124992 - }, - "id": 2569880, - "non_hive": { - "close": 606, - "high": 241, - "low": 606, - "open": 4462, - "volume": 38155 - }, - "open": "2020-03-26T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9, - "high": 9, - "low": 9, - "open": 9, - "volume": 9 - }, - "id": 2569895, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 3, - "volume": 3 - }, - "open": "2020-03-26T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 64408, - "high": 2520, - "low": 10850, - "open": 1806, - "volume": 269169 - }, - "id": 2569899, - "non_hive": { - "close": 20160, - "high": 789, - "low": 3363, - "open": 560, - "volume": 84022 - }, - "open": "2020-03-26T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9959, - "high": 9959, - "low": 114539, - "open": 114539, - "volume": 369817 - }, - "id": 2569918, - "non_hive": { - "close": 3476, - "high": 3476, - "low": 35850, - "open": 35850, - "volume": 118883 - }, - "open": "2020-03-26T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3675, - "high": 3675, - "low": 72858, - "open": 25126, - "volume": 1307650 - }, - "id": 2569956, - "non_hive": { - "close": 1360, - "high": 1360, - "low": 25427, - "open": 8769, - "volume": 459233 - }, - "open": "2020-03-26T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74901, - "high": 1432, - "low": 88, - "open": 5200, - "volume": 176725 - }, - "id": 2570024, - "non_hive": { - "close": 27713, - "high": 530, - "low": 28, - "open": 1924, - "volume": 65382 - }, - "open": "2020-03-26T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32338, - "high": 55269, - "low": 32338, - "open": 426, - "volume": 269794 - }, - "id": 2570037, - "non_hive": { - "close": 11368, - "high": 28740, - "low": 11368, - "open": 151, - "volume": 105168 - }, - "open": "2020-03-26T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9006, - "high": 513, - "low": 11, - "open": 11, - "volume": 48129 - }, - "id": 2570053, - "non_hive": { - "close": 4413, - "high": 262, - "low": 4, - "open": 4, - "volume": 24364 - }, - "open": "2020-03-26T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1065, - "high": 1065, - "low": 1065, - "open": 1065, - "volume": 1065 - }, - "id": 2570063, - "non_hive": { - "close": 520, - "high": 520, - "low": 520, - "open": 520, - "volume": 520 - }, - "open": "2020-03-26T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8160, - "high": 1612, - "low": 25, - "open": 10060, - "volume": 384119 - }, - "id": 2570067, - "non_hive": { - "close": 3830, - "high": 773, - "low": 10, - "open": 4823, - "volume": 180868 - }, - "open": "2020-03-26T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1300, - "high": 14, - "low": 115858, - "open": 21937, - "volume": 473051 - }, - "id": 2570099, - "non_hive": { - "close": 585, - "high": 7, - "low": 47535, - "open": 10464, - "volume": 201307 - }, - "open": "2020-03-26T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8755, - "high": 2, - "low": 175549, - "open": 19046, - "volume": 313821 - }, - "id": 2570141, - "non_hive": { - "close": 3765, - "high": 1, - "low": 72010, - "open": 8190, - "volume": 131587 - }, - "open": "2020-03-26T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7884, - "high": 11, - "low": 381, - "open": 3658, - "volume": 60346 - }, - "id": 2570182, - "non_hive": { - "close": 3351, - "high": 5, - "low": 160, - "open": 1573, - "volume": 25782 - }, - "open": "2020-03-26T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18, - "high": 2, - "low": 12, - "open": 70, - "volume": 5150 - }, - "id": 2570211, - "non_hive": { - "close": 8, - "high": 1, - "low": 5, - "open": 30, - "volume": 2190 - }, - "open": "2020-03-26T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 823, - "high": 2, - "low": 20576, - "open": 870, - "volume": 22341 - }, - "id": 2570231, - "non_hive": { - "close": 350, - "high": 1, - "low": 8745, - "open": 370, - "volume": 9496 - }, - "open": "2020-03-26T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16600, - "high": 2433, - "low": 56735, - "open": 2433, - "volume": 643665 - }, - "id": 2570246, - "non_hive": { - "close": 6307, - "high": 1034, - "low": 19857, - "open": 1034, - "volume": 256332 - }, - "open": "2020-03-26T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7002, - "high": 92, - "low": 15598, - "open": 25719, - "volume": 213349 - }, - "id": 2570270, - "non_hive": { - "close": 2661, - "high": 38, - "low": 5459, - "open": 10287, - "volume": 84196 - }, - "open": "2020-03-26T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 2, - "low": 12, - "open": 5205, - "volume": 92607 - }, - "id": 2570303, - "non_hive": { - "close": 4, - "high": 1, - "low": 4, - "open": 1978, - "volume": 34848 - }, - "open": "2020-03-26T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4001, - "high": 42, - "low": 207945, - "open": 207945, - "volume": 214891 - }, - "id": 2570338, - "non_hive": { - "close": 1519, - "high": 16, - "low": 73196, - "open": 73196, - "volume": 75833 - }, - "open": "2020-03-26T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 410, - "high": 94, - "low": 6, - "open": 100000, - "volume": 675204 - }, - "id": 2570360, - "non_hive": { - "close": 156, - "high": 36, - "low": 2, - "open": 37964, - "volume": 256399 - }, - "open": "2020-03-26T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1021, - "high": 58733, - "low": 2500, - "open": 2500, - "volume": 193738 - }, - "id": 2570451, - "non_hive": { - "close": 402, - "high": 23199, - "low": 920, - "open": 920, - "volume": 74483 - }, - "open": "2020-03-26T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2, - "high": 2, - "low": 380, - "open": 400, - "volume": 120186 - }, - "id": 2570462, - "non_hive": { - "close": 1, - "high": 1, - "low": 148, - "open": 156, - "volume": 47206 - }, - "open": "2020-03-26T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 2, - "low": 100, - "open": 210, - "volume": 71372 - }, - "id": 2570505, - "non_hive": { - "close": 39, - "high": 1, - "low": 39, - "open": 83, - "volume": 28083 - }, - "open": "2020-03-26T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 289, - "high": 2, - "low": 3, - "open": 2, - "volume": 21572 - }, - "id": 2570572, - "non_hive": { - "close": 114, - "high": 1, - "low": 1, - "open": 1, - "volume": 8489 - }, - "open": "2020-03-27T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 7, - "low": 17026, - "open": 5, - "volume": 19257 - }, - "id": 2570609, - "non_hive": { - "close": 3, - "high": 3, - "low": 6442, - "open": 2, - "volume": 7320 - }, - "open": "2020-03-27T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 2, - "low": 11, - "open": 101, - "volume": 35194 - }, - "id": 2570623, - "non_hive": { - "close": 2, - "high": 1, - "low": 4, - "open": 40, - "volume": 13841 - }, - "open": "2020-03-27T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1739, - "high": 2, - "low": 16071, - "open": 7, - "volume": 53892 - }, - "id": 2570643, - "non_hive": { - "close": 684, - "high": 1, - "low": 6318, - "open": 3, - "volume": 21193 - }, - "open": "2020-03-27T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3329, - "high": 3329, - "low": 83974, - "open": 34728, - "volume": 122031 - }, - "id": 2570667, - "non_hive": { - "close": 1307, - "high": 1307, - "low": 31909, - "open": 13631, - "volume": 46847 - }, - "open": "2020-03-27T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100127, - "high": 17128, - "low": 100127, - "open": 174547, - "volume": 1239900 - }, - "id": 2570677, - "non_hive": { - "close": 31301, - "high": 6723, - "low": 31301, - "open": 68510, - "volume": 429381 - }, - "open": "2020-03-27T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11992, - "high": 62850, - "low": 88, - "open": 67496, - "volume": 220010 - }, - "id": 2570704, - "non_hive": { - "close": 3749, - "high": 19671, - "low": 27, - "open": 21100, - "volume": 68800 - }, - "open": "2020-03-27T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 63387, - "high": 9, - "low": 23026, - "open": 211812, - "volume": 349215 - }, - "id": 2570727, - "non_hive": { - "close": 19815, - "high": 3, - "low": 7197, - "open": 66213, - "volume": 109166 - }, - "open": "2020-03-27T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21544, - "high": 3, - "low": 10, - "open": 2600, - "volume": 251658 - }, - "id": 2570755, - "non_hive": { - "close": 6735, - "high": 1, - "low": 3, - "open": 813, - "volume": 78670 - }, - "open": "2020-03-27T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21461, - "high": 63, - "low": 1000, - "open": 447, - "volume": 492728 - }, - "id": 2570781, - "non_hive": { - "close": 6709, - "high": 20, - "low": 312, - "open": 140, - "volume": 154028 - }, - "open": "2020-03-27T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 374, - "high": 374, - "low": 26, - "open": 3125, - "volume": 199607 - }, - "id": 2570805, - "non_hive": { - "close": 117, - "high": 117, - "low": 8, - "open": 977, - "volume": 62399 - }, - "open": "2020-03-27T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56554, - "high": 1650, - "low": 245672, - "open": 3602, - "volume": 332975 - }, - "id": 2570828, - "non_hive": { - "close": 17679, - "high": 516, - "low": 76798, - "open": 1126, - "volume": 104090 - }, - "open": "2020-03-27T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4179, - "high": 12757, - "low": 153816, - "open": 153816, - "volume": 364291 - }, - "id": 2570848, - "non_hive": { - "close": 1308, - "high": 3993, - "low": 48083, - "open": 48083, - "volume": 113959 - }, - "open": "2020-03-27T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 70487, - "high": 482, - "low": 7843, - "open": 482, - "volume": 202681 - }, - "id": 2570865, - "non_hive": { - "close": 21146, - "high": 151, - "low": 2352, - "open": 151, - "volume": 61895 - }, - "open": "2020-03-27T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 47, - "low": 100, - "open": 25586, - "volume": 1880318 - }, - "id": 2570888, - "non_hive": { - "close": 312, - "high": 15, - "low": 30, - "open": 8007, - "volume": 588504 - }, - "open": "2020-03-27T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 907, - "high": 907, - "low": 46002, - "open": 2316, - "volume": 60349 - }, - "id": 2570919, - "non_hive": { - "close": 284, - "high": 284, - "low": 14398, - "open": 725, - "volume": 18889 - }, - "open": "2020-03-27T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15818, - "high": 380, - "low": 8548, - "open": 11297, - "volume": 183456 - }, - "id": 2570934, - "non_hive": { - "close": 4951, - "high": 119, - "low": 2675, - "open": 3536, - "volume": 57416 - }, - "open": "2020-03-27T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37005, - "high": 159, - "low": 3000, - "open": 22349, - "volume": 160210 - }, - "id": 2570963, - "non_hive": { - "close": 11582, - "high": 50, - "low": 938, - "open": 6995, - "volume": 50142 - }, - "open": "2020-03-27T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 552, - "high": 456, - "low": 13, - "open": 88000, - "volume": 681438 - }, - "id": 2570988, - "non_hive": { - "close": 173, - "high": 143, - "low": 4, - "open": 27542, - "volume": 213278 - }, - "open": "2020-03-27T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6182, - "high": 38, - "low": 17429, - "open": 3846, - "volume": 101208 - }, - "id": 2571019, - "non_hive": { - "close": 1935, - "high": 12, - "low": 5455, - "open": 1204, - "volume": 31679 - }, - "open": "2020-03-27T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1037381, - "high": 73, - "low": 37, - "open": 6182, - "volume": 5987361 - }, - "id": 2571060, - "non_hive": { - "close": 324690, - "high": 23, - "low": 11, - "open": 1935, - "volume": 1872166 - }, - "open": "2020-03-27T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 612, - "high": 612, - "low": 211159, - "open": 12856, - "volume": 2803133 - }, - "id": 2571103, - "non_hive": { - "close": 193, - "high": 193, - "low": 66090, - "open": 4024, - "volume": 878074 - }, - "open": "2020-03-27T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1227, - "high": 1227, - "low": 2647, - "open": 397, - "volume": 47189 - }, - "id": 2571126, - "non_hive": { - "close": 427, - "high": 427, - "low": 833, - "open": 125, - "volume": 16307 - }, - "open": "2020-03-27T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 147964, - "low": 82759, - "open": 147964, - "volume": 2654778 - }, - "id": 2571144, - "non_hive": { - "close": 29, - "high": 46631, - "low": 24000, - "open": 46631, - "volume": 819849 - }, - "open": "2020-03-27T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1529, - "high": 8043, - "low": 4, - "open": 42, - "volume": 212998 - }, - "id": 2571190, - "non_hive": { - "close": 484, - "high": 2548, - "low": 1, - "open": 12, - "volume": 62587 - }, - "open": "2020-03-28T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 251, - "high": 251, - "low": 944, - "open": 944, - "volume": 1195 - }, - "id": 2571216, - "non_hive": { - "close": 80, - "high": 80, - "low": 300, - "open": 300, - "volume": 380 - }, - "open": "2020-03-28T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3149, - "high": 3147, - "low": 12, - "open": 69256, - "volume": 94447 - }, - "id": 2571222, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 3, - "open": 22000, - "volume": 30003 - }, - "open": "2020-03-28T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20135, - "high": 56, - "low": 2681, - "open": 3165, - "volume": 34495 - }, - "id": 2571237, - "non_hive": { - "close": 6394, - "high": 18, - "low": 847, - "open": 1000, - "volume": 10945 - }, - "open": "2020-03-28T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26621, - "high": 8137, - "low": 26621, - "open": 8137, - "volume": 86930 - }, - "id": 2571257, - "non_hive": { - "close": 8447, - "high": 2582, - "low": 8447, - "open": 2582, - "volume": 27584 - }, - "open": "2020-03-28T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3236, - "high": 29248, - "low": 12462, - "open": 3415, - "volume": 95030 - }, - "id": 2571267, - "non_hive": { - "close": 1000, - "high": 9067, - "low": 3801, - "open": 1048, - "volume": 29340 - }, - "open": "2020-03-28T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9654, - "high": 637, - "low": 93, - "open": 3169, - "volume": 224868 - }, - "id": 2571289, - "non_hive": { - "close": 2943, - "high": 197, - "low": 25, - "open": 979, - "volume": 69275 - }, - "open": "2020-03-28T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 4419, - "low": 1000, - "open": 4419, - "volume": 175232 - }, - "id": 2571310, - "non_hive": { - "close": 305, - "high": 1348, - "low": 272, - "open": 1348, - "volume": 53087 - }, - "open": "2020-03-28T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2066, - "high": 1016, - "low": 1000, - "open": 1000, - "volume": 69682 - }, - "id": 2571350, - "non_hive": { - "close": 630, - "high": 310, - "low": 272, - "open": 272, - "volume": 20837 - }, - "open": "2020-03-28T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3350, - "high": 1806, - "low": 71662, - "open": 29496, - "volume": 205379 - }, - "id": 2571369, - "non_hive": { - "close": 1022, - "high": 551, - "low": 19457, - "open": 8991, - "volume": 59786 - }, - "open": "2020-03-28T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3000, - "high": 56213, - "low": 27, - "open": 27, - "volume": 140600 - }, - "id": 2571385, - "non_hive": { - "close": 924, - "high": 17426, - "low": 8, - "open": 8, - "volume": 43516 - }, - "open": "2020-03-28T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3546, - "high": 1954, - "low": 1035, - "open": 20051, - "volume": 517068 - }, - "id": 2571395, - "non_hive": { - "close": 1081, - "high": 606, - "low": 315, - "open": 6216, - "volume": 160029 - }, - "open": "2020-03-28T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 77, - "high": 77, - "low": 8702, - "open": 70001, - "volume": 90537 - }, - "id": 2571434, - "non_hive": { - "close": 24, - "high": 24, - "low": 2696, - "open": 21699, - "volume": 28062 - }, - "open": "2020-03-28T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9918, - "high": 3576, - "low": 44050, - "open": 3228, - "volume": 1112798 - }, - "id": 2571453, - "non_hive": { - "close": 3063, - "high": 1108, - "low": 12121, - "open": 1000, - "volume": 332219 - }, - "open": "2020-03-28T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4525, - "high": 50689, - "low": 2938, - "open": 116703, - "volume": 300628 - }, - "id": 2571486, - "non_hive": { - "close": 1398, - "high": 15663, - "low": 906, - "open": 36041, - "volume": 92846 - }, - "open": "2020-03-28T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10154, - "high": 544, - "low": 3000, - "open": 94224, - "volume": 146504 - }, - "id": 2571525, - "non_hive": { - "close": 3097, - "high": 166, - "low": 906, - "open": 28738, - "volume": 44675 - }, - "open": "2020-03-28T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12463, - "high": 42, - "low": 12463, - "open": 42, - "volume": 115579 - }, - "id": 2571553, - "non_hive": { - "close": 3764, - "high": 13, - "low": 3764, - "open": 13, - "volume": 35129 - }, - "open": "2020-03-28T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3417, - "high": 2752, - "low": 3417, - "open": 99924, - "volume": 171902 - }, - "id": 2571572, - "non_hive": { - "close": 1032, - "high": 837, - "low": 1032, - "open": 30381, - "volume": 52256 - }, - "open": "2020-03-28T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 248, - "high": 6982, - "low": 14, - "open": 6982, - "volume": 24709 - }, - "id": 2571594, - "non_hive": { - "close": 75, - "high": 2123, - "low": 4, - "open": 2123, - "volume": 7503 - }, - "open": "2020-03-28T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 917, - "high": 917, - "low": 14826, - "open": 11186, - "volume": 186330 - }, - "id": 2571609, - "non_hive": { - "close": 279, - "high": 279, - "low": 4462, - "open": 3388, - "volume": 56408 - }, - "open": "2020-03-28T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27318, - "high": 3, - "low": 1667, - "open": 4000, - "volume": 35100 - }, - "id": 2571629, - "non_hive": { - "close": 8194, - "high": 1, - "low": 500, - "open": 1204, - "volume": 10534 - }, - "open": "2020-03-28T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37196, - "high": 385, - "low": 37196, - "open": 3000, - "volume": 154989 - }, - "id": 2571647, - "non_hive": { - "close": 10416, - "high": 116, - "low": 10416, - "open": 888, - "volume": 44637 - }, - "open": "2020-03-28T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 151384, - "high": 3, - "low": 72, - "open": 399, - "volume": 450086 - }, - "id": 2571668, - "non_hive": { - "close": 42394, - "high": 1, - "low": 20, - "open": 117, - "volume": 126700 - }, - "open": "2020-03-28T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1885, - "high": 47, - "low": 5, - "open": 28414, - "volume": 68027 - }, - "id": 2571697, - "non_hive": { - "close": 552, - "high": 14, - "low": 1, - "open": 8240, - "volume": 19892 - }, - "open": "2020-03-28T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 952, - "low": 4, - "open": 104, - "volume": 26655 - }, - "id": 2571716, - "non_hive": { - "close": 1, - "high": 279, - "low": 1, - "open": 29, - "volume": 7768 - }, - "open": "2020-03-29T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1183, - "high": 16302, - "low": 200, - "open": 16302, - "volume": 31594 - }, - "id": 2571738, - "non_hive": { - "close": 342, - "high": 4730, - "low": 56, - "open": 4730, - "volume": 9148 - }, - "open": "2020-03-29T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3491, - "high": 1694, - "low": 11, - "open": 1694, - "volume": 8516 - }, - "id": 2571749, - "non_hive": { - "close": 1000, - "high": 488, - "low": 3, - "open": 488, - "volume": 2445 - }, - "open": "2020-03-29T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3350, - "high": 9368, - "low": 3350, - "open": 9368, - "volume": 14975 - }, - "id": 2571762, - "non_hive": { - "close": 948, - "high": 2682, - "low": 948, - "open": 2682, - "volume": 4275 - }, - "open": "2020-03-29T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1210, - "high": 681, - "low": 18104, - "open": 94756, - "volume": 155727 - }, - "id": 2571772, - "non_hive": { - "close": 344, - "high": 194, - "low": 5146, - "open": 26980, - "volume": 44326 - }, - "open": "2020-03-29T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2844, - "high": 1144, - "low": 314, - "open": 1144, - "volume": 18212 - }, - "id": 2571790, - "non_hive": { - "close": 803, - "high": 324, - "low": 87, - "open": 324, - "volume": 5142 - }, - "open": "2020-03-29T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 93, - "high": 225998, - "low": 93, - "open": 225998, - "volume": 226091 - }, - "id": 2571811, - "non_hive": { - "close": 26, - "high": 63802, - "low": 26, - "open": 63802, - "volume": 63828 - }, - "open": "2020-03-29T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 240, - "high": 3084, - "low": 4290, - "open": 3084, - "volume": 137274 - }, - "id": 2571818, - "non_hive": { - "close": 68, - "high": 875, - "low": 1215, - "open": 875, - "volume": 38898 - }, - "open": "2020-03-29T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9275, - "high": 1477, - "low": 11, - "open": 11, - "volume": 1039243 - }, - "id": 2571829, - "non_hive": { - "close": 2599, - "high": 418, - "low": 3, - "open": 3, - "volume": 291530 - }, - "open": "2020-03-29T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 68443, - "high": 2561, - "low": 346083, - "open": 2561, - "volume": 1247358 - }, - "id": 2571849, - "non_hive": { - "close": 19341, - "high": 725, - "low": 97797, - "open": 725, - "volume": 352815 - }, - "open": "2020-03-29T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1732, - "high": 746, - "low": 29, - "open": 746, - "volume": 133443 - }, - "id": 2571885, - "non_hive": { - "close": 489, - "high": 211, - "low": 8, - "open": 211, - "volume": 37505 - }, - "open": "2020-03-29T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53, - "high": 134446, - "low": 8534, - "open": 134446, - "volume": 1487302 - }, - "id": 2571911, - "non_hive": { - "close": 14, - "high": 37942, - "low": 2133, - "open": 37942, - "volume": 397507 - }, - "open": "2020-03-29T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3880, - "high": 4234, - "low": 4977, - "open": 20844, - "volume": 200834 - }, - "id": 2571976, - "non_hive": { - "close": 1000, - "high": 1175, - "low": 1208, - "open": 5211, - "volume": 51127 - }, - "open": "2020-03-29T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2865, - "high": 2725, - "low": 3300, - "open": 3300, - "volume": 52085 - }, - "id": 2572009, - "non_hive": { - "close": 802, - "high": 763, - "low": 839, - "open": 839, - "volume": 14483 - }, - "open": "2020-03-29T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 11194, - "low": 28957, - "open": 689, - "volume": 528332 - }, - "id": 2572033, - "non_hive": { - "close": 25, - "high": 3130, - "low": 7208, - "open": 187, - "volume": 136377 - }, - "open": "2020-03-29T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41571, - "high": 849, - "low": 41571, - "open": 849, - "volume": 1004123 - }, - "id": 2572064, - "non_hive": { - "close": 10100, - "high": 234, - "low": 10100, - "open": 234, - "volume": 248140 - }, - "open": "2020-03-29T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2000, - "high": 5612, - "low": 2000, - "open": 61465, - "volume": 3668621 - }, - "id": 2572104, - "non_hive": { - "close": 460, - "high": 1403, - "low": 460, - "open": 14935, - "volume": 868404 - }, - "open": "2020-03-29T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1528, - "high": 4, - "low": 273, - "open": 300141, - "volume": 2450347 - }, - "id": 2572156, - "non_hive": { - "close": 380, - "high": 1, - "low": 63, - "open": 70000, - "volume": 571711 - }, - "open": "2020-03-29T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1310, - "high": 4, - "low": 13, - "open": 4, - "volume": 716790 - }, - "id": 2572199, - "non_hive": { - "close": 323, - "high": 1, - "low": 3, - "open": 1, - "volume": 166614 - }, - "open": "2020-03-29T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3321, - "high": 25, - "low": 3321, - "open": 8337, - "volume": 122014 - }, - "id": 2572242, - "non_hive": { - "close": 782, - "high": 6, - "low": 782, - "open": 2000, - "volume": 29063 - }, - "open": "2020-03-29T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6165, - "high": 4, - "low": 9, - "open": 679, - "volume": 318597 - }, - "id": 2572267, - "non_hive": { - "close": 1418, - "high": 1, - "low": 2, - "open": 156, - "volume": 73965 - }, - "open": "2020-03-29T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1715, - "high": 1715, - "low": 6, - "open": 6000, - "volume": 45052 - }, - "id": 2572315, - "non_hive": { - "close": 405, - "high": 405, - "low": 1, - "open": 1372, - "volume": 10444 - }, - "open": "2020-03-29T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32902, - "high": 8, - "low": 122, - "open": 2360, - "volume": 188118 - }, - "id": 2572346, - "non_hive": { - "close": 7733, - "high": 2, - "low": 27, - "open": 557, - "volume": 43503 - }, - "open": "2020-03-29T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 4, - "low": 6932, - "open": 4, - "volume": 38652 - }, - "id": 2572367, - "non_hive": { - "close": 3, - "high": 1, - "low": 1635, - "open": 1, - "volume": 9122 - }, - "open": "2020-03-29T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 321, - "high": 4, - "low": 61, - "open": 4, - "volume": 18162 - }, - "id": 2572405, - "non_hive": { - "close": 74, - "high": 1, - "low": 13, - "open": 1, - "volume": 4184 - }, - "open": "2020-03-30T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30, - "high": 30, - "low": 114, - "open": 11244, - "volume": 1142178 - }, - "id": 2572438, - "non_hive": { - "close": 7, - "high": 7, - "low": 26, - "open": 2589, - "volume": 262323 - }, - "open": "2020-03-30T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8, - "high": 8, - "low": 9, - "open": 56, - "volume": 169843 - }, - "id": 2572472, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 13, - "volume": 38664 - }, - "open": "2020-03-30T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 88, - "high": 4, - "low": 90308, - "open": 4, - "volume": 178690 - }, - "id": 2572499, - "non_hive": { - "close": 20, - "high": 1, - "low": 20303, - "open": 1, - "volume": 40177 - }, - "open": "2020-03-30T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20720, - "high": 4, - "low": 1241, - "open": 14921, - "volume": 270301 - }, - "id": 2572526, - "non_hive": { - "close": 4659, - "high": 1, - "low": 278, - "open": 3355, - "volume": 60766 - }, - "open": "2020-03-30T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2686, - "high": 26, - "low": 14585, - "open": 2374, - "volume": 267444 - }, - "id": 2572561, - "non_hive": { - "close": 618, - "high": 6, - "low": 3210, - "open": 534, - "volume": 59861 - }, - "open": "2020-03-30T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 142506, - "high": 1060, - "low": 200000, - "open": 1060, - "volume": 376412 - }, - "id": 2572579, - "non_hive": { - "close": 31503, - "high": 244, - "low": 44206, - "open": 244, - "volume": 83373 - }, - "open": "2020-03-30T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 8, - "low": 9944, - "open": 25152, - "volume": 36969 - }, - "id": 2572605, - "non_hive": { - "close": 1, - "high": 2, - "low": 2192, - "open": 5550, - "volume": 8168 - }, - "open": "2020-03-30T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74, - "high": 4, - "low": 46692, - "open": 7248, - "volume": 70026 - }, - "id": 2572628, - "non_hive": { - "close": 17, - "high": 1, - "low": 10292, - "open": 1598, - "volume": 15530 - }, - "open": "2020-03-30T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74886, - "high": 8, - "low": 1674, - "open": 17, - "volume": 309520 - }, - "id": 2572640, - "non_hive": { - "close": 17184, - "high": 2, - "low": 379, - "open": 4, - "volume": 70569 - }, - "open": "2020-03-30T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13539, - "high": 7808, - "low": 28, - "open": 2276, - "volume": 269720 - }, - "id": 2572666, - "non_hive": { - "close": 3019, - "high": 1792, - "low": 6, - "open": 516, - "volume": 59583 - }, - "open": "2020-03-30T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 106017, - "high": 8, - "low": 49908, - "open": 9144, - "volume": 2712360 - }, - "id": 2572681, - "non_hive": { - "close": 22263, - "high": 2, - "low": 10480, - "open": 2039, - "volume": 586580 - }, - "open": "2020-03-30T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38644, - "high": 26, - "low": 42047, - "open": 30152, - "volume": 500688 - }, - "id": 2572734, - "non_hive": { - "close": 8887, - "high": 6, - "low": 9077, - "open": 6597, - "volume": 112171 - }, - "open": "2020-03-30T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7373, - "high": 1634, - "low": 42658, - "open": 42658, - "volume": 1667932 - }, - "id": 2572772, - "non_hive": { - "close": 1696, - "high": 376, - "low": 9598, - "open": 9598, - "volume": 383404 - }, - "open": "2020-03-30T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4369, - "high": 4369, - "low": 9, - "open": 4369, - "volume": 141346 - }, - "id": 2572808, - "non_hive": { - "close": 1005, - "high": 1005, - "low": 2, - "open": 1005, - "volume": 32510 - }, - "open": "2020-03-30T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12221, - "high": 12221, - "low": 75468, - "open": 6061, - "volume": 295381 - }, - "id": 2572831, - "non_hive": { - "close": 2811, - "high": 2811, - "low": 16997, - "open": 1394, - "volume": 67577 - }, - "open": "2020-03-30T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13054, - "high": 4, - "low": 576306, - "open": 4369, - "volume": 780010 - }, - "id": 2572848, - "non_hive": { - "close": 3002, - "high": 1, - "low": 129783, - "open": 1005, - "volume": 175903 - }, - "open": "2020-03-30T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4352, - "high": 17, - "low": 66907, - "open": 10271, - "volume": 161901 - }, - "id": 2572867, - "non_hive": { - "close": 1001, - "high": 4, - "low": 15385, - "open": 2362, - "volume": 37231 - }, - "open": "2020-03-30T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4355, - "high": 4, - "low": 14, - "open": 217, - "volume": 286331 - }, - "id": 2572893, - "non_hive": { - "close": 1000, - "high": 1, - "low": 3, - "open": 50, - "volume": 64952 - }, - "open": "2020-03-30T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6254, - "high": 4355, - "low": 64, - "open": 4355, - "volume": 35695 - }, - "id": 2572937, - "non_hive": { - "close": 1436, - "high": 1000, - "low": 13, - "open": 1000, - "volume": 8185 - }, - "open": "2020-03-30T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2275, - "high": 767, - "low": 14, - "open": 767, - "volume": 77010 - }, - "id": 2572955, - "non_hive": { - "close": 522, - "high": 176, - "low": 3, - "open": 176, - "volume": 17669 - }, - "open": "2020-03-30T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1902, - "high": 47, - "low": 29666, - "open": 29666, - "volume": 180249 - }, - "id": 2572973, - "non_hive": { - "close": 437, - "high": 11, - "low": 6806, - "open": 6806, - "volume": 41393 - }, - "open": "2020-03-30T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4362, - "high": 4, - "low": 410, - "open": 410, - "volume": 14239 - }, - "id": 2572984, - "non_hive": { - "close": 1002, - "high": 1, - "low": 94, - "open": 94, - "volume": 3271 - }, - "open": "2020-03-30T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 77, - "open": 4, - "volume": 770 - }, - "id": 2573006, - "non_hive": { - "close": 1, - "high": 1, - "low": 17, - "open": 1, - "volume": 177 - }, - "open": "2020-03-30T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 204, - "high": 4, - "low": 41, - "open": 4, - "volume": 203047 - }, - "id": 2573032, - "non_hive": { - "close": 47, - "high": 1, - "low": 9, - "open": 1, - "volume": 46635 - }, - "open": "2020-03-31T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4793, - "high": 4, - "low": 9151, - "open": 4, - "volume": 13978 - }, - "id": 2573064, - "non_hive": { - "close": 1101, - "high": 1, - "low": 2102, - "open": 1, - "volume": 3211 - }, - "open": "2020-03-31T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 9, - "open": 56, - "volume": 5600 - }, - "id": 2573078, - "non_hive": { - "close": 1, - "high": 1, - "low": 2, - "open": 13, - "volume": 1287 - }, - "open": "2020-03-31T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 65002, - "high": 65002, - "low": 10653, - "open": 10653, - "volume": 75655 - }, - "id": 2573099, - "non_hive": { - "close": 14931, - "high": 14931, - "low": 2447, - "open": 2447, - "volume": 17378 - }, - "open": "2020-03-31T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57193, - "high": 1053, - "low": 57193, - "open": 1053, - "volume": 91211 - }, - "id": 2573106, - "non_hive": { - "close": 13134, - "high": 242, - "low": 13134, - "open": 242, - "volume": 20948 - }, - "open": "2020-03-31T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 64766, - "high": 65, - "low": 105, - "open": 8302, - "volume": 264578 - }, - "id": 2573119, - "non_hive": { - "close": 14873, - "high": 15, - "low": 24, - "open": 1907, - "volume": 60766 - }, - "open": "2020-03-31T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21211, - "high": 1300, - "low": 95, - "open": 1300, - "volume": 101716 - }, - "id": 2573142, - "non_hive": { - "close": 4857, - "high": 299, - "low": 21, - "open": 299, - "volume": 23315 - }, - "open": "2020-03-31T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 8, - "low": 144621, - "open": 17344, - "volume": 1668453 - }, - "id": 2573176, - "non_hive": { - "close": 1, - "high": 2, - "low": 31948, - "open": 3972, - "volume": 370192 - }, - "open": "2020-03-31T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 638, - "high": 23682, - "low": 18, - "open": 15747, - "volume": 45270 - }, - "id": 2573225, - "non_hive": { - "close": 141, - "high": 5441, - "low": 3, - "open": 3616, - "volume": 10392 - }, - "open": "2020-03-31T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3080, - "high": 1773, - "low": 643, - "open": 638, - "volume": 77306 - }, - "id": 2573243, - "non_hive": { - "close": 707, - "high": 407, - "low": 142, - "open": 146, - "volume": 17712 - }, - "open": "2020-03-31T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4670, - "high": 4670, - "low": 27, - "open": 47949, - "volume": 278910 - }, - "id": 2573262, - "non_hive": { - "close": 1072, - "high": 1072, - "low": 6, - "open": 11005, - "volume": 64016 - }, - "open": "2020-03-31T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 933, - "high": 17, - "low": 933, - "open": 3010, - "volume": 74294 - }, - "id": 2573291, - "non_hive": { - "close": 214, - "high": 4, - "low": 214, - "open": 691, - "volume": 17052 - }, - "open": "2020-03-31T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6606, - "high": 4, - "low": 1077, - "open": 12063, - "volume": 137769 - }, - "id": 2573309, - "non_hive": { - "close": 1515, - "high": 1, - "low": 238, - "open": 2767, - "volume": 30996 - }, - "open": "2020-03-31T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6636, - "high": 16846, - "low": 23348, - "open": 9623, - "volume": 115676 - }, - "id": 2573334, - "non_hive": { - "close": 1522, - "high": 3864, - "low": 5354, - "open": 2207, - "volume": 26530 - }, - "open": "2020-03-31T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 872, - "high": 514, - "low": 9, - "open": 22444, - "volume": 173246 - }, - "id": 2573355, - "non_hive": { - "close": 200, - "high": 118, - "low": 2, - "open": 5147, - "volume": 39729 - }, - "open": "2020-03-31T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34260, - "high": 165, - "low": 30000, - "open": 3519, - "volume": 298241 - }, - "id": 2573377, - "non_hive": { - "close": 7855, - "high": 38, - "low": 6878, - "open": 807, - "volume": 68383 - }, - "open": "2020-03-31T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10928, - "high": 1324, - "low": 10928, - "open": 4401, - "volume": 425690 - }, - "id": 2573408, - "non_hive": { - "close": 2407, - "high": 301, - "low": 2407, - "open": 1000, - "volume": 94233 - }, - "open": "2020-03-31T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8836, - "high": 912, - "low": 30961, - "open": 57542, - "volume": 138627 - }, - "id": 2573421, - "non_hive": { - "close": 1947, - "high": 201, - "low": 6822, - "open": 12679, - "volume": 30546 - }, - "open": "2020-03-31T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2707, - "high": 245, - "low": 10, - "open": 4539, - "volume": 282205 - }, - "id": 2573445, - "non_hive": { - "close": 596, - "high": 54, - "low": 2, - "open": 1000, - "volume": 60944 - }, - "open": "2020-03-31T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45335, - "high": 4565, - "low": 45335, - "open": 45430, - "volume": 95330 - }, - "id": 2573476, - "non_hive": { - "close": 9979, - "high": 1005, - "low": 9979, - "open": 10000, - "volume": 20984 - }, - "open": "2020-03-31T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4397, - "high": 4391, - "low": 10, - "open": 10, - "volume": 727524 - }, - "id": 2573486, - "non_hive": { - "close": 1002, - "high": 1001, - "low": 2, - "open": 2, - "volume": 165804 - }, - "open": "2020-03-31T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30035, - "high": 15794, - "low": 3400, - "open": 3400, - "volume": 49229 - }, - "id": 2573504, - "non_hive": { - "close": 6846, - "high": 3600, - "low": 765, - "open": 765, - "volume": 11211 - }, - "open": "2020-03-31T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 655, - "high": 655, - "low": 404, - "open": 404, - "volume": 359999 - }, - "id": 2573514, - "non_hive": { - "close": 150, - "high": 150, - "low": 92, - "open": 92, - "volume": 82080 - }, - "open": "2020-03-31T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2366, - "high": 283, - "low": 6768, - "open": 60899, - "volume": 145586 - }, - "id": 2573534, - "non_hive": { - "close": 542, - "high": 65, - "low": 1522, - "open": 13946, - "volume": 33264 - }, - "open": "2020-03-31T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 134215, - "high": 4305, - "low": 41, - "open": 72783, - "volume": 267373 - }, - "id": 2573565, - "non_hive": { - "close": 30734, - "high": 986, - "low": 9, - "open": 16666, - "volume": 61208 - }, - "open": "2020-04-01T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 475, - "high": 475, - "low": 4344, - "open": 5454, - "volume": 47338 - }, - "id": 2573587, - "non_hive": { - "close": 109, - "high": 109, - "low": 994, - "open": 1249, - "volume": 10840 - }, - "open": "2020-04-01T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16218, - "high": 7000, - "low": 108735, - "open": 108735, - "volume": 250186 - }, - "id": 2573597, - "non_hive": { - "close": 3712, - "high": 1603, - "low": 23935, - "open": 23935, - "volume": 56322 - }, - "open": "2020-04-01T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43827, - "high": 7091, - "low": 3494, - "open": 16858, - "volume": 1825382 - }, - "id": 2573610, - "non_hive": { - "close": 9722, - "high": 1623, - "low": 765, - "open": 3712, - "volume": 404207 - }, - "open": "2020-04-01T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3385, - "high": 3385, - "low": 4355, - "open": 4071, - "volume": 37646 - }, - "id": 2573650, - "non_hive": { - "close": 751, - "high": 751, - "low": 958, - "open": 903, - "volume": 8342 - }, - "open": "2020-04-01T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 909, - "high": 9047, - "low": 4709, - "open": 29458, - "volume": 682990 - }, - "id": 2573663, - "non_hive": { - "close": 200, - "high": 2007, - "low": 1036, - "open": 6534, - "volume": 151478 - }, - "open": "2020-04-01T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10188, - "high": 1502, - "low": 8, - "open": 24677, - "volume": 733392 - }, - "id": 2573698, - "non_hive": { - "close": 2251, - "high": 332, - "low": 1, - "open": 5381, - "volume": 160279 - }, - "open": "2020-04-01T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10066, - "high": 76, - "low": 11905, - "open": 3286, - "volume": 1988093 - }, - "id": 2573728, - "non_hive": { - "close": 2114, - "high": 17, - "low": 2500, - "open": 726, - "volume": 421197 - }, - "open": "2020-04-01T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2881, - "high": 20534, - "low": 6500, - "open": 20534, - "volume": 51944 - }, - "id": 2573748, - "non_hive": { - "close": 634, - "high": 4533, - "low": 1430, - "open": 4533, - "volume": 11460 - }, - "open": "2020-04-01T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12888, - "high": 11116, - "low": 29, - "open": 29, - "volume": 48921 - }, - "id": 2573761, - "non_hive": { - "close": 2832, - "high": 2454, - "low": 6, - "open": 6, - "volume": 10784 - }, - "open": "2020-04-01T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 738, - "high": 1305, - "low": 19746, - "open": 101116, - "volume": 317373 - }, - "id": 2573777, - "non_hive": { - "close": 157, - "high": 288, - "low": 4148, - "open": 21243, - "volume": 68490 - }, - "open": "2020-04-01T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6902, - "high": 16197, - "low": 215, - "open": 14440, - "volume": 152199 - }, - "id": 2573803, - "non_hive": { - "close": 1517, - "high": 3565, - "low": 45, - "open": 3178, - "volume": 33463 - }, - "open": "2020-04-01T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4596, - "high": 5293, - "low": 176, - "open": 208159, - "volume": 437519 - }, - "id": 2573832, - "non_hive": { - "close": 1006, - "high": 1162, - "low": 36, - "open": 45180, - "volume": 95412 - }, - "open": "2020-04-01T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 201, - "high": 201, - "low": 10, - "open": 5652, - "volume": 53491 - }, - "id": 2573868, - "non_hive": { - "close": 44, - "high": 44, - "low": 2, - "open": 1235, - "volume": 11381 - }, - "open": "2020-04-01T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15004, - "high": 15619, - "low": 27, - "open": 4672, - "volume": 54507 - }, - "id": 2573881, - "non_hive": { - "close": 3283, - "high": 3419, - "low": 5, - "open": 1000, - "volume": 11823 - }, - "open": "2020-04-01T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 215302, - "high": 32, - "low": 3925, - "open": 32, - "volume": 267758 - }, - "id": 2573898, - "non_hive": { - "close": 47086, - "high": 7, - "low": 858, - "open": 7, - "volume": 58558 - }, - "open": "2020-04-01T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8345, - "high": 8345, - "low": 32, - "open": 100000, - "volume": 1775527 - }, - "id": 2573917, - "non_hive": { - "close": 1827, - "high": 1827, - "low": 6, - "open": 21869, - "volume": 383900 - }, - "open": "2020-04-01T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 487, - "high": 9475, - "low": 487, - "open": 12556, - "volume": 192783 - }, - "id": 2573951, - "non_hive": { - "close": 104, - "high": 2075, - "low": 104, - "open": 2749, - "volume": 42211 - }, - "open": "2020-04-01T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 138737, - "high": 200, - "low": 10000, - "open": 3274, - "volume": 176408 - }, - "id": 2573983, - "non_hive": { - "close": 30380, - "high": 44, - "low": 2160, - "open": 717, - "volume": 38600 - }, - "open": "2020-04-01T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2429, - "high": 2429, - "low": 10, - "open": 10, - "volume": 26900 - }, - "id": 2574003, - "non_hive": { - "close": 532, - "high": 532, - "low": 2, - "open": 2, - "volume": 5887 - }, - "open": "2020-04-01T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74127, - "high": 853, - "low": 19883, - "open": 853, - "volume": 262177 - }, - "id": 2574015, - "non_hive": { - "close": 16234, - "high": 187, - "low": 4354, - "open": 187, - "volume": 57415 - }, - "open": "2020-04-01T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3118, - "high": 3118, - "low": 111, - "open": 100474, - "volume": 1765189 - }, - "id": 2574032, - "non_hive": { - "close": 683, - "high": 683, - "low": 24, - "open": 22002, - "volume": 386575 - }, - "open": "2020-04-01T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13611, - "high": 1342, - "low": 24242, - "open": 662, - "volume": 189588 - }, - "id": 2574059, - "non_hive": { - "close": 2981, - "high": 294, - "low": 5309, - "open": 145, - "volume": 41521 - }, - "open": "2020-04-01T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17841, - "high": 11409, - "low": 42, - "open": 42, - "volume": 717244 - }, - "id": 2574086, - "non_hive": { - "close": 3978, - "high": 2544, - "low": 9, - "open": 9, - "volume": 157198 - }, - "open": "2020-04-02T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53830, - "high": 3330, - "low": 1344, - "open": 35525, - "volume": 382044 - }, - "id": 2574112, - "non_hive": { - "close": 12347, - "high": 764, - "low": 299, - "open": 7921, - "volume": 86176 - }, - "open": "2020-04-02T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 114742, - "high": 4, - "low": 10, - "open": 13532, - "volume": 657950 - }, - "id": 2574157, - "non_hive": { - "close": 26318, - "high": 1, - "low": 2, - "open": 3104, - "volume": 144005 - }, - "open": "2020-04-02T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4373, - "high": 2834, - "low": 344555, - "open": 35763, - "volume": 1380868 - }, - "id": 2574180, - "non_hive": { - "close": 1001, - "high": 650, - "low": 73149, - "open": 8200, - "volume": 298003 - }, - "open": "2020-04-02T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 75, - "low": 12, - "open": 52688, - "volume": 215402 - }, - "id": 2574206, - "non_hive": { - "close": 2, - "high": 17, - "low": 2, - "open": 11190, - "volume": 45745 - }, - "open": "2020-04-02T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 113603, - "high": 9995, - "low": 5, - "open": 9995, - "volume": 123603 - }, - "id": 2574234, - "non_hive": { - "close": 25560, - "high": 2249, - "low": 1, - "open": 2249, - "volume": 27810 - }, - "open": "2020-04-02T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18273, - "high": 88320, - "low": 60114, - "open": 128, - "volume": 778723 - }, - "id": 2574241, - "non_hive": { - "close": 4109, - "high": 20069, - "low": 12766, - "open": 29, - "volume": 173085 - }, - "open": "2020-04-02T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27315, - "high": 2686, - "low": 27315, - "open": 2686, - "volume": 168874 - }, - "id": 2574272, - "non_hive": { - "close": 6140, - "high": 604, - "low": 6140, - "open": 604, - "volume": 37971 - }, - "open": "2020-04-02T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16000, - "high": 214, - "low": 10, - "open": 214, - "volume": 1014083 - }, - "id": 2574285, - "non_hive": { - "close": 3520, - "high": 48, - "low": 2, - "open": 48, - "volume": 216088 - }, - "open": "2020-04-02T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5514, - "high": 4486, - "low": 3600, - "open": 3600, - "volume": 61429 - }, - "id": 2574318, - "non_hive": { - "close": 1229, - "high": 1000, - "low": 783, - "open": 783, - "volume": 13593 - }, - "open": "2020-04-02T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48, - "high": 4, - "low": 388757, - "open": 2876, - "volume": 1109394 - }, - "id": 2574337, - "non_hive": { - "close": 11, - "high": 1, - "low": 81665, - "open": 644, - "volume": 235410 - }, - "open": "2020-04-02T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 147089, - "open": 5084, - "volume": 1777625 - }, - "id": 2574381, - "non_hive": { - "close": 1, - "high": 1, - "low": 30447, - "open": 1142, - "volume": 391483 - }, - "open": "2020-04-02T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2304, - "high": 4, - "low": 35970, - "open": 35970, - "volume": 255110 - }, - "id": 2574428, - "non_hive": { - "close": 514, - "high": 1, - "low": 7446, - "open": 7446, - "volume": 56002 - }, - "open": "2020-04-02T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20000, - "high": 1163, - "low": 3700, - "open": 3700, - "volume": 174784 - }, - "id": 2574457, - "non_hive": { - "close": 4467, - "high": 260, - "low": 814, - "open": 814, - "volume": 38989 - }, - "open": "2020-04-02T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19771, - "high": 1069, - "low": 22728, - "open": 595, - "volume": 68817 - }, - "id": 2574487, - "non_hive": { - "close": 4417, - "high": 239, - "low": 5000, - "open": 133, - "volume": 15295 - }, - "open": "2020-04-02T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15514, - "high": 3755, - "low": 135, - "open": 4252, - "volume": 170773 - }, - "id": 2574509, - "non_hive": { - "close": 3466, - "high": 839, - "low": 30, - "open": 950, - "volume": 38141 - }, - "open": "2020-04-02T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30000, - "high": 71, - "low": 30000, - "open": 7202, - "volume": 126602 - }, - "id": 2574547, - "non_hive": { - "close": 6689, - "high": 16, - "low": 6689, - "open": 1609, - "volume": 28276 - }, - "open": "2020-04-02T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11723, - "high": 31, - "low": 4174, - "open": 3156, - "volume": 92190 - }, - "id": 2574576, - "non_hive": { - "close": 2619, - "high": 7, - "low": 921, - "open": 704, - "volume": 20559 - }, - "open": "2020-04-02T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3016, - "high": 2202, - "low": 10, - "open": 55335, - "volume": 805650 - }, - "id": 2574599, - "non_hive": { - "close": 673, - "high": 492, - "low": 2, - "open": 12362, - "volume": 178323 - }, - "open": "2020-04-02T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9373, - "high": 1066, - "low": 337880, - "open": 37959, - "volume": 1198136 - }, - "id": 2574640, - "non_hive": { - "close": 2088, - "high": 238, - "low": 70000, - "open": 7970, - "volume": 248873 - }, - "open": "2020-04-02T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 292933, - "high": 8581, - "low": 10, - "open": 8581, - "volume": 633238 - }, - "id": 2574672, - "non_hive": { - "close": 61516, - "high": 1912, - "low": 2, - "open": 1912, - "volume": 133509 - }, - "open": "2020-04-02T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7603, - "high": 14591, - "low": 344715, - "open": 4761, - "volume": 1173752 - }, - "id": 2574706, - "non_hive": { - "close": 1695, - "high": 3254, - "low": 72390, - "open": 1000, - "volume": 256582 - }, - "open": "2020-04-02T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1436, - "high": 420, - "low": 50001, - "open": 2157, - "volume": 2208457 - }, - "id": 2574727, - "non_hive": { - "close": 321, - "high": 94, - "low": 10303, - "open": 481, - "volume": 475346 - }, - "open": "2020-04-02T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9473, - "high": 4916, - "low": 53, - "open": 34176, - "volume": 161897 - }, - "id": 2574776, - "non_hive": { - "close": 2115, - "high": 1098, - "low": 10, - "open": 7632, - "volume": 34965 - }, - "open": "2020-04-02T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44949, - "high": 2145, - "low": 291186, - "open": 41, - "volume": 433878 - }, - "id": 2574807, - "non_hive": { - "close": 10024, - "high": 479, - "low": 58825, - "open": 9, - "volume": 90595 - }, - "open": "2020-04-03T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14481, - "high": 1809, - "low": 3680, - "open": 3680, - "volume": 418336 - }, - "id": 2574840, - "non_hive": { - "close": 3218, - "high": 404, - "low": 817, - "open": 817, - "volume": 92978 - }, - "open": "2020-04-03T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21, - "high": 99, - "low": 21, - "open": 10, - "volume": 130 - }, - "id": 2574865, - "non_hive": { - "close": 4, - "high": 22, - "low": 4, - "open": 2, - "volume": 28 - }, - "open": "2020-04-03T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8170, - "high": 76, - "low": 3789, - "open": 76, - "volume": 1157900 - }, - "id": 2574875, - "non_hive": { - "close": 1644, - "high": 17, - "low": 762, - "open": 17, - "volume": 233154 - }, - "open": "2020-04-03T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 86, - "high": 4513, - "low": 8417, - "open": 8251, - "volume": 345828 - }, - "id": 2574927, - "non_hive": { - "close": 19, - "high": 1000, - "low": 1693, - "open": 1660, - "volume": 72455 - }, - "open": "2020-04-03T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6490, - "high": 4799, - "low": 56286, - "open": 6138, - "volume": 1113013 - }, - "id": 2574984, - "non_hive": { - "close": 1432, - "high": 1061, - "low": 11355, - "open": 1354, - "volume": 225131 - }, - "open": "2020-04-03T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1610, - "high": 18860, - "low": 24928, - "open": 22476, - "volume": 2732974 - }, - "id": 2575012, - "non_hive": { - "close": 353, - "high": 4149, - "low": 4985, - "open": 4630, - "volume": 556211 - }, - "open": "2020-04-03T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3243, - "high": 7283, - "low": 340233, - "open": 4380, - "volume": 366353 - }, - "id": 2575045, - "non_hive": { - "close": 712, - "high": 1599, - "low": 68046, - "open": 960, - "volume": 73771 - }, - "open": "2020-04-03T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5827, - "high": 3862, - "low": 10, - "open": 10, - "volume": 104479 - }, - "id": 2575069, - "non_hive": { - "close": 1267, - "high": 841, - "low": 2, - "open": 2, - "volume": 22298 - }, - "open": "2020-04-03T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 64, - "high": 64, - "low": 10058, - "open": 23029, - "volume": 262179 - }, - "id": 2575094, - "non_hive": { - "close": 14, - "high": 14, - "low": 2173, - "open": 5000, - "volume": 56729 - }, - "open": "2020-04-03T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2052, - "high": 1033, - "low": 175989, - "open": 3127, - "volume": 1419600 - }, - "id": 2575130, - "non_hive": { - "close": 440, - "high": 223, - "low": 34988, - "open": 675, - "volume": 284284 - }, - "open": "2020-04-03T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8317, - "high": 2984, - "low": 8317, - "open": 2984, - "volume": 295499 - }, - "id": 2575154, - "non_hive": { - "close": 1655, - "high": 650, - "low": 1655, - "open": 650, - "volume": 63047 - }, - "open": "2020-04-03T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3987, - "high": 3075, - "low": 34949, - "open": 23990, - "volume": 198452 - }, - "id": 2575182, - "non_hive": { - "close": 862, - "high": 670, - "low": 6855, - "open": 5051, - "volume": 41791 - }, - "open": "2020-04-03T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31665, - "high": 7935, - "low": 174099, - "open": 7935, - "volume": 307411 - }, - "id": 2575214, - "non_hive": { - "close": 6799, - "high": 1711, - "low": 34170, - "open": 1711, - "volume": 62665 - }, - "open": "2020-04-03T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2438, - "high": 1498, - "low": 11, - "open": 61636, - "volume": 200944 - }, - "id": 2575240, - "non_hive": { - "close": 525, - "high": 323, - "low": 2, - "open": 12096, - "volume": 40729 - }, - "open": "2020-04-03T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 505, - "high": 3744, - "low": 37, - "open": 45684, - "volume": 139469 - }, - "id": 2575272, - "non_hive": { - "close": 108, - "high": 807, - "low": 7, - "open": 9822, - "volume": 29654 - }, - "open": "2020-04-03T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1067, - "high": 4, - "low": 334643, - "open": 3148, - "volume": 1345067 - }, - "id": 2575314, - "non_hive": { - "close": 221, - "high": 1, - "low": 68591, - "open": 670, - "volume": 277051 - }, - "open": "2020-04-03T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22892, - "high": 53, - "low": 100000, - "open": 153073, - "volume": 988161 - }, - "id": 2575367, - "non_hive": { - "close": 4670, - "high": 11, - "low": 20297, - "open": 31375, - "volume": 201066 - }, - "open": "2020-04-03T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7676, - "high": 4, - "low": 24165, - "open": 15691, - "volume": 281731 - }, - "id": 2575403, - "non_hive": { - "close": 1566, - "high": 1, - "low": 4929, - "open": 3201, - "volume": 57472 - }, - "open": "2020-04-03T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14142, - "high": 24, - "low": 16353, - "open": 627, - "volume": 131122 - }, - "id": 2575426, - "non_hive": { - "close": 2899, - "high": 5, - "low": 3336, - "open": 128, - "volume": 26853 - }, - "open": "2020-04-03T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6683, - "high": 18320, - "low": 6683, - "open": 18320, - "volume": 25003 - }, - "id": 2575445, - "non_hive": { - "close": 1400, - "high": 3839, - "low": 1400, - "open": 3839, - "volume": 5239 - }, - "open": "2020-04-03T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 552, - "high": 4, - "low": 10, - "open": 4, - "volume": 124041 - }, - "id": 2575452, - "non_hive": { - "close": 112, - "high": 1, - "low": 2, - "open": 1, - "volume": 25824 - }, - "open": "2020-04-03T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1097, - "high": 23, - "low": 404, - "open": 1590, - "volume": 146230 - }, - "id": 2575476, - "non_hive": { - "close": 226, - "high": 5, - "low": 82, - "open": 331, - "volume": 29712 - }, - "open": "2020-04-03T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7961, - "high": 7961, - "low": 32326, - "open": 33417, - "volume": 133345 - }, - "id": 2575496, - "non_hive": { - "close": 1664, - "high": 1664, - "low": 6755, - "open": 6983, - "volume": 27865 - }, - "open": "2020-04-03T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2803, - "high": 2803, - "low": 40, - "open": 40, - "volume": 14792 - }, - "id": 2575511, - "non_hive": { - "close": 588, - "high": 588, - "low": 8, - "open": 8, - "volume": 3093 - }, - "open": "2020-04-04T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 77, - "high": 58862, - "low": 386989, - "open": 58862, - "volume": 811838 - }, - "id": 2575522, - "non_hive": { - "close": 16, - "high": 12345, - "low": 78547, - "open": 12345, - "volume": 166035 - }, - "open": "2020-04-04T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7342, - "high": 531, - "low": 26, - "open": 29888, - "volume": 589996 - }, - "id": 2575544, - "non_hive": { - "close": 1520, - "high": 110, - "low": 5, - "open": 6187, - "volume": 119673 - }, - "open": "2020-04-04T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 4, - "low": 5, - "open": 48, - "volume": 1030323 - }, - "id": 2575581, - "non_hive": { - "close": 2, - "high": 1, - "low": 1, - "open": 10, - "volume": 208323 - }, - "open": "2020-04-04T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3213, - "high": 3213, - "low": 1812, - "open": 1812, - "volume": 5025 - }, - "id": 2575624, - "non_hive": { - "close": 660, - "high": 660, - "low": 372, - "open": 372, - "volume": 1032 - }, - "open": "2020-04-04T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4594, - "high": 150, - "low": 10527, - "open": 472, - "volume": 81033 - }, - "id": 2575630, - "non_hive": { - "close": 948, - "high": 31, - "low": 2109, - "open": 97, - "volume": 16487 - }, - "open": "2020-04-04T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 318, - "high": 4, - "low": 90, - "open": 5798, - "volume": 42082 - }, - "id": 2575652, - "non_hive": { - "close": 64, - "high": 1, - "low": 18, - "open": 1196, - "volume": 8740 - }, - "open": "2020-04-04T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16, - "high": 1055, - "low": 16, - "open": 10492, - "volume": 34771 - }, - "id": 2575672, - "non_hive": { - "close": 3, - "high": 218, - "low": 3, - "open": 2144, - "volume": 7157 - }, - "open": "2020-04-04T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8367, - "high": 4, - "low": 46, - "open": 818, - "volume": 32299 - }, - "id": 2575686, - "non_hive": { - "close": 1727, - "high": 1, - "low": 9, - "open": 169, - "volume": 6669 - }, - "open": "2020-04-04T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9, - "high": 4, - "low": 10, - "open": 13877, - "volume": 105173 - }, - "id": 2575716, - "non_hive": { - "close": 2, - "high": 1, - "low": 2, - "open": 2864, - "volume": 21707 - }, - "open": "2020-04-04T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1980, - "high": 9, - "low": 25, - "open": 25, - "volume": 1389501 - }, - "id": 2575742, - "non_hive": { - "close": 408, - "high": 2, - "low": 5, - "open": 5, - "volume": 286214 - }, - "open": "2020-04-04T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6534, - "high": 82, - "low": 6534, - "open": 1029, - "volume": 144537 - }, - "id": 2575777, - "non_hive": { - "close": 1335, - "high": 17, - "low": 1335, - "open": 212, - "volume": 29628 - }, - "open": "2020-04-04T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2474, - "high": 4, - "low": 73328, - "open": 636, - "volume": 162801 - }, - "id": 2575801, - "non_hive": { - "close": 505, - "high": 1, - "low": 14694, - "open": 130, - "volume": 32892 - }, - "open": "2020-04-04T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 620, - "high": 6292, - "low": 85937, - "open": 100000, - "volume": 3705664 - }, - "id": 2575828, - "non_hive": { - "close": 124, - "high": 1284, - "low": 16924, - "open": 20200, - "volume": 738188 - }, - "open": "2020-04-04T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50086, - "high": 5, - "low": 10655, - "open": 59093, - "volume": 397324 - }, - "id": 2575870, - "non_hive": { - "close": 10000, - "high": 1, - "low": 2118, - "open": 11807, - "volume": 79355 - }, - "open": "2020-04-04T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 101188, - "high": 75, - "low": 11, - "open": 10453, - "volume": 547951 - }, - "id": 2575892, - "non_hive": { - "close": 20187, - "high": 15, - "low": 2, - "open": 2087, - "volume": 109337 - }, - "open": "2020-04-04T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 684548, - "high": 170, - "low": 684548, - "open": 170, - "volume": 1092440 - }, - "id": 2575926, - "non_hive": { - "close": 136479, - "high": 34, - "low": 136479, - "open": 34, - "volume": 217854 - }, - "open": "2020-04-04T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1664, - "high": 19, - "low": 132, - "open": 10684, - "volume": 395702 - }, - "id": 2575959, - "non_hive": { - "close": 341, - "high": 4, - "low": 26, - "open": 2127, - "volume": 78930 - }, - "open": "2020-04-04T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26, - "high": 2318, - "low": 26, - "open": 4227, - "volume": 644560 - }, - "id": 2576000, - "non_hive": { - "close": 5, - "high": 475, - "low": 5, - "open": 833, - "volume": 127483 - }, - "open": "2020-04-04T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22681, - "high": 4325, - "low": 49, - "open": 3702, - "volume": 224893 - }, - "id": 2576027, - "non_hive": { - "close": 4644, - "high": 886, - "low": 9, - "open": 758, - "volume": 46046 - }, - "open": "2020-04-04T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4476, - "high": 1426, - "low": 31, - "open": 9935, - "volume": 184190 - }, - "id": 2576054, - "non_hive": { - "close": 914, - "high": 292, - "low": 6, - "open": 2034, - "volume": 37629 - }, - "open": "2020-04-04T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 4, - "low": 11, - "open": 4, - "volume": 33761 - }, - "id": 2576086, - "non_hive": { - "close": 2, - "high": 1, - "low": 2, - "open": 1, - "volume": 6873 - }, - "open": "2020-04-04T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 98, - "high": 4, - "low": 98, - "open": 417, - "volume": 108465 - }, - "id": 2576112, - "non_hive": { - "close": 19, - "high": 1, - "low": 19, - "open": 82, - "volume": 22062 - }, - "open": "2020-04-04T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20082, - "high": 4, - "low": 42, - "open": 1577, - "volume": 500325 - }, - "id": 2576149, - "non_hive": { - "close": 4087, - "high": 1, - "low": 8, - "open": 321, - "volume": 101845 - }, - "open": "2020-04-04T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 84, - "open": 4, - "volume": 28091 - }, - "id": 2576168, - "non_hive": { - "close": 1, - "high": 1, - "low": 17, - "open": 1, - "volume": 5719 - }, - "open": "2020-04-05T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34, - "high": 4, - "low": 5, - "open": 5, - "volume": 90368 - }, - "id": 2576197, - "non_hive": { - "close": 7, - "high": 1, - "low": 1, - "open": 1, - "volume": 18397 - }, - "open": "2020-04-05T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 628, - "high": 4, - "low": 375, - "open": 4, - "volume": 26529 - }, - "id": 2576232, - "non_hive": { - "close": 128, - "high": 1, - "low": 76, - "open": 1, - "volume": 5401 - }, - "open": "2020-04-05T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 4, - "low": 10, - "open": 4, - "volume": 5588 - }, - "id": 2576267, - "non_hive": { - "close": 3, - "high": 1, - "low": 2, - "open": 1, - "volume": 1138 - }, - "open": "2020-04-05T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2050, - "high": 4, - "low": 7424, - "open": 4, - "volume": 710912 - }, - "id": 2576281, - "non_hive": { - "close": 410, - "high": 1, - "low": 1477, - "open": 1, - "volume": 141836 - }, - "open": "2020-04-05T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2940, - "high": 55, - "low": 28731, - "open": 55, - "volume": 598374 - }, - "id": 2576312, - "non_hive": { - "close": 588, - "high": 11, - "low": 5632, - "open": 11, - "volume": 118717 - }, - "open": "2020-04-05T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17491, - "high": 5, - "low": 13, - "open": 20580, - "volume": 560303 - }, - "id": 2576346, - "non_hive": { - "close": 3498, - "high": 1, - "low": 2, - "open": 4075, - "volume": 110664 - }, - "open": "2020-04-05T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111, - "high": 10, - "low": 505130, - "open": 16099, - "volume": 1071173 - }, - "id": 2576386, - "non_hive": { - "close": 22, - "high": 2, - "low": 98990, - "open": 3202, - "volume": 210314 - }, - "open": "2020-04-05T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 138421, - "high": 25, - "low": 14599, - "open": 476, - "volume": 691544 - }, - "id": 2576413, - "non_hive": { - "close": 26846, - "high": 5, - "low": 2831, - "open": 94, - "volume": 134386 - }, - "open": "2020-04-05T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 6437, - "low": 11, - "open": 1248, - "volume": 164561 - }, - "id": 2576437, - "non_hive": { - "close": 2, - "high": 1277, - "low": 2, - "open": 246, - "volume": 32212 - }, - "open": "2020-04-05T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2702, - "high": 6660, - "low": 99856, - "open": 26, - "volume": 1219549 - }, - "id": 2576467, - "non_hive": { - "close": 529, - "high": 1316, - "low": 18972, - "open": 5, - "volume": 232705 - }, - "open": "2020-04-05T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1560, - "high": 86, - "low": 52936, - "open": 462, - "volume": 272345 - }, - "id": 2576481, - "non_hive": { - "close": 298, - "high": 17, - "low": 10100, - "open": 90, - "volume": 52694 - }, - "open": "2020-04-05T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5244, - "high": 68, - "low": 23842, - "open": 3232, - "volume": 687992 - }, - "id": 2576511, - "non_hive": { - "close": 1001, - "high": 13, - "low": 4506, - "open": 617, - "volume": 130412 - }, - "open": "2020-04-05T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4820, - "high": 5060, - "low": 5561, - "open": 183, - "volume": 25802 - }, - "id": 2576538, - "non_hive": { - "close": 940, - "high": 1000, - "low": 1056, - "open": 35, - "volume": 4964 - }, - "open": "2020-04-05T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 163376, - "high": 546, - "low": 10, - "open": 4947, - "volume": 801392 - }, - "id": 2576566, - "non_hive": { - "close": 31205, - "high": 106, - "low": 1, - "open": 940, - "volume": 153657 - }, - "open": "2020-04-05T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 199, - "low": 11, - "open": 199, - "volume": 70658 - }, - "id": 2576604, - "non_hive": { - "close": 2, - "high": 38, - "low": 2, - "open": 38, - "volume": 13449 - }, - "open": "2020-04-05T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14522, - "high": 18220, - "low": 4800, - "open": 10296, - "volume": 926090 - }, - "id": 2576629, - "non_hive": { - "close": 2888, - "high": 3624, - "low": 915, - "open": 1964, - "volume": 177622 - }, - "open": "2020-04-05T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 164, - "high": 11213, - "low": 64, - "open": 10760, - "volume": 1971041 - }, - "id": 2576668, - "non_hive": { - "close": 31, - "high": 2218, - "low": 12, - "open": 2119, - "volume": 375459 - }, - "open": "2020-04-05T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3131, - "high": 15576, - "low": 1144, - "open": 15576, - "volume": 1033710 - }, - "id": 2576695, - "non_hive": { - "close": 606, - "high": 3081, - "low": 219, - "open": 3081, - "volume": 201492 - }, - "open": "2020-04-05T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6345, - "high": 6345, - "low": 4000, - "open": 124, - "volume": 20887 - }, - "id": 2576727, - "non_hive": { - "close": 1229, - "high": 1229, - "low": 770, - "open": 24, - "volume": 4039 - }, - "open": "2020-04-05T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1408, - "high": 20, - "low": 414359, - "open": 16979, - "volume": 1052901 - }, - "id": 2576740, - "non_hive": { - "close": 273, - "high": 4, - "low": 77803, - "open": 3294, - "volume": 198604 - }, - "open": "2020-04-05T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 10601, - "low": 11, - "open": 11, - "volume": 680154 - }, - "id": 2576771, - "non_hive": { - "close": 2, - "high": 2051, - "low": 2, - "open": 2, - "volume": 127247 - }, - "open": "2020-04-05T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 277, - "high": 277, - "low": 68, - "open": 2773, - "volume": 89194 - }, - "id": 2576787, - "non_hive": { - "close": 53, - "high": 53, - "low": 12, - "open": 518, - "volume": 16913 - }, - "open": "2020-04-05T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4169, - "high": 3597, - "low": 456, - "open": 456, - "volume": 14293 - }, - "id": 2576809, - "non_hive": { - "close": 788, - "high": 680, - "low": 84, - "open": 84, - "volume": 2699 - }, - "open": "2020-04-05T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8592, - "high": 3095, - "low": 444, - "open": 3095, - "volume": 2898945 - }, - "id": 2576827, - "non_hive": { - "close": 1623, - "high": 585, - "low": 79, - "open": 585, - "volume": 532804 - }, - "open": "2020-04-06T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45568, - "high": 10150, - "low": 28, - "open": 11844, - "volume": 127770 - }, - "id": 2576858, - "non_hive": { - "close": 8000, - "high": 1916, - "low": 4, - "open": 2227, - "volume": 23216 - }, - "open": "2020-04-06T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 572, - "high": 572, - "low": 178, - "open": 178, - "volume": 116378 - }, - "id": 2576883, - "non_hive": { - "close": 108, - "high": 108, - "low": 31, - "open": 31, - "volume": 21192 - }, - "open": "2020-04-06T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6256, - "high": 1690, - "low": 6256, - "open": 8700, - "volume": 20752 - }, - "id": 2576907, - "non_hive": { - "close": 1175, - "high": 319, - "low": 1175, - "open": 1642, - "volume": 3911 - }, - "open": "2020-04-06T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15, - "high": 15, - "low": 11, - "open": 2140, - "volume": 90576 - }, - "id": 2576919, - "non_hive": { - "close": 3, - "high": 3, - "low": 2, - "open": 402, - "volume": 17010 - }, - "open": "2020-04-06T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 79, - "high": 51633, - "low": 79, - "open": 24985, - "volume": 228746 - }, - "id": 2576936, - "non_hive": { - "close": 14, - "high": 9737, - "low": 14, - "open": 4694, - "volume": 43066 - }, - "open": "2020-04-06T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 77801, - "high": 77801, - "low": 102, - "open": 25000, - "volume": 46885646 - }, - "id": 2576954, - "non_hive": { - "close": 33463, - "high": 33463, - "low": 19, - "open": 4682, - "volume": 15166401 - }, - "open": "2020-04-06T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5011, - "high": 800, - "low": 4260, - "open": 8286, - "volume": 12098367 - }, - "id": 2577000, - "non_hive": { - "close": 957, - "high": 270, - "low": 800, - "open": 1995, - "volume": 2662820 - }, - "open": "2020-04-06T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7653, - "high": 20, - "low": 44, - "open": 5061, - "volume": 204460 - }, - "id": 2577097, - "non_hive": { - "close": 1910, - "high": 5, - "low": 8, - "open": 967, - "volume": 44076 - }, - "open": "2020-04-06T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 169, - "high": 169, - "low": 5966, - "open": 2900, - "volume": 3648873 - }, - "id": 2577172, - "non_hive": { - "close": 44, - "high": 44, - "low": 1252, - "open": 638, - "volume": 824284 - }, - "open": "2020-04-06T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1606, - "high": 6514, - "low": 10, - "open": 5570, - "volume": 5934084 - }, - "id": 2577229, - "non_hive": { - "close": 337, - "high": 1759, - "low": 2, - "open": 1448, - "volume": 1275364 - }, - "open": "2020-04-06T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21, - "high": 1586, - "low": 21, - "open": 1622, - "volume": 6584930 - }, - "id": 2577327, - "non_hive": { - "close": 3, - "high": 357, - "low": 3, - "open": 341, - "volume": 1196646 - }, - "open": "2020-04-06T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9495, - "high": 1002, - "low": 830, - "open": 8152, - "volume": 215156 - }, - "id": 2577433, - "non_hive": { - "close": 1899, - "high": 220, - "low": 135, - "open": 1630, - "volume": 44217 - }, - "open": "2020-04-06T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 165, - "high": 3057, - "low": 24000, - "open": 6018, - "volume": 718099 - }, - "id": 2577481, - "non_hive": { - "close": 36, - "high": 669, - "low": 4102, - "open": 1263, - "volume": 146308 - }, - "open": "2020-04-06T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 396, - "high": 32, - "low": 29258, - "open": 25462, - "volume": 1047710 - }, - "id": 2577525, - "non_hive": { - "close": 79, - "high": 7, - "low": 5563, - "open": 5525, - "volume": 211955 - }, - "open": "2020-04-06T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9237, - "high": 4, - "low": 186, - "open": 8773, - "volume": 1773909 - }, - "id": 2577601, - "non_hive": { - "close": 1958, - "high": 1, - "low": 37, - "open": 1840, - "volume": 372072 - }, - "open": "2020-04-06T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11085, - "high": 23, - "low": 56, - "open": 9143, - "volume": 3088366 - }, - "id": 2577680, - "non_hive": { - "close": 2328, - "high": 5, - "low": 11, - "open": 1938, - "volume": 639584 - }, - "open": "2020-04-06T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 603, - "high": 47, - "low": 11385, - "open": 927000, - "volume": 1631318 - }, - "id": 2577736, - "non_hive": { - "close": 128, - "high": 10, - "low": 2334, - "open": 194670, - "volume": 343293 - }, - "open": "2020-04-06T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90, - "high": 636, - "low": 310988, - "open": 636, - "volume": 1731108 - }, - "id": 2577772, - "non_hive": { - "close": 18, - "high": 128, - "low": 59294, - "open": 128, - "volume": 340601 - }, - "open": "2020-04-06T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3600, - "high": 3600, - "low": 36, - "open": 5702, - "volume": 244497 - }, - "id": 2577830, - "non_hive": { - "close": 762, - "high": 762, - "low": 7, - "open": 1134, - "volume": 50993 - }, - "open": "2020-04-06T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 938, - "high": 226, - "low": 153, - "open": 5755, - "volume": 1249786 - }, - "id": 2577866, - "non_hive": { - "close": 190, - "high": 48, - "low": 30, - "open": 1218, - "volume": 262305 - }, - "open": "2020-04-06T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37, - "high": 4289, - "low": 37, - "open": 4289, - "volume": 714136 - }, - "id": 2577918, - "non_hive": { - "close": 7, - "high": 903, - "low": 7, - "open": 903, - "volume": 141111 - }, - "open": "2020-04-06T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2114, - "high": 6644, - "low": 11, - "open": 74, - "volume": 1757427 - }, - "id": 2578025, - "non_hive": { - "close": 425, - "high": 1390, - "low": 2, - "open": 15, - "volume": 350953 - }, - "open": "2020-04-06T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111, - "high": 4, - "low": 402, - "open": 198743, - "volume": 1720658 - }, - "id": 2578065, - "non_hive": { - "close": 22, - "high": 1, - "low": 79, - "open": 39550, - "volume": 343457 - }, - "open": "2020-04-06T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 109380, - "high": 4991, - "low": 9, - "open": 198, - "volume": 137018 - }, - "id": 2578094, - "non_hive": { - "close": 21525, - "high": 1022, - "low": 1, - "open": 40, - "volume": 27130 - }, - "open": "2020-04-07T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60851, - "high": 49, - "low": 42, - "open": 272628, - "volume": 1222694 - }, - "id": 2578139, - "non_hive": { - "close": 12359, - "high": 10, - "low": 8, - "open": 53653, - "volume": 237697 - }, - "open": "2020-04-07T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1487, - "high": 1487, - "low": 1487, - "open": 1487, - "volume": 1487 - }, - "id": 2578160, - "non_hive": { - "close": 299, - "high": 299, - "low": 299, - "open": 299, - "volume": 299 - }, - "open": "2020-04-07T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 201, - "high": 201, - "low": 318628, - "open": 318628, - "volume": 318829 - }, - "id": 2578164, - "non_hive": { - "close": 41, - "high": 41, - "low": 63313, - "open": 63313, - "volume": 63354 - }, - "open": "2020-04-07T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 732, - "low": 603191, - "open": 10859, - "volume": 2333787 - }, - "id": 2578171, - "non_hive": { - "close": 2, - "high": 150, - "low": 115169, - "open": 2206, - "volume": 447056 - }, - "open": "2020-04-07T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 221847, - "high": 268, - "low": 18583, - "open": 10001, - "volume": 866362 - }, - "id": 2578192, - "non_hive": { - "close": 42991, - "high": 55, - "low": 3553, - "open": 2048, - "volume": 170032 - }, - "open": "2020-04-07T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3191, - "high": 3191, - "low": 6400, - "open": 20126, - "volume": 38201 - }, - "id": 2578236, - "non_hive": { - "close": 632, - "high": 632, - "low": 1267, - "open": 3985, - "volume": 7564 - }, - "open": "2020-04-07T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13000, - "high": 4, - "low": 93, - "open": 10, - "volume": 2600782 - }, - "id": 2578252, - "non_hive": { - "close": 2626, - "high": 1, - "low": 18, - "open": 2, - "volume": 523780 - }, - "open": "2020-04-07T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3361, - "high": 1158, - "low": 40, - "open": 1158, - "volume": 112332 - }, - "id": 2578294, - "non_hive": { - "close": 684, - "high": 237, - "low": 7, - "open": 237, - "volume": 22606 - }, - "open": "2020-04-07T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1748, - "high": 39, - "low": 3403, - "open": 3126, - "volume": 169086 - }, - "id": 2578339, - "non_hive": { - "close": 355, - "high": 8, - "low": 674, - "open": 636, - "volume": 33899 - }, - "open": "2020-04-07T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12679, - "high": 773, - "low": 11, - "open": 773, - "volume": 881046 - }, - "id": 2578380, - "non_hive": { - "close": 2469, - "high": 157, - "low": 2, - "open": 157, - "volume": 171840 - }, - "open": "2020-04-07T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 126102, - "high": 126102, - "low": 2779, - "open": 14644, - "volume": 281556 - }, - "id": 2578415, - "non_hive": { - "close": 24964, - "high": 24964, - "low": 531, - "open": 2870, - "volume": 55034 - }, - "open": "2020-04-07T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 79, - "high": 12352, - "low": 79, - "open": 196080, - "volume": 1090471 - }, - "id": 2578466, - "non_hive": { - "close": 15, - "high": 2442, - "low": 15, - "open": 38421, - "volume": 211767 - }, - "open": "2020-04-07T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54574, - "high": 5094, - "low": 296, - "open": 28642, - "volume": 4714345 - }, - "id": 2578519, - "non_hive": { - "close": 10641, - "high": 1002, - "low": 54, - "open": 5529, - "volume": 890729 - }, - "open": "2020-04-07T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59096, - "high": 9, - "low": 49, - "open": 113, - "volume": 4216303 - }, - "id": 2578576, - "non_hive": { - "close": 11582, - "high": 2, - "low": 8, - "open": 22, - "volume": 801329 - }, - "open": "2020-04-07T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50134, - "high": 344, - "low": 30000, - "open": 118, - "volume": 3148088 - }, - "id": 2578631, - "non_hive": { - "close": 9976, - "high": 70, - "low": 5734, - "open": 23, - "volume": 615301 - }, - "open": "2020-04-07T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6202, - "high": 1728, - "low": 10, - "open": 1111000, - "volume": 7021257 - }, - "id": 2578699, - "non_hive": { - "close": 1239, - "high": 350, - "low": 1, - "open": 211202, - "volume": 1341444 - }, - "open": "2020-04-07T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4116, - "high": 5898, - "low": 4116, - "open": 132, - "volume": 521752 - }, - "id": 2578769, - "non_hive": { - "close": 791, - "high": 1168, - "low": 791, - "open": 26, - "volume": 101508 - }, - "open": "2020-04-07T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 527754, - "high": 1852, - "low": 2139, - "open": 1852, - "volume": 715262 - }, - "id": 2578809, - "non_hive": { - "close": 99096, - "high": 361, - "low": 401, - "open": 361, - "volume": 135144 - }, - "open": "2020-04-07T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5539, - "high": 654, - "low": 117058, - "open": 117058, - "volume": 248473 - }, - "id": 2578851, - "non_hive": { - "close": 1041, - "high": 125, - "low": 21980, - "open": 21980, - "volume": 46858 - }, - "open": "2020-04-07T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 994, - "high": 994, - "low": 24940, - "open": 4625, - "volume": 230860 - }, - "id": 2578888, - "non_hive": { - "close": 189, - "high": 189, - "low": 4683, - "open": 869, - "volume": 43391 - }, - "open": "2020-04-07T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40116, - "high": 5258, - "low": 114, - "open": 1200000, - "volume": 1276540 - }, - "id": 2578916, - "non_hive": { - "close": 7611, - "high": 1000, - "low": 21, - "open": 225320, - "volume": 239813 - }, - "open": "2020-04-07T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7590, - "high": 1002, - "low": 11, - "open": 5083, - "volume": 130149 - }, - "id": 2578944, - "non_hive": { - "close": 1425, - "high": 198, - "low": 2, - "open": 955, - "volume": 24831 - }, - "open": "2020-04-07T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16794, - "high": 41740, - "low": 405, - "open": 3003, - "volume": 1359454 - }, - "id": 2578988, - "non_hive": { - "close": 3153, - "high": 8344, - "low": 76, - "open": 587, - "volume": 263978 - }, - "open": "2020-04-07T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1200000, - "high": 3715, - "low": 10041, - "open": 74780, - "volume": 3838418 - }, - "id": 2579039, - "non_hive": { - "close": 225320, - "high": 732, - "low": 1885, - "open": 14046, - "volume": 725772 - }, - "open": "2020-04-08T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 51, - "low": 7, - "open": 1200000, - "volume": 3487484 - }, - "id": 2579067, - "non_hive": { - "close": 1, - "high": 10, - "low": 1, - "open": 225320, - "volume": 654927 - }, - "open": "2020-04-08T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 1005, - "low": 800, - "open": 1002, - "volume": 1705811 - }, - "id": 2579095, - "non_hive": { - "close": 194, - "high": 196, - "low": 151, - "open": 193, - "volume": 331963 - }, - "open": "2020-04-08T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5155, - "high": 634, - "low": 37, - "open": 1000, - "volume": 3941225 - }, - "id": 2579122, - "non_hive": { - "close": 1001, - "high": 126, - "low": 6, - "open": 194, - "volume": 739722 - }, - "open": "2020-04-08T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17307, - "high": 1397, - "low": 582784, - "open": 5466, - "volume": 10836651 - }, - "id": 2579158, - "non_hive": { - "close": 3236, - "high": 269, - "low": 75761, - "open": 1001, - "volume": 1654071 - }, - "open": "2020-04-08T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 5, - "low": 290423, - "open": 18428, - "volume": 12173528 - }, - "id": 2579199, - "non_hive": { - "close": 1, - "high": 1, - "low": 38248, - "open": 3429, - "volume": 1944067 - }, - "open": "2020-04-08T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 124794, - "high": 5500, - "low": 349, - "open": 1906, - "volume": 3913324 - }, - "id": 2579287, - "non_hive": { - "close": 20022, - "high": 962, - "low": 55, - "open": 332, - "volume": 654112 - }, - "open": "2020-04-08T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1276224, - "high": 5, - "low": 91, - "open": 20000, - "volume": 7848000 - }, - "id": 2579324, - "non_hive": { - "close": 222958, - "high": 1, - "low": 15, - "open": 3400, - "volume": 1341134 - }, - "open": "2020-04-08T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 11861, - "low": 565993, - "open": 11861, - "volume": 3631501 - }, - "id": 2579365, - "non_hive": { - "close": 100860, - "high": 2205, - "low": 94634, - "open": 2205, - "volume": 618038 - }, - "open": "2020-04-08T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600261, - "high": 356591, - "low": 133241, - "open": 511111, - "volume": 6023045 - }, - "id": 2579401, - "non_hive": { - "close": 104325, - "high": 62341, - "low": 22280, - "open": 86480, - "volume": 1025971 - }, - "open": "2020-04-08T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 216, - "low": 12, - "open": 600000, - "volume": 6800588 - }, - "id": 2579441, - "non_hive": { - "close": 2, - "high": 39, - "low": 2, - "open": 100860, - "volume": 1166859 - }, - "open": "2020-04-08T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51, - "high": 11634, - "low": 51, - "open": 600000, - "volume": 4913249 - }, - "id": 2579487, - "non_hive": { - "close": 8, - "high": 2152, - "low": 8, - "open": 103981, - "volume": 863038 - }, - "open": "2020-04-08T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 21, - "low": 600000, - "open": 490000, - "volume": 3807016 - }, - "id": 2579530, - "non_hive": { - "close": 102001, - "high": 4, - "low": 102001, - "open": 86348, - "volume": 668656 - }, - "open": "2020-04-08T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14419, - "high": 486, - "low": 182160, - "open": 450000, - "volume": 5427668 - }, - "id": 2579561, - "non_hive": { - "close": 2667, - "high": 90, - "low": 30967, - "open": 77490, - "volume": 966135 - }, - "open": "2020-04-08T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 582546, - "high": 554, - "low": 580000, - "open": 453700, - "volume": 3650875 - }, - "id": 2579608, - "non_hive": { - "close": 107422, - "high": 103, - "low": 102084, - "open": 82695, - "volume": 655859 - }, - "open": "2020-04-08T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 593807, - "high": 5158, - "low": 593807, - "open": 20000, - "volume": 5197496 - }, - "id": 2579638, - "non_hive": { - "close": 105838, - "high": 1006, - "low": 105838, - "open": 3711, - "volume": 947338 - }, - "open": "2020-04-08T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 13818, - "low": 12, - "open": 50000, - "volume": 3773059 - }, - "id": 2579667, - "non_hive": { - "close": 2, - "high": 2752, - "low": 2, - "open": 9750, - "volume": 670835 - }, - "open": "2020-04-08T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12544, - "high": 10, - "low": 958, - "open": 12000, - "volume": 4260902 - }, - "id": 2579701, - "non_hive": { - "close": 2195, - "high": 2, - "low": 167, - "open": 2268, - "volume": 752849 - }, - "open": "2020-04-08T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 18655, - "low": 323083, - "open": 600000, - "volume": 4957477 - }, - "id": 2579753, - "non_hive": { - "close": 104100, - "high": 3447, - "low": 55571, - "open": 105000, - "volume": 875472 - }, - "open": "2020-04-08T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49831, - "high": 954, - "low": 213583, - "open": 600000, - "volume": 6463471 - }, - "id": 2579784, - "non_hive": { - "close": 9178, - "high": 176, - "low": 36736, - "open": 107152, - "volume": 1146072 - }, - "open": "2020-04-08T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 400, - "high": 1753, - "low": 204157, - "open": 1753, - "volume": 4138020 - }, - "id": 2579845, - "non_hive": { - "close": 71, - "high": 323, - "low": 36095, - "open": 323, - "volume": 739906 - }, - "open": "2020-04-08T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6835, - "high": 6835, - "low": 245077, - "open": 120, - "volume": 5040494 - }, - "id": 2579884, - "non_hive": { - "close": 1258, - "high": 1258, - "low": 41664, - "open": 21, - "volume": 886166 - }, - "open": "2020-04-08T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39265, - "high": 652, - "low": 12, - "open": 3831, - "volume": 3516491 - }, - "id": 2579923, - "non_hive": { - "close": 7165, - "high": 120, - "low": 2, - "open": 704, - "volume": 622022 - }, - "open": "2020-04-08T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 460560, - "high": 581, - "low": 406, - "open": 133, - "volume": 2810272 - }, - "id": 2579965, - "non_hive": { - "close": 78296, - "high": 107, - "low": 69, - "open": 24, - "volume": 478244 - }, - "open": "2020-04-08T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 159, - "high": 3845, - "low": 70, - "open": 3845, - "volume": 10644 - }, - "id": 2580004, - "non_hive": { - "close": 29, - "high": 706, - "low": 11, - "open": 706, - "volume": 1862 - }, - "open": "2020-04-09T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 132, - "high": 4038, - "low": 42, - "open": 12355, - "volume": 38425 - }, - "id": 2580033, - "non_hive": { - "close": 24, - "high": 736, - "low": 7, - "open": 2250, - "volume": 6988 - }, - "open": "2020-04-09T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10194, - "high": 154, - "low": 42308, - "open": 7594, - "volume": 60250 - }, - "id": 2580052, - "non_hive": { - "close": 1850, - "high": 28, - "low": 7649, - "open": 1373, - "volume": 10900 - }, - "open": "2020-04-09T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16683, - "high": 10073, - "low": 26337, - "open": 10073, - "volume": 279977 - }, - "id": 2580064, - "non_hive": { - "close": 2864, - "high": 1821, - "low": 4521, - "open": 1821, - "volume": 48685 - }, - "open": "2020-04-09T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 234, - "low": 12, - "open": 18861, - "volume": 69807 - }, - "id": 2580090, - "non_hive": { - "close": 2, - "high": 42, - "low": 2, - "open": 3374, - "volume": 12488 - }, - "open": "2020-04-09T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 308, - "high": 3041, - "low": 293109, - "open": 3041, - "volume": 843716 - }, - "id": 2580104, - "non_hive": { - "close": 53, - "high": 529, - "low": 50317, - "open": 529, - "volume": 144866 - }, - "open": "2020-04-09T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19020, - "high": 4769, - "low": 24, - "open": 24, - "volume": 23813 - }, - "id": 2580115, - "non_hive": { - "close": 3340, - "high": 839, - "low": 4, - "open": 4, - "volume": 4183 - }, - "open": "2020-04-09T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 94, - "high": 94, - "low": 94, - "open": 94, - "volume": 94 - }, - "id": 2580124, - "non_hive": { - "close": 16, - "high": 16, - "low": 16, - "open": 16, - "volume": 16 - }, - "open": "2020-04-09T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24388, - "high": 24388, - "low": 30912, - "open": 193, - "volume": 2819301 - }, - "id": 2580128, - "non_hive": { - "close": 4325, - "high": 4325, - "low": 5268, - "open": 33, - "volume": 495332 - }, - "open": "2020-04-09T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3000, - "high": 2169, - "low": 130, - "open": 130, - "volume": 13419 - }, - "id": 2580162, - "non_hive": { - "close": 532, - "high": 390, - "low": 23, - "open": 23, - "volume": 2405 - }, - "open": "2020-04-09T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 44214, - "low": 12, - "open": 17993, - "volume": 393316 - }, - "id": 2580175, - "non_hive": { - "close": 2, - "high": 8002, - "low": 2, - "open": 3190, - "volume": 70395 - }, - "open": "2020-04-09T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39972, - "high": 960536, - "low": 12, - "open": 4511, - "volume": 2043549 - }, - "id": 2580213, - "non_hive": { - "close": 7194, - "high": 175100, - "low": 2, - "open": 822, - "volume": 372234 - }, - "open": "2020-04-09T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42, - "high": 42, - "low": 25415, - "open": 18585, - "volume": 144042 - }, - "id": 2580232, - "non_hive": { - "close": 8, - "high": 8, - "low": 4633, - "open": 3388, - "volume": 26397 - }, - "open": "2020-04-09T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 335, - "high": 162494, - "low": 514, - "open": 449486, - "volume": 1593943 - }, - "id": 2580243, - "non_hive": { - "close": 64, - "high": 31199, - "low": 97, - "open": 84953, - "volume": 304475 - }, - "open": "2020-04-09T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1354, - "high": 539, - "low": 1354, - "open": 539, - "volume": 8175466 - }, - "id": 2580263, - "non_hive": { - "close": 230, - "high": 105, - "low": 230, - "open": 105, - "volume": 1458314 - }, - "open": "2020-04-09T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 122, - "high": 297, - "low": 170964, - "open": 399923, - "volume": 5123649 - }, - "id": 2580307, - "non_hive": { - "close": 22, - "high": 56, - "low": 30099, - "open": 71829, - "volume": 932712 - }, - "open": "2020-04-09T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 790276, - "high": 790276, - "low": 999900, - "open": 3749, - "volume": 3979400 - }, - "id": 2580356, - "non_hive": { - "close": 157197, - "high": 157197, - "low": 179990, - "open": 705, - "volume": 751512 - }, - "open": "2020-04-09T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 697314, - "high": 75, - "low": 3004966, - "open": 3760, - "volume": 6332575 - }, - "id": 2580379, - "non_hive": { - "close": 138974, - "high": 15, - "low": 540927, - "open": 748, - "volume": 1195466 - }, - "open": "2020-04-09T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47, - "high": 60002, - "low": 275, - "open": 49063, - "volume": 1644937 - }, - "id": 2580403, - "non_hive": { - "close": 10, - "high": 12900, - "low": 52, - "open": 9778, - "volume": 320425 - }, - "open": "2020-04-09T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3548, - "high": 3548, - "low": 136986, - "open": 96521, - "volume": 7902047 - }, - "id": 2580432, - "non_hive": { - "close": 693, - "high": 693, - "low": 24698, - "open": 18339, - "volume": 1452782 - }, - "open": "2020-04-09T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 614, - "high": 14582, - "low": 5323630, - "open": 1152, - "volume": 10057304 - }, - "id": 2580452, - "non_hive": { - "close": 131, - "high": 3126, - "low": 958296, - "open": 225, - "volume": 1833437 - }, - "open": "2020-04-09T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 742, - "high": 30, - "low": 81957, - "open": 5031, - "volume": 3718306 - }, - "id": 2580481, - "non_hive": { - "close": 144, - "high": 6, - "low": 14133, - "open": 916, - "volume": 648285 - }, - "open": "2020-04-09T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 10, - "low": 12, - "open": 10, - "volume": 4542373 - }, - "id": 2580524, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 802630 - }, - "open": "2020-04-09T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18888, - "high": 277026, - "low": 376954, - "open": 158350, - "volume": 4630297 - }, - "id": 2580563, - "non_hive": { - "close": 3400, - "high": 52584, - "low": 64466, - "open": 27340, - "volume": 816097 - }, - "open": "2020-04-09T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 5, - "low": 600000, - "open": 233586, - "volume": 4598469 - }, - "id": 2580591, - "non_hive": { - "close": 1, - "high": 1, - "low": 104160, - "open": 42028, - "volume": 806166 - }, - "open": "2020-04-10T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 600000, - "low": 41, - "open": 600000, - "volume": 3600041 - }, - "id": 2580624, - "non_hive": { - "close": 106820, - "high": 106830, - "low": 7, - "open": 104420, - "volume": 633549 - }, - "open": "2020-04-10T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 78, - "low": 599476, - "open": 78, - "volume": 3600602 - }, - "id": 2580646, - "non_hive": { - "close": 109518, - "high": 15, - "low": 106846, - "open": 15, - "volume": 646508 - }, - "open": "2020-04-10T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 114758, - "high": 11564, - "low": 600000, - "open": 600000, - "volume": 3737860 - }, - "id": 2580671, - "non_hive": { - "close": 21909, - "high": 2208, - "low": 108054, - "open": 111353, - "volume": 686415 - }, - "open": "2020-04-10T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 48581, - "low": 12, - "open": 600000, - "volume": 4798556 - }, - "id": 2580696, - "non_hive": { - "close": 2, - "high": 9274, - "low": 2, - "open": 108781, - "volume": 873263 - }, - "open": "2020-04-10T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 462236, - "high": 95560, - "low": 43281, - "open": 2908, - "volume": 3827022 - }, - "id": 2580726, - "non_hive": { - "close": 81355, - "high": 17851, - "low": 7617, - "open": 515, - "volume": 675950 - }, - "open": "2020-04-10T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8762, - "high": 251, - "low": 47363, - "open": 11300, - "volume": 3767921 - }, - "id": 2580751, - "non_hive": { - "close": 1586, - "high": 46, - "low": 8146, - "open": 2000, - "volume": 650993 - }, - "open": "2020-04-10T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 1613, - "low": 90, - "open": 600000, - "volume": 4576887 - }, - "id": 2580787, - "non_hive": { - "close": 101618, - "high": 292, - "low": 15, - "open": 103367, - "volume": 779384 - }, - "open": "2020-04-10T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 19360, - "low": 322710, - "open": 19360, - "volume": 3291825 - }, - "id": 2580813, - "non_hive": { - "close": 96748, - "high": 3388, - "low": 52035, - "open": 3388, - "volume": 548793 - }, - "open": "2020-04-10T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 13569, - "low": 149, - "open": 600000, - "volume": 3640171 - }, - "id": 2580844, - "non_hive": { - "close": 96747, - "high": 2334, - "low": 24, - "open": 96748, - "volume": 587177 - }, - "open": "2020-04-10T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 560000, - "high": 5782, - "low": 232334, - "open": 1246, - "volume": 3605782 - }, - "id": 2580871, - "non_hive": { - "close": 90160, - "high": 1000, - "low": 37405, - "open": 201, - "volume": 580985 - }, - "open": "2020-04-10T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19502, - "high": 133, - "low": 25, - "open": 600000, - "volume": 3639456 - }, - "id": 2580892, - "non_hive": { - "close": 3296, - "high": 23, - "low": 4, - "open": 96600, - "volume": 588364 - }, - "open": "2020-04-10T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 5917, - "low": 40456, - "open": 19921, - "volume": 4010802 - }, - "id": 2580924, - "non_hive": { - "close": 99347, - "high": 1000, - "low": 6513, - "open": 3287, - "volume": 653317 - }, - "open": "2020-04-10T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 569041, - "high": 5, - "low": 57041, - "open": 173, - "volume": 8674105 - }, - "id": 2580962, - "non_hive": { - "close": 98028, - "high": 1, - "low": 9426, - "open": 29, - "volume": 1491676 - }, - "open": "2020-04-10T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 108, - "high": 23993, - "low": 107553, - "open": 463315, - "volume": 6103212 - }, - "id": 2581034, - "non_hive": { - "close": 19, - "high": 4542, - "low": 17869, - "open": 80377, - "volume": 1073722 - }, - "open": "2020-04-10T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 99827, - "high": 99827, - "low": 198479, - "open": 208163, - "volume": 3471675 - }, - "id": 2581074, - "non_hive": { - "close": 17949, - "high": 17949, - "low": 33056, - "open": 35700, - "volume": 608580 - }, - "open": "2020-04-10T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 5, - "low": 12, - "open": 61, - "volume": 6721744 - }, - "id": 2581116, - "non_hive": { - "close": 1, - "high": 1, - "low": 2, - "open": 11, - "volume": 1261751 - }, - "open": "2020-04-10T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 306, - "high": 1066, - "low": 92574, - "open": 14426, - "volume": 2640357 - }, - "id": 2581158, - "non_hive": { - "close": 58, - "high": 212, - "low": 16703, - "open": 2603, - "volume": 493232 - }, - "open": "2020-04-10T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1148, - "high": 3429, - "low": 1148, - "open": 11664, - "volume": 211858 - }, - "id": 2581197, - "non_hive": { - "close": 218, - "high": 665, - "low": 218, - "open": 2228, - "volume": 40551 - }, - "open": "2020-04-10T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2727, - "high": 377, - "low": 2727, - "open": 377, - "volume": 3104 - }, - "id": 2581213, - "non_hive": { - "close": 528, - "high": 73, - "low": 528, - "open": 73, - "volume": 601 - }, - "open": "2020-04-10T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2379, - "high": 73, - "low": 33, - "open": 33, - "volume": 70774 - }, - "id": 2581220, - "non_hive": { - "close": 452, - "high": 14, - "low": 6, - "open": 6, - "volume": 13444 - }, - "open": "2020-04-10T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19120, - "high": 52947, - "low": 19120, - "open": 1001, - "volume": 2879114 - }, - "id": 2581235, - "non_hive": { - "close": 3480, - "high": 10061, - "low": 3480, - "open": 190, - "volume": 532385 - }, - "open": "2020-04-10T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72571, - "high": 72571, - "low": 12, - "open": 11764, - "volume": 685489 - }, - "id": 2581255, - "non_hive": { - "close": 14000, - "high": 14000, - "low": 2, - "open": 2228, - "volume": 122347 - }, - "open": "2020-04-10T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48000, - "high": 1395, - "low": 398, - "open": 398, - "volume": 49793 - }, - "id": 2581280, - "non_hive": { - "close": 8453, - "high": 269, - "low": 70, - "open": 70, - "volume": 8792 - }, - "open": "2020-04-10T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 211262, - "high": 388738, - "low": 4163, - "open": 4163, - "volume": 604163 - }, - "id": 2581290, - "non_hive": { - "close": 38027, - "high": 70000, - "low": 737, - "open": 737, - "volume": 108764 - }, - "open": "2020-04-11T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 657, - "high": 41, - "low": 98064, - "open": 39, - "volume": 1249864 - }, - "id": 2581298, - "non_hive": { - "close": 125, - "high": 8, - "low": 17358, - "open": 7, - "volume": 224700 - }, - "open": "2020-04-11T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 570289, - "high": 29711, - "low": 3953, - "open": 16334, - "volume": 2400000 - }, - "id": 2581316, - "non_hive": { - "close": 100371, - "high": 5348, - "low": 695, - "open": 2940, - "volume": 423623 - }, - "open": "2020-04-11T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 198764, - "high": 576, - "low": 198764, - "open": 576, - "volume": 6467979 - }, - "id": 2581329, - "non_hive": { - "close": 33988, - "high": 106, - "low": 33988, - "open": 106, - "volume": 1137714 - }, - "open": "2020-04-11T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 18743, - "low": 12, - "open": 18743, - "volume": 4082497 - }, - "id": 2581342, - "non_hive": { - "close": 2, - "high": 3445, - "low": 2, - "open": 3445, - "volume": 698246 - }, - "open": "2020-04-11T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11396, - "high": 213426, - "low": 11396, - "open": 113985, - "volume": 4669196 - }, - "id": 2581374, - "non_hive": { - "close": 1891, - "high": 37447, - "low": 1891, - "open": 19446, - "volume": 788177 - }, - "open": "2020-04-11T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 705, - "high": 3605, - "low": 24, - "open": 1000, - "volume": 33156 - }, - "id": 2581398, - "non_hive": { - "close": 123, - "high": 631, - "low": 4, - "open": 170, - "volume": 5778 - }, - "open": "2020-04-11T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 263308, - "high": 2413, - "low": 91, - "open": 57946, - "volume": 2201542 - }, - "id": 2581416, - "non_hive": { - "close": 45016, - "high": 421, - "low": 15, - "open": 9853, - "volume": 371234 - }, - "open": "2020-04-11T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2171, - "high": 4941, - "low": 2517, - "open": 4941, - "volume": 53625 - }, - "id": 2581443, - "non_hive": { - "close": 375, - "high": 859, - "low": 422, - "open": 859, - "volume": 9290 - }, - "open": "2020-04-11T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 262, - "high": 346, - "low": 542153, - "open": 67779, - "volume": 7081629 - }, - "id": 2581466, - "non_hive": { - "close": 46, - "high": 61, - "low": 89997, - "open": 11387, - "volume": 1213546 - }, - "open": "2020-04-11T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 561952, - "high": 74, - "low": 1000, - "open": 1000, - "volume": 3381656 - }, - "id": 2581509, - "non_hive": { - "close": 105647, - "high": 14, - "low": 180, - "open": 180, - "volume": 633667 - }, - "open": "2020-04-11T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 192272, - "high": 1507, - "low": 192272, - "open": 437542, - "volume": 1109574 - }, - "id": 2581533, - "non_hive": { - "close": 34039, - "high": 285, - "low": 34039, - "open": 82258, - "volume": 202083 - }, - "open": "2020-04-11T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7589, - "high": 120143, - "low": 599660, - "open": 6889, - "volume": 1629507 - }, - "id": 2581554, - "non_hive": { - "close": 1404, - "high": 22698, - "low": 105000, - "open": 1300, - "volume": 289270 - }, - "open": "2020-04-11T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2092, - "high": 1172, - "low": 496886, - "open": 1172, - "volume": 3639230 - }, - "id": 2581590, - "non_hive": { - "close": 385, - "high": 217, - "low": 87037, - "open": 217, - "volume": 639077 - }, - "open": "2020-04-11T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1949, - "high": 5, - "low": 595785, - "open": 1897, - "volume": 1936056 - }, - "id": 2581627, - "non_hive": { - "close": 356, - "high": 1, - "low": 104464, - "open": 349, - "volume": 341061 - }, - "open": "2020-04-11T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 222, - "high": 5, - "low": 262433, - "open": 3274, - "volume": 1924662 - }, - "id": 2581661, - "non_hive": { - "close": 40, - "high": 1, - "low": 45208, - "open": 598, - "volume": 334053 - }, - "open": "2020-04-11T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 260, - "high": 5, - "low": 12, - "open": 21403, - "volume": 5898137 - }, - "id": 2581716, - "non_hive": { - "close": 45, - "high": 1, - "low": 2, - "open": 3745, - "volume": 1020288 - }, - "open": "2020-04-11T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 77360, - "high": 232, - "low": 600000, - "open": 299690, - "volume": 13093327 - }, - "id": 2581765, - "non_hive": { - "close": 11604, - "high": 41, - "low": 80400, - "open": 51556, - "volume": 2084903 - }, - "open": "2020-04-11T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 646203, - "high": 41, - "low": 131927, - "open": 22443, - "volume": 6454845 - }, - "id": 2581833, - "non_hive": { - "close": 102100, - "high": 7, - "low": 20712, - "open": 3562, - "volume": 1035131 - }, - "open": "2020-04-11T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 229, - "high": 36, - "low": 279051, - "open": 47000, - "volume": 3701654 - }, - "id": 2581890, - "non_hive": { - "close": 38, - "high": 6, - "low": 36391, - "open": 7427, - "volume": 546303 - }, - "open": "2020-04-11T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 267151, - "high": 6, - "low": 37577, - "open": 600000, - "volume": 5004746 - }, - "id": 2581927, - "non_hive": { - "close": 43118, - "high": 1, - "low": 5840, - "open": 96005, - "volume": 793608 - }, - "open": "2020-04-11T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 367544, - "high": 6, - "low": 99773, - "open": 445585, - "volume": 3865878 - }, - "id": 2581958, - "non_hive": { - "close": 52948, - "high": 1, - "low": 14373, - "open": 70000, - "volume": 578534 - }, - "open": "2020-04-11T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 6, - "low": 8, - "open": 520091, - "volume": 5367410 - }, - "id": 2582001, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 75008, - "volume": 755492 - }, - "open": "2020-04-11T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30, - "high": 6, - "low": 300000, - "open": 590000, - "volume": 3600543 - }, - "id": 2582043, - "non_hive": { - "close": 5, - "high": 1, - "low": 39636, - "open": 91450, - "volume": 530797 - }, - "open": "2020-04-11T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299997, - "high": 6, - "low": 76, - "open": 300000, - "volume": 3600100 - }, - "id": 2582068, - "non_hive": { - "close": 39788, - "high": 1, - "low": 10, - "open": 39640, - "volume": 476300 - }, - "open": "2020-04-12T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 12, - "low": 38, - "open": 49982, - "volume": 3892562 - }, - "id": 2582100, - "non_hive": { - "close": 2, - "high": 2, - "low": 5, - "open": 7941, - "volume": 524129 - }, - "open": "2020-04-12T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 168, - "high": 18, - "low": 249997, - "open": 300003, - "volume": 3610995 - }, - "id": 2582137, - "non_hive": { - "close": 27, - "high": 3, - "low": 33161, - "open": 39964, - "volume": 482301 - }, - "open": "2020-04-12T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18, - "high": 18, - "low": 300000, - "open": 47390, - "volume": 3669924 - }, - "id": 2582187, - "non_hive": { - "close": 3, - "high": 3, - "low": 39630, - "open": 7582, - "volume": 489593 - }, - "open": "2020-04-12T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8, - "high": 12, - "low": 8, - "open": 300003, - "volume": 3641430 - }, - "id": 2582231, - "non_hive": { - "close": 1, - "high": 2, - "low": 1, - "open": 40561, - "volume": 486941 - }, - "open": "2020-04-12T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299997, - "high": 6, - "low": 299997, - "open": 88, - "volume": 3600192 - }, - "id": 2582272, - "non_hive": { - "close": 40200, - "high": 1, - "low": 39740, - "open": 14, - "volume": 479760 - }, - "open": "2020-04-12T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7321, - "high": 7321, - "low": 30, - "open": 50000, - "volume": 5027621 - }, - "id": 2582312, - "non_hive": { - "close": 1093, - "high": 1093, - "low": 4, - "open": 6850, - "volume": 695461 - }, - "open": "2020-04-12T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127332, - "high": 6, - "low": 127332, - "open": 300000, - "volume": 3763294 - }, - "id": 2582353, - "non_hive": { - "close": 17203, - "high": 1, - "low": 17203, - "open": 42006, - "volume": 529335 - }, - "open": "2020-04-12T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33, - "high": 34153, - "low": 253531, - "open": 34153, - "volume": 4991870 - }, - "id": 2582384, - "non_hive": { - "close": 5, - "high": 5330, - "low": 34254, - "open": 5330, - "volume": 700165 - }, - "open": "2020-04-12T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56, - "high": 986, - "low": 192938, - "open": 2689, - "volume": 4864316 - }, - "id": 2582428, - "non_hive": { - "close": 8, - "high": 146, - "low": 26089, - "open": 398, - "volume": 680557 - }, - "open": "2020-04-12T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8, - "high": 2200, - "low": 8, - "open": 7786, - "volume": 3616322 - }, - "id": 2582478, - "non_hive": { - "close": 1, - "high": 319, - "low": 1, - "open": 1053, - "volume": 489123 - }, - "open": "2020-04-12T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 200000, - "high": 149824, - "low": 27, - "open": 30000, - "volume": 7955934 - }, - "id": 2582509, - "non_hive": { - "close": 27073, - "high": 21758, - "low": 3, - "open": 4071, - "volume": 1119044 - }, - "open": "2020-04-12T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 80562, - "high": 80562, - "low": 300000, - "open": 300000, - "volume": 6534168 - }, - "id": 2582545, - "non_hive": { - "close": 11994, - "high": 11994, - "low": 40609, - "open": 40611, - "volume": 917976 - }, - "open": "2020-04-12T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12794, - "high": 10075, - "low": 506425, - "open": 300000, - "volume": 4517404 - }, - "id": 2582583, - "non_hive": { - "close": 1900, - "high": 1500, - "low": 68402, - "open": 41398, - "volume": 629172 - }, - "open": "2020-04-12T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49827, - "high": 45431, - "low": 41951, - "open": 300003, - "volume": 6830946 - }, - "id": 2582619, - "non_hive": { - "close": 7791, - "high": 7125, - "low": 5666, - "open": 42454, - "volume": 996650 - }, - "open": "2020-04-12T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19, - "high": 6, - "low": 600000, - "open": 600000, - "volume": 2382698 - }, - "id": 2582676, - "non_hive": { - "close": 3, - "high": 1, - "low": 89400, - "open": 89400, - "volume": 368207 - }, - "open": "2020-04-12T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 2045, - "low": 7, - "open": 20529, - "volume": 2622596 - }, - "id": 2582709, - "non_hive": { - "close": 1, - "high": 328, - "low": 1, - "open": 3223, - "volume": 416113 - }, - "open": "2020-04-12T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6345, - "high": 18, - "low": 7, - "open": 251, - "volume": 146554 - }, - "id": 2582737, - "non_hive": { - "close": 980, - "high": 3, - "low": 1, - "open": 40, - "volume": 23518 - }, - "open": "2020-04-12T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 133, - "high": 25607, - "low": 7, - "open": 7, - "volume": 761351 - }, - "id": 2582762, - "non_hive": { - "close": 22, - "high": 4250, - "low": 1, - "open": 1, - "volume": 124335 - }, - "open": "2020-04-12T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 199733, - "high": 199733, - "low": 658, - "open": 2629, - "volume": 2422528 - }, - "id": 2582801, - "non_hive": { - "close": 33735, - "high": 33735, - "low": 111, - "open": 444, - "volume": 408935 - }, - "open": "2020-04-12T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34535, - "high": 34535, - "low": 12875, - "open": 26209, - "volume": 92111 - }, - "id": 2582829, - "non_hive": { - "close": 5871, - "high": 5871, - "low": 2023, - "open": 4424, - "volume": 15334 - }, - "open": "2020-04-12T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7398, - "high": 7398, - "low": 177, - "open": 177, - "volume": 101036 - }, - "id": 2582847, - "non_hive": { - "close": 1316, - "high": 1316, - "low": 30, - "open": 30, - "volume": 17819 - }, - "open": "2020-04-12T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1068, - "high": 1068, - "low": 12, - "open": 73762, - "volume": 75094 - }, - "id": 2582854, - "non_hive": { - "close": 190, - "high": 190, - "low": 2, - "open": 12613, - "volume": 12848 - }, - "open": "2020-04-12T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 73946, - "high": 73946, - "low": 50, - "open": 38728, - "volume": 192541 - }, - "id": 2582866, - "non_hive": { - "close": 12571, - "high": 12571, - "low": 8, - "open": 6506, - "volume": 32573 - }, - "open": "2020-04-12T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42090, - "high": 2960, - "low": 42090, - "open": 12968, - "volume": 58018 - }, - "id": 2582883, - "non_hive": { - "close": 6949, - "high": 512, - "low": 6949, - "open": 2243, - "volume": 9704 - }, - "open": "2020-04-13T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15385, - "high": 15385, - "low": 43, - "open": 42702, - "volume": 429502 - }, - "id": 2582894, - "non_hive": { - "close": 2677, - "high": 2677, - "low": 7, - "open": 7088, - "volume": 71602 - }, - "open": "2020-04-13T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 510, - "high": 1591, - "low": 510, - "open": 1591, - "volume": 2195901 - }, - "id": 2582924, - "non_hive": { - "close": 83, - "high": 277, - "low": 83, - "open": 277, - "volume": 365212 - }, - "open": "2020-04-13T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 52456, - "high": 5, - "low": 90976, - "open": 24493, - "volume": 389672 - }, - "id": 2582997, - "non_hive": { - "close": 8393, - "high": 1, - "low": 14556, - "open": 4017, - "volume": 64887 - }, - "open": "2020-04-13T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 5, - "low": 1281, - "open": 2256, - "volume": 402292 - }, - "id": 2583019, - "non_hive": { - "close": 1, - "high": 1, - "low": 204, - "open": 361, - "volume": 64373 - }, - "open": "2020-04-13T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25, - "high": 25, - "low": 25, - "open": 25, - "volume": 25 - }, - "id": 2583034, - "non_hive": { - "close": 4, - "high": 4, - "low": 4, - "open": 4, - "volume": 4 - }, - "open": "2020-04-13T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 94, - "high": 94, - "low": 94, - "open": 94, - "volume": 94 - }, - "id": 2583038, - "non_hive": { - "close": 15, - "high": 15, - "low": 15, - "open": 15, - "volume": 15 - }, - "open": "2020-04-13T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 160, - "high": 160, - "low": 93, - "open": 93, - "volume": 49450 - }, - "id": 2583042, - "non_hive": { - "close": 26, - "high": 26, - "low": 15, - "open": 15, - "volume": 8011 - }, - "open": "2020-04-13T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3152, - "high": 11989, - "low": 78973, - "open": 643, - "volume": 3012632 - }, - "id": 2583052, - "non_hive": { - "close": 498, - "high": 1951, - "low": 12477, - "open": 104, - "volume": 480688 - }, - "open": "2020-04-13T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 164, - "high": 31, - "low": 7, - "open": 12347, - "volume": 5381259 - }, - "id": 2583072, - "non_hive": { - "close": 26, - "high": 5, - "low": 1, - "open": 1951, - "volume": 840418 - }, - "open": "2020-04-13T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33063, - "high": 345668, - "low": 49054, - "open": 612, - "volume": 7083055 - }, - "id": 2583118, - "non_hive": { - "close": 5270, - "high": 56102, - "low": 7091, - "open": 97, - "volume": 1125488 - }, - "open": "2020-04-13T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 493887, - "high": 680, - "low": 10, - "open": 775, - "volume": 2725699 - }, - "id": 2583171, - "non_hive": { - "close": 78112, - "high": 115, - "low": 1, - "open": 127, - "volume": 438283 - }, - "open": "2020-04-13T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49843, - "high": 21775, - "low": 382377, - "open": 49994, - "volume": 4603470 - }, - "id": 2583194, - "non_hive": { - "close": 7975, - "high": 3546, - "low": 55291, - "open": 7906, - "volume": 692599 - }, - "open": "2020-04-13T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 567799, - "high": 63522, - "low": 258025, - "open": 7334, - "volume": 17047628 - }, - "id": 2583229, - "non_hive": { - "close": 100898, - "high": 11288, - "low": 37369, - "open": 1144, - "volume": 2889837 - }, - "open": "2020-04-13T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 292, - "high": 21206, - "low": 11137, - "open": 1805807, - "volume": 8549023 - }, - "id": 2583288, - "non_hive": { - "close": 52, - "high": 3902, - "low": 1871, - "open": 320891, - "volume": 1490960 - }, - "open": "2020-04-13T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 692, - "high": 309, - "low": 170753, - "open": 309, - "volume": 3600698 - }, - "id": 2583336, - "non_hive": { - "close": 115, - "high": 52, - "low": 27349, - "open": 52, - "volume": 597675 - }, - "open": "2020-04-13T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 170760, - "high": 22424, - "low": 150054, - "open": 255345, - "volume": 4959971 - }, - "id": 2583360, - "non_hive": { - "close": 28397, - "high": 3857, - "low": 24047, - "open": 42576, - "volume": 814222 - }, - "open": "2020-04-13T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20, - "high": 1523, - "low": 20, - "open": 5953, - "volume": 3604777 - }, - "id": 2583390, - "non_hive": { - "close": 3, - "high": 262, - "low": 3, - "open": 1000, - "volume": 595732 - }, - "open": "2020-04-13T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 300000, - "high": 13201, - "low": 299997, - "open": 1585, - "volume": 3791201 - }, - "id": 2583414, - "non_hive": { - "close": 49575, - "high": 2263, - "low": 49559, - "open": 262, - "volume": 626826 - }, - "open": "2020-04-13T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 440211, - "high": 182451, - "low": 21724, - "open": 300003, - "volume": 7070502 - }, - "id": 2583438, - "non_hive": { - "close": 71403, - "high": 31545, - "low": 3523, - "open": 49577, - "volume": 1159396 - }, - "open": "2020-04-13T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 288976, - "high": 5, - "low": 199790, - "open": 87, - "volume": 4156137 - }, - "id": 2583522, - "non_hive": { - "close": 46875, - "high": 1, - "low": 32406, - "open": 15, - "volume": 677419 - }, - "open": "2020-04-13T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 413741, - "low": 7, - "open": 300003, - "volume": 9298364 - }, - "id": 2583562, - "non_hive": { - "close": 1, - "high": 71314, - "low": 1, - "open": 48665, - "volume": 1498076 - }, - "open": "2020-04-13T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 199600, - "high": 1974, - "low": 401, - "open": 460534, - "volume": 3623777 - }, - "id": 2583612, - "non_hive": { - "close": 31193, - "high": 340, - "low": 62, - "open": 71313, - "volume": 563890 - }, - "open": "2020-04-13T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 49994, - "low": 565531, - "open": 49994, - "volume": 4428756 - }, - "id": 2583637, - "non_hive": { - "close": 87306, - "high": 7843, - "low": 82116, - "open": 7843, - "volume": 668562 - }, - "open": "2020-04-14T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 1500, - "low": 42, - "open": 600000, - "volume": 3601542 - }, - "id": 2583659, - "non_hive": { - "close": 89053, - "high": 246, - "low": 6, - "open": 87665, - "volume": 531872 - }, - "open": "2020-04-14T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 8363, - "low": 600000, - "open": 1500, - "volume": 3608363 - }, - "id": 2583683, - "non_hive": { - "close": 87353, - "high": 1371, - "low": 87353, - "open": 224, - "volume": 531321 - }, - "open": "2020-04-14T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13002, - "high": 24855, - "low": 13002, - "open": 20000, - "volume": 3758278 - }, - "id": 2583703, - "non_hive": { - "close": 1886, - "high": 4140, - "low": 1886, - "open": 3200, - "volume": 553520 - }, - "open": "2020-04-14T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11268, - "high": 323, - "low": 7, - "open": 21939, - "volume": 3516357 - }, - "id": 2583729, - "non_hive": { - "close": 1800, - "high": 53, - "low": 1, - "open": 3225, - "volume": 518255 - }, - "open": "2020-04-14T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4436, - "high": 55, - "low": 5605, - "open": 3831, - "volume": 57500 - }, - "id": 2583762, - "non_hive": { - "close": 725, - "high": 9, - "low": 840, - "open": 612, - "volume": 9235 - }, - "open": "2020-04-14T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18207, - "high": 6, - "low": 27, - "open": 6, - "volume": 86421 - }, - "id": 2583779, - "non_hive": { - "close": 2907, - "high": 1, - "low": 4, - "open": 1, - "volume": 13320 - }, - "open": "2020-04-14T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49830, - "high": 432, - "low": 95, - "open": 95, - "volume": 100357 - }, - "id": 2583791, - "non_hive": { - "close": 7941, - "high": 69, - "low": 14, - "open": 14, - "volume": 15991 - }, - "open": "2020-04-14T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 452, - "high": 452, - "low": 452, - "open": 452, - "volume": 452 - }, - "id": 2583800, - "non_hive": { - "close": 72, - "high": 72, - "low": 72, - "open": 72, - "volume": 72 - }, - "open": "2020-04-14T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 63, - "high": 63, - "low": 27797, - "open": 439070, - "volume": 602063 - }, - "id": 2583804, - "non_hive": { - "close": 10, - "high": 10, - "low": 4138, - "open": 65891, - "volume": 90315 - }, - "open": "2020-04-14T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 49829, - "low": 13, - "open": 1405, - "volume": 301779 - }, - "id": 2583814, - "non_hive": { - "close": 2, - "high": 7884, - "low": 2, - "open": 222, - "volume": 47732 - }, - "open": "2020-04-14T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25240, - "high": 8499, - "low": 27, - "open": 8499, - "volume": 625267 - }, - "id": 2583826, - "non_hive": { - "close": 3887, - "high": 1319, - "low": 4, - "open": 1319, - "volume": 93430 - }, - "open": "2020-04-14T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22522, - "high": 115, - "low": 22522, - "open": 24760, - "volume": 979943 - }, - "id": 2583836, - "non_hive": { - "close": 3310, - "high": 18, - "low": 3310, - "open": 3813, - "volume": 148257 - }, - "open": "2020-04-14T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18537, - "high": 231, - "low": 18537, - "open": 187, - "volume": 1220024 - }, - "id": 2583848, - "non_hive": { - "close": 2706, - "high": 37, - "low": 2706, - "open": 29, - "volume": 179872 - }, - "open": "2020-04-14T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 147495, - "high": 369, - "low": 147495, - "open": 600000, - "volume": 3050356 - }, - "id": 2583866, - "non_hive": { - "close": 19911, - "high": 59, - "low": 19911, - "open": 87600, - "volume": 443021 - }, - "open": "2020-04-14T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 398, - "high": 398, - "low": 9286, - "open": 9286, - "volume": 4052499 - }, - "id": 2583898, - "non_hive": { - "close": 63, - "high": 63, - "low": 1391, - "open": 1391, - "volume": 638023 - }, - "open": "2020-04-14T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 6, - "low": 389220, - "open": 210780, - "volume": 6844964 - }, - "id": 2583936, - "non_hive": { - "close": 1, - "high": 1, - "low": 55265, - "open": 31617, - "volume": 1037522 - }, - "open": "2020-04-14T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 244466, - "low": 10, - "open": 600000, - "volume": 5589090 - }, - "id": 2583978, - "non_hive": { - "close": 1, - "high": 38377, - "low": 1, - "open": 87120, - "volume": 829505 - }, - "open": "2020-04-14T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 675, - "high": 2745, - "low": 100, - "open": 80028, - "volume": 3230196 - }, - "id": 2584022, - "non_hive": { - "close": 104, - "high": 431, - "low": 15, - "open": 12563, - "volume": 506862 - }, - "open": "2020-04-14T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30124, - "high": 30124, - "low": 549678, - "open": 600000, - "volume": 1348690 - }, - "id": 2584043, - "non_hive": { - "close": 4800, - "high": 4800, - "low": 85200, - "open": 93000, - "volume": 209593 - }, - "open": "2020-04-14T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 375550, - "high": 630518, - "low": 2014, - "open": 25104, - "volume": 1583062 - }, - "id": 2584056, - "non_hive": { - "close": 60088, - "high": 100883, - "low": 320, - "open": 4000, - "volume": 252933 - }, - "open": "2020-04-14T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53000, - "high": 11390, - "low": 99542, - "open": 12549, - "volume": 1857771 - }, - "id": 2584071, - "non_hive": { - "close": 8480, - "high": 1868, - "low": 15456, - "open": 1994, - "volume": 293422 - }, - "open": "2020-04-14T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 264, - "high": 16192, - "low": 13, - "open": 176082, - "volume": 726990 - }, - "id": 2584093, - "non_hive": { - "close": 41, - "high": 2650, - "low": 2, - "open": 27821, - "volume": 114343 - }, - "open": "2020-04-14T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14152, - "high": 2841, - "low": 13, - "open": 2841, - "volume": 27976 - }, - "id": 2584110, - "non_hive": { - "close": 2308, - "high": 464, - "low": 2, - "open": 464, - "volume": 4502 - }, - "open": "2020-04-14T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 360600, - "high": 179668, - "low": 78, - "open": 18743, - "volume": 3727688 - }, - "id": 2584128, - "non_hive": { - "close": 56093, - "high": 28731, - "low": 12, - "open": 2919, - "volume": 580938 - }, - "open": "2020-04-15T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 190, - "low": 82309, - "open": 600000, - "volume": 3600046 - }, - "id": 2584151, - "non_hive": { - "close": 87078, - "high": 30, - "low": 11945, - "open": 93333, - "volume": 547148 - }, - "open": "2020-04-15T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3200, - "high": 180997, - "low": 500850, - "open": 600000, - "volume": 3499973 - }, - "id": 2584173, - "non_hive": { - "close": 496, - "high": 28954, - "low": 72683, - "open": 87078, - "volume": 514695 - }, - "open": "2020-04-15T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 18, - "low": 100, - "open": 46800, - "volume": 1741348 - }, - "id": 2584203, - "non_hive": { - "close": 14, - "high": 3, - "low": 14, - "open": 7254, - "volume": 273175 - }, - "open": "2020-04-15T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299997, - "high": 12832, - "low": 100, - "open": 170, - "volume": 3912671 - }, - "id": 2584228, - "non_hive": { - "close": 44490, - "high": 2053, - "low": 14, - "open": 27, - "volume": 583432 - }, - "open": "2020-04-15T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1871, - "high": 1871, - "low": 14, - "open": 300003, - "volume": 8122911 - }, - "id": 2584261, - "non_hive": { - "close": 304, - "high": 304, - "low": 2, - "open": 44679, - "volume": 1279978 - }, - "open": "2020-04-15T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4244, - "high": 580611, - "low": 491, - "open": 19000, - "volume": 6072367 - }, - "id": 2584310, - "non_hive": { - "close": 665, - "high": 94346, - "low": 76, - "open": 3002, - "volume": 966058 - }, - "open": "2020-04-15T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 249230, - "high": 36, - "low": 136, - "open": 300001, - "volume": 3640265 - }, - "id": 2584346, - "non_hive": { - "close": 39589, - "high": 6, - "low": 21, - "open": 47461, - "volume": 579771 - }, - "open": "2020-04-15T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16000, - "high": 8527, - "low": 7, - "open": 300000, - "volume": 4162601 - }, - "id": 2584376, - "non_hive": { - "close": 2592, - "high": 1385, - "low": 1, - "open": 47958, - "volume": 654225 - }, - "open": "2020-04-15T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2228, - "high": 2228, - "low": 401, - "open": 66657, - "volume": 3002228 - }, - "id": 2584410, - "non_hive": { - "close": 361, - "high": 361, - "low": 62, - "open": 10433, - "volume": 469668 - }, - "open": "2020-04-15T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26, - "high": 1933, - "low": 26, - "open": 18500, - "volume": 3001959 - }, - "id": 2584427, - "non_hive": { - "close": 4, - "high": 313, - "low": 4, - "open": 2896, - "volume": 470008 - }, - "open": "2020-04-15T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 605280, - "low": 7, - "open": 533664, - "volume": 7329859 - }, - "id": 2584445, - "non_hive": { - "close": 94531, - "high": 98358, - "low": 1, - "open": 83842, - "volume": 1172635 - }, - "open": "2020-04-15T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47790, - "high": 26349, - "low": 100, - "open": 100, - "volume": 3635022 - }, - "id": 2584482, - "non_hive": { - "close": 7475, - "high": 4220, - "low": 15, - "open": 15, - "volume": 571951 - }, - "open": "2020-04-15T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45003, - "high": 5021, - "low": 34620, - "open": 145000, - "volume": 2644642 - }, - "id": 2584507, - "non_hive": { - "close": 7133, - "high": 808, - "low": 5366, - "open": 22693, - "volume": 411658 - }, - "open": "2020-04-15T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 300000, - "high": 15378, - "low": 372359, - "open": 15378, - "volume": 3001000 - }, - "id": 2584535, - "non_hive": { - "close": 45008, - "high": 2384, - "low": 55859, - "open": 2384, - "volume": 453770 - }, - "open": "2020-04-15T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 249453, - "high": 9115, - "low": 157036, - "open": 300003, - "volume": 3609115 - }, - "id": 2584553, - "non_hive": { - "close": 37425, - "high": 1390, - "low": 23559, - "open": 45010, - "volume": 541533 - }, - "open": "2020-04-15T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 129, - "high": 129, - "low": 338351, - "open": 144361, - "volume": 2510337 - }, - "id": 2584575, - "non_hive": { - "close": 20, - "high": 20, - "low": 50761, - "open": 21660, - "volume": 377114 - }, - "open": "2020-04-15T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 49812, - "low": 7, - "open": 300003, - "volume": 4217299 - }, - "id": 2584597, - "non_hive": { - "close": 90773, - "high": 7721, - "low": 1, - "open": 45031, - "volume": 640651 - }, - "open": "2020-04-15T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3410, - "high": 1127, - "low": 100, - "open": 300003, - "volume": 3225185 - }, - "id": 2584632, - "non_hive": { - "close": 515, - "high": 177, - "low": 15, - "open": 45307, - "volume": 488100 - }, - "open": "2020-04-15T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3536, - "high": 655, - "low": 3536, - "open": 300000, - "volume": 3752298 - }, - "id": 2584661, - "non_hive": { - "close": 514, - "high": 101, - "low": 514, - "open": 45312, - "volume": 562421 - }, - "open": "2020-04-15T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 1493, - "low": 283305, - "open": 298, - "volume": 3780748 - }, - "id": 2584689, - "non_hive": { - "close": 87361, - "high": 230, - "low": 41242, - "open": 45, - "volume": 551794 - }, - "open": "2020-04-15T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 600000, - "low": 600000, - "open": 600000, - "volume": 2400000 - }, - "id": 2584714, - "non_hive": { - "close": 87348, - "high": 87361, - "low": 87348, - "open": 87361, - "volume": 349405 - }, - "open": "2020-04-15T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 514611, - "high": 966, - "low": 14, - "open": 600000, - "volume": 3002222 - }, - "id": 2584727, - "non_hive": { - "close": 74917, - "high": 145, - "low": 2, - "open": 87348, - "volume": 437072 - }, - "open": "2020-04-15T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27303, - "high": 15469, - "low": 7, - "open": 600000, - "volume": 3000427 - }, - "id": 2584754, - "non_hive": { - "close": 3958, - "high": 2252, - "low": 1, - "open": 87348, - "volume": 436777 - }, - "open": "2020-04-15T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299997, - "high": 122703, - "low": 37043, - "open": 122703, - "volume": 3000000 - }, - "id": 2584775, - "non_hive": { - "close": 42894, - "high": 17792, - "low": 5296, - "open": 17792, - "volume": 429207 - }, - "open": "2020-04-16T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 206320, - "high": 27875, - "low": 39, - "open": 27875, - "volume": 3000042 - }, - "id": 2584792, - "non_hive": { - "close": 29500, - "high": 4000, - "low": 5, - "open": 4000, - "volume": 428972 - }, - "open": "2020-04-16T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 386, - "low": 398781, - "open": 361574, - "volume": 3000386 - }, - "id": 2584811, - "non_hive": { - "close": 81030, - "high": 56, - "low": 53855, - "open": 51699, - "volume": 413203 - }, - "open": "2020-04-16T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 620165, - "high": 113399, - "low": 600000, - "open": 600000, - "volume": 4182918 - }, - "id": 2584830, - "non_hive": { - "close": 89924, - "high": 16443, - "low": 81030, - "open": 81030, - "volume": 580469 - }, - "open": "2020-04-16T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 210590, - "high": 148796, - "low": 210590, - "open": 148796, - "volume": 359386 - }, - "id": 2584868, - "non_hive": { - "close": 30525, - "high": 21568, - "low": 30525, - "open": 21568, - "volume": 52093 - }, - "open": "2020-04-16T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16927, - "high": 16927, - "low": 7, - "open": 7, - "volume": 3582281 - }, - "id": 2584875, - "non_hive": { - "close": 2573, - "high": 2573, - "low": 1, - "open": 1, - "volume": 519549 - }, - "open": "2020-04-16T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22, - "high": 217, - "low": 22, - "open": 217, - "volume": 70499 - }, - "id": 2584892, - "non_hive": { - "close": 3, - "high": 31, - "low": 3, - "open": 31, - "volume": 10061 - }, - "open": "2020-04-16T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49826, - "high": 49826, - "low": 93, - "open": 476, - "volume": 97779 - }, - "id": 2584902, - "non_hive": { - "close": 7175, - "high": 7175, - "low": 13, - "open": 68, - "volume": 14025 - }, - "open": "2020-04-16T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 120, - "high": 18732, - "low": 120, - "open": 50000, - "volume": 439468 - }, - "id": 2584915, - "non_hive": { - "close": 18, - "high": 2895, - "low": 17, - "open": 7242, - "volume": 63964 - }, - "open": "2020-04-16T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50000, - "high": 13, - "low": 120, - "open": 120, - "volume": 112124 - }, - "id": 2584940, - "non_hive": { - "close": 7645, - "high": 2, - "low": 18, - "open": 18, - "volume": 17084 - }, - "open": "2020-04-16T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 89, - "high": 5033, - "low": 89, - "open": 5033, - "volume": 8314 - }, - "id": 2584961, - "non_hive": { - "close": 13, - "high": 760, - "low": 13, - "open": 760, - "volume": 1255 - }, - "open": "2020-04-16T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 220, - "high": 25, - "low": 7, - "open": 188, - "volume": 35125 - }, - "id": 2584968, - "non_hive": { - "close": 34, - "high": 4, - "low": 1, - "open": 29, - "volume": 5238 - }, - "open": "2020-04-16T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1688, - "high": 51, - "low": 1688, - "open": 51, - "volume": 1739 - }, - "id": 2584993, - "non_hive": { - "close": 260, - "high": 8, - "low": 260, - "open": 8, - "volume": 268 - }, - "open": "2020-04-16T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 324719, - "high": 18688, - "low": 378702, - "open": 17684, - "volume": 1018776 - }, - "id": 2585000, - "non_hive": { - "close": 49982, - "high": 2878, - "low": 58291, - "open": 2722, - "volume": 156815 - }, - "open": "2020-04-16T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 95576, - "high": 1133, - "low": 95576, - "open": 1133, - "volume": 145576 - }, - "id": 2585009, - "non_hive": { - "close": 14670, - "high": 174, - "low": 14670, - "open": 174, - "volume": 22345 - }, - "open": "2020-04-16T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14076, - "high": 14076, - "low": 2970, - "open": 80216, - "volume": 192079 - }, - "id": 2585019, - "non_hive": { - "close": 2161, - "high": 2161, - "low": 455, - "open": 12313, - "volume": 29485 - }, - "open": "2020-04-16T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 403, - "high": 7341, - "low": 403, - "open": 7341, - "volume": 7744 - }, - "id": 2585034, - "non_hive": { - "close": 61, - "high": 1127, - "low": 61, - "open": 1127, - "volume": 1188 - }, - "open": "2020-04-16T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 7, - "low": 7, - "open": 7, - "volume": 7 - }, - "id": 2585041, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 1, - "volume": 1 - }, - "open": "2020-04-16T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3301, - "high": 191975, - "low": 3301, - "open": 797700, - "volume": 2585020 - }, - "id": 2585045, - "non_hive": { - "close": 505, - "high": 29472, - "low": 505, - "open": 122462, - "volume": 396848 - }, - "open": "2020-04-16T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9771, - "high": 4352, - "low": 9771, - "open": 4352, - "volume": 14123 - }, - "id": 2585059, - "non_hive": { - "close": 1495, - "high": 668, - "low": 1495, - "open": 668, - "volume": 2163 - }, - "open": "2020-04-16T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13246, - "high": 78306, - "low": 13246, - "open": 7169, - "volume": 208617 - }, - "id": 2585066, - "non_hive": { - "close": 2000, - "high": 12017, - "low": 2000, - "open": 1086, - "volume": 31898 - }, - "open": "2020-04-16T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7188, - "high": 7188, - "low": 7188, - "open": 7188, - "volume": 7188 - }, - "id": 2585083, - "non_hive": { - "close": 1094, - "high": 1094, - "low": 1094, - "open": 1094, - "volume": 1094 - }, - "open": "2020-04-16T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20030, - "high": 96574, - "low": 14, - "open": 14, - "volume": 1905889 - }, - "id": 2585087, - "non_hive": { - "close": 2986, - "high": 14820, - "low": 2, - "open": 2, - "volume": 285339 - }, - "open": "2020-04-16T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 283596, - "high": 666590, - "low": 7, - "open": 57993, - "volume": 5679109 - }, - "id": 2585108, - "non_hive": { - "close": 41229, - "high": 100000, - "low": 1, - "open": 8657, - "volume": 845269 - }, - "open": "2020-04-16T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 160, - "high": 2226, - "low": 160, - "open": 2226, - "volume": 5245960 - }, - "id": 2585140, - "non_hive": { - "close": 23, - "high": 339, - "low": 23, - "open": 339, - "volume": 771348 - }, - "open": "2020-04-17T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299999, - "high": 4585, - "low": 41, - "open": 300001, - "volume": 3610483 - }, - "id": 2585175, - "non_hive": { - "close": 44399, - "high": 690, - "low": 6, - "open": 44401, - "volume": 534393 - }, - "open": "2020-04-17T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299999, - "high": 568829, - "low": 198224, - "open": 300001, - "volume": 4310871 - }, - "id": 2585199, - "non_hive": { - "close": 43691, - "high": 88081, - "low": 28817, - "open": 44401, - "volume": 637695 - }, - "open": "2020-04-17T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19055, - "high": 11217, - "low": 19055, - "open": 300001, - "volume": 3708934 - }, - "id": 2585220, - "non_hive": { - "close": 2775, - "high": 1737, - "low": 2775, - "open": 43693, - "volume": 540278 - }, - "open": "2020-04-17T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299567, - "high": 299567, - "low": 100, - "open": 10000, - "volume": 4973607 - }, - "id": 2585247, - "non_hive": { - "close": 46371, - "high": 46371, - "low": 14, - "open": 1457, - "volume": 734918 - }, - "open": "2020-04-17T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 196989, - "high": 6, - "low": 7, - "open": 300001, - "volume": 9690129 - }, - "id": 2585276, - "non_hive": { - "close": 28884, - "high": 1, - "low": 1, - "open": 45121, - "volume": 1486953 - }, - "open": "2020-04-17T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48177, - "high": 8570, - "low": 48177, - "open": 153673, - "volume": 4762866 - }, - "id": 2585314, - "non_hive": { - "close": 6868, - "high": 1359, - "low": 6868, - "open": 23178, - "volume": 699685 - }, - "open": "2020-04-17T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 2591, - "low": 94, - "open": 598000, - "volume": 4943776 - }, - "id": 2585346, - "non_hive": { - "close": 14, - "high": 391, - "low": 13, - "open": 83242, - "volume": 699152 - }, - "open": "2020-04-17T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38739, - "high": 31760, - "low": 35, - "open": 185, - "volume": 4848563 - }, - "id": 2585377, - "non_hive": { - "close": 6000, - "high": 4987, - "low": 5, - "open": 28, - "volume": 729641 - }, - "open": "2020-04-17T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 445994, - "high": 211, - "low": 237, - "open": 17316, - "volume": 3906817 - }, - "id": 2585422, - "non_hive": { - "close": 67500, - "high": 33, - "low": 35, - "open": 2682, - "volume": 593999 - }, - "open": "2020-04-17T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29377, - "high": 1675, - "low": 600000, - "open": 214729, - "volume": 3079377 - }, - "id": 2585455, - "non_hive": { - "close": 4524, - "high": 258, - "low": 89967, - "open": 32499, - "volume": 463614 - }, - "open": "2020-04-17T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 942, - "high": 942, - "low": 7, - "open": 30000, - "volume": 8754387 - }, - "id": 2585477, - "non_hive": { - "close": 147, - "high": 147, - "low": 1, - "open": 4620, - "volume": 1339548 - }, - "open": "2020-04-17T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59259, - "high": 49833, - "low": 300000, - "open": 600000, - "volume": 4444136 - }, - "id": 2585526, - "non_hive": { - "close": 9242, - "high": 7772, - "low": 45000, - "open": 91868, - "volume": 673223 - }, - "open": "2020-04-17T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 300000, - "high": 101184, - "low": 300000, - "open": 565072, - "volume": 3666256 - }, - "id": 2585558, - "non_hive": { - "close": 45048, - "high": 15636, - "low": 45015, - "open": 87320, - "volume": 554669 - }, - "open": "2020-04-17T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 72583, - "low": 299999, - "open": 300001, - "volume": 10541432 - }, - "id": 2585577, - "non_hive": { - "close": 96408, - "high": 12847, - "low": 45048, - "open": 45050, - "volume": 1737304 - }, - "open": "2020-04-17T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 49835, - "low": 110, - "open": 300001, - "volume": 4062795 - }, - "id": 2585622, - "non_hive": { - "close": 96074, - "high": 8469, - "low": 16, - "open": 49134, - "volume": 653667 - }, - "open": "2020-04-17T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 68939, - "high": 123, - "low": 535482, - "open": 123, - "volume": 5733786 - }, - "id": 2585652, - "non_hive": { - "close": 11374, - "high": 21, - "low": 85678, - "open": 21, - "volume": 931355 - }, - "open": "2020-04-17T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 228, - "high": 131, - "low": 48309, - "open": 34411, - "volume": 184134 - }, - "id": 2585698, - "non_hive": { - "close": 40, - "high": 23, - "low": 7738, - "open": 5850, - "volume": 30308 - }, - "open": "2020-04-17T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3235, - "high": 21958, - "low": 173, - "open": 10737, - "volume": 138193 - }, - "id": 2585723, - "non_hive": { - "close": 525, - "high": 3835, - "low": 28, - "open": 1751, - "volume": 23443 - }, - "open": "2020-04-17T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2436, - "high": 572, - "low": 39561, - "open": 572, - "volume": 42569 - }, - "id": 2585745, - "non_hive": { - "close": 425, - "high": 100, - "low": 6899, - "open": 100, - "volume": 7424 - }, - "open": "2020-04-17T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10343, - "high": 140389, - "low": 10343, - "open": 48309, - "volume": 537223 - }, - "id": 2585752, - "non_hive": { - "close": 1758, - "high": 24526, - "low": 1758, - "open": 8424, - "volume": 93715 - }, - "open": "2020-04-17T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 733, - "high": 3344, - "low": 570287, - "open": 3564, - "volume": 9157850 - }, - "id": 2585760, - "non_hive": { - "close": 131, - "high": 598, - "low": 94667, - "open": 621, - "volume": 1620399 - }, - "open": "2020-04-17T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 549, - "high": 8736, - "low": 13, - "open": 3559, - "volume": 177345 - }, - "id": 2585772, - "non_hive": { - "close": 90, - "high": 1560, - "low": 2, - "open": 598, - "volume": 29954 - }, - "open": "2020-04-17T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 411930, - "high": 12489, - "low": 400, - "open": 12489, - "volume": 4571992 - }, - "id": 2585796, - "non_hive": { - "close": 67585, - "high": 2107, - "low": 64, - "open": 2107, - "volume": 748411 - }, - "open": "2020-04-17T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 201, - "high": 201, - "low": 80, - "open": 75, - "volume": 2025588 - }, - "id": 2585820, - "non_hive": { - "close": 33, - "high": 33, - "low": 12, - "open": 12, - "volume": 330024 - }, - "open": "2020-04-18T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 5, - "low": 38, - "open": 38, - "volume": 710978 - }, - "id": 2585837, - "non_hive": { - "close": 96144, - "high": 1, - "low": 6, - "open": 6, - "volume": 115883 - }, - "open": "2020-04-18T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 200004, - "low": 399996, - "open": 600000, - "volume": 2400000 - }, - "id": 2585853, - "non_hive": { - "close": 96144, - "high": 32049, - "low": 64095, - "open": 96144, - "volume": 384576 - }, - "open": "2020-04-18T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29095, - "high": 29095, - "low": 100, - "open": 600000, - "volume": 629195 - }, - "id": 2585866, - "non_hive": { - "close": 5000, - "high": 5000, - "low": 16, - "open": 96150, - "volume": 101166 - }, - "open": "2020-04-18T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 5356, - "low": 568815, - "open": 31185, - "volume": 2413686 - }, - "id": 2585876, - "non_hive": { - "close": 96210, - "high": 920, - "low": 91185, - "open": 5001, - "volume": 387268 - }, - "open": "2020-04-18T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51242, - "high": 38082, - "low": 289032, - "open": 600000, - "volume": 4173059 - }, - "id": 2585897, - "non_hive": { - "close": 8250, - "high": 6545, - "low": 46370, - "open": 96260, - "volume": 671358 - }, - "open": "2020-04-18T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25, - "high": 600000, - "low": 25, - "open": 600000, - "volume": 1200025 - }, - "id": 2585932, - "non_hive": { - "close": 4, - "high": 96539, - "low": 4, - "open": 96515, - "volume": 193058 - }, - "open": "2020-04-18T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 355800, - "high": 348306, - "low": 88, - "open": 348306, - "volume": 1800088 - }, - "id": 2585942, - "non_hive": { - "close": 56966, - "high": 56077, - "low": 14, - "open": 56077, - "volume": 288570 - }, - "open": "2020-04-18T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 48409, - "low": 7, - "open": 48409, - "volume": 158976 - }, - "id": 2585954, - "non_hive": { - "close": 1, - "high": 7738, - "low": 1, - "open": 7738, - "volume": 25318 - }, - "open": "2020-04-18T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2422, - "high": 2422, - "low": 488039, - "open": 111961, - "volume": 1259013 - }, - "id": 2585964, - "non_hive": { - "close": 390, - "high": 390, - "low": 76548, - "open": 17578, - "volume": 197728 - }, - "open": "2020-04-18T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5379, - "high": 29838, - "low": 162, - "open": 2655, - "volume": 396362 - }, - "id": 2585982, - "non_hive": { - "close": 867, - "high": 4816, - "low": 26, - "open": 428, - "volume": 63971 - }, - "open": "2020-04-18T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5523, - "high": 5523, - "low": 7, - "open": 520948, - "volume": 632027 - }, - "id": 2585994, - "non_hive": { - "close": 890, - "high": 890, - "low": 1, - "open": 83945, - "volume": 101844 - }, - "open": "2020-04-18T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27330, - "high": 29838, - "low": 522466, - "open": 53081, - "volume": 1703901 - }, - "id": 2586006, - "non_hive": { - "close": 4412, - "high": 4817, - "low": 81973, - "open": 8440, - "volume": 272221 - }, - "open": "2020-04-18T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29851, - "high": 29845, - "low": 567176, - "open": 600000, - "volume": 5618712 - }, - "id": 2586019, - "non_hive": { - "close": 4816, - "high": 4819, - "low": 89613, - "open": 94810, - "volume": 897262 - }, - "open": "2020-04-18T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16120, - "high": 17232, - "low": 1534, - "open": 149, - "volume": 3932461 - }, - "id": 2586055, - "non_hive": { - "close": 2547, - "high": 2875, - "low": 242, - "open": 24, - "volume": 623906 - }, - "open": "2020-04-18T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19880, - "high": 27419, - "low": 8, - "open": 27419, - "volume": 440626 - }, - "id": 2586087, - "non_hive": { - "close": 3141, - "high": 4442, - "low": 1, - "open": 4442, - "volume": 70343 - }, - "open": "2020-04-18T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5956, - "high": 5956, - "low": 383822, - "open": 20139, - "volume": 3069688 - }, - "id": 2586111, - "non_hive": { - "close": 941, - "high": 941, - "low": 58376, - "open": 3160, - "volume": 471719 - }, - "open": "2020-04-18T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 924, - "high": 658, - "low": 7, - "open": 300000, - "volume": 3815466 - }, - "id": 2586133, - "non_hive": { - "close": 146, - "high": 104, - "low": 1, - "open": 45740, - "volume": 587405 - }, - "open": "2020-04-18T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3295, - "high": 15005, - "low": 110, - "open": 127176, - "volume": 5798664 - }, - "id": 2586160, - "non_hive": { - "close": 514, - "high": 2400, - "low": 17, - "open": 20088, - "volume": 907894 - }, - "open": "2020-04-18T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18335, - "high": 135177, - "low": 117887, - "open": 11705, - "volume": 5331087 - }, - "id": 2586196, - "non_hive": { - "close": 2844, - "high": 21628, - "low": 18226, - "open": 1826, - "volume": 838673 - }, - "open": "2020-04-18T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 934, - "high": 28122, - "low": 600000, - "open": 29997, - "volume": 3849797 - }, - "id": 2586221, - "non_hive": { - "close": 157, - "high": 4769, - "low": 93211, - "open": 4695, - "volume": 605807 - }, - "open": "2020-04-18T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21137, - "high": 21137, - "low": 600000, - "open": 600000, - "volume": 3621137 - }, - "id": 2586248, - "non_hive": { - "close": 3382, - "high": 3382, - "low": 93223, - "open": 93223, - "volume": 562770 - }, - "open": "2020-04-18T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 599994, - "high": 37139, - "low": 13, - "open": 600000, - "volume": 3114807 - }, - "id": 2586270, - "non_hive": { - "close": 93232, - "high": 6295, - "low": 2, - "open": 93294, - "volume": 485001 - }, - "open": "2020-04-18T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3275, - "high": 1002, - "low": 13, - "open": 600000, - "volume": 3008698 - }, - "id": 2586300, - "non_hive": { - "close": 507, - "high": 168, - "low": 2, - "open": 93233, - "volume": 467010 - }, - "open": "2020-04-18T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74294, - "high": 343457, - "low": 535897, - "open": 64103, - "volume": 2400000 - }, - "id": 2586329, - "non_hive": { - "close": 11551, - "high": 54000, - "low": 82968, - "open": 10000, - "volume": 374170 - }, - "open": "2020-04-19T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 101487, - "high": 101487, - "low": 39, - "open": 600000, - "volume": 2550068 - }, - "id": 2586343, - "non_hive": { - "close": 16467, - "high": 16467, - "low": 6, - "open": 93294, - "volume": 397410 - }, - "open": "2020-04-19T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 48642, - "low": 54194, - "open": 105878, - "volume": 3048642 - }, - "id": 2586362, - "non_hive": { - "close": 93294, - "high": 7758, - "low": 8426, - "open": 16467, - "volume": 474238 - }, - "open": "2020-04-19T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 238979, - "high": 343237, - "low": 238979, - "open": 343237, - "volume": 3013983 - }, - "id": 2586381, - "non_hive": { - "close": 37041, - "high": 53370, - "low": 37041, - "open": 53370, - "volume": 468346 - }, - "open": "2020-04-19T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 475304, - "high": 1400, - "low": 475304, - "open": 1400, - "volume": 2419370 - }, - "id": 2586400, - "non_hive": { - "close": 73196, - "high": 227, - "low": 73196, - "open": 227, - "volume": 374013 - }, - "open": "2020-04-19T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 25, - "low": 7, - "open": 524676, - "volume": 4413207 - }, - "id": 2586416, - "non_hive": { - "close": 90199, - "high": 4, - "low": 1, - "open": 80800, - "volume": 668946 - }, - "open": "2020-04-19T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 369669, - "high": 15674, - "low": 27, - "open": 15674, - "volume": 5065839 - }, - "id": 2586442, - "non_hive": { - "close": 56542, - "high": 2447, - "low": 4, - "open": 2447, - "volume": 770557 - }, - "open": "2020-04-19T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 530, - "high": 465, - "low": 599517, - "open": 465, - "volume": 3624827 - }, - "id": 2586476, - "non_hive": { - "close": 82, - "high": 72, - "low": 90780, - "open": 72, - "volume": 553278 - }, - "open": "2020-04-19T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 51421, - "low": 7, - "open": 51421, - "volume": 8239097 - }, - "id": 2586514, - "non_hive": { - "close": 1, - "high": 7878, - "low": 1, - "open": 7878, - "volume": 1221710 - }, - "open": "2020-04-19T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 39543, - "low": 10, - "open": 600000, - "volume": 3196822 - }, - "id": 2586550, - "non_hive": { - "close": 1, - "high": 6110, - "low": 1, - "open": 87600, - "volume": 474714 - }, - "open": "2020-04-19T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15222, - "high": 13344, - "low": 78, - "open": 299961, - "volume": 3057393 - }, - "id": 2586573, - "non_hive": { - "close": 2284, - "high": 2055, - "low": 11, - "open": 45049, - "volume": 459568 - }, - "open": "2020-04-19T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47207, - "high": 110495, - "low": 7, - "open": 600000, - "volume": 4664784 - }, - "id": 2586592, - "non_hive": { - "close": 7080, - "high": 16795, - "low": 1, - "open": 90112, - "volume": 703221 - }, - "open": "2020-04-19T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 300000, - "high": 227249, - "low": 600000, - "open": 600000, - "volume": 4107654 - }, - "id": 2586624, - "non_hive": { - "close": 44265, - "high": 34301, - "low": 86873, - "open": 90000, - "volume": 610904 - }, - "open": "2020-04-19T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 234173, - "high": 1235, - "low": 30, - "open": 50000, - "volume": 4584492 - }, - "id": 2586648, - "non_hive": { - "close": 35187, - "high": 190, - "low": 4, - "open": 7500, - "volume": 688468 - }, - "open": "2020-04-19T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16031, - "high": 57678, - "low": 126587, - "open": 157626, - "volume": 3424048 - }, - "id": 2586684, - "non_hive": { - "close": 2420, - "high": 8859, - "low": 18230, - "open": 23707, - "volume": 511519 - }, - "open": "2020-04-19T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 91152, - "high": 154401, - "low": 470631, - "open": 43969, - "volume": 1547682 - }, - "id": 2586718, - "non_hive": { - "close": 13750, - "high": 23549, - "low": 67723, - "open": 6637, - "volume": 229132 - }, - "open": "2020-04-19T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15963, - "high": 10706, - "low": 15963, - "open": 10706, - "volume": 26669 - }, - "id": 2586740, - "non_hive": { - "close": 2408, - "high": 1615, - "low": 2408, - "open": 1615, - "volume": 4023 - }, - "open": "2020-04-19T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6135, - "high": 21028, - "low": 7, - "open": 21028, - "volume": 187685 - }, - "id": 2586747, - "non_hive": { - "close": 908, - "high": 3172, - "low": 1, - "open": 3172, - "volume": 28149 - }, - "open": "2020-04-19T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3351, - "high": 114, - "low": 16422, - "open": 16422, - "volume": 607785 - }, - "id": 2586762, - "non_hive": { - "close": 496, - "high": 17, - "low": 2430, - "open": 2430, - "volume": 90434 - }, - "open": "2020-04-19T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 523, - "high": 523, - "low": 40000, - "open": 40000, - "volume": 42602 - }, - "id": 2586775, - "non_hive": { - "close": 79, - "high": 79, - "low": 5926, - "open": 5926, - "volume": 6319 - }, - "open": "2020-04-19T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 182, - "high": 182, - "low": 182, - "open": 182, - "volume": 182 - }, - "id": 2586785, - "non_hive": { - "close": 27, - "high": 27, - "low": 27, - "open": 27, - "volume": 27 - }, - "open": "2020-04-19T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10333, - "high": 10333, - "low": 14, - "open": 14, - "volume": 10602 - }, - "id": 2586789, - "non_hive": { - "close": 1540, - "high": 1540, - "low": 2, - "open": 2, - "volume": 1580 - }, - "open": "2020-04-19T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 6076, - "low": 7, - "open": 4578, - "volume": 99794 - }, - "id": 2586799, - "non_hive": { - "close": 1, - "high": 924, - "low": 1, - "open": 696, - "volume": 15171 - }, - "open": "2020-04-19T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32746, - "high": 32746, - "low": 41, - "open": 41, - "volume": 32787 - }, - "id": 2586810, - "non_hive": { - "close": 4980, - "high": 4980, - "low": 6, - "open": 6, - "volume": 4986 - }, - "open": "2020-04-20T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 105423, - "high": 105423, - "low": 551963, - "open": 551963, - "volume": 657386 - }, - "id": 2586818, - "non_hive": { - "close": 16032, - "high": 16032, - "low": 83938, - "open": 83938, - "volume": 99970 - }, - "open": "2020-04-20T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6, - "high": 6, - "low": 192, - "open": 192, - "volume": 853452 - }, - "id": 2586822, - "non_hive": { - "close": 1, - "high": 1, - "low": 29, - "open": 29, - "volume": 129789 - }, - "open": "2020-04-20T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 149249, - "high": 6, - "low": 7, - "open": 2435, - "volume": 162442 - }, - "id": 2586827, - "non_hive": { - "close": 22675, - "high": 1, - "low": 1, - "open": 380, - "volume": 24690 - }, - "open": "2020-04-20T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 93, - "high": 749, - "low": 27, - "open": 76000, - "volume": 236057 - }, - "id": 2586840, - "non_hive": { - "close": 14, - "high": 117, - "low": 4, - "open": 11625, - "volume": 36433 - }, - "open": "2020-04-20T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8578, - "high": 9623, - "low": 99341, - "open": 99341, - "volume": 560613 - }, - "id": 2586853, - "non_hive": { - "close": 1354, - "high": 1519, - "low": 15201, - "open": 15201, - "volume": 87171 - }, - "open": "2020-04-20T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 647179, - "high": 20515, - "low": 7, - "open": 20515, - "volume": 2512408 - }, - "id": 2586874, - "non_hive": { - "close": 100000, - "high": 3217, - "low": 1, - "open": 3217, - "volume": 388324 - }, - "open": "2020-04-20T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3567, - "high": 3567, - "low": 376655, - "open": 376655, - "volume": 380222 - }, - "id": 2586891, - "non_hive": { - "close": 560, - "high": 560, - "low": 58162, - "open": 58162, - "volume": 58722 - }, - "open": "2020-04-20T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 97, - "low": 422590, - "open": 3636, - "volume": 2463655 - }, - "id": 2586898, - "non_hive": { - "close": 90016, - "high": 15, - "low": 63388, - "open": 560, - "volume": 371734 - }, - "open": "2020-04-20T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2143, - "high": 536, - "low": 14, - "open": 229952, - "volume": 3034684 - }, - "id": 2586915, - "non_hive": { - "close": 327, - "high": 82, - "low": 2, - "open": 35175, - "volume": 457363 - }, - "open": "2020-04-20T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 399648, - "high": 19, - "low": 34, - "open": 300000, - "volume": 3616766 - }, - "id": 2586943, - "non_hive": { - "close": 59987, - "high": 3, - "low": 5, - "open": 45396, - "volume": 543931 - }, - "open": "2020-04-20T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 242647, - "high": 234399, - "low": 600000, - "open": 600000, - "volume": 3234399 - }, - "id": 2586968, - "non_hive": { - "close": 36455, - "high": 36093, - "low": 90060, - "open": 90060, - "volume": 487265 - }, - "open": "2020-04-20T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1559900, - "high": 68696, - "low": 600000, - "open": 600000, - "volume": 7148186 - }, - "id": 2586987, - "non_hive": { - "close": 233985, - "high": 10321, - "low": 87012, - "open": 90144, - "volume": 1062981 - }, - "open": "2020-04-20T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1876, - "high": 6, - "low": 53913, - "open": 600000, - "volume": 6807076 - }, - "id": 2587022, - "non_hive": { - "close": 290, - "high": 1, - "low": 7818, - "open": 87024, - "volume": 1031311 - }, - "open": "2020-04-20T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 289582, - "high": 35070, - "low": 159492, - "open": 35070, - "volume": 3600000 - }, - "id": 2587049, - "non_hive": { - "close": 43644, - "high": 5301, - "low": 24027, - "open": 5301, - "volume": 542565 - }, - "open": "2020-04-20T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50000, - "high": 50000, - "low": 7, - "open": 10417, - "volume": 3670986 - }, - "id": 2587068, - "non_hive": { - "close": 7871, - "high": 7871, - "low": 1, - "open": 1570, - "volume": 548365 - }, - "open": "2020-04-20T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2815, - "high": 1015, - "low": 9021, - "open": 1638, - "volume": 4418781 - }, - "id": 2587093, - "non_hive": { - "close": 424, - "high": 160, - "low": 1354, - "open": 258, - "volume": 668943 - }, - "open": "2020-04-20T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 298492, - "high": 177275, - "low": 508, - "open": 300001, - "volume": 3877275 - }, - "id": 2587131, - "non_hive": { - "close": 44985, - "high": 27474, - "low": 76, - "open": 45212, - "volume": 585224 - }, - "open": "2020-04-20T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 471014, - "high": 32860, - "low": 600000, - "open": 600000, - "volume": 4264621 - }, - "id": 2587155, - "non_hive": { - "close": 71008, - "high": 5118, - "low": 90450, - "open": 90450, - "volume": 645692 - }, - "open": "2020-04-20T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 50986, - "low": 538522, - "open": 50986, - "volume": 3000000 - }, - "id": 2587175, - "non_hive": { - "close": 90454, - "high": 7750, - "low": 81185, - "open": 7750, - "volume": 452339 - }, - "open": "2020-04-20T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590000, - "high": 2892, - "low": 14, - "open": 134130, - "volume": 8834501 - }, - "id": 2587191, - "non_hive": { - "close": 85668, - "high": 436, - "low": 2, - "open": 20221, - "volume": 1316366 - }, - "open": "2020-04-20T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 9573, - "low": 597879, - "open": 10000, - "volume": 4186092 - }, - "id": 2587221, - "non_hive": { - "close": 84667, - "high": 1437, - "low": 84367, - "open": 1501, - "volume": 602689 - }, - "open": "2020-04-20T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 3669, - "low": 260817, - "open": 600000, - "volume": 4992402 - }, - "id": 2587242, - "non_hive": { - "close": 15, - "high": 560, - "low": 35340, - "open": 84667, - "volume": 701926 - }, - "open": "2020-04-21T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 209455, - "high": 1035, - "low": 44, - "open": 300000, - "volume": 3156393 - }, - "id": 2587278, - "non_hive": { - "close": 28382, - "high": 155, - "low": 5, - "open": 40652, - "volume": 428438 - }, - "open": "2020-04-21T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 858647, - "high": 29, - "low": 20425, - "open": 79248, - "volume": 5533147 - }, - "id": 2587301, - "non_hive": { - "close": 114200, - "high": 4, - "low": 2716, - "open": 10762, - "volume": 742070 - }, - "open": "2020-04-21T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24739, - "high": 299197, - "low": 543063, - "open": 398, - "volume": 7475347 - }, - "id": 2587325, - "non_hive": { - "close": 3513, - "high": 42855, - "low": 68589, - "open": 53, - "volume": 1015067 - }, - "open": "2020-04-21T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 600000, - "low": 591445, - "open": 8555, - "volume": 3600000 - }, - "id": 2587367, - "non_hive": { - "close": 82140, - "high": 82268, - "low": 79786, - "open": 1166, - "volume": 489915 - }, - "open": "2020-04-21T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 77728, - "high": 53389, - "low": 15, - "open": 53389, - "volume": 2138192 - }, - "id": 2587386, - "non_hive": { - "close": 11269, - "high": 7885, - "low": 2, - "open": 7885, - "volume": 311437 - }, - "open": "2020-04-21T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 93886, - "high": 123324, - "low": 29, - "open": 132461, - "volume": 3356358 - }, - "id": 2587405, - "non_hive": { - "close": 13895, - "high": 18252, - "low": 4, - "open": 19204, - "volume": 495355 - }, - "open": "2020-04-21T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 71052, - "high": 71052, - "low": 104, - "open": 104, - "volume": 71156 - }, - "id": 2587421, - "non_hive": { - "close": 10282, - "high": 10282, - "low": 15, - "open": 15, - "volume": 10297 - }, - "open": "2020-04-21T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 50000, - "low": 7, - "open": 70867, - "volume": 728166 - }, - "id": 2587427, - "non_hive": { - "close": 1, - "high": 7405, - "low": 1, - "open": 10488, - "volume": 107773 - }, - "open": "2020-04-21T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 464476, - "high": 98819, - "low": 464476, - "open": 98819, - "volume": 600000 - }, - "id": 2587437, - "non_hive": { - "close": 67924, - "high": 14650, - "low": 67924, - "open": 14650, - "volume": 87945 - }, - "open": "2020-04-21T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 914, - "low": 10, - "open": 1229, - "volume": 52153 - }, - "id": 2587441, - "non_hive": { - "close": 1, - "high": 137, - "low": 1, - "open": 182, - "volume": 7813 - }, - "open": "2020-04-21T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 552, - "high": 552, - "low": 28, - "open": 1230, - "volume": 600587 - }, - "id": 2587451, - "non_hive": { - "close": 82, - "high": 82, - "low": 4, - "open": 182, - "volume": 88358 - }, - "open": "2020-04-21T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590592, - "high": 9408, - "low": 8887, - "open": 8887, - "volume": 2164789 - }, - "id": 2587464, - "non_hive": { - "close": 88802, - "high": 1433, - "low": 1319, - "open": 1319, - "volume": 326693 - }, - "open": "2020-04-21T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 565000, - "high": 234175, - "low": 565000, - "open": 589339, - "volume": 2389408 - }, - "id": 2587475, - "non_hive": { - "close": 85482, - "high": 36535, - "low": 85482, - "open": 91248, - "volume": 368205 - }, - "open": "2020-04-21T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 489626, - "high": 1275, - "low": 600000, - "open": 600000, - "volume": 3314346 - }, - "id": 2587484, - "non_hive": { - "close": 74930, - "high": 199, - "low": 90960, - "open": 90960, - "volume": 509889 - }, - "open": "2020-04-21T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72971, - "high": 483, - "low": 72971, - "open": 483, - "volume": 1200000 - }, - "id": 2587505, - "non_hive": { - "close": 10748, - "high": 74, - "low": 10748, - "open": 74, - "volume": 177921 - }, - "open": "2020-04-21T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 299326, - "low": 558820, - "open": 592662, - "volume": 5432699 - }, - "id": 2587512, - "non_hive": { - "close": 91810, - "high": 46661, - "low": 82485, - "open": 91547, - "volume": 827439 - }, - "open": "2020-04-21T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 551461, - "high": 600000, - "low": 7, - "open": 20000, - "volume": 3064156 - }, - "id": 2587546, - "non_hive": { - "close": 84302, - "high": 92220, - "low": 1, - "open": 3060, - "volume": 469078 - }, - "open": "2020-04-21T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3545, - "high": 32, - "low": 319034, - "open": 20019, - "volume": 3048025 - }, - "id": 2587568, - "non_hive": { - "close": 542, - "high": 5, - "low": 48599, - "open": 3063, - "volume": 469477 - }, - "open": "2020-04-21T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 573749, - "high": 156943, - "low": 342225, - "open": 16829, - "volume": 1476710 - }, - "id": 2587590, - "non_hive": { - "close": 86120, - "high": 24443, - "low": 51337, - "open": 2575, - "volume": 223232 - }, - "open": "2020-04-21T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7574, - "high": 7574, - "low": 233, - "open": 233, - "volume": 24594 - }, - "id": 2587606, - "non_hive": { - "close": 1174, - "high": 1174, - "low": 35, - "open": 35, - "volume": 3811 - }, - "open": "2020-04-21T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 562520, - "low": 12, - "open": 25639, - "volume": 2023707 - }, - "id": 2587616, - "non_hive": { - "close": 1, - "high": 87621, - "low": 1, - "open": 3974, - "volume": 308544 - }, - "open": "2020-04-21T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 582467, - "high": 582467, - "low": 14, - "open": 14, - "volume": 582755 - }, - "id": 2587632, - "non_hive": { - "close": 90724, - "high": 90724, - "low": 2, - "open": 2, - "volume": 90767 - }, - "open": "2020-04-21T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 199522, - "high": 1220, - "low": 22, - "open": 1220, - "volume": 650108 - }, - "id": 2587642, - "non_hive": { - "close": 30952, - "high": 190, - "low": 3, - "open": 190, - "volume": 100620 - }, - "open": "2020-04-21T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3569, - "high": 3569, - "low": 590343, - "open": 585356, - "volume": 1438900 - }, - "id": 2587665, - "non_hive": { - "close": 556, - "high": 556, - "low": 89056, - "open": 90716, - "volume": 220529 - }, - "open": "2020-04-22T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 192851, - "high": 61889, - "low": 40, - "open": 600000, - "volume": 3000040 - }, - "id": 2587679, - "non_hive": { - "close": 28928, - "high": 9350, - "low": 6, - "open": 90644, - "volume": 452103 - }, - "open": "2020-04-22T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 594604, - "high": 2080, - "low": 594604, - "open": 600000, - "volume": 3622713 - }, - "id": 2587697, - "non_hive": { - "close": 87585, - "high": 324, - "low": 87585, - "open": 90001, - "volume": 538038 - }, - "open": "2020-04-22T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90, - "high": 90, - "low": 10330, - "open": 10330, - "volume": 610420 - }, - "id": 2587721, - "non_hive": { - "close": 14, - "high": 14, - "low": 1521, - "open": 1521, - "volume": 89915 - }, - "open": "2020-04-22T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 600000, - "low": 600000, - "open": 600000, - "volume": 3010000 - }, - "id": 2587730, - "non_hive": { - "close": 88380, - "high": 88380, - "low": 88380, - "open": 88380, - "volume": 443373 - }, - "open": "2020-04-22T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 210, - "low": 7, - "open": 600000, - "volume": 2400007 - }, - "id": 2587747, - "non_hive": { - "close": 88560, - "high": 31, - "low": 1, - "open": 88440, - "volume": 353971 - }, - "open": "2020-04-22T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 195000, - "high": 20001, - "low": 600000, - "open": 600000, - "volume": 6222657 - }, - "id": 2587763, - "non_hive": { - "close": 28798, - "high": 3060, - "low": 88517, - "open": 88563, - "volume": 933298 - }, - "open": "2020-04-22T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 500, - "high": 587, - "low": 500, - "open": 600000, - "volume": 2401180 - }, - "id": 2587801, - "non_hive": { - "close": 74, - "high": 90, - "low": 74, - "open": 89415, - "volume": 359322 - }, - "open": "2020-04-22T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 29915, - "low": 15, - "open": 7517, - "volume": 4877757 - }, - "id": 2587821, - "non_hive": { - "close": 1, - "high": 4577, - "low": 2, - "open": 1150, - "volume": 729857 - }, - "open": "2020-04-22T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 599857, - "high": 92794, - "low": 590488, - "open": 600000, - "volume": 5189113 - }, - "id": 2587855, - "non_hive": { - "close": 87849, - "high": 13743, - "low": 86329, - "open": 88860, - "volume": 766045 - }, - "open": "2020-04-22T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13121, - "high": 6, - "low": 537634, - "open": 143, - "volume": 4230375 - }, - "id": 2587877, - "non_hive": { - "close": 1942, - "high": 1, - "low": 78602, - "open": 21, - "volume": 619662 - }, - "open": "2020-04-22T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 251, - "low": 28, - "open": 13283, - "volume": 3009650 - }, - "id": 2587916, - "non_hive": { - "close": 87059, - "high": 38, - "low": 4, - "open": 1942, - "volume": 438733 - }, - "open": "2020-04-22T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2584, - "high": 2584, - "low": 100000, - "open": 600000, - "volume": 2402584 - }, - "id": 2587941, - "non_hive": { - "close": 388, - "high": 388, - "low": 14509, - "open": 87059, - "volume": 348624 - }, - "open": "2020-04-22T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 597189, - "high": 41840, - "low": 600000, - "open": 600000, - "volume": 2444560 - }, - "id": 2587954, - "non_hive": { - "close": 86652, - "high": 6278, - "low": 87059, - "open": 87059, - "volume": 354923 - }, - "open": "2020-04-22T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 652, - "high": 652, - "low": 595918, - "open": 600000, - "volume": 5592528 - }, - "id": 2587972, - "non_hive": { - "close": 97, - "high": 97, - "low": 82475, - "open": 87060, - "volume": 800006 - }, - "open": "2020-04-22T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 75409, - "high": 2438, - "low": 2000, - "open": 2438, - "volume": 2479847 - }, - "id": 2587996, - "non_hive": { - "close": 10962, - "high": 362, - "low": 277, - "open": 362, - "volume": 344484 - }, - "open": "2020-04-22T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 259345, - "high": 99597, - "low": 600000, - "open": 105179, - "volume": 8318040 - }, - "id": 2588017, - "non_hive": { - "close": 37405, - "high": 14840, - "low": 83219, - "open": 14620, - "volume": 1203821 - }, - "open": "2020-04-22T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20690, - "high": 20690, - "low": 7, - "open": 350650, - "volume": 3021100 - }, - "id": 2588050, - "non_hive": { - "close": 3083, - "high": 3083, - "low": 1, - "open": 51651, - "volume": 442571 - }, - "open": "2020-04-22T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3296, - "high": 46810, - "low": 3296, - "open": 102225, - "volume": 4133717 - }, - "id": 2588071, - "non_hive": { - "close": 470, - "high": 7018, - "low": 470, - "open": 15035, - "volume": 602634 - }, - "open": "2020-04-22T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 96, - "low": 500000, - "open": 96, - "volume": 1823598 - }, - "id": 2588100, - "non_hive": { - "close": 86358, - "high": 14, - "low": 71303, - "open": 14, - "volume": 261714 - }, - "open": "2020-04-22T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 3953, - "low": 459223, - "open": 23666, - "volume": 3003953 - }, - "id": 2588118, - "non_hive": { - "close": 86360, - "high": 591, - "low": 66096, - "open": 3408, - "volume": 432432 - }, - "open": "2020-04-22T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 582256, - "high": 99725, - "low": 600000, - "open": 600000, - "volume": 2985401 - }, - "id": 2588137, - "non_hive": { - "close": 87087, - "high": 14918, - "low": 86360, - "open": 86360, - "volume": 436361 - }, - "open": "2020-04-22T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 210850, - "high": 296425, - "low": 14, - "open": 54770, - "volume": 9508670 - }, - "id": 2588156, - "non_hive": { - "close": 30761, - "high": 49755, - "low": 2, - "open": 8190, - "volume": 1451033 - }, - "open": "2020-04-22T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 31864, - "low": 7, - "open": 600000, - "volume": 7772214 - }, - "id": 2588200, - "non_hive": { - "close": 88717, - "high": 5097, - "low": 1, - "open": 90470, - "volume": 1180610 - }, - "open": "2020-04-22T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299997, - "high": 6, - "low": 500000, - "open": 100000, - "volume": 4972700 - }, - "id": 2588236, - "non_hive": { - "close": 44700, - "high": 1, - "low": 74000, - "open": 14900, - "volume": 743513 - }, - "open": "2020-04-23T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 528386, - "high": 78633, - "low": 41, - "open": 300003, - "volume": 1882209 - }, - "id": 2588274, - "non_hive": { - "close": 78137, - "high": 12162, - "low": 6, - "open": 44704, - "volume": 279768 - }, - "open": "2020-04-23T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 600000, - "low": 600000, - "open": 82223, - "volume": 3600000 - }, - "id": 2588290, - "non_hive": { - "close": 88775, - "high": 88775, - "low": 88727, - "open": 12162, - "volume": 532505 - }, - "open": "2020-04-23T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 169743, - "high": 4500, - "low": 169743, - "open": 3001, - "volume": 607501 - }, - "id": 2588309, - "non_hive": { - "close": 25125, - "high": 696, - "low": 25125, - "open": 464, - "volume": 90818 - }, - "open": "2020-04-23T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10330, - "high": 19015, - "low": 10330, - "open": 19015, - "volume": 29345 - }, - "id": 2588319, - "non_hive": { - "close": 1549, - "high": 2947, - "low": 1549, - "open": 2947, - "volume": 4496 - }, - "open": "2020-04-23T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33382, - "high": 33382, - "low": 57, - "open": 3629, - "volume": 487791 - }, - "id": 2588326, - "non_hive": { - "close": 5170, - "high": 5170, - "low": 8, - "open": 562, - "volume": 74349 - }, - "open": "2020-04-23T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29731, - "high": 29731, - "low": 657, - "open": 291721, - "volume": 15894945 - }, - "id": 2588350, - "non_hive": { - "close": 5289, - "high": 5289, - "low": 101, - "open": 44882, - "volume": 2584831 - }, - "open": "2020-04-23T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3175, - "high": 360715, - "low": 95, - "open": 64637, - "volume": 6303679 - }, - "id": 2588420, - "non_hive": { - "close": 581, - "high": 66011, - "low": 16, - "open": 10889, - "volume": 1113019 - }, - "open": "2020-04-23T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11391, - "high": 147, - "low": 6, - "open": 3825, - "volume": 7023100 - }, - "id": 2588464, - "non_hive": { - "close": 1955, - "high": 27, - "low": 1, - "open": 699, - "volume": 1222528 - }, - "open": "2020-04-23T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 415649, - "high": 99492, - "low": 130794, - "open": 3530, - "volume": 5286539 - }, - "id": 2588494, - "non_hive": { - "close": 74973, - "high": 18802, - "low": 22234, - "open": 638, - "volume": 964821 - }, - "open": "2020-04-23T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 110741, - "high": 10303, - "low": 437916, - "open": 10303, - "volume": 558960 - }, - "id": 2588537, - "non_hive": { - "close": 20000, - "high": 1946, - "low": 79062, - "open": 1946, - "volume": 101008 - }, - "open": "2020-04-23T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 101962, - "high": 1108, - "low": 28, - "open": 110703, - "volume": 8577377 - }, - "id": 2588547, - "non_hive": { - "close": 18507, - "high": 215, - "low": 5, - "open": 20000, - "volume": 1620585 - }, - "open": "2020-04-23T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 461, - "high": 7084, - "low": 114585, - "open": 410689, - "volume": 1195816 - }, - "id": 2588593, - "non_hive": { - "close": 84, - "high": 1371, - "low": 20831, - "open": 74669, - "volume": 219011 - }, - "open": "2020-04-23T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45036, - "high": 572, - "low": 30259, - "open": 30259, - "volume": 196967 - }, - "id": 2588622, - "non_hive": { - "close": 8625, - "high": 110, - "low": 5548, - "open": 5548, - "volume": 37526 - }, - "open": "2020-04-23T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 414, - "high": 338, - "low": 11897, - "open": 338, - "volume": 12649 - }, - "id": 2588638, - "non_hive": { - "close": 78, - "high": 65, - "low": 2238, - "open": 65, - "volume": 2381 - }, - "open": "2020-04-23T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2520, - "high": 1010, - "low": 1599, - "open": 1758, - "volume": 653396 - }, - "id": 2588648, - "non_hive": { - "close": 462, - "high": 191, - "low": 293, - "open": 330, - "volume": 123287 - }, - "open": "2020-04-23T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1084, - "high": 337534, - "low": 2616, - "open": 1646, - "volume": 480760 - }, - "id": 2588680, - "non_hive": { - "close": 208, - "high": 64769, - "low": 483, - "open": 311, - "volume": 91562 - }, - "open": "2020-04-23T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10406, - "high": 5351, - "low": 11, - "open": 5000, - "volume": 262886 - }, - "id": 2588699, - "non_hive": { - "close": 1995, - "high": 1027, - "low": 2, - "open": 924, - "volume": 48987 - }, - "open": "2020-04-23T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3237, - "high": 22210, - "low": 2026, - "open": 20000, - "volume": 521119 - }, - "id": 2588721, - "non_hive": { - "close": 607, - "high": 4309, - "low": 374, - "open": 3820, - "volume": 100074 - }, - "open": "2020-04-23T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 143307, - "high": 143307, - "low": 5000, - "open": 1897, - "volume": 230219 - }, - "id": 2588738, - "non_hive": { - "close": 28074, - "high": 28074, - "low": 937, - "open": 369, - "volume": 44983 - }, - "open": "2020-04-23T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6256, - "high": 15, - "low": 100000, - "open": 15, - "volume": 106301 - }, - "id": 2588753, - "non_hive": { - "close": 1225, - "high": 3, - "low": 19575, - "open": 3, - "volume": 20809 - }, - "open": "2020-04-23T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 363287, - "high": 3503, - "low": 732, - "open": 1001, - "volume": 3206194 - }, - "id": 2588761, - "non_hive": { - "close": 70314, - "high": 686, - "low": 141, - "open": 196, - "volume": 620569 - }, - "open": "2020-04-23T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8231, - "high": 332494, - "low": 11, - "open": 787, - "volume": 3572951 - }, - "id": 2588780, - "non_hive": { - "close": 1510, - "high": 65169, - "low": 2, - "open": 152, - "volume": 690435 - }, - "open": "2020-04-23T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 243522, - "high": 150859, - "low": 11, - "open": 731, - "volume": 2608971 - }, - "id": 2588808, - "non_hive": { - "close": 45539, - "high": 30153, - "low": 2, - "open": 143, - "volume": 489443 - }, - "open": "2020-04-23T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 119470, - "high": 5, - "low": 81, - "open": 600000, - "volume": 3690395 - }, - "id": 2588832, - "non_hive": { - "close": 22352, - "high": 1, - "low": 15, - "open": 112201, - "volume": 692639 - }, - "open": "2020-04-24T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 129390, - "high": 1228, - "low": 74096, - "open": 220, - "volume": 9400258 - }, - "id": 2588866, - "non_hive": { - "close": 25917, - "high": 246, - "low": 14085, - "open": 44, - "volume": 1857493 - }, - "open": "2020-04-24T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 87770, - "high": 87770, - "low": 299999, - "open": 300001, - "volume": 3196668 - }, - "id": 2588913, - "non_hive": { - "close": 19149, - "high": 19149, - "low": 58510, - "open": 58511, - "volume": 665355 - }, - "open": "2020-04-24T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50000, - "high": 50000, - "low": 153, - "open": 77, - "volume": 1621310 - }, - "id": 2588935, - "non_hive": { - "close": 11500, - "high": 11500, - "low": 33, - "open": 17, - "volume": 369506 - }, - "open": "2020-04-24T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53843, - "high": 53843, - "low": 56, - "open": 56, - "volume": 53899 - }, - "id": 2588951, - "non_hive": { - "close": 12384, - "high": 12384, - "low": 11, - "open": 11, - "volume": 12395 - }, - "open": "2020-04-24T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23458, - "high": 621, - "low": 10, - "open": 10, - "volume": 35341 - }, - "id": 2588958, - "non_hive": { - "close": 5548, - "high": 147, - "low": 2, - "open": 2, - "volume": 8285 - }, - "open": "2020-04-24T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 258296, - "high": 253, - "low": 258296, - "open": 3319, - "volume": 1870752 - }, - "id": 2588971, - "non_hive": { - "close": 48288, - "high": 60, - "low": 48288, - "open": 667, - "volume": 367544 - }, - "open": "2020-04-24T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 370309, - "high": 370309, - "low": 100, - "open": 13427, - "volume": 450610 - }, - "id": 2588987, - "non_hive": { - "close": 82764, - "high": 82764, - "low": 18, - "open": 2994, - "volume": 100691 - }, - "open": "2020-04-24T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 566, - "low": 234111, - "open": 566, - "volume": 1200000 - }, - "id": 2589001, - "non_hive": { - "close": 113400, - "high": 119, - "low": 44246, - "open": 119, - "volume": 226896 - }, - "open": "2020-04-24T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 89754, - "high": 89754, - "low": 89754, - "open": 89754, - "volume": 89754 - }, - "id": 2589008, - "non_hive": { - "close": 20000, - "high": 20000, - "low": 20000, - "open": 20000, - "volume": 20000 - }, - "open": "2020-04-24T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 119660, - "high": 119660, - "low": 11, - "open": 543, - "volume": 9877329 - }, - "id": 2589012, - "non_hive": { - "close": 30693, - "high": 30693, - "low": 2, - "open": 120, - "volume": 2304906 - }, - "open": "2020-04-24T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2938, - "high": 2938, - "low": 340, - "open": 340, - "volume": 4281036 - }, - "id": 2589068, - "non_hive": { - "close": 1005, - "high": 1005, - "low": 87, - "open": 87, - "volume": 1283781 - }, - "open": "2020-04-24T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27687, - "high": 4771, - "low": 800, - "open": 1629, - "volume": 500729 - }, - "id": 2589112, - "non_hive": { - "close": 8036, - "high": 1632, - "low": 201, - "open": 501, - "volume": 162931 - }, - "open": "2020-04-24T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 251572, - "high": 10486, - "low": 32, - "open": 28266, - "volume": 9899578 - }, - "id": 2589142, - "non_hive": { - "close": 72956, - "high": 3542, - "low": 8, - "open": 8197, - "volume": 2870101 - }, - "open": "2020-04-24T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2198, - "high": 20900, - "low": 548, - "open": 548, - "volume": 1137866 - }, - "id": 2589196, - "non_hive": { - "close": 725, - "high": 7148, - "low": 158, - "open": 158, - "volume": 365118 - }, - "open": "2020-04-24T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 448, - "high": 15224, - "low": 382, - "open": 40522, - "volume": 5722564 - }, - "id": 2589231, - "non_hive": { - "close": 177, - "high": 6241, - "low": 127, - "open": 13506, - "volume": 2164973 - }, - "open": "2020-04-24T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35526, - "high": 6835, - "low": 3, - "open": 195815, - "volume": 1588147 - }, - "id": 2589291, - "non_hive": { - "close": 15009, - "high": 2888, - "low": 1, - "open": 77347, - "volume": 639947 - }, - "open": "2020-04-24T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11507, - "high": 7, - "low": 1969, - "open": 85, - "volume": 2790624 - }, - "id": 2589326, - "non_hive": { - "close": 4717, - "high": 3, - "low": 748, - "open": 36, - "volume": 1125494 - }, - "open": "2020-04-24T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1163, - "high": 33, - "low": 99, - "open": 4891, - "volume": 686810 - }, - "id": 2589393, - "non_hive": { - "close": 492, - "high": 14, - "low": 37, - "open": 2000, - "volume": 274451 - }, - "open": "2020-04-24T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4529, - "high": 4529, - "low": 9837, - "open": 3560, - "volume": 34748 - }, - "id": 2589421, - "non_hive": { - "close": 1916, - "high": 1916, - "low": 3698, - "open": 1353, - "volume": 13357 - }, - "open": "2020-04-24T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9893, - "high": 241, - "low": 298, - "open": 29217, - "volume": 886493 - }, - "id": 2589442, - "non_hive": { - "close": 4254, - "high": 104, - "low": 119, - "open": 12271, - "volume": 370065 - }, - "open": "2020-04-24T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6681, - "high": 2222, - "low": 110, - "open": 35354, - "volume": 274205 - }, - "id": 2589473, - "non_hive": { - "close": 2873, - "high": 1000, - "low": 40, - "open": 14848, - "volume": 116216 - }, - "open": "2020-04-24T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 66, - "low": 11, - "open": 415, - "volume": 20063 - }, - "id": 2589512, - "non_hive": { - "close": 4, - "high": 30, - "low": 4, - "open": 166, - "volume": 8844 - }, - "open": "2020-04-24T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24392, - "high": 6482, - "low": 501, - "open": 3801, - "volume": 49035 - }, - "id": 2589534, - "non_hive": { - "close": 10976, - "high": 2917, - "low": 215, - "open": 1709, - "volume": 21946 - }, - "open": "2020-04-25T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16545, - "high": 14903, - "low": 44, - "open": 44238, - "volume": 91064 - }, - "id": 2589547, - "non_hive": { - "close": 7346, - "high": 6632, - "low": 18, - "open": 19686, - "volume": 40264 - }, - "open": "2020-04-25T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11488, - "high": 97, - "low": 3, - "open": 3, - "volume": 51547 - }, - "id": 2589562, - "non_hive": { - "close": 5090, - "high": 43, - "low": 1, - "open": 1, - "volume": 21775 - }, - "open": "2020-04-25T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30673, - "high": 2720, - "low": 89, - "open": 27263, - "volume": 122131 - }, - "id": 2589575, - "non_hive": { - "close": 13619, - "high": 1208, - "low": 39, - "open": 12078, - "volume": 54178 - }, - "open": "2020-04-25T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 188548, - "high": 19276, - "low": 5000, - "open": 981, - "volume": 6899369 - }, - "id": 2589601, - "non_hive": { - "close": 77305, - "high": 8578, - "low": 2000, - "open": 436, - "volume": 2830907 - }, - "open": "2020-04-25T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23857, - "high": 513, - "low": 11, - "open": 188548, - "volume": 3241503 - }, - "id": 2589655, - "non_hive": { - "close": 10493, - "high": 226, - "low": 4, - "open": 77305, - "volume": 1330588 - }, - "open": "2020-04-25T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12792, - "high": 795, - "low": 27, - "open": 30192, - "volume": 95785 - }, - "id": 2589685, - "non_hive": { - "close": 5626, - "high": 350, - "low": 10, - "open": 13279, - "volume": 42127 - }, - "open": "2020-04-25T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1802, - "high": 13287, - "low": 95, - "open": 13287, - "volume": 73899 - }, - "id": 2589703, - "non_hive": { - "close": 789, - "high": 5844, - "low": 36, - "open": 5844, - "volume": 32457 - }, - "open": "2020-04-25T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 486, - "low": 5, - "open": 486, - "volume": 1957 - }, - "id": 2589726, - "non_hive": { - "close": 2, - "high": 213, - "low": 2, - "open": 213, - "volume": 857 - }, - "open": "2020-04-25T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 354, - "high": 1000, - "low": 5242, - "open": 5242, - "volume": 10570 - }, - "id": 2589736, - "non_hive": { - "close": 155, - "high": 438, - "low": 2295, - "open": 2295, - "volume": 4628 - }, - "open": "2020-04-25T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2097, - "high": 50, - "low": 17289, - "open": 4420, - "volume": 451846 - }, - "id": 2589749, - "non_hive": { - "close": 902, - "high": 22, - "low": 6414, - "open": 1768, - "volume": 175420 - }, - "open": "2020-04-25T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 175, - "high": 367, - "low": 175, - "open": 22010, - "volume": 862948 - }, - "id": 2589779, - "non_hive": { - "close": 68, - "high": 158, - "low": 68, - "open": 9105, - "volume": 361009 - }, - "open": "2020-04-25T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18356, - "high": 202, - "low": 18489, - "open": 121, - "volume": 211545 - }, - "id": 2589810, - "non_hive": { - "close": 7981, - "high": 88, - "low": 7395, - "open": 51, - "volume": 87144 - }, - "open": "2020-04-25T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12576, - "high": 48, - "low": 100000, - "open": 102, - "volume": 128197 - }, - "id": 2589843, - "non_hive": { - "close": 5408, - "high": 21, - "low": 41000, - "open": 44, - "volume": 53126 - }, - "open": "2020-04-25T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 320, - "high": 18, - "low": 8458, - "open": 6633, - "volume": 203899 - }, - "id": 2589870, - "non_hive": { - "close": 138, - "high": 8, - "low": 3140, - "open": 2852, - "volume": 87180 - }, - "open": "2020-04-25T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17185, - "high": 21190, - "low": 31, - "open": 31, - "volume": 15574440 - }, - "id": 2589893, - "non_hive": { - "close": 9451, - "high": 11655, - "low": 13, - "open": 13, - "volume": 6897447 - }, - "open": "2020-04-25T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7846, - "high": 25, - "low": 1660, - "open": 3550, - "volume": 107554 - }, - "id": 2589985, - "non_hive": { - "close": 4628, - "high": 15, - "low": 887, - "open": 2095, - "volume": 62608 - }, - "open": "2020-04-25T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15, - "high": 15, - "low": 11, - "open": 1802, - "volume": 257812 - }, - "id": 2590030, - "non_hive": { - "close": 9, - "high": 9, - "low": 5, - "open": 1063, - "volume": 126844 - }, - "open": "2020-04-25T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 228, - "high": 78, - "low": 5, - "open": 33576, - "volume": 73764 - }, - "id": 2590061, - "non_hive": { - "close": 111, - "high": 46, - "low": 2, - "open": 19776, - "volume": 41765 - }, - "open": "2020-04-25T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 432, - "high": 76, - "low": 13, - "open": 1054, - "volume": 57847 - }, - "id": 2590100, - "non_hive": { - "close": 238, - "high": 42, - "low": 6, - "open": 558, - "volume": 30811 - }, - "open": "2020-04-25T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3794, - "high": 125, - "low": 10104, - "open": 1273, - "volume": 49857 - }, - "id": 2590133, - "non_hive": { - "close": 2087, - "high": 74, - "low": 5395, - "open": 700, - "volume": 27350 - }, - "open": "2020-04-25T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6631, - "high": 2114, - "low": 35550, - "open": 10517, - "volume": 736281 - }, - "id": 2590161, - "non_hive": { - "close": 3312, - "high": 1140, - "low": 13580, - "open": 5395, - "volume": 308055 - }, - "open": "2020-04-25T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3511, - "high": 3338, - "low": 13, - "open": 6499, - "volume": 1351514 - }, - "id": 2590213, - "non_hive": { - "close": 1893, - "high": 1800, - "low": 6, - "open": 3246, - "volume": 675801 - }, - "open": "2020-04-25T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6910, - "high": 40, - "low": 401, - "open": 2212, - "volume": 98431 - }, - "id": 2590283, - "non_hive": { - "close": 3726, - "high": 22, - "low": 188, - "open": 1193, - "volume": 53035 - }, - "open": "2020-04-25T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 83, - "high": 16, - "low": 266, - "open": 777, - "volume": 4854 - }, - "id": 2590314, - "non_hive": { - "close": 45, - "high": 9, - "low": 125, - "open": 419, - "volume": 2600 - }, - "open": "2020-04-26T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 307, - "high": 1272, - "low": 41, - "open": 41, - "volume": 1908 - }, - "id": 2590331, - "non_hive": { - "close": 165, - "high": 685, - "low": 22, - "open": 22, - "volume": 1027 - }, - "open": "2020-04-26T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16368, - "high": 5967, - "low": 6608, - "open": 832, - "volume": 1544626 - }, - "id": 2590344, - "non_hive": { - "close": 6072, - "high": 3217, - "low": 2451, - "open": 391, - "volume": 650247 - }, - "open": "2020-04-26T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3178, - "high": 1730, - "low": 2, - "open": 13684, - "volume": 570365 - }, - "id": 2590491, - "non_hive": { - "close": 1713, - "high": 933, - "low": 1, - "open": 7377, - "volume": 307488 - }, - "open": "2020-04-26T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24786, - "high": 36065, - "low": 75, - "open": 10812, - "volume": 9797311 - }, - "id": 2590581, - "non_hive": { - "close": 13384, - "high": 19475, - "low": 40, - "open": 5828, - "volume": 5281654 - }, - "open": "2020-04-26T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 866, - "high": 866, - "low": 17097, - "open": 9230, - "volume": 1246838 - }, - "id": 2590694, - "non_hive": { - "close": 511, - "high": 511, - "low": 7608, - "open": 5343, - "volume": 664405 - }, - "open": "2020-04-26T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6412, - "high": 181, - "low": 426, - "open": 14039, - "volume": 402945 - }, - "id": 2590827, - "non_hive": { - "close": 3719, - "high": 107, - "low": 187, - "open": 6458, - "volume": 225673 - }, - "open": "2020-04-26T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 8, - "low": 117, - "open": 138, - "volume": 301146 - }, - "id": 2590910, - "non_hive": { - "close": 6000, - "high": 5, - "low": 67, - "open": 80, - "volume": 174878 - }, - "open": "2020-04-26T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 68360, - "high": 1, - "low": 7, - "open": 1293, - "volume": 231255 - }, - "id": 2590946, - "non_hive": { - "close": 41052, - "high": 1, - "low": 4, - "open": 838, - "volume": 144958 - }, - "open": "2020-04-26T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 161, - "high": 161, - "low": 7, - "open": 158, - "volume": 668874 - }, - "id": 2590989, - "non_hive": { - "close": 113, - "high": 113, - "low": 4, - "open": 94, - "volume": 448623 - }, - "open": "2020-04-26T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1307, - "high": 518, - "low": 48, - "open": 337, - "volume": 663108 - }, - "id": 2591051, - "non_hive": { - "close": 1046, - "high": 415, - "low": 33, - "open": 236, - "volume": 494141 - }, - "open": "2020-04-26T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14205, - "high": 42, - "low": 12, - "open": 2509, - "volume": 1266285 - }, - "id": 2591115, - "non_hive": { - "close": 12075, - "high": 36, - "low": 9, - "open": 2007, - "volume": 992967 - }, - "open": "2020-04-26T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 244, - "high": 4, - "low": 108, - "open": 2353, - "volume": 452904 - }, - "id": 2591197, - "non_hive": { - "close": 208, - "high": 4, - "low": 84, - "open": 2000, - "volume": 356564 - }, - "open": "2020-04-26T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 156, - "high": 493, - "low": 359, - "open": 1177, - "volume": 1099671 - }, - "id": 2591241, - "non_hive": { - "close": 132, - "high": 463, - "low": 280, - "open": 1000, - "volume": 968878 - }, - "open": "2020-04-26T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7266, - "high": 286, - "low": 1209, - "open": 151, - "volume": 756442 - }, - "id": 2591327, - "non_hive": { - "close": 6808, - "high": 269, - "low": 858, - "open": 142, - "volume": 605327 - }, - "open": "2020-04-26T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8641, - "high": 267, - "low": 91011, - "open": 867, - "volume": 394059 - }, - "id": 2591392, - "non_hive": { - "close": 6739, - "high": 251, - "low": 65763, - "open": 812, - "volume": 301319 - }, - "open": "2020-04-26T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 336, - "high": 35, - "low": 80187, - "open": 98425, - "volume": 1882800 - }, - "id": 2591440, - "non_hive": { - "close": 276, - "high": 29, - "low": 57818, - "open": 79182, - "volume": 1493373 - }, - "open": "2020-04-26T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 595, - "high": 237, - "low": 3696, - "open": 1525, - "volume": 224838 - }, - "id": 2591518, - "non_hive": { - "close": 488, - "high": 195, - "low": 2956, - "open": 1250, - "volume": 183947 - }, - "open": "2020-04-26T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10975, - "high": 185, - "low": 34402, - "open": 2756, - "volume": 113822 - }, - "id": 2591551, - "non_hive": { - "close": 9000, - "high": 152, - "low": 27521, - "open": 2260, - "volume": 92577 - }, - "open": "2020-04-26T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3343, - "high": 64, - "low": 58095, - "open": 6109, - "volume": 206960 - }, - "id": 2591592, - "non_hive": { - "close": 3000, - "high": 58, - "low": 47637, - "open": 5010, - "volume": 180752 - }, - "open": "2020-04-26T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2115, - "high": 140, - "low": 63, - "open": 140, - "volume": 53684 - }, - "id": 2591615, - "non_hive": { - "close": 1897, - "high": 126, - "low": 50, - "open": 126, - "volume": 48157 - }, - "open": "2020-04-26T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7800, - "high": 3268, - "low": 6287, - "open": 15739, - "volume": 2826283 - }, - "id": 2591637, - "non_hive": { - "close": 7020, - "high": 2942, - "low": 5033, - "open": 13680, - "volume": 2488723 - }, - "open": "2020-04-26T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 268, - "high": 268, - "low": 251, - "open": 87286, - "volume": 4068951 - }, - "id": 2591700, - "non_hive": { - "close": 255, - "high": 255, - "low": 193, - "open": 78558, - "volume": 3665332 - }, - "open": "2020-04-26T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10535, - "high": 367, - "low": 12, - "open": 367, - "volume": 90252 - }, - "id": 2591767, - "non_hive": { - "close": 9935, - "high": 349, - "low": 9, - "open": 349, - "volume": 85168 - }, - "open": "2020-04-26T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24188, - "high": 154, - "low": 61, - "open": 10324, - "volume": 722252 - }, - "id": 2591809, - "non_hive": { - "close": 22801, - "high": 146, - "low": 57, - "open": 9736, - "volume": 680995 - }, - "open": "2020-04-27T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3828, - "high": 15, - "low": 28, - "open": 61, - "volume": 390288 - }, - "id": 2591859, - "non_hive": { - "close": 3614, - "high": 15, - "low": 26, - "open": 57, - "volume": 376038 - }, - "open": "2020-04-27T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4866, - "high": 208, - "low": 122, - "open": 172, - "volume": 631703 - }, - "id": 2591891, - "non_hive": { - "close": 4693, - "high": 201, - "low": 114, - "open": 163, - "volume": 596929 - }, - "open": "2020-04-27T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 324, - "high": 43, - "low": 65, - "open": 453, - "volume": 991568 - }, - "id": 2591961, - "non_hive": { - "close": 291, - "high": 42, - "low": 58, - "open": 437, - "volume": 930047 - }, - "open": "2020-04-27T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6986, - "high": 48, - "low": 182, - "open": 103706, - "volume": 1311635 - }, - "id": 2592011, - "non_hive": { - "close": 6739, - "high": 47, - "low": 163, - "open": 99999, - "volume": 1208037 - }, - "open": "2020-04-27T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 872, - "high": 78, - "low": 12, - "open": 1070, - "volume": 378419 - }, - "id": 2592075, - "non_hive": { - "close": 838, - "high": 76, - "low": 10, - "open": 1032, - "volume": 349451 - }, - "open": "2020-04-27T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 802, - "high": 2855, - "low": 29, - "open": 2855, - "volume": 63786 - }, - "id": 2592120, - "non_hive": { - "close": 766, - "high": 2741, - "low": 23, - "open": 2741, - "volume": 59201 - }, - "open": "2020-04-27T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 903, - "high": 8668, - "low": 94, - "open": 1709, - "volume": 264933 - }, - "id": 2592146, - "non_hive": { - "close": 813, - "high": 8235, - "low": 75, - "open": 1623, - "volume": 230106 - }, - "open": "2020-04-27T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13175, - "high": 423, - "low": 3, - "open": 19226, - "volume": 163738 - }, - "id": 2592190, - "non_hive": { - "close": 12241, - "high": 398, - "low": 2, - "open": 17300, - "volume": 149783 - }, - "open": "2020-04-27T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1968, - "high": 22, - "low": 4758, - "open": 22, - "volume": 20151 - }, - "id": 2592237, - "non_hive": { - "close": 1821, - "high": 21, - "low": 4283, - "open": 21, - "volume": 18572 - }, - "open": "2020-04-27T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1823, - "high": 947, - "low": 2220, - "open": 1182, - "volume": 120169 - }, - "id": 2592253, - "non_hive": { - "close": 1679, - "high": 877, - "low": 1898, - "open": 1088, - "volume": 108153 - }, - "open": "2020-04-27T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7491, - "high": 2673, - "low": 6957, - "open": 601, - "volume": 1292812 - }, - "id": 2592286, - "non_hive": { - "close": 6654, - "high": 2470, - "low": 5411, - "open": 553, - "volume": 1101737 - }, - "open": "2020-04-27T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10981, - "high": 1, - "low": 854, - "open": 4923, - "volume": 89014 - }, - "id": 2592327, - "non_hive": { - "close": 9006, - "high": 1, - "low": 614, - "open": 3829, - "volume": 69997 - }, - "open": "2020-04-27T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3408, - "high": 8, - "low": 2, - "open": 67, - "volume": 311214 - }, - "id": 2592363, - "non_hive": { - "close": 2900, - "high": 8, - "low": 1, - "open": 54, - "volume": 249588 - }, - "open": "2020-04-27T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 93403, - "high": 14, - "low": 48, - "open": 3312, - "volume": 390429 - }, - "id": 2592421, - "non_hive": { - "close": 79392, - "high": 12, - "low": 39, - "open": 2816, - "volume": 324027 - }, - "open": "2020-04-27T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32, - "high": 9880, - "low": 32, - "open": 1636, - "volume": 1234319 - }, - "id": 2592457, - "non_hive": { - "close": 21, - "high": 8398, - "low": 21, - "open": 1375, - "volume": 902011 - }, - "open": "2020-04-27T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1044, - "high": 56, - "low": 1218, - "open": 9484, - "volume": 211323 - }, - "id": 2592504, - "non_hive": { - "close": 888, - "high": 48, - "low": 1000, - "open": 8061, - "volume": 179585 - }, - "open": "2020-04-27T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1039, - "high": 5747, - "low": 1039, - "open": 906, - "volume": 528251 - }, - "id": 2592530, - "non_hive": { - "close": 634, - "high": 4598, - "low": 634, - "open": 618, - "volume": 358572 - }, - "open": "2020-04-27T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3243, - "high": 32, - "low": 1539, - "open": 1539, - "volume": 360167 - }, - "id": 2592578, - "non_hive": { - "close": 2043, - "high": 23, - "low": 938, - "open": 938, - "volume": 237207 - }, - "open": "2020-04-27T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 868, - "high": 868, - "low": 19950, - "open": 1039, - "volume": 140291 - }, - "id": 2592613, - "non_hive": { - "close": 608, - "high": 608, - "low": 12967, - "open": 727, - "volume": 93793 - }, - "open": "2020-04-27T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29285, - "high": 118, - "low": 5337, - "open": 3747, - "volume": 335327 - }, - "id": 2592640, - "non_hive": { - "close": 20500, - "high": 83, - "low": 3468, - "open": 2586, - "volume": 226658 - }, - "open": "2020-04-27T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6791, - "high": 13002, - "low": 3581, - "open": 12810, - "volume": 106979 - }, - "id": 2592686, - "non_hive": { - "close": 4753, - "high": 9102, - "low": 2506, - "open": 8967, - "volume": 74885 - }, - "open": "2020-04-27T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20433, - "high": 651, - "low": 13, - "open": 651, - "volume": 36595 - }, - "id": 2592706, - "non_hive": { - "close": 14302, - "high": 456, - "low": 9, - "open": 456, - "volume": 25615 - }, - "open": "2020-04-27T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 367, - "high": 52, - "low": 11, - "open": 52, - "volume": 261951 - }, - "id": 2592722, - "non_hive": { - "close": 239, - "high": 37, - "low": 7, - "open": 37, - "volume": 173509 - }, - "open": "2020-04-27T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 770, - "high": 770, - "low": 18195, - "open": 137304, - "volume": 315418 - }, - "id": 2592750, - "non_hive": { - "close": 501, - "high": 501, - "low": 11106, - "open": 83813, - "volume": 197000 - }, - "open": "2020-04-28T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5009, - "high": 5009, - "low": 42, - "open": 5554, - "volume": 68542 - }, - "id": 2592775, - "non_hive": { - "close": 3256, - "high": 3256, - "low": 27, - "open": 3610, - "volume": 44550 - }, - "open": "2020-04-28T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1531, - "high": 161, - "low": 1531, - "open": 35801, - "volume": 51036 - }, - "id": 2592798, - "non_hive": { - "close": 995, - "high": 105, - "low": 995, - "open": 23269, - "volume": 33172 - }, - "open": "2020-04-28T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2, - "high": 183, - "low": 2, - "open": 5207, - "volume": 33221 - }, - "id": 2592812, - "non_hive": { - "close": 1, - "high": 119, - "low": 1, - "open": 3384, - "volume": 21227 - }, - "open": "2020-04-28T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3242, - "high": 496, - "low": 64, - "open": 15084, - "volume": 26030 - }, - "id": 2592828, - "non_hive": { - "close": 2107, - "high": 323, - "low": 38, - "open": 9804, - "volume": 16740 - }, - "open": "2020-04-28T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1278, - "high": 1278, - "low": 12, - "open": 13111, - "volume": 18081 - }, - "id": 2592851, - "non_hive": { - "close": 831, - "high": 831, - "low": 7, - "open": 8521, - "volume": 11751 - }, - "open": "2020-04-28T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23330, - "high": 70, - "low": 27, - "open": 530, - "volume": 123687 - }, - "id": 2592863, - "non_hive": { - "close": 15162, - "high": 46, - "low": 16, - "open": 345, - "volume": 80381 - }, - "open": "2020-04-28T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17473, - "high": 46, - "low": 17473, - "open": 3367, - "volume": 196712 - }, - "id": 2592890, - "non_hive": { - "close": 9610, - "high": 30, - "low": 9610, - "open": 2188, - "volume": 117156 - }, - "open": "2020-04-28T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13393, - "high": 1588, - "low": 13, - "open": 709, - "volume": 426547 - }, - "id": 2592923, - "non_hive": { - "close": 6961, - "high": 950, - "low": 6, - "open": 390, - "volume": 215913 - }, - "open": "2020-04-28T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 283, - "high": 372, - "low": 3000, - "open": 13057, - "volume": 1066741 - }, - "id": 2592964, - "non_hive": { - "close": 144, - "high": 196, - "low": 1470, - "open": 6786, - "volume": 544825 - }, - "open": "2020-04-28T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21229, - "high": 1073, - "low": 127500, - "open": 684, - "volume": 660958 - }, - "id": 2593026, - "non_hive": { - "close": 12483, - "high": 640, - "low": 65080, - "open": 389, - "volume": 382608 - }, - "open": "2020-04-28T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 53, - "low": 28, - "open": 24392, - "volume": 431782 - }, - "id": 2593060, - "non_hive": { - "close": 5596, - "high": 31, - "low": 14, - "open": 12452, - "volume": 225403 - }, - "open": "2020-04-28T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1408, - "high": 1485, - "low": 40503, - "open": 7013, - "volume": 83936 - }, - "id": 2593114, - "non_hive": { - "close": 816, - "high": 861, - "low": 20656, - "open": 3925, - "volume": 45052 - }, - "open": "2020-04-28T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39701, - "high": 119, - "low": 39701, - "open": 175, - "volume": 118719 - }, - "id": 2593135, - "non_hive": { - "close": 19096, - "high": 69, - "low": 19096, - "open": 101, - "volume": 58609 - }, - "open": "2020-04-28T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 78, - "high": 75, - "low": 149774, - "open": 551, - "volume": 477367 - }, - "id": 2593151, - "non_hive": { - "close": 40, - "high": 42, - "low": 71891, - "open": 306, - "volume": 241133 - }, - "open": "2020-04-28T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 828, - "high": 57, - "low": 7088, - "open": 947, - "volume": 1879549 - }, - "id": 2593180, - "non_hive": { - "close": 419, - "high": 31, - "low": 3012, - "open": 481, - "volume": 872526 - }, - "open": "2020-04-28T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 149900, - "high": 38169, - "low": 46283, - "open": 61, - "volume": 17171321 - }, - "id": 2593234, - "non_hive": { - "close": 49467, - "high": 19175, - "low": 9258, - "open": 26, - "volume": 5089604 - }, - "open": "2020-04-28T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1297, - "high": 824, - "low": 10, - "open": 159797, - "volume": 4083555 - }, - "id": 2593367, - "non_hive": { - "close": 506, - "high": 366, - "low": 2, - "open": 52733, - "volume": 1428070 - }, - "open": "2020-04-28T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 204, - "high": 3597, - "low": 132, - "open": 3597, - "volume": 4254453 - }, - "id": 2593455, - "non_hive": { - "close": 65, - "high": 1367, - "low": 35, - "open": 1367, - "volume": 1489421 - }, - "open": "2020-04-28T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16333, - "high": 16333, - "low": 14578, - "open": 3961, - "volume": 3639577 - }, - "id": 2593518, - "non_hive": { - "close": 6852, - "high": 6852, - "low": 5373, - "open": 1460, - "volume": 1393817 - }, - "open": "2020-04-28T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17, - "high": 17, - "low": 8601, - "open": 2212, - "volume": 3094061 - }, - "id": 2593594, - "non_hive": { - "close": 8, - "high": 8, - "low": 3608, - "open": 928, - "volume": 1401744 - }, - "open": "2020-04-28T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7224, - "high": 3393, - "low": 889, - "open": 167, - "volume": 60103 - }, - "id": 2593655, - "non_hive": { - "close": 3540, - "high": 1663, - "low": 399, - "open": 78, - "volume": 28639 - }, - "open": "2020-04-28T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3869, - "high": 2, - "low": 14, - "open": 2, - "volume": 1510011 - }, - "id": 2593682, - "non_hive": { - "close": 1817, - "high": 1, - "low": 5, - "open": 1, - "volume": 643177 - }, - "open": "2020-04-28T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42026, - "high": 38, - "low": 401, - "open": 4255, - "volume": 561659 - }, - "id": 2593713, - "non_hive": { - "close": 19752, - "high": 18, - "low": 147, - "open": 2000, - "volume": 263925 - }, - "open": "2020-04-28T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13185, - "high": 327, - "low": 32, - "open": 50043, - "volume": 385493 - }, - "id": 2593755, - "non_hive": { - "close": 6189, - "high": 154, - "low": 15, - "open": 23520, - "volume": 181167 - }, - "open": "2020-04-29T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4494, - "high": 157, - "low": 41, - "open": 3000, - "volume": 58570 - }, - "id": 2593777, - "non_hive": { - "close": 2110, - "high": 77, - "low": 19, - "open": 1410, - "volume": 27975 - }, - "open": "2020-04-29T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42684, - "high": 1341, - "low": 42684, - "open": 2000, - "volume": 77521 - }, - "id": 2593805, - "non_hive": { - "close": 17942, - "high": 630, - "low": 17942, - "open": 938, - "volume": 33777 - }, - "open": "2020-04-29T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21672, - "high": 2448, - "low": 135427, - "open": 192467, - "volume": 437991 - }, - "id": 2593829, - "non_hive": { - "close": 10170, - "high": 1149, - "low": 56879, - "open": 80903, - "volume": 187745 - }, - "open": "2020-04-29T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8186, - "high": 19, - "low": 815, - "open": 815, - "volume": 1298201 - }, - "id": 2593848, - "non_hive": { - "close": 3840, - "high": 9, - "low": 346, - "open": 346, - "volume": 554847 - }, - "open": "2020-04-29T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2071, - "high": 2, - "low": 12, - "open": 4589, - "volume": 154541 - }, - "id": 2593879, - "non_hive": { - "close": 970, - "high": 1, - "low": 5, - "open": 2153, - "volume": 67068 - }, - "open": "2020-04-29T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36450, - "high": 960, - "low": 29, - "open": 23968, - "volume": 139456 - }, - "id": 2593905, - "non_hive": { - "close": 17069, - "high": 450, - "low": 12, - "open": 11224, - "volume": 65305 - }, - "open": "2020-04-29T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 167, - "high": 339, - "low": 94, - "open": 123988, - "volume": 215315 - }, - "id": 2593930, - "non_hive": { - "close": 78, - "high": 159, - "low": 40, - "open": 52968, - "volume": 93359 - }, - "open": "2020-04-29T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 2, - "low": 12, - "open": 1021, - "volume": 260488 - }, - "id": 2593961, - "non_hive": { - "close": 5, - "high": 1, - "low": 5, - "open": 478, - "volume": 113983 - }, - "open": "2020-04-29T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9220, - "high": 15480, - "low": 288, - "open": 5247, - "volume": 159184 - }, - "id": 2593993, - "non_hive": { - "close": 4101, - "high": 7149, - "low": 121, - "open": 2233, - "volume": 71651 - }, - "open": "2020-04-29T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 128802, - "high": 5004, - "low": 123, - "open": 3940, - "volume": 2513452 - }, - "id": 2594014, - "non_hive": { - "close": 59633, - "high": 2317, - "low": 53, - "open": 1753, - "volume": 1118665 - }, - "open": "2020-04-29T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 372, - "high": 300, - "low": 27, - "open": 179638, - "volume": 1821406 - }, - "id": 2594066, - "non_hive": { - "close": 185, - "high": 150, - "low": 11, - "open": 83169, - "volume": 897797 - }, - "open": "2020-04-29T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 471, - "high": 364, - "low": 222, - "open": 18231, - "volume": 477052 - }, - "id": 2594106, - "non_hive": { - "close": 244, - "high": 189, - "low": 110, - "open": 9114, - "volume": 239135 - }, - "open": "2020-04-29T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1947, - "high": 1947, - "low": 98, - "open": 6438, - "volume": 48635 - }, - "id": 2594151, - "non_hive": { - "close": 1009, - "high": 1009, - "low": 49, - "open": 3335, - "volume": 24870 - }, - "open": "2020-04-29T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16316, - "high": 297093, - "low": 32, - "open": 447, - "volume": 380232 - }, - "id": 2594180, - "non_hive": { - "close": 8462, - "high": 154459, - "low": 15, - "open": 232, - "volume": 197537 - }, - "open": "2020-04-29T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 384207, - "high": 300, - "low": 384207, - "open": 300, - "volume": 818615 - }, - "id": 2594208, - "non_hive": { - "close": 180450, - "high": 156, - "low": 180450, - "open": 156, - "volume": 395379 - }, - "open": "2020-04-29T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 48, - "low": 10, - "open": 212568, - "volume": 2562584 - }, - "id": 2594238, - "non_hive": { - "close": 4, - "high": 25, - "low": 4, - "open": 100000, - "volume": 1190878 - }, - "open": "2020-04-29T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7289, - "high": 19256, - "low": 10, - "open": 10, - "volume": 1639118 - }, - "id": 2594267, - "non_hive": { - "close": 3497, - "high": 9899, - "low": 4, - "open": 4, - "volume": 719612 - }, - "open": "2020-04-29T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3244, - "high": 783, - "low": 3244, - "open": 783, - "volume": 83551 - }, - "id": 2594305, - "non_hive": { - "close": 1378, - "high": 376, - "low": 1378, - "open": 376, - "volume": 39193 - }, - "open": "2020-04-29T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12038, - "high": 220, - "low": 10000, - "open": 220, - "volume": 170076 - }, - "id": 2594337, - "non_hive": { - "close": 5630, - "high": 103, - "low": 4263, - "open": 103, - "volume": 77839 - }, - "open": "2020-04-29T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 97, - "high": 1511, - "low": 197262, - "open": 1900, - "volume": 607375 - }, - "id": 2594366, - "non_hive": { - "close": 45, - "high": 707, - "low": 84387, - "open": 889, - "volume": 261343 - }, - "open": "2020-04-29T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 83288, - "high": 1000, - "low": 36, - "open": 9313, - "volume": 541453 - }, - "id": 2594388, - "non_hive": { - "close": 38795, - "high": 467, - "low": 16, - "open": 4301, - "volume": 252231 - }, - "open": "2020-04-29T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 95358, - "high": 95358, - "low": 12, - "open": 118448, - "volume": 1568504 - }, - "id": 2594416, - "non_hive": { - "close": 49014, - "high": 49014, - "low": 5, - "open": 55315, - "volume": 731826 - }, - "open": "2020-04-29T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3684, - "high": 5097, - "low": 5, - "open": 13126, - "volume": 791806 - }, - "id": 2594465, - "non_hive": { - "close": 1831, - "high": 2620, - "low": 2, - "open": 6747, - "volume": 365099 - }, - "open": "2020-04-29T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7698, - "high": 5630, - "low": 77, - "open": 13728, - "volume": 2168738 - }, - "id": 2594490, - "non_hive": { - "close": 3957, - "high": 2894, - "low": 34, - "open": 6828, - "volume": 1012929 - }, - "open": "2020-04-30T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 377944, - "high": 5743, - "low": 9521, - "open": 221744, - "volume": 2402726 - }, - "id": 2594519, - "non_hive": { - "close": 170081, - "high": 2950, - "low": 4284, - "open": 100000, - "volume": 1119568 - }, - "open": "2020-04-30T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 187, - "high": 889, - "low": 573866, - "open": 889, - "volume": 729031 - }, - "id": 2594544, - "non_hive": { - "close": 92, - "high": 455, - "low": 255784, - "open": 455, - "volume": 331341 - }, - "open": "2020-04-30T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1120, - "high": 2, - "low": 15541, - "open": 9722, - "volume": 815391 - }, - "id": 2594574, - "non_hive": { - "close": 533, - "high": 1, - "low": 7009, - "open": 4759, - "volume": 384642 - }, - "open": "2020-04-30T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6143, - "high": 18767, - "low": 65663, - "open": 65663, - "volume": 2051305 - }, - "id": 2594612, - "non_hive": { - "close": 3157, - "high": 9645, - "low": 30204, - "open": 30204, - "volume": 965534 - }, - "open": "2020-04-30T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 203506, - "high": 651, - "low": 11, - "open": 2936, - "volume": 1522714 - }, - "id": 2594650, - "non_hive": { - "close": 104000, - "high": 335, - "low": 5, - "open": 1509, - "volume": 739054 - }, - "open": "2020-04-30T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7004, - "high": 9311, - "low": 28, - "open": 9311, - "volume": 1622506 - }, - "id": 2594681, - "non_hive": { - "close": 3502, - "high": 4758, - "low": 13, - "open": 4758, - "volume": 776676 - }, - "open": "2020-04-30T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21836, - "high": 3136, - "low": 94, - "open": 3136, - "volume": 669612 - }, - "id": 2594723, - "non_hive": { - "close": 10896, - "high": 1568, - "low": 44, - "open": 1568, - "volume": 317395 - }, - "open": "2020-04-30T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 186, - "low": 12, - "open": 2653, - "volume": 812742 - }, - "id": 2594753, - "non_hive": { - "close": 5, - "high": 93, - "low": 5, - "open": 1324, - "volume": 378964 - }, - "open": "2020-04-30T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40, - "high": 925, - "low": 103158, - "open": 10867, - "volume": 1912196 - }, - "id": 2594787, - "non_hive": { - "close": 19, - "high": 452, - "low": 46420, - "open": 4893, - "volume": 863855 - }, - "open": "2020-04-30T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21740, - "high": 69, - "low": 3, - "open": 142510, - "volume": 293027 - }, - "id": 2594820, - "non_hive": { - "close": 10235, - "high": 33, - "low": 1, - "open": 67406, - "volume": 138425 - }, - "open": "2020-04-30T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24484, - "high": 6170, - "low": 12, - "open": 10000, - "volume": 177381 - }, - "id": 2594854, - "non_hive": { - "close": 11458, - "high": 2911, - "low": 5, - "open": 4717, - "volume": 83288 - }, - "open": "2020-04-30T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7561, - "high": 8077, - "low": 22201, - "open": 8077, - "volume": 192769 - }, - "id": 2594882, - "non_hive": { - "close": 3531, - "high": 3776, - "low": 10364, - "open": 3776, - "volume": 90025 - }, - "open": "2020-04-30T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 123741, - "high": 10373, - "low": 181779, - "open": 5259, - "volume": 747574 - }, - "id": 2594906, - "non_hive": { - "close": 57539, - "high": 4830, - "low": 79982, - "open": 2332, - "volume": 332684 - }, - "open": "2020-04-30T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5555, - "high": 55936, - "low": 152, - "open": 1642, - "volume": 1007164 - }, - "id": 2594920, - "non_hive": { - "close": 2589, - "high": 26091, - "low": 67, - "open": 765, - "volume": 469387 - }, - "open": "2020-04-30T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 160, - "high": 567, - "low": 39, - "open": 567, - "volume": 183413 - }, - "id": 2594955, - "non_hive": { - "close": 74, - "high": 264, - "low": 17, - "open": 264, - "volume": 85256 - }, - "open": "2020-04-30T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 274, - "high": 274, - "low": 32, - "open": 69001, - "volume": 3394756 - }, - "id": 2595005, - "non_hive": { - "close": 140, - "high": 140, - "low": 14, - "open": 32077, - "volume": 1582061 - }, - "open": "2020-04-30T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16000, - "high": 203, - "low": 11, - "open": 201832, - "volume": 6838219 - }, - "id": 2595077, - "non_hive": { - "close": 8173, - "high": 104, - "low": 5, - "open": 102874, - "volume": 3367380 - }, - "open": "2020-04-30T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1260, - "high": 2440, - "low": 11464, - "open": 635, - "volume": 3249125 - }, - "id": 2595147, - "non_hive": { - "close": 579, - "high": 1246, - "low": 5159, - "open": 305, - "volume": 1522886 - }, - "open": "2020-04-30T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1078, - "high": 1328, - "low": 124615, - "open": 10000, - "volume": 1240655 - }, - "id": 2595211, - "non_hive": { - "close": 524, - "high": 651, - "low": 54834, - "open": 4601, - "volume": 568099 - }, - "open": "2020-04-30T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4781, - "high": 52, - "low": 6944, - "open": 18700, - "volume": 779790 - }, - "id": 2595234, - "non_hive": { - "close": 2217, - "high": 25, - "low": 3021, - "open": 8976, - "volume": 347836 - }, - "open": "2020-04-30T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 78259, - "high": 759, - "low": 25962, - "open": 43390, - "volume": 263791 - }, - "id": 2595275, - "non_hive": { - "close": 36472, - "high": 354, - "low": 12067, - "open": 20211, - "volume": 122889 - }, - "open": "2020-04-30T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4053, - "high": 448, - "low": 14, - "open": 43118, - "volume": 172024 - }, - "id": 2595305, - "non_hive": { - "close": 1887, - "high": 209, - "low": 6, - "open": 20065, - "volume": 79916 - }, - "open": "2020-04-30T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8842, - "high": 47, - "low": 53313, - "open": 258, - "volume": 733451 - }, - "id": 2595332, - "non_hive": { - "close": 4120, - "high": 22, - "low": 22531, - "open": 120, - "volume": 322348 - }, - "open": "2020-04-30T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2264, - "high": 329, - "low": 2264, - "open": 854, - "volume": 3447 - }, - "id": 2595362, - "non_hive": { - "close": 1052, - "high": 153, - "low": 1052, - "open": 397, - "volume": 1602 - }, - "open": "2020-05-01T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3302, - "high": 8799, - "low": 43, - "open": 225416, - "volume": 1734995 - }, - "id": 2595373, - "non_hive": { - "close": 1615, - "high": 4312, - "low": 18, - "open": 104688, - "volume": 756977 - }, - "open": "2020-05-01T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5501, - "high": 5501, - "low": 13171, - "open": 107, - "volume": 717599 - }, - "id": 2595393, - "non_hive": { - "close": 2744, - "high": 2744, - "low": 5329, - "open": 46, - "volume": 299702 - }, - "open": "2020-05-01T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1001, - "high": 6802, - "low": 1001, - "open": 6802, - "volume": 15642 - }, - "id": 2595418, - "non_hive": { - "close": 492, - "high": 3382, - "low": 492, - "open": 3382, - "volume": 7768 - }, - "open": "2020-05-01T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8385, - "high": 53, - "low": 8385, - "open": 14561, - "volume": 60520 - }, - "id": 2595431, - "non_hive": { - "close": 4065, - "high": 26, - "low": 4065, - "open": 7136, - "volume": 29553 - }, - "open": "2020-05-01T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 1026, - "low": 10, - "open": 1026, - "volume": 164342 - }, - "id": 2595449, - "non_hive": { - "close": 4, - "high": 482, - "low": 4, - "open": 482, - "volume": 74653 - }, - "open": "2020-05-01T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 87799, - "high": 2, - "low": 7316, - "open": 52798, - "volume": 253115 - }, - "id": 2595463, - "non_hive": { - "close": 36000, - "high": 1, - "low": 2999, - "open": 21648, - "volume": 106479 - }, - "open": "2020-05-01T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 814, - "high": 2, - "low": 91, - "open": 2186, - "volume": 218341 - }, - "id": 2595492, - "non_hive": { - "close": 358, - "high": 1, - "low": 37, - "open": 991, - "volume": 91991 - }, - "open": "2020-05-01T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10327, - "high": 495, - "low": 4235, - "open": 17283, - "volume": 571504 - }, - "id": 2595522, - "non_hive": { - "close": 4439, - "high": 218, - "low": 1744, - "open": 7596, - "volume": 245463 - }, - "open": "2020-05-01T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2879, - "high": 2879, - "low": 106, - "open": 763, - "volume": 112769 - }, - "id": 2595563, - "non_hive": { - "close": 1267, - "high": 1267, - "low": 45, - "open": 328, - "volume": 48972 - }, - "open": "2020-05-01T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1213, - "high": 14071, - "low": 118, - "open": 9361, - "volume": 166218 - }, - "id": 2595585, - "non_hive": { - "close": 534, - "high": 6370, - "low": 51, - "open": 4117, - "volume": 73466 - }, - "open": "2020-05-01T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 229, - "high": 128, - "low": 12, - "open": 28, - "volume": 336466 - }, - "id": 2595607, - "non_hive": { - "close": 102, - "high": 58, - "low": 5, - "open": 12, - "volume": 151419 - }, - "open": "2020-05-01T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 322, - "high": 92, - "low": 12716, - "open": 4945, - "volume": 83801 - }, - "id": 2595628, - "non_hive": { - "close": 146, - "high": 42, - "low": 5755, - "open": 2239, - "volume": 37934 - }, - "open": "2020-05-01T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13740, - "high": 13740, - "low": 123040, - "open": 42522, - "volume": 407058 - }, - "id": 2595654, - "non_hive": { - "close": 6242, - "high": 6242, - "low": 53555, - "open": 19245, - "volume": 182024 - }, - "open": "2020-05-01T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 71, - "high": 7484, - "low": 12650, - "open": 3500, - "volume": 342699 - }, - "id": 2595678, - "non_hive": { - "close": 32, - "high": 3400, - "low": 5628, - "open": 1590, - "volume": 152923 - }, - "open": "2020-05-01T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2464, - "high": 145, - "low": 90131, - "open": 2440, - "volume": 695701 - }, - "id": 2595706, - "non_hive": { - "close": 1099, - "high": 65, - "low": 36052, - "open": 1088, - "volume": 296621 - }, - "open": "2020-05-01T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15815, - "high": 170, - "low": 20342, - "open": 249588, - "volume": 1396969 - }, - "id": 2595733, - "non_hive": { - "close": 6643, - "high": 76, - "low": 8136, - "open": 100000, - "volume": 566899 - }, - "open": "2020-05-01T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27601, - "high": 11, - "low": 27601, - "open": 61411, - "volume": 395228 - }, - "id": 2595779, - "non_hive": { - "close": 11316, - "high": 5, - "low": 11316, - "open": 27163, - "volume": 167729 - }, - "open": "2020-05-01T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3247, - "high": 28498, - "low": 8, - "open": 28498, - "volume": 116991 - }, - "id": 2595811, - "non_hive": { - "close": 1332, - "high": 12596, - "low": 3, - "open": 12596, - "volume": 50609 - }, - "open": "2020-05-01T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12522, - "high": 12522, - "low": 305, - "open": 479, - "volume": 136037 - }, - "id": 2595851, - "non_hive": { - "close": 5447, - "high": 5447, - "low": 125, - "open": 203, - "volume": 57733 - }, - "open": "2020-05-01T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 211, - "low": 598545, - "open": 21090, - "volume": 1313131 - }, - "id": 2595873, - "non_hive": { - "close": 4308, - "high": 92, - "low": 227626, - "open": 9172, - "volume": 523244 - }, - "open": "2020-05-01T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27001, - "high": 58, - "low": 4, - "open": 10000, - "volume": 1371675 - }, - "id": 2595905, - "non_hive": { - "close": 11588, - "high": 25, - "low": 1, - "open": 4308, - "volume": 529697 - }, - "open": "2020-05-01T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 540, - "high": 2, - "low": 10526, - "open": 77304, - "volume": 818477 - }, - "id": 2595945, - "non_hive": { - "close": 209, - "high": 1, - "low": 4000, - "open": 30164, - "volume": 322800 - }, - "open": "2020-05-01T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32305, - "high": 3157, - "low": 32305, - "open": 3157, - "volume": 482699 - }, - "id": 2595980, - "non_hive": { - "close": 12637, - "high": 1359, - "low": 12637, - "open": 1359, - "volume": 191304 - }, - "open": "2020-05-01T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1633, - "high": 1101, - "low": 34779, - "open": 17025, - "volume": 54538 - }, - "id": 2596013, - "non_hive": { - "close": 703, - "high": 474, - "low": 14965, - "open": 7326, - "volume": 23468 - }, - "open": "2020-05-02T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5521, - "high": 104, - "low": 41, - "open": 28317, - "volume": 98503 - }, - "id": 2596027, - "non_hive": { - "close": 2369, - "high": 45, - "low": 16, - "open": 12185, - "volume": 42138 - }, - "open": "2020-05-02T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3308, - "high": 4801, - "low": 3, - "open": 3, - "volume": 29873 - }, - "id": 2596053, - "non_hive": { - "close": 1416, - "high": 2059, - "low": 1, - "open": 1, - "volume": 12783 - }, - "open": "2020-05-02T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 302, - "high": 19090, - "low": 2809, - "open": 19090, - "volume": 39206 - }, - "id": 2596066, - "non_hive": { - "close": 128, - "high": 8168, - "low": 1099, - "open": 8168, - "volume": 16537 - }, - "open": "2020-05-02T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5711, - "high": 2156, - "low": 109, - "open": 109, - "volume": 26631 - }, - "id": 2596081, - "non_hive": { - "close": 2383, - "high": 912, - "low": 42, - "open": 42, - "volume": 11120 - }, - "open": "2020-05-02T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 207, - "high": 1168, - "low": 11, - "open": 1168, - "volume": 57053 - }, - "id": 2596091, - "non_hive": { - "close": 83, - "high": 487, - "low": 4, - "open": 487, - "volume": 22619 - }, - "open": "2020-05-02T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45, - "high": 9992, - "low": 28, - "open": 10750, - "volume": 156940 - }, - "id": 2596113, - "non_hive": { - "close": 18, - "high": 3997, - "low": 11, - "open": 4300, - "volume": 62775 - }, - "open": "2020-05-02T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20488, - "high": 10295, - "low": 93, - "open": 4345, - "volume": 173671 - }, - "id": 2596138, - "non_hive": { - "close": 8296, - "high": 4221, - "low": 36, - "open": 1738, - "volume": 69982 - }, - "open": "2020-05-02T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 169341, - "low": 13, - "open": 10000, - "volume": 819219 - }, - "id": 2596169, - "non_hive": { - "close": 5, - "high": 69723, - "low": 5, - "open": 4117, - "volume": 323522 - }, - "open": "2020-05-02T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1534, - "high": 33011, - "low": 12256, - "open": 33011, - "volume": 770294 - }, - "id": 2596193, - "non_hive": { - "close": 617, - "high": 13525, - "low": 4695, - "open": 13525, - "volume": 300830 - }, - "open": "2020-05-02T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3624, - "high": 27, - "low": 381, - "open": 10000, - "volume": 69944 - }, - "id": 2596231, - "non_hive": { - "close": 1458, - "high": 11, - "low": 148, - "open": 4021, - "volume": 28077 - }, - "open": "2020-05-02T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 61414, - "high": 355, - "low": 11, - "open": 45409, - "volume": 324734 - }, - "id": 2596259, - "non_hive": { - "close": 24710, - "high": 143, - "low": 4, - "open": 18268, - "volume": 130398 - }, - "open": "2020-05-02T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 306, - "high": 504, - "low": 163, - "open": 23897, - "volume": 1057746 - }, - "id": 2596293, - "non_hive": { - "close": 119, - "high": 203, - "low": 63, - "open": 9558, - "volume": 418932 - }, - "open": "2020-05-02T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 497, - "high": 29, - "low": 214844, - "open": 13042, - "volume": 3160408 - }, - "id": 2596328, - "non_hive": { - "close": 199, - "high": 12, - "low": 79710, - "open": 5215, - "volume": 1198017 - }, - "open": "2020-05-02T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 96184, - "high": 322, - "low": 130276, - "open": 882, - "volume": 709363 - }, - "id": 2596374, - "non_hive": { - "close": 38451, - "high": 129, - "low": 48244, - "open": 331, - "volume": 278824 - }, - "open": "2020-05-02T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1154, - "high": 6000, - "low": 17816, - "open": 6000, - "volume": 2372990 - }, - "id": 2596403, - "non_hive": { - "close": 460, - "high": 2398, - "low": 6591, - "open": 2398, - "volume": 892808 - }, - "open": "2020-05-02T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 106170, - "high": 112, - "low": 106170, - "open": 7256, - "volume": 791835 - }, - "id": 2596435, - "non_hive": { - "close": 38224, - "high": 45, - "low": 38224, - "open": 2891, - "volume": 293194 - }, - "open": "2020-05-02T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56347, - "high": 27, - "low": 10, - "open": 12953, - "volume": 3552459 - }, - "id": 2596472, - "non_hive": { - "close": 22481, - "high": 11, - "low": 3, - "open": 4666, - "volume": 1281737 - }, - "open": "2020-05-02T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3241, - "high": 8783, - "low": 572686, - "open": 8783, - "volume": 3388189 - }, - "id": 2596538, - "non_hive": { - "close": 1198, - "high": 3502, - "low": 200738, - "open": 3502, - "volume": 1196456 - }, - "open": "2020-05-02T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 135933, - "high": 2, - "low": 135933, - "open": 316, - "volume": 2868417 - }, - "id": 2596580, - "non_hive": { - "close": 46229, - "high": 1, - "low": 46229, - "open": 117, - "volume": 1007444 - }, - "open": "2020-05-02T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 10000, - "low": 3992, - "open": 6000, - "volume": 135206 - }, - "id": 2596629, - "non_hive": { - "close": 3897, - "high": 3897, - "low": 1294, - "open": 2036, - "volume": 48107 - }, - "open": "2020-05-02T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 715, - "high": 1901, - "low": 14528, - "open": 4190, - "volume": 21334 - }, - "id": 2596657, - "non_hive": { - "close": 269, - "high": 741, - "low": 4983, - "open": 1633, - "volume": 7626 - }, - "open": "2020-05-02T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59, - "high": 59, - "low": 3, - "open": 3298, - "volume": 74783 - }, - "id": 2596670, - "non_hive": { - "close": 23, - "high": 23, - "low": 1, - "open": 1237, - "volume": 28171 - }, - "open": "2020-05-02T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26328, - "high": 20, - "low": 15517, - "open": 9940, - "volume": 264056 - }, - "id": 2596706, - "non_hive": { - "close": 9419, - "high": 8, - "low": 5431, - "open": 3864, - "volume": 95787 - }, - "open": "2020-05-02T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2144, - "high": 2, - "low": 28195, - "open": 18020, - "volume": 167836 - }, - "id": 2596750, - "non_hive": { - "close": 836, - "high": 1, - "low": 9642, - "open": 6447, - "volume": 61256 - }, - "open": "2020-05-03T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 68589, - "high": 2, - "low": 68589, - "open": 502, - "volume": 104900 - }, - "id": 2596774, - "non_hive": { - "close": 23458, - "high": 1, - "low": 23458, - "open": 195, - "volume": 37870 - }, - "open": "2020-05-03T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45563, - "high": 1270, - "low": 3, - "open": 10231, - "volume": 211984 - }, - "id": 2596795, - "non_hive": { - "close": 17739, - "high": 495, - "low": 1, - "open": 3987, - "volume": 80551 - }, - "open": "2020-05-03T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1338, - "high": 5, - "low": 4434, - "open": 102, - "volume": 13248 - }, - "id": 2596824, - "non_hive": { - "close": 509, - "high": 2, - "low": 1517, - "open": 40, - "volume": 4881 - }, - "open": "2020-05-03T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9286, - "high": 18, - "low": 9286, - "open": 18, - "volume": 47033 - }, - "id": 2596836, - "non_hive": { - "close": 3436, - "high": 7, - "low": 3436, - "open": 7, - "volume": 17787 - }, - "open": "2020-05-03T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22789, - "high": 6281, - "low": 12, - "open": 6281, - "volume": 210178 - }, - "id": 2596850, - "non_hive": { - "close": 8421, - "high": 2324, - "low": 4, - "open": 2324, - "volume": 77678 - }, - "open": "2020-05-03T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22840, - "high": 70, - "low": 291296, - "open": 1434, - "volume": 1340243 - }, - "id": 2596866, - "non_hive": { - "close": 7994, - "high": 26, - "low": 99186, - "open": 530, - "volume": 458786 - }, - "open": "2020-05-03T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2709, - "high": 94, - "low": 92, - "open": 60954, - "volume": 3039635 - }, - "id": 2596905, - "non_hive": { - "close": 975, - "high": 35, - "low": 31, - "open": 20783, - "volume": 1036242 - }, - "open": "2020-05-03T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57494, - "high": 2, - "low": 12, - "open": 7421, - "volume": 3354585 - }, - "id": 2596932, - "non_hive": { - "close": 19689, - "high": 1, - "low": 4, - "open": 2657, - "volume": 1144928 - }, - "open": "2020-05-03T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5629, - "high": 1515, - "low": 10002, - "open": 1515, - "volume": 70584 - }, - "id": 2596996, - "non_hive": { - "close": 1926, - "high": 519, - "low": 3400, - "open": 519, - "volume": 24142 - }, - "open": "2020-05-03T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 484, - "high": 239, - "low": 100, - "open": 12969, - "volume": 1245356 - }, - "id": 2597030, - "non_hive": { - "close": 166, - "high": 82, - "low": 33, - "open": 4440, - "volume": 421233 - }, - "open": "2020-05-03T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25056, - "high": 2058, - "low": 27, - "open": 5613, - "volume": 1958231 - }, - "id": 2597074, - "non_hive": { - "close": 8519, - "high": 718, - "low": 9, - "open": 1921, - "volume": 662556 - }, - "open": "2020-05-03T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 145, - "high": 2, - "low": 2547, - "open": 2547, - "volume": 281382 - }, - "id": 2597110, - "non_hive": { - "close": 49, - "high": 1, - "low": 860, - "open": 860, - "volume": 97283 - }, - "open": "2020-05-03T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 197, - "high": 197, - "low": 186, - "open": 10000, - "volume": 750071 - }, - "id": 2597169, - "non_hive": { - "close": 69, - "high": 69, - "low": 61, - "open": 3487, - "volume": 253909 - }, - "open": "2020-05-03T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3869, - "high": 1483, - "low": 3869, - "open": 832, - "volume": 108088 - }, - "id": 2597223, - "non_hive": { - "close": 1246, - "high": 517, - "low": 1246, - "open": 290, - "volume": 36835 - }, - "open": "2020-05-03T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 130824, - "high": 14138, - "low": 54593, - "open": 755, - "volume": 1382358 - }, - "id": 2597253, - "non_hive": { - "close": 39986, - "high": 4804, - "low": 16686, - "open": 248, - "volume": 443141 - }, - "open": "2020-05-03T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14935, - "high": 116, - "low": 333333, - "open": 20402, - "volume": 1942497 - }, - "id": 2597300, - "non_hive": { - "close": 5363, - "high": 42, - "low": 99999, - "open": 6236, - "volume": 653590 - }, - "open": "2020-05-03T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5778, - "high": 2, - "low": 692, - "open": 3384, - "volume": 71546 - }, - "id": 2597356, - "non_hive": { - "close": 2077, - "high": 1, - "low": 248, - "open": 1216, - "volume": 25718 - }, - "open": "2020-05-03T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9756, - "high": 3097, - "low": 10402, - "open": 10402, - "volume": 146136 - }, - "id": 2597386, - "non_hive": { - "close": 3685, - "high": 1193, - "low": 3740, - "open": 3740, - "volume": 54590 - }, - "open": "2020-05-03T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17199, - "high": 21, - "low": 1477, - "open": 1768, - "volume": 304598 - }, - "id": 2597426, - "non_hive": { - "close": 6535, - "high": 8, - "low": 540, - "open": 668, - "volume": 115555 - }, - "open": "2020-05-03T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5729, - "high": 2, - "low": 88, - "open": 2468, - "volume": 1138449 - }, - "id": 2597459, - "non_hive": { - "close": 2177, - "high": 1, - "low": 32, - "open": 938, - "volume": 426779 - }, - "open": "2020-05-03T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20877, - "high": 27248, - "low": 600000, - "open": 5265, - "volume": 2878971 - }, - "id": 2597507, - "non_hive": { - "close": 8332, - "high": 10878, - "low": 222060, - "open": 2000, - "volume": 1086741 - }, - "open": "2020-05-03T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 500, - "high": 207, - "low": 13, - "open": 64770, - "volume": 834459 - }, - "id": 2597568, - "non_hive": { - "close": 207, - "high": 86, - "low": 5, - "open": 25848, - "volume": 333349 - }, - "open": "2020-05-03T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1061, - "high": 2, - "low": 403, - "open": 500, - "volume": 428869 - }, - "id": 2597608, - "non_hive": { - "close": 476, - "high": 1, - "low": 161, - "open": 207, - "volume": 189325 - }, - "open": "2020-05-03T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40520, - "high": 504, - "low": 205563, - "open": 21204, - "volume": 747661 - }, - "id": 2597658, - "non_hive": { - "close": 18234, - "high": 227, - "low": 82603, - "open": 9521, - "volume": 307324 - }, - "open": "2020-05-04T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6767, - "high": 3365, - "low": 6767, - "open": 93, - "volume": 32210 - }, - "id": 2597686, - "non_hive": { - "close": 2717, - "high": 1614, - "low": 2717, - "open": 42, - "volume": 13918 - }, - "open": "2020-05-04T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 918, - "high": 5588, - "low": 3, - "open": 3, - "volume": 3045607 - }, - "id": 2597703, - "non_hive": { - "close": 436, - "high": 2665, - "low": 1, - "open": 1, - "volume": 1226617 - }, - "open": "2020-05-04T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2285, - "high": 7573, - "low": 587370, - "open": 12788, - "volume": 1913914 - }, - "id": 2597739, - "non_hive": { - "close": 1028, - "high": 3475, - "low": 229133, - "open": 5371, - "volume": 758555 - }, - "open": "2020-05-04T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38730, - "high": 29211, - "low": 38730, - "open": 270, - "volume": 3790429 - }, - "id": 2597772, - "non_hive": { - "close": 15108, - "high": 13120, - "low": 15108, - "open": 108, - "volume": 1506669 - }, - "open": "2020-05-04T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9841, - "high": 232, - "low": 11, - "open": 273, - "volume": 1291672 - }, - "id": 2597813, - "non_hive": { - "close": 4232, - "high": 100, - "low": 4, - "open": 106, - "volume": 488997 - }, - "open": "2020-05-04T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5251, - "high": 9, - "low": 1611, - "open": 9, - "volume": 18304 - }, - "id": 2597842, - "non_hive": { - "close": 2254, - "high": 4, - "low": 579, - "open": 4, - "volume": 7655 - }, - "open": "2020-05-04T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3561, - "high": 9575, - "low": 92, - "open": 9999, - "volume": 667453 - }, - "id": 2597860, - "non_hive": { - "close": 1421, - "high": 3830, - "low": 34, - "open": 3999, - "volume": 248947 - }, - "open": "2020-05-04T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5052, - "high": 55, - "low": 11, - "open": 3302, - "volume": 75431 - }, - "id": 2597898, - "non_hive": { - "close": 1875, - "high": 22, - "low": 4, - "open": 1318, - "volume": 29705 - }, - "open": "2020-05-04T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15750, - "high": 55, - "low": 15750, - "open": 10000, - "volume": 260390 - }, - "id": 2597923, - "non_hive": { - "close": 5985, - "high": 22, - "low": 5985, - "open": 3990, - "volume": 102587 - }, - "open": "2020-05-04T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2, - "high": 2, - "low": 543, - "open": 543, - "volume": 123840 - }, - "id": 2597953, - "non_hive": { - "close": 1, - "high": 1, - "low": 216, - "open": 216, - "volume": 49463 - }, - "open": "2020-05-04T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1639, - "high": 14327, - "low": 11, - "open": 500, - "volume": 93983 - }, - "id": 2597987, - "non_hive": { - "close": 654, - "high": 5723, - "low": 4, - "open": 191, - "volume": 37355 - }, - "open": "2020-05-04T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13706, - "high": 2, - "low": 33045, - "open": 3282, - "volume": 1646579 - }, - "id": 2598028, - "non_hive": { - "close": 5606, - "high": 1, - "low": 12891, - "open": 1309, - "volume": 657303 - }, - "open": "2020-05-04T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1952, - "high": 2701, - "low": 2000, - "open": 1757, - "volume": 356720 - }, - "id": 2598087, - "non_hive": { - "close": 818, - "high": 1132, - "low": 817, - "open": 719, - "volume": 148162 - }, - "open": "2020-05-04T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29811, - "high": 238, - "low": 1036, - "open": 100, - "volume": 454037 - }, - "id": 2598118, - "non_hive": { - "close": 12442, - "high": 100, - "low": 404, - "open": 42, - "volume": 187758 - }, - "open": "2020-05-04T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2946, - "high": 536, - "low": 7009, - "open": 9980, - "volume": 180225 - }, - "id": 2598193, - "non_hive": { - "close": 1263, - "high": 230, - "low": 2811, - "open": 4165, - "volume": 76920 - }, - "open": "2020-05-04T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 760, - "high": 2, - "low": 13312, - "open": 2, - "volume": 804121 - }, - "id": 2598232, - "non_hive": { - "close": 361, - "high": 1, - "low": 5590, - "open": 1, - "volume": 363848 - }, - "open": "2020-05-04T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23405, - "high": 60, - "low": 11, - "open": 21000, - "volume": 391489 - }, - "id": 2598293, - "non_hive": { - "close": 11211, - "high": 29, - "low": 5, - "open": 9974, - "volume": 185484 - }, - "open": "2020-05-04T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 217, - "high": 66, - "low": 15, - "open": 10000, - "volume": 622099 - }, - "id": 2598351, - "non_hive": { - "close": 104, - "high": 32, - "low": 6, - "open": 4789, - "volume": 269385 - }, - "open": "2020-05-04T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1466, - "high": 54, - "low": 5, - "open": 3243, - "volume": 185020 - }, - "id": 2598385, - "non_hive": { - "close": 701, - "high": 26, - "low": 2, - "open": 1460, - "volume": 85929 - }, - "open": "2020-05-04T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5412, - "high": 1439, - "low": 2000, - "open": 1226, - "volume": 185299 - }, - "id": 2598423, - "non_hive": { - "close": 2295, - "high": 688, - "low": 848, - "open": 586, - "volume": 79949 - }, - "open": "2020-05-04T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2400, - "high": 2, - "low": 12792, - "open": 523, - "volume": 222165 - }, - "id": 2598456, - "non_hive": { - "close": 1110, - "high": 1, - "low": 5423, - "open": 222, - "volume": 102078 - }, - "open": "2020-05-04T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29318, - "high": 8649, - "low": 12, - "open": 1406, - "volume": 126574 - }, - "id": 2598494, - "non_hive": { - "close": 13568, - "high": 4003, - "low": 5, - "open": 647, - "volume": 58101 - }, - "open": "2020-05-04T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 4711, - "low": 12, - "open": 2892, - "volume": 619988 - }, - "id": 2598522, - "non_hive": { - "close": 5, - "high": 2252, - "low": 5, - "open": 1339, - "volume": 262680 - }, - "open": "2020-05-04T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 775, - "high": 2223, - "low": 13273, - "open": 15586, - "volume": 60495 - }, - "id": 2598542, - "non_hive": { - "close": 366, - "high": 1051, - "low": 6268, - "open": 7366, - "volume": 28583 - }, - "open": "2020-05-05T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10001, - "high": 582, - "low": 10001, - "open": 11497, - "volume": 23302 - }, - "id": 2598567, - "non_hive": { - "close": 4718, - "high": 275, - "low": 4718, - "open": 5424, - "volume": 10994 - }, - "open": "2020-05-05T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44351, - "high": 508, - "low": 44351, - "open": 508, - "volume": 54179 - }, - "id": 2598579, - "non_hive": { - "close": 18725, - "high": 240, - "low": 18725, - "open": 240, - "volume": 23365 - }, - "open": "2020-05-05T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3961, - "high": 3961, - "low": 2566, - "open": 2566, - "volume": 2150302 - }, - "id": 2598590, - "non_hive": { - "close": 1872, - "high": 1872, - "low": 1206, - "open": 1206, - "volume": 1014690 - }, - "open": "2020-05-05T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42976, - "high": 279, - "low": 42976, - "open": 4570, - "volume": 383903 - }, - "id": 2598603, - "non_hive": { - "close": 17981, - "high": 132, - "low": 17981, - "open": 2160, - "volume": 165381 - }, - "open": "2020-05-05T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 469, - "high": 469, - "low": 12, - "open": 2985, - "volume": 292003 - }, - "id": 2598627, - "non_hive": { - "close": 221, - "high": 221, - "low": 5, - "open": 1246, - "volume": 122032 - }, - "open": "2020-05-05T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29, - "high": 76, - "low": 29, - "open": 76, - "volume": 5556 - }, - "id": 2598651, - "non_hive": { - "close": 13, - "high": 36, - "low": 13, - "open": 36, - "volume": 2596 - }, - "open": "2020-05-05T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1829, - "high": 352, - "low": 23643, - "open": 352, - "volume": 33981 - }, - "id": 2598668, - "non_hive": { - "close": 841, - "high": 165, - "low": 10402, - "open": 165, - "volume": 15098 - }, - "open": "2020-05-05T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 60, - "low": 5, - "open": 3134, - "volume": 133634 - }, - "id": 2598684, - "non_hive": { - "close": 6, - "high": 28, - "low": 2, - "open": 1440, - "volume": 61145 - }, - "open": "2020-05-05T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 560, - "high": 269, - "low": 105, - "open": 4122, - "volume": 74962 - }, - "id": 2598727, - "non_hive": { - "close": 255, - "high": 124, - "low": 46, - "open": 1895, - "volume": 34428 - }, - "open": "2020-05-05T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8397, - "high": 168, - "low": 212793, - "open": 1513, - "volume": 1246438 - }, - "id": 2598753, - "non_hive": { - "close": 3775, - "high": 77, - "low": 85164, - "open": 689, - "volume": 502242 - }, - "open": "2020-05-05T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4140, - "high": 2, - "low": 5, - "open": 100, - "volume": 93493 - }, - "id": 2598779, - "non_hive": { - "close": 1852, - "high": 1, - "low": 2, - "open": 44, - "volume": 40987 - }, - "open": "2020-05-05T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1692, - "high": 2238, - "low": 85, - "open": 2238, - "volume": 147946 - }, - "id": 2598806, - "non_hive": { - "close": 749, - "high": 1001, - "low": 35, - "open": 1001, - "volume": 63636 - }, - "open": "2020-05-05T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299, - "high": 6, - "low": 4, - "open": 27, - "volume": 131897 - }, - "id": 2598837, - "non_hive": { - "close": 132, - "high": 3, - "low": 1, - "open": 12, - "volume": 57556 - }, - "open": "2020-05-05T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3963, - "high": 61, - "low": 441, - "open": 1152, - "volume": 139840 - }, - "id": 2598883, - "non_hive": { - "close": 1744, - "high": 27, - "low": 194, - "open": 508, - "volume": 61584 - }, - "open": "2020-05-05T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3455, - "high": 79, - "low": 9593, - "open": 20701, - "volume": 410437 - }, - "id": 2598919, - "non_hive": { - "close": 1522, - "high": 35, - "low": 4048, - "open": 9111, - "volume": 179805 - }, - "open": "2020-05-05T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5375, - "high": 265, - "low": 5210, - "open": 136221, - "volume": 663942 - }, - "id": 2598955, - "non_hive": { - "close": 2311, - "high": 117, - "low": 2240, - "open": 60000, - "volume": 290438 - }, - "open": "2020-05-05T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 340, - "high": 352, - "low": 12, - "open": 15000, - "volume": 216457 - }, - "id": 2598996, - "non_hive": { - "close": 150, - "high": 156, - "low": 5, - "open": 6450, - "volume": 93783 - }, - "open": "2020-05-05T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23127, - "high": 124, - "low": 4, - "open": 3622, - "volume": 347563 - }, - "id": 2599040, - "non_hive": { - "close": 10173, - "high": 55, - "low": 1, - "open": 1597, - "volume": 153186 - }, - "open": "2020-05-05T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 839, - "high": 432, - "low": 199, - "open": 3228, - "volume": 53053 - }, - "id": 2599067, - "non_hive": { - "close": 365, - "high": 191, - "low": 83, - "open": 1359, - "volume": 23371 - }, - "open": "2020-05-05T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 178469, - "high": 142, - "low": 8861, - "open": 20695, - "volume": 250704 - }, - "id": 2599094, - "non_hive": { - "close": 73617, - "high": 63, - "low": 3654, - "open": 9000, - "volume": 104668 - }, - "open": "2020-05-05T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 827, - "high": 43, - "low": 5648, - "open": 5513, - "volume": 70696 - }, - "id": 2599121, - "non_hive": { - "close": 365, - "high": 19, - "low": 2484, - "open": 2431, - "volume": 31168 - }, - "open": "2020-05-05T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 71, - "high": 781, - "low": 10, - "open": 300, - "volume": 27693 - }, - "id": 2599160, - "non_hive": { - "close": 29, - "high": 344, - "low": 4, - "open": 132, - "volume": 12083 - }, - "open": "2020-05-05T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14257, - "high": 57, - "low": 197, - "open": 4300, - "volume": 216246 - }, - "id": 2599195, - "non_hive": { - "close": 5984, - "high": 25, - "low": 81, - "open": 1859, - "volume": 92937 - }, - "open": "2020-05-05T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 366, - "high": 71, - "low": 3, - "open": 5404, - "volume": 63719 - }, - "id": 2599221, - "non_hive": { - "close": 153, - "high": 31, - "low": 1, - "open": 2336, - "volume": 27327 - }, - "open": "2020-05-06T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 87, - "high": 87, - "low": 147, - "open": 4850, - "volume": 523901 - }, - "id": 2599245, - "non_hive": { - "close": 38, - "high": 38, - "low": 61, - "open": 2023, - "volume": 226345 - }, - "open": "2020-05-06T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 582, - "high": 582, - "low": 5, - "open": 804, - "volume": 624873 - }, - "id": 2599273, - "non_hive": { - "close": 252, - "high": 252, - "low": 2, - "open": 348, - "volume": 256200 - }, - "open": "2020-05-06T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6231, - "high": 31784, - "low": 5382, - "open": 31784, - "volume": 46088 - }, - "id": 2599293, - "non_hive": { - "close": 2683, - "high": 13690, - "low": 2317, - "open": 13690, - "volume": 19849 - }, - "open": "2020-05-06T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2188, - "high": 3047, - "low": 3480, - "open": 63018, - "volume": 111360 - }, - "id": 2599305, - "non_hive": { - "close": 903, - "high": 1310, - "low": 1436, - "open": 27090, - "volume": 47093 - }, - "open": "2020-05-06T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 249, - "high": 564, - "low": 13, - "open": 1197, - "volume": 656154 - }, - "id": 2599327, - "non_hive": { - "close": 100, - "high": 233, - "low": 5, - "open": 494, - "volume": 264773 - }, - "open": "2020-05-06T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5059, - "high": 16, - "low": 29, - "open": 16, - "volume": 38243 - }, - "id": 2599352, - "non_hive": { - "close": 2087, - "high": 7, - "low": 11, - "open": 7, - "volume": 15755 - }, - "open": "2020-05-06T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2077, - "high": 2077, - "low": 98, - "open": 8179, - "volume": 599939 - }, - "id": 2599377, - "non_hive": { - "close": 867, - "high": 867, - "low": 39, - "open": 3374, - "volume": 244196 - }, - "open": "2020-05-06T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5774, - "high": 611, - "low": 12, - "open": 404, - "volume": 74030 - }, - "id": 2599416, - "non_hive": { - "close": 2410, - "high": 264, - "low": 5, - "open": 169, - "volume": 30907 - }, - "open": "2020-05-06T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 71, - "high": 48, - "low": 400, - "open": 1305, - "volume": 122347 - }, - "id": 2599448, - "non_hive": { - "close": 31, - "high": 21, - "low": 158, - "open": 564, - "volume": 52672 - }, - "open": "2020-05-06T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15836, - "high": 1141, - "low": 26705, - "open": 14926, - "volume": 1060393 - }, - "id": 2599485, - "non_hive": { - "close": 6804, - "high": 492, - "low": 10414, - "open": 6434, - "volume": 424981 - }, - "open": "2020-05-06T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2153, - "high": 20097, - "low": 29, - "open": 13289, - "volume": 117419 - }, - "id": 2599528, - "non_hive": { - "close": 920, - "high": 8613, - "low": 11, - "open": 5695, - "volume": 50217 - }, - "open": "2020-05-06T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 175, - "high": 2, - "low": 44, - "open": 2335, - "volume": 65583 - }, - "id": 2599560, - "non_hive": { - "close": 75, - "high": 1, - "low": 18, - "open": 997, - "volume": 27474 - }, - "open": "2020-05-06T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 533007, - "high": 9145, - "low": 432941, - "open": 12897, - "volume": 1344065 - }, - "id": 2599584, - "non_hive": { - "close": 213418, - "high": 3903, - "low": 173349, - "open": 5494, - "volume": 543941 - }, - "open": "2020-05-06T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 702, - "high": 978, - "low": 3034, - "open": 9057, - "volume": 1847974 - }, - "id": 2599622, - "non_hive": { - "close": 295, - "high": 411, - "low": 1214, - "open": 3804, - "volume": 740485 - }, - "open": "2020-05-06T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6810, - "high": 23, - "low": 529, - "open": 15485, - "volume": 1030983 - }, - "id": 2599660, - "non_hive": { - "close": 2858, - "high": 10, - "low": 211, - "open": 6500, - "volume": 421024 - }, - "open": "2020-05-06T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7133, - "high": 369, - "low": 11269, - "open": 9911, - "volume": 924183 - }, - "id": 2599707, - "non_hive": { - "close": 2993, - "high": 155, - "low": 4450, - "open": 4161, - "volume": 372875 - }, - "open": "2020-05-06T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11542, - "high": 452, - "low": 13, - "open": 49318, - "volume": 68653 - }, - "id": 2599752, - "non_hive": { - "close": 4838, - "high": 190, - "low": 5, - "open": 20693, - "volume": 28802 - }, - "open": "2020-05-06T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5125, - "high": 5458, - "low": 1329, - "open": 5458, - "volume": 193992 - }, - "id": 2599773, - "non_hive": { - "close": 2101, - "high": 2288, - "low": 531, - "open": 2288, - "volume": 80021 - }, - "open": "2020-05-06T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 387, - "high": 38, - "low": 606, - "open": 3437, - "volume": 938368 - }, - "id": 2599805, - "non_hive": { - "close": 161, - "high": 16, - "low": 241, - "open": 1375, - "volume": 378351 - }, - "open": "2020-05-06T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8432, - "high": 831, - "low": 2016, - "open": 3453, - "volume": 81142 - }, - "id": 2599853, - "non_hive": { - "close": 3500, - "high": 345, - "low": 836, - "open": 1433, - "volume": 33668 - }, - "open": "2020-05-06T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13065, - "high": 38, - "low": 16409, - "open": 38, - "volume": 1337302 - }, - "id": 2599872, - "non_hive": { - "close": 5416, - "high": 16, - "low": 6234, - "open": 16, - "volume": 519576 - }, - "open": "2020-05-06T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41582, - "high": 50, - "low": 14, - "open": 50, - "volume": 546170 - }, - "id": 2599900, - "non_hive": { - "close": 16000, - "high": 21, - "low": 5, - "open": 21, - "volume": 205617 - }, - "open": "2020-05-06T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7040, - "high": 7729, - "low": 11, - "open": 38895, - "volume": 85758 - }, - "id": 2599962, - "non_hive": { - "close": 2707, - "high": 2972, - "low": 4, - "open": 14952, - "volume": 32957 - }, - "open": "2020-05-06T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20694, - "high": 2, - "low": 7872, - "open": 1300, - "volume": 78687 - }, - "id": 2599988, - "non_hive": { - "close": 7957, - "high": 1, - "low": 2870, - "open": 474, - "volume": 29767 - }, - "open": "2020-05-07T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 80, - "high": 10, - "low": 10291, - "open": 17994, - "volume": 33345 - }, - "id": 2600012, - "non_hive": { - "close": 31, - "high": 4, - "low": 3957, - "open": 6919, - "volume": 12823 - }, - "open": "2020-05-07T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7997, - "high": 610, - "low": 3, - "open": 1157, - "volume": 371231 - }, - "id": 2600036, - "non_hive": { - "close": 3119, - "high": 238, - "low": 1, - "open": 445, - "volume": 142850 - }, - "open": "2020-05-07T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4891, - "high": 23897, - "low": 36733, - "open": 23897, - "volume": 667283 - }, - "id": 2600070, - "non_hive": { - "close": 1904, - "high": 9320, - "low": 14123, - "open": 9320, - "volume": 257009 - }, - "open": "2020-05-07T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 148, - "high": 148, - "low": 40, - "open": 3182, - "volume": 175326 - }, - "id": 2600089, - "non_hive": { - "close": 58, - "high": 58, - "low": 15, - "open": 1239, - "volume": 68340 - }, - "open": "2020-05-07T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5414, - "high": 261, - "low": 14, - "open": 556, - "volume": 627373 - }, - "id": 2600116, - "non_hive": { - "close": 2109, - "high": 102, - "low": 5, - "open": 217, - "volume": 237892 - }, - "open": "2020-05-07T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 52, - "high": 15, - "low": 110, - "open": 5658, - "volume": 95696 - }, - "id": 2600137, - "non_hive": { - "close": 20, - "high": 6, - "low": 40, - "open": 2204, - "volume": 37286 - }, - "open": "2020-05-07T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16465, - "high": 320, - "low": 99, - "open": 692, - "volume": 200984 - }, - "id": 2600170, - "non_hive": { - "close": 6415, - "high": 125, - "low": 36, - "open": 270, - "volume": 78090 - }, - "open": "2020-05-07T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 6005, - "low": 14, - "open": 17455, - "volume": 122661 - }, - "id": 2600201, - "non_hive": { - "close": 5, - "high": 2341, - "low": 5, - "open": 6798, - "volume": 47382 - }, - "open": "2020-05-07T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 620, - "high": 620, - "low": 16, - "open": 6125, - "volume": 71242 - }, - "id": 2600227, - "non_hive": { - "close": 242, - "high": 242, - "low": 6, - "open": 2387, - "volume": 27768 - }, - "open": "2020-05-07T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 940, - "high": 205, - "low": 8593, - "open": 20000, - "volume": 677866 - }, - "id": 2600263, - "non_hive": { - "close": 366, - "high": 80, - "low": 3133, - "open": 7798, - "volume": 249717 - }, - "open": "2020-05-07T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9657, - "high": 6066, - "low": 31, - "open": 20754, - "volume": 305867 - }, - "id": 2600291, - "non_hive": { - "close": 3755, - "high": 2365, - "low": 11, - "open": 8091, - "volume": 115382 - }, - "open": "2020-05-07T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2640, - "high": 48, - "low": 5436, - "open": 18022, - "volume": 790427 - }, - "id": 2600323, - "non_hive": { - "close": 1022, - "high": 19, - "low": 2104, - "open": 7003, - "volume": 306878 - }, - "open": "2020-05-07T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 419, - "high": 2, - "low": 31, - "open": 8600, - "volume": 603493 - }, - "id": 2600361, - "non_hive": { - "close": 160, - "high": 1, - "low": 11, - "open": 3328, - "volume": 223798 - }, - "open": "2020-05-07T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1462, - "high": 940, - "low": 87, - "open": 87, - "volume": 12256 - }, - "id": 2600397, - "non_hive": { - "close": 558, - "high": 359, - "low": 33, - "open": 33, - "volume": 4677 - }, - "open": "2020-05-07T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5464, - "high": 23, - "low": 199, - "open": 3182, - "volume": 246532 - }, - "id": 2600410, - "non_hive": { - "close": 2049, - "high": 9, - "low": 72, - "open": 1209, - "volume": 93477 - }, - "open": "2020-05-07T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21940, - "high": 3461, - "low": 29921, - "open": 3461, - "volume": 364081 - }, - "id": 2600455, - "non_hive": { - "close": 8301, - "high": 1314, - "low": 11071, - "open": 1314, - "volume": 137470 - }, - "open": "2020-05-07T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7770, - "high": 567, - "low": 14, - "open": 562, - "volume": 604769 - }, - "id": 2600495, - "non_hive": { - "close": 2984, - "high": 218, - "low": 5, - "open": 213, - "volume": 230962 - }, - "open": "2020-05-07T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 359, - "high": 44, - "low": 4677, - "open": 30794, - "volume": 242792 - }, - "id": 2600542, - "non_hive": { - "close": 138, - "high": 17, - "low": 1795, - "open": 11825, - "volume": 93233 - }, - "open": "2020-05-07T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1351, - "high": 78, - "low": 11093, - "open": 3463, - "volume": 194040 - }, - "id": 2600576, - "non_hive": { - "close": 519, - "high": 30, - "low": 4258, - "open": 1330, - "volume": 74509 - }, - "open": "2020-05-07T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8026, - "high": 62, - "low": 608, - "open": 12896, - "volume": 95540 - }, - "id": 2600610, - "non_hive": { - "close": 3082, - "high": 24, - "low": 228, - "open": 4952, - "volume": 36659 - }, - "open": "2020-05-07T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 473, - "high": 473, - "low": 10632, - "open": 601, - "volume": 620401 - }, - "id": 2600647, - "non_hive": { - "close": 182, - "high": 182, - "low": 4082, - "open": 231, - "volume": 238235 - }, - "open": "2020-05-07T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1072, - "high": 114930, - "low": 14, - "open": 101, - "volume": 500307 - }, - "id": 2600681, - "non_hive": { - "close": 414, - "high": 44477, - "low": 5, - "open": 39, - "volume": 192625 - }, - "open": "2020-05-07T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1261, - "high": 2, - "low": 441, - "open": 398, - "volume": 425424 - }, - "id": 2600707, - "non_hive": { - "close": 487, - "high": 1, - "low": 165, - "open": 154, - "volume": 164205 - }, - "open": "2020-05-07T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15712, - "high": 8826, - "low": 11381, - "open": 8826, - "volume": 55429 - }, - "id": 2600740, - "non_hive": { - "close": 6065, - "high": 3407, - "low": 4392, - "open": 3407, - "volume": 21395 - }, - "open": "2020-05-08T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 76, - "high": 18, - "low": 50, - "open": 15544, - "volume": 786888 - }, - "id": 2600755, - "non_hive": { - "close": 28, - "high": 7, - "low": 18, - "open": 6000, - "volume": 302481 - }, - "open": "2020-05-08T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16928, - "high": 431, - "low": 3, - "open": 8631, - "volume": 80647 - }, - "id": 2600787, - "non_hive": { - "close": 6353, - "high": 167, - "low": 1, - "open": 3339, - "volume": 30984 - }, - "open": "2020-05-08T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59975, - "high": 312, - "low": 21161, - "open": 5722, - "volume": 126296 - }, - "id": 2600810, - "non_hive": { - "close": 23201, - "high": 121, - "low": 8186, - "open": 2214, - "volume": 48858 - }, - "open": "2020-05-08T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 290, - "high": 860, - "low": 290, - "open": 442, - "volume": 696650 - }, - "id": 2600828, - "non_hive": { - "close": 108, - "high": 333, - "low": 108, - "open": 171, - "volume": 262667 - }, - "open": "2020-05-08T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10439, - "high": 1098, - "low": 14, - "open": 7007, - "volume": 204388 - }, - "id": 2600859, - "non_hive": { - "close": 4035, - "high": 425, - "low": 5, - "open": 2711, - "volume": 79006 - }, - "open": "2020-05-08T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16773, - "high": 2, - "low": 44, - "open": 362, - "volume": 135869 - }, - "id": 2600895, - "non_hive": { - "close": 6490, - "high": 1, - "low": 16, - "open": 140, - "volume": 52569 - }, - "open": "2020-05-08T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14735, - "high": 31, - "low": 251, - "open": 22029, - "volume": 117963 - }, - "id": 2600923, - "non_hive": { - "close": 5683, - "high": 12, - "low": 94, - "open": 8284, - "volume": 45262 - }, - "open": "2020-05-08T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 15794, - "low": 14, - "open": 15794, - "volume": 103285 - }, - "id": 2600954, - "non_hive": { - "close": 5, - "high": 6076, - "low": 5, - "open": 6076, - "volume": 39277 - }, - "open": "2020-05-08T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3302, - "high": 800, - "low": 8, - "open": 2243, - "volume": 142176 - }, - "id": 2600981, - "non_hive": { - "close": 1268, - "high": 308, - "low": 3, - "open": 848, - "volume": 54618 - }, - "open": "2020-05-08T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1304, - "high": 1145, - "low": 3902, - "open": 13733, - "volume": 900858 - }, - "id": 2601012, - "non_hive": { - "close": 501, - "high": 440, - "low": 1464, - "open": 5273, - "volume": 341580 - }, - "open": "2020-05-08T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19660, - "high": 295, - "low": 31, - "open": 18792, - "volume": 108725 - }, - "id": 2601037, - "non_hive": { - "close": 7467, - "high": 113, - "low": 11, - "open": 7051, - "volume": 41177 - }, - "open": "2020-05-08T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15442, - "high": 2, - "low": 113, - "open": 2812, - "volume": 216249 - }, - "id": 2601065, - "non_hive": { - "close": 5856, - "high": 1, - "low": 41, - "open": 1068, - "volume": 82041 - }, - "open": "2020-05-08T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 411, - "high": 34, - "low": 232, - "open": 6587, - "volume": 195539 - }, - "id": 2601105, - "non_hive": { - "close": 156, - "high": 13, - "low": 84, - "open": 2498, - "volume": 74100 - }, - "open": "2020-05-08T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4122, - "high": 4122, - "low": 158, - "open": 232, - "volume": 119960 - }, - "id": 2601133, - "non_hive": { - "close": 1566, - "high": 1566, - "low": 57, - "open": 87, - "volume": 45503 - }, - "open": "2020-05-08T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8811, - "high": 2958, - "low": 933, - "open": 18189, - "volume": 249375 - }, - "id": 2601168, - "non_hive": { - "close": 3336, - "high": 1124, - "low": 353, - "open": 6910, - "volume": 94582 - }, - "open": "2020-05-08T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2727, - "high": 804, - "low": 8549, - "open": 9374, - "volume": 214573 - }, - "id": 2601205, - "non_hive": { - "close": 1033, - "high": 311, - "low": 3236, - "open": 3549, - "volume": 82301 - }, - "open": "2020-05-08T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 411, - "high": 411, - "low": 14, - "open": 99800, - "volume": 142901 - }, - "id": 2601240, - "non_hive": { - "close": 159, - "high": 159, - "low": 5, - "open": 38592, - "volume": 55253 - }, - "open": "2020-05-08T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3998, - "high": 884, - "low": 53999, - "open": 1858, - "volume": 2394932 - }, - "id": 2601258, - "non_hive": { - "close": 1552, - "high": 344, - "low": 19770, - "open": 719, - "volume": 890908 - }, - "open": "2020-05-08T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 237, - "high": 407, - "low": 11774, - "open": 1096, - "volume": 676768 - }, - "id": 2601300, - "non_hive": { - "close": 92, - "high": 158, - "low": 4274, - "open": 425, - "volume": 250768 - }, - "open": "2020-05-08T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2002, - "high": 12, - "low": 12, - "open": 8758, - "volume": 80192 - }, - "id": 2601324, - "non_hive": { - "close": 774, - "high": 5, - "low": 4, - "open": 3391, - "volume": 31001 - }, - "open": "2020-05-08T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1551, - "high": 530, - "low": 106849, - "open": 530, - "volume": 570412 - }, - "id": 2601362, - "non_hive": { - "close": 599, - "high": 205, - "low": 39058, - "open": 205, - "volume": 212177 - }, - "open": "2020-05-08T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 158, - "high": 158, - "low": 14, - "open": 54423, - "volume": 140330 - }, - "id": 2601390, - "non_hive": { - "close": 61, - "high": 61, - "low": 5, - "open": 20681, - "volume": 53455 - }, - "open": "2020-05-08T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 52650, - "high": 57, - "low": 273, - "open": 6561, - "volume": 1883318 - }, - "id": 2601422, - "non_hive": { - "close": 20000, - "high": 22, - "low": 99, - "open": 2529, - "volume": 698793 - }, - "open": "2020-05-08T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1666, - "high": 2049, - "low": 81000, - "open": 81000, - "volume": 284960 - }, - "id": 2601463, - "non_hive": { - "close": 638, - "high": 790, - "low": 29490, - "open": 29490, - "volume": 107274 - }, - "open": "2020-05-09T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3723, - "high": 2869, - "low": 42, - "open": 2869, - "volume": 23410 - }, - "id": 2601508, - "non_hive": { - "close": 1421, - "high": 1097, - "low": 15, - "open": 1097, - "volume": 8685 - }, - "open": "2020-05-09T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 207936, - "high": 326, - "low": 3, - "open": 23909, - "volume": 1288326 - }, - "id": 2601526, - "non_hive": { - "close": 76815, - "high": 126, - "low": 1, - "open": 9114, - "volume": 477132 - }, - "open": "2020-05-09T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 638, - "high": 52, - "low": 638, - "open": 10122, - "volume": 47770 - }, - "id": 2601558, - "non_hive": { - "close": 244, - "high": 20, - "low": 244, - "open": 3875, - "volume": 18284 - }, - "open": "2020-05-09T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 71801, - "high": 149, - "low": 80, - "open": 547, - "volume": 661637 - }, - "id": 2601576, - "non_hive": { - "close": 26325, - "high": 57, - "low": 29, - "open": 203, - "volume": 243646 - }, - "open": "2020-05-09T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2673, - "high": 2, - "low": 14, - "open": 383, - "volume": 282435 - }, - "id": 2601602, - "non_hive": { - "close": 969, - "high": 1, - "low": 5, - "open": 146, - "volume": 105204 - }, - "open": "2020-05-09T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 422, - "high": 18004, - "low": 77, - "open": 13602, - "volume": 282682 - }, - "id": 2601639, - "non_hive": { - "close": 160, - "high": 6836, - "low": 27, - "open": 5164, - "volume": 107148 - }, - "open": "2020-05-09T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16995, - "high": 243, - "low": 11236, - "open": 27795, - "volume": 2127327 - }, - "id": 2601680, - "non_hive": { - "close": 6214, - "high": 92, - "low": 4055, - "open": 10512, - "volume": 789425 - }, - "open": "2020-05-09T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 201795, - "low": 14, - "open": 4407, - "volume": 3224194 - }, - "id": 2601719, - "non_hive": { - "close": 5, - "high": 75925, - "low": 5, - "open": 1591, - "volume": 1164786 - }, - "open": "2020-05-09T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3796, - "high": 601, - "low": 48, - "open": 1335, - "volume": 154564 - }, - "id": 2601753, - "non_hive": { - "close": 1396, - "high": 222, - "low": 17, - "open": 493, - "volume": 56381 - }, - "open": "2020-05-09T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 412, - "high": 29, - "low": 1061, - "open": 125634, - "volume": 2191767 - }, - "id": 2601791, - "non_hive": { - "close": 151, - "high": 11, - "low": 383, - "open": 46191, - "volume": 793030 - }, - "open": "2020-05-09T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 272, - "high": 272, - "low": 31, - "open": 418, - "volume": 632751 - }, - "id": 2601830, - "non_hive": { - "close": 100, - "high": 100, - "low": 11, - "open": 151, - "volume": 228635 - }, - "open": "2020-05-09T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2030, - "high": 23143, - "low": 15, - "open": 7901, - "volume": 826939 - }, - "id": 2601855, - "non_hive": { - "close": 753, - "high": 8596, - "low": 5, - "open": 2900, - "volume": 300480 - }, - "open": "2020-05-09T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 7485, - "low": 13, - "open": 923, - "volume": 690128 - }, - "id": 2601893, - "non_hive": { - "close": 1, - "high": 2774, - "low": 4, - "open": 342, - "volume": 251099 - }, - "open": "2020-05-09T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4841, - "high": 502, - "low": 19, - "open": 10192, - "volume": 1515980 - }, - "id": 2601924, - "non_hive": { - "close": 1792, - "high": 186, - "low": 6, - "open": 3700, - "volume": 551852 - }, - "open": "2020-05-09T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16148, - "high": 3116, - "low": 424180, - "open": 33000, - "volume": 2459223 - }, - "id": 2601980, - "non_hive": { - "close": 6213, - "high": 1199, - "low": 154401, - "open": 12210, - "volume": 910166 - }, - "open": "2020-05-09T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 436726, - "high": 75, - "low": 436726, - "open": 326624, - "volume": 3720415 - }, - "id": 2602034, - "non_hive": { - "close": 155263, - "high": 29, - "low": 155263, - "open": 121518, - "volume": 1350290 - }, - "open": "2020-05-09T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1332, - "high": 4212, - "low": 12, - "open": 10181, - "volume": 3076676 - }, - "id": 2602082, - "non_hive": { - "close": 488, - "high": 1553, - "low": 4, - "open": 3677, - "volume": 1094475 - }, - "open": "2020-05-09T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 92184, - "high": 46, - "low": 92184, - "open": 10729, - "volume": 1308828 - }, - "id": 2602117, - "non_hive": { - "close": 32771, - "high": 17, - "low": 32771, - "open": 3930, - "volume": 466701 - }, - "open": "2020-05-09T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 639, - "high": 2, - "low": 3, - "open": 2736, - "volume": 685343 - }, - "id": 2602148, - "non_hive": { - "close": 234, - "high": 1, - "low": 1, - "open": 1001, - "volume": 250103 - }, - "open": "2020-05-09T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 397, - "high": 672, - "low": 161661, - "open": 2253, - "volume": 3065791 - }, - "id": 2602209, - "non_hive": { - "close": 145, - "high": 246, - "low": 56742, - "open": 824, - "volume": 1092862 - }, - "open": "2020-05-09T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 534, - "high": 274, - "low": 28521, - "open": 274, - "volume": 712187 - }, - "id": 2602247, - "non_hive": { - "close": 193, - "high": 100, - "low": 9982, - "open": 100, - "volume": 250598 - }, - "open": "2020-05-09T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3071, - "high": 4286, - "low": 15, - "open": 3484, - "volume": 105154 - }, - "id": 2602265, - "non_hive": { - "close": 1109, - "high": 1549, - "low": 5, - "open": 1259, - "volume": 37913 - }, - "open": "2020-05-09T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 252374, - "high": 2738, - "low": 12, - "open": 182, - "volume": 753784 - }, - "id": 2602293, - "non_hive": { - "close": 88419, - "high": 1004, - "low": 4, - "open": 66, - "volume": 266740 - }, - "open": "2020-05-09T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 758, - "high": 303, - "low": 184, - "open": 303, - "volume": 380708 - }, - "id": 2602325, - "non_hive": { - "close": 245, - "high": 111, - "low": 59, - "open": 111, - "volume": 129162 - }, - "open": "2020-05-10T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 240, - "high": 16, - "low": 8200, - "open": 437, - "volume": 109078 - }, - "id": 2602358, - "non_hive": { - "close": 87, - "high": 6, - "low": 2727, - "open": 159, - "volume": 39239 - }, - "open": "2020-05-10T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41329, - "high": 930, - "low": 41329, - "open": 3, - "volume": 303705 - }, - "id": 2602393, - "non_hive": { - "close": 13725, - "high": 341, - "low": 13725, - "open": 1, - "volume": 104148 - }, - "open": "2020-05-10T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3628, - "high": 78, - "low": 3628, - "open": 9805, - "volume": 117347 - }, - "id": 2602423, - "non_hive": { - "close": 1172, - "high": 26, - "low": 1172, - "open": 3265, - "volume": 38936 - }, - "open": "2020-05-10T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3961, - "high": 189, - "low": 1220, - "open": 1220, - "volume": 113579 - }, - "id": 2602448, - "non_hive": { - "close": 1318, - "high": 63, - "low": 405, - "open": 405, - "volume": 37792 - }, - "open": "2020-05-10T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10532, - "high": 10532, - "low": 533, - "open": 4553, - "volume": 489461 - }, - "id": 2602474, - "non_hive": { - "close": 3859, - "high": 3859, - "low": 177, - "open": 1515, - "volume": 165930 - }, - "open": "2020-05-10T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20000, - "high": 73, - "low": 5, - "open": 16790, - "volume": 137936 - }, - "id": 2602502, - "non_hive": { - "close": 6680, - "high": 27, - "low": 1, - "open": 6152, - "volume": 49847 - }, - "open": "2020-05-10T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1772, - "high": 1772, - "low": 102, - "open": 1513, - "volume": 11644 - }, - "id": 2602536, - "non_hive": { - "close": 651, - "high": 651, - "low": 34, - "open": 553, - "volume": 4262 - }, - "open": "2020-05-10T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7876, - "high": 1606, - "low": 51, - "open": 743, - "volume": 75261 - }, - "id": 2602559, - "non_hive": { - "close": 2990, - "high": 610, - "low": 17, - "open": 275, - "volume": 27880 - }, - "open": "2020-05-10T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15774, - "high": 5088, - "low": 7888, - "open": 5088, - "volume": 546726 - }, - "id": 2602600, - "non_hive": { - "close": 5599, - "high": 1930, - "low": 2641, - "open": 1930, - "volume": 194824 - }, - "open": "2020-05-10T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3767, - "high": 52, - "low": 19476, - "open": 52, - "volume": 652570 - }, - "id": 2602638, - "non_hive": { - "close": 1411, - "high": 20, - "low": 6855, - "open": 20, - "volume": 237556 - }, - "open": "2020-05-10T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 393, - "high": 6298, - "low": 13, - "open": 6298, - "volume": 502373 - }, - "id": 2602668, - "non_hive": { - "close": 133, - "high": 2314, - "low": 4, - "open": 2314, - "volume": 168024 - }, - "open": "2020-05-10T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5472, - "high": 22322, - "low": 2232, - "open": 1390, - "volume": 618498 - }, - "id": 2602709, - "non_hive": { - "close": 1970, - "high": 8205, - "low": 719, - "open": 469, - "volume": 212618 - }, - "open": "2020-05-10T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10929, - "high": 2439, - "low": 17665, - "open": 2439, - "volume": 789011 - }, - "id": 2602753, - "non_hive": { - "close": 3879, - "high": 890, - "low": 6093, - "open": 890, - "volume": 273490 - }, - "open": "2020-05-10T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1147, - "high": 520, - "low": 5, - "open": 5635, - "volume": 1130370 - }, - "id": 2602788, - "non_hive": { - "close": 408, - "high": 186, - "low": 1, - "open": 2000, - "volume": 393374 - }, - "open": "2020-05-10T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53, - "high": 53, - "low": 74311, - "open": 10000, - "volume": 577144 - }, - "id": 2602835, - "non_hive": { - "close": 19, - "high": 19, - "low": 25278, - "open": 3551, - "volume": 203015 - }, - "open": "2020-05-10T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6921, - "high": 8298, - "low": 26, - "open": 81928, - "volume": 1407747 - }, - "id": 2602890, - "non_hive": { - "close": 2284, - "high": 3048, - "low": 8, - "open": 28973, - "volume": 478985 - }, - "open": "2020-05-10T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31617, - "high": 1966, - "low": 125397, - "open": 81, - "volume": 1575467 - }, - "id": 2602945, - "non_hive": { - "close": 10129, - "high": 649, - "low": 37619, - "open": 26, - "volume": 492825 - }, - "open": "2020-05-10T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 409, - "high": 24, - "low": 19218, - "open": 1384, - "volume": 1077361 - }, - "id": 2602986, - "non_hive": { - "close": 135, - "high": 8, - "low": 5965, - "open": 443, - "volume": 345644 - }, - "open": "2020-05-10T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9606, - "high": 3535, - "low": 3566, - "open": 3566, - "volume": 1088713 - }, - "id": 2603022, - "non_hive": { - "close": 3074, - "high": 1234, - "low": 1130, - "open": 1130, - "volume": 348413 - }, - "open": "2020-05-10T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 392, - "high": 50616, - "low": 15, - "open": 2250, - "volume": 288172 - }, - "id": 2603062, - "non_hive": { - "close": 129, - "high": 16952, - "low": 4, - "open": 720, - "volume": 94959 - }, - "open": "2020-05-10T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1818, - "high": 888, - "low": 192, - "open": 888, - "volume": 75619 - }, - "id": 2603118, - "non_hive": { - "close": 597, - "high": 292, - "low": 61, - "open": 292, - "volume": 24826 - }, - "open": "2020-05-10T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 3194, - "low": 7, - "open": 746, - "volume": 1929195 - }, - "id": 2603139, - "non_hive": { - "close": 4, - "high": 1149, - "low": 2, - "open": 238, - "volume": 615939 - }, - "open": "2020-05-10T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10046, - "high": 13956, - "low": 13, - "open": 14776, - "volume": 493262 - }, - "id": 2603201, - "non_hive": { - "close": 3652, - "high": 5120, - "low": 4, - "open": 4802, - "volume": 161385 - }, - "open": "2020-05-10T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8954, - "high": 106, - "low": 7082, - "open": 75606, - "volume": 262973 - }, - "id": 2603256, - "non_hive": { - "close": 2954, - "high": 35, - "low": 2202, - "open": 24950, - "volume": 86295 - }, - "open": "2020-05-11T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16991, - "high": 16991, - "low": 3127, - "open": 1976, - "volume": 338746 - }, - "id": 2603280, - "non_hive": { - "close": 6178, - "high": 6178, - "low": 1031, - "open": 652, - "volume": 112318 - }, - "open": "2020-05-11T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5264, - "high": 2, - "low": 3, - "open": 2730, - "volume": 59248 - }, - "id": 2603308, - "non_hive": { - "close": 1928, - "high": 1, - "low": 1, - "open": 992, - "volume": 21678 - }, - "open": "2020-05-11T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 406, - "high": 2512, - "low": 250484, - "open": 1244, - "volume": 623954 - }, - "id": 2603335, - "non_hive": { - "close": 148, - "high": 917, - "low": 84345, - "open": 454, - "volume": 210942 - }, - "open": "2020-05-11T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26582, - "high": 41, - "low": 17759, - "open": 28526, - "volume": 133106 - }, - "id": 2603352, - "non_hive": { - "close": 9687, - "high": 15, - "low": 6379, - "open": 10316, - "volume": 48036 - }, - "open": "2020-05-11T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 13288, - "low": 12, - "open": 13288, - "volume": 214423 - }, - "id": 2603377, - "non_hive": { - "close": 1, - "high": 4842, - "low": 4, - "open": 4842, - "volume": 77870 - }, - "open": "2020-05-11T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 180, - "high": 115, - "low": 3, - "open": 3, - "volume": 105804 - }, - "id": 2603396, - "non_hive": { - "close": 65, - "high": 42, - "low": 1, - "open": 1, - "volume": 38457 - }, - "open": "2020-05-11T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21136, - "high": 1289, - "low": 270898, - "open": 1289, - "volume": 646476 - }, - "id": 2603441, - "non_hive": { - "close": 7609, - "high": 467, - "low": 90901, - "open": 467, - "volume": 218137 - }, - "open": "2020-05-11T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15, - "high": 83, - "low": 51, - "open": 83, - "volume": 175762 - }, - "id": 2603467, - "non_hive": { - "close": 5, - "high": 30, - "low": 17, - "open": 30, - "volume": 62703 - }, - "open": "2020-05-11T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1281, - "high": 42971, - "low": 17227, - "open": 18060, - "volume": 103522 - }, - "id": 2603501, - "non_hive": { - "close": 455, - "high": 15371, - "low": 5953, - "open": 6460, - "volume": 36785 - }, - "open": "2020-05-11T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14211, - "high": 158569, - "low": 5883, - "open": 158569, - "volume": 252748 - }, - "id": 2603523, - "non_hive": { - "close": 5000, - "high": 56331, - "low": 2000, - "open": 56331, - "volume": 89447 - }, - "open": "2020-05-11T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 151, - "high": 33, - "low": 30, - "open": 15355, - "volume": 754347 - }, - "id": 2603549, - "non_hive": { - "close": 53, - "high": 12, - "low": 10, - "open": 5402, - "volume": 256032 - }, - "open": "2020-05-11T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45363, - "high": 4331, - "low": 94, - "open": 11888, - "volume": 722204 - }, - "id": 2603583, - "non_hive": { - "close": 15692, - "high": 1516, - "low": 31, - "open": 4090, - "volume": 249885 - }, - "open": "2020-05-11T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10966, - "high": 10966, - "low": 3, - "open": 4339, - "volume": 242302 - }, - "id": 2603620, - "non_hive": { - "close": 3837, - "high": 3837, - "low": 1, - "open": 1501, - "volume": 83802 - }, - "open": "2020-05-11T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4978, - "high": 205, - "low": 302176, - "open": 205, - "volume": 908686 - }, - "id": 2603659, - "non_hive": { - "close": 1740, - "high": 72, - "low": 101497, - "open": 72, - "volume": 309114 - }, - "open": "2020-05-11T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3989, - "high": 32171, - "low": 21342, - "open": 21342, - "volume": 130325 - }, - "id": 2603702, - "non_hive": { - "close": 1396, - "high": 11387, - "low": 7458, - "open": 7458, - "volume": 45712 - }, - "open": "2020-05-11T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2235, - "high": 2816, - "low": 37869, - "open": 45328, - "volume": 1851558 - }, - "id": 2603729, - "non_hive": { - "close": 790, - "high": 1000, - "low": 12595, - "open": 15862, - "volume": 638520 - }, - "open": "2020-05-11T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 141, - "low": 12, - "open": 18394, - "volume": 135200 - }, - "id": 2603758, - "non_hive": { - "close": 4, - "high": 50, - "low": 4, - "open": 6500, - "volume": 47539 - }, - "open": "2020-05-11T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 996, - "high": 2496, - "low": 15, - "open": 2692, - "volume": 740552 - }, - "id": 2603807, - "non_hive": { - "close": 328, - "high": 873, - "low": 4, - "open": 929, - "volume": 251167 - }, - "open": "2020-05-11T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 64717, - "high": 38, - "low": 15371, - "open": 3563, - "volume": 786154 - }, - "id": 2603857, - "non_hive": { - "close": 22000, - "high": 13, - "low": 4953, - "open": 1175, - "volume": 262416 - }, - "open": "2020-05-11T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8817, - "high": 67, - "low": 38, - "open": 2150, - "volume": 690712 - }, - "id": 2603909, - "non_hive": { - "close": 2997, - "high": 23, - "low": 12, - "open": 731, - "volume": 229002 - }, - "open": "2020-05-11T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3796, - "high": 3796, - "low": 1566, - "open": 86, - "volume": 2596498 - }, - "id": 2603953, - "non_hive": { - "close": 1291, - "high": 1291, - "low": 516, - "open": 29, - "volume": 872291 - }, - "open": "2020-05-11T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8750, - "high": 8750, - "low": 12, - "open": 90007, - "volume": 260765 - }, - "id": 2603994, - "non_hive": { - "close": 3031, - "high": 3031, - "low": 4, - "open": 30600, - "volume": 88719 - }, - "open": "2020-05-11T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6603, - "high": 231, - "low": 440, - "open": 17388, - "volume": 860711 - }, - "id": 2604029, - "non_hive": { - "close": 2285, - "high": 80, - "low": 145, - "open": 6017, - "volume": 288467 - }, - "open": "2020-05-11T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2629, - "high": 8115, - "low": 245, - "open": 7936, - "volume": 75876 - }, - "id": 2604075, - "non_hive": { - "close": 918, - "high": 2836, - "low": 80, - "open": 2744, - "volume": 26203 - }, - "open": "2020-05-12T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 564, - "high": 31, - "low": 73471, - "open": 17199, - "volume": 933320 - }, - "id": 2604104, - "non_hive": { - "close": 191, - "high": 11, - "low": 24465, - "open": 6000, - "volume": 318848 - }, - "open": "2020-05-12T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38, - "high": 363, - "low": 3, - "open": 3, - "volume": 36143 - }, - "id": 2604120, - "non_hive": { - "close": 13, - "high": 127, - "low": 1, - "open": 1, - "volume": 12374 - }, - "open": "2020-05-12T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 267, - "high": 23574, - "low": 9065, - "open": 23574, - "volume": 96174 - }, - "id": 2604143, - "non_hive": { - "close": 92, - "high": 8217, - "low": 3122, - "open": 8217, - "volume": 33439 - }, - "open": "2020-05-12T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 106, - "high": 275, - "low": 106, - "open": 38915, - "volume": 64308 - }, - "id": 2604161, - "non_hive": { - "close": 35, - "high": 96, - "low": 35, - "open": 13565, - "volume": 22415 - }, - "open": "2020-05-12T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11924, - "high": 2, - "low": 375, - "open": 20058, - "volume": 58591 - }, - "id": 2604177, - "non_hive": { - "close": 4121, - "high": 1, - "low": 127, - "open": 6986, - "volume": 20157 - }, - "open": "2020-05-12T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6030, - "high": 6030, - "low": 31, - "open": 46, - "volume": 59430 - }, - "id": 2604198, - "non_hive": { - "close": 2104, - "high": 2104, - "low": 10, - "open": 16, - "volume": 20574 - }, - "open": "2020-05-12T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18428, - "high": 2, - "low": 21992, - "open": 95649, - "volume": 771912 - }, - "id": 2604245, - "non_hive": { - "close": 6340, - "high": 1, - "low": 7136, - "open": 33370, - "volume": 259000 - }, - "open": "2020-05-12T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 337, - "low": 13, - "open": 9589, - "volume": 105269 - }, - "id": 2604278, - "non_hive": { - "close": 4, - "high": 116, - "low": 4, - "open": 3290, - "volume": 36147 - }, - "open": "2020-05-12T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 421, - "high": 421, - "low": 13, - "open": 13, - "volume": 224930 - }, - "id": 2604323, - "non_hive": { - "close": 145, - "high": 145, - "low": 4, - "open": 4, - "volume": 76564 - }, - "open": "2020-05-12T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4896, - "high": 1518, - "low": 1850, - "open": 2985, - "volume": 126241 - }, - "id": 2604362, - "non_hive": { - "close": 1683, - "high": 522, - "low": 619, - "open": 1000, - "volume": 43143 - }, - "open": "2020-05-12T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1364, - "high": 1230, - "low": 30, - "open": 23737, - "volume": 54063 - }, - "id": 2604393, - "non_hive": { - "close": 469, - "high": 423, - "low": 10, - "open": 8159, - "volume": 18581 - }, - "open": "2020-05-12T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1452, - "high": 654, - "low": 13865, - "open": 23023, - "volume": 221162 - }, - "id": 2604427, - "non_hive": { - "close": 499, - "high": 225, - "low": 4764, - "open": 7911, - "volume": 75996 - }, - "open": "2020-05-12T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5841, - "high": 759, - "low": 8, - "open": 10996, - "volume": 524620 - }, - "id": 2604456, - "non_hive": { - "close": 2007, - "high": 261, - "low": 2, - "open": 3778, - "volume": 177373 - }, - "open": "2020-05-12T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8891, - "high": 1018, - "low": 1418, - "open": 32633, - "volume": 465574 - }, - "id": 2604504, - "non_hive": { - "close": 3051, - "high": 350, - "low": 476, - "open": 11212, - "volume": 158914 - }, - "open": "2020-05-12T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6708, - "high": 26, - "low": 3158, - "open": 26, - "volume": 81740 - }, - "id": 2604541, - "non_hive": { - "close": 2299, - "high": 9, - "low": 1080, - "open": 9, - "volume": 27998 - }, - "open": "2020-05-12T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5168, - "high": 1064, - "low": 45000, - "open": 235764, - "volume": 1619587 - }, - "id": 2604563, - "non_hive": { - "close": 1770, - "high": 365, - "low": 14381, - "open": 80799, - "volume": 539646 - }, - "open": "2020-05-12T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4095, - "high": 157, - "low": 13, - "open": 32, - "volume": 619368 - }, - "id": 2604612, - "non_hive": { - "close": 1404, - "high": 54, - "low": 4, - "open": 11, - "volume": 212211 - }, - "open": "2020-05-12T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 207, - "high": 309, - "low": 207, - "open": 1391, - "volume": 1220841 - }, - "id": 2604659, - "non_hive": { - "close": 67, - "high": 106, - "low": 67, - "open": 477, - "volume": 401165 - }, - "open": "2020-05-12T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20030, - "high": 314, - "low": 33, - "open": 3563, - "volume": 674472 - }, - "id": 2604680, - "non_hive": { - "close": 6804, - "high": 107, - "low": 10, - "open": 1165, - "volume": 221500 - }, - "open": "2020-05-12T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2496, - "high": 20, - "low": 31, - "open": 6506, - "volume": 151520 - }, - "id": 2604715, - "non_hive": { - "close": 845, - "high": 7, - "low": 10, - "open": 2210, - "volume": 51507 - }, - "open": "2020-05-12T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 645, - "high": 73, - "low": 38, - "open": 31, - "volume": 385980 - }, - "id": 2604742, - "non_hive": { - "close": 219, - "high": 25, - "low": 12, - "open": 10, - "volume": 129078 - }, - "open": "2020-05-12T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2603, - "high": 7577, - "low": 13, - "open": 2348, - "volume": 1439124 - }, - "id": 2604776, - "non_hive": { - "close": 849, - "high": 2572, - "low": 4, - "open": 792, - "volume": 475394 - }, - "open": "2020-05-12T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 289523, - "high": 6279, - "low": 21, - "open": 111535, - "volume": 1664957 - }, - "id": 2604809, - "non_hive": { - "close": 93225, - "high": 2132, - "low": 6, - "open": 37782, - "volume": 544951 - }, - "open": "2020-05-12T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 160710, - "high": 112, - "low": 7328, - "open": 11144, - "volume": 224287 - }, - "id": 2604848, - "non_hive": { - "close": 54521, - "high": 38, - "low": 2471, - "open": 3768, - "volume": 76041 - }, - "open": "2020-05-13T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 553, - "high": 29696, - "low": 406, - "open": 34076, - "volume": 112290 - }, - "id": 2604868, - "non_hive": { - "close": 182, - "high": 10078, - "low": 130, - "open": 11560, - "volume": 38083 - }, - "open": "2020-05-13T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 66457, - "high": 9366, - "low": 4, - "open": 9366, - "volume": 77772 - }, - "id": 2604884, - "non_hive": { - "close": 22489, - "high": 3180, - "low": 1, - "open": 3180, - "volume": 26330 - }, - "open": "2020-05-13T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16509, - "high": 103, - "low": 1547, - "open": 21855, - "volume": 181612 - }, - "id": 2604900, - "non_hive": { - "close": 5594, - "high": 35, - "low": 510, - "open": 7419, - "volume": 61597 - }, - "open": "2020-05-13T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5248, - "high": 2005, - "low": 5248, - "open": 2005, - "volume": 7253 - }, - "id": 2604923, - "non_hive": { - "close": 1732, - "high": 662, - "low": 1732, - "open": 662, - "volume": 2394 - }, - "open": "2020-05-13T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4201, - "high": 20106, - "low": 13, - "open": 8030, - "volume": 684508 - }, - "id": 2604930, - "non_hive": { - "close": 1395, - "high": 6794, - "low": 4, - "open": 2713, - "volume": 224884 - }, - "open": "2020-05-13T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 601, - "high": 1476, - "low": 31, - "open": 326, - "volume": 187686 - }, - "id": 2604956, - "non_hive": { - "close": 199, - "high": 490, - "low": 10, - "open": 108, - "volume": 62086 - }, - "open": "2020-05-13T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 912, - "high": 912, - "low": 39, - "open": 151, - "volume": 115770 - }, - "id": 2604990, - "non_hive": { - "close": 303, - "high": 303, - "low": 12, - "open": 50, - "volume": 38415 - }, - "open": "2020-05-13T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29389, - "high": 39, - "low": 13, - "open": 3974, - "volume": 82291 - }, - "id": 2605018, - "non_hive": { - "close": 9753, - "high": 13, - "low": 4, - "open": 1319, - "volume": 27314 - }, - "open": "2020-05-13T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 367, - "high": 367, - "low": 146150, - "open": 18037, - "volume": 680972 - }, - "id": 2605055, - "non_hive": { - "close": 122, - "high": 122, - "low": 47498, - "open": 5988, - "volume": 222505 - }, - "open": "2020-05-13T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11886, - "high": 60, - "low": 10, - "open": 2829, - "volume": 1065965 - }, - "id": 2605082, - "non_hive": { - "close": 3945, - "high": 20, - "low": 3, - "open": 939, - "volume": 353758 - }, - "open": "2020-05-13T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 427, - "high": 126, - "low": 13, - "open": 412, - "volume": 129662 - }, - "id": 2605124, - "non_hive": { - "close": 142, - "high": 42, - "low": 4, - "open": 137, - "volume": 43046 - }, - "open": "2020-05-13T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 876, - "high": 165, - "low": 1276, - "open": 165, - "volume": 29042 - }, - "id": 2605162, - "non_hive": { - "close": 291, - "high": 55, - "low": 423, - "open": 55, - "volume": 9642 - }, - "open": "2020-05-13T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 454, - "high": 454, - "low": 19792, - "open": 2518, - "volume": 47123 - }, - "id": 2605176, - "non_hive": { - "close": 151, - "high": 151, - "low": 6571, - "open": 836, - "volume": 15646 - }, - "open": "2020-05-13T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16000, - "high": 96, - "low": 42592, - "open": 39157, - "volume": 534614 - }, - "id": 2605201, - "non_hive": { - "close": 5312, - "high": 32, - "low": 14140, - "open": 13000, - "volume": 177492 - }, - "open": "2020-05-13T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 67846, - "high": 12873, - "low": 1540, - "open": 4759, - "volume": 2521063 - }, - "id": 2605231, - "non_hive": { - "close": 22543, - "high": 4380, - "low": 496, - "open": 1580, - "volume": 849087 - }, - "open": "2020-05-13T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 85110, - "high": 132, - "low": 155303, - "open": 92848, - "volume": 2661916 - }, - "id": 2605289, - "non_hive": { - "close": 28341, - "high": 44, - "low": 50121, - "open": 30000, - "volume": 872816 - }, - "open": "2020-05-13T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2220, - "high": 3591, - "low": 13, - "open": 3591, - "volume": 1549919 - }, - "id": 2605317, - "non_hive": { - "close": 751, - "high": 1221, - "low": 4, - "open": 1221, - "volume": 505898 - }, - "open": "2020-05-13T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12204, - "high": 292, - "low": 20, - "open": 11835, - "volume": 126418 - }, - "id": 2605354, - "non_hive": { - "close": 4126, - "high": 99, - "low": 6, - "open": 4004, - "volume": 42684 - }, - "open": "2020-05-13T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20945, - "high": 2855, - "low": 3562, - "open": 10962, - "volume": 96729 - }, - "id": 2605394, - "non_hive": { - "close": 7076, - "high": 965, - "low": 1175, - "open": 3705, - "volume": 32567 - }, - "open": "2020-05-13T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11123, - "high": 20, - "low": 308, - "open": 1021, - "volume": 410484 - }, - "id": 2605419, - "non_hive": { - "close": 3756, - "high": 7, - "low": 102, - "open": 345, - "volume": 138511 - }, - "open": "2020-05-13T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 315, - "high": 27555, - "low": 315, - "open": 36699, - "volume": 783638 - }, - "id": 2605468, - "non_hive": { - "close": 104, - "high": 9312, - "low": 104, - "open": 12400, - "volume": 261914 - }, - "open": "2020-05-13T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 172, - "high": 62, - "low": 13, - "open": 12624, - "volume": 959807 - }, - "id": 2605488, - "non_hive": { - "close": 55, - "high": 21, - "low": 4, - "open": 4165, - "volume": 318151 - }, - "open": "2020-05-13T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 69, - "high": 67528, - "low": 13, - "open": 307402, - "volume": 516869 - }, - "id": 2605521, - "non_hive": { - "close": 22, - "high": 22785, - "low": 4, - "open": 100000, - "volume": 169288 - }, - "open": "2020-05-13T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2544, - "high": 11, - "low": 207, - "open": 2961, - "volume": 829975 - }, - "id": 2605547, - "non_hive": { - "close": 848, - "high": 4, - "low": 66, - "open": 996, - "volume": 271898 - }, - "open": "2020-05-14T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 581, - "high": 32, - "low": 12270, - "open": 12270, - "volume": 60693 - }, - "id": 2605572, - "non_hive": { - "close": 196, - "high": 11, - "low": 4090, - "open": 4090, - "volume": 20427 - }, - "open": "2020-05-14T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3296, - "high": 575, - "low": 3, - "open": 5087, - "volume": 70173 - }, - "id": 2605601, - "non_hive": { - "close": 1112, - "high": 194, - "low": 1, - "open": 1716, - "volume": 23673 - }, - "open": "2020-05-14T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 166, - "high": 166, - "low": 140, - "open": 3279, - "volume": 725716 - }, - "id": 2605622, - "non_hive": { - "close": 56, - "high": 56, - "low": 45, - "open": 1093, - "volume": 238652 - }, - "open": "2020-05-14T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25673, - "high": 1304, - "low": 128, - "open": 3430, - "volume": 86973 - }, - "id": 2605649, - "non_hive": { - "close": 8662, - "high": 440, - "low": 41, - "open": 1157, - "volume": 29321 - }, - "open": "2020-05-14T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4871, - "high": 5101, - "low": 232, - "open": 8504, - "volume": 64781 - }, - "id": 2605674, - "non_hive": { - "close": 1640, - "high": 1721, - "low": 75, - "open": 2869, - "volume": 21829 - }, - "open": "2020-05-14T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15681, - "high": 18150, - "low": 29, - "open": 18150, - "volume": 328208 - }, - "id": 2605698, - "non_hive": { - "close": 5201, - "high": 6095, - "low": 9, - "open": 6095, - "volume": 106236 - }, - "open": "2020-05-14T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2162, - "high": 18, - "low": 103, - "open": 2551, - "volume": 46742 - }, - "id": 2605726, - "non_hive": { - "close": 693, - "high": 6, - "low": 33, - "open": 846, - "volume": 15255 - }, - "open": "2020-05-14T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 13309, - "low": 13, - "open": 4831, - "volume": 2349741 - }, - "id": 2605756, - "non_hive": { - "close": 4, - "high": 4487, - "low": 4, - "open": 1548, - "volume": 777239 - }, - "open": "2020-05-14T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5882, - "high": 267, - "low": 595901, - "open": 1327, - "volume": 1676224 - }, - "id": 2605808, - "non_hive": { - "close": 1936, - "high": 90, - "low": 190767, - "open": 447, - "volume": 541907 - }, - "open": "2020-05-14T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 576619, - "high": 18, - "low": 162, - "open": 2212, - "volume": 3355581 - }, - "id": 2605845, - "non_hive": { - "close": 184595, - "high": 6, - "low": 51, - "open": 728, - "volume": 1077610 - }, - "open": "2020-05-14T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1074, - "high": 42, - "low": 13, - "open": 42, - "volume": 1445748 - }, - "id": 2605908, - "non_hive": { - "close": 353, - "high": 14, - "low": 4, - "open": 14, - "volume": 464927 - }, - "open": "2020-05-14T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7962, - "high": 3, - "low": 889, - "open": 3114, - "volume": 1984467 - }, - "id": 2605943, - "non_hive": { - "close": 2551, - "high": 1, - "low": 284, - "open": 1000, - "volume": 636859 - }, - "open": "2020-05-14T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 79, - "high": 404, - "low": 7, - "open": 26282, - "volume": 651955 - }, - "id": 2605992, - "non_hive": { - "close": 25, - "high": 133, - "low": 2, - "open": 8638, - "volume": 209254 - }, - "open": "2020-05-14T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15464, - "high": 6429, - "low": 26, - "open": 11107, - "volume": 136065 - }, - "id": 2606021, - "non_hive": { - "close": 5070, - "high": 2108, - "low": 8, - "open": 3641, - "volume": 44591 - }, - "open": "2020-05-14T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 203, - "high": 8952, - "low": 203, - "open": 8952, - "volume": 804552 - }, - "id": 2606048, - "non_hive": { - "close": 66, - "high": 2935, - "low": 64, - "open": 2935, - "volume": 259527 - }, - "open": "2020-05-14T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 153, - "high": 579, - "low": 20, - "open": 249999, - "volume": 918276 - }, - "id": 2606091, - "non_hive": { - "close": 51, - "high": 193, - "low": 6, - "open": 81950, - "volume": 303191 - }, - "open": "2020-05-14T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14965, - "high": 326, - "low": 377, - "open": 377, - "volume": 887788 - }, - "id": 2606162, - "non_hive": { - "close": 5039, - "high": 110, - "low": 125, - "open": 125, - "volume": 297212 - }, - "open": "2020-05-14T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3002, - "high": 1256, - "low": 253, - "open": 29700, - "volume": 184771 - }, - "id": 2606213, - "non_hive": { - "close": 1000, - "high": 423, - "low": 84, - "open": 10001, - "volume": 62105 - }, - "open": "2020-05-14T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3029, - "high": 7790, - "low": 2402, - "open": 3002, - "volume": 146627 - }, - "id": 2606252, - "non_hive": { - "close": 1000, - "high": 2618, - "low": 784, - "open": 1000, - "volume": 49079 - }, - "open": "2020-05-14T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 122, - "high": 44, - "low": 223827, - "open": 3029, - "volume": 1283762 - }, - "id": 2606297, - "non_hive": { - "close": 41, - "high": 15, - "low": 72743, - "open": 1000, - "volume": 418903 - }, - "open": "2020-05-14T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 70, - "high": 26, - "low": 20, - "open": 2610, - "volume": 110593 - }, - "id": 2606343, - "non_hive": { - "close": 23, - "high": 9, - "low": 6, - "open": 872, - "volume": 37023 - }, - "open": "2020-05-14T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5867, - "high": 4174, - "low": 13, - "open": 4174, - "volume": 104749 - }, - "id": 2606383, - "non_hive": { - "close": 1952, - "high": 1402, - "low": 4, - "open": 1402, - "volume": 34870 - }, - "open": "2020-05-14T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8873, - "high": 120, - "low": 13, - "open": 120, - "volume": 111636 - }, - "id": 2606407, - "non_hive": { - "close": 2952, - "high": 40, - "low": 4, - "open": 40, - "volume": 36995 - }, - "open": "2020-05-14T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 39, - "low": 4, - "open": 5913, - "volume": 348664 - }, - "id": 2606434, - "non_hive": { - "close": 1, - "high": 13, - "low": 1, - "open": 1967, - "volume": 115990 - }, - "open": "2020-05-15T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 99, - "high": 48, - "low": 99, - "open": 395, - "volume": 688892 - }, - "id": 2606478, - "non_hive": { - "close": 32, - "high": 16, - "low": 32, - "open": 131, - "volume": 225772 - }, - "open": "2020-05-15T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16925, - "high": 16925, - "low": 99, - "open": 99, - "volume": 57589 - }, - "id": 2606516, - "non_hive": { - "close": 5685, - "high": 5685, - "low": 32, - "open": 32, - "volume": 19150 - }, - "open": "2020-05-15T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3117, - "high": 5249, - "low": 123, - "open": 5249, - "volume": 1373809 - }, - "id": 2606528, - "non_hive": { - "close": 1000, - "high": 1759, - "low": 39, - "open": 1759, - "volume": 445208 - }, - "open": "2020-05-15T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3117, - "high": 3244, - "low": 152, - "open": 3117, - "volume": 85846 - }, - "id": 2606599, - "non_hive": { - "close": 1000, - "high": 1089, - "low": 48, - "open": 1000, - "volume": 28111 - }, - "open": "2020-05-15T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10105, - "high": 3663, - "low": 13, - "open": 3117, - "volume": 690452 - }, - "id": 2606648, - "non_hive": { - "close": 3335, - "high": 1230, - "low": 4, - "open": 1000, - "volume": 222632 - }, - "open": "2020-05-15T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 424, - "high": 8851, - "low": 31, - "open": 8851, - "volume": 75064 - }, - "id": 2606685, - "non_hive": { - "close": 137, - "high": 2946, - "low": 10, - "open": 2946, - "volume": 24915 - }, - "open": "2020-05-15T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4543, - "high": 7321, - "low": 17, - "open": 20000, - "volume": 45591 - }, - "id": 2606705, - "non_hive": { - "close": 1507, - "high": 2429, - "low": 5, - "open": 6632, - "volume": 15119 - }, - "open": "2020-05-15T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 1000, - "low": 13, - "open": 673, - "volume": 16819 - }, - "id": 2606727, - "non_hive": { - "close": 4, - "high": 332, - "low": 4, - "open": 217, - "volume": 5557 - }, - "open": "2020-05-15T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2407, - "high": 1618, - "low": 19, - "open": 6788, - "volume": 929112 - }, - "id": 2606758, - "non_hive": { - "close": 794, - "high": 537, - "low": 6, - "open": 2252, - "volume": 303834 - }, - "open": "2020-05-15T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8899, - "high": 153, - "low": 228, - "open": 67289, - "volume": 1278900 - }, - "id": 2606798, - "non_hive": { - "close": 2949, - "high": 51, - "low": 73, - "open": 22207, - "volume": 415120 - }, - "open": "2020-05-15T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2596, - "high": 1074, - "low": 13, - "open": 7185, - "volume": 104789 - }, - "id": 2606840, - "non_hive": { - "close": 860, - "high": 356, - "low": 4, - "open": 2381, - "volume": 34670 - }, - "open": "2020-05-15T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5294, - "high": 21, - "low": 16, - "open": 12125, - "volume": 851274 - }, - "id": 2606882, - "non_hive": { - "close": 1754, - "high": 7, - "low": 5, - "open": 4016, - "volume": 282045 - }, - "open": "2020-05-15T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4642, - "high": 299, - "low": 677, - "open": 4541, - "volume": 836713 - }, - "id": 2606921, - "non_hive": { - "close": 1547, - "high": 100, - "low": 217, - "open": 1505, - "volume": 274582 - }, - "open": "2020-05-15T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 354, - "low": 15772, - "open": 1322, - "volume": 203375 - }, - "id": 2606972, - "non_hive": { - "close": 3331, - "high": 118, - "low": 5205, - "open": 440, - "volume": 67667 - }, - "open": "2020-05-15T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1022, - "high": 3, - "low": 252884, - "open": 44470, - "volume": 3236618 - }, - "id": 2607010, - "non_hive": { - "close": 340, - "high": 1, - "low": 82186, - "open": 14813, - "volume": 1058061 - }, - "open": "2020-05-15T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 190496, - "high": 27, - "low": 190496, - "open": 1984, - "volume": 1166455 - }, - "id": 2607071, - "non_hive": { - "close": 61066, - "high": 9, - "low": 61066, - "open": 660, - "volume": 378261 - }, - "open": "2020-05-15T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 215, - "high": 313, - "low": 13, - "open": 3558, - "volume": 101561 - }, - "id": 2607117, - "non_hive": { - "close": 70, - "high": 102, - "low": 4, - "open": 1156, - "volume": 33002 - }, - "open": "2020-05-15T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5057, - "high": 49, - "low": 9, - "open": 1018, - "volume": 327213 - }, - "id": 2607162, - "non_hive": { - "close": 1643, - "high": 16, - "low": 2, - "open": 331, - "volume": 106316 - }, - "open": "2020-05-15T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5001, - "high": 1018, - "low": 7, - "open": 221, - "volume": 2113259 - }, - "id": 2607209, - "non_hive": { - "close": 1625, - "high": 331, - "low": 2, - "open": 71, - "volume": 677834 - }, - "open": "2020-05-15T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55, - "high": 110, - "low": 4466, - "open": 6484, - "volume": 201143 - }, - "id": 2607246, - "non_hive": { - "close": 18, - "high": 36, - "low": 1430, - "open": 2107, - "volume": 65332 - }, - "open": "2020-05-15T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5743, - "high": 338, - "low": 60890, - "open": 338, - "volume": 528978 - }, - "id": 2607282, - "non_hive": { - "close": 1866, - "high": 110, - "low": 19781, - "open": 110, - "volume": 171858 - }, - "open": "2020-05-15T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37, - "high": 824, - "low": 13, - "open": 824, - "volume": 195556 - }, - "id": 2607315, - "non_hive": { - "close": 12, - "high": 268, - "low": 4, - "open": 268, - "volume": 63534 - }, - "open": "2020-05-15T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3082, - "high": 3, - "low": 13, - "open": 333, - "volume": 2282579 - }, - "id": 2607343, - "non_hive": { - "close": 999, - "high": 1, - "low": 4, - "open": 108, - "volume": 731301 - }, - "open": "2020-05-15T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 302, - "high": 9171, - "low": 58, - "open": 6596, - "volume": 195705 - }, - "id": 2607391, - "non_hive": { - "close": 98, - "high": 3030, - "low": 18, - "open": 2138, - "volume": 63396 - }, - "open": "2020-05-16T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15135, - "high": 60, - "low": 124, - "open": 533239, - "volume": 671809 - }, - "id": 2607441, - "non_hive": { - "close": 5000, - "high": 20, - "low": 40, - "open": 175590, - "volume": 220995 - }, - "open": "2020-05-16T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27, - "high": 42, - "low": 12, - "open": 53612, - "volume": 88610 - }, - "id": 2607462, - "non_hive": { - "close": 8, - "high": 14, - "low": 3, - "open": 17710, - "volume": 29205 - }, - "open": "2020-05-16T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 94, - "high": 12321, - "low": 3325, - "open": 6153, - "volume": 1062872 - }, - "id": 2607492, - "non_hive": { - "close": 31, - "high": 4069, - "low": 1000, - "open": 2000, - "volume": 340224 - }, - "open": "2020-05-16T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35270, - "high": 618, - "low": 35270, - "open": 2348, - "volume": 128979 - }, - "id": 2607512, - "non_hive": { - "close": 11373, - "high": 200, - "low": 11373, - "open": 759, - "volume": 41654 - }, - "open": "2020-05-16T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6938, - "high": 9004, - "low": 13, - "open": 7570, - "volume": 90864 - }, - "id": 2607528, - "non_hive": { - "close": 2286, - "high": 2968, - "low": 4, - "open": 2439, - "volume": 29776 - }, - "open": "2020-05-16T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2359, - "high": 303, - "low": 31, - "open": 2185, - "volume": 614389 - }, - "id": 2607552, - "non_hive": { - "close": 776, - "high": 100, - "low": 10, - "open": 720, - "volume": 199006 - }, - "open": "2020-05-16T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 190, - "high": 5464, - "low": 45, - "open": 587, - "volume": 635044 - }, - "id": 2607572, - "non_hive": { - "close": 62, - "high": 1799, - "low": 14, - "open": 193, - "volume": 205090 - }, - "open": "2020-05-16T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 41843, - "low": 52, - "open": 118, - "volume": 47028 - }, - "id": 2607599, - "non_hive": { - "close": 4, - "high": 13469, - "low": 16, - "open": 37, - "volume": 15135 - }, - "open": "2020-05-16T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31116, - "high": 1015, - "low": 22876, - "open": 10372, - "volume": 91366 - }, - "id": 2607614, - "non_hive": { - "close": 10016, - "high": 327, - "low": 7353, - "open": 3334, - "volume": 29389 - }, - "open": "2020-05-16T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 524, - "high": 55, - "low": 33350, - "open": 12128, - "volume": 222225 - }, - "id": 2607631, - "non_hive": { - "close": 168, - "high": 18, - "low": 10669, - "open": 3904, - "volume": 71409 - }, - "open": "2020-05-16T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 129, - "high": 114, - "low": 13, - "open": 8767, - "volume": 76942 - }, - "id": 2607658, - "non_hive": { - "close": 40, - "high": 37, - "low": 4, - "open": 2821, - "volume": 24717 - }, - "open": "2020-05-16T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16679, - "high": 3, - "low": 275, - "open": 341, - "volume": 192100 - }, - "id": 2607691, - "non_hive": { - "close": 5369, - "high": 1, - "low": 88, - "open": 110, - "volume": 61826 - }, - "open": "2020-05-16T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11823, - "high": 546, - "low": 485, - "open": 14306, - "volume": 74926 - }, - "id": 2607727, - "non_hive": { - "close": 3806, - "high": 176, - "low": 156, - "open": 4605, - "volume": 24116 - }, - "open": "2020-05-16T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2117, - "high": 2214, - "low": 16623, - "open": 17169, - "volume": 445229 - }, - "id": 2607760, - "non_hive": { - "close": 696, - "high": 728, - "low": 5351, - "open": 5527, - "volume": 143481 - }, - "open": "2020-05-16T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 188, - "high": 544, - "low": 188, - "open": 310616, - "volume": 1272034 - }, - "id": 2607784, - "non_hive": { - "close": 60, - "high": 179, - "low": 60, - "open": 100000, - "volume": 409466 - }, - "open": "2020-05-16T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 438, - "high": 438, - "low": 245, - "open": 198, - "volume": 697339 - }, - "id": 2607815, - "non_hive": { - "close": 144, - "high": 144, - "low": 78, - "open": 65, - "volume": 224988 - }, - "open": "2020-05-16T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6552, - "high": 85, - "low": 13, - "open": 18535, - "volume": 139187 - }, - "id": 2607848, - "non_hive": { - "close": 2148, - "high": 28, - "low": 4, - "open": 6081, - "volume": 45655 - }, - "open": "2020-05-16T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3263, - "high": 341, - "low": 51, - "open": 341, - "volume": 225711 - }, - "id": 2607892, - "non_hive": { - "close": 1065, - "high": 112, - "low": 16, - "open": 112, - "volume": 73704 - }, - "open": "2020-05-16T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1465, - "high": 180, - "low": 1465, - "open": 456, - "volume": 685161 - }, - "id": 2607929, - "non_hive": { - "close": 471, - "high": 59, - "low": 471, - "open": 149, - "volume": 220988 - }, - "open": "2020-05-16T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 973, - "high": 96, - "low": 44002, - "open": 96, - "volume": 50178 - }, - "id": 2607949, - "non_hive": { - "close": 313, - "high": 31, - "low": 14143, - "open": 31, - "volume": 16129 - }, - "open": "2020-05-16T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10816, - "high": 13504, - "low": 13, - "open": 2585, - "volume": 65261 - }, - "id": 2607964, - "non_hive": { - "close": 3477, - "high": 4342, - "low": 4, - "open": 831, - "volume": 20979 - }, - "open": "2020-05-16T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 345, - "high": 345, - "low": 13, - "open": 3472, - "volume": 1022689 - }, - "id": 2607985, - "non_hive": { - "close": 111, - "high": 111, - "low": 4, - "open": 1116, - "volume": 324453 - }, - "open": "2020-05-16T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18704, - "high": 7108, - "low": 23, - "open": 7108, - "volume": 910162 - }, - "id": 2608015, - "non_hive": { - "close": 5948, - "high": 2283, - "low": 7, - "open": 2283, - "volume": 285547 - }, - "open": "2020-05-16T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10818, - "high": 22614, - "low": 306, - "open": 6291, - "volume": 276124 - }, - "id": 2608058, - "non_hive": { - "close": 3375, - "high": 7186, - "low": 94, - "open": 1999, - "volume": 86563 - }, - "open": "2020-05-17T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10577, - "high": 15573, - "low": 204, - "open": 15573, - "volume": 661782 - }, - "id": 2608087, - "non_hive": { - "close": 3331, - "high": 5000, - "low": 63, - "open": 5000, - "volume": 207348 - }, - "open": "2020-05-17T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5453, - "high": 339, - "low": 90, - "open": 6237, - "volume": 84732 - }, - "id": 2608112, - "non_hive": { - "close": 1750, - "high": 109, - "low": 28, - "open": 2002, - "volume": 27195 - }, - "open": "2020-05-17T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53, - "high": 538, - "low": 301, - "open": 2810, - "volume": 120097 - }, - "id": 2608135, - "non_hive": { - "close": 17, - "high": 173, - "low": 93, - "open": 902, - "volume": 38546 - }, - "open": "2020-05-17T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4763, - "high": 2090, - "low": 279, - "open": 29638, - "volume": 977958 - }, - "id": 2608161, - "non_hive": { - "close": 1527, - "high": 671, - "low": 87, - "open": 9470, - "volume": 308469 - }, - "open": "2020-05-17T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 40, - "low": 46, - "open": 68833, - "volume": 229957 - }, - "id": 2608182, - "non_hive": { - "close": 4, - "high": 13, - "low": 14, - "open": 22088, - "volume": 73478 - }, - "open": "2020-05-17T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7494, - "high": 882, - "low": 57, - "open": 12500, - "volume": 30071 - }, - "id": 2608209, - "non_hive": { - "close": 2342, - "high": 282, - "low": 17, - "open": 3995, - "volume": 9517 - }, - "open": "2020-05-17T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 588, - "high": 153, - "low": 70, - "open": 160, - "volume": 692866 - }, - "id": 2608234, - "non_hive": { - "close": 186, - "high": 49, - "low": 21, - "open": 51, - "volume": 215533 - }, - "open": "2020-05-17T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 296, - "low": 13, - "open": 12460, - "volume": 60632 - }, - "id": 2608273, - "non_hive": { - "close": 4, - "high": 95, - "low": 4, - "open": 3991, - "volume": 19397 - }, - "open": "2020-05-17T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 611, - "high": 13580, - "low": 504, - "open": 13580, - "volume": 298188 - }, - "id": 2608304, - "non_hive": { - "close": 195, - "high": 4340, - "low": 156, - "open": 4340, - "volume": 94827 - }, - "open": "2020-05-17T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5420, - "high": 229, - "low": 104, - "open": 229, - "volume": 96912 - }, - "id": 2608329, - "non_hive": { - "close": 1680, - "high": 73, - "low": 32, - "open": 73, - "volume": 30518 - }, - "open": "2020-05-17T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7952, - "high": 546, - "low": 30, - "open": 944, - "volume": 130424 - }, - "id": 2608355, - "non_hive": { - "close": 2520, - "high": 174, - "low": 9, - "open": 300, - "volume": 41437 - }, - "open": "2020-05-17T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 70155, - "high": 47, - "low": 51, - "open": 268, - "volume": 307792 - }, - "id": 2608393, - "non_hive": { - "close": 22169, - "high": 15, - "low": 15, - "open": 85, - "volume": 97791 - }, - "open": "2020-05-17T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 167464, - "high": 167464, - "low": 23, - "open": 4774, - "volume": 351647 - }, - "id": 2608431, - "non_hive": { - "close": 53312, - "high": 53312, - "low": 7, - "open": 1519, - "volume": 111865 - }, - "open": "2020-05-17T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16496, - "high": 97, - "low": 4881, - "open": 67190, - "volume": 221852 - }, - "id": 2608463, - "non_hive": { - "close": 5263, - "high": 31, - "low": 1553, - "open": 21385, - "volume": 70676 - }, - "open": "2020-05-17T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40020, - "high": 68779, - "low": 11592, - "open": 86624, - "volume": 1093113 - }, - "id": 2608500, - "non_hive": { - "close": 12999, - "high": 22344, - "low": 3698, - "open": 27636, - "volume": 349399 - }, - "open": "2020-05-17T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 396008, - "high": 7259, - "low": 123, - "open": 15536, - "volume": 871824 - }, - "id": 2608532, - "non_hive": { - "close": 125671, - "high": 2359, - "low": 38, - "open": 5001, - "volume": 277317 - }, - "open": "2020-05-17T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 69, - "high": 76, - "low": 4, - "open": 215, - "volume": 1396260 - }, - "id": 2608573, - "non_hive": { - "close": 22, - "high": 25, - "low": 1, - "open": 70, - "volume": 452135 - }, - "open": "2020-05-17T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10044, - "high": 1284, - "low": 127027, - "open": 5569, - "volume": 1295548 - }, - "id": 2608635, - "non_hive": { - "close": 3212, - "high": 417, - "low": 40141, - "open": 1808, - "volume": 411518 - }, - "open": "2020-05-17T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 272, - "high": 200, - "low": 272, - "open": 3567, - "volume": 682315 - }, - "id": 2608673, - "non_hive": { - "close": 84, - "high": 65, - "low": 84, - "open": 1130, - "volume": 215839 - }, - "open": "2020-05-17T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8399, - "high": 88, - "low": 8, - "open": 17010, - "volume": 794243 - }, - "id": 2608706, - "non_hive": { - "close": 2671, - "high": 28, - "low": 2, - "open": 5378, - "volume": 249654 - }, - "open": "2020-05-17T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 252089, - "high": 252089, - "low": 197, - "open": 81, - "volume": 1304892 - }, - "id": 2608753, - "non_hive": { - "close": 81897, - "high": 81897, - "low": 62, - "open": 26, - "volume": 418195 - }, - "open": "2020-05-17T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1803, - "high": 1332, - "low": 13, - "open": 1893, - "volume": 641355 - }, - "id": 2608794, - "non_hive": { - "close": 586, - "high": 433, - "low": 4, - "open": 615, - "volume": 205151 - }, - "open": "2020-05-17T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33967, - "high": 60, - "low": 13, - "open": 12604, - "volume": 3172678 - }, - "id": 2608822, - "non_hive": { - "close": 11257, - "high": 20, - "low": 4, - "open": 4094, - "volume": 1039710 - }, - "open": "2020-05-17T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11835, - "high": 8757, - "low": 85, - "open": 13801, - "volume": 134827 - }, - "id": 2608886, - "non_hive": { - "close": 3988, - "high": 2951, - "low": 28, - "open": 4574, - "volume": 44867 - }, - "open": "2020-05-18T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3299, - "high": 29, - "low": 78891, - "open": 6000, - "volume": 667675 - }, - "id": 2608932, - "non_hive": { - "close": 1116, - "high": 10, - "low": 25951, - "open": 2022, - "volume": 224300 - }, - "open": "2020-05-18T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 357, - "high": 357, - "low": 3, - "open": 2205, - "volume": 50414 - }, - "id": 2608969, - "non_hive": { - "close": 121, - "high": 121, - "low": 1, - "open": 746, - "volume": 17002 - }, - "open": "2020-05-18T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10868, - "high": 112, - "low": 49270, - "open": 20621, - "volume": 92652 - }, - "id": 2608986, - "non_hive": { - "close": 3676, - "high": 38, - "low": 16663, - "open": 6974, - "volume": 31336 - }, - "open": "2020-05-18T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6214, - "high": 12889, - "low": 2940, - "open": 3001, - "volume": 764116 - }, - "id": 2609001, - "non_hive": { - "close": 2075, - "high": 4509, - "low": 981, - "open": 1015, - "volume": 257058 - }, - "open": "2020-05-18T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2920, - "high": 4360, - "low": 13, - "open": 4360, - "volume": 166049 - }, - "id": 2609029, - "non_hive": { - "close": 964, - "high": 1522, - "low": 4, - "open": 1522, - "volume": 55255 - }, - "open": "2020-05-18T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 251, - "high": 245, - "low": 31, - "open": 4132, - "volume": 924963 - }, - "id": 2609080, - "non_hive": { - "close": 83, - "high": 83, - "low": 10, - "open": 1364, - "volume": 306190 - }, - "open": "2020-05-18T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5738, - "high": 163, - "low": 101, - "open": 9700, - "volume": 1255991 - }, - "id": 2609114, - "non_hive": { - "close": 1928, - "high": 55, - "low": 33, - "open": 3270, - "volume": 412652 - }, - "open": "2020-05-18T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 1619, - "low": 13, - "open": 142505, - "volume": 259334 - }, - "id": 2609146, - "non_hive": { - "close": 4, - "high": 536, - "low": 4, - "open": 47159, - "volume": 85815 - }, - "open": "2020-05-18T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 208, - "high": 2133, - "low": 208, - "open": 2133, - "volume": 124993 - }, - "id": 2609160, - "non_hive": { - "close": 68, - "high": 706, - "low": 68, - "open": 706, - "volume": 41360 - }, - "open": "2020-05-18T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 96083, - "high": 1237, - "low": 96083, - "open": 4942, - "volume": 305290 - }, - "id": 2609181, - "non_hive": { - "close": 31534, - "high": 409, - "low": 31534, - "open": 1633, - "volume": 100633 - }, - "open": "2020-05-18T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 463, - "high": 65, - "low": 13, - "open": 1083, - "volume": 145984 - }, - "id": 2609206, - "non_hive": { - "close": 156, - "high": 22, - "low": 4, - "open": 358, - "volume": 48319 - }, - "open": "2020-05-18T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11582, - "high": 346, - "low": 14968, - "open": 14968, - "volume": 172826 - }, - "id": 2609244, - "non_hive": { - "close": 3880, - "high": 117, - "low": 5000, - "open": 5000, - "volume": 57897 - }, - "open": "2020-05-18T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13281, - "high": 4709, - "low": 150, - "open": 2540, - "volume": 845281 - }, - "id": 2609271, - "non_hive": { - "close": 4484, - "high": 1590, - "low": 50, - "open": 851, - "volume": 283692 - }, - "open": "2020-05-18T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17470, - "high": 106, - "low": 24000, - "open": 7929, - "volume": 152813 - }, - "id": 2609309, - "non_hive": { - "close": 5870, - "high": 36, - "low": 8064, - "open": 2677, - "volume": 51429 - }, - "open": "2020-05-18T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2765, - "high": 505, - "low": 2765, - "open": 8717, - "volume": 118212 - }, - "id": 2609340, - "non_hive": { - "close": 929, - "high": 170, - "low": 929, - "open": 2929, - "volume": 39720 - }, - "open": "2020-05-18T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 279, - "high": 178, - "low": 266247, - "open": 10661, - "volume": 761621 - }, - "id": 2609367, - "non_hive": { - "close": 94, - "high": 60, - "low": 86172, - "open": 3581, - "volume": 250038 - }, - "open": "2020-05-18T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 178, - "high": 139, - "low": 12, - "open": 916, - "volume": 212153 - }, - "id": 2609401, - "non_hive": { - "close": 60, - "high": 47, - "low": 3, - "open": 308, - "volume": 71251 - }, - "open": "2020-05-18T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 822, - "high": 2022, - "low": 5, - "open": 4430, - "volume": 357105 - }, - "id": 2609446, - "non_hive": { - "close": 276, - "high": 679, - "low": 1, - "open": 1487, - "volume": 119865 - }, - "open": "2020-05-18T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9760, - "high": 1733, - "low": 3568, - "open": 1733, - "volume": 240825 - }, - "id": 2609491, - "non_hive": { - "close": 3276, - "high": 582, - "low": 1188, - "open": 582, - "volume": 80843 - }, - "open": "2020-05-18T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1183, - "high": 631, - "low": 292667, - "open": 72003, - "volume": 690775 - }, - "id": 2609515, - "non_hive": { - "close": 397, - "high": 212, - "low": 96103, - "open": 24168, - "volume": 229645 - }, - "open": "2020-05-18T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 76380, - "high": 2, - "low": 22, - "open": 12506, - "volume": 390887 - }, - "id": 2609558, - "non_hive": { - "close": 25618, - "high": 1, - "low": 7, - "open": 4196, - "volume": 131081 - }, - "open": "2020-05-18T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1136, - "high": 700, - "low": 42, - "open": 12499, - "volume": 276551 - }, - "id": 2609588, - "non_hive": { - "close": 381, - "high": 235, - "low": 14, - "open": 4192, - "volume": 92756 - }, - "open": "2020-05-18T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5989, - "high": 20, - "low": 12, - "open": 5964, - "volume": 85317 - }, - "id": 2609623, - "non_hive": { - "close": 2009, - "high": 7, - "low": 4, - "open": 2000, - "volume": 28614 - }, - "open": "2020-05-18T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6467, - "high": 5882, - "low": 274202, - "open": 5882, - "volume": 1314380 - }, - "id": 2609655, - "non_hive": { - "close": 2167, - "high": 1973, - "low": 86221, - "open": 1973, - "volume": 418713 - }, - "open": "2020-05-19T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1433, - "high": 235, - "low": 1433, - "open": 235, - "volume": 157114 - }, - "id": 2609687, - "non_hive": { - "close": 471, - "high": 79, - "low": 471, - "open": 79, - "volume": 52220 - }, - "open": "2020-05-19T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4090, - "high": 65, - "low": 348, - "open": 83498, - "volume": 227203 - }, - "id": 2609714, - "non_hive": { - "close": 1371, - "high": 22, - "low": 114, - "open": 27434, - "volume": 74758 - }, - "open": "2020-05-19T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 246858, - "high": 2066, - "low": 246858, - "open": 2066, - "volume": 688826 - }, - "id": 2609737, - "non_hive": { - "close": 78253, - "high": 692, - "low": 78253, - "open": 692, - "volume": 220023 - }, - "open": "2020-05-19T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1048, - "high": 266, - "low": 136508, - "open": 266, - "volume": 650492 - }, - "id": 2609760, - "non_hive": { - "close": 343, - "high": 89, - "low": 43317, - "open": 89, - "volume": 211724 - }, - "open": "2020-05-19T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33374, - "high": 3, - "low": 12, - "open": 9000, - "volume": 1487878 - }, - "id": 2609797, - "non_hive": { - "close": 10846, - "high": 1, - "low": 3, - "open": 2942, - "volume": 485605 - }, - "open": "2020-05-19T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3991, - "high": 68, - "low": 10, - "open": 2081, - "volume": 38931 - }, - "id": 2609838, - "non_hive": { - "close": 1333, - "high": 23, - "low": 3, - "open": 681, - "volume": 12981 - }, - "open": "2020-05-19T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 81423, - "high": 4997, - "low": 100, - "open": 542, - "volume": 158862 - }, - "id": 2609866, - "non_hive": { - "close": 27190, - "high": 1669, - "low": 33, - "open": 181, - "volume": 53022 - }, - "open": "2020-05-19T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1348363, - "high": 15048, - "low": 13, - "open": 7725, - "volume": 3315434 - }, - "id": 2609896, - "non_hive": { - "close": 422037, - "high": 5018, - "low": 4, - "open": 2576, - "volume": 1041962 - }, - "open": "2020-05-19T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19700, - "high": 64736, - "low": 77239, - "open": 511, - "volume": 13856361 - }, - "id": 2609922, - "non_hive": { - "close": 7678, - "high": 26541, - "low": 19947, - "open": 170, - "volume": 4571986 - }, - "open": "2020-05-19T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 75323, - "high": 80398, - "low": 600000, - "open": 19317, - "volume": 7787504 - }, - "id": 2610025, - "non_hive": { - "close": 31507, - "high": 34734, - "low": 205680, - "open": 7524, - "volume": 3016864 - }, - "open": "2020-05-19T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 500000, - "high": 1153, - "low": 600000, - "open": 182, - "volume": 14136233 - }, - "id": 2610098, - "non_hive": { - "close": 190001, - "high": 519, - "low": 199955, - "open": 76, - "volume": 5334174 - }, - "open": "2020-05-19T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19149, - "high": 18985, - "low": 508, - "open": 310524, - "volume": 19725028 - }, - "id": 2610198, - "non_hive": { - "close": 8023, - "high": 8031, - "low": 193, - "open": 119552, - "volume": 7802749 - }, - "open": "2020-05-19T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 165142, - "high": 484, - "low": 600000, - "open": 18728, - "volume": 8151310 - }, - "id": 2610306, - "non_hive": { - "close": 67896, - "high": 211, - "low": 240360, - "open": 7861, - "volume": 3390114 - }, - "open": "2020-05-19T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 30174, - "low": 117, - "open": 600000, - "volume": 4631281 - }, - "id": 2610375, - "non_hive": { - "close": 4149, - "high": 13135, - "low": 48, - "open": 247445, - "volume": 1933731 - }, - "open": "2020-05-19T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2577, - "high": 439, - "low": 3, - "open": 300000, - "volume": 7240828 - }, - "id": 2610451, - "non_hive": { - "close": 1103, - "high": 189, - "low": 1, - "open": 124809, - "volume": 2969540 - }, - "open": "2020-05-19T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38826, - "high": 2, - "low": 571428, - "open": 456, - "volume": 11379771 - }, - "id": 2610519, - "non_hive": { - "close": 13974, - "high": 1, - "low": 194268, - "open": 182, - "volume": 4362019 - }, - "open": "2020-05-19T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 12544, - "low": 5, - "open": 5038, - "volume": 4908346 - }, - "id": 2610594, - "non_hive": { - "close": 1, - "high": 4999, - "low": 1, - "open": 1811, - "volume": 1730475 - }, - "open": "2020-05-19T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 101, - "high": 2, - "low": 101, - "open": 6871, - "volume": 4061769 - }, - "id": 2610655, - "non_hive": { - "close": 33, - "high": 1, - "low": 33, - "open": 2629, - "volume": 1446014 - }, - "open": "2020-05-19T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1120, - "high": 2, - "low": 1161, - "open": 6001, - "volume": 4797153 - }, - "id": 2610699, - "non_hive": { - "close": 435, - "high": 1, - "low": 388, - "open": 2382, - "volume": 1676537 - }, - "open": "2020-05-19T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27278, - "high": 3731, - "low": 144, - "open": 11666, - "volume": 5107940 - }, - "id": 2610777, - "non_hive": { - "close": 10000, - "high": 1451, - "low": 51, - "open": 4269, - "volume": 1839329 - }, - "open": "2020-05-19T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 599552, - "high": 853, - "low": 182765, - "open": 1054, - "volume": 4803336 - }, - "id": 2610836, - "non_hive": { - "close": 213266, - "high": 316, - "low": 64980, - "open": 386, - "volume": 1719892 - }, - "open": "2020-05-19T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1896, - "high": 282, - "low": 15, - "open": 600000, - "volume": 6457002 - }, - "id": 2610876, - "non_hive": { - "close": 729, - "high": 110, - "low": 5, - "open": 213389, - "volume": 2300959 - }, - "open": "2020-05-19T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20364, - "high": 74, - "low": 12, - "open": 8500, - "volume": 4027217 - }, - "id": 2610929, - "non_hive": { - "close": 7717, - "high": 29, - "low": 4, - "open": 3023, - "volume": 1445459 - }, - "open": "2020-05-19T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299998, - "high": 512, - "low": 54, - "open": 300011, - "volume": 5244498 - }, - "id": 2610993, - "non_hive": { - "close": 109229, - "high": 205, - "low": 19, - "open": 106690, - "volume": 1910549 - }, - "open": "2020-05-20T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 595248, - "high": 1380, - "low": 206, - "open": 300001, - "volume": 3951054 - }, - "id": 2611034, - "non_hive": { - "close": 211793, - "high": 552, - "low": 73, - "open": 109171, - "volume": 1414901 - }, - "open": "2020-05-20T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 465, - "high": 465, - "low": 3, - "open": 1320, - "volume": 4162473 - }, - "id": 2611072, - "non_hive": { - "close": 186, - "high": 186, - "low": 1, - "open": 470, - "volume": 1503416 - }, - "open": "2020-05-20T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 597956, - "high": 1353, - "low": 528466, - "open": 12510, - "volume": 5287510 - }, - "id": 2611109, - "non_hive": { - "close": 214253, - "high": 544, - "low": 188424, - "open": 4999, - "volume": 1980579 - }, - "open": "2020-05-20T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1796, - "high": 2, - "low": 21158, - "open": 600000, - "volume": 5929119 - }, - "id": 2611148, - "non_hive": { - "close": 711, - "high": 1, - "low": 7582, - "open": 216431, - "volume": 2176625 - }, - "open": "2020-05-20T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17794, - "high": 159, - "low": 65441, - "open": 3142, - "volume": 6032217 - }, - "id": 2611217, - "non_hive": { - "close": 7258, - "high": 65, - "low": 23631, - "open": 1244, - "volume": 2317736 - }, - "open": "2020-05-20T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1536, - "high": 17436, - "low": 30, - "open": 17436, - "volume": 4802987 - }, - "id": 2611291, - "non_hive": { - "close": 624, - "high": 7112, - "low": 11, - "open": 7112, - "volume": 1811185 - }, - "open": "2020-05-20T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 354, - "high": 1804, - "low": 49984, - "open": 52269, - "volume": 5632213 - }, - "id": 2611349, - "non_hive": { - "close": 139, - "high": 734, - "low": 18049, - "open": 19610, - "volume": 2148087 - }, - "open": "2020-05-20T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1023974, - "high": 51037, - "low": 14, - "open": 485, - "volume": 6225220 - }, - "id": 2611415, - "non_hive": { - "close": 409589, - "high": 20415, - "low": 5, - "open": 190, - "volume": 2397915 - }, - "open": "2020-05-20T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 298792, - "high": 298854, - "low": 595930, - "open": 240964, - "volume": 4912492 - }, - "id": 2611472, - "non_hive": { - "close": 116688, - "high": 121901, - "low": 230984, - "open": 98037, - "volume": 1924963 - }, - "open": "2020-05-20T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 289, - "high": 485, - "low": 189615, - "open": 2417, - "volume": 5094269 - }, - "id": 2611511, - "non_hive": { - "close": 115, - "high": 197, - "low": 62771, - "open": 944, - "volume": 1846925 - }, - "open": "2020-05-20T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32475, - "high": 472, - "low": 30, - "open": 300000, - "volume": 2793504 - }, - "id": 2611577, - "non_hive": { - "close": 12662, - "high": 187, - "low": 10, - "open": 100320, - "volume": 947419 - }, - "open": "2020-05-20T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 292406, - "high": 25, - "low": 28, - "open": 600000, - "volume": 3663112 - }, - "id": 2611620, - "non_hive": { - "close": 106631, - "high": 10, - "low": 10, - "open": 227040, - "volume": 1360720 - }, - "open": "2020-05-20T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8539, - "high": 2, - "low": 228189, - "open": 2096, - "volume": 7864149 - }, - "id": 2611659, - "non_hive": { - "close": 3422, - "high": 1, - "low": 84434, - "open": 814, - "volume": 3034882 - }, - "open": "2020-05-20T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 88, - "high": 2, - "low": 27, - "open": 600000, - "volume": 9059669 - }, - "id": 2611751, - "non_hive": { - "close": 34, - "high": 1, - "low": 10, - "open": 234302, - "volume": 3511191 - }, - "open": "2020-05-20T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 221675, - "high": 89, - "low": 300000, - "open": 100000, - "volume": 4263262 - }, - "id": 2611820, - "non_hive": { - "close": 88837, - "high": 36, - "low": 114936, - "open": 39105, - "volume": 1659781 - }, - "open": "2020-05-20T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4482, - "high": 9, - "low": 30451, - "open": 298532, - "volume": 6238537 - }, - "id": 2611862, - "non_hive": { - "close": 1797, - "high": 4, - "low": 11730, - "open": 119671, - "volume": 2440137 - }, - "open": "2020-05-20T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30549, - "high": 254, - "low": 94366, - "open": 7500, - "volume": 4086856 - }, - "id": 2611916, - "non_hive": { - "close": 11518, - "high": 103, - "low": 34240, - "open": 3007, - "volume": 1548348 - }, - "open": "2020-05-20T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5040, - "high": 2, - "low": 3, - "open": 13260, - "volume": 3482503 - }, - "id": 2611974, - "non_hive": { - "close": 1990, - "high": 1, - "low": 1, - "open": 4999, - "volume": 1300870 - }, - "open": "2020-05-20T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34614, - "high": 1851, - "low": 212, - "open": 3565, - "volume": 3111209 - }, - "id": 2612032, - "non_hive": { - "close": 13499, - "high": 731, - "low": 80, - "open": 1350, - "volume": 1188325 - }, - "open": "2020-05-20T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 979, - "high": 2, - "low": 5, - "open": 1962, - "volume": 596699 - }, - "id": 2612081, - "non_hive": { - "close": 384, - "high": 1, - "low": 1, - "open": 765, - "volume": 232685 - }, - "open": "2020-05-20T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26473, - "high": 2, - "low": 67, - "open": 13257, - "volume": 301565 - }, - "id": 2612130, - "non_hive": { - "close": 10351, - "high": 1, - "low": 25, - "open": 5197, - "volume": 118099 - }, - "open": "2020-05-20T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 2884, - "low": 42, - "open": 734, - "volume": 1559670 - }, - "id": 2612155, - "non_hive": { - "close": 5, - "high": 1226, - "low": 16, - "open": 286, - "volume": 630509 - }, - "open": "2020-05-20T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 207690, - "high": 548, - "low": 13, - "open": 548, - "volume": 684832 - }, - "id": 2612192, - "non_hive": { - "close": 81782, - "high": 233, - "low": 5, - "open": 233, - "volume": 270011 - }, - "open": "2020-05-20T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 571, - "high": 571, - "low": 54, - "open": 1378, - "volume": 112719 - }, - "id": 2612217, - "non_hive": { - "close": 242, - "high": 242, - "low": 21, - "open": 543, - "volume": 44406 - }, - "open": "2020-05-21T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7481, - "high": 8495, - "low": 13010, - "open": 1402, - "volume": 995968 - }, - "id": 2612250, - "non_hive": { - "close": 3161, - "high": 3599, - "low": 5018, - "open": 552, - "volume": 392403 - }, - "open": "2020-05-21T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4094, - "high": 9027, - "low": 3, - "open": 3, - "volume": 173822 - }, - "id": 2612280, - "non_hive": { - "close": 1678, - "high": 3805, - "low": 1, - "open": 1, - "volume": 69969 - }, - "open": "2020-05-21T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1232, - "high": 1232, - "low": 3124, - "open": 19809, - "volume": 251055 - }, - "id": 2612305, - "non_hive": { - "close": 505, - "high": 505, - "low": 1197, - "open": 7800, - "volume": 97697 - }, - "open": "2020-05-21T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24841, - "high": 173, - "low": 24841, - "open": 84, - "volume": 988022 - }, - "id": 2612329, - "non_hive": { - "close": 8880, - "high": 71, - "low": 8880, - "open": 33, - "volume": 369054 - }, - "open": "2020-05-21T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20826, - "high": 101, - "low": 85, - "open": 2795, - "volume": 1492914 - }, - "id": 2612359, - "non_hive": { - "close": 8330, - "high": 41, - "low": 30, - "open": 1130, - "volume": 559470 - }, - "open": "2020-05-21T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9082, - "high": 9082, - "low": 32, - "open": 20514, - "volume": 3461445 - }, - "id": 2612389, - "non_hive": { - "close": 3717, - "high": 3717, - "low": 12, - "open": 8201, - "volume": 1343571 - }, - "open": "2020-05-21T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1736, - "high": 1599, - "low": 103, - "open": 12235, - "volume": 1729606 - }, - "id": 2612461, - "non_hive": { - "close": 711, - "high": 655, - "low": 39, - "open": 4999, - "volume": 673252 - }, - "open": "2020-05-21T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18756, - "high": 9267, - "low": 14, - "open": 1320, - "volume": 2852485 - }, - "id": 2612507, - "non_hive": { - "close": 7502, - "high": 3793, - "low": 5, - "open": 504, - "volume": 1104835 - }, - "open": "2020-05-21T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42, - "high": 9542, - "low": 40, - "open": 18384, - "volume": 270069 - }, - "id": 2612561, - "non_hive": { - "close": 16, - "high": 3835, - "low": 15, - "open": 7351, - "volume": 106182 - }, - "open": "2020-05-21T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3202, - "high": 3202, - "low": 177250, - "open": 607, - "volume": 1544473 - }, - "id": 2612602, - "non_hive": { - "close": 1287, - "high": 1287, - "low": 69127, - "open": 239, - "volume": 611754 - }, - "open": "2020-05-21T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5388, - "high": 1701, - "low": 13, - "open": 600000, - "volume": 767461 - }, - "id": 2612621, - "non_hive": { - "close": 2153, - "high": 684, - "low": 5, - "open": 234236, - "volume": 300568 - }, - "open": "2020-05-21T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7102, - "high": 1639, - "low": 287543, - "open": 498, - "volume": 746190 - }, - "id": 2612659, - "non_hive": { - "close": 2853, - "high": 659, - "low": 114169, - "open": 200, - "volume": 296756 - }, - "open": "2020-05-21T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1342, - "high": 276, - "low": 289, - "open": 4952, - "volume": 594624 - }, - "id": 2612701, - "non_hive": { - "close": 534, - "high": 111, - "low": 111, - "open": 1989, - "volume": 234055 - }, - "open": "2020-05-21T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 86909, - "high": 9243, - "low": 84120, - "open": 9243, - "volume": 207686 - }, - "id": 2612747, - "non_hive": { - "close": 33675, - "high": 3677, - "low": 32594, - "open": 3677, - "volume": 80661 - }, - "open": "2020-05-21T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 302991, - "high": 3962, - "low": 50, - "open": 309920, - "volume": 1738683 - }, - "id": 2612774, - "non_hive": { - "close": 118840, - "high": 1571, - "low": 19, - "open": 120085, - "volume": 678337 - }, - "open": "2020-05-21T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3051, - "high": 27, - "low": 398, - "open": 302991, - "volume": 2708142 - }, - "id": 2612830, - "non_hive": { - "close": 1213, - "high": 11, - "low": 154, - "open": 118840, - "volume": 1063148 - }, - "open": "2020-05-21T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 628, - "low": 6, - "open": 1635, - "volume": 949473 - }, - "id": 2612912, - "non_hive": { - "close": 4, - "high": 250, - "low": 2, - "open": 650, - "volume": 366139 - }, - "open": "2020-05-21T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17421, - "high": 3402, - "low": 101, - "open": 136716, - "volume": 1798198 - }, - "id": 2612952, - "non_hive": { - "close": 6533, - "high": 1319, - "low": 35, - "open": 52113, - "volume": 640415 - }, - "open": "2020-05-21T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11578, - "high": 11578, - "low": 575857, - "open": 1799, - "volume": 3240646 - }, - "id": 2612993, - "non_hive": { - "close": 4543, - "high": 4543, - "low": 201551, - "open": 673, - "volume": 1167089 - }, - "open": "2020-05-21T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45495, - "high": 2, - "low": 34, - "open": 2, - "volume": 2921859 - }, - "id": 2613040, - "non_hive": { - "close": 17173, - "high": 1, - "low": 12, - "open": 1, - "volume": 1088930 - }, - "open": "2020-05-21T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1689, - "high": 230, - "low": 61815, - "open": 256, - "volume": 735831 - }, - "id": 2613099, - "non_hive": { - "close": 636, - "high": 90, - "low": 21659, - "open": 97, - "volume": 265878 - }, - "open": "2020-05-21T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9190, - "high": 216987, - "low": 14, - "open": 334, - "volume": 448142 - }, - "id": 2613126, - "non_hive": { - "close": 3638, - "high": 86171, - "low": 5, - "open": 125, - "volume": 175823 - }, - "open": "2020-05-21T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1223, - "high": 1873, - "low": 5, - "open": 491, - "volume": 654636 - }, - "id": 2613164, - "non_hive": { - "close": 483, - "high": 744, - "low": 1, - "open": 194, - "volume": 234376 - }, - "open": "2020-05-21T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20947, - "high": 93, - "low": 54, - "open": 5117, - "volume": 39429 - }, - "id": 2613195, - "non_hive": { - "close": 8264, - "high": 37, - "low": 21, - "open": 2019, - "volume": 15556 - }, - "open": "2020-05-22T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127194, - "high": 476, - "low": 99116, - "open": 4071, - "volume": 1332444 - }, - "id": 2613213, - "non_hive": { - "close": 46312, - "high": 189, - "low": 36000, - "open": 1606, - "volume": 490041 - }, - "open": "2020-05-22T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16377, - "high": 549, - "low": 3, - "open": 549, - "volume": 52483 - }, - "id": 2613248, - "non_hive": { - "close": 5963, - "high": 216, - "low": 1, - "open": 216, - "volume": 19199 - }, - "open": "2020-05-22T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42, - "high": 11578, - "low": 42, - "open": 11578, - "volume": 75095 - }, - "id": 2613269, - "non_hive": { - "close": 15, - "high": 4548, - "low": 15, - "open": 4548, - "volume": 28716 - }, - "open": "2020-05-22T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1895, - "high": 421, - "low": 22607, - "open": 1898, - "volume": 620674 - }, - "id": 2613297, - "non_hive": { - "close": 692, - "high": 154, - "low": 7811, - "open": 693, - "volume": 218023 - }, - "open": "2020-05-22T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3144, - "high": 345, - "low": 12, - "open": 345, - "volume": 69538 - }, - "id": 2613313, - "non_hive": { - "close": 1084, - "high": 126, - "low": 4, - "open": 126, - "volume": 25318 - }, - "open": "2020-05-22T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11131, - "high": 156, - "low": 29, - "open": 12678, - "volume": 328707 - }, - "id": 2613334, - "non_hive": { - "close": 4059, - "high": 57, - "low": 10, - "open": 4626, - "volume": 119961 - }, - "open": "2020-05-22T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 105, - "high": 80, - "low": 29, - "open": 919, - "volume": 136140 - }, - "id": 2613369, - "non_hive": { - "close": 38, - "high": 29, - "low": 10, - "open": 332, - "volume": 48556 - }, - "open": "2020-05-22T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 310024, - "high": 13, - "low": 392, - "open": 9698, - "volume": 2173395 - }, - "id": 2613415, - "non_hive": { - "close": 110658, - "high": 5, - "low": 139, - "open": 3490, - "volume": 779922 - }, - "open": "2020-05-22T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 75, - "low": 144, - "open": 28015, - "volume": 3715753 - }, - "id": 2613457, - "non_hive": { - "close": 15, - "high": 27, - "low": 49, - "open": 10000, - "volume": 1284755 - }, - "open": "2020-05-22T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 422, - "high": 449, - "low": 12696, - "open": 698, - "volume": 16121 - }, - "id": 2613503, - "non_hive": { - "close": 146, - "high": 157, - "low": 4333, - "open": 244, - "volume": 5525 - }, - "open": "2020-05-22T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3361, - "high": 103, - "low": 30, - "open": 1361, - "volume": 238762 - }, - "id": 2613519, - "non_hive": { - "close": 1163, - "high": 36, - "low": 10, - "open": 471, - "volume": 82659 - }, - "open": "2020-05-22T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12117, - "high": 5433, - "low": 22, - "open": 5577, - "volume": 1825731 - }, - "id": 2613567, - "non_hive": { - "close": 4232, - "high": 1898, - "low": 7, - "open": 1924, - "volume": 628852 - }, - "open": "2020-05-22T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6374, - "high": 2, - "low": 34, - "open": 14616, - "volume": 1243934 - }, - "id": 2613600, - "non_hive": { - "close": 2225, - "high": 1, - "low": 11, - "open": 5102, - "volume": 430778 - }, - "open": "2020-05-22T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10216, - "high": 28, - "low": 93, - "open": 2586, - "volume": 1143192 - }, - "id": 2613643, - "non_hive": { - "close": 3626, - "high": 10, - "low": 32, - "open": 903, - "volume": 400124 - }, - "open": "2020-05-22T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43, - "high": 2, - "low": 43, - "open": 293, - "volume": 1359541 - }, - "id": 2613683, - "non_hive": { - "close": 15, - "high": 1, - "low": 15, - "open": 104, - "volume": 478849 - }, - "open": "2020-05-22T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30434, - "high": 57, - "low": 3, - "open": 13004, - "volume": 1314956 - }, - "id": 2613733, - "non_hive": { - "close": 10804, - "high": 21, - "low": 1, - "open": 4736, - "volume": 465222 - }, - "open": "2020-05-22T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 8238, - "low": 12, - "open": 98, - "volume": 669829 - }, - "id": 2613784, - "non_hive": { - "close": 4, - "high": 2966, - "low": 4, - "open": 35, - "volume": 233437 - }, - "open": "2020-05-22T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 107736, - "high": 17295, - "low": 113, - "open": 10000, - "volume": 308241 - }, - "id": 2613811, - "non_hive": { - "close": 37949, - "high": 6209, - "low": 38, - "open": 3590, - "volume": 107175 - }, - "open": "2020-05-22T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15817, - "high": 2, - "low": 14612, - "open": 77306, - "volume": 5785531 - }, - "id": 2613842, - "non_hive": { - "close": 5284, - "high": 1, - "low": 4881, - "open": 27231, - "volume": 1963993 - }, - "open": "2020-05-22T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11070, - "high": 2, - "low": 16785, - "open": 16133, - "volume": 980804 - }, - "id": 2613937, - "non_hive": { - "close": 3919, - "high": 1, - "low": 5607, - "open": 5390, - "volume": 341096 - }, - "open": "2020-05-22T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43, - "high": 2, - "low": 43, - "open": 723, - "volume": 2148634 - }, - "id": 2613989, - "non_hive": { - "close": 14, - "high": 1, - "low": 14, - "open": 256, - "volume": 721647 - }, - "open": "2020-05-22T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2627, - "high": 223, - "low": 14, - "open": 6016, - "volume": 3858813 - }, - "id": 2614051, - "non_hive": { - "close": 894, - "high": 79, - "low": 4, - "open": 1986, - "volume": 1252657 - }, - "open": "2020-05-22T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 3204, - "low": 14, - "open": 40, - "volume": 3863115 - }, - "id": 2614108, - "non_hive": { - "close": 4, - "high": 1087, - "low": 4, - "open": 12, - "volume": 1168995 - }, - "open": "2020-05-22T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 4000, - "low": 586181, - "open": 3026, - "volume": 5496162 - }, - "id": 2614160, - "non_hive": { - "close": 184500, - "high": 1319, - "low": 176036, - "open": 997, - "volume": 1716392 - }, - "open": "2020-05-23T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 332190, - "high": 215726, - "low": 100, - "open": 100, - "volume": 5957456 - }, - "id": 2614212, - "non_hive": { - "close": 106754, - "high": 71621, - "low": 31, - "open": 31, - "volume": 1930231 - }, - "open": "2020-05-23T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 238, - "low": 49, - "open": 12537, - "volume": 3642756 - }, - "id": 2614252, - "non_hive": { - "close": 193650, - "high": 82, - "low": 15, - "open": 4161, - "volume": 1175849 - }, - "open": "2020-05-23T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 81, - "low": 44, - "open": 600000, - "volume": 3208310 - }, - "id": 2614282, - "non_hive": { - "close": 14, - "high": 28, - "low": 14, - "open": 193693, - "volume": 1038322 - }, - "open": "2020-05-23T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 584068, - "high": 609, - "low": 250607, - "open": 600000, - "volume": 5820084 - }, - "id": 2614317, - "non_hive": { - "close": 175408, - "high": 210, - "low": 75259, - "open": 193511, - "volume": 1846218 - }, - "open": "2020-05-23T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6093, - "high": 787, - "low": 14357, - "open": 787, - "volume": 6080821 - }, - "id": 2614350, - "non_hive": { - "close": 1910, - "high": 252, - "low": 4311, - "open": 252, - "volume": 1862375 - }, - "open": "2020-05-23T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14986, - "high": 162, - "low": 130, - "open": 660, - "volume": 4011664 - }, - "id": 2614408, - "non_hive": { - "close": 4688, - "high": 51, - "low": 39, - "open": 207, - "volume": 1208908 - }, - "open": "2020-05-23T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 319, - "high": 319, - "low": 594967, - "open": 15985, - "volume": 5795716 - }, - "id": 2614464, - "non_hive": { - "close": 109, - "high": 109, - "low": 178732, - "open": 5000, - "volume": 1781387 - }, - "open": "2020-05-23T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 20548, - "low": 13, - "open": 75895, - "volume": 4765954 - }, - "id": 2614516, - "non_hive": { - "close": 4, - "high": 7006, - "low": 4, - "open": 23907, - "volume": 1513349 - }, - "open": "2020-05-23T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9702, - "high": 23743, - "low": 42, - "open": 23743, - "volume": 4246520 - }, - "id": 2614571, - "non_hive": { - "close": 3051, - "high": 7715, - "low": 13, - "open": 7715, - "volume": 1336529 - }, - "open": "2020-05-23T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 561519, - "high": 257, - "low": 16801, - "open": 490257, - "volume": 6153807 - }, - "id": 2614613, - "non_hive": { - "close": 169804, - "high": 85, - "low": 5078, - "open": 154186, - "volume": 1898220 - }, - "open": "2020-05-23T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 571, - "high": 1538, - "low": 9, - "open": 1538, - "volume": 6864305 - }, - "id": 2614652, - "non_hive": { - "close": 177, - "high": 490, - "low": 2, - "open": 490, - "volume": 2090193 - }, - "open": "2020-05-23T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30538, - "high": 12, - "low": 113, - "open": 19429, - "volume": 4046372 - }, - "id": 2614710, - "non_hive": { - "close": 9434, - "high": 4, - "low": 33, - "open": 6015, - "volume": 1221409 - }, - "open": "2020-05-23T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 9, - "low": 8, - "open": 8045, - "volume": 4024782 - }, - "id": 2614761, - "non_hive": { - "close": 180540, - "high": 3, - "low": 2, - "open": 2485, - "volume": 1214761 - }, - "open": "2020-05-23T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 566614, - "high": 3, - "low": 125, - "open": 10000, - "volume": 5167815 - }, - "id": 2614807, - "non_hive": { - "close": 173383, - "high": 1, - "low": 38, - "open": 3079, - "volume": 1586340 - }, - "open": "2020-05-23T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43, - "high": 3, - "low": 1000, - "open": 10000, - "volume": 4650007 - }, - "id": 2614882, - "non_hive": { - "close": 13, - "high": 1, - "low": 302, - "open": 3091, - "volume": 1413186 - }, - "open": "2020-05-23T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4715, - "high": 152417, - "low": 9, - "open": 3596, - "volume": 4379715 - }, - "id": 2614957, - "non_hive": { - "close": 1460, - "high": 52148, - "low": 2, - "open": 1112, - "volume": 1348123 - }, - "open": "2020-05-23T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 89990, - "high": 805, - "low": 89, - "open": 6617, - "volume": 5036496 - }, - "id": 2615000, - "non_hive": { - "close": 27034, - "high": 249, - "low": 26, - "open": 2000, - "volume": 1518310 - }, - "open": "2020-05-23T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 999412, - "high": 102, - "low": 15, - "open": 11254, - "volume": 11095222 - }, - "id": 2615054, - "non_hive": { - "close": 299824, - "high": 31, - "low": 4, - "open": 3381, - "volume": 3330157 - }, - "open": "2020-05-23T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 86083, - "high": 3, - "low": 6, - "open": 5231, - "volume": 2801718 - }, - "id": 2615109, - "non_hive": { - "close": 25911, - "high": 1, - "low": 1, - "open": 1601, - "volume": 830316 - }, - "open": "2020-05-23T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9884, - "high": 4651, - "low": 185, - "open": 880, - "volume": 3600569 - }, - "id": 2615170, - "non_hive": { - "close": 2999, - "high": 1413, - "low": 54, - "open": 265, - "volume": 1067908 - }, - "open": "2020-05-23T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 3, - "low": 44, - "open": 35880, - "volume": 3367033 - }, - "id": 2615218, - "non_hive": { - "close": 13, - "high": 1, - "low": 13, - "open": 10764, - "volume": 1001579 - }, - "open": "2020-05-23T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11630, - "high": 235, - "low": 14, - "open": 73265, - "volume": 4854469 - }, - "id": 2615263, - "non_hive": { - "close": 3555, - "high": 72, - "low": 4, - "open": 22222, - "volume": 1462680 - }, - "open": "2020-05-23T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1706, - "high": 1380, - "low": 11, - "open": 19986, - "volume": 1289053 - }, - "id": 2615322, - "non_hive": { - "close": 518, - "high": 422, - "low": 3, - "open": 5996, - "volume": 385298 - }, - "open": "2020-05-23T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2817, - "high": 16, - "low": 107, - "open": 6286, - "volume": 663105 - }, - "id": 2615353, - "non_hive": { - "close": 854, - "high": 5, - "low": 31, - "open": 1907, - "volume": 199130 - }, - "open": "2020-05-24T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10703, - "high": 683, - "low": 16, - "open": 9481, - "volume": 100164 - }, - "id": 2615396, - "non_hive": { - "close": 3258, - "high": 208, - "low": 4, - "open": 2873, - "volume": 30392 - }, - "open": "2020-05-24T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 851, - "high": 851, - "low": 595486, - "open": 318240, - "volume": 6557695 - }, - "id": 2615424, - "non_hive": { - "close": 261, - "high": 261, - "low": 174170, - "open": 96871, - "volume": 1979196 - }, - "open": "2020-05-24T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 307211, - "high": 363, - "low": 11, - "open": 363, - "volume": 4353489 - }, - "id": 2615481, - "non_hive": { - "close": 93074, - "high": 111, - "low": 3, - "open": 111, - "volume": 1293171 - }, - "open": "2020-05-24T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9, - "high": 9, - "low": 233, - "open": 6988, - "volume": 5657583 - }, - "id": 2615521, - "non_hive": { - "close": 3, - "high": 3, - "low": 68, - "open": 2117, - "volume": 1695622 - }, - "open": "2020-05-24T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8140, - "high": 32668, - "low": 25, - "open": 10108, - "volume": 5243521 - }, - "id": 2615562, - "non_hive": { - "close": 2478, - "high": 10133, - "low": 7, - "open": 3101, - "volume": 1589255 - }, - "open": "2020-05-24T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1317, - "high": 4556, - "low": 52, - "open": 6576, - "volume": 5087363 - }, - "id": 2615610, - "non_hive": { - "close": 397, - "high": 1390, - "low": 15, - "open": 1999, - "volume": 1524060 - }, - "open": "2020-05-24T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 563309, - "high": 19516, - "low": 102, - "open": 6872, - "volume": 3744833 - }, - "id": 2615669, - "non_hive": { - "close": 168992, - "high": 5966, - "low": 30, - "open": 2070, - "volume": 1120356 - }, - "open": "2020-05-24T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3178, - "high": 7318, - "low": 43, - "open": 7112, - "volume": 4380383 - }, - "id": 2615713, - "non_hive": { - "close": 965, - "high": 2228, - "low": 12, - "open": 2161, - "volume": 1311044 - }, - "open": "2020-05-24T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 63341, - "high": 740, - "low": 14, - "open": 14, - "volume": 3743139 - }, - "id": 2615770, - "non_hive": { - "close": 19016, - "high": 225, - "low": 4, - "open": 4, - "volume": 1124419 - }, - "open": "2020-05-24T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 217006, - "high": 1000, - "low": 44, - "open": 44, - "volume": 3643298 - }, - "id": 2615815, - "non_hive": { - "close": 65113, - "high": 304, - "low": 13, - "open": 13, - "volume": 1094419 - }, - "open": "2020-05-24T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 567428, - "high": 167446, - "low": 52, - "open": 500000, - "volume": 4266447 - }, - "id": 2615852, - "non_hive": { - "close": 168298, - "high": 50898, - "low": 15, - "open": 150029, - "volume": 1273274 - }, - "open": "2020-05-24T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 99756, - "high": 392, - "low": 7370, - "open": 6657, - "volume": 3271156 - }, - "id": 2615894, - "non_hive": { - "close": 29528, - "high": 118, - "low": 2170, - "open": 1999, - "volume": 968812 - }, - "open": "2020-05-24T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8422, - "high": 6, - "low": 64, - "open": 184, - "volume": 5051696 - }, - "id": 2615941, - "non_hive": { - "close": 2560, - "high": 2, - "low": 18, - "open": 55, - "volume": 1504089 - }, - "open": "2020-05-24T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 86759, - "high": 927, - "low": 5, - "open": 927, - "volume": 3313638 - }, - "id": 2615997, - "non_hive": { - "close": 25793, - "high": 282, - "low": 1, - "open": 282, - "volume": 986329 - }, - "open": "2020-05-24T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16000, - "high": 12730, - "low": 443370, - "open": 183, - "volume": 3756822 - }, - "id": 2616049, - "non_hive": { - "close": 4768, - "high": 3857, - "low": 131797, - "open": 55, - "volume": 1120685 - }, - "open": "2020-05-24T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72620, - "high": 2515, - "low": 5, - "open": 6628, - "volume": 3356664 - }, - "id": 2616105, - "non_hive": { - "close": 20987, - "high": 759, - "low": 1, - "open": 1999, - "volume": 983340 - }, - "open": "2020-05-24T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6000, - "high": 3, - "low": 11, - "open": 1798, - "volume": 4297274 - }, - "id": 2616151, - "non_hive": { - "close": 1692, - "high": 1, - "low": 3, - "open": 521, - "volume": 1226172 - }, - "open": "2020-05-24T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12768, - "high": 3, - "low": 16, - "open": 3453, - "volume": 3788667 - }, - "id": 2616214, - "non_hive": { - "close": 3802, - "high": 1, - "low": 4, - "open": 999, - "volume": 1070934 - }, - "open": "2020-05-24T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2185, - "high": 3, - "low": 3063, - "open": 3568, - "volume": 2230531 - }, - "id": 2616269, - "non_hive": { - "close": 650, - "high": 1, - "low": 861, - "open": 1062, - "volume": 643967 - }, - "open": "2020-05-24T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 480, - "low": 6, - "open": 3445, - "volume": 371252 - }, - "id": 2616323, - "non_hive": { - "close": 3, - "high": 143, - "low": 1, - "open": 1000, - "volume": 109877 - }, - "open": "2020-05-24T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8465, - "high": 40323, - "low": 6, - "open": 12, - "volume": 842368 - }, - "id": 2616361, - "non_hive": { - "close": 2517, - "high": 11998, - "low": 1, - "open": 3, - "volume": 248647 - }, - "open": "2020-05-24T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9169, - "high": 1872, - "low": 13, - "open": 41, - "volume": 1195751 - }, - "id": 2616413, - "non_hive": { - "close": 2726, - "high": 557, - "low": 3, - "open": 12, - "volume": 354561 - }, - "open": "2020-05-24T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1236, - "high": 188, - "low": 11, - "open": 14, - "volume": 5126006 - }, - "id": 2616458, - "non_hive": { - "close": 358, - "high": 56, - "low": 3, - "open": 4, - "volume": 1508172 - }, - "open": "2020-05-24T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51225, - "high": 934, - "low": 52, - "open": 934, - "volume": 3548130 - }, - "id": 2616521, - "non_hive": { - "close": 14843, - "high": 278, - "low": 15, - "open": 278, - "volume": 1031832 - }, - "open": "2020-05-25T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 975, - "high": 113, - "low": 98, - "open": 113, - "volume": 214413 - }, - "id": 2616566, - "non_hive": { - "close": 282, - "high": 33, - "low": 27, - "open": 33, - "volume": 61922 - }, - "open": "2020-05-25T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1388, - "high": 463, - "low": 4, - "open": 85036, - "volume": 121206 - }, - "id": 2616592, - "non_hive": { - "close": 401, - "high": 134, - "low": 1, - "open": 24568, - "volume": 35005 - }, - "open": "2020-05-25T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 554, - "high": 1387, - "low": 16, - "open": 7612, - "volume": 174256 - }, - "id": 2616612, - "non_hive": { - "close": 160, - "high": 401, - "low": 4, - "open": 2198, - "volume": 50335 - }, - "open": "2020-05-25T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 115, - "high": 644, - "low": 59, - "open": 43, - "volume": 162204 - }, - "id": 2616636, - "non_hive": { - "close": 32, - "high": 186, - "low": 16, - "open": 12, - "volume": 46823 - }, - "open": "2020-05-25T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1336, - "high": 1336, - "low": 11, - "open": 214085, - "volume": 1414028 - }, - "id": 2616660, - "non_hive": { - "close": 386, - "high": 386, - "low": 3, - "open": 60618, - "volume": 404963 - }, - "open": "2020-05-25T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16467, - "high": 92, - "low": 32, - "open": 229, - "volume": 1820530 - }, - "id": 2616680, - "non_hive": { - "close": 4799, - "high": 27, - "low": 9, - "open": 66, - "volume": 523978 - }, - "open": "2020-05-25T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 176097, - "high": 2000, - "low": 39017, - "open": 9802, - "volume": 2626226 - }, - "id": 2616726, - "non_hive": { - "close": 50927, - "high": 597, - "low": 11002, - "open": 2856, - "volume": 755434 - }, - "open": "2020-05-25T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49505, - "high": 23, - "low": 49505, - "open": 54199, - "volume": 323243 - }, - "id": 2616772, - "non_hive": { - "close": 14357, - "high": 7, - "low": 14357, - "open": 15845, - "volume": 95137 - }, - "open": "2020-05-25T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20550, - "high": 2534, - "low": 14, - "open": 14, - "volume": 204123 - }, - "id": 2616810, - "non_hive": { - "close": 6097, - "high": 752, - "low": 4, - "open": 4, - "volume": 59738 - }, - "open": "2020-05-25T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19337, - "high": 134, - "low": 629, - "open": 44, - "volume": 228955 - }, - "id": 2616842, - "non_hive": { - "close": 5736, - "high": 40, - "low": 185, - "open": 13, - "volume": 67906 - }, - "open": "2020-05-25T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 896, - "low": 11, - "open": 11638, - "volume": 241548 - }, - "id": 2616880, - "non_hive": { - "close": 3, - "high": 267, - "low": 3, - "open": 3452, - "volume": 71020 - }, - "open": "2020-05-25T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15290, - "high": 3, - "low": 4, - "open": 630, - "volume": 472599 - }, - "id": 2616908, - "non_hive": { - "close": 4478, - "high": 1, - "low": 1, - "open": 188, - "volume": 139907 - }, - "open": "2020-05-25T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9572, - "high": 154, - "low": 309, - "open": 11190, - "volume": 216756 - }, - "id": 2616951, - "non_hive": { - "close": 2853, - "high": 46, - "low": 90, - "open": 3335, - "volume": 64602 - }, - "open": "2020-05-25T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2505, - "high": 1788, - "low": 117, - "open": 1031, - "volume": 1983456 - }, - "id": 2616981, - "non_hive": { - "close": 746, - "high": 533, - "low": 33, - "open": 307, - "volume": 584730 - }, - "open": "2020-05-25T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2662, - "high": 219299, - "low": 211, - "open": 10415, - "volume": 1351918 - }, - "id": 2617024, - "non_hive": { - "close": 790, - "high": 65309, - "low": 61, - "open": 3100, - "volume": 401538 - }, - "open": "2020-05-25T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 1157, - "low": 42, - "open": 1467, - "volume": 4693036 - }, - "id": 2617057, - "non_hive": { - "close": 2986, - "high": 346, - "low": 12, - "open": 435, - "volume": 1385355 - }, - "open": "2020-05-25T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1405, - "high": 103, - "low": 122, - "open": 20388, - "volume": 515068 - }, - "id": 2617110, - "non_hive": { - "close": 420, - "high": 31, - "low": 35, - "open": 6094, - "volume": 153739 - }, - "open": "2020-05-25T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2000, - "high": 130, - "low": 284, - "open": 3816, - "volume": 775516 - }, - "id": 2617170, - "non_hive": { - "close": 597, - "high": 39, - "low": 83, - "open": 1140, - "volume": 231708 - }, - "open": "2020-05-25T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 202064, - "high": 103, - "low": 202064, - "open": 14232, - "volume": 2044343 - }, - "id": 2617218, - "non_hive": { - "close": 59053, - "high": 31, - "low": 59053, - "open": 4245, - "volume": 600678 - }, - "open": "2020-05-25T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100528, - "high": 618, - "low": 23, - "open": 3420, - "volume": 1215264 - }, - "id": 2617269, - "non_hive": { - "close": 28664, - "high": 183, - "low": 6, - "open": 1000, - "volume": 350172 - }, - "open": "2020-05-25T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7834, - "high": 1513, - "low": 13225, - "open": 859, - "volume": 164426 - }, - "id": 2617337, - "non_hive": { - "close": 2271, - "high": 439, - "low": 3800, - "open": 247, - "volume": 47492 - }, - "open": "2020-05-25T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31, - "high": 227, - "low": 121, - "open": 42, - "volume": 448901 - }, - "id": 2617377, - "non_hive": { - "close": 9, - "high": 66, - "low": 34, - "open": 12, - "volume": 129649 - }, - "open": "2020-05-25T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1047, - "high": 789, - "low": 38, - "open": 3430, - "volume": 116236 - }, - "id": 2617428, - "non_hive": { - "close": 302, - "high": 228, - "low": 10, - "open": 990, - "volume": 33511 - }, - "open": "2020-05-25T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8221, - "high": 2654, - "low": 53, - "open": 1699, - "volume": 89773 - }, - "id": 2617461, - "non_hive": { - "close": 2375, - "high": 767, - "low": 15, - "open": 488, - "volume": 25872 - }, - "open": "2020-05-26T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14598, - "high": 626, - "low": 36, - "open": 4001, - "volume": 47792 - }, - "id": 2617498, - "non_hive": { - "close": 4193, - "high": 181, - "low": 10, - "open": 1156, - "volume": 13780 - }, - "open": "2020-05-26T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 223085, - "high": 223085, - "low": 4, - "open": 4564, - "volume": 918359 - }, - "id": 2617514, - "non_hive": { - "close": 64470, - "high": 64470, - "low": 1, - "open": 1318, - "volume": 265189 - }, - "open": "2020-05-26T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 928, - "high": 6543, - "low": 8599, - "open": 6543, - "volume": 16070 - }, - "id": 2617549, - "non_hive": { - "close": 268, - "high": 1890, - "low": 2483, - "open": 1890, - "volume": 4641 - }, - "open": "2020-05-26T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 92, - "high": 4412, - "low": 43, - "open": 42001, - "volume": 371144 - }, - "id": 2617559, - "non_hive": { - "close": 26, - "high": 1275, - "low": 12, - "open": 12137, - "volume": 107081 - }, - "open": "2020-05-26T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 83, - "low": 11, - "open": 20005, - "volume": 138217 - }, - "id": 2617595, - "non_hive": { - "close": 3, - "high": 24, - "low": 3, - "open": 5779, - "volume": 39788 - }, - "open": "2020-05-26T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 439, - "high": 439, - "low": 28, - "open": 19322, - "volume": 408824 - }, - "id": 2617628, - "non_hive": { - "close": 127, - "high": 127, - "low": 8, - "open": 5581, - "volume": 118139 - }, - "open": "2020-05-26T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1539, - "high": 155, - "low": 1224, - "open": 9088, - "volume": 5867814 - }, - "id": 2617679, - "non_hive": { - "close": 443, - "high": 45, - "low": 339, - "open": 2626, - "volume": 1661076 - }, - "open": "2020-05-26T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18894, - "high": 730, - "low": 18524, - "open": 470, - "volume": 141507 - }, - "id": 2617745, - "non_hive": { - "close": 5290, - "high": 210, - "low": 5186, - "open": 135, - "volume": 39862 - }, - "open": "2020-05-26T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27204, - "high": 3, - "low": 4, - "open": 15, - "volume": 2650291 - }, - "id": 2617765, - "non_hive": { - "close": 7714, - "high": 1, - "low": 1, - "open": 4, - "volume": 751515 - }, - "open": "2020-05-26T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47054, - "high": 737, - "low": 43, - "open": 27748, - "volume": 226599 - }, - "id": 2617814, - "non_hive": { - "close": 13467, - "high": 211, - "low": 12, - "open": 7869, - "volume": 64625 - }, - "open": "2020-05-26T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 173130, - "low": 28, - "open": 26425, - "volume": 811800 - }, - "id": 2617845, - "non_hive": { - "close": 4, - "high": 50000, - "low": 8, - "open": 7563, - "volume": 233271 - }, - "open": "2020-05-26T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34508, - "high": 8784, - "low": 49798, - "open": 81476, - "volume": 481694 - }, - "id": 2617885, - "non_hive": { - "close": 9966, - "high": 2537, - "low": 14253, - "open": 23530, - "volume": 138981 - }, - "open": "2020-05-26T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 69461, - "high": 1485, - "low": 69461, - "open": 17985, - "volume": 1012595 - }, - "id": 2617921, - "non_hive": { - "close": 20000, - "high": 429, - "low": 20000, - "open": 5194, - "volume": 291722 - }, - "open": "2020-05-26T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13965, - "high": 13965, - "low": 5, - "open": 5325, - "volume": 8742139 - }, - "id": 2617947, - "non_hive": { - "close": 4244, - "high": 4244, - "low": 1, - "open": 1533, - "volume": 2551844 - }, - "open": "2020-05-26T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18874, - "high": 3, - "low": 17786, - "open": 86035, - "volume": 2048987 - }, - "id": 2617991, - "non_hive": { - "close": 5624, - "high": 1, - "low": 5299, - "open": 26146, - "volume": 618395 - }, - "open": "2020-05-26T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 88, - "high": 9240, - "low": 88, - "open": 19252, - "volume": 2136107 - }, - "id": 2618052, - "non_hive": { - "close": 25, - "high": 2835, - "low": 25, - "open": 5736, - "volume": 623313 - }, - "open": "2020-05-26T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 224, - "high": 224, - "low": 9, - "open": 12191, - "volume": 130038 - }, - "id": 2618107, - "non_hive": { - "close": 68, - "high": 68, - "low": 2, - "open": 3654, - "volume": 38901 - }, - "open": "2020-05-26T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25, - "high": 92, - "low": 25, - "open": 1420, - "volume": 50151 - }, - "id": 2618141, - "non_hive": { - "close": 7, - "high": 28, - "low": 7, - "open": 429, - "volume": 15135 - }, - "open": "2020-05-26T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 200, - "high": 4634, - "low": 3566, - "open": 3566, - "volume": 140554 - }, - "id": 2618168, - "non_hive": { - "close": 60, - "high": 1395, - "low": 1034, - "open": 1034, - "volume": 41956 - }, - "open": "2020-05-26T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 340, - "high": 505, - "low": 20, - "open": 18698, - "volume": 1223956 - }, - "id": 2618202, - "non_hive": { - "close": 100, - "high": 152, - "low": 5, - "open": 5626, - "volume": 356873 - }, - "open": "2020-05-26T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6541, - "high": 3, - "low": 53, - "open": 6460, - "volume": 1677221 - }, - "id": 2618237, - "non_hive": { - "close": 1883, - "high": 1, - "low": 15, - "open": 1899, - "volume": 489471 - }, - "open": "2020-05-26T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25, - "high": 3, - "low": 5, - "open": 42, - "volume": 242239 - }, - "id": 2618272, - "non_hive": { - "close": 7, - "high": 1, - "low": 1, - "open": 12, - "volume": 71471 - }, - "open": "2020-05-26T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25, - "high": 3, - "low": 25, - "open": 6723, - "volume": 1015400 - }, - "id": 2618308, - "non_hive": { - "close": 7, - "high": 1, - "low": 7, - "open": 1999, - "volume": 295779 - }, - "open": "2020-05-26T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4827, - "high": 511, - "low": 53, - "open": 511, - "volume": 98957 - }, - "id": 2618356, - "non_hive": { - "close": 1424, - "high": 151, - "low": 15, - "open": 151, - "volume": 28716 - }, - "open": "2020-05-27T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46, - "high": 6130, - "low": 46, - "open": 6585, - "volume": 1087871 - }, - "id": 2618382, - "non_hive": { - "close": 13, - "high": 1808, - "low": 13, - "open": 1882, - "volume": 311115 - }, - "open": "2020-05-27T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8350, - "high": 3, - "low": 4, - "open": 27793, - "volume": 583502 - }, - "id": 2618406, - "non_hive": { - "close": 2421, - "high": 1, - "low": 1, - "open": 8167, - "volume": 169516 - }, - "open": "2020-05-27T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3901, - "high": 286, - "low": 30, - "open": 286, - "volume": 115240 - }, - "id": 2618457, - "non_hive": { - "close": 1131, - "high": 83, - "low": 8, - "open": 83, - "volume": 33399 - }, - "open": "2020-05-27T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23344, - "high": 1973, - "low": 37, - "open": 1694, - "volume": 527575 - }, - "id": 2618492, - "non_hive": { - "close": 6767, - "high": 572, - "low": 10, - "open": 491, - "volume": 152930 - }, - "open": "2020-05-27T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 89, - "high": 89, - "low": 41, - "open": 3007, - "volume": 328319 - }, - "id": 2618509, - "non_hive": { - "close": 26, - "high": 26, - "low": 11, - "open": 871, - "volume": 95170 - }, - "open": "2020-05-27T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8522, - "high": 86, - "low": 32, - "open": 125354, - "volume": 275546 - }, - "id": 2618542, - "non_hive": { - "close": 2471, - "high": 25, - "low": 9, - "open": 36336, - "volume": 79877 - }, - "open": "2020-05-27T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 609, - "high": 920, - "low": 101, - "open": 50000, - "volume": 188918 - }, - "id": 2618576, - "non_hive": { - "close": 176, - "high": 267, - "low": 29, - "open": 14496, - "volume": 54671 - }, - "open": "2020-05-27T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 417, - "high": 417, - "low": 3000, - "open": 13133, - "volume": 37821 - }, - "id": 2618600, - "non_hive": { - "close": 121, - "high": 121, - "low": 864, - "open": 3806, - "volume": 10954 - }, - "open": "2020-05-27T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 655, - "high": 17, - "low": 14, - "open": 14, - "volume": 75099 - }, - "id": 2618625, - "non_hive": { - "close": 190, - "high": 5, - "low": 4, - "open": 4, - "volume": 21764 - }, - "open": "2020-05-27T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26432, - "high": 41, - "low": 42, - "open": 42, - "volume": 707328 - }, - "id": 2618652, - "non_hive": { - "close": 7660, - "high": 12, - "low": 12, - "open": 12, - "volume": 203704 - }, - "open": "2020-05-27T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 3222, - "low": 11, - "open": 72283, - "volume": 1605691 - }, - "id": 2618681, - "non_hive": { - "close": 3, - "high": 934, - "low": 3, - "open": 20799, - "volume": 461996 - }, - "open": "2020-05-27T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3638261, - "high": 348, - "low": 438849, - "open": 15000, - "volume": 4446021 - }, - "id": 2618714, - "non_hive": { - "close": 1046000, - "high": 101, - "low": 126169, - "open": 4347, - "volume": 1278805 - }, - "open": "2020-05-27T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 103842, - "high": 3, - "low": 93, - "open": 17249, - "volume": 379201 - }, - "id": 2618757, - "non_hive": { - "close": 30000, - "high": 1, - "low": 26, - "open": 4997, - "volume": 109644 - }, - "open": "2020-05-27T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9690, - "high": 20, - "low": 6956, - "open": 3955, - "volume": 690440 - }, - "id": 2618804, - "non_hive": { - "close": 2790, - "high": 6, - "low": 1988, - "open": 1145, - "volume": 197520 - }, - "open": "2020-05-27T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16610, - "high": 6228, - "low": 4, - "open": 2463, - "volume": 320782 - }, - "id": 2618832, - "non_hive": { - "close": 4768, - "high": 1793, - "low": 1, - "open": 709, - "volume": 92189 - }, - "open": "2020-05-27T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11670, - "high": 640, - "low": 43, - "open": 3958, - "volume": 114402 - }, - "id": 2618870, - "non_hive": { - "close": 3348, - "high": 184, - "low": 12, - "open": 1136, - "volume": 32840 - }, - "open": "2020-05-27T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10602, - "high": 10602, - "low": 11, - "open": 2136, - "volume": 1044134 - }, - "id": 2618898, - "non_hive": { - "close": 3045, - "high": 3045, - "low": 3, - "open": 613, - "volume": 299701 - }, - "open": "2020-05-27T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9885, - "high": 198, - "low": 18784, - "open": 34692, - "volume": 142191 - }, - "id": 2618959, - "non_hive": { - "close": 2835, - "high": 57, - "low": 5290, - "open": 9963, - "volume": 40712 - }, - "open": "2020-05-27T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1017, - "high": 17, - "low": 3570, - "open": 8717, - "volume": 133284 - }, - "id": 2618995, - "non_hive": { - "close": 292, - "high": 5, - "low": 1006, - "open": 2500, - "volume": 38210 - }, - "open": "2020-05-27T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10439, - "high": 3, - "low": 5575, - "open": 191150, - "volume": 291320 - }, - "id": 2619035, - "non_hive": { - "close": 2992, - "high": 1, - "low": 1594, - "open": 54669, - "volume": 83349 - }, - "open": "2020-05-27T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4661, - "high": 1045, - "low": 1183, - "open": 16108, - "volume": 382341 - }, - "id": 2619071, - "non_hive": { - "close": 1337, - "high": 300, - "low": 339, - "open": 4616, - "volume": 109634 - }, - "open": "2020-05-27T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 210428, - "low": 42, - "open": 18947, - "volume": 852331 - }, - "id": 2619130, - "non_hive": { - "close": 4, - "high": 60949, - "low": 12, - "open": 5434, - "volume": 245210 - }, - "open": "2020-05-27T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 839, - "high": 24, - "low": 273, - "open": 545, - "volume": 98271 - }, - "id": 2619169, - "non_hive": { - "close": 243, - "high": 7, - "low": 78, - "open": 158, - "volume": 28441 - }, - "open": "2020-05-27T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 313830, - "high": 6069, - "low": 52, - "open": 11097, - "volume": 473893 - }, - "id": 2619207, - "non_hive": { - "close": 90889, - "high": 1758, - "low": 15, - "open": 3214, - "volume": 137247 - }, - "open": "2020-05-28T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17529, - "high": 2102, - "low": 16806, - "open": 2102, - "volume": 88573 - }, - "id": 2619239, - "non_hive": { - "close": 5074, - "high": 609, - "low": 4820, - "open": 609, - "volume": 25600 - }, - "open": "2020-05-28T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5255, - "high": 120, - "low": 4, - "open": 18608, - "volume": 197589 - }, - "id": 2619257, - "non_hive": { - "close": 1522, - "high": 35, - "low": 1, - "open": 5388, - "volume": 57225 - }, - "open": "2020-05-28T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27390, - "high": 31073, - "low": 27390, - "open": 17553, - "volume": 754556 - }, - "id": 2619285, - "non_hive": { - "close": 7850, - "high": 9000, - "low": 7850, - "open": 5082, - "volume": 216838 - }, - "open": "2020-05-28T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29838, - "high": 477, - "low": 42, - "open": 42, - "volume": 36699 - }, - "id": 2619310, - "non_hive": { - "close": 8618, - "high": 138, - "low": 12, - "open": 12, - "volume": 10602 - }, - "open": "2020-05-28T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 2893, - "low": 11, - "open": 14, - "volume": 131134 - }, - "id": 2619328, - "non_hive": { - "close": 3, - "high": 838, - "low": 3, - "open": 4, - "volume": 37967 - }, - "open": "2020-05-28T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48, - "high": 48, - "low": 162, - "open": 1886, - "volume": 199448 - }, - "id": 2619361, - "non_hive": { - "close": 14, - "high": 14, - "low": 46, - "open": 546, - "volume": 57532 - }, - "open": "2020-05-28T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38, - "high": 38, - "low": 102, - "open": 3503, - "volume": 157242 - }, - "id": 2619407, - "non_hive": { - "close": 11, - "high": 11, - "low": 29, - "open": 1013, - "volume": 45206 - }, - "open": "2020-05-28T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27, - "high": 3, - "low": 8316, - "open": 173, - "volume": 308222 - }, - "id": 2619433, - "non_hive": { - "close": 8, - "high": 1, - "low": 2377, - "open": 50, - "volume": 88951 - }, - "open": "2020-05-28T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 553988, - "high": 96, - "low": 14, - "open": 2406, - "volume": 657031 - }, - "id": 2619457, - "non_hive": { - "close": 160047, - "high": 28, - "low": 4, - "open": 696, - "volume": 189811 - }, - "open": "2020-05-28T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16605, - "high": 955, - "low": 16425, - "open": 1298, - "volume": 1786557 - }, - "id": 2619493, - "non_hive": { - "close": 4687, - "high": 276, - "low": 4635, - "open": 375, - "volume": 509743 - }, - "open": "2020-05-28T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 611, - "low": 11, - "open": 1567, - "volume": 112817 - }, - "id": 2619538, - "non_hive": { - "close": 3, - "high": 177, - "low": 3, - "open": 442, - "volume": 32629 - }, - "open": "2020-05-28T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17471, - "high": 231, - "low": 13730, - "open": 942, - "volume": 1151374 - }, - "id": 2619582, - "non_hive": { - "close": 5059, - "high": 67, - "low": 3968, - "open": 273, - "volume": 332947 - }, - "open": "2020-05-28T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 70793, - "high": 528, - "low": 235181, - "open": 4945, - "volume": 762591 - }, - "id": 2619622, - "non_hive": { - "close": 20492, - "high": 153, - "low": 67262, - "open": 1432, - "volume": 218639 - }, - "open": "2020-05-28T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6401, - "high": 459, - "low": 18284, - "open": 459, - "volume": 99380 - }, - "id": 2619649, - "non_hive": { - "close": 1849, - "high": 133, - "low": 5211, - "open": 133, - "volume": 28632 - }, - "open": "2020-05-28T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7673, - "high": 5205, - "low": 53, - "open": 77379, - "volume": 631995 - }, - "id": 2619677, - "non_hive": { - "close": 2217, - "high": 1504, - "low": 15, - "open": 22093, - "volume": 181440 - }, - "open": "2020-05-28T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 692972, - "high": 449, - "low": 43, - "open": 449, - "volume": 983571 - }, - "id": 2619713, - "non_hive": { - "close": 197497, - "high": 130, - "low": 12, - "open": 130, - "volume": 280648 - }, - "open": "2020-05-28T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 10, - "low": 11, - "open": 380, - "volume": 1210010 - }, - "id": 2619750, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 110, - "volume": 349506 - }, - "open": "2020-05-28T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18806, - "high": 20, - "low": 936, - "open": 1087, - "volume": 118495 - }, - "id": 2619789, - "non_hive": { - "close": 5423, - "high": 6, - "low": 269, - "open": 314, - "volume": 34186 - }, - "open": "2020-05-28T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1068, - "high": 31, - "low": 326966, - "open": 8591, - "volume": 863134 - }, - "id": 2619823, - "non_hive": { - "close": 308, - "high": 9, - "low": 93046, - "open": 2477, - "volume": 246021 - }, - "open": "2020-05-28T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1594, - "high": 48, - "low": 14748, - "open": 48, - "volume": 472457 - }, - "id": 2619868, - "non_hive": { - "close": 458, - "high": 14, - "low": 4231, - "open": 14, - "volume": 135771 - }, - "open": "2020-05-28T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5370, - "high": 919, - "low": 10, - "open": 16678, - "volume": 3680725 - }, - "id": 2619907, - "non_hive": { - "close": 1540, - "high": 264, - "low": 2, - "open": 4790, - "volume": 1046411 - }, - "open": "2020-05-28T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 3, - "low": 51, - "open": 43, - "volume": 177689 - }, - "id": 2619960, - "non_hive": { - "close": 4, - "high": 1, - "low": 14, - "open": 12, - "volume": 50632 - }, - "open": "2020-05-28T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 52, - "low": 24, - "open": 8348, - "volume": 1836233 - }, - "id": 2620005, - "non_hive": { - "close": 3, - "high": 15, - "low": 6, - "open": 2391, - "volume": 517826 - }, - "open": "2020-05-28T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3559, - "high": 3, - "low": 6305, - "open": 357, - "volume": 106740 - }, - "id": 2620065, - "non_hive": { - "close": 1015, - "high": 1, - "low": 1743, - "open": 100, - "volume": 30306 - }, - "open": "2020-05-29T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 84, - "high": 3502, - "low": 84, - "open": 7016, - "volume": 59807 - }, - "id": 2620104, - "non_hive": { - "close": 23, - "high": 998, - "low": 23, - "open": 1999, - "volume": 16937 - }, - "open": "2020-05-29T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 6495, - "low": 10, - "open": 6495, - "volume": 7093 - }, - "id": 2620119, - "non_hive": { - "close": 2, - "high": 1837, - "low": 2, - "open": 1837, - "volume": 2005 - }, - "open": "2020-05-29T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6904, - "high": 6904, - "low": 93764, - "open": 353, - "volume": 349343 - }, - "id": 2620128, - "non_hive": { - "close": 1971, - "high": 1971, - "low": 25784, - "open": 100, - "volume": 96159 - }, - "open": "2020-05-29T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2428, - "high": 3, - "low": 44, - "open": 986, - "volume": 118151 - }, - "id": 2620138, - "non_hive": { - "close": 688, - "high": 1, - "low": 12, - "open": 270, - "volume": 32763 - }, - "open": "2020-05-29T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 84, - "low": 37, - "open": 2683, - "volume": 433440 - }, - "id": 2620168, - "non_hive": { - "close": 3, - "high": 24, - "low": 10, - "open": 760, - "volume": 122049 - }, - "open": "2020-05-29T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 2803, - "low": 51, - "open": 7575, - "volume": 130966 - }, - "id": 2620202, - "non_hive": { - "close": 2820, - "high": 803, - "low": 14, - "open": 2140, - "volume": 37115 - }, - "open": "2020-05-29T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4448, - "high": 105, - "low": 103, - "open": 5001, - "volume": 200538 - }, - "id": 2620240, - "non_hive": { - "close": 1263, - "high": 30, - "low": 29, - "open": 1420, - "volume": 56934 - }, - "open": "2020-05-29T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6126, - "high": 28, - "low": 2000, - "open": 975, - "volume": 229996 - }, - "id": 2620278, - "non_hive": { - "close": 1739, - "high": 8, - "low": 567, - "open": 277, - "volume": 65287 - }, - "open": "2020-05-29T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2418, - "high": 1828, - "low": 15, - "open": 15, - "volume": 87159 - }, - "id": 2620316, - "non_hive": { - "close": 686, - "high": 519, - "low": 4, - "open": 4, - "volume": 24722 - }, - "open": "2020-05-29T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42191, - "high": 28, - "low": 43, - "open": 1040, - "volume": 803182 - }, - "id": 2620339, - "non_hive": { - "close": 11972, - "high": 8, - "low": 12, - "open": 295, - "volume": 227898 - }, - "open": "2020-05-29T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 322, - "high": 274, - "low": 29, - "open": 5000, - "volume": 278068 - }, - "id": 2620378, - "non_hive": { - "close": 91, - "high": 78, - "low": 8, - "open": 1419, - "volume": 78400 - }, - "open": "2020-05-29T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 229, - "high": 35, - "low": 11, - "open": 11, - "volume": 242058 - }, - "id": 2620406, - "non_hive": { - "close": 65, - "high": 10, - "low": 3, - "open": 3, - "volume": 68634 - }, - "open": "2020-05-29T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 727, - "high": 955, - "low": 4650, - "open": 17092, - "volume": 160031 - }, - "id": 2620437, - "non_hive": { - "close": 206, - "high": 271, - "low": 1307, - "open": 4845, - "volume": 45334 - }, - "open": "2020-05-29T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6900, - "high": 1942, - "low": 14351, - "open": 2001, - "volume": 298115 - }, - "id": 2620462, - "non_hive": { - "close": 1957, - "high": 551, - "low": 4066, - "open": 567, - "volume": 84541 - }, - "open": "2020-05-29T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1955, - "high": 3, - "low": 8, - "open": 3734, - "volume": 1498235 - }, - "id": 2620485, - "non_hive": { - "close": 551, - "high": 1, - "low": 2, - "open": 1059, - "volume": 421271 - }, - "open": "2020-05-29T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2382, - "high": 413, - "low": 43, - "open": 702, - "volume": 143493 - }, - "id": 2620521, - "non_hive": { - "close": 673, - "high": 117, - "low": 12, - "open": 198, - "volume": 40444 - }, - "open": "2020-05-29T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 184309, - "high": 3, - "low": 110, - "open": 110, - "volume": 419955 - }, - "id": 2620548, - "non_hive": { - "close": 52188, - "high": 1, - "low": 30, - "open": 30, - "volume": 118347 - }, - "open": "2020-05-29T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1844, - "high": 3, - "low": 11, - "open": 11, - "volume": 468691 - }, - "id": 2620586, - "non_hive": { - "close": 520, - "high": 1, - "low": 3, - "open": 3, - "volume": 131582 - }, - "open": "2020-05-29T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7133, - "high": 3, - "low": 49, - "open": 1842, - "volume": 136689 - }, - "id": 2620635, - "non_hive": { - "close": 1999, - "high": 1, - "low": 13, - "open": 519, - "volume": 38332 - }, - "open": "2020-05-29T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 92, - "high": 3, - "low": 87, - "open": 8021, - "volume": 125955 - }, - "id": 2620674, - "non_hive": { - "close": 25, - "high": 1, - "low": 23, - "open": 2233, - "volume": 35092 - }, - "open": "2020-05-29T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 418, - "high": 357, - "low": 17449, - "open": 17476, - "volume": 328613 - }, - "id": 2620715, - "non_hive": { - "close": 117, - "high": 100, - "low": 4878, - "open": 4890, - "volume": 91955 - }, - "open": "2020-05-29T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15, - "high": 63, - "low": 15, - "open": 32193, - "volume": 207564 - }, - "id": 2620735, - "non_hive": { - "close": 4, - "high": 18, - "low": 4, - "open": 9000, - "volume": 57964 - }, - "open": "2020-05-29T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16167, - "high": 188, - "low": 40, - "open": 70000, - "volume": 768541 - }, - "id": 2620770, - "non_hive": { - "close": 4556, - "high": 53, - "low": 11, - "open": 19598, - "volume": 215648 - }, - "open": "2020-05-29T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2617, - "high": 99, - "low": 11, - "open": 31778, - "volume": 653839 - }, - "id": 2620822, - "non_hive": { - "close": 737, - "high": 28, - "low": 3, - "open": 8950, - "volume": 184000 - }, - "open": "2020-05-30T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11964, - "high": 934, - "low": 4100, - "open": 934, - "volume": 46673 - }, - "id": 2620853, - "non_hive": { - "close": 3360, - "high": 263, - "low": 1151, - "open": 263, - "volume": 13112 - }, - "open": "2020-05-30T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 837, - "high": 475, - "low": 4, - "open": 475, - "volume": 240128 - }, - "id": 2620882, - "non_hive": { - "close": 236, - "high": 134, - "low": 1, - "open": 134, - "volume": 66842 - }, - "open": "2020-05-30T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5384, - "high": 7, - "low": 406, - "open": 15870, - "volume": 724848 - }, - "id": 2620917, - "non_hive": { - "close": 1506, - "high": 2, - "low": 111, - "open": 4464, - "volume": 199034 - }, - "open": "2020-05-30T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35571, - "high": 192, - "low": 44, - "open": 1416, - "volume": 252061 - }, - "id": 2620938, - "non_hive": { - "close": 9746, - "high": 54, - "low": 12, - "open": 395, - "volume": 70118 - }, - "open": "2020-05-30T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9384, - "high": 6344, - "low": 3645, - "open": 6344, - "volume": 55095 - }, - "id": 2620963, - "non_hive": { - "close": 2617, - "high": 1777, - "low": 1015, - "open": 1777, - "volume": 15378 - }, - "open": "2020-05-30T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5666, - "high": 75, - "low": 11, - "open": 12088, - "volume": 154228 - }, - "id": 2620991, - "non_hive": { - "close": 1577, - "high": 21, - "low": 3, - "open": 3370, - "volume": 42818 - }, - "open": "2020-05-30T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4545, - "high": 143, - "low": 4545, - "open": 10000, - "volume": 1331473 - }, - "id": 2621023, - "non_hive": { - "close": 1246, - "high": 40, - "low": 1246, - "open": 2784, - "volume": 370700 - }, - "open": "2020-05-30T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14266, - "high": 138, - "low": 13424, - "open": 142, - "volume": 342236 - }, - "id": 2621048, - "non_hive": { - "close": 4013, - "high": 39, - "low": 3697, - "open": 40, - "volume": 95721 - }, - "open": "2020-05-30T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 3, - "low": 4, - "open": 8607, - "volume": 2674600 - }, - "id": 2621101, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 2421, - "volume": 736533 - }, - "open": "2020-05-30T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 3, - "low": 44, - "open": 44, - "volume": 209133 - }, - "id": 2621146, - "non_hive": { - "close": 1, - "high": 1, - "low": 12, - "open": 12, - "volume": 57801 - }, - "open": "2020-05-30T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13697, - "high": 32, - "low": 30, - "open": 8819, - "volume": 221842 - }, - "id": 2621166, - "non_hive": { - "close": 3784, - "high": 9, - "low": 8, - "open": 2437, - "volume": 61299 - }, - "open": "2020-05-30T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2262, - "high": 130, - "low": 11, - "open": 11, - "volume": 61708 - }, - "id": 2621221, - "non_hive": { - "close": 625, - "high": 36, - "low": 3, - "open": 3, - "volume": 17047 - }, - "open": "2020-05-30T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36757, - "high": 25, - "low": 26633, - "open": 25, - "volume": 419482 - }, - "id": 2621254, - "non_hive": { - "close": 10155, - "high": 7, - "low": 7274, - "open": 7, - "volume": 115428 - }, - "open": "2020-05-30T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7223, - "high": 21, - "low": 7040, - "open": 36197, - "volume": 155128 - }, - "id": 2621276, - "non_hive": { - "close": 1996, - "high": 6, - "low": 1939, - "open": 10000, - "volume": 42812 - }, - "open": "2020-05-30T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 117194, - "high": 3, - "low": 33, - "open": 1518, - "volume": 748309 - }, - "id": 2621312, - "non_hive": { - "close": 32369, - "high": 1, - "low": 9, - "open": 418, - "volume": 206483 - }, - "open": "2020-05-30T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33, - "high": 47, - "low": 33, - "open": 91, - "volume": 1204358 - }, - "id": 2621341, - "non_hive": { - "close": 8, - "high": 13, - "low": 8, - "open": 25, - "volume": 329210 - }, - "open": "2020-05-30T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32, - "high": 2619, - "low": 32, - "open": 5624, - "volume": 174339 - }, - "id": 2621379, - "non_hive": { - "close": 8, - "high": 721, - "low": 8, - "open": 1548, - "volume": 47634 - }, - "open": "2020-05-30T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11271, - "high": 3, - "low": 6, - "open": 12, - "volume": 793502 - }, - "id": 2621406, - "non_hive": { - "close": 3095, - "high": 1, - "low": 1, - "open": 3, - "volume": 214808 - }, - "open": "2020-05-30T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17081, - "high": 182, - "low": 207, - "open": 1198, - "volume": 1305067 - }, - "id": 2621449, - "non_hive": { - "close": 4681, - "high": 50, - "low": 55, - "open": 329, - "volume": 356768 - }, - "open": "2020-05-30T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38, - "high": 98, - "low": 38, - "open": 1572, - "volume": 1213059 - }, - "id": 2621498, - "non_hive": { - "close": 10, - "high": 27, - "low": 10, - "open": 431, - "volume": 328891 - }, - "open": "2020-05-30T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21659, - "high": 325, - "low": 11, - "open": 2418, - "volume": 943062 - }, - "id": 2621532, - "non_hive": { - "close": 5884, - "high": 89, - "low": 2, - "open": 662, - "volume": 254068 - }, - "open": "2020-05-30T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 176557, - "high": 22, - "low": 56, - "open": 22, - "volume": 773782 - }, - "id": 2621570, - "non_hive": { - "close": 47873, - "high": 6, - "low": 14, - "open": 6, - "volume": 206280 - }, - "open": "2020-05-30T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33, - "high": 103, - "low": 33, - "open": 8011, - "volume": 199048 - }, - "id": 2621619, - "non_hive": { - "close": 8, - "high": 28, - "low": 8, - "open": 2171, - "volume": 53610 - }, - "open": "2020-05-30T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20560, - "high": 37410, - "low": 12, - "open": 175, - "volume": 115045 - }, - "id": 2621661, - "non_hive": { - "close": 5566, - "high": 10162, - "low": 3, - "open": 47, - "volume": 31093 - }, - "open": "2020-05-31T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4986, - "high": 263, - "low": 271, - "open": 8001, - "volume": 72573 - }, - "id": 2621683, - "non_hive": { - "close": 1338, - "high": 71, - "low": 71, - "open": 2155, - "volume": 19518 - }, - "open": "2020-05-31T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 133, - "high": 908, - "low": 4, - "open": 7306, - "volume": 41344 - }, - "id": 2621705, - "non_hive": { - "close": 34, - "high": 243, - "low": 1, - "open": 1917, - "volume": 11001 - }, - "open": "2020-05-31T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10140, - "high": 1128, - "low": 12, - "open": 18743, - "volume": 303493 - }, - "id": 2621730, - "non_hive": { - "close": 2729, - "high": 304, - "low": 3, - "open": 5000, - "volume": 80845 - }, - "open": "2020-05-31T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10644, - "high": 14105, - "low": 42, - "open": 14105, - "volume": 45972 - }, - "id": 2621748, - "non_hive": { - "close": 2850, - "high": 3784, - "low": 11, - "open": 3784, - "volume": 12320 - }, - "open": "2020-05-31T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7693, - "high": 601, - "low": 38282, - "open": 26109, - "volume": 134444 - }, - "id": 2621762, - "non_hive": { - "close": 2060, - "high": 161, - "low": 10249, - "open": 6991, - "volume": 35997 - }, - "open": "2020-05-31T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23346, - "high": 1213, - "low": 12, - "open": 351, - "volume": 158191 - }, - "id": 2621780, - "non_hive": { - "close": 6253, - "high": 325, - "low": 3, - "open": 94, - "volume": 42368 - }, - "open": "2020-05-31T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3286, - "high": 369, - "low": 101, - "open": 10044, - "volume": 105392 - }, - "id": 2621806, - "non_hive": { - "close": 880, - "high": 99, - "low": 27, - "open": 2690, - "volume": 28222 - }, - "open": "2020-05-31T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2252, - "high": 3, - "low": 1566, - "open": 70, - "volume": 64080 - }, - "id": 2621838, - "non_hive": { - "close": 603, - "high": 1, - "low": 419, - "open": 19, - "volume": 17155 - }, - "open": "2020-05-31T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24308, - "high": 70, - "low": 12, - "open": 4434, - "volume": 198915 - }, - "id": 2621862, - "non_hive": { - "close": 6509, - "high": 19, - "low": 3, - "open": 1187, - "volume": 53254 - }, - "open": "2020-05-31T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13620, - "high": 354, - "low": 42, - "open": 336, - "volume": 158306 - }, - "id": 2621900, - "non_hive": { - "close": 3649, - "high": 95, - "low": 11, - "open": 90, - "volume": 42395 - }, - "open": "2020-05-31T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4334, - "high": 1230, - "low": 30, - "open": 21059, - "volume": 100011 - }, - "id": 2621920, - "non_hive": { - "close": 1169, - "high": 332, - "low": 8, - "open": 5642, - "volume": 26881 - }, - "open": "2020-05-31T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7956, - "high": 800, - "low": 12, - "open": 12, - "volume": 246444 - }, - "id": 2621954, - "non_hive": { - "close": 2146, - "high": 216, - "low": 3, - "open": 3, - "volume": 66466 - }, - "open": "2020-05-31T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10930, - "high": 126, - "low": 936, - "open": 1894, - "volume": 540274 - }, - "id": 2621979, - "non_hive": { - "close": 2948, - "high": 34, - "low": 252, - "open": 511, - "volume": 145713 - }, - "open": "2020-05-31T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 133, - "high": 133, - "low": 34, - "open": 370, - "volume": 933820 - }, - "id": 2622006, - "non_hive": { - "close": 36, - "high": 36, - "low": 9, - "open": 100, - "volume": 251848 - }, - "open": "2020-05-31T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 124, - "high": 85, - "low": 94, - "open": 1291, - "volume": 263437 - }, - "id": 2622037, - "non_hive": { - "close": 33, - "high": 23, - "low": 25, - "open": 348, - "volume": 70918 - }, - "open": "2020-05-31T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 730, - "high": 48, - "low": 117, - "open": 1062, - "volume": 987042 - }, - "id": 2622074, - "non_hive": { - "close": 196, - "high": 13, - "low": 31, - "open": 286, - "volume": 263414 - }, - "open": "2020-05-31T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5018, - "high": 7, - "low": 4, - "open": 7454, - "volume": 1963384 - }, - "id": 2622122, - "non_hive": { - "close": 1337, - "high": 2, - "low": 1, - "open": 1999, - "volume": 520095 - }, - "open": "2020-05-31T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 124056, - "high": 86, - "low": 12, - "open": 12, - "volume": 422121 - }, - "id": 2622166, - "non_hive": { - "close": 32875, - "high": 23, - "low": 3, - "open": 3, - "volume": 112122 - }, - "open": "2020-05-31T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3802, - "high": 3, - "low": 22364, - "open": 83, - "volume": 1920585 - }, - "id": 2622215, - "non_hive": { - "close": 1000, - "high": 1, - "low": 5830, - "open": 22, - "volume": 502899 - }, - "open": "2020-05-31T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1758, - "high": 460, - "low": 1336560, - "open": 25461, - "volume": 2869550 - }, - "id": 2622258, - "non_hive": { - "close": 462, - "high": 121, - "low": 347505, - "open": 6696, - "volume": 748364 - }, - "open": "2020-05-31T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39488, - "high": 9867, - "low": 1440301, - "open": 2520, - "volume": 2744042 - }, - "id": 2622303, - "non_hive": { - "close": 10267, - "high": 2595, - "low": 374478, - "open": 662, - "volume": 714206 - }, - "open": "2020-05-31T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 76, - "low": 12, - "open": 376, - "volume": 321775 - }, - "id": 2622332, - "non_hive": { - "close": 3, - "high": 20, - "low": 3, - "open": 98, - "volume": 83170 - }, - "open": "2020-05-31T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7167, - "high": 7, - "low": 2311, - "open": 7696, - "volume": 151779 - }, - "id": 2622387, - "non_hive": { - "close": 1855, - "high": 2, - "low": 579, - "open": 1999, - "volume": 38956 - }, - "open": "2020-05-31T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 92496, - "high": 7710, - "low": 12, - "open": 456, - "volume": 8376781 - }, - "id": 2622420, - "non_hive": { - "close": 23124, - "high": 2001, - "low": 3, - "open": 118, - "volume": 2096025 - }, - "open": "2020-06-01T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 560129, - "high": 6032, - "low": 63, - "open": 6032, - "volume": 2141519 - }, - "id": 2622478, - "non_hive": { - "close": 134627, - "high": 1536, - "low": 15, - "open": 1536, - "volume": 516434 - }, - "open": "2020-06-01T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49096, - "high": 34147, - "low": 175711, - "open": 7937, - "volume": 5643178 - }, - "id": 2622509, - "non_hive": { - "close": 11802, - "high": 8603, - "low": 42232, - "open": 1999, - "volume": 1370884 - }, - "open": "2020-06-01T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7870, - "high": 9083, - "low": 36618, - "open": 2365, - "volume": 6122531 - }, - "id": 2622549, - "non_hive": { - "close": 1999, - "high": 2311, - "low": 8801, - "open": 586, - "volume": 1488301 - }, - "open": "2020-06-01T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2230, - "high": 7208, - "low": 7, - "open": 7208, - "volume": 1462131 - }, - "id": 2622592, - "non_hive": { - "close": 555, - "high": 1831, - "low": 1, - "open": 1831, - "volume": 353022 - }, - "open": "2020-06-01T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 109100, - "high": 597, - "low": 109100, - "open": 7921, - "volume": 1594849 - }, - "id": 2622626, - "non_hive": { - "close": 26630, - "high": 151, - "low": 26630, - "open": 1999, - "volume": 392360 - }, - "open": "2020-06-01T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 859, - "high": 164, - "low": 13, - "open": 4338, - "volume": 3286546 - }, - "id": 2622643, - "non_hive": { - "close": 213, - "high": 41, - "low": 3, - "open": 1084, - "volume": 792636 - }, - "open": "2020-06-01T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 391670, - "low": 49, - "open": 22839, - "volume": 7042960 - }, - "id": 2622689, - "non_hive": { - "close": 144660, - "high": 97234, - "low": 11, - "open": 5660, - "volume": 1720646 - }, - "open": "2020-06-01T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1256, - "high": 328, - "low": 5, - "open": 1301, - "volume": 4894888 - }, - "id": 2622734, - "non_hive": { - "close": 316, - "high": 83, - "low": 1, - "open": 323, - "volume": 1205104 - }, - "open": "2020-06-01T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 540687, - "high": 3046, - "low": 13, - "open": 13, - "volume": 3651907 - }, - "id": 2622780, - "non_hive": { - "close": 130269, - "high": 764, - "low": 3, - "open": 3, - "volume": 892155 - }, - "open": "2020-06-01T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28746, - "high": 28746, - "low": 42, - "open": 4560, - "volume": 6384383 - }, - "id": 2622815, - "non_hive": { - "close": 7216, - "high": 7216, - "low": 10, - "open": 1135, - "volume": 1573603 - }, - "open": "2020-06-01T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 585924, - "high": 881, - "low": 29, - "open": 363, - "volume": 6937007 - }, - "id": 2622847, - "non_hive": { - "close": 144207, - "high": 221, - "low": 7, - "open": 90, - "volume": 1703914 - }, - "open": "2020-06-01T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24354, - "high": 1097, - "low": 13, - "open": 4347, - "volume": 3838728 - }, - "id": 2622882, - "non_hive": { - "close": 5994, - "high": 275, - "low": 3, - "open": 1089, - "volume": 946791 - }, - "open": "2020-06-01T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 175, - "high": 175, - "low": 18, - "open": 7985, - "volume": 3827591 - }, - "id": 2622936, - "non_hive": { - "close": 44, - "high": 44, - "low": 4, - "open": 1999, - "volume": 943095 - }, - "open": "2020-06-01T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 198620, - "high": 649284, - "low": 6453, - "open": 68, - "volume": 5769489 - }, - "id": 2622992, - "non_hive": { - "close": 49617, - "high": 162901, - "low": 1583, - "open": 17, - "volume": 1427180 - }, - "open": "2020-06-01T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12725, - "high": 12, - "low": 15, - "open": 28036, - "volume": 6174168 - }, - "id": 2623035, - "non_hive": { - "close": 3179, - "high": 3, - "low": 3, - "open": 6992, - "volume": 1524074 - }, - "open": "2020-06-01T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7420, - "high": 144, - "low": 353, - "open": 272448, - "volume": 10769103 - }, - "id": 2623110, - "non_hive": { - "close": 2002, - "high": 40, - "low": 88, - "open": 68063, - "volume": 2731781 - }, - "open": "2020-06-01T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 203, - "high": 3, - "low": 32, - "open": 3, - "volume": 6156811 - }, - "id": 2623179, - "non_hive": { - "close": 54, - "high": 1, - "low": 8, - "open": 1, - "volume": 1621979 - }, - "open": "2020-06-01T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37940, - "high": 3121, - "low": 12, - "open": 7744, - "volume": 6526381 - }, - "id": 2623248, - "non_hive": { - "close": 10000, - "high": 830, - "low": 3, - "open": 2000, - "volume": 1692293 - }, - "open": "2020-06-01T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4323, - "high": 37, - "low": 3567, - "open": 8594, - "volume": 739838 - }, - "id": 2623301, - "non_hive": { - "close": 1147, - "high": 10, - "low": 926, - "open": 2265, - "volume": 194190 - }, - "open": "2020-06-01T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59209, - "high": 296569, - "low": 71, - "open": 3437, - "volume": 7250885 - }, - "id": 2623341, - "non_hive": { - "close": 15792, - "high": 79175, - "low": 18, - "open": 911, - "volume": 1915268 - }, - "open": "2020-06-01T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37181, - "high": 33399, - "low": 409476, - "open": 54251, - "volume": 3591992 - }, - "id": 2623395, - "non_hive": { - "close": 10000, - "high": 8984, - "low": 107369, - "open": 14466, - "volume": 950833 - }, - "open": "2020-06-01T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3984, - "high": 9600, - "low": 27, - "open": 3992, - "volume": 290337 - }, - "id": 2623432, - "non_hive": { - "close": 1075, - "high": 2592, - "low": 7, - "open": 1073, - "volume": 78216 - }, - "open": "2020-06-01T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1074, - "high": 3, - "low": 113, - "open": 3770, - "volume": 5060283 - }, - "id": 2623466, - "non_hive": { - "close": 289, - "high": 1, - "low": 29, - "open": 1018, - "volume": 1329516 - }, - "open": "2020-06-01T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 598078, - "high": 3, - "low": 5, - "open": 7431, - "volume": 1022577 - }, - "id": 2623521, - "non_hive": { - "close": 155531, - "high": 1, - "low": 1, - "open": 1999, - "volume": 268437 - }, - "open": "2020-06-02T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24183, - "high": 4968, - "low": 15, - "open": 4968, - "volume": 2247812 - }, - "id": 2623573, - "non_hive": { - "close": 6335, - "high": 1309, - "low": 3, - "open": 1309, - "volume": 583363 - }, - "open": "2020-06-02T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20220, - "high": 35536, - "low": 4, - "open": 4, - "volume": 3193256 - }, - "id": 2623606, - "non_hive": { - "close": 5071, - "high": 9210, - "low": 1, - "open": 1, - "volume": 818044 - }, - "open": "2020-06-02T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20002, - "high": 20002, - "low": 4, - "open": 1258, - "volume": 2660563 - }, - "id": 2623626, - "non_hive": { - "close": 5266, - "high": 5266, - "low": 1, - "open": 326, - "volume": 689648 - }, - "open": "2020-06-02T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1921, - "high": 34, - "low": 43, - "open": 4038, - "volume": 1969236 - }, - "id": 2623664, - "non_hive": { - "close": 507, - "high": 9, - "low": 11, - "open": 1063, - "volume": 511152 - }, - "open": "2020-06-02T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4026, - "high": 5363, - "low": 592286, - "open": 7714, - "volume": 4700751 - }, - "id": 2623695, - "non_hive": { - "close": 1069, - "high": 1426, - "low": 153532, - "open": 2000, - "volume": 1231914 - }, - "open": "2020-06-02T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 146, - "low": 26, - "open": 802, - "volume": 4640547 - }, - "id": 2623738, - "non_hive": { - "close": 3, - "high": 39, - "low": 6, - "open": 213, - "volume": 1207357 - }, - "open": "2020-06-02T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 610, - "high": 7106, - "low": 60, - "open": 7745, - "volume": 1286719 - }, - "id": 2623795, - "non_hive": { - "close": 159, - "high": 1875, - "low": 15, - "open": 1997, - "volume": 331023 - }, - "open": "2020-06-02T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5129, - "high": 49, - "low": 37, - "open": 13274, - "volume": 3360154 - }, - "id": 2623826, - "non_hive": { - "close": 1342, - "high": 13, - "low": 9, - "open": 3498, - "volume": 865583 - }, - "open": "2020-06-02T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 892, - "high": 29798, - "low": 12, - "open": 12, - "volume": 3649292 - }, - "id": 2623869, - "non_hive": { - "close": 233, - "high": 7794, - "low": 3, - "open": 3, - "volume": 935804 - }, - "open": "2020-06-02T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59, - "high": 75952, - "low": 59, - "open": 8789, - "volume": 1917750 - }, - "id": 2623907, - "non_hive": { - "close": 15, - "high": 20000, - "low": 15, - "open": 2294, - "volume": 491752 - }, - "open": "2020-06-02T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23597, - "high": 23597, - "low": 28, - "open": 4641, - "volume": 2930110 - }, - "id": 2623936, - "non_hive": { - "close": 6219, - "high": 6219, - "low": 7, - "open": 1179, - "volume": 752859 - }, - "open": "2020-06-02T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28953, - "high": 34, - "low": 12, - "open": 34, - "volume": 5331687 - }, - "id": 2623968, - "non_hive": { - "close": 7573, - "high": 9, - "low": 3, - "open": 9, - "volume": 1376218 - }, - "open": "2020-06-02T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 380802, - "high": 3, - "low": 53, - "open": 22645, - "volume": 3992518 - }, - "id": 2624012, - "non_hive": { - "close": 96723, - "high": 1, - "low": 13, - "open": 5778, - "volume": 1021446 - }, - "open": "2020-06-02T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6376, - "high": 6454, - "low": 10136, - "open": 830, - "volume": 1652275 - }, - "id": 2624051, - "non_hive": { - "close": 1657, - "high": 1680, - "low": 2565, - "open": 216, - "volume": 420265 - }, - "open": "2020-06-02T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12981, - "high": 10859, - "low": 297466, - "open": 47722, - "volume": 8288795 - }, - "id": 2624086, - "non_hive": { - "close": 3323, - "high": 2816, - "low": 71663, - "open": 12090, - "volume": 2082062 - }, - "open": "2020-06-02T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42358, - "high": 1748, - "low": 595898, - "open": 20087, - "volume": 5192714 - }, - "id": 2624134, - "non_hive": { - "close": 10853, - "high": 449, - "low": 143814, - "open": 5140, - "volume": 1277055 - }, - "open": "2020-06-02T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12118, - "high": 46, - "low": 541337, - "open": 11000, - "volume": 3728238 - }, - "id": 2624191, - "non_hive": { - "close": 3079, - "high": 12, - "low": 130147, - "open": 2674, - "volume": 904955 - }, - "open": "2020-06-02T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1472, - "high": 4559, - "low": 13, - "open": 24839, - "volume": 3113139 - }, - "id": 2624234, - "non_hive": { - "close": 372, - "high": 1155, - "low": 3, - "open": 6000, - "volume": 756090 - }, - "open": "2020-06-02T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6963, - "high": 15865, - "low": 84, - "open": 7897, - "volume": 3285083 - }, - "id": 2624274, - "non_hive": { - "close": 1726, - "high": 4016, - "low": 20, - "open": 1999, - "volume": 801367 - }, - "open": "2020-06-02T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3091, - "high": 3091, - "low": 298372, - "open": 6728, - "volume": 2612669 - }, - "id": 2624321, - "non_hive": { - "close": 781, - "high": 781, - "low": 71922, - "open": 1654, - "volume": 635356 - }, - "open": "2020-06-02T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5003, - "high": 932, - "low": 2401, - "open": 8190, - "volume": 1898229 - }, - "id": 2624367, - "non_hive": { - "close": 1229, - "high": 233, - "low": 579, - "open": 2000, - "volume": 462882 - }, - "open": "2020-06-02T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 75, - "low": 7, - "open": 977, - "volume": 4920333 - }, - "id": 2624404, - "non_hive": { - "close": 3, - "high": 19, - "low": 1, - "open": 240, - "volume": 1206335 - }, - "open": "2020-06-02T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 373, - "high": 87150, - "low": 273, - "open": 6551, - "volume": 4932474 - }, - "id": 2624458, - "non_hive": { - "close": 91, - "high": 21922, - "low": 66, - "open": 1593, - "volume": 1208807 - }, - "open": "2020-06-02T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13755, - "high": 390677, - "low": 13, - "open": 390677, - "volume": 1281646 - }, - "id": 2624513, - "non_hive": { - "close": 3434, - "high": 98000, - "low": 3, - "open": 98000, - "volume": 321275 - }, - "open": "2020-06-03T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3384, - "high": 132181, - "low": 7, - "open": 132181, - "volume": 1973492 - }, - "id": 2624551, - "non_hive": { - "close": 832, - "high": 33374, - "low": 1, - "open": 33374, - "volume": 489532 - }, - "open": "2020-06-03T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1250, - "high": 2509, - "low": 27723, - "open": 7904, - "volume": 2632081 - }, - "id": 2624583, - "non_hive": { - "close": 315, - "high": 634, - "low": 6764, - "open": 1992, - "volume": 651326 - }, - "open": "2020-06-03T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 678, - "high": 273, - "low": 9299, - "open": 3269, - "volume": 2532332 - }, - "id": 2624623, - "non_hive": { - "close": 171, - "high": 69, - "low": 2243, - "open": 822, - "volume": 618476 - }, - "open": "2020-06-03T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 311, - "high": 12239, - "low": 1000, - "open": 12239, - "volume": 2603191 - }, - "id": 2624656, - "non_hive": { - "close": 75, - "high": 3084, - "low": 241, - "open": 3084, - "volume": 635817 - }, - "open": "2020-06-03T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56878, - "high": 42680, - "low": 32, - "open": 7975, - "volume": 4010694 - }, - "id": 2624693, - "non_hive": { - "close": 13883, - "high": 10701, - "low": 7, - "open": 1999, - "volume": 977478 - }, - "open": "2020-06-03T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15, - "high": 697, - "low": 15, - "open": 8026, - "volume": 4346397 - }, - "id": 2624749, - "non_hive": { - "close": 3, - "high": 176, - "low": 3, - "open": 1999, - "volume": 1063247 - }, - "open": "2020-06-03T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 202, - "high": 23, - "low": 28, - "open": 23, - "volume": 3686631 - }, - "id": 2624805, - "non_hive": { - "close": 50, - "high": 6, - "low": 6, - "open": 6, - "volume": 894308 - }, - "open": "2020-06-03T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14989, - "high": 1074, - "low": 588335, - "open": 8251, - "volume": 3671846 - }, - "id": 2624844, - "non_hive": { - "close": 3698, - "high": 265, - "low": 142496, - "open": 1999, - "volume": 895774 - }, - "open": "2020-06-03T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 299997, - "high": 201, - "low": 13, - "open": 13, - "volume": 1979907 - }, - "id": 2624874, - "non_hive": { - "close": 72812, - "high": 50, - "low": 3, - "open": 3, - "volume": 481326 - }, - "open": "2020-06-03T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 4, - "low": 42, - "open": 14933, - "volume": 2064291 - }, - "id": 2624900, - "non_hive": { - "close": 146701, - "high": 1, - "low": 10, - "open": 3703, - "volume": 505731 - }, - "open": "2020-06-03T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55455, - "high": 426, - "low": 32, - "open": 14939, - "volume": 3734935 - }, - "id": 2624946, - "non_hive": { - "close": 13780, - "high": 106, - "low": 7, - "open": 3711, - "volume": 916957 - }, - "open": "2020-06-03T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24942, - "high": 24942, - "low": 13, - "open": 549913, - "volume": 3889520 - }, - "id": 2624989, - "non_hive": { - "close": 6198, - "high": 6198, - "low": 3, - "open": 135176, - "volume": 956930 - }, - "open": "2020-06-03T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 555, - "low": 66, - "open": 3489, - "volume": 4064129 - }, - "id": 2625027, - "non_hive": { - "close": 147600, - "high": 138, - "low": 16, - "open": 867, - "volume": 1000452 - }, - "open": "2020-06-03T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42309, - "high": 4817, - "low": 568849, - "open": 467, - "volume": 4653320 - }, - "id": 2625070, - "non_hive": { - "close": 10497, - "high": 1217, - "low": 139692, - "open": 116, - "volume": 1150055 - }, - "open": "2020-06-03T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9, - "high": 153, - "low": 9, - "open": 842, - "volume": 11729364 - }, - "id": 2625135, - "non_hive": { - "close": 2, - "high": 40, - "low": 2, - "open": 209, - "volume": 2951029 - }, - "open": "2020-06-03T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 183984, - "low": 11, - "open": 4132, - "volume": 4477945 - }, - "id": 2625202, - "non_hive": { - "close": 254, - "high": 47158, - "low": 2, - "open": 1050, - "volume": 1129099 - }, - "open": "2020-06-03T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1089, - "high": 129, - "low": 30, - "open": 4730, - "volume": 1033823 - }, - "id": 2625253, - "non_hive": { - "close": 277, - "high": 33, - "low": 7, - "open": 1205, - "volume": 260608 - }, - "open": "2020-06-03T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 869, - "high": 4428, - "low": 13, - "open": 4428, - "volume": 3085582 - }, - "id": 2625294, - "non_hive": { - "close": 219, - "high": 1124, - "low": 3, - "open": 1124, - "volume": 766376 - }, - "open": "2020-06-03T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4155, - "high": 67, - "low": 342833, - "open": 7948, - "volume": 1623665 - }, - "id": 2625332, - "non_hive": { - "close": 1045, - "high": 17, - "low": 85050, - "open": 1999, - "volume": 404145 - }, - "open": "2020-06-03T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13768, - "high": 3, - "low": 25, - "open": 14131, - "volume": 216506 - }, - "id": 2625383, - "non_hive": { - "close": 3442, - "high": 1, - "low": 6, - "open": 3555, - "volume": 54748 - }, - "open": "2020-06-03T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2436, - "high": 7248, - "low": 297, - "open": 7248, - "volume": 675180 - }, - "id": 2625421, - "non_hive": { - "close": 605, - "high": 1841, - "low": 73, - "open": 1841, - "volume": 167156 - }, - "open": "2020-06-03T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3814, - "high": 24, - "low": 29, - "open": 3729, - "volume": 163931 - }, - "id": 2625446, - "non_hive": { - "close": 953, - "high": 6, - "low": 7, - "open": 932, - "volume": 40701 - }, - "open": "2020-06-03T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6032, - "high": 64, - "low": 13, - "open": 13, - "volume": 276806 - }, - "id": 2625491, - "non_hive": { - "close": 1507, - "high": 16, - "low": 3, - "open": 3, - "volume": 69147 - }, - "open": "2020-06-03T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2701, - "high": 448, - "low": 13, - "open": 448, - "volume": 625461 - }, - "id": 2625537, - "non_hive": { - "close": 671, - "high": 112, - "low": 3, - "open": 112, - "volume": 154142 - }, - "open": "2020-06-04T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 719, - "high": 12861, - "low": 1024, - "open": 1024, - "volume": 27465 - }, - "id": 2625565, - "non_hive": { - "close": 179, - "high": 3207, - "low": 254, - "open": 254, - "volume": 6847 - }, - "open": "2020-06-04T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13155, - "high": 316, - "low": 13318, - "open": 44030, - "volume": 3967502 - }, - "id": 2625576, - "non_hive": { - "close": 3288, - "high": 79, - "low": 3276, - "open": 11000, - "volume": 977800 - }, - "open": "2020-06-04T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19399, - "high": 468, - "low": 377, - "open": 1977, - "volume": 1988718 - }, - "id": 2625608, - "non_hive": { - "close": 4849, - "high": 117, - "low": 92, - "open": 494, - "volume": 489868 - }, - "open": "2020-06-04T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 530871, - "high": 1316, - "low": 41, - "open": 44020, - "volume": 1383128 - }, - "id": 2625645, - "non_hive": { - "close": 131673, - "high": 329, - "low": 10, - "open": 11000, - "volume": 342984 - }, - "open": "2020-06-04T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1036, - "high": 7, - "low": 5, - "open": 300000, - "volume": 4324106 - }, - "id": 2625674, - "non_hive": { - "close": 264, - "high": 2, - "low": 1, - "open": 74424, - "volume": 1077921 - }, - "open": "2020-06-04T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1797, - "high": 1797, - "low": 12, - "open": 203, - "volume": 160590 - }, - "id": 2625701, - "non_hive": { - "close": 466, - "high": 466, - "low": 3, - "open": 51, - "volume": 41425 - }, - "open": "2020-06-04T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13327, - "high": 1651, - "low": 102, - "open": 197, - "volume": 49852 - }, - "id": 2625741, - "non_hive": { - "close": 3447, - "high": 428, - "low": 26, - "open": 51, - "volume": 12808 - }, - "open": "2020-06-04T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 933, - "high": 233936, - "low": 13590, - "open": 769, - "volume": 4010094 - }, - "id": 2625756, - "non_hive": { - "close": 238, - "high": 60475, - "low": 3435, - "open": 197, - "volume": 1020451 - }, - "open": "2020-06-04T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2799, - "high": 62, - "low": 12, - "open": 12, - "volume": 1568471 - }, - "id": 2625785, - "non_hive": { - "close": 711, - "high": 16, - "low": 3, - "open": 3, - "volume": 397793 - }, - "open": "2020-06-04T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 406, - "high": 102, - "low": 44, - "open": 14554, - "volume": 698276 - }, - "id": 2625817, - "non_hive": { - "close": 103, - "high": 26, - "low": 11, - "open": 3696, - "volume": 177170 - }, - "open": "2020-06-04T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 210148, - "high": 210148, - "low": 32, - "open": 13828, - "volume": 2130777 - }, - "id": 2625852, - "non_hive": { - "close": 53798, - "high": 53798, - "low": 8, - "open": 3502, - "volume": 540518 - }, - "open": "2020-06-04T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 120, - "high": 7523, - "low": 57, - "open": 11480, - "volume": 3431620 - }, - "id": 2625883, - "non_hive": { - "close": 30, - "high": 1926, - "low": 14, - "open": 2939, - "volume": 865966 - }, - "open": "2020-06-04T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 193749, - "high": 160, - "low": 601, - "open": 14471, - "volume": 1271832 - }, - "id": 2625922, - "non_hive": { - "close": 49212, - "high": 41, - "low": 150, - "open": 3689, - "volume": 323147 - }, - "open": "2020-06-04T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 173000, - "high": 70, - "low": 17601, - "open": 14183, - "volume": 478865 - }, - "id": 2625955, - "non_hive": { - "close": 44242, - "high": 18, - "low": 4417, - "open": 3602, - "volume": 121875 - }, - "open": "2020-06-04T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2637, - "high": 3, - "low": 199, - "open": 2822, - "volume": 776324 - }, - "id": 2625996, - "non_hive": { - "close": 695, - "high": 1, - "low": 50, - "open": 722, - "volume": 199291 - }, - "open": "2020-06-04T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 155, - "high": 79, - "low": 11, - "open": 6628, - "volume": 1745834 - }, - "id": 2626054, - "non_hive": { - "close": 40, - "high": 21, - "low": 2, - "open": 1727, - "volume": 450200 - }, - "open": "2020-06-04T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8000, - "high": 37867, - "low": 100, - "open": 819, - "volume": 1644126 - }, - "id": 2626100, - "non_hive": { - "close": 2024, - "high": 9936, - "low": 25, - "open": 214, - "volume": 418139 - }, - "open": "2020-06-04T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 801, - "high": 64, - "low": 12, - "open": 18007, - "volume": 441091 - }, - "id": 2626139, - "non_hive": { - "close": 210, - "high": 17, - "low": 3, - "open": 4679, - "volume": 115472 - }, - "open": "2020-06-04T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13402, - "high": 34, - "low": 10, - "open": 7874, - "volume": 4090001 - }, - "id": 2626170, - "non_hive": { - "close": 3477, - "high": 9, - "low": 2, - "open": 2000, - "volume": 1038132 - }, - "open": "2020-06-04T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 381, - "high": 893, - "low": 17, - "open": 3000, - "volume": 2052103 - }, - "id": 2626226, - "non_hive": { - "close": 100, - "high": 235, - "low": 4, - "open": 778, - "volume": 532088 - }, - "open": "2020-06-04T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 34996, - "low": 7, - "open": 7721, - "volume": 2700257 - }, - "id": 2626273, - "non_hive": { - "close": 1, - "high": 9165, - "low": 1, - "open": 2000, - "volume": 694475 - }, - "open": "2020-06-04T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6135, - "high": 6983, - "low": 114, - "open": 7811, - "volume": 775648 - }, - "id": 2626330, - "non_hive": { - "close": 1589, - "high": 1815, - "low": 28, - "open": 2000, - "volume": 198753 - }, - "open": "2020-06-04T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3076, - "high": 3076, - "low": 12, - "open": 12, - "volume": 5200899 - }, - "id": 2626355, - "non_hive": { - "close": 818, - "high": 818, - "low": 3, - "open": 3, - "volume": 1360637 - }, - "open": "2020-06-04T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8, - "high": 29992, - "low": 12, - "open": 394, - "volume": 91742 - }, - "id": 2626410, - "non_hive": { - "close": 2, - "high": 8038, - "low": 3, - "open": 105, - "volume": 24453 - }, - "open": "2020-06-05T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4076, - "high": 12588, - "low": 584633, - "open": 2000, - "volume": 1843190 - }, - "id": 2626449, - "non_hive": { - "close": 1100, - "high": 3399, - "low": 152134, - "open": 539, - "volume": 482186 - }, - "open": "2020-06-05T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2968, - "high": 300, - "low": 22, - "open": 5813, - "volume": 690975 - }, - "id": 2626471, - "non_hive": { - "close": 774, - "high": 81, - "low": 5, - "open": 1568, - "volume": 180274 - }, - "open": "2020-06-05T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 472, - "high": 456, - "low": 472, - "open": 456, - "volume": 2138759 - }, - "id": 2626510, - "non_hive": { - "close": 122, - "high": 123, - "low": 122, - "open": 123, - "volume": 560048 - }, - "open": "2020-06-05T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16322, - "high": 16322, - "low": 4, - "open": 2597, - "volume": 3050715 - }, - "id": 2626537, - "non_hive": { - "close": 4456, - "high": 4456, - "low": 1, - "open": 694, - "volume": 821774 - }, - "open": "2020-06-05T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1560, - "high": 470734, - "low": 24, - "open": 7327, - "volume": 734093 - }, - "id": 2626580, - "non_hive": { - "close": 429, - "high": 129452, - "low": 6, - "open": 1999, - "volume": 201054 - }, - "open": "2020-06-05T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1080, - "high": 12153, - "low": 592593, - "open": 9848, - "volume": 1232120 - }, - "id": 2626609, - "non_hive": { - "close": 296, - "high": 3342, - "low": 156485, - "open": 2708, - "volume": 325767 - }, - "open": "2020-06-05T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35579, - "high": 21889, - "low": 1088, - "open": 1018, - "volume": 78075 - }, - "id": 2626636, - "non_hive": { - "close": 9765, - "high": 6014, - "low": 285, - "open": 279, - "volume": 21218 - }, - "open": "2020-06-05T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 672, - "high": 94951, - "low": 672, - "open": 94951, - "volume": 102623 - }, - "id": 2626648, - "non_hive": { - "close": 181, - "high": 26015, - "low": 181, - "open": 26015, - "volume": 28087 - }, - "open": "2020-06-05T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43, - "high": 115, - "low": 43, - "open": 13927, - "volume": 88159 - }, - "id": 2626661, - "non_hive": { - "close": 11, - "high": 31, - "low": 11, - "open": 3746, - "volume": 23711 - }, - "open": "2020-06-05T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1379, - "high": 319, - "low": 3991, - "open": 319, - "volume": 15389 - }, - "id": 2626685, - "non_hive": { - "close": 371, - "high": 86, - "low": 1073, - "open": 86, - "volume": 4139 - }, - "open": "2020-06-05T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 691, - "high": 29, - "low": 9971, - "open": 5718, - "volume": 55778 - }, - "id": 2626701, - "non_hive": { - "close": 186, - "high": 8, - "low": 2681, - "open": 1538, - "volume": 15001 - }, - "open": "2020-06-05T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8335, - "high": 866, - "low": 30087, - "open": 11748, - "volume": 106399 - }, - "id": 2626717, - "non_hive": { - "close": 2242, - "high": 233, - "low": 7973, - "open": 3160, - "volume": 28411 - }, - "open": "2020-06-05T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49930, - "high": 1145, - "low": 131, - "open": 1145, - "volume": 98334 - }, - "id": 2626737, - "non_hive": { - "close": 13426, - "high": 308, - "low": 35, - "open": 308, - "volume": 26444 - }, - "open": "2020-06-05T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17009, - "high": 1751, - "low": 34, - "open": 91792, - "volume": 327957 - }, - "id": 2626754, - "non_hive": { - "close": 4575, - "high": 471, - "low": 9, - "open": 24682, - "volume": 88201 - }, - "open": "2020-06-05T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10942, - "high": 462, - "low": 161617, - "open": 4647, - "volume": 557577 - }, - "id": 2626789, - "non_hive": { - "close": 2954, - "high": 125, - "low": 43468, - "open": 1250, - "volume": 150264 - }, - "open": "2020-06-05T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 92, - "high": 92, - "low": 14982, - "open": 18805, - "volume": 2467217 - }, - "id": 2626821, - "non_hive": { - "close": 25, - "high": 25, - "low": 3955, - "open": 5077, - "volume": 654573 - }, - "open": "2020-06-05T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 454, - "high": 286, - "low": 180, - "open": 2190, - "volume": 2831963 - }, - "id": 2626851, - "non_hive": { - "close": 122, - "high": 77, - "low": 47, - "open": 589, - "volume": 749049 - }, - "open": "2020-06-05T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12124, - "high": 10374, - "low": 8, - "open": 10374, - "volume": 881735 - }, - "id": 2626885, - "non_hive": { - "close": 3232, - "high": 2780, - "low": 2, - "open": 2780, - "volume": 235092 - }, - "open": "2020-06-05T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13653, - "high": 22, - "low": 61, - "open": 41941, - "volume": 230762 - }, - "id": 2626920, - "non_hive": { - "close": 3611, - "high": 6, - "low": 15, - "open": 11038, - "volume": 61123 - }, - "open": "2020-06-05T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6894, - "high": 56, - "low": 12, - "open": 75626, - "volume": 618818 - }, - "id": 2626947, - "non_hive": { - "close": 1834, - "high": 15, - "low": 3, - "open": 20001, - "volume": 163737 - }, - "open": "2020-06-05T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 85055, - "high": 18, - "low": 16526, - "open": 5632, - "volume": 789796 - }, - "id": 2626985, - "non_hive": { - "close": 22623, - "high": 5, - "low": 4309, - "open": 1498, - "volume": 207205 - }, - "open": "2020-06-05T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3605, - "high": 263, - "low": 612, - "open": 1211, - "volume": 670138 - }, - "id": 2627023, - "non_hive": { - "close": 954, - "high": 70, - "low": 158, - "open": 322, - "volume": 174645 - }, - "open": "2020-06-05T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17971, - "high": 51614, - "low": 6, - "open": 12, - "volume": 3238330 - }, - "id": 2627051, - "non_hive": { - "close": 4654, - "high": 13720, - "low": 1, - "open": 3, - "volume": 842037 - }, - "open": "2020-06-05T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 417, - "high": 174, - "low": 51, - "open": 4115, - "volume": 3078042 - }, - "id": 2627101, - "non_hive": { - "close": 109, - "high": 46, - "low": 13, - "open": 1066, - "volume": 788824 - }, - "open": "2020-06-06T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 610, - "high": 1819, - "low": 386, - "open": 1819, - "volume": 107117 - }, - "id": 2627145, - "non_hive": { - "close": 157, - "high": 474, - "low": 98, - "open": 474, - "volume": 27558 - }, - "open": "2020-06-06T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3247, - "high": 3247, - "low": 4, - "open": 1653, - "volume": 4221899 - }, - "id": 2627165, - "non_hive": { - "close": 845, - "high": 845, - "low": 1, - "open": 425, - "volume": 1095271 - }, - "open": "2020-06-06T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 635064, - "high": 42, - "low": 43, - "open": 2305, - "volume": 5807805 - }, - "id": 2627198, - "non_hive": { - "close": 165238, - "high": 11, - "low": 10, - "open": 600, - "volume": 1499023 - }, - "open": "2020-06-06T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 563872, - "high": 466, - "low": 321437, - "open": 3251, - "volume": 5636724 - }, - "id": 2627235, - "non_hive": { - "close": 148637, - "high": 123, - "low": 82144, - "open": 846, - "volume": 1460224 - }, - "open": "2020-06-06T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 189, - "high": 189, - "low": 595828, - "open": 600000, - "volume": 3617292 - }, - "id": 2627276, - "non_hive": { - "close": 50, - "high": 50, - "low": 151986, - "open": 154908, - "volume": 926236 - }, - "open": "2020-06-06T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 410, - "high": 22291, - "low": 574573, - "open": 3334, - "volume": 3711423 - }, - "id": 2627304, - "non_hive": { - "close": 108, - "high": 5876, - "low": 146835, - "open": 867, - "volume": 949383 - }, - "open": "2020-06-06T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 73310, - "high": 23250, - "low": 323517, - "open": 23250, - "volume": 3127360 - }, - "id": 2627335, - "non_hive": { - "close": 18474, - "high": 6116, - "low": 81525, - "open": 6116, - "volume": 798105 - }, - "open": "2020-06-06T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 215, - "high": 398057, - "low": 26, - "open": 8326, - "volume": 2045475 - }, - "id": 2627365, - "non_hive": { - "close": 55, - "high": 103307, - "low": 6, - "open": 2123, - "volume": 521328 - }, - "open": "2020-06-06T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22382, - "high": 22382, - "low": 42, - "open": 1219, - "volume": 110466 - }, - "id": 2627416, - "non_hive": { - "close": 5794, - "high": 5794, - "low": 10, - "open": 309, - "volume": 28239 - }, - "open": "2020-06-06T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 192762, - "high": 70, - "low": 5, - "open": 78138, - "volume": 736804 - }, - "id": 2627456, - "non_hive": { - "close": 49156, - "high": 18, - "low": 1, - "open": 19996, - "volume": 187433 - }, - "open": "2020-06-06T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 436, - "high": 158, - "low": 262297, - "open": 10338, - "volume": 2943231 - }, - "id": 2627489, - "non_hive": { - "close": 113, - "high": 41, - "low": 66679, - "open": 2641, - "volume": 751513 - }, - "open": "2020-06-06T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12084, - "high": 30, - "low": 51173, - "open": 706, - "volume": 1397544 - }, - "id": 2627537, - "non_hive": { - "close": 3123, - "high": 8, - "low": 12947, - "open": 183, - "volume": 355995 - }, - "open": "2020-06-06T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 102, - "high": 220, - "low": 40, - "open": 136933, - "volume": 3438711 - }, - "id": 2627577, - "non_hive": { - "close": 26, - "high": 57, - "low": 10, - "open": 35364, - "volume": 878476 - }, - "open": "2020-06-06T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 853, - "high": 93, - "low": 19, - "open": 93, - "volume": 2202573 - }, - "id": 2627624, - "non_hive": { - "close": 220, - "high": 24, - "low": 4, - "open": 24, - "volume": 560698 - }, - "open": "2020-06-06T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3001, - "high": 27, - "low": 8501, - "open": 391, - "volume": 2656887 - }, - "id": 2627674, - "non_hive": { - "close": 768, - "high": 7, - "low": 2151, - "open": 99, - "volume": 673600 - }, - "open": "2020-06-06T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3346, - "high": 809, - "low": 173381, - "open": 114, - "volume": 1229576 - }, - "id": 2627723, - "non_hive": { - "close": 853, - "high": 207, - "low": 43691, - "open": 29, - "volume": 311936 - }, - "open": "2020-06-06T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11584, - "high": 7764, - "low": 62, - "open": 4442, - "volume": 2732160 - }, - "id": 2627757, - "non_hive": { - "close": 2946, - "high": 1986, - "low": 15, - "open": 1130, - "volume": 691051 - }, - "open": "2020-06-06T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39896, - "high": 212, - "low": 10369, - "open": 212, - "volume": 870856 - }, - "id": 2627805, - "non_hive": { - "close": 9975, - "high": 54, - "low": 2592, - "open": 54, - "volume": 218005 - }, - "open": "2020-06-06T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3661, - "high": 3661, - "low": 85, - "open": 4884, - "volume": 266667 - }, - "id": 2627857, - "non_hive": { - "close": 919, - "high": 919, - "low": 21, - "open": 1221, - "volume": 66873 - }, - "open": "2020-06-06T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41, - "high": 1278, - "low": 66, - "open": 13468, - "volume": 164278 - }, - "id": 2627898, - "non_hive": { - "close": 10, - "high": 321, - "low": 16, - "open": 3380, - "volume": 41218 - }, - "open": "2020-06-06T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9849, - "high": 398, - "low": 10846, - "open": 13381, - "volume": 361376 - }, - "id": 2627938, - "non_hive": { - "close": 2472, - "high": 100, - "low": 2720, - "open": 3358, - "volume": 90701 - }, - "open": "2020-06-06T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 71, - "high": 71, - "low": 28, - "open": 12498, - "volume": 14065 - }, - "id": 2627990, - "non_hive": { - "close": 18, - "high": 18, - "low": 7, - "open": 3137, - "volume": 3530 - }, - "open": "2020-06-06T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1003, - "high": 3, - "low": 71, - "open": 12, - "volume": 2349203 - }, - "id": 2628003, - "non_hive": { - "close": 258, - "high": 1, - "low": 17, - "open": 3, - "volume": 591725 - }, - "open": "2020-06-06T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 341, - "high": 341, - "low": 71, - "open": 1678, - "volume": 873888 - }, - "id": 2628054, - "non_hive": { - "close": 88, - "high": 88, - "low": 17, - "open": 432, - "volume": 221213 - }, - "open": "2020-06-07T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1002, - "high": 222, - "low": 24, - "open": 24, - "volume": 125291 - }, - "id": 2628081, - "non_hive": { - "close": 255, - "high": 57, - "low": 6, - "open": 6, - "volume": 32031 - }, - "open": "2020-06-07T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 39, - "low": 10, - "open": 225, - "volume": 1275243 - }, - "id": 2628099, - "non_hive": { - "close": 3, - "high": 10, - "low": 2, - "open": 57, - "volume": 320129 - }, - "open": "2020-06-07T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 246, - "high": 273, - "low": 24, - "open": 3109, - "volume": 1133593 - }, - "id": 2628132, - "non_hive": { - "close": 63, - "high": 70, - "low": 6, - "open": 791, - "volume": 288485 - }, - "open": "2020-06-07T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 429, - "high": 269, - "low": 17, - "open": 10000, - "volume": 1014120 - }, - "id": 2628167, - "non_hive": { - "close": 110, - "high": 69, - "low": 4, - "open": 2559, - "volume": 259029 - }, - "open": "2020-06-07T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9634, - "high": 17697, - "low": 24, - "open": 8626, - "volume": 2386216 - }, - "id": 2628197, - "non_hive": { - "close": 2484, - "high": 4563, - "low": 6, - "open": 2208, - "volume": 611333 - }, - "open": "2020-06-07T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 934, - "high": 147, - "low": 24, - "open": 4343, - "volume": 159601 - }, - "id": 2628238, - "non_hive": { - "close": 241, - "high": 38, - "low": 6, - "open": 1120, - "volume": 41153 - }, - "open": "2020-06-07T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2047, - "high": 387, - "low": 24, - "open": 484, - "volume": 79008 - }, - "id": 2628278, - "non_hive": { - "close": 528, - "high": 100, - "low": 6, - "open": 125, - "volume": 20372 - }, - "open": "2020-06-07T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50, - "high": 73, - "low": 50, - "open": 73, - "volume": 1303418 - }, - "id": 2628310, - "non_hive": { - "close": 12, - "high": 19, - "low": 12, - "open": 19, - "volume": 334035 - }, - "open": "2020-06-07T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 1612, - "low": 24, - "open": 10000, - "volume": 840784 - }, - "id": 2628366, - "non_hive": { - "close": 6, - "high": 416, - "low": 6, - "open": 2561, - "volume": 215056 - }, - "open": "2020-06-07T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 674, - "low": 24, - "open": 16281, - "volume": 124363 - }, - "id": 2628388, - "non_hive": { - "close": 6, - "high": 174, - "low": 6, - "open": 4199, - "volume": 32072 - }, - "open": "2020-06-07T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1424, - "high": 38, - "low": 24, - "open": 14670, - "volume": 2216691 - }, - "id": 2628420, - "non_hive": { - "close": 367, - "high": 10, - "low": 6, - "open": 3783, - "volume": 564360 - }, - "open": "2020-06-07T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5101, - "high": 1882, - "low": 41, - "open": 1882, - "volume": 3663633 - }, - "id": 2628471, - "non_hive": { - "close": 1308, - "high": 485, - "low": 10, - "open": 485, - "volume": 928530 - }, - "open": "2020-06-07T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 103, - "high": 6061, - "low": 90, - "open": 7867, - "volume": 3741493 - }, - "id": 2628511, - "non_hive": { - "close": 26, - "high": 1563, - "low": 22, - "open": 1994, - "volume": 949590 - }, - "open": "2020-06-07T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32, - "high": 69, - "low": 51, - "open": 69, - "volume": 3694566 - }, - "id": 2628559, - "non_hive": { - "close": 8, - "high": 18, - "low": 12, - "open": 18, - "volume": 933676 - }, - "open": "2020-06-07T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6383, - "high": 1073, - "low": 52, - "open": 16649, - "volume": 2129563 - }, - "id": 2628624, - "non_hive": { - "close": 1615, - "high": 276, - "low": 12, - "open": 4276, - "volume": 535023 - }, - "open": "2020-06-07T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5000, - "high": 104, - "low": 5, - "open": 137, - "volume": 3082204 - }, - "id": 2628670, - "non_hive": { - "close": 1262, - "high": 27, - "low": 1, - "open": 35, - "volume": 773104 - }, - "open": "2020-06-07T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28, - "high": 2101, - "low": 173, - "open": 2101, - "volume": 2610070 - }, - "id": 2628730, - "non_hive": { - "close": 7, - "high": 541, - "low": 43, - "open": 541, - "volume": 653581 - }, - "open": "2020-06-07T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20601, - "high": 20601, - "low": 7, - "open": 7895, - "volume": 203591 - }, - "id": 2628783, - "non_hive": { - "close": 5298, - "high": 5298, - "low": 1, - "open": 1999, - "volume": 51905 - }, - "open": "2020-06-07T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 75372, - "high": 3275, - "low": 6, - "open": 13830, - "volume": 161687 - }, - "id": 2628831, - "non_hive": { - "close": 19218, - "high": 843, - "low": 1, - "open": 3559, - "volume": 41308 - }, - "open": "2020-06-07T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13503, - "high": 70, - "low": 89, - "open": 86, - "volume": 824023 - }, - "id": 2628869, - "non_hive": { - "close": 3383, - "high": 18, - "low": 22, - "open": 22, - "volume": 208448 - }, - "open": "2020-06-07T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 339, - "high": 286, - "low": 19, - "open": 1101, - "volume": 183277 - }, - "id": 2628928, - "non_hive": { - "close": 85, - "high": 73, - "low": 4, - "open": 281, - "volume": 46618 - }, - "open": "2020-06-07T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5940, - "high": 70, - "low": 13, - "open": 841, - "volume": 807747 - }, - "id": 2628955, - "non_hive": { - "close": 1514, - "high": 18, - "low": 3, - "open": 214, - "volume": 204718 - }, - "open": "2020-06-07T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10929, - "high": 1480, - "low": 25, - "open": 12, - "volume": 1225581 - }, - "id": 2629008, - "non_hive": { - "close": 2759, - "high": 377, - "low": 6, - "open": 3, - "volume": 309280 - }, - "open": "2020-06-07T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6181, - "high": 3, - "low": 24, - "open": 1445, - "volume": 2495213 - }, - "id": 2629041, - "non_hive": { - "close": 1557, - "high": 1, - "low": 6, - "open": 365, - "volume": 629633 - }, - "open": "2020-06-08T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4202, - "high": 1737, - "low": 5, - "open": 3385, - "volume": 2027803 - }, - "id": 2629083, - "non_hive": { - "close": 1061, - "high": 439, - "low": 1, - "open": 854, - "volume": 512160 - }, - "open": "2020-06-08T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 229, - "low": 4, - "open": 4, - "volume": 582309 - }, - "id": 2629123, - "non_hive": { - "close": 3, - "high": 58, - "low": 1, - "open": 1, - "volume": 147110 - }, - "open": "2020-06-08T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 547795, - "high": 3, - "low": 361, - "open": 5846, - "volume": 6414636 - }, - "id": 2629150, - "non_hive": { - "close": 136951, - "high": 1, - "low": 90, - "open": 1477, - "volume": 1616144 - }, - "open": "2020-06-08T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6568, - "high": 157554, - "low": 18, - "open": 7998, - "volume": 3643781 - }, - "id": 2629199, - "non_hive": { - "close": 1658, - "high": 39822, - "low": 4, - "open": 2000, - "volume": 911533 - }, - "open": "2020-06-08T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 491, - "high": 395, - "low": 24, - "open": 10748, - "volume": 193681 - }, - "id": 2629234, - "non_hive": { - "close": 124, - "high": 100, - "low": 6, - "open": 2713, - "volume": 48910 - }, - "open": "2020-06-08T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6850, - "high": 43, - "low": 24, - "open": 13837, - "volume": 64253 - }, - "id": 2629264, - "non_hive": { - "close": 1730, - "high": 11, - "low": 6, - "open": 3494, - "volume": 16226 - }, - "open": "2020-06-08T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 118865, - "high": 19, - "low": 24, - "open": 13837, - "volume": 316025 - }, - "id": 2629304, - "non_hive": { - "close": 30001, - "high": 5, - "low": 6, - "open": 3494, - "volume": 79802 - }, - "open": "2020-06-08T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 930, - "low": 25, - "open": 2657, - "volume": 1815199 - }, - "id": 2629354, - "non_hive": { - "close": 3, - "high": 235, - "low": 6, - "open": 671, - "volume": 453859 - }, - "open": "2020-06-08T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7313, - "high": 47, - "low": 1150, - "open": 3414, - "volume": 826273 - }, - "id": 2629383, - "non_hive": { - "close": 1850, - "high": 12, - "low": 287, - "open": 861, - "volume": 207038 - }, - "open": "2020-06-08T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1409, - "high": 33573, - "low": 26, - "open": 7899, - "volume": 1656980 - }, - "id": 2629421, - "non_hive": { - "close": 358, - "high": 8564, - "low": 6, - "open": 1999, - "volume": 417542 - }, - "open": "2020-06-08T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3366, - "high": 19, - "low": 24, - "open": 252, - "volume": 2477299 - }, - "id": 2629461, - "non_hive": { - "close": 850, - "high": 5, - "low": 6, - "open": 64, - "volume": 619834 - }, - "open": "2020-06-08T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24978, - "high": 2512, - "low": 38, - "open": 28867, - "volume": 769151 - }, - "id": 2629493, - "non_hive": { - "close": 6332, - "high": 637, - "low": 9, - "open": 7220, - "volume": 192727 - }, - "open": "2020-06-08T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 347, - "low": 24, - "open": 13118, - "volume": 1339519 - }, - "id": 2629537, - "non_hive": { - "close": 25, - "high": 88, - "low": 6, - "open": 3325, - "volume": 336474 - }, - "open": "2020-06-08T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 35, - "low": 99, - "open": 4289, - "volume": 3072746 - }, - "id": 2629577, - "non_hive": { - "close": 3, - "high": 9, - "low": 24, - "open": 1077, - "volume": 769890 - }, - "open": "2020-06-08T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 35489, - "low": 38, - "open": 28, - "volume": 3840129 - }, - "id": 2629633, - "non_hive": { - "close": 6, - "high": 9029, - "low": 9, - "open": 7, - "volume": 961279 - }, - "open": "2020-06-08T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 188671, - "high": 63, - "low": 94, - "open": 7966, - "volume": 2117917 - }, - "id": 2629684, - "non_hive": { - "close": 47650, - "high": 16, - "low": 23, - "open": 1999, - "volume": 530119 - }, - "open": "2020-06-08T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 2918, - "low": 107, - "open": 606, - "volume": 220761 - }, - "id": 2629725, - "non_hive": { - "close": 6, - "high": 737, - "low": 26, - "open": 152, - "volume": 55704 - }, - "open": "2020-06-08T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 75, - "low": 400, - "open": 14342, - "volume": 1046911 - }, - "id": 2629772, - "non_hive": { - "close": 6, - "high": 19, - "low": 100, - "open": 3622, - "volume": 263331 - }, - "open": "2020-06-08T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 201, - "low": 99, - "open": 600000, - "volume": 2527035 - }, - "id": 2629820, - "non_hive": { - "close": 6, - "high": 51, - "low": 24, - "open": 150364, - "volume": 633529 - }, - "open": "2020-06-08T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 562, - "low": 44, - "open": 13750, - "volume": 493110 - }, - "id": 2629863, - "non_hive": { - "close": 6, - "high": 142, - "low": 11, - "open": 3468, - "volume": 124404 - }, - "open": "2020-06-08T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 245, - "low": 306310, - "open": 245, - "volume": 963628 - }, - "id": 2629897, - "non_hive": { - "close": 6, - "high": 62, - "low": 76577, - "open": 62, - "volume": 241917 - }, - "open": "2020-06-08T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 637, - "high": 114, - "low": 637, - "open": 15808, - "volume": 388512 - }, - "id": 2629947, - "non_hive": { - "close": 160, - "high": 29, - "low": 159, - "open": 3992, - "volume": 98076 - }, - "open": "2020-06-08T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13736, - "high": 75, - "low": 41, - "open": 23238, - "volume": 106133 - }, - "id": 2630003, - "non_hive": { - "close": 3468, - "high": 19, - "low": 10, - "open": 5868, - "volume": 26798 - }, - "open": "2020-06-08T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2294, - "high": 1081, - "low": 28, - "open": 1081, - "volume": 1220327 - }, - "id": 2630030, - "non_hive": { - "close": 579, - "high": 273, - "low": 7, - "open": 273, - "volume": 305438 - }, - "open": "2020-06-09T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24133, - "high": 59656, - "low": 32, - "open": 5739, - "volume": 206335 - }, - "id": 2630051, - "non_hive": { - "close": 6089, - "high": 15062, - "low": 8, - "open": 1448, - "volume": 52073 - }, - "open": "2020-06-09T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 163, - "high": 324, - "low": 4, - "open": 4, - "volume": 56291 - }, - "id": 2630075, - "non_hive": { - "close": 41, - "high": 82, - "low": 1, - "open": 1, - "volume": 14147 - }, - "open": "2020-06-09T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1454, - "high": 2721, - "low": 140, - "open": 2721, - "volume": 2747563 - }, - "id": 2630106, - "non_hive": { - "close": 367, - "high": 687, - "low": 35, - "open": 687, - "volume": 693484 - }, - "open": "2020-06-09T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 580289, - "high": 580289, - "low": 233, - "open": 6125, - "volume": 6685917 - }, - "id": 2630132, - "non_hive": { - "close": 146560, - "high": 146560, - "low": 58, - "open": 1546, - "volume": 1687787 - }, - "open": "2020-06-09T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3048, - "high": 994, - "low": 4, - "open": 4353, - "volume": 169731 - }, - "id": 2630170, - "non_hive": { - "close": 766, - "high": 250, - "low": 1, - "open": 1093, - "volume": 42643 - }, - "open": "2020-06-09T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24472, - "high": 2836, - "low": 24, - "open": 7954, - "volume": 1632345 - }, - "id": 2630191, - "non_hive": { - "close": 6264, - "high": 726, - "low": 6, - "open": 2000, - "volume": 411523 - }, - "open": "2020-06-09T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14464, - "high": 6365, - "low": 24, - "open": 6365, - "volume": 1250235 - }, - "id": 2630233, - "non_hive": { - "close": 3694, - "high": 1629, - "low": 6, - "open": 1629, - "volume": 315579 - }, - "open": "2020-06-09T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 12504, - "low": 24, - "open": 7935, - "volume": 3793943 - }, - "id": 2630255, - "non_hive": { - "close": 3, - "high": 3195, - "low": 6, - "open": 2000, - "volume": 956821 - }, - "open": "2020-06-09T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5302, - "high": 1375, - "low": 22481, - "open": 72181, - "volume": 2708797 - }, - "id": 2630299, - "non_hive": { - "close": 1349, - "high": 350, - "low": 5620, - "open": 18192, - "volume": 679652 - }, - "open": "2020-06-09T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 71148, - "high": 94, - "low": 4253, - "open": 22675, - "volume": 2472144 - }, - "id": 2630344, - "non_hive": { - "close": 17787, - "high": 24, - "low": 1063, - "open": 5770, - "volume": 618342 - }, - "open": "2020-06-09T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2622, - "high": 319, - "low": 142846, - "open": 328843, - "volume": 655416 - }, - "id": 2630375, - "non_hive": { - "close": 664, - "high": 81, - "low": 35425, - "open": 82212, - "volume": 163559 - }, - "open": "2020-06-09T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24272, - "high": 94, - "low": 25, - "open": 15687, - "volume": 796567 - }, - "id": 2630408, - "non_hive": { - "close": 6138, - "high": 24, - "low": 6, - "open": 3971, - "volume": 199814 - }, - "open": "2020-06-09T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 146, - "low": 4317, - "open": 35526, - "volume": 1080847 - }, - "id": 2630447, - "non_hive": { - "close": 25, - "high": 37, - "low": 1079, - "open": 8976, - "volume": 271610 - }, - "open": "2020-06-09T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 3000, - "low": 36, - "open": 2012, - "volume": 387086 - }, - "id": 2630489, - "non_hive": { - "close": 3, - "high": 759, - "low": 9, - "open": 509, - "volume": 97766 - }, - "open": "2020-06-09T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 190, - "low": 42, - "open": 32, - "volume": 1661853 - }, - "id": 2630529, - "non_hive": { - "close": 6, - "high": 48, - "low": 10, - "open": 8, - "volume": 416407 - }, - "open": "2020-06-09T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 3444, - "low": 64, - "open": 8310, - "volume": 868225 - }, - "id": 2630577, - "non_hive": { - "close": 6, - "high": 868, - "low": 16, - "open": 2090, - "volume": 217967 - }, - "open": "2020-06-09T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 71, - "low": 24, - "open": 357, - "volume": 48439 - }, - "id": 2630617, - "non_hive": { - "close": 6, - "high": 18, - "low": 6, - "open": 90, - "volume": 12205 - }, - "open": "2020-06-09T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25, - "high": 103, - "low": 25, - "open": 103, - "volume": 712144 - }, - "id": 2630643, - "non_hive": { - "close": 6, - "high": 26, - "low": 6, - "open": 26, - "volume": 178124 - }, - "open": "2020-06-09T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12919, - "high": 170, - "low": 3572, - "open": 3572, - "volume": 394431 - }, - "id": 2630692, - "non_hive": { - "close": 3255, - "high": 43, - "low": 886, - "open": 886, - "volume": 99276 - }, - "open": "2020-06-09T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 1140705, - "low": 20, - "open": 24, - "volume": 3007500 - }, - "id": 2630726, - "non_hive": { - "close": 3, - "high": 288015, - "low": 4, - "open": 6, - "volume": 754737 - }, - "open": "2020-06-09T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2000, - "high": 839, - "low": 5, - "open": 25, - "volume": 71377 - }, - "id": 2630756, - "non_hive": { - "close": 505, - "high": 212, - "low": 1, - "open": 6, - "volume": 17997 - }, - "open": "2020-06-09T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53, - "high": 28209, - "low": 25, - "open": 26132, - "volume": 2537859 - }, - "id": 2630795, - "non_hive": { - "close": 13, - "high": 7113, - "low": 6, - "open": 6588, - "volume": 630748 - }, - "open": "2020-06-09T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3919, - "high": 280573, - "low": 11, - "open": 13, - "volume": 3538766 - }, - "id": 2630826, - "non_hive": { - "close": 988, - "high": 70981, - "low": 2, - "open": 3, - "volume": 882624 - }, - "open": "2020-06-09T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19, - "high": 19, - "low": 32, - "open": 5536, - "volume": 35706 - }, - "id": 2630895, - "non_hive": { - "close": 5, - "high": 5, - "low": 7, - "open": 1397, - "volume": 9006 - }, - "open": "2020-06-10T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 555528, - "high": 229, - "low": 25, - "open": 13392, - "volume": 1603233 - }, - "id": 2630919, - "non_hive": { - "close": 137771, - "high": 58, - "low": 6, - "open": 3377, - "volume": 399344 - }, - "open": "2020-06-10T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 384, - "low": 9, - "open": 384, - "volume": 766543 - }, - "id": 2630950, - "non_hive": { - "close": 3, - "high": 97, - "low": 2, - "open": 97, - "volume": 190764 - }, - "open": "2020-06-10T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41, - "high": 528, - "low": 145, - "open": 437, - "volume": 202621 - }, - "id": 2630975, - "non_hive": { - "close": 10, - "high": 133, - "low": 35, - "open": 110, - "volume": 50980 - }, - "open": "2020-06-10T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 3, - "low": 37, - "open": 37, - "volume": 688338 - }, - "id": 2630996, - "non_hive": { - "close": 1, - "high": 1, - "low": 9, - "open": 9, - "volume": 171126 - }, - "open": "2020-06-10T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 181, - "high": 764, - "low": 25, - "open": 25, - "volume": 133917 - }, - "id": 2631030, - "non_hive": { - "close": 45, - "high": 192, - "low": 6, - "open": 6, - "volume": 33627 - }, - "open": "2020-06-10T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1471, - "high": 10831, - "low": 288763, - "open": 11948, - "volume": 708851 - }, - "id": 2631059, - "non_hive": { - "close": 370, - "high": 2725, - "low": 71076, - "open": 3000, - "volume": 175999 - }, - "open": "2020-06-10T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6200, - "high": 2430, - "low": 25, - "open": 2430, - "volume": 97486 - }, - "id": 2631087, - "non_hive": { - "close": 1550, - "high": 611, - "low": 6, - "open": 611, - "volume": 24339 - }, - "open": "2020-06-10T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 15, - "low": 13, - "open": 21609, - "volume": 2070832 - }, - "id": 2631128, - "non_hive": { - "close": 3, - "high": 4, - "low": 3, - "open": 5415, - "volume": 510803 - }, - "open": "2020-06-10T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3481, - "high": 1173, - "low": 25, - "open": 2542, - "volume": 3949187 - }, - "id": 2631179, - "non_hive": { - "close": 863, - "high": 294, - "low": 6, - "open": 636, - "volume": 976051 - }, - "open": "2020-06-10T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8990, - "high": 3000, - "low": 25, - "open": 1984, - "volume": 732972 - }, - "id": 2631231, - "non_hive": { - "close": 2229, - "high": 744, - "low": 6, - "open": 492, - "volume": 180656 - }, - "open": "2020-06-10T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8537, - "high": 104, - "low": 25, - "open": 10790, - "volume": 1490734 - }, - "id": 2631258, - "non_hive": { - "close": 2117, - "high": 26, - "low": 6, - "open": 2676, - "volume": 368163 - }, - "open": "2020-06-10T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 599891, - "high": 1431, - "low": 25, - "open": 42127, - "volume": 1127761 - }, - "id": 2631301, - "non_hive": { - "close": 148176, - "high": 355, - "low": 6, - "open": 10446, - "volume": 279070 - }, - "open": "2020-06-10T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60, - "high": 28, - "low": 25, - "open": 12559, - "volume": 130368 - }, - "id": 2631333, - "non_hive": { - "close": 15, - "high": 7, - "low": 6, - "open": 3114, - "volume": 32409 - }, - "open": "2020-06-10T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41, - "high": 36, - "low": 29, - "open": 102, - "volume": 1264831 - }, - "id": 2631373, - "non_hive": { - "close": 10, - "high": 9, - "low": 7, - "open": 25, - "volume": 311626 - }, - "open": "2020-06-10T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 480, - "high": 1016, - "low": 7, - "open": 32753, - "volume": 821519 - }, - "id": 2631415, - "non_hive": { - "close": 120, - "high": 254, - "low": 1, - "open": 8185, - "volume": 205048 - }, - "open": "2020-06-10T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11787, - "high": 168, - "low": 6, - "open": 8015, - "volume": 732739 - }, - "id": 2631476, - "non_hive": { - "close": 2935, - "high": 42, - "low": 1, - "open": 2001, - "volume": 181294 - }, - "open": "2020-06-10T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 327, - "high": 12, - "low": 25, - "open": 13000, - "volume": 1930600 - }, - "id": 2631520, - "non_hive": { - "close": 81, - "high": 3, - "low": 6, - "open": 3237, - "volume": 476462 - }, - "open": "2020-06-10T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 307, - "high": 52323, - "low": 25, - "open": 4373, - "volume": 1161614 - }, - "id": 2631568, - "non_hive": { - "close": 76, - "high": 13006, - "low": 6, - "open": 1078, - "volume": 286822 - }, - "open": "2020-06-10T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 126, - "high": 125, - "low": 25, - "open": 12141, - "volume": 2506119 - }, - "id": 2631633, - "non_hive": { - "close": 31, - "high": 31, - "low": 6, - "open": 2999, - "volume": 616915 - }, - "open": "2020-06-10T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41, - "high": 224, - "low": 43, - "open": 4284, - "volume": 775742 - }, - "id": 2631671, - "non_hive": { - "close": 10, - "high": 56, - "low": 10, - "open": 1054, - "volume": 190628 - }, - "open": "2020-06-10T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15, - "high": 4, - "low": 15, - "open": 13, - "volume": 591325 - }, - "id": 2631727, - "non_hive": { - "close": 3, - "high": 1, - "low": 3, - "open": 3, - "volume": 146111 - }, - "open": "2020-06-10T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54, - "high": 8935, - "low": 20, - "open": 806, - "volume": 147380 - }, - "id": 2631782, - "non_hive": { - "close": 13, - "high": 2229, - "low": 4, - "open": 201, - "volume": 36730 - }, - "open": "2020-06-10T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10057, - "high": 172, - "low": 25, - "open": 8882, - "volume": 613942 - }, - "id": 2631818, - "non_hive": { - "close": 2464, - "high": 43, - "low": 6, - "open": 2215, - "volume": 151123 - }, - "open": "2020-06-10T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5493, - "high": 1092, - "low": 25, - "open": 8159, - "volume": 3928831 - }, - "id": 2631859, - "non_hive": { - "close": 1367, - "high": 273, - "low": 6, - "open": 2000, - "volume": 979782 - }, - "open": "2020-06-11T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32, - "high": 838, - "low": 134, - "open": 7659, - "volume": 546856 - }, - "id": 2631919, - "non_hive": { - "close": 8, - "high": 210, - "low": 33, - "open": 1908, - "volume": 136769 - }, - "open": "2020-06-11T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 866, - "low": 4, - "open": 13499, - "volume": 71273 - }, - "id": 2631961, - "non_hive": { - "close": 11, - "high": 217, - "low": 1, - "open": 3382, - "volume": 17855 - }, - "open": "2020-06-11T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5612, - "high": 7455, - "low": 50, - "open": 12, - "volume": 3380543 - }, - "id": 2631981, - "non_hive": { - "close": 1404, - "high": 1868, - "low": 12, - "open": 3, - "volume": 834492 - }, - "open": "2020-06-11T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 251130, - "high": 35, - "low": 25, - "open": 5291, - "volume": 3666783 - }, - "id": 2632028, - "non_hive": { - "close": 61791, - "high": 9, - "low": 6, - "open": 1323, - "volume": 902462 - }, - "open": "2020-06-11T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45573, - "high": 5384, - "low": 25, - "open": 8127, - "volume": 3618173 - }, - "id": 2632060, - "non_hive": { - "close": 11168, - "high": 1343, - "low": 6, - "open": 2000, - "volume": 890210 - }, - "open": "2020-06-11T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100000, - "high": 662, - "low": 25, - "open": 827, - "volume": 3792908 - }, - "id": 2632095, - "non_hive": { - "close": 24603, - "high": 166, - "low": 6, - "open": 204, - "volume": 930830 - }, - "open": "2020-06-11T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 289372, - "high": 176, - "low": 7, - "open": 984, - "volume": 9421957 - }, - "id": 2632141, - "non_hive": { - "close": 73790, - "high": 45, - "low": 1, - "open": 244, - "volume": 2366370 - }, - "open": "2020-06-11T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 19, - "low": 5, - "open": 313, - "volume": 859714 - }, - "id": 2632213, - "non_hive": { - "close": 11, - "high": 5, - "low": 1, - "open": 80, - "volume": 220304 - }, - "open": "2020-06-11T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25841, - "high": 25841, - "low": 12, - "open": 12, - "volume": 98294 - }, - "id": 2632263, - "non_hive": { - "close": 6667, - "high": 6667, - "low": 3, - "open": 3, - "volume": 25278 - }, - "open": "2020-06-11T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 393, - "high": 101705, - "low": 24, - "open": 500, - "volume": 643306 - }, - "id": 2632294, - "non_hive": { - "close": 101, - "high": 26341, - "low": 6, - "open": 129, - "volume": 165993 - }, - "open": "2020-06-11T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 404735, - "high": 23, - "low": 24, - "open": 31300, - "volume": 1316215 - }, - "id": 2632341, - "non_hive": { - "close": 103636, - "high": 6, - "low": 6, - "open": 8014, - "volume": 337567 - }, - "open": "2020-06-11T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1712, - "high": 191270, - "low": 24, - "open": 14445, - "volume": 3256136 - }, - "id": 2632376, - "non_hive": { - "close": 440, - "high": 49517, - "low": 6, - "open": 3713, - "volume": 832016 - }, - "open": "2020-06-11T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 568535, - "high": 303, - "low": 24, - "open": 6068, - "volume": 1931401 - }, - "id": 2632411, - "non_hive": { - "close": 144319, - "high": 78, - "low": 6, - "open": 1559, - "volume": 491290 - }, - "open": "2020-06-11T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 929815, - "high": 1435, - "low": 97, - "open": 103, - "volume": 4722908 - }, - "id": 2632460, - "non_hive": { - "close": 237959, - "high": 368, - "low": 24, - "open": 26, - "volume": 1200970 - }, - "open": "2020-06-11T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14208, - "high": 19, - "low": 13, - "open": 12, - "volume": 7190984 - }, - "id": 2632509, - "non_hive": { - "close": 3670, - "high": 5, - "low": 3, - "open": 3, - "volume": 1837989 - }, - "open": "2020-06-11T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 174, - "high": 254, - "low": 24, - "open": 7739, - "volume": 7020497 - }, - "id": 2632574, - "non_hive": { - "close": 45, - "high": 66, - "low": 6, - "open": 1999, - "volume": 1803507 - }, - "open": "2020-06-11T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7352, - "high": 62, - "low": 23, - "open": 52295, - "volume": 4358305 - }, - "id": 2632638, - "non_hive": { - "close": 1893, - "high": 16, - "low": 5, - "open": 13473, - "volume": 1112469 - }, - "open": "2020-06-11T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 200000, - "high": 15, - "low": 19, - "open": 7760, - "volume": 6588396 - }, - "id": 2632693, - "non_hive": { - "close": 51598, - "high": 4, - "low": 4, - "open": 1999, - "volume": 1686735 - }, - "open": "2020-06-11T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 1158, - "low": 584349, - "open": 1901, - "volume": 9385384 - }, - "id": 2632771, - "non_hive": { - "close": 153642, - "high": 318, - "low": 148424, - "open": 492, - "volume": 2437140 - }, - "open": "2020-06-11T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 277, - "low": 24, - "open": 990, - "volume": 3695149 - }, - "id": 2632848, - "non_hive": { - "close": 11, - "high": 72, - "low": 6, - "open": 257, - "volume": 939915 - }, - "open": "2020-06-11T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4758, - "high": 466, - "low": 12, - "open": 12, - "volume": 3731367 - }, - "id": 2632906, - "non_hive": { - "close": 1222, - "high": 120, - "low": 3, - "open": 3, - "volume": 937781 - }, - "open": "2020-06-11T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6211, - "high": 128, - "low": 27850, - "open": 7992, - "volume": 3879864 - }, - "id": 2632953, - "non_hive": { - "close": 1581, - "high": 33, - "low": 6962, - "open": 1999, - "volume": 972021 - }, - "open": "2020-06-11T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7782, - "high": 609, - "low": 25, - "open": 7860, - "volume": 4524901 - }, - "id": 2633016, - "non_hive": { - "close": 1964, - "high": 155, - "low": 6, - "open": 1999, - "volume": 1124981 - }, - "open": "2020-06-11T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 183, - "high": 3757, - "low": 25, - "open": 3757, - "volume": 3017947 - }, - "id": 2633069, - "non_hive": { - "close": 46, - "high": 947, - "low": 6, - "open": 947, - "volume": 743150 - }, - "open": "2020-06-12T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29, - "high": 1035, - "low": 305, - "open": 1035, - "volume": 3695064 - }, - "id": 2633108, - "non_hive": { - "close": 7, - "high": 259, - "low": 73, - "open": 259, - "volume": 904531 - }, - "open": "2020-06-12T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42, - "high": 2032, - "low": 42, - "open": 300003, - "volume": 4606708 - }, - "id": 2633144, - "non_hive": { - "close": 10, - "high": 505, - "low": 10, - "open": 72769, - "volume": 1117228 - }, - "open": "2020-06-12T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25, - "high": 280, - "low": 10, - "open": 460, - "volume": 3631985 - }, - "id": 2633175, - "non_hive": { - "close": 6, - "high": 70, - "low": 2, - "open": 114, - "volume": 889815 - }, - "open": "2020-06-12T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1205, - "high": 1794, - "low": 17, - "open": 1794, - "volume": 3811278 - }, - "id": 2633221, - "non_hive": { - "close": 300, - "high": 447, - "low": 4, - "open": 447, - "volume": 930852 - }, - "open": "2020-06-12T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 377905, - "high": 36, - "low": 7, - "open": 25, - "volume": 7871329 - }, - "id": 2633245, - "non_hive": { - "close": 94312, - "high": 9, - "low": 1, - "open": 6, - "volume": 1942252 - }, - "open": "2020-06-12T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59, - "high": 9490, - "low": 55, - "open": 25, - "volume": 6062323 - }, - "id": 2633304, - "non_hive": { - "close": 14, - "high": 2416, - "low": 13, - "open": 6, - "volume": 1508208 - }, - "open": "2020-06-12T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 88408, - "high": 393, - "low": 72, - "open": 393, - "volume": 5029178 - }, - "id": 2633353, - "non_hive": { - "close": 22059, - "high": 100, - "low": 17, - "open": 100, - "volume": 1244606 - }, - "open": "2020-06-12T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41, - "high": 279, - "low": 26, - "open": 8016, - "volume": 4143482 - }, - "id": 2633418, - "non_hive": { - "close": 10, - "high": 70, - "low": 6, - "open": 1999, - "volume": 1028611 - }, - "open": "2020-06-12T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 735, - "high": 52, - "low": 13, - "open": 13, - "volume": 2512481 - }, - "id": 2633490, - "non_hive": { - "close": 183, - "high": 13, - "low": 3, - "open": 3, - "volume": 620225 - }, - "open": "2020-06-12T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3103, - "high": 3, - "low": 25, - "open": 25, - "volume": 2157724 - }, - "id": 2633531, - "non_hive": { - "close": 777, - "high": 1, - "low": 6, - "open": 6, - "volume": 533308 - }, - "open": "2020-06-12T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 139, - "high": 27, - "low": 5, - "open": 7989, - "volume": 3704123 - }, - "id": 2633578, - "non_hive": { - "close": 34, - "high": 7, - "low": 1, - "open": 1999, - "volume": 926865 - }, - "open": "2020-06-12T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 120657, - "high": 157, - "low": 5, - "open": 25, - "volume": 3936609 - }, - "id": 2633622, - "non_hive": { - "close": 30063, - "high": 40, - "low": 1, - "open": 6, - "volume": 982373 - }, - "open": "2020-06-12T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30252, - "high": 8248, - "low": 46, - "open": 8026, - "volume": 3657049 - }, - "id": 2633662, - "non_hive": { - "close": 7532, - "high": 2085, - "low": 11, - "open": 2000, - "volume": 912424 - }, - "open": "2020-06-12T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7943, - "high": 16178, - "low": 32, - "open": 101, - "volume": 1317777 - }, - "id": 2633699, - "non_hive": { - "close": 1999, - "high": 4078, - "low": 7, - "open": 25, - "volume": 328189 - }, - "open": "2020-06-12T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9306, - "high": 8089, - "low": 8, - "open": 12, - "volume": 3988419 - }, - "id": 2633734, - "non_hive": { - "close": 2326, - "high": 2037, - "low": 1, - "open": 3, - "volume": 994564 - }, - "open": "2020-06-12T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5758, - "high": 3, - "low": 24, - "open": 21632, - "volume": 4550608 - }, - "id": 2633786, - "non_hive": { - "close": 1460, - "high": 1, - "low": 6, - "open": 5429, - "volume": 1139619 - }, - "open": "2020-06-12T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 199554, - "high": 199554, - "low": 6, - "open": 2126, - "volume": 7432441 - }, - "id": 2633826, - "non_hive": { - "close": 51089, - "high": 51089, - "low": 1, - "open": 539, - "volume": 1883954 - }, - "open": "2020-06-12T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2867, - "high": 15073, - "low": 24, - "open": 446, - "volume": 1956191 - }, - "id": 2633889, - "non_hive": { - "close": 735, - "high": 3889, - "low": 6, - "open": 114, - "volume": 496903 - }, - "open": "2020-06-12T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9265, - "high": 139, - "low": 329, - "open": 3571, - "volume": 2342383 - }, - "id": 2633943, - "non_hive": { - "close": 2375, - "high": 36, - "low": 82, - "open": 907, - "volume": 595769 - }, - "open": "2020-06-12T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 3, - "low": 24, - "open": 3001, - "volume": 1255143 - }, - "id": 2634001, - "non_hive": { - "close": 11, - "high": 1, - "low": 6, - "open": 769, - "volume": 317268 - }, - "open": "2020-06-12T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3392, - "high": 1025, - "low": 12, - "open": 12, - "volume": 86132 - }, - "id": 2634038, - "non_hive": { - "close": 864, - "high": 263, - "low": 3, - "open": 3, - "volume": 21939 - }, - "open": "2020-06-12T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3124, - "high": 23, - "low": 16, - "open": 1947, - "volume": 13097 - }, - "id": 2634074, - "non_hive": { - "close": 806, - "high": 6, - "low": 4, - "open": 500, - "volume": 3372 - }, - "open": "2020-06-12T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 582389, - "high": 337, - "low": 12, - "open": 12, - "volume": 2451336 - }, - "id": 2634101, - "non_hive": { - "close": 147985, - "high": 87, - "low": 3, - "open": 3, - "volume": 624827 - }, - "open": "2020-06-12T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 601, - "high": 3, - "low": 32, - "open": 13864, - "volume": 4091901 - }, - "id": 2634145, - "non_hive": { - "close": 155, - "high": 1, - "low": 8, - "open": 3571, - "volume": 1041559 - }, - "open": "2020-06-13T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 114, - "high": 387, - "low": 114, - "open": 387, - "volume": 1875800 - }, - "id": 2634195, - "non_hive": { - "close": 28, - "high": 100, - "low": 28, - "open": 100, - "volume": 473793 - }, - "open": "2020-06-13T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 121, - "high": 2618, - "low": 121, - "open": 4, - "volume": 2594359 - }, - "id": 2634221, - "non_hive": { - "close": 30, - "high": 671, - "low": 30, - "open": 1, - "volume": 652081 - }, - "open": "2020-06-13T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 592033, - "high": 23, - "low": 177, - "open": 12, - "volume": 3013903 - }, - "id": 2634254, - "non_hive": { - "close": 148600, - "high": 6, - "low": 44, - "open": 3, - "volume": 756548 - }, - "open": "2020-06-13T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 396, - "high": 32241, - "low": 24, - "open": 7967, - "volume": 3758428 - }, - "id": 2634278, - "non_hive": { - "close": 100, - "high": 8185, - "low": 6, - "open": 2000, - "volume": 943582 - }, - "open": "2020-06-13T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2088, - "high": 1398, - "low": 24, - "open": 140191, - "volume": 4068911 - }, - "id": 2634313, - "non_hive": { - "close": 530, - "high": 355, - "low": 6, - "open": 35188, - "volume": 1023359 - }, - "open": "2020-06-13T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 386424, - "high": 1901, - "low": 116, - "open": 7960, - "volume": 3630363 - }, - "id": 2634362, - "non_hive": { - "close": 96992, - "high": 481, - "low": 29, - "open": 2000, - "volume": 911539 - }, - "open": "2020-06-13T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13454, - "high": 80632, - "low": 134, - "open": 5001, - "volume": 3752039 - }, - "id": 2634397, - "non_hive": { - "close": 3406, - "high": 20436, - "low": 33, - "open": 1262, - "volume": 942165 - }, - "open": "2020-06-13T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 149, - "low": 24, - "open": 59941, - "volume": 3718969 - }, - "id": 2634435, - "non_hive": { - "close": 11, - "high": 38, - "low": 6, - "open": 15179, - "volume": 933906 - }, - "open": "2020-06-13T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19, - "high": 19, - "low": 245, - "open": 12, - "volume": 3794133 - }, - "id": 2634479, - "non_hive": { - "close": 5, - "high": 5, - "low": 61, - "open": 3, - "volume": 952702 - }, - "open": "2020-06-13T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 201, - "high": 201, - "low": 24, - "open": 9628, - "volume": 2312261 - }, - "id": 2634535, - "non_hive": { - "close": 51, - "high": 51, - "low": 6, - "open": 2435, - "volume": 584994 - }, - "open": "2020-06-13T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12063, - "high": 220, - "low": 24, - "open": 220, - "volume": 3726659 - }, - "id": 2634577, - "non_hive": { - "close": 3058, - "high": 56, - "low": 6, - "open": 56, - "volume": 942914 - }, - "open": "2020-06-13T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 97423, - "high": 97423, - "low": 24, - "open": 8567, - "volume": 7041278 - }, - "id": 2634620, - "non_hive": { - "close": 25330, - "high": 25330, - "low": 6, - "open": 2172, - "volume": 1792621 - }, - "open": "2020-06-13T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7707, - "high": 6442, - "low": 24, - "open": 7703, - "volume": 653159 - }, - "id": 2634670, - "non_hive": { - "close": 1999, - "high": 1672, - "low": 6, - "open": 1999, - "volume": 167236 - }, - "open": "2020-06-13T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43, - "high": 6415, - "low": 24, - "open": 101, - "volume": 2094670 - }, - "id": 2634694, - "non_hive": { - "close": 11, - "high": 1668, - "low": 6, - "open": 26, - "volume": 539932 - }, - "open": "2020-06-13T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18839, - "high": 80, - "low": 12, - "open": 12, - "volume": 275886 - }, - "id": 2634745, - "non_hive": { - "close": 4822, - "high": 21, - "low": 3, - "open": 3, - "volume": 71615 - }, - "open": "2020-06-13T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7236, - "high": 42, - "low": 24, - "open": 7701, - "volume": 861598 - }, - "id": 2634792, - "non_hive": { - "close": 1876, - "high": 11, - "low": 6, - "open": 1999, - "volume": 221640 - }, - "open": "2020-06-13T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 519, - "high": 146, - "low": 6, - "open": 15075, - "volume": 193465 - }, - "id": 2634832, - "non_hive": { - "close": 135, - "high": 38, - "low": 1, - "open": 3909, - "volume": 50217 - }, - "open": "2020-06-13T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9959, - "high": 30, - "low": 24, - "open": 392, - "volume": 152415 - }, - "id": 2634869, - "non_hive": { - "close": 2549, - "high": 8, - "low": 6, - "open": 101, - "volume": 39355 - }, - "open": "2020-06-13T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18, - "high": 84, - "low": 18, - "open": 7713, - "volume": 725011 - }, - "id": 2634937, - "non_hive": { - "close": 4, - "high": 22, - "low": 4, - "open": 1999, - "volume": 186024 - }, - "open": "2020-06-13T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 69, - "low": 24, - "open": 2340, - "volume": 2650012 - }, - "id": 2634983, - "non_hive": { - "close": 11, - "high": 18, - "low": 6, - "open": 608, - "volume": 675093 - }, - "open": "2020-06-13T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3185, - "high": 11, - "low": 7, - "open": 12, - "volume": 332006 - }, - "id": 2635040, - "non_hive": { - "close": 814, - "high": 3, - "low": 1, - "open": 3, - "volume": 84705 - }, - "open": "2020-06-13T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 62700, - "high": 7390, - "low": 28, - "open": 9528, - "volume": 724675 - }, - "id": 2635083, - "non_hive": { - "close": 16119, - "high": 1919, - "low": 7, - "open": 2474, - "volume": 186314 - }, - "open": "2020-06-13T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1942, - "high": 46, - "low": 12, - "open": 2065, - "volume": 1056114 - }, - "id": 2635120, - "non_hive": { - "close": 505, - "high": 12, - "low": 3, - "open": 531, - "volume": 273945 - }, - "open": "2020-06-13T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27, - "high": 3, - "low": 696, - "open": 14110, - "volume": 1234109 - }, - "id": 2635164, - "non_hive": { - "close": 7, - "high": 1, - "low": 180, - "open": 3667, - "volume": 320861 - }, - "open": "2020-06-14T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13582, - "high": 16683, - "low": 36, - "open": 11435, - "volume": 3763561 - }, - "id": 2635201, - "non_hive": { - "close": 3725, - "high": 4587, - "low": 9, - "open": 3035, - "volume": 1004216 - }, - "open": "2020-06-14T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 25, - "low": 4, - "open": 4058, - "volume": 6162050 - }, - "id": 2635241, - "non_hive": { - "close": 12, - "high": 7, - "low": 1, - "open": 1111, - "volume": 1689197 - }, - "open": "2020-06-14T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5186, - "high": 3, - "low": 12, - "open": 33091, - "volume": 3195781 - }, - "id": 2635290, - "non_hive": { - "close": 1400, - "high": 1, - "low": 3, - "open": 9190, - "volume": 858822 - }, - "open": "2020-06-14T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23, - "high": 62, - "low": 4, - "open": 368, - "volume": 277661 - }, - "id": 2635331, - "non_hive": { - "close": 6, - "high": 17, - "low": 1, - "open": 100, - "volume": 75694 - }, - "open": "2020-06-14T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23, - "high": 351022, - "low": 23, - "open": 351022, - "volume": 2509101 - }, - "id": 2635358, - "non_hive": { - "close": 6, - "high": 95766, - "low": 6, - "open": 95766, - "volume": 680818 - }, - "open": "2020-06-14T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 573395, - "high": 11, - "low": 566777, - "open": 920, - "volume": 1422986 - }, - "id": 2635388, - "non_hive": { - "close": 153687, - "high": 3, - "low": 151913, - "open": 250, - "volume": 381901 - }, - "open": "2020-06-14T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12416, - "high": 12416, - "low": 23, - "open": 23, - "volume": 612439 - }, - "id": 2635434, - "non_hive": { - "close": 3371, - "high": 3371, - "low": 6, - "open": 6, - "volume": 163659 - }, - "open": "2020-06-14T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8720, - "high": 8720, - "low": 16, - "open": 6353, - "volume": 3110377 - }, - "id": 2635442, - "non_hive": { - "close": 2401, - "high": 2401, - "low": 4, - "open": 1725, - "volume": 839464 - }, - "open": "2020-06-14T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 131891, - "high": 68, - "low": 6854, - "open": 41, - "volume": 2567736 - }, - "id": 2635489, - "non_hive": { - "close": 36347, - "high": 19, - "low": 1837, - "open": 11, - "volume": 696784 - }, - "open": "2020-06-14T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 409264, - "high": 8281, - "low": 591, - "open": 8281, - "volume": 2483566 - }, - "id": 2635526, - "non_hive": { - "close": 111988, - "high": 2280, - "low": 158, - "open": 2280, - "volume": 679552 - }, - "open": "2020-06-14T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4621, - "high": 25, - "low": 76, - "open": 50318, - "volume": 281277 - }, - "id": 2635550, - "non_hive": { - "close": 1266, - "high": 7, - "low": 20, - "open": 13788, - "volume": 77067 - }, - "open": "2020-06-14T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7476, - "high": 21, - "low": 7301, - "open": 21, - "volume": 913275 - }, - "id": 2635576, - "non_hive": { - "close": 2064, - "high": 6, - "low": 1999, - "open": 6, - "volume": 252212 - }, - "open": "2020-06-14T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21465, - "high": 112, - "low": 77, - "open": 7244, - "volume": 51516 - }, - "id": 2635614, - "non_hive": { - "close": 5925, - "high": 31, - "low": 21, - "open": 1999, - "volume": 14217 - }, - "open": "2020-06-14T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5089, - "high": 23001, - "low": 30, - "open": 23001, - "volume": 566820 - }, - "id": 2635646, - "non_hive": { - "close": 1399, - "high": 6349, - "low": 8, - "open": 6349, - "volume": 155051 - }, - "open": "2020-06-14T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12491, - "high": 39, - "low": 30, - "open": 41, - "volume": 2674988 - }, - "id": 2635698, - "non_hive": { - "close": 3403, - "high": 11, - "low": 8, - "open": 11, - "volume": 727226 - }, - "open": "2020-06-14T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 592148, - "high": 1020, - "low": 269003, - "open": 1020, - "volume": 4472966 - }, - "id": 2635758, - "non_hive": { - "close": 157512, - "high": 278, - "low": 71301, - "open": 278, - "volume": 1205364 - }, - "open": "2020-06-14T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3711, - "high": 33, - "low": 154, - "open": 304, - "volume": 1715423 - }, - "id": 2635794, - "non_hive": { - "close": 1001, - "high": 9, - "low": 40, - "open": 82, - "volume": 457802 - }, - "open": "2020-06-14T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 918, - "high": 40, - "low": 8, - "open": 46501, - "volume": 4907400 - }, - "id": 2635830, - "non_hive": { - "close": 243, - "high": 11, - "low": 2, - "open": 12500, - "volume": 1303543 - }, - "open": "2020-06-14T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 80, - "high": 10709, - "low": 69, - "open": 14684, - "volume": 3179599 - }, - "id": 2635883, - "non_hive": { - "close": 22, - "high": 2976, - "low": 18, - "open": 3886, - "volume": 848109 - }, - "open": "2020-06-14T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1038, - "high": 353, - "low": 116, - "open": 7452, - "volume": 3952423 - }, - "id": 2635926, - "non_hive": { - "close": 282, - "high": 97, - "low": 30, - "open": 2000, - "volume": 1058931 - }, - "open": "2020-06-14T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13927, - "high": 232, - "low": 12, - "open": 232, - "volume": 1307669 - }, - "id": 2635964, - "non_hive": { - "close": 3776, - "high": 63, - "low": 3, - "open": 63, - "volume": 349727 - }, - "open": "2020-06-14T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 361717, - "high": 3, - "low": 319388, - "open": 66, - "volume": 1316092 - }, - "id": 2636001, - "non_hive": { - "close": 98186, - "high": 1, - "low": 85308, - "open": 18, - "volume": 355674 - }, - "open": "2020-06-14T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 709447, - "high": 1260, - "low": 12, - "open": 715, - "volume": 1427775 - }, - "id": 2636034, - "non_hive": { - "close": 188690, - "high": 342, - "low": 3, - "open": 191, - "volume": 376875 - }, - "open": "2020-06-14T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 66650, - "high": 598, - "low": 52, - "open": 407, - "volume": 2631380 - }, - "id": 2636075, - "non_hive": { - "close": 17614, - "high": 159, - "low": 13, - "open": 106, - "volume": 673542 - }, - "open": "2020-06-15T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3431, - "high": 90, - "low": 45, - "open": 1000, - "volume": 4575859 - }, - "id": 2636142, - "non_hive": { - "close": 881, - "high": 24, - "low": 11, - "open": 264, - "volume": 1170242 - }, - "open": "2020-06-15T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7120, - "high": 214, - "low": 2925, - "open": 10496, - "volume": 4179285 - }, - "id": 2636223, - "non_hive": { - "close": 1822, - "high": 55, - "low": 731, - "open": 2695, - "volume": 1049884 - }, - "open": "2020-06-15T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31, - "high": 383, - "low": 31, - "open": 44, - "volume": 3719713 - }, - "id": 2636275, - "non_hive": { - "close": 7, - "high": 98, - "low": 7, - "open": 11, - "volume": 930487 - }, - "open": "2020-06-15T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 592130, - "high": 31, - "low": 34, - "open": 7843, - "volume": 5001222 - }, - "id": 2636330, - "non_hive": { - "close": 150439, - "high": 8, - "low": 8, - "open": 1999, - "volume": 1264981 - }, - "open": "2020-06-15T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 3, - "low": 59, - "open": 7876, - "volume": 3723651 - }, - "id": 2636385, - "non_hive": { - "close": 11, - "high": 1, - "low": 14, - "open": 2000, - "volume": 939158 - }, - "open": "2020-06-15T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100542, - "high": 35, - "low": 96349, - "open": 7885, - "volume": 5238936 - }, - "id": 2636432, - "non_hive": { - "close": 25157, - "high": 9, - "low": 24087, - "open": 1989, - "volume": 1317044 - }, - "open": "2020-06-15T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1744, - "high": 3333, - "low": 10, - "open": 3333, - "volume": 3690065 - }, - "id": 2636480, - "non_hive": { - "close": 443, - "high": 849, - "low": 2, - "open": 849, - "volume": 923536 - }, - "open": "2020-06-15T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7901, - "high": 1394, - "low": 30, - "open": 1394, - "volume": 5327365 - }, - "id": 2636520, - "non_hive": { - "close": 1999, - "high": 354, - "low": 7, - "open": 354, - "volume": 1339590 - }, - "open": "2020-06-15T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41, - "high": 3336, - "low": 99, - "open": 3027, - "volume": 3792837 - }, - "id": 2636566, - "non_hive": { - "close": 10, - "high": 848, - "low": 24, - "open": 766, - "volume": 944285 - }, - "open": "2020-06-15T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7953, - "high": 265, - "low": 5, - "open": 8049, - "volume": 5210526 - }, - "id": 2636618, - "non_hive": { - "close": 2001, - "high": 67, - "low": 1, - "open": 1990, - "volume": 1293609 - }, - "open": "2020-06-15T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7432, - "high": 6710, - "low": 39, - "open": 8076, - "volume": 3690382 - }, - "id": 2636674, - "non_hive": { - "close": 1859, - "high": 1688, - "low": 9, - "open": 1999, - "volume": 911476 - }, - "open": "2020-06-15T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 151, - "high": 151, - "low": 72, - "open": 621, - "volume": 2753667 - }, - "id": 2636725, - "non_hive": { - "close": 38, - "high": 38, - "low": 17, - "open": 155, - "volume": 679459 - }, - "open": "2020-06-15T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1943, - "high": 79, - "low": 127, - "open": 8104, - "volume": 3753504 - }, - "id": 2636767, - "non_hive": { - "close": 486, - "high": 20, - "low": 31, - "open": 2000, - "volume": 924965 - }, - "open": "2020-06-15T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2853, - "high": 655, - "low": 76, - "open": 102, - "volume": 2382468 - }, - "id": 2636817, - "non_hive": { - "close": 713, - "high": 164, - "low": 18, - "open": 25, - "volume": 588165 - }, - "open": "2020-06-15T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28841, - "high": 596, - "low": 13, - "open": 41, - "volume": 414079 - }, - "id": 2636881, - "non_hive": { - "close": 7200, - "high": 149, - "low": 3, - "open": 10, - "volume": 103261 - }, - "open": "2020-06-15T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 96, - "high": 44, - "low": 121, - "open": 4006, - "volume": 405082 - }, - "id": 2636928, - "non_hive": { - "close": 24, - "high": 11, - "low": 30, - "open": 1000, - "volume": 101088 - }, - "open": "2020-06-15T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 212, - "low": 9903, - "open": 57007, - "volume": 430506 - }, - "id": 2637000, - "non_hive": { - "close": 2497, - "high": 53, - "low": 2470, - "open": 14246, - "volume": 107500 - }, - "open": "2020-06-15T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40, - "high": 620, - "low": 10, - "open": 27090, - "volume": 162284 - }, - "id": 2637045, - "non_hive": { - "close": 10, - "high": 155, - "low": 2, - "open": 6769, - "volume": 40547 - }, - "open": "2020-06-15T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 797652, - "high": 39, - "low": 571023, - "open": 768, - "volume": 5096306 - }, - "id": 2637086, - "non_hive": { - "close": 200961, - "high": 10, - "low": 142116, - "open": 192, - "volume": 1274610 - }, - "open": "2020-06-15T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36, - "high": 134, - "low": 36, - "open": 8033, - "volume": 2056337 - }, - "id": 2637134, - "non_hive": { - "close": 8, - "high": 34, - "low": 8, - "open": 2000, - "volume": 513120 - }, - "open": "2020-06-15T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10008, - "high": 23, - "low": 9, - "open": 41, - "volume": 309481 - }, - "id": 2637172, - "non_hive": { - "close": 2521, - "high": 6, - "low": 2, - "open": 10, - "volume": 77682 - }, - "open": "2020-06-15T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26677, - "high": 174, - "low": 7, - "open": 61563, - "volume": 140589 - }, - "id": 2637215, - "non_hive": { - "close": 6720, - "high": 44, - "low": 1, - "open": 15507, - "volume": 35394 - }, - "open": "2020-06-15T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2337, - "high": 91, - "low": 12, - "open": 4191, - "volume": 752248 - }, - "id": 2637245, - "non_hive": { - "close": 589, - "high": 23, - "low": 3, - "open": 1055, - "volume": 189544 - }, - "open": "2020-06-15T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 781, - "high": 19, - "low": 33, - "open": 9361, - "volume": 80616 - }, - "id": 2637278, - "non_hive": { - "close": 197, - "high": 5, - "low": 8, - "open": 2359, - "volume": 20316 - }, - "open": "2020-06-16T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 368917, - "high": 374826, - "low": 6, - "open": 10631, - "volume": 3525182 - }, - "id": 2637308, - "non_hive": { - "close": 92553, - "high": 95640, - "low": 1, - "open": 2679, - "volume": 887106 - }, - "open": "2020-06-16T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 387056, - "high": 19746, - "low": 22, - "open": 7970, - "volume": 3711942 - }, - "id": 2637345, - "non_hive": { - "close": 98023, - "high": 5013, - "low": 5, - "open": 2000, - "volume": 931384 - }, - "open": "2020-06-16T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 351, - "high": 11, - "low": 13, - "open": 44, - "volume": 2387589 - }, - "id": 2637385, - "non_hive": { - "close": 89, - "high": 3, - "low": 3, - "open": 11, - "volume": 600346 - }, - "open": "2020-06-16T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 585862, - "high": 1074, - "low": 189, - "open": 397, - "volume": 1345841 - }, - "id": 2637408, - "non_hive": { - "close": 147062, - "high": 272, - "low": 46, - "open": 100, - "volume": 338128 - }, - "open": "2020-06-16T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 427, - "high": 50, - "low": 493111, - "open": 50, - "volume": 4295674 - }, - "id": 2637433, - "non_hive": { - "close": 108, - "high": 13, - "low": 123779, - "open": 13, - "volume": 1086259 - }, - "open": "2020-06-16T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1421, - "high": 27, - "low": 15, - "open": 8491, - "volume": 3704034 - }, - "id": 2637484, - "non_hive": { - "close": 362, - "high": 7, - "low": 3, - "open": 2167, - "volume": 930904 - }, - "open": "2020-06-16T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 298, - "high": 1036, - "low": 298, - "open": 6428, - "volume": 1876117 - }, - "id": 2637543, - "non_hive": { - "close": 74, - "high": 264, - "low": 74, - "open": 1637, - "volume": 470894 - }, - "open": "2020-06-16T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 175152, - "high": 27, - "low": 539939, - "open": 7966, - "volume": 2819714 - }, - "id": 2637572, - "non_hive": { - "close": 44510, - "high": 7, - "low": 135032, - "open": 2000, - "volume": 710446 - }, - "open": "2020-06-16T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 795, - "high": 413, - "low": 29, - "open": 44, - "volume": 2639249 - }, - "id": 2637609, - "non_hive": { - "close": 202, - "high": 105, - "low": 7, - "open": 11, - "volume": 661591 - }, - "open": "2020-06-16T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 851, - "high": 23, - "low": 23, - "open": 5003, - "volume": 1309380 - }, - "id": 2637664, - "non_hive": { - "close": 216, - "high": 6, - "low": 5, - "open": 1270, - "volume": 328030 - }, - "open": "2020-06-16T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 169, - "high": 47, - "low": 13, - "open": 7165, - "volume": 642207 - }, - "id": 2637698, - "non_hive": { - "close": 43, - "high": 12, - "low": 3, - "open": 1817, - "volume": 161324 - }, - "open": "2020-06-16T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9184, - "high": 23, - "low": 515, - "open": 86, - "volume": 940905 - }, - "id": 2637733, - "non_hive": { - "close": 2330, - "high": 6, - "low": 130, - "open": 22, - "volume": 238408 - }, - "open": "2020-06-16T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4181, - "high": 39, - "low": 264617, - "open": 15322, - "volume": 435250 - }, - "id": 2637774, - "non_hive": { - "close": 1060, - "high": 10, - "low": 66895, - "open": 3887, - "volume": 110179 - }, - "open": "2020-06-16T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32, - "high": 441, - "low": 5, - "open": 455, - "volume": 3029288 - }, - "id": 2637829, - "non_hive": { - "close": 8, - "high": 112, - "low": 1, - "open": 115, - "volume": 766461 - }, - "open": "2020-06-16T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 52771, - "high": 19, - "low": 44, - "open": 135772, - "volume": 3452002 - }, - "id": 2637884, - "non_hive": { - "close": 13361, - "high": 5, - "low": 11, - "open": 34446, - "volume": 872071 - }, - "open": "2020-06-16T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19, - "high": 19, - "low": 9, - "open": 201, - "volume": 1271779 - }, - "id": 2637949, - "non_hive": { - "close": 5, - "high": 5, - "low": 2, - "open": 51, - "volume": 319524 - }, - "open": "2020-06-16T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 68, - "high": 302, - "low": 68, - "open": 32819, - "volume": 2164900 - }, - "id": 2637975, - "non_hive": { - "close": 17, - "high": 77, - "low": 17, - "open": 8351, - "volume": 545264 - }, - "open": "2020-06-16T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 230440, - "high": 31, - "low": 16, - "open": 620, - "volume": 3049181 - }, - "id": 2638018, - "non_hive": { - "close": 57717, - "high": 8, - "low": 4, - "open": 158, - "volume": 764757 - }, - "open": "2020-06-16T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1125, - "high": 19, - "low": 30, - "open": 1013, - "volume": 249657 - }, - "id": 2638057, - "non_hive": { - "close": 282, - "high": 5, - "low": 7, - "open": 258, - "volume": 63480 - }, - "open": "2020-06-16T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3837, - "high": 19, - "low": 486, - "open": 6611, - "volume": 238532 - }, - "id": 2638091, - "non_hive": { - "close": 963, - "high": 5, - "low": 121, - "open": 1682, - "volume": 60051 - }, - "open": "2020-06-16T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39395, - "high": 3, - "low": 5, - "open": 7865, - "volume": 1033033 - }, - "id": 2638125, - "non_hive": { - "close": 10000, - "high": 1, - "low": 1, - "open": 1999, - "volume": 260569 - }, - "open": "2020-06-16T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 207025, - "high": 114, - "low": 5, - "open": 12, - "volume": 3935886 - }, - "id": 2638162, - "non_hive": { - "close": 52455, - "high": 29, - "low": 1, - "open": 3, - "volume": 990557 - }, - "open": "2020-06-16T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5132, - "high": 35, - "low": 12, - "open": 3948, - "volume": 1540504 - }, - "id": 2638249, - "non_hive": { - "close": 1300, - "high": 9, - "low": 3, - "open": 1000, - "volume": 390150 - }, - "open": "2020-06-16T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 402607, - "high": 32361, - "low": 162, - "open": 8371, - "volume": 1901314 - }, - "id": 2638297, - "non_hive": { - "close": 102380, - "high": 8230, - "low": 40, - "open": 2123, - "volume": 480666 - }, - "open": "2020-06-17T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11618, - "high": 251, - "low": 395021, - "open": 7974, - "volume": 2976470 - }, - "id": 2638350, - "non_hive": { - "close": 2918, - "high": 64, - "low": 99056, - "open": 2000, - "volume": 753288 - }, - "open": "2020-06-17T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 302, - "high": 3, - "low": 4, - "open": 7873, - "volume": 1157723 - }, - "id": 2638388, - "non_hive": { - "close": 77, - "high": 1, - "low": 1, - "open": 1999, - "volume": 293010 - }, - "open": "2020-06-17T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 15, - "low": 4, - "open": 4, - "volume": 3128253 - }, - "id": 2638432, - "non_hive": { - "close": 11, - "high": 4, - "low": 1, - "open": 1, - "volume": 789728 - }, - "open": "2020-06-17T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7968, - "high": 39378, - "low": 12, - "open": 12, - "volume": 2455813 - }, - "id": 2638479, - "non_hive": { - "close": 2000, - "high": 10001, - "low": 3, - "open": 3, - "volume": 616531 - }, - "open": "2020-06-17T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7915, - "high": 5886, - "low": 2000, - "open": 343417, - "volume": 625801 - }, - "id": 2638505, - "non_hive": { - "close": 1999, - "high": 1490, - "low": 501, - "open": 86181, - "volume": 157070 - }, - "open": "2020-06-17T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 397, - "high": 395, - "low": 397, - "open": 19140, - "volume": 607760 - }, - "id": 2638522, - "non_hive": { - "close": 100, - "high": 100, - "low": 100, - "open": 4834, - "volume": 153537 - }, - "open": "2020-06-17T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4613, - "high": 704, - "low": 7557, - "open": 7557, - "volume": 1770263 - }, - "id": 2638558, - "non_hive": { - "close": 1171, - "high": 179, - "low": 1909, - "open": 1909, - "volume": 448298 - }, - "open": "2020-06-17T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1679, - "high": 59, - "low": 15255, - "open": 5111, - "volume": 124743 - }, - "id": 2638587, - "non_hive": { - "close": 426, - "high": 15, - "low": 3870, - "open": 1297, - "volume": 31650 - }, - "open": "2020-06-17T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 951689, - "low": 44, - "open": 1885, - "volume": 7816253 - }, - "id": 2638623, - "non_hive": { - "close": 11, - "high": 247083, - "low": 11, - "open": 478, - "volume": 1994116 - }, - "open": "2020-06-17T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1098, - "high": 7, - "low": 12, - "open": 411194, - "volume": 1071830 - }, - "id": 2638673, - "non_hive": { - "close": 285, - "high": 2, - "low": 3, - "open": 104550, - "volume": 272904 - }, - "open": "2020-06-17T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3852, - "high": 50, - "low": 6902, - "open": 6902, - "volume": 159273 - }, - "id": 2638704, - "non_hive": { - "close": 1000, - "high": 13, - "low": 1791, - "open": 1791, - "volume": 41337 - }, - "open": "2020-06-17T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 3, - "low": 100, - "open": 7843, - "volume": 13111907 - }, - "id": 2638744, - "non_hive": { - "close": 25, - "high": 1, - "low": 25, - "open": 2000, - "volume": 3378989 - }, - "open": "2020-06-17T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22943, - "high": 18999, - "low": 200, - "open": 2688, - "volume": 113145 - }, - "id": 2638837, - "non_hive": { - "close": 5965, - "high": 4995, - "low": 51, - "open": 698, - "volume": 29427 - }, - "open": "2020-06-17T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1812, - "high": 19, - "low": 102, - "open": 102, - "volume": 2588614 - }, - "id": 2638874, - "non_hive": { - "close": 469, - "high": 5, - "low": 26, - "open": 26, - "volume": 662630 - }, - "open": "2020-06-17T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 301, - "low": 28, - "open": 28, - "volume": 4113618 - }, - "id": 2638917, - "non_hive": { - "close": 11, - "high": 78, - "low": 7, - "open": 7, - "volume": 1049023 - }, - "open": "2020-06-17T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 98, - "high": 3444, - "low": 12, - "open": 7783, - "volume": 3932312 - }, - "id": 2638979, - "non_hive": { - "close": 25, - "high": 885, - "low": 3, - "open": 1999, - "volume": 990949 - }, - "open": "2020-06-17T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44428, - "high": 122, - "low": 14, - "open": 203, - "volume": 3781721 - }, - "id": 2639033, - "non_hive": { - "close": 11136, - "high": 31, - "low": 3, - "open": 51, - "volume": 948475 - }, - "open": "2020-06-17T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3225, - "high": 11062, - "low": 8, - "open": 620, - "volume": 3647553 - }, - "id": 2639078, - "non_hive": { - "close": 818, - "high": 2807, - "low": 2, - "open": 157, - "volume": 915032 - }, - "open": "2020-06-17T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7959, - "high": 176, - "low": 33884, - "open": 4657, - "volume": 3551304 - }, - "id": 2639109, - "non_hive": { - "close": 2000, - "high": 45, - "low": 8493, - "open": 1181, - "volume": 894978 - }, - "open": "2020-06-17T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2840, - "high": 3, - "low": 4, - "open": 1420, - "volume": 4151454 - }, - "id": 2639152, - "non_hive": { - "close": 721, - "high": 1, - "low": 1, - "open": 361, - "volume": 1048236 - }, - "open": "2020-06-17T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 141, - "low": 44, - "open": 55, - "volume": 3438924 - }, - "id": 2639237, - "non_hive": { - "close": 11, - "high": 36, - "low": 11, - "open": 14, - "volume": 863736 - }, - "open": "2020-06-17T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111, - "high": 63, - "low": 19, - "open": 12, - "volume": 1721126 - }, - "id": 2639282, - "non_hive": { - "close": 27, - "high": 16, - "low": 4, - "open": 3, - "volume": 433101 - }, - "open": "2020-06-17T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1296, - "high": 51, - "low": 154, - "open": 275, - "volume": 1515031 - }, - "id": 2639327, - "non_hive": { - "close": 329, - "high": 13, - "low": 38, - "open": 70, - "volume": 382629 - }, - "open": "2020-06-17T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 443210, - "high": 35, - "low": 52, - "open": 68881, - "volume": 1937391 - }, - "id": 2639372, - "non_hive": { - "close": 111165, - "high": 9, - "low": 13, - "open": 17481, - "volume": 486441 - }, - "open": "2020-06-18T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 256, - "high": 736, - "low": 545687, - "open": 87, - "volume": 1893519 - }, - "id": 2639406, - "non_hive": { - "close": 65, - "high": 187, - "low": 136967, - "open": 22, - "volume": 475599 - }, - "open": "2020-06-18T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 239073, - "high": 1619, - "low": 7, - "open": 5900, - "volume": 3199817 - }, - "id": 2639435, - "non_hive": { - "close": 59944, - "high": 411, - "low": 1, - "open": 1496, - "volume": 803568 - }, - "open": "2020-06-18T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 6028, - "low": 44, - "open": 434, - "volume": 3630440 - }, - "id": 2639483, - "non_hive": { - "close": 11, - "high": 1529, - "low": 11, - "open": 110, - "volume": 910370 - }, - "open": "2020-06-18T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 176794, - "high": 7854, - "low": 12, - "open": 12, - "volume": 3861075 - }, - "id": 2639524, - "non_hive": { - "close": 44328, - "high": 1990, - "low": 3, - "open": 3, - "volume": 968699 - }, - "open": "2020-06-18T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1500, - "high": 23, - "low": 88815, - "open": 1670, - "volume": 3724724 - }, - "id": 2639556, - "non_hive": { - "close": 379, - "high": 6, - "low": 22261, - "open": 422, - "volume": 934150 - }, - "open": "2020-06-18T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 585766, - "high": 55, - "low": 2212, - "open": 1423, - "volume": 3646301 - }, - "id": 2639608, - "non_hive": { - "close": 146851, - "high": 14, - "low": 554, - "open": 357, - "volume": 914220 - }, - "open": "2020-06-18T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 435, - "high": 43, - "low": 107233, - "open": 530199, - "volume": 3629279 - }, - "id": 2639642, - "non_hive": { - "close": 110, - "high": 11, - "low": 26883, - "open": 132921, - "volume": 909955 - }, - "open": "2020-06-18T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 226564, - "high": 79, - "low": 226564, - "open": 665, - "volume": 3010910 - }, - "id": 2639675, - "non_hive": { - "close": 56746, - "high": 20, - "low": 56746, - "open": 168, - "volume": 754501 - }, - "open": "2020-06-18T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 47603, - "low": 188359, - "open": 39926, - "volume": 1930733 - }, - "id": 2639702, - "non_hive": { - "close": 11, - "high": 12024, - "low": 47089, - "open": 10000, - "volume": 482960 - }, - "open": "2020-06-18T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100188, - "high": 23, - "low": 2671, - "open": 447, - "volume": 250383 - }, - "id": 2639743, - "non_hive": { - "close": 25173, - "high": 6, - "low": 667, - "open": 112, - "volume": 62894 - }, - "open": "2020-06-18T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3985, - "high": 2759, - "low": 94270, - "open": 7998, - "volume": 954787 - }, - "id": 2639780, - "non_hive": { - "close": 1001, - "high": 697, - "low": 23567, - "open": 2000, - "volume": 239242 - }, - "open": "2020-06-18T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3934, - "high": 3, - "low": 77, - "open": 401, - "volume": 301431 - }, - "id": 2639822, - "non_hive": { - "close": 983, - "high": 1, - "low": 19, - "open": 100, - "volume": 75750 - }, - "open": "2020-06-18T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1540, - "high": 31, - "low": 21, - "open": 3974, - "volume": 4316403 - }, - "id": 2639872, - "non_hive": { - "close": 385, - "high": 8, - "low": 5, - "open": 998, - "volume": 1074553 - }, - "open": "2020-06-18T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 64046, - "high": 48, - "low": 36, - "open": 48, - "volume": 1936693 - }, - "id": 2639930, - "non_hive": { - "close": 15825, - "high": 12, - "low": 8, - "open": 12, - "volume": 478727 - }, - "open": "2020-06-18T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28988, - "high": 401454, - "low": 5, - "open": 29, - "volume": 553597 - }, - "id": 2639968, - "non_hive": { - "close": 7246, - "high": 100381, - "low": 1, - "open": 7, - "volume": 138318 - }, - "open": "2020-06-18T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 128, - "high": 160, - "low": 13, - "open": 13, - "volume": 1982690 - }, - "id": 2640017, - "non_hive": { - "close": 32, - "high": 40, - "low": 3, - "open": 3, - "volume": 487507 - }, - "open": "2020-06-18T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7000, - "high": 108, - "low": 8, - "open": 11000, - "volume": 3158180 - }, - "id": 2640059, - "non_hive": { - "close": 1736, - "high": 27, - "low": 1, - "open": 2735, - "volume": 776485 - }, - "open": "2020-06-18T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59260, - "high": 4, - "low": 7000, - "open": 645, - "volume": 305596 - }, - "id": 2640120, - "non_hive": { - "close": 14699, - "high": 1, - "low": 1731, - "open": 160, - "volume": 75785 - }, - "open": "2020-06-18T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16055, - "high": 748, - "low": 56, - "open": 12931, - "volume": 926677 - }, - "id": 2640162, - "non_hive": { - "close": 4001, - "high": 187, - "low": 13, - "open": 3231, - "volume": 229100 - }, - "open": "2020-06-18T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4006, - "high": 96, - "low": 46, - "open": 8125, - "volume": 242401 - }, - "id": 2640194, - "non_hive": { - "close": 998, - "high": 24, - "low": 11, - "open": 2000, - "volume": 59876 - }, - "open": "2020-06-18T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 629, - "high": 610, - "low": 51575, - "open": 610, - "volume": 746532 - }, - "id": 2640229, - "non_hive": { - "close": 156, - "high": 152, - "low": 12478, - "open": 152, - "volume": 183361 - }, - "open": "2020-06-18T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 591774, - "high": 26936, - "low": 13, - "open": 42, - "volume": 3113335 - }, - "id": 2640252, - "non_hive": { - "close": 143845, - "high": 6676, - "low": 3, - "open": 10, - "volume": 757311 - }, - "open": "2020-06-18T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22, - "high": 4047, - "low": 15, - "open": 8103, - "volume": 1701782 - }, - "id": 2640294, - "non_hive": { - "close": 5, - "high": 1000, - "low": 3, - "open": 1999, - "volume": 419834 - }, - "open": "2020-06-18T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29, - "high": 52, - "low": 10, - "open": 22, - "volume": 2997874 - }, - "id": 2640321, - "non_hive": { - "close": 7, - "high": 13, - "low": 2, - "open": 5, - "volume": 733823 - }, - "open": "2020-06-19T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 669, - "high": 669, - "low": 29, - "open": 3887, - "volume": 1701568 - }, - "id": 2640353, - "non_hive": { - "close": 165, - "high": 165, - "low": 7, - "open": 958, - "volume": 417693 - }, - "open": "2020-06-19T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35, - "high": 16, - "low": 35, - "open": 8215, - "volume": 666191 - }, - "id": 2640390, - "non_hive": { - "close": 8, - "high": 4, - "low": 8, - "open": 2000, - "volume": 161869 - }, - "open": "2020-06-19T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 215067, - "high": 220, - "low": 15, - "open": 220, - "volume": 1417409 - }, - "id": 2640421, - "non_hive": { - "close": 51616, - "high": 54, - "low": 3, - "open": 54, - "volume": 341619 - }, - "open": "2020-06-19T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 102, - "high": 102, - "low": 13, - "open": 42, - "volume": 1371433 - }, - "id": 2640453, - "non_hive": { - "close": 25, - "high": 25, - "low": 3, - "open": 10, - "volume": 330434 - }, - "open": "2020-06-19T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7621, - "high": 98, - "low": 318, - "open": 7920, - "volume": 1108665 - }, - "id": 2640493, - "non_hive": { - "close": 1854, - "high": 24, - "low": 76, - "open": 1929, - "volume": 267905 - }, - "open": "2020-06-19T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36, - "high": 333, - "low": 28, - "open": 8263, - "volume": 325499 - }, - "id": 2640529, - "non_hive": { - "close": 8, - "high": 81, - "low": 6, - "open": 2000, - "volume": 79082 - }, - "open": "2020-06-19T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 534, - "high": 4, - "low": 38, - "open": 27101, - "volume": 1163676 - }, - "id": 2640561, - "non_hive": { - "close": 130, - "high": 1, - "low": 9, - "open": 6587, - "volume": 282072 - }, - "open": "2020-06-19T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1210, - "high": 222, - "low": 42290, - "open": 222, - "volume": 92602 - }, - "id": 2640588, - "non_hive": { - "close": 294, - "high": 54, - "low": 10275, - "open": 54, - "volume": 22500 - }, - "open": "2020-06-19T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12379, - "high": 20, - "low": 115785, - "open": 2906, - "volume": 952608 - }, - "id": 2640602, - "non_hive": { - "close": 3007, - "high": 5, - "low": 28043, - "open": 706, - "volume": 231370 - }, - "open": "2020-06-19T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 85, - "high": 85, - "low": 13, - "open": 15832, - "volume": 2229022 - }, - "id": 2640641, - "non_hive": { - "close": 21, - "high": 21, - "low": 3, - "open": 3847, - "volume": 540142 - }, - "open": "2020-06-19T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 146, - "open": 27211, - "volume": 132834 - }, - "id": 2640676, - "non_hive": { - "close": 1, - "high": 1, - "low": 35, - "open": 6667, - "volume": 32536 - }, - "open": "2020-06-19T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6901, - "high": 220, - "low": 69, - "open": 4212, - "volume": 1966757 - }, - "id": 2640697, - "non_hive": { - "close": 1688, - "high": 54, - "low": 16, - "open": 1031, - "volume": 476673 - }, - "open": "2020-06-19T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3045, - "high": 36, - "low": 46, - "open": 1272, - "volume": 2875979 - }, - "id": 2640743, - "non_hive": { - "close": 744, - "high": 9, - "low": 11, - "open": 311, - "volume": 697436 - }, - "open": "2020-06-19T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 310, - "high": 171, - "low": 63, - "open": 37, - "volume": 2525363 - }, - "id": 2640780, - "non_hive": { - "close": 75, - "high": 42, - "low": 15, - "open": 9, - "volume": 611548 - }, - "open": "2020-06-19T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12395, - "high": 1977, - "low": 13, - "open": 29, - "volume": 30329 - }, - "id": 2640830, - "non_hive": { - "close": 3027, - "high": 483, - "low": 3, - "open": 7, - "volume": 7397 - }, - "open": "2020-06-19T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4802, - "high": 196, - "low": 13, - "open": 196, - "volume": 896388 - }, - "id": 2640862, - "non_hive": { - "close": 1172, - "high": 48, - "low": 3, - "open": 48, - "volume": 217352 - }, - "open": "2020-06-19T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9000, - "high": 313983, - "low": 65, - "open": 313983, - "volume": 1009530 - }, - "id": 2640894, - "non_hive": { - "close": 2188, - "high": 76617, - "low": 15, - "open": 76617, - "volume": 244394 - }, - "open": "2020-06-19T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 305, - "high": 16, - "low": 45, - "open": 12333, - "volume": 652317 - }, - "id": 2640921, - "non_hive": { - "close": 74, - "high": 4, - "low": 10, - "open": 3000, - "volume": 156944 - }, - "open": "2020-06-19T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2033, - "high": 2033, - "low": 350, - "open": 5215, - "volume": 162085 - }, - "id": 2640949, - "non_hive": { - "close": 494, - "high": 494, - "low": 84, - "open": 1263, - "volume": 39357 - }, - "open": "2020-06-19T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34243, - "high": 4, - "low": 8295, - "open": 2239, - "volume": 119006 - }, - "id": 2640978, - "non_hive": { - "close": 8287, - "high": 1, - "low": 1999, - "open": 544, - "volume": 28738 - }, - "open": "2020-06-19T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8817, - "high": 8817, - "low": 85134, - "open": 5904, - "volume": 1556200 - }, - "id": 2640998, - "non_hive": { - "close": 2153, - "high": 2153, - "low": 20425, - "open": 1429, - "volume": 376317 - }, - "open": "2020-06-19T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3977, - "high": 2939, - "low": 6, - "open": 2432, - "volume": 868433 - }, - "id": 2641041, - "non_hive": { - "close": 970, - "high": 718, - "low": 1, - "open": 594, - "volume": 211315 - }, - "open": "2020-06-19T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 746, - "high": 45, - "low": 13, - "open": 1374, - "volume": 1268576 - }, - "id": 2641099, - "non_hive": { - "close": 182, - "high": 11, - "low": 3, - "open": 335, - "volume": 309373 - }, - "open": "2020-06-19T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 162054, - "high": 30636, - "low": 54, - "open": 21438, - "volume": 2814861 - }, - "id": 2641148, - "non_hive": { - "close": 39509, - "high": 7474, - "low": 13, - "open": 5230, - "volume": 685537 - }, - "open": "2020-06-20T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 373, - "high": 373, - "low": 83, - "open": 83, - "volume": 2282292 - }, - "id": 2641186, - "non_hive": { - "close": 91, - "high": 91, - "low": 20, - "open": 20, - "volume": 555369 - }, - "open": "2020-06-20T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7382, - "high": 45, - "low": 4411, - "open": 45, - "volume": 56996 - }, - "id": 2641207, - "non_hive": { - "close": 1799, - "high": 11, - "low": 1067, - "open": 11, - "volume": 13861 - }, - "open": "2020-06-20T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7428, - "high": 578, - "low": 12451, - "open": 1137, - "volume": 1361765 - }, - "id": 2641225, - "non_hive": { - "close": 1811, - "high": 141, - "low": 3033, - "open": 277, - "volume": 331951 - }, - "open": "2020-06-20T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1817, - "high": 360, - "low": 13, - "open": 27698, - "volume": 345954 - }, - "id": 2641240, - "non_hive": { - "close": 443, - "high": 88, - "low": 3, - "open": 6753, - "volume": 84344 - }, - "open": "2020-06-20T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 159918, - "high": 32, - "low": 598139, - "open": 352, - "volume": 4170111 - }, - "id": 2641263, - "non_hive": { - "close": 38988, - "high": 8, - "low": 145826, - "open": 86, - "volume": 1016677 - }, - "open": "2020-06-20T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8613, - "high": 61, - "low": 590543, - "open": 3358, - "volume": 3653479 - }, - "id": 2641305, - "non_hive": { - "close": 2105, - "high": 15, - "low": 143974, - "open": 819, - "volume": 890745 - }, - "open": "2020-06-20T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 119364, - "high": 377, - "low": 436, - "open": 12985, - "volume": 2618222 - }, - "id": 2641346, - "non_hive": { - "close": 29076, - "high": 93, - "low": 106, - "open": 3176, - "volume": 638409 - }, - "open": "2020-06-20T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3379, - "high": 1708, - "low": 27, - "open": 4542, - "volume": 71270 - }, - "id": 2641375, - "non_hive": { - "close": 832, - "high": 421, - "low": 6, - "open": 1118, - "volume": 17506 - }, - "open": "2020-06-20T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 8117, - "open": 8117, - "volume": 286946 - }, - "id": 2641396, - "non_hive": { - "close": 1, - "high": 1, - "low": 1999, - "open": 1999, - "volume": 70675 - }, - "open": "2020-06-20T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13748, - "high": 2297, - "low": 13, - "open": 41, - "volume": 1843139 - }, - "id": 2641419, - "non_hive": { - "close": 3382, - "high": 566, - "low": 3, - "open": 10, - "volume": 449858 - }, - "open": "2020-06-20T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60709, - "high": 365, - "low": 787, - "open": 13178, - "volume": 224480 - }, - "id": 2641449, - "non_hive": { - "close": 14915, - "high": 90, - "low": 193, - "open": 3239, - "volume": 55166 - }, - "open": "2020-06-20T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 73601, - "high": 4064, - "low": 100, - "open": 4064, - "volume": 1593491 - }, - "id": 2641487, - "non_hive": { - "close": 18030, - "high": 999, - "low": 24, - "open": 999, - "volume": 389295 - }, - "open": "2020-06-20T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13203, - "high": 976, - "low": 524, - "open": 95175, - "volume": 4466534 - }, - "id": 2641519, - "non_hive": { - "close": 3314, - "high": 245, - "low": 128, - "open": 23313, - "volume": 1100086 - }, - "open": "2020-06-20T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25542, - "high": 3, - "low": 735, - "open": 675, - "volume": 1530211 - }, - "id": 2641572, - "non_hive": { - "close": 6385, - "high": 1, - "low": 182, - "open": 168, - "volume": 380249 - }, - "open": "2020-06-20T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 976, - "high": 64, - "low": 29, - "open": 6887, - "volume": 2045020 - }, - "id": 2641616, - "non_hive": { - "close": 242, - "high": 16, - "low": 7, - "open": 1721, - "volume": 505924 - }, - "open": "2020-06-20T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1054, - "high": 44, - "low": 13, - "open": 23838, - "volume": 581060 - }, - "id": 2641671, - "non_hive": { - "close": 261, - "high": 11, - "low": 3, - "open": 5906, - "volume": 143877 - }, - "open": "2020-06-20T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12655, - "high": 969, - "low": 251, - "open": 21728, - "volume": 2011767 - }, - "id": 2641722, - "non_hive": { - "close": 3159, - "high": 242, - "low": 62, - "open": 5376, - "volume": 498227 - }, - "open": "2020-06-20T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5268, - "high": 31, - "low": 12093, - "open": 6872, - "volume": 3562383 - }, - "id": 2641760, - "non_hive": { - "close": 1322, - "high": 8, - "low": 3018, - "open": 1716, - "volume": 890146 - }, - "open": "2020-06-20T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4237, - "high": 533, - "low": 13, - "open": 2223, - "volume": 1382511 - }, - "id": 2641811, - "non_hive": { - "close": 1059, - "high": 134, - "low": 3, - "open": 558, - "volume": 346861 - }, - "open": "2020-06-20T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2864, - "high": 3776, - "low": 13344, - "open": 9112, - "volume": 128624 - }, - "id": 2641864, - "non_hive": { - "close": 716, - "high": 944, - "low": 3334, - "open": 2277, - "volume": 32147 - }, - "open": "2020-06-20T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 526, - "high": 247, - "low": 10828, - "open": 10828, - "volume": 406625 - }, - "id": 2641896, - "non_hive": { - "close": 132, - "high": 62, - "low": 2706, - "open": 2706, - "volume": 101800 - }, - "open": "2020-06-20T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60467, - "high": 235, - "low": 29, - "open": 41, - "volume": 124436 - }, - "id": 2641935, - "non_hive": { - "close": 15111, - "high": 59, - "low": 7, - "open": 10, - "volume": 31131 - }, - "open": "2020-06-20T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 996, - "high": 3325, - "low": 290, - "open": 107, - "volume": 1091560 - }, - "id": 2641974, - "non_hive": { - "close": 254, - "high": 848, - "low": 72, - "open": 27, - "volume": 274935 - }, - "open": "2020-06-20T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24845, - "high": 3876, - "low": 52, - "open": 713, - "volume": 183354 - }, - "id": 2642016, - "non_hive": { - "close": 6321, - "high": 988, - "low": 13, - "open": 181, - "volume": 46569 - }, - "open": "2020-06-21T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1369, - "high": 2244, - "low": 54, - "open": 3443, - "volume": 1841167 - }, - "id": 2642042, - "non_hive": { - "close": 348, - "high": 572, - "low": 13, - "open": 874, - "volume": 461474 - }, - "open": "2020-06-21T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 500340, - "high": 165, - "low": 23, - "open": 3734, - "volume": 3050356 - }, - "id": 2642073, - "non_hive": { - "close": 125131, - "high": 42, - "low": 5, - "open": 949, - "volume": 763579 - }, - "open": "2020-06-21T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 592004, - "high": 12625, - "low": 168968, - "open": 7943, - "volume": 3035085 - }, - "id": 2642115, - "non_hive": { - "close": 148056, - "high": 3179, - "low": 42257, - "open": 1999, - "volume": 759361 - }, - "open": "2020-06-21T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 186130, - "high": 557, - "low": 44, - "open": 44, - "volume": 201037 - }, - "id": 2642140, - "non_hive": { - "close": 46747, - "high": 140, - "low": 11, - "open": 11, - "volume": 50491 - }, - "open": "2020-06-21T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3514, - "high": 277, - "low": 190450, - "open": 10255, - "volume": 1228114 - }, - "id": 2642158, - "non_hive": { - "close": 887, - "high": 70, - "low": 47254, - "open": 2574, - "volume": 306784 - }, - "open": "2020-06-21T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25088, - "high": 95, - "low": 25088, - "open": 13566, - "volume": 430023 - }, - "id": 2642185, - "non_hive": { - "close": 6252, - "high": 24, - "low": 6252, - "open": 3423, - "volume": 108208 - }, - "open": "2020-06-21T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12169, - "high": 12169, - "low": 13022, - "open": 13022, - "volume": 2705967 - }, - "id": 2642220, - "non_hive": { - "close": 3070, - "high": 3070, - "low": 3240, - "open": 3240, - "volume": 676575 - }, - "open": "2020-06-21T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9167, - "high": 257, - "low": 5, - "open": 32915, - "volume": 320341 - }, - "id": 2642246, - "non_hive": { - "close": 2310, - "high": 65, - "low": 1, - "open": 8303, - "volume": 80781 - }, - "open": "2020-06-21T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1392, - "high": 22014, - "low": 1182, - "open": 198440, - "volume": 794149 - }, - "id": 2642280, - "non_hive": { - "close": 349, - "high": 5547, - "low": 295, - "open": 49999, - "volume": 199793 - }, - "open": "2020-06-21T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4949, - "high": 35, - "low": 345425, - "open": 2921, - "volume": 1150727 - }, - "id": 2642316, - "non_hive": { - "close": 1244, - "high": 9, - "low": 85998, - "open": 732, - "volume": 287625 - }, - "open": "2020-06-21T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5168, - "high": 163, - "low": 285984, - "open": 4553, - "volume": 1832501 - }, - "id": 2642362, - "non_hive": { - "close": 1292, - "high": 41, - "low": 71067, - "open": 1145, - "volume": 456169 - }, - "open": "2020-06-21T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14594, - "high": 14594, - "low": 5593, - "open": 13951, - "volume": 412762 - }, - "id": 2642386, - "non_hive": { - "close": 3656, - "high": 3656, - "low": 1400, - "open": 3494, - "volume": 103375 - }, - "open": "2020-06-21T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6433, - "high": 1345, - "low": 560, - "open": 27862, - "volume": 265443 - }, - "id": 2642409, - "non_hive": { - "close": 1610, - "high": 337, - "low": 140, - "open": 6977, - "volume": 66478 - }, - "open": "2020-06-21T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1410, - "high": 427, - "low": 829, - "open": 22133, - "volume": 215626 - }, - "id": 2642438, - "non_hive": { - "close": 353, - "high": 107, - "low": 207, - "open": 5541, - "volume": 53973 - }, - "open": "2020-06-21T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2945, - "high": 475, - "low": 1330, - "open": 32, - "volume": 267961 - }, - "id": 2642482, - "non_hive": { - "close": 736, - "high": 119, - "low": 332, - "open": 8, - "volume": 67056 - }, - "open": "2020-06-21T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 204, - "high": 919, - "low": 201323, - "open": 44, - "volume": 1261936 - }, - "id": 2642537, - "non_hive": { - "close": 51, - "high": 230, - "low": 49525, - "open": 11, - "volume": 312838 - }, - "open": "2020-06-21T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5500, - "high": 36484, - "low": 165, - "open": 36484, - "volume": 238329 - }, - "id": 2642569, - "non_hive": { - "close": 1366, - "high": 9121, - "low": 40, - "open": 9121, - "volume": 59524 - }, - "open": "2020-06-21T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9571, - "high": 104, - "low": 51, - "open": 8006, - "volume": 226649 - }, - "id": 2642610, - "non_hive": { - "close": 2390, - "high": 26, - "low": 12, - "open": 1999, - "volume": 56584 - }, - "open": "2020-06-21T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12582, - "high": 332, - "low": 12, - "open": 12466, - "volume": 4336888 - }, - "id": 2642653, - "non_hive": { - "close": 3145, - "high": 83, - "low": 2, - "open": 3111, - "volume": 1083444 - }, - "open": "2020-06-21T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14014, - "high": 2788, - "low": 1486, - "open": 12638, - "volume": 210193 - }, - "id": 2642710, - "non_hive": { - "close": 3503, - "high": 697, - "low": 371, - "open": 3158, - "volume": 52534 - }, - "open": "2020-06-21T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8361, - "high": 392, - "low": 350, - "open": 12685, - "volume": 284703 - }, - "id": 2642757, - "non_hive": { - "close": 2090, - "high": 98, - "low": 87, - "open": 3170, - "volume": 71161 - }, - "open": "2020-06-21T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5106, - "high": 480, - "low": 25, - "open": 12680, - "volume": 210970 - }, - "id": 2642803, - "non_hive": { - "close": 1276, - "high": 120, - "low": 6, - "open": 3168, - "volume": 52727 - }, - "open": "2020-06-21T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11070, - "high": 457495, - "low": 13, - "open": 13, - "volume": 682070 - }, - "id": 2642855, - "non_hive": { - "close": 2770, - "high": 114519, - "low": 3, - "open": 3, - "volume": 170723 - }, - "open": "2020-06-21T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3115, - "high": 10962, - "low": 3115, - "open": 1759, - "volume": 217350 - }, - "id": 2642878, - "non_hive": { - "close": 777, - "high": 2744, - "low": 777, - "open": 440, - "volume": 54401 - }, - "open": "2020-06-22T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 2532, - "low": 11807, - "open": 2532, - "volume": 37298 - }, - "id": 2642912, - "non_hive": { - "close": 3, - "high": 633, - "low": 2950, - "open": 633, - "volume": 9321 - }, - "open": "2020-06-22T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9962, - "high": 591, - "low": 12401, - "open": 2454, - "volume": 83667 - }, - "id": 2642936, - "non_hive": { - "close": 2492, - "high": 148, - "low": 3099, - "open": 614, - "volume": 20929 - }, - "open": "2020-06-22T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1915, - "high": 83, - "low": 1915, - "open": 8581, - "volume": 68275 - }, - "id": 2642961, - "non_hive": { - "close": 476, - "high": 21, - "low": 476, - "open": 2147, - "volume": 17068 - }, - "open": "2020-06-22T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12267, - "high": 771, - "low": 4922, - "open": 6573, - "volume": 72521 - }, - "id": 2642994, - "non_hive": { - "close": 3068, - "high": 193, - "low": 1230, - "open": 1644, - "volume": 18140 - }, - "open": "2020-06-22T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3994, - "high": 87, - "low": 13, - "open": 5351, - "volume": 1913944 - }, - "id": 2643024, - "non_hive": { - "close": 999, - "high": 22, - "low": 3, - "open": 1339, - "volume": 475113 - }, - "open": "2020-06-22T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6549, - "high": 6549, - "low": 424108, - "open": 11124, - "volume": 747443 - }, - "id": 2643060, - "non_hive": { - "close": 1638, - "high": 1638, - "low": 105178, - "open": 2781, - "volume": 185675 - }, - "open": "2020-06-22T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4337, - "high": 2583, - "low": 6833, - "open": 2583, - "volume": 644039 - }, - "id": 2643084, - "non_hive": { - "close": 1084, - "high": 646, - "low": 1692, - "open": 646, - "volume": 159895 - }, - "open": "2020-06-22T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10473, - "high": 671, - "low": 49909, - "open": 7956, - "volume": 754883 - }, - "id": 2643103, - "non_hive": { - "close": 2608, - "high": 168, - "low": 12377, - "open": 1990, - "volume": 187636 - }, - "open": "2020-06-22T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12900, - "high": 38620, - "low": 15224, - "open": 38620, - "volume": 143468 - }, - "id": 2643134, - "non_hive": { - "close": 3225, - "high": 9655, - "low": 3805, - "open": 9655, - "volume": 35866 - }, - "open": "2020-06-22T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1320, - "high": 5961, - "low": 13, - "open": 1989, - "volume": 1503463 - }, - "id": 2643162, - "non_hive": { - "close": 330, - "high": 1491, - "low": 3, - "open": 497, - "volume": 373057 - }, - "open": "2020-06-22T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7352, - "high": 1372, - "low": 6029, - "open": 397, - "volume": 1254042 - }, - "id": 2643208, - "non_hive": { - "close": 1833, - "high": 343, - "low": 1488, - "open": 99, - "volume": 312518 - }, - "open": "2020-06-22T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 70002, - "high": 128, - "low": 3418, - "open": 95258, - "volume": 644772 - }, - "id": 2643268, - "non_hive": { - "close": 17445, - "high": 32, - "low": 851, - "open": 23748, - "volume": 160708 - }, - "open": "2020-06-22T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5686, - "high": 20, - "low": 8086, - "open": 8086, - "volume": 53059 - }, - "id": 2643327, - "non_hive": { - "close": 1413, - "high": 5, - "low": 2000, - "open": 2000, - "volume": 13164 - }, - "open": "2020-06-22T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10818, - "high": 28, - "low": 101, - "open": 28, - "volume": 1364190 - }, - "id": 2643354, - "non_hive": { - "close": 2693, - "high": 7, - "low": 25, - "open": 7, - "volume": 338631 - }, - "open": "2020-06-22T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5100, - "high": 489, - "low": 13, - "open": 2543, - "volume": 1402337 - }, - "id": 2643390, - "non_hive": { - "close": 1270, - "high": 122, - "low": 3, - "open": 633, - "volume": 347751 - }, - "open": "2020-06-22T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5000, - "high": 52, - "low": 13, - "open": 52, - "volume": 981243 - }, - "id": 2643443, - "non_hive": { - "close": 1245, - "high": 13, - "low": 3, - "open": 13, - "volume": 243753 - }, - "open": "2020-06-22T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8063, - "high": 40, - "low": 317016, - "open": 4365, - "volume": 1123081 - }, - "id": 2643493, - "non_hive": { - "close": 2000, - "high": 10, - "low": 78617, - "open": 1087, - "volume": 279020 - }, - "open": "2020-06-22T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 324563, - "high": 132, - "low": 255411, - "open": 928, - "volume": 1956608 - }, - "id": 2643540, - "non_hive": { - "close": 80189, - "high": 33, - "low": 63029, - "open": 231, - "volume": 484827 - }, - "open": "2020-06-22T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1031, - "high": 24, - "low": 306731, - "open": 24, - "volume": 731425 - }, - "id": 2643607, - "non_hive": { - "close": 256, - "high": 6, - "low": 75455, - "open": 6, - "volume": 180321 - }, - "open": "2020-06-22T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1059, - "high": 4, - "low": 157964, - "open": 4029, - "volume": 1075028 - }, - "id": 2643636, - "non_hive": { - "close": 262, - "high": 1, - "low": 38922, - "open": 999, - "volume": 265793 - }, - "open": "2020-06-22T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 333, - "high": 473, - "low": 3160, - "open": 30399, - "volume": 1554320 - }, - "id": 2643677, - "non_hive": { - "close": 83, - "high": 118, - "low": 781, - "open": 7539, - "volume": 386011 - }, - "open": "2020-06-22T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1072, - "high": 955, - "low": 13, - "open": 1258, - "volume": 46081 - }, - "id": 2643724, - "non_hive": { - "close": 267, - "high": 238, - "low": 3, - "open": 313, - "volume": 11471 - }, - "open": "2020-06-22T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11173, - "high": 14477, - "low": 13, - "open": 65002, - "volume": 1364972 - }, - "id": 2643761, - "non_hive": { - "close": 2810, - "high": 3641, - "low": 3, - "open": 16185, - "volume": 340557 - }, - "open": "2020-06-22T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5792, - "high": 1065, - "low": 53, - "open": 2966, - "volume": 1965040 - }, - "id": 2643799, - "non_hive": { - "close": 1456, - "high": 268, - "low": 13, - "open": 746, - "volume": 488210 - }, - "open": "2020-06-23T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1521, - "high": 234, - "low": 30, - "open": 2161, - "volume": 4203725 - }, - "id": 2643837, - "non_hive": { - "close": 382, - "high": 59, - "low": 7, - "open": 543, - "volume": 1044921 - }, - "open": "2020-06-23T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3491, - "high": 17199, - "low": 121020, - "open": 7734, - "volume": 1236384 - }, - "id": 2643893, - "non_hive": { - "close": 877, - "high": 4321, - "low": 30012, - "open": 1942, - "volume": 306789 - }, - "open": "2020-06-23T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 386, - "high": 386, - "low": 32261, - "open": 8084, - "volume": 5538852 - }, - "id": 2643907, - "non_hive": { - "close": 97, - "high": 97, - "low": 7968, - "open": 2000, - "volume": 1376970 - }, - "open": "2020-06-23T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56180, - "high": 1044, - "low": 13, - "open": 36, - "volume": 2457163 - }, - "id": 2643949, - "non_hive": { - "close": 13822, - "high": 262, - "low": 3, - "open": 9, - "volume": 607368 - }, - "open": "2020-06-23T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 55, - "low": 274, - "open": 803, - "volume": 1993225 - }, - "id": 2643976, - "non_hive": { - "close": 11, - "high": 14, - "low": 67, - "open": 200, - "volume": 492905 - }, - "open": "2020-06-23T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 66000, - "high": 4, - "low": 16947, - "open": 8614, - "volume": 1433998 - }, - "id": 2644017, - "non_hive": { - "close": 16430, - "high": 1, - "low": 4189, - "open": 2152, - "volume": 355174 - }, - "open": "2020-06-23T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1666, - "high": 99736, - "low": 77343, - "open": 390329, - "volume": 2063144 - }, - "id": 2644068, - "non_hive": { - "close": 416, - "high": 25023, - "low": 19193, - "open": 97192, - "volume": 513549 - }, - "open": "2020-06-23T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20744, - "high": 24576, - "low": 2062, - "open": 2543, - "volume": 1335345 - }, - "id": 2644095, - "non_hive": { - "close": 5186, - "high": 6144, - "low": 511, - "open": 635, - "volume": 331678 - }, - "open": "2020-06-23T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2140, - "high": 7580, - "low": 339069, - "open": 7580, - "volume": 692465 - }, - "id": 2644136, - "non_hive": { - "close": 535, - "high": 1895, - "low": 84276, - "open": 1895, - "volume": 172256 - }, - "open": "2020-06-23T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60001, - "high": 684, - "low": 13, - "open": 684, - "volume": 794317 - }, - "id": 2644167, - "non_hive": { - "close": 15000, - "high": 171, - "low": 3, - "open": 171, - "volume": 197788 - }, - "open": "2020-06-23T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11048, - "high": 2640, - "low": 58, - "open": 136230, - "volume": 1994810 - }, - "id": 2644192, - "non_hive": { - "close": 2762, - "high": 660, - "low": 14, - "open": 34056, - "volume": 494682 - }, - "open": "2020-06-23T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1416, - "high": 14288, - "low": 940, - "open": 4828, - "volume": 2931562 - }, - "id": 2644233, - "non_hive": { - "close": 354, - "high": 3572, - "low": 232, - "open": 1206, - "volume": 727979 - }, - "open": "2020-06-23T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20000, - "high": 3300, - "low": 10, - "open": 50292, - "volume": 280335 - }, - "id": 2644272, - "non_hive": { - "close": 4999, - "high": 825, - "low": 2, - "open": 12572, - "volume": 70020 - }, - "open": "2020-06-23T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 172983, - "high": 1744, - "low": 44, - "open": 11290, - "volume": 1712512 - }, - "id": 2644300, - "non_hive": { - "close": 43241, - "high": 436, - "low": 10, - "open": 2822, - "volume": 424896 - }, - "open": "2020-06-23T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12212, - "high": 4016, - "low": 13, - "open": 4016, - "volume": 213170 - }, - "id": 2644328, - "non_hive": { - "close": 3053, - "high": 1004, - "low": 3, - "open": 1004, - "volume": 53257 - }, - "open": "2020-06-23T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24056, - "high": 164, - "low": 9, - "open": 164, - "volume": 1261786 - }, - "id": 2644382, - "non_hive": { - "close": 6014, - "high": 41, - "low": 2, - "open": 41, - "volume": 314226 - }, - "open": "2020-06-23T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 364, - "high": 7412, - "low": 377515, - "open": 4437, - "volume": 656153 - }, - "id": 2644430, - "non_hive": { - "close": 91, - "high": 1853, - "low": 93695, - "open": 1109, - "volume": 162963 - }, - "open": "2020-06-23T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8006, - "high": 69480, - "low": 16, - "open": 17773, - "volume": 1677604 - }, - "id": 2644455, - "non_hive": { - "close": 1999, - "high": 17370, - "low": 3, - "open": 4443, - "volume": 416596 - }, - "open": "2020-06-23T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15611, - "high": 44, - "low": 9, - "open": 8006, - "volume": 1511118 - }, - "id": 2644513, - "non_hive": { - "close": 3887, - "high": 11, - "low": 2, - "open": 1999, - "volume": 374097 - }, - "open": "2020-06-23T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1563, - "high": 477, - "low": 371994, - "open": 477, - "volume": 637102 - }, - "id": 2644573, - "non_hive": { - "close": 389, - "high": 119, - "low": 91892, - "open": 119, - "volume": 157470 - }, - "open": "2020-06-23T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100901, - "high": 7617, - "low": 11195, - "open": 1048, - "volume": 866820 - }, - "id": 2644600, - "non_hive": { - "close": 25124, - "high": 1903, - "low": 2786, - "open": 261, - "volume": 215840 - }, - "open": "2020-06-23T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57778, - "high": 235, - "low": 13, - "open": 9000, - "volume": 1689691 - }, - "id": 2644625, - "non_hive": { - "close": 14438, - "high": 59, - "low": 3, - "open": 2247, - "volume": 421923 - }, - "open": "2020-06-23T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 591970, - "high": 2217, - "low": 5, - "open": 8036, - "volume": 7153024 - }, - "id": 2644671, - "non_hive": { - "close": 147420, - "high": 560, - "low": 1, - "open": 2008, - "volume": 1793170 - }, - "open": "2020-06-23T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51, - "high": 30158, - "low": 53, - "open": 26745, - "volume": 4126288 - }, - "id": 2644726, - "non_hive": { - "close": 13, - "high": 7690, - "low": 13, - "open": 6746, - "volume": 1030360 - }, - "open": "2020-06-24T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7969, - "high": 1047, - "low": 50904, - "open": 701, - "volume": 2459539 - }, - "id": 2644776, - "non_hive": { - "close": 1999, - "high": 263, - "low": 12573, - "open": 175, - "volume": 610133 - }, - "open": "2020-06-24T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31086, - "high": 70, - "low": 4, - "open": 7845, - "volume": 352483 - }, - "id": 2644808, - "non_hive": { - "close": 7895, - "high": 18, - "low": 1, - "open": 1999, - "volume": 89581 - }, - "open": "2020-06-24T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 771, - "high": 2581, - "low": 3727, - "open": 2581, - "volume": 47775 - }, - "id": 2644839, - "non_hive": { - "close": 193, - "high": 656, - "low": 932, - "open": 656, - "volume": 12120 - }, - "open": "2020-06-24T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2001, - "high": 34995, - "low": 139, - "open": 775, - "volume": 1852627 - }, - "id": 2644856, - "non_hive": { - "close": 500, - "high": 8749, - "low": 34, - "open": 193, - "volume": 459790 - }, - "open": "2020-06-24T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45866, - "high": 177, - "low": 5999, - "open": 5999, - "volume": 2788135 - }, - "id": 2644881, - "non_hive": { - "close": 11552, - "high": 45, - "low": 1499, - "open": 1499, - "volume": 697558 - }, - "open": "2020-06-24T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1454, - "high": 67, - "low": 565290, - "open": 330, - "volume": 709307 - }, - "id": 2644903, - "non_hive": { - "close": 368, - "high": 17, - "low": 141932, - "open": 83, - "volume": 178305 - }, - "open": "2020-06-24T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7922, - "high": 45908, - "low": 2901, - "open": 7964, - "volume": 4141325 - }, - "id": 2644938, - "non_hive": { - "close": 1999, - "high": 11609, - "low": 725, - "open": 2000, - "volume": 1037678 - }, - "open": "2020-06-24T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 580877, - "high": 9748, - "low": 576, - "open": 9748, - "volume": 3719869 - }, - "id": 2644981, - "non_hive": { - "close": 144104, - "high": 2460, - "low": 142, - "open": 2460, - "volume": 923945 - }, - "open": "2020-06-24T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1824, - "high": 572, - "low": 401491, - "open": 329170, - "volume": 3877795 - }, - "id": 2645023, - "non_hive": { - "close": 452, - "high": 143, - "low": 97963, - "open": 81662, - "volume": 958866 - }, - "open": "2020-06-24T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1432, - "high": 88751, - "low": 328817, - "open": 77821, - "volume": 1452025 - }, - "id": 2645071, - "non_hive": { - "close": 355, - "high": 22092, - "low": 79901, - "open": 19291, - "volume": 355045 - }, - "open": "2020-06-24T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6022, - "high": 4, - "low": 2053, - "open": 4, - "volume": 2458654 - }, - "id": 2645103, - "non_hive": { - "close": 1494, - "high": 1, - "low": 492, - "open": 1, - "volume": 592026 - }, - "open": "2020-06-24T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 236, - "high": 40, - "low": 236, - "open": 4502, - "volume": 3141537 - }, - "id": 2645146, - "non_hive": { - "close": 56, - "high": 10, - "low": 56, - "open": 1115, - "volume": 755852 - }, - "open": "2020-06-24T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1796, - "high": 282, - "low": 21, - "open": 1217, - "volume": 1338671 - }, - "id": 2645192, - "non_hive": { - "close": 445, - "high": 70, - "low": 5, - "open": 300, - "volume": 324588 - }, - "open": "2020-06-24T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5063, - "high": 28, - "low": 85, - "open": 104, - "volume": 3477193 - }, - "id": 2645233, - "non_hive": { - "close": 1240, - "high": 7, - "low": 20, - "open": 25, - "volume": 843173 - }, - "open": "2020-06-24T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 97, - "low": 7, - "open": 788, - "volume": 3662899 - }, - "id": 2645315, - "non_hive": { - "close": 1, - "high": 24, - "low": 1, - "open": 193, - "volume": 887970 - }, - "open": "2020-06-24T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2355, - "high": 4, - "low": 8, - "open": 1223, - "volume": 1061041 - }, - "id": 2645373, - "non_hive": { - "close": 581, - "high": 1, - "low": 1, - "open": 300, - "volume": 257530 - }, - "open": "2020-06-24T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8179, - "high": 113, - "low": 11, - "open": 1217, - "volume": 1444028 - }, - "id": 2645441, - "non_hive": { - "close": 1999, - "high": 28, - "low": 2, - "open": 300, - "volume": 350683 - }, - "open": "2020-06-24T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1526, - "high": 1023, - "low": 1930, - "open": 8886, - "volume": 181275 - }, - "id": 2645480, - "non_hive": { - "close": 377, - "high": 253, - "low": 469, - "open": 2173, - "volume": 44666 - }, - "open": "2020-06-24T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 258218, - "high": 28, - "low": 24, - "open": 2386, - "volume": 496768 - }, - "id": 2645513, - "non_hive": { - "close": 63843, - "high": 7, - "low": 5, - "open": 590, - "volume": 122774 - }, - "open": "2020-06-24T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1156, - "high": 355, - "low": 9008, - "open": 38710, - "volume": 551479 - }, - "id": 2645559, - "non_hive": { - "close": 286, - "high": 88, - "low": 2217, - "open": 9571, - "volume": 136284 - }, - "open": "2020-06-24T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1479, - "high": 1023, - "low": 28, - "open": 42134, - "volume": 1328635 - }, - "id": 2645605, - "non_hive": { - "close": 364, - "high": 253, - "low": 6, - "open": 10419, - "volume": 324761 - }, - "open": "2020-06-24T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 105000, - "high": 1000, - "low": 29, - "open": 1000, - "volume": 124966 - }, - "id": 2645643, - "non_hive": { - "close": 25658, - "high": 246, - "low": 7, - "open": 246, - "volume": 30530 - }, - "open": "2020-06-24T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 576288, - "high": 405888, - "low": 13, - "open": 10445, - "volume": 4062299 - }, - "id": 2645668, - "non_hive": { - "close": 138310, - "high": 100343, - "low": 3, - "open": 2553, - "volume": 979637 - }, - "open": "2020-06-24T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 579425, - "high": 2001, - "low": 28, - "open": 8331, - "volume": 3752765 - }, - "id": 2645698, - "non_hive": { - "close": 139062, - "high": 494, - "low": 6, - "open": 2000, - "volume": 901716 - }, - "open": "2020-06-25T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 423410, - "high": 13331, - "low": 305, - "open": 8332, - "volume": 3703387 - }, - "id": 2645736, - "non_hive": { - "close": 101618, - "high": 3288, - "low": 73, - "open": 2000, - "volume": 889430 - }, - "open": "2020-06-25T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 591668, - "high": 98001, - "low": 136, - "open": 226, - "volume": 3822736 - }, - "id": 2645767, - "non_hive": { - "close": 142000, - "high": 24115, - "low": 32, - "open": 55, - "volume": 918871 - }, - "open": "2020-06-25T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4678, - "high": 65, - "low": 56300, - "open": 8065, - "volume": 3792115 - }, - "id": 2645811, - "non_hive": { - "close": 1119, - "high": 16, - "low": 13240, - "open": 1936, - "volume": 904555 - }, - "open": "2020-06-25T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 886, - "high": 12, - "low": 14327, - "open": 600000, - "volume": 7227295 - }, - "id": 2645848, - "non_hive": { - "close": 218, - "high": 3, - "low": 3369, - "open": 143618, - "volume": 1717838 - }, - "open": "2020-06-25T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5768, - "high": 8003, - "low": 7465, - "open": 8133, - "volume": 2521318 - }, - "id": 2645891, - "non_hive": { - "close": 1401, - "high": 1968, - "low": 1754, - "open": 1999, - "volume": 594195 - }, - "open": "2020-06-25T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7682, - "high": 7682, - "low": 80, - "open": 2463, - "volume": 1381349 - }, - "id": 2645930, - "non_hive": { - "close": 1890, - "high": 1890, - "low": 18, - "open": 598, - "volume": 324085 - }, - "open": "2020-06-25T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17568, - "high": 778, - "low": 597429, - "open": 8545, - "volume": 3787353 - }, - "id": 2645991, - "non_hive": { - "close": 4278, - "high": 191, - "low": 139213, - "open": 2000, - "volume": 887144 - }, - "open": "2020-06-25T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 582570, - "high": 151, - "low": 20, - "open": 1232, - "volume": 853671 - }, - "id": 2646034, - "non_hive": { - "close": 136911, - "high": 37, - "low": 4, - "open": 300, - "volume": 202390 - }, - "open": "2020-06-25T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 333, - "high": 29, - "low": 203, - "open": 1238, - "volume": 1563667 - }, - "id": 2646077, - "non_hive": { - "close": 80, - "high": 7, - "low": 47, - "open": 298, - "volume": 369510 - }, - "open": "2020-06-25T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4310, - "high": 166, - "low": 88, - "open": 4000, - "volume": 48531 - }, - "id": 2646116, - "non_hive": { - "close": 1034, - "high": 40, - "low": 20, - "open": 960, - "volume": 11646 - }, - "open": "2020-06-25T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6529, - "high": 8321, - "low": 6, - "open": 4023, - "volume": 241985 - }, - "id": 2646142, - "non_hive": { - "close": 1568, - "high": 2000, - "low": 1, - "open": 965, - "volume": 58069 - }, - "open": "2020-06-25T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2925, - "high": 4718, - "low": 31, - "open": 4718, - "volume": 3809307 - }, - "id": 2646180, - "non_hive": { - "close": 704, - "high": 1139, - "low": 7, - "open": 1139, - "volume": 900284 - }, - "open": "2020-06-25T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6400, - "high": 499, - "low": 59825, - "open": 8472, - "volume": 3649445 - }, - "id": 2646225, - "non_hive": { - "close": 1538, - "high": 120, - "low": 14088, - "open": 2000, - "volume": 861222 - }, - "open": "2020-06-25T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50, - "high": 4, - "low": 35, - "open": 1919, - "volume": 2170764 - }, - "id": 2646272, - "non_hive": { - "close": 12, - "high": 1, - "low": 8, - "open": 461, - "volume": 510394 - }, - "open": "2020-06-25T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5793, - "high": 4, - "low": 5, - "open": 5399, - "volume": 2395090 - }, - "id": 2646328, - "non_hive": { - "close": 1399, - "high": 1, - "low": 1, - "open": 1294, - "volume": 565346 - }, - "open": "2020-06-25T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8284, - "high": 4, - "low": 18, - "open": 11234, - "volume": 1063357 - }, - "id": 2646412, - "non_hive": { - "close": 1988, - "high": 1, - "low": 4, - "open": 2713, - "volume": 251339 - }, - "open": "2020-06-25T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 196, - "high": 7619, - "low": 5, - "open": 49, - "volume": 3543905 - }, - "id": 2646458, - "non_hive": { - "close": 48, - "high": 1873, - "low": 1, - "open": 12, - "volume": 851194 - }, - "open": "2020-06-25T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8183, - "high": 20, - "low": 32, - "open": 8175, - "volume": 2873320 - }, - "id": 2646518, - "non_hive": { - "close": 1999, - "high": 5, - "low": 7, - "open": 1999, - "volume": 689772 - }, - "open": "2020-06-25T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 209107, - "high": 641, - "low": 67, - "open": 8176, - "volume": 1145134 - }, - "id": 2646590, - "non_hive": { - "close": 49777, - "high": 157, - "low": 15, - "open": 1999, - "volume": 273942 - }, - "open": "2020-06-25T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 812, - "high": 4, - "low": 5, - "open": 8200, - "volume": 584044 - }, - "id": 2646627, - "non_hive": { - "close": 195, - "high": 1, - "low": 1, - "open": 1999, - "volume": 140815 - }, - "open": "2020-06-25T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 226877, - "high": 360, - "low": 226877, - "open": 1267, - "volume": 2053680 - }, - "id": 2646693, - "non_hive": { - "close": 53321, - "high": 88, - "low": 53321, - "open": 304, - "volume": 485590 - }, - "open": "2020-06-25T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4532, - "high": 8, - "low": 10, - "open": 1871, - "volume": 849834 - }, - "id": 2646730, - "non_hive": { - "close": 1103, - "high": 2, - "low": 2, - "open": 447, - "volume": 201355 - }, - "open": "2020-06-25T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2191, - "high": 424, - "low": 13, - "open": 424, - "volume": 1984228 - }, - "id": 2646771, - "non_hive": { - "close": 536, - "high": 104, - "low": 3, - "open": 104, - "volume": 470331 - }, - "open": "2020-06-25T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10550, - "high": 10550, - "low": 67, - "open": 2244, - "volume": 43356 - }, - "id": 2646827, - "non_hive": { - "close": 2586, - "high": 2586, - "low": 16, - "open": 549, - "volume": 10604 - }, - "open": "2020-06-26T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 397, - "high": 40, - "low": 86, - "open": 86, - "volume": 17807 - }, - "id": 2646851, - "non_hive": { - "close": 97, - "high": 10, - "low": 21, - "open": 21, - "volume": 4363 - }, - "open": "2020-06-26T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2572, - "high": 69, - "low": 8402, - "open": 114, - "volume": 124587 - }, - "id": 2646866, - "non_hive": { - "close": 630, - "high": 17, - "low": 2000, - "open": 28, - "volume": 30064 - }, - "open": "2020-06-26T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 108704, - "high": 1185, - "low": 108704, - "open": 4794, - "volume": 653528 - }, - "id": 2646898, - "non_hive": { - "close": 25545, - "high": 290, - "low": 25545, - "open": 1173, - "volume": 154096 - }, - "open": "2020-06-26T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1605, - "high": 4542, - "low": 95, - "open": 4542, - "volume": 2429291 - }, - "id": 2646919, - "non_hive": { - "close": 384, - "high": 1111, - "low": 22, - "open": 1111, - "volume": 571068 - }, - "open": "2020-06-26T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 401, - "high": 7050, - "low": 401, - "open": 5613, - "volume": 833342 - }, - "id": 2646951, - "non_hive": { - "close": 94, - "high": 1688, - "low": 94, - "open": 1339, - "volume": 196669 - }, - "open": "2020-06-26T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9688, - "high": 6849, - "low": 46, - "open": 12829, - "volume": 735473 - }, - "id": 2646986, - "non_hive": { - "close": 2306, - "high": 1638, - "low": 10, - "open": 3038, - "volume": 173212 - }, - "open": "2020-06-26T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 89381, - "high": 58, - "low": 89381, - "open": 58, - "volume": 3841610 - }, - "id": 2647027, - "non_hive": { - "close": 21004, - "high": 14, - "low": 21004, - "open": 14, - "volume": 902997 - }, - "open": "2020-06-26T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 398300, - "high": 573, - "low": 73, - "open": 21391, - "volume": 7621062 - }, - "id": 2647057, - "non_hive": { - "close": 91688, - "high": 135, - "low": 16, - "open": 5027, - "volume": 1768299 - }, - "open": "2020-06-26T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 121257, - "high": 2984, - "low": 121257, - "open": 2984, - "volume": 3852246 - }, - "id": 2647107, - "non_hive": { - "close": 27889, - "high": 699, - "low": 27889, - "open": 699, - "volume": 887035 - }, - "open": "2020-06-26T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6000, - "high": 4681, - "low": 33953, - "open": 8690, - "volume": 3590744 - }, - "id": 2647148, - "non_hive": { - "close": 1385, - "high": 1090, - "low": 7809, - "open": 1999, - "volume": 826201 - }, - "open": "2020-06-26T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5218, - "high": 268, - "low": 472803, - "open": 268, - "volume": 3695014 - }, - "id": 2647187, - "non_hive": { - "close": 1188, - "high": 62, - "low": 106480, - "open": 62, - "volume": 841186 - }, - "open": "2020-06-26T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5069, - "high": 48, - "low": 1040, - "open": 8879, - "volume": 5214103 - }, - "id": 2647231, - "non_hive": { - "close": 1144, - "high": 11, - "low": 231, - "open": 2000, - "volume": 1171380 - }, - "open": "2020-06-26T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35126, - "high": 31, - "low": 48, - "open": 8862, - "volume": 3211897 - }, - "id": 2647278, - "non_hive": { - "close": 7911, - "high": 7, - "low": 10, - "open": 1999, - "volume": 718826 - }, - "open": "2020-06-26T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6066, - "high": 4, - "low": 196, - "open": 221490, - "volume": 4614043 - }, - "id": 2647324, - "non_hive": { - "close": 1374, - "high": 1, - "low": 43, - "open": 49888, - "volume": 1036475 - }, - "open": "2020-06-26T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 522003, - "high": 101, - "low": 14, - "open": 27, - "volume": 3258624 - }, - "id": 2647396, - "non_hive": { - "close": 116415, - "high": 23, - "low": 3, - "open": 6, - "volume": 728795 - }, - "open": "2020-06-26T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 44, - "low": 510119, - "open": 887, - "volume": 3803880 - }, - "id": 2647448, - "non_hive": { - "close": 10, - "high": 10, - "low": 112317, - "open": 200, - "volume": 847141 - }, - "open": "2020-06-26T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6561, - "high": 71, - "low": 433227, - "open": 9077, - "volume": 3813724 - }, - "id": 2647509, - "non_hive": { - "close": 1463, - "high": 16, - "low": 95386, - "open": 2000, - "volume": 844861 - }, - "open": "2020-06-26T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 574214, - "high": 251, - "low": 574214, - "open": 9044, - "volume": 4036976 - }, - "id": 2647591, - "non_hive": { - "close": 126441, - "high": 56, - "low": 126441, - "open": 2000, - "volume": 890171 - }, - "open": "2020-06-26T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33133, - "high": 241381, - "low": 5, - "open": 2089, - "volume": 4938690 - }, - "id": 2647628, - "non_hive": { - "close": 7299, - "high": 53684, - "low": 1, - "open": 463, - "volume": 1090274 - }, - "open": "2020-06-26T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 1564, - "low": 7, - "open": 600000, - "volume": 2588109 - }, - "id": 2647676, - "non_hive": { - "close": 1, - "high": 348, - "low": 1, - "open": 132157, - "volume": 569860 - }, - "open": "2020-06-26T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 180, - "high": 3186, - "low": 520, - "open": 9009, - "volume": 1568541 - }, - "id": 2647698, - "non_hive": { - "close": 40, - "high": 708, - "low": 114, - "open": 1999, - "volume": 345272 - }, - "open": "2020-06-26T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 167690, - "high": 787, - "low": 28, - "open": 1833, - "volume": 453520 - }, - "id": 2647744, - "non_hive": { - "close": 37192, - "high": 175, - "low": 6, - "open": 407, - "volume": 100630 - }, - "open": "2020-06-26T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2000, - "high": 63, - "low": 14, - "open": 9018, - "volume": 1485308 - }, - "id": 2647796, - "non_hive": { - "close": 440, - "high": 14, - "low": 3, - "open": 1997, - "volume": 328950 - }, - "open": "2020-06-26T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8400, - "high": 4, - "low": 87, - "open": 5517, - "volume": 2599721 - }, - "id": 2647848, - "non_hive": { - "close": 1855, - "high": 1, - "low": 18, - "open": 1215, - "volume": 566379 - }, - "open": "2020-06-27T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 581399, - "high": 22, - "low": 581399, - "open": 22, - "volume": 3051302 - }, - "id": 2647904, - "non_hive": { - "close": 125010, - "high": 5, - "low": 125010, - "open": 5, - "volume": 657770 - }, - "open": "2020-06-27T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 587076, - "high": 3624, - "low": 5, - "open": 9084, - "volume": 2410153 - }, - "id": 2647939, - "non_hive": { - "close": 126230, - "high": 800, - "low": 1, - "open": 2000, - "volume": 518954 - }, - "open": "2020-06-27T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 113, - "high": 67, - "low": 353375, - "open": 9300, - "volume": 1872441 - }, - "id": 2647958, - "non_hive": { - "close": 25, - "high": 15, - "low": 75981, - "open": 2000, - "volume": 403030 - }, - "open": "2020-06-27T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1768, - "high": 228, - "low": 56, - "open": 984, - "volume": 647198 - }, - "id": 2647988, - "non_hive": { - "close": 394, - "high": 51, - "low": 12, - "open": 217, - "volume": 141259 - }, - "open": "2020-06-27T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 170, - "high": 170, - "low": 5, - "open": 1859, - "volume": 58152 - }, - "id": 2648021, - "non_hive": { - "close": 39, - "high": 39, - "low": 1, - "open": 413, - "volume": 13022 - }, - "open": "2020-06-27T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7737, - "high": 4093, - "low": 509181, - "open": 4093, - "volume": 1269919 - }, - "id": 2648036, - "non_hive": { - "close": 1753, - "high": 936, - "low": 113591, - "open": 936, - "volume": 284212 - }, - "open": "2020-06-27T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22472, - "high": 5776, - "low": 144, - "open": 5776, - "volume": 1858770 - }, - "id": 2648055, - "non_hive": { - "close": 5000, - "high": 1308, - "low": 31, - "open": 1308, - "volume": 412031 - }, - "open": "2020-06-27T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11246, - "high": 9360, - "low": 2765, - "open": 9036, - "volume": 3221230 - }, - "id": 2648094, - "non_hive": { - "close": 2508, - "high": 2137, - "low": 611, - "open": 2000, - "volume": 713587 - }, - "open": "2020-06-27T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6313, - "high": 139, - "low": 421176, - "open": 8969, - "volume": 1828925 - }, - "id": 2648161, - "non_hive": { - "close": 1405, - "high": 31, - "low": 93194, - "open": 1999, - "volume": 404774 - }, - "open": "2020-06-27T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1375, - "high": 78, - "low": 58729, - "open": 707, - "volume": 1291521 - }, - "id": 2648190, - "non_hive": { - "close": 302, - "high": 18, - "low": 12809, - "open": 157, - "volume": 285663 - }, - "open": "2020-06-27T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 1051, - "low": 129, - "open": 9026, - "volume": 770544 - }, - "id": 2648245, - "non_hive": { - "close": 2211, - "high": 233, - "low": 28, - "open": 1999, - "volume": 169759 - }, - "open": "2020-06-27T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 88, - "high": 698, - "low": 581388, - "open": 9041, - "volume": 1745154 - }, - "id": 2648276, - "non_hive": { - "close": 20, - "high": 164, - "low": 127905, - "open": 1999, - "volume": 385065 - }, - "open": "2020-06-27T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14536, - "high": 196, - "low": 5, - "open": 8929, - "volume": 3819866 - }, - "id": 2648339, - "non_hive": { - "close": 3227, - "high": 46, - "low": 1, - "open": 1999, - "volume": 844393 - }, - "open": "2020-06-27T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 557, - "high": 73504, - "low": 23469, - "open": 2762, - "volume": 4025359 - }, - "id": 2648396, - "non_hive": { - "close": 122, - "high": 16318, - "low": 5048, - "open": 613, - "volume": 878287 - }, - "open": "2020-06-27T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8211, - "high": 22, - "low": 33, - "open": 28, - "volume": 2489668 - }, - "id": 2648449, - "non_hive": { - "close": 1794, - "high": 5, - "low": 7, - "open": 6, - "volume": 537975 - }, - "open": "2020-06-27T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 125063, - "high": 1006, - "low": 742, - "open": 12000, - "volume": 3125432 - }, - "id": 2648499, - "non_hive": { - "close": 27338, - "high": 220, - "low": 160, - "open": 2622, - "volume": 676628 - }, - "open": "2020-06-27T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2146, - "high": 5064, - "low": 9869, - "open": 1512, - "volume": 1053824 - }, - "id": 2648570, - "non_hive": { - "close": 468, - "high": 1107, - "low": 2131, - "open": 330, - "volume": 228366 - }, - "open": "2020-06-27T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 554419, - "high": 14654, - "low": 554419, - "open": 14654, - "volume": 2508612 - }, - "id": 2648604, - "non_hive": { - "close": 116570, - "high": 3196, - "low": 116570, - "open": 3196, - "volume": 531905 - }, - "open": "2020-06-27T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6065, - "high": 4977, - "low": 412, - "open": 1525, - "volume": 3685663 - }, - "id": 2648642, - "non_hive": { - "close": 1306, - "high": 1078, - "low": 86, - "open": 330, - "volume": 775524 - }, - "open": "2020-06-27T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15415, - "high": 37570, - "low": 680, - "open": 99588, - "volume": 5153695 - }, - "id": 2648701, - "non_hive": { - "close": 3254, - "high": 7961, - "low": 137, - "open": 20824, - "volume": 1057533 - }, - "open": "2020-06-27T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32472, - "high": 3748, - "low": 100, - "open": 9825, - "volume": 3133258 - }, - "id": 2648759, - "non_hive": { - "close": 6785, - "high": 785, - "low": 20, - "open": 2000, - "volume": 640725 - }, - "open": "2020-06-27T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590247, - "high": 23, - "low": 7, - "open": 1201, - "volume": 3247050 - }, - "id": 2648794, - "non_hive": { - "close": 121024, - "high": 5, - "low": 1, - "open": 251, - "volume": 667976 - }, - "open": "2020-06-27T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8806, - "high": 33, - "low": 26, - "open": 9329, - "volume": 2392783 - }, - "id": 2648848, - "non_hive": { - "close": 1854, - "high": 7, - "low": 5, - "open": 1943, - "volume": 490935 - }, - "open": "2020-06-27T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1133, - "high": 1133, - "low": 54, - "open": 9502, - "volume": 2605025 - }, - "id": 2648891, - "non_hive": { - "close": 239, - "high": 239, - "low": 11, - "open": 1999, - "volume": 535913 - }, - "open": "2020-06-28T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 125248, - "high": 199, - "low": 281, - "open": 2000, - "volume": 3234803 - }, - "id": 2648945, - "non_hive": { - "close": 26175, - "high": 42, - "low": 57, - "open": 421, - "volume": 663603 - }, - "open": "2020-06-28T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48866, - "high": 666, - "low": 48866, - "open": 666, - "volume": 712922 - }, - "id": 2648979, - "non_hive": { - "close": 9870, - "high": 139, - "low": 9870, - "open": 139, - "volume": 144625 - }, - "open": "2020-06-28T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 104, - "high": 104, - "low": 590101, - "open": 9899, - "volume": 4168646 - }, - "id": 2649002, - "non_hive": { - "close": 22, - "high": 22, - "low": 119206, - "open": 2000, - "volume": 851805 - }, - "open": "2020-06-28T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7139, - "high": 1011, - "low": 58, - "open": 58, - "volume": 240120 - }, - "id": 2649039, - "non_hive": { - "close": 1499, - "high": 213, - "low": 12, - "open": 12, - "volume": 50366 - }, - "open": "2020-06-28T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11785, - "high": 563, - "low": 111810, - "open": 214, - "volume": 233278 - }, - "id": 2649069, - "non_hive": { - "close": 2463, - "high": 119, - "low": 23231, - "open": 45, - "volume": 48735 - }, - "open": "2020-06-28T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5023, - "high": 1167, - "low": 37, - "open": 1968, - "volume": 143768 - }, - "id": 2649101, - "non_hive": { - "close": 1050, - "high": 244, - "low": 7, - "open": 411, - "volume": 30033 - }, - "open": "2020-06-28T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2153, - "high": 224, - "low": 9367, - "open": 71771, - "volume": 250926 - }, - "id": 2649128, - "non_hive": { - "close": 450, - "high": 47, - "low": 1957, - "open": 15000, - "volume": 52443 - }, - "open": "2020-06-28T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23753, - "high": 23, - "low": 437, - "open": 995, - "volume": 99587 - }, - "id": 2649157, - "non_hive": { - "close": 5000, - "high": 5, - "low": 91, - "open": 208, - "volume": 20883 - }, - "open": "2020-06-28T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16308, - "high": 322, - "low": 174726, - "open": 9468, - "volume": 2540742 - }, - "id": 2649193, - "non_hive": { - "close": 3441, - "high": 68, - "low": 36504, - "open": 1993, - "volume": 535281 - }, - "open": "2020-06-28T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9796, - "high": 819, - "low": 2701, - "open": 1255, - "volume": 1672615 - }, - "id": 2649230, - "non_hive": { - "close": 2067, - "high": 173, - "low": 569, - "open": 265, - "volume": 352918 - }, - "open": "2020-06-28T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12883, - "high": 77, - "low": 9381, - "open": 9381, - "volume": 4066924 - }, - "id": 2649275, - "non_hive": { - "close": 2795, - "high": 17, - "low": 1979, - "open": 1979, - "volume": 858680 - }, - "open": "2020-06-28T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12996, - "high": 114, - "low": 12996, - "open": 17187, - "volume": 430469 - }, - "id": 2649328, - "non_hive": { - "close": 2807, - "high": 25, - "low": 2807, - "open": 3744, - "volume": 93599 - }, - "open": "2020-06-28T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13832, - "high": 4, - "low": 190, - "open": 9258, - "volume": 488457 - }, - "id": 2649368, - "non_hive": { - "close": 3001, - "high": 1, - "low": 40, - "open": 2000, - "volume": 105846 - }, - "open": "2020-06-28T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7692, - "high": 4, - "low": 5, - "open": 102, - "volume": 4220330 - }, - "id": 2649423, - "non_hive": { - "close": 1670, - "high": 1, - "low": 1, - "open": 22, - "volume": 909641 - }, - "open": "2020-06-28T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6587, - "high": 4, - "low": 15, - "open": 9217, - "volume": 499624 - }, - "id": 2649475, - "non_hive": { - "close": 1404, - "high": 1, - "low": 3, - "open": 1999, - "volume": 107820 - }, - "open": "2020-06-28T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4276, - "high": 418, - "low": 61, - "open": 103, - "volume": 376887 - }, - "id": 2649530, - "non_hive": { - "close": 950, - "high": 98, - "low": 13, - "open": 22, - "volume": 86507 - }, - "open": "2020-06-28T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10891, - "high": 25, - "low": 700, - "open": 8621, - "volume": 363708 - }, - "id": 2649555, - "non_hive": { - "close": 2396, - "high": 6, - "low": 150, - "open": 1999, - "volume": 80091 - }, - "open": "2020-06-28T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5363, - "high": 18, - "low": 41790, - "open": 11440, - "volume": 558444 - }, - "id": 2649596, - "non_hive": { - "close": 1180, - "high": 4, - "low": 9047, - "open": 2516, - "volume": 121859 - }, - "open": "2020-06-28T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5470, - "high": 4, - "low": 230269, - "open": 4, - "volume": 709812 - }, - "id": 2649651, - "non_hive": { - "close": 1203, - "high": 1, - "low": 49897, - "open": 1, - "volume": 157809 - }, - "open": "2020-06-28T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4794, - "high": 4794, - "low": 255, - "open": 9094, - "volume": 481137 - }, - "id": 2649690, - "non_hive": { - "close": 1102, - "high": 1102, - "low": 55, - "open": 1999, - "volume": 104597 - }, - "open": "2020-06-28T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5228, - "high": 4, - "low": 5, - "open": 9217, - "volume": 2416973 - }, - "id": 2649726, - "non_hive": { - "close": 1179, - "high": 1, - "low": 1, - "open": 2000, - "volume": 525952 - }, - "open": "2020-06-28T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4459, - "high": 26, - "low": 6, - "open": 9236, - "volume": 2138392 - }, - "id": 2649781, - "non_hive": { - "close": 1000, - "high": 6, - "low": 1, - "open": 2000, - "volume": 468342 - }, - "open": "2020-06-28T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 141577, - "high": 4, - "low": 96, - "open": 1223248, - "volume": 3283318 - }, - "id": 2649837, - "non_hive": { - "close": 30647, - "high": 1, - "low": 20, - "open": 273468, - "volume": 720364 - }, - "open": "2020-06-28T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 937, - "high": 1535, - "low": 12, - "open": 1535, - "volume": 65246 - }, - "id": 2649870, - "non_hive": { - "close": 203, - "high": 341, - "low": 2, - "open": 341, - "volume": 14297 - }, - "open": "2020-06-29T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 323, - "high": 3427, - "low": 59, - "open": 907, - "volume": 278189 - }, - "id": 2649894, - "non_hive": { - "close": 70, - "high": 769, - "low": 12, - "open": 203, - "volume": 61926 - }, - "open": "2020-06-29T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 217, - "high": 377, - "low": 20, - "open": 323, - "volume": 3289054 - }, - "id": 2649934, - "non_hive": { - "close": 48, - "high": 85, - "low": 4, - "open": 71, - "volume": 732957 - }, - "open": "2020-06-29T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12893, - "high": 336, - "low": 12044, - "open": 6422, - "volume": 427736 - }, - "id": 2649980, - "non_hive": { - "close": 2986, - "high": 78, - "low": 2787, - "open": 1488, - "volume": 99065 - }, - "open": "2020-06-29T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19593, - "high": 60, - "low": 58, - "open": 474951, - "volume": 523217 - }, - "id": 2650010, - "non_hive": { - "close": 4538, - "high": 14, - "low": 13, - "open": 110000, - "volume": 121167 - }, - "open": "2020-06-29T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4088, - "high": 13528, - "low": 484690, - "open": 13528, - "volume": 545367 - }, - "id": 2650036, - "non_hive": { - "close": 936, - "high": 3133, - "low": 110000, - "open": 3133, - "volume": 123996 - }, - "open": "2020-06-29T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1192, - "high": 1192, - "low": 11481, - "open": 17956, - "volume": 2818166 - }, - "id": 2650053, - "non_hive": { - "close": 273, - "high": 273, - "low": 2624, - "open": 4110, - "volume": 644805 - }, - "open": "2020-06-29T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8734, - "high": 288, - "low": 329245, - "open": 18954, - "volume": 3956353 - }, - "id": 2650087, - "non_hive": { - "close": 2000, - "high": 66, - "low": 74568, - "open": 4333, - "volume": 902105 - }, - "open": "2020-06-29T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18069, - "high": 1043, - "low": 148170, - "open": 3870, - "volume": 368748 - }, - "id": 2650110, - "non_hive": { - "close": 4138, - "high": 239, - "low": 33867, - "open": 886, - "volume": 84372 - }, - "open": "2020-06-29T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1663, - "high": 200, - "low": 12515, - "open": 12515, - "volume": 40546 - }, - "id": 2650156, - "non_hive": { - "close": 381, - "high": 46, - "low": 2866, - "open": 2866, - "volume": 9286 - }, - "open": "2020-06-29T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1069, - "high": 69, - "low": 76, - "open": 4491, - "volume": 545950 - }, - "id": 2650177, - "non_hive": { - "close": 245, - "high": 16, - "low": 17, - "open": 1028, - "volume": 124421 - }, - "open": "2020-06-29T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 96311, - "high": 1186, - "low": 723499, - "open": 723499, - "volume": 1282149 - }, - "id": 2650221, - "non_hive": { - "close": 22151, - "high": 273, - "low": 165665, - "open": 165665, - "volume": 293848 - }, - "open": "2020-06-29T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10341, - "high": 4, - "low": 1143, - "open": 52, - "volume": 1564708 - }, - "id": 2650258, - "non_hive": { - "close": 2395, - "high": 1, - "low": 262, - "open": 12, - "volume": 361378 - }, - "open": "2020-06-29T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2179, - "high": 21635, - "low": 7623, - "open": 865426, - "volume": 1101616 - }, - "id": 2650306, - "non_hive": { - "close": 503, - "high": 5000, - "low": 1759, - "open": 200000, - "volume": 254568 - }, - "open": "2020-06-29T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2771, - "high": 1018, - "low": 3932, - "open": 4294, - "volume": 804983 - }, - "id": 2650336, - "non_hive": { - "close": 639, - "high": 236, - "low": 902, - "open": 992, - "volume": 185728 - }, - "open": "2020-06-29T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11285, - "high": 18693, - "low": 61, - "open": 4942, - "volume": 466460 - }, - "id": 2650384, - "non_hive": { - "close": 2596, - "high": 4310, - "low": 13, - "open": 1139, - "volume": 107049 - }, - "open": "2020-06-29T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 912, - "high": 10245, - "low": 5, - "open": 44719, - "volume": 8515380 - }, - "id": 2650426, - "non_hive": { - "close": 212, - "high": 2418, - "low": 1, - "open": 10289, - "volume": 1971473 - }, - "open": "2020-06-29T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6952, - "high": 179, - "low": 167, - "open": 46, - "volume": 2757185 - }, - "id": 2650492, - "non_hive": { - "close": 1573, - "high": 43, - "low": 37, - "open": 11, - "volume": 641559 - }, - "open": "2020-06-29T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 182, - "high": 23003, - "low": 12083, - "open": 8836, - "volume": 324165 - }, - "id": 2650554, - "non_hive": { - "close": 43, - "high": 5448, - "low": 2721, - "open": 2000, - "volume": 74683 - }, - "open": "2020-06-29T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8779, - "high": 4, - "low": 3227, - "open": 2291, - "volume": 360640 - }, - "id": 2650588, - "non_hive": { - "close": 1999, - "high": 1, - "low": 729, - "open": 536, - "volume": 82398 - }, - "open": "2020-06-29T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8740, - "high": 8740, - "low": 100000, - "open": 9494, - "volume": 685124 - }, - "id": 2650620, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 22700, - "open": 2162, - "volume": 155912 - }, - "open": "2020-06-29T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11429, - "high": 26, - "low": 11429, - "open": 3883, - "volume": 802735 - }, - "id": 2650671, - "non_hive": { - "close": 2584, - "high": 6, - "low": 2584, - "open": 888, - "volume": 182098 - }, - "open": "2020-06-29T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7095, - "high": 888, - "low": 27, - "open": 11429, - "volume": 780338 - }, - "id": 2650716, - "non_hive": { - "close": 1605, - "high": 201, - "low": 6, - "open": 2584, - "volume": 176474 - }, - "open": "2020-06-29T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3003, - "high": 236, - "low": 14, - "open": 7502, - "volume": 1374818 - }, - "id": 2650749, - "non_hive": { - "close": 686, - "high": 54, - "low": 3, - "open": 1697, - "volume": 313863 - }, - "open": "2020-06-29T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 280, - "high": 280, - "low": 53, - "open": 11810, - "volume": 268714 - }, - "id": 2650791, - "non_hive": { - "close": 64, - "high": 64, - "low": 12, - "open": 2695, - "volume": 61376 - }, - "open": "2020-06-30T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3475, - "high": 923, - "low": 10823, - "open": 3900, - "volume": 227374 - }, - "id": 2650826, - "non_hive": { - "close": 794, - "high": 211, - "low": 2471, - "open": 891, - "volume": 51939 - }, - "open": "2020-06-30T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 163, - "high": 99599, - "low": 40290, - "open": 2044, - "volume": 236022 - }, - "id": 2650848, - "non_hive": { - "close": 37, - "high": 23771, - "low": 9116, - "open": 467, - "volume": 55053 - }, - "open": "2020-06-30T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6971, - "high": 6965, - "low": 34, - "open": 6965, - "volume": 23535 - }, - "id": 2650867, - "non_hive": { - "close": 1618, - "high": 1621, - "low": 7, - "open": 1621, - "volume": 5469 - }, - "open": "2020-06-30T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2070, - "high": 103, - "low": 16, - "open": 103, - "volume": 77280 - }, - "id": 2650885, - "non_hive": { - "close": 474, - "high": 24, - "low": 3, - "open": 24, - "volume": 17878 - }, - "open": "2020-06-30T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43003, - "high": 1620, - "low": 288, - "open": 13043, - "volume": 641402 - }, - "id": 2650916, - "non_hive": { - "close": 10007, - "high": 377, - "low": 65, - "open": 2974, - "volume": 146922 - }, - "open": "2020-06-30T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1277418, - "high": 4, - "low": 371, - "open": 5062, - "volume": 3218310 - }, - "id": 2650954, - "non_hive": { - "close": 297383, - "high": 1, - "low": 86, - "open": 1178, - "volume": 748336 - }, - "open": "2020-06-30T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4839, - "high": 364, - "low": 20, - "open": 94067, - "volume": 723086 - }, - "id": 2650971, - "non_hive": { - "close": 1147, - "high": 87, - "low": 4, - "open": 21899, - "volume": 168757 - }, - "open": "2020-06-30T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13300, - "high": 836, - "low": 21, - "open": 25561, - "volume": 2458450 - }, - "id": 2651002, - "non_hive": { - "close": 3105, - "high": 200, - "low": 4, - "open": 6058, - "volume": 575202 - }, - "open": "2020-06-30T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 301350, - "high": 1311, - "low": 103276, - "open": 7152, - "volume": 1055674 - }, - "id": 2651051, - "non_hive": { - "close": 69996, - "high": 308, - "low": 23753, - "open": 1674, - "volume": 244091 - }, - "open": "2020-06-30T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4280, - "high": 7886, - "low": 4280, - "open": 8363, - "volume": 957509 - }, - "id": 2651086, - "non_hive": { - "close": 998, - "high": 1885, - "low": 998, - "open": 1999, - "volume": 223457 - }, - "open": "2020-06-30T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5362, - "high": 4, - "low": 905, - "open": 4294, - "volume": 375788 - }, - "id": 2651116, - "non_hive": { - "close": 1254, - "high": 1, - "low": 208, - "open": 1001, - "volume": 87621 - }, - "open": "2020-06-30T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1025, - "high": 180, - "low": 1364, - "open": 180, - "volume": 933526 - }, - "id": 2651151, - "non_hive": { - "close": 239, - "high": 42, - "low": 317, - "open": 42, - "volume": 217617 - }, - "open": "2020-06-30T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17047, - "high": 38, - "low": 17047, - "open": 4429, - "volume": 33363 - }, - "id": 2651174, - "non_hive": { - "close": 3972, - "high": 9, - "low": 3972, - "open": 1032, - "volume": 7774 - }, - "open": "2020-06-30T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2074, - "high": 64, - "low": 99, - "open": 99, - "volume": 597918 - }, - "id": 2651190, - "non_hive": { - "close": 483, - "high": 15, - "low": 23, - "open": 23, - "volume": 139304 - }, - "open": "2020-06-30T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39904, - "high": 39904, - "low": 31, - "open": 22853, - "volume": 1696563 - }, - "id": 2651234, - "non_hive": { - "close": 9300, - "high": 9300, - "low": 7, - "open": 5322, - "volume": 392767 - }, - "open": "2020-06-30T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40582, - "high": 7238, - "low": 84, - "open": 8660, - "volume": 2148372 - }, - "id": 2651286, - "non_hive": { - "close": 9300, - "high": 1686, - "low": 19, - "open": 2000, - "volume": 495701 - }, - "open": "2020-06-30T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 560, - "high": 4, - "low": 11, - "open": 103, - "volume": 673546 - }, - "id": 2651331, - "non_hive": { - "close": 129, - "high": 1, - "low": 2, - "open": 24, - "volume": 155148 - }, - "open": "2020-06-30T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15643, - "high": 4, - "low": 7729, - "open": 5793, - "volume": 2152473 - }, - "id": 2651384, - "non_hive": { - "close": 3565, - "high": 1, - "low": 1741, - "open": 1333, - "volume": 490411 - }, - "open": "2020-06-30T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 171, - "high": 4, - "low": 26, - "open": 1233, - "volume": 283996 - }, - "id": 2651448, - "non_hive": { - "close": 39, - "high": 1, - "low": 5, - "open": 281, - "volume": 64728 - }, - "open": "2020-06-30T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12643, - "high": 295, - "low": 188, - "open": 93, - "volume": 2534380 - }, - "id": 2651494, - "non_hive": { - "close": 2956, - "high": 69, - "low": 42, - "open": 21, - "volume": 581297 - }, - "open": "2020-06-30T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8580, - "high": 129, - "low": 7728, - "open": 7728, - "volume": 1296504 - }, - "id": 2651538, - "non_hive": { - "close": 2000, - "high": 31, - "low": 1750, - "open": 1750, - "volume": 300879 - }, - "open": "2020-06-30T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9080, - "high": 4, - "low": 3594, - "open": 8548, - "volume": 210919 - }, - "id": 2651574, - "non_hive": { - "close": 2143, - "high": 1, - "low": 828, - "open": 1999, - "volume": 49511 - }, - "open": "2020-06-30T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40000, - "high": 16575, - "low": 16, - "open": 15888, - "volume": 3534189 - }, - "id": 2651617, - "non_hive": { - "close": 9435, - "high": 3968, - "low": 3, - "open": 3749, - "volume": 834392 - }, - "open": "2020-06-30T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4156, - "high": 1526, - "low": 51, - "open": 8199, - "volume": 4626807 - }, - "id": 2651649, - "non_hive": { - "close": 953, - "high": 360, - "low": 11, - "open": 1934, - "volume": 1039297 - }, - "open": "2020-07-01T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8971, - "high": 48800, - "low": 47151, - "open": 9121, - "volume": 3699692 - }, - "id": 2651701, - "non_hive": { - "close": 1999, - "high": 11160, - "low": 10326, - "open": 2000, - "volume": 811176 - }, - "open": "2020-07-01T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 576164, - "high": 2354, - "low": 22, - "open": 2354, - "volume": 4111254 - }, - "id": 2651737, - "non_hive": { - "close": 125045, - "high": 525, - "low": 4, - "open": 525, - "volume": 898545 - }, - "open": "2020-07-01T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590833, - "high": 1482, - "low": 44772, - "open": 573, - "volume": 3093959 - }, - "id": 2651769, - "non_hive": { - "close": 128818, - "high": 326, - "low": 9761, - "open": 126, - "volume": 674788 - }, - "open": "2020-07-01T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7969, - "high": 2060, - "low": 557799, - "open": 22754, - "volume": 2578467 - }, - "id": 2651805, - "non_hive": { - "close": 1749, - "high": 453, - "low": 121066, - "open": 5000, - "volume": 560231 - }, - "open": "2020-07-01T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30000, - "high": 1631, - "low": 59042, - "open": 1631, - "volume": 3808757 - }, - "id": 2651833, - "non_hive": { - "close": 6522, - "high": 358, - "low": 12829, - "open": 358, - "volume": 828024 - }, - "open": "2020-07-01T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9107, - "high": 11543, - "low": 468171, - "open": 33253, - "volume": 4042050 - }, - "id": 2651881, - "non_hive": { - "close": 1999, - "high": 2534, - "low": 101640, - "open": 7226, - "volume": 878346 - }, - "open": "2020-07-01T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11547, - "high": 200, - "low": 401552, - "open": 3463, - "volume": 2466277 - }, - "id": 2651925, - "non_hive": { - "close": 2500, - "high": 44, - "low": 86775, - "open": 760, - "volume": 534443 - }, - "open": "2020-07-01T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20438, - "high": 17796, - "low": 517825, - "open": 1305, - "volume": 2403656 - }, - "id": 2651962, - "non_hive": { - "close": 4486, - "high": 3908, - "low": 112575, - "open": 286, - "volume": 523697 - }, - "open": "2020-07-01T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2295, - "high": 2295, - "low": 500216, - "open": 4955, - "volume": 1366869 - }, - "id": 2652011, - "non_hive": { - "close": 522, - "high": 522, - "low": 109047, - "open": 1083, - "volume": 298643 - }, - "open": "2020-07-01T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 562117, - "high": 3663, - "low": 544122, - "open": 1452, - "volume": 3686800 - }, - "id": 2652043, - "non_hive": { - "close": 122035, - "high": 835, - "low": 118128, - "open": 330, - "volume": 801376 - }, - "open": "2020-07-01T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30, - "high": 30, - "low": 1520, - "open": 1519, - "volume": 4586919 - }, - "id": 2652081, - "non_hive": { - "close": 7, - "high": 7, - "low": 330, - "open": 330, - "volume": 1011331 - }, - "open": "2020-07-01T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 484869, - "high": 8151, - "low": 1426, - "open": 109681, - "volume": 2859593 - }, - "id": 2652125, - "non_hive": { - "close": 107151, - "high": 1849, - "low": 311, - "open": 23970, - "volume": 630650 - }, - "open": "2020-07-01T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90, - "high": 277, - "low": 21163, - "open": 277, - "volume": 4780025 - }, - "id": 2652156, - "non_hive": { - "close": 20, - "high": 63, - "low": 4613, - "open": 63, - "volume": 1052118 - }, - "open": "2020-07-01T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5426, - "high": 5426, - "low": 125, - "open": 8060, - "volume": 2339891 - }, - "id": 2652200, - "non_hive": { - "close": 1231, - "high": 1231, - "low": 27, - "open": 1791, - "volume": 518375 - }, - "open": "2020-07-01T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11153, - "high": 260, - "low": 14, - "open": 2182, - "volume": 2058759 - }, - "id": 2652245, - "non_hive": { - "close": 2475, - "high": 59, - "low": 3, - "open": 495, - "volume": 454455 - }, - "open": "2020-07-01T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5166, - "high": 44274, - "low": 986, - "open": 1437, - "volume": 1367900 - }, - "id": 2652292, - "non_hive": { - "close": 1147, - "high": 9831, - "low": 214, - "open": 319, - "volume": 300922 - }, - "open": "2020-07-01T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127790, - "high": 4, - "low": 174, - "open": 21898, - "volume": 1210981 - }, - "id": 2652326, - "non_hive": { - "close": 27878, - "high": 1, - "low": 37, - "open": 4764, - "volume": 263371 - }, - "open": "2020-07-01T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1992, - "high": 276627, - "low": 456799, - "open": 7737, - "volume": 3418655 - }, - "id": 2652365, - "non_hive": { - "close": 444, - "high": 62745, - "low": 98703, - "open": 1710, - "volume": 748075 - }, - "open": "2020-07-01T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 290787, - "high": 164, - "low": 290762, - "open": 9235, - "volume": 3673765 - }, - "id": 2652413, - "non_hive": { - "close": 63132, - "high": 37, - "low": 62828, - "open": 1999, - "volume": 797925 - }, - "open": "2020-07-01T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 428, - "high": 31, - "low": 22, - "open": 5041, - "volume": 3999559 - }, - "id": 2652468, - "non_hive": { - "close": 95, - "high": 7, - "low": 4, - "open": 1130, - "volume": 869566 - }, - "open": "2020-07-01T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9201, - "high": 86, - "low": 5, - "open": 470, - "volume": 2788164 - }, - "id": 2652523, - "non_hive": { - "close": 1999, - "high": 19, - "low": 1, - "open": 102, - "volume": 601298 - }, - "open": "2020-07-01T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 206159, - "high": 82225, - "low": 54, - "open": 25690, - "volume": 4510341 - }, - "id": 2652556, - "non_hive": { - "close": 46613, - "high": 18602, - "low": 11, - "open": 5584, - "volume": 977721 - }, - "open": "2020-07-01T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29469, - "high": 4, - "low": 5, - "open": 4, - "volume": 3331370 - }, - "id": 2652621, - "non_hive": { - "close": 6321, - "high": 1, - "low": 1, - "open": 1, - "volume": 717276 - }, - "open": "2020-07-01T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1006, - "high": 227, - "low": 1006, - "open": 36419, - "volume": 82006 - }, - "id": 2652677, - "non_hive": { - "close": 216, - "high": 50, - "low": 216, - "open": 8000, - "volume": 17996 - }, - "open": "2020-07-02T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25193, - "high": 1563, - "low": 84, - "open": 8294, - "volume": 743818 - }, - "id": 2652710, - "non_hive": { - "close": 5542, - "high": 344, - "low": 18, - "open": 1784, - "volume": 160379 - }, - "open": "2020-07-02T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16893, - "high": 252, - "low": 5, - "open": 50754, - "volume": 2563798 - }, - "id": 2652751, - "non_hive": { - "close": 3812, - "high": 57, - "low": 1, - "open": 11459, - "volume": 555250 - }, - "open": "2020-07-02T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 957, - "high": 5803, - "low": 2948, - "open": 9320, - "volume": 86965 - }, - "id": 2652789, - "non_hive": { - "close": 215, - "high": 1304, - "low": 632, - "open": 2000, - "volume": 18823 - }, - "open": "2020-07-02T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 760, - "high": 88283, - "low": 57, - "open": 8174, - "volume": 3912529 - }, - "id": 2652817, - "non_hive": { - "close": 167, - "high": 19828, - "low": 12, - "open": 1832, - "volume": 853419 - }, - "open": "2020-07-02T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6002, - "high": 141, - "low": 9611, - "open": 141, - "volume": 61663 - }, - "id": 2652850, - "non_hive": { - "close": 1318, - "high": 31, - "low": 2107, - "open": 31, - "volume": 13533 - }, - "open": "2020-07-02T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4926, - "high": 487, - "low": 366589, - "open": 1507, - "volume": 3279888 - }, - "id": 2652874, - "non_hive": { - "close": 1078, - "high": 107, - "low": 76986, - "open": 330, - "volume": 693362 - }, - "open": "2020-07-02T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 225047, - "high": 237, - "low": 12, - "open": 1510, - "volume": 1211883 - }, - "id": 2652918, - "non_hive": { - "close": 47259, - "high": 52, - "low": 2, - "open": 330, - "volume": 255369 - }, - "open": "2020-07-02T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35, - "high": 46, - "low": 35, - "open": 9523, - "volume": 98727 - }, - "id": 2652935, - "non_hive": { - "close": 7, - "high": 10, - "low": 7, - "open": 2000, - "volume": 21187 - }, - "open": "2020-07-02T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10381, - "high": 566, - "low": 576, - "open": 464, - "volume": 206112 - }, - "id": 2652963, - "non_hive": { - "close": 2234, - "high": 122, - "low": 122, - "open": 100, - "volume": 44299 - }, - "open": "2020-07-02T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 614, - "high": 59236, - "low": 31, - "open": 10400, - "volume": 366495 - }, - "id": 2652993, - "non_hive": { - "close": 132, - "high": 12760, - "low": 6, - "open": 2240, - "volume": 78684 - }, - "open": "2020-07-02T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4740, - "high": 432, - "low": 12, - "open": 9475, - "volume": 2011086 - }, - "id": 2653031, - "non_hive": { - "close": 1000, - "high": 93, - "low": 2, - "open": 2000, - "volume": 423931 - }, - "open": "2020-07-02T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6145, - "high": 88, - "low": 226, - "open": 9517, - "volume": 3772201 - }, - "id": 2653074, - "non_hive": { - "close": 1310, - "high": 19, - "low": 47, - "open": 2000, - "volume": 792781 - }, - "open": "2020-07-02T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1274, - "high": 32, - "low": 45, - "open": 32, - "volume": 4254691 - }, - "id": 2653124, - "non_hive": { - "close": 267, - "high": 7, - "low": 9, - "open": 7, - "volume": 895268 - }, - "open": "2020-07-02T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 129056, - "high": 6872, - "low": 100, - "open": 9434, - "volume": 4029426 - }, - "id": 2653174, - "non_hive": { - "close": 27104, - "high": 1457, - "low": 21, - "open": 1999, - "volume": 847136 - }, - "open": "2020-07-02T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 496431, - "high": 45217, - "low": 15, - "open": 9442, - "volume": 4551347 - }, - "id": 2653225, - "non_hive": { - "close": 105188, - "high": 9628, - "low": 3, - "open": 1999, - "volume": 957689 - }, - "open": "2020-07-02T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12300, - "high": 4, - "low": 7623, - "open": 1560, - "volume": 2460625 - }, - "id": 2653305, - "non_hive": { - "close": 2590, - "high": 1, - "low": 1570, - "open": 330, - "volume": 514156 - }, - "open": "2020-07-02T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 132949, - "high": 11457, - "low": 67, - "open": 1567, - "volume": 6713974 - }, - "id": 2653368, - "non_hive": { - "close": 27786, - "high": 2412, - "low": 13, - "open": 329, - "volume": 1396919 - }, - "open": "2020-07-02T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9409, - "high": 90, - "low": 9, - "open": 23732, - "volume": 2803638 - }, - "id": 2653437, - "non_hive": { - "close": 1974, - "high": 19, - "low": 1, - "open": 5000, - "volume": 582251 - }, - "open": "2020-07-02T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 279, - "high": 279, - "low": 47592, - "open": 120, - "volume": 2052429 - }, - "id": 2653501, - "non_hive": { - "close": 59, - "high": 59, - "low": 9886, - "open": 25, - "volume": 428747 - }, - "open": "2020-07-02T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2879, - "high": 322, - "low": 264, - "open": 9604, - "volume": 3216983 - }, - "id": 2653546, - "non_hive": { - "close": 601, - "high": 68, - "low": 54, - "open": 2000, - "volume": 667413 - }, - "open": "2020-07-02T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 974, - "high": 44289, - "low": 580274, - "open": 44289, - "volume": 3152797 - }, - "id": 2653597, - "non_hive": { - "close": 202, - "high": 9239, - "low": 120116, - "open": 9239, - "volume": 652893 - }, - "open": "2020-07-02T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 440, - "high": 971, - "low": 71, - "open": 9620, - "volume": 284925 - }, - "id": 2653639, - "non_hive": { - "close": 91, - "high": 204, - "low": 14, - "open": 1999, - "volume": 59691 - }, - "open": "2020-07-02T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2479, - "high": 23, - "low": 15, - "open": 7698, - "volume": 2593098 - }, - "id": 2653671, - "non_hive": { - "close": 523, - "high": 5, - "low": 3, - "open": 1614, - "volume": 539936 - }, - "open": "2020-07-02T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53, - "high": 5579, - "low": 53, - "open": 5579, - "volume": 723503 - }, - "id": 2653722, - "non_hive": { - "close": 11, - "high": 1176, - "low": 11, - "open": 1176, - "volume": 150549 - }, - "open": "2020-07-03T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14318, - "high": 8147, - "low": 241435, - "open": 28718, - "volume": 668763 - }, - "id": 2653744, - "non_hive": { - "close": 3018, - "high": 1719, - "low": 50218, - "open": 6059, - "volume": 139342 - }, - "open": "2020-07-03T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33, - "high": 1847, - "low": 33, - "open": 521, - "volume": 65326 - }, - "id": 2653760, - "non_hive": { - "close": 6, - "high": 389, - "low": 6, - "open": 109, - "volume": 13741 - }, - "open": "2020-07-03T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15728, - "high": 644, - "low": 5309, - "open": 644, - "volume": 116373 - }, - "id": 2653786, - "non_hive": { - "close": 3304, - "high": 136, - "low": 1110, - "open": 136, - "volume": 24516 - }, - "open": "2020-07-03T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 598059, - "high": 42, - "low": 26, - "open": 5309, - "volume": 1254476 - }, - "id": 2653814, - "non_hive": { - "close": 124396, - "high": 9, - "low": 5, - "open": 1120, - "volume": 261138 - }, - "open": "2020-07-03T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2389, - "high": 1325, - "low": 179959, - "open": 9613, - "volume": 3154310 - }, - "id": 2653838, - "non_hive": { - "close": 501, - "high": 279, - "low": 37431, - "open": 2000, - "volume": 656447 - }, - "open": "2020-07-03T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2426, - "high": 162, - "low": 4940, - "open": 9088, - "volume": 3160459 - }, - "id": 2653884, - "non_hive": { - "close": 505, - "high": 34, - "low": 1027, - "open": 1906, - "volume": 657535 - }, - "open": "2020-07-03T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 370255, - "high": 28, - "low": 214550, - "open": 9615, - "volume": 3713319 - }, - "id": 2653926, - "non_hive": { - "close": 77013, - "high": 6, - "low": 44626, - "open": 2000, - "volume": 772479 - }, - "open": "2020-07-03T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 884, - "high": 38, - "low": 548694, - "open": 18505, - "volume": 2170314 - }, - "id": 2653960, - "non_hive": { - "close": 185, - "high": 8, - "low": 114128, - "open": 3879, - "volume": 452096 - }, - "open": "2020-07-03T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3144, - "high": 114, - "low": 292297, - "open": 784, - "volume": 2363117 - }, - "id": 2654007, - "non_hive": { - "close": 657, - "high": 24, - "low": 60797, - "open": 164, - "volume": 492163 - }, - "open": "2020-07-03T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18734, - "high": 7034, - "low": 158979, - "open": 600000, - "volume": 3727537 - }, - "id": 2654062, - "non_hive": { - "close": 3946, - "high": 1484, - "low": 33226, - "open": 125400, - "volume": 779297 - }, - "open": "2020-07-03T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 276, - "high": 564, - "low": 315834, - "open": 564, - "volume": 3793733 - }, - "id": 2654106, - "non_hive": { - "close": 58, - "high": 119, - "low": 65693, - "open": 119, - "volume": 790242 - }, - "open": "2020-07-03T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9552, - "high": 124, - "low": 590340, - "open": 10000, - "volume": 3342872 - }, - "id": 2654160, - "non_hive": { - "close": 1999, - "high": 26, - "low": 122200, - "open": 2081, - "volume": 694087 - }, - "open": "2020-07-03T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12097, - "high": 27046, - "low": 40340, - "open": 105, - "volume": 3038214 - }, - "id": 2654198, - "non_hive": { - "close": 2535, - "high": 5669, - "low": 8350, - "open": 22, - "volume": 630399 - }, - "open": "2020-07-03T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9983, - "high": 148, - "low": 9, - "open": 18424, - "volume": 3174961 - }, - "id": 2654232, - "non_hive": { - "close": 2074, - "high": 31, - "low": 1, - "open": 3858, - "volume": 657559 - }, - "open": "2020-07-03T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29, - "high": 258, - "low": 15, - "open": 8101, - "volume": 4616663 - }, - "id": 2654298, - "non_hive": { - "close": 6, - "high": 54, - "low": 3, - "open": 1683, - "volume": 961970 - }, - "open": "2020-07-03T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 328670, - "high": 80099, - "low": 574946, - "open": 9567, - "volume": 3449703 - }, - "id": 2654357, - "non_hive": { - "close": 68377, - "high": 16901, - "low": 119588, - "open": 1999, - "volume": 718887 - }, - "open": "2020-07-03T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1574, - "high": 4, - "low": 84, - "open": 10806, - "volume": 1997115 - }, - "id": 2654402, - "non_hive": { - "close": 330, - "high": 1, - "low": 17, - "open": 2264, - "volume": 415624 - }, - "open": "2020-07-03T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 454, - "high": 90, - "low": 425196, - "open": 2197, - "volume": 1816780 - }, - "id": 2654444, - "non_hive": { - "close": 95, - "high": 19, - "low": 88440, - "open": 461, - "volume": 378685 - }, - "open": "2020-07-03T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9613, - "high": 939, - "low": 130280, - "open": 9606, - "volume": 3109006 - }, - "id": 2654503, - "non_hive": { - "close": 2000, - "high": 197, - "low": 27098, - "open": 2000, - "volume": 646777 - }, - "open": "2020-07-03T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9614, - "high": 57, - "low": 75626, - "open": 9568, - "volume": 1204831 - }, - "id": 2654561, - "non_hive": { - "close": 2000, - "high": 12, - "low": 15730, - "open": 1999, - "volume": 250899 - }, - "open": "2020-07-03T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29044, - "high": 593, - "low": 52634, - "open": 52634, - "volume": 173233 - }, - "id": 2654612, - "non_hive": { - "close": 6065, - "high": 124, - "low": 10948, - "open": 10948, - "volume": 36119 - }, - "open": "2020-07-03T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3150, - "high": 243242, - "low": 29, - "open": 18287, - "volume": 2729078 - }, - "id": 2654649, - "non_hive": { - "close": 656, - "high": 50793, - "low": 6, - "open": 3817, - "volume": 565798 - }, - "open": "2020-07-03T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 575561, - "high": 44864, - "low": 5, - "open": 44864, - "volume": 3627497 - }, - "id": 2654679, - "non_hive": { - "close": 119141, - "high": 9344, - "low": 1, - "open": 9344, - "volume": 751252 - }, - "open": "2020-07-03T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 591629, - "high": 8792, - "low": 125, - "open": 600000, - "volume": 3662733 - }, - "id": 2654718, - "non_hive": { - "close": 122467, - "high": 1830, - "low": 25, - "open": 124800, - "volume": 758853 - }, - "open": "2020-07-04T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 279, - "high": 23, - "low": 582266, - "open": 18231, - "volume": 1839945 - }, - "id": 2654752, - "non_hive": { - "close": 58, - "high": 5, - "low": 120529, - "open": 3786, - "volume": 380946 - }, - "open": "2020-07-04T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28, - "high": 4, - "low": 5, - "open": 279, - "volume": 5569752 - }, - "id": 2654779, - "non_hive": { - "close": 6, - "high": 1, - "low": 1, - "open": 58, - "volume": 1153077 - }, - "open": "2020-07-04T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 591034, - "high": 412, - "low": 305405, - "open": 9599, - "volume": 3710843 - }, - "id": 2654821, - "non_hive": { - "close": 122344, - "high": 86, - "low": 63218, - "open": 1999, - "volume": 768253 - }, - "open": "2020-07-04T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 574207, - "high": 604, - "low": 58, - "open": 18300, - "volume": 3462006 - }, - "id": 2654857, - "non_hive": { - "close": 118860, - "high": 126, - "low": 12, - "open": 3813, - "volume": 717713 - }, - "open": "2020-07-04T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1334, - "high": 14, - "low": 112, - "open": 18148, - "volume": 3329247 - }, - "id": 2654904, - "non_hive": { - "close": 278, - "high": 3, - "low": 23, - "open": 3766, - "volume": 690897 - }, - "open": "2020-07-04T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 95, - "high": 639, - "low": 6, - "open": 22000, - "volume": 7486121 - }, - "id": 2654944, - "non_hive": { - "close": 19, - "high": 135, - "low": 1, - "open": 4568, - "volume": 1557797 - }, - "open": "2020-07-04T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23852, - "high": 190, - "low": 48, - "open": 9630, - "volume": 841588 - }, - "id": 2654972, - "non_hive": { - "close": 4973, - "high": 40, - "low": 9, - "open": 2000, - "volume": 175078 - }, - "open": "2020-07-04T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 87438, - "high": 87438, - "low": 39, - "open": 1584, - "volume": 443638 - }, - "id": 2655019, - "non_hive": { - "close": 18449, - "high": 18449, - "low": 8, - "open": 330, - "volume": 92951 - }, - "open": "2020-07-04T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2289, - "high": 113, - "low": 470, - "open": 48759, - "volume": 415370 - }, - "id": 2655059, - "non_hive": { - "close": 483, - "high": 24, - "low": 98, - "open": 10288, - "volume": 87630 - }, - "open": "2020-07-04T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5890, - "high": 3976, - "low": 3483, - "open": 18802, - "volume": 174432 - }, - "id": 2655107, - "non_hive": { - "close": 1242, - "high": 839, - "low": 731, - "open": 3966, - "volume": 36782 - }, - "open": "2020-07-04T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 528, - "high": 528, - "low": 24, - "open": 683, - "volume": 2321856 - }, - "id": 2655145, - "non_hive": { - "close": 113, - "high": 113, - "low": 5, - "open": 144, - "volume": 489930 - }, - "open": "2020-07-04T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8495, - "high": 92622, - "low": 8, - "open": 103045, - "volume": 3692568 - }, - "id": 2655189, - "non_hive": { - "close": 1820, - "high": 19847, - "low": 1, - "open": 22000, - "volume": 786989 - }, - "open": "2020-07-04T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19266, - "high": 168, - "low": 6, - "open": 211610, - "volume": 468695 - }, - "id": 2655252, - "non_hive": { - "close": 4124, - "high": 36, - "low": 1, - "open": 45333, - "volume": 100405 - }, - "open": "2020-07-04T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1491, - "high": 3479, - "low": 587594, - "open": 103, - "volume": 3532330 - }, - "id": 2655289, - "non_hive": { - "close": 322, - "high": 752, - "low": 125306, - "open": 22, - "volume": 756074 - }, - "open": "2020-07-04T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33, - "high": 10409, - "low": 29, - "open": 9340, - "volume": 4671081 - }, - "id": 2655341, - "non_hive": { - "close": 7, - "high": 2250, - "low": 6, - "open": 2000, - "volume": 1001466 - }, - "open": "2020-07-04T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 582430, - "high": 4078, - "low": 582430, - "open": 9283, - "volume": 3685269 - }, - "id": 2655397, - "non_hive": { - "close": 124117, - "high": 880, - "low": 124117, - "open": 1999, - "volume": 787269 - }, - "open": "2020-07-04T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 892, - "high": 4, - "low": 892, - "open": 1537, - "volume": 6661083 - }, - "id": 2655434, - "non_hive": { - "close": 187, - "high": 1, - "low": 187, - "open": 330, - "volume": 1413143 - }, - "open": "2020-07-04T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4680, - "high": 126, - "low": 11, - "open": 9281, - "volume": 1862595 - }, - "id": 2655480, - "non_hive": { - "close": 1000, - "high": 27, - "low": 2, - "open": 1983, - "volume": 396630 - }, - "open": "2020-07-04T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4145, - "high": 2360, - "low": 10508, - "open": 9492, - "volume": 953807 - }, - "id": 2655538, - "non_hive": { - "close": 883, - "high": 505, - "low": 2213, - "open": 2000, - "volume": 202156 - }, - "open": "2020-07-04T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13574, - "high": 1483, - "low": 52, - "open": 11451, - "volume": 1552682 - }, - "id": 2655584, - "non_hive": { - "close": 2891, - "high": 316, - "low": 10, - "open": 2439, - "volume": 328565 - }, - "open": "2020-07-04T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47034, - "high": 314, - "low": 11, - "open": 9510, - "volume": 1859319 - }, - "id": 2655627, - "non_hive": { - "close": 10000, - "high": 67, - "low": 2, - "open": 2000, - "volume": 390524 - }, - "open": "2020-07-04T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590485, - "high": 4000, - "low": 25, - "open": 2613, - "volume": 3983798 - }, - "id": 2655650, - "non_hive": { - "close": 124098, - "high": 851, - "low": 5, - "open": 555, - "volume": 838585 - }, - "open": "2020-07-04T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28451, - "high": 738, - "low": 31, - "open": 300000, - "volume": 4074357 - }, - "id": 2655704, - "non_hive": { - "close": 5982, - "high": 157, - "low": 6, - "open": 63059, - "volume": 857267 - }, - "open": "2020-07-04T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 595652, - "high": 84426, - "low": 172, - "open": 7192, - "volume": 1059450 - }, - "id": 2655756, - "non_hive": { - "close": 126861, - "high": 17981, - "low": 36, - "open": 1530, - "volume": 225299 - }, - "open": "2020-07-05T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 248, - "low": 86, - "open": 2878, - "volume": 1907402 - }, - "id": 2655789, - "non_hive": { - "close": 126008, - "high": 53, - "low": 18, - "open": 613, - "volume": 400662 - }, - "open": "2020-07-05T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23128, - "high": 37, - "low": 204, - "open": 108, - "volume": 2708483 - }, - "id": 2655813, - "non_hive": { - "close": 4920, - "high": 8, - "low": 42, - "open": 23, - "volume": 569052 - }, - "open": "2020-07-05T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 581767, - "high": 122, - "low": 173138, - "open": 9522, - "volume": 3037668 - }, - "id": 2655849, - "non_hive": { - "close": 121591, - "high": 26, - "low": 36186, - "open": 2000, - "volume": 637508 - }, - "open": "2020-07-05T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6392, - "high": 10321, - "low": 58, - "open": 9391, - "volume": 3619671 - }, - "id": 2655875, - "non_hive": { - "close": 1360, - "high": 2197, - "low": 12, - "open": 1999, - "volume": 756695 - }, - "open": "2020-07-05T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 588466, - "high": 126, - "low": 341, - "open": 14906, - "volume": 3035767 - }, - "id": 2655905, - "non_hive": { - "close": 122548, - "high": 27, - "low": 71, - "open": 3173, - "volume": 633357 - }, - "open": "2020-07-05T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 560164, - "high": 216, - "low": 16400, - "open": 9393, - "volume": 3841747 - }, - "id": 2655932, - "non_hive": { - "close": 116514, - "high": 46, - "low": 3411, - "open": 1999, - "volume": 799633 - }, - "open": "2020-07-05T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 719, - "high": 719, - "low": 590894, - "open": 48290, - "volume": 3619635 - }, - "id": 2655987, - "non_hive": { - "close": 153, - "high": 153, - "low": 122905, - "open": 10059, - "volume": 753045 - }, - "open": "2020-07-05T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 583414, - "high": 84, - "low": 72376, - "open": 84, - "volume": 3053399 - }, - "id": 2656013, - "non_hive": { - "close": 121356, - "high": 18, - "low": 15054, - "open": 18, - "volume": 635422 - }, - "open": "2020-07-05T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4931, - "high": 94, - "low": 55, - "open": 9479, - "volume": 3766583 - }, - "id": 2656058, - "non_hive": { - "close": 1034, - "high": 20, - "low": 11, - "open": 1999, - "volume": 783844 - }, - "open": "2020-07-05T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1574, - "high": 5262, - "low": 16, - "open": 1586, - "volume": 3663721 - }, - "id": 2656122, - "non_hive": { - "close": 331, - "high": 1108, - "low": 3, - "open": 330, - "volume": 762063 - }, - "open": "2020-07-05T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3476, - "high": 308, - "low": 71, - "open": 1568, - "volume": 3114324 - }, - "id": 2656169, - "non_hive": { - "close": 731, - "high": 65, - "low": 14, - "open": 330, - "volume": 642073 - }, - "open": "2020-07-05T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5000, - "high": 575, - "low": 38, - "open": 1570, - "volume": 3858159 - }, - "id": 2656214, - "non_hive": { - "close": 1030, - "high": 122, - "low": 7, - "open": 329, - "volume": 795632 - }, - "open": "2020-07-05T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8523, - "high": 477, - "low": 48, - "open": 17311, - "volume": 3930770 - }, - "id": 2656274, - "non_hive": { - "close": 1782, - "high": 100, - "low": 9, - "open": 3616, - "volume": 810687 - }, - "open": "2020-07-05T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43527, - "high": 4, - "low": 30, - "open": 9701, - "volume": 3245267 - }, - "id": 2656316, - "non_hive": { - "close": 8971, - "high": 1, - "low": 6, - "open": 2000, - "volume": 670574 - }, - "open": "2020-07-05T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72, - "high": 16967, - "low": 72, - "open": 16967, - "volume": 318500 - }, - "id": 2656357, - "non_hive": { - "close": 14, - "high": 3601, - "low": 14, - "open": 3601, - "volume": 67258 - }, - "open": "2020-07-05T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 941, - "high": 14, - "low": 46, - "open": 7293, - "volume": 2149852 - }, - "id": 2656411, - "non_hive": { - "close": 198, - "high": 3, - "low": 9, - "open": 1545, - "volume": 444248 - }, - "open": "2020-07-05T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12210, - "high": 1264, - "low": 127, - "open": 1319, - "volume": 1619088 - }, - "id": 2656457, - "non_hive": { - "close": 2526, - "high": 267, - "low": 26, - "open": 277, - "volume": 335622 - }, - "open": "2020-07-05T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 585144, - "high": 308, - "low": 66, - "open": 1565, - "volume": 1974746 - }, - "id": 2656500, - "non_hive": { - "close": 120540, - "high": 65, - "low": 13, - "open": 330, - "volume": 408014 - }, - "open": "2020-07-05T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4868, - "high": 62, - "low": 589485, - "open": 2405, - "volume": 1967574 - }, - "id": 2656543, - "non_hive": { - "close": 1012, - "high": 13, - "low": 121434, - "open": 500, - "volume": 405724 - }, - "open": "2020-07-05T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11391, - "high": 6731, - "low": 18589, - "open": 5183, - "volume": 811043 - }, - "id": 2656587, - "non_hive": { - "close": 2391, - "high": 1421, - "low": 3810, - "open": 1078, - "volume": 167360 - }, - "open": "2020-07-05T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5009, - "high": 10500, - "low": 198, - "open": 18722, - "volume": 255144 - }, - "id": 2656623, - "non_hive": { - "close": 1051, - "high": 2204, - "low": 41, - "open": 3913, - "volume": 53461 - }, - "open": "2020-07-05T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31907, - "high": 2837, - "low": 29, - "open": 5325, - "volume": 233506 - }, - "id": 2656657, - "non_hive": { - "close": 6667, - "high": 593, - "low": 6, - "open": 1113, - "volume": 48787 - }, - "open": "2020-07-05T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44214, - "high": 44214, - "low": 15, - "open": 1416, - "volume": 3074715 - }, - "id": 2656683, - "non_hive": { - "close": 9286, - "high": 9286, - "low": 3, - "open": 296, - "volume": 639876 - }, - "open": "2020-07-05T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3357, - "high": 208, - "low": 33, - "open": 208, - "volume": 2451561 - }, - "id": 2656720, - "non_hive": { - "close": 708, - "high": 44, - "low": 6, - "open": 44, - "volume": 505302 - }, - "open": "2020-07-06T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1709, - "high": 139654, - "low": 73, - "open": 5588, - "volume": 3817686 - }, - "id": 2656759, - "non_hive": { - "close": 352, - "high": 29486, - "low": 15, - "open": 1178, - "volume": 793450 - }, - "open": "2020-07-06T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 644, - "high": 393, - "low": 5, - "open": 9612, - "volume": 1190558 - }, - "id": 2656797, - "non_hive": { - "close": 136, - "high": 83, - "low": 1, - "open": 2000, - "volume": 247878 - }, - "open": "2020-07-06T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111955, - "high": 867, - "low": 10, - "open": 9479, - "volume": 2466804 - }, - "id": 2656818, - "non_hive": { - "close": 23066, - "high": 183, - "low": 2, - "open": 1999, - "volume": 509034 - }, - "open": "2020-07-06T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5057, - "high": 787, - "low": 58, - "open": 9492, - "volume": 630787 - }, - "id": 2656846, - "non_hive": { - "close": 1065, - "high": 166, - "low": 12, - "open": 1999, - "volume": 131903 - }, - "open": "2020-07-06T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47, - "high": 47, - "low": 590297, - "open": 244, - "volume": 1050039 - }, - "id": 2656863, - "non_hive": { - "close": 10, - "high": 10, - "low": 122799, - "open": 51, - "volume": 219847 - }, - "open": "2020-07-06T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1661, - "high": 253, - "low": 8, - "open": 371, - "volume": 579695 - }, - "id": 2656885, - "non_hive": { - "close": 353, - "high": 54, - "low": 1, - "open": 79, - "volume": 121954 - }, - "open": "2020-07-06T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3588, - "high": 32, - "low": 11, - "open": 1082, - "volume": 923140 - }, - "id": 2656922, - "non_hive": { - "close": 771, - "high": 7, - "low": 2, - "open": 230, - "volume": 194572 - }, - "open": "2020-07-06T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 429, - "high": 820, - "low": 5, - "open": 9517, - "volume": 181944 - }, - "id": 2656974, - "non_hive": { - "close": 92, - "high": 176, - "low": 1, - "open": 2000, - "volume": 38672 - }, - "open": "2020-07-06T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 179860, - "high": 9, - "low": 223, - "open": 29076, - "volume": 3444315 - }, - "id": 2657006, - "non_hive": { - "close": 37519, - "high": 2, - "low": 46, - "open": 6135, - "volume": 721504 - }, - "open": "2020-07-06T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18670, - "high": 19693, - "low": 590414, - "open": 9497, - "volume": 823027 - }, - "id": 2657073, - "non_hive": { - "close": 4000, - "high": 4224, - "low": 123164, - "open": 1999, - "volume": 172322 - }, - "open": "2020-07-06T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1544, - "high": 5276, - "low": 71, - "open": 154, - "volume": 196567 - }, - "id": 2657104, - "non_hive": { - "close": 330, - "high": 1131, - "low": 14, - "open": 33, - "volume": 41474 - }, - "open": "2020-07-06T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15636, - "high": 16321, - "low": 304, - "open": 46788, - "volume": 1552541 - }, - "id": 2657141, - "non_hive": { - "close": 3341, - "high": 3492, - "low": 63, - "open": 10000, - "volume": 329887 - }, - "open": "2020-07-06T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5290, - "high": 65, - "low": 11669, - "open": 9356, - "volume": 690059 - }, - "id": 2657178, - "non_hive": { - "close": 1131, - "high": 14, - "low": 2462, - "open": 1999, - "volume": 147465 - }, - "open": "2020-07-06T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14939, - "high": 56, - "low": 11, - "open": 56, - "volume": 1599365 - }, - "id": 2657214, - "non_hive": { - "close": 3194, - "high": 12, - "low": 2, - "open": 12, - "volume": 339135 - }, - "open": "2020-07-06T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10184, - "high": 406, - "low": 15, - "open": 2501, - "volume": 436247 - }, - "id": 2657258, - "non_hive": { - "close": 2178, - "high": 87, - "low": 3, - "open": 532, - "volume": 93250 - }, - "open": "2020-07-06T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5509, - "high": 5509, - "low": 796, - "open": 4002, - "volume": 2711507 - }, - "id": 2657296, - "non_hive": { - "close": 1211, - "high": 1211, - "low": 169, - "open": 856, - "volume": 584605 - }, - "open": "2020-07-06T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5854, - "high": 259, - "low": 27, - "open": 9190, - "volume": 811596 - }, - "id": 2657338, - "non_hive": { - "close": 1329, - "high": 59, - "low": 5, - "open": 2000, - "volume": 177175 - }, - "open": "2020-07-06T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1663, - "high": 2612, - "low": 578588, - "open": 5146, - "volume": 2267270 - }, - "id": 2657395, - "non_hive": { - "close": 369, - "high": 593, - "low": 125612, - "open": 1168, - "volume": 500147 - }, - "open": "2020-07-06T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5666, - "high": 63, - "low": 42, - "open": 9209, - "volume": 2174724 - }, - "id": 2657450, - "non_hive": { - "close": 1256, - "high": 14, - "low": 9, - "open": 2000, - "volume": 473227 - }, - "open": "2020-07-06T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 505, - "high": 505, - "low": 357, - "open": 9130, - "volume": 2446645 - }, - "id": 2657497, - "non_hive": { - "close": 112, - "high": 112, - "low": 77, - "open": 2000, - "volume": 530917 - }, - "open": "2020-07-06T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 177, - "high": 36, - "low": 1218, - "open": 36, - "volume": 112562 - }, - "id": 2657529, - "non_hive": { - "close": 39, - "high": 8, - "low": 263, - "open": 8, - "volume": 24687 - }, - "open": "2020-07-06T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5006, - "high": 225, - "low": 28, - "open": 687, - "volume": 50685 - }, - "id": 2657556, - "non_hive": { - "close": 1111, - "high": 50, - "low": 6, - "open": 151, - "volume": 11210 - }, - "open": "2020-07-06T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8834, - "high": 13258, - "low": 133, - "open": 46364, - "volume": 1192176 - }, - "id": 2657580, - "non_hive": { - "close": 1999, - "high": 3010, - "low": 28, - "open": 10287, - "volume": 267831 - }, - "open": "2020-07-06T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 338, - "high": 338, - "low": 388726, - "open": 45, - "volume": 456513 - }, - "id": 2657604, - "non_hive": { - "close": 77, - "high": 77, - "low": 84567, - "open": 10, - "volume": 99844 - }, - "open": "2020-07-07T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4704, - "high": 325, - "low": 3551, - "open": 15959, - "volume": 93536 - }, - "id": 2657628, - "non_hive": { - "close": 1069, - "high": 74, - "low": 806, - "open": 3626, - "volume": 21255 - }, - "open": "2020-07-07T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1302, - "high": 303, - "low": 1501, - "open": 17865, - "volume": 2483350 - }, - "id": 2657654, - "non_hive": { - "close": 296, - "high": 69, - "low": 325, - "open": 4060, - "volume": 540495 - }, - "open": "2020-07-07T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8955, - "high": 83, - "low": 12387, - "open": 145000, - "volume": 764065 - }, - "id": 2657685, - "non_hive": { - "close": 1993, - "high": 19, - "low": 2689, - "open": 32914, - "volume": 167469 - }, - "open": "2020-07-07T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18290, - "high": 18290, - "low": 55, - "open": 1623, - "volume": 47380 - }, - "id": 2657703, - "non_hive": { - "close": 4070, - "high": 4070, - "low": 12, - "open": 361, - "volume": 10540 - }, - "open": "2020-07-07T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4296, - "high": 5560, - "low": 218, - "open": 5560, - "volume": 921536 - }, - "id": 2657716, - "non_hive": { - "close": 941, - "high": 1237, - "low": 47, - "open": 1237, - "volume": 201098 - }, - "open": "2020-07-07T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1500, - "high": 436, - "low": 155111, - "open": 9212, - "volume": 1040615 - }, - "id": 2657760, - "non_hive": { - "close": 328, - "high": 96, - "low": 32728, - "open": 2000, - "volume": 225879 - }, - "open": "2020-07-07T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23995, - "high": 23995, - "low": 83, - "open": 4500, - "volume": 2773198 - }, - "id": 2657790, - "non_hive": { - "close": 5263, - "high": 5263, - "low": 18, - "open": 984, - "volume": 602583 - }, - "open": "2020-07-07T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1951344, - "high": 714, - "low": 2100, - "open": 9200, - "volume": 11205068 - }, - "id": 2657838, - "non_hive": { - "close": 425393, - "high": 157, - "low": 455, - "open": 2000, - "volume": 2443459 - }, - "open": "2020-07-07T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 500, - "high": 10583, - "low": 220, - "open": 981, - "volume": 3563447 - }, - "id": 2657885, - "non_hive": { - "close": 109, - "high": 2325, - "low": 47, - "open": 215, - "volume": 777614 - }, - "open": "2020-07-07T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16653, - "high": 136, - "low": 843, - "open": 136, - "volume": 4825572 - }, - "id": 2657931, - "non_hive": { - "close": 3658, - "high": 30, - "low": 183, - "open": 30, - "volume": 1054534 - }, - "open": "2020-07-07T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 200048, - "high": 236, - "low": 175, - "open": 4937, - "volume": 3524527 - }, - "id": 2657974, - "non_hive": { - "close": 43993, - "high": 52, - "low": 38, - "open": 1084, - "volume": 770658 - }, - "open": "2020-07-07T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4364, - "high": 118, - "low": 79, - "open": 321218, - "volume": 4042517 - }, - "id": 2658012, - "non_hive": { - "close": 959, - "high": 26, - "low": 17, - "open": 70459, - "volume": 884354 - }, - "open": "2020-07-07T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5024, - "high": 329, - "low": 319, - "open": 332155, - "volume": 4947874 - }, - "id": 2658054, - "non_hive": { - "close": 1128, - "high": 74, - "low": 69, - "open": 72526, - "volume": 1085781 - }, - "open": "2020-07-07T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1397, - "high": 535108, - "low": 109, - "open": 976, - "volume": 3618739 - }, - "id": 2658089, - "non_hive": { - "close": 312, - "high": 120192, - "low": 23, - "open": 219, - "volume": 798844 - }, - "open": "2020-07-07T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 6, - "open": 421, - "volume": 3277472 - }, - "id": 2658142, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 94, - "volume": 718503 - }, - "open": "2020-07-07T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590869, - "high": 262, - "low": 590827, - "open": 6939, - "volume": 3973152 - }, - "id": 2658214, - "non_hive": { - "close": 129401, - "high": 59, - "low": 128800, - "open": 1536, - "volume": 870166 - }, - "open": "2020-07-07T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 756, - "high": 49042, - "low": 52, - "open": 3625, - "volume": 4719948 - }, - "id": 2658251, - "non_hive": { - "close": 167, - "high": 11001, - "low": 11, - "open": 811, - "volume": 1037830 - }, - "open": "2020-07-07T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590829, - "high": 4, - "low": 62840, - "open": 9052, - "volume": 4103490 - }, - "id": 2658321, - "non_hive": { - "close": 128820, - "high": 1, - "low": 13658, - "open": 1999, - "volume": 898868 - }, - "open": "2020-07-07T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590830, - "high": 7592, - "low": 100, - "open": 4537, - "volume": 3801351 - }, - "id": 2658374, - "non_hive": { - "close": 128846, - "high": 1674, - "low": 21, - "open": 1000, - "volume": 830888 - }, - "open": "2020-07-07T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13208, - "high": 222, - "low": 187, - "open": 7886, - "volume": 2139753 - }, - "id": 2658418, - "non_hive": { - "close": 2913, - "high": 49, - "low": 40, - "open": 1737, - "volume": 466923 - }, - "open": "2020-07-07T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9007, - "high": 113, - "low": 6296, - "open": 18146, - "volume": 877229 - }, - "id": 2658476, - "non_hive": { - "close": 1985, - "high": 25, - "low": 1361, - "open": 4000, - "volume": 191299 - }, - "open": "2020-07-07T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 176, - "high": 176, - "low": 28, - "open": 9130, - "volume": 1869937 - }, - "id": 2658519, - "non_hive": { - "close": 39, - "high": 39, - "low": 6, - "open": 2000, - "volume": 404862 - }, - "open": "2020-07-07T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 433518, - "high": 4, - "low": 5, - "open": 9282, - "volume": 2017816 - }, - "id": 2658565, - "non_hive": { - "close": 93379, - "high": 1, - "low": 1, - "open": 2000, - "volume": 435731 - }, - "open": "2020-07-07T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 556599, - "high": 40, - "low": 87, - "open": 322, - "volume": 2506028 - }, - "id": 2658599, - "non_hive": { - "close": 119668, - "high": 9, - "low": 18, - "open": 71, - "volume": 539314 - }, - "open": "2020-07-08T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 304, - "high": 27, - "low": 137, - "open": 9091, - "volume": 1242731 - }, - "id": 2658637, - "non_hive": { - "close": 67, - "high": 6, - "low": 29, - "open": 1999, - "volume": 267463 - }, - "open": "2020-07-08T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 148, - "high": 312, - "low": 104, - "open": 560, - "volume": 54006 - }, - "id": 2658668, - "non_hive": { - "close": 32, - "high": 69, - "low": 22, - "open": 123, - "volume": 11904 - }, - "open": "2020-07-08T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57367, - "high": 2189, - "low": 12, - "open": 2189, - "volume": 2483970 - }, - "id": 2658698, - "non_hive": { - "close": 12633, - "high": 483, - "low": 2, - "open": 483, - "volume": 539582 - }, - "open": "2020-07-08T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 295, - "high": 176436, - "low": 56, - "open": 554, - "volume": 5379939 - }, - "id": 2658727, - "non_hive": { - "close": 65, - "high": 38917, - "low": 12, - "open": 122, - "volume": 1169213 - }, - "open": "2020-07-08T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 685, - "high": 4, - "low": 14, - "open": 9175, - "volume": 3031026 - }, - "id": 2658772, - "non_hive": { - "close": 151, - "high": 1, - "low": 3, - "open": 2000, - "volume": 659390 - }, - "open": "2020-07-08T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 523202, - "high": 172, - "low": 133, - "open": 9208, - "volume": 2620707 - }, - "id": 2658803, - "non_hive": { - "close": 112493, - "high": 38, - "low": 28, - "open": 2000, - "volume": 566937 - }, - "open": "2020-07-08T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590800, - "high": 2554, - "low": 7, - "open": 1499, - "volume": 3710552 - }, - "id": 2658846, - "non_hive": { - "close": 128407, - "high": 562, - "low": 1, - "open": 329, - "volume": 805282 - }, - "open": "2020-07-08T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 424632, - "high": 36, - "low": 557355, - "open": 3068, - "volume": 5045534 - }, - "id": 2658899, - "non_hive": { - "close": 92619, - "high": 8, - "low": 121230, - "open": 668, - "volume": 1099645 - }, - "open": "2020-07-08T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4309, - "high": 4309, - "low": 26, - "open": 9172, - "volume": 4907547 - }, - "id": 2658938, - "non_hive": { - "close": 950, - "high": 950, - "low": 5, - "open": 2000, - "volume": 1070455 - }, - "open": "2020-07-08T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 581613, - "high": 9, - "low": 560420, - "open": 27628, - "volume": 3784449 - }, - "id": 2658990, - "non_hive": { - "close": 126506, - "high": 2, - "low": 121896, - "open": 6023, - "volume": 824583 - }, - "open": "2020-07-08T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 217, - "high": 217, - "low": 70, - "open": 9166, - "volume": 3866896 - }, - "id": 2659039, - "non_hive": { - "close": 48, - "high": 48, - "low": 15, - "open": 1994, - "volume": 840086 - }, - "open": "2020-07-08T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 392882, - "high": 4, - "low": 6, - "open": 9084, - "volume": 2029416 - }, - "id": 2659082, - "non_hive": { - "close": 85255, - "high": 1, - "low": 1, - "open": 1999, - "volume": 441138 - }, - "open": "2020-07-08T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 345866, - "high": 7101, - "low": 23, - "open": 9123, - "volume": 3477100 - }, - "id": 2659131, - "non_hive": { - "close": 76123, - "high": 1563, - "low": 4, - "open": 1999, - "volume": 755917 - }, - "open": "2020-07-08T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18193, - "high": 11104, - "low": 24, - "open": 9168, - "volume": 6247367 - }, - "id": 2659175, - "non_hive": { - "close": 3997, - "high": 2444, - "low": 5, - "open": 1999, - "volume": 1358970 - }, - "open": "2020-07-08T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7989, - "high": 22, - "low": 33, - "open": 9105, - "volume": 222919 - }, - "id": 2659224, - "non_hive": { - "close": 1756, - "high": 5, - "low": 7, - "open": 1999, - "volume": 48935 - }, - "open": "2020-07-08T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3466462, - "high": 172, - "low": 30171, - "open": 9100, - "volume": 5978084 - }, - "id": 2659270, - "non_hive": { - "close": 752225, - "high": 38, - "low": 6547, - "open": 1999, - "volume": 1297804 - }, - "open": "2020-07-08T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 541833, - "high": 10992, - "low": 99, - "open": 9115, - "volume": 3149321 - }, - "id": 2659313, - "non_hive": { - "close": 117578, - "high": 2412, - "low": 21, - "open": 1999, - "volume": 683672 - }, - "open": "2020-07-08T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 435460, - "high": 6823, - "low": 3856, - "open": 2152, - "volume": 5201634 - }, - "id": 2659358, - "non_hive": { - "close": 95540, - "high": 1497, - "low": 836, - "open": 470, - "volume": 1139548 - }, - "open": "2020-07-08T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1703, - "high": 4, - "low": 241, - "open": 227388, - "volume": 7518879 - }, - "id": 2659418, - "non_hive": { - "close": 378, - "high": 1, - "low": 52, - "open": 49888, - "volume": 1650110 - }, - "open": "2020-07-08T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 581677, - "high": 49, - "low": 4257, - "open": 9146, - "volume": 3311461 - }, - "id": 2659511, - "non_hive": { - "close": 126893, - "high": 11, - "low": 928, - "open": 2000, - "volume": 723353 - }, - "open": "2020-07-08T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 927, - "high": 8378, - "low": 594345, - "open": 9166, - "volume": 2082859 - }, - "id": 2659561, - "non_hive": { - "close": 204, - "high": 1902, - "low": 129656, - "open": 2000, - "volume": 455405 - }, - "open": "2020-07-08T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4392, - "high": 2895, - "low": 28, - "open": 2836, - "volume": 727074 - }, - "id": 2659605, - "non_hive": { - "close": 966, - "high": 637, - "low": 6, - "open": 624, - "volume": 158864 - }, - "open": "2020-07-08T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1177, - "high": 695, - "low": 14, - "open": 15127, - "volume": 1172330 - }, - "id": 2659639, - "non_hive": { - "close": 259, - "high": 153, - "low": 3, - "open": 3327, - "volume": 256883 - }, - "open": "2020-07-08T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2527, - "high": 77, - "low": 61, - "open": 19427, - "volume": 150603 - }, - "id": 2659672, - "non_hive": { - "close": 556, - "high": 17, - "low": 13, - "open": 4273, - "volume": 33127 - }, - "open": "2020-07-09T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11627, - "high": 11627, - "low": 19763, - "open": 9136, - "volume": 120823 - }, - "id": 2659701, - "non_hive": { - "close": 2637, - "high": 2637, - "low": 4347, - "open": 2010, - "volume": 26659 - }, - "open": "2020-07-09T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 286, - "high": 853, - "low": 5, - "open": 18300, - "volume": 58191 - }, - "id": 2659717, - "non_hive": { - "close": 65, - "high": 194, - "low": 1, - "open": 4149, - "volume": 13208 - }, - "open": "2020-07-09T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 643, - "high": 396, - "low": 643, - "open": 152754, - "volume": 383250 - }, - "id": 2659742, - "non_hive": { - "close": 141, - "high": 90, - "low": 141, - "open": 34703, - "volume": 87046 - }, - "open": "2020-07-09T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 889, - "high": 1300, - "low": 11, - "open": 250, - "volume": 1820025 - }, - "id": 2659766, - "non_hive": { - "close": 200, - "high": 293, - "low": 2, - "open": 56, - "volume": 400567 - }, - "open": "2020-07-09T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 91012, - "high": 195, - "low": 6, - "open": 4916, - "volume": 193831 - }, - "id": 2659784, - "non_hive": { - "close": 20294, - "high": 44, - "low": 1, - "open": 1105, - "volume": 43382 - }, - "open": "2020-07-09T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1050, - "high": 22, - "low": 6234, - "open": 950, - "volume": 261518 - }, - "id": 2659824, - "non_hive": { - "close": 238, - "high": 5, - "low": 1388, - "open": 212, - "volume": 58437 - }, - "open": "2020-07-09T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 284, - "high": 35, - "low": 4701, - "open": 1025, - "volume": 108974 - }, - "id": 2659892, - "non_hive": { - "close": 64, - "high": 8, - "low": 1050, - "open": 232, - "volume": 24569 - }, - "open": "2020-07-09T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56, - "high": 9699, - "low": 56, - "open": 1471, - "volume": 727222 - }, - "id": 2659933, - "non_hive": { - "close": 12, - "high": 2199, - "low": 12, - "open": 330, - "volume": 162948 - }, - "open": "2020-07-09T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 448, - "high": 398, - "low": 448, - "open": 398, - "volume": 2302082 - }, - "id": 2659964, - "non_hive": { - "close": 97, - "high": 90, - "low": 97, - "open": 90, - "volume": 504537 - }, - "open": "2020-07-09T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6433, - "high": 19523, - "low": 15, - "open": 621, - "volume": 223169 - }, - "id": 2660003, - "non_hive": { - "close": 1434, - "high": 4354, - "low": 3, - "open": 138, - "volume": 49698 - }, - "open": "2020-07-09T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29001, - "high": 291, - "low": 209, - "open": 21726, - "volume": 870170 - }, - "id": 2660034, - "non_hive": { - "close": 6470, - "high": 65, - "low": 46, - "open": 4840, - "volume": 194002 - }, - "open": "2020-07-09T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23027, - "high": 49, - "low": 5, - "open": 1066, - "volume": 2384395 - }, - "id": 2660050, - "non_hive": { - "close": 5113, - "high": 11, - "low": 1, - "open": 238, - "volume": 527675 - }, - "open": "2020-07-09T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 550456, - "high": 928, - "low": 379, - "open": 167906, - "volume": 1268310 - }, - "id": 2660082, - "non_hive": { - "close": 122219, - "high": 208, - "low": 84, - "open": 37300, - "volume": 282113 - }, - "open": "2020-07-09T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2850, - "high": 11228, - "low": 46, - "open": 8939, - "volume": 4281452 - }, - "id": 2660113, - "non_hive": { - "close": 631, - "high": 2512, - "low": 10, - "open": 1999, - "volume": 951043 - }, - "open": "2020-07-09T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28, - "high": 27, - "low": 28, - "open": 123249, - "volume": 3743042 - }, - "id": 2660173, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 27353, - "volume": 824310 - }, - "open": "2020-07-09T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6185, - "high": 47128, - "low": 578575, - "open": 160, - "volume": 3431115 - }, - "id": 2660227, - "non_hive": { - "close": 1346, - "high": 10425, - "low": 124972, - "open": 35, - "volume": 742883 - }, - "open": "2020-07-09T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5681, - "high": 261, - "low": 2460, - "open": 191028, - "volume": 3772148 - }, - "id": 2660280, - "non_hive": { - "close": 1236, - "high": 57, - "low": 531, - "open": 41310, - "volume": 815670 - }, - "open": "2020-07-09T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1511, - "high": 18, - "low": 240984, - "open": 9258, - "volume": 2836048 - }, - "id": 2660330, - "non_hive": { - "close": 329, - "high": 4, - "low": 52052, - "open": 2000, - "volume": 615411 - }, - "open": "2020-07-09T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 616, - "high": 616, - "low": 223, - "open": 1511, - "volume": 3980812 - }, - "id": 2660393, - "non_hive": { - "close": 135, - "high": 135, - "low": 48, - "open": 330, - "volume": 864183 - }, - "open": "2020-07-09T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9215, - "high": 4, - "low": 579857, - "open": 9137, - "volume": 3726610 - }, - "id": 2660449, - "non_hive": { - "close": 2000, - "high": 1, - "low": 125828, - "open": 1999, - "volume": 809009 - }, - "open": "2020-07-09T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 256, - "high": 99744, - "low": 550883, - "open": 21469, - "volume": 1263417 - }, - "id": 2660500, - "non_hive": { - "close": 56, - "high": 21844, - "low": 119541, - "open": 4684, - "volume": 275453 - }, - "open": "2020-07-09T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9100, - "high": 14168, - "low": 28, - "open": 10373, - "volume": 723221 - }, - "id": 2660527, - "non_hive": { - "close": 2002, - "high": 3117, - "low": 6, - "open": 2272, - "volume": 158061 - }, - "open": "2020-07-09T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 454, - "high": 27, - "low": 14, - "open": 71714, - "volume": 3529000 - }, - "id": 2660561, - "non_hive": { - "close": 99, - "high": 6, - "low": 3, - "open": 15777, - "volume": 770836 - }, - "open": "2020-07-09T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590846, - "high": 63, - "low": 19, - "open": 11012, - "volume": 3650526 - }, - "id": 2660604, - "non_hive": { - "close": 128808, - "high": 14, - "low": 4, - "open": 2401, - "volume": 795915 - }, - "open": "2020-07-10T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3064, - "high": 49, - "low": 8, - "open": 4744, - "volume": 2552485 - }, - "id": 2660644, - "non_hive": { - "close": 668, - "high": 11, - "low": 1, - "open": 1047, - "volume": 560351 - }, - "open": "2020-07-10T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 64, - "high": 1562, - "low": 64, - "open": 154, - "volume": 700420 - }, - "id": 2660677, - "non_hive": { - "close": 13, - "high": 345, - "low": 13, - "open": 34, - "volume": 154623 - }, - "open": "2020-07-10T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 800000, - "high": 54, - "low": 22349, - "open": 2392, - "volume": 3348281 - }, - "id": 2660699, - "non_hive": { - "close": 176000, - "high": 12, - "low": 4914, - "open": 526, - "volume": 736614 - }, - "open": "2020-07-10T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590828, - "high": 86, - "low": 563372, - "open": 600000, - "volume": 3017295 - }, - "id": 2660730, - "non_hive": { - "close": 128816, - "high": 19, - "low": 122821, - "open": 132000, - "volume": 661512 - }, - "open": "2020-07-10T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 145526, - "high": 9425, - "low": 5, - "open": 9172, - "volume": 3754120 - }, - "id": 2660752, - "non_hive": { - "close": 31726, - "high": 2081, - "low": 1, - "open": 2000, - "volume": 818810 - }, - "open": "2020-07-10T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 586585, - "high": 7767, - "low": 39, - "open": 9172, - "volume": 2428370 - }, - "id": 2660798, - "non_hive": { - "close": 127291, - "high": 1711, - "low": 8, - "open": 2000, - "volume": 527658 - }, - "open": "2020-07-10T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590785, - "high": 17415, - "low": 14894, - "open": 6277, - "volume": 3694351 - }, - "id": 2660824, - "non_hive": { - "close": 128203, - "high": 3832, - "low": 3232, - "open": 1379, - "volume": 801952 - }, - "open": "2020-07-10T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 275610, - "high": 86, - "low": 591413, - "open": 206, - "volume": 1282053 - }, - "id": 2660856, - "non_hive": { - "close": 60682, - "high": 19, - "low": 128339, - "open": 45, - "volume": 280223 - }, - "open": "2020-07-10T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8512, - "high": 8512, - "low": 6094, - "open": 15000, - "volume": 5579623 - }, - "id": 2660899, - "non_hive": { - "close": 1904, - "high": 1904, - "low": 1340, - "open": 3300, - "volume": 1231996 - }, - "open": "2020-07-10T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1273, - "high": 1273, - "low": 253474, - "open": 425, - "volume": 7284906 - }, - "id": 2660947, - "non_hive": { - "close": 289, - "high": 289, - "low": 56325, - "open": 95, - "volume": 1626559 - }, - "open": "2020-07-10T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8801, - "high": 302570, - "low": 12, - "open": 6183, - "volume": 5785492 - }, - "id": 2660981, - "non_hive": { - "close": 1993, - "high": 68682, - "low": 2, - "open": 1403, - "volume": 1313248 - }, - "open": "2020-07-10T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90309, - "high": 66, - "low": 27, - "open": 27, - "volume": 846855 - }, - "id": 2661008, - "non_hive": { - "close": 20500, - "high": 15, - "low": 6, - "open": 6, - "volume": 192218 - }, - "open": "2020-07-10T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35000, - "high": 515, - "low": 5, - "open": 16973, - "volume": 370206 - }, - "id": 2661047, - "non_hive": { - "close": 8142, - "high": 120, - "low": 1, - "open": 3853, - "volume": 84890 - }, - "open": "2020-07-10T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1615, - "high": 179, - "low": 73, - "open": 451, - "volume": 401249 - }, - "id": 2661088, - "non_hive": { - "close": 382, - "high": 43, - "low": 16, - "open": 105, - "volume": 95673 - }, - "open": "2020-07-10T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4052, - "high": 37818, - "low": 67, - "open": 26250, - "volume": 598640 - }, - "id": 2661138, - "non_hive": { - "close": 1000, - "high": 9369, - "low": 15, - "open": 6205, - "volume": 144392 - }, - "open": "2020-07-10T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15735, - "high": 24, - "low": 18932, - "open": 66737, - "volume": 2617820 - }, - "id": 2661194, - "non_hive": { - "close": 3621, - "high": 6, - "low": 4303, - "open": 16472, - "volume": 619799 - }, - "open": "2020-07-10T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 183411, - "high": 157, - "low": 582675, - "open": 8688, - "volume": 3626595 - }, - "id": 2661258, - "non_hive": { - "close": 42001, - "high": 37, - "low": 132267, - "open": 2000, - "volume": 827531 - }, - "open": "2020-07-10T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 389, - "high": 12, - "low": 118572, - "open": 218342, - "volume": 2954098 - }, - "id": 2661305, - "non_hive": { - "close": 91, - "high": 3, - "low": 26915, - "open": 50000, - "volume": 680214 - }, - "open": "2020-07-10T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7502, - "high": 571, - "low": 6457, - "open": 571, - "volume": 2183207 - }, - "id": 2661364, - "non_hive": { - "close": 1709, - "high": 133, - "low": 1462, - "open": 133, - "volume": 496218 - }, - "open": "2020-07-10T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90003, - "high": 78244, - "low": 146495, - "open": 8781, - "volume": 853489 - }, - "id": 2661412, - "non_hive": { - "close": 20478, - "high": 17820, - "low": 32847, - "open": 1999, - "volume": 193323 - }, - "open": "2020-07-10T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 170, - "high": 40507, - "low": 136, - "open": 476, - "volume": 401316 - }, - "id": 2661428, - "non_hive": { - "close": 40, - "high": 9716, - "low": 31, - "open": 109, - "volume": 93610 - }, - "open": "2020-07-10T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 71499, - "high": 4, - "low": 170, - "open": 4261, - "volume": 2111508 - }, - "id": 2661461, - "non_hive": { - "close": 16382, - "high": 1, - "low": 38, - "open": 1000, - "volume": 488779 - }, - "open": "2020-07-10T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74, - "high": 106848, - "low": 64, - "open": 682660, - "volume": 1069010 - }, - "id": 2661512, - "non_hive": { - "close": 17, - "high": 25638, - "low": 14, - "open": 156411, - "volume": 247620 - }, - "open": "2020-07-10T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56, - "high": 3873, - "low": 56, - "open": 5890, - "volume": 1481440 - }, - "id": 2661556, - "non_hive": { - "close": 12, - "high": 924, - "low": 12, - "open": 1405, - "volume": 344705 - }, - "open": "2020-07-11T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3715, - "high": 4688, - "low": 310365, - "open": 5530, - "volume": 2477956 - }, - "id": 2661602, - "non_hive": { - "close": 875, - "high": 1106, - "low": 71086, - "open": 1282, - "volume": 572221 - }, - "open": "2020-07-11T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30, - "high": 828, - "low": 30, - "open": 28041, - "volume": 205994 - }, - "id": 2661628, - "non_hive": { - "close": 6, - "high": 195, - "low": 6, - "open": 6600, - "volume": 47429 - }, - "open": "2020-07-11T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17201, - "high": 12, - "low": 107, - "open": 1065, - "volume": 329128 - }, - "id": 2661642, - "non_hive": { - "close": 4055, - "high": 3, - "low": 24, - "open": 251, - "volume": 77588 - }, - "open": "2020-07-11T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 694, - "high": 16160, - "low": 43, - "open": 5709, - "volume": 93637 - }, - "id": 2661671, - "non_hive": { - "close": 159, - "high": 3808, - "low": 9, - "open": 1345, - "volume": 22051 - }, - "open": "2020-07-11T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9872, - "high": 233, - "low": 177, - "open": 694, - "volume": 50587 - }, - "id": 2661695, - "non_hive": { - "close": 2324, - "high": 55, - "low": 40, - "open": 162, - "volume": 11884 - }, - "open": "2020-07-11T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6356, - "high": 233, - "low": 20, - "open": 6330, - "volume": 365816 - }, - "id": 2661714, - "non_hive": { - "close": 1495, - "high": 55, - "low": 4, - "open": 1487, - "volume": 86037 - }, - "open": "2020-07-11T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12532, - "high": 59, - "low": 542808, - "open": 5407, - "volume": 1394792 - }, - "id": 2661746, - "non_hive": { - "close": 2945, - "high": 14, - "low": 125389, - "open": 1273, - "volume": 323621 - }, - "open": "2020-07-11T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1276, - "high": 1452, - "low": 621, - "open": 6392, - "volume": 1221375 - }, - "id": 2661796, - "non_hive": { - "close": 300, - "high": 342, - "low": 145, - "open": 1501, - "volume": 287111 - }, - "open": "2020-07-11T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1042, - "high": 403, - "low": 24616, - "open": 6219, - "volume": 1513865 - }, - "id": 2661818, - "non_hive": { - "close": 245, - "high": 95, - "low": 5739, - "open": 1463, - "volume": 355374 - }, - "open": "2020-07-11T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3108, - "high": 3846, - "low": 672, - "open": 6430, - "volume": 115624 - }, - "id": 2661845, - "non_hive": { - "close": 726, - "high": 905, - "low": 156, - "open": 1512, - "volume": 27124 - }, - "open": "2020-07-11T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590406, - "high": 977, - "low": 142808, - "open": 32183, - "volume": 5842369 - }, - "id": 2661876, - "non_hive": { - "close": 137001, - "high": 231, - "low": 33131, - "open": 7517, - "volume": 1362849 - }, - "open": "2020-07-11T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8475, - "high": 792, - "low": 516740, - "open": 6437, - "volume": 2137088 - }, - "id": 2661907, - "non_hive": { - "close": 1999, - "high": 187, - "low": 119937, - "open": 1517, - "volume": 497079 - }, - "open": "2020-07-11T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 465338, - "high": 10374, - "low": 513946, - "open": 3484, - "volume": 3943423 - }, - "id": 2661944, - "non_hive": { - "close": 108427, - "high": 2447, - "low": 119749, - "open": 821, - "volume": 919021 - }, - "open": "2020-07-11T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 995, - "high": 51, - "low": 1817, - "open": 51, - "volume": 3963508 - }, - "id": 2661978, - "non_hive": { - "close": 233, - "high": 12, - "low": 423, - "open": 12, - "volume": 924167 - }, - "open": "2020-07-11T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 172968, - "high": 20062, - "low": 31, - "open": 68, - "volume": 5930816 - }, - "id": 2662039, - "non_hive": { - "close": 39828, - "high": 4869, - "low": 7, - "open": 16, - "volume": 1376957 - }, - "open": "2020-07-11T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 286, - "high": 1449, - "low": 12, - "open": 35000, - "volume": 1489290 - }, - "id": 2662081, - "non_hive": { - "close": 65, - "high": 339, - "low": 2, - "open": 8059, - "volume": 343172 - }, - "open": "2020-07-11T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 697, - "high": 815, - "low": 23, - "open": 8694, - "volume": 691726 - }, - "id": 2662136, - "non_hive": { - "close": 162, - "high": 190, - "low": 5, - "open": 2000, - "volume": 159254 - }, - "open": "2020-07-11T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9458, - "high": 202, - "low": 18, - "open": 8603, - "volume": 1781989 - }, - "id": 2662177, - "non_hive": { - "close": 2185, - "high": 47, - "low": 4, - "open": 1999, - "volume": 411210 - }, - "open": "2020-07-11T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100000, - "high": 51, - "low": 46, - "open": 7122, - "volume": 2862289 - }, - "id": 2662214, - "non_hive": { - "close": 23100, - "high": 12, - "low": 10, - "open": 1645, - "volume": 659440 - }, - "open": "2020-07-11T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25297, - "high": 4980, - "low": 800112, - "open": 4569, - "volume": 4510832 - }, - "id": 2662264, - "non_hive": { - "close": 6000, - "high": 1213, - "low": 184810, - "open": 1060, - "volume": 1050263 - }, - "open": "2020-07-11T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 152356, - "high": 59, - "low": 57448, - "open": 923, - "volume": 317012 - }, - "id": 2662311, - "non_hive": { - "close": 35292, - "high": 14, - "low": 13213, - "open": 217, - "volume": 73588 - }, - "open": "2020-07-11T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27, - "high": 195, - "low": 27, - "open": 3865, - "volume": 468870 - }, - "id": 2662339, - "non_hive": { - "close": 6, - "high": 46, - "low": 6, - "open": 908, - "volume": 110063 - }, - "open": "2020-07-11T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 251, - "high": 63417, - "low": 246, - "open": 7629, - "volume": 217309 - }, - "id": 2662363, - "non_hive": { - "close": 58, - "high": 15197, - "low": 56, - "open": 1793, - "volume": 51419 - }, - "open": "2020-07-11T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 379, - "high": 379, - "low": 462644, - "open": 270, - "volume": 621697 - }, - "id": 2662402, - "non_hive": { - "close": 90, - "high": 90, - "low": 107796, - "open": 64, - "volume": 144995 - }, - "open": "2020-07-12T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10756, - "high": 7863, - "low": 6253, - "open": 6253, - "volume": 58684 - }, - "id": 2662426, - "non_hive": { - "close": 2581, - "high": 1887, - "low": 1481, - "open": 1481, - "volume": 13960 - }, - "open": "2020-07-12T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 906, - "high": 892, - "low": 159658, - "open": 892, - "volume": 660380 - }, - "id": 2662444, - "non_hive": { - "close": 217, - "high": 214, - "low": 36752, - "open": 214, - "volume": 153783 - }, - "open": "2020-07-12T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56093, - "high": 37, - "low": 591346, - "open": 94, - "volume": 731930 - }, - "id": 2662467, - "non_hive": { - "close": 13413, - "high": 9, - "low": 136634, - "open": 22, - "volume": 170182 - }, - "open": "2020-07-12T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13643, - "high": 125, - "low": 6, - "open": 125, - "volume": 36026 - }, - "id": 2662490, - "non_hive": { - "close": 3195, - "high": 30, - "low": 1, - "open": 30, - "volume": 8435 - }, - "open": "2020-07-12T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12838, - "high": 15000, - "low": 8667, - "open": 8646, - "volume": 2537975 - }, - "id": 2662510, - "non_hive": { - "close": 3000, - "high": 3513, - "low": 2000, - "open": 2000, - "volume": 587574 - }, - "open": "2020-07-12T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8180, - "high": 4932, - "low": 546473, - "open": 8649, - "volume": 3644922 - }, - "id": 2662542, - "non_hive": { - "close": 1891, - "high": 1152, - "low": 125797, - "open": 2000, - "volume": 840479 - }, - "open": "2020-07-12T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 872, - "high": 12, - "low": 1053, - "open": 468, - "volume": 4109392 - }, - "id": 2662573, - "non_hive": { - "close": 204, - "high": 3, - "low": 242, - "open": 108, - "volume": 947536 - }, - "open": "2020-07-12T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2968, - "high": 3059, - "low": 345351, - "open": 885, - "volume": 3111822 - }, - "id": 2662622, - "non_hive": { - "close": 691, - "high": 716, - "low": 79086, - "open": 204, - "volume": 716126 - }, - "open": "2020-07-12T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 188962, - "high": 288, - "low": 5, - "open": 8605, - "volume": 8035206 - }, - "id": 2662674, - "non_hive": { - "close": 43177, - "high": 67, - "low": 1, - "open": 1998, - "volume": 1851372 - }, - "open": "2020-07-12T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 291, - "high": 146, - "low": 282, - "open": 8747, - "volume": 4022402 - }, - "id": 2662745, - "non_hive": { - "close": 67, - "high": 34, - "low": 64, - "open": 2000, - "volume": 920976 - }, - "open": "2020-07-12T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8142, - "high": 2512, - "low": 3564, - "open": 290, - "volume": 5556741 - }, - "id": 2662784, - "non_hive": { - "close": 1887, - "high": 583, - "low": 819, - "open": 67, - "volume": 1281308 - }, - "open": "2020-07-12T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 124732, - "high": 5070, - "low": 1000, - "open": 8198, - "volume": 2675015 - }, - "id": 2662845, - "non_hive": { - "close": 28905, - "high": 1175, - "low": 230, - "open": 1887, - "volume": 615945 - }, - "open": "2020-07-12T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1660, - "high": 17, - "low": 152556, - "open": 8678, - "volume": 3128494 - }, - "id": 2662893, - "non_hive": { - "close": 385, - "high": 4, - "low": 35087, - "open": 2000, - "volume": 720614 - }, - "open": "2020-07-12T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5504, - "high": 802, - "low": 546698, - "open": 17041, - "volume": 3720403 - }, - "id": 2662938, - "non_hive": { - "close": 1265, - "high": 186, - "low": 124201, - "open": 3951, - "volume": 848666 - }, - "open": "2020-07-12T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2056, - "high": 2056, - "low": 14, - "open": 8788, - "volume": 4123526 - }, - "id": 2662989, - "non_hive": { - "close": 473, - "high": 473, - "low": 3, - "open": 2000, - "volume": 940446 - }, - "open": "2020-07-12T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 73, - "high": 86, - "low": 16, - "open": 6220, - "volume": 4157075 - }, - "id": 2663034, - "non_hive": { - "close": 16, - "high": 20, - "low": 3, - "open": 1430, - "volume": 947475 - }, - "open": "2020-07-12T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 61, - "high": 47, - "low": 47, - "open": 7444, - "volume": 2574478 - }, - "id": 2663097, - "non_hive": { - "close": 14, - "high": 11, - "low": 10, - "open": 1706, - "volume": 582313 - }, - "open": "2020-07-12T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4743, - "high": 11593, - "low": 15, - "open": 227, - "volume": 1507871 - }, - "id": 2663144, - "non_hive": { - "close": 1081, - "high": 2664, - "low": 3, - "open": 52, - "volume": 341547 - }, - "open": "2020-07-12T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 532094, - "high": 191, - "low": 92, - "open": 2000, - "volume": 7079340 - }, - "id": 2663194, - "non_hive": { - "close": 119604, - "high": 44, - "low": 20, - "open": 459, - "volume": 1601731 - }, - "open": "2020-07-12T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7329, - "high": 8731, - "low": 35, - "open": 8817, - "volume": 2649701 - }, - "id": 2663239, - "non_hive": { - "close": 1667, - "high": 1988, - "low": 7, - "open": 1999, - "volume": 596699 - }, - "open": "2020-07-12T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 269, - "high": 962, - "low": 10, - "open": 8869, - "volume": 1310880 - }, - "id": 2663286, - "non_hive": { - "close": 60, - "high": 220, - "low": 2, - "open": 2000, - "volume": 295236 - }, - "open": "2020-07-12T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7245, - "high": 4474, - "low": 34, - "open": 4474, - "volume": 573123 - }, - "id": 2663319, - "non_hive": { - "close": 1644, - "high": 1018, - "low": 7, - "open": 1018, - "volume": 128536 - }, - "open": "2020-07-12T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 330, - "high": 143491, - "low": 14, - "open": 847, - "volume": 201640 - }, - "id": 2663344, - "non_hive": { - "close": 74, - "high": 32885, - "low": 3, - "open": 192, - "volume": 46149 - }, - "open": "2020-07-12T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 306, - "high": 192, - "low": 7, - "open": 330, - "volume": 71556 - }, - "id": 2663365, - "non_hive": { - "close": 69, - "high": 44, - "low": 1, - "open": 75, - "volume": 16374 - }, - "open": "2020-07-13T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 58, - "high": 493908, - "low": 58, - "open": 5343, - "volume": 2426417 - }, - "id": 2663399, - "non_hive": { - "close": 13, - "high": 112910, - "low": 13, - "open": 1220, - "volume": 548218 - }, - "open": "2020-07-13T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11545, - "high": 63669, - "low": 21, - "open": 18804, - "volume": 2849452 - }, - "id": 2663428, - "non_hive": { - "close": 2620, - "high": 14483, - "low": 4, - "open": 4276, - "volume": 641834 - }, - "open": "2020-07-13T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8830, - "high": 1472, - "low": 5879, - "open": 6997, - "volume": 1817299 - }, - "id": 2663470, - "non_hive": { - "close": 1999, - "high": 334, - "low": 1317, - "open": 1587, - "volume": 407180 - }, - "open": "2020-07-13T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31549, - "high": 309, - "low": 31549, - "open": 11744, - "volume": 810318 - }, - "id": 2663488, - "non_hive": { - "close": 7067, - "high": 70, - "low": 7067, - "open": 2660, - "volume": 183376 - }, - "open": "2020-07-13T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30940, - "high": 99003, - "low": 13497, - "open": 8836, - "volume": 261068 - }, - "id": 2663502, - "non_hive": { - "close": 7000, - "high": 22410, - "low": 3023, - "open": 1999, - "volume": 58951 - }, - "open": "2020-07-13T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 368, - "high": 13000, - "low": 15530, - "open": 2898, - "volume": 88317 - }, - "id": 2663541, - "non_hive": { - "close": 84, - "high": 2995, - "low": 3510, - "open": 655, - "volume": 20246 - }, - "open": "2020-07-13T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 350987, - "high": 43, - "low": 11, - "open": 26136, - "volume": 644705 - }, - "id": 2663560, - "non_hive": { - "close": 78626, - "high": 10, - "low": 2, - "open": 5950, - "volume": 144593 - }, - "open": "2020-07-13T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 82, - "high": 658, - "low": 8, - "open": 2982, - "volume": 378325 - }, - "id": 2663581, - "non_hive": { - "close": 18, - "high": 150, - "low": 1, - "open": 668, - "volume": 85589 - }, - "open": "2020-07-13T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7901, - "high": 26, - "low": 27, - "open": 322, - "volume": 1879516 - }, - "id": 2663626, - "non_hive": { - "close": 1796, - "high": 6, - "low": 6, - "open": 73, - "volume": 423132 - }, - "open": "2020-07-13T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5389, - "high": 446, - "low": 24, - "open": 3694, - "volume": 4969346 - }, - "id": 2663660, - "non_hive": { - "close": 1230, - "high": 102, - "low": 5, - "open": 840, - "volume": 1126254 - }, - "open": "2020-07-13T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9566, - "high": 4, - "low": 12, - "open": 5390, - "volume": 5353754 - }, - "id": 2663706, - "non_hive": { - "close": 2184, - "high": 1, - "low": 2, - "open": 1230, - "volume": 1211353 - }, - "open": "2020-07-13T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9218, - "high": 17, - "low": 56, - "open": 188, - "volume": 922603 - }, - "id": 2663768, - "non_hive": { - "close": 2100, - "high": 4, - "low": 12, - "open": 43, - "volume": 208212 - }, - "open": "2020-07-13T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 63, - "high": 43, - "low": 63, - "open": 43, - "volume": 1283395 - }, - "id": 2663811, - "non_hive": { - "close": 14, - "high": 10, - "low": 14, - "open": 10, - "volume": 289580 - }, - "open": "2020-07-13T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5431, - "high": 359, - "low": 319503, - "open": 3490, - "volume": 1941619 - }, - "id": 2663852, - "non_hive": { - "close": 1239, - "high": 82, - "low": 71857, - "open": 797, - "volume": 438707 - }, - "open": "2020-07-13T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 311, - "low": 5, - "open": 5460, - "volume": 849658 - }, - "id": 2663886, - "non_hive": { - "close": 1, - "high": 71, - "low": 1, - "open": 1245, - "volume": 191582 - }, - "open": "2020-07-13T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 659, - "high": 659, - "low": 2675, - "open": 34001, - "volume": 105886 - }, - "id": 2663922, - "non_hive": { - "close": 151, - "high": 151, - "low": 606, - "open": 7738, - "volume": 24074 - }, - "open": "2020-07-13T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42680, - "high": 104, - "low": 34, - "open": 27216, - "volume": 1082903 - }, - "id": 2663948, - "non_hive": { - "close": 9771, - "high": 24, - "low": 7, - "open": 6232, - "volume": 247906 - }, - "open": "2020-07-13T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 578, - "high": 148, - "low": 591230, - "open": 148, - "volume": 766386 - }, - "id": 2663992, - "non_hive": { - "close": 132, - "high": 34, - "low": 134800, - "open": 34, - "volume": 174896 - }, - "open": "2020-07-13T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 67, - "high": 3012, - "low": 67, - "open": 49480, - "volume": 2533287 - }, - "id": 2664024, - "non_hive": { - "close": 15, - "high": 692, - "low": 15, - "open": 11331, - "volume": 572156 - }, - "open": "2020-07-13T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9699, - "high": 4803, - "low": 49848, - "open": 8886, - "volume": 842630 - }, - "id": 2664061, - "non_hive": { - "close": 2181, - "high": 1099, - "low": 11196, - "open": 2000, - "volume": 189624 - }, - "open": "2020-07-13T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 85804, - "high": 325, - "low": 14382, - "open": 8788, - "volume": 920916 - }, - "id": 2664082, - "non_hive": { - "close": 19302, - "high": 74, - "low": 3197, - "open": 1973, - "volume": 206892 - }, - "open": "2020-07-13T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7910, - "high": 6573, - "low": 27, - "open": 399048, - "volume": 2107474 - }, - "id": 2664130, - "non_hive": { - "close": 1798, - "high": 1500, - "low": 6, - "open": 89745, - "volume": 474210 - }, - "open": "2020-07-13T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 145882, - "high": 4, - "low": 14, - "open": 18762, - "volume": 4748332 - }, - "id": 2664164, - "non_hive": { - "close": 33207, - "high": 1, - "low": 3, - "open": 4279, - "volume": 1072089 - }, - "open": "2020-07-13T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7549, - "high": 1600, - "low": 451, - "open": 8789, - "volume": 399661 - }, - "id": 2664230, - "non_hive": { - "close": 1714, - "high": 364, - "low": 99, - "open": 1999, - "volume": 89061 - }, - "open": "2020-07-14T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2855, - "high": 9990, - "low": 82, - "open": 1370, - "volume": 184711 - }, - "id": 2664256, - "non_hive": { - "close": 645, - "high": 2268, - "low": 18, - "open": 311, - "volume": 41771 - }, - "open": "2020-07-14T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4231, - "high": 22, - "low": 148, - "open": 240000, - "volume": 943692 - }, - "id": 2664273, - "non_hive": { - "close": 931, - "high": 5, - "low": 32, - "open": 54186, - "volume": 208650 - }, - "open": "2020-07-14T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30645, - "high": 917, - "low": 30645, - "open": 917, - "volume": 112392 - }, - "id": 2664296, - "non_hive": { - "close": 6910, - "high": 207, - "low": 6910, - "open": 207, - "volume": 25354 - }, - "open": "2020-07-14T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5704, - "high": 9707, - "low": 2368, - "open": 216, - "volume": 2554422 - }, - "id": 2664319, - "non_hive": { - "close": 1260, - "high": 2187, - "low": 513, - "open": 48, - "volume": 556192 - }, - "open": "2020-07-14T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 348951, - "high": 36, - "low": 9221, - "open": 5150, - "volume": 1455395 - }, - "id": 2664350, - "non_hive": { - "close": 75792, - "high": 8, - "low": 2000, - "open": 1137, - "volume": 316732 - }, - "open": "2020-07-14T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1929, - "high": 1146, - "low": 502504, - "open": 3003, - "volume": 1244652 - }, - "id": 2664398, - "non_hive": { - "close": 424, - "high": 252, - "low": 108942, - "open": 660, - "volume": 270220 - }, - "open": "2020-07-14T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3189, - "high": 723, - "low": 63359, - "open": 13897, - "volume": 2598028 - }, - "id": 2664427, - "non_hive": { - "close": 701, - "high": 159, - "low": 13685, - "open": 3054, - "volume": 562613 - }, - "open": "2020-07-14T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6368, - "high": 778, - "low": 124763, - "open": 263, - "volume": 3622502 - }, - "id": 2664464, - "non_hive": { - "close": 1398, - "high": 171, - "low": 26949, - "open": 57, - "volume": 782598 - }, - "open": "2020-07-14T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590481, - "high": 447, - "low": 394862, - "open": 6793, - "volume": 3042639 - }, - "id": 2664493, - "non_hive": { - "close": 126957, - "high": 98, - "low": 84896, - "open": 1487, - "volume": 654492 - }, - "open": "2020-07-14T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12907, - "high": 1372, - "low": 31, - "open": 2121, - "volume": 955618 - }, - "id": 2664531, - "non_hive": { - "close": 2811, - "high": 299, - "low": 6, - "open": 458, - "volume": 206176 - }, - "open": "2020-07-14T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20172, - "high": 41, - "low": 20172, - "open": 1321, - "volume": 1267393 - }, - "id": 2664589, - "non_hive": { - "close": 4296, - "high": 9, - "low": 4296, - "open": 287, - "volume": 273932 - }, - "open": "2020-07-14T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18991, - "high": 8592, - "low": 128, - "open": 1316, - "volume": 1137407 - }, - "id": 2664617, - "non_hive": { - "close": 4168, - "high": 1886, - "low": 27, - "open": 285, - "volume": 247409 - }, - "open": "2020-07-14T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4712, - "high": 154, - "low": 15737, - "open": 2118, - "volume": 65034 - }, - "id": 2664650, - "non_hive": { - "close": 1034, - "high": 34, - "low": 3453, - "open": 465, - "volume": 14272 - }, - "open": "2020-07-14T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1319, - "high": 59, - "low": 107567, - "open": 59, - "volume": 2043134 - }, - "id": 2664673, - "non_hive": { - "close": 289, - "high": 13, - "low": 23150, - "open": 13, - "volume": 444068 - }, - "open": "2020-07-14T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12865, - "high": 296, - "low": 9, - "open": 9114, - "volume": 986940 - }, - "id": 2664717, - "non_hive": { - "close": 2823, - "high": 65, - "low": 1, - "open": 1999, - "volume": 216000 - }, - "open": "2020-07-14T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7994, - "high": 82, - "low": 321422, - "open": 82, - "volume": 731861 - }, - "id": 2664760, - "non_hive": { - "close": 1754, - "high": 18, - "low": 69227, - "open": 18, - "volume": 158331 - }, - "open": "2020-07-14T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1864, - "high": 232, - "low": 28375, - "open": 898, - "volume": 700956 - }, - "id": 2664798, - "non_hive": { - "close": 409, - "high": 51, - "low": 6134, - "open": 197, - "volume": 151883 - }, - "open": "2020-07-14T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 209, - "high": 168, - "low": 13468, - "open": 11485, - "volume": 877535 - }, - "id": 2664833, - "non_hive": { - "close": 46, - "high": 37, - "low": 2923, - "open": 2520, - "volume": 191072 - }, - "open": "2020-07-14T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4556, - "high": 9, - "low": 120, - "open": 9115, - "volume": 1014226 - }, - "id": 2664874, - "non_hive": { - "close": 1000, - "high": 2, - "low": 26, - "open": 1999, - "volume": 221826 - }, - "open": "2020-07-14T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 373, - "high": 373, - "low": 9304, - "open": 12722, - "volume": 102360 - }, - "id": 2664912, - "non_hive": { - "close": 82, - "high": 82, - "low": 2041, - "open": 2792, - "volume": 22464 - }, - "open": "2020-07-14T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40097, - "high": 428, - "low": 22, - "open": 428, - "volume": 2507419 - }, - "id": 2664932, - "non_hive": { - "close": 8794, - "high": 94, - "low": 4, - "open": 94, - "volume": 544844 - }, - "open": "2020-07-14T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6266, - "high": 36, - "low": 28, - "open": 160, - "volume": 499962 - }, - "id": 2664969, - "non_hive": { - "close": 1373, - "high": 8, - "low": 6, - "open": 35, - "volume": 109550 - }, - "open": "2020-07-14T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90713, - "high": 55928, - "low": 22, - "open": 1602, - "volume": 1706141 - }, - "id": 2665002, - "non_hive": { - "close": 19900, - "high": 12274, - "low": 4, - "open": 351, - "volume": 372561 - }, - "open": "2020-07-14T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 257, - "high": 565, - "low": 257, - "open": 1317, - "volume": 366317 - }, - "id": 2665047, - "non_hive": { - "close": 55, - "high": 124, - "low": 55, - "open": 289, - "volume": 80303 - }, - "open": "2020-07-15T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15898, - "high": 54, - "low": 31, - "open": 12833, - "volume": 330107 - }, - "id": 2665076, - "non_hive": { - "close": 3488, - "high": 12, - "low": 6, - "open": 2814, - "volume": 72390 - }, - "open": "2020-07-15T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 592, - "high": 592, - "low": 399, - "open": 1595, - "volume": 633745 - }, - "id": 2665106, - "non_hive": { - "close": 130, - "high": 130, - "low": 87, - "open": 350, - "volume": 138274 - }, - "open": "2020-07-15T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 319, - "high": 557, - "low": 392438, - "open": 11322, - "volume": 10623951 - }, - "id": 2665127, - "non_hive": { - "close": 70, - "high": 123, - "low": 85935, - "open": 2484, - "volume": 2328884 - }, - "open": "2020-07-15T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1126, - "high": 1126, - "low": 590124, - "open": 122, - "volume": 4032737 - }, - "id": 2665156, - "non_hive": { - "close": 257, - "high": 257, - "low": 129289, - "open": 27, - "volume": 887228 - }, - "open": "2020-07-15T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8355, - "high": 4, - "low": 590873, - "open": 9125, - "volume": 1288116 - }, - "id": 2665191, - "non_hive": { - "close": 1846, - "high": 1, - "low": 129453, - "open": 2000, - "volume": 282349 - }, - "open": "2020-07-15T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 140026, - "high": 1198, - "low": 40362, - "open": 1705, - "volume": 1247362 - }, - "id": 2665222, - "non_hive": { - "close": 30751, - "high": 265, - "low": 8863, - "open": 377, - "volume": 274811 - }, - "open": "2020-07-15T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2017, - "high": 2017, - "low": 1890, - "open": 9106, - "volume": 636189 - }, - "id": 2665249, - "non_hive": { - "close": 446, - "high": 446, - "low": 415, - "open": 2000, - "volume": 139748 - }, - "open": "2020-07-15T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 84822, - "high": 158, - "low": 119, - "open": 104, - "volume": 183966 - }, - "id": 2665271, - "non_hive": { - "close": 18750, - "high": 35, - "low": 26, - "open": 23, - "volume": 40663 - }, - "open": "2020-07-15T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3875, - "high": 3875, - "low": 443398, - "open": 10160, - "volume": 2580907 - }, - "id": 2665308, - "non_hive": { - "close": 857, - "high": 857, - "low": 96700, - "open": 2246, - "volume": 564491 - }, - "open": "2020-07-15T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6050, - "high": 194, - "low": 1709, - "open": 5164, - "volume": 210511 - }, - "id": 2665347, - "non_hive": { - "close": 1338, - "high": 43, - "low": 377, - "open": 1142, - "volume": 46555 - }, - "open": "2020-07-15T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 615, - "high": 203, - "low": 580535, - "open": 11979, - "volume": 1397182 - }, - "id": 2665379, - "non_hive": { - "close": 136, - "high": 45, - "low": 126608, - "open": 2649, - "volume": 305667 - }, - "open": "2020-07-15T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11684, - "high": 11684, - "low": 89424, - "open": 9169, - "volume": 806326 - }, - "id": 2665411, - "non_hive": { - "close": 2584, - "high": 2584, - "low": 19502, - "open": 2000, - "volume": 176457 - }, - "open": "2020-07-15T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72163, - "high": 58, - "low": 223, - "open": 58, - "volume": 710494 - }, - "id": 2665437, - "non_hive": { - "close": 15958, - "high": 13, - "low": 49, - "open": 13, - "volume": 156332 - }, - "open": "2020-07-15T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47155, - "high": 27, - "low": 100, - "open": 100, - "volume": 127776 - }, - "id": 2665462, - "non_hive": { - "close": 10427, - "high": 6, - "low": 22, - "open": 22, - "volume": 28217 - }, - "open": "2020-07-15T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32, - "high": 673, - "low": 28, - "open": 4443, - "volume": 1941026 - }, - "id": 2665486, - "non_hive": { - "close": 7, - "high": 149, - "low": 6, - "open": 982, - "volume": 425333 - }, - "open": "2020-07-15T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4569, - "high": 94, - "low": 415356, - "open": 7748, - "volume": 702084 - }, - "id": 2665523, - "non_hive": { - "close": 1010, - "high": 21, - "low": 90158, - "open": 1713, - "volume": 153062 - }, - "open": "2020-07-15T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60226, - "high": 58, - "low": 16785, - "open": 17478, - "volume": 4030306 - }, - "id": 2665555, - "non_hive": { - "close": 13215, - "high": 13, - "low": 3642, - "open": 3863, - "volume": 877562 - }, - "open": "2020-07-15T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 124, - "high": 74068, - "low": 124, - "open": 9100, - "volume": 1339199 - }, - "id": 2665609, - "non_hive": { - "close": 26, - "high": 16364, - "low": 26, - "open": 2000, - "volume": 291111 - }, - "open": "2020-07-15T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 114, - "high": 114, - "low": 6637, - "open": 9097, - "volume": 1275245 - }, - "id": 2665640, - "non_hive": { - "close": 25, - "high": 25, - "low": 1434, - "open": 1974, - "volume": 275839 - }, - "open": "2020-07-15T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 94309, - "high": 1139, - "low": 122618, - "open": 9040, - "volume": 1104729 - }, - "id": 2665661, - "non_hive": { - "close": 20531, - "high": 249, - "low": 26397, - "open": 1974, - "volume": 239712 - }, - "open": "2020-07-15T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2899, - "high": 41, - "low": 426435, - "open": 7576, - "volume": 702740 - }, - "id": 2665685, - "non_hive": { - "close": 631, - "high": 9, - "low": 92814, - "open": 1649, - "volume": 152954 - }, - "open": "2020-07-15T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2461, - "high": 95741, - "low": 28, - "open": 307609, - "volume": 1839010 - }, - "id": 2665728, - "non_hive": { - "close": 544, - "high": 21178, - "low": 6, - "open": 66951, - "volume": 400900 - }, - "open": "2020-07-15T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 138, - "high": 138, - "low": 14, - "open": 36725, - "volume": 793204 - }, - "id": 2665783, - "non_hive": { - "close": 32, - "high": 32, - "low": 3, - "open": 8153, - "volume": 179355 - }, - "open": "2020-07-15T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 982, - "high": 3012, - "low": 34, - "open": 10000, - "volume": 2342545 - }, - "id": 2665810, - "non_hive": { - "close": 225, - "high": 696, - "low": 7, - "open": 2310, - "volume": 514897 - }, - "open": "2020-07-16T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 370428, - "high": 3619, - "low": 370428, - "open": 8742, - "volume": 612486 - }, - "id": 2665840, - "non_hive": { - "close": 80036, - "high": 828, - "low": 80036, - "open": 1999, - "volume": 132544 - }, - "open": "2020-07-16T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15802, - "high": 10885, - "low": 5, - "open": 4657, - "volume": 679895 - }, - "id": 2665851, - "non_hive": { - "close": 3512, - "high": 2484, - "low": 1, - "open": 1062, - "volume": 147659 - }, - "open": "2020-07-16T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72378, - "high": 193, - "low": 72378, - "open": 1709, - "volume": 1377490 - }, - "id": 2665876, - "non_hive": { - "close": 15705, - "high": 43, - "low": 15705, - "open": 380, - "volume": 299754 - }, - "open": "2020-07-16T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 523, - "high": 108, - "low": 198, - "open": 9029, - "volume": 61384 - }, - "id": 2665906, - "non_hive": { - "close": 116, - "high": 24, - "low": 43, - "open": 1999, - "volume": 13556 - }, - "open": "2020-07-16T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1488, - "high": 9651, - "low": 296, - "open": 1128, - "volume": 919719 - }, - "id": 2665929, - "non_hive": { - "close": 329, - "high": 2228, - "low": 64, - "open": 250, - "volume": 201674 - }, - "open": "2020-07-16T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1402, - "high": 624, - "low": 108054, - "open": 9045, - "volume": 926621 - }, - "id": 2665971, - "non_hive": { - "close": 310, - "high": 138, - "low": 23351, - "open": 1999, - "volume": 202428 - }, - "open": "2020-07-16T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1085, - "high": 4, - "low": 476323, - "open": 9252, - "volume": 1947985 - }, - "id": 2665996, - "non_hive": { - "close": 233, - "high": 1, - "low": 100599, - "open": 2000, - "volume": 414849 - }, - "open": "2020-07-16T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 561560, - "high": 724, - "low": 561560, - "open": 19296, - "volume": 3686281 - }, - "id": 2666028, - "non_hive": { - "close": 118602, - "high": 160, - "low": 118602, - "open": 4148, - "volume": 780878 - }, - "open": "2020-07-16T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 79, - "high": 18, - "low": 79, - "open": 1639, - "volume": 4315362 - }, - "id": 2666072, - "non_hive": { - "close": 16, - "high": 4, - "low": 16, - "open": 362, - "volume": 906881 - }, - "open": "2020-07-16T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6930, - "high": 23, - "low": 129992, - "open": 30130, - "volume": 1388570 - }, - "id": 2666119, - "non_hive": { - "close": 1447, - "high": 5, - "low": 27027, - "open": 6434, - "volume": 289225 - }, - "open": "2020-07-16T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8257, - "high": 47, - "low": 17791, - "open": 6003, - "volume": 4752841 - }, - "id": 2666150, - "non_hive": { - "close": 1734, - "high": 10, - "low": 3699, - "open": 1260, - "volume": 996547 - }, - "open": "2020-07-16T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6690, - "high": 389, - "low": 311784, - "open": 1860, - "volume": 3057977 - }, - "id": 2666189, - "non_hive": { - "close": 1426, - "high": 83, - "low": 64851, - "open": 390, - "volume": 636612 - }, - "open": "2020-07-16T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21739, - "high": 63, - "low": 3731, - "open": 1501, - "volume": 2843429 - }, - "id": 2666237, - "non_hive": { - "close": 4763, - "high": 14, - "low": 776, - "open": 320, - "volume": 594877 - }, - "open": "2020-07-16T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9205, - "high": 91, - "low": 75, - "open": 5754, - "volume": 2850122 - }, - "id": 2666279, - "non_hive": { - "close": 1999, - "high": 20, - "low": 15, - "open": 1260, - "volume": 601202 - }, - "open": "2020-07-16T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33, - "high": 248, - "low": 15, - "open": 2310, - "volume": 5899346 - }, - "id": 2666331, - "non_hive": { - "close": 7, - "high": 54, - "low": 3, - "open": 501, - "volume": 1251216 - }, - "open": "2020-07-16T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 597600, - "high": 687, - "low": 34, - "open": 2749, - "volume": 3779582 - }, - "id": 2666393, - "non_hive": { - "close": 126701, - "high": 149, - "low": 7, - "open": 586, - "volume": 801480 - }, - "open": "2020-07-16T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7846, - "high": 92, - "low": 59, - "open": 9253, - "volume": 4261051 - }, - "id": 2666430, - "non_hive": { - "close": 1688, - "high": 20, - "low": 12, - "open": 1999, - "volume": 905509 - }, - "open": "2020-07-16T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 232, - "high": 227, - "low": 13, - "open": 42, - "volume": 5108967 - }, - "id": 2666490, - "non_hive": { - "close": 50, - "high": 49, - "low": 2, - "open": 9, - "volume": 1081277 - }, - "open": "2020-07-16T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 733, - "high": 55, - "low": 358, - "open": 9470, - "volume": 4088355 - }, - "id": 2666575, - "non_hive": { - "close": 157, - "high": 12, - "low": 75, - "open": 2000, - "volume": 864231 - }, - "open": "2020-07-16T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2380, - "high": 1481, - "low": 2380, - "open": 9443, - "volume": 4361756 - }, - "id": 2666621, - "non_hive": { - "close": 500, - "high": 314, - "low": 500, - "open": 2000, - "volume": 919323 - }, - "open": "2020-07-16T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1890, - "high": 1000, - "low": 328, - "open": 2361, - "volume": 3136355 - }, - "id": 2666666, - "non_hive": { - "close": 400, - "high": 212, - "low": 68, - "open": 500, - "volume": 658242 - }, - "open": "2020-07-16T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29, - "high": 79, - "low": 54, - "open": 2033, - "volume": 35670 - }, - "id": 2666732, - "non_hive": { - "close": 6, - "high": 17, - "low": 11, - "open": 435, - "volume": 7621 - }, - "open": "2020-07-16T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1759, - "high": 217, - "low": 5, - "open": 13524, - "volume": 1419374 - }, - "id": 2666755, - "non_hive": { - "close": 388, - "high": 48, - "low": 1, - "open": 2895, - "volume": 303912 - }, - "open": "2020-07-16T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1319, - "high": 644, - "low": 119, - "open": 47177, - "volume": 188680 - }, - "id": 2666793, - "non_hive": { - "close": 290, - "high": 142, - "low": 24, - "open": 10391, - "volume": 41179 - }, - "open": "2020-07-17T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45067, - "high": 16912, - "low": 87, - "open": 9103, - "volume": 671292 - }, - "id": 2666821, - "non_hive": { - "close": 9370, - "high": 3714, - "low": 18, - "open": 1999, - "volume": 140642 - }, - "open": "2020-07-17T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6945, - "high": 50, - "low": 464, - "open": 13698, - "volume": 96152 - }, - "id": 2666842, - "non_hive": { - "close": 1518, - "high": 11, - "low": 96, - "open": 3000, - "volume": 20736 - }, - "open": "2020-07-17T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25202, - "high": 9, - "low": 518630, - "open": 9156, - "volume": 1874840 - }, - "id": 2666867, - "non_hive": { - "close": 5481, - "high": 2, - "low": 107878, - "open": 1999, - "volume": 391173 - }, - "open": "2020-07-17T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 584337, - "high": 211, - "low": 23764, - "open": 441196, - "volume": 10401490 - }, - "id": 2666898, - "non_hive": { - "close": 122520, - "high": 46, - "low": 4806, - "open": 95950, - "volume": 2211585 - }, - "open": "2020-07-17T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 204522, - "high": 1171, - "low": 11, - "open": 6202, - "volume": 4927845 - }, - "id": 2666968, - "non_hive": { - "close": 43178, - "high": 254, - "low": 2, - "open": 1345, - "volume": 1044657 - }, - "open": "2020-07-17T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13452, - "high": 702, - "low": 28, - "open": 600000, - "volume": 5155329 - }, - "id": 2667012, - "non_hive": { - "close": 2889, - "high": 151, - "low": 5, - "open": 126910, - "volume": 1092000 - }, - "open": "2020-07-17T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4667, - "high": 9, - "low": 40, - "open": 8313, - "volume": 3686311 - }, - "id": 2667066, - "non_hive": { - "close": 1000, - "high": 2, - "low": 8, - "open": 1784, - "volume": 779324 - }, - "open": "2020-07-17T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590542, - "high": 4, - "low": 41, - "open": 6331, - "volume": 3457319 - }, - "id": 2667121, - "non_hive": { - "close": 124850, - "high": 1, - "low": 8, - "open": 1356, - "volume": 731973 - }, - "open": "2020-07-17T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 958, - "high": 280, - "low": 14, - "open": 33310, - "volume": 5405056 - }, - "id": 2667166, - "non_hive": { - "close": 200, - "high": 60, - "low": 2, - "open": 7057, - "volume": 1139200 - }, - "open": "2020-07-17T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 850, - "high": 4497, - "low": 139955, - "open": 956, - "volume": 1715783 - }, - "id": 2667241, - "non_hive": { - "close": 177, - "high": 941, - "low": 28157, - "open": 200, - "volume": 355028 - }, - "open": "2020-07-17T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3576, - "high": 3576, - "low": 159, - "open": 1119, - "volume": 643980 - }, - "id": 2667285, - "non_hive": { - "close": 750, - "high": 750, - "low": 33, - "open": 233, - "volume": 133981 - }, - "open": "2020-07-17T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3528, - "high": 3396, - "low": 3168, - "open": 3936, - "volume": 365695 - }, - "id": 2667318, - "non_hive": { - "close": 750, - "high": 727, - "low": 666, - "open": 828, - "volume": 77708 - }, - "open": "2020-07-17T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4705, - "high": 42, - "low": 23103, - "open": 9439, - "volume": 1526746 - }, - "id": 2667356, - "non_hive": { - "close": 993, - "high": 9, - "low": 4845, - "open": 2000, - "volume": 321452 - }, - "open": "2020-07-17T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9161, - "high": 52, - "low": 100, - "open": 341, - "volume": 618261 - }, - "id": 2667404, - "non_hive": { - "close": 1933, - "high": 11, - "low": 21, - "open": 72, - "volume": 130500 - }, - "open": "2020-07-17T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3540, - "high": 32, - "low": 32, - "open": 426501, - "volume": 3496750 - }, - "id": 2667461, - "non_hive": { - "close": 750, - "high": 7, - "low": 6, - "open": 90046, - "volume": 739367 - }, - "open": "2020-07-17T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20653, - "high": 392, - "low": 29, - "open": 29, - "volume": 1327228 - }, - "id": 2667523, - "non_hive": { - "close": 4405, - "high": 84, - "low": 6, - "open": 6, - "volume": 280531 - }, - "open": "2020-07-17T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 181, - "high": 88, - "low": 181, - "open": 9346, - "volume": 1487382 - }, - "id": 2667559, - "non_hive": { - "close": 38, - "high": 19, - "low": 38, - "open": 1999, - "volume": 315084 - }, - "open": "2020-07-17T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3540, - "high": 4, - "low": 13, - "open": 9439, - "volume": 1844131 - }, - "id": 2667621, - "non_hive": { - "close": 750, - "high": 1, - "low": 2, - "open": 2000, - "volume": 385088 - }, - "open": "2020-07-17T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 146, - "high": 6451, - "low": 44549, - "open": 3370, - "volume": 1703812 - }, - "id": 2667656, - "non_hive": { - "close": 30, - "high": 1378, - "low": 8954, - "open": 714, - "volume": 356779 - }, - "open": "2020-07-17T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1158, - "high": 37, - "low": 60, - "open": 9567, - "volume": 1494365 - }, - "id": 2667709, - "non_hive": { - "close": 247, - "high": 8, - "low": 12, - "open": 2000, - "volume": 317489 - }, - "open": "2020-07-17T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 309756, - "high": 32, - "low": 580479, - "open": 3492, - "volume": 1898552 - }, - "id": 2667750, - "non_hive": { - "close": 66494, - "high": 7, - "low": 124063, - "open": 750, - "volume": 407659 - }, - "open": "2020-07-17T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 308941, - "high": 1693, - "low": 114392, - "open": 8905, - "volume": 1830921 - }, - "id": 2667785, - "non_hive": { - "close": 65896, - "high": 365, - "low": 24250, - "open": 1919, - "volume": 390084 - }, - "open": "2020-07-17T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 712, - "high": 23, - "low": 15, - "open": 7731, - "volume": 813658 - }, - "id": 2667812, - "non_hive": { - "close": 152, - "high": 5, - "low": 3, - "open": 1660, - "volume": 172715 - }, - "open": "2020-07-17T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 630, - "high": 9, - "low": 3693, - "open": 8664, - "volume": 2458273 - }, - "id": 2667859, - "non_hive": { - "close": 134, - "high": 2, - "low": 779, - "open": 1847, - "volume": 519280 - }, - "open": "2020-07-18T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 413, - "high": 4004, - "low": 826, - "open": 1309, - "volume": 64888 - }, - "id": 2667898, - "non_hive": { - "close": 87, - "high": 851, - "low": 173, - "open": 278, - "volume": 13673 - }, - "open": "2020-07-18T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3564, - "high": 223, - "low": 5, - "open": 223, - "volume": 1209755 - }, - "id": 2667932, - "non_hive": { - "close": 750, - "high": 47, - "low": 1, - "open": 47, - "volume": 253385 - }, - "open": "2020-07-18T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9123, - "high": 180040, - "low": 20079, - "open": 29435, - "volume": 270861 - }, - "id": 2667954, - "non_hive": { - "close": 1930, - "high": 38102, - "low": 4225, - "open": 6195, - "volume": 57260 - }, - "open": "2020-07-18T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 747, - "high": 94, - "low": 178248, - "open": 132, - "volume": 712979 - }, - "id": 2667972, - "non_hive": { - "close": 157, - "high": 20, - "low": 37141, - "open": 28, - "volume": 149077 - }, - "open": "2020-07-18T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47284, - "high": 837, - "low": 47284, - "open": 60851, - "volume": 160983 - }, - "id": 2668006, - "non_hive": { - "close": 9990, - "high": 177, - "low": 9990, - "open": 12862, - "volume": 34021 - }, - "open": "2020-07-18T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7502, - "high": 322, - "low": 2180, - "open": 322, - "volume": 25714 - }, - "id": 2668024, - "non_hive": { - "close": 1583, - "high": 68, - "low": 460, - "open": 68, - "volume": 5427 - }, - "open": "2020-07-18T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3294, - "high": 7109, - "low": 572320, - "open": 7109, - "volume": 745311 - }, - "id": 2668040, - "non_hive": { - "close": 691, - "high": 1500, - "low": 119061, - "open": 1500, - "volume": 155483 - }, - "open": "2020-07-18T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3004, - "high": 7618, - "low": 2270, - "open": 1207, - "volume": 3053548 - }, - "id": 2668061, - "non_hive": { - "close": 628, - "high": 1598, - "low": 472, - "open": 253, - "volume": 635299 - }, - "open": "2020-07-18T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34215, - "high": 1831, - "low": 625, - "open": 584, - "volume": 171005 - }, - "id": 2668099, - "non_hive": { - "close": 7192, - "high": 385, - "low": 130, - "open": 122, - "volume": 35942 - }, - "open": "2020-07-18T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9525, - "high": 2135, - "low": 10000, - "open": 4635, - "volume": 2319229 - }, - "id": 2668122, - "non_hive": { - "close": 1999, - "high": 449, - "low": 2090, - "open": 974, - "volume": 485369 - }, - "open": "2020-07-18T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 223480, - "high": 128, - "low": 223480, - "open": 128, - "volume": 3580796 - }, - "id": 2668163, - "non_hive": { - "close": 45988, - "high": 27, - "low": 45988, - "open": 27, - "volume": 751878 - }, - "open": "2020-07-18T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 543, - "high": 543, - "low": 217, - "open": 17704, - "volume": 410586 - }, - "id": 2668189, - "non_hive": { - "close": 114, - "high": 114, - "low": 44, - "open": 3658, - "volume": 85772 - }, - "open": "2020-07-18T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2143, - "high": 1713, - "low": 38, - "open": 5000, - "volume": 252124 - }, - "id": 2668212, - "non_hive": { - "close": 449, - "high": 359, - "low": 7, - "open": 1035, - "volume": 52717 - }, - "open": "2020-07-18T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4510, - "high": 9, - "low": 184, - "open": 6226, - "volume": 483376 - }, - "id": 2668253, - "non_hive": { - "close": 949, - "high": 2, - "low": 38, - "open": 1304, - "volume": 101234 - }, - "open": "2020-07-18T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15, - "high": 604, - "low": 15, - "open": 760, - "volume": 3402634 - }, - "id": 2668298, - "non_hive": { - "close": 3, - "high": 128, - "low": 3, - "open": 160, - "volume": 716456 - }, - "open": "2020-07-18T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 92, - "high": 11340, - "low": 16, - "open": 29, - "volume": 2580952 - }, - "id": 2668332, - "non_hive": { - "close": 19, - "high": 2432, - "low": 3, - "open": 6, - "volume": 545107 - }, - "open": "2020-07-18T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590497, - "high": 3402, - "low": 137724, - "open": 2153, - "volume": 3618664 - }, - "id": 2668402, - "non_hive": { - "close": 124260, - "high": 727, - "low": 28981, - "open": 460, - "volume": 762851 - }, - "open": "2020-07-18T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5740, - "high": 7520, - "low": 590497, - "open": 5552, - "volume": 2023199 - }, - "id": 2668427, - "non_hive": { - "close": 1216, - "high": 1604, - "low": 124260, - "open": 1174, - "volume": 426725 - }, - "open": "2020-07-18T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 262, - "high": 248, - "low": 262, - "open": 3697, - "volume": 4535966 - }, - "id": 2668486, - "non_hive": { - "close": 55, - "high": 53, - "low": 55, - "open": 783, - "volume": 958761 - }, - "open": "2020-07-18T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 280757, - "high": 380, - "low": 5000, - "open": 9216, - "volume": 4501126 - }, - "id": 2668530, - "non_hive": { - "close": 59080, - "high": 81, - "low": 1052, - "open": 1945, - "volume": 948455 - }, - "open": "2020-07-18T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4000, - "high": 785, - "low": 5000, - "open": 9420, - "volume": 1922267 - }, - "id": 2668574, - "non_hive": { - "close": 850, - "high": 167, - "low": 1052, - "open": 1999, - "volume": 404990 - }, - "open": "2020-07-18T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1179, - "high": 51, - "low": 24, - "open": 18863, - "volume": 36122 - }, - "id": 2668610, - "non_hive": { - "close": 250, - "high": 11, - "low": 5, - "open": 4002, - "volume": 7664 - }, - "open": "2020-07-18T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4289, - "high": 47, - "low": 15, - "open": 18829, - "volume": 484796 - }, - "id": 2668636, - "non_hive": { - "close": 909, - "high": 10, - "low": 3, - "open": 3991, - "volume": 102794 - }, - "open": "2020-07-18T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74366, - "high": 65, - "low": 15, - "open": 9450, - "volume": 127636 - }, - "id": 2668660, - "non_hive": { - "close": 15781, - "high": 14, - "low": 3, - "open": 2002, - "volume": 27064 - }, - "open": "2020-07-19T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2440, - "high": 3733, - "low": 482, - "open": 19193, - "volume": 1542300 - }, - "id": 2668688, - "non_hive": { - "close": 519, - "high": 797, - "low": 102, - "open": 4072, - "volume": 327241 - }, - "open": "2020-07-19T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 239, - "high": 2951, - "low": 588697, - "open": 2651, - "volume": 2441978 - }, - "id": 2668712, - "non_hive": { - "close": 51, - "high": 630, - "low": 124807, - "open": 564, - "volume": 517764 - }, - "open": "2020-07-19T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1001, - "high": 1555, - "low": 3063, - "open": 9432, - "volume": 2467709 - }, - "id": 2668741, - "non_hive": { - "close": 213, - "high": 331, - "low": 648, - "open": 2000, - "volume": 523224 - }, - "open": "2020-07-19T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1860, - "high": 1860, - "low": 325, - "open": 14206, - "volume": 4363021 - }, - "id": 2668768, - "non_hive": { - "close": 400, - "high": 400, - "low": 69, - "open": 3022, - "volume": 931622 - }, - "open": "2020-07-19T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4540, - "high": 1200, - "low": 81896, - "open": 11702, - "volume": 4049451 - }, - "id": 2668806, - "non_hive": { - "close": 987, - "high": 261, - "low": 17611, - "open": 2522, - "volume": 871960 - }, - "open": "2020-07-19T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 157, - "high": 157, - "low": 568368, - "open": 9279, - "volume": 3881451 - }, - "id": 2668845, - "non_hive": { - "close": 34, - "high": 34, - "low": 121713, - "open": 2000, - "volume": 834678 - }, - "open": "2020-07-19T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25649, - "high": 634, - "low": 555115, - "open": 6940, - "volume": 912870 - }, - "id": 2668872, - "non_hive": { - "close": 5538, - "high": 137, - "low": 119353, - "open": 1495, - "volume": 196576 - }, - "open": "2020-07-19T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 599856, - "high": 180, - "low": 4806, - "open": 1042, - "volume": 2535578 - }, - "id": 2668899, - "non_hive": { - "close": 129035, - "high": 39, - "low": 1033, - "open": 225, - "volume": 545659 - }, - "open": "2020-07-19T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72298, - "high": 514, - "low": 311, - "open": 600000, - "volume": 3054601 - }, - "id": 2668935, - "non_hive": { - "close": 15579, - "high": 111, - "low": 66, - "open": 129066, - "volume": 655379 - }, - "open": "2020-07-19T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 130, - "high": 501, - "low": 574699, - "open": 501, - "volume": 3668569 - }, - "id": 2668965, - "non_hive": { - "close": 28, - "high": 108, - "low": 122561, - "open": 108, - "volume": 782774 - }, - "open": "2020-07-19T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2898, - "high": 37, - "low": 39432, - "open": 19377, - "volume": 2771724 - }, - "id": 2669008, - "non_hive": { - "close": 619, - "high": 8, - "low": 8368, - "open": 4145, - "volume": 591280 - }, - "open": "2020-07-19T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19149, - "high": 9, - "low": 475698, - "open": 1090, - "volume": 2688724 - }, - "id": 2669065, - "non_hive": { - "close": 4097, - "high": 2, - "low": 100769, - "open": 233, - "volume": 570409 - }, - "open": "2020-07-19T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48912, - "high": 1093, - "low": 19424, - "open": 9353, - "volume": 2104917 - }, - "id": 2669113, - "non_hive": { - "close": 10465, - "high": 234, - "low": 4141, - "open": 1999, - "volume": 449005 - }, - "open": "2020-07-19T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 565, - "high": 565, - "low": 152, - "open": 152, - "volume": 889069 - }, - "id": 2669146, - "non_hive": { - "close": 121, - "high": 121, - "low": 32, - "open": 32, - "volume": 190052 - }, - "open": "2020-07-19T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1860200, - "high": 696, - "low": 15, - "open": 4002, - "volume": 2114958 - }, - "id": 2669192, - "non_hive": { - "close": 398001, - "high": 149, - "low": 3, - "open": 856, - "volume": 452502 - }, - "open": "2020-07-19T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1638, - "high": 3408, - "low": 29, - "open": 26000, - "volume": 2020784 - }, - "id": 2669243, - "non_hive": { - "close": 349, - "high": 732, - "low": 6, - "open": 5563, - "volume": 428996 - }, - "open": "2020-07-19T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9377, - "high": 206, - "low": 166761, - "open": 206, - "volume": 1852406 - }, - "id": 2669280, - "non_hive": { - "close": 1999, - "high": 44, - "low": 35333, - "open": 44, - "volume": 392609 - }, - "open": "2020-07-19T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 322243, - "high": 490, - "low": 4942, - "open": 7882, - "volume": 804421 - }, - "id": 2669308, - "non_hive": { - "close": 68967, - "high": 105, - "low": 1047, - "open": 1681, - "volume": 171917 - }, - "open": "2020-07-19T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9387, - "high": 42, - "low": 207087, - "open": 411, - "volume": 1250581 - }, - "id": 2669362, - "non_hive": { - "close": 1999, - "high": 9, - "low": 43878, - "open": 88, - "volume": 265168 - }, - "open": "2020-07-19T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2303, - "high": 207273, - "low": 10347, - "open": 4874, - "volume": 288191 - }, - "id": 2669385, - "non_hive": { - "close": 490, - "high": 44360, - "low": 2198, - "open": 1038, - "volume": 61586 - }, - "open": "2020-07-19T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 267, - "high": 1313, - "low": 114346, - "open": 10427, - "volume": 1010149 - }, - "id": 2669405, - "non_hive": { - "close": 57, - "high": 283, - "low": 24124, - "open": 2223, - "volume": 214599 - }, - "open": "2020-07-19T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15465, - "high": 2666, - "low": 29, - "open": 9100, - "volume": 133102 - }, - "id": 2669433, - "non_hive": { - "close": 3294, - "high": 568, - "low": 6, - "open": 1938, - "volume": 28297 - }, - "open": "2020-07-19T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60198, - "high": 18, - "low": 15, - "open": 9259, - "volume": 185296 - }, - "id": 2669467, - "non_hive": { - "close": 12822, - "high": 4, - "low": 3, - "open": 1972, - "volume": 39453 - }, - "open": "2020-07-19T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10233, - "high": 18, - "low": 10252, - "open": 211, - "volume": 159414 - }, - "id": 2669518, - "non_hive": { - "close": 2179, - "high": 4, - "low": 2182, - "open": 45, - "volume": 33953 - }, - "open": "2020-07-20T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 223606, - "high": 84, - "low": 223606, - "open": 6409, - "volume": 1206578 - }, - "id": 2669561, - "non_hive": { - "close": 47180, - "high": 18, - "low": 47180, - "open": 1365, - "volume": 255035 - }, - "open": "2020-07-20T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3662, - "high": 2161, - "low": 5, - "open": 9501, - "volume": 1249822 - }, - "id": 2669576, - "non_hive": { - "close": 776, - "high": 458, - "low": 1, - "open": 2000, - "volume": 263135 - }, - "open": "2020-07-20T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 214565, - "high": 216, - "low": 583906, - "open": 5961, - "volume": 1110245 - }, - "id": 2669605, - "non_hive": { - "close": 45609, - "high": 46, - "low": 122887, - "open": 1263, - "volume": 234685 - }, - "open": "2020-07-20T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2353, - "high": 42, - "low": 1554, - "open": 42, - "volume": 182469 - }, - "id": 2669630, - "non_hive": { - "close": 500, - "high": 9, - "low": 330, - "open": 9, - "volume": 38784 - }, - "open": "2020-07-20T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3024, - "high": 9, - "low": 5167, - "open": 9, - "volume": 29252 - }, - "id": 2669649, - "non_hive": { - "close": 643, - "high": 2, - "low": 1097, - "open": 2, - "volume": 6216 - }, - "open": "2020-07-20T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4046, - "high": 18, - "low": 584261, - "open": 470, - "volume": 1860545 - }, - "id": 2669666, - "non_hive": { - "close": 857, - "high": 4, - "low": 122961, - "open": 100, - "volume": 392541 - }, - "open": "2020-07-20T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 372708, - "high": 2008, - "low": 372708, - "open": 5538, - "volume": 1261674 - }, - "id": 2669698, - "non_hive": { - "close": 78438, - "high": 425, - "low": 78438, - "open": 1172, - "volume": 265586 - }, - "open": "2020-07-20T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 235368, - "high": 155, - "low": 9436, - "open": 9436, - "volume": 797538 - }, - "id": 2669724, - "non_hive": { - "close": 49942, - "high": 33, - "low": 1999, - "open": 1999, - "volume": 169289 - }, - "open": "2020-07-20T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3885, - "high": 23, - "low": 72365, - "open": 9469, - "volume": 1990843 - }, - "id": 2669755, - "non_hive": { - "close": 822, - "high": 5, - "low": 15268, - "open": 2000, - "volume": 420487 - }, - "open": "2020-07-20T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18705, - "high": 301, - "low": 10133, - "open": 3148, - "volume": 3828913 - }, - "id": 2669803, - "non_hive": { - "close": 3970, - "high": 64, - "low": 2149, - "open": 668, - "volume": 812506 - }, - "open": "2020-07-20T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 108, - "high": 108, - "low": 6323, - "open": 116004, - "volume": 221870 - }, - "id": 2669872, - "non_hive": { - "close": 23, - "high": 23, - "low": 1341, - "open": 24621, - "volume": 47086 - }, - "open": "2020-07-20T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3201, - "high": 3201, - "low": 19210, - "open": 858, - "volume": 558946 - }, - "id": 2669902, - "non_hive": { - "close": 682, - "high": 682, - "low": 4072, - "open": 182, - "volume": 118913 - }, - "open": "2020-07-20T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 98, - "high": 98, - "low": 8250, - "open": 11140, - "volume": 745651 - }, - "id": 2669949, - "non_hive": { - "close": 21, - "high": 21, - "low": 1755, - "open": 2373, - "volume": 158818 - }, - "open": "2020-07-20T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3762, - "high": 3762, - "low": 99, - "open": 75, - "volume": 3706329 - }, - "id": 2669987, - "non_hive": { - "close": 812, - "high": 812, - "low": 21, - "open": 16, - "volume": 790732 - }, - "open": "2020-07-20T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46, - "high": 6295, - "low": 34, - "open": 6456, - "volume": 199340 - }, - "id": 2670028, - "non_hive": { - "close": 10, - "high": 1372, - "low": 7, - "open": 1393, - "volume": 43336 - }, - "open": "2020-07-20T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5742, - "high": 55, - "low": 33, - "open": 1514, - "volume": 554860 - }, - "id": 2670068, - "non_hive": { - "close": 1251, - "high": 12, - "low": 7, - "open": 330, - "volume": 120910 - }, - "open": "2020-07-20T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1001, - "high": 100, - "low": 1001, - "open": 10576, - "volume": 6279938 - }, - "id": 2670121, - "non_hive": { - "close": 214, - "high": 22, - "low": 214, - "open": 2315, - "volume": 1361333 - }, - "open": "2020-07-20T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9955, - "high": 9, - "low": 853, - "open": 853, - "volume": 4737322 - }, - "id": 2670185, - "non_hive": { - "close": 2147, - "high": 2, - "low": 182, - "open": 182, - "volume": 1018221 - }, - "open": "2020-07-20T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10010, - "high": 300, - "low": 121, - "open": 746, - "volume": 226557 - }, - "id": 2670225, - "non_hive": { - "close": 2164, - "high": 65, - "low": 26, - "open": 161, - "volume": 49014 - }, - "open": "2020-07-20T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 258658, - "high": 64, - "low": 33354, - "open": 54986, - "volume": 1696525 - }, - "id": 2670250, - "non_hive": { - "close": 55781, - "high": 14, - "low": 7094, - "open": 11888, - "volume": 365141 - }, - "open": "2020-07-20T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72356, - "high": 32, - "low": 72356, - "open": 14578, - "volume": 656190 - }, - "id": 2670308, - "non_hive": { - "close": 15585, - "high": 7, - "low": 15585, - "open": 3144, - "volume": 141488 - }, - "open": "2020-07-20T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10143, - "high": 2660, - "low": 28, - "open": 465, - "volume": 85288 - }, - "id": 2670351, - "non_hive": { - "close": 2183, - "high": 573, - "low": 6, - "open": 100, - "volume": 18357 - }, - "open": "2020-07-20T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10158, - "high": 18, - "low": 14, - "open": 374393, - "volume": 856993 - }, - "id": 2670384, - "non_hive": { - "close": 2186, - "high": 4, - "low": 3, - "open": 80589, - "volume": 184468 - }, - "open": "2020-07-20T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9954, - "high": 232, - "low": 705148, - "open": 77174, - "volume": 1041001 - }, - "id": 2670439, - "non_hive": { - "close": 2139, - "high": 50, - "low": 149994, - "open": 16612, - "volume": 222149 - }, - "open": "2020-07-21T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17003, - "high": 1084, - "low": 6139, - "open": 3727, - "volume": 115186 - }, - "id": 2670467, - "non_hive": { - "close": 3654, - "high": 233, - "low": 1319, - "open": 801, - "volume": 24753 - }, - "open": "2020-07-21T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9472, - "high": 1557, - "low": 3649, - "open": 609062, - "volume": 4137667 - }, - "id": 2670495, - "non_hive": { - "close": 2055, - "high": 338, - "low": 784, - "open": 130883, - "volume": 889878 - }, - "open": "2020-07-21T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 370396, - "high": 12993, - "low": 370396, - "open": 125, - "volume": 3113469 - }, - "id": 2670541, - "non_hive": { - "close": 79325, - "high": 2819, - "low": 79325, - "open": 27, - "volume": 669776 - }, - "open": "2020-07-21T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 590666, - "high": 23, - "low": 590666, - "open": 283, - "volume": 3644333 - }, - "id": 2670570, - "non_hive": { - "close": 126538, - "high": 5, - "low": 126538, - "open": 61, - "volume": 782455 - }, - "open": "2020-07-21T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 593043, - "high": 571, - "low": 593043, - "open": 571, - "volume": 8022421 - }, - "id": 2670599, - "non_hive": { - "close": 126558, - "high": 123, - "low": 126558, - "open": 123, - "volume": 1722300 - }, - "open": "2020-07-21T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6632, - "high": 158, - "low": 6, - "open": 7232, - "volume": 3049295 - }, - "id": 2670628, - "non_hive": { - "close": 1426, - "high": 34, - "low": 1, - "open": 1553, - "volume": 652963 - }, - "open": "2020-07-21T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10651, - "high": 7311, - "low": 610, - "open": 610, - "volume": 9244601 - }, - "id": 2670664, - "non_hive": { - "close": 2631, - "high": 1806, - "low": 131, - "open": 131, - "volume": 2071033 - }, - "open": "2020-07-21T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1167, - "high": 1167, - "low": 25546, - "open": 8451, - "volume": 1568597 - }, - "id": 2670733, - "non_hive": { - "close": 292, - "high": 292, - "low": 6045, - "open": 2000, - "volume": 385860 - }, - "open": "2020-07-21T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3674, - "high": 5314, - "low": 441000, - "open": 5314, - "volume": 9173142 - }, - "id": 2670775, - "non_hive": { - "close": 916, - "high": 1329, - "low": 106189, - "open": 1329, - "volume": 2245986 - }, - "open": "2020-07-21T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9997, - "high": 405, - "low": 784375, - "open": 8188, - "volume": 8152467 - }, - "id": 2670845, - "non_hive": { - "close": 2302, - "high": 101, - "low": 173373, - "open": 1999, - "volume": 1933247 - }, - "open": "2020-07-21T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3957, - "high": 25, - "low": 181, - "open": 394220, - "volume": 7078320 - }, - "id": 2670916, - "non_hive": { - "close": 943, - "high": 6, - "low": 41, - "open": 90740, - "volume": 1650343 - }, - "open": "2020-07-21T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7430, - "high": 144, - "low": 5, - "open": 8399, - "volume": 4682431 - }, - "id": 2670978, - "non_hive": { - "close": 1850, - "high": 36, - "low": 1, - "open": 1999, - "volume": 1107854 - }, - "open": "2020-07-21T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2852, - "high": 24, - "low": 180, - "open": 78316, - "volume": 6117002 - }, - "id": 2671039, - "non_hive": { - "close": 705, - "high": 6, - "low": 43, - "open": 19500, - "volume": 1484096 - }, - "open": "2020-07-21T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 210485, - "high": 20, - "low": 10, - "open": 103, - "volume": 3476326 - }, - "id": 2671102, - "non_hive": { - "close": 49884, - "high": 5, - "low": 2, - "open": 25, - "volume": 834112 - }, - "open": "2020-07-21T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1017, - "high": 8018, - "low": 13, - "open": 8439, - "volume": 806595 - }, - "id": 2671157, - "non_hive": { - "close": 239, - "high": 1960, - "low": 3, - "open": 1999, - "volume": 191882 - }, - "open": "2020-07-21T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 186, - "high": 16, - "low": 186, - "open": 29515, - "volume": 1371670 - }, - "id": 2671210, - "non_hive": { - "close": 43, - "high": 4, - "low": 43, - "open": 7079, - "volume": 328962 - }, - "open": "2020-07-21T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 980, - "high": 49, - "low": 101, - "open": 4247, - "volume": 1480176 - }, - "id": 2671241, - "non_hive": { - "close": 236, - "high": 12, - "low": 23, - "open": 1028, - "volume": 351063 - }, - "open": "2020-07-21T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 979, - "high": 228, - "low": 22, - "open": 21557, - "volume": 171703 - }, - "id": 2671288, - "non_hive": { - "close": 235, - "high": 55, - "low": 5, - "open": 5182, - "volume": 41243 - }, - "open": "2020-07-21T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1375, - "high": 54, - "low": 90, - "open": 212, - "volume": 4335097 - }, - "id": 2671333, - "non_hive": { - "close": 323, - "high": 13, - "low": 21, - "open": 51, - "volume": 1034648 - }, - "open": "2020-07-21T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 794, - "high": 225, - "low": 177, - "open": 225, - "volume": 105603 - }, - "id": 2671371, - "non_hive": { - "close": 186, - "high": 53, - "low": 40, - "open": 53, - "volume": 24686 - }, - "open": "2020-07-21T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 72, - "low": 10000, - "open": 72, - "volume": 494871 - }, - "id": 2671398, - "non_hive": { - "close": 2349, - "high": 17, - "low": 2338, - "open": 17, - "volume": 115736 - }, - "open": "2020-07-21T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3665, - "high": 16803, - "low": 47, - "open": 16803, - "volume": 55965 - }, - "id": 2671426, - "non_hive": { - "close": 860, - "high": 3948, - "low": 10, - "open": 3948, - "volume": 13144 - }, - "open": "2020-07-21T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127650, - "high": 33, - "low": 277, - "open": 38858, - "volume": 8730191 - }, - "id": 2671449, - "non_hive": { - "close": 29487, - "high": 8, - "low": 63, - "open": 9131, - "volume": 2043869 - }, - "open": "2020-07-21T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 119, - "high": 8, - "low": 119, - "open": 8521, - "volume": 15552095 - }, - "id": 2671495, - "non_hive": { - "close": 26, - "high": 2, - "low": 26, - "open": 1968, - "volume": 3612377 - }, - "open": "2020-07-22T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 386, - "high": 297, - "low": 88, - "open": 297, - "volume": 60613 - }, - "id": 2671554, - "non_hive": { - "close": 91, - "high": 71, - "low": 19, - "open": 71, - "volume": 14427 - }, - "open": "2020-07-22T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21, - "high": 1001, - "low": 21, - "open": 8050, - "volume": 1826120 - }, - "id": 2671583, - "non_hive": { - "close": 4, - "high": 238, - "low": 4, - "open": 1909, - "volume": 418016 - }, - "open": "2020-07-22T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90, - "high": 4664, - "low": 90, - "open": 8769, - "volume": 2446422 - }, - "id": 2671603, - "non_hive": { - "close": 20, - "high": 1104, - "low": 20, - "open": 2000, - "volume": 558089 - }, - "open": "2020-07-22T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 591268, - "high": 3520, - "low": 42, - "open": 3520, - "volume": 1818095 - }, - "id": 2671629, - "non_hive": { - "close": 135401, - "high": 829, - "low": 9, - "open": 829, - "volume": 414783 - }, - "open": "2020-07-22T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23, - "high": 571, - "low": 23, - "open": 8650, - "volume": 2830291 - }, - "id": 2671650, - "non_hive": { - "close": 5, - "high": 136, - "low": 5, - "open": 1999, - "volume": 654998 - }, - "open": "2020-07-22T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34, - "high": 420, - "low": 34, - "open": 3002, - "volume": 77316 - }, - "id": 2671673, - "non_hive": { - "close": 8, - "high": 100, - "low": 7, - "open": 714, - "volume": 18351 - }, - "open": "2020-07-22T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1390, - "high": 1000, - "low": 34, - "open": 34, - "volume": 21393 - }, - "id": 2671697, - "non_hive": { - "close": 329, - "high": 237, - "low": 8, - "open": 8, - "volume": 5067 - }, - "open": "2020-07-22T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 5526, - "low": 17, - "open": 2981, - "volume": 4015780 - }, - "id": 2671718, - "non_hive": { - "close": 139800, - "high": 1314, - "low": 3, - "open": 705, - "volume": 936210 - }, - "open": "2020-07-22T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24505, - "high": 4509, - "low": 29, - "open": 4207, - "volume": 3180523 - }, - "id": 2671747, - "non_hive": { - "close": 5831, - "high": 1073, - "low": 6, - "open": 1000, - "volume": 738255 - }, - "open": "2020-07-22T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6693, - "high": 7568, - "low": 623, - "open": 1984, - "volume": 976801 - }, - "id": 2671787, - "non_hive": { - "close": 1591, - "high": 1801, - "low": 144, - "open": 472, - "volume": 229139 - }, - "open": "2020-07-22T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 255619, - "high": 6838, - "low": 219, - "open": 6838, - "volume": 1393576 - }, - "id": 2671816, - "non_hive": { - "close": 59399, - "high": 1625, - "low": 50, - "open": 1625, - "volume": 324730 - }, - "open": "2020-07-22T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31, - "high": 4781, - "low": 28, - "open": 8407, - "volume": 1968439 - }, - "id": 2671850, - "non_hive": { - "close": 7, - "high": 1137, - "low": 6, - "open": 1999, - "volume": 458001 - }, - "open": "2020-07-22T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13689, - "high": 13689, - "low": 42, - "open": 8074, - "volume": 693003 - }, - "id": 2671898, - "non_hive": { - "close": 3256, - "high": 3256, - "low": 9, - "open": 1911, - "volume": 160103 - }, - "open": "2020-07-22T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 244, - "high": 4, - "low": 161, - "open": 64, - "volume": 1058084 - }, - "id": 2671936, - "non_hive": { - "close": 57, - "high": 1, - "low": 37, - "open": 15, - "volume": 246025 - }, - "open": "2020-07-22T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 725, - "high": 154, - "low": 31, - "open": 28014, - "volume": 192768 - }, - "id": 2671978, - "non_hive": { - "close": 169, - "high": 36, - "low": 7, - "open": 6528, - "volume": 44922 - }, - "open": "2020-07-22T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 600000, - "high": 25, - "low": 31, - "open": 31, - "volume": 3025874 - }, - "id": 2672017, - "non_hive": { - "close": 138625, - "high": 6, - "low": 7, - "open": 7, - "volume": 700281 - }, - "open": "2020-07-22T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 564, - "high": 571, - "low": 4770, - "open": 8655, - "volume": 629970 - }, - "id": 2672063, - "non_hive": { - "close": 131, - "high": 133, - "low": 1095, - "open": 2000, - "volume": 144777 - }, - "open": "2020-07-22T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8627, - "high": 56, - "low": 105, - "open": 3247, - "volume": 2524000 - }, - "id": 2672090, - "non_hive": { - "close": 1999, - "high": 13, - "low": 24, - "open": 753, - "volume": 583115 - }, - "open": "2020-07-22T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5183, - "high": 60, - "low": 122, - "open": 9956, - "volume": 692078 - }, - "id": 2672135, - "non_hive": { - "close": 1200, - "high": 14, - "low": 28, - "open": 2307, - "volume": 159344 - }, - "open": "2020-07-22T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21567, - "high": 105330, - "low": 2310, - "open": 2000, - "volume": 1018561 - }, - "id": 2672169, - "non_hive": { - "close": 4995, - "high": 24434, - "low": 531, - "open": 460, - "volume": 234708 - }, - "open": "2020-07-22T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 540, - "high": 2987, - "low": 8694, - "open": 4313, - "volume": 169437 - }, - "id": 2672211, - "non_hive": { - "close": 125, - "high": 692, - "low": 2000, - "open": 999, - "volume": 39214 - }, - "open": "2020-07-22T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 168, - "high": 168, - "low": 27, - "open": 8694, - "volume": 3729467 - }, - "id": 2672242, - "non_hive": { - "close": 39, - "high": 39, - "low": 6, - "open": 2000, - "volume": 856704 - }, - "open": "2020-07-22T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6529, - "high": 21, - "low": 14, - "open": 8654, - "volume": 3275079 - }, - "id": 2672279, - "non_hive": { - "close": 1505, - "high": 5, - "low": 3, - "open": 1999, - "volume": 748325 - }, - "open": "2020-07-22T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49919, - "high": 4, - "low": 20224, - "open": 7450, - "volume": 154250 - }, - "id": 2672336, - "non_hive": { - "close": 11500, - "high": 1, - "low": 4659, - "open": 1717, - "volume": 35539 - }, - "open": "2020-07-23T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 84, - "high": 34, - "low": 84, - "open": 2605, - "volume": 21116 - }, - "id": 2672359, - "non_hive": { - "close": 19, - "high": 8, - "low": 19, - "open": 600, - "volume": 4862 - }, - "open": "2020-07-23T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1424, - "high": 4358, - "low": 5, - "open": 4358, - "volume": 2859804 - }, - "id": 2672374, - "non_hive": { - "close": 325, - "high": 1003, - "low": 1, - "open": 1003, - "volume": 652249 - }, - "open": "2020-07-23T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6039, - "high": 710, - "low": 14, - "open": 11129, - "volume": 187553 - }, - "id": 2672396, - "non_hive": { - "close": 1385, - "high": 163, - "low": 3, - "open": 2552, - "volume": 43015 - }, - "open": "2020-07-23T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7225, - "high": 4, - "low": 78975, - "open": 8740, - "volume": 2456160 - }, - "id": 2672412, - "non_hive": { - "close": 1656, - "high": 1, - "low": 17929, - "open": 1997, - "volume": 561832 - }, - "open": "2020-07-23T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8201, - "high": 7283, - "low": 4994, - "open": 7283, - "volume": 1408739 - }, - "id": 2672452, - "non_hive": { - "close": 1870, - "high": 1669, - "low": 1128, - "open": 1669, - "volume": 319619 - }, - "open": "2020-07-23T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8886, - "high": 438, - "low": 58, - "open": 438, - "volume": 59475 - }, - "id": 2672482, - "non_hive": { - "close": 2028, - "high": 100, - "low": 13, - "open": 100, - "volume": 13556 - }, - "open": "2020-07-23T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 401142, - "high": 1354, - "low": 11145, - "open": 16146, - "volume": 4051711 - }, - "id": 2672503, - "non_hive": { - "close": 93580, - "high": 336, - "low": 2539, - "open": 3685, - "volume": 937597 - }, - "open": "2020-07-23T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 746280, - "high": 3016, - "low": 2148, - "open": 8547, - "volume": 3093403 - }, - "id": 2672540, - "non_hive": { - "close": 173478, - "high": 718, - "low": 490, - "open": 2000, - "volume": 720856 - }, - "open": "2020-07-23T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1026, - "high": 21, - "low": 22136, - "open": 150, - "volume": 2580098 - }, - "id": 2672591, - "non_hive": { - "close": 235, - "high": 5, - "low": 5000, - "open": 35, - "volume": 589436 - }, - "open": "2020-07-23T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8618, - "high": 4, - "low": 43, - "open": 8850, - "volume": 6955271 - }, - "id": 2672632, - "non_hive": { - "close": 1999, - "high": 1, - "low": 9, - "open": 1999, - "volume": 1580646 - }, - "open": "2020-07-23T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3185, - "high": 206, - "low": 3185, - "open": 8074, - "volume": 527207 - }, - "id": 2672687, - "non_hive": { - "close": 729, - "high": 48, - "low": 729, - "open": 1873, - "volume": 122520 - }, - "open": "2020-07-23T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9873, - "high": 1966, - "low": 36969, - "open": 8607, - "volume": 457422 - }, - "id": 2672720, - "non_hive": { - "close": 2299, - "high": 458, - "low": 8467, - "open": 1999, - "volume": 106249 - }, - "open": "2020-07-23T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14599, - "high": 2571, - "low": 3082, - "open": 8686, - "volume": 210752 - }, - "id": 2672767, - "non_hive": { - "close": 3361, - "high": 592, - "low": 706, - "open": 2000, - "volume": 48495 - }, - "open": "2020-07-23T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8730, - "high": 30, - "low": 391, - "open": 1268, - "volume": 190989 - }, - "id": 2672805, - "non_hive": { - "close": 2000, - "high": 7, - "low": 89, - "open": 292, - "volume": 43951 - }, - "open": "2020-07-23T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 225680, - "low": 14, - "open": 6814, - "volume": 394749 - }, - "id": 2672852, - "non_hive": { - "close": 3, - "high": 51964, - "low": 3, - "open": 1561, - "volume": 90819 - }, - "open": "2020-07-23T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1245, - "high": 115, - "low": 31, - "open": 7197, - "volume": 838724 - }, - "id": 2672878, - "non_hive": { - "close": 292, - "high": 27, - "low": 7, - "open": 1656, - "volume": 195514 - }, - "open": "2020-07-23T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11246, - "high": 153, - "low": 15, - "open": 1112, - "volume": 3631661 - }, - "id": 2672926, - "non_hive": { - "close": 2589, - "high": 36, - "low": 3, - "open": 256, - "volume": 830879 - }, - "open": "2020-07-23T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 161, - "high": 176, - "low": 13, - "open": 3192, - "volume": 450342 - }, - "id": 2672963, - "non_hive": { - "close": 37, - "high": 41, - "low": 2, - "open": 735, - "volume": 103430 - }, - "open": "2020-07-23T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1305, - "high": 14705, - "low": 55, - "open": 8701, - "volume": 4810450 - }, - "id": 2672995, - "non_hive": { - "close": 297, - "high": 3411, - "low": 12, - "open": 2000, - "volume": 1094197 - }, - "open": "2020-07-23T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1608, - "high": 48, - "low": 8000, - "open": 518, - "volume": 866939 - }, - "id": 2673059, - "non_hive": { - "close": 367, - "high": 11, - "low": 1815, - "open": 118, - "volume": 196868 - }, - "open": "2020-07-23T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5715, - "high": 1998, - "low": 2368, - "open": 671, - "volume": 181157 - }, - "id": 2673091, - "non_hive": { - "close": 1304, - "high": 456, - "low": 535, - "open": 152, - "volume": 41323 - }, - "open": "2020-07-23T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5142, - "high": 376, - "low": 27, - "open": 1161, - "volume": 82554 - }, - "id": 2673125, - "non_hive": { - "close": 1173, - "high": 86, - "low": 6, - "open": 265, - "volume": 18831 - }, - "open": "2020-07-23T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21377, - "high": 179, - "low": 14, - "open": 14308, - "volume": 148903 - }, - "id": 2673157, - "non_hive": { - "close": 4876, - "high": 41, - "low": 3, - "open": 3264, - "volume": 33875 - }, - "open": "2020-07-23T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25867, - "high": 43, - "low": 43441, - "open": 6400, - "volume": 1880587 - }, - "id": 2673188, - "non_hive": { - "close": 5901, - "high": 10, - "low": 9822, - "open": 1460, - "volume": 428812 - }, - "open": "2020-07-24T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 188, - "high": 174734, - "low": 413, - "open": 413, - "volume": 3151704 - }, - "id": 2673230, - "non_hive": { - "close": 45, - "high": 41847, - "low": 94, - "open": 94, - "volume": 729915 - }, - "open": "2020-07-24T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8003, - "high": 112, - "low": 10191, - "open": 10726, - "volume": 804060 - }, - "id": 2673263, - "non_hive": { - "close": 1917, - "high": 27, - "low": 2436, - "open": 2564, - "volume": 192392 - }, - "open": "2020-07-24T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 320506, - "high": 13578, - "low": 320506, - "open": 13578, - "volume": 448072 - }, - "id": 2673286, - "non_hive": { - "close": 76611, - "high": 3364, - "low": 76611, - "open": 3364, - "volume": 107893 - }, - "open": "2020-07-24T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 106, - "high": 4, - "low": 8532, - "open": 2000, - "volume": 3535409 - }, - "id": 2673304, - "non_hive": { - "close": 25, - "high": 1, - "low": 1999, - "open": 494, - "volume": 832421 - }, - "open": "2020-07-24T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3877, - "high": 144, - "low": 24000, - "open": 17830, - "volume": 142782 - }, - "id": 2673339, - "non_hive": { - "close": 907, - "high": 34, - "low": 5613, - "open": 4179, - "volume": 33419 - }, - "open": "2020-07-24T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18787, - "high": 23448, - "low": 137, - "open": 23448, - "volume": 625179 - }, - "id": 2673377, - "non_hive": { - "close": 4333, - "high": 5497, - "low": 31, - "open": 5497, - "volume": 143339 - }, - "open": "2020-07-24T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13615, - "high": 6710, - "low": 13615, - "open": 8382, - "volume": 747117 - }, - "id": 2673406, - "non_hive": { - "close": 3103, - "high": 1574, - "low": 3103, - "open": 1933, - "volume": 170703 - }, - "open": "2020-07-24T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 64453, - "high": 4591, - "low": 1551, - "open": 8767, - "volume": 2842605 - }, - "id": 2673428, - "non_hive": { - "close": 15056, - "high": 1077, - "low": 353, - "open": 1997, - "volume": 650185 - }, - "open": "2020-07-24T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39, - "high": 78629, - "low": 39, - "open": 6920, - "volume": 238713 - }, - "id": 2673462, - "non_hive": { - "close": 8, - "high": 18391, - "low": 8, - "open": 1616, - "volume": 55514 - }, - "open": "2020-07-24T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 784, - "high": 29, - "low": 761, - "open": 6487, - "volume": 467305 - }, - "id": 2673500, - "non_hive": { - "close": 183, - "high": 7, - "low": 177, - "open": 1515, - "volume": 109281 - }, - "open": "2020-07-24T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5068, - "high": 1000, - "low": 2043, - "open": 27697, - "volume": 114694 - }, - "id": 2673529, - "non_hive": { - "close": 1200, - "high": 237, - "low": 476, - "open": 6459, - "volume": 26778 - }, - "open": "2020-07-24T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 89, - "high": 6119, - "low": 60809, - "open": 634, - "volume": 3176058 - }, - "id": 2673552, - "non_hive": { - "close": 21, - "high": 1449, - "low": 14104, - "open": 150, - "volume": 737817 - }, - "open": "2020-07-24T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1467, - "high": 286, - "low": 6582, - "open": 8621, - "volume": 891234 - }, - "id": 2673579, - "non_hive": { - "close": 342, - "high": 67, - "low": 1526, - "open": 2000, - "volume": 207677 - }, - "open": "2020-07-24T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16011, - "high": 20991, - "low": 16011, - "open": 103, - "volume": 75426 - }, - "id": 2673621, - "non_hive": { - "close": 3729, - "high": 4897, - "low": 3729, - "open": 24, - "volume": 17586 - }, - "open": "2020-07-24T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 4, - "low": 31, - "open": 5543, - "volume": 706121 - }, - "id": 2673644, - "non_hive": { - "close": 3, - "high": 1, - "low": 7, - "open": 1291, - "volume": 164537 - }, - "open": "2020-07-24T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19398, - "high": 329, - "low": 21, - "open": 39976, - "volume": 1258450 - }, - "id": 2673699, - "non_hive": { - "close": 4551, - "high": 78, - "low": 4, - "open": 9457, - "volume": 296368 - }, - "open": "2020-07-24T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 231335, - "high": 2576, - "low": 699249, - "open": 2576, - "volume": 9648150 - }, - "id": 2673762, - "non_hive": { - "close": 53901, - "high": 604, - "low": 160117, - "open": 604, - "volume": 2228326 - }, - "open": "2020-07-24T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 85, - "high": 6795, - "low": 4327, - "open": 6795, - "volume": 1050328 - }, - "id": 2673820, - "non_hive": { - "close": 20, - "high": 1606, - "low": 1000, - "open": 1606, - "volume": 245547 - }, - "open": "2020-07-24T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8597, - "high": 8, - "low": 2089, - "open": 517, - "volume": 4618836 - }, - "id": 2673845, - "non_hive": { - "close": 2000, - "high": 2, - "low": 483, - "open": 121, - "volume": 1072905 - }, - "open": "2020-07-24T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5655, - "high": 2468, - "low": 5775, - "open": 5775, - "volume": 1158429 - }, - "id": 2673896, - "non_hive": { - "close": 1321, - "high": 577, - "low": 1348, - "open": 1348, - "volume": 270653 - }, - "open": "2020-07-24T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59634, - "high": 4, - "low": 4273, - "open": 13395, - "volume": 162791 - }, - "id": 2673939, - "non_hive": { - "close": 13935, - "high": 1, - "low": 994, - "open": 3128, - "volume": 37985 - }, - "open": "2020-07-24T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8898, - "high": 51, - "low": 26, - "open": 8559, - "volume": 2023574 - }, - "id": 2673966, - "non_hive": { - "close": 2079, - "high": 12, - "low": 6, - "open": 1999, - "volume": 472843 - }, - "open": "2020-07-24T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 335, - "high": 458444, - "low": 14, - "open": 3304, - "volume": 6083629 - }, - "id": 2674000, - "non_hive": { - "close": 77, - "high": 110018, - "low": 3, - "open": 772, - "volume": 1421050 - }, - "open": "2020-07-24T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12117, - "high": 8355, - "low": 25726, - "open": 596, - "volume": 138900 - }, - "id": 2674040, - "non_hive": { - "close": 2900, - "high": 2000, - "low": 5881, - "open": 137, - "volume": 32287 - }, - "open": "2020-07-25T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 222, - "high": 3156, - "low": 7406, - "open": 8734, - "volume": 40080 - }, - "id": 2674071, - "non_hive": { - "close": 52, - "high": 754, - "low": 1695, - "open": 2000, - "volume": 9344 - }, - "open": "2020-07-25T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8567, - "high": 1089, - "low": 26402, - "open": 26402, - "volume": 36058 - }, - "id": 2674087, - "non_hive": { - "close": 1998, - "high": 254, - "low": 6157, - "open": 6157, - "volume": 8409 - }, - "open": "2020-07-25T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28555, - "high": 12865, - "low": 198841, - "open": 12865, - "volume": 627839 - }, - "id": 2674096, - "non_hive": { - "close": 6569, - "high": 3000, - "low": 45734, - "open": 3000, - "volume": 144509 - }, - "open": "2020-07-25T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30873, - "high": 334, - "low": 1260, - "open": 334, - "volume": 754146 - }, - "id": 2674127, - "non_hive": { - "close": 7102, - "high": 77, - "low": 288, - "open": 77, - "volume": 173467 - }, - "open": "2020-07-25T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3447, - "high": 56, - "low": 3447, - "open": 170, - "volume": 349724 - }, - "id": 2674173, - "non_hive": { - "close": 789, - "high": 13, - "low": 789, - "open": 39, - "volume": 80444 - }, - "open": "2020-07-25T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10502, - "high": 1638, - "low": 5369, - "open": 3135, - "volume": 112130 - }, - "id": 2674216, - "non_hive": { - "close": 2416, - "high": 377, - "low": 1228, - "open": 721, - "volume": 25784 - }, - "open": "2020-07-25T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8072, - "high": 1317, - "low": 300, - "open": 13271, - "volume": 102342 - }, - "id": 2674245, - "non_hive": { - "close": 1857, - "high": 303, - "low": 68, - "open": 3053, - "volume": 23503 - }, - "open": "2020-07-25T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13128, - "high": 682, - "low": 10917, - "open": 10917, - "volume": 63586 - }, - "id": 2674270, - "non_hive": { - "close": 3020, - "high": 157, - "low": 2510, - "open": 2510, - "volume": 14626 - }, - "open": "2020-07-25T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13375, - "high": 343, - "low": 61861, - "open": 14062, - "volume": 325939 - }, - "id": 2674286, - "non_hive": { - "close": 3077, - "high": 79, - "low": 14230, - "open": 3235, - "volume": 74980 - }, - "open": "2020-07-25T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34776, - "high": 956, - "low": 1425, - "open": 5003, - "volume": 321295 - }, - "id": 2674313, - "non_hive": { - "close": 8000, - "high": 220, - "low": 326, - "open": 1151, - "volume": 73888 - }, - "open": "2020-07-25T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5290, - "high": 108, - "low": 1971, - "open": 6723, - "volume": 84956 - }, - "id": 2674339, - "non_hive": { - "close": 1217, - "high": 25, - "low": 453, - "open": 1546, - "volume": 19543 - }, - "open": "2020-07-25T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23566, - "high": 26, - "low": 242, - "open": 4000, - "volume": 1169213 - }, - "id": 2674369, - "non_hive": { - "close": 5420, - "high": 6, - "low": 55, - "open": 920, - "volume": 268882 - }, - "open": "2020-07-25T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4308, - "high": 115569, - "low": 3444, - "open": 46603, - "volume": 464827 - }, - "id": 2674421, - "non_hive": { - "close": 999, - "high": 26808, - "low": 789, - "open": 10719, - "volume": 107260 - }, - "open": "2020-07-25T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43133, - "high": 1000, - "low": 104, - "open": 1000, - "volume": 1061657 - }, - "id": 2674455, - "non_hive": { - "close": 9998, - "high": 232, - "low": 24, - "open": 232, - "volume": 246112 - }, - "open": "2020-07-25T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 80095, - "high": 80095, - "low": 31, - "open": 16062, - "volume": 352423 - }, - "id": 2674493, - "non_hive": { - "close": 18735, - "high": 18735, - "low": 7, - "open": 3726, - "volume": 81779 - }, - "open": "2020-07-25T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19025, - "high": 1179, - "low": 31, - "open": 30785, - "volume": 458606 - }, - "id": 2674531, - "non_hive": { - "close": 4430, - "high": 276, - "low": 7, - "open": 7201, - "volume": 106576 - }, - "open": "2020-07-25T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11191, - "high": 893, - "low": 2160, - "open": 8550, - "volume": 873671 - }, - "id": 2674569, - "non_hive": { - "close": 2618, - "high": 209, - "low": 503, - "open": 1999, - "volume": 204351 - }, - "open": "2020-07-25T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2347, - "high": 19095, - "low": 1500042, - "open": 19095, - "volume": 1655414 - }, - "id": 2674604, - "non_hive": { - "close": 547, - "high": 4467, - "low": 348865, - "open": 4467, - "volume": 385085 - }, - "open": "2020-07-25T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6480, - "high": 154, - "low": 1376, - "open": 1376, - "volume": 64354 - }, - "id": 2674623, - "non_hive": { - "close": 1510, - "high": 36, - "low": 320, - "open": 320, - "volume": 14995 - }, - "open": "2020-07-25T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 564950, - "high": 25, - "low": 2025, - "open": 4549, - "volume": 908479 - }, - "id": 2674651, - "non_hive": { - "close": 132159, - "high": 6, - "low": 469, - "open": 1060, - "volume": 212178 - }, - "open": "2020-07-25T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1120, - "high": 2962, - "low": 187, - "open": 39349, - "volume": 1115383 - }, - "id": 2674684, - "non_hive": { - "close": 262, - "high": 693, - "low": 43, - "open": 9205, - "volume": 260888 - }, - "open": "2020-07-25T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4293, - "high": 123, - "low": 26, - "open": 123, - "volume": 281711 - }, - "id": 2674720, - "non_hive": { - "close": 1000, - "high": 29, - "low": 6, - "open": 29, - "volume": 65743 - }, - "open": "2020-07-25T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 275, - "high": 12175, - "low": 13, - "open": 8581, - "volume": 348304 - }, - "id": 2674751, - "non_hive": { - "close": 64, - "high": 2837, - "low": 3, - "open": 1999, - "volume": 81117 - }, - "open": "2020-07-25T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 416, - "high": 416, - "low": 6000, - "open": 10214, - "volume": 120892 - }, - "id": 2674778, - "non_hive": { - "close": 97, - "high": 97, - "low": 1397, - "open": 2380, - "volume": 28168 - }, - "open": "2020-07-26T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7575, - "high": 4343, - "low": 86, - "open": 25982, - "volume": 104444 - }, - "id": 2674809, - "non_hive": { - "close": 1765, - "high": 1012, - "low": 20, - "open": 6054, - "volume": 24336 - }, - "open": "2020-07-26T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4433, - "high": 90, - "low": 4433, - "open": 90, - "volume": 4523 - }, - "id": 2674828, - "non_hive": { - "close": 1033, - "high": 21, - "low": 1033, - "open": 21, - "volume": 1054 - }, - "open": "2020-07-26T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9145, - "high": 9145, - "low": 2278, - "open": 60657, - "volume": 194870 - }, - "id": 2674835, - "non_hive": { - "close": 2131, - "high": 2131, - "low": 530, - "open": 14133, - "volume": 45404 - }, - "open": "2020-07-26T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 1695, - "low": 7760, - "open": 6868, - "volume": 42888 - }, - "id": 2674850, - "non_hive": { - "close": 233, - "high": 395, - "low": 1807, - "open": 1600, - "volume": 9990 - }, - "open": "2020-07-26T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3518, - "high": 29360, - "low": 1981, - "open": 98019, - "volume": 514945 - }, - "id": 2674871, - "non_hive": { - "close": 820, - "high": 6867, - "low": 461, - "open": 22831, - "volume": 119991 - }, - "open": "2020-07-26T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1233, - "high": 1478, - "low": 87, - "open": 2009, - "volume": 223728 - }, - "id": 2674914, - "non_hive": { - "close": 286, - "high": 346, - "low": 20, - "open": 470, - "volume": 51991 - }, - "open": "2020-07-26T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6547, - "high": 4, - "low": 668834, - "open": 1026, - "volume": 3764210 - }, - "id": 2674962, - "non_hive": { - "close": 1515, - "high": 1, - "low": 152752, - "open": 238, - "volume": 864377 - }, - "open": "2020-07-26T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3340, - "high": 21073, - "low": 311, - "open": 8701, - "volume": 490699 - }, - "id": 2674995, - "non_hive": { - "close": 774, - "high": 4885, - "low": 71, - "open": 1999, - "volume": 112988 - }, - "open": "2020-07-26T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 619, - "high": 38, - "low": 392, - "open": 8642, - "volume": 2750871 - }, - "id": 2675032, - "non_hive": { - "close": 138, - "high": 9, - "low": 87, - "open": 1999, - "volume": 623880 - }, - "open": "2020-07-26T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1409, - "high": 8729, - "low": 117, - "open": 8729, - "volume": 3909785 - }, - "id": 2675081, - "non_hive": { - "close": 305, - "high": 2000, - "low": 25, - "open": 2000, - "volume": 859991 - }, - "open": "2020-07-26T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46322, - "high": 7087, - "low": 31996, - "open": 9174, - "volume": 459440 - }, - "id": 2675157, - "non_hive": { - "close": 9999, - "high": 1545, - "low": 6906, - "open": 1999, - "volume": 99284 - }, - "open": "2020-07-26T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7110, - "high": 7110, - "low": 301296, - "open": 46327, - "volume": 3660466 - }, - "id": 2675199, - "non_hive": { - "close": 1557, - "high": 1557, - "low": 65029, - "open": 9999, - "volume": 790236 - }, - "open": "2020-07-26T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 66524, - "high": 4301, - "low": 7082, - "open": 7082, - "volume": 295891 - }, - "id": 2675271, - "non_hive": { - "close": 15092, - "high": 976, - "low": 1558, - "open": 1558, - "volume": 66720 - }, - "open": "2020-07-26T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19445, - "high": 2543, - "low": 102, - "open": 8052, - "volume": 793425 - }, - "id": 2675309, - "non_hive": { - "close": 4472, - "high": 585, - "low": 23, - "open": 1828, - "volume": 182354 - }, - "open": "2020-07-26T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2617, - "high": 8732, - "low": 14, - "open": 8732, - "volume": 243523 - }, - "id": 2675355, - "non_hive": { - "close": 598, - "high": 2000, - "low": 3, - "open": 2000, - "volume": 55466 - }, - "open": "2020-07-26T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44102, - "high": 100, - "low": 31, - "open": 31, - "volume": 774739 - }, - "id": 2675382, - "non_hive": { - "close": 10050, - "high": 23, - "low": 7, - "open": 7, - "volume": 176424 - }, - "open": "2020-07-26T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1501, - "high": 52, - "low": 1258, - "open": 52, - "volume": 414753 - }, - "id": 2675433, - "non_hive": { - "close": 343, - "high": 12, - "low": 286, - "open": 12, - "volume": 94725 - }, - "open": "2020-07-26T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6803, - "high": 6803, - "low": 228, - "open": 7575, - "volume": 405893 - }, - "id": 2675472, - "non_hive": { - "close": 1558, - "high": 1558, - "low": 51, - "open": 1731, - "volume": 92749 - }, - "open": "2020-07-26T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4397, - "high": 152, - "low": 7690, - "open": 8731, - "volume": 2762547 - }, - "id": 2675513, - "non_hive": { - "close": 1007, - "high": 35, - "low": 1730, - "open": 2000, - "volume": 627012 - }, - "open": "2020-07-26T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5444, - "high": 757, - "low": 5444, - "open": 8733, - "volume": 154483 - }, - "id": 2675571, - "non_hive": { - "close": 1244, - "high": 174, - "low": 1244, - "open": 1999, - "volume": 35444 - }, - "open": "2020-07-26T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2302, - "high": 52, - "low": 683, - "open": 4417, - "volume": 325130 - }, - "id": 2675594, - "non_hive": { - "close": 525, - "high": 12, - "low": 154, - "open": 1007, - "volume": 73719 - }, - "open": "2020-07-26T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4438, - "high": 65192, - "low": 27, - "open": 1708, - "volume": 2312710 - }, - "id": 2675640, - "non_hive": { - "close": 1000, - "high": 14863, - "low": 6, - "open": 389, - "volume": 519316 - }, - "open": "2020-07-26T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 276, - "high": 323, - "low": 14, - "open": 3093, - "volume": 395495 - }, - "id": 2675689, - "non_hive": { - "close": 62, - "high": 73, - "low": 3, - "open": 697, - "volume": 89111 - }, - "open": "2020-07-26T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5488, - "high": 514, - "low": 20000, - "open": 11056, - "volume": 109045 - }, - "id": 2675720, - "non_hive": { - "close": 1237, - "high": 116, - "low": 4507, - "open": 2492, - "volume": 24578 - }, - "open": "2020-07-27T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28825, - "high": 381, - "low": 2191, - "open": 381, - "volume": 232030 - }, - "id": 2675750, - "non_hive": { - "close": 6497, - "high": 86, - "low": 493, - "open": 86, - "volume": 52299 - }, - "open": "2020-07-27T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6999, - "high": 6999, - "low": 3243, - "open": 13039, - "volume": 157913 - }, - "id": 2675775, - "non_hive": { - "close": 1599, - "high": 1599, - "low": 730, - "open": 2938, - "volume": 35755 - }, - "open": "2020-07-27T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2900, - "high": 4, - "low": 123, - "open": 4001, - "volume": 4352568 - }, - "id": 2675796, - "non_hive": { - "close": 661, - "high": 1, - "low": 27, - "open": 914, - "volume": 973825 - }, - "open": "2020-07-27T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 621789, - "high": 302, - "low": 37, - "open": 2976, - "volume": 1097814 - }, - "id": 2675839, - "non_hive": { - "close": 138001, - "high": 69, - "low": 8, - "open": 661, - "volume": 245042 - }, - "open": "2020-07-27T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 64694, - "high": 94, - "low": 11629, - "open": 9019, - "volume": 299646 - }, - "id": 2675871, - "non_hive": { - "close": 14200, - "high": 21, - "low": 2552, - "open": 1999, - "volume": 66228 - }, - "open": "2020-07-27T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6157, - "high": 1299, - "low": 7610, - "open": 9023, - "volume": 310106 - }, - "id": 2675912, - "non_hive": { - "close": 1365, - "high": 288, - "low": 1670, - "open": 1999, - "volume": 68562 - }, - "open": "2020-07-27T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9101, - "high": 4, - "low": 26170, - "open": 252, - "volume": 313417 - }, - "id": 2675948, - "non_hive": { - "close": 2000, - "high": 1, - "low": 5744, - "open": 56, - "volume": 69290 - }, - "open": "2020-07-27T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40988, - "high": 275, - "low": 320995, - "open": 30000, - "volume": 4512633 - }, - "id": 2676000, - "non_hive": { - "close": 9059, - "high": 61, - "low": 69700, - "open": 6647, - "volume": 996098 - }, - "open": "2020-07-27T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 227, - "high": 227, - "low": 51961, - "open": 9048, - "volume": 1531281 - }, - "id": 2676071, - "non_hive": { - "close": 52, - "high": 52, - "low": 11436, - "open": 1999, - "volume": 344266 - }, - "open": "2020-07-27T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21085, - "high": 100, - "low": 100, - "open": 9026, - "volume": 97445 - }, - "id": 2676113, - "non_hive": { - "close": 4729, - "high": 23, - "low": 22, - "open": 2064, - "volume": 21973 - }, - "open": "2020-07-27T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30000, - "high": 65, - "low": 51, - "open": 8914, - "volume": 2107506 - }, - "id": 2676140, - "non_hive": { - "close": 6863, - "high": 15, - "low": 11, - "open": 2000, - "volume": 475327 - }, - "open": "2020-07-27T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 741617, - "high": 875, - "low": 31443, - "open": 15744, - "volume": 4405608 - }, - "id": 2676197, - "non_hive": { - "close": 169098, - "high": 201, - "low": 7169, - "open": 3604, - "volume": 1006477 - }, - "open": "2020-07-27T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17581, - "high": 17581, - "low": 49, - "open": 8684, - "volume": 1361096 - }, - "id": 2676263, - "non_hive": { - "close": 4038, - "high": 4038, - "low": 11, - "open": 1988, - "volume": 311676 - }, - "open": "2020-07-27T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8230, - "high": 191022, - "low": 102, - "open": 19524, - "volume": 2528723 - }, - "id": 2676287, - "non_hive": { - "close": 2000, - "high": 46786, - "low": 23, - "open": 4473, - "volume": 591929 - }, - "open": "2020-07-27T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 226, - "high": 81, - "low": 48, - "open": 4308, - "volume": 4733269 - }, - "id": 2676347, - "non_hive": { - "close": 53, - "high": 20, - "low": 11, - "open": 1047, - "volume": 1115062 - }, - "open": "2020-07-27T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1856, - "high": 4, - "low": 23543, - "open": 8205, - "volume": 1670958 - }, - "id": 2676420, - "non_hive": { - "close": 427, - "high": 1, - "low": 5368, - "open": 1999, - "volume": 392719 - }, - "open": "2020-07-27T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3000, - "high": 591, - "low": 2844, - "open": 2849, - "volume": 6395141 - }, - "id": 2676473, - "non_hive": { - "close": 684, - "high": 136, - "low": 629, - "open": 655, - "volume": 1442665 - }, - "open": "2020-07-27T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1974, - "high": 95, - "low": 22, - "open": 8772, - "volume": 658317 - }, - "id": 2676583, - "non_hive": { - "close": 454, - "high": 22, - "low": 4, - "open": 1999, - "volume": 150141 - }, - "open": "2020-07-27T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42801, - "high": 1746, - "low": 165, - "open": 14754, - "volume": 274335 - }, - "id": 2676647, - "non_hive": { - "close": 9844, - "high": 405, - "low": 37, - "open": 3393, - "volume": 63200 - }, - "open": "2020-07-27T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1841, - "high": 1000, - "low": 30000, - "open": 30000, - "volume": 430399 - }, - "id": 2676690, - "non_hive": { - "close": 422, - "high": 232, - "low": 6870, - "open": 6870, - "volume": 99513 - }, - "open": "2020-07-27T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57, - "high": 288, - "low": 12, - "open": 1318, - "volume": 273168 - }, - "id": 2676720, - "non_hive": { - "close": 12, - "high": 67, - "low": 2, - "open": 305, - "volume": 62876 - }, - "open": "2020-07-27T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6784, - "high": 30, - "low": 6, - "open": 8769, - "volume": 5210952 - }, - "id": 2676762, - "non_hive": { - "close": 1525, - "high": 7, - "low": 1, - "open": 2000, - "volume": 1178935 - }, - "open": "2020-07-27T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 274, - "high": 30, - "low": 52, - "open": 8773, - "volume": 1502490 - }, - "id": 2676820, - "non_hive": { - "close": 63, - "high": 7, - "low": 11, - "open": 1999, - "volume": 344632 - }, - "open": "2020-07-27T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 66074, - "high": 298, - "low": 66074, - "open": 298, - "volume": 491775 - }, - "id": 2676880, - "non_hive": { - "close": 14766, - "high": 69, - "low": 14766, - "open": 69, - "volume": 111882 - }, - "open": "2020-07-28T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2685, - "high": 29, - "low": 610, - "open": 610, - "volume": 4318394 - }, - "id": 2676914, - "non_hive": { - "close": 623, - "high": 7, - "low": 136, - "open": 136, - "volume": 1002134 - }, - "open": "2020-07-28T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 199, - "high": 461445, - "low": 16892, - "open": 2126, - "volume": 512527 - }, - "id": 2676962, - "non_hive": { - "close": 47, - "high": 109847, - "low": 3908, - "open": 500, - "volume": 121810 - }, - "open": "2020-07-28T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 396573, - "high": 58, - "low": 115, - "open": 928, - "volume": 855470 - }, - "id": 2676981, - "non_hive": { - "close": 94404, - "high": 14, - "low": 27, - "open": 218, - "volume": 203633 - }, - "open": "2020-07-28T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 980057, - "high": 5946, - "low": 3998, - "open": 2097, - "volume": 2529196 - }, - "id": 2677016, - "non_hive": { - "close": 232277, - "high": 1420, - "low": 947, - "open": 500, - "volume": 600996 - }, - "open": "2020-07-28T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4626, - "high": 11844, - "low": 499706, - "open": 8376, - "volume": 3572509 - }, - "id": 2677049, - "non_hive": { - "close": 1104, - "high": 2828, - "low": 117358, - "open": 1999, - "volume": 843979 - }, - "open": "2020-07-28T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2329605, - "high": 16, - "low": 2329605, - "open": 11198, - "volume": 2704202 - }, - "id": 2677081, - "non_hive": { - "close": 543541, - "high": 4, - "low": 543541, - "open": 2630, - "volume": 631336 - }, - "open": "2020-07-28T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 113514, - "high": 54195, - "low": 1144, - "open": 8483, - "volume": 1818657 - }, - "id": 2677116, - "non_hive": { - "close": 26744, - "high": 12771, - "low": 269, - "open": 1999, - "volume": 428464 - }, - "open": "2020-07-28T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5373, - "high": 12113, - "low": 8571, - "open": 431, - "volume": 732948 - }, - "id": 2677142, - "non_hive": { - "close": 1265, - "high": 2854, - "low": 2000, - "open": 101, - "volume": 172579 - }, - "open": "2020-07-28T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3707, - "high": 1965, - "low": 3118, - "open": 3118, - "volume": 150140 - }, - "id": 2677174, - "non_hive": { - "close": 873, - "high": 463, - "low": 734, - "open": 734, - "volume": 35356 - }, - "open": "2020-07-28T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 645, - "high": 645, - "low": 220932, - "open": 220932, - "volume": 262935 - }, - "id": 2677193, - "non_hive": { - "close": 152, - "high": 152, - "low": 52024, - "open": 52024, - "volume": 61915 - }, - "open": "2020-07-28T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7428, - "high": 276, - "low": 24, - "open": 4247, - "volume": 2434477 - }, - "id": 2677211, - "non_hive": { - "close": 1771, - "high": 66, - "low": 5, - "open": 1000, - "volume": 577084 - }, - "open": "2020-07-28T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4187, - "high": 299159, - "low": 421, - "open": 421, - "volume": 450514 - }, - "id": 2677251, - "non_hive": { - "close": 999, - "high": 71388, - "low": 100, - "open": 100, - "volume": 107500 - }, - "open": "2020-07-28T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18922, - "high": 2950, - "low": 9002, - "open": 2950, - "volume": 123758 - }, - "id": 2677275, - "non_hive": { - "close": 4513, - "high": 704, - "low": 2147, - "open": 704, - "volume": 29519 - }, - "open": "2020-07-28T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14583, - "high": 25, - "low": 226129, - "open": 306, - "volume": 2182489 - }, - "id": 2677303, - "non_hive": { - "close": 3480, - "high": 6, - "low": 53699, - "open": 73, - "volume": 520369 - }, - "open": "2020-07-28T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 2543, - "low": 13, - "open": 5016, - "volume": 530495 - }, - "id": 2677349, - "non_hive": { - "close": 3, - "high": 607, - "low": 3, - "open": 1197, - "volume": 126574 - }, - "open": "2020-07-28T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8385, - "high": 7141, - "low": 30, - "open": 30, - "volume": 359827 - }, - "id": 2677388, - "non_hive": { - "close": 2000, - "high": 1704, - "low": 7, - "open": 7, - "volume": 85850 - }, - "open": "2020-07-28T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14012, - "high": 201, - "low": 20792, - "open": 12355, - "volume": 221352 - }, - "id": 2677425, - "non_hive": { - "close": 3343, - "high": 48, - "low": 4960, - "open": 2948, - "volume": 52815 - }, - "open": "2020-07-28T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 686, - "high": 50, - "low": 14689, - "open": 14689, - "volume": 1182813 - }, - "id": 2677464, - "non_hive": { - "close": 164, - "high": 12, - "low": 3504, - "open": 3504, - "volume": 282373 - }, - "open": "2020-07-28T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16274, - "high": 188, - "low": 11689, - "open": 11689, - "volume": 95535 - }, - "id": 2677494, - "non_hive": { - "close": 3886, - "high": 45, - "low": 2791, - "open": 2791, - "volume": 22812 - }, - "open": "2020-07-28T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10042, - "high": 33, - "low": 683351, - "open": 10788, - "volume": 2407595 - }, - "id": 2677523, - "non_hive": { - "close": 2398, - "high": 8, - "low": 163164, - "open": 2576, - "volume": 574876 - }, - "open": "2020-07-28T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 76003, - "high": 1000, - "low": 5769, - "open": 825, - "volume": 579175 - }, - "id": 2677564, - "non_hive": { - "close": 18148, - "high": 239, - "low": 1377, - "open": 197, - "volume": 138294 - }, - "open": "2020-07-28T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1950, - "high": 137, - "low": 26, - "open": 3203, - "volume": 106114 - }, - "id": 2677591, - "non_hive": { - "close": 467, - "high": 33, - "low": 6, - "open": 765, - "volume": 25340 - }, - "open": "2020-07-28T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2073, - "high": 2073, - "low": 13, - "open": 5856, - "volume": 737979 - }, - "id": 2677623, - "non_hive": { - "close": 500, - "high": 500, - "low": 3, - "open": 1405, - "volume": 177346 - }, - "open": "2020-07-28T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2036, - "high": 2036, - "low": 2658, - "open": 2070, - "volume": 2693842 - }, - "id": 2677668, - "non_hive": { - "close": 500, - "high": 500, - "low": 637, - "open": 500, - "volume": 656913 - }, - "open": "2020-07-29T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1199, - "high": 117, - "low": 86, - "open": 1744, - "volume": 17488 - }, - "id": 2677720, - "non_hive": { - "close": 295, - "high": 29, - "low": 21, - "open": 429, - "volume": 4301 - }, - "open": "2020-07-29T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2009, - "high": 40, - "low": 2009, - "open": 2422, - "volume": 409163 - }, - "id": 2677742, - "non_hive": { - "close": 494, - "high": 10, - "low": 494, - "open": 596, - "volume": 100871 - }, - "open": "2020-07-29T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40994, - "high": 5575, - "low": 8579, - "open": 8128, - "volume": 689439 - }, - "id": 2677760, - "non_hive": { - "close": 10162, - "high": 1382, - "low": 2107, - "open": 2000, - "volume": 170814 - }, - "open": "2020-07-29T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50000, - "high": 100, - "low": 605, - "open": 100, - "volume": 506471 - }, - "id": 2677781, - "non_hive": { - "close": 12160, - "high": 25, - "low": 145, - "open": 25, - "volume": 122977 - }, - "open": "2020-07-29T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45, - "high": 45, - "low": 164341, - "open": 11705, - "volume": 194587 - }, - "id": 2677814, - "non_hive": { - "close": 11, - "high": 11, - "low": 40000, - "open": 2849, - "volume": 47362 - }, - "open": "2020-07-29T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 65290, - "high": 270, - "low": 65290, - "open": 4568, - "volume": 3114787 - }, - "id": 2677829, - "non_hive": { - "close": 15800, - "high": 66, - "low": 15800, - "open": 1112, - "volume": 756912 - }, - "open": "2020-07-29T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24177, - "high": 967, - "low": 243, - "open": 6889, - "volume": 49228 - }, - "id": 2677850, - "non_hive": { - "close": 5895, - "high": 236, - "low": 59, - "open": 1679, - "volume": 12002 - }, - "open": "2020-07-29T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13141, - "high": 57, - "low": 2487, - "open": 6492, - "volume": 32476 - }, - "id": 2677874, - "non_hive": { - "close": 3202, - "high": 14, - "low": 597, - "open": 1582, - "volume": 7890 - }, - "open": "2020-07-29T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11173, - "high": 201, - "low": 2080, - "open": 2487, - "volume": 827516 - }, - "id": 2677892, - "non_hive": { - "close": 2723, - "high": 49, - "low": 500, - "open": 606, - "volume": 201627 - }, - "open": "2020-07-29T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3374, - "high": 299, - "low": 1961, - "open": 622, - "volume": 264402 - }, - "id": 2677916, - "non_hive": { - "close": 822, - "high": 73, - "low": 472, - "open": 151, - "volume": 64358 - }, - "open": "2020-07-29T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2520, - "high": 237, - "low": 703209, - "open": 3943, - "volume": 1995349 - }, - "id": 2677949, - "non_hive": { - "close": 614, - "high": 58, - "low": 169467, - "open": 960, - "volume": 484523 - }, - "open": "2020-07-29T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5610, - "high": 4, - "low": 3188, - "open": 22257, - "volume": 222893 - }, - "id": 2677983, - "non_hive": { - "close": 1368, - "high": 1, - "low": 777, - "open": 5428, - "volume": 54356 - }, - "open": "2020-07-29T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3091, - "high": 32, - "low": 117, - "open": 269424, - "volume": 668507 - }, - "id": 2678015, - "non_hive": { - "close": 754, - "high": 8, - "low": 28, - "open": 65699, - "volume": 163023 - }, - "open": "2020-07-29T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4399, - "high": 127, - "low": 103, - "open": 103, - "volume": 213621 - }, - "id": 2678040, - "non_hive": { - "close": 1073, - "high": 31, - "low": 25, - "open": 25, - "volume": 52095 - }, - "open": "2020-07-29T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 537, - "low": 13, - "open": 13097, - "volume": 251976 - }, - "id": 2678072, - "non_hive": { - "close": 3, - "high": 131, - "low": 3, - "open": 3194, - "volume": 61447 - }, - "open": "2020-07-29T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 139424, - "high": 53, - "low": 29, - "open": 53, - "volume": 212457 - }, - "id": 2678111, - "non_hive": { - "close": 34004, - "high": 13, - "low": 7, - "open": 13, - "volume": 51814 - }, - "open": "2020-07-29T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24689, - "high": 217, - "low": 1006, - "open": 5400, - "volume": 283027 - }, - "id": 2678135, - "non_hive": { - "close": 6023, - "high": 53, - "low": 245, - "open": 1317, - "volume": 69034 - }, - "open": "2020-07-29T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7064, - "high": 3889, - "low": 28611, - "open": 28611, - "volume": 789047 - }, - "id": 2678172, - "non_hive": { - "close": 1758, - "high": 968, - "low": 6980, - "open": 6980, - "volume": 194196 - }, - "open": "2020-07-29T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3441, - "high": 36, - "low": 30680, - "open": 30680, - "volume": 3030699 - }, - "id": 2678218, - "non_hive": { - "close": 855, - "high": 9, - "low": 7582, - "open": 7582, - "volume": 749659 - }, - "open": "2020-07-29T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 84661, - "high": 32, - "low": 3523, - "open": 3523, - "volume": 203674 - }, - "id": 2678253, - "non_hive": { - "close": 21038, - "high": 8, - "low": 875, - "open": 875, - "volume": 50614 - }, - "open": "2020-07-29T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31072, - "high": 28, - "low": 33208, - "open": 8064, - "volume": 449239 - }, - "id": 2678294, - "non_hive": { - "close": 7768, - "high": 7, - "low": 8252, - "open": 2004, - "volume": 111874 - }, - "open": "2020-07-29T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 710, - "high": 710, - "low": 28, - "open": 3322, - "volume": 635769 - }, - "id": 2678333, - "non_hive": { - "close": 179, - "high": 179, - "low": 7, - "open": 837, - "volume": 160143 - }, - "open": "2020-07-29T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 666, - "low": 321, - "open": 22967, - "volume": 108481 - }, - "id": 2678357, - "non_hive": { - "close": 3, - "high": 168, - "low": 80, - "open": 5785, - "volume": 27324 - }, - "open": "2020-07-29T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 125869, - "high": 31, - "low": 222, - "open": 183774, - "volume": 1733814 - }, - "id": 2678382, - "non_hive": { - "close": 31215, - "high": 8, - "low": 55, - "open": 46289, - "volume": 431854 - }, - "open": "2020-07-30T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 24, - "low": 43214, - "open": 1445, - "volume": 177316 - }, - "id": 2678424, - "non_hive": { - "close": 6, - "high": 6, - "low": 10673, - "open": 359, - "volume": 43908 - }, - "open": "2020-07-30T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3000, - "high": 1154, - "low": 57050, - "open": 1154, - "volume": 233100 - }, - "id": 2678452, - "non_hive": { - "close": 732, - "high": 287, - "low": 13911, - "open": 287, - "volume": 57141 - }, - "open": "2020-07-30T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11963, - "high": 11963, - "low": 6187, - "open": 1000, - "volume": 195291 - }, - "id": 2678470, - "non_hive": { - "close": 2943, - "high": 2943, - "low": 1515, - "open": 246, - "volume": 47973 - }, - "open": "2020-07-30T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4807, - "high": 4195, - "low": 15617, - "open": 8139, - "volume": 103065 - }, - "id": 2678492, - "non_hive": { - "close": 1182, - "high": 1032, - "low": 3824, - "open": 1999, - "volume": 25318 - }, - "open": "2020-07-30T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2596, - "high": 410, - "low": 2596, - "open": 8135, - "volume": 2033161 - }, - "id": 2678512, - "non_hive": { - "close": 623, - "high": 101, - "low": 623, - "open": 1999, - "volume": 491754 - }, - "open": "2020-07-30T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5871, - "high": 1787, - "low": 82, - "open": 4167, - "volume": 349114 - }, - "id": 2678548, - "non_hive": { - "close": 1429, - "high": 438, - "low": 19, - "open": 1000, - "volume": 84470 - }, - "open": "2020-07-30T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1755, - "high": 4, - "low": 40, - "open": 2052, - "volume": 1174014 - }, - "id": 2678580, - "non_hive": { - "close": 429, - "high": 1, - "low": 9, - "open": 499, - "volume": 284569 - }, - "open": "2020-07-30T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2635, - "high": 24, - "low": 97, - "open": 79649, - "volume": 562513 - }, - "id": 2678625, - "non_hive": { - "close": 628, - "high": 6, - "low": 23, - "open": 19351, - "volume": 135535 - }, - "open": "2020-07-30T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4140, - "high": 128, - "low": 6800, - "open": 8387, - "volume": 88013 - }, - "id": 2678653, - "non_hive": { - "close": 1000, - "high": 31, - "low": 1618, - "open": 2000, - "volume": 21120 - }, - "open": "2020-07-30T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6400, - "high": 78, - "low": 6400, - "open": 78, - "volume": 14811 - }, - "id": 2678679, - "non_hive": { - "close": 1536, - "high": 19, - "low": 1536, - "open": 19, - "volume": 3555 - }, - "open": "2020-07-30T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 58181, - "high": 762, - "low": 216, - "open": 216, - "volume": 1515390 - }, - "id": 2678689, - "non_hive": { - "close": 13964, - "high": 184, - "low": 51, - "open": 51, - "volume": 363225 - }, - "open": "2020-07-30T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 471, - "high": 33, - "low": 122, - "open": 5617, - "volume": 246140 - }, - "id": 2678738, - "non_hive": { - "close": 113, - "high": 8, - "low": 28, - "open": 1348, - "volume": 59041 - }, - "open": "2020-07-30T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2500, - "high": 460, - "low": 40, - "open": 4171, - "volume": 1414828 - }, - "id": 2678773, - "non_hive": { - "close": 592, - "high": 111, - "low": 9, - "open": 1000, - "volume": 340935 - }, - "open": "2020-07-30T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6078, - "high": 211, - "low": 97, - "open": 5941, - "volume": 425010 - }, - "id": 2678810, - "non_hive": { - "close": 1466, - "high": 51, - "low": 22, - "open": 1408, - "volume": 102208 - }, - "open": "2020-07-30T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 1250, - "low": 13, - "open": 8398, - "volume": 162252 - }, - "id": 2678870, - "non_hive": { - "close": 3, - "high": 302, - "low": 3, - "open": 2000, - "volume": 38976 - }, - "open": "2020-07-30T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16764, - "high": 161, - "low": 30, - "open": 58, - "volume": 1615710 - }, - "id": 2678909, - "non_hive": { - "close": 4047, - "high": 39, - "low": 7, - "open": 14, - "volume": 387992 - }, - "open": "2020-07-30T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11940, - "high": 343, - "low": 708241, - "open": 343, - "volume": 1359305 - }, - "id": 2678954, - "non_hive": { - "close": 2882, - "high": 83, - "low": 169091, - "open": 83, - "volume": 325235 - }, - "open": "2020-07-30T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 76792, - "high": 5361, - "low": 76792, - "open": 5361, - "volume": 111040 - }, - "id": 2678995, - "non_hive": { - "close": 18500, - "high": 1294, - "low": 18500, - "open": 1294, - "volume": 26757 - }, - "open": "2020-07-30T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12057, - "high": 195, - "low": 9, - "open": 195, - "volume": 4151635 - }, - "id": 2679014, - "non_hive": { - "close": 2821, - "high": 47, - "low": 2, - "open": 47, - "volume": 973385 - }, - "open": "2020-07-30T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4609, - "high": 191, - "low": 2321, - "open": 2321, - "volume": 22664 - }, - "id": 2679073, - "non_hive": { - "close": 1105, - "high": 46, - "low": 543, - "open": 543, - "volume": 5431 - }, - "open": "2020-07-30T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 105, - "high": 3861, - "low": 53, - "open": 1276, - "volume": 129250 - }, - "id": 2679092, - "non_hive": { - "close": 24, - "high": 925, - "low": 12, - "open": 305, - "volume": 30857 - }, - "open": "2020-07-30T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3169, - "high": 47265, - "low": 26, - "open": 47265, - "volume": 113048 - }, - "id": 2679125, - "non_hive": { - "close": 754, - "high": 11269, - "low": 6, - "open": 11269, - "volume": 26931 - }, - "open": "2020-07-30T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12562, - "high": 196, - "low": 24, - "open": 4205, - "volume": 695691 - }, - "id": 2679154, - "non_hive": { - "close": 3000, - "high": 47, - "low": 5, - "open": 1000, - "volume": 165896 - }, - "open": "2020-07-30T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5688, - "high": 347, - "low": 277, - "open": 716, - "volume": 8553 - }, - "id": 2679198, - "non_hive": { - "close": 1357, - "high": 83, - "low": 65, - "open": 171, - "volume": 2040 - }, - "open": "2020-07-31T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16007, - "high": 247884, - "low": 172, - "open": 1576, - "volume": 957145 - }, - "id": 2679213, - "non_hive": { - "close": 3883, - "high": 60209, - "low": 40, - "open": 377, - "volume": 230226 - }, - "open": "2020-07-31T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1860, - "high": 840, - "low": 157, - "open": 52454, - "volume": 144538 - }, - "id": 2679245, - "non_hive": { - "close": 437, - "high": 204, - "low": 36, - "open": 12726, - "volume": 35021 - }, - "open": "2020-07-31T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2945, - "high": 2945, - "low": 3000, - "open": 8499, - "volume": 83895 - }, - "id": 2679268, - "non_hive": { - "close": 701, - "high": 701, - "low": 705, - "open": 2000, - "volume": 19874 - }, - "open": "2020-07-31T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22042, - "high": 50, - "low": 16477, - "open": 5924, - "volume": 1120767 - }, - "id": 2679286, - "non_hive": { - "close": 5246, - "high": 12, - "low": 3921, - "open": 1410, - "volume": 266761 - }, - "open": "2020-07-31T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7389, - "high": 5617, - "low": 7389, - "open": 14381, - "volume": 104366 - }, - "id": 2679321, - "non_hive": { - "close": 1758, - "high": 1337, - "low": 1758, - "open": 3423, - "volume": 24836 - }, - "open": "2020-07-31T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43924, - "high": 2386, - "low": 2279, - "open": 2279, - "volume": 158320 - }, - "id": 2679348, - "non_hive": { - "close": 10454, - "high": 568, - "low": 542, - "open": 542, - "volume": 37682 - }, - "open": "2020-07-31T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1461, - "high": 8, - "low": 80, - "open": 8470, - "volume": 536631 - }, - "id": 2679364, - "non_hive": { - "close": 346, - "high": 2, - "low": 18, - "open": 2016, - "volume": 126719 - }, - "open": "2020-07-31T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 422092, - "high": 25, - "low": 422092, - "open": 12122, - "volume": 1489790 - }, - "id": 2679391, - "non_hive": { - "close": 98856, - "high": 6, - "low": 98856, - "open": 2879, - "volume": 350833 - }, - "open": "2020-07-31T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 491492, - "high": 113, - "low": 73, - "open": 11967, - "volume": 765916 - }, - "id": 2679431, - "non_hive": { - "close": 115510, - "high": 27, - "low": 17, - "open": 2839, - "volume": 180519 - }, - "open": "2020-07-31T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38547, - "high": 105, - "low": 38547, - "open": 36509, - "volume": 565294 - }, - "id": 2679467, - "non_hive": { - "close": 9027, - "high": 25, - "low": 9027, - "open": 8645, - "volume": 132867 - }, - "open": "2020-07-31T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 796, - "high": 63, - "low": 8459, - "open": 8459, - "volume": 93383 - }, - "id": 2679491, - "non_hive": { - "close": 189, - "high": 15, - "low": 1999, - "open": 1999, - "volume": 22102 - }, - "open": "2020-07-31T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13799, - "high": 13728, - "low": 144, - "open": 289898, - "volume": 909414 - }, - "id": 2679517, - "non_hive": { - "close": 3273, - "high": 3258, - "low": 34, - "open": 68788, - "volume": 215729 - }, - "open": "2020-07-31T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2000, - "high": 67, - "low": 2000, - "open": 5499, - "volume": 170149 - }, - "id": 2679552, - "non_hive": { - "close": 472, - "high": 16, - "low": 472, - "open": 1304, - "volume": 40322 - }, - "open": "2020-07-31T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6707, - "high": 1725, - "low": 103, - "open": 58218, - "volume": 206333 - }, - "id": 2679581, - "non_hive": { - "close": 1590, - "high": 409, - "low": 24, - "open": 13740, - "volume": 48612 - }, - "open": "2020-07-31T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 421, - "high": 2672, - "low": 421, - "open": 2672, - "volume": 326684 - }, - "id": 2679624, - "non_hive": { - "close": 99, - "high": 634, - "low": 98, - "open": 634, - "volume": 77128 - }, - "open": "2020-07-31T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27559, - "high": 3561, - "low": 27, - "open": 13, - "volume": 1523638 - }, - "id": 2679670, - "non_hive": { - "close": 6521, - "high": 844, - "low": 6, - "open": 3, - "volume": 356197 - }, - "open": "2020-07-31T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13570, - "high": 59, - "low": 49, - "open": 8458, - "volume": 3321712 - }, - "id": 2679723, - "non_hive": { - "close": 3193, - "high": 14, - "low": 11, - "open": 1999, - "volume": 769186 - }, - "open": "2020-07-31T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49161, - "high": 174, - "low": 20, - "open": 779, - "volume": 397237 - }, - "id": 2679784, - "non_hive": { - "close": 11306, - "high": 41, - "low": 4, - "open": 183, - "volume": 92259 - }, - "open": "2020-07-31T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21, - "high": 21, - "low": 21805, - "open": 100, - "volume": 320339 - }, - "id": 2679844, - "non_hive": { - "close": 5, - "high": 5, - "low": 5001, - "open": 23, - "volume": 73498 - }, - "open": "2020-07-31T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3808, - "high": 4457, - "low": 25736, - "open": 8723, - "volume": 126074 - }, - "id": 2679881, - "non_hive": { - "close": 873, - "high": 1022, - "low": 5900, - "open": 2000, - "volume": 28905 - }, - "open": "2020-07-31T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36348, - "high": 604, - "low": 8759, - "open": 24580, - "volume": 345025 - }, - "id": 2679908, - "non_hive": { - "close": 8467, - "high": 141, - "low": 1997, - "open": 5635, - "volume": 79336 - }, - "open": "2020-07-31T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12143, - "high": 948, - "low": 27, - "open": 3715, - "volume": 390001 - }, - "id": 2679941, - "non_hive": { - "close": 2823, - "high": 221, - "low": 6, - "open": 865, - "volume": 90689 - }, - "open": "2020-07-31T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 458, - "high": 140, - "low": 14, - "open": 7709, - "volume": 1019094 - }, - "id": 2679967, - "non_hive": { - "close": 106, - "high": 33, - "low": 3, - "open": 1789, - "volume": 239610 - }, - "open": "2020-07-31T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127, - "high": 714, - "low": 57, - "open": 39, - "volume": 3052395 - }, - "id": 2680001, - "non_hive": { - "close": 28, - "high": 167, - "low": 12, - "open": 9, - "volume": 697844 - }, - "open": "2020-08-01T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 121648, - "high": 116, - "low": 85, - "open": 8610, - "volume": 2030757 - }, - "id": 2680043, - "non_hive": { - "close": 27498, - "high": 27, - "low": 19, - "open": 1999, - "volume": 460285 - }, - "open": "2020-08-01T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 591126, - "high": 1876, - "low": 12, - "open": 8846, - "volume": 3080395 - }, - "id": 2680074, - "non_hive": { - "close": 133122, - "high": 432, - "low": 2, - "open": 2000, - "volume": 694769 - }, - "open": "2020-08-01T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2731, - "high": 74, - "low": 111236, - "open": 2524, - "volume": 1209590 - }, - "id": 2680105, - "non_hive": { - "close": 621, - "high": 17, - "low": 25050, - "open": 574, - "volume": 272429 - }, - "open": "2020-08-01T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36917, - "high": 26, - "low": 8792, - "open": 5995, - "volume": 303155 - }, - "id": 2680117, - "non_hive": { - "close": 8373, - "high": 6, - "low": 1993, - "open": 1360, - "volume": 68756 - }, - "open": "2020-08-01T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8827, - "high": 370, - "low": 493274, - "open": 3618, - "volume": 1971836 - }, - "id": 2680148, - "non_hive": { - "close": 1999, - "high": 84, - "low": 111097, - "open": 820, - "volume": 444338 - }, - "open": "2020-08-01T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2983, - "high": 2771, - "low": 637, - "open": 17631, - "volume": 3757862 - }, - "id": 2680185, - "non_hive": { - "close": 676, - "high": 628, - "low": 141, - "open": 3993, - "volume": 843699 - }, - "open": "2020-08-01T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 366, - "high": 52, - "low": 582497, - "open": 10587, - "volume": 3298480 - }, - "id": 2680235, - "non_hive": { - "close": 83, - "high": 12, - "low": 131073, - "open": 2399, - "volume": 742769 - }, - "open": "2020-08-01T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6851, - "high": 8, - "low": 110, - "open": 20588, - "volume": 2179281 - }, - "id": 2680281, - "non_hive": { - "close": 1551, - "high": 2, - "low": 24, - "open": 4665, - "volume": 491120 - }, - "open": "2020-08-01T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3843, - "high": 68794, - "low": 413843, - "open": 8919, - "volume": 2252128 - }, - "id": 2680341, - "non_hive": { - "close": 868, - "high": 15574, - "low": 92559, - "open": 2000, - "volume": 505374 - }, - "open": "2020-08-01T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27135, - "high": 234, - "low": 399468, - "open": 15062, - "volume": 1541809 - }, - "id": 2680383, - "non_hive": { - "close": 6125, - "high": 53, - "low": 89359, - "open": 3398, - "volume": 345551 - }, - "open": "2020-08-01T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3300, - "high": 1477, - "low": 392733, - "open": 7011, - "volume": 2092134 - }, - "id": 2680426, - "non_hive": { - "close": 746, - "high": 334, - "low": 88225, - "open": 1575, - "volume": 470854 - }, - "open": "2020-08-01T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29109, - "high": 242, - "low": 31358, - "open": 8887, - "volume": 2340987 - }, - "id": 2680467, - "non_hive": { - "close": 6602, - "high": 55, - "low": 7045, - "open": 2000, - "volume": 529454 - }, - "open": "2020-08-01T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11759, - "high": 92, - "low": 380925, - "open": 36719, - "volume": 2197424 - }, - "id": 2680519, - "non_hive": { - "close": 2667, - "high": 21, - "low": 85612, - "open": 8328, - "volume": 494965 - }, - "open": "2020-08-01T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 62600, - "high": 9228, - "low": 103, - "open": 9228, - "volume": 3854422 - }, - "id": 2680577, - "non_hive": { - "close": 14179, - "high": 2093, - "low": 23, - "open": 2093, - "volume": 867230 - }, - "open": "2020-08-01T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26759, - "high": 3125, - "low": 32, - "open": 3125, - "volume": 2537458 - }, - "id": 2680623, - "non_hive": { - "close": 6061, - "high": 708, - "low": 7, - "open": 708, - "volume": 569635 - }, - "open": "2020-08-01T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2322, - "high": 22, - "low": 14, - "open": 14, - "volume": 919847 - }, - "id": 2680662, - "non_hive": { - "close": 526, - "high": 5, - "low": 3, - "open": 3, - "volume": 206726 - }, - "open": "2020-08-01T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8733, - "high": 3000, - "low": 249437, - "open": 2491, - "volume": 861417 - }, - "id": 2680715, - "non_hive": { - "close": 1976, - "high": 680, - "low": 55864, - "open": 564, - "volume": 193762 - }, - "open": "2020-08-01T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1279, - "high": 1279, - "low": 386492, - "open": 251583, - "volume": 2169708 - }, - "id": 2680749, - "non_hive": { - "close": 290, - "high": 290, - "low": 86707, - "open": 56925, - "volume": 489010 - }, - "open": "2020-08-01T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16412, - "high": 317, - "low": 5, - "open": 596, - "volume": 126051 - }, - "id": 2680788, - "non_hive": { - "close": 3719, - "high": 72, - "low": 1, - "open": 135, - "volume": 28540 - }, - "open": "2020-08-01T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2290, - "high": 185, - "low": 69226, - "open": 11007, - "volume": 1872553 - }, - "id": 2680832, - "non_hive": { - "close": 519, - "high": 42, - "low": 15685, - "open": 2494, - "volume": 424314 - }, - "open": "2020-08-01T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16840, - "high": 8, - "low": 30524, - "open": 79, - "volume": 759025 - }, - "id": 2680882, - "non_hive": { - "close": 3816, - "high": 2, - "low": 6867, - "open": 18, - "volume": 171925 - }, - "open": "2020-08-01T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46242, - "high": 4002, - "low": 27, - "open": 11850, - "volume": 1924536 - }, - "id": 2680921, - "non_hive": { - "close": 10478, - "high": 907, - "low": 6, - "open": 2685, - "volume": 433269 - }, - "open": "2020-08-01T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12586, - "high": 66, - "low": 14, - "open": 12265, - "volume": 274574 - }, - "id": 2680955, - "non_hive": { - "close": 2852, - "high": 15, - "low": 3, - "open": 2779, - "volume": 62218 - }, - "open": "2020-08-01T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 323, - "high": 450, - "low": 11596, - "open": 10591, - "volume": 1113371 - }, - "id": 2680997, - "non_hive": { - "close": 73, - "high": 102, - "low": 2600, - "open": 2400, - "volume": 252180 - }, - "open": "2020-08-02T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19037, - "high": 639, - "low": 8697, - "open": 508, - "volume": 475341 - }, - "id": 2681027, - "non_hive": { - "close": 4283, - "high": 145, - "low": 1956, - "open": 115, - "volume": 107249 - }, - "open": "2020-08-02T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6597, - "high": 2210, - "low": 2036, - "open": 47092, - "volume": 1367416 - }, - "id": 2681079, - "non_hive": { - "close": 1495, - "high": 501, - "low": 461, - "open": 10671, - "volume": 309855 - }, - "open": "2020-08-02T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11191, - "high": 8623, - "low": 4244, - "open": 14280, - "volume": 240592 - }, - "id": 2681111, - "non_hive": { - "close": 2540, - "high": 1983, - "low": 961, - "open": 3236, - "volume": 54581 - }, - "open": "2020-08-02T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 371933, - "high": 130865, - "low": 62, - "open": 8698, - "volume": 1420465 - }, - "id": 2681133, - "non_hive": { - "close": 84800, - "high": 30559, - "low": 14, - "open": 2000, - "volume": 324993 - }, - "open": "2020-08-02T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8460, - "high": 21, - "low": 6, - "open": 8767, - "volume": 5839996 - }, - "id": 2681163, - "non_hive": { - "close": 1939, - "high": 5, - "low": 1, - "open": 2000, - "volume": 1316530 - }, - "open": "2020-08-02T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3412, - "high": 1878, - "low": 28, - "open": 9005, - "volume": 3612985 - }, - "id": 2681206, - "non_hive": { - "close": 779, - "high": 430, - "low": 6, - "open": 2000, - "volume": 802238 - }, - "open": "2020-08-02T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 240995, - "high": 179, - "low": 20, - "open": 8764, - "volume": 5016301 - }, - "id": 2681239, - "non_hive": { - "close": 53524, - "high": 41, - "low": 4, - "open": 1999, - "volume": 1128441 - }, - "open": "2020-08-02T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 122, - "high": 1259, - "low": 122, - "open": 8806, - "volume": 2423972 - }, - "id": 2681303, - "non_hive": { - "close": 26, - "high": 286, - "low": 26, - "open": 1999, - "volume": 537362 - }, - "open": "2020-08-02T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27032, - "high": 855, - "low": 2065, - "open": 9009, - "volume": 76002 - }, - "id": 2681326, - "non_hive": { - "close": 6001, - "high": 190, - "low": 458, - "open": 1999, - "volume": 16871 - }, - "open": "2020-08-02T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4683, - "high": 549, - "low": 337210, - "open": 10063, - "volume": 1054925 - }, - "id": 2681350, - "non_hive": { - "close": 1038, - "high": 122, - "low": 73331, - "open": 2234, - "volume": 231873 - }, - "open": "2020-08-02T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57325, - "high": 8767, - "low": 49, - "open": 9027, - "volume": 2585372 - }, - "id": 2681379, - "non_hive": { - "close": 12326, - "high": 1942, - "low": 10, - "open": 1999, - "volume": 560080 - }, - "open": "2020-08-02T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12670, - "high": 122, - "low": 37, - "open": 5790, - "volume": 1130524 - }, - "id": 2681417, - "non_hive": { - "close": 2773, - "high": 27, - "low": 7, - "open": 1245, - "volume": 245528 - }, - "open": "2020-08-02T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11837, - "high": 169, - "low": 36, - "open": 6156, - "volume": 2293868 - }, - "id": 2681459, - "non_hive": { - "close": 2588, - "high": 37, - "low": 7, - "open": 1347, - "volume": 498676 - }, - "open": "2020-08-02T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4893, - "high": 13411, - "low": 37, - "open": 202, - "volume": 177679 - }, - "id": 2681505, - "non_hive": { - "close": 1067, - "high": 2930, - "low": 7, - "open": 44, - "volume": 38756 - }, - "open": "2020-08-02T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 174, - "high": 348, - "low": 28, - "open": 16433, - "volume": 95563 - }, - "id": 2681543, - "non_hive": { - "close": 38, - "high": 76, - "low": 6, - "open": 3583, - "volume": 20819 - }, - "open": "2020-08-02T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3507, - "high": 419, - "low": 33, - "open": 14, - "volume": 90359 - }, - "id": 2681572, - "non_hive": { - "close": 769, - "high": 92, - "low": 7, - "open": 3, - "volume": 19811 - }, - "open": "2020-08-02T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2348, - "high": 1003, - "low": 2971, - "open": 2971, - "volume": 491436 - }, - "id": 2681604, - "non_hive": { - "close": 515, - "high": 220, - "low": 651, - "open": 651, - "volume": 107751 - }, - "open": "2020-08-02T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 27, - "low": 14444, - "open": 102000, - "volume": 200165 - }, - "id": 2681636, - "non_hive": { - "close": 22, - "high": 6, - "low": 3166, - "open": 22362, - "volume": 43885 - }, - "open": "2020-08-02T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5112, - "high": 13, - "low": 322783, - "open": 5377, - "volume": 1002810 - }, - "id": 2681671, - "non_hive": { - "close": 1121, - "high": 3, - "low": 70774, - "open": 1179, - "volume": 219879 - }, - "open": "2020-08-02T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2047, - "high": 27, - "low": 19165, - "open": 10494, - "volume": 156757 - }, - "id": 2681697, - "non_hive": { - "close": 449, - "high": 6, - "low": 4202, - "open": 2301, - "volume": 34371 - }, - "open": "2020-08-02T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48739, - "high": 250, - "low": 276275, - "open": 11593, - "volume": 887744 - }, - "id": 2681737, - "non_hive": { - "close": 10684, - "high": 55, - "low": 60259, - "open": 2542, - "volume": 194016 - }, - "open": "2020-08-02T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28, - "high": 236, - "low": 28, - "open": 7003, - "volume": 991860 - }, - "id": 2681772, - "non_hive": { - "close": 6, - "high": 52, - "low": 6, - "open": 1535, - "volume": 217716 - }, - "open": "2020-08-02T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22, - "high": 22, - "low": 14, - "open": 26844, - "volume": 409718 - }, - "id": 2681807, - "non_hive": { - "close": 5, - "high": 5, - "low": 3, - "open": 5893, - "volume": 89240 - }, - "open": "2020-08-02T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27409, - "high": 82, - "low": 277, - "open": 277, - "volume": 67026 - }, - "id": 2681831, - "non_hive": { - "close": 6008, - "high": 18, - "low": 60, - "open": 60, - "volume": 14690 - }, - "open": "2020-08-03T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14782, - "high": 18052, - "low": 1209513, - "open": 1209513, - "volume": 2233785 - }, - "id": 2681850, - "non_hive": { - "close": 3295, - "high": 4024, - "low": 265093, - "open": 265093, - "volume": 490475 - }, - "open": "2020-08-03T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 250867, - "high": 56302, - "low": 99, - "open": 9029, - "volume": 2493393 - }, - "id": 2681868, - "non_hive": { - "close": 54943, - "high": 12490, - "low": 21, - "open": 2000, - "volume": 545222 - }, - "open": "2020-08-03T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7862, - "high": 2442, - "low": 8533, - "open": 1150, - "volume": 85217 - }, - "id": 2681888, - "non_hive": { - "close": 1751, - "high": 544, - "low": 1844, - "open": 255, - "volume": 18825 - }, - "open": "2020-08-03T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2759, - "high": 1211, - "low": 299695, - "open": 8977, - "volume": 2658544 - }, - "id": 2681909, - "non_hive": { - "close": 615, - "high": 270, - "low": 65934, - "open": 1999, - "volume": 587523 - }, - "open": "2020-08-03T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 380160, - "high": 619, - "low": 380160, - "open": 619, - "volume": 2468977 - }, - "id": 2681940, - "non_hive": { - "close": 83274, - "high": 138, - "low": 83274, - "open": 138, - "volume": 540983 - }, - "open": "2020-08-03T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 198, - "high": 17, - "low": 12, - "open": 8983, - "volume": 506206 - }, - "id": 2681976, - "non_hive": { - "close": 44, - "high": 4, - "low": 2, - "open": 1999, - "volume": 112702 - }, - "open": "2020-08-03T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1158, - "high": 161, - "low": 7, - "open": 48551, - "volume": 835946 - }, - "id": 2682004, - "non_hive": { - "close": 258, - "high": 36, - "low": 1, - "open": 10705, - "volume": 184396 - }, - "open": "2020-08-03T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1973, - "high": 408, - "low": 16259, - "open": 5049, - "volume": 80788 - }, - "id": 2682042, - "non_hive": { - "close": 440, - "high": 91, - "low": 3622, - "open": 1125, - "volume": 17999 - }, - "open": "2020-08-03T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7238, - "high": 565, - "low": 1608, - "open": 224523, - "volume": 447759 - }, - "id": 2682057, - "non_hive": { - "close": 1612, - "high": 126, - "low": 358, - "open": 49999, - "volume": 99713 - }, - "open": "2020-08-03T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2133, - "high": 278, - "low": 73757, - "open": 7853, - "volume": 173080 - }, - "id": 2682078, - "non_hive": { - "close": 475, - "high": 62, - "low": 16424, - "open": 1749, - "volume": 38542 - }, - "open": "2020-08-03T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23255, - "high": 543, - "low": 23255, - "open": 10000, - "volume": 252825 - }, - "id": 2682102, - "non_hive": { - "close": 5178, - "high": 121, - "low": 5178, - "open": 2227, - "volume": 56298 - }, - "open": "2020-08-03T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16369, - "high": 2375, - "low": 22140, - "open": 2375, - "volume": 52793 - }, - "id": 2682130, - "non_hive": { - "close": 3645, - "high": 529, - "low": 4930, - "open": 529, - "volume": 11756 - }, - "open": "2020-08-03T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8097, - "high": 7587, - "low": 21, - "open": 13006, - "volume": 1882626 - }, - "id": 2682143, - "non_hive": { - "close": 1804, - "high": 1691, - "low": 4, - "open": 2896, - "volume": 416471 - }, - "open": "2020-08-03T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22, - "high": 597, - "low": 22, - "open": 21593, - "volume": 1539237 - }, - "id": 2682177, - "non_hive": { - "close": 4, - "high": 135, - "low": 4, - "open": 4810, - "volume": 341725 - }, - "open": "2020-08-03T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2286, - "high": 1284, - "low": 32, - "open": 1284, - "volume": 35177 - }, - "id": 2682222, - "non_hive": { - "close": 516, - "high": 290, - "low": 7, - "open": 290, - "volume": 7942 - }, - "open": "2020-08-03T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9051, - "high": 22, - "low": 5, - "open": 14, - "volume": 4745759 - }, - "id": 2682243, - "non_hive": { - "close": 1999, - "high": 5, - "low": 1, - "open": 3, - "volume": 1043733 - }, - "open": "2020-08-03T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 407, - "high": 289, - "low": 4603, - "open": 4842, - "volume": 532810 - }, - "id": 2682306, - "non_hive": { - "close": 90, - "high": 64, - "low": 1016, - "open": 1070, - "volume": 117673 - }, - "open": "2020-08-03T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26573, - "high": 742, - "low": 73, - "open": 294396, - "volume": 437778 - }, - "id": 2682354, - "non_hive": { - "close": 5952, - "high": 167, - "low": 16, - "open": 65050, - "volume": 97185 - }, - "open": "2020-08-03T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29855, - "high": 29855, - "low": 24535, - "open": 6732, - "volume": 1347807 - }, - "id": 2682387, - "non_hive": { - "close": 6746, - "high": 6746, - "low": 5349, - "open": 1489, - "volume": 297878 - }, - "open": "2020-08-03T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 305, - "high": 2979, - "low": 207, - "open": 10000, - "volume": 306909 - }, - "id": 2682424, - "non_hive": { - "close": 68, - "high": 673, - "low": 45, - "open": 2259, - "volume": 68453 - }, - "open": "2020-08-03T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6, - "high": 10052, - "low": 6, - "open": 9088, - "volume": 412236 - }, - "id": 2682471, - "non_hive": { - "close": 1, - "high": 2241, - "low": 1, - "open": 2000, - "volume": 91770 - }, - "open": "2020-08-03T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28, - "high": 53, - "low": 28, - "open": 6400, - "volume": 92678 - }, - "id": 2682496, - "non_hive": { - "close": 6, - "high": 12, - "low": 6, - "open": 1424, - "volume": 20721 - }, - "open": "2020-08-03T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 357, - "low": 14, - "open": 30693, - "volume": 1850579 - }, - "id": 2682518, - "non_hive": { - "close": 3, - "high": 80, - "low": 3, - "open": 6870, - "volume": 409677 - }, - "open": "2020-08-03T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 138, - "high": 58, - "low": 138, - "open": 177, - "volume": 423082 - }, - "id": 2682548, - "non_hive": { - "close": 30, - "high": 13, - "low": 30, - "open": 39, - "volume": 94707 - }, - "open": "2020-08-04T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 134015, - "high": 8880, - "low": 87, - "open": 44670, - "volume": 1450401 - }, - "id": 2682577, - "non_hive": { - "close": 30000, - "high": 1988, - "low": 19, - "open": 10000, - "volume": 320341 - }, - "open": "2020-08-04T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1032, - "high": 31, - "low": 5, - "open": 26807, - "volume": 2453386 - }, - "id": 2682600, - "non_hive": { - "close": 231, - "high": 7, - "low": 1, - "open": 6001, - "volume": 540455 - }, - "open": "2020-08-04T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 778061, - "high": 4, - "low": 167457, - "open": 7528, - "volume": 2560254 - }, - "id": 2682628, - "non_hive": { - "close": 171318, - "high": 1, - "low": 36868, - "open": 1684, - "volume": 564309 - }, - "open": "2020-08-04T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9387, - "high": 120, - "low": 959870, - "open": 8934, - "volume": 4848515 - }, - "id": 2682653, - "non_hive": { - "close": 2093, - "high": 27, - "low": 210293, - "open": 1999, - "volume": 1066666 - }, - "open": "2020-08-04T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 927, - "high": 1255, - "low": 927, - "open": 2789, - "volume": 179426 - }, - "id": 2682684, - "non_hive": { - "close": 203, - "high": 281, - "low": 203, - "open": 624, - "volume": 39970 - }, - "open": "2020-08-04T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111, - "high": 4481, - "low": 111, - "open": 7525, - "volume": 1499531 - }, - "id": 2682699, - "non_hive": { - "close": 24, - "high": 1003, - "low": 24, - "open": 1683, - "volume": 330696 - }, - "open": "2020-08-04T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16755, - "high": 53, - "low": 364, - "open": 413167, - "volume": 2137032 - }, - "id": 2682745, - "non_hive": { - "close": 3750, - "high": 12, - "low": 80, - "open": 92397, - "volume": 474544 - }, - "open": "2020-08-04T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8977, - "high": 18844, - "low": 58, - "open": 18844, - "volume": 1349195 - }, - "id": 2682776, - "non_hive": { - "close": 2006, - "high": 4217, - "low": 12, - "open": 4217, - "volume": 301423 - }, - "open": "2020-08-04T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38845, - "high": 518, - "low": 115, - "open": 9128, - "volume": 5383363 - }, - "id": 2682814, - "non_hive": { - "close": 8692, - "high": 116, - "low": 25, - "open": 2000, - "volume": 1176134 - }, - "open": "2020-08-04T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27189, - "high": 129, - "low": 338, - "open": 7935, - "volume": 1304863 - }, - "id": 2682854, - "non_hive": { - "close": 5924, - "high": 29, - "low": 73, - "open": 1775, - "volume": 285334 - }, - "open": "2020-08-04T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 860143, - "high": 10407, - "low": 6, - "open": 5965, - "volume": 1257330 - }, - "id": 2682886, - "non_hive": { - "close": 186650, - "high": 2323, - "low": 1, - "open": 1331, - "volume": 273401 - }, - "open": "2020-08-04T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9380, - "high": 76, - "low": 113, - "open": 9036, - "volume": 1499576 - }, - "id": 2682915, - "non_hive": { - "close": 2042, - "high": 17, - "low": 24, - "open": 1999, - "volume": 325987 - }, - "open": "2020-08-04T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 442, - "high": 442, - "low": 106998, - "open": 7906, - "volume": 285442 - }, - "id": 2682941, - "non_hive": { - "close": 97, - "high": 97, - "low": 23430, - "open": 1735, - "volume": 62570 - }, - "open": "2020-08-04T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22756, - "high": 180, - "low": 101, - "open": 1232, - "volume": 377242 - }, - "id": 2682974, - "non_hive": { - "close": 5038, - "high": 40, - "low": 22, - "open": 271, - "volume": 83490 - }, - "open": "2020-08-04T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5464, - "high": 23800, - "low": 28, - "open": 23800, - "volume": 136043 - }, - "id": 2683019, - "non_hive": { - "close": 1209, - "high": 5269, - "low": 6, - "open": 5269, - "volume": 30103 - }, - "open": "2020-08-04T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 3218, - "low": 14, - "open": 14, - "volume": 338687 - }, - "id": 2683054, - "non_hive": { - "close": 2215, - "high": 713, - "low": 3, - "open": 3, - "volume": 74938 - }, - "open": "2020-08-04T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1059, - "high": 412, - "low": 36782, - "open": 36782, - "volume": 458875 - }, - "id": 2683092, - "non_hive": { - "close": 236, - "high": 92, - "low": 8147, - "open": 8147, - "volume": 102204 - }, - "open": "2020-08-04T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 272973, - "high": 59250, - "low": 272973, - "open": 111293, - "volume": 4429894 - }, - "id": 2683125, - "non_hive": { - "close": 59835, - "high": 13269, - "low": 59835, - "open": 24795, - "volume": 981371 - }, - "open": "2020-08-04T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1033, - "high": 80, - "low": 8549, - "open": 6426, - "volume": 301679 - }, - "id": 2683177, - "non_hive": { - "close": 231, - "high": 18, - "low": 1911, - "open": 1439, - "volume": 67508 - }, - "open": "2020-08-04T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2951, - "high": 61, - "low": 2951, - "open": 10195, - "volume": 677093 - }, - "id": 2683222, - "non_hive": { - "close": 659, - "high": 14, - "low": 659, - "open": 2278, - "volume": 153545 - }, - "open": "2020-08-04T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 168, - "high": 5811, - "low": 30, - "open": 18680, - "volume": 5651832 - }, - "id": 2683262, - "non_hive": { - "close": 37, - "high": 1318, - "low": 6, - "open": 4233, - "volume": 1251691 - }, - "open": "2020-08-04T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1171571, - "high": 5439, - "low": 28, - "open": 23380, - "volume": 10121773 - }, - "id": 2683301, - "non_hive": { - "close": 256536, - "high": 1330, - "low": 6, - "open": 5289, - "volume": 2289199 - }, - "open": "2020-08-04T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8965, - "high": 4, - "low": 14, - "open": 358, - "volume": 2759761 - }, - "id": 2683355, - "non_hive": { - "close": 2000, - "high": 1, - "low": 3, - "open": 86, - "volume": 611545 - }, - "open": "2020-08-04T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22422, - "high": 1670, - "low": 352, - "open": 8965, - "volume": 368965 - }, - "id": 2683401, - "non_hive": { - "close": 5000, - "high": 392, - "low": 78, - "open": 2000, - "volume": 82958 - }, - "open": "2020-08-05T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7533, - "high": 500, - "low": 21535, - "open": 1244, - "volume": 1217473 - }, - "id": 2683444, - "non_hive": { - "close": 1731, - "high": 115, - "low": 4740, - "open": 286, - "volume": 268255 - }, - "open": "2020-08-05T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3152, - "high": 16592, - "low": 848, - "open": 16592, - "volume": 38773 - }, - "id": 2683462, - "non_hive": { - "close": 722, - "high": 3816, - "low": 194, - "open": 3816, - "volume": 8895 - }, - "open": "2020-08-05T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 139, - "high": 139, - "low": 207, - "open": 9340, - "volume": 103188 - }, - "id": 2683479, - "non_hive": { - "close": 32, - "high": 32, - "low": 46, - "open": 2139, - "volume": 23622 - }, - "open": "2020-08-05T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3725, - "high": 1537, - "low": 5033, - "open": 7425, - "volume": 617071 - }, - "id": 2683496, - "non_hive": { - "close": 849, - "high": 352, - "low": 1122, - "open": 1699, - "volume": 138313 - }, - "open": "2020-08-05T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6208, - "high": 7604, - "low": 10536, - "open": 7604, - "volume": 1478383 - }, - "id": 2683537, - "non_hive": { - "close": 1397, - "high": 1731, - "low": 2314, - "open": 1731, - "volume": 326134 - }, - "open": "2020-08-05T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 8, - "low": 1505, - "open": 2000, - "volume": 1776348 - }, - "id": 2683576, - "non_hive": { - "close": 3, - "high": 2, - "low": 331, - "open": 450, - "volume": 393607 - }, - "open": "2020-08-05T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26513, - "high": 26, - "low": 5445, - "open": 5963, - "volume": 116675 - }, - "id": 2683642, - "non_hive": { - "close": 6000, - "high": 6, - "low": 1198, - "open": 1353, - "volume": 26313 - }, - "open": "2020-08-05T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7454, - "high": 48, - "low": 204715, - "open": 2294, - "volume": 1843632 - }, - "id": 2683692, - "non_hive": { - "close": 1676, - "high": 11, - "low": 45036, - "open": 519, - "volume": 409529 - }, - "open": "2020-08-05T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 116477, - "high": 4, - "low": 1905, - "open": 22404, - "volume": 337981 - }, - "id": 2683730, - "non_hive": { - "close": 26191, - "high": 1, - "low": 420, - "open": 5037, - "volume": 75952 - }, - "open": "2020-08-05T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2034, - "high": 489, - "low": 141812, - "open": 75711, - "volume": 1506235 - }, - "id": 2683771, - "non_hive": { - "close": 457, - "high": 110, - "low": 31198, - "open": 17018, - "volume": 334122 - }, - "open": "2020-08-05T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 76382, - "high": 3334, - "low": 7117, - "open": 5308, - "volume": 214313 - }, - "id": 2683799, - "non_hive": { - "close": 17178, - "high": 750, - "low": 1600, - "open": 1194, - "volume": 48199 - }, - "open": "2020-08-05T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7460, - "high": 115, - "low": 20000, - "open": 115, - "volume": 82133 - }, - "id": 2683825, - "non_hive": { - "close": 1678, - "high": 26, - "low": 4498, - "open": 26, - "volume": 18473 - }, - "open": "2020-08-05T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45690, - "high": 12440, - "low": 45690, - "open": 15326, - "volume": 365966 - }, - "id": 2683843, - "non_hive": { - "close": 10143, - "high": 2798, - "low": 10143, - "open": 3447, - "volume": 81868 - }, - "open": "2020-08-05T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25452, - "high": 48, - "low": 17103, - "open": 16256, - "volume": 2543317 - }, - "id": 2683882, - "non_hive": { - "close": 5742, - "high": 11, - "low": 3754, - "open": 3609, - "volume": 560249 - }, - "open": "2020-08-05T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7477, - "high": 1235, - "low": 32, - "open": 1213, - "volume": 627155 - }, - "id": 2683923, - "non_hive": { - "close": 1680, - "high": 279, - "low": 7, - "open": 274, - "volume": 141484 - }, - "open": "2020-08-05T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36356, - "high": 6942, - "low": 14, - "open": 6650, - "volume": 2647969 - }, - "id": 2683965, - "non_hive": { - "close": 7998, - "high": 1566, - "low": 3, - "open": 1500, - "volume": 586865 - }, - "open": "2020-08-05T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16083, - "high": 62, - "low": 2000, - "open": 17463, - "volume": 416428 - }, - "id": 2684018, - "non_hive": { - "close": 3586, - "high": 14, - "low": 440, - "open": 3906, - "volume": 92875 - }, - "open": "2020-08-05T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4792, - "high": 8, - "low": 648593, - "open": 96243, - "volume": 1949036 - }, - "id": 2684066, - "non_hive": { - "close": 1074, - "high": 2, - "low": 142690, - "open": 21566, - "volume": 431842 - }, - "open": "2020-08-05T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11405, - "high": 72677, - "low": 5365, - "open": 18246, - "volume": 353204 - }, - "id": 2684109, - "non_hive": { - "close": 2578, - "high": 16439, - "low": 1191, - "open": 4089, - "volume": 79200 - }, - "open": "2020-08-05T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 915, - "high": 915, - "low": 321, - "open": 8626, - "volume": 145729 - }, - "id": 2684152, - "non_hive": { - "close": 207, - "high": 207, - "low": 72, - "open": 1950, - "volume": 32938 - }, - "open": "2020-08-05T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 92, - "low": 426, - "open": 19642, - "volume": 535652 - }, - "id": 2684186, - "non_hive": { - "close": 226, - "high": 21, - "low": 93, - "open": 4439, - "volume": 120950 - }, - "open": "2020-08-05T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28, - "high": 1110, - "low": 28, - "open": 5753, - "volume": 67181 - }, - "id": 2684228, - "non_hive": { - "close": 6, - "high": 251, - "low": 6, - "open": 1300, - "volume": 15184 - }, - "open": "2020-08-05T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 1150, - "low": 14, - "open": 3360, - "volume": 1712827 - }, - "id": 2684254, - "non_hive": { - "close": 3, - "high": 260, - "low": 3, - "open": 756, - "volume": 385789 - }, - "open": "2020-08-05T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14703, - "high": 336, - "low": 277, - "open": 2363, - "volume": 28151 - }, - "id": 2684305, - "non_hive": { - "close": 3322, - "high": 76, - "low": 61, - "open": 534, - "volume": 6359 - }, - "open": "2020-08-06T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4529, - "high": 6173, - "low": 382681, - "open": 8952, - "volume": 1228730 - }, - "id": 2684329, - "non_hive": { - "close": 1022, - "high": 1395, - "low": 84189, - "open": 2000, - "volume": 270869 - }, - "open": "2020-08-06T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10487, - "high": 10487, - "low": 5, - "open": 16660, - "volume": 1303108 - }, - "id": 2684348, - "non_hive": { - "close": 2369, - "high": 2369, - "low": 1, - "open": 3740, - "volume": 287183 - }, - "open": "2020-08-06T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2759, - "high": 637, - "low": 65428, - "open": 30001, - "volume": 201581 - }, - "id": 2684375, - "non_hive": { - "close": 623, - "high": 144, - "low": 14400, - "open": 6777, - "volume": 44874 - }, - "open": "2020-08-06T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 112552, - "high": 8, - "low": 112552, - "open": 79720, - "volume": 1303916 - }, - "id": 2684402, - "non_hive": { - "close": 24648, - "high": 2, - "low": 24648, - "open": 18000, - "volume": 288482 - }, - "open": "2020-08-06T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 141098, - "high": 44, - "low": 32483, - "open": 8879, - "volume": 379848 - }, - "id": 2684419, - "non_hive": { - "close": 30843, - "high": 10, - "low": 7100, - "open": 1999, - "volume": 83862 - }, - "open": "2020-08-06T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9023, - "high": 1076, - "low": 9023, - "open": 1076, - "volume": 10679 - }, - "id": 2684437, - "non_hive": { - "close": 2022, - "high": 242, - "low": 2022, - "open": 242, - "volume": 2394 - }, - "open": "2020-08-06T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5589, - "high": 84, - "low": 1624, - "open": 3785, - "volume": 100718 - }, - "id": 2684447, - "non_hive": { - "close": 1250, - "high": 19, - "low": 363, - "open": 848, - "volume": 22551 - }, - "open": "2020-08-06T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 125159, - "high": 3597, - "low": 458, - "open": 7982, - "volume": 922177 - }, - "id": 2684488, - "non_hive": { - "close": 27359, - "high": 812, - "low": 100, - "open": 1785, - "volume": 203908 - }, - "open": "2020-08-06T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11163, - "high": 57, - "low": 4120, - "open": 4201, - "volume": 123133 - }, - "id": 2684516, - "non_hive": { - "close": 2511, - "high": 13, - "low": 924, - "open": 943, - "volume": 27715 - }, - "open": "2020-08-06T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 75, - "high": 75, - "low": 1563, - "open": 2173, - "volume": 126860 - }, - "id": 2684553, - "non_hive": { - "close": 17, - "high": 17, - "low": 344, - "open": 489, - "volume": 28522 - }, - "open": "2020-08-06T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1862, - "high": 6509, - "low": 1862, - "open": 8479, - "volume": 125244 - }, - "id": 2684583, - "non_hive": { - "close": 418, - "high": 1464, - "low": 409, - "open": 1907, - "volume": 28154 - }, - "open": "2020-08-06T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12593, - "high": 1507, - "low": 18348, - "open": 8062, - "volume": 239453 - }, - "id": 2684620, - "non_hive": { - "close": 2832, - "high": 339, - "low": 4126, - "open": 1813, - "volume": 53850 - }, - "open": "2020-08-06T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4651, - "high": 44, - "low": 4651, - "open": 44, - "volume": 182084 - }, - "id": 2684653, - "non_hive": { - "close": 1044, - "high": 10, - "low": 1044, - "open": 10, - "volume": 40944 - }, - "open": "2020-08-06T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5533, - "high": 70, - "low": 21922, - "open": 349, - "volume": 940917 - }, - "id": 2684698, - "non_hive": { - "close": 1248, - "high": 16, - "low": 4826, - "open": 78, - "volume": 211971 - }, - "open": "2020-08-06T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36992, - "high": 36992, - "low": 32, - "open": 2172, - "volume": 499736 - }, - "id": 2684752, - "non_hive": { - "close": 8349, - "high": 8349, - "low": 7, - "open": 488, - "volume": 112746 - }, - "open": "2020-08-06T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19402, - "high": 44, - "low": 14, - "open": 14, - "volume": 348212 - }, - "id": 2684779, - "non_hive": { - "close": 4401, - "high": 10, - "low": 3, - "open": 3, - "volume": 78642 - }, - "open": "2020-08-06T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8445, - "high": 1449, - "low": 3028, - "open": 10000, - "volume": 76746 - }, - "id": 2684828, - "non_hive": { - "close": 1917, - "high": 329, - "low": 687, - "open": 2270, - "volume": 17420 - }, - "open": "2020-08-06T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4760, - "high": 61, - "low": 4760, - "open": 19425, - "volume": 244007 - }, - "id": 2684848, - "non_hive": { - "close": 1063, - "high": 14, - "low": 1063, - "open": 4409, - "volume": 55260 - }, - "open": "2020-08-06T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13897, - "high": 1381, - "low": 3644, - "open": 3644, - "volume": 69671 - }, - "id": 2684890, - "non_hive": { - "close": 3109, - "high": 313, - "low": 814, - "open": 814, - "volume": 15641 - }, - "open": "2020-08-06T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9183, - "high": 48, - "low": 4286, - "open": 8947, - "volume": 265579 - }, - "id": 2684921, - "non_hive": { - "close": 2103, - "high": 11, - "low": 957, - "open": 2000, - "volume": 60233 - }, - "open": "2020-08-06T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 173378, - "high": 8, - "low": 19394, - "open": 8, - "volume": 248163 - }, - "id": 2684968, - "non_hive": { - "close": 39703, - "high": 2, - "low": 4440, - "open": 2, - "volume": 56826 - }, - "open": "2020-08-06T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27, - "high": 565, - "low": 27, - "open": 7938, - "volume": 65952 - }, - "id": 2684994, - "non_hive": { - "close": 6, - "high": 130, - "low": 6, - "open": 1821, - "volume": 15146 - }, - "open": "2020-08-06T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5213, - "high": 395, - "low": 14, - "open": 20308, - "volume": 993163 - }, - "id": 2685011, - "non_hive": { - "close": 1224, - "high": 93, - "low": 3, - "open": 4667, - "volume": 232835 - }, - "open": "2020-08-06T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6, - "high": 4, - "low": 6, - "open": 13595, - "volume": 109467 - }, - "id": 2685057, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 3194, - "volume": 25706 - }, - "open": "2020-08-07T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1694, - "high": 2264, - "low": 87, - "open": 20793, - "volume": 753010 - }, - "id": 2685094, - "non_hive": { - "close": 398, - "high": 532, - "low": 20, - "open": 4883, - "volume": 176839 - }, - "open": "2020-08-07T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9640, - "high": 821, - "low": 534, - "open": 6126, - "volume": 127879 - }, - "id": 2685120, - "non_hive": { - "close": 2264, - "high": 193, - "low": 125, - "open": 1439, - "volume": 30034 - }, - "open": "2020-08-07T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12737, - "high": 4, - "low": 2182, - "open": 5868, - "volume": 161564 - }, - "id": 2685151, - "non_hive": { - "close": 2991, - "high": 1, - "low": 512, - "open": 1378, - "volume": 37941 - }, - "open": "2020-08-07T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4254, - "high": 6493, - "low": 474, - "open": 3311, - "volume": 116399 - }, - "id": 2685183, - "non_hive": { - "close": 999, - "high": 1525, - "low": 111, - "open": 777, - "volume": 27333 - }, - "open": "2020-08-07T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2673, - "high": 1064, - "low": 11796, - "open": 31452, - "volume": 727873 - }, - "id": 2685207, - "non_hive": { - "close": 628, - "high": 250, - "low": 2770, - "open": 7386, - "volume": 170968 - }, - "open": "2020-08-07T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7079, - "high": 29, - "low": 1117, - "open": 12758, - "volume": 399758 - }, - "id": 2685242, - "non_hive": { - "close": 1663, - "high": 7, - "low": 262, - "open": 2997, - "volume": 93899 - }, - "open": "2020-08-07T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32200, - "high": 1174, - "low": 653, - "open": 20805, - "volume": 1278850 - }, - "id": 2685283, - "non_hive": { - "close": 7567, - "high": 276, - "low": 153, - "open": 4886, - "volume": 300419 - }, - "open": "2020-08-07T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1478, - "high": 1478, - "low": 744, - "open": 16183, - "volume": 2996422 - }, - "id": 2685324, - "non_hive": { - "close": 350, - "high": 350, - "low": 174, - "open": 3803, - "volume": 708594 - }, - "open": "2020-08-07T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1689, - "high": 4, - "low": 3881, - "open": 13899, - "volume": 411007 - }, - "id": 2685348, - "non_hive": { - "close": 400, - "high": 1, - "low": 918, - "open": 3290, - "volume": 97284 - }, - "open": "2020-08-07T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8900, - "high": 25, - "low": 3889, - "open": 20721, - "volume": 352843 - }, - "id": 2685393, - "non_hive": { - "close": 2105, - "high": 6, - "low": 919, - "open": 4904, - "volume": 83477 - }, - "open": "2020-08-07T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59373, - "high": 194, - "low": 360, - "open": 20684, - "volume": 146773 - }, - "id": 2685435, - "non_hive": { - "close": 14054, - "high": 46, - "low": 85, - "open": 4891, - "volume": 34729 - }, - "open": "2020-08-07T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5822, - "high": 1140, - "low": 5822, - "open": 16038, - "volume": 164528 - }, - "id": 2685463, - "non_hive": { - "close": 1377, - "high": 270, - "low": 1377, - "open": 3796, - "volume": 38940 - }, - "open": "2020-08-07T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 774, - "high": 15699, - "low": 2049, - "open": 21080, - "volume": 41651 - }, - "id": 2685496, - "non_hive": { - "close": 183, - "high": 3713, - "low": 481, - "open": 4984, - "volume": 9845 - }, - "open": "2020-08-07T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4618, - "high": 633, - "low": 103, - "open": 8522, - "volume": 1777042 - }, - "id": 2685509, - "non_hive": { - "close": 1093, - "high": 150, - "low": 24, - "open": 2013, - "volume": 420586 - }, - "open": "2020-08-07T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54407, - "high": 8109, - "low": 63, - "open": 21530, - "volume": 1316325 - }, - "id": 2685551, - "non_hive": { - "close": 12881, - "high": 1920, - "low": 14, - "open": 5095, - "volume": 311603 - }, - "open": "2020-08-07T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30190, - "high": 300, - "low": 13, - "open": 13, - "volume": 621262 - }, - "id": 2685599, - "non_hive": { - "close": 7237, - "high": 72, - "low": 3, - "open": 3, - "volume": 147740 - }, - "open": "2020-08-07T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3265, - "high": 1033, - "low": 1196, - "open": 1196, - "volume": 343668 - }, - "id": 2685635, - "non_hive": { - "close": 780, - "high": 247, - "low": 283, - "open": 283, - "volume": 82128 - }, - "open": "2020-08-07T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 146, - "high": 146, - "low": 114, - "open": 8373, - "volume": 115460 - }, - "id": 2685669, - "non_hive": { - "close": 35, - "high": 35, - "low": 27, - "open": 2000, - "volume": 27572 - }, - "open": "2020-08-07T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22281, - "high": 696, - "low": 100, - "open": 696, - "volume": 266999 - }, - "id": 2685686, - "non_hive": { - "close": 5311, - "high": 166, - "low": 23, - "open": 166, - "volume": 63544 - }, - "open": "2020-08-07T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4918, - "high": 347, - "low": 367652, - "open": 8368, - "volume": 1027369 - }, - "id": 2685718, - "non_hive": { - "close": 1174, - "high": 83, - "low": 84563, - "open": 1999, - "volume": 240857 - }, - "open": "2020-08-07T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 562, - "high": 2613, - "low": 5122, - "open": 22339, - "volume": 122338 - }, - "id": 2685753, - "non_hive": { - "close": 134, - "high": 624, - "low": 1185, - "open": 5332, - "volume": 29164 - }, - "open": "2020-08-07T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20952, - "high": 2403, - "low": 504, - "open": 12211, - "volume": 70368 - }, - "id": 2685780, - "non_hive": { - "close": 4995, - "high": 573, - "low": 116, - "open": 2911, - "volume": 16771 - }, - "open": "2020-08-07T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 50, - "low": 13, - "open": 50303, - "volume": 618539 - }, - "id": 2685807, - "non_hive": { - "close": 3, - "high": 12, - "low": 3, - "open": 11992, - "volume": 147452 - }, - "open": "2020-08-07T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3810, - "high": 18586, - "low": 119, - "open": 38, - "volume": 454798 - }, - "id": 2685835, - "non_hive": { - "close": 882, - "high": 4427, - "low": 27, - "open": 9, - "volume": 108282 - }, - "open": "2020-08-08T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21166, - "high": 713, - "low": 3810, - "open": 3810, - "volume": 566919 - }, - "id": 2685854, - "non_hive": { - "close": 5042, - "high": 170, - "low": 907, - "open": 907, - "volume": 135072 - }, - "open": "2020-08-08T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 155, - "high": 155, - "low": 14, - "open": 12600, - "volume": 223658 - }, - "id": 2685874, - "non_hive": { - "close": 37, - "high": 37, - "low": 3, - "open": 3000, - "volume": 53140 - }, - "open": "2020-08-08T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3142, - "high": 8623, - "low": 3142, - "open": 8623, - "volume": 11765 - }, - "id": 2685902, - "non_hive": { - "close": 728, - "high": 2000, - "low": 728, - "open": 2000, - "volume": 2728 - }, - "open": "2020-08-08T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16148, - "high": 16148, - "low": 165, - "open": 8475, - "volume": 244813 - }, - "id": 2685906, - "non_hive": { - "close": 3845, - "high": 3845, - "low": 38, - "open": 1999, - "volume": 57999 - }, - "open": "2020-08-08T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12649, - "high": 26253, - "low": 2050, - "open": 16434, - "volume": 5715904 - }, - "id": 2685928, - "non_hive": { - "close": 3099, - "high": 6432, - "low": 488, - "open": 3913, - "volume": 1367608 - }, - "open": "2020-08-08T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9532, - "high": 2137, - "low": 12099, - "open": 41330, - "volume": 99405 - }, - "id": 2685959, - "non_hive": { - "close": 2341, - "high": 525, - "low": 2964, - "open": 10126, - "volume": 24367 - }, - "open": "2020-08-08T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13780, - "high": 789, - "low": 21452, - "open": 2036, - "volume": 56951 - }, - "id": 2685974, - "non_hive": { - "close": 3384, - "high": 194, - "low": 5268, - "open": 500, - "volume": 13986 - }, - "open": "2020-08-08T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 206610, - "high": 25275, - "low": 206610, - "open": 526, - "volume": 482426 - }, - "id": 2685987, - "non_hive": { - "close": 50000, - "high": 6207, - "low": 50000, - "open": 129, - "volume": 117271 - }, - "open": "2020-08-08T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 867464, - "high": 5462, - "low": 7596, - "open": 5462, - "volume": 1286694 - }, - "id": 2686006, - "non_hive": { - "close": 209927, - "high": 1322, - "low": 1808, - "open": 1322, - "volume": 311349 - }, - "open": "2020-08-08T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24474, - "high": 122, - "low": 16382, - "open": 4425, - "volume": 506576 - }, - "id": 2686038, - "non_hive": { - "close": 6010, - "high": 30, - "low": 3964, - "open": 1071, - "volume": 122837 - }, - "open": "2020-08-08T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8014, - "high": 553, - "low": 21296, - "open": 19665, - "volume": 92451 - }, - "id": 2686067, - "non_hive": { - "close": 1968, - "high": 136, - "low": 5229, - "open": 4829, - "volume": 22703 - }, - "open": "2020-08-08T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15674, - "high": 2121, - "low": 20439, - "open": 2687, - "volume": 191871 - }, - "id": 2686095, - "non_hive": { - "close": 3791, - "high": 521, - "low": 4922, - "open": 660, - "volume": 46565 - }, - "open": "2020-08-08T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1888, - "high": 1888, - "low": 36663, - "open": 967, - "volume": 102640 - }, - "id": 2686123, - "non_hive": { - "close": 457, - "high": 457, - "low": 8870, - "open": 234, - "volume": 24833 - }, - "open": "2020-08-08T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8014, - "high": 40, - "low": 100, - "open": 10832, - "volume": 252458 - }, - "id": 2686150, - "non_hive": { - "close": 1968, - "high": 10, - "low": 24, - "open": 2620, - "volume": 61108 - }, - "open": "2020-08-08T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15140, - "high": 20, - "low": 63, - "open": 57, - "volume": 178117 - }, - "id": 2686186, - "non_hive": { - "close": 3718, - "high": 5, - "low": 15, - "open": 14, - "volume": 43740 - }, - "open": "2020-08-08T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 319562, - "high": 24, - "low": 13, - "open": 24, - "volume": 623519 - }, - "id": 2686230, - "non_hive": { - "close": 78478, - "high": 6, - "low": 3, - "open": 6, - "volume": 153124 - }, - "open": "2020-08-08T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39072, - "high": 10071, - "low": 62902, - "open": 8128, - "volume": 1005468 - }, - "id": 2686277, - "non_hive": { - "close": 10000, - "high": 2578, - "low": 15455, - "open": 2000, - "volume": 249743 - }, - "open": "2020-08-08T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14850, - "high": 382, - "low": 70, - "open": 5543, - "volume": 2573141 - }, - "id": 2686351, - "non_hive": { - "close": 3799, - "high": 98, - "low": 17, - "open": 1413, - "volume": 656547 - }, - "open": "2020-08-08T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6004, - "high": 66, - "low": 4656, - "open": 5254, - "volume": 226123 - }, - "id": 2686401, - "non_hive": { - "close": 1537, - "high": 17, - "low": 1191, - "open": 1345, - "volume": 57881 - }, - "open": "2020-08-08T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3625, - "high": 390, - "low": 23065, - "open": 50220, - "volume": 238800 - }, - "id": 2686444, - "non_hive": { - "close": 928, - "high": 100, - "low": 5904, - "open": 12855, - "volume": 61127 - }, - "open": "2020-08-08T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11772, - "high": 46, - "low": 13011, - "open": 14337, - "volume": 521455 - }, - "id": 2686476, - "non_hive": { - "close": 3010, - "high": 12, - "low": 3259, - "open": 3669, - "volume": 131538 - }, - "open": "2020-08-08T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1761, - "high": 54, - "low": 28, - "open": 1093, - "volume": 179792 - }, - "id": 2686509, - "non_hive": { - "close": 451, - "high": 14, - "low": 7, - "open": 280, - "volume": 45367 - }, - "open": "2020-08-08T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 500, - "high": 1580, - "low": 5, - "open": 20838, - "volume": 617240 - }, - "id": 2686546, - "non_hive": { - "close": 130, - "high": 411, - "low": 1, - "open": 5333, - "volume": 158122 - }, - "open": "2020-08-08T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50000, - "high": 140, - "low": 12, - "open": 12, - "volume": 464898 - }, - "id": 2686585, - "non_hive": { - "close": 13150, - "high": 37, - "low": 3, - "open": 3, - "volume": 121486 - }, - "open": "2020-08-09T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 955, - "high": 955, - "low": 219, - "open": 50000, - "volume": 181838 - }, - "id": 2686620, - "non_hive": { - "close": 283, - "high": 283, - "low": 58, - "open": 13300, - "volume": 48878 - }, - "open": "2020-08-09T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9849, - "high": 17388, - "low": 4, - "open": 1743, - "volume": 34861 - }, - "id": 2686645, - "non_hive": { - "close": 2816, - "high": 4988, - "low": 1, - "open": 500, - "volume": 9985 - }, - "open": "2020-08-09T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14240, - "high": 9640, - "low": 3773, - "open": 8860, - "volume": 313527 - }, - "id": 2686658, - "non_hive": { - "close": 4080, - "high": 2765, - "low": 1080, - "open": 2539, - "volume": 89836 - }, - "open": "2020-08-09T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3534, - "high": 20490, - "low": 289, - "open": 3773, - "volume": 38932 - }, - "id": 2686701, - "non_hive": { - "close": 954, - "high": 5867, - "low": 78, - "open": 1080, - "volume": 11075 - }, - "open": "2020-08-09T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1269, - "high": 780, - "low": 23643, - "open": 1505, - "volume": 92510 - }, - "id": 2686718, - "non_hive": { - "close": 361, - "high": 222, - "low": 6162, - "open": 428, - "volume": 24431 - }, - "open": "2020-08-09T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49508, - "high": 334, - "low": 4353, - "open": 5760, - "volume": 229167 - }, - "id": 2686733, - "non_hive": { - "close": 14054, - "high": 95, - "low": 1230, - "open": 1638, - "volume": 65115 - }, - "open": "2020-08-09T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2554, - "high": 21, - "low": 21748, - "open": 10568, - "volume": 77070 - }, - "id": 2686773, - "non_hive": { - "close": 702, - "high": 6, - "low": 5872, - "open": 2979, - "volume": 21324 - }, - "open": "2020-08-09T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1223, - "high": 7, - "low": 36, - "open": 14573, - "volume": 3303207 - }, - "id": 2686794, - "non_hive": { - "close": 344, - "high": 2, - "low": 9, - "open": 3964, - "volume": 885238 - }, - "open": "2020-08-09T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 912, - "high": 14, - "low": 56285, - "open": 5888, - "volume": 325167 - }, - "id": 2686864, - "non_hive": { - "close": 256, - "high": 4, - "low": 14971, - "open": 1655, - "volume": 89974 - }, - "open": "2020-08-09T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 200000, - "high": 9348, - "low": 1626, - "open": 653, - "volume": 462626 - }, - "id": 2686888, - "non_hive": { - "close": 53400, - "high": 2523, - "low": 432, - "open": 174, - "volume": 123626 - }, - "open": "2020-08-09T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46476, - "high": 622, - "low": 46476, - "open": 15762, - "volume": 158629 - }, - "id": 2686905, - "non_hive": { - "close": 12316, - "high": 174, - "low": 12316, - "open": 4395, - "volume": 42855 - }, - "open": "2020-08-09T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9078, - "high": 9078, - "low": 8, - "open": 4096, - "volume": 47869 - }, - "id": 2686929, - "non_hive": { - "close": 2537, - "high": 2537, - "low": 2, - "open": 1144, - "volume": 13366 - }, - "open": "2020-08-09T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26744, - "high": 3842, - "low": 31, - "open": 7543, - "volume": 135999 - }, - "id": 2686956, - "non_hive": { - "close": 7221, - "high": 1073, - "low": 8, - "open": 2000, - "volume": 36575 - }, - "open": "2020-08-09T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4694, - "high": 4694, - "low": 374881, - "open": 44, - "volume": 4126786 - }, - "id": 2686981, - "non_hive": { - "close": 1293, - "high": 1293, - "low": 93695, - "open": 12, - "volume": 1040173 - }, - "open": "2020-08-09T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 58293, - "high": 15449, - "low": 15666, - "open": 203, - "volume": 130010 - }, - "id": 2687017, - "non_hive": { - "close": 16019, - "high": 4255, - "low": 3870, - "open": 55, - "volume": 34937 - }, - "open": "2020-08-09T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34279, - "high": 18, - "low": 63, - "open": 5000, - "volume": 1463706 - }, - "id": 2687045, - "non_hive": { - "close": 8891, - "high": 5, - "low": 15, - "open": 1372, - "volume": 368561 - }, - "open": "2020-08-09T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8092, - "high": 1242, - "low": 139, - "open": 214, - "volume": 192635 - }, - "id": 2687102, - "non_hive": { - "close": 2096, - "high": 323, - "low": 34, - "open": 54, - "volume": 48543 - }, - "open": "2020-08-09T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 511, - "low": 10, - "open": 4296, - "volume": 1469816 - }, - "id": 2687148, - "non_hive": { - "close": 2, - "high": 131, - "low": 2, - "open": 1100, - "volume": 367296 - }, - "open": "2020-08-09T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1737, - "high": 3, - "low": 7941, - "open": 2318, - "volume": 176003 - }, - "id": 2687181, - "non_hive": { - "close": 439, - "high": 1, - "low": 1997, - "open": 586, - "volume": 44376 - }, - "open": "2020-08-09T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3637, - "high": 237, - "low": 106, - "open": 2693, - "volume": 77988 - }, - "id": 2687222, - "non_hive": { - "close": 917, - "high": 60, - "low": 26, - "open": 680, - "volume": 19682 - }, - "open": "2020-08-09T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10661, - "high": 106581, - "low": 44, - "open": 41410, - "volume": 331444 - }, - "id": 2687251, - "non_hive": { - "close": 2727, - "high": 27263, - "low": 11, - "open": 10435, - "volume": 84225 - }, - "open": "2020-08-09T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4605, - "high": 316, - "low": 28, - "open": 10571, - "volume": 127390 - }, - "id": 2687275, - "non_hive": { - "close": 1178, - "high": 81, - "low": 7, - "open": 2704, - "volume": 32586 - }, - "open": "2020-08-09T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8342, - "high": 35, - "low": 442, - "open": 190866, - "volume": 597145 - }, - "id": 2687303, - "non_hive": { - "close": 2119, - "high": 9, - "low": 111, - "open": 48821, - "volume": 151354 - }, - "open": "2020-08-09T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1521, - "high": 74, - "low": 12, - "open": 12, - "volume": 84232 - }, - "id": 2687328, - "non_hive": { - "close": 386, - "high": 19, - "low": 3, - "open": 3, - "volume": 21393 - }, - "open": "2020-08-10T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18179, - "high": 216, - "low": 4947, - "open": 1001, - "volume": 137370 - }, - "id": 2687352, - "non_hive": { - "close": 4615, - "high": 55, - "low": 1255, - "open": 254, - "volume": 34874 - }, - "open": "2020-08-10T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 73725, - "high": 19, - "low": 14937, - "open": 1469, - "volume": 1469651 - }, - "id": 2687373, - "non_hive": { - "close": 18700, - "high": 5, - "low": 3704, - "open": 373, - "volume": 367162 - }, - "open": "2020-08-10T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 222000, - "high": 222000, - "low": 2287, - "open": 8060, - "volume": 970499 - }, - "id": 2687400, - "non_hive": { - "close": 55508, - "high": 55508, - "low": 567, - "open": 2000, - "volume": 242386 - }, - "open": "2020-08-10T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4983, - "high": 27, - "low": 13, - "open": 7997, - "volume": 3903426 - }, - "id": 2687420, - "non_hive": { - "close": 1264, - "high": 7, - "low": 3, - "open": 2000, - "volume": 976153 - }, - "open": "2020-08-10T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1808, - "high": 27, - "low": 64, - "open": 7889, - "volume": 117055 - }, - "id": 2687452, - "non_hive": { - "close": 458, - "high": 7, - "low": 15, - "open": 1999, - "volume": 29658 - }, - "open": "2020-08-10T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8060, - "high": 47, - "low": 7, - "open": 537, - "volume": 158959 - }, - "id": 2687478, - "non_hive": { - "close": 2000, - "high": 12, - "low": 1, - "open": 136, - "volume": 39605 - }, - "open": "2020-08-10T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2740, - "high": 2740, - "low": 405, - "open": 405, - "volume": 65803 - }, - "id": 2687514, - "non_hive": { - "close": 680, - "high": 680, - "low": 100, - "open": 100, - "volume": 16328 - }, - "open": "2020-08-10T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 382, - "high": 2001, - "low": 13, - "open": 5420, - "volume": 440482 - }, - "id": 2687526, - "non_hive": { - "close": 94, - "high": 503, - "low": 3, - "open": 1345, - "volume": 109938 - }, - "open": "2020-08-10T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45397, - "high": 262, - "low": 10, - "open": 479, - "volume": 190614 - }, - "id": 2687563, - "non_hive": { - "close": 11404, - "high": 66, - "low": 2, - "open": 120, - "volume": 47894 - }, - "open": "2020-08-10T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13135, - "high": 13135, - "low": 716, - "open": 716, - "volume": 855899 - }, - "id": 2687592, - "non_hive": { - "close": 3297, - "high": 3297, - "low": 179, - "open": 179, - "volume": 214730 - }, - "open": "2020-08-10T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30000, - "high": 402, - "low": 471, - "open": 1892, - "volume": 316800 - }, - "id": 2687625, - "non_hive": { - "close": 7528, - "high": 101, - "low": 118, - "open": 475, - "volume": 79499 - }, - "open": "2020-08-10T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13500, - "high": 433, - "low": 523919, - "open": 14681, - "volume": 1879834 - }, - "id": 2687654, - "non_hive": { - "close": 3396, - "high": 109, - "low": 130473, - "open": 3684, - "volume": 470099 - }, - "open": "2020-08-10T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11431, - "high": 682, - "low": 10142, - "open": 7239, - "volume": 376279 - }, - "id": 2687693, - "non_hive": { - "close": 2898, - "high": 173, - "low": 2551, - "open": 1821, - "volume": 94787 - }, - "open": "2020-08-10T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20903, - "high": 543, - "low": 61, - "open": 47, - "volume": 1064040 - }, - "id": 2687730, - "non_hive": { - "close": 5347, - "high": 139, - "low": 15, - "open": 12, - "volume": 270939 - }, - "open": "2020-08-10T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18837, - "high": 261, - "low": 213, - "open": 10242, - "volume": 847493 - }, - "id": 2687774, - "non_hive": { - "close": 4815, - "high": 67, - "low": 53, - "open": 2620, - "volume": 216688 - }, - "open": "2020-08-10T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7901, - "high": 1001, - "low": 12, - "open": 7896, - "volume": 319211 - }, - "id": 2687831, - "non_hive": { - "close": 2000, - "high": 256, - "low": 3, - "open": 2000, - "volume": 81246 - }, - "open": "2020-08-10T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5148, - "high": 82, - "low": 25, - "open": 34952, - "volume": 3287894 - }, - "id": 2687885, - "non_hive": { - "close": 1317, - "high": 21, - "low": 6, - "open": 8847, - "volume": 835205 - }, - "open": "2020-08-10T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 739, - "high": 739, - "low": 4328808, - "open": 2462, - "volume": 4823149 - }, - "id": 2687930, - "non_hive": { - "close": 192, - "high": 192, - "low": 1107309, - "open": 630, - "volume": 1233997 - }, - "open": "2020-08-10T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20720, - "high": 291, - "low": 187, - "open": 500, - "volume": 107638 - }, - "id": 2687966, - "non_hive": { - "close": 5536, - "high": 78, - "low": 48, - "open": 130, - "volume": 28499 - }, - "open": "2020-08-10T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45587, - "high": 32828, - "low": 35290, - "open": 76587, - "volume": 638175 - }, - "id": 2687999, - "non_hive": { - "close": 11761, - "high": 8858, - "low": 9000, - "open": 20464, - "volume": 169745 - }, - "open": "2020-08-10T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11478, - "high": 98, - "low": 37809, - "open": 32762, - "volume": 614117 - }, - "id": 2688049, - "non_hive": { - "close": 3020, - "high": 26, - "low": 9830, - "open": 8680, - "volume": 160870 - }, - "open": "2020-08-10T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3943, - "high": 7, - "low": 5104, - "open": 915, - "volume": 1776253 - }, - "id": 2688103, - "non_hive": { - "close": 1035, - "high": 2, - "low": 1312, - "open": 238, - "volume": 470229 - }, - "open": "2020-08-10T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1716, - "high": 414660, - "low": 20, - "open": 7404, - "volume": 2879243 - }, - "id": 2688150, - "non_hive": { - "close": 450, - "high": 111908, - "low": 5, - "open": 1997, - "volume": 741048 - }, - "open": "2020-08-10T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 122, - "high": 4398, - "low": 12, - "open": 157, - "volume": 1278411 - }, - "id": 2688182, - "non_hive": { - "close": 32, - "high": 1184, - "low": 3, - "open": 41, - "volume": 325035 - }, - "open": "2020-08-11T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 451, - "high": 1792, - "low": 10080, - "open": 1894, - "volume": 241712 - }, - "id": 2688215, - "non_hive": { - "close": 119, - "high": 479, - "low": 2561, - "open": 500, - "volume": 63541 - }, - "open": "2020-08-11T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34612, - "high": 8270, - "low": 4, - "open": 8270, - "volume": 109934 - }, - "id": 2688239, - "non_hive": { - "close": 9132, - "high": 2182, - "low": 1, - "open": 2182, - "volume": 28942 - }, - "open": "2020-08-11T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1209, - "high": 1209, - "low": 1400, - "open": 2767, - "volume": 57090 - }, - "id": 2688262, - "non_hive": { - "close": 319, - "high": 319, - "low": 369, - "open": 730, - "volume": 15055 - }, - "open": "2020-08-11T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1431, - "high": 3462, - "low": 4749, - "open": 3462, - "volume": 275705 - }, - "id": 2688279, - "non_hive": { - "close": 365, - "high": 913, - "low": 1211, - "open": 913, - "volume": 71063 - }, - "open": "2020-08-11T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 379, - "high": 379, - "low": 253, - "open": 521, - "volume": 100551 - }, - "id": 2688294, - "non_hive": { - "close": 100, - "high": 100, - "low": 64, - "open": 133, - "volume": 26271 - }, - "open": "2020-08-11T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 70215, - "high": 21760, - "low": 70215, - "open": 21760, - "volume": 4925537 - }, - "id": 2688327, - "non_hive": { - "close": 17764, - "high": 5737, - "low": 17764, - "open": 5737, - "volume": 1252244 - }, - "open": "2020-08-11T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5774, - "high": 212061, - "low": 530, - "open": 7589, - "volume": 1702412 - }, - "id": 2688354, - "non_hive": { - "close": 1520, - "high": 55878, - "low": 134, - "open": 1999, - "volume": 433902 - }, - "open": "2020-08-11T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24470, - "high": 7, - "low": 446952, - "open": 2451, - "volume": 10866023 - }, - "id": 2688378, - "non_hive": { - "close": 6240, - "high": 2, - "low": 113078, - "open": 625, - "volume": 2758047 - }, - "open": "2020-08-11T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 61, - "high": 30, - "low": 10981, - "open": 1777, - "volume": 7288958 - }, - "id": 2688427, - "non_hive": { - "close": 16, - "high": 8, - "low": 2778, - "open": 453, - "volume": 1844808 - }, - "open": "2020-08-11T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2329, - "high": 15, - "low": 1177762, - "open": 7904, - "volume": 5053977 - }, - "id": 2688466, - "non_hive": { - "close": 594, - "high": 4, - "low": 294446, - "open": 2000, - "volume": 1275917 - }, - "open": "2020-08-11T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4530, - "high": 238, - "low": 667, - "open": 7631, - "volume": 1038753 - }, - "id": 2688528, - "non_hive": { - "close": 1195, - "high": 63, - "low": 170, - "open": 1999, - "volume": 273682 - }, - "open": "2020-08-11T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9421, - "high": 18, - "low": 234760, - "open": 3941, - "volume": 2463785 - }, - "id": 2688569, - "non_hive": { - "close": 2480, - "high": 5, - "low": 58454, - "open": 1038, - "volume": 619379 - }, - "open": "2020-08-11T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41347, - "high": 1407, - "low": 1048391, - "open": 8029, - "volume": 6852203 - }, - "id": 2688605, - "non_hive": { - "close": 10130, - "high": 370, - "low": 251615, - "open": 2000, - "volume": 1672279 - }, - "open": "2020-08-11T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8287, - "high": 115970, - "low": 434, - "open": 11008, - "volume": 764049 - }, - "id": 2688657, - "non_hive": { - "close": 2033, - "high": 30535, - "low": 106, - "open": 2697, - "volume": 193233 - }, - "open": "2020-08-11T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9005, - "high": 110, - "low": 29, - "open": 7599, - "volume": 378100 - }, - "id": 2688701, - "non_hive": { - "close": 2314, - "high": 29, - "low": 7, - "open": 1999, - "volume": 97397 - }, - "open": "2020-08-11T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8032, - "high": 38, - "low": 33, - "open": 1143, - "volume": 4882806 - }, - "id": 2688750, - "non_hive": { - "close": 2000, - "high": 10, - "low": 8, - "open": 294, - "volume": 1212051 - }, - "open": "2020-08-11T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4284, - "high": 15413, - "low": 661995, - "open": 27920, - "volume": 5163101 - }, - "id": 2688801, - "non_hive": { - "close": 1087, - "high": 3941, - "low": 158878, - "open": 6842, - "volume": 1262490 - }, - "open": "2020-08-11T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5369, - "high": 39, - "low": 309630, - "open": 406429, - "volume": 3215700 - }, - "id": 2688850, - "non_hive": { - "close": 1359, - "high": 10, - "low": 74930, - "open": 100000, - "volume": 782039 - }, - "open": "2020-08-11T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1296, - "high": 1296, - "low": 77, - "open": 12240, - "volume": 37107 - }, - "id": 2688887, - "non_hive": { - "close": 328, - "high": 328, - "low": 18, - "open": 3096, - "volume": 9184 - }, - "open": "2020-08-11T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6061, - "high": 94, - "low": 5000, - "open": 94, - "volume": 34273 - }, - "id": 2688902, - "non_hive": { - "close": 1532, - "high": 24, - "low": 1208, - "open": 24, - "volume": 8610 - }, - "open": "2020-08-11T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10476, - "high": 225, - "low": 4093, - "open": 383, - "volume": 96009 - }, - "id": 2688924, - "non_hive": { - "close": 2653, - "high": 57, - "low": 1032, - "open": 97, - "volume": 24241 - }, - "open": "2020-08-11T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5884, - "high": 16980, - "low": 7, - "open": 11523, - "volume": 290337 - }, - "id": 2688945, - "non_hive": { - "close": 1424, - "high": 4300, - "low": 1, - "open": 2918, - "volume": 72959 - }, - "open": "2020-08-11T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 445, - "high": 98, - "low": 445, - "open": 1955, - "volume": 1647254 - }, - "id": 2689001, - "non_hive": { - "close": 107, - "high": 25, - "low": 107, - "open": 494, - "volume": 402764 - }, - "open": "2020-08-11T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 119094, - "high": 11185, - "low": 13, - "open": 356, - "volume": 195735 - }, - "id": 2689047, - "non_hive": { - "close": 30000, - "high": 2820, - "low": 3, - "open": 89, - "volume": 49310 - }, - "open": "2020-08-12T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1198808, - "high": 10462, - "low": 1198808, - "open": 204298, - "volume": 6314074 - }, - "id": 2689072, - "non_hive": { - "close": 282918, - "high": 2634, - "low": 282918, - "open": 51434, - "volume": 1513632 - }, - "open": "2020-08-12T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1168474, - "high": 55, - "low": 80059, - "open": 55, - "volume": 7056028 - }, - "id": 2689100, - "non_hive": { - "close": 270508, - "high": 14, - "low": 18534, - "open": 14, - "volume": 1645264 - }, - "open": "2020-08-12T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1198896, - "high": 11459, - "low": 225566, - "open": 664, - "volume": 7332419 - }, - "id": 2689144, - "non_hive": { - "close": 277551, - "high": 2796, - "low": 52219, - "open": 162, - "volume": 1698290 - }, - "open": "2020-08-12T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 176154, - "high": 28, - "low": 15219, - "open": 224567, - "volume": 5312910 - }, - "id": 2689176, - "non_hive": { - "close": 43001, - "high": 7, - "low": 3523, - "open": 54731, - "volume": 1239498 - }, - "open": "2020-08-12T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 320, - "high": 12354, - "low": 107, - "open": 8193, - "volume": 249967 - }, - "id": 2689208, - "non_hive": { - "close": 78, - "high": 3015, - "low": 26, - "open": 1999, - "volume": 60942 - }, - "open": "2020-08-12T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1099275, - "high": 4, - "low": 264470, - "open": 8631, - "volume": 5874663 - }, - "id": 2689237, - "non_hive": { - "close": 255284, - "high": 1, - "low": 60830, - "open": 2000, - "volume": 1369664 - }, - "open": "2020-08-12T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4353, - "high": 24, - "low": 1191397, - "open": 8227, - "volume": 6268651 - }, - "id": 2689269, - "non_hive": { - "close": 1060, - "high": 6, - "low": 276908, - "open": 1999, - "volume": 1462243 - }, - "open": "2020-08-12T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6115, - "high": 8, - "low": 7326, - "open": 7662, - "volume": 5006441 - }, - "id": 2689317, - "non_hive": { - "close": 1488, - "high": 2, - "low": 1714, - "open": 1865, - "volume": 1183529 - }, - "open": "2020-08-12T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7087, - "high": 8202, - "low": 8667, - "open": 2100, - "volume": 5063852 - }, - "id": 2689361, - "non_hive": { - "close": 1727, - "high": 1999, - "low": 2036, - "open": 511, - "volume": 1195831 - }, - "open": "2020-08-12T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 736, - "high": 131, - "low": 685813, - "open": 9096, - "volume": 5833075 - }, - "id": 2689409, - "non_hive": { - "close": 179, - "high": 32, - "low": 161240, - "open": 2217, - "volume": 1383605 - }, - "open": "2020-08-12T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10419, - "high": 420, - "low": 38, - "open": 420, - "volume": 131792 - }, - "id": 2689465, - "non_hive": { - "close": 2511, - "high": 102, - "low": 9, - "open": 102, - "volume": 31956 - }, - "open": "2020-08-12T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1401, - "high": 86220, - "low": 4241, - "open": 5014, - "volume": 538187 - }, - "id": 2689494, - "non_hive": { - "close": 339, - "high": 20928, - "low": 991, - "open": 1208, - "volume": 129931 - }, - "open": "2020-08-12T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8204, - "high": 4, - "low": 20, - "open": 11047, - "volume": 3505055 - }, - "id": 2689533, - "non_hive": { - "close": 1999, - "high": 1, - "low": 4, - "open": 2673, - "volume": 845623 - }, - "open": "2020-08-12T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 52, - "high": 24, - "low": 102, - "open": 59471, - "volume": 1688749 - }, - "id": 2689587, - "non_hive": { - "close": 13, - "high": 6, - "low": 24, - "open": 14491, - "volume": 410438 - }, - "open": "2020-08-12T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7928, - "high": 5208, - "low": 229, - "open": 408164, - "volume": 1653557 - }, - "id": 2689640, - "non_hive": { - "close": 2047, - "high": 1348, - "low": 57, - "open": 102020, - "volume": 418687 - }, - "open": "2020-08-12T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54812, - "high": 3, - "low": 180579, - "open": 12, - "volume": 1534245 - }, - "id": 2689705, - "non_hive": { - "close": 14120, - "high": 1, - "low": 44964, - "open": 3, - "volume": 384943 - }, - "open": "2020-08-12T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 226, - "high": 7843, - "low": 168, - "open": 7843, - "volume": 4332560 - }, - "id": 2689766, - "non_hive": { - "close": 56, - "high": 2000, - "low": 41, - "open": 2000, - "volume": 1082350 - }, - "open": "2020-08-12T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2256, - "high": 486, - "low": 227865, - "open": 4119, - "volume": 2657000 - }, - "id": 2689827, - "non_hive": { - "close": 566, - "high": 124, - "low": 56461, - "open": 1028, - "volume": 659444 - }, - "open": "2020-08-12T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19521, - "high": 135, - "low": 1175577, - "open": 4438, - "volume": 1311418 - }, - "id": 2689901, - "non_hive": { - "close": 4897, - "high": 34, - "low": 291290, - "open": 1113, - "volume": 325297 - }, - "open": "2020-08-12T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 168495, - "high": 617, - "low": 168495, - "open": 1575, - "volume": 1012070 - }, - "id": 2689954, - "non_hive": { - "close": 41618, - "high": 155, - "low": 41618, - "open": 395, - "volume": 252411 - }, - "open": "2020-08-12T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 451, - "high": 15, - "low": 57013, - "open": 801, - "volume": 1444302 - }, - "id": 2689984, - "non_hive": { - "close": 115, - "high": 4, - "low": 14139, - "open": 201, - "volume": 362028 - }, - "open": "2020-08-12T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15690, - "high": 43510, - "low": 1191985, - "open": 2238, - "volume": 1296912 - }, - "id": 2690012, - "non_hive": { - "close": 3996, - "high": 11083, - "low": 297400, - "open": 569, - "volume": 324078 - }, - "open": "2020-08-12T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5257, - "high": 19600, - "low": 446, - "open": 23537, - "volume": 2049747 - }, - "id": 2690034, - "non_hive": { - "close": 1337, - "high": 4998, - "low": 111, - "open": 5994, - "volume": 516290 - }, - "open": "2020-08-12T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2418, - "high": 90, - "low": 2418, - "open": 8028, - "volume": 284197 - }, - "id": 2690086, - "non_hive": { - "close": 602, - "high": 23, - "low": 602, - "open": 2000, - "volume": 71227 - }, - "open": "2020-08-13T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12976, - "high": 447, - "low": 7849, - "open": 7849, - "volume": 80475 - }, - "id": 2690124, - "non_hive": { - "close": 3305, - "high": 114, - "low": 1999, - "open": 1999, - "volume": 20500 - }, - "open": "2020-08-13T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 30283, - "low": 214643, - "open": 3543, - "volume": 2441867 - }, - "id": 2690140, - "non_hive": { - "close": 1, - "high": 7722, - "low": 52802, - "open": 901, - "volume": 604935 - }, - "open": "2020-08-13T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2480, - "high": 20204, - "low": 398, - "open": 20204, - "volume": 3624454 - }, - "id": 2690158, - "non_hive": { - "close": 600, - "high": 5148, - "low": 96, - "open": 5148, - "volume": 884201 - }, - "open": "2020-08-13T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 163405, - "high": 11926, - "low": 29, - "open": 11926, - "volume": 211104 - }, - "id": 2690183, - "non_hive": { - "close": 40851, - "high": 3032, - "low": 7, - "open": 3032, - "volume": 52690 - }, - "open": "2020-08-13T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 291, - "high": 30117, - "low": 8, - "open": 30117, - "volume": 30764 - }, - "id": 2690206, - "non_hive": { - "close": 73, - "high": 7629, - "low": 1, - "open": 7629, - "volume": 7790 - }, - "open": "2020-08-13T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6387, - "high": 7601, - "low": 262, - "open": 10112, - "volume": 139760 - }, - "id": 2690219, - "non_hive": { - "close": 1601, - "high": 1926, - "low": 63, - "open": 2537, - "volume": 35163 - }, - "open": "2020-08-13T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 124396, - "high": 124396, - "low": 77608, - "open": 15670, - "volume": 257812 - }, - "id": 2690244, - "non_hive": { - "close": 31517, - "high": 31517, - "low": 19448, - "open": 3927, - "volume": 64951 - }, - "open": "2020-08-13T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11692, - "high": 75972, - "low": 129951, - "open": 8209, - "volume": 3613914 - }, - "id": 2690262, - "non_hive": { - "close": 2921, - "high": 19069, - "low": 31191, - "open": 2000, - "volume": 873238 - }, - "open": "2020-08-13T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6654, - "high": 111, - "low": 35, - "open": 8006, - "volume": 5583618 - }, - "id": 2690290, - "non_hive": { - "close": 1674, - "high": 28, - "low": 8, - "open": 1999, - "volume": 1338429 - }, - "open": "2020-08-13T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3692, - "high": 5002, - "low": 473280, - "open": 8277, - "volume": 2415050 - }, - "id": 2690338, - "non_hive": { - "close": 927, - "high": 1257, - "low": 114064, - "open": 2000, - "volume": 582621 - }, - "open": "2020-08-13T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3829, - "high": 67, - "low": 7969, - "open": 7969, - "volume": 112891 - }, - "id": 2690356, - "non_hive": { - "close": 963, - "high": 17, - "low": 1999, - "open": 1999, - "volume": 28381 - }, - "open": "2020-08-13T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1124414, - "high": 19, - "low": 20, - "open": 11029, - "volume": 3671634 - }, - "id": 2690377, - "non_hive": { - "close": 274357, - "high": 5, - "low": 4, - "open": 2773, - "volume": 900874 - }, - "open": "2020-08-13T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9496, - "high": 27, - "low": 865242, - "open": 3221, - "volume": 2604148 - }, - "id": 2690422, - "non_hive": { - "close": 2370, - "high": 7, - "low": 211215, - "open": 809, - "volume": 637587 - }, - "open": "2020-08-13T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8193, - "high": 3061, - "low": 18, - "open": 145, - "volume": 5377722 - }, - "id": 2690459, - "non_hive": { - "close": 2000, - "high": 764, - "low": 4, - "open": 36, - "volume": 1319654 - }, - "open": "2020-08-13T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46260, - "high": 80, - "low": 25, - "open": 70044, - "volume": 945287 - }, - "id": 2690519, - "non_hive": { - "close": 11426, - "high": 20, - "low": 6, - "open": 17098, - "volume": 234255 - }, - "open": "2020-08-13T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 289705, - "high": 289705, - "low": 13, - "open": 2036, - "volume": 1884167 - }, - "id": 2690573, - "non_hive": { - "close": 73471, - "high": 73471, - "low": 3, - "open": 503, - "volume": 468415 - }, - "open": "2020-08-13T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7729, - "high": 23, - "low": 366163, - "open": 631, - "volume": 3567557 - }, - "id": 2690627, - "non_hive": { - "close": 1999, - "high": 6, - "low": 89725, - "open": 160, - "volume": 913462 - }, - "open": "2020-08-13T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 297668, - "high": 146, - "low": 297668, - "open": 3740, - "volume": 935051 - }, - "id": 2690691, - "non_hive": { - "close": 76500, - "high": 38, - "low": 76500, - "open": 968, - "volume": 241360 - }, - "open": "2020-08-13T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3969, - "high": 130, - "low": 6903, - "open": 6903, - "volume": 111003 - }, - "id": 2690739, - "non_hive": { - "close": 1020, - "high": 34, - "low": 1774, - "open": 1774, - "volume": 28710 - }, - "open": "2020-08-13T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7722, - "high": 2519, - "low": 310, - "open": 3812, - "volume": 737642 - }, - "id": 2690775, - "non_hive": { - "close": 1999, - "high": 653, - "low": 79, - "open": 980, - "volume": 190118 - }, - "open": "2020-08-13T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 15, - "low": 100, - "open": 5539, - "volume": 2819931 - }, - "id": 2690809, - "non_hive": { - "close": 25, - "high": 4, - "low": 25, - "open": 1434, - "volume": 709820 - }, - "open": "2020-08-13T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28, - "high": 23, - "low": 13, - "open": 2747, - "volume": 1049399 - }, - "id": 2690847, - "non_hive": { - "close": 7, - "high": 6, - "low": 3, - "open": 710, - "volume": 263717 - }, - "open": "2020-08-13T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3779, - "high": 85, - "low": 11, - "open": 7759, - "volume": 1225371 - }, - "id": 2690894, - "non_hive": { - "close": 963, - "high": 22, - "low": 2, - "open": 1999, - "volume": 311833 - }, - "open": "2020-08-13T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22, - "high": 135311, - "low": 22, - "open": 8055, - "volume": 335454 - }, - "id": 2690947, - "non_hive": { - "close": 5, - "high": 34435, - "low": 5, - "open": 2000, - "volume": 84644 - }, - "open": "2020-08-14T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 782, - "high": 157, - "low": 35, - "open": 157, - "volume": 65805 - }, - "id": 2690977, - "non_hive": { - "close": 198, - "high": 40, - "low": 8, - "open": 40, - "volume": 16683 - }, - "open": "2020-08-14T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 83, - "high": 22624, - "low": 15, - "open": 19759, - "volume": 217060 - }, - "id": 2691001, - "non_hive": { - "close": 20, - "high": 5725, - "low": 3, - "open": 5000, - "volume": 54876 - }, - "open": "2020-08-14T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 138270, - "high": 35, - "low": 734, - "open": 35, - "volume": 470533 - }, - "id": 2691040, - "non_hive": { - "close": 34985, - "high": 9, - "low": 183, - "open": 9, - "volume": 119046 - }, - "open": "2020-08-14T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 302369, - "high": 438, - "low": 1721, - "open": 7935, - "volume": 2014438 - }, - "id": 2691059, - "non_hive": { - "close": 76520, - "high": 111, - "low": 433, - "open": 2000, - "volume": 509771 - }, - "open": "2020-08-14T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2437, - "high": 50, - "low": 761, - "open": 84471, - "volume": 1637263 - }, - "id": 2691095, - "non_hive": { - "close": 623, - "high": 13, - "low": 192, - "open": 21377, - "volume": 415000 - }, - "open": "2020-08-14T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23690, - "high": 69, - "low": 15394, - "open": 24641, - "volume": 404368 - }, - "id": 2691119, - "non_hive": { - "close": 6112, - "high": 18, - "low": 3849, - "open": 6308, - "volume": 103726 - }, - "open": "2020-08-14T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 792, - "high": 46, - "low": 130, - "open": 4487, - "volume": 309710 - }, - "id": 2691158, - "non_hive": { - "close": 206, - "high": 12, - "low": 33, - "open": 1157, - "volume": 79201 - }, - "open": "2020-08-14T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3616, - "high": 503, - "low": 624, - "open": 80804, - "volume": 447042 - }, - "id": 2691200, - "non_hive": { - "close": 940, - "high": 131, - "low": 162, - "open": 21001, - "volume": 116211 - }, - "open": "2020-08-14T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 530, - "high": 457, - "low": 3196, - "open": 984, - "volume": 24393 - }, - "id": 2691231, - "non_hive": { - "close": 138, - "high": 119, - "low": 830, - "open": 256, - "volume": 6342 - }, - "open": "2020-08-14T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12338, - "high": 4766, - "low": 7845, - "open": 8438, - "volume": 187422 - }, - "id": 2691246, - "non_hive": { - "close": 3208, - "high": 1244, - "low": 2039, - "open": 2194, - "volume": 48800 - }, - "open": "2020-08-14T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2837, - "high": 19, - "low": 50463, - "open": 27373, - "volume": 415789 - }, - "id": 2691290, - "non_hive": { - "close": 737, - "high": 5, - "low": 13069, - "open": 7117, - "volume": 107755 - }, - "open": "2020-08-14T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2116, - "high": 1273, - "low": 344, - "open": 4594, - "volume": 1058289 - }, - "id": 2691311, - "non_hive": { - "close": 550, - "high": 331, - "low": 88, - "open": 1193, - "volume": 273745 - }, - "open": "2020-08-14T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10208, - "high": 38, - "low": 118, - "open": 1482, - "volume": 2575558 - }, - "id": 2691348, - "non_hive": { - "close": 2644, - "high": 10, - "low": 30, - "open": 385, - "volume": 663482 - }, - "open": "2020-08-14T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10831, - "high": 15, - "low": 7860, - "open": 16676, - "volume": 583123 - }, - "id": 2691394, - "non_hive": { - "close": 2804, - "high": 4, - "low": 2019, - "open": 4317, - "volume": 150304 - }, - "open": "2020-08-14T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3, - "high": 3, - "low": 13, - "open": 1923, - "volume": 138984 - }, - "id": 2691440, - "non_hive": { - "close": 1, - "high": 1, - "low": 3, - "open": 498, - "volume": 36064 - }, - "open": "2020-08-14T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11571, - "high": 196, - "low": 61, - "open": 251, - "volume": 114862 - }, - "id": 2691482, - "non_hive": { - "close": 2973, - "high": 51, - "low": 15, - "open": 65, - "volume": 29751 - }, - "open": "2020-08-14T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 232240, - "high": 2278, - "low": 48816, - "open": 7726, - "volume": 405819 - }, - "id": 2691520, - "non_hive": { - "close": 60097, - "high": 590, - "low": 12544, - "open": 1999, - "volume": 104846 - }, - "open": "2020-08-14T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30000, - "high": 2159, - "low": 32297, - "open": 1016, - "volume": 753312 - }, - "id": 2691544, - "non_hive": { - "close": 7749, - "high": 558, - "low": 8268, - "open": 262, - "volume": 193794 - }, - "open": "2020-08-14T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1238, - "high": 16529, - "low": 102921, - "open": 102921, - "volume": 309938 - }, - "id": 2691573, - "non_hive": { - "close": 320, - "high": 4276, - "low": 26584, - "open": 26584, - "volume": 80096 - }, - "open": "2020-08-14T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 199555, - "high": 199555, - "low": 227, - "open": 11052, - "volume": 507723 - }, - "id": 2691601, - "non_hive": { - "close": 51625, - "high": 51625, - "low": 58, - "open": 2855, - "volume": 131224 - }, - "open": "2020-08-14T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 91, - "high": 91, - "low": 607596, - "open": 445, - "volume": 8278305 - }, - "id": 2691622, - "non_hive": { - "close": 24, - "high": 24, - "low": 155848, - "open": 115, - "volume": 2151468 - }, - "open": "2020-08-14T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28, - "high": 520, - "low": 28, - "open": 7502, - "volume": 2652308 - }, - "id": 2691668, - "non_hive": { - "close": 7, - "high": 137, - "low": 7, - "open": 1975, - "volume": 680794 - }, - "open": "2020-08-14T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3922, - "high": 307, - "low": 445, - "open": 1494, - "volume": 3610388 - }, - "id": 2691715, - "non_hive": { - "close": 1027, - "high": 81, - "low": 113, - "open": 388, - "volume": 934829 - }, - "open": "2020-08-14T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1028513, - "high": 326, - "low": 12, - "open": 3712, - "volume": 1419736 - }, - "id": 2691765, - "non_hive": { - "close": 261396, - "high": 86, - "low": 3, - "open": 973, - "volume": 362917 - }, - "open": "2020-08-15T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 679, - "high": 679, - "low": 22, - "open": 196733, - "volume": 283124 - }, - "id": 2691828, - "non_hive": { - "close": 179, - "high": 179, - "low": 5, - "open": 50000, - "volume": 72517 - }, - "open": "2020-08-15T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 6749, - "low": 45, - "open": 7643, - "volume": 237219 - }, - "id": 2691866, - "non_hive": { - "close": 11, - "high": 1779, - "low": 11, - "open": 1999, - "volume": 62310 - }, - "open": "2020-08-15T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46947, - "high": 63636, - "low": 959841, - "open": 3912, - "volume": 1447568 - }, - "id": 2691934, - "non_hive": { - "close": 12137, - "high": 16754, - "low": 242844, - "open": 1026, - "volume": 368459 - }, - "open": "2020-08-15T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5352, - "high": 38281, - "low": 149708, - "open": 7736, - "volume": 1285430 - }, - "id": 2691963, - "non_hive": { - "close": 1383, - "high": 9900, - "low": 37876, - "open": 1999, - "volume": 325916 - }, - "open": "2020-08-15T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14545, - "high": 100, - "low": 199, - "open": 646, - "volume": 57414 - }, - "id": 2691980, - "non_hive": { - "close": 3752, - "high": 26, - "low": 50, - "open": 167, - "volume": 14805 - }, - "open": "2020-08-15T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11198, - "high": 267, - "low": 292, - "open": 2005, - "volume": 50746 - }, - "id": 2692001, - "non_hive": { - "close": 2887, - "high": 69, - "low": 75, - "open": 517, - "volume": 13084 - }, - "open": "2020-08-15T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31, - "high": 31, - "low": 34938, - "open": 6956, - "volume": 226815 - }, - "id": 2692022, - "non_hive": { - "close": 8, - "high": 8, - "low": 9000, - "open": 1793, - "volume": 58446 - }, - "open": "2020-08-15T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25003, - "high": 910, - "low": 7780, - "open": 7780, - "volume": 588290 - }, - "id": 2692048, - "non_hive": { - "close": 6450, - "high": 235, - "low": 1999, - "open": 2000, - "volume": 151344 - }, - "open": "2020-08-15T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37618, - "high": 26, - "low": 622, - "open": 116, - "volume": 1606210 - }, - "id": 2692071, - "non_hive": { - "close": 9826, - "high": 7, - "low": 160, - "open": 30, - "volume": 416682 - }, - "open": "2020-08-15T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7522, - "high": 294, - "low": 3986, - "open": 18437, - "volume": 848609 - }, - "id": 2692112, - "non_hive": { - "close": 1965, - "high": 77, - "low": 1036, - "open": 4816, - "volume": 221662 - }, - "open": "2020-08-15T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51408, - "high": 45, - "low": 2478, - "open": 2478, - "volume": 990469 - }, - "id": 2692149, - "non_hive": { - "close": 13433, - "high": 12, - "low": 647, - "open": 647, - "volume": 258738 - }, - "open": "2020-08-15T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4570, - "high": 5797, - "low": 9188, - "open": 146, - "volume": 1285936 - }, - "id": 2692174, - "non_hive": { - "close": 1202, - "high": 1527, - "low": 2389, - "open": 38, - "volume": 335838 - }, - "open": "2020-08-15T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5028, - "high": 497, - "low": 606, - "open": 4672, - "volume": 50189 - }, - "id": 2692207, - "non_hive": { - "close": 1322, - "high": 131, - "low": 157, - "open": 1230, - "volume": 13177 - }, - "open": "2020-08-15T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 119659, - "high": 208, - "low": 104, - "open": 43267, - "volume": 1928456 - }, - "id": 2692227, - "non_hive": { - "close": 31501, - "high": 55, - "low": 27, - "open": 11387, - "volume": 507571 - }, - "open": "2020-08-15T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9710, - "high": 326, - "low": 8, - "open": 274342, - "volume": 3416701 - }, - "id": 2692280, - "non_hive": { - "close": 2559, - "high": 86, - "low": 2, - "open": 72152, - "volume": 899551 - }, - "open": "2020-08-15T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9714, - "high": 26, - "low": 12, - "open": 136, - "volume": 218709 - }, - "id": 2692333, - "non_hive": { - "close": 2560, - "high": 7, - "low": 3, - "open": 36, - "volume": 57636 - }, - "open": "2020-08-15T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22227, - "high": 9759, - "low": 54, - "open": 9759, - "volume": 352979 - }, - "id": 2692365, - "non_hive": { - "close": 5823, - "high": 2572, - "low": 14, - "open": 2572, - "volume": 92588 - }, - "open": "2020-08-15T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 918, - "high": 918, - "low": 5830, - "open": 5830, - "volume": 877755 - }, - "id": 2692416, - "non_hive": { - "close": 248, - "high": 248, - "low": 1527, - "open": 1527, - "volume": 232369 - }, - "open": "2020-08-15T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 130, - "high": 218, - "low": 2329, - "open": 1404, - "volume": 440187 - }, - "id": 2692444, - "non_hive": { - "close": 36, - "high": 61, - "low": 617, - "open": 379, - "volume": 121064 - }, - "open": "2020-08-15T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6038, - "high": 8311, - "low": 106, - "open": 7233, - "volume": 1025343 - }, - "id": 2692483, - "non_hive": { - "close": 1618, - "high": 2297, - "low": 28, - "open": 1999, - "volume": 276968 - }, - "open": "2020-08-15T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5461, - "high": 3, - "low": 20, - "open": 218, - "volume": 1822279 - }, - "id": 2692519, - "non_hive": { - "close": 1506, - "high": 1, - "low": 5, - "open": 58, - "volume": 487422 - }, - "open": "2020-08-15T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6929, - "high": 1323, - "low": 8, - "open": 2974, - "volume": 376591 - }, - "id": 2692568, - "non_hive": { - "close": 1843, - "high": 365, - "low": 2, - "open": 820, - "volume": 103205 - }, - "open": "2020-08-15T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2152, - "high": 1702, - "low": 22, - "open": 20816, - "volume": 2450921 - }, - "id": 2692607, - "non_hive": { - "close": 581, - "high": 468, - "low": 5, - "open": 5538, - "volume": 647371 - }, - "open": "2020-08-15T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2140, - "high": 21733, - "low": 15, - "open": 1352, - "volume": 29839 - }, - "id": 2692636, - "non_hive": { - "close": 588, - "high": 5972, - "low": 4, - "open": 365, - "volume": 8191 - }, - "open": "2020-08-16T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12796, - "high": 142, - "low": 9721, - "open": 16017, - "volume": 97811 - }, - "id": 2692652, - "non_hive": { - "close": 3512, - "high": 39, - "low": 2668, - "open": 4399, - "volume": 26852 - }, - "open": "2020-08-16T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8103, - "high": 120, - "low": 4, - "open": 29064, - "volume": 83454 - }, - "id": 2692672, - "non_hive": { - "close": 2224, - "high": 33, - "low": 1, - "open": 7977, - "volume": 22905 - }, - "open": "2020-08-16T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1223, - "high": 437, - "low": 3337, - "open": 3774, - "volume": 79717 - }, - "id": 2692701, - "non_hive": { - "close": 334, - "high": 120, - "low": 910, - "open": 1035, - "volume": 21871 - }, - "open": "2020-08-16T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17831, - "high": 154, - "low": 28578, - "open": 717, - "volume": 96829 - }, - "id": 2692720, - "non_hive": { - "close": 4812, - "high": 42, - "low": 7712, - "open": 195, - "volume": 26153 - }, - "open": "2020-08-16T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57000, - "high": 15831, - "low": 67, - "open": 11714, - "volume": 2122770 - }, - "id": 2692738, - "non_hive": { - "close": 15374, - "high": 4272, - "low": 17, - "open": 3161, - "volume": 556652 - }, - "open": "2020-08-16T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11008, - "high": 11008, - "low": 4, - "open": 10740, - "volume": 1714825 - }, - "id": 2692762, - "non_hive": { - "close": 2991, - "high": 2991, - "low": 1, - "open": 2897, - "volume": 463012 - }, - "open": "2020-08-16T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2393, - "high": 2393, - "low": 186, - "open": 67935, - "volume": 153520 - }, - "id": 2692799, - "non_hive": { - "close": 677, - "high": 677, - "low": 50, - "open": 18458, - "volume": 42179 - }, - "open": "2020-08-16T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3841, - "high": 7115, - "low": 7770, - "open": 7115, - "volume": 181503 - }, - "id": 2692836, - "non_hive": { - "close": 1067, - "high": 2000, - "low": 2148, - "open": 2000, - "volume": 50573 - }, - "open": "2020-08-16T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 260, - "high": 1359, - "low": 196, - "open": 3841, - "volume": 1897049 - }, - "id": 2692871, - "non_hive": { - "close": 70, - "high": 378, - "low": 52, - "open": 1067, - "volume": 516073 - }, - "open": "2020-08-16T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3789, - "high": 3789, - "low": 260, - "open": 3832, - "volume": 496571 - }, - "id": 2692942, - "non_hive": { - "close": 1042, - "high": 1042, - "low": 70, - "open": 1035, - "volume": 134528 - }, - "open": "2020-08-16T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 261, - "high": 601, - "low": 245, - "open": 3789, - "volume": 120239 - }, - "id": 2692992, - "non_hive": { - "close": 71, - "high": 169, - "low": 66, - "open": 1042, - "volume": 33089 - }, - "open": "2020-08-16T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7103, - "high": 252, - "low": 189565, - "open": 252, - "volume": 1131981 - }, - "id": 2693050, - "non_hive": { - "close": 1915, - "high": 70, - "low": 50000, - "open": 70, - "volume": 300133 - }, - "open": "2020-08-16T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4464, - "high": 185, - "low": 1273, - "open": 7529, - "volume": 443179 - }, - "id": 2693102, - "non_hive": { - "close": 1252, - "high": 53, - "low": 338, - "open": 2000, - "volume": 123195 - }, - "open": "2020-08-16T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 102, - "high": 114, - "low": 68, - "open": 239, - "volume": 193789 - }, - "id": 2693151, - "non_hive": { - "close": 28, - "high": 32, - "low": 18, - "open": 67, - "volume": 54124 - }, - "open": "2020-08-16T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 228, - "high": 3796, - "low": 30, - "open": 3730, - "volume": 124227 - }, - "id": 2693201, - "non_hive": { - "close": 62, - "high": 1065, - "low": 8, - "open": 1025, - "volume": 34598 - }, - "open": "2020-08-16T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6528, - "high": 17, - "low": 15, - "open": 17, - "volume": 173651 - }, - "id": 2693251, - "non_hive": { - "close": 1802, - "high": 5, - "low": 4, - "open": 5, - "volume": 48316 - }, - "open": "2020-08-16T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3586, - "high": 99, - "low": 12, - "open": 4479, - "volume": 812498 - }, - "id": 2693307, - "non_hive": { - "close": 990, - "high": 28, - "low": 3, - "open": 1236, - "volume": 222664 - }, - "open": "2020-08-16T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7184, - "high": 1162, - "low": 25, - "open": 3038, - "volume": 154025 - }, - "id": 2693345, - "non_hive": { - "close": 1983, - "high": 323, - "low": 6, - "open": 837, - "volume": 42053 - }, - "open": "2020-08-16T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9353, - "high": 1625, - "low": 2500, - "open": 3815, - "volume": 344969 - }, - "id": 2693372, - "non_hive": { - "close": 2600, - "high": 452, - "low": 680, - "open": 1053, - "volume": 95694 - }, - "open": "2020-08-16T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1178, - "high": 135, - "low": 7002, - "open": 100000, - "volume": 167126 - }, - "id": 2693416, - "non_hive": { - "close": 330, - "high": 38, - "low": 1933, - "open": 27800, - "volume": 46567 - }, - "open": "2020-08-16T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46747, - "high": 214, - "low": 195, - "open": 214, - "volume": 86595 - }, - "id": 2693444, - "non_hive": { - "close": 13089, - "high": 60, - "low": 54, - "open": 60, - "volume": 24192 - }, - "open": "2020-08-16T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7000, - "high": 53, - "low": 15, - "open": 8985, - "volume": 31858 - }, - "id": 2693470, - "non_hive": { - "close": 1960, - "high": 15, - "low": 4, - "open": 2516, - "volume": 8920 - }, - "open": "2020-08-16T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2525, - "high": 2525, - "low": 34797, - "open": 297407, - "volume": 701067 - }, - "id": 2693496, - "non_hive": { - "close": 730, - "high": 730, - "low": 9742, - "open": 83271, - "volume": 196763 - }, - "open": "2020-08-16T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3844, - "high": 100, - "low": 15, - "open": 3399, - "volume": 290550 - }, - "id": 2693544, - "non_hive": { - "close": 1111, - "high": 29, - "low": 4, - "open": 969, - "volume": 83335 - }, - "open": "2020-08-17T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 148, - "high": 148, - "low": 17865, - "open": 6920, - "volume": 57994 - }, - "id": 2693595, - "non_hive": { - "close": 43, - "high": 43, - "low": 5163, - "open": 2000, - "volume": 16761 - }, - "open": "2020-08-17T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3612, - "high": 18906, - "low": 4, - "open": 18906, - "volume": 26134 - }, - "id": 2693614, - "non_hive": { - "close": 1043, - "high": 5464, - "low": 1, - "open": 5464, - "volume": 7551 - }, - "open": "2020-08-17T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36904, - "high": 3010, - "low": 10162, - "open": 3612, - "volume": 123839 - }, - "id": 2693627, - "non_hive": { - "close": 10180, - "high": 870, - "low": 2802, - "open": 1043, - "volume": 34508 - }, - "open": "2020-08-17T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72, - "high": 3759, - "low": 72, - "open": 944, - "volume": 18650 - }, - "id": 2693639, - "non_hive": { - "close": 20, - "high": 1086, - "low": 20, - "open": 270, - "volume": 5343 - }, - "open": "2020-08-17T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1528, - "high": 432, - "low": 168, - "open": 432, - "volume": 10087 - }, - "id": 2693647, - "non_hive": { - "close": 430, - "high": 123, - "low": 47, - "open": 123, - "volume": 2852 - }, - "open": "2020-08-17T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8763, - "high": 571, - "low": 713, - "open": 355, - "volume": 131728 - }, - "id": 2693660, - "non_hive": { - "close": 2531, - "high": 165, - "low": 200, - "open": 100, - "volume": 38007 - }, - "open": "2020-08-17T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127840, - "high": 48, - "low": 1207, - "open": 7137, - "volume": 178857 - }, - "id": 2693688, - "non_hive": { - "close": 36929, - "high": 14, - "low": 338, - "open": 2000, - "volume": 51586 - }, - "open": "2020-08-17T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3624, - "high": 2166, - "low": 3851, - "open": 20538, - "volume": 142337 - }, - "id": 2693717, - "non_hive": { - "close": 1027, - "high": 639, - "low": 1087, - "open": 5956, - "volume": 41363 - }, - "open": "2020-08-17T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3627, - "high": 6, - "low": 476, - "open": 30, - "volume": 114777 - }, - "id": 2693768, - "non_hive": { - "close": 1077, - "high": 2, - "low": 134, - "open": 9, - "volume": 33469 - }, - "open": "2020-08-17T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 345, - "high": 16676, - "low": 234, - "open": 3627, - "volume": 3703730 - }, - "id": 2693832, - "non_hive": { - "close": 102, - "high": 5000, - "low": 66, - "open": 1080, - "volume": 1052692 - }, - "open": "2020-08-17T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39910, - "high": 47, - "low": 372, - "open": 785, - "volume": 246972 - }, - "id": 2693884, - "non_hive": { - "close": 11805, - "high": 14, - "low": 105, - "open": 233, - "volume": 72158 - }, - "open": "2020-08-17T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3750, - "high": 91, - "low": 572, - "open": 91, - "volume": 269324 - }, - "id": 2693939, - "non_hive": { - "close": 1097, - "high": 27, - "low": 162, - "open": 27, - "volume": 78044 - }, - "open": "2020-08-17T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3765, - "high": 33, - "low": 3670, - "open": 33, - "volume": 613972 - }, - "id": 2694003, - "non_hive": { - "close": 1069, - "high": 10, - "low": 1042, - "open": 10, - "volume": 175862 - }, - "open": "2020-08-17T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3795, - "high": 62382, - "low": 3773, - "open": 3277, - "volume": 749482 - }, - "id": 2694049, - "non_hive": { - "close": 1048, - "high": 18407, - "low": 1041, - "open": 931, - "volume": 211162 - }, - "open": "2020-08-17T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3558, - "high": 250, - "low": 13, - "open": 91595, - "volume": 841336 - }, - "id": 2694133, - "non_hive": { - "close": 985, - "high": 74, - "low": 3, - "open": 25646, - "volume": 236966 - }, - "open": "2020-08-17T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 302, - "high": 2848, - "low": 27, - "open": 3663, - "volume": 1600241 - }, - "id": 2694203, - "non_hive": { - "close": 89, - "high": 841, - "low": 7, - "open": 1015, - "volume": 448380 - }, - "open": "2020-08-17T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3823, - "high": 74569, - "low": 1611, - "open": 3620, - "volume": 1432813 - }, - "id": 2694265, - "non_hive": { - "close": 1046, - "high": 21998, - "low": 440, - "open": 1053, - "volume": 395596 - }, - "open": "2020-08-17T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10493, - "high": 3, - "low": 61, - "open": 3824, - "volume": 3070034 - }, - "id": 2694333, - "non_hive": { - "close": 3053, - "high": 1, - "low": 16, - "open": 1047, - "volume": 851613 - }, - "open": "2020-08-17T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 707, - "high": 3063, - "low": 122, - "open": 1039, - "volume": 74731 - }, - "id": 2694424, - "non_hive": { - "close": 202, - "high": 890, - "low": 34, - "open": 299, - "volume": 21544 - }, - "open": "2020-08-17T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12617, - "high": 3, - "low": 7308, - "open": 6943, - "volume": 651581 - }, - "id": 2694451, - "non_hive": { - "close": 3596, - "high": 1, - "low": 2010, - "open": 1999, - "volume": 183397 - }, - "open": "2020-08-17T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6936, - "high": 24, - "low": 6936, - "open": 6372, - "volume": 154721 - }, - "id": 2694507, - "non_hive": { - "close": 1915, - "high": 7, - "low": 1915, - "open": 1816, - "volume": 43963 - }, - "open": "2020-08-17T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 118158, - "high": 200, - "low": 140, - "open": 7240, - "volume": 1052055 - }, - "id": 2694548, - "non_hive": { - "close": 34845, - "high": 59, - "low": 38, - "open": 2000, - "volume": 309028 - }, - "open": "2020-08-17T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 876, - "high": 2500, - "low": 585901, - "open": 2500, - "volume": 2563922 - }, - "id": 2694587, - "non_hive": { - "close": 247, - "high": 737, - "low": 158913, - "open": 737, - "volume": 705540 - }, - "open": "2020-08-17T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17731, - "high": 303725, - "low": 15, - "open": 6391, - "volume": 816139 - }, - "id": 2694621, - "non_hive": { - "close": 5000, - "high": 88047, - "low": 4, - "open": 1801, - "volume": 233001 - }, - "open": "2020-08-18T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10645, - "high": 4247, - "low": 509, - "open": 33534, - "volume": 966541 - }, - "id": 2694657, - "non_hive": { - "close": 3001, - "high": 1227, - "low": 143, - "open": 9454, - "volume": 272592 - }, - "open": "2020-08-18T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 41, - "low": 4, - "open": 41, - "volume": 62923 - }, - "id": 2694690, - "non_hive": { - "close": 1, - "high": 12, - "low": 1, - "open": 12, - "volume": 18053 - }, - "open": "2020-08-18T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27557, - "high": 2336, - "low": 10588, - "open": 10588, - "volume": 195928 - }, - "id": 2694714, - "non_hive": { - "close": 7961, - "high": 675, - "low": 3051, - "open": 3051, - "volume": 56593 - }, - "open": "2020-08-18T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1416, - "high": 24, - "low": 14994, - "open": 439, - "volume": 27308 - }, - "id": 2694727, - "non_hive": { - "close": 409, - "high": 7, - "low": 4289, - "open": 127, - "volume": 7828 - }, - "open": "2020-08-18T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 2830, - "low": 1000, - "open": 5507, - "volume": 2111789 - }, - "id": 2694743, - "non_hive": { - "close": 285, - "high": 818, - "low": 285, - "open": 1590, - "volume": 610221 - }, - "open": "2020-08-18T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1985, - "high": 138, - "low": 612290, - "open": 6069, - "volume": 1343097 - }, - "id": 2694768, - "non_hive": { - "close": 546, - "high": 40, - "low": 168379, - "open": 1753, - "volume": 371574 - }, - "open": "2020-08-18T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12657, - "high": 5626, - "low": 222, - "open": 5626, - "volume": 4895281 - }, - "id": 2694798, - "non_hive": { - "close": 3599, - "high": 1603, - "low": 60, - "open": 1603, - "volume": 1346212 - }, - "open": "2020-08-18T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1327, - "high": 2346, - "low": 109558, - "open": 4949, - "volume": 3892931 - }, - "id": 2694838, - "non_hive": { - "close": 370, - "high": 667, - "low": 29799, - "open": 1407, - "volume": 1065063 - }, - "open": "2020-08-18T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 706, - "high": 7995, - "low": 1192649, - "open": 7351, - "volume": 2746952 - }, - "id": 2694867, - "non_hive": { - "close": 199, - "high": 2282, - "low": 324400, - "open": 2000, - "volume": 748295 - }, - "open": "2020-08-18T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3824, - "high": 99, - "low": 2709, - "open": 3030, - "volume": 233970 - }, - "id": 2694902, - "non_hive": { - "close": 1050, - "high": 28, - "low": 742, - "open": 844, - "volume": 65650 - }, - "open": "2020-08-18T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11545, - "high": 2558, - "low": 1074, - "open": 364, - "volume": 90434 - }, - "id": 2694943, - "non_hive": { - "close": 3247, - "high": 720, - "low": 292, - "open": 100, - "volume": 24946 - }, - "open": "2020-08-18T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3572, - "high": 2027, - "low": 10, - "open": 7108, - "volume": 120851 - }, - "id": 2694987, - "non_hive": { - "close": 1006, - "high": 571, - "low": 2, - "open": 1999, - "volume": 33929 - }, - "open": "2020-08-18T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29581, - "high": 110, - "low": 56, - "open": 3355, - "volume": 618723 - }, - "id": 2695045, - "non_hive": { - "close": 7993, - "high": 31, - "low": 15, - "open": 940, - "volume": 169301 - }, - "open": "2020-08-18T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2623, - "high": 28, - "low": 33276, - "open": 99, - "volume": 5889087 - }, - "id": 2695104, - "non_hive": { - "close": 737, - "high": 8, - "low": 8585, - "open": 27, - "volume": 1540480 - }, - "open": "2020-08-18T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1343, - "high": 3051, - "low": 31, - "open": 3051, - "volume": 5230008 - }, - "id": 2695151, - "non_hive": { - "close": 354, - "high": 856, - "low": 8, - "open": 856, - "volume": 1376361 - }, - "open": "2020-08-18T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 323, - "high": 39, - "low": 12, - "open": 2004, - "volume": 1528210 - }, - "id": 2695199, - "non_hive": { - "close": 89, - "high": 11, - "low": 3, - "open": 555, - "volume": 407634 - }, - "open": "2020-08-18T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 754, - "high": 159, - "low": 325, - "open": 1364, - "volume": 104114 - }, - "id": 2695254, - "non_hive": { - "close": 210, - "high": 45, - "low": 90, - "open": 381, - "volume": 29231 - }, - "open": "2020-08-18T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 320, - "high": 44702, - "low": 191893, - "open": 3851, - "volume": 1540799 - }, - "id": 2695290, - "non_hive": { - "close": 87, - "high": 12583, - "low": 51240, - "open": 1066, - "volume": 420452 - }, - "open": "2020-08-18T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 323, - "high": 323, - "low": 195, - "open": 3850, - "volume": 427418 - }, - "id": 2695345, - "non_hive": { - "close": 91, - "high": 91, - "low": 53, - "open": 1047, - "volume": 120127 - }, - "open": "2020-08-18T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2366, - "high": 479, - "low": 2366, - "open": 1603, - "volume": 37807 - }, - "id": 2695378, - "non_hive": { - "close": 644, - "high": 135, - "low": 644, - "open": 451, - "volume": 10497 - }, - "open": "2020-08-18T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8453, - "high": 1690, - "low": 2397, - "open": 5399, - "volume": 328154 - }, - "id": 2695395, - "non_hive": { - "close": 2375, - "high": 475, - "low": 655, - "open": 1489, - "volume": 91196 - }, - "open": "2020-08-18T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29, - "high": 298, - "low": 202, - "open": 6049, - "volume": 865947 - }, - "id": 2695434, - "non_hive": { - "close": 8, - "high": 84, - "low": 54, - "open": 1700, - "volume": 243320 - }, - "open": "2020-08-18T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 10, - "low": 60, - "open": 512187, - "volume": 1010695 - }, - "id": 2695473, - "non_hive": { - "close": 3, - "high": 3, - "low": 16, - "open": 143929, - "volume": 283993 - }, - "open": "2020-08-18T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6186, - "high": 28, - "low": 15, - "open": 12650, - "volume": 390976 - }, - "id": 2695512, - "non_hive": { - "close": 1738, - "high": 8, - "low": 4, - "open": 3554, - "volume": 109800 - }, - "open": "2020-08-19T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 68680, - "high": 135, - "low": 68680, - "open": 5090, - "volume": 91980 - }, - "id": 2695541, - "non_hive": { - "close": 19293, - "high": 38, - "low": 19293, - "open": 1430, - "volume": 25839 - }, - "open": "2020-08-19T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72748, - "high": 85159, - "low": 4, - "open": 276, - "volume": 1204941 - }, - "id": 2695558, - "non_hive": { - "close": 19642, - "high": 23923, - "low": 1, - "open": 77, - "volume": 329148 - }, - "open": "2020-08-19T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9178, - "high": 28, - "low": 9178, - "open": 7404, - "volume": 4751804 - }, - "id": 2695580, - "non_hive": { - "close": 2386, - "high": 8, - "low": 2386, - "open": 2000, - "volume": 1260540 - }, - "open": "2020-08-19T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7849, - "high": 13718, - "low": 23897, - "open": 6009, - "volume": 7482046 - }, - "id": 2695617, - "non_hive": { - "close": 1994, - "high": 3852, - "low": 6069, - "open": 1563, - "volume": 1931943 - }, - "open": "2020-08-19T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1446, - "high": 4789, - "low": 4049, - "open": 685, - "volume": 6527867 - }, - "id": 2695661, - "non_hive": { - "close": 376, - "high": 1292, - "low": 1016, - "open": 174, - "volume": 1658773 - }, - "open": "2020-08-19T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 99501, - "high": 8308, - "low": 1037283, - "open": 9000, - "volume": 7519593 - }, - "id": 2695718, - "non_hive": { - "close": 24975, - "high": 2168, - "low": 258288, - "open": 2345, - "volume": 1904685 - }, - "open": "2020-08-19T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7900, - "high": 28702, - "low": 6168, - "open": 7890, - "volume": 285981 - }, - "id": 2695779, - "non_hive": { - "close": 2138, - "high": 7797, - "low": 1534, - "open": 1999, - "volume": 74517 - }, - "open": "2020-08-19T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9307, - "high": 51, - "low": 176, - "open": 8911, - "volume": 311292 - }, - "id": 2695816, - "non_hive": { - "close": 2525, - "high": 14, - "low": 45, - "open": 2326, - "volume": 81858 - }, - "open": "2020-08-19T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9265, - "high": 25, - "low": 2408, - "open": 4226, - "volume": 344104 - }, - "id": 2695856, - "non_hive": { - "close": 2434, - "high": 7, - "low": 626, - "open": 1147, - "volume": 90316 - }, - "open": "2020-08-19T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 862621, - "high": 7, - "low": 249767, - "open": 7392, - "volume": 11542290 - }, - "id": 2695896, - "non_hive": { - "close": 220927, - "high": 2, - "low": 63968, - "open": 1999, - "volume": 2985255 - }, - "open": "2020-08-19T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3747, - "high": 131, - "low": 167946, - "open": 337380, - "volume": 3675993 - }, - "id": 2695950, - "non_hive": { - "close": 1000, - "high": 35, - "low": 42827, - "open": 86407, - "volume": 939416 - }, - "open": "2020-08-19T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18897, - "high": 2796, - "low": 1189647, - "open": 4807, - "volume": 1359151 - }, - "id": 2695977, - "non_hive": { - "close": 4875, - "high": 749, - "low": 305750, - "open": 1283, - "volume": 350721 - }, - "open": "2020-08-19T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2422, - "high": 302, - "low": 4667, - "open": 4667, - "volume": 944525 - }, - "id": 2696011, - "non_hive": { - "close": 657, - "high": 82, - "low": 1250, - "open": 1250, - "volume": 254934 - }, - "open": "2020-08-19T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5744, - "high": 833, - "low": 104, - "open": 7382, - "volume": 1329155 - }, - "id": 2696071, - "non_hive": { - "close": 1558, - "high": 226, - "low": 27, - "open": 1999, - "volume": 347394 - }, - "open": "2020-08-19T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1201, - "high": 3690, - "low": 962, - "open": 3690, - "volume": 6922503 - }, - "id": 2696103, - "non_hive": { - "close": 319, - "high": 1001, - "low": 248, - "open": 1001, - "volume": 1811710 - }, - "open": "2020-08-19T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 139, - "low": 5, - "open": 4531, - "volume": 527139 - }, - "id": 2696148, - "non_hive": { - "close": 1, - "high": 37, - "low": 1, - "open": 1203, - "volume": 139531 - }, - "open": "2020-08-19T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 196, - "high": 75, - "low": 18545, - "open": 7712, - "volume": 2016178 - }, - "id": 2696197, - "non_hive": { - "close": 52, - "high": 20, - "low": 4786, - "open": 2000, - "volume": 525704 - }, - "open": "2020-08-19T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16249, - "high": 52, - "low": 1614, - "open": 1614, - "volume": 54943 - }, - "id": 2696228, - "non_hive": { - "close": 4353, - "high": 14, - "low": 426, - "open": 426, - "volume": 14711 - }, - "open": "2020-08-19T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18461, - "high": 2177, - "low": 293468, - "open": 2000, - "volume": 1403575 - }, - "id": 2696260, - "non_hive": { - "close": 5001, - "high": 590, - "low": 76887, - "open": 536, - "volume": 369498 - }, - "open": "2020-08-19T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 114, - "high": 143, - "low": 241, - "open": 11186, - "volume": 446190 - }, - "id": 2696301, - "non_hive": { - "close": 31, - "high": 39, - "low": 65, - "open": 3030, - "volume": 120874 - }, - "open": "2020-08-19T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 447, - "high": 88, - "low": 1980, - "open": 155, - "volume": 535739 - }, - "id": 2696325, - "non_hive": { - "close": 121, - "high": 24, - "low": 522, - "open": 42, - "volume": 145118 - }, - "open": "2020-08-19T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26, - "high": 199558, - "low": 26, - "open": 2310, - "volume": 945291 - }, - "id": 2696370, - "non_hive": { - "close": 7, - "high": 54180, - "low": 7, - "open": 626, - "volume": 256540 - }, - "open": "2020-08-19T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 444, - "high": 162, - "low": 442, - "open": 442, - "volume": 488087 - }, - "id": 2696395, - "non_hive": { - "close": 123, - "high": 47, - "low": 120, - "open": 120, - "volume": 140206 - }, - "open": "2020-08-19T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 844, - "high": 467, - "low": 27644, - "open": 444, - "volume": 3306079 - }, - "id": 2696413, - "non_hive": { - "close": 234, - "high": 135, - "low": 7206, - "open": 128, - "volume": 900687 - }, - "open": "2020-08-20T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6869, - "high": 4920, - "low": 453, - "open": 967, - "volume": 106524 - }, - "id": 2696462, - "non_hive": { - "close": 1964, - "high": 1407, - "low": 125, - "open": 268, - "volume": 30382 - }, - "open": "2020-08-20T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 118, - "low": 4, - "open": 118, - "volume": 1270536 - }, - "id": 2696480, - "non_hive": { - "close": 1, - "high": 34, - "low": 1, - "open": 34, - "volume": 349438 - }, - "open": "2020-08-20T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 395172, - "high": 31280, - "low": 3504, - "open": 7067, - "volume": 2885525 - }, - "id": 2696516, - "non_hive": { - "close": 114201, - "high": 9040, - "low": 968, - "open": 1999, - "volume": 826177 - }, - "open": "2020-08-20T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 78363, - "high": 114, - "low": 20297, - "open": 346053, - "volume": 3419693 - }, - "id": 2696551, - "non_hive": { - "close": 25428, - "high": 40, - "low": 5865, - "open": 100000, - "volume": 1012430 - }, - "open": "2020-08-20T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21217, - "high": 169, - "low": 21217, - "open": 209, - "volume": 930028 - }, - "id": 2696627, - "non_hive": { - "close": 5958, - "high": 55, - "low": 5958, - "open": 68, - "volume": 285330 - }, - "open": "2020-08-20T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 199542, - "high": 199542, - "low": 357, - "open": 7109, - "volume": 1231524 - }, - "id": 2696682, - "non_hive": { - "close": 62055, - "high": 62055, - "low": 100, - "open": 1999, - "volume": 377979 - }, - "open": "2020-08-20T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4974, - "high": 77, - "low": 2910, - "open": 6430, - "volume": 2168951 - }, - "id": 2696730, - "non_hive": { - "close": 1589, - "high": 27, - "low": 846, - "open": 1999, - "volume": 673583 - }, - "open": "2020-08-20T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2042, - "high": 301, - "low": 1624, - "open": 658, - "volume": 1631793 - }, - "id": 2696775, - "non_hive": { - "close": 653, - "high": 103, - "low": 472, - "open": 210, - "volume": 511316 - }, - "open": "2020-08-20T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18226, - "high": 109, - "low": 2882, - "open": 900, - "volume": 148892 - }, - "id": 2696827, - "non_hive": { - "close": 5830, - "high": 35, - "low": 822, - "open": 288, - "volume": 47233 - }, - "open": "2020-08-20T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100000, - "high": 233, - "low": 331, - "open": 1017, - "volume": 262472 - }, - "id": 2696853, - "non_hive": { - "close": 29965, - "high": 70, - "low": 94, - "open": 305, - "volume": 78660 - }, - "open": "2020-08-20T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 339, - "high": 226, - "low": 163168, - "open": 5322, - "volume": 3667107 - }, - "id": 2696885, - "non_hive": { - "close": 95, - "high": 68, - "low": 45687, - "open": 1595, - "volume": 1032055 - }, - "open": "2020-08-20T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2994, - "high": 5206, - "low": 2400, - "open": 6671, - "volume": 1697227 - }, - "id": 2696920, - "non_hive": { - "close": 866, - "high": 1561, - "low": 660, - "open": 1999, - "volume": 472666 - }, - "open": "2020-08-20T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1205, - "high": 327, - "low": 1112127, - "open": 3474, - "volume": 1335558 - }, - "id": 2696974, - "non_hive": { - "close": 341, - "high": 95, - "low": 305908, - "open": 1000, - "volume": 369542 - }, - "open": "2020-08-20T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 791224, - "high": 3, - "low": 791224, - "open": 1270, - "volume": 1364191 - }, - "id": 2696990, - "non_hive": { - "close": 217646, - "high": 1, - "low": 217646, - "open": 358, - "volume": 378403 - }, - "open": "2020-08-20T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2216, - "high": 380, - "low": 1181989, - "open": 190, - "volume": 1270150 - }, - "id": 2697047, - "non_hive": { - "close": 622, - "high": 109, - "low": 325135, - "open": 54, - "volume": 350044 - }, - "open": "2020-08-20T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 354, - "high": 215, - "low": 5, - "open": 29, - "volume": 1356356 - }, - "id": 2697090, - "non_hive": { - "close": 100, - "high": 61, - "low": 1, - "open": 8, - "volume": 367972 - }, - "open": "2020-08-20T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1836, - "high": 3, - "low": 372640, - "open": 7026, - "volume": 3126386 - }, - "id": 2697131, - "non_hive": { - "close": 512, - "high": 1, - "low": 99882, - "open": 1953, - "volume": 850414 - }, - "open": "2020-08-20T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 605, - "high": 2813, - "low": 23538, - "open": 5443, - "volume": 1807255 - }, - "id": 2697206, - "non_hive": { - "close": 170, - "high": 799, - "low": 6485, - "open": 1518, - "volume": 506697 - }, - "open": "2020-08-20T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 101401, - "high": 85, - "low": 1167265, - "open": 5966, - "volume": 1582541 - }, - "id": 2697228, - "non_hive": { - "close": 27378, - "high": 24, - "low": 311715, - "open": 1674, - "volume": 425383 - }, - "open": "2020-08-20T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1705, - "high": 3, - "low": 8547, - "open": 487, - "volume": 276543 - }, - "id": 2697275, - "non_hive": { - "close": 464, - "high": 1, - "low": 2291, - "open": 131, - "volume": 74479 - }, - "open": "2020-08-20T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4849, - "high": 18, - "low": 60368, - "open": 9114, - "volume": 197728 - }, - "id": 2697317, - "non_hive": { - "close": 1319, - "high": 5, - "low": 16420, - "open": 2479, - "volume": 53783 - }, - "open": "2020-08-20T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3544, - "high": 128, - "low": 26, - "open": 128, - "volume": 62130 - }, - "id": 2697354, - "non_hive": { - "close": 963, - "high": 35, - "low": 7, - "open": 35, - "volume": 16899 - }, - "open": "2020-08-20T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14956, - "high": 594, - "low": 101483, - "open": 21296, - "volume": 2226167 - }, - "id": 2697376, - "non_hive": { - "close": 4113, - "high": 167, - "low": 27197, - "open": 5792, - "volume": 606263 - }, - "open": "2020-08-20T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2272, - "high": 16313, - "low": 15, - "open": 183, - "volume": 863540 - }, - "id": 2697427, - "non_hive": { - "close": 611, - "high": 4486, - "low": 4, - "open": 49, - "volume": 233083 - }, - "open": "2020-08-21T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 517, - "high": 87, - "low": 32445, - "open": 1181, - "volume": 1283374 - }, - "id": 2697492, - "non_hive": { - "close": 137, - "high": 24, - "low": 8533, - "open": 318, - "volume": 338464 - }, - "open": "2020-08-21T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 871, - "high": 621, - "low": 4, - "open": 4009, - "volume": 6456524 - }, - "id": 2697538, - "non_hive": { - "close": 245, - "high": 175, - "low": 1, - "open": 1067, - "volume": 1761757 - }, - "open": "2020-08-21T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19796, - "high": 32, - "low": 722, - "open": 4081, - "volume": 1354349 - }, - "id": 2697637, - "non_hive": { - "close": 5087, - "high": 9, - "low": 184, - "open": 1073, - "volume": 350526 - }, - "open": "2020-08-21T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7464, - "high": 208, - "low": 826, - "open": 4145, - "volume": 71867 - }, - "id": 2697684, - "non_hive": { - "close": 1999, - "high": 56, - "low": 211, - "open": 1065, - "volume": 18857 - }, - "open": "2020-08-21T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4131, - "high": 262, - "low": 619, - "open": 261, - "volume": 1577954 - }, - "id": 2697724, - "non_hive": { - "close": 1091, - "high": 71, - "low": 162, - "open": 70, - "volume": 416957 - }, - "open": "2020-08-21T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10467, - "high": 211, - "low": 7491, - "open": 7491, - "volume": 541082 - }, - "id": 2697769, - "non_hive": { - "close": 2868, - "high": 60, - "low": 1999, - "open": 1999, - "volume": 145934 - }, - "open": "2020-08-21T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12233, - "high": 3037, - "low": 12233, - "open": 7323, - "volume": 337175 - }, - "id": 2697809, - "non_hive": { - "close": 3291, - "high": 835, - "low": 3291, - "open": 2000, - "volume": 91736 - }, - "open": "2020-08-21T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 95208, - "high": 15743, - "low": 233, - "open": 15743, - "volume": 1480366 - }, - "id": 2697843, - "non_hive": { - "close": 25522, - "high": 4235, - "low": 61, - "open": 4235, - "volume": 392672 - }, - "open": "2020-08-21T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 77, - "high": 181, - "low": 35, - "open": 7461, - "volume": 422858 - }, - "id": 2697882, - "non_hive": { - "close": 21, - "high": 51, - "low": 9, - "open": 1999, - "volume": 115179 - }, - "open": "2020-08-21T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6564, - "high": 1512, - "low": 4, - "open": 4, - "volume": 709602 - }, - "id": 2697913, - "non_hive": { - "close": 1805, - "high": 423, - "low": 1, - "open": 1, - "volume": 193626 - }, - "open": "2020-08-21T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1173, - "high": 5500, - "low": 794107, - "open": 5500, - "volume": 3098555 - }, - "id": 2697956, - "non_hive": { - "close": 312, - "high": 1512, - "low": 203382, - "open": 1512, - "volume": 801508 - }, - "open": "2020-08-21T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13628, - "high": 266, - "low": 1148090, - "open": 7517, - "volume": 8136877 - }, - "id": 2698012, - "non_hive": { - "close": 3543, - "high": 71, - "low": 289461, - "open": 2000, - "volume": 2077528 - }, - "open": "2020-08-21T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5234, - "high": 41, - "low": 77546, - "open": 1346, - "volume": 1311032 - }, - "id": 2698074, - "non_hive": { - "close": 1380, - "high": 11, - "low": 19719, - "open": 354, - "volume": 334599 - }, - "open": "2020-08-21T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21658, - "high": 300, - "low": 102, - "open": 545, - "volume": 1619311 - }, - "id": 2698103, - "non_hive": { - "close": 5759, - "high": 80, - "low": 26, - "open": 144, - "volume": 417299 - }, - "open": "2020-08-21T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2238, - "high": 146, - "low": 509, - "open": 319, - "volume": 82832 - }, - "id": 2698151, - "non_hive": { - "close": 591, - "high": 39, - "low": 129, - "open": 85, - "volume": 21822 - }, - "open": "2020-08-21T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7717, - "high": 26, - "low": 12, - "open": 1462, - "volume": 488945 - }, - "id": 2698192, - "non_hive": { - "close": 2035, - "high": 7, - "low": 3, - "open": 386, - "volume": 127125 - }, - "open": "2020-08-21T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4897, - "high": 3, - "low": 2087, - "open": 2851, - "volume": 653166 - }, - "id": 2698236, - "non_hive": { - "close": 1289, - "high": 1, - "low": 535, - "open": 753, - "volume": 172971 - }, - "open": "2020-08-21T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7640, - "high": 3, - "low": 135762, - "open": 7590, - "volume": 2601091 - }, - "id": 2698282, - "non_hive": { - "close": 1999, - "high": 1, - "low": 34106, - "open": 1997, - "volume": 666826 - }, - "open": "2020-08-21T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12360, - "high": 8872, - "low": 48, - "open": 13000, - "volume": 87873 - }, - "id": 2698326, - "non_hive": { - "close": 3234, - "high": 2324, - "low": 12, - "open": 3401, - "volume": 22777 - }, - "open": "2020-08-21T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1115, - "high": 221, - "low": 7, - "open": 194, - "volume": 1368708 - }, - "id": 2698345, - "non_hive": { - "close": 289, - "high": 58, - "low": 1, - "open": 50, - "volume": 353048 - }, - "open": "2020-08-21T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3003, - "high": 563, - "low": 289128, - "open": 7759, - "volume": 3797740 - }, - "id": 2698378, - "non_hive": { - "close": 768, - "high": 146, - "low": 71382, - "open": 2000, - "volume": 955208 - }, - "open": "2020-08-21T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3823, - "high": 38409, - "low": 221776, - "open": 8063, - "volume": 484625 - }, - "id": 2698424, - "non_hive": { - "close": 1000, - "high": 10052, - "low": 55000, - "open": 2000, - "volume": 123505 - }, - "open": "2020-08-21T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2163, - "high": 10988, - "low": 445, - "open": 7164, - "volume": 1258852 - }, - "id": 2698445, - "non_hive": { - "close": 569, - "high": 2917, - "low": 110, - "open": 1874, - "volume": 328194 - }, - "open": "2020-08-21T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 275, - "high": 923, - "low": 13, - "open": 1233, - "volume": 6028132 - }, - "id": 2698492, - "non_hive": { - "close": 65, - "high": 240, - "low": 3, - "open": 312, - "volume": 1475732 - }, - "open": "2020-08-22T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1835, - "high": 48, - "low": 463879, - "open": 48, - "volume": 2488093 - }, - "id": 2698521, - "non_hive": { - "close": 455, - "high": 12, - "low": 109427, - "open": 12, - "volume": 590227 - }, - "open": "2020-08-22T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 81, - "high": 1976, - "low": 16, - "open": 3606, - "volume": 1016364 - }, - "id": 2698548, - "non_hive": { - "close": 20, - "high": 492, - "low": 3, - "open": 894, - "volume": 252504 - }, - "open": "2020-08-22T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 866, - "high": 1594, - "low": 12448, - "open": 1594, - "volume": 1370208 - }, - "id": 2698588, - "non_hive": { - "close": 215, - "high": 397, - "low": 2968, - "open": 397, - "volume": 327471 - }, - "open": "2020-08-22T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1258, - "high": 180, - "low": 1146627, - "open": 392, - "volume": 2480451 - }, - "id": 2698617, - "non_hive": { - "close": 312, - "high": 45, - "low": 274135, - "open": 97, - "volume": 593912 - }, - "open": "2020-08-22T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23824, - "high": 24, - "low": 1191617, - "open": 11501, - "volume": 2521216 - }, - "id": 2698648, - "non_hive": { - "close": 5931, - "high": 6, - "low": 284259, - "open": 2858, - "volume": 603321 - }, - "open": "2020-08-22T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9580, - "high": 791, - "low": 7269, - "open": 6575, - "volume": 354306 - }, - "id": 2698684, - "non_hive": { - "close": 2385, - "high": 197, - "low": 1808, - "open": 1636, - "volume": 88202 - }, - "open": "2020-08-22T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27311, - "high": 4, - "low": 753298, - "open": 6733, - "volume": 1328572 - }, - "id": 2698712, - "non_hive": { - "close": 6761, - "high": 1, - "low": 180098, - "open": 1676, - "volume": 318937 - }, - "open": "2020-08-22T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 99706, - "high": 80, - "low": 34, - "open": 45954, - "volume": 1389433 - }, - "id": 2698744, - "non_hive": { - "close": 24577, - "high": 20, - "low": 8, - "open": 11380, - "volume": 343150 - }, - "open": "2020-08-22T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2350, - "high": 2350, - "low": 536, - "open": 16048, - "volume": 826559 - }, - "id": 2698786, - "non_hive": { - "close": 627, - "high": 627, - "low": 130, - "open": 3996, - "volume": 209742 - }, - "open": "2020-08-22T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 802, - "high": 3046, - "low": 802, - "open": 2434, - "volume": 1320281 - }, - "id": 2698810, - "non_hive": { - "close": 200, - "high": 820, - "low": 200, - "open": 645, - "volume": 333523 - }, - "open": "2020-08-22T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1233, - "high": 1714, - "low": 44, - "open": 1714, - "volume": 1404180 - }, - "id": 2698861, - "non_hive": { - "close": 312, - "high": 447, - "low": 10, - "open": 447, - "volume": 343286 - }, - "open": "2020-08-22T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19, - "high": 88, - "low": 11, - "open": 1461, - "volume": 644939 - }, - "id": 2698879, - "non_hive": { - "close": 4, - "high": 23, - "low": 2, - "open": 371, - "volume": 166377 - }, - "open": "2020-08-22T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41842, - "high": 3, - "low": 16797, - "open": 7154, - "volume": 2703861 - }, - "id": 2698927, - "non_hive": { - "close": 10670, - "high": 1, - "low": 4064, - "open": 1866, - "volume": 661994 - }, - "open": "2020-08-22T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 509, - "high": 19, - "low": 8, - "open": 4450, - "volume": 51479 - }, - "id": 2698980, - "non_hive": { - "close": 132, - "high": 5, - "low": 2, - "open": 1139, - "volume": 13267 - }, - "open": "2020-08-22T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2946, - "high": 50, - "low": 2946, - "open": 19063, - "volume": 219574 - }, - "id": 2699008, - "non_hive": { - "close": 713, - "high": 13, - "low": 713, - "open": 4922, - "volume": 55913 - }, - "open": "2020-08-22T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4000, - "high": 2658, - "low": 29, - "open": 7875, - "volume": 1711667 - }, - "id": 2699038, - "non_hive": { - "close": 1000, - "high": 686, - "low": 7, - "open": 1999, - "volume": 421542 - }, - "open": "2020-08-22T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1228, - "high": 3, - "low": 84, - "open": 18512, - "volume": 1713833 - }, - "id": 2699089, - "non_hive": { - "close": 312, - "high": 1, - "low": 20, - "open": 4628, - "volume": 427569 - }, - "open": "2020-08-22T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34005, - "high": 43759, - "low": 9, - "open": 7843, - "volume": 3218859 - }, - "id": 2699148, - "non_hive": { - "close": 8501, - "high": 11284, - "low": 2, - "open": 1999, - "volume": 792692 - }, - "open": "2020-08-22T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11235, - "high": 11235, - "low": 158310, - "open": 1136, - "volume": 3622974 - }, - "id": 2699197, - "non_hive": { - "close": 2973, - "high": 2973, - "low": 38632, - "open": 286, - "volume": 888097 - }, - "open": "2020-08-22T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14534, - "high": 3631, - "low": 1037718, - "open": 2805, - "volume": 1301623 - }, - "id": 2699241, - "non_hive": { - "close": 3547, - "high": 922, - "low": 252168, - "open": 701, - "volume": 317096 - }, - "open": "2020-08-22T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11626, - "high": 6949, - "low": 480, - "open": 997, - "volume": 299707 - }, - "id": 2699282, - "non_hive": { - "close": 2964, - "high": 1772, - "low": 119, - "open": 249, - "volume": 75318 - }, - "open": "2020-08-22T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29426, - "high": 8936, - "low": 53, - "open": 8936, - "volume": 449504 - }, - "id": 2699332, - "non_hive": { - "close": 7342, - "high": 2261, - "low": 13, - "open": 2261, - "volume": 112093 - }, - "open": "2020-08-22T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 446, - "high": 36333, - "low": 29, - "open": 29, - "volume": 805318 - }, - "id": 2699363, - "non_hive": { - "close": 111, - "high": 9374, - "low": 7, - "open": 7, - "volume": 205263 - }, - "open": "2020-08-22T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 275, - "high": 624, - "low": 48, - "open": 1446, - "volume": 428019 - }, - "id": 2699387, - "non_hive": { - "close": 69, - "high": 161, - "low": 11, - "open": 371, - "volume": 110061 - }, - "open": "2020-08-23T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1224, - "high": 2147, - "low": 37454, - "open": 7916, - "volume": 2462969 - }, - "id": 2699429, - "non_hive": { - "close": 312, - "high": 554, - "low": 9176, - "open": 2000, - "volume": 614068 - }, - "open": "2020-08-23T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 7810, - "low": 47, - "open": 7812, - "volume": 1345610 - }, - "id": 2699458, - "non_hive": { - "close": 1, - "high": 2000, - "low": 11, - "open": 1999, - "volume": 330354 - }, - "open": "2020-08-23T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 268004, - "high": 268004, - "low": 1176969, - "open": 2959, - "volume": 2762129 - }, - "id": 2699495, - "non_hive": { - "close": 71597, - "high": 71597, - "low": 287347, - "open": 754, - "volume": 699625 - }, - "open": "2020-08-23T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5436, - "high": 7811, - "low": 1247, - "open": 7811, - "volume": 705501 - }, - "id": 2699519, - "non_hive": { - "close": 1353, - "high": 2000, - "low": 305, - "open": 2000, - "volume": 174538 - }, - "open": "2020-08-23T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1653, - "high": 113, - "low": 11408, - "open": 8035, - "volume": 66275 - }, - "id": 2699529, - "non_hive": { - "close": 422, - "high": 29, - "low": 2839, - "open": 2000, - "volume": 16653 - }, - "open": "2020-08-23T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7874, - "high": 191, - "low": 349471, - "open": 2005, - "volume": 1356290 - }, - "id": 2699552, - "non_hive": { - "close": 2000, - "high": 49, - "low": 84924, - "open": 512, - "volume": 335078 - }, - "open": "2020-08-23T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3377, - "high": 663, - "low": 2649, - "open": 663, - "volume": 25093 - }, - "id": 2699568, - "non_hive": { - "close": 851, - "high": 169, - "low": 667, - "open": 169, - "volume": 6336 - }, - "open": "2020-08-23T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127, - "high": 7932, - "low": 921, - "open": 7932, - "volume": 63666 - }, - "id": 2699592, - "non_hive": { - "close": 32, - "high": 2000, - "low": 225, - "open": 2000, - "volume": 16024 - }, - "open": "2020-08-23T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1534, - "high": 5889, - "low": 266256, - "open": 4293, - "volume": 1274447 - }, - "id": 2699615, - "non_hive": { - "close": 388, - "high": 1501, - "low": 64429, - "open": 1080, - "volume": 311224 - }, - "open": "2020-08-23T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19087, - "high": 5177, - "low": 983111, - "open": 180, - "volume": 1480269 - }, - "id": 2699648, - "non_hive": { - "close": 4676, - "high": 1305, - "low": 237898, - "open": 45, - "volume": 359631 - }, - "open": "2020-08-23T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 190806, - "high": 23785, - "low": 54551, - "open": 23785, - "volume": 4928521 - }, - "id": 2699671, - "non_hive": { - "close": 45793, - "high": 5824, - "low": 13043, - "open": 5824, - "volume": 1180287 - }, - "open": "2020-08-23T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6390, - "high": 6390, - "low": 33139, - "open": 8332, - "volume": 1433403 - }, - "id": 2699699, - "non_hive": { - "close": 1622, - "high": 1622, - "low": 7903, - "open": 2000, - "volume": 344591 - }, - "open": "2020-08-23T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3783, - "high": 24, - "low": 913824, - "open": 24, - "volume": 1578487 - }, - "id": 2699735, - "non_hive": { - "close": 926, - "high": 6, - "low": 218000, - "open": 6, - "volume": 378919 - }, - "open": "2020-08-23T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16662, - "high": 38605, - "low": 101, - "open": 265, - "volume": 95564 - }, - "id": 2699794, - "non_hive": { - "close": 4029, - "high": 9446, - "low": 24, - "open": 64, - "volume": 23331 - }, - "open": "2020-08-23T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15347, - "high": 554, - "low": 22637, - "open": 13767, - "volume": 3810292 - }, - "id": 2699815, - "non_hive": { - "close": 3708, - "high": 134, - "low": 5412, - "open": 3329, - "volume": 914774 - }, - "open": "2020-08-23T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2936, - "high": 2936, - "low": 13, - "open": 13613, - "volume": 405437 - }, - "id": 2699862, - "non_hive": { - "close": 710, - "high": 710, - "low": 3, - "open": 3289, - "volume": 97960 - }, - "open": "2020-08-23T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14302, - "high": 12, - "low": 366405, - "open": 23003, - "volume": 2389047 - }, - "id": 2699917, - "non_hive": { - "close": 3502, - "high": 3, - "low": 87937, - "open": 5562, - "volume": 575846 - }, - "open": "2020-08-23T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8074, - "high": 130, - "low": 109, - "open": 8168, - "volume": 704524 - }, - "id": 2699967, - "non_hive": { - "close": 1953, - "high": 32, - "low": 26, - "open": 1999, - "volume": 171264 - }, - "open": "2020-08-23T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16800, - "high": 73, - "low": 406, - "open": 32501, - "volume": 1829816 - }, - "id": 2699999, - "non_hive": { - "close": 4112, - "high": 18, - "low": 98, - "open": 7857, - "volume": 444728 - }, - "open": "2020-08-23T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22524, - "high": 5000, - "low": 12000, - "open": 14471, - "volume": 75125 - }, - "id": 2700047, - "non_hive": { - "close": 5513, - "high": 1224, - "low": 2937, - "open": 3542, - "volume": 18388 - }, - "open": "2020-08-23T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6315, - "high": 116, - "low": 197, - "open": 1634, - "volume": 1445383 - }, - "id": 2700067, - "non_hive": { - "close": 1578, - "high": 29, - "low": 48, - "open": 400, - "volume": 353988 - }, - "open": "2020-08-23T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10970, - "high": 2586, - "low": 15, - "open": 2586, - "volume": 177889 - }, - "id": 2700098, - "non_hive": { - "close": 2656, - "high": 646, - "low": 3, - "open": 646, - "volume": 44226 - }, - "open": "2020-08-23T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 446, - "high": 36, - "low": 25, - "open": 25, - "volume": 704850 - }, - "id": 2700121, - "non_hive": { - "close": 108, - "high": 9, - "low": 6, - "open": 6, - "volume": 174864 - }, - "open": "2020-08-23T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7244, - "high": 6135, - "low": 83218, - "open": 16782, - "volume": 209784 - }, - "id": 2700145, - "non_hive": { - "close": 1810, - "high": 1533, - "low": 20155, - "open": 4193, - "volume": 51766 - }, - "open": "2020-08-24T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 976, - "high": 1304, - "low": 25078, - "open": 25078, - "volume": 46719 - }, - "id": 2700171, - "non_hive": { - "close": 244, - "high": 326, - "low": 6265, - "open": 6265, - "volume": 11673 - }, - "open": "2020-08-24T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15222, - "high": 1452, - "low": 83348, - "open": 104244, - "volume": 1620981 - }, - "id": 2700187, - "non_hive": { - "close": 3803, - "high": 363, - "low": 20179, - "open": 26047, - "volume": 398617 - }, - "open": "2020-08-24T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60548, - "high": 1072, - "low": 60548, - "open": 5003, - "volume": 73758 - }, - "id": 2700218, - "non_hive": { - "close": 15127, - "high": 268, - "low": 15127, - "open": 1250, - "volume": 18428 - }, - "open": "2020-08-24T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4235, - "high": 1172, - "low": 4215, - "open": 5407, - "volume": 140001 - }, - "id": 2700236, - "non_hive": { - "close": 1058, - "high": 293, - "low": 1053, - "open": 1351, - "volume": 34978 - }, - "open": "2020-08-24T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4322, - "high": 1556, - "low": 63136, - "open": 10198, - "volume": 688903 - }, - "id": 2700262, - "non_hive": { - "close": 1058, - "high": 389, - "low": 15323, - "open": 2548, - "volume": 168530 - }, - "open": "2020-08-24T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2694, - "high": 44, - "low": 2641, - "open": 1675, - "volume": 176163 - }, - "id": 2700308, - "non_hive": { - "close": 672, - "high": 11, - "low": 646, - "open": 418, - "volume": 43785 - }, - "open": "2020-08-24T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4343, - "high": 5433, - "low": 2483, - "open": 4318, - "volume": 322770 - }, - "id": 2700359, - "non_hive": { - "close": 1072, - "high": 1355, - "low": 605, - "open": 1062, - "volume": 80109 - }, - "open": "2020-08-24T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 324, - "low": 47, - "open": 4339, - "volume": 243025 - }, - "id": 2700416, - "non_hive": { - "close": 2487, - "high": 81, - "low": 11, - "open": 1067, - "volume": 60482 - }, - "open": "2020-08-24T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4258, - "high": 13145, - "low": 34, - "open": 4299, - "volume": 272223 - }, - "id": 2700479, - "non_hive": { - "close": 1051, - "high": 3287, - "low": 8, - "open": 1053, - "volume": 67699 - }, - "open": "2020-08-24T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4254, - "high": 691, - "low": 18, - "open": 7027, - "volume": 320608 - }, - "id": 2700529, - "non_hive": { - "close": 1063, - "high": 173, - "low": 4, - "open": 1757, - "volume": 80134 - }, - "open": "2020-08-24T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 173, - "high": 1019, - "low": 173, - "open": 17496, - "volume": 2100345 - }, - "id": 2700589, - "non_hive": { - "close": 42, - "high": 261, - "low": 42, - "open": 4375, - "volume": 525705 - }, - "open": "2020-08-24T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1877, - "high": 1941, - "low": 1122, - "open": 4996, - "volume": 203459 - }, - "id": 2700646, - "non_hive": { - "close": 469, - "high": 499, - "low": 274, - "open": 1269, - "volume": 51898 - }, - "open": "2020-08-24T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4273, - "high": 852, - "low": 238, - "open": 852, - "volume": 1020990 - }, - "id": 2700699, - "non_hive": { - "close": 1082, - "high": 219, - "low": 58, - "open": 219, - "volume": 253442 - }, - "open": "2020-08-24T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2886, - "high": 23, - "low": 2362, - "open": 4274, - "volume": 650168 - }, - "id": 2700758, - "non_hive": { - "close": 717, - "high": 6, - "low": 586, - "open": 1082, - "volume": 164440 - }, - "open": "2020-08-24T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4887, - "high": 38448, - "low": 4258, - "open": 4303, - "volume": 1451623 - }, - "id": 2700812, - "non_hive": { - "close": 1236, - "high": 9728, - "low": 1058, - "open": 1080, - "volume": 364206 - }, - "open": "2020-08-24T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4279, - "high": 29387, - "low": 570, - "open": 5502, - "volume": 516452 - }, - "id": 2700871, - "non_hive": { - "close": 1082, - "high": 7436, - "low": 142, - "open": 1392, - "volume": 130453 - }, - "open": "2020-08-24T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1883, - "high": 133, - "low": 25, - "open": 1004, - "volume": 211833 - }, - "id": 2700936, - "non_hive": { - "close": 478, - "high": 34, - "low": 6, - "open": 254, - "volume": 53745 - }, - "open": "2020-08-24T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6383, - "high": 1717, - "low": 2319, - "open": 4412, - "volume": 966945 - }, - "id": 2701007, - "non_hive": { - "close": 1613, - "high": 438, - "low": 586, - "open": 1116, - "volume": 245590 - }, - "open": "2020-08-24T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 66223, - "high": 54, - "low": 4304, - "open": 1821, - "volume": 1157839 - }, - "id": 2701078, - "non_hive": { - "close": 16868, - "high": 14, - "low": 1076, - "open": 464, - "volume": 294151 - }, - "open": "2020-08-24T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1572, - "high": 23, - "low": 574, - "open": 4277, - "volume": 330102 - }, - "id": 2701147, - "non_hive": { - "close": 401, - "high": 6, - "low": 144, - "open": 1074, - "volume": 84001 - }, - "open": "2020-08-24T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1215, - "high": 54, - "low": 553, - "open": 4221, - "volume": 164601 - }, - "id": 2701218, - "non_hive": { - "close": 310, - "high": 14, - "low": 139, - "open": 1068, - "volume": 41864 - }, - "open": "2020-08-24T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3471, - "high": 109, - "low": 654, - "open": 1362, - "volume": 104478 - }, - "id": 2701276, - "non_hive": { - "close": 878, - "high": 28, - "low": 165, - "open": 347, - "volume": 26551 - }, - "open": "2020-08-24T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4268, - "high": 499, - "low": 28, - "open": 7843, - "volume": 1142226 - }, - "id": 2701330, - "non_hive": { - "close": 1094, - "high": 129, - "low": 7, - "open": 1999, - "volume": 291178 - }, - "open": "2020-08-24T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 277, - "high": 3, - "low": 12, - "open": 3532, - "volume": 2157173 - }, - "id": 2701396, - "non_hive": { - "close": 70, - "high": 1, - "low": 3, - "open": 906, - "volume": 547320 - }, - "open": "2020-08-25T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4309, - "high": 3009, - "low": 4, - "open": 3159, - "volume": 142479 - }, - "id": 2701449, - "non_hive": { - "close": 1120, - "high": 787, - "low": 1, - "open": 800, - "volume": 36739 - }, - "open": "2020-08-25T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 619, - "high": 7, - "low": 4, - "open": 3385, - "volume": 311327 - }, - "id": 2701499, - "non_hive": { - "close": 163, - "high": 2, - "low": 1, - "open": 880, - "volume": 81532 - }, - "open": "2020-08-25T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4205, - "high": 5800, - "low": 68, - "open": 4209, - "volume": 62497 - }, - "id": 2701545, - "non_hive": { - "close": 1065, - "high": 1525, - "low": 17, - "open": 1085, - "volume": 16010 - }, - "open": "2020-08-25T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4214, - "high": 6395, - "low": 34, - "open": 2900, - "volume": 108423 - }, - "id": 2701585, - "non_hive": { - "close": 1097, - "high": 1694, - "low": 8, - "open": 735, - "volume": 27787 - }, - "open": "2020-08-25T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4189, - "high": 15, - "low": 3509, - "open": 4218, - "volume": 192847 - }, - "id": 2701646, - "non_hive": { - "close": 1061, - "high": 4, - "low": 888, - "open": 1098, - "volume": 49429 - }, - "open": "2020-08-25T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4213, - "high": 45, - "low": 64, - "open": 4264, - "volume": 97751 - }, - "id": 2701753, - "non_hive": { - "close": 1115, - "high": 12, - "low": 16, - "open": 1110, - "volume": 25444 - }, - "open": "2020-08-25T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31248, - "high": 71, - "low": 457, - "open": 4193, - "volume": 220631 - }, - "id": 2701807, - "non_hive": { - "close": 8276, - "high": 19, - "low": 118, - "open": 1109, - "volume": 58346 - }, - "open": "2020-08-25T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4298, - "high": 426, - "low": 817, - "open": 4247, - "volume": 485757 - }, - "id": 2701870, - "non_hive": { - "close": 1104, - "high": 113, - "low": 209, - "open": 1124, - "volume": 125316 - }, - "open": "2020-08-25T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6098, - "high": 2984, - "low": 1470, - "open": 4298, - "volume": 122828 - }, - "id": 2701986, - "non_hive": { - "close": 1614, - "high": 790, - "low": 372, - "open": 1104, - "volume": 31976 - }, - "open": "2020-08-25T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12714, - "high": 56, - "low": 4, - "open": 213, - "volume": 659054 - }, - "id": 2702047, - "non_hive": { - "close": 3231, - "high": 15, - "low": 1, - "open": 56, - "volume": 172570 - }, - "open": "2020-08-25T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7673, - "high": 672, - "low": 4978, - "open": 672, - "volume": 436871 - }, - "id": 2702099, - "non_hive": { - "close": 1999, - "high": 178, - "low": 1265, - "open": 178, - "volume": 115072 - }, - "open": "2020-08-25T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 69558, - "high": 4627, - "low": 4444, - "open": 12648, - "volume": 467756 - }, - "id": 2702141, - "non_hive": { - "close": 18353, - "high": 1221, - "low": 1145, - "open": 3295, - "volume": 122262 - }, - "open": "2020-08-25T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 58, - "high": 348, - "low": 8677, - "open": 1902, - "volume": 593740 - }, - "id": 2702188, - "non_hive": { - "close": 15, - "high": 92, - "low": 2197, - "open": 502, - "volume": 153650 - }, - "open": "2020-08-25T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12560, - "high": 1967, - "low": 103, - "open": 7225, - "volume": 267544 - }, - "id": 2702248, - "non_hive": { - "close": 3190, - "high": 515, - "low": 26, - "open": 1850, - "volume": 68362 - }, - "open": "2020-08-25T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3911, - "high": 1527, - "low": 3911, - "open": 12548, - "volume": 1104446 - }, - "id": 2702304, - "non_hive": { - "close": 990, - "high": 395, - "low": 990, - "open": 3187, - "volume": 280123 - }, - "open": "2020-08-25T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 353, - "high": 2275, - "low": 16881, - "open": 10018, - "volume": 941338 - }, - "id": 2702353, - "non_hive": { - "close": 89, - "high": 576, - "low": 4119, - "open": 2536, - "volume": 236149 - }, - "open": "2020-08-25T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4701, - "high": 43, - "low": 4074, - "open": 8875, - "volume": 246792 - }, - "id": 2702411, - "non_hive": { - "close": 1182, - "high": 11, - "low": 1018, - "open": 2235, - "volume": 61940 - }, - "open": "2020-08-25T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17313, - "high": 1023, - "low": 4811, - "open": 1882, - "volume": 180894 - }, - "id": 2702451, - "non_hive": { - "close": 4363, - "high": 258, - "low": 1202, - "open": 473, - "volume": 45512 - }, - "open": "2020-08-25T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3610, - "high": 9076, - "low": 3343, - "open": 7937, - "volume": 698213 - }, - "id": 2702491, - "non_hive": { - "close": 909, - "high": 2300, - "low": 835, - "open": 1999, - "volume": 175989 - }, - "open": "2020-08-25T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4629, - "high": 106, - "low": 856, - "open": 7888, - "volume": 1800562 - }, - "id": 2702548, - "non_hive": { - "close": 1212, - "high": 28, - "low": 215, - "open": 1999, - "volume": 464012 - }, - "open": "2020-08-25T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 586, - "high": 160, - "low": 2132, - "open": 3819, - "volume": 401533 - }, - "id": 2702601, - "non_hive": { - "close": 153, - "high": 42, - "low": 546, - "open": 999, - "volume": 104530 - }, - "open": "2020-08-25T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12822, - "high": 858, - "low": 12822, - "open": 8908, - "volume": 69973 - }, - "id": 2702649, - "non_hive": { - "close": 3310, - "high": 224, - "low": 3310, - "open": 2325, - "volume": 18160 - }, - "open": "2020-08-25T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2212690, - "high": 11973, - "low": 28, - "open": 12774, - "volume": 3367192 - }, - "id": 2702674, - "non_hive": { - "close": 571377, - "high": 3125, - "low": 7, - "open": 3299, - "volume": 871857 - }, - "open": "2020-08-25T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6102, - "high": 5808, - "low": 12, - "open": 7745, - "volume": 96005 - }, - "id": 2702724, - "non_hive": { - "close": 1575, - "high": 1500, - "low": 3, - "open": 1999, - "volume": 24787 - }, - "open": "2020-08-26T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13620, - "high": 9251, - "low": 277, - "open": 277, - "volume": 135855 - }, - "id": 2702752, - "non_hive": { - "close": 3517, - "high": 2389, - "low": 70, - "open": 70, - "volume": 35079 - }, - "open": "2020-08-26T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7636, - "high": 7, - "low": 4, - "open": 921, - "volume": 240858 - }, - "id": 2702778, - "non_hive": { - "close": 1999, - "high": 2, - "low": 1, - "open": 237, - "volume": 62516 - }, - "open": "2020-08-26T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20292, - "high": 554, - "low": 1029361, - "open": 554, - "volume": 1671714 - }, - "id": 2702797, - "non_hive": { - "close": 5240, - "high": 145, - "low": 265809, - "open": 145, - "volume": 431693 - }, - "open": "2020-08-26T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 93114, - "high": 17190, - "low": 23613, - "open": 17190, - "volume": 235188 - }, - "id": 2702816, - "non_hive": { - "close": 24032, - "high": 4439, - "low": 6029, - "open": 4439, - "volume": 60617 - }, - "open": "2020-08-26T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7629, - "high": 1681, - "low": 2157, - "open": 2157, - "volume": 412688 - }, - "id": 2702842, - "non_hive": { - "close": 1969, - "high": 434, - "low": 556, - "open": 556, - "volume": 106511 - }, - "open": "2020-08-26T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 449, - "high": 449, - "low": 714, - "open": 16238, - "volume": 1423765 - }, - "id": 2702871, - "non_hive": { - "close": 116, - "high": 116, - "low": 182, - "open": 4191, - "volume": 364715 - }, - "open": "2020-08-26T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37640, - "high": 1383, - "low": 78, - "open": 124, - "volume": 60122 - }, - "id": 2702897, - "non_hive": { - "close": 9708, - "high": 357, - "low": 20, - "open": 32, - "volume": 15509 - }, - "open": "2020-08-26T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5762, - "high": 20898, - "low": 676, - "open": 20898, - "volume": 2540811 - }, - "id": 2702918, - "non_hive": { - "close": 1478, - "high": 5393, - "low": 168, - "open": 5393, - "volume": 641253 - }, - "open": "2020-08-26T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7445, - "high": 254, - "low": 3622, - "open": 7933, - "volume": 121731 - }, - "id": 2702945, - "non_hive": { - "close": 1899, - "high": 65, - "low": 890, - "open": 2000, - "volume": 30307 - }, - "open": "2020-08-26T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 58653, - "high": 4725, - "low": 12265, - "open": 4725, - "volume": 144905 - }, - "id": 2702958, - "non_hive": { - "close": 14946, - "high": 1205, - "low": 3092, - "open": 1205, - "volume": 36894 - }, - "open": "2020-08-26T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 646, - "high": 7483, - "low": 79, - "open": 8091, - "volume": 152691 - }, - "id": 2702986, - "non_hive": { - "close": 161, - "high": 1887, - "low": 19, - "open": 2000, - "volume": 38176 - }, - "open": "2020-08-26T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8092, - "high": 176, - "low": 5, - "open": 8089, - "volume": 1560348 - }, - "id": 2703021, - "non_hive": { - "close": 1975, - "high": 44, - "low": 1, - "open": 2000, - "volume": 384067 - }, - "open": "2020-08-26T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44, - "high": 4, - "low": 3225, - "open": 4245, - "volume": 228376 - }, - "id": 2703071, - "non_hive": { - "close": 11, - "high": 1, - "low": 787, - "open": 1045, - "volume": 56045 - }, - "open": "2020-08-26T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 103059, - "high": 103059, - "low": 102, - "open": 13600, - "volume": 662370 - }, - "id": 2703111, - "non_hive": { - "close": 25856, - "high": 25856, - "low": 25, - "open": 3347, - "volume": 163616 - }, - "open": "2020-08-26T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7400, - "high": 582, - "low": 7975, - "open": 4195, - "volume": 495917 - }, - "id": 2703144, - "non_hive": { - "close": 1856, - "high": 146, - "low": 1999, - "open": 1052, - "volume": 124397 - }, - "open": "2020-08-26T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22803, - "high": 237, - "low": 33, - "open": 227, - "volume": 547390 - }, - "id": 2703167, - "non_hive": { - "close": 5749, - "high": 60, - "low": 8, - "open": 57, - "volume": 135860 - }, - "open": "2020-08-26T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 641, - "high": 641, - "low": 80, - "open": 7932, - "volume": 123607 - }, - "id": 2703219, - "non_hive": { - "close": 165, - "high": 165, - "low": 19, - "open": 1999, - "volume": 31486 - }, - "open": "2020-08-26T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1652, - "high": 7003, - "low": 4633, - "open": 3985, - "volume": 107148 - }, - "id": 2703256, - "non_hive": { - "close": 425, - "high": 1802, - "low": 1168, - "open": 1012, - "volume": 27469 - }, - "open": "2020-08-26T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4859, - "high": 112, - "low": 219, - "open": 3827, - "volume": 131224 - }, - "id": 2703284, - "non_hive": { - "close": 1237, - "high": 29, - "low": 55, - "open": 984, - "volume": 33601 - }, - "open": "2020-08-26T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4245, - "high": 200, - "low": 3647, - "open": 200, - "volume": 931377 - }, - "id": 2703326, - "non_hive": { - "close": 1074, - "high": 51, - "low": 897, - "open": 51, - "volume": 231314 - }, - "open": "2020-08-26T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3828, - "high": 108, - "low": 7981, - "open": 4024, - "volume": 135298 - }, - "id": 2703361, - "non_hive": { - "close": 952, - "high": 27, - "low": 1963, - "open": 990, - "volume": 33635 - }, - "open": "2020-08-26T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 132, - "high": 132, - "low": 43, - "open": 1635, - "volume": 70971 - }, - "id": 2703396, - "non_hive": { - "close": 33, - "high": 33, - "low": 10, - "open": 407, - "volume": 17662 - }, - "open": "2020-08-26T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3050, - "high": 291807, - "low": 29, - "open": 29, - "volume": 1348487 - }, - "id": 2703418, - "non_hive": { - "close": 768, - "high": 73970, - "low": 7, - "open": 7, - "volume": 340473 - }, - "open": "2020-08-26T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 4106, - "low": 12, - "open": 4011, - "volume": 521527 - }, - "id": 2703455, - "non_hive": { - "close": 3, - "high": 1039, - "low": 3, - "open": 1013, - "volume": 131741 - }, - "open": "2020-08-27T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14545, - "high": 14545, - "low": 21795, - "open": 277, - "volume": 270150 - }, - "id": 2703475, - "non_hive": { - "close": 3687, - "high": 3687, - "low": 5489, - "open": 70, - "volume": 68271 - }, - "open": "2020-08-27T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18124, - "high": 106, - "low": 2032, - "open": 12476, - "volume": 84643 - }, - "id": 2703501, - "non_hive": { - "close": 4531, - "high": 27, - "low": 506, - "open": 3161, - "volume": 21208 - }, - "open": "2020-08-27T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100, - "high": 7999, - "low": 2399, - "open": 348, - "volume": 111843 - }, - "id": 2703522, - "non_hive": { - "close": 25, - "high": 2000, - "low": 590, - "open": 87, - "volume": 27918 - }, - "open": "2020-08-27T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60, - "high": 336, - "low": 3303, - "open": 7712, - "volume": 41120 - }, - "id": 2703542, - "non_hive": { - "close": 15, - "high": 84, - "low": 816, - "open": 1927, - "volume": 10266 - }, - "open": "2020-08-27T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 367167, - "high": 4, - "low": 47260, - "open": 481, - "volume": 743660 - }, - "id": 2703565, - "non_hive": { - "close": 90777, - "high": 1, - "low": 11679, - "open": 120, - "volume": 184333 - }, - "open": "2020-08-27T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 65, - "high": 740, - "low": 542, - "open": 8090, - "volume": 539652 - }, - "id": 2703586, - "non_hive": { - "close": 16, - "high": 185, - "low": 133, - "open": 2000, - "volume": 133494 - }, - "open": "2020-08-27T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9594, - "high": 276, - "low": 13045, - "open": 276, - "volume": 45940 - }, - "id": 2703610, - "non_hive": { - "close": 2392, - "high": 69, - "low": 3248, - "open": 69, - "volume": 11446 - }, - "open": "2020-08-27T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7842, - "high": 32, - "low": 6, - "open": 32, - "volume": 1151370 - }, - "id": 2703622, - "non_hive": { - "close": 1929, - "high": 8, - "low": 1, - "open": 8, - "volume": 285861 - }, - "open": "2020-08-27T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2267, - "high": 200, - "low": 452, - "open": 200, - "volume": 202647 - }, - "id": 2703666, - "non_hive": { - "close": 560, - "high": 50, - "low": 111, - "open": 50, - "volume": 50045 - }, - "open": "2020-08-27T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5915, - "high": 400, - "low": 67503, - "open": 400, - "volume": 2154743 - }, - "id": 2703693, - "non_hive": { - "close": 1460, - "high": 99, - "low": 16360, - "open": 99, - "volume": 524265 - }, - "open": "2020-08-27T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 815, - "high": 116, - "low": 815, - "open": 7504, - "volume": 596385 - }, - "id": 2703722, - "non_hive": { - "close": 201, - "high": 29, - "low": 201, - "open": 1853, - "volume": 148216 - }, - "open": "2020-08-27T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2863, - "high": 290, - "low": 700, - "open": 8072, - "volume": 166427 - }, - "id": 2703749, - "non_hive": { - "close": 709, - "high": 72, - "low": 171, - "open": 1999, - "volume": 41207 - }, - "open": "2020-08-27T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 197226, - "high": 674, - "low": 85936, - "open": 674, - "volume": 1642558 - }, - "id": 2703786, - "non_hive": { - "close": 48315, - "high": 167, - "low": 20827, - "open": 167, - "volume": 401774 - }, - "open": "2020-08-27T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11101, - "high": 540, - "low": 100, - "open": 204, - "volume": 358568 - }, - "id": 2703829, - "non_hive": { - "close": 2751, - "high": 134, - "low": 24, - "open": 50, - "volume": 87552 - }, - "open": "2020-08-27T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6000, - "high": 68, - "low": 6000, - "open": 201803, - "volume": 467565 - }, - "id": 2703866, - "non_hive": { - "close": 1456, - "high": 17, - "low": 1456, - "open": 49999, - "volume": 115801 - }, - "open": "2020-08-27T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40760, - "high": 1906, - "low": 13, - "open": 241, - "volume": 2015569 - }, - "id": 2703891, - "non_hive": { - "close": 9986, - "high": 471, - "low": 3, - "open": 59, - "volume": 489220 - }, - "open": "2020-08-27T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15, - "high": 4, - "low": 8, - "open": 481, - "volume": 2348440 - }, - "id": 2703932, - "non_hive": { - "close": 3, - "high": 1, - "low": 1, - "open": 118, - "volume": 568663 - }, - "open": "2020-08-27T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27323, - "high": 20, - "low": 388, - "open": 590, - "volume": 395773 - }, - "id": 2703989, - "non_hive": { - "close": 6742, - "high": 5, - "low": 92, - "open": 144, - "volume": 96607 - }, - "open": "2020-08-27T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7, - "high": 8, - "low": 7, - "open": 27433, - "volume": 628889 - }, - "id": 2704031, - "non_hive": { - "close": 1, - "high": 2, - "low": 1, - "open": 6769, - "volume": 155873 - }, - "open": "2020-08-27T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39741, - "high": 6146, - "low": 39741, - "open": 4478, - "volume": 928067 - }, - "id": 2704089, - "non_hive": { - "close": 9428, - "high": 1534, - "low": 9428, - "open": 1117, - "volume": 221760 - }, - "open": "2020-08-27T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 490991, - "high": 4, - "low": 465002, - "open": 1079, - "volume": 2117853 - }, - "id": 2704122, - "non_hive": { - "close": 115530, - "high": 1, - "low": 109414, - "open": 267, - "volume": 499395 - }, - "open": "2020-08-27T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5327, - "high": 5327, - "low": 64592, - "open": 1269, - "volume": 877687 - }, - "id": 2704167, - "non_hive": { - "close": 1325, - "high": 1325, - "low": 15017, - "open": 311, - "volume": 209894 - }, - "open": "2020-08-27T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1777, - "high": 232850, - "low": 26, - "open": 26, - "volume": 638387 - }, - "id": 2704204, - "non_hive": { - "close": 437, - "high": 57901, - "low": 6, - "open": 6, - "volume": 157256 - }, - "open": "2020-08-27T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8168, - "high": 289, - "low": 13, - "open": 138478, - "volume": 534943 - }, - "id": 2704228, - "non_hive": { - "close": 1999, - "high": 71, - "low": 3, - "open": 33929, - "volume": 130803 - }, - "open": "2020-08-28T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7757, - "high": 8, - "low": 5, - "open": 278, - "volume": 785736 - }, - "id": 2704258, - "non_hive": { - "close": 1931, - "high": 2, - "low": 1, - "open": 68, - "volume": 189231 - }, - "open": "2020-08-28T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9345, - "high": 268, - "low": 1494, - "open": 274, - "volume": 23804 - }, - "id": 2704290, - "non_hive": { - "close": 2327, - "high": 67, - "low": 366, - "open": 68, - "volume": 5920 - }, - "open": "2020-08-28T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26556, - "high": 100, - "low": 26556, - "open": 100, - "volume": 124937 - }, - "id": 2704312, - "non_hive": { - "close": 6383, - "high": 25, - "low": 6383, - "open": 25, - "volume": 30609 - }, - "open": "2020-08-28T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13611, - "high": 153, - "low": 84, - "open": 84, - "volume": 226638 - }, - "id": 2704331, - "non_hive": { - "close": 3379, - "high": 38, - "low": 20, - "open": 20, - "volume": 56232 - }, - "open": "2020-08-28T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 540, - "high": 2469, - "low": 84, - "open": 2469, - "volume": 89082 - }, - "id": 2704345, - "non_hive": { - "close": 134, - "high": 613, - "low": 20, - "open": 613, - "volume": 22107 - }, - "open": "2020-08-28T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3469, - "high": 3006, - "low": 139, - "open": 3006, - "volume": 218156 - }, - "id": 2704371, - "non_hive": { - "close": 843, - "high": 746, - "low": 33, - "open": 746, - "volume": 53751 - }, - "open": "2020-08-28T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 15718, - "open": 5164, - "volume": 673287 - }, - "id": 2704404, - "non_hive": { - "close": 1, - "high": 1, - "low": 3788, - "open": 1249, - "volume": 163191 - }, - "open": "2020-08-28T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5361, - "high": 49, - "low": 20571, - "open": 8293, - "volume": 595763 - }, - "id": 2704431, - "non_hive": { - "close": 1296, - "high": 12, - "low": 4909, - "open": 2000, - "volume": 143680 - }, - "open": "2020-08-28T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7764, - "high": 57, - "low": 104, - "open": 2909, - "volume": 275869 - }, - "id": 2704474, - "non_hive": { - "close": 1879, - "high": 14, - "low": 25, - "open": 703, - "volume": 66759 - }, - "open": "2020-08-28T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3068, - "high": 11386, - "low": 10892, - "open": 15462, - "volume": 89585 - }, - "id": 2704504, - "non_hive": { - "close": 757, - "high": 2814, - "low": 2635, - "open": 3742, - "volume": 21958 - }, - "open": "2020-08-28T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11900, - "high": 85, - "low": 5, - "open": 9001, - "volume": 171367 - }, - "id": 2704523, - "non_hive": { - "close": 2879, - "high": 21, - "low": 1, - "open": 2220, - "volume": 42007 - }, - "open": "2020-08-28T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 113, - "high": 40, - "low": 62, - "open": 2002, - "volume": 255294 - }, - "id": 2704558, - "non_hive": { - "close": 28, - "high": 10, - "low": 14, - "open": 492, - "volume": 62585 - }, - "open": "2020-08-28T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1221, - "high": 9544, - "low": 10773, - "open": 5000, - "volume": 1035781 - }, - "id": 2704598, - "non_hive": { - "close": 301, - "high": 2354, - "low": 2607, - "open": 1210, - "volume": 252080 - }, - "open": "2020-08-28T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2000, - "high": 312, - "low": 104, - "open": 227, - "volume": 20705 - }, - "id": 2704633, - "non_hive": { - "close": 489, - "high": 77, - "low": 25, - "open": 56, - "volume": 5070 - }, - "open": "2020-08-28T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1421, - "high": 105, - "low": 7744, - "open": 6578, - "volume": 193336 - }, - "id": 2704662, - "non_hive": { - "close": 349, - "high": 26, - "low": 1859, - "open": 1608, - "volume": 47168 - }, - "open": "2020-08-28T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9474, - "high": 629, - "low": 13, - "open": 8148, - "volume": 259396 - }, - "id": 2704703, - "non_hive": { - "close": 2333, - "high": 155, - "low": 3, - "open": 1999, - "volume": 63124 - }, - "open": "2020-08-28T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 300, - "high": 300, - "low": 142, - "open": 12189, - "volume": 126537 - }, - "id": 2704754, - "non_hive": { - "close": 74, - "high": 74, - "low": 34, - "open": 3001, - "volume": 31135 - }, - "open": "2020-08-28T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11207, - "high": 1866, - "low": 3879, - "open": 1866, - "volume": 207515 - }, - "id": 2704787, - "non_hive": { - "close": 2723, - "high": 459, - "low": 932, - "open": 459, - "volume": 50683 - }, - "open": "2020-08-28T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1722, - "high": 41756, - "low": 4269, - "open": 8320, - "volume": 1169798 - }, - "id": 2704819, - "non_hive": { - "close": 415, - "high": 10243, - "low": 1019, - "open": 2000, - "volume": 280956 - }, - "open": "2020-08-28T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8243, - "high": 4473, - "low": 99, - "open": 8160, - "volume": 54257 - }, - "id": 2704861, - "non_hive": { - "close": 1999, - "high": 1096, - "low": 23, - "open": 1999, - "volume": 13244 - }, - "open": "2020-08-28T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30067, - "high": 220, - "low": 142394, - "open": 1000, - "volume": 1859016 - }, - "id": 2704883, - "non_hive": { - "close": 7363, - "high": 54, - "low": 33610, - "open": 245, - "volume": 444710 - }, - "open": "2020-08-28T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6699, - "high": 102, - "low": 494, - "open": 4668, - "volume": 131992 - }, - "id": 2704911, - "non_hive": { - "close": 1639, - "high": 25, - "low": 118, - "open": 1143, - "volume": 32303 - }, - "open": "2020-08-28T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37, - "high": 8, - "low": 445, - "open": 29, - "volume": 1289976 - }, - "id": 2704935, - "non_hive": { - "close": 9, - "high": 2, - "low": 105, - "open": 7, - "volume": 313379 - }, - "open": "2020-08-28T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1254, - "high": 8, - "low": 13, - "open": 8, - "volume": 16469 - }, - "id": 2704969, - "non_hive": { - "close": 306, - "high": 2, - "low": 3, - "open": 2, - "volume": 4019 - }, - "open": "2020-08-29T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 81919, - "high": 81919, - "low": 8, - "open": 275, - "volume": 147134 - }, - "id": 2704982, - "non_hive": { - "close": 20000, - "high": 20000, - "low": 1, - "open": 65, - "volume": 35879 - }, - "open": "2020-08-29T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 927752, - "high": 927752, - "low": 43025, - "open": 5051, - "volume": 2001180 - }, - "id": 2705003, - "non_hive": { - "close": 226510, - "high": 226510, - "low": 10500, - "open": 1233, - "volume": 488543 - }, - "open": "2020-08-29T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56881, - "high": 421, - "low": 32821, - "open": 9281, - "volume": 701945 - }, - "id": 2705028, - "non_hive": { - "close": 13894, - "high": 103, - "low": 7784, - "open": 2266, - "volume": 170983 - }, - "open": "2020-08-29T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3718, - "high": 199, - "low": 38, - "open": 126, - "volume": 221786 - }, - "id": 2705057, - "non_hive": { - "close": 903, - "high": 49, - "low": 9, - "open": 31, - "volume": 54040 - }, - "open": "2020-08-29T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11995, - "high": 11995, - "low": 12962, - "open": 41278, - "volume": 141098 - }, - "id": 2705083, - "non_hive": { - "close": 2917, - "high": 2917, - "low": 3074, - "open": 10024, - "volume": 34095 - }, - "open": "2020-08-29T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1656, - "high": 407, - "low": 4228, - "open": 4000, - "volume": 317184 - }, - "id": 2705099, - "non_hive": { - "close": 406, - "high": 100, - "low": 1027, - "open": 972, - "volume": 77260 - }, - "open": "2020-08-29T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 263981, - "high": 4313, - "low": 395, - "open": 4313, - "volume": 492272 - }, - "id": 2705152, - "non_hive": { - "close": 64280, - "high": 1057, - "low": 96, - "open": 1057, - "volume": 119975 - }, - "open": "2020-08-29T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1370, - "high": 8, - "low": 26, - "open": 2000, - "volume": 164220 - }, - "id": 2705177, - "non_hive": { - "close": 328, - "high": 2, - "low": 6, - "open": 487, - "volume": 39917 - }, - "open": "2020-08-29T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3876, - "high": 800, - "low": 40477, - "open": 932, - "volume": 292079 - }, - "id": 2705216, - "non_hive": { - "close": 935, - "high": 196, - "low": 9755, - "open": 228, - "volume": 71173 - }, - "open": "2020-08-29T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5392, - "high": 1388, - "low": 1653, - "open": 1388, - "volume": 196006 - }, - "id": 2705244, - "non_hive": { - "close": 1318, - "high": 340, - "low": 399, - "open": 340, - "volume": 47858 - }, - "open": "2020-08-29T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8197, - "high": 237, - "low": 4142, - "open": 1975, - "volume": 337961 - }, - "id": 2705279, - "non_hive": { - "close": 1999, - "high": 58, - "low": 982, - "open": 482, - "volume": 81474 - }, - "open": "2020-08-29T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4219, - "high": 28, - "low": 12789, - "open": 2279, - "volume": 117976 - }, - "id": 2705312, - "non_hive": { - "close": 1024, - "high": 7, - "low": 3033, - "open": 556, - "volume": 28506 - }, - "open": "2020-08-29T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 650, - "high": 650, - "low": 16, - "open": 762, - "volume": 316006 - }, - "id": 2705334, - "non_hive": { - "close": 158, - "high": 158, - "low": 3, - "open": 184, - "volume": 75786 - }, - "open": "2020-08-29T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21419, - "high": 16, - "low": 54, - "open": 23144, - "volume": 228595 - }, - "id": 2705360, - "non_hive": { - "close": 5102, - "high": 4, - "low": 12, - "open": 5622, - "volume": 55053 - }, - "open": "2020-08-29T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6649, - "high": 49, - "low": 36443, - "open": 2043, - "volume": 236682 - }, - "id": 2705394, - "non_hive": { - "close": 1611, - "high": 12, - "low": 8681, - "open": 495, - "volume": 57019 - }, - "open": "2020-08-29T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39573, - "high": 11455, - "low": 13, - "open": 587, - "volume": 529326 - }, - "id": 2705424, - "non_hive": { - "close": 9596, - "high": 2778, - "low": 3, - "open": 142, - "volume": 128308 - }, - "open": "2020-08-29T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3587, - "high": 131, - "low": 100000, - "open": 12958, - "volume": 304894 - }, - "id": 2705461, - "non_hive": { - "close": 870, - "high": 32, - "low": 24200, - "open": 3142, - "volume": 73882 - }, - "open": "2020-08-29T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9500, - "high": 45, - "low": 9500, - "open": 11345, - "volume": 161904 - }, - "id": 2705494, - "non_hive": { - "close": 2298, - "high": 11, - "low": 2298, - "open": 2751, - "volume": 39234 - }, - "open": "2020-08-29T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13299, - "high": 2886, - "low": 1234, - "open": 8569, - "volume": 510728 - }, - "id": 2705531, - "non_hive": { - "close": 3217, - "high": 700, - "low": 298, - "open": 2078, - "volume": 123691 - }, - "open": "2020-08-29T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40988, - "high": 32, - "low": 2874, - "open": 1353, - "volume": 234518 - }, - "id": 2705581, - "non_hive": { - "close": 10001, - "high": 8, - "low": 696, - "open": 328, - "volume": 57065 - }, - "open": "2020-08-29T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1127, - "high": 16, - "low": 232, - "open": 8451, - "volume": 135855 - }, - "id": 2705613, - "non_hive": { - "close": 275, - "high": 4, - "low": 56, - "open": 2062, - "volume": 33115 - }, - "open": "2020-08-29T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12979, - "high": 102, - "low": 3000, - "open": 102, - "volume": 25868 - }, - "id": 2705650, - "non_hive": { - "close": 3167, - "high": 25, - "low": 731, - "open": 25, - "volume": 6311 - }, - "open": "2020-08-29T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3212, - "high": 261, - "low": 29, - "open": 29, - "volume": 1271179 - }, - "id": 2705663, - "non_hive": { - "close": 787, - "high": 64, - "low": 7, - "open": 7, - "volume": 310395 - }, - "open": "2020-08-29T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 91804, - "high": 6443, - "low": 13, - "open": 5779, - "volume": 132333 - }, - "id": 2705690, - "non_hive": { - "close": 22398, - "high": 1585, - "low": 3, - "open": 1416, - "volume": 32321 - }, - "open": "2020-08-30T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1885, - "high": 5129, - "low": 279, - "open": 279, - "volume": 55210 - }, - "id": 2705704, - "non_hive": { - "close": 460, - "high": 1261, - "low": 68, - "open": 68, - "volume": 13480 - }, - "open": "2020-08-30T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 52439, - "high": 1852, - "low": 1376, - "open": 164932, - "volume": 799004 - }, - "id": 2705720, - "non_hive": { - "close": 12796, - "high": 452, - "low": 332, - "open": 40241, - "volume": 194186 - }, - "open": "2020-08-30T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1015388, - "high": 1015388, - "low": 132590, - "open": 18019, - "volume": 1165997 - }, - "id": 2705742, - "non_hive": { - "close": 247776, - "high": 247776, - "low": 32354, - "open": 4397, - "volume": 284527 - }, - "open": "2020-08-30T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4654, - "high": 4, - "low": 4654, - "open": 22658, - "volume": 61639 - }, - "id": 2705749, - "non_hive": { - "close": 1126, - "high": 1, - "low": 1126, - "open": 5529, - "volume": 15012 - }, - "open": "2020-08-30T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49619, - "high": 53, - "low": 196, - "open": 824, - "volume": 384228 - }, - "id": 2705761, - "non_hive": { - "close": 12002, - "high": 13, - "low": 47, - "open": 201, - "volume": 93630 - }, - "open": "2020-08-30T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 348, - "high": 348, - "low": 8143, - "open": 8143, - "volume": 67236 - }, - "id": 2705777, - "non_hive": { - "close": 85, - "high": 85, - "low": 1986, - "open": 1986, - "volume": 16406 - }, - "open": "2020-08-30T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7413, - "high": 4, - "low": 225, - "open": 2738, - "volume": 125440 - }, - "id": 2705793, - "non_hive": { - "close": 1809, - "high": 1, - "low": 54, - "open": 668, - "volume": 30573 - }, - "open": "2020-08-30T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9293, - "high": 52073, - "low": 509, - "open": 52073, - "volume": 228404 - }, - "id": 2705833, - "non_hive": { - "close": 2264, - "high": 12707, - "low": 124, - "open": 12707, - "volume": 55722 - }, - "open": "2020-08-30T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 344, - "high": 344, - "low": 9167, - "open": 58428, - "volume": 231113 - }, - "id": 2705859, - "non_hive": { - "close": 84, - "high": 84, - "low": 2233, - "open": 14234, - "volume": 56364 - }, - "open": "2020-08-30T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5602, - "high": 8601, - "low": 246, - "open": 3450, - "volume": 669413 - }, - "id": 2705882, - "non_hive": { - "close": 1367, - "high": 2099, - "low": 59, - "open": 840, - "volume": 163337 - }, - "open": "2020-08-30T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8200, - "high": 7995, - "low": 47000, - "open": 7995, - "volume": 411433 - }, - "id": 2705920, - "non_hive": { - "close": 2001, - "high": 1951, - "low": 11468, - "open": 1951, - "volume": 100391 - }, - "open": "2020-08-30T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 663, - "high": 65, - "low": 11472, - "open": 65, - "volume": 124958 - }, - "id": 2705947, - "non_hive": { - "close": 162, - "high": 16, - "low": 2787, - "open": 16, - "volume": 30470 - }, - "open": "2020-08-30T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7524, - "high": 1561, - "low": 8127, - "open": 19696, - "volume": 129241 - }, - "id": 2705978, - "non_hive": { - "close": 1836, - "high": 381, - "low": 1983, - "open": 4806, - "volume": 31536 - }, - "open": "2020-08-30T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 684, - "high": 233, - "low": 396, - "open": 360, - "volume": 96174 - }, - "id": 2706004, - "non_hive": { - "close": 167, - "high": 57, - "low": 96, - "open": 88, - "volume": 23467 - }, - "open": "2020-08-30T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1269, - "high": 438, - "low": 1269, - "open": 211194, - "volume": 981139 - }, - "id": 2706036, - "non_hive": { - "close": 309, - "high": 107, - "low": 309, - "open": 51531, - "volume": 239404 - }, - "open": "2020-08-30T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13550, - "high": 2467, - "low": 13, - "open": 29, - "volume": 32764 - }, - "id": 2706074, - "non_hive": { - "close": 3305, - "high": 602, - "low": 3, - "open": 7, - "volume": 7992 - }, - "open": "2020-08-30T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 557, - "high": 4, - "low": 557, - "open": 4, - "volume": 505513 - }, - "id": 2706096, - "non_hive": { - "close": 135, - "high": 1, - "low": 135, - "open": 1, - "volume": 123342 - }, - "open": "2020-08-30T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51528, - "high": 4, - "low": 70, - "open": 8954, - "volume": 3086550 - }, - "id": 2706128, - "non_hive": { - "close": 12567, - "high": 1, - "low": 17, - "open": 2185, - "volume": 753223 - }, - "open": "2020-08-30T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 666, - "high": 4, - "low": 176281, - "open": 8226, - "volume": 353627 - }, - "id": 2706207, - "non_hive": { - "close": 163, - "high": 1, - "low": 42847, - "open": 2000, - "volume": 86173 - }, - "open": "2020-08-30T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 784, - "open": 45366, - "volume": 1245332 - }, - "id": 2706249, - "non_hive": { - "close": 1, - "high": 1, - "low": 191, - "open": 11092, - "volume": 304761 - }, - "open": "2020-08-30T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6101, - "high": 4, - "low": 198, - "open": 1902, - "volume": 39536 - }, - "id": 2706299, - "non_hive": { - "close": 1494, - "high": 1, - "low": 48, - "open": 466, - "volume": 9663 - }, - "open": "2020-08-30T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1973, - "high": 5341, - "low": 19780, - "open": 72699, - "volume": 178293 - }, - "id": 2706330, - "non_hive": { - "close": 483, - "high": 1308, - "low": 4811, - "open": 17801, - "volume": 43612 - }, - "open": "2020-08-30T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 444, - "high": 10956, - "low": 29, - "open": 29, - "volume": 669545 - }, - "id": 2706360, - "non_hive": { - "close": 108, - "high": 2684, - "low": 7, - "open": 7, - "volume": 163991 - }, - "open": "2020-08-30T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27387, - "high": 2032, - "low": 13, - "open": 769, - "volume": 42900 - }, - "id": 2706376, - "non_hive": { - "close": 6708, - "high": 498, - "low": 3, - "open": 188, - "volume": 10508 - }, - "open": "2020-08-31T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6019, - "high": 351, - "low": 6019, - "open": 278, - "volume": 87283 - }, - "id": 2706394, - "non_hive": { - "close": 1464, - "high": 86, - "low": 1464, - "open": 68, - "volume": 21356 - }, - "open": "2020-08-31T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3576, - "high": 81, - "low": 69110, - "open": 81, - "volume": 183214 - }, - "id": 2706418, - "non_hive": { - "close": 876, - "high": 20, - "low": 16862, - "open": 20, - "volume": 44812 - }, - "open": "2020-08-31T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6531, - "high": 726, - "low": 20412, - "open": 15055, - "volume": 96048 - }, - "id": 2706433, - "non_hive": { - "close": 1600, - "high": 178, - "low": 5000, - "open": 3688, - "volume": 23528 - }, - "open": "2020-08-31T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8157, - "high": 5278, - "low": 86, - "open": 5278, - "volume": 103404 - }, - "id": 2706453, - "non_hive": { - "close": 1998, - "high": 1293, - "low": 21, - "open": 1293, - "volume": 25328 - }, - "open": "2020-08-31T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12003, - "high": 28, - "low": 4736, - "open": 669, - "volume": 26649 - }, - "id": 2706481, - "non_hive": { - "close": 2940, - "high": 7, - "low": 1159, - "open": 164, - "volume": 6526 - }, - "open": "2020-08-31T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2367, - "high": 4, - "low": 6607, - "open": 2523, - "volume": 375362 - }, - "id": 2706494, - "non_hive": { - "close": 580, - "high": 1, - "low": 1608, - "open": 618, - "volume": 91931 - }, - "open": "2020-08-31T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 478235, - "high": 5012, - "low": 154, - "open": 4296, - "volume": 1535250 - }, - "id": 2706529, - "non_hive": { - "close": 117138, - "high": 1228, - "low": 37, - "open": 1052, - "volume": 376027 - }, - "open": "2020-08-31T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4391, - "high": 114, - "low": 24415, - "open": 47775, - "volume": 206544 - }, - "id": 2706566, - "non_hive": { - "close": 1076, - "high": 28, - "low": 5980, - "open": 11702, - "volume": 50599 - }, - "open": "2020-08-31T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 334, - "high": 334, - "low": 2737, - "open": 6057, - "volume": 434189 - }, - "id": 2706593, - "non_hive": { - "close": 82, - "high": 82, - "low": 670, - "open": 1484, - "volume": 106376 - }, - "open": "2020-08-31T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51441, - "high": 134, - "low": 10589, - "open": 134, - "volume": 4853992 - }, - "id": 2706621, - "non_hive": { - "close": 12654, - "high": 33, - "low": 2578, - "open": 33, - "volume": 1189319 - }, - "open": "2020-08-31T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 790, - "high": 32, - "low": 940, - "open": 151, - "volume": 169423 - }, - "id": 2706674, - "non_hive": { - "close": 195, - "high": 8, - "low": 229, - "open": 37, - "volume": 41842 - }, - "open": "2020-08-31T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13888, - "high": 214, - "low": 1952, - "open": 793, - "volume": 94199 - }, - "id": 2706708, - "non_hive": { - "close": 3430, - "high": 53, - "low": 480, - "open": 196, - "volume": 23263 - }, - "open": "2020-08-31T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7324, - "high": 32, - "low": 9440, - "open": 9440, - "volume": 197277 - }, - "id": 2706743, - "non_hive": { - "close": 1809, - "high": 8, - "low": 2331, - "open": 2331, - "volume": 48725 - }, - "open": "2020-08-31T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22674, - "high": 12, - "low": 102, - "open": 413, - "volume": 245097 - }, - "id": 2706773, - "non_hive": { - "close": 5638, - "high": 3, - "low": 25, - "open": 102, - "volume": 60823 - }, - "open": "2020-08-31T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3000, - "high": 3000, - "low": 24794, - "open": 5131, - "volume": 320355 - }, - "id": 2706823, - "non_hive": { - "close": 754, - "high": 754, - "low": 6157, - "open": 1277, - "volume": 79926 - }, - "open": "2020-08-31T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7000, - "high": 35, - "low": 28640, - "open": 2782, - "volume": 818047 - }, - "id": 2706877, - "non_hive": { - "close": 1772, - "high": 9, - "low": 7113, - "open": 701, - "volume": 206771 - }, - "open": "2020-08-31T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2421, - "high": 90, - "low": 7, - "open": 900, - "volume": 850167 - }, - "id": 2706941, - "non_hive": { - "close": 613, - "high": 23, - "low": 1, - "open": 228, - "volume": 215195 - }, - "open": "2020-08-31T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5779, - "high": 28374, - "low": 5779, - "open": 17972, - "volume": 1236353 - }, - "id": 2706995, - "non_hive": { - "close": 1463, - "high": 7184, - "low": 1463, - "open": 4550, - "volume": 313008 - }, - "open": "2020-08-31T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4155, - "high": 1299, - "low": 142, - "open": 19754, - "volume": 135879 - }, - "id": 2707026, - "non_hive": { - "close": 1052, - "high": 329, - "low": 35, - "open": 5001, - "volume": 34395 - }, - "open": "2020-08-31T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27722, - "high": 94, - "low": 256, - "open": 23408, - "volume": 662235 - }, - "id": 2707062, - "non_hive": { - "close": 7015, - "high": 24, - "low": 64, - "open": 5925, - "volume": 167622 - }, - "open": "2020-08-31T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7973, - "high": 3, - "low": 3933, - "open": 2442, - "volume": 172065 - }, - "id": 2707110, - "non_hive": { - "close": 2000, - "high": 1, - "low": 976, - "open": 618, - "volume": 43404 - }, - "open": "2020-08-31T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27323, - "high": 7, - "low": 26, - "open": 7978, - "volume": 325640 - }, - "id": 2707125, - "non_hive": { - "close": 6914, - "high": 2, - "low": 6, - "open": 1999, - "volume": 82184 - }, - "open": "2020-08-31T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15565, - "high": 39, - "low": 229, - "open": 28, - "volume": 1014695 - }, - "id": 2707176, - "non_hive": { - "close": 3940, - "high": 10, - "low": 56, - "open": 7, - "volume": 256618 - }, - "open": "2020-08-31T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2552, - "high": 1086, - "low": 13, - "open": 37945, - "volume": 5718335 - }, - "id": 2707215, - "non_hive": { - "close": 633, - "high": 275, - "low": 3, - "open": 9605, - "volume": 1415226 - }, - "open": "2020-09-01T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1807, - "high": 3955, - "low": 80488, - "open": 23827, - "volume": 2754760 - }, - "id": 2707257, - "non_hive": { - "close": 448, - "high": 1001, - "low": 19478, - "open": 5909, - "volume": 674911 - }, - "open": "2020-09-01T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12098, - "high": 209, - "low": 64, - "open": 209, - "volume": 4057894 - }, - "id": 2707303, - "non_hive": { - "close": 3000, - "high": 52, - "low": 15, - "open": 52, - "volume": 986076 - }, - "open": "2020-09-01T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 849, - "high": 23, - "low": 20, - "open": 90660, - "volume": 3047913 - }, - "id": 2707363, - "non_hive": { - "close": 209, - "high": 6, - "low": 4, - "open": 22479, - "volume": 753984 - }, - "open": "2020-09-01T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1170813, - "high": 43, - "low": 42, - "open": 7977, - "volume": 5465160 - }, - "id": 2707412, - "non_hive": { - "close": 285699, - "high": 11, - "low": 10, - "open": 1999, - "volume": 1339488 - }, - "open": "2020-09-01T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 64, - "high": 88830, - "low": 256, - "open": 8070, - "volume": 2604274 - }, - "id": 2707454, - "non_hive": { - "close": 16, - "high": 22284, - "low": 62, - "open": 1999, - "volume": 636738 - }, - "open": "2020-09-01T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 66500, - "high": 8, - "low": 18, - "open": 7541, - "volume": 3079651 - }, - "id": 2707481, - "non_hive": { - "close": 16492, - "high": 2, - "low": 4, - "open": 1879, - "volume": 754808 - }, - "open": "2020-09-01T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40002, - "high": 40, - "low": 66, - "open": 8026, - "volume": 1494219 - }, - "id": 2707535, - "non_hive": { - "close": 9964, - "high": 10, - "low": 16, - "open": 1999, - "volume": 366041 - }, - "open": "2020-09-01T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 317, - "high": 8, - "low": 8, - "open": 8, - "volume": 58450 - }, - "id": 2707576, - "non_hive": { - "close": 79, - "high": 2, - "low": 1, - "open": 2, - "volume": 14556 - }, - "open": "2020-09-01T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 193, - "high": 196, - "low": 56, - "open": 369272, - "volume": 3145094 - }, - "id": 2707595, - "non_hive": { - "close": 48, - "high": 49, - "low": 13, - "open": 91940, - "volume": 774786 - }, - "open": "2020-09-01T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55664, - "high": 1578, - "low": 8, - "open": 8030, - "volume": 260749 - }, - "id": 2707643, - "non_hive": { - "close": 13853, - "high": 393, - "low": 1, - "open": 1999, - "volume": 64895 - }, - "open": "2020-09-01T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17467, - "high": 8, - "low": 84, - "open": 17089, - "volume": 2645007 - }, - "id": 2707673, - "non_hive": { - "close": 4228, - "high": 2, - "low": 20, - "open": 4253, - "volume": 640179 - }, - "open": "2020-09-01T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7346, - "high": 52, - "low": 51, - "open": 17353, - "volume": 2857852 - }, - "id": 2707715, - "non_hive": { - "close": 1792, - "high": 13, - "low": 12, - "open": 4301, - "volume": 686908 - }, - "open": "2020-09-01T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20877, - "high": 56, - "low": 46, - "open": 25048, - "volume": 2346402 - }, - "id": 2707798, - "non_hive": { - "close": 5172, - "high": 14, - "low": 11, - "open": 6110, - "volume": 569138 - }, - "open": "2020-09-01T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 322609, - "high": 56, - "low": 1172719, - "open": 327, - "volume": 5894735 - }, - "id": 2707860, - "non_hive": { - "close": 79917, - "high": 14, - "low": 279129, - "open": 80, - "volume": 1421868 - }, - "open": "2020-09-01T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 678, - "high": 193, - "low": 142, - "open": 14343, - "volume": 1308966 - }, - "id": 2707938, - "non_hive": { - "close": 167, - "high": 48, - "low": 34, - "open": 3553, - "volume": 319913 - }, - "open": "2020-09-01T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6008, - "high": 20, - "low": 13, - "open": 5003, - "volume": 731065 - }, - "id": 2708004, - "non_hive": { - "close": 1487, - "high": 5, - "low": 3, - "open": 1231, - "volume": 179827 - }, - "open": "2020-09-01T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7000, - "high": 68, - "low": 1183604, - "open": 68, - "volume": 2882736 - }, - "id": 2708050, - "non_hive": { - "close": 1725, - "high": 17, - "low": 282927, - "open": 17, - "volume": 697388 - }, - "open": "2020-09-01T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 400000, - "high": 5263, - "low": 632944, - "open": 7000, - "volume": 4075379 - }, - "id": 2708118, - "non_hive": { - "close": 98000, - "high": 1303, - "low": 151931, - "open": 1725, - "volume": 984284 - }, - "open": "2020-09-01T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14198, - "high": 88, - "low": 25028, - "open": 88, - "volume": 1916675 - }, - "id": 2708168, - "non_hive": { - "close": 3507, - "high": 22, - "low": 6008, - "open": 22, - "volume": 464668 - }, - "open": "2020-09-01T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 73768, - "high": 222, - "low": 395573, - "open": 178, - "volume": 1370323 - }, - "id": 2708208, - "non_hive": { - "close": 18269, - "high": 55, - "low": 97297, - "open": 44, - "volume": 337663 - }, - "open": "2020-09-01T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3752, - "high": 8, - "low": 94, - "open": 12502, - "volume": 4091584 - }, - "id": 2708259, - "non_hive": { - "close": 906, - "high": 2, - "low": 22, - "open": 3096, - "volume": 995520 - }, - "open": "2020-09-01T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8691, - "high": 60, - "low": 281, - "open": 60, - "volume": 123165 - }, - "id": 2708324, - "non_hive": { - "close": 2164, - "high": 15, - "low": 69, - "open": 15, - "volume": 30633 - }, - "open": "2020-09-01T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3333, - "high": 63, - "low": 446, - "open": 5300, - "volume": 2530748 - }, - "id": 2708358, - "non_hive": { - "close": 800, - "high": 16, - "low": 107, - "open": 1319, - "volume": 623679 - }, - "open": "2020-09-01T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 338, - "high": 15020, - "low": 13, - "open": 15020, - "volume": 15371 - }, - "id": 2708394, - "non_hive": { - "close": 83, - "high": 3748, - "low": 3, - "open": 3748, - "volume": 3834 - }, - "open": "2020-09-02T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3392, - "high": 1086, - "low": 112, - "open": 277, - "volume": 1237025 - }, - "id": 2708405, - "non_hive": { - "close": 846, - "high": 271, - "low": 26, - "open": 67, - "volume": 295486 - }, - "open": "2020-09-02T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2855, - "high": 1512, - "low": 2855, - "open": 1512, - "volume": 200312 - }, - "id": 2708439, - "non_hive": { - "close": 711, - "high": 377, - "low": 711, - "open": 377, - "volume": 49900 - }, - "open": "2020-09-02T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4358, - "high": 6112, - "low": 82268, - "open": 17207, - "volume": 253193 - }, - "id": 2708461, - "non_hive": { - "close": 1074, - "high": 1534, - "low": 19867, - "open": 4283, - "volume": 61986 - }, - "open": "2020-09-02T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 1166797, - "open": 1295, - "volume": 5042378 - }, - "id": 2708487, - "non_hive": { - "close": 1, - "high": 1, - "low": 272097, - "open": 312, - "volume": 1186373 - }, - "open": "2020-09-02T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 279298, - "high": 9906, - "low": 28, - "open": 9906, - "volume": 2489609 - }, - "id": 2708539, - "non_hive": { - "close": 65526, - "high": 2394, - "low": 6, - "open": 2394, - "volume": 584814 - }, - "open": "2020-09-02T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 329, - "high": 8, - "low": 23, - "open": 7801, - "volume": 7628615 - }, - "id": 2708560, - "non_hive": { - "close": 79, - "high": 2, - "low": 5, - "open": 1880, - "volume": 1787389 - }, - "open": "2020-09-02T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 776, - "high": 483, - "low": 114783, - "open": 4582, - "volume": 2864422 - }, - "id": 2708621, - "non_hive": { - "close": 186, - "high": 116, - "low": 26769, - "open": 1100, - "volume": 670420 - }, - "open": "2020-09-02T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 819, - "high": 9487, - "low": 378626, - "open": 5090, - "volume": 2751190 - }, - "id": 2708653, - "non_hive": { - "close": 196, - "high": 2275, - "low": 88300, - "open": 1220, - "volume": 643908 - }, - "open": "2020-09-02T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 819, - "high": 355, - "low": 1167552, - "open": 58505, - "volume": 2484242 - }, - "id": 2708678, - "non_hive": { - "close": 196, - "high": 85, - "low": 270886, - "open": 14000, - "volume": 578304 - }, - "open": "2020-09-02T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4699, - "high": 898, - "low": 312552, - "open": 9181, - "volume": 5683998 - }, - "id": 2708697, - "non_hive": { - "close": 1123, - "high": 215, - "low": 71278, - "open": 2196, - "volume": 1309405 - }, - "open": "2020-09-02T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 165, - "high": 5612, - "low": 1520, - "open": 11455, - "volume": 7361285 - }, - "id": 2708732, - "non_hive": { - "close": 39, - "high": 1341, - "low": 342, - "open": 2737, - "volume": 1660620 - }, - "open": "2020-09-02T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5572, - "high": 4420, - "low": 5572, - "open": 5131, - "volume": 7471595 - }, - "id": 2708769, - "non_hive": { - "close": 1253, - "high": 1043, - "low": 1253, - "open": 1206, - "volume": 1682784 - }, - "open": "2020-09-02T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40073, - "high": 4650, - "low": 610218, - "open": 13943, - "volume": 9426621 - }, - "id": 2708814, - "non_hive": { - "close": 9000, - "high": 1090, - "low": 134858, - "open": 3268, - "volume": 2113617 - }, - "open": "2020-09-02T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 720070, - "high": 2506, - "low": 36949, - "open": 17621, - "volume": 8131713 - }, - "id": 2708867, - "non_hive": { - "close": 159935, - "high": 584, - "low": 8167, - "open": 3957, - "volume": 1811553 - }, - "open": "2020-09-02T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3472, - "high": 4021, - "low": 39193, - "open": 1361, - "volume": 8399378 - }, - "id": 2708939, - "non_hive": { - "close": 802, - "high": 937, - "low": 8633, - "open": 313, - "volume": 1870441 - }, - "open": "2020-09-02T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12315, - "high": 4, - "low": 28, - "open": 5281, - "volume": 12794783 - }, - "id": 2709008, - "non_hive": { - "close": 2793, - "high": 1, - "low": 6, - "open": 1222, - "volume": 2836665 - }, - "open": "2020-09-02T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20084, - "high": 8557, - "low": 1200000, - "open": 3056, - "volume": 6276882 - }, - "id": 2709084, - "non_hive": { - "close": 4551, - "high": 1941, - "low": 260535, - "open": 693, - "volume": 1374657 - }, - "open": "2020-09-02T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24410, - "high": 10836, - "low": 400389, - "open": 20685, - "volume": 5275814 - }, - "id": 2709126, - "non_hive": { - "close": 5457, - "high": 2453, - "low": 87687, - "open": 4551, - "volume": 1157937 - }, - "open": "2020-09-02T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10524, - "high": 18093, - "low": 21, - "open": 20430, - "volume": 2338197 - }, - "id": 2709179, - "non_hive": { - "close": 2385, - "high": 4104, - "low": 4, - "open": 4563, - "volume": 522598 - }, - "open": "2020-09-02T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 61, - "high": 4, - "low": 66, - "open": 20400, - "volume": 1821634 - }, - "id": 2709246, - "non_hive": { - "close": 13, - "high": 1, - "low": 14, - "open": 4623, - "volume": 408868 - }, - "open": "2020-09-02T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1376, - "high": 114, - "low": 101, - "open": 6440, - "volume": 3176066 - }, - "id": 2709317, - "non_hive": { - "close": 312, - "high": 26, - "low": 22, - "open": 1460, - "volume": 706911 - }, - "open": "2020-09-02T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 253336, - "high": 392, - "low": 482, - "open": 23190, - "volume": 598617 - }, - "id": 2709384, - "non_hive": { - "close": 57376, - "high": 89, - "low": 107, - "open": 5255, - "volume": 135596 - }, - "open": "2020-09-02T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 106399, - "high": 2113, - "low": 97, - "open": 561, - "volume": 2201622 - }, - "id": 2709418, - "non_hive": { - "close": 24000, - "high": 486, - "low": 21, - "open": 127, - "volume": 495791 - }, - "open": "2020-09-02T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 107665, - "high": 34, - "low": 14, - "open": 8866, - "volume": 6500481 - }, - "id": 2709468, - "non_hive": { - "close": 23901, - "high": 8, - "low": 3, - "open": 2000, - "volume": 1446249 - }, - "open": "2020-09-03T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5040, - "high": 19850, - "low": 275, - "open": 10117, - "volume": 4724463 - }, - "id": 2709506, - "non_hive": { - "close": 1149, - "high": 4550, - "low": 61, - "open": 2319, - "volume": 1055218 - }, - "open": "2020-09-03T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13177, - "high": 135999, - "low": 15897, - "open": 135999, - "volume": 1397907 - }, - "id": 2709549, - "non_hive": { - "close": 3000, - "high": 31000, - "low": 3544, - "open": 31000, - "volume": 313021 - }, - "open": "2020-09-03T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17155, - "high": 4691, - "low": 5, - "open": 5, - "volume": 1480134 - }, - "id": 2709568, - "non_hive": { - "close": 3908, - "high": 1069, - "low": 1, - "open": 1, - "volume": 331060 - }, - "open": "2020-09-03T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2262, - "high": 153, - "low": 87, - "open": 4000, - "volume": 1356957 - }, - "id": 2709603, - "non_hive": { - "close": 515, - "high": 35, - "low": 19, - "open": 911, - "volume": 300361 - }, - "open": "2020-09-03T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 140, - "high": 105, - "low": 36354, - "open": 1002, - "volume": 1008650 - }, - "id": 2709626, - "non_hive": { - "close": 32, - "high": 24, - "low": 7999, - "open": 228, - "volume": 223645 - }, - "open": "2020-09-03T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 586, - "high": 67, - "low": 250, - "open": 67, - "volume": 2551259 - }, - "id": 2709660, - "non_hive": { - "close": 131, - "high": 15, - "low": 54, - "open": 15, - "volume": 552452 - }, - "open": "2020-09-03T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127, - "high": 127, - "low": 8946, - "open": 2870, - "volume": 89648 - }, - "id": 2709697, - "non_hive": { - "close": 29, - "high": 29, - "low": 1997, - "open": 641, - "volume": 20110 - }, - "open": "2020-09-03T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15470, - "high": 5929, - "low": 99302, - "open": 3000, - "volume": 316959 - }, - "id": 2709724, - "non_hive": { - "close": 3507, - "high": 1352, - "low": 21466, - "open": 674, - "volume": 70804 - }, - "open": "2020-09-03T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90768, - "high": 88, - "low": 90768, - "open": 100466, - "volume": 3193933 - }, - "id": 2709758, - "non_hive": { - "close": 19881, - "high": 20, - "low": 19881, - "open": 22604, - "volume": 711226 - }, - "open": "2020-09-03T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 355533, - "high": 114, - "low": 355533, - "open": 2128, - "volume": 2093535 - }, - "id": 2709793, - "non_hive": { - "close": 76812, - "high": 26, - "low": 76812, - "open": 482, - "volume": 456330 - }, - "open": "2020-09-03T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18598, - "high": 15385, - "low": 18598, - "open": 3000, - "volume": 3808231 - }, - "id": 2709819, - "non_hive": { - "close": 3961, - "high": 3483, - "low": 3961, - "open": 673, - "volume": 828575 - }, - "open": "2020-09-03T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1200000, - "high": 204, - "low": 118141, - "open": 4665, - "volume": 6702768 - }, - "id": 2709854, - "non_hive": { - "close": 253286, - "high": 46, - "low": 24933, - "open": 1049, - "volume": 1420644 - }, - "open": "2020-09-03T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8452, - "high": 9, - "low": 156598, - "open": 3042, - "volume": 7347086 - }, - "id": 2709892, - "non_hive": { - "close": 1868, - "high": 2, - "low": 33121, - "open": 673, - "volume": 1556814 - }, - "open": "2020-09-03T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4, - "high": 4, - "low": 100, - "open": 294, - "volume": 6350852 - }, - "id": 2709938, - "non_hive": { - "close": 1, - "high": 1, - "low": 21, - "open": 65, - "volume": 1349073 - }, - "open": "2020-09-03T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3229, - "high": 49, - "low": 2770, - "open": 3154, - "volume": 4990665 - }, - "id": 2709994, - "non_hive": { - "close": 717, - "high": 11, - "low": 587, - "open": 700, - "volume": 1064786 - }, - "open": "2020-09-03T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 418, - "high": 4, - "low": 6, - "open": 415, - "volume": 423169 - }, - "id": 2710046, - "non_hive": { - "close": 93, - "high": 1, - "low": 1, - "open": 92, - "volume": 91359 - }, - "open": "2020-09-03T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4503, - "high": 126, - "low": 74999, - "open": 13519, - "volume": 859036 - }, - "id": 2710083, - "non_hive": { - "close": 999, - "high": 28, - "low": 16034, - "open": 3000, - "volume": 184579 - }, - "open": "2020-09-03T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5140, - "high": 9, - "low": 36732, - "open": 6497, - "volume": 3448492 - }, - "id": 2710104, - "non_hive": { - "close": 1128, - "high": 2, - "low": 7732, - "open": 1441, - "volume": 732237 - }, - "open": "2020-09-03T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6930, - "high": 9, - "low": 23662, - "open": 18233, - "volume": 2196936 - }, - "id": 2710162, - "non_hive": { - "close": 1498, - "high": 2, - "low": 4968, - "open": 4000, - "volume": 467261 - }, - "open": "2020-09-03T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17448, - "high": 134, - "low": 20301, - "open": 11452, - "volume": 426398 - }, - "id": 2710218, - "non_hive": { - "close": 3768, - "high": 29, - "low": 4267, - "open": 2475, - "volume": 90549 - }, - "open": "2020-09-03T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13146, - "high": 9, - "low": 7619, - "open": 24218, - "volume": 247874 - }, - "id": 2710256, - "non_hive": { - "close": 2841, - "high": 2, - "low": 1600, - "open": 5230, - "volume": 53697 - }, - "open": "2020-09-03T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49860, - "high": 4962, - "low": 49860, - "open": 11573, - "volume": 1861276 - }, - "id": 2710287, - "non_hive": { - "close": 10370, - "high": 1086, - "low": 10370, - "open": 2488, - "volume": 391437 - }, - "open": "2020-09-03T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38171, - "high": 9, - "low": 28309, - "open": 144, - "volume": 6356988 - }, - "id": 2710341, - "non_hive": { - "close": 8017, - "high": 2, - "low": 5661, - "open": 30, - "volume": 1309397 - }, - "open": "2020-09-03T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48165, - "high": 9, - "low": 310, - "open": 1738, - "volume": 813846 - }, - "id": 2710414, - "non_hive": { - "close": 9682, - "high": 2, - "low": 62, - "open": 379, - "volume": 164729 - }, - "open": "2020-09-04T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7355, - "high": 9, - "low": 10651, - "open": 47959, - "volume": 328752 - }, - "id": 2710448, - "non_hive": { - "close": 1573, - "high": 2, - "low": 2141, - "open": 9998, - "volume": 69200 - }, - "open": "2020-09-04T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43505, - "high": 9, - "low": 43505, - "open": 1992, - "volume": 6332444 - }, - "id": 2710490, - "non_hive": { - "close": 8574, - "high": 2, - "low": 8574, - "open": 426, - "volume": 1270111 - }, - "open": "2020-09-04T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 110641, - "high": 110641, - "low": 2000, - "open": 4805, - "volume": 211833 - }, - "id": 2710525, - "non_hive": { - "close": 23203, - "high": 23203, - "low": 394, - "open": 999, - "volume": 44224 - }, - "open": "2020-09-04T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6525, - "high": 6238, - "low": 6525, - "open": 6238, - "volume": 52706 - }, - "id": 2710557, - "non_hive": { - "close": 1305, - "high": 1308, - "low": 1305, - "open": 1308, - "volume": 10873 - }, - "open": "2020-09-04T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 649, - "high": 201542, - "low": 30848, - "open": 14415, - "volume": 1090871 - }, - "id": 2710572, - "non_hive": { - "close": 134, - "high": 41966, - "low": 6169, - "open": 2994, - "volume": 222844 - }, - "open": "2020-09-04T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100235, - "high": 9, - "low": 83, - "open": 13000, - "volume": 836445 - }, - "id": 2710613, - "non_hive": { - "close": 19946, - "high": 2, - "low": 16, - "open": 2681, - "volume": 167617 - }, - "open": "2020-09-04T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 147017, - "high": 5948, - "low": 3632, - "open": 5948, - "volume": 1358571 - }, - "id": 2710653, - "non_hive": { - "close": 29420, - "high": 1222, - "low": 701, - "open": 1222, - "volume": 268193 - }, - "open": "2020-09-04T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15100, - "high": 1914, - "low": 4941, - "open": 10334, - "volume": 1314753 - }, - "id": 2710709, - "non_hive": { - "close": 3000, - "high": 383, - "low": 938, - "open": 2000, - "volume": 251774 - }, - "open": "2020-09-04T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6809, - "high": 6809, - "low": 653, - "open": 10077, - "volume": 395608 - }, - "id": 2710750, - "non_hive": { - "close": 1368, - "high": 1368, - "low": 128, - "open": 2000, - "volume": 78576 - }, - "open": "2020-09-04T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4930, - "high": 78, - "low": 10146, - "open": 3142, - "volume": 1339146 - }, - "id": 2710790, - "non_hive": { - "close": 1001, - "high": 16, - "low": 1978, - "open": 631, - "volume": 262108 - }, - "open": "2020-09-04T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21929, - "high": 22700, - "low": 19, - "open": 1848, - "volume": 908154 - }, - "id": 2710836, - "non_hive": { - "close": 4369, - "high": 4722, - "low": 3, - "open": 375, - "volume": 182438 - }, - "open": "2020-09-04T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 973749, - "high": 87, - "low": 673963, - "open": 291, - "volume": 6920898 - }, - "id": 2710881, - "non_hive": { - "close": 188366, - "high": 18, - "low": 130374, - "open": 58, - "volume": 1344709 - }, - "open": "2020-09-04T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7868, - "high": 18987, - "low": 15, - "open": 20000, - "volume": 3169901 - }, - "id": 2710944, - "non_hive": { - "close": 1580, - "high": 3816, - "low": 2, - "open": 3868, - "volume": 627227 - }, - "open": "2020-09-04T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5017, - "high": 134, - "low": 62, - "open": 9956, - "volume": 5773119 - }, - "id": 2711013, - "non_hive": { - "close": 1001, - "high": 27, - "low": 12, - "open": 1999, - "volume": 1139338 - }, - "open": "2020-09-04T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1099834, - "high": 10, - "low": 1099834, - "open": 10145, - "volume": 12109020 - }, - "id": 2711077, - "non_hive": { - "close": 210071, - "high": 2, - "low": 210071, - "open": 2000, - "volume": 2344094 - }, - "open": "2020-09-04T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1189363, - "high": 30, - "low": 14, - "open": 100166, - "volume": 10919203 - }, - "id": 2711159, - "non_hive": { - "close": 223600, - "high": 6, - "low": 2, - "open": 19132, - "volume": 2064928 - }, - "open": "2020-09-04T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2346, - "high": 25, - "low": 918872, - "open": 10301, - "volume": 10648096 - }, - "id": 2711239, - "non_hive": { - "close": 455, - "high": 5, - "low": 170037, - "open": 1999, - "volume": 1991386 - }, - "open": "2020-09-04T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 443728, - "high": 90, - "low": 443728, - "open": 10751, - "volume": 4679220 - }, - "id": 2711308, - "non_hive": { - "close": 82538, - "high": 18, - "low": 82538, - "open": 2088, - "volume": 885259 - }, - "open": "2020-09-04T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4140, - "high": 33982, - "low": 519792, - "open": 10025, - "volume": 2743149 - }, - "id": 2711388, - "non_hive": { - "close": 795, - "high": 6782, - "low": 97218, - "open": 1999, - "volume": 518880 - }, - "open": "2020-09-04T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 110535, - "high": 125, - "low": 110535, - "open": 14000, - "volume": 4998742 - }, - "id": 2711453, - "non_hive": { - "close": 21443, - "high": 25, - "low": 21443, - "open": 2785, - "volume": 983541 - }, - "open": "2020-09-04T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13552, - "high": 11429, - "low": 28, - "open": 71, - "volume": 2001698 - }, - "id": 2711523, - "non_hive": { - "close": 2707, - "high": 2283, - "low": 5, - "open": 14, - "volume": 386616 - }, - "open": "2020-09-04T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3303, - "high": 65, - "low": 99, - "open": 7945, - "volume": 223418 - }, - "id": 2711586, - "non_hive": { - "close": 654, - "high": 13, - "low": 19, - "open": 1587, - "volume": 44471 - }, - "open": "2020-09-04T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2241, - "high": 576460, - "low": 438804, - "open": 15153, - "volume": 6289778 - }, - "id": 2711626, - "non_hive": { - "close": 445, - "high": 131433, - "low": 83811, - "open": 3000, - "volume": 1247675 - }, - "open": "2020-09-04T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 4982, - "low": 11, - "open": 12182, - "volume": 1384190 - }, - "id": 2711692, - "non_hive": { - "close": 2, - "high": 991, - "low": 2, - "open": 2418, - "volume": 269491 - }, - "open": "2020-09-05T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15113, - "high": 23211, - "low": 1043546, - "open": 15102, - "volume": 1361051 - }, - "id": 2711726, - "non_hive": { - "close": 3000, - "high": 4617, - "low": 202523, - "open": 3000, - "volume": 265504 - }, - "open": "2020-09-05T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22969, - "high": 1384, - "low": 1152860, - "open": 15113, - "volume": 3690066 - }, - "id": 2711762, - "non_hive": { - "close": 4557, - "high": 275, - "low": 223780, - "open": 3000, - "volume": 716769 - }, - "open": "2020-09-05T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 800, - "high": 800, - "low": 447, - "open": 2752, - "volume": 101338 - }, - "id": 2711793, - "non_hive": { - "close": 159, - "high": 159, - "low": 88, - "open": 546, - "volume": 20114 - }, - "open": "2020-09-05T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23229, - "high": 448, - "low": 41, - "open": 6091, - "volume": 220669 - }, - "id": 2711811, - "non_hive": { - "close": 4614, - "high": 89, - "low": 8, - "open": 1210, - "volume": 43831 - }, - "open": "2020-09-05T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 75, - "high": 15, - "low": 798945, - "open": 15, - "volume": 1658276 - }, - "id": 2711847, - "non_hive": { - "close": 15, - "high": 3, - "low": 154211, - "open": 3, - "volume": 323138 - }, - "open": "2020-09-05T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18290, - "high": 256, - "low": 810883, - "open": 15103, - "volume": 2610838 - }, - "id": 2711896, - "non_hive": { - "close": 3633, - "high": 51, - "low": 156515, - "open": 3000, - "volume": 505230 - }, - "open": "2020-09-05T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 203902, - "high": 11586, - "low": 203902, - "open": 10, - "volume": 1550007 - }, - "id": 2711937, - "non_hive": { - "close": 38741, - "high": 2375, - "low": 38741, - "open": 2, - "volume": 300754 - }, - "open": "2020-09-05T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11586, - "high": 430, - "low": 36653, - "open": 9907, - "volume": 2842130 - }, - "id": 2711966, - "non_hive": { - "close": 2304, - "high": 87, - "low": 6817, - "open": 1999, - "volume": 540889 - }, - "open": "2020-09-05T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1269, - "high": 7960, - "low": 1189065, - "open": 10072, - "volume": 2795006 - }, - "id": 2712022, - "non_hive": { - "close": 248, - "high": 1580, - "low": 217874, - "open": 1999, - "volume": 519079 - }, - "open": "2020-09-05T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42, - "high": 2797, - "low": 15841, - "open": 2797, - "volume": 1042135 - }, - "id": 2712062, - "non_hive": { - "close": 8, - "high": 546, - "low": 2992, - "open": 546, - "volume": 202644 - }, - "open": "2020-09-05T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 114, - "high": 5273, - "low": 972489, - "open": 15841, - "volume": 1495408 - }, - "id": 2712089, - "non_hive": { - "close": 21, - "high": 1001, - "low": 178258, - "open": 2986, - "volume": 275721 - }, - "open": "2020-09-05T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15961, - "high": 52, - "low": 138, - "open": 2240, - "volume": 406531 - }, - "id": 2712139, - "non_hive": { - "close": 3001, - "high": 10, - "low": 25, - "open": 425, - "volume": 76861 - }, - "open": "2020-09-05T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2448, - "high": 10, - "low": 196524, - "open": 26035, - "volume": 1839104 - }, - "id": 2712194, - "non_hive": { - "close": 464, - "high": 2, - "low": 36043, - "open": 4895, - "volume": 340268 - }, - "open": "2020-09-05T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15862, - "high": 15830, - "low": 102, - "open": 15830, - "volume": 273043 - }, - "id": 2712248, - "non_hive": { - "close": 3000, - "high": 3000, - "low": 19, - "open": 3000, - "volume": 51691 - }, - "open": "2020-09-05T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7413, - "high": 79, - "low": 10, - "open": 79, - "volume": 188472 - }, - "id": 2712291, - "non_hive": { - "close": 1401, - "high": 15, - "low": 1, - "open": 15, - "volume": 35617 - }, - "open": "2020-09-05T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16667, - "high": 412, - "low": 7, - "open": 15875, - "volume": 8946332 - }, - "id": 2712327, - "non_hive": { - "close": 3000, - "high": 78, - "low": 1, - "open": 3000, - "volume": 1628140 - }, - "open": "2020-09-05T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 985343, - "high": 63, - "low": 583711, - "open": 10604, - "volume": 4954710 - }, - "id": 2712383, - "non_hive": { - "close": 176381, - "high": 12, - "low": 102751, - "open": 1999, - "volume": 887313 - }, - "open": "2020-09-05T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 446, - "high": 10856, - "low": 573930, - "open": 6975, - "volume": 9970113 - }, - "id": 2712445, - "non_hive": { - "close": 82, - "high": 2000, - "low": 98744, - "open": 1272, - "volume": 1755449 - }, - "open": "2020-09-05T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2682, - "high": 237596, - "low": 43, - "open": 1947, - "volume": 6516493 - }, - "id": 2712517, - "non_hive": { - "close": 490, - "high": 44752, - "low": 7, - "open": 357, - "volume": 1129152 - }, - "open": "2020-09-05T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5518, - "high": 59, - "low": 420011, - "open": 4333, - "volume": 2924873 - }, - "id": 2712575, - "non_hive": { - "close": 1008, - "high": 11, - "low": 71408, - "open": 792, - "volume": 504901 - }, - "open": "2020-09-05T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6012, - "high": 10806, - "low": 11274, - "open": 218, - "volume": 1330290 - }, - "id": 2712608, - "non_hive": { - "close": 1098, - "high": 2000, - "low": 1917, - "open": 40, - "volume": 227526 - }, - "open": "2020-09-05T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13730, - "high": 1062, - "low": 588248, - "open": 1062, - "volume": 4005542 - }, - "id": 2712640, - "non_hive": { - "close": 2484, - "high": 194, - "low": 100086, - "open": 194, - "volume": 686296 - }, - "open": "2020-09-05T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 278426, - "high": 10, - "low": 24, - "open": 868, - "volume": 8353280 - }, - "id": 2712672, - "non_hive": { - "close": 47369, - "high": 2, - "low": 4, - "open": 157, - "volume": 1437835 - }, - "open": "2020-09-05T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2038, - "high": 1401, - "low": 12, - "open": 10661, - "volume": 7301875 - }, - "id": 2712744, - "non_hive": { - "close": 375, - "high": 262, - "low": 2, - "open": 1950, - "volume": 1244522 - }, - "open": "2020-09-06T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4091, - "high": 5, - "low": 21023, - "open": 14931, - "volume": 3914108 - }, - "id": 2712786, - "non_hive": { - "close": 753, - "high": 1, - "low": 3573, - "open": 2760, - "volume": 669173 - }, - "open": "2020-09-06T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16738, - "high": 10, - "low": 241, - "open": 10870, - "volume": 10332054 - }, - "id": 2712842, - "non_hive": { - "close": 2956, - "high": 2, - "low": 40, - "open": 1999, - "volume": 1810918 - }, - "open": "2020-09-06T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 98616, - "high": 90, - "low": 98616, - "open": 36444, - "volume": 7242208 - }, - "id": 2712896, - "non_hive": { - "close": 16370, - "high": 16, - "low": 16370, - "open": 6050, - "volume": 1202881 - }, - "open": "2020-09-06T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5803, - "high": 5, - "low": 85, - "open": 11555, - "volume": 7829695 - }, - "id": 2712932, - "non_hive": { - "close": 1001, - "high": 1, - "low": 14, - "open": 1999, - "volume": 1299882 - }, - "open": "2020-09-06T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6998, - "high": 11, - "low": 550635, - "open": 17423, - "volume": 6327946 - }, - "id": 2712987, - "non_hive": { - "close": 1211, - "high": 2, - "low": 88679, - "open": 2992, - "volume": 1036008 - }, - "open": "2020-09-06T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12005, - "high": 11, - "low": 426775, - "open": 12413, - "volume": 8435321 - }, - "id": 2713035, - "non_hive": { - "close": 2000, - "high": 2, - "low": 68744, - "open": 2000, - "volume": 1373822 - }, - "open": "2020-09-06T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8957, - "high": 10, - "low": 1044393, - "open": 743, - "volume": 5271517 - }, - "id": 2713141, - "non_hive": { - "close": 1514, - "high": 2, - "low": 170262, - "open": 126, - "volume": 865008 - }, - "open": "2020-09-06T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54, - "high": 59, - "low": 25, - "open": 10996, - "volume": 10158249 - }, - "id": 2713207, - "non_hive": { - "close": 10, - "high": 11, - "low": 4, - "open": 1999, - "volume": 1865995 - }, - "open": "2020-09-06T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 631, - "high": 10, - "low": 12709, - "open": 1200000, - "volume": 3347676 - }, - "id": 2713259, - "non_hive": { - "close": 118, - "high": 2, - "low": 2269, - "open": 220519, - "volume": 615157 - }, - "open": "2020-09-06T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11415, - "high": 10, - "low": 56583, - "open": 10729, - "volume": 949615 - }, - "id": 2713309, - "non_hive": { - "close": 2000, - "high": 2, - "low": 9908, - "open": 1999, - "volume": 172358 - }, - "open": "2020-09-06T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 609, - "high": 10, - "low": 927742, - "open": 11415, - "volume": 1599558 - }, - "id": 2713358, - "non_hive": { - "close": 110, - "high": 2, - "low": 157719, - "open": 2000, - "volume": 276000 - }, - "open": "2020-09-06T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24563, - "high": 16, - "low": 11161, - "open": 16, - "volume": 167202 - }, - "id": 2713410, - "non_hive": { - "close": 4401, - "high": 3, - "low": 1999, - "open": 3, - "volume": 29998 - }, - "open": "2020-09-06T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5982, - "high": 2530, - "low": 227368, - "open": 7720, - "volume": 3327207 - }, - "id": 2713452, - "non_hive": { - "close": 1132, - "high": 479, - "low": 39108, - "open": 1381, - "volume": 577434 - }, - "open": "2020-09-06T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 739, - "high": 12938, - "low": 140851, - "open": 18000, - "volume": 2015903 - }, - "id": 2713535, - "non_hive": { - "close": 133, - "high": 2448, - "low": 24227, - "open": 3387, - "volume": 353476 - }, - "open": "2020-09-06T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6655, - "high": 10, - "low": 95213, - "open": 10007, - "volume": 834140 - }, - "id": 2713615, - "non_hive": { - "close": 1195, - "high": 2, - "low": 16471, - "open": 1880, - "volume": 150948 - }, - "open": "2020-09-06T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2001, - "high": 142, - "low": 27, - "open": 11141, - "volume": 5023698 - }, - "id": 2713668, - "non_hive": { - "close": 376, - "high": 27, - "low": 4, - "open": 1999, - "volume": 894898 - }, - "open": "2020-09-06T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4148, - "high": 2518, - "low": 268703, - "open": 2518, - "volume": 5785025 - }, - "id": 2713739, - "non_hive": { - "close": 775, - "high": 473, - "low": 46760, - "open": 473, - "volume": 1032365 - }, - "open": "2020-09-06T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 632, - "high": 2482, - "low": 1160969, - "open": 91, - "volume": 5344749 - }, - "id": 2713776, - "non_hive": { - "close": 116, - "high": 457, - "low": 203173, - "open": 16, - "volume": 946352 - }, - "open": "2020-09-06T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23121, - "high": 1903, - "low": 3000, - "open": 16913, - "volume": 3142556 - }, - "id": 2713822, - "non_hive": { - "close": 4251, - "high": 350, - "low": 531, - "open": 3108, - "volume": 559950 - }, - "open": "2020-09-06T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2560, - "high": 86, - "low": 732053, - "open": 16321, - "volume": 2008974 - }, - "id": 2713868, - "non_hive": { - "close": 471, - "high": 16, - "low": 130689, - "open": 3000, - "volume": 361091 - }, - "open": "2020-09-06T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20185, - "high": 35843, - "low": 125, - "open": 17832, - "volume": 2304028 - }, - "id": 2713921, - "non_hive": { - "close": 3708, - "high": 6593, - "low": 22, - "open": 3280, - "volume": 417721 - }, - "open": "2020-09-06T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7897, - "high": 5, - "low": 6104, - "open": 3043, - "volume": 664879 - }, - "id": 2713972, - "non_hive": { - "close": 1453, - "high": 1, - "low": 1121, - "open": 559, - "volume": 122259 - }, - "open": "2020-09-06T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3761, - "high": 70, - "low": 28, - "open": 163274, - "volume": 556633 - }, - "id": 2714005, - "non_hive": { - "close": 692, - "high": 13, - "low": 5, - "open": 30037, - "volume": 102405 - }, - "open": "2020-09-06T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 1434, - "low": 11, - "open": 9811, - "volume": 75780 - }, - "id": 2714047, - "non_hive": { - "close": 2, - "high": 264, - "low": 2, - "open": 1805, - "volume": 13941 - }, - "open": "2020-09-07T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5, - "high": 5, - "low": 479037, - "open": 278, - "volume": 1274566 - }, - "id": 2714072, - "non_hive": { - "close": 1, - "high": 1, - "low": 84752, - "open": 51, - "volume": 227952 - }, - "open": "2020-09-07T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32855, - "high": 202941, - "low": 119, - "open": 10882, - "volume": 4717115 - }, - "id": 2714098, - "non_hive": { - "close": 6000, - "high": 37309, - "low": 21, - "open": 1999, - "volume": 853270 - }, - "open": "2020-09-07T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 84, - "high": 223, - "low": 143, - "open": 10949, - "volume": 3837311 - }, - "id": 2714123, - "non_hive": { - "close": 15, - "high": 41, - "low": 25, - "open": 2000, - "volume": 692819 - }, - "open": "2020-09-07T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 271525, - "high": 5, - "low": 49, - "open": 10874, - "volume": 997292 - }, - "id": 2714164, - "non_hive": { - "close": 49889, - "high": 1, - "low": 8, - "open": 1999, - "volume": 183230 - }, - "open": "2020-09-07T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 778, - "high": 464, - "low": 21118, - "open": 605, - "volume": 2657563 - }, - "id": 2714192, - "non_hive": { - "close": 144, - "high": 86, - "low": 3839, - "open": 111, - "volume": 489717 - }, - "open": "2020-09-07T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3232, - "high": 718, - "low": 95285, - "open": 718, - "volume": 7444004 - }, - "id": 2714231, - "non_hive": { - "close": 585, - "high": 133, - "low": 16960, - "open": 133, - "volume": 1326885 - }, - "open": "2020-09-07T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11025, - "high": 27, - "low": 8, - "open": 397, - "volume": 22690527 - }, - "id": 2714269, - "non_hive": { - "close": 1994, - "high": 5, - "low": 1, - "open": 72, - "volume": 4037371 - }, - "open": "2020-09-07T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54, - "high": 2348, - "low": 54, - "open": 5130, - "volume": 5060915 - }, - "id": 2714330, - "non_hive": { - "close": 9, - "high": 425, - "low": 9, - "open": 928, - "volume": 898125 - }, - "open": "2020-09-07T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5531, - "high": 33, - "low": 9, - "open": 11200, - "volume": 7476300 - }, - "id": 2714371, - "non_hive": { - "close": 1001, - "high": 6, - "low": 1, - "open": 2000, - "volume": 1329521 - }, - "open": "2020-09-07T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5723, - "high": 154, - "low": 10, - "open": 11261, - "volume": 12909699 - }, - "id": 2714431, - "non_hive": { - "close": 1001, - "high": 28, - "low": 1, - "open": 2000, - "volume": 2259747 - }, - "open": "2020-09-07T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41024, - "high": 145, - "low": 16259, - "open": 11687, - "volume": 1601316 - }, - "id": 2714498, - "non_hive": { - "close": 7339, - "high": 26, - "low": 2747, - "open": 2000, - "volume": 275622 - }, - "open": "2020-09-07T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29947, - "high": 11, - "low": 100, - "open": 8782, - "volume": 334045 - }, - "id": 2714542, - "non_hive": { - "close": 5121, - "high": 2, - "low": 17, - "open": 1572, - "volume": 57828 - }, - "open": "2020-09-07T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45000, - "high": 235, - "low": 249, - "open": 118, - "volume": 2722724 - }, - "id": 2714569, - "non_hive": { - "close": 7964, - "high": 42, - "low": 42, - "open": 21, - "volume": 481927 - }, - "open": "2020-09-07T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24172, - "high": 1912, - "low": 99, - "open": 1000, - "volume": 1181323 - }, - "id": 2714627, - "non_hive": { - "close": 4175, - "high": 350, - "low": 17, - "open": 178, - "volume": 213262 - }, - "open": "2020-09-07T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 125, - "high": 10, - "low": 6, - "open": 1947, - "volume": 1331617 - }, - "id": 2714685, - "non_hive": { - "close": 23, - "high": 2, - "low": 1, - "open": 356, - "volume": 243631 - }, - "open": "2020-09-07T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34069, - "high": 43, - "low": 12, - "open": 27689, - "volume": 271639 - }, - "id": 2714733, - "non_hive": { - "close": 6233, - "high": 8, - "low": 2, - "open": 5091, - "volume": 49809 - }, - "open": "2020-09-07T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 218, - "low": 11940, - "open": 6903, - "volume": 1674173 - }, - "id": 2714793, - "non_hive": { - "close": 1786, - "high": 40, - "low": 2096, - "open": 1263, - "volume": 305514 - }, - "open": "2020-09-07T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11007, - "high": 147, - "low": 2000, - "open": 57487, - "volume": 312871 - }, - "id": 2714856, - "non_hive": { - "close": 2000, - "high": 27, - "low": 353, - "open": 10503, - "volume": 56912 - }, - "open": "2020-09-07T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11049, - "high": 49, - "low": 17670, - "open": 203, - "volume": 2284626 - }, - "id": 2714920, - "non_hive": { - "close": 2000, - "high": 9, - "low": 3123, - "open": 37, - "volume": 413995 - }, - "open": "2020-09-07T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 379, - "high": 251, - "low": 499, - "open": 9710, - "volume": 2889895 - }, - "id": 2714989, - "non_hive": { - "close": 68, - "high": 46, - "low": 86, - "open": 1748, - "volume": 516791 - }, - "open": "2020-09-07T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16788, - "high": 33, - "low": 116, - "open": 5901, - "volume": 317619 - }, - "id": 2715061, - "non_hive": { - "close": 3000, - "high": 6, - "low": 20, - "open": 1058, - "volume": 56807 - }, - "open": "2020-09-07T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16771, - "high": 134048, - "low": 450773, - "open": 16790, - "volume": 1678118 - }, - "id": 2715112, - "non_hive": { - "close": 3000, - "high": 23981, - "low": 80475, - "open": 3000, - "volume": 299690 - }, - "open": "2020-09-07T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1762, - "high": 1403, - "low": 23, - "open": 1403, - "volume": 100799 - }, - "id": 2715145, - "non_hive": { - "close": 315, - "high": 251, - "low": 4, - "open": 251, - "volume": 18021 - }, - "open": "2020-09-07T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1744, - "high": 782, - "low": 12, - "open": 16786, - "volume": 4230165 - }, - "id": 2715178, - "non_hive": { - "close": 312, - "high": 140, - "low": 2, - "open": 3000, - "volume": 743271 - }, - "open": "2020-09-08T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 172807, - "high": 16405, - "low": 274, - "open": 10000, - "volume": 1963850 - }, - "id": 2715219, - "non_hive": { - "close": 31585, - "high": 3000, - "low": 48, - "open": 1753, - "volume": 347838 - }, - "open": "2020-09-08T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 858, - "high": 114, - "low": 33, - "open": 7951, - "volume": 2576227 - }, - "id": 2715256, - "non_hive": { - "close": 157, - "high": 21, - "low": 5, - "open": 1453, - "volume": 463134 - }, - "open": "2020-09-08T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14679, - "high": 666, - "low": 14679, - "open": 10932, - "volume": 6619981 - }, - "id": 2715304, - "non_hive": { - "close": 2583, - "high": 122, - "low": 2583, - "open": 1999, - "volume": 1168114 - }, - "open": "2020-09-08T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19667, - "high": 13637, - "low": 68337, - "open": 11079, - "volume": 2478202 - }, - "id": 2715353, - "non_hive": { - "close": 3570, - "high": 2482, - "low": 11704, - "open": 1999, - "volume": 433001 - }, - "open": "2020-09-08T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 537013, - "high": 5659, - "low": 12, - "open": 6278, - "volume": 5141438 - }, - "id": 2715399, - "non_hive": { - "close": 97001, - "high": 1024, - "low": 2, - "open": 1136, - "volume": 899195 - }, - "open": "2020-09-08T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16468, - "high": 5, - "low": 280494, - "open": 11290, - "volume": 7547054 - }, - "id": 2715441, - "non_hive": { - "close": 3000, - "high": 1, - "low": 49092, - "open": 2000, - "volume": 1336244 - }, - "open": "2020-09-08T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5566, - "high": 882, - "low": 1182271, - "open": 6000, - "volume": 2568344 - }, - "id": 2715498, - "non_hive": { - "close": 1001, - "high": 161, - "low": 208079, - "open": 1059, - "volume": 452999 - }, - "open": "2020-09-08T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11224, - "high": 5563, - "low": 106, - "open": 208, - "volume": 1590880 - }, - "id": 2715536, - "non_hive": { - "close": 2017, - "high": 1001, - "low": 18, - "open": 37, - "volume": 281404 - }, - "open": "2020-09-08T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17587, - "high": 153, - "low": 26, - "open": 4279, - "volume": 1624174 - }, - "id": 2715581, - "non_hive": { - "close": 3207, - "high": 28, - "low": 4, - "open": 769, - "volume": 285776 - }, - "open": "2020-09-08T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7884, - "high": 148, - "low": 30495, - "open": 1234, - "volume": 208554 - }, - "id": 2715613, - "non_hive": { - "close": 1418, - "high": 27, - "low": 5374, - "open": 225, - "volume": 37582 - }, - "open": "2020-09-08T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 78, - "high": 2475, - "low": 1125238, - "open": 9177, - "volume": 8938869 - }, - "id": 2715642, - "non_hive": { - "close": 14, - "high": 445, - "low": 191409, - "open": 1650, - "volume": 1551349 - }, - "open": "2020-09-08T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9858, - "high": 809, - "low": 1188441, - "open": 2390, - "volume": 1436954 - }, - "id": 2715681, - "non_hive": { - "close": 1763, - "high": 145, - "low": 205600, - "open": 428, - "volume": 250009 - }, - "open": "2020-09-08T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1440, - "high": 1440, - "low": 7603, - "open": 7603, - "volume": 88193 - }, - "id": 2715713, - "non_hive": { - "close": 258, - "high": 258, - "low": 1350, - "open": 1350, - "volume": 15776 - }, - "open": "2020-09-08T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 346, - "high": 3602, - "low": 35, - "open": 11418, - "volume": 4058330 - }, - "id": 2715742, - "non_hive": { - "close": 60, - "high": 645, - "low": 6, - "open": 2000, - "volume": 704559 - }, - "open": "2020-09-08T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28625, - "high": 3812, - "low": 504, - "open": 167834, - "volume": 588842 - }, - "id": 2715799, - "non_hive": { - "close": 5210, - "high": 694, - "low": 88, - "open": 29582, - "volume": 104495 - }, - "open": "2020-09-08T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10920, - "high": 43, - "low": 12, - "open": 13006, - "volume": 181233 - }, - "id": 2715851, - "non_hive": { - "close": 1980, - "high": 8, - "low": 2, - "open": 2315, - "volume": 32784 - }, - "open": "2020-09-08T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 524833, - "high": 11965, - "low": 158759, - "open": 11029, - "volume": 2551971 - }, - "id": 2715895, - "non_hive": { - "close": 92011, - "high": 2187, - "low": 27832, - "open": 1999, - "volume": 449513 - }, - "open": "2020-09-08T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 585205, - "high": 4710, - "low": 585205, - "open": 4710, - "volume": 1304419 - }, - "id": 2715933, - "non_hive": { - "close": 102604, - "high": 859, - "low": 102604, - "open": 859, - "volume": 229353 - }, - "open": "2020-09-08T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 850, - "high": 71, - "low": 60808, - "open": 2520, - "volume": 1663397 - }, - "id": 2715969, - "non_hive": { - "close": 154, - "high": 13, - "low": 10663, - "open": 457, - "volume": 293467 - }, - "open": "2020-09-08T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7866, - "high": 2089, - "low": 21719, - "open": 366, - "volume": 1573343 - }, - "id": 2716005, - "non_hive": { - "close": 1413, - "high": 379, - "low": 3808, - "open": 66, - "volume": 277478 - }, - "open": "2020-09-08T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3357, - "high": 3357, - "low": 3600, - "open": 11223, - "volume": 2601336 - }, - "id": 2716037, - "non_hive": { - "close": 609, - "high": 609, - "low": 631, - "open": 2000, - "volume": 457495 - }, - "open": "2020-09-08T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8038, - "high": 1047, - "low": 957, - "open": 2002, - "volume": 67265 - }, - "id": 2716084, - "non_hive": { - "close": 1466, - "high": 191, - "low": 174, - "open": 365, - "volume": 12266 - }, - "open": "2020-09-08T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27750, - "high": 415190, - "low": 29, - "open": 3649, - "volume": 2527219 - }, - "id": 2716101, - "non_hive": { - "close": 5020, - "high": 75977, - "low": 5, - "open": 666, - "volume": 453679 - }, - "open": "2020-09-08T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 403, - "low": 12, - "open": 403, - "volume": 62901 - }, - "id": 2716142, - "non_hive": { - "close": 2, - "high": 73, - "low": 2, - "open": 73, - "volume": 11377 - }, - "open": "2020-09-09T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33340, - "high": 5265, - "low": 148880, - "open": 278, - "volume": 1268735 - }, - "id": 2716158, - "non_hive": { - "close": 6028, - "high": 952, - "low": 26206, - "open": 49, - "volume": 223817 - }, - "open": "2020-09-09T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7675, - "high": 591, - "low": 7675, - "open": 11327, - "volume": 50322 - }, - "id": 2716178, - "non_hive": { - "close": 1386, - "high": 107, - "low": 1386, - "open": 2048, - "volume": 9096 - }, - "open": "2020-09-09T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3437, - "high": 193, - "low": 161, - "open": 9344, - "volume": 3775828 - }, - "id": 2716196, - "non_hive": { - "close": 621, - "high": 35, - "low": 29, - "open": 1689, - "volume": 682109 - }, - "open": "2020-09-09T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2591, - "high": 5, - "low": 86, - "open": 273339, - "volume": 3599783 - }, - "id": 2716223, - "non_hive": { - "close": 466, - "high": 1, - "low": 15, - "open": 49378, - "volume": 636760 - }, - "open": "2020-09-09T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9378, - "high": 55, - "low": 588442, - "open": 11415, - "volume": 3735123 - }, - "id": 2716254, - "non_hive": { - "close": 1683, - "high": 10, - "low": 101819, - "open": 2000, - "volume": 647516 - }, - "open": "2020-09-09T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3283, - "high": 89, - "low": 22017, - "open": 2192, - "volume": 6131819 - }, - "id": 2716287, - "non_hive": { - "close": 590, - "high": 16, - "low": 3831, - "open": 393, - "volume": 1067958 - }, - "open": "2020-09-09T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 588508, - "high": 372, - "low": 24116, - "open": 11481, - "volume": 7314898 - }, - "id": 2716329, - "non_hive": { - "close": 102410, - "high": 67, - "low": 4196, - "open": 2000, - "volume": 1273322 - }, - "open": "2020-09-09T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 529399, - "high": 44, - "low": 570542, - "open": 1345, - "volume": 2494599 - }, - "id": 2716365, - "non_hive": { - "close": 92133, - "high": 8, - "low": 99293, - "open": 241, - "volume": 434694 - }, - "open": "2020-09-09T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2000, - "high": 44, - "low": 7000, - "open": 11177, - "volume": 1450515 - }, - "id": 2716393, - "non_hive": { - "close": 348, - "high": 8, - "low": 1218, - "open": 1999, - "volume": 253049 - }, - "open": "2020-09-09T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15671, - "high": 5616, - "low": 517213, - "open": 11210, - "volume": 2551294 - }, - "id": 2716433, - "non_hive": { - "close": 2788, - "high": 1002, - "low": 90512, - "open": 1999, - "volume": 446871 - }, - "open": "2020-09-09T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11427, - "high": 567, - "low": 6, - "open": 611, - "volume": 6121141 - }, - "id": 2716468, - "non_hive": { - "close": 2000, - "high": 101, - "low": 1, - "open": 107, - "volume": 1071447 - }, - "open": "2020-09-09T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 118750, - "high": 5, - "low": 57, - "open": 11261, - "volume": 7998463 - }, - "id": 2716507, - "non_hive": { - "close": 20900, - "high": 1, - "low": 9, - "open": 1999, - "volume": 1401219 - }, - "open": "2020-09-09T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11424, - "high": 129, - "low": 186, - "open": 120021, - "volume": 5067145 - }, - "id": 2716579, - "non_hive": { - "close": 2000, - "high": 23, - "low": 32, - "open": 21005, - "volume": 887144 - }, - "open": "2020-09-09T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55, - "high": 11754, - "low": 13, - "open": 28, - "volume": 5312510 - }, - "id": 2716610, - "non_hive": { - "close": 9, - "high": 2104, - "low": 2, - "open": 5, - "volume": 933885 - }, - "open": "2020-09-09T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 82034, - "high": 5, - "low": 71, - "open": 4395, - "volume": 1500011 - }, - "id": 2716668, - "non_hive": { - "close": 14716, - "high": 1, - "low": 12, - "open": 786, - "volume": 266083 - }, - "open": "2020-09-09T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5899, - "high": 1426, - "low": 12, - "open": 11803, - "volume": 3644070 - }, - "id": 2716724, - "non_hive": { - "close": 1075, - "high": 261, - "low": 2, - "open": 2117, - "volume": 653327 - }, - "open": "2020-09-09T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57679, - "high": 9345, - "low": 304371, - "open": 104, - "volume": 1983947 - }, - "id": 2716789, - "non_hive": { - "close": 10477, - "high": 1709, - "low": 54289, - "open": 19, - "volume": 357383 - }, - "open": "2020-09-09T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 275, - "high": 418, - "low": 85603, - "open": 9243, - "volume": 1770686 - }, - "id": 2716820, - "non_hive": { - "close": 50, - "high": 76, - "low": 15237, - "open": 1678, - "volume": 318205 - }, - "open": "2020-09-09T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127851, - "high": 27, - "low": 10767, - "open": 74688, - "volume": 1111153 - }, - "id": 2716858, - "non_hive": { - "close": 23230, - "high": 5, - "low": 1953, - "open": 13553, - "volume": 201661 - }, - "open": "2020-09-09T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 3753, - "low": 29806, - "open": 11093, - "volume": 341253 - }, - "id": 2716889, - "non_hive": { - "close": 1817, - "high": 682, - "low": 5308, - "open": 2000, - "volume": 61805 - }, - "open": "2020-09-09T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16511, - "high": 44, - "low": 608, - "open": 16511, - "volume": 109789 - }, - "id": 2716936, - "non_hive": { - "close": 3000, - "high": 8, - "low": 110, - "open": 3000, - "volume": 19948 - }, - "open": "2020-09-09T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16510, - "high": 44, - "low": 2000, - "open": 2000, - "volume": 194781 - }, - "id": 2716966, - "non_hive": { - "close": 3000, - "high": 8, - "low": 363, - "open": 363, - "volume": 35391 - }, - "open": "2020-09-09T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16420, - "high": 103, - "low": 28, - "open": 69383, - "volume": 944350 - }, - "id": 2717013, - "non_hive": { - "close": 3000, - "high": 19, - "low": 5, - "open": 12606, - "volume": 171797 - }, - "open": "2020-09-09T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5407, - "high": 300, - "low": 2994, - "open": 7038, - "volume": 227442 - }, - "id": 2717058, - "non_hive": { - "close": 989, - "high": 55, - "low": 544, - "open": 1286, - "volume": 41532 - }, - "open": "2020-09-10T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54, - "high": 54, - "low": 274, - "open": 5523, - "volume": 1810173 - }, - "id": 2717091, - "non_hive": { - "close": 10, - "high": 10, - "low": 50, - "open": 1010, - "volume": 331252 - }, - "open": "2020-09-10T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 657, - "high": 54, - "low": 2089, - "open": 2089, - "volume": 724258 - }, - "id": 2717119, - "non_hive": { - "close": 121, - "high": 10, - "low": 382, - "open": 382, - "volume": 133261 - }, - "open": "2020-09-10T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9351, - "high": 2489, - "low": 369488, - "open": 391, - "volume": 1668748 - }, - "id": 2717133, - "non_hive": { - "close": 1776, - "high": 483, - "low": 67612, - "open": 72, - "volume": 306856 - }, - "open": "2020-09-10T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5089, - "high": 110827, - "low": 446671, - "open": 1806, - "volume": 5304014 - }, - "id": 2717161, - "non_hive": { - "close": 949, - "high": 21053, - "low": 82682, - "open": 342, - "volume": 990618 - }, - "open": "2020-09-10T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 118039, - "high": 139, - "low": 1174304, - "open": 7300, - "volume": 2624446 - }, - "id": 2717196, - "non_hive": { - "close": 21919, - "high": 26, - "low": 216071, - "open": 1359, - "volume": 485599 - }, - "open": "2020-09-10T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2000, - "high": 32, - "low": 10, - "open": 21610, - "volume": 1992670 - }, - "id": 2717216, - "non_hive": { - "close": 370, - "high": 6, - "low": 1, - "open": 4013, - "volume": 369183 - }, - "open": "2020-09-10T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14660, - "high": 42623, - "low": 107, - "open": 8809, - "volume": 1676262 - }, - "id": 2717270, - "non_hive": { - "close": 2682, - "high": 7928, - "low": 19, - "open": 1630, - "volume": 308868 - }, - "open": "2020-09-10T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10003, - "high": 129, - "low": 75, - "open": 4950, - "volume": 5808140 - }, - "id": 2717328, - "non_hive": { - "close": 1852, - "high": 24, - "low": 13, - "open": 919, - "volume": 1052810 - }, - "open": "2020-09-10T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3200, - "high": 70, - "low": 88830, - "open": 5526, - "volume": 6052331 - }, - "id": 2717384, - "non_hive": { - "close": 588, - "high": 13, - "low": 15901, - "open": 1023, - "volume": 1086765 - }, - "open": "2020-09-10T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10516, - "high": 5, - "low": 142, - "open": 10984, - "volume": 10204222 - }, - "id": 2717418, - "non_hive": { - "close": 1935, - "high": 1, - "low": 25, - "open": 2000, - "volume": 1861721 - }, - "open": "2020-09-10T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11653, - "high": 5440, - "low": 31163, - "open": 5440, - "volume": 7405122 - }, - "id": 2717486, - "non_hive": { - "close": 2136, - "high": 1001, - "low": 5586, - "open": 1001, - "volume": 1342645 - }, - "open": "2020-09-10T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8865, - "high": 43, - "low": 1062698, - "open": 38, - "volume": 8124168 - }, - "id": 2717529, - "non_hive": { - "close": 1630, - "high": 8, - "low": 191286, - "open": 7, - "volume": 1480592 - }, - "open": "2020-09-10T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55303, - "high": 133097, - "low": 24, - "open": 29000, - "volume": 9019083 - }, - "id": 2717588, - "non_hive": { - "close": 10148, - "high": 24605, - "low": 4, - "open": 5333, - "volume": 1650323 - }, - "open": "2020-09-10T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2278, - "high": 545, - "low": 18, - "open": 10818, - "volume": 7088419 - }, - "id": 2717653, - "non_hive": { - "close": 426, - "high": 102, - "low": 3, - "open": 1999, - "volume": 1314770 - }, - "open": "2020-09-10T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 243262, - "high": 47, - "low": 128, - "open": 394, - "volume": 7576467 - }, - "id": 2717737, - "non_hive": { - "close": 45039, - "high": 9, - "low": 23, - "open": 73, - "volume": 1409783 - }, - "open": "2020-09-10T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27394, - "high": 2373, - "low": 11, - "open": 10598, - "volume": 232589 - }, - "id": 2717787, - "non_hive": { - "close": 5170, - "high": 448, - "low": 2, - "open": 1999, - "volume": 43889 - }, - "open": "2020-09-10T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4463, - "high": 28648, - "low": 28, - "open": 21144, - "volume": 219228 - }, - "id": 2717816, - "non_hive": { - "close": 839, - "high": 5386, - "low": 5, - "open": 3973, - "volume": 41197 - }, - "open": "2020-09-10T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 589265, - "high": 1920, - "low": 47, - "open": 7705, - "volume": 6134735 - }, - "id": 2717855, - "non_hive": { - "close": 109326, - "high": 361, - "low": 8, - "open": 1448, - "volume": 1138600 - }, - "open": "2020-09-10T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5529, - "high": 42, - "low": 27756, - "open": 713, - "volume": 7412787 - }, - "id": 2717896, - "non_hive": { - "close": 1039, - "high": 8, - "low": 5148, - "open": 134, - "volume": 1375776 - }, - "open": "2020-09-10T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 559447, - "high": 569, - "low": 74, - "open": 3816, - "volume": 7312492 - }, - "id": 2717940, - "non_hive": { - "close": 103806, - "high": 107, - "low": 13, - "open": 717, - "volume": 1357028 - }, - "open": "2020-09-10T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1005, - "high": 1005, - "low": 33075, - "open": 10777, - "volume": 6969444 - }, - "id": 2717984, - "non_hive": { - "close": 190, - "high": 190, - "low": 6137, - "open": 2000, - "volume": 1295803 - }, - "open": "2020-09-10T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 529, - "high": 524, - "low": 529, - "open": 5456, - "volume": 4904227 - }, - "id": 2718023, - "non_hive": { - "close": 98, - "high": 99, - "low": 98, - "open": 1014, - "volume": 910499 - }, - "open": "2020-09-10T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 444, - "high": 105, - "low": 444, - "open": 1477, - "volume": 4408587 - }, - "id": 2718053, - "non_hive": { - "close": 82, - "high": 20, - "low": 82, - "open": 279, - "volume": 820710 - }, - "open": "2020-09-10T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1644, - "high": 26486, - "low": 11, - "open": 26486, - "volume": 1229641 - }, - "id": 2718087, - "non_hive": { - "close": 310, - "high": 5000, - "low": 2, - "open": 5000, - "volume": 227835 - }, - "open": "2020-09-11T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17973, - "high": 683, - "low": 41, - "open": 2700, - "volume": 72115 - }, - "id": 2718101, - "non_hive": { - "close": 3392, - "high": 129, - "low": 7, - "open": 509, - "volume": 13599 - }, - "open": "2020-09-11T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18956, - "high": 3083, - "low": 10707, - "open": 2914, - "volume": 309343 - }, - "id": 2718124, - "non_hive": { - "close": 3541, - "high": 582, - "low": 1999, - "open": 550, - "volume": 57979 - }, - "open": "2020-09-11T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 813, - "high": 2271, - "low": 929, - "open": 5342, - "volume": 17255 - }, - "id": 2718150, - "non_hive": { - "close": 153, - "high": 428, - "low": 173, - "open": 998, - "volume": 3240 - }, - "open": "2020-09-11T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6802, - "high": 16972, - "low": 6925, - "open": 16972, - "volume": 38484 - }, - "id": 2718169, - "non_hive": { - "close": 1272, - "high": 3198, - "low": 1295, - "open": 3198, - "volume": 7221 - }, - "open": "2020-09-11T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2328, - "high": 1032, - "low": 2328, - "open": 1032, - "volume": 129862 - }, - "id": 2718185, - "non_hive": { - "close": 431, - "high": 193, - "low": 431, - "open": 193, - "volume": 24265 - }, - "open": "2020-09-11T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1524, - "high": 1524, - "low": 10698, - "open": 6817, - "volume": 80437 - }, - "id": 2718205, - "non_hive": { - "close": 285, - "high": 285, - "low": 1999, - "open": 1274, - "volume": 15034 - }, - "open": "2020-09-11T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 10, - "low": 40803, - "open": 1328, - "volume": 189651 - }, - "id": 2718231, - "non_hive": { - "close": 2, - "high": 2, - "low": 7549, - "open": 248, - "volume": 35377 - }, - "open": "2020-09-11T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90, - "high": 90, - "low": 12757, - "open": 8374, - "volume": 34786 - }, - "id": 2718257, - "non_hive": { - "close": 17, - "high": 17, - "low": 2384, - "open": 1565, - "volume": 6501 - }, - "open": "2020-09-11T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7042, - "high": 32, - "low": 181, - "open": 6817, - "volume": 427419 - }, - "id": 2718270, - "non_hive": { - "close": 1308, - "high": 6, - "low": 33, - "open": 1274, - "volume": 79301 - }, - "open": "2020-09-11T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6792, - "high": 6207, - "low": 15, - "open": 3896, - "volume": 488978 - }, - "id": 2718326, - "non_hive": { - "close": 1267, - "high": 1159, - "low": 2, - "open": 727, - "volume": 91028 - }, - "open": "2020-09-11T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12093, - "high": 12093, - "low": 299, - "open": 10719, - "volume": 73063 - }, - "id": 2718397, - "non_hive": { - "close": 2258, - "high": 2258, - "low": 55, - "open": 1999, - "volume": 13628 - }, - "open": "2020-09-11T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10868, - "high": 133, - "low": 118487, - "open": 16067, - "volume": 3586391 - }, - "id": 2718407, - "non_hive": { - "close": 2000, - "high": 25, - "low": 21487, - "open": 3000, - "volume": 658698 - }, - "open": "2020-09-11T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10237, - "high": 2852, - "low": 11, - "open": 6005, - "volume": 2545897 - }, - "id": 2718442, - "non_hive": { - "close": 1912, - "high": 533, - "low": 2, - "open": 1115, - "volume": 471703 - }, - "open": "2020-09-11T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 862243, - "high": 10, - "low": 22, - "open": 5826, - "volume": 2115892 - }, - "id": 2718504, - "non_hive": { - "close": 159523, - "high": 2, - "low": 4, - "open": 1088, - "volume": 393209 - }, - "open": "2020-09-11T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1938, - "high": 1938, - "low": 284, - "open": 10695, - "volume": 6370119 - }, - "id": 2718546, - "non_hive": { - "close": 376, - "high": 376, - "low": 53, - "open": 1999, - "volume": 1199979 - }, - "open": "2020-09-11T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5020, - "high": 100, - "low": 11, - "open": 8000, - "volume": 4223456 - }, - "id": 2718610, - "non_hive": { - "close": 1003, - "high": 20, - "low": 2, - "open": 1552, - "volume": 826539 - }, - "open": "2020-09-11T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 24, - "low": 40, - "open": 10006, - "volume": 3115501 - }, - "id": 2718660, - "non_hive": { - "close": 5, - "high": 5, - "low": 7, - "open": 1999, - "volume": 608039 - }, - "open": "2020-09-11T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2660, - "high": 2844, - "low": 43455, - "open": 471, - "volume": 1211319 - }, - "id": 2718691, - "non_hive": { - "close": 526, - "high": 572, - "low": 8464, - "open": 92, - "volume": 236539 - }, - "open": "2020-09-11T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14755, - "high": 904, - "low": 23, - "open": 10112, - "volume": 94524 - }, - "id": 2718724, - "non_hive": { - "close": 2935, - "high": 180, - "low": 4, - "open": 1999, - "volume": 18773 - }, - "open": "2020-09-11T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14376, - "high": 10304, - "low": 5424, - "open": 10053, - "volume": 577713 - }, - "id": 2718753, - "non_hive": { - "close": 2825, - "high": 2050, - "low": 1065, - "open": 1999, - "volume": 114264 - }, - "open": "2020-09-11T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8466, - "high": 10, - "low": 8466, - "open": 11094, - "volume": 555234 - }, - "id": 2718806, - "non_hive": { - "close": 1663, - "high": 2, - "low": 1663, - "open": 2180, - "volume": 110372 - }, - "open": "2020-09-11T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10264, - "high": 708, - "low": 8000, - "open": 26551, - "volume": 95637 - }, - "id": 2718832, - "non_hive": { - "close": 2042, - "high": 141, - "low": 1591, - "open": 5282, - "volume": 19026 - }, - "open": "2020-09-11T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 145, - "high": 702, - "low": 26, - "open": 26, - "volume": 1341973 - }, - "id": 2718863, - "non_hive": { - "close": 29, - "high": 142, - "low": 5, - "open": 5, - "volume": 268474 - }, - "open": "2020-09-11T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2102, - "high": 1170, - "low": 11, - "open": 1170, - "volume": 1263736 - }, - "id": 2718904, - "non_hive": { - "close": 420, - "high": 234, - "low": 2, - "open": 234, - "volume": 247353 - }, - "open": "2020-09-12T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2432, - "high": 100, - "low": 16440, - "open": 274, - "volume": 369946 - }, - "id": 2718932, - "non_hive": { - "close": 486, - "high": 20, - "low": 3172, - "open": 53, - "volume": 71893 - }, - "open": "2020-09-12T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127, - "high": 5, - "low": 10, - "open": 10, - "volume": 142 - }, - "id": 2718953, - "non_hive": { - "close": 25, - "high": 1, - "low": 1, - "open": 1, - "volume": 27 - }, - "open": "2020-09-12T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2273, - "high": 795, - "low": 1958, - "open": 2838, - "volume": 114764 - }, - "id": 2718963, - "non_hive": { - "close": 454, - "high": 159, - "low": 389, - "open": 567, - "volume": 22924 - }, - "open": "2020-09-12T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 706287, - "high": 165, - "low": 37, - "open": 74998, - "volume": 2805956 - }, - "id": 2718996, - "non_hive": { - "close": 134911, - "high": 33, - "low": 7, - "open": 14983, - "volume": 539615 - }, - "open": "2020-09-12T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1227, - "high": 21248, - "low": 102, - "open": 21248, - "volume": 55113 - }, - "id": 2719033, - "non_hive": { - "close": 242, - "high": 4200, - "low": 20, - "open": 4200, - "volume": 10883 - }, - "open": "2020-09-12T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 336, - "high": 121, - "low": 25051, - "open": 3798, - "volume": 262228 - }, - "id": 2719049, - "non_hive": { - "close": 65, - "high": 24, - "low": 4791, - "open": 749, - "volume": 50810 - }, - "open": "2020-09-12T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2176, - "high": 7968, - "low": 4361, - "open": 10415, - "volume": 138297 - }, - "id": 2719086, - "non_hive": { - "close": 420, - "high": 1538, - "low": 834, - "open": 2000, - "volume": 26637 - }, - "open": "2020-09-12T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 84431, - "high": 1911, - "low": 6592, - "open": 1911, - "volume": 724117 - }, - "id": 2719104, - "non_hive": { - "close": 16294, - "high": 369, - "low": 1260, - "open": 369, - "volume": 139158 - }, - "open": "2020-09-12T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34333, - "high": 36, - "low": 524, - "open": 5854, - "volume": 86049 - }, - "id": 2719152, - "non_hive": { - "close": 6609, - "high": 7, - "low": 100, - "open": 1131, - "volume": 16568 - }, - "open": "2020-09-12T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13703, - "high": 5797, - "low": 936610, - "open": 12971, - "volume": 3255224 - }, - "id": 2719178, - "non_hive": { - "close": 2638, - "high": 1116, - "low": 178101, - "open": 2497, - "volume": 622198 - }, - "open": "2020-09-12T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11179, - "high": 10, - "low": 147, - "open": 7177, - "volume": 2936043 - }, - "id": 2719218, - "non_hive": { - "close": 2191, - "high": 2, - "low": 28, - "open": 1378, - "volume": 568290 - }, - "open": "2020-09-12T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3621, - "high": 459, - "low": 152415, - "open": 17906, - "volume": 6364975 - }, - "id": 2719265, - "non_hive": { - "close": 702, - "high": 90, - "low": 29141, - "open": 3509, - "volume": 1225182 - }, - "open": "2020-09-12T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15715, - "high": 15476, - "low": 813, - "open": 15476, - "volume": 2965894 - }, - "id": 2719327, - "non_hive": { - "close": 3000, - "high": 3000, - "low": 154, - "open": 3000, - "volume": 566676 - }, - "open": "2020-09-12T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17, - "high": 3410, - "low": 17, - "open": 13384, - "volume": 340942 - }, - "id": 2719370, - "non_hive": { - "close": 3, - "high": 658, - "low": 3, - "open": 2555, - "volume": 65089 - }, - "open": "2020-09-12T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2575, - "high": 5192, - "low": 765308, - "open": 10469, - "volume": 1666686 - }, - "id": 2719420, - "non_hive": { - "close": 496, - "high": 1001, - "low": 145478, - "open": 2000, - "volume": 317848 - }, - "open": "2020-09-12T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20258, - "high": 20258, - "low": 11, - "open": 115, - "volume": 509682 - }, - "id": 2719467, - "non_hive": { - "close": 3903, - "high": 3903, - "low": 2, - "open": 22, - "volume": 97681 - }, - "open": "2020-09-12T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3857, - "high": 228, - "low": 8, - "open": 228, - "volume": 435027 - }, - "id": 2719503, - "non_hive": { - "close": 743, - "high": 44, - "low": 1, - "open": 44, - "volume": 83649 - }, - "open": "2020-09-12T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8103, - "high": 903, - "low": 843135, - "open": 1820, - "volume": 2548264 - }, - "id": 2719535, - "non_hive": { - "close": 1561, - "high": 174, - "low": 160677, - "open": 347, - "volume": 487580 - }, - "open": "2020-09-12T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51949, - "high": 4329, - "low": 51949, - "open": 4329, - "volume": 182720 - }, - "id": 2719570, - "non_hive": { - "close": 10000, - "high": 834, - "low": 10000, - "open": 834, - "volume": 35179 - }, - "open": "2020-09-12T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 58477, - "high": 1116, - "low": 3667, - "open": 78443, - "volume": 421558 - }, - "id": 2719596, - "non_hive": { - "close": 11257, - "high": 215, - "low": 705, - "open": 15100, - "volume": 81147 - }, - "open": "2020-09-12T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21012, - "high": 57, - "low": 5200, - "open": 14394, - "volume": 264128 - }, - "id": 2719638, - "non_hive": { - "close": 4045, - "high": 11, - "low": 1001, - "open": 2771, - "volume": 50846 - }, - "open": "2020-09-12T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 360268, - "high": 46, - "low": 12788, - "open": 43802, - "volume": 640728 - }, - "id": 2719679, - "non_hive": { - "close": 69836, - "high": 9, - "low": 2461, - "open": 8432, - "volume": 123916 - }, - "open": "2020-09-12T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 334, - "high": 577, - "low": 193, - "open": 26, - "volume": 1348348 - }, - "id": 2719695, - "non_hive": { - "close": 63, - "high": 112, - "low": 36, - "open": 5, - "volume": 259340 - }, - "open": "2020-09-12T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 7552, - "low": 11, - "open": 7552, - "volume": 127960 - }, - "id": 2719729, - "non_hive": { - "close": 2, - "high": 1464, - "low": 2, - "open": 1464, - "volume": 24793 - }, - "open": "2020-09-13T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44845, - "high": 36, - "low": 44845, - "open": 18203, - "volume": 5002960 - }, - "id": 2719759, - "non_hive": { - "close": 8206, - "high": 7, - "low": 8206, - "open": 3525, - "volume": 933530 - }, - "open": "2020-09-13T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10333, - "high": 377, - "low": 162, - "open": 1374, - "volume": 173721 - }, - "id": 2719796, - "non_hive": { - "close": 1999, - "high": 73, - "low": 31, - "open": 266, - "volume": 33621 - }, - "open": "2020-09-13T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8498, - "high": 10029, - "low": 8498, - "open": 10029, - "volume": 143296 - }, - "id": 2719815, - "non_hive": { - "close": 1565, - "high": 1941, - "low": 1565, - "open": 1941, - "volume": 27624 - }, - "open": "2020-09-13T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41130, - "high": 67, - "low": 39, - "open": 7773, - "volume": 223032 - }, - "id": 2719832, - "non_hive": { - "close": 7912, - "high": 13, - "low": 7, - "open": 1494, - "volume": 42879 - }, - "open": "2020-09-13T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15452, - "high": 10, - "low": 155, - "open": 7216, - "volume": 190956 - }, - "id": 2719863, - "non_hive": { - "close": 2951, - "high": 2, - "low": 28, - "open": 1388, - "volume": 36305 - }, - "open": "2020-09-13T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1010, - "high": 19461, - "low": 258667, - "open": 258667, - "volume": 695280 - }, - "id": 2719896, - "non_hive": { - "close": 193, - "high": 3736, - "low": 49270, - "open": 49270, - "volume": 132487 - }, - "open": "2020-09-13T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9749, - "high": 11658, - "low": 2316, - "open": 3990, - "volume": 144540 - }, - "id": 2719914, - "non_hive": { - "close": 1862, - "high": 2238, - "low": 431, - "open": 762, - "volume": 27571 - }, - "open": "2020-09-13T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15706, - "high": 15706, - "low": 24322, - "open": 16432, - "volume": 605622 - }, - "id": 2719946, - "non_hive": { - "close": 3000, - "high": 3000, - "low": 4621, - "open": 3138, - "volume": 115631 - }, - "open": "2020-09-13T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2500, - "high": 6203, - "low": 1546, - "open": 13047, - "volume": 92940 - }, - "id": 2719980, - "non_hive": { - "close": 480, - "high": 1191, - "low": 295, - "open": 2492, - "volume": 17770 - }, - "open": "2020-09-13T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 537112, - "high": 5334, - "low": 52, - "open": 5334, - "volume": 4128116 - }, - "id": 2720009, - "non_hive": { - "close": 99999, - "high": 1024, - "low": 9, - "open": 1024, - "volume": 774391 - }, - "open": "2020-09-13T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 999304, - "high": 63, - "low": 52, - "open": 10582, - "volume": 3164929 - }, - "id": 2720050, - "non_hive": { - "close": 182023, - "high": 12, - "low": 9, - "open": 1999, - "volume": 583797 - }, - "open": "2020-09-13T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 61171, - "high": 301, - "low": 27594, - "open": 10588, - "volume": 7842750 - }, - "id": 2720087, - "non_hive": { - "close": 11427, - "high": 57, - "low": 4966, - "open": 1999, - "volume": 1423268 - }, - "open": "2020-09-13T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10298, - "high": 1536, - "low": 36, - "open": 2367, - "volume": 2557831 - }, - "id": 2720143, - "non_hive": { - "close": 1920, - "high": 287, - "low": 6, - "open": 442, - "volume": 462281 - }, - "open": "2020-09-13T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5510, - "high": 5, - "low": 1206, - "open": 1002, - "volume": 3976395 - }, - "id": 2720177, - "non_hive": { - "close": 1029, - "high": 1, - "low": 219, - "open": 187, - "volume": 725282 - }, - "open": "2020-09-13T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 311, - "high": 10, - "low": 108, - "open": 10957, - "volume": 1347988 - }, - "id": 2720240, - "non_hive": { - "close": 58, - "high": 2, - "low": 19, - "open": 2000, - "volume": 246454 - }, - "open": "2020-09-13T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 921982, - "high": 386, - "low": 8, - "open": 7971, - "volume": 2337545 - }, - "id": 2720287, - "non_hive": { - "close": 164140, - "high": 72, - "low": 1, - "open": 1481, - "volume": 420136 - }, - "open": "2020-09-13T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6182, - "high": 43, - "low": 14, - "open": 6570, - "volume": 1425226 - }, - "id": 2720329, - "non_hive": { - "close": 1142, - "high": 8, - "low": 2, - "open": 1215, - "volume": 255253 - }, - "open": "2020-09-13T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38675, - "high": 146, - "low": 16, - "open": 16518, - "volume": 2616338 - }, - "id": 2720379, - "non_hive": { - "close": 7000, - "high": 27, - "low": 2, - "open": 3051, - "volume": 468342 - }, - "open": "2020-09-13T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 255, - "high": 5, - "low": 4716, - "open": 11049, - "volume": 122865 - }, - "id": 2720439, - "non_hive": { - "close": 47, - "high": 1, - "low": 833, - "open": 2000, - "volume": 22209 - }, - "open": "2020-09-13T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 322422, - "high": 228, - "low": 6015, - "open": 10870, - "volume": 713703 - }, - "id": 2720470, - "non_hive": { - "close": 59318, - "high": 42, - "low": 1094, - "open": 1999, - "volume": 131205 - }, - "open": "2020-09-13T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 101160, - "high": 101160, - "low": 8, - "open": 7167, - "volume": 2764769 - }, - "id": 2720507, - "non_hive": { - "close": 18611, - "high": 18611, - "low": 1, - "open": 1318, - "volume": 503593 - }, - "open": "2020-09-13T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1157923, - "high": 6188, - "low": 1157923, - "open": 10927, - "volume": 3005411 - }, - "id": 2720544, - "non_hive": { - "close": 207326, - "high": 1138, - "low": 207326, - "open": 2000, - "volume": 544359 - }, - "open": "2020-09-13T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10870, - "high": 282, - "low": 28, - "open": 42310, - "volume": 5386266 - }, - "id": 2720574, - "non_hive": { - "close": 1999, - "high": 52, - "low": 5, - "open": 7658, - "volume": 971669 - }, - "open": "2020-09-13T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 885, - "low": 11, - "open": 8212, - "volume": 161296 - }, - "id": 2720619, - "non_hive": { - "close": 2, - "high": 163, - "low": 2, - "open": 1511, - "volume": 29677 - }, - "open": "2020-09-14T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44839, - "high": 48, - "low": 278, - "open": 1891, - "volume": 116667 - }, - "id": 2720645, - "non_hive": { - "close": 8250, - "high": 9, - "low": 51, - "open": 348, - "volume": 21466 - }, - "open": "2020-09-14T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 10000, - "low": 55545, - "open": 55545, - "volume": 322497 - }, - "id": 2720669, - "non_hive": { - "close": 1845, - "high": 1845, - "low": 10219, - "open": 10219, - "volume": 59367 - }, - "open": "2020-09-14T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4327, - "high": 19848, - "low": 1099234, - "open": 19848, - "volume": 6283684 - }, - "id": 2720702, - "non_hive": { - "close": 799, - "high": 3667, - "low": 198963, - "open": 3667, - "volume": 1138778 - }, - "open": "2020-09-14T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 589, - "high": 589, - "low": 2931, - "open": 2931, - "volume": 71934 - }, - "id": 2720728, - "non_hive": { - "close": 109, - "high": 109, - "low": 541, - "open": 541, - "volume": 13286 - }, - "open": "2020-09-14T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1117105, - "high": 91, - "low": 1117105, - "open": 8384, - "volume": 1370902 - }, - "id": 2720752, - "non_hive": { - "close": 201080, - "high": 17, - "low": 201080, - "open": 1549, - "volume": 247638 - }, - "open": "2020-09-14T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 542, - "high": 32, - "low": 1187979, - "open": 29825, - "volume": 3988151 - }, - "id": 2720779, - "non_hive": { - "close": 100, - "high": 6, - "low": 215232, - "open": 5500, - "volume": 723810 - }, - "open": "2020-09-14T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9063, - "high": 10, - "low": 72046, - "open": 10, - "volume": 356491 - }, - "id": 2720828, - "non_hive": { - "close": 1674, - "high": 2, - "low": 13201, - "open": 2, - "volume": 65745 - }, - "open": "2020-09-14T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1065149, - "high": 10, - "low": 1065149, - "open": 210, - "volume": 1260811 - }, - "id": 2720862, - "non_hive": { - "close": 195036, - "high": 2, - "low": 195036, - "open": 39, - "volume": 231219 - }, - "open": "2020-09-14T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10817, - "high": 32, - "low": 1524, - "open": 14027, - "volume": 4867699 - }, - "id": 2720883, - "non_hive": { - "close": 1999, - "high": 6, - "low": 276, - "open": 2557, - "volume": 886635 - }, - "open": "2020-09-14T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30396, - "high": 5478, - "low": 10823, - "open": 10817, - "volume": 132177 - }, - "id": 2720923, - "non_hive": { - "close": 5617, - "high": 1013, - "low": 1999, - "open": 1999, - "volume": 24433 - }, - "open": "2020-09-14T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12772, - "high": 113, - "low": 31404, - "open": 703, - "volume": 194177 - }, - "id": 2720941, - "non_hive": { - "close": 2361, - "high": 21, - "low": 5803, - "open": 130, - "volume": 35909 - }, - "open": "2020-09-14T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42764, - "high": 4908, - "low": 298551, - "open": 21289, - "volume": 7964342 - }, - "id": 2720970, - "non_hive": { - "close": 7908, - "high": 908, - "low": 54129, - "open": 3937, - "volume": 1454846 - }, - "open": "2020-09-14T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17450, - "high": 113, - "low": 767, - "open": 113, - "volume": 6398259 - }, - "id": 2721012, - "non_hive": { - "close": 3226, - "high": 21, - "low": 139, - "open": 21, - "volume": 1169768 - }, - "open": "2020-09-14T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39984, - "high": 232, - "low": 39984, - "open": 8766, - "volume": 5445701 - }, - "id": 2721075, - "non_hive": { - "close": 7283, - "high": 43, - "low": 7283, - "open": 1620, - "volume": 999102 - }, - "open": "2020-09-14T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16221, - "high": 10, - "low": 348, - "open": 146, - "volume": 409179 - }, - "id": 2721139, - "non_hive": { - "close": 3001, - "high": 2, - "low": 64, - "open": 27, - "volume": 75583 - }, - "open": "2020-09-14T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8372, - "high": 210, - "low": 28, - "open": 3735, - "volume": 1497693 - }, - "id": 2721199, - "non_hive": { - "close": 1549, - "high": 39, - "low": 5, - "open": 691, - "volume": 274434 - }, - "open": "2020-09-14T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 373, - "high": 5, - "low": 10, - "open": 772, - "volume": 1414570 - }, - "id": 2721263, - "non_hive": { - "close": 69, - "high": 1, - "low": 1, - "open": 143, - "volume": 260791 - }, - "open": "2020-09-14T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23493, - "high": 54, - "low": 10207, - "open": 200, - "volume": 249476 - }, - "id": 2721300, - "non_hive": { - "close": 4344, - "high": 10, - "low": 1887, - "open": 37, - "volume": 46138 - }, - "open": "2020-09-14T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8361, - "high": 1730, - "low": 30308, - "open": 10931, - "volume": 1466865 - }, - "id": 2721347, - "non_hive": { - "close": 1546, - "high": 320, - "low": 5455, - "open": 2000, - "volume": 267050 - }, - "open": "2020-09-14T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 503829, - "high": 113, - "low": 503829, - "open": 2147, - "volume": 1419126 - }, - "id": 2721392, - "non_hive": { - "close": 91696, - "high": 21, - "low": 91696, - "open": 397, - "volume": 258876 - }, - "open": "2020-09-14T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 546351, - "high": 37, - "low": 264, - "open": 10988, - "volume": 3351434 - }, - "id": 2721424, - "non_hive": { - "close": 99999, - "high": 7, - "low": 48, - "open": 2000, - "volume": 613765 - }, - "open": "2020-09-14T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6121, - "high": 151, - "low": 130, - "open": 1749, - "volume": 2497297 - }, - "id": 2721495, - "non_hive": { - "close": 1129, - "high": 28, - "low": 23, - "open": 320, - "volume": 452361 - }, - "open": "2020-09-14T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 81179, - "high": 5, - "low": 13, - "open": 4507, - "volume": 271048 - }, - "id": 2721521, - "non_hive": { - "close": 15002, - "high": 1, - "low": 2, - "open": 831, - "volume": 49790 - }, - "open": "2020-09-14T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 128603, - "low": 19932, - "open": 10342, - "volume": 2450504 - }, - "id": 2721568, - "non_hive": { - "close": 2, - "high": 23766, - "low": 3587, - "open": 1910, - "volume": 447126 - }, - "open": "2020-09-15T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 10, - "low": 277, - "open": 32462, - "volume": 321386 - }, - "id": 2721590, - "non_hive": { - "close": 2, - "high": 2, - "low": 51, - "open": 5983, - "volume": 59299 - }, - "open": "2020-09-15T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 62527, - "high": 3162, - "low": 862, - "open": 23089, - "volume": 1108198 - }, - "id": 2721623, - "non_hive": { - "close": 11545, - "high": 584, - "low": 159, - "open": 4263, - "volume": 204607 - }, - "open": "2020-09-15T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 541599, - "high": 5, - "low": 88, - "open": 245619, - "volume": 1659259 - }, - "id": 2721655, - "non_hive": { - "close": 99111, - "high": 1, - "low": 16, - "open": 45352, - "volume": 304386 - }, - "open": "2020-09-15T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 83, - "high": 270, - "low": 83, - "open": 10832, - "volume": 52910 - }, - "id": 2721683, - "non_hive": { - "close": 15, - "high": 50, - "low": 15, - "open": 1999, - "volume": 9766 - }, - "open": "2020-09-15T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1822, - "high": 10490, - "low": 104221, - "open": 10490, - "volume": 1230161 - }, - "id": 2721707, - "non_hive": { - "close": 336, - "high": 1936, - "low": 18820, - "open": 1936, - "volume": 224454 - }, - "open": "2020-09-15T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 222, - "high": 5, - "low": 446667, - "open": 1291, - "volume": 2400564 - }, - "id": 2721726, - "non_hive": { - "close": 41, - "high": 1, - "low": 80153, - "open": 238, - "volume": 437501 - }, - "open": "2020-09-15T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4666, - "high": 32, - "low": 902, - "open": 472, - "volume": 479446 - }, - "id": 2721784, - "non_hive": { - "close": 860, - "high": 6, - "low": 162, - "open": 87, - "volume": 88079 - }, - "open": "2020-09-15T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49913, - "high": 19207, - "low": 222, - "open": 2549, - "volume": 158400 - }, - "id": 2721832, - "non_hive": { - "close": 9214, - "high": 3551, - "low": 40, - "open": 470, - "volume": 29191 - }, - "open": "2020-09-15T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 444024, - "high": 39000, - "low": 1157497, - "open": 39000, - "volume": 3279488 - }, - "id": 2721864, - "non_hive": { - "close": 79948, - "high": 7191, - "low": 206076, - "open": 7191, - "volume": 589228 - }, - "open": "2020-09-15T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1130, - "high": 4666, - "low": 694922, - "open": 7742, - "volume": 3463106 - }, - "id": 2721889, - "non_hive": { - "close": 207, - "high": 860, - "low": 124460, - "open": 1406, - "volume": 629560 - }, - "open": "2020-09-15T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16892, - "high": 49, - "low": 36, - "open": 7058, - "volume": 2543400 - }, - "id": 2721928, - "non_hive": { - "close": 3089, - "high": 9, - "low": 6, - "open": 1291, - "volume": 460725 - }, - "open": "2020-09-15T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16053, - "high": 5, - "low": 95180, - "open": 13469, - "volume": 2459490 - }, - "id": 2721969, - "non_hive": { - "close": 2960, - "high": 1, - "low": 17227, - "open": 2463, - "volume": 448761 - }, - "open": "2020-09-15T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5410, - "high": 97, - "low": 40, - "open": 106012, - "volume": 1475699 - }, - "id": 2722009, - "non_hive": { - "close": 995, - "high": 18, - "low": 7, - "open": 19536, - "volume": 267894 - }, - "open": "2020-09-15T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8202, - "high": 43, - "low": 115, - "open": 1082, - "volume": 1486273 - }, - "id": 2722058, - "non_hive": { - "close": 1500, - "high": 8, - "low": 20, - "open": 199, - "volume": 263077 - }, - "open": "2020-09-15T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50528, - "high": 27, - "low": 907318, - "open": 382482, - "volume": 5009721 - }, - "id": 2722104, - "non_hive": { - "close": 9211, - "high": 5, - "low": 157056, - "open": 67317, - "volume": 880382 - }, - "open": "2020-09-15T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6757, - "high": 10, - "low": 12, - "open": 5000, - "volume": 6540059 - }, - "id": 2722168, - "non_hive": { - "close": 1199, - "high": 2, - "low": 2, - "open": 912, - "volume": 1136072 - }, - "open": "2020-09-15T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7222, - "high": 39, - "low": 20, - "open": 11559, - "volume": 4346752 - }, - "id": 2722213, - "non_hive": { - "close": 1278, - "high": 7, - "low": 3, - "open": 2000, - "volume": 751235 - }, - "open": "2020-09-15T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 67, - "high": 5, - "low": 359, - "open": 11365, - "volume": 2336238 - }, - "id": 2722264, - "non_hive": { - "close": 12, - "high": 1, - "low": 62, - "open": 1999, - "volume": 407400 - }, - "open": "2020-09-15T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 334, - "high": 118, - "low": 15, - "open": 11487, - "volume": 1821754 - }, - "id": 2722327, - "non_hive": { - "close": 59, - "high": 21, - "low": 2, - "open": 2000, - "volume": 318283 - }, - "open": "2020-09-15T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5804, - "high": 3608, - "low": 264, - "open": 322, - "volume": 4756585 - }, - "id": 2722381, - "non_hive": { - "close": 1016, - "high": 639, - "low": 45, - "open": 57, - "volume": 827855 - }, - "open": "2020-09-15T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3948, - "high": 756, - "low": 544, - "open": 6018, - "volume": 1559464 - }, - "id": 2722437, - "non_hive": { - "close": 699, - "high": 134, - "low": 94, - "open": 1065, - "volume": 272469 - }, - "open": "2020-09-15T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 174265, - "high": 62, - "low": 218, - "open": 28244, - "volume": 2206654 - }, - "id": 2722490, - "non_hive": { - "close": 30856, - "high": 11, - "low": 37, - "open": 5000, - "volume": 386554 - }, - "open": "2020-09-15T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13183, - "high": 344, - "low": 29, - "open": 212, - "volume": 1132843 - }, - "id": 2722536, - "non_hive": { - "close": 2334, - "high": 61, - "low": 5, - "open": 37, - "volume": 198600 - }, - "open": "2020-09-15T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 2336, - "low": 13, - "open": 10715, - "volume": 6122798 - }, - "id": 2722579, - "non_hive": { - "close": 2, - "high": 420, - "low": 2, - "open": 1925, - "volume": 1030714 - }, - "open": "2020-09-16T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111, - "high": 66635, - "low": 197, - "open": 2843, - "volume": 4319960 - }, - "id": 2722607, - "non_hive": { - "close": 20, - "high": 12081, - "low": 32, - "open": 506, - "volume": 716681 - }, - "open": "2020-09-16T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1275, - "high": 17157, - "low": 85, - "open": 11434, - "volume": 113631 - }, - "id": 2722644, - "non_hive": { - "close": 223, - "high": 3001, - "low": 14, - "open": 1999, - "volume": 19871 - }, - "open": "2020-09-16T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1115, - "high": 62, - "low": 25, - "open": 5005, - "volume": 138695 - }, - "id": 2722663, - "non_hive": { - "close": 195, - "high": 11, - "low": 4, - "open": 875, - "volume": 24117 - }, - "open": "2020-09-16T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2862, - "high": 11, - "low": 1758, - "open": 11665, - "volume": 198460 - }, - "id": 2722699, - "non_hive": { - "close": 500, - "high": 2, - "low": 293, - "open": 1996, - "volume": 34366 - }, - "open": "2020-09-16T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 574, - "high": 223, - "low": 2128, - "open": 223, - "volume": 116960 - }, - "id": 2722722, - "non_hive": { - "close": 100, - "high": 39, - "low": 370, - "open": 39, - "volume": 20377 - }, - "open": "2020-09-16T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8984, - "high": 8984, - "low": 11, - "open": 9426, - "volume": 221573 - }, - "id": 2722743, - "non_hive": { - "close": 1571, - "high": 1571, - "low": 1, - "open": 1641, - "volume": 38610 - }, - "open": "2020-09-16T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5727, - "high": 17166, - "low": 3591, - "open": 11627, - "volume": 409911 - }, - "id": 2722789, - "non_hive": { - "close": 1001, - "high": 3001, - "low": 604, - "open": 2000, - "volume": 71075 - }, - "open": "2020-09-16T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9270, - "high": 474, - "low": 10, - "open": 230850, - "volume": 434386 - }, - "id": 2722815, - "non_hive": { - "close": 1594, - "high": 83, - "low": 1, - "open": 40345, - "volume": 75715 - }, - "open": "2020-09-16T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18073, - "high": 11, - "low": 153072, - "open": 827, - "volume": 3772842 - }, - "id": 2722859, - "non_hive": { - "close": 3157, - "high": 2, - "low": 25426, - "open": 140, - "volume": 631609 - }, - "open": "2020-09-16T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34714, - "high": 34714, - "low": 12857, - "open": 980, - "volume": 1366915 - }, - "id": 2722899, - "non_hive": { - "close": 6070, - "high": 6070, - "low": 2137, - "open": 170, - "volume": 230713 - }, - "open": "2020-09-16T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9455, - "high": 154, - "low": 9410, - "open": 13380, - "volume": 328805 - }, - "id": 2722921, - "non_hive": { - "close": 1653, - "high": 27, - "low": 1578, - "open": 2339, - "volume": 57357 - }, - "open": "2020-09-16T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22527, - "high": 11, - "low": 11918, - "open": 43060, - "volume": 888041 - }, - "id": 2722961, - "non_hive": { - "close": 3936, - "high": 2, - "low": 2000, - "open": 7526, - "volume": 155012 - }, - "open": "2020-09-16T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12161, - "high": 57, - "low": 32680, - "open": 17722, - "volume": 193164 - }, - "id": 2722991, - "non_hive": { - "close": 2127, - "high": 10, - "low": 5708, - "open": 3098, - "volume": 33753 - }, - "open": "2020-09-16T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 339, - "high": 17986, - "low": 102, - "open": 34235, - "volume": 2498250 - }, - "id": 2723035, - "non_hive": { - "close": 59, - "high": 3145, - "low": 17, - "open": 5986, - "volume": 423386 - }, - "open": "2020-09-16T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1597, - "high": 74, - "low": 4332, - "open": 161, - "volume": 107493 - }, - "id": 2723081, - "non_hive": { - "close": 277, - "high": 13, - "low": 736, - "open": 28, - "volume": 18627 - }, - "open": "2020-09-16T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 97262, - "high": 97262, - "low": 12, - "open": 15474, - "volume": 440604 - }, - "id": 2723111, - "non_hive": { - "close": 17015, - "high": 17015, - "low": 2, - "open": 2684, - "volume": 76651 - }, - "open": "2020-09-16T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13786, - "high": 34, - "low": 141257, - "open": 1885, - "volume": 2210148 - }, - "id": 2723148, - "non_hive": { - "close": 2400, - "high": 6, - "low": 23773, - "open": 327, - "volume": 378470 - }, - "open": "2020-09-16T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5737, - "high": 17, - "low": 19937, - "open": 286359, - "volume": 1633066 - }, - "id": 2723192, - "non_hive": { - "close": 997, - "high": 3, - "low": 3343, - "open": 50001, - "volume": 277102 - }, - "open": "2020-09-16T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5288, - "high": 11, - "low": 285, - "open": 11505, - "volume": 1286048 - }, - "id": 2723236, - "non_hive": { - "close": 914, - "high": 2, - "low": 48, - "open": 2000, - "volume": 221070 - }, - "open": "2020-09-16T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17190, - "high": 74, - "low": 8258, - "open": 584, - "volume": 776638 - }, - "id": 2723306, - "non_hive": { - "close": 3000, - "high": 13, - "low": 1428, - "open": 101, - "volume": 135483 - }, - "open": "2020-09-16T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6143, - "high": 11, - "low": 3740, - "open": 9398, - "volume": 1582277 - }, - "id": 2723345, - "non_hive": { - "close": 1070, - "high": 2, - "low": 628, - "open": 1640, - "volume": 269788 - }, - "open": "2020-09-16T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8403, - "high": 17, - "low": 9, - "open": 5335, - "volume": 111893 - }, - "id": 2723387, - "non_hive": { - "close": 1463, - "high": 3, - "low": 1, - "open": 929, - "volume": 19262 - }, - "open": "2020-09-16T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 442, - "high": 129848, - "low": 24, - "open": 3079, - "volume": 1241730 - }, - "id": 2723422, - "non_hive": { - "close": 77, - "high": 22639, - "low": 4, - "open": 536, - "volume": 216335 - }, - "open": "2020-09-16T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 315, - "low": 12, - "open": 40230, - "volume": 58760 - }, - "id": 2723447, - "non_hive": { - "close": 2, - "high": 55, - "low": 2, - "open": 7012, - "volume": 10241 - }, - "open": "2020-09-17T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 269619, - "high": 120, - "low": 494, - "open": 6253, - "volume": 933033 - }, - "id": 2723463, - "non_hive": { - "close": 47000, - "high": 21, - "low": 85, - "open": 1090, - "volume": 162632 - }, - "open": "2020-09-17T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10975, - "high": 183, - "low": 434, - "open": 183, - "volume": 355290 - }, - "id": 2723508, - "non_hive": { - "close": 1911, - "high": 32, - "low": 75, - "open": 32, - "volume": 61937 - }, - "open": "2020-09-17T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7884, - "high": 1510, - "low": 397, - "open": 2838, - "volume": 828070 - }, - "id": 2723530, - "non_hive": { - "close": 1373, - "high": 263, - "low": 69, - "open": 494, - "volume": 144196 - }, - "open": "2020-09-17T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 325495, - "high": 3358, - "low": 325495, - "open": 34012, - "volume": 3350035 - }, - "id": 2723548, - "non_hive": { - "close": 55985, - "high": 601, - "low": 55985, - "open": 5923, - "volume": 579014 - }, - "open": "2020-09-17T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 238, - "high": 238, - "low": 649, - "open": 1376, - "volume": 57047 - }, - "id": 2723590, - "non_hive": { - "close": 42, - "high": 42, - "low": 114, - "open": 242, - "volume": 10033 - }, - "open": "2020-09-17T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 405, - "high": 62, - "low": 1000, - "open": 1000, - "volume": 46416 - }, - "id": 2723614, - "non_hive": { - "close": 71, - "high": 11, - "low": 170, - "open": 170, - "volume": 8160 - }, - "open": "2020-09-17T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7148, - "high": 4335, - "low": 11429, - "open": 6824, - "volume": 300567 - }, - "id": 2723643, - "non_hive": { - "close": 1258, - "high": 763, - "low": 1999, - "open": 1201, - "volume": 52814 - }, - "open": "2020-09-17T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11513, - "high": 3994, - "low": 483551, - "open": 53865, - "volume": 4604697 - }, - "id": 2723680, - "non_hive": { - "close": 1999, - "high": 703, - "low": 81239, - "open": 9479, - "volume": 779514 - }, - "open": "2020-09-17T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33688, - "high": 74, - "low": 24, - "open": 787, - "volume": 223305 - }, - "id": 2723733, - "non_hive": { - "close": 5854, - "high": 13, - "low": 4, - "open": 136, - "volume": 38791 - }, - "open": "2020-09-17T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11627, - "high": 8603, - "low": 3694, - "open": 265, - "volume": 127619 - }, - "id": 2723771, - "non_hive": { - "close": 1990, - "high": 1495, - "low": 626, - "open": 46, - "volume": 21928 - }, - "open": "2020-09-17T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48341, - "high": 535, - "low": 48341, - "open": 535, - "volume": 204160 - }, - "id": 2723790, - "non_hive": { - "close": 8218, - "high": 93, - "low": 8218, - "open": 93, - "volume": 34931 - }, - "open": "2020-09-17T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29, - "high": 52, - "low": 6494, - "open": 11758, - "volume": 629365 - }, - "id": 2723809, - "non_hive": { - "close": 5, - "high": 9, - "low": 1092, - "open": 1998, - "volume": 108047 - }, - "open": "2020-09-17T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6925, - "high": 121, - "low": 49923, - "open": 11667, - "volume": 3097497 - }, - "id": 2723864, - "non_hive": { - "close": 1193, - "high": 21, - "low": 8294, - "open": 1994, - "volume": 522186 - }, - "open": "2020-09-17T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18975, - "high": 110, - "low": 8, - "open": 11610, - "volume": 4074435 - }, - "id": 2723914, - "non_hive": { - "close": 3244, - "high": 19, - "low": 1, - "open": 1999, - "volume": 687039 - }, - "open": "2020-09-17T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7778, - "high": 40, - "low": 1026514, - "open": 95362, - "volume": 1688053 - }, - "id": 2723976, - "non_hive": { - "close": 1296, - "high": 7, - "low": 170646, - "open": 16304, - "volume": 282985 - }, - "open": "2020-09-17T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2346, - "high": 296, - "low": 3422, - "open": 83, - "volume": 246888 - }, - "id": 2724023, - "non_hive": { - "close": 399, - "high": 51, - "low": 570, - "open": 14, - "volume": 41970 - }, - "open": "2020-09-17T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5060, - "high": 694, - "low": 121, - "open": 131809, - "volume": 546855 - }, - "id": 2724074, - "non_hive": { - "close": 860, - "high": 118, - "low": 20, - "open": 22402, - "volume": 92943 - }, - "open": "2020-09-17T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1010426, - "high": 76, - "low": 1010426, - "open": 76, - "volume": 1904906 - }, - "id": 2724124, - "non_hive": { - "close": 167858, - "high": 13, - "low": 167858, - "open": 13, - "volume": 319238 - }, - "open": "2020-09-17T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 52, - "low": 219, - "open": 11860, - "volume": 426321 - }, - "id": 2724167, - "non_hive": { - "close": 172, - "high": 9, - "low": 36, - "open": 2015, - "volume": 71909 - }, - "open": "2020-09-17T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57, - "high": 28, - "low": 1006361, - "open": 5666, - "volume": 2822859 - }, - "id": 2724205, - "non_hive": { - "close": 10, - "high": 5, - "low": 170121, - "open": 986, - "volume": 482454 - }, - "open": "2020-09-17T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 126, - "high": 14743, - "low": 126, - "open": 4194, - "volume": 325299 - }, - "id": 2724264, - "non_hive": { - "close": 21, - "high": 2580, - "low": 21, - "open": 729, - "volume": 56447 - }, - "open": "2020-09-17T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47908, - "high": 2176, - "low": 47908, - "open": 2176, - "volume": 2297292 - }, - "id": 2724311, - "non_hive": { - "close": 8110, - "high": 381, - "low": 8110, - "open": 381, - "volume": 393669 - }, - "open": "2020-09-17T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 447, - "high": 22, - "low": 11, - "open": 542764, - "volume": 3929354 - }, - "id": 2724352, - "non_hive": { - "close": 75, - "high": 4, - "low": 1, - "open": 91890, - "volume": 669918 - }, - "open": "2020-09-17T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 13104, - "low": 6, - "open": 11696, - "volume": 2259855 - }, - "id": 2724387, - "non_hive": { - "close": 2, - "high": 2284, - "low": 1, - "open": 1999, - "volume": 383369 - }, - "open": "2020-09-18T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12064, - "high": 6344, - "low": 102, - "open": 494, - "volume": 198333 - }, - "id": 2724430, - "non_hive": { - "close": 2027, - "high": 1110, - "low": 17, - "open": 84, - "volume": 33982 - }, - "open": "2020-09-18T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7968, - "high": 199889, - "low": 11539, - "open": 11539, - "volume": 458643 - }, - "id": 2724456, - "non_hive": { - "close": 1392, - "high": 34968, - "low": 1999, - "open": 1999, - "volume": 79827 - }, - "open": "2020-09-18T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 944, - "high": 944, - "low": 48160, - "open": 2195, - "volume": 51299 - }, - "id": 2724466, - "non_hive": { - "close": 163, - "high": 163, - "low": 8315, - "open": 379, - "volume": 8857 - }, - "open": "2020-09-18T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 20179, - "low": 83, - "open": 20179, - "volume": 164909 - }, - "id": 2724475, - "non_hive": { - "close": 170, - "high": 3483, - "low": 14, - "open": 3483, - "volume": 28457 - }, - "open": "2020-09-18T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19974, - "high": 1628, - "low": 12, - "open": 274, - "volume": 3003119 - }, - "id": 2724496, - "non_hive": { - "close": 3494, - "high": 285, - "low": 2, - "open": 47, - "volume": 518619 - }, - "open": "2020-09-18T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37109, - "high": 68, - "low": 34, - "open": 64011, - "volume": 1924876 - }, - "id": 2724546, - "non_hive": { - "close": 6486, - "high": 12, - "low": 5, - "open": 11197, - "volume": 332440 - }, - "open": "2020-09-18T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10258, - "high": 6986, - "low": 11561, - "open": 20151, - "volume": 480012 - }, - "id": 2724579, - "non_hive": { - "close": 1790, - "high": 1222, - "low": 1999, - "open": 3522, - "volume": 83287 - }, - "open": "2020-09-18T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57529, - "high": 7736, - "low": 32, - "open": 24311, - "volume": 294054 - }, - "id": 2724614, - "non_hive": { - "close": 10113, - "high": 1360, - "low": 5, - "open": 4242, - "volume": 51489 - }, - "open": "2020-09-18T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5732, - "high": 830, - "low": 574945, - "open": 20311, - "volume": 2796856 - }, - "id": 2724648, - "non_hive": { - "close": 1001, - "high": 146, - "low": 100000, - "open": 3571, - "volume": 489556 - }, - "open": "2020-09-18T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55893, - "high": 11, - "low": 1145917, - "open": 22214, - "volume": 5861746 - }, - "id": 2724692, - "non_hive": { - "close": 10000, - "high": 2, - "low": 198299, - "open": 3879, - "volume": 1030151 - }, - "open": "2020-09-18T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 127, - "high": 127, - "low": 537469, - "open": 39791, - "volume": 2561171 - }, - "id": 2724747, - "non_hive": { - "close": 23, - "high": 23, - "low": 94775, - "open": 7122, - "volume": 452886 - }, - "open": "2020-09-18T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 383, - "high": 16, - "low": 383, - "open": 439, - "volume": 1724740 - }, - "id": 2724773, - "non_hive": { - "close": 67, - "high": 3, - "low": 67, - "open": 79, - "volume": 305787 - }, - "open": "2020-09-18T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2653, - "high": 139, - "low": 162, - "open": 11347, - "volume": 663112 - }, - "id": 2724831, - "non_hive": { - "close": 477, - "high": 25, - "low": 28, - "open": 2000, - "volume": 118055 - }, - "open": "2020-09-18T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11125, - "high": 72, - "low": 103, - "open": 72, - "volume": 19932 - }, - "id": 2724873, - "non_hive": { - "close": 1999, - "high": 13, - "low": 18, - "open": 13, - "volume": 3582 - }, - "open": "2020-09-18T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 328934, - "high": 11, - "low": 59, - "open": 4300, - "volume": 4763538 - }, - "id": 2724897, - "non_hive": { - "close": 57974, - "high": 2, - "low": 10, - "open": 773, - "volume": 840175 - }, - "open": "2020-09-18T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5184, - "high": 9576, - "low": 10, - "open": 4638, - "volume": 3449382 - }, - "id": 2724945, - "non_hive": { - "close": 916, - "high": 1716, - "low": 1, - "open": 817, - "volume": 605616 - }, - "open": "2020-09-18T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 101710, - "high": 2067, - "low": 32045, - "open": 75158, - "volume": 1884355 - }, - "id": 2724988, - "non_hive": { - "close": 17793, - "high": 362, - "low": 5465, - "open": 13135, - "volume": 324025 - }, - "open": "2020-09-18T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 517423, - "high": 51, - "low": 132, - "open": 503, - "volume": 2128333 - }, - "id": 2725029, - "non_hive": { - "close": 90008, - "high": 9, - "low": 22, - "open": 88, - "volume": 370330 - }, - "open": "2020-09-18T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48736, - "high": 4195, - "low": 2087, - "open": 6956, - "volume": 319429 - }, - "id": 2725077, - "non_hive": { - "close": 8526, - "high": 734, - "low": 363, - "open": 1217, - "volume": 55830 - }, - "open": "2020-09-18T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5278, - "high": 5, - "low": 148, - "open": 2075, - "volume": 2562964 - }, - "id": 2725116, - "non_hive": { - "close": 909, - "high": 1, - "low": 25, - "open": 361, - "volume": 443108 - }, - "open": "2020-09-18T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 222545, - "high": 11314, - "low": 8238, - "open": 11601, - "volume": 767361 - }, - "id": 2725160, - "non_hive": { - "close": 38923, - "high": 1980, - "low": 1420, - "open": 2000, - "volume": 134163 - }, - "open": "2020-09-18T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 131, - "high": 74, - "low": 32, - "open": 12355, - "volume": 3206654 - }, - "id": 2725194, - "non_hive": { - "close": 23, - "high": 13, - "low": 5, - "open": 2161, - "volume": 552384 - }, - "open": "2020-09-18T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1462, - "high": 4001, - "low": 97, - "open": 6762, - "volume": 2008678 - }, - "id": 2725235, - "non_hive": { - "close": 255, - "high": 700, - "low": 16, - "open": 1183, - "volume": 345346 - }, - "open": "2020-09-18T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 22, - "low": 34, - "open": 340, - "volume": 91107 - }, - "id": 2725271, - "non_hive": { - "close": 2, - "high": 4, - "low": 5, - "open": 59, - "volume": 16029 - }, - "open": "2020-09-19T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 153, - "high": 5700, - "low": 11, - "open": 67072, - "volume": 123143 - }, - "id": 2725292, - "non_hive": { - "close": 26, - "high": 1003, - "low": 1, - "open": 11802, - "volume": 21637 - }, - "open": "2020-09-19T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4156, - "high": 875, - "low": 73, - "open": 10990, - "volume": 171897 - }, - "id": 2725308, - "non_hive": { - "close": 731, - "high": 154, - "low": 12, - "open": 1925, - "volume": 29904 - }, - "open": "2020-09-19T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3434, - "high": 3434, - "low": 6380, - "open": 7466, - "volume": 39330 - }, - "id": 2725333, - "non_hive": { - "close": 604, - "high": 604, - "low": 1122, - "open": 1313, - "volume": 6917 - }, - "open": "2020-09-19T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 897, - "high": 119, - "low": 41, - "open": 19031, - "volume": 89916 - }, - "id": 2725340, - "non_hive": { - "close": 157, - "high": 21, - "low": 7, - "open": 3347, - "volume": 15758 - }, - "open": "2020-09-19T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7954, - "high": 5, - "low": 713, - "open": 22989, - "volume": 240033 - }, - "id": 2725366, - "non_hive": { - "close": 1384, - "high": 1, - "low": 124, - "open": 4000, - "volume": 41765 - }, - "open": "2020-09-19T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11496, - "high": 1166, - "low": 16, - "open": 10000, - "volume": 3431306 - }, - "id": 2725394, - "non_hive": { - "close": 1999, - "high": 203, - "low": 2, - "open": 1731, - "volume": 592879 - }, - "open": "2020-09-19T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31260, - "high": 166, - "low": 409, - "open": 16848, - "volume": 1179939 - }, - "id": 2725440, - "non_hive": { - "close": 5439, - "high": 29, - "low": 70, - "open": 2931, - "volume": 204893 - }, - "open": "2020-09-19T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12951, - "high": 7218, - "low": 136, - "open": 7218, - "volume": 4532710 - }, - "id": 2725484, - "non_hive": { - "close": 2248, - "high": 1256, - "low": 23, - "open": 1256, - "volume": 780988 - }, - "open": "2020-09-19T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7525, - "high": 36077, - "low": 7525, - "open": 11523, - "volume": 195731 - }, - "id": 2725529, - "non_hive": { - "close": 1305, - "high": 6277, - "low": 1305, - "open": 1999, - "volume": 34024 - }, - "open": "2020-09-19T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1698, - "high": 690, - "low": 1698, - "open": 1652, - "volume": 1498359 - }, - "id": 2725564, - "non_hive": { - "close": 291, - "high": 120, - "low": 291, - "open": 286, - "volume": 258455 - }, - "open": "2020-09-19T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44005, - "high": 224, - "low": 158377, - "open": 12234, - "volume": 330076 - }, - "id": 2725583, - "non_hive": { - "close": 7646, - "high": 39, - "low": 27248, - "open": 2126, - "volume": 57056 - }, - "open": "2020-09-19T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14379, - "high": 1005, - "low": 806, - "open": 14330, - "volume": 812671 - }, - "id": 2725610, - "non_hive": { - "close": 2500, - "high": 175, - "low": 138, - "open": 2490, - "volume": 141189 - }, - "open": "2020-09-19T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4768, - "high": 3375, - "low": 235, - "open": 235, - "volume": 213839 - }, - "id": 2725647, - "non_hive": { - "close": 829, - "high": 587, - "low": 40, - "open": 40, - "volume": 37182 - }, - "open": "2020-09-19T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 154825, - "high": 28, - "low": 99, - "open": 28, - "volume": 1903897 - }, - "id": 2725680, - "non_hive": { - "close": 26918, - "high": 5, - "low": 17, - "open": 5, - "volume": 329085 - }, - "open": "2020-09-19T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2485, - "high": 471, - "low": 3867, - "open": 471, - "volume": 132767 - }, - "id": 2725731, - "non_hive": { - "close": 430, - "high": 82, - "low": 669, - "open": 82, - "volume": 23046 - }, - "open": "2020-09-19T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 638, - "high": 638, - "low": 12, - "open": 139, - "volume": 251356 - }, - "id": 2725760, - "non_hive": { - "close": 111, - "high": 111, - "low": 2, - "open": 24, - "volume": 43487 - }, - "open": "2020-09-19T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13301, - "high": 379, - "low": 1048786, - "open": 6257, - "volume": 2349710 - }, - "id": 2725798, - "non_hive": { - "close": 2313, - "high": 66, - "low": 180918, - "open": 1088, - "volume": 406973 - }, - "open": "2020-09-19T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2875, - "high": 57, - "low": 1594, - "open": 86267, - "volume": 542168 - }, - "id": 2725833, - "non_hive": { - "close": 500, - "high": 10, - "low": 277, - "open": 15001, - "volume": 94276 - }, - "open": "2020-09-19T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8132, - "high": 310, - "low": 28436, - "open": 11854, - "volume": 1620477 - }, - "id": 2725878, - "non_hive": { - "close": 1401, - "high": 54, - "low": 4896, - "open": 2061, - "volume": 279464 - }, - "open": "2020-09-19T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23, - "high": 80, - "low": 17424, - "open": 4772, - "volume": 1059939 - }, - "id": 2725914, - "non_hive": { - "close": 4, - "high": 14, - "low": 3000, - "open": 822, - "volume": 184168 - }, - "open": "2020-09-19T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11450, - "high": 11450, - "low": 107758, - "open": 24298, - "volume": 1348915 - }, - "id": 2725952, - "non_hive": { - "close": 1991, - "high": 1991, - "low": 18553, - "open": 4223, - "volume": 232499 - }, - "open": "2020-09-19T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 386802, - "high": 178, - "low": 116, - "open": 143772, - "volume": 663007 - }, - "id": 2725974, - "non_hive": { - "close": 67261, - "high": 31, - "low": 20, - "open": 25000, - "volume": 115289 - }, - "open": "2020-09-19T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8304, - "high": 258, - "low": 24, - "open": 24, - "volume": 77472 - }, - "id": 2725994, - "non_hive": { - "close": 1444, - "high": 45, - "low": 4, - "open": 4, - "volume": 13471 - }, - "open": "2020-09-19T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20576, - "high": 5049, - "low": 37116, - "open": 5049, - "volume": 114843 - }, - "id": 2726022, - "non_hive": { - "close": 3578, - "high": 878, - "low": 6454, - "open": 878, - "volume": 19970 - }, - "open": "2020-09-20T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 793, - "high": 879, - "low": 12, - "open": 12, - "volume": 1225896 - }, - "id": 2726038, - "non_hive": { - "close": 138, - "high": 153, - "low": 2, - "open": 2, - "volume": 211257 - }, - "open": "2020-09-20T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22353, - "high": 9356, - "low": 47108, - "open": 17724, - "volume": 180324 - }, - "id": 2726055, - "non_hive": { - "close": 3887, - "high": 1627, - "low": 8191, - "open": 3082, - "volume": 31356 - }, - "open": "2020-09-20T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 500, - "high": 500, - "low": 8778, - "open": 21974, - "volume": 1409486 - }, - "id": 2726071, - "non_hive": { - "close": 87, - "high": 87, - "low": 1497, - "open": 3821, - "volume": 241536 - }, - "open": "2020-09-20T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1184554, - "high": 3118, - "low": 140, - "open": 2538, - "volume": 2512786 - }, - "id": 2726091, - "non_hive": { - "close": 203802, - "high": 542, - "low": 23, - "open": 433, - "volume": 430695 - }, - "open": "2020-09-20T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9373, - "high": 2563, - "low": 32, - "open": 4956, - "volume": 26286 - }, - "id": 2726119, - "non_hive": { - "close": 1627, - "high": 445, - "low": 5, - "open": 860, - "volume": 4562 - }, - "open": "2020-09-20T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5009, - "high": 1295, - "low": 11093, - "open": 1544, - "volume": 94042 - }, - "id": 2726133, - "non_hive": { - "close": 869, - "high": 225, - "low": 1909, - "open": 268, - "volume": 16299 - }, - "open": "2020-09-20T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4582, - "high": 109, - "low": 2500, - "open": 883, - "volume": 182326 - }, - "id": 2726163, - "non_hive": { - "close": 796, - "high": 19, - "low": 433, - "open": 153, - "volume": 31669 - }, - "open": "2020-09-20T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10562, - "high": 4915, - "low": 15941, - "open": 30222, - "volume": 109819 - }, - "id": 2726205, - "non_hive": { - "close": 1835, - "high": 854, - "low": 2762, - "open": 5250, - "volume": 19071 - }, - "open": "2020-09-20T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60257, - "high": 34, - "low": 17270, - "open": 1403, - "volume": 180299 - }, - "id": 2726225, - "non_hive": { - "close": 10478, - "high": 6, - "low": 3000, - "open": 244, - "volume": 31349 - }, - "open": "2020-09-20T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23, - "high": 235, - "low": 11134, - "open": 730, - "volume": 12122 - }, - "id": 2726249, - "non_hive": { - "close": 4, - "high": 41, - "low": 1936, - "open": 127, - "volume": 2108 - }, - "open": "2020-09-20T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2749, - "high": 379, - "low": 20468, - "open": 6820, - "volume": 47714 - }, - "id": 2726261, - "non_hive": { - "close": 478, - "high": 66, - "low": 3559, - "open": 1186, - "volume": 8297 - }, - "open": "2020-09-20T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 333, - "high": 333, - "low": 1220, - "open": 573893, - "volume": 635734 - }, - "id": 2726278, - "non_hive": { - "close": 58, - "high": 58, - "low": 212, - "open": 99788, - "volume": 110541 - }, - "open": "2020-09-20T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3421, - "high": 685, - "low": 168520, - "open": 685, - "volume": 2494986 - }, - "id": 2726299, - "non_hive": { - "close": 592, - "high": 119, - "low": 28648, - "open": 119, - "volume": 427500 - }, - "open": "2020-09-20T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 179194, - "high": 530, - "low": 104, - "open": 27005, - "volume": 652690 - }, - "id": 2726327, - "non_hive": { - "close": 31054, - "high": 92, - "low": 18, - "open": 4679, - "volume": 113091 - }, - "open": "2020-09-20T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 202, - "high": 5089, - "low": 18067, - "open": 404, - "volume": 251265 - }, - "id": 2726361, - "non_hive": { - "close": 35, - "high": 885, - "low": 3130, - "open": 70, - "volume": 43626 - }, - "open": "2020-09-20T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 297136, - "high": 11, - "low": 12, - "open": 11, - "volume": 4273470 - }, - "id": 2726398, - "non_hive": { - "close": 49888, - "high": 2, - "low": 2, - "open": 2, - "volume": 720305 - }, - "open": "2020-09-20T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1086550, - "high": 4420, - "low": 1086550, - "open": 4932, - "volume": 4957782 - }, - "id": 2726446, - "non_hive": { - "close": 182544, - "high": 760, - "low": 182544, - "open": 848, - "volume": 833633 - }, - "open": "2020-09-20T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10062, - "high": 30774, - "low": 248897, - "open": 11662, - "volume": 1396333 - }, - "id": 2726478, - "non_hive": { - "close": 1722, - "high": 5281, - "low": 41565, - "open": 1999, - "volume": 234973 - }, - "open": "2020-09-20T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19475, - "high": 81, - "low": 64678, - "open": 35046, - "volume": 461196 - }, - "id": 2726503, - "non_hive": { - "close": 3333, - "high": 14, - "low": 11069, - "open": 6001, - "volume": 78962 - }, - "open": "2020-09-20T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29227, - "high": 1325, - "low": 17, - "open": 1188, - "volume": 2582508 - }, - "id": 2726532, - "non_hive": { - "close": 5001, - "high": 227, - "low": 2, - "open": 201, - "volume": 435085 - }, - "open": "2020-09-20T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17938, - "high": 420, - "low": 69, - "open": 29226, - "volume": 1656180 - }, - "id": 2726598, - "non_hive": { - "close": 3073, - "high": 72, - "low": 11, - "open": 5000, - "volume": 281048 - }, - "open": "2020-09-20T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26764, - "high": 70, - "low": 40857, - "open": 22550, - "volume": 1037537 - }, - "id": 2726646, - "non_hive": { - "close": 4585, - "high": 12, - "low": 6998, - "open": 3863, - "volume": 177737 - }, - "open": "2020-09-20T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29181, - "high": 11, - "low": 24, - "open": 350, - "volume": 2415238 - }, - "id": 2726686, - "non_hive": { - "close": 5000, - "high": 2, - "low": 4, - "open": 60, - "volume": 413618 - }, - "open": "2020-09-20T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28891, - "high": 638, - "low": 117454, - "open": 13342, - "volume": 1997473 - }, - "id": 2726728, - "non_hive": { - "close": 5000, - "high": 111, - "low": 20124, - "open": 2286, - "volume": 345473 - }, - "open": "2020-09-21T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 126, - "high": 9591, - "low": 12, - "open": 12, - "volume": 3383180 - }, - "id": 2726761, - "non_hive": { - "close": 22, - "high": 1678, - "low": 2, - "open": 2, - "volume": 588358 - }, - "open": "2020-09-21T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7760, - "high": 4058, - "low": 11451, - "open": 11432, - "volume": 138592 - }, - "id": 2726811, - "non_hive": { - "close": 1357, - "high": 710, - "low": 1992, - "open": 1999, - "volume": 24221 - }, - "open": "2020-09-21T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33753, - "high": 240, - "low": 11081, - "open": 3928, - "volume": 214268 - }, - "id": 2726830, - "non_hive": { - "close": 5900, - "high": 42, - "low": 1928, - "open": 685, - "volume": 37400 - }, - "open": "2020-09-21T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 82, - "high": 1298, - "low": 82, - "open": 37000, - "volume": 1110692 - }, - "id": 2726853, - "non_hive": { - "close": 14, - "high": 227, - "low": 14, - "open": 6467, - "volume": 191444 - }, - "open": "2020-09-21T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1413, - "high": 1413, - "low": 39316, - "open": 5349, - "volume": 135531 - }, - "id": 2726886, - "non_hive": { - "close": 246, - "high": 246, - "low": 6776, - "open": 922, - "volume": 23502 - }, - "open": "2020-09-21T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1017, - "high": 120, - "low": 17687, - "open": 3298, - "volume": 100302 - }, - "id": 2726907, - "non_hive": { - "close": 177, - "high": 21, - "low": 3051, - "open": 574, - "volume": 17369 - }, - "open": "2020-09-21T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13938, - "high": 97, - "low": 1188442, - "open": 97, - "volume": 2746295 - }, - "id": 2726937, - "non_hive": { - "close": 2422, - "high": 17, - "low": 205618, - "open": 17, - "volume": 475428 - }, - "open": "2020-09-21T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42422, - "high": 74, - "low": 1165780, - "open": 5421, - "volume": 9914368 - }, - "id": 2726984, - "non_hive": { - "close": 7313, - "high": 13, - "low": 196001, - "open": 943, - "volume": 1699544 - }, - "open": "2020-09-21T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 685, - "high": 11, - "low": 1100790, - "open": 6694, - "volume": 6992141 - }, - "id": 2727037, - "non_hive": { - "close": 117, - "high": 2, - "low": 182735, - "open": 1154, - "volume": 1173804 - }, - "open": "2020-09-21T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13, - "high": 543, - "low": 12, - "open": 12047, - "volume": 10535794 - }, - "id": 2727086, - "non_hive": { - "close": 2, - "high": 91, - "low": 1, - "open": 2000, - "volume": 1725891 - }, - "open": "2020-09-21T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9889, - "high": 74078, - "low": 922661, - "open": 3151, - "volume": 18157491 - }, - "id": 2727135, - "non_hive": { - "close": 1623, - "high": 12380, - "low": 147718, - "open": 514, - "volume": 2958550 - }, - "open": "2020-09-21T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 628724, - "high": 14018, - "low": 1123824, - "open": 2291, - "volume": 10262116 - }, - "id": 2727194, - "non_hive": { - "close": 100000, - "high": 2302, - "low": 176641, - "open": 376, - "volume": 1633235 - }, - "open": "2020-09-21T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20000, - "high": 96711, - "low": 132, - "open": 132, - "volume": 7026625 - }, - "id": 2727250, - "non_hive": { - "close": 3258, - "high": 15953, - "low": 20, - "open": 20, - "volume": 1125827 - }, - "open": "2020-09-21T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 185, - "high": 5614, - "low": 185, - "open": 2768, - "volume": 9021567 - }, - "id": 2727317, - "non_hive": { - "close": 29, - "high": 926, - "low": 29, - "open": 451, - "volume": 1448876 - }, - "open": "2020-09-21T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18659, - "high": 56899, - "low": 240, - "open": 56899, - "volume": 4607751 - }, - "id": 2727394, - "non_hive": { - "close": 3001, - "high": 9222, - "low": 37, - "open": 9222, - "volume": 728439 - }, - "open": "2020-09-21T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1331, - "high": 3036, - "low": 10, - "open": 623, - "volume": 3005520 - }, - "id": 2727462, - "non_hive": { - "close": 213, - "high": 488, - "low": 1, - "open": 100, - "volume": 476834 - }, - "open": "2020-09-21T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30258, - "high": 30258, - "low": 841580, - "open": 106000, - "volume": 11130303 - }, - "id": 2727524, - "non_hive": { - "close": 5001, - "high": 5001, - "low": 132969, - "open": 16960, - "volume": 1809551 - }, - "open": "2020-09-21T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49058, - "high": 836, - "low": 256, - "open": 24146, - "volume": 13832067 - }, - "id": 2727600, - "non_hive": { - "close": 8264, - "high": 143, - "low": 41, - "open": 4000, - "volume": 2297944 - }, - "open": "2020-09-21T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21396, - "high": 7001, - "low": 21396, - "open": 7001, - "volume": 7953135 - }, - "id": 2727675, - "non_hive": { - "close": 3466, - "high": 1178, - "low": 3466, - "open": 1178, - "volume": 1298110 - }, - "open": "2020-09-21T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11851, - "high": 671678, - "low": 233181, - "open": 8209, - "volume": 8422607 - }, - "id": 2727724, - "non_hive": { - "close": 1999, - "high": 115527, - "low": 37780, - "open": 1367, - "volume": 1389296 - }, - "open": "2020-09-21T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 357, - "high": 237, - "low": 32000, - "open": 38837, - "volume": 3689648 - }, - "id": 2727768, - "non_hive": { - "close": 60, - "high": 40, - "low": 5248, - "open": 6554, - "volume": 609330 - }, - "open": "2020-09-21T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29231, - "high": 248114, - "low": 14, - "open": 11913, - "volume": 947800 - }, - "id": 2727824, - "non_hive": { - "close": 5000, - "high": 42639, - "low": 2, - "open": 1999, - "volume": 160555 - }, - "open": "2020-09-21T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5029, - "high": 132, - "low": 25, - "open": 29275, - "volume": 7011325 - }, - "id": 2727873, - "non_hive": { - "close": 874, - "high": 23, - "low": 4, - "open": 5000, - "volume": 1196089 - }, - "open": "2020-09-21T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 129687, - "high": 129687, - "low": 203, - "open": 7679, - "volume": 3094551 - }, - "id": 2727942, - "non_hive": { - "close": 23473, - "high": 23473, - "low": 35, - "open": 1334, - "volume": 539338 - }, - "open": "2020-09-22T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19059, - "high": 17, - "low": 12, - "open": 12, - "volume": 1406968 - }, - "id": 2727988, - "non_hive": { - "close": 3270, - "high": 3, - "low": 2, - "open": 2, - "volume": 242610 - }, - "open": "2020-09-22T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1526, - "high": 154374, - "low": 112601, - "open": 11378, - "volume": 1448793 - }, - "id": 2728011, - "non_hive": { - "close": 267, - "high": 27136, - "low": 19142, - "open": 1999, - "volume": 248431 - }, - "open": "2020-09-22T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8715, - "high": 1420, - "low": 36623, - "open": 1581, - "volume": 2513259 - }, - "id": 2728045, - "non_hive": { - "close": 1467, - "high": 247, - "low": 6042, - "open": 275, - "volume": 422879 - }, - "open": "2020-09-22T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6071, - "high": 5, - "low": 27, - "open": 3534, - "volume": 56456 - }, - "id": 2728064, - "non_hive": { - "close": 1021, - "high": 1, - "low": 4, - "open": 595, - "volume": 9497 - }, - "open": "2020-09-22T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 118, - "high": 374, - "low": 118, - "open": 59402, - "volume": 240128 - }, - "id": 2728085, - "non_hive": { - "close": 19, - "high": 63, - "low": 19, - "open": 9990, - "volume": 40368 - }, - "open": "2020-09-22T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2967, - "high": 3677, - "low": 144050, - "open": 10000, - "volume": 3634838 - }, - "id": 2728116, - "non_hive": { - "close": 494, - "high": 618, - "low": 23336, - "open": 1680, - "volume": 596410 - }, - "open": "2020-09-22T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8496, - "high": 37293, - "low": 43, - "open": 7573, - "volume": 4090382 - }, - "id": 2728140, - "non_hive": { - "close": 1402, - "high": 6198, - "low": 6, - "open": 1258, - "volume": 658478 - }, - "open": "2020-09-22T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12251, - "high": 249, - "low": 1187231, - "open": 19183, - "volume": 6265173 - }, - "id": 2728177, - "non_hive": { - "close": 1999, - "high": 41, - "low": 189016, - "open": 3157, - "volume": 1001772 - }, - "open": "2020-09-22T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11400, - "high": 52825, - "low": 416637, - "open": 3000, - "volume": 6096578 - }, - "id": 2728225, - "non_hive": { - "close": 1860, - "high": 8632, - "low": 66253, - "open": 489, - "volume": 977919 - }, - "open": "2020-09-22T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 79326, - "high": 4001, - "low": 195934, - "open": 4001, - "volume": 4985077 - }, - "id": 2728266, - "non_hive": { - "close": 12929, - "high": 653, - "low": 31349, - "open": 653, - "volume": 799419 - }, - "open": "2020-09-22T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6874, - "high": 4908, - "low": 17, - "open": 12265, - "volume": 3841593 - }, - "id": 2728298, - "non_hive": { - "close": 1121, - "high": 801, - "low": 2, - "open": 1999, - "volume": 620525 - }, - "open": "2020-09-22T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6911, - "high": 12933, - "low": 33, - "open": 754, - "volume": 5154112 - }, - "id": 2728333, - "non_hive": { - "close": 1127, - "high": 2111, - "low": 5, - "open": 123, - "volume": 837150 - }, - "open": "2020-09-22T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16210, - "high": 16210, - "low": 421756, - "open": 129, - "volume": 4889798 - }, - "id": 2728381, - "non_hive": { - "close": 2682, - "high": 2682, - "low": 68144, - "open": 21, - "volume": 797435 - }, - "open": "2020-09-22T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36096, - "high": 929, - "low": 7, - "open": 3293, - "volume": 6902246 - }, - "id": 2728417, - "non_hive": { - "close": 6008, - "high": 156, - "low": 1, - "open": 545, - "volume": 1129982 - }, - "open": "2020-09-22T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 261909, - "high": 174, - "low": 300, - "open": 18284, - "volume": 3365881 - }, - "id": 2728472, - "non_hive": { - "close": 43210, - "high": 29, - "low": 48, - "open": 3041, - "volume": 546645 - }, - "open": "2020-09-22T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1003812, - "high": 1182, - "low": 92, - "open": 10678, - "volume": 3939436 - }, - "id": 2728527, - "non_hive": { - "close": 163626, - "high": 195, - "low": 14, - "open": 1761, - "volume": 640405 - }, - "open": "2020-09-22T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16912, - "high": 9212, - "low": 278, - "open": 12121, - "volume": 6618449 - }, - "id": 2728589, - "non_hive": { - "close": 2785, - "high": 1520, - "low": 45, - "open": 1999, - "volume": 1077202 - }, - "open": "2020-09-22T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 243, - "high": 2569, - "low": 203, - "open": 12155, - "volume": 163439 - }, - "id": 2728640, - "non_hive": { - "close": 40, - "high": 423, - "low": 32, - "open": 1999, - "volume": 26896 - }, - "open": "2020-09-22T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9771, - "high": 4740, - "low": 183210, - "open": 64005, - "volume": 5995559 - }, - "id": 2728663, - "non_hive": { - "close": 1633, - "high": 795, - "low": 29680, - "open": 10535, - "volume": 982547 - }, - "open": "2020-09-22T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27929, - "high": 24125, - "low": 10, - "open": 59881, - "volume": 7984471 - }, - "id": 2728725, - "non_hive": { - "close": 4637, - "high": 4098, - "low": 1, - "open": 10000, - "volume": 1336601 - }, - "open": "2020-09-22T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8276, - "high": 29761, - "low": 304535, - "open": 29761, - "volume": 2881294 - }, - "id": 2728810, - "non_hive": { - "close": 1367, - "high": 5000, - "low": 49029, - "open": 5000, - "volume": 470911 - }, - "open": "2020-09-22T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30223, - "high": 5610, - "low": 154929, - "open": 2003, - "volume": 1658447 - }, - "id": 2728855, - "non_hive": { - "close": 5001, - "high": 940, - "low": 24974, - "open": 331, - "volume": 270084 - }, - "open": "2020-09-22T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30231, - "high": 6365, - "low": 48, - "open": 30222, - "volume": 3510194 - }, - "id": 2728904, - "non_hive": { - "close": 5000, - "high": 1070, - "low": 7, - "open": 5000, - "volume": 572217 - }, - "open": "2020-09-22T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4366, - "high": 5954, - "low": 8, - "open": 30348, - "volume": 281496 - }, - "id": 2728966, - "non_hive": { - "close": 729, - "high": 999, - "low": 1, - "open": 5001, - "volume": 46448 - }, - "open": "2020-09-23T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 128977, - "high": 5698, - "low": 13, - "open": 13, - "volume": 287116 - }, - "id": 2728999, - "non_hive": { - "close": 21002, - "high": 961, - "low": 2, - "open": 2, - "volume": 47368 - }, - "open": "2020-09-23T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26600, - "high": 114, - "low": 3663, - "open": 6044, - "volume": 107865 - }, - "id": 2729021, - "non_hive": { - "close": 4428, - "high": 19, - "low": 609, - "open": 1006, - "volume": 17950 - }, - "open": "2020-09-23T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30082, - "high": 42, - "low": 14895, - "open": 90158, - "volume": 237379 - }, - "id": 2729042, - "non_hive": { - "close": 5000, - "high": 7, - "low": 2425, - "open": 15001, - "volume": 39380 - }, - "open": "2020-09-23T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15000, - "high": 2177, - "low": 3250, - "open": 30095, - "volume": 3816392 - }, - "id": 2729063, - "non_hive": { - "close": 2400, - "high": 365, - "low": 520, - "open": 5000, - "volume": 612814 - }, - "open": "2020-09-23T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2446, - "high": 10990, - "low": 263185, - "open": 10990, - "volume": 3712434 - }, - "id": 2729094, - "non_hive": { - "close": 412, - "high": 1855, - "low": 41583, - "open": 1855, - "volume": 591254 - }, - "open": "2020-09-23T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4273, - "high": 5, - "low": 42, - "open": 2002, - "volume": 1353295 - }, - "id": 2729125, - "non_hive": { - "close": 717, - "high": 1, - "low": 6, - "open": 337, - "volume": 216215 - }, - "open": "2020-09-23T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3098, - "high": 11, - "low": 2073, - "open": 2718, - "volume": 343840 - }, - "id": 2729165, - "non_hive": { - "close": 520, - "high": 2, - "low": 331, - "open": 456, - "volume": 56902 - }, - "open": "2020-09-23T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1297, - "high": 11, - "low": 23, - "open": 2980, - "volume": 1564113 - }, - "id": 2729194, - "non_hive": { - "close": 217, - "high": 2, - "low": 3, - "open": 499, - "volume": 248898 - }, - "open": "2020-09-23T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7712, - "high": 18411, - "low": 221456, - "open": 1000, - "volume": 3726811 - }, - "id": 2729238, - "non_hive": { - "close": 1254, - "high": 3001, - "low": 34990, - "open": 158, - "volume": 589633 - }, - "open": "2020-09-23T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2886, - "high": 97398, - "low": 10, - "open": 67706, - "volume": 3072414 - }, - "id": 2729273, - "non_hive": { - "close": 456, - "high": 15823, - "low": 1, - "open": 10999, - "volume": 487391 - }, - "open": "2020-09-23T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 209, - "high": 676, - "low": 53312, - "open": 481, - "volume": 1424187 - }, - "id": 2729314, - "non_hive": { - "close": 34, - "high": 110, - "low": 8423, - "open": 78, - "volume": 227236 - }, - "open": "2020-09-23T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3338, - "high": 12, - "low": 3636, - "open": 36395, - "volume": 172400 - }, - "id": 2729344, - "non_hive": { - "close": 543, - "high": 2, - "low": 581, - "open": 5912, - "volume": 28013 - }, - "open": "2020-09-23T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 516, - "high": 780, - "low": 28159, - "open": 4003, - "volume": 780924 - }, - "id": 2729388, - "non_hive": { - "close": 84, - "high": 127, - "low": 4558, - "open": 651, - "volume": 126830 - }, - "open": "2020-09-23T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 358, - "high": 67, - "low": 54434, - "open": 6777, - "volume": 1609576 - }, - "id": 2729443, - "non_hive": { - "close": 58, - "high": 11, - "low": 8709, - "open": 1102, - "volume": 260600 - }, - "open": "2020-09-23T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7364, - "high": 2305, - "low": 31250, - "open": 10000, - "volume": 1098913 - }, - "id": 2729489, - "non_hive": { - "close": 1207, - "high": 378, - "low": 5000, - "open": 1620, - "volume": 177515 - }, - "open": "2020-09-23T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30938, - "high": 36, - "low": 8, - "open": 6211, - "volume": 824916 - }, - "id": 2729539, - "non_hive": { - "close": 5000, - "high": 6, - "low": 1, - "open": 1018, - "volume": 134659 - }, - "open": "2020-09-23T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 124861, - "high": 18, - "low": 10757, - "open": 12375, - "volume": 480391 - }, - "id": 2729595, - "non_hive": { - "close": 20178, - "high": 3, - "low": 1736, - "open": 1999, - "volume": 77631 - }, - "open": "2020-09-23T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7969, - "high": 12, - "low": 125018, - "open": 135719, - "volume": 1790917 - }, - "id": 2729628, - "non_hive": { - "close": 1303, - "high": 2, - "low": 20126, - "open": 22000, - "volume": 289735 - }, - "open": "2020-09-23T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16117, - "high": 25443, - "low": 16117, - "open": 25443, - "volume": 2438350 - }, - "id": 2729655, - "non_hive": { - "close": 2449, - "high": 4145, - "low": 2449, - "open": 4145, - "volume": 381024 - }, - "open": "2020-09-23T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 277603, - "high": 25, - "low": 10000, - "open": 10000, - "volume": 1733176 - }, - "id": 2729689, - "non_hive": { - "close": 42796, - "high": 4, - "low": 1520, - "open": 1520, - "volume": 269268 - }, - "open": "2020-09-23T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7311, - "high": 16364, - "low": 20, - "open": 4605, - "volume": 2465144 - }, - "id": 2729747, - "non_hive": { - "close": 1147, - "high": 2569, - "low": 3, - "open": 719, - "volume": 376868 - }, - "open": "2020-09-23T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3684, - "high": 2002, - "low": 12987, - "open": 1988, - "volume": 3804895 - }, - "id": 2729809, - "non_hive": { - "close": 580, - "high": 326, - "low": 1999, - "open": 312, - "volume": 603336 - }, - "open": "2020-09-23T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13334, - "high": 6, - "low": 13334, - "open": 9014, - "volume": 9098430 - }, - "id": 2729867, - "non_hive": { - "close": 2000, - "high": 1, - "low": 2000, - "open": 1419, - "volume": 1403629 - }, - "open": "2020-09-23T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2579, - "high": 10517, - "low": 1189927, - "open": 10517, - "volume": 2619084 - }, - "id": 2729925, - "non_hive": { - "close": 397, - "high": 1640, - "low": 172559, - "open": 1640, - "volume": 382251 - }, - "open": "2020-09-24T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 245979, - "high": 3662, - "low": 14, - "open": 14, - "volume": 384307 - }, - "id": 2729954, - "non_hive": { - "close": 37843, - "high": 575, - "low": 2, - "open": 2, - "volume": 59240 - }, - "open": "2020-09-24T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19915, - "high": 3162, - "low": 271, - "open": 16232, - "volume": 232718 - }, - "id": 2729984, - "non_hive": { - "close": 3121, - "high": 496, - "low": 41, - "open": 2497, - "volume": 36210 - }, - "open": "2020-09-24T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 38, - "low": 109, - "open": 6276, - "volume": 76338 - }, - "id": 2730009, - "non_hive": { - "close": 153, - "high": 6, - "low": 16, - "open": 983, - "volume": 11811 - }, - "open": "2020-09-24T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 81, - "high": 9375, - "low": 81, - "open": 9375, - "volume": 926013 - }, - "id": 2730033, - "non_hive": { - "close": 12, - "high": 1466, - "low": 12, - "open": 1466, - "volume": 139054 - }, - "open": "2020-09-24T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34238, - "high": 1193, - "low": 42746, - "open": 4326, - "volume": 737094 - }, - "id": 2730063, - "non_hive": { - "close": 5135, - "high": 179, - "low": 6370, - "open": 645, - "volume": 110002 - }, - "open": "2020-09-24T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 61800, - "high": 141, - "low": 13335, - "open": 13335, - "volume": 1581812 - }, - "id": 2730102, - "non_hive": { - "close": 9362, - "high": 22, - "low": 1999, - "open": 1999, - "volume": 238209 - }, - "open": "2020-09-24T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8918, - "high": 38, - "low": 54235, - "open": 6433, - "volume": 1557540 - }, - "id": 2730144, - "non_hive": { - "close": 1386, - "high": 6, - "low": 8081, - "open": 999, - "volume": 239076 - }, - "open": "2020-09-24T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32406, - "high": 362, - "low": 11, - "open": 2812, - "volume": 126575 - }, - "id": 2730191, - "non_hive": { - "close": 5001, - "high": 56, - "low": 1, - "open": 432, - "volume": 19503 - }, - "open": "2020-09-24T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 315, - "high": 301, - "low": 100, - "open": 10458, - "volume": 121191 - }, - "id": 2730218, - "non_hive": { - "close": 49, - "high": 47, - "low": 15, - "open": 1614, - "volume": 18503 - }, - "open": "2020-09-24T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15445, - "high": 385, - "low": 19, - "open": 385, - "volume": 131630 - }, - "id": 2730242, - "non_hive": { - "close": 2401, - "high": 60, - "low": 2, - "open": 60, - "volume": 20470 - }, - "open": "2020-09-24T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12855, - "high": 26383, - "low": 431717, - "open": 3759, - "volume": 1835428 - }, - "id": 2730270, - "non_hive": { - "close": 1999, - "high": 4106, - "low": 65146, - "open": 571, - "volume": 278344 - }, - "open": "2020-09-24T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51, - "high": 21241, - "low": 51, - "open": 32247, - "volume": 261587 - }, - "id": 2730294, - "non_hive": { - "close": 7, - "high": 3307, - "low": 7, - "open": 5017, - "volume": 40706 - }, - "open": "2020-09-24T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2403, - "high": 160, - "low": 6681, - "open": 1948, - "volume": 303621 - }, - "id": 2730329, - "non_hive": { - "close": 374, - "high": 25, - "low": 1002, - "open": 303, - "volume": 47147 - }, - "open": "2020-09-24T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 523, - "high": 604, - "low": 180, - "open": 12792, - "volume": 1228037 - }, - "id": 2730364, - "non_hive": { - "close": 81, - "high": 94, - "low": 27, - "open": 1990, - "volume": 190904 - }, - "open": "2020-09-24T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31558, - "high": 31558, - "low": 17, - "open": 32149, - "volume": 1004114 - }, - "id": 2730416, - "non_hive": { - "close": 4940, - "high": 4940, - "low": 2, - "open": 5000, - "volume": 156367 - }, - "open": "2020-09-24T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5974, - "high": 383, - "low": 26, - "open": 4306, - "volume": 332796 - }, - "id": 2730455, - "non_hive": { - "close": 935, - "high": 60, - "low": 4, - "open": 674, - "volume": 52065 - }, - "open": "2020-09-24T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41, - "high": 3022, - "low": 41, - "open": 121741, - "volume": 259668 - }, - "id": 2730495, - "non_hive": { - "close": 6, - "high": 473, - "low": 6, - "open": 19052, - "volume": 40635 - }, - "open": "2020-09-24T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31959, - "high": 51, - "low": 495211, - "open": 5068, - "volume": 1272306 - }, - "id": 2730528, - "non_hive": { - "close": 5000, - "high": 8, - "low": 77474, - "open": 793, - "volume": 199050 - }, - "open": "2020-09-24T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2601, - "high": 2601, - "low": 12247, - "open": 9095, - "volume": 336212 - }, - "id": 2730585, - "non_hive": { - "close": 407, - "high": 407, - "low": 1916, - "open": 1423, - "volume": 52601 - }, - "open": "2020-09-24T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2099, - "high": 140, - "low": 2099, - "open": 44993, - "volume": 428122 - }, - "id": 2730616, - "non_hive": { - "close": 328, - "high": 22, - "low": 328, - "open": 7039, - "volume": 66979 - }, - "open": "2020-09-24T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31959, - "high": 255, - "low": 37508, - "open": 10220, - "volume": 341153 - }, - "id": 2730655, - "non_hive": { - "close": 5000, - "high": 40, - "low": 5868, - "open": 1599, - "volume": 53374 - }, - "open": "2020-09-24T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 66406, - "high": 198, - "low": 66406, - "open": 31959, - "volume": 373313 - }, - "id": 2730703, - "non_hive": { - "close": 10389, - "high": 31, - "low": 10389, - "open": 5000, - "volume": 58405 - }, - "open": "2020-09-24T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31965, - "high": 185, - "low": 26, - "open": 540825, - "volume": 1763052 - }, - "id": 2730740, - "non_hive": { - "close": 5001, - "high": 29, - "low": 4, - "open": 84610, - "volume": 275828 - }, - "open": "2020-09-24T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31563, - "high": 31563, - "low": 226372, - "open": 31965, - "volume": 1434856 - }, - "id": 2730779, - "non_hive": { - "close": 5142, - "high": 5142, - "low": 35415, - "open": 5001, - "volume": 228655 - }, - "open": "2020-09-25T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15601, - "high": 6, - "low": 13, - "open": 13, - "volume": 62178 - }, - "id": 2730818, - "non_hive": { - "close": 2549, - "high": 1, - "low": 2, - "open": 2, - "volume": 10088 - }, - "open": "2020-09-25T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 157, - "high": 157, - "low": 5646, - "open": 5646, - "volume": 7530 - }, - "id": 2730845, - "non_hive": { - "close": 26, - "high": 26, - "low": 926, - "open": 926, - "volume": 1237 - }, - "open": "2020-09-25T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4495, - "high": 490, - "low": 135, - "open": 1258, - "volume": 79248 - }, - "id": 2730855, - "non_hive": { - "close": 741, - "high": 82, - "low": 22, - "open": 207, - "volume": 13138 - }, - "open": "2020-09-25T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 782, - "high": 1982, - "low": 33, - "open": 1982, - "volume": 2269331 - }, - "id": 2730896, - "non_hive": { - "close": 124, - "high": 327, - "low": 5, - "open": 327, - "volume": 364172 - }, - "open": "2020-09-25T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90769, - "high": 606, - "low": 10418, - "open": 1060, - "volume": 1346117 - }, - "id": 2730959, - "non_hive": { - "close": 14430, - "high": 97, - "low": 1634, - "open": 169, - "volume": 212725 - }, - "open": "2020-09-25T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 320, - "high": 100, - "low": 251, - "open": 12511, - "volume": 112436 - }, - "id": 2730988, - "non_hive": { - "close": 50, - "high": 16, - "low": 39, - "open": 1999, - "volume": 17872 - }, - "open": "2020-09-25T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3962, - "high": 3568, - "low": 4000, - "open": 320, - "volume": 1264457 - }, - "id": 2731016, - "non_hive": { - "close": 634, - "high": 571, - "low": 626, - "open": 51, - "volume": 202071 - }, - "open": "2020-09-25T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1956, - "high": 191, - "low": 6322, - "open": 26438, - "volume": 298450 - }, - "id": 2731052, - "non_hive": { - "close": 315, - "high": 31, - "low": 1005, - "open": 4230, - "volume": 48115 - }, - "open": "2020-09-25T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 31, - "low": 1000, - "open": 6484, - "volume": 76237 - }, - "id": 2731088, - "non_hive": { - "close": 159, - "high": 5, - "low": 159, - "open": 1044, - "volume": 12272 - }, - "open": "2020-09-25T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 319604, - "high": 6668, - "low": 319604, - "open": 450, - "volume": 629324 - }, - "id": 2731112, - "non_hive": { - "close": 50000, - "high": 1067, - "low": 50000, - "open": 72, - "volume": 98822 - }, - "open": "2020-09-25T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4587, - "high": 787, - "low": 18, - "open": 843, - "volume": 400836 - }, - "id": 2731132, - "non_hive": { - "close": 711, - "high": 126, - "low": 2, - "open": 131, - "volume": 63409 - }, - "open": "2020-09-25T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3302, - "high": 6, - "low": 36, - "open": 8315, - "volume": 159613 - }, - "id": 2731168, - "non_hive": { - "close": 525, - "high": 1, - "low": 5, - "open": 1289, - "volume": 24999 - }, - "open": "2020-09-25T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20790, - "high": 131, - "low": 196715, - "open": 12577, - "volume": 418110 - }, - "id": 2731211, - "non_hive": { - "close": 3326, - "high": 21, - "low": 30544, - "open": 2000, - "volume": 65550 - }, - "open": "2020-09-25T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12000, - "high": 23, - "low": 565, - "open": 8020, - "volume": 2655738 - }, - "id": 2731237, - "non_hive": { - "close": 1948, - "high": 4, - "low": 90, - "open": 1283, - "volume": 432599 - }, - "open": "2020-09-25T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2839, - "high": 512, - "low": 11725, - "open": 1655, - "volume": 129391 - }, - "id": 2731315, - "non_hive": { - "close": 464, - "high": 84, - "low": 1904, - "open": 271, - "volume": 21145 - }, - "open": "2020-09-25T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44155, - "high": 807, - "low": 13, - "open": 10322, - "volume": 439132 - }, - "id": 2731346, - "non_hive": { - "close": 7197, - "high": 132, - "low": 2, - "open": 1687, - "volume": 71643 - }, - "open": "2020-09-25T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 153386, - "high": 650, - "low": 5535, - "open": 9282, - "volume": 236949 - }, - "id": 2731400, - "non_hive": { - "close": 25002, - "high": 106, - "low": 902, - "open": 1513, - "volume": 38623 - }, - "open": "2020-09-25T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20692, - "high": 31114, - "low": 81, - "open": 12454, - "volume": 1114362 - }, - "id": 2731425, - "non_hive": { - "close": 3317, - "high": 5000, - "low": 12, - "open": 2000, - "volume": 175203 - }, - "open": "2020-09-25T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 462, - "high": 1466, - "low": 462, - "open": 22729, - "volume": 218605 - }, - "id": 2731468, - "non_hive": { - "close": 74, - "high": 235, - "low": 74, - "open": 3643, - "volume": 35034 - }, - "open": "2020-09-25T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 321, - "high": 4290, - "low": 21, - "open": 31702, - "volume": 1202928 - }, - "id": 2731498, - "non_hive": { - "close": 52, - "high": 695, - "low": 3, - "open": 5077, - "volume": 193326 - }, - "open": "2020-09-25T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11496, - "high": 11496, - "low": 2960, - "open": 1370, - "volume": 776361 - }, - "id": 2731544, - "non_hive": { - "close": 1874, - "high": 1874, - "low": 479, - "open": 222, - "volume": 126524 - }, - "open": "2020-09-25T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1049, - "high": 18, - "low": 30218, - "open": 18, - "volume": 203201 - }, - "id": 2731578, - "non_hive": { - "close": 171, - "high": 3, - "low": 4894, - "open": 3, - "volume": 32939 - }, - "open": "2020-09-25T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5473, - "high": 5473, - "low": 25, - "open": 9059, - "volume": 833027 - }, - "id": 2731605, - "non_hive": { - "close": 919, - "high": 919, - "low": 4, - "open": 1476, - "volume": 136944 - }, - "open": "2020-09-25T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 988, - "high": 988, - "low": 2500, - "open": 9770, - "volume": 315581 - }, - "id": 2731636, - "non_hive": { - "close": 170, - "high": 170, - "low": 418, - "open": 1641, - "volume": 53265 - }, - "open": "2020-09-26T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29892, - "high": 5752, - "low": 12, - "open": 12, - "volume": 226714 - }, - "id": 2731671, - "non_hive": { - "close": 5094, - "high": 1000, - "low": 2, - "open": 2, - "volume": 38865 - }, - "open": "2020-09-26T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29633, - "high": 17, - "low": 7084, - "open": 11732, - "volume": 342374 - }, - "id": 2731713, - "non_hive": { - "close": 5070, - "high": 3, - "low": 1207, - "open": 2000, - "volume": 58500 - }, - "open": "2020-09-26T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29644, - "high": 87, - "low": 2950, - "open": 4911, - "volume": 1748505 - }, - "id": 2731766, - "non_hive": { - "close": 5049, - "high": 15, - "low": 502, - "open": 840, - "volume": 298740 - }, - "open": "2020-09-26T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8872, - "high": 1196, - "low": 34, - "open": 2804, - "volume": 265519 - }, - "id": 2731799, - "non_hive": { - "close": 1520, - "high": 205, - "low": 5, - "open": 480, - "volume": 45233 - }, - "open": "2020-09-26T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2427, - "high": 11626, - "low": 2427, - "open": 1739, - "volume": 60326 - }, - "id": 2731836, - "non_hive": { - "close": 413, - "high": 2000, - "low": 413, - "open": 297, - "volume": 10348 - }, - "open": "2020-09-26T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44138, - "high": 57, - "low": 79, - "open": 2893, - "volume": 366336 - }, - "id": 2731852, - "non_hive": { - "close": 7621, - "high": 10, - "low": 13, - "open": 492, - "volume": 63300 - }, - "open": "2020-09-26T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60281, - "high": 747, - "low": 521, - "open": 52548, - "volume": 335458 - }, - "id": 2731889, - "non_hive": { - "close": 10400, - "high": 129, - "low": 88, - "open": 9073, - "volume": 57848 - }, - "open": "2020-09-26T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 93, - "high": 521, - "low": 599, - "open": 10237, - "volume": 236039 - }, - "id": 2731927, - "non_hive": { - "close": 16, - "high": 90, - "low": 103, - "open": 1766, - "volume": 40712 - }, - "open": "2020-09-26T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11041, - "high": 121, - "low": 23172, - "open": 1912, - "volume": 585024 - }, - "id": 2731965, - "non_hive": { - "close": 1909, - "high": 21, - "low": 3986, - "open": 329, - "volume": 100859 - }, - "open": "2020-09-26T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11978, - "high": 1347, - "low": 521, - "open": 3430, - "volume": 107942 - }, - "id": 2731993, - "non_hive": { - "close": 2071, - "high": 233, - "low": 90, - "open": 593, - "volume": 18662 - }, - "open": "2020-09-26T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5680, - "high": 8264, - "low": 1522, - "open": 9300, - "volume": 428208 - }, - "id": 2732016, - "non_hive": { - "close": 982, - "high": 1429, - "low": 262, - "open": 1608, - "volume": 74031 - }, - "open": "2020-09-26T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 884, - "high": 300, - "low": 5604, - "open": 1093, - "volume": 186257 - }, - "id": 2732044, - "non_hive": { - "close": 153, - "high": 52, - "low": 966, - "open": 189, - "volume": 32173 - }, - "open": "2020-09-26T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4537, - "high": 184, - "low": 89220, - "open": 7890, - "volume": 123957 - }, - "id": 2732073, - "non_hive": { - "close": 785, - "high": 32, - "low": 15435, - "open": 1365, - "volume": 21445 - }, - "open": "2020-09-26T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11000, - "high": 346, - "low": 99, - "open": 10104, - "volume": 669127 - }, - "id": 2732091, - "non_hive": { - "close": 1903, - "high": 60, - "low": 17, - "open": 1748, - "volume": 115758 - }, - "open": "2020-09-26T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2318, - "high": 28, - "low": 1194, - "open": 1364, - "volume": 96716 - }, - "id": 2732133, - "non_hive": { - "close": 401, - "high": 5, - "low": 205, - "open": 236, - "volume": 16677 - }, - "open": "2020-09-26T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29, - "high": 6549, - "low": 12, - "open": 11624, - "volume": 449189 - }, - "id": 2732162, - "non_hive": { - "close": 5, - "high": 1133, - "low": 2, - "open": 2000, - "volume": 77651 - }, - "open": "2020-09-26T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34, - "high": 34, - "low": 5384, - "open": 51881, - "volume": 78536 - }, - "id": 2732195, - "non_hive": { - "close": 6, - "high": 6, - "low": 931, - "open": 8975, - "volume": 13586 - }, - "open": "2020-09-26T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4133, - "high": 109, - "low": 4614, - "open": 2985, - "volume": 1868327 - }, - "id": 2732214, - "non_hive": { - "close": 715, - "high": 19, - "low": 797, - "open": 516, - "volume": 323049 - }, - "open": "2020-09-26T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 306, - "high": 69, - "low": 12544, - "open": 13671, - "volume": 68896 - }, - "id": 2732240, - "non_hive": { - "close": 53, - "high": 12, - "low": 2170, - "open": 2365, - "volume": 11919 - }, - "open": "2020-09-26T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8632, - "high": 8632, - "low": 9161, - "open": 9161, - "volume": 67837 - }, - "id": 2732266, - "non_hive": { - "close": 1502, - "high": 1502, - "low": 1584, - "open": 1584, - "volume": 11778 - }, - "open": "2020-09-26T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15607, - "high": 137, - "low": 90, - "open": 11490, - "volume": 1587156 - }, - "id": 2732288, - "non_hive": { - "close": 2726, - "high": 24, - "low": 15, - "open": 2000, - "volume": 276265 - }, - "open": "2020-09-26T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34432, - "high": 85, - "low": 9, - "open": 59344, - "volume": 1857423 - }, - "id": 2732317, - "non_hive": { - "close": 6054, - "high": 15, - "low": 1, - "open": 10365, - "volume": 323038 - }, - "open": "2020-09-26T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 302, - "high": 9128, - "low": 594, - "open": 1406, - "volume": 1431744 - }, - "id": 2732369, - "non_hive": { - "close": 52, - "high": 1606, - "low": 102, - "open": 243, - "volume": 251629 - }, - "open": "2020-09-26T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1699, - "high": 1699, - "low": 23637, - "open": 28423, - "volume": 53759 - }, - "id": 2732391, - "non_hive": { - "close": 299, - "high": 299, - "low": 4158, - "open": 5000, - "volume": 9457 - }, - "open": "2020-09-27T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 201, - "high": 6327, - "low": 12, - "open": 28424, - "volume": 54096 - }, - "id": 2732402, - "non_hive": { - "close": 35, - "high": 1113, - "low": 2, - "open": 5000, - "volume": 9481 - }, - "open": "2020-09-27T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11481, - "high": 1637, - "low": 13969, - "open": 13969, - "volume": 121262 - }, - "id": 2732421, - "non_hive": { - "close": 2000, - "high": 288, - "low": 2432, - "open": 2432, - "volume": 21216 - }, - "open": "2020-09-27T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90488, - "high": 111000, - "low": 13, - "open": 1234, - "volume": 2362290 - }, - "id": 2732450, - "non_hive": { - "close": 15759, - "high": 19557, - "low": 2, - "open": 217, - "volume": 412460 - }, - "open": "2020-09-27T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 82, - "high": 246, - "low": 58, - "open": 246, - "volume": 1378683 - }, - "id": 2732490, - "non_hive": { - "close": 14, - "high": 43, - "low": 9, - "open": 43, - "volume": 239414 - }, - "open": "2020-09-27T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2463, - "high": 22280, - "low": 195, - "open": 11497, - "volume": 179183 - }, - "id": 2732515, - "non_hive": { - "close": 426, - "high": 3876, - "low": 33, - "open": 1999, - "volume": 31019 - }, - "open": "2020-09-27T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 702, - "high": 4810, - "low": 478, - "open": 6361, - "volume": 195064 - }, - "id": 2732546, - "non_hive": { - "close": 122, - "high": 837, - "low": 81, - "open": 1100, - "volume": 33495 - }, - "open": "2020-09-27T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56660, - "high": 869, - "low": 14271, - "open": 869, - "volume": 250627 - }, - "id": 2732570, - "non_hive": { - "close": 9792, - "high": 151, - "low": 2426, - "open": 151, - "volume": 43128 - }, - "open": "2020-09-27T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3738, - "high": 2098, - "low": 42, - "open": 11725, - "volume": 153819 - }, - "id": 2732599, - "non_hive": { - "close": 635, - "high": 362, - "low": 7, - "open": 2000, - "volume": 26223 - }, - "open": "2020-09-27T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43246, - "high": 15336, - "low": 540, - "open": 11719, - "volume": 221443 - }, - "id": 2732627, - "non_hive": { - "close": 7392, - "high": 2622, - "low": 91, - "open": 1992, - "volume": 37827 - }, - "open": "2020-09-27T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4979, - "high": 10039, - "low": 4979, - "open": 10039, - "volume": 50747 - }, - "id": 2732660, - "non_hive": { - "close": 851, - "high": 1716, - "low": 851, - "open": 1716, - "volume": 8674 - }, - "open": "2020-09-27T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18528, - "high": 263, - "low": 2721, - "open": 2931, - "volume": 68144 - }, - "id": 2732670, - "non_hive": { - "close": 3166, - "high": 45, - "low": 459, - "open": 501, - "volume": 11639 - }, - "open": "2020-09-27T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 216, - "high": 216, - "low": 4803, - "open": 2364, - "volume": 132118 - }, - "id": 2732701, - "non_hive": { - "close": 37, - "high": 37, - "low": 820, - "open": 404, - "volume": 22575 - }, - "open": "2020-09-27T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 720, - "high": 5, - "low": 2539, - "open": 11241, - "volume": 202686 - }, - "id": 2732726, - "non_hive": { - "close": 123, - "high": 1, - "low": 428, - "open": 1921, - "volume": 34312 - }, - "open": "2020-09-27T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 133, - "high": 105, - "low": 15, - "open": 3793, - "volume": 1345211 - }, - "id": 2732757, - "non_hive": { - "close": 22, - "high": 18, - "low": 2, - "open": 647, - "volume": 227420 - }, - "open": "2020-09-27T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13452, - "high": 804, - "low": 13, - "open": 11744, - "volume": 206075 - }, - "id": 2732789, - "non_hive": { - "close": 2288, - "high": 137, - "low": 2, - "open": 1999, - "volume": 35017 - }, - "open": "2020-09-27T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 396759, - "high": 6408, - "low": 98, - "open": 56224, - "volume": 1241340 - }, - "id": 2732819, - "non_hive": { - "close": 67058, - "high": 1091, - "low": 16, - "open": 9561, - "volume": 209847 - }, - "open": "2020-09-27T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2224, - "high": 2224, - "low": 118, - "open": 17645, - "volume": 168285 - }, - "id": 2732873, - "non_hive": { - "close": 376, - "high": 376, - "low": 19, - "open": 2982, - "volume": 28436 - }, - "open": "2020-09-27T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 240236, - "high": 29, - "low": 240236, - "open": 29, - "volume": 1546729 - }, - "id": 2732908, - "non_hive": { - "close": 40576, - "high": 5, - "low": 40576, - "open": 5, - "volume": 261373 - }, - "open": "2020-09-27T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 91552, - "high": 414, - "low": 1144, - "open": 414, - "volume": 182611 - }, - "id": 2732938, - "non_hive": { - "close": 15463, - "high": 70, - "low": 193, - "open": 70, - "volume": 30843 - }, - "open": "2020-09-27T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6565, - "high": 53, - "low": 75567, - "open": 32566, - "volume": 1731560 - }, - "id": 2732967, - "non_hive": { - "close": 1107, - "high": 9, - "low": 12636, - "open": 5500, - "volume": 290893 - }, - "open": "2020-09-27T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 148, - "high": 148, - "low": 197, - "open": 11848, - "volume": 66688 - }, - "id": 2733015, - "non_hive": { - "close": 25, - "high": 25, - "low": 33, - "open": 1999, - "volume": 11255 - }, - "open": "2020-09-27T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8988, - "high": 35, - "low": 44, - "open": 611, - "volume": 632498 - }, - "id": 2733035, - "non_hive": { - "close": 1492, - "high": 6, - "low": 7, - "open": 103, - "volume": 105639 - }, - "open": "2020-09-27T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 528, - "high": 481, - "low": 25, - "open": 11054, - "volume": 453621 - }, - "id": 2733085, - "non_hive": { - "close": 88, - "high": 81, - "low": 4, - "open": 1835, - "volume": 75344 - }, - "open": "2020-09-27T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 88648, - "high": 29781, - "low": 816, - "open": 29781, - "volume": 275440 - }, - "id": 2733120, - "non_hive": { - "close": 14912, - "high": 5014, - "low": 136, - "open": 5014, - "volume": 46350 - }, - "open": "2020-09-28T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1012, - "high": 8354, - "low": 12, - "open": 12, - "volume": 9654 - }, - "id": 2733138, - "non_hive": { - "close": 170, - "high": 1405, - "low": 2, - "open": 2, - "volume": 1623 - }, - "open": "2020-09-28T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21244, - "high": 54202, - "low": 2823, - "open": 500, - "volume": 81508 - }, - "id": 2733150, - "non_hive": { - "close": 3569, - "high": 9106, - "low": 474, - "open": 84, - "volume": 13693 - }, - "open": "2020-09-28T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38577, - "high": 101, - "low": 38577, - "open": 1172, - "volume": 93700 - }, - "id": 2733162, - "non_hive": { - "close": 6476, - "high": 17, - "low": 6476, - "open": 197, - "volume": 15733 - }, - "open": "2020-09-28T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3414, - "high": 166, - "low": 83505, - "open": 166, - "volume": 394912 - }, - "id": 2733184, - "non_hive": { - "close": 560, - "high": 28, - "low": 13362, - "open": 28, - "volume": 64711 - }, - "open": "2020-09-28T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36129, - "high": 21466, - "low": 36129, - "open": 10901, - "volume": 1116654 - }, - "id": 2733225, - "non_hive": { - "close": 5783, - "high": 3522, - "low": 5783, - "open": 1788, - "volume": 181257 - }, - "open": "2020-09-28T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29057, - "high": 19120, - "low": 12217, - "open": 1708, - "volume": 2275751 - }, - "id": 2733270, - "non_hive": { - "close": 4823, - "high": 3177, - "low": 1999, - "open": 280, - "volume": 373001 - }, - "open": "2020-09-28T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5000, - "high": 132, - "low": 112, - "open": 95602, - "volume": 503908 - }, - "id": 2733306, - "non_hive": { - "close": 818, - "high": 22, - "low": 18, - "open": 15865, - "volume": 83536 - }, - "open": "2020-09-28T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1362, - "high": 2061, - "low": 8274, - "open": 3047, - "volume": 695699 - }, - "id": 2733343, - "non_hive": { - "close": 226, - "high": 342, - "low": 1338, - "open": 504, - "volume": 115165 - }, - "open": "2020-09-28T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8132, - "high": 970, - "low": 8132, - "open": 11909, - "volume": 256113 - }, - "id": 2733385, - "non_hive": { - "close": 1343, - "high": 161, - "low": 1343, - "open": 1976, - "volume": 42483 - }, - "open": "2020-09-28T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1838, - "high": 132, - "low": 302, - "open": 16913, - "volume": 732373 - }, - "id": 2733411, - "non_hive": { - "close": 305, - "high": 22, - "low": 49, - "open": 2793, - "volume": 121261 - }, - "open": "2020-09-28T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55737, - "high": 295, - "low": 602, - "open": 295, - "volume": 758502 - }, - "id": 2733439, - "non_hive": { - "close": 9203, - "high": 49, - "low": 99, - "open": 49, - "volume": 125749 - }, - "open": "2020-09-28T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30136, - "high": 24, - "low": 276, - "open": 12055, - "volume": 785392 - }, - "id": 2733503, - "non_hive": { - "close": 5000, - "high": 4, - "low": 45, - "open": 1999, - "volume": 130303 - }, - "open": "2020-09-28T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17100, - "high": 11142, - "low": 602, - "open": 6021, - "volume": 1740803 - }, - "id": 2733561, - "non_hive": { - "close": 2907, - "high": 1897, - "low": 99, - "open": 999, - "volume": 292807 - }, - "open": "2020-09-28T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6011, - "high": 193, - "low": 192, - "open": 353, - "volume": 468635 - }, - "id": 2733617, - "non_hive": { - "close": 1022, - "high": 33, - "low": 32, - "open": 60, - "volume": 79738 - }, - "open": "2020-09-28T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1316, - "high": 5, - "low": 41, - "open": 294133, - "volume": 1365570 - }, - "id": 2733668, - "non_hive": { - "close": 226, - "high": 1, - "low": 6, - "open": 50000, - "volume": 232311 - }, - "open": "2020-09-28T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9306, - "high": 320, - "low": 47, - "open": 320, - "volume": 199936 - }, - "id": 2733723, - "non_hive": { - "close": 1595, - "high": 55, - "low": 7, - "open": 55, - "volume": 34252 - }, - "open": "2020-09-28T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13661, - "high": 419, - "low": 6000, - "open": 33420, - "volume": 1058402 - }, - "id": 2733780, - "non_hive": { - "close": 2343, - "high": 72, - "low": 1028, - "open": 5728, - "volume": 181419 - }, - "open": "2020-09-28T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7437, - "high": 5, - "low": 23127, - "open": 7370, - "volume": 508734 - }, - "id": 2733820, - "non_hive": { - "close": 1277, - "high": 1, - "low": 3963, - "open": 1264, - "volume": 87248 - }, - "open": "2020-09-28T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29320, - "high": 879, - "low": 7, - "open": 33750, - "volume": 1890026 - }, - "id": 2733850, - "non_hive": { - "close": 4939, - "high": 151, - "low": 1, - "open": 5795, - "volume": 319289 - }, - "open": "2020-09-28T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 290, - "high": 1404, - "low": 44, - "open": 11698, - "volume": 216654 - }, - "id": 2733900, - "non_hive": { - "close": 49, - "high": 240, - "low": 7, - "open": 1999, - "volume": 37008 - }, - "open": "2020-09-28T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33588, - "high": 281, - "low": 76, - "open": 334, - "volume": 282160 - }, - "id": 2733935, - "non_hive": { - "close": 5735, - "high": 48, - "low": 12, - "open": 57, - "volume": 48165 - }, - "open": "2020-09-28T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2442, - "high": 5, - "low": 2442, - "open": 20107, - "volume": 772665 - }, - "id": 2733970, - "non_hive": { - "close": 417, - "high": 1, - "low": 417, - "open": 3434, - "volume": 131962 - }, - "open": "2020-09-28T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 445, - "high": 309, - "low": 24, - "open": 1371, - "volume": 892981 - }, - "id": 2734003, - "non_hive": { - "close": 76, - "high": 53, - "low": 4, - "open": 234, - "volume": 152608 - }, - "open": "2020-09-28T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17559, - "high": 304, - "low": 297, - "open": 29252, - "volume": 87664 - }, - "id": 2734024, - "non_hive": { - "close": 3002, - "high": 52, - "low": 50, - "open": 5001, - "volume": 14987 - }, - "open": "2020-09-29T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18334, - "high": 8153, - "low": 12, - "open": 12, - "volume": 1090159 - }, - "id": 2734051, - "non_hive": { - "close": 3083, - "high": 1394, - "low": 2, - "open": 2, - "volume": 184523 - }, - "open": "2020-09-29T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7103, - "high": 5405, - "low": 2611, - "open": 2611, - "volume": 69021 - }, - "id": 2734073, - "non_hive": { - "close": 1214, - "high": 924, - "low": 446, - "open": 446, - "volume": 11797 - }, - "open": "2020-09-29T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8257, - "high": 8257, - "low": 1239, - "open": 11889, - "volume": 78881 - }, - "id": 2734086, - "non_hive": { - "close": 1410, - "high": 1410, - "low": 208, - "open": 2000, - "volume": 13284 - }, - "open": "2020-09-29T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2155, - "high": 12133, - "low": 22, - "open": 3450, - "volume": 1003445 - }, - "id": 2734101, - "non_hive": { - "close": 361, - "high": 2072, - "low": 3, - "open": 589, - "volume": 168444 - }, - "open": "2020-09-29T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7480, - "high": 5217, - "low": 661, - "open": 661, - "volume": 79050 - }, - "id": 2734126, - "non_hive": { - "close": 1253, - "high": 874, - "low": 110, - "open": 110, - "volume": 13238 - }, - "open": "2020-09-29T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40161, - "high": 298, - "low": 205379, - "open": 298, - "volume": 403261 - }, - "id": 2734151, - "non_hive": { - "close": 6727, - "high": 50, - "low": 34401, - "open": 50, - "volume": 67547 - }, - "open": "2020-09-29T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3408, - "high": 3408, - "low": 57003, - "open": 13146, - "volume": 128438 - }, - "id": 2734178, - "non_hive": { - "close": 571, - "high": 571, - "low": 9548, - "open": 2202, - "volume": 21514 - }, - "open": "2020-09-29T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29, - "high": 29, - "low": 29600, - "open": 11868, - "volume": 705298 - }, - "id": 2734201, - "non_hive": { - "close": 5, - "high": 5, - "low": 4958, - "open": 1988, - "volume": 118138 - }, - "open": "2020-09-29T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11910, - "high": 131, - "low": 2184, - "open": 7570, - "volume": 68119 - }, - "id": 2734223, - "non_hive": { - "close": 1995, - "high": 22, - "low": 365, - "open": 1268, - "volume": 11410 - }, - "open": "2020-09-29T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10372, - "high": 101, - "low": 47, - "open": 20250, - "volume": 1164032 - }, - "id": 2734255, - "non_hive": { - "close": 1732, - "high": 17, - "low": 7, - "open": 3392, - "volume": 192836 - }, - "open": "2020-09-29T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 57101, - "high": 3838, - "low": 66, - "open": 499, - "volume": 539807 - }, - "id": 2734293, - "non_hive": { - "close": 9536, - "high": 641, - "low": 10, - "open": 83, - "volume": 90143 - }, - "open": "2020-09-29T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10465, - "high": 83, - "low": 9039, - "open": 28757, - "volume": 165644 - }, - "id": 2734328, - "non_hive": { - "close": 1747, - "high": 14, - "low": 1508, - "open": 4800, - "volume": 27657 - }, - "open": "2020-09-29T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9154, - "high": 227, - "low": 31227, - "open": 18975, - "volume": 438844 - }, - "id": 2734364, - "non_hive": { - "close": 1527, - "high": 38, - "low": 5183, - "open": 3150, - "volume": 73117 - }, - "open": "2020-09-29T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51853, - "high": 3016, - "low": 572, - "open": 3016, - "volume": 511896 - }, - "id": 2734394, - "non_hive": { - "close": 8591, - "high": 503, - "low": 94, - "open": 503, - "volume": 84840 - }, - "open": "2020-09-29T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29583, - "high": 30, - "low": 29583, - "open": 16338, - "volume": 325867 - }, - "id": 2734436, - "non_hive": { - "close": 4901, - "high": 5, - "low": 4901, - "open": 2707, - "volume": 53989 - }, - "open": "2020-09-29T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 63937, - "high": 36, - "low": 13, - "open": 2601, - "volume": 414917 - }, - "id": 2734468, - "non_hive": { - "close": 10593, - "high": 6, - "low": 2, - "open": 431, - "volume": 68738 - }, - "open": "2020-09-29T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6000, - "high": 126, - "low": 696, - "open": 6029, - "volume": 4128506 - }, - "id": 2734506, - "non_hive": { - "close": 979, - "high": 21, - "low": 113, - "open": 999, - "volume": 681674 - }, - "open": "2020-09-29T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1234, - "high": 644, - "low": 467, - "open": 30426, - "volume": 1972457 - }, - "id": 2734556, - "non_hive": { - "close": 203, - "high": 106, - "low": 76, - "open": 5000, - "volume": 324384 - }, - "open": "2020-09-29T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 79, - "high": 455, - "low": 9436, - "open": 18570, - "volume": 467351 - }, - "id": 2734601, - "non_hive": { - "close": 13, - "high": 75, - "low": 1534, - "open": 3054, - "volume": 76675 - }, - "open": "2020-09-29T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2865, - "high": 28407, - "low": 2785, - "open": 3271, - "volume": 293842 - }, - "id": 2734642, - "non_hive": { - "close": 468, - "high": 4673, - "low": 453, - "open": 538, - "volume": 48192 - }, - "open": "2020-09-29T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1539, - "high": 36, - "low": 32181, - "open": 1596, - "volume": 156611 - }, - "id": 2734679, - "non_hive": { - "close": 252, - "high": 6, - "low": 5261, - "open": 261, - "volume": 25620 - }, - "open": "2020-09-29T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16908, - "high": 4753, - "low": 16908, - "open": 33803, - "volume": 119635 - }, - "id": 2734713, - "non_hive": { - "close": 2767, - "high": 778, - "low": 2767, - "open": 5532, - "volume": 19579 - }, - "open": "2020-09-29T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30551, - "high": 366, - "low": 25, - "open": 9020, - "volume": 836527 - }, - "id": 2734731, - "non_hive": { - "close": 5000, - "high": 60, - "low": 4, - "open": 1476, - "volume": 136900 - }, - "open": "2020-09-29T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 965, - "high": 470, - "low": 121, - "open": 21826, - "volume": 97644 - }, - "id": 2734755, - "non_hive": { - "close": 158, - "high": 77, - "low": 19, - "open": 3572, - "volume": 15980 - }, - "open": "2020-09-30T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50147, - "high": 9800, - "low": 13, - "open": 13, - "volume": 172252 - }, - "id": 2734780, - "non_hive": { - "close": 8207, - "high": 1604, - "low": 2, - "open": 2, - "volume": 28190 - }, - "open": "2020-09-30T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 88635, - "high": 1264, - "low": 88635, - "open": 3941, - "volume": 577554 - }, - "id": 2734796, - "non_hive": { - "close": 14427, - "high": 207, - "low": 14427, - "open": 645, - "volume": 94154 - }, - "open": "2020-09-30T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12532, - "high": 12, - "low": 16406, - "open": 751, - "volume": 51160 - }, - "id": 2734808, - "non_hive": { - "close": 2051, - "high": 2, - "low": 2685, - "open": 123, - "volume": 8373 - }, - "open": "2020-09-30T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 86, - "high": 334, - "low": 3000, - "open": 3000, - "volume": 197576 - }, - "id": 2734821, - "non_hive": { - "close": 14, - "high": 55, - "low": 487, - "open": 487, - "volume": 32446 - }, - "open": "2020-09-30T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14446, - "high": 2905, - "low": 76, - "open": 2905, - "volume": 273096 - }, - "id": 2734854, - "non_hive": { - "close": 2376, - "high": 478, - "low": 12, - "open": 478, - "volume": 44867 - }, - "open": "2020-09-30T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 76811, - "high": 3557, - "low": 7828, - "open": 9693, - "volume": 434286 - }, - "id": 2734881, - "non_hive": { - "close": 12617, - "high": 585, - "low": 1272, - "open": 1594, - "volume": 71330 - }, - "open": "2020-09-30T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9994, - "high": 16333, - "low": 9994, - "open": 16333, - "volume": 840128 - }, - "id": 2734905, - "non_hive": { - "close": 1619, - "high": 2683, - "low": 1619, - "open": 2683, - "volume": 136556 - }, - "open": "2020-09-30T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9187, - "high": 5801, - "low": 42735, - "open": 5801, - "volume": 724429 - }, - "id": 2734935, - "non_hive": { - "close": 1470, - "high": 947, - "low": 6837, - "open": 947, - "volume": 116913 - }, - "open": "2020-09-30T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10974, - "high": 1035, - "low": 5437, - "open": 6793, - "volume": 323706 - }, - "id": 2734972, - "non_hive": { - "close": 1791, - "high": 169, - "low": 869, - "open": 1087, - "volume": 52183 - }, - "open": "2020-09-30T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51, - "high": 24, - "low": 51, - "open": 2341, - "volume": 216769 - }, - "id": 2735020, - "non_hive": { - "close": 8, - "high": 4, - "low": 8, - "open": 382, - "volume": 34945 - }, - "open": "2020-09-30T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2290, - "high": 24, - "low": 9498, - "open": 2783, - "volume": 130283 - }, - "id": 2735038, - "non_hive": { - "close": 373, - "high": 4, - "low": 1546, - "open": 453, - "volume": 21214 - }, - "open": "2020-09-30T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 160, - "high": 2671, - "low": 11274, - "open": 6029, - "volume": 89624 - }, - "id": 2735060, - "non_hive": { - "close": 26, - "high": 435, - "low": 1826, - "open": 981, - "volume": 14571 - }, - "open": "2020-09-30T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49, - "high": 49, - "low": 2401, - "open": 4419, - "volume": 70579 - }, - "id": 2735084, - "non_hive": { - "close": 8, - "high": 8, - "low": 384, - "open": 718, - "volume": 11433 - }, - "open": "2020-09-30T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14115, - "high": 388, - "low": 69, - "open": 3496, - "volume": 103029 - }, - "id": 2735123, - "non_hive": { - "close": 2289, - "high": 63, - "low": 11, - "open": 566, - "volume": 16702 - }, - "open": "2020-09-30T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54774, - "high": 55, - "low": 177, - "open": 12464, - "volume": 478531 - }, - "id": 2735167, - "non_hive": { - "close": 8883, - "high": 9, - "low": 28, - "open": 2000, - "volume": 77567 - }, - "open": "2020-09-30T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25788, - "high": 110, - "low": 13, - "open": 1000, - "volume": 3169472 - }, - "id": 2735216, - "non_hive": { - "close": 4152, - "high": 18, - "low": 2, - "open": 162, - "volume": 510013 - }, - "open": "2020-09-30T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31697, - "high": 320, - "low": 26, - "open": 12494, - "volume": 1085949 - }, - "id": 2735264, - "non_hive": { - "close": 5137, - "high": 52, - "low": 4, - "open": 2000, - "volume": 174067 - }, - "open": "2020-09-30T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1313, - "high": 413, - "low": 395, - "open": 888, - "volume": 252246 - }, - "id": 2735296, - "non_hive": { - "close": 213, - "high": 67, - "low": 64, - "open": 144, - "volume": 40900 - }, - "open": "2020-09-30T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11267, - "high": 345, - "low": 11267, - "open": 6889, - "volume": 244733 - }, - "id": 2735336, - "non_hive": { - "close": 1825, - "high": 56, - "low": 1825, - "open": 1117, - "volume": 39667 - }, - "open": "2020-09-30T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1696, - "high": 135, - "low": 406128, - "open": 340, - "volume": 1135318 - }, - "id": 2735395, - "non_hive": { - "close": 275, - "high": 22, - "low": 65677, - "open": 55, - "volume": 183887 - }, - "open": "2020-09-30T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14730, - "high": 135, - "low": 305, - "open": 1301, - "volume": 276808 - }, - "id": 2735436, - "non_hive": { - "close": 2387, - "high": 22, - "low": 49, - "open": 211, - "volume": 44867 - }, - "open": "2020-09-30T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53684, - "high": 1023, - "low": 274, - "open": 19735, - "volume": 1203072 - }, - "id": 2735467, - "non_hive": { - "close": 8706, - "high": 166, - "low": 44, - "open": 3198, - "volume": 195034 - }, - "open": "2020-09-30T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 427, - "high": 2256, - "low": 8819, - "open": 16453, - "volume": 4053644 - }, - "id": 2735501, - "non_hive": { - "close": 68, - "high": 366, - "low": 1393, - "open": 2668, - "volume": 649377 - }, - "open": "2020-09-30T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 540, - "high": 25150, - "low": 8, - "open": 12572, - "volume": 4576694 - }, - "id": 2735538, - "non_hive": { - "close": 83, - "high": 4001, - "low": 1, - "open": 1999, - "volume": 704400 - }, - "open": "2020-10-01T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 382, - "high": 3569, - "low": 275, - "open": 12983, - "volume": 1393663 - }, - "id": 2735573, - "non_hive": { - "close": 59, - "high": 560, - "low": 42, - "open": 2000, - "volume": 215000 - }, - "open": "2020-10-01T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 89, - "high": 89, - "low": 2383, - "open": 2383, - "volume": 371361 - }, - "id": 2735595, - "non_hive": { - "close": 14, - "high": 14, - "low": 373, - "open": 373, - "volume": 58203 - }, - "open": "2020-10-01T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9157, - "high": 15800, - "low": 5924, - "open": 15800, - "volume": 45126 - }, - "id": 2735610, - "non_hive": { - "close": 1433, - "high": 2475, - "low": 927, - "open": 2475, - "volume": 7065 - }, - "open": "2020-10-01T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6354, - "high": 31961, - "low": 18, - "open": 31961, - "volume": 425805 - }, - "id": 2735624, - "non_hive": { - "close": 993, - "high": 5000, - "low": 2, - "open": 5000, - "volume": 66564 - }, - "open": "2020-10-01T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19382, - "high": 15531, - "low": 1390, - "open": 396, - "volume": 323942 - }, - "id": 2735670, - "non_hive": { - "close": 3027, - "high": 2433, - "low": 217, - "open": 62, - "volume": 50685 - }, - "open": "2020-10-01T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14855, - "high": 1703, - "low": 259468, - "open": 1825, - "volume": 1521581 - }, - "id": 2735695, - "non_hive": { - "close": 2344, - "high": 269, - "low": 39992, - "open": 286, - "volume": 236984 - }, - "open": "2020-10-01T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24500, - "high": 38147, - "low": 800, - "open": 38147, - "volume": 398484 - }, - "id": 2735734, - "non_hive": { - "close": 3797, - "high": 5940, - "low": 122, - "open": 5940, - "volume": 61881 - }, - "open": "2020-10-01T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 457, - "high": 39257, - "low": 655, - "open": 34958, - "volume": 1956739 - }, - "id": 2735766, - "non_hive": { - "close": 72, - "high": 6200, - "low": 101, - "open": 5436, - "volume": 304820 - }, - "open": "2020-10-01T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 193172, - "high": 69, - "low": 157, - "open": 8741, - "volume": 293836 - }, - "id": 2735807, - "non_hive": { - "close": 30436, - "high": 11, - "low": 24, - "open": 1377, - "volume": 46303 - }, - "open": "2020-10-01T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19840, - "high": 3839, - "low": 1104, - "open": 102069, - "volume": 263579 - }, - "id": 2735840, - "non_hive": { - "close": 3109, - "high": 605, - "low": 173, - "open": 16082, - "volume": 41484 - }, - "open": "2020-10-01T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24374, - "high": 642, - "low": 7, - "open": 731, - "volume": 204050 - }, - "id": 2735868, - "non_hive": { - "close": 3830, - "high": 101, - "low": 1, - "open": 115, - "volume": 32063 - }, - "open": "2020-10-01T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12227, - "high": 5187, - "low": 596209, - "open": 5187, - "volume": 1838907 - }, - "id": 2735891, - "non_hive": { - "close": 1915, - "high": 815, - "low": 92622, - "open": 815, - "volume": 287000 - }, - "open": "2020-10-01T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1025, - "high": 197, - "low": 3521, - "open": 28866, - "volume": 1116768 - }, - "id": 2735922, - "non_hive": { - "close": 166, - "high": 32, - "low": 551, - "open": 4520, - "volume": 179046 - }, - "open": "2020-10-01T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 163, - "high": 4164, - "low": 163, - "open": 12277, - "volume": 3450718 - }, - "id": 2735976, - "non_hive": { - "close": 25, - "high": 671, - "low": 25, - "open": 1978, - "volume": 543980 - }, - "open": "2020-10-01T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12355, - "high": 30440, - "low": 25, - "open": 12523, - "volume": 2061432 - }, - "id": 2736026, - "non_hive": { - "close": 1939, - "high": 4867, - "low": 3, - "open": 1999, - "volume": 321676 - }, - "open": "2020-10-01T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60549, - "high": 10497, - "low": 11, - "open": 10497, - "volume": 372446 - }, - "id": 2736088, - "non_hive": { - "close": 9320, - "high": 1630, - "low": 1, - "open": 1630, - "volume": 57463 - }, - "open": "2020-10-01T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 238, - "high": 83, - "low": 227, - "open": 10455, - "volume": 549115 - }, - "id": 2736135, - "non_hive": { - "close": 36, - "high": 13, - "low": 34, - "open": 1609, - "volume": 84654 - }, - "open": "2020-10-01T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 83984, - "high": 154, - "low": 349, - "open": 8782, - "volume": 865056 - }, - "id": 2736162, - "non_hive": { - "close": 13000, - "high": 24, - "low": 53, - "open": 1362, - "volume": 133289 - }, - "open": "2020-10-01T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6668, - "high": 6, - "low": 10, - "open": 5928, - "volume": 1578079 - }, - "id": 2736204, - "non_hive": { - "close": 1000, - "high": 1, - "low": 1, - "open": 918, - "volume": 238366 - }, - "open": "2020-10-01T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1576, - "high": 1804, - "low": 1576, - "open": 1804, - "volume": 284748 - }, - "id": 2736259, - "non_hive": { - "close": 237, - "high": 277, - "low": 237, - "open": 277, - "volume": 43370 - }, - "open": "2020-10-01T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11001, - "high": 3745, - "low": 5467, - "open": 6107, - "volume": 36493 - }, - "id": 2736276, - "non_hive": { - "close": 1675, - "high": 571, - "low": 831, - "open": 931, - "volume": 5558 - }, - "open": "2020-10-01T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1890, - "high": 1503, - "low": 394725, - "open": 99334, - "volume": 1333771 - }, - "id": 2736294, - "non_hive": { - "close": 287, - "high": 229, - "low": 59304, - "open": 15132, - "volume": 201367 - }, - "open": "2020-10-01T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 376, - "high": 311, - "low": 27, - "open": 575718, - "volume": 652465 - }, - "id": 2736321, - "non_hive": { - "close": 58, - "high": 48, - "low": 4, - "open": 87561, - "volume": 99349 - }, - "open": "2020-10-01T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1292, - "high": 85000, - "low": 437, - "open": 5807, - "volume": 1413609 - }, - "id": 2736342, - "non_hive": { - "close": 196, - "high": 13249, - "low": 66, - "open": 894, - "volume": 217093 - }, - "open": "2020-10-02T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 219, - "high": 147, - "low": 14, - "open": 14, - "volume": 87962 - }, - "id": 2736373, - "non_hive": { - "close": 34, - "high": 23, - "low": 2, - "open": 2, - "volume": 13629 - }, - "open": "2020-10-02T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6002, - "high": 173, - "low": 11, - "open": 4001, - "volume": 593011 - }, - "id": 2736394, - "non_hive": { - "close": 934, - "high": 27, - "low": 1, - "open": 623, - "volume": 90564 - }, - "open": "2020-10-02T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2845, - "high": 4136, - "low": 11, - "open": 12864, - "volume": 129970 - }, - "id": 2736425, - "non_hive": { - "close": 432, - "high": 645, - "low": 1, - "open": 1999, - "volume": 20157 - }, - "open": "2020-10-02T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 421, - "high": 256, - "low": 185, - "open": 256, - "volume": 72462 - }, - "id": 2736450, - "non_hive": { - "close": 64, - "high": 40, - "low": 28, - "open": 40, - "volume": 11288 - }, - "open": "2020-10-02T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27096, - "high": 5556, - "low": 92711, - "open": 13153, - "volume": 611525 - }, - "id": 2736467, - "non_hive": { - "close": 4196, - "high": 866, - "low": 14096, - "open": 2000, - "volume": 93682 - }, - "open": "2020-10-02T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3740, - "high": 10947, - "low": 191, - "open": 10947, - "volume": 3719761 - }, - "id": 2736500, - "non_hive": { - "close": 561, - "high": 1694, - "low": 27, - "open": 1694, - "volume": 543528 - }, - "open": "2020-10-02T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2860, - "high": 2860, - "low": 1126225, - "open": 240, - "volume": 7334386 - }, - "id": 2736529, - "non_hive": { - "close": 432, - "high": 432, - "low": 163988, - "open": 36, - "volume": 1070676 - }, - "open": "2020-10-02T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 96784, - "high": 2388, - "low": 96784, - "open": 13644, - "volume": 7616700 - }, - "id": 2736570, - "non_hive": { - "close": 14034, - "high": 363, - "low": 14034, - "open": 1999, - "volume": 1107867 - }, - "open": "2020-10-02T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43369, - "high": 353, - "low": 314059, - "open": 2905, - "volume": 5068065 - }, - "id": 2736616, - "non_hive": { - "close": 6371, - "high": 53, - "low": 45224, - "open": 435, - "volume": 740712 - }, - "open": "2020-10-02T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16, - "high": 6817, - "low": 16, - "open": 5095, - "volume": 3748033 - }, - "id": 2736660, - "non_hive": { - "close": 2, - "high": 1001, - "low": 2, - "open": 748, - "volume": 540606 - }, - "open": "2020-10-02T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1765, - "high": 81, - "low": 70, - "open": 729, - "volume": 287520 - }, - "id": 2736690, - "non_hive": { - "close": 259, - "high": 12, - "low": 10, - "open": 107, - "volume": 42156 - }, - "open": "2020-10-02T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6218, - "high": 48983, - "low": 196, - "open": 1167, - "volume": 241010 - }, - "id": 2736717, - "non_hive": { - "close": 911, - "high": 7186, - "low": 28, - "open": 171, - "volume": 35327 - }, - "open": "2020-10-02T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1765, - "high": 46537, - "low": 1765, - "open": 46537, - "volume": 306357 - }, - "id": 2736739, - "non_hive": { - "close": 255, - "high": 6818, - "low": 255, - "open": 6818, - "volume": 44851 - }, - "open": "2020-10-02T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7622, - "high": 7622, - "low": 1765, - "open": 1765, - "volume": 69097 - }, - "id": 2736756, - "non_hive": { - "close": 1173, - "high": 1173, - "low": 258, - "open": 258, - "volume": 10450 - }, - "open": "2020-10-02T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32000, - "high": 2876, - "low": 10, - "open": 11274, - "volume": 1854711 - }, - "id": 2736784, - "non_hive": { - "close": 4861, - "high": 443, - "low": 1, - "open": 1735, - "volume": 282546 - }, - "open": "2020-10-02T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1013, - "high": 922182, - "low": 28, - "open": 322, - "volume": 8273207 - }, - "id": 2736840, - "non_hive": { - "close": 154, - "high": 148452, - "low": 4, - "open": 49, - "volume": 1247653 - }, - "open": "2020-10-02T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 129213, - "high": 262656, - "low": 3361, - "open": 13167, - "volume": 2225174 - }, - "id": 2736909, - "non_hive": { - "close": 19381, - "high": 40396, - "low": 497, - "open": 1999, - "volume": 333125 - }, - "open": "2020-10-02T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3342, - "high": 124, - "low": 278, - "open": 13083, - "volume": 1439742 - }, - "id": 2736935, - "non_hive": { - "close": 501, - "high": 19, - "low": 41, - "open": 1999, - "volume": 218923 - }, - "open": "2020-10-02T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8047, - "high": 943, - "low": 1179147, - "open": 66000, - "volume": 1948765 - }, - "id": 2736991, - "non_hive": { - "close": 1201, - "high": 144, - "low": 174592, - "open": 10008, - "volume": 290162 - }, - "open": "2020-10-02T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13508, - "high": 1106, - "low": 8926, - "open": 1106, - "volume": 1588513 - }, - "id": 2737042, - "non_hive": { - "close": 2000, - "high": 169, - "low": 1321, - "open": 169, - "volume": 237482 - }, - "open": "2020-10-02T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6612, - "high": 117, - "low": 12, - "open": 13300, - "volume": 856183 - }, - "id": 2737072, - "non_hive": { - "close": 990, - "high": 18, - "low": 1, - "open": 1999, - "volume": 128142 - }, - "open": "2020-10-02T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2310, - "high": 165, - "low": 7, - "open": 1851, - "volume": 1372824 - }, - "id": 2737122, - "non_hive": { - "close": 348, - "high": 25, - "low": 1, - "open": 279, - "volume": 203739 - }, - "open": "2020-10-02T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 403, - "high": 18999, - "low": 27, - "open": 6026, - "volume": 1179183 - }, - "id": 2737156, - "non_hive": { - "close": 62, - "high": 2926, - "low": 4, - "open": 916, - "volume": 181461 - }, - "open": "2020-10-02T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 83820, - "high": 32517, - "low": 1000, - "open": 162554, - "volume": 442170 - }, - "id": 2737187, - "non_hive": { - "close": 12867, - "high": 5001, - "low": 152, - "open": 25000, - "volume": 67949 - }, - "open": "2020-10-03T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3139, - "high": 2375, - "low": 14, - "open": 14, - "volume": 22883 - }, - "id": 2737226, - "non_hive": { - "close": 482, - "high": 365, - "low": 2, - "open": 2, - "volume": 3514 - }, - "open": "2020-10-03T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 78097, - "high": 19542, - "low": 139, - "open": 19542, - "volume": 272154 - }, - "id": 2737245, - "non_hive": { - "close": 11978, - "high": 3000, - "low": 21, - "open": 3000, - "volume": 41753 - }, - "open": "2020-10-03T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7294, - "high": 2485, - "low": 287, - "open": 58339, - "volume": 181903 - }, - "id": 2737274, - "non_hive": { - "close": 1110, - "high": 382, - "low": 43, - "open": 8965, - "volume": 27932 - }, - "open": "2020-10-03T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 478433, - "high": 45483, - "low": 40, - "open": 7294, - "volume": 3096840 - }, - "id": 2737303, - "non_hive": { - "close": 71794, - "high": 6987, - "low": 6, - "open": 1119, - "volume": 467977 - }, - "open": "2020-10-03T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9799, - "high": 52, - "low": 87, - "open": 87, - "volume": 375561 - }, - "id": 2737335, - "non_hive": { - "close": 1489, - "high": 8, - "low": 13, - "open": 13, - "volume": 57127 - }, - "open": "2020-10-03T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4738, - "high": 17664, - "low": 29334, - "open": 209, - "volume": 1483504 - }, - "id": 2737355, - "non_hive": { - "close": 715, - "high": 2719, - "low": 4400, - "open": 32, - "volume": 224310 - }, - "open": "2020-10-03T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 259, - "high": 30558, - "low": 262, - "open": 709, - "volume": 1367827 - }, - "id": 2737401, - "non_hive": { - "close": 40, - "high": 4734, - "low": 39, - "open": 107, - "volume": 206163 - }, - "open": "2020-10-03T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 329, - "high": 870, - "low": 329, - "open": 12867, - "volume": 294550 - }, - "id": 2737454, - "non_hive": { - "close": 50, - "high": 135, - "low": 49, - "open": 1993, - "volume": 45572 - }, - "open": "2020-10-03T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12995, - "high": 13691, - "low": 286, - "open": 19203, - "volume": 1046754 - }, - "id": 2737488, - "non_hive": { - "close": 1999, - "high": 2110, - "low": 42, - "open": 2959, - "volume": 160892 - }, - "open": "2020-10-03T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 67, - "high": 136, - "low": 12, - "open": 21556, - "volume": 65510 - }, - "id": 2737528, - "non_hive": { - "close": 10, - "high": 21, - "low": 1, - "open": 3316, - "volume": 10076 - }, - "open": "2020-10-03T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 77788, - "high": 97, - "low": 78607, - "open": 1476, - "volume": 4402932 - }, - "id": 2737550, - "non_hive": { - "close": 11990, - "high": 15, - "low": 12035, - "open": 227, - "volume": 677090 - }, - "open": "2020-10-03T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1701770, - "high": 2189628, - "low": 54310, - "open": 310561, - "volume": 9872602 - }, - "id": 2737580, - "non_hive": { - "close": 275976, - "high": 355092, - "low": 8371, - "open": 47868, - "volume": 1577915 - }, - "open": "2020-10-03T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 142963, - "high": 49, - "low": 3184, - "open": 1184, - "volume": 841063 - }, - "id": 2737621, - "non_hive": { - "close": 23185, - "high": 8, - "low": 496, - "open": 192, - "volume": 136300 - }, - "open": "2020-10-03T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1171025, - "high": 6, - "low": 40, - "open": 333, - "volume": 2491963 - }, - "id": 2737657, - "non_hive": { - "close": 184692, - "high": 1, - "low": 6, - "open": 54, - "volume": 394166 - }, - "open": "2020-10-03T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4123, - "high": 364, - "low": 138, - "open": 364, - "volume": 7369150 - }, - "id": 2737691, - "non_hive": { - "close": 655, - "high": 59, - "low": 21, - "open": 59, - "volume": 1160235 - }, - "open": "2020-10-03T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7262, - "high": 49, - "low": 26, - "open": 12439, - "volume": 158294 - }, - "id": 2737733, - "non_hive": { - "close": 1140, - "high": 8, - "low": 4, - "open": 1999, - "volume": 25249 - }, - "open": "2020-10-03T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19, - "high": 992, - "low": 19, - "open": 992, - "volume": 71765 - }, - "id": 2737773, - "non_hive": { - "close": 2, - "high": 159, - "low": 2, - "open": 159, - "volume": 11458 - }, - "open": "2020-10-03T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29005, - "high": 11190, - "low": 29005, - "open": 11190, - "volume": 144383 - }, - "id": 2737798, - "non_hive": { - "close": 4612, - "high": 1784, - "low": 4612, - "open": 1784, - "volume": 22974 - }, - "open": "2020-10-03T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3782, - "high": 6, - "low": 2242, - "open": 7653, - "volume": 319650 - }, - "id": 2737817, - "non_hive": { - "close": 600, - "high": 1, - "low": 345, - "open": 1216, - "volume": 50641 - }, - "open": "2020-10-03T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17899, - "high": 131, - "low": 2042, - "open": 8598, - "volume": 1498364 - }, - "id": 2737853, - "non_hive": { - "close": 2854, - "high": 21, - "low": 316, - "open": 1364, - "volume": 233580 - }, - "open": "2020-10-03T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 603, - "high": 6267, - "low": 14, - "open": 25103, - "volume": 1380225 - }, - "id": 2737896, - "non_hive": { - "close": 96, - "high": 999, - "low": 2, - "open": 4001, - "volume": 215489 - }, - "open": "2020-10-03T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1664, - "high": 9542, - "low": 221, - "open": 12580, - "volume": 3302419 - }, - "id": 2737946, - "non_hive": { - "close": 259, - "high": 1517, - "low": 33, - "open": 1999, - "volume": 505748 - }, - "open": "2020-10-03T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 171, - "high": 289, - "low": 27, - "open": 12858, - "volume": 1569382 - }, - "id": 2737973, - "non_hive": { - "close": 26, - "high": 45, - "low": 4, - "open": 1999, - "volume": 239678 - }, - "open": "2020-10-03T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8311, - "high": 12741, - "low": 520869, - "open": 12741, - "volume": 2498061 - }, - "id": 2738017, - "non_hive": { - "close": 1268, - "high": 1974, - "low": 77611, - "open": 1974, - "volume": 374373 - }, - "open": "2020-10-04T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8466, - "high": 8882, - "low": 14, - "open": 14, - "volume": 89185 - }, - "id": 2738046, - "non_hive": { - "close": 1289, - "high": 1355, - "low": 2, - "open": 2, - "volume": 13591 - }, - "open": "2020-10-04T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6662, - "high": 2050, - "low": 895, - "open": 2050, - "volume": 115989 - }, - "id": 2738067, - "non_hive": { - "close": 1012, - "high": 312, - "low": 133, - "open": 312, - "volume": 17627 - }, - "open": "2020-10-04T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 701, - "high": 701, - "low": 7960, - "open": 7917, - "volume": 85771 - }, - "id": 2738084, - "non_hive": { - "close": 107, - "high": 107, - "low": 1206, - "open": 1201, - "volume": 13007 - }, - "open": "2020-10-04T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31967, - "high": 14782, - "low": 31967, - "open": 1389, - "volume": 236292 - }, - "id": 2738099, - "non_hive": { - "close": 4879, - "high": 2259, - "low": 4879, - "open": 212, - "volume": 36100 - }, - "open": "2020-10-04T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1309, - "high": 111, - "low": 5830, - "open": 111, - "volume": 3075249 - }, - "id": 2738115, - "non_hive": { - "close": 198, - "high": 17, - "low": 868, - "open": 17, - "volume": 461503 - }, - "open": "2020-10-04T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1003, - "high": 6, - "low": 41, - "open": 3181, - "volume": 108268 - }, - "id": 2738146, - "non_hive": { - "close": 148, - "high": 1, - "low": 6, - "open": 481, - "volume": 16148 - }, - "open": "2020-10-04T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 109583, - "high": 12234, - "low": 33932, - "open": 1459, - "volume": 1044311 - }, - "id": 2738166, - "non_hive": { - "close": 16510, - "high": 1847, - "low": 4987, - "open": 219, - "volume": 156656 - }, - "open": "2020-10-04T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5786, - "high": 5786, - "low": 90747, - "open": 13085, - "volume": 453715 - }, - "id": 2738215, - "non_hive": { - "close": 885, - "high": 885, - "low": 13459, - "open": 1962, - "volume": 68183 - }, - "open": "2020-10-04T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3449, - "high": 61758, - "low": 3771, - "open": 3569, - "volume": 189066 - }, - "id": 2738254, - "non_hive": { - "close": 527, - "high": 9500, - "low": 576, - "open": 546, - "volume": 29012 - }, - "open": "2020-10-04T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17667, - "high": 45, - "low": 4497, - "open": 27351, - "volume": 263739 - }, - "id": 2738282, - "non_hive": { - "close": 2703, - "high": 7, - "low": 687, - "open": 4179, - "volume": 40316 - }, - "open": "2020-10-04T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 253612, - "high": 51, - "low": 253612, - "open": 18000, - "volume": 2839848 - }, - "id": 2738316, - "non_hive": { - "close": 38310, - "high": 8, - "low": 38310, - "open": 2768, - "volume": 431392 - }, - "open": "2020-10-04T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 255, - "high": 255, - "low": 594348, - "open": 6298, - "volume": 1560522 - }, - "id": 2738350, - "non_hive": { - "close": 39, - "high": 39, - "low": 89151, - "open": 945, - "volume": 235092 - }, - "open": "2020-10-04T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12083, - "high": 99187, - "low": 12083, - "open": 99187, - "volume": 111270 - }, - "id": 2738374, - "non_hive": { - "close": 1845, - "high": 15147, - "low": 1845, - "open": 15147, - "volume": 16992 - }, - "open": "2020-10-04T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9593, - "high": 2756, - "low": 99, - "open": 1799, - "volume": 98644 - }, - "id": 2738381, - "non_hive": { - "close": 1465, - "high": 421, - "low": 15, - "open": 274, - "volume": 15059 - }, - "open": "2020-10-04T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7296, - "high": 1393, - "low": 13745, - "open": 13895, - "volume": 88058 - }, - "id": 2738404, - "non_hive": { - "close": 1126, - "high": 215, - "low": 2098, - "open": 2122, - "volume": 13479 - }, - "open": "2020-10-04T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24350, - "high": 4394, - "low": 14, - "open": 137, - "volume": 1520271 - }, - "id": 2738430, - "non_hive": { - "close": 3681, - "high": 678, - "low": 2, - "open": 21, - "volume": 229523 - }, - "open": "2020-10-04T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 51705, - "high": 38, - "low": 1581, - "open": 13419, - "volume": 4321189 - }, - "id": 2738459, - "non_hive": { - "close": 7980, - "high": 6, - "low": 235, - "open": 2000, - "volume": 650840 - }, - "open": "2020-10-04T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 461, - "high": 330, - "low": 93, - "open": 4000, - "volume": 4946131 - }, - "id": 2738516, - "non_hive": { - "close": 71, - "high": 51, - "low": 13, - "open": 617, - "volume": 741259 - }, - "open": "2020-10-04T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4531, - "high": 32393, - "low": 4531, - "open": 12541, - "volume": 336152 - }, - "id": 2738576, - "non_hive": { - "close": 695, - "high": 4983, - "low": 695, - "open": 1928, - "volume": 51644 - }, - "open": "2020-10-04T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32757, - "high": 7369, - "low": 141, - "open": 13327, - "volume": 331039 - }, - "id": 2738609, - "non_hive": { - "close": 5000, - "high": 1128, - "low": 21, - "open": 2000, - "volume": 50240 - }, - "open": "2020-10-04T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30951, - "high": 35429, - "low": 195, - "open": 32763, - "volume": 1105084 - }, - "id": 2738638, - "non_hive": { - "close": 4741, - "high": 5456, - "low": 29, - "open": 5000, - "volume": 169033 - }, - "open": "2020-10-04T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13062, - "high": 194, - "low": 659139, - "open": 4449, - "volume": 2969356 - }, - "id": 2738668, - "non_hive": { - "close": 2000, - "high": 30, - "low": 99681, - "open": 685, - "volume": 452779 - }, - "open": "2020-10-04T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2934, - "high": 156, - "low": 27, - "open": 6128, - "volume": 3274950 - }, - "id": 2738730, - "non_hive": { - "close": 438, - "high": 24, - "low": 4, - "open": 932, - "volume": 490936 - }, - "open": "2020-10-04T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 516, - "high": 3055, - "low": 39, - "open": 444, - "volume": 79290 - }, - "id": 2738756, - "non_hive": { - "close": 79, - "high": 468, - "low": 5, - "open": 67, - "volume": 12134 - }, - "open": "2020-10-05T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 977, - "high": 36216, - "low": 14, - "open": 14, - "volume": 113715 - }, - "id": 2738775, - "non_hive": { - "close": 149, - "high": 5535, - "low": 2, - "open": 2, - "volume": 17267 - }, - "open": "2020-10-05T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13125, - "high": 51, - "low": 6052, - "open": 13118, - "volume": 165662 - }, - "id": 2738793, - "non_hive": { - "close": 2000, - "high": 8, - "low": 922, - "open": 1999, - "volume": 25332 - }, - "open": "2020-10-05T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3830, - "high": 175, - "low": 65, - "open": 5304, - "volume": 418085 - }, - "id": 2738825, - "non_hive": { - "close": 586, - "high": 27, - "low": 9, - "open": 816, - "volume": 63721 - }, - "open": "2020-10-05T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1517, - "high": 1863, - "low": 15, - "open": 15636, - "volume": 1458708 - }, - "id": 2738856, - "non_hive": { - "close": 232, - "high": 286, - "low": 2, - "open": 2400, - "volume": 218895 - }, - "open": "2020-10-05T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 687102, - "high": 17202, - "low": 37, - "open": 18381, - "volume": 6609525 - }, - "id": 2738887, - "non_hive": { - "close": 106535, - "high": 2701, - "low": 5, - "open": 2808, - "volume": 1016446 - }, - "open": "2020-10-05T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1670, - "high": 2628, - "low": 87, - "open": 12659, - "volume": 2222141 - }, - "id": 2738942, - "non_hive": { - "close": 265, - "high": 418, - "low": 13, - "open": 1999, - "volume": 341288 - }, - "open": "2020-10-05T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19768, - "high": 9719, - "low": 804, - "open": 12984, - "volume": 375613 - }, - "id": 2738979, - "non_hive": { - "close": 3078, - "high": 1525, - "low": 123, - "open": 2000, - "volume": 58237 - }, - "open": "2020-10-05T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1198103, - "high": 12730, - "low": 40, - "open": 8178, - "volume": 2957751 - }, - "id": 2739020, - "non_hive": { - "close": 188192, - "high": 2000, - "low": 6, - "open": 1272, - "volume": 458866 - }, - "open": "2020-10-05T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3022, - "high": 43, - "low": 188057, - "open": 31840, - "volume": 1822414 - }, - "id": 2739068, - "non_hive": { - "close": 482, - "high": 7, - "low": 28862, - "open": 5000, - "volume": 285740 - }, - "open": "2020-10-05T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 225, - "high": 31, - "low": 7, - "open": 12721, - "volume": 2977444 - }, - "id": 2739118, - "non_hive": { - "close": 36, - "high": 5, - "low": 1, - "open": 2000, - "volume": 468568 - }, - "open": "2020-10-05T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54847, - "high": 770, - "low": 239, - "open": 7992, - "volume": 2809266 - }, - "id": 2739176, - "non_hive": { - "close": 8891, - "high": 125, - "low": 38, - "open": 1274, - "volume": 448905 - }, - "open": "2020-10-05T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7230, - "high": 746, - "low": 107, - "open": 746, - "volume": 1290274 - }, - "id": 2739215, - "non_hive": { - "close": 1172, - "high": 121, - "low": 17, - "open": 121, - "volume": 206465 - }, - "open": "2020-10-05T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13802, - "high": 80, - "low": 8419, - "open": 2170, - "volume": 568791 - }, - "id": 2739249, - "non_hive": { - "close": 2238, - "high": 13, - "low": 1365, - "open": 352, - "volume": 92237 - }, - "open": "2020-10-05T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10917, - "high": 11871, - "low": 7180, - "open": 149, - "volume": 3201675 - }, - "id": 2739290, - "non_hive": { - "close": 1771, - "high": 1933, - "low": 1153, - "open": 24, - "volume": 519801 - }, - "open": "2020-10-05T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 585552, - "high": 542, - "low": 13, - "open": 4204, - "volume": 3245540 - }, - "id": 2739330, - "non_hive": { - "close": 93245, - "high": 88, - "low": 2, - "open": 682, - "volume": 520135 - }, - "open": "2020-10-05T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5002, - "high": 2104, - "low": 13, - "open": 12339, - "volume": 138210 - }, - "id": 2739369, - "non_hive": { - "close": 810, - "high": 341, - "low": 2, - "open": 1999, - "volume": 22375 - }, - "open": "2020-10-05T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2675, - "high": 159, - "low": 527, - "open": 13278, - "volume": 1527908 - }, - "id": 2739408, - "non_hive": { - "close": 433, - "high": 26, - "low": 83, - "open": 2150, - "volume": 245301 - }, - "open": "2020-10-05T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1863, - "high": 9846, - "low": 77, - "open": 9846, - "volume": 383781 - }, - "id": 2739453, - "non_hive": { - "close": 301, - "high": 1594, - "low": 12, - "open": 1594, - "volume": 61553 - }, - "open": "2020-10-05T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20470, - "high": 8280, - "low": 116, - "open": 11940, - "volume": 1581090 - }, - "id": 2739488, - "non_hive": { - "close": 3232, - "high": 1338, - "low": 18, - "open": 1928, - "volume": 253214 - }, - "open": "2020-10-05T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14881, - "high": 375, - "low": 8, - "open": 12508, - "volume": 1671885 - }, - "id": 2739540, - "non_hive": { - "close": 2381, - "high": 61, - "low": 1, - "open": 1999, - "volume": 271138 - }, - "open": "2020-10-05T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1338, - "high": 3218, - "low": 134166, - "open": 3218, - "volume": 2727179 - }, - "id": 2739577, - "non_hive": { - "close": 214, - "high": 515, - "low": 20929, - "open": 515, - "volume": 429402 - }, - "open": "2020-10-05T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 62, - "high": 62, - "low": 1039348, - "open": 11515, - "volume": 2478742 - }, - "id": 2739609, - "non_hive": { - "close": 10, - "high": 10, - "low": 161291, - "open": 1841, - "volume": 385220 - }, - "open": "2020-10-05T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 975, - "high": 12885, - "low": 14, - "open": 12885, - "volume": 5087097 - }, - "id": 2739637, - "non_hive": { - "close": 151, - "high": 2000, - "low": 2, - "open": 2000, - "volume": 786616 - }, - "open": "2020-10-05T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9113, - "high": 58003, - "low": 446, - "open": 12914, - "volume": 530589 - }, - "id": 2739674, - "non_hive": { - "close": 1411, - "high": 8983, - "low": 69, - "open": 1999, - "volume": 82168 - }, - "open": "2020-10-06T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4539, - "high": 916, - "low": 13, - "open": 13, - "volume": 64771 - }, - "id": 2739700, - "non_hive": { - "close": 703, - "high": 142, - "low": 2, - "open": 2, - "volume": 10029 - }, - "open": "2020-10-06T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14074, - "high": 14074, - "low": 7891, - "open": 26382, - "volume": 54281 - }, - "id": 2739721, - "non_hive": { - "close": 2180, - "high": 2180, - "low": 1219, - "open": 4082, - "volume": 8399 - }, - "open": "2020-10-06T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12395, - "high": 677, - "low": 5000, - "open": 10923, - "volume": 50824 - }, - "id": 2739734, - "non_hive": { - "close": 1920, - "high": 105, - "low": 770, - "open": 1691, - "volume": 7867 - }, - "open": "2020-10-06T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21465, - "high": 387, - "low": 2849, - "open": 2151, - "volume": 64490 - }, - "id": 2739756, - "non_hive": { - "close": 3325, - "high": 60, - "low": 441, - "open": 333, - "volume": 9988 - }, - "open": "2020-10-06T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8211, - "high": 8211, - "low": 84, - "open": 84, - "volume": 81779 - }, - "id": 2739780, - "non_hive": { - "close": 1272, - "high": 1272, - "low": 13, - "open": 13, - "volume": 12667 - }, - "open": "2020-10-06T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1196, - "high": 426, - "low": 497450, - "open": 55075, - "volume": 1273590 - }, - "id": 2739793, - "non_hive": { - "close": 185, - "high": 66, - "low": 75611, - "open": 8531, - "volume": 195130 - }, - "open": "2020-10-06T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 333334, - "high": 717, - "low": 23190, - "open": 1604, - "volume": 2926634 - }, - "id": 2739817, - "non_hive": { - "close": 50000, - "high": 111, - "low": 3478, - "open": 248, - "volume": 441370 - }, - "open": "2020-10-06T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6152, - "high": 12513, - "low": 1073923, - "open": 12513, - "volume": 1721652 - }, - "id": 2739841, - "non_hive": { - "close": 922, - "high": 1876, - "low": 156900, - "open": 1876, - "volume": 253505 - }, - "open": "2020-10-06T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 192295, - "high": 12986, - "low": 125506, - "open": 12434, - "volume": 2195951 - }, - "id": 2739872, - "non_hive": { - "close": 29000, - "high": 2000, - "low": 18577, - "open": 1863, - "volume": 328672 - }, - "open": "2020-10-06T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29773, - "high": 1644, - "low": 37590, - "open": 610, - "volume": 1288567 - }, - "id": 2739935, - "non_hive": { - "close": 4489, - "high": 248, - "low": 5525, - "open": 92, - "volume": 190820 - }, - "open": "2020-10-06T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5657, - "high": 16061, - "low": 1020142, - "open": 663350, - "volume": 3693582 - }, - "id": 2739952, - "non_hive": { - "close": 820, - "high": 2486, - "low": 145890, - "open": 100000, - "volume": 541475 - }, - "open": "2020-10-06T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 116132, - "high": 116132, - "low": 1361, - "open": 8415, - "volume": 4332481 - }, - "id": 2739986, - "non_hive": { - "close": 17641, - "high": 17641, - "low": 194, - "open": 1210, - "volume": 622313 - }, - "open": "2020-10-06T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1414, - "high": 351, - "low": 626, - "open": 2680, - "volume": 326371 - }, - "id": 2740051, - "non_hive": { - "close": 209, - "high": 52, - "low": 91, - "open": 397, - "volume": 48274 - }, - "open": "2020-10-06T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 313, - "high": 737, - "low": 120, - "open": 10208, - "volume": 2735436 - }, - "id": 2740076, - "non_hive": { - "close": 47, - "high": 112, - "low": 17, - "open": 1508, - "volume": 396335 - }, - "open": "2020-10-06T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1907, - "high": 44533, - "low": 87, - "open": 9137, - "volume": 238543 - }, - "id": 2740149, - "non_hive": { - "close": 288, - "high": 6763, - "low": 13, - "open": 1370, - "volume": 35916 - }, - "open": "2020-10-06T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 746, - "high": 52, - "low": 28, - "open": 3940, - "volume": 46735 - }, - "id": 2740196, - "non_hive": { - "close": 112, - "high": 8, - "low": 4, - "open": 595, - "volume": 7044 - }, - "open": "2020-10-06T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27, - "high": 4033, - "low": 27, - "open": 25027, - "volume": 146204 - }, - "id": 2740227, - "non_hive": { - "close": 3, - "high": 605, - "low": 3, - "open": 3753, - "volume": 21924 - }, - "open": "2020-10-06T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1383, - "high": 333, - "low": 146, - "open": 50458, - "volume": 2930916 - }, - "id": 2740253, - "non_hive": { - "close": 202, - "high": 50, - "low": 21, - "open": 7566, - "volume": 432350 - }, - "open": "2020-10-06T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6697, - "high": 6049, - "low": 85519, - "open": 2176, - "volume": 3856837 - }, - "id": 2740296, - "non_hive": { - "close": 998, - "high": 907, - "low": 11972, - "open": 318, - "volume": 544756 - }, - "open": "2020-10-06T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 61762, - "high": 7704, - "low": 61762, - "open": 1045, - "volume": 1244911 - }, - "id": 2740339, - "non_hive": { - "close": 8646, - "high": 1154, - "low": 8646, - "open": 154, - "volume": 174842 - }, - "open": "2020-10-06T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11, - "high": 120, - "low": 11, - "open": 3823, - "volume": 42557 - }, - "id": 2740366, - "non_hive": { - "close": 1, - "high": 18, - "low": 1, - "open": 568, - "volume": 6320 - }, - "open": "2020-10-06T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12493, - "high": 367802, - "low": 49647, - "open": 8659, - "volume": 1854535 - }, - "id": 2740390, - "non_hive": { - "close": 1848, - "high": 54724, - "low": 6519, - "open": 1285, - "volume": 264174 - }, - "open": "2020-10-06T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2914, - "high": 13, - "low": 23, - "open": 1027, - "volume": 1123847 - }, - "id": 2740419, - "non_hive": { - "close": 433, - "high": 2, - "low": 3, - "open": 152, - "volume": 166566 - }, - "open": "2020-10-06T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1088, - "high": 1088, - "low": 9782, - "open": 444, - "volume": 163325 - }, - "id": 2740452, - "non_hive": { - "close": 162, - "high": 162, - "low": 1439, - "open": 66, - "volume": 24258 - }, - "open": "2020-10-07T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24844, - "high": 30727, - "low": 15, - "open": 30727, - "volume": 1264559 - }, - "id": 2740477, - "non_hive": { - "close": 3613, - "high": 4573, - "low": 2, - "open": 4573, - "volume": 179676 - }, - "open": "2020-10-07T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1805, - "high": 40, - "low": 59, - "open": 34387, - "volume": 161517 - }, - "id": 2740501, - "non_hive": { - "close": 266, - "high": 6, - "low": 8, - "open": 5000, - "volume": 23517 - }, - "open": "2020-10-07T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1219, - "high": 504, - "low": 432367, - "open": 13562, - "volume": 1984955 - }, - "id": 2740530, - "non_hive": { - "close": 174, - "high": 75, - "low": 60983, - "open": 1992, - "volume": 284674 - }, - "open": "2020-10-07T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 520218, - "high": 259, - "low": 14717, - "open": 259, - "volume": 4876720 - }, - "id": 2740576, - "non_hive": { - "close": 71738, - "high": 37, - "low": 1999, - "open": 37, - "volume": 672949 - }, - "open": "2020-10-07T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3269, - "high": 103176, - "low": 5028, - "open": 7421, - "volume": 595660 - }, - "id": 2740630, - "non_hive": { - "close": 464, - "high": 14651, - "low": 693, - "open": 1023, - "volume": 83486 - }, - "open": "2020-10-07T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6443, - "high": 1772, - "low": 8097, - "open": 2719, - "volume": 155126 - }, - "id": 2740674, - "non_hive": { - "close": 934, - "high": 257, - "low": 1149, - "open": 386, - "volume": 22200 - }, - "open": "2020-10-07T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26831, - "high": 369, - "low": 26831, - "open": 13240, - "volume": 3121020 - }, - "id": 2740700, - "non_hive": { - "close": 3810, - "high": 55, - "low": 3810, - "open": 1919, - "volume": 452916 - }, - "open": "2020-10-07T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18480, - "high": 63, - "low": 1023, - "open": 324, - "volume": 3289937 - }, - "id": 2740764, - "non_hive": { - "close": 2626, - "high": 9, - "low": 144, - "open": 46, - "volume": 465830 - }, - "open": "2020-10-07T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8159, - "high": 7487, - "low": 200000, - "open": 63, - "volume": 7239023 - }, - "id": 2740808, - "non_hive": { - "close": 1162, - "high": 1107, - "low": 28300, - "open": 9, - "volume": 1041192 - }, - "open": "2020-10-07T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100000, - "high": 926, - "low": 10630, - "open": 67700, - "volume": 2974149 - }, - "id": 2740864, - "non_hive": { - "close": 14400, - "high": 137, - "low": 1500, - "open": 9641, - "volume": 432381 - }, - "open": "2020-10-07T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42, - "high": 446, - "low": 14090, - "open": 14083, - "volume": 12393972 - }, - "id": 2740901, - "non_hive": { - "close": 6, - "high": 66, - "low": 1999, - "open": 1999, - "volume": 1784949 - }, - "open": "2020-10-07T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4078, - "high": 5475, - "low": 14085, - "open": 14085, - "volume": 2960076 - }, - "id": 2740953, - "non_hive": { - "close": 587, - "high": 799, - "low": 1999, - "open": 1999, - "volume": 421693 - }, - "open": "2020-10-07T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3828, - "high": 469, - "low": 82, - "open": 3663, - "volume": 7357677 - }, - "id": 2740980, - "non_hive": { - "close": 545, - "high": 68, - "low": 11, - "open": 527, - "volume": 1043602 - }, - "open": "2020-10-07T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23476, - "high": 5699, - "low": 35, - "open": 149866, - "volume": 7075959 - }, - "id": 2741032, - "non_hive": { - "close": 3365, - "high": 826, - "low": 4, - "open": 21332, - "volume": 996138 - }, - "open": "2020-10-07T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35659, - "high": 13610, - "low": 14286, - "open": 496382, - "volume": 10405256 - }, - "id": 2741100, - "non_hive": { - "close": 5000, - "high": 2000, - "low": 1999, - "open": 70993, - "volume": 1469997 - }, - "open": "2020-10-07T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42, - "high": 4869, - "low": 15, - "open": 9158, - "volume": 9899921 - }, - "id": 2741178, - "non_hive": { - "close": 6, - "high": 715, - "low": 2, - "open": 1283, - "volume": 1409265 - }, - "open": "2020-10-07T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3000000, - "high": 39840, - "low": 1087, - "open": 22549, - "volume": 17125852 - }, - "id": 2741241, - "non_hive": { - "close": 421347, - "high": 5891, - "low": 152, - "open": 3243, - "volume": 2436537 - }, - "open": "2020-10-07T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 107002, - "high": 171, - "low": 401, - "open": 14065, - "volume": 10126114 - }, - "id": 2741309, - "non_hive": { - "close": 15067, - "high": 25, - "low": 55, - "open": 2006, - "volume": 1436873 - }, - "open": "2020-10-07T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 200000, - "high": 13704, - "low": 457, - "open": 12664, - "volume": 2438273 - }, - "id": 2741363, - "non_hive": { - "close": 28400, - "high": 2000, - "low": 64, - "open": 1848, - "volume": 346512 - }, - "open": "2020-10-07T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4894, - "high": 1225, - "low": 9383, - "open": 14082, - "volume": 6761732 - }, - "id": 2741421, - "non_hive": { - "close": 697, - "high": 180, - "low": 1330, - "open": 2000, - "volume": 965974 - }, - "open": "2020-10-07T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35560, - "high": 8744, - "low": 1169, - "open": 2960748, - "volume": 17903199 - }, - "id": 2741478, - "non_hive": { - "close": 5000, - "high": 1300, - "low": 164, - "open": 422001, - "volume": 2573169 - }, - "open": "2020-10-07T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 138370, - "high": 10938, - "low": 123, - "open": 430382, - "volume": 8562310 - }, - "id": 2741558, - "non_hive": { - "close": 20070, - "high": 1617, - "low": 17, - "open": 60501, - "volume": 1232126 - }, - "open": "2020-10-07T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 419906, - "high": 127, - "low": 29, - "open": 285047, - "volume": 16827175 - }, - "id": 2741603, - "non_hive": { - "close": 60637, - "high": 19, - "low": 4, - "open": 41339, - "volume": 2443399 - }, - "open": "2020-10-07T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20163, - "high": 866, - "low": 446, - "open": 446, - "volume": 2829846 - }, - "id": 2741663, - "non_hive": { - "close": 3001, - "high": 129, - "low": 64, - "open": 64, - "volume": 412286 - }, - "open": "2020-10-08T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47, - "high": 248, - "low": 11, - "open": 14, - "volume": 1238103 - }, - "id": 2741703, - "non_hive": { - "close": 7, - "high": 37, - "low": 1, - "open": 2, - "volume": 180706 - }, - "open": "2020-10-08T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34030, - "high": 226, - "low": 13745, - "open": 57179, - "volume": 15447910 - }, - "id": 2741730, - "non_hive": { - "close": 5000, - "high": 34, - "low": 1999, - "open": 8509, - "volume": 2271699 - }, - "open": "2020-10-08T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20814, - "high": 48, - "low": 400, - "open": 48, - "volume": 4353351 - }, - "id": 2741783, - "non_hive": { - "close": 3025, - "high": 7, - "low": 58, - "open": 7, - "volume": 633497 - }, - "open": "2020-10-08T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17162, - "high": 881, - "low": 3112, - "open": 291, - "volume": 12063023 - }, - "id": 2741809, - "non_hive": { - "close": 2507, - "high": 132, - "low": 448, - "open": 42, - "volume": 1770492 - }, - "open": "2020-10-08T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1415, - "high": 5615, - "low": 119, - "open": 13690, - "volume": 9870293 - }, - "id": 2741849, - "non_hive": { - "close": 208, - "high": 841, - "low": 17, - "open": 2000, - "volume": 1432396 - }, - "open": "2020-10-08T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13706, - "high": 47, - "low": 1184672, - "open": 47, - "volume": 2606415 - }, - "id": 2741894, - "non_hive": { - "close": 2000, - "high": 7, - "low": 170627, - "open": 7, - "volume": 375772 - }, - "open": "2020-10-08T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17531, - "high": 20480, - "low": 8720, - "open": 13837, - "volume": 1416465 - }, - "id": 2741917, - "non_hive": { - "close": 2612, - "high": 3060, - "low": 1256, - "open": 2000, - "volume": 205170 - }, - "open": "2020-10-08T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19011, - "high": 8628, - "low": 8, - "open": 20000, - "volume": 548940 - }, - "id": 2741951, - "non_hive": { - "close": 2794, - "high": 1293, - "low": 1, - "open": 2996, - "volume": 81175 - }, - "open": "2020-10-08T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34022, - "high": 34022, - "low": 34022, - "open": 34022, - "volume": 34022 - }, - "id": 2741981, - "non_hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "open": "2020-10-08T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34043, - "high": 34027, - "low": 118, - "open": 118, - "volume": 102248 - }, - "id": 2741985, - "non_hive": { - "close": 5001, - "high": 5000, - "low": 17, - "open": 17, - "volume": 15019 - }, - "open": "2020-10-08T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18509, - "high": 18509, - "low": 18509, - "open": 18509, - "volume": 18509 - }, - "id": 2741998, - "non_hive": { - "close": 2719, - "high": 2719, - "low": 2719, - "open": 2719, - "volume": 2719 - }, - "open": "2020-10-08T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2873, - "high": 2873, - "low": 88419, - "open": 88419, - "volume": 91292 - }, - "id": 2742002, - "non_hive": { - "close": 422, - "high": 422, - "low": 12987, - "open": 12987, - "volume": 13409 - }, - "open": "2020-10-08T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1752, - "high": 194582, - "low": 1752, - "open": 8867, - "volume": 205201 - }, - "id": 2742009, - "non_hive": { - "close": 253, - "high": 28576, - "low": 253, - "open": 1302, - "volume": 30131 - }, - "open": "2020-10-08T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 104, - "high": 104, - "low": 104, - "open": 104, - "volume": 104 - }, - "id": 2742016, - "non_hive": { - "close": 15, - "high": 15, - "low": 15, - "open": 15, - "volume": 15 - }, - "open": "2020-10-08T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10399, - "high": 10399, - "low": 140, - "open": 1856, - "volume": 47734 - }, - "id": 2742020, - "non_hive": { - "close": 1528, - "high": 1528, - "low": 20, - "open": 272, - "volume": 7003 - }, - "open": "2020-10-08T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28, - "high": 9502, - "low": 28, - "open": 9502, - "volume": 23514 - }, - "id": 2742033, - "non_hive": { - "close": 4, - "high": 1396, - "low": 4, - "open": 1396, - "volume": 3454 - }, - "open": "2020-10-08T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3029, - "high": 850, - "low": 117, - "open": 266, - "volume": 944841 - }, - "id": 2742049, - "non_hive": { - "close": 445, - "high": 125, - "low": 16, - "open": 39, - "volume": 138449 - }, - "open": "2020-10-08T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35486, - "high": 13, - "low": 1225, - "open": 303786, - "volume": 921780 - }, - "id": 2742124, - "non_hive": { - "close": 5215, - "high": 2, - "low": 179, - "open": 44618, - "volume": 135390 - }, - "open": "2020-10-08T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 856, - "high": 898, - "low": 1204, - "open": 34023, - "volume": 7648913 - }, - "id": 2742184, - "non_hive": { - "close": 125, - "high": 133, - "low": 169, - "open": 5000, - "volume": 1093213 - }, - "open": "2020-10-08T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 345995, - "high": 13, - "low": 345995, - "open": 13719, - "volume": 663579 - }, - "id": 2742275, - "non_hive": { - "close": 50000, - "high": 2, - "low": 50000, - "open": 1999, - "volume": 96194 - }, - "open": "2020-10-08T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 118, - "high": 89, - "low": 712, - "open": 13851, - "volume": 5654332 - }, - "id": 2742324, - "non_hive": { - "close": 17, - "high": 13, - "low": 100, - "open": 2000, - "volume": 807212 - }, - "open": "2020-10-08T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2999464, - "high": 68, - "low": 1138, - "open": 13887, - "volume": 19779011 - }, - "id": 2742393, - "non_hive": { - "close": 426002, - "high": 10, - "low": 160, - "open": 2000, - "volume": 2827393 - }, - "open": "2020-10-08T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13750, - "high": 19137, - "low": 22, - "open": 13580, - "volume": 25319697 - }, - "id": 2742461, - "non_hive": { - "close": 1999, - "high": 2784, - "low": 3, - "open": 1928, - "volume": 3625121 - }, - "open": "2020-10-08T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 784, - "high": 307901, - "low": 448, - "open": 5564, - "volume": 4678377 - }, - "id": 2742527, - "non_hive": { - "close": 114, - "high": 44792, - "low": 63, - "open": 809, - "volume": 668414 - }, - "open": "2020-10-09T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8241, - "high": 116, - "low": 14, - "open": 14, - "volume": 103346 - }, - "id": 2742582, - "non_hive": { - "close": 1199, - "high": 17, - "low": 2, - "open": 2, - "volume": 15021 - }, - "open": "2020-10-09T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25433, - "high": 6853, - "low": 1000, - "open": 1000, - "volume": 183063 - }, - "id": 2742610, - "non_hive": { - "close": 3700, - "high": 997, - "low": 145, - "open": 145, - "volume": 26631 - }, - "open": "2020-10-09T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6878, - "high": 13, - "low": 6878, - "open": 13, - "volume": 166974 - }, - "id": 2742625, - "non_hive": { - "close": 1000, - "high": 2, - "low": 1000, - "open": 2, - "volume": 24290 - }, - "open": "2020-10-09T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2993590, - "high": 18394, - "low": 389, - "open": 2249, - "volume": 37808249 - }, - "id": 2742640, - "non_hive": { - "close": 425030, - "high": 2676, - "low": 54, - "open": 326, - "volume": 5370441 - }, - "open": "2020-10-09T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 85, - "open": 6410, - "volume": 763652 - }, - "id": 2742706, - "non_hive": { - "close": 145, - "high": 145, - "low": 12, - "open": 910, - "volume": 109121 - }, - "open": "2020-10-09T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4950, - "high": 253273, - "low": 6776, - "open": 855, - "volume": 4829715 - }, - "id": 2742746, - "non_hive": { - "close": 717, - "high": 36845, - "low": 968, - "open": 124, - "volume": 691864 - }, - "open": "2020-10-09T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 106558, - "high": 440, - "low": 14283, - "open": 13986, - "volume": 9027586 - }, - "id": 2742786, - "non_hive": { - "close": 14921, - "high": 63, - "low": 1999, - "open": 2000, - "volume": 1280288 - }, - "open": "2020-10-09T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6620, - "high": 6983, - "low": 419, - "open": 14000, - "volume": 4653393 - }, - "id": 2742822, - "non_hive": { - "close": 920, - "high": 1001, - "low": 58, - "open": 1959, - "volume": 654503 - }, - "open": "2020-10-09T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21151, - "high": 21150, - "low": 178, - "open": 14184, - "volume": 29175361 - }, - "id": 2742872, - "non_hive": { - "close": 3001, - "high": 3001, - "low": 24, - "open": 2000, - "volume": 4041985 - }, - "open": "2020-10-09T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8186, - "high": 826, - "low": 12, - "open": 14096, - "volume": 19004799 - }, - "id": 2742950, - "non_hive": { - "close": 1145, - "high": 119, - "low": 1, - "open": 1999, - "volume": 2638128 - }, - "open": "2020-10-09T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29281, - "high": 8411, - "low": 19, - "open": 6107, - "volume": 6146480 - }, - "id": 2743019, - "non_hive": { - "close": 4140, - "high": 1219, - "low": 2, - "open": 854, - "volume": 853427 - }, - "open": "2020-10-09T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13987, - "high": 503, - "low": 200115, - "open": 5382, - "volume": 9265794 - }, - "id": 2743084, - "non_hive": { - "close": 1999, - "high": 73, - "low": 27616, - "open": 780, - "volume": 1303834 - }, - "open": "2020-10-09T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1105, - "high": 6, - "low": 44, - "open": 8867, - "volume": 3940820 - }, - "id": 2743146, - "non_hive": { - "close": 158, - "high": 1, - "low": 6, - "open": 1268, - "volume": 565681 - }, - "open": "2020-10-09T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3388114, - "high": 3388114, - "low": 2653209, - "open": 13847, - "volume": 7518020 - }, - "id": 2743196, - "non_hive": { - "close": 492886, - "high": 492886, - "low": 381611, - "open": 2000, - "volume": 1088153 - }, - "open": "2020-10-09T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21101, - "high": 21101, - "low": 13799, - "open": 2392, - "volume": 1472368 - }, - "id": 2743241, - "non_hive": { - "close": 3122, - "high": 3122, - "low": 1997, - "open": 348, - "volume": 213898 - }, - "open": "2020-10-09T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35495, - "high": 35495, - "low": 28, - "open": 8196, - "volume": 3502825 - }, - "id": 2743273, - "non_hive": { - "close": 5309, - "high": 5309, - "low": 4, - "open": 1213, - "volume": 515138 - }, - "open": "2020-10-09T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1041376, - "high": 153, - "low": 13748, - "open": 15732, - "volume": 7052040 - }, - "id": 2743331, - "non_hive": { - "close": 152988, - "high": 23, - "low": 1999, - "open": 2353, - "volume": 1039903 - }, - "open": "2020-10-09T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13369, - "high": 735, - "low": 33236, - "open": 674152, - "volume": 2049537 - }, - "id": 2743369, - "non_hive": { - "close": 1999, - "high": 110, - "low": 4863, - "open": 98986, - "volume": 301783 - }, - "open": "2020-10-09T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5184, - "high": 33429, - "low": 6642, - "open": 12519, - "volume": 5549347 - }, - "id": 2743414, - "non_hive": { - "close": 775, - "high": 5001, - "low": 956, - "open": 1872, - "volume": 822551 - }, - "open": "2020-10-09T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4427, - "high": 100000, - "low": 197, - "open": 6774, - "volume": 17794945 - }, - "id": 2743444, - "non_hive": { - "close": 653, - "high": 14840, - "low": 27, - "open": 988, - "volume": 2554201 - }, - "open": "2020-10-09T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1868, - "high": 1868, - "low": 1415, - "open": 266505, - "volume": 16239548 - }, - "id": 2743526, - "non_hive": { - "close": 279, - "high": 279, - "low": 197, - "open": 39352, - "volume": 2337434 - }, - "open": "2020-10-09T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2937, - "high": 381, - "low": 929, - "open": 7019, - "volume": 13446275 - }, - "id": 2743602, - "non_hive": { - "close": 414, - "high": 57, - "low": 130, - "open": 1048, - "volume": 1944038 - }, - "open": "2020-10-09T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1187021, - "high": 1000, - "low": 14186, - "open": 10000, - "volume": 17703469 - }, - "id": 2743657, - "non_hive": { - "close": 182892, - "high": 160, - "low": 1999, - "open": 1495, - "volume": 2684846 - }, - "open": "2020-10-09T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41602, - "high": 270, - "low": 13896, - "open": 499689, - "volume": 9175857 - }, - "id": 2743712, - "non_hive": { - "close": 6490, - "high": 43, - "low": 1999, - "open": 77000, - "volume": 1388851 - }, - "open": "2020-10-10T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 680846, - "high": 18890, - "low": 13895, - "open": 13881, - "volume": 17372786 - }, - "id": 2743766, - "non_hive": { - "close": 98001, - "high": 3000, - "low": 1999, - "open": 1999, - "volume": 2589172 - }, - "open": "2020-10-10T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14000, - "high": 409, - "low": 13895, - "open": 13895, - "volume": 6275384 - }, - "id": 2743825, - "non_hive": { - "close": 2224, - "high": 65, - "low": 1999, - "open": 1999, - "volume": 950119 - }, - "open": "2020-10-10T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2388, - "high": 251, - "low": 922046, - "open": 9831, - "volume": 2553031 - }, - "id": 2743873, - "non_hive": { - "close": 374, - "high": 40, - "low": 144392, - "open": 1562, - "volume": 400906 - }, - "open": "2020-10-10T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6096, - "high": 8300, - "low": 39, - "open": 10382, - "volume": 3978991 - }, - "id": 2743904, - "non_hive": { - "close": 968, - "high": 1318, - "low": 6, - "open": 1626, - "volume": 620664 - }, - "open": "2020-10-10T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 529, - "high": 8446, - "low": 279, - "open": 6884, - "volume": 4204839 - }, - "id": 2743931, - "non_hive": { - "close": 82, - "high": 1342, - "low": 42, - "open": 1093, - "volume": 655299 - }, - "open": "2020-10-10T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16623, - "high": 6298, - "low": 126749, - "open": 245, - "volume": 2597034 - }, - "id": 2743965, - "non_hive": { - "close": 2636, - "high": 999, - "low": 19430, - "open": 38, - "volume": 398709 - }, - "open": "2020-10-10T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 86956, - "high": 1127, - "low": 312, - "open": 6521, - "volume": 366861 - }, - "id": 2743991, - "non_hive": { - "close": 13332, - "high": 179, - "low": 47, - "open": 1033, - "volume": 57142 - }, - "open": "2020-10-10T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 601, - "high": 34747, - "low": 601, - "open": 13158, - "volume": 4890679 - }, - "id": 2744021, - "non_hive": { - "close": 90, - "high": 5515, - "low": 90, - "open": 1999, - "volume": 748129 - }, - "open": "2020-10-10T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 551195, - "high": 1240, - "low": 13062, - "open": 13062, - "volume": 2176327 - }, - "id": 2744068, - "non_hive": { - "close": 84942, - "high": 197, - "low": 2000, - "open": 2000, - "volume": 338482 - }, - "open": "2020-10-10T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35008, - "high": 90540, - "low": 35008, - "open": 6309, - "volume": 3028090 - }, - "id": 2744120, - "non_hive": { - "close": 4986, - "high": 14375, - "low": 4986, - "open": 1001, - "volume": 451969 - }, - "open": "2020-10-10T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7019, - "high": 5228, - "low": 6812, - "open": 6812, - "volume": 97663 - }, - "id": 2744165, - "non_hive": { - "close": 1074, - "high": 810, - "low": 1035, - "open": 1035, - "volume": 15068 - }, - "open": "2020-10-10T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 666, - "high": 6442, - "low": 5896, - "open": 189, - "volume": 465493 - }, - "id": 2744194, - "non_hive": { - "close": 102, - "high": 998, - "low": 855, - "open": 29, - "volume": 70922 - }, - "open": "2020-10-10T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 412713, - "high": 412713, - "low": 13334, - "open": 13334, - "volume": 882293 - }, - "id": 2744226, - "non_hive": { - "close": 63942, - "high": 63942, - "low": 1999, - "open": 1999, - "volume": 135762 - }, - "open": "2020-10-10T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4196, - "high": 107, - "low": 48709, - "open": 99700, - "volume": 1121251 - }, - "id": 2744270, - "non_hive": { - "close": 658, - "high": 17, - "low": 7455, - "open": 15449, - "volume": 176761 - }, - "open": "2020-10-10T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12943, - "high": 69, - "low": 337468, - "open": 69, - "volume": 909373 - }, - "id": 2744333, - "non_hive": { - "close": 2000, - "high": 11, - "low": 52138, - "open": 11, - "volume": 141250 - }, - "open": "2020-10-10T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54788, - "high": 38, - "low": 13, - "open": 12682, - "volume": 171024 - }, - "id": 2744374, - "non_hive": { - "close": 8630, - "high": 6, - "low": 2, - "open": 1999, - "volume": 26861 - }, - "open": "2020-10-10T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6206, - "high": 6206, - "low": 8001, - "open": 10000, - "volume": 1140870 - }, - "id": 2744413, - "non_hive": { - "close": 993, - "high": 993, - "low": 1237, - "open": 1575, - "volume": 181086 - }, - "open": "2020-10-10T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15626, - "high": 868, - "low": 47984, - "open": 1700, - "volume": 2352743 - }, - "id": 2744436, - "non_hive": { - "close": 2499, - "high": 139, - "low": 7491, - "open": 272, - "volume": 372855 - }, - "open": "2020-10-10T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8148, - "high": 106, - "low": 299, - "open": 1813, - "volume": 342522 - }, - "id": 2744473, - "non_hive": { - "close": 1303, - "high": 17, - "low": 47, - "open": 290, - "volume": 54757 - }, - "open": "2020-10-10T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2087, - "high": 12664, - "low": 6718, - "open": 2384, - "volume": 3131739 - }, - "id": 2744509, - "non_hive": { - "close": 339, - "high": 2058, - "low": 1044, - "open": 375, - "volume": 499581 - }, - "open": "2020-10-10T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1163, - "high": 1163, - "low": 4255, - "open": 4255, - "volume": 17596 - }, - "id": 2744562, - "non_hive": { - "close": 189, - "high": 189, - "low": 689, - "open": 689, - "volume": 2855 - }, - "open": "2020-10-10T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 196, - "high": 196, - "low": 10243, - "open": 8043, - "volume": 569778 - }, - "id": 2744572, - "non_hive": { - "close": 32, - "high": 32, - "low": 1651, - "open": 1307, - "volume": 92404 - }, - "open": "2020-10-10T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3878, - "high": 6, - "low": 19, - "open": 55187, - "volume": 378730 - }, - "id": 2744617, - "non_hive": { - "close": 628, - "high": 1, - "low": 3, - "open": 8968, - "volume": 61458 - }, - "open": "2020-10-10T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9595, - "high": 6, - "low": 289237, - "open": 5170, - "volume": 762918 - }, - "id": 2744655, - "non_hive": { - "close": 1558, - "high": 1, - "low": 44849, - "open": 837, - "volume": 120825 - }, - "open": "2020-10-11T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11790, - "high": 6, - "low": 13, - "open": 12330, - "volume": 102588 - }, - "id": 2744711, - "non_hive": { - "close": 1909, - "high": 1, - "low": 2, - "open": 1999, - "volume": 16614 - }, - "open": "2020-10-11T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20392, - "high": 6, - "low": 1196, - "open": 556, - "volume": 475414 - }, - "id": 2744746, - "non_hive": { - "close": 3309, - "high": 1, - "low": 193, - "open": 90, - "volume": 77026 - }, - "open": "2020-10-11T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1015, - "high": 1015, - "low": 98000, - "open": 11107, - "volume": 142881 - }, - "id": 2744789, - "non_hive": { - "close": 165, - "high": 165, - "low": 15898, - "open": 1805, - "volume": 23188 - }, - "open": "2020-10-11T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6499, - "high": 9673, - "low": 1054, - "open": 35495, - "volume": 145151 - }, - "id": 2744807, - "non_hive": { - "close": 1056, - "high": 1572, - "low": 171, - "open": 5768, - "volume": 23587 - }, - "open": "2020-10-11T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 209, - "high": 209, - "low": 43346, - "open": 1230, - "volume": 71778 - }, - "id": 2744830, - "non_hive": { - "close": 34, - "high": 34, - "low": 7043, - "open": 200, - "volume": 11663 - }, - "open": "2020-10-11T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 80895, - "high": 36, - "low": 8455, - "open": 726, - "volume": 317671 - }, - "id": 2744846, - "non_hive": { - "close": 12942, - "high": 6, - "low": 1337, - "open": 118, - "volume": 50852 - }, - "open": "2020-10-11T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39027, - "high": 6, - "low": 136030, - "open": 12642, - "volume": 484761 - }, - "id": 2744871, - "non_hive": { - "close": 6338, - "high": 1, - "low": 21369, - "open": 2000, - "volume": 77792 - }, - "open": "2020-10-11T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 634, - "high": 634, - "low": 12404, - "open": 53197, - "volume": 316858 - }, - "id": 2744905, - "non_hive": { - "close": 103, - "high": 103, - "low": 2000, - "open": 8618, - "volume": 51421 - }, - "open": "2020-10-11T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16468, - "high": 15003, - "low": 5300, - "open": 2678, - "volume": 128645 - }, - "id": 2744936, - "non_hive": { - "close": 2676, - "high": 2438, - "low": 829, - "open": 435, - "volume": 20861 - }, - "open": "2020-10-11T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 123, - "high": 861, - "low": 7502, - "open": 5300, - "volume": 65552 - }, - "id": 2744967, - "non_hive": { - "close": 20, - "high": 140, - "low": 1200, - "open": 861, - "volume": 10601 - }, - "open": "2020-10-11T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9453, - "high": 424, - "low": 9453, - "open": 424, - "volume": 9877 - }, - "id": 2744983, - "non_hive": { - "close": 1535, - "high": 69, - "low": 1535, - "open": 69, - "volume": 1604 - }, - "open": "2020-10-11T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 203, - "high": 203, - "low": 9873, - "open": 5649, - "volume": 479088 - }, - "id": 2744990, - "non_hive": { - "close": 33, - "high": 33, - "low": 1579, - "open": 918, - "volume": 76959 - }, - "open": "2020-10-11T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12221, - "high": 1267, - "low": 10000, - "open": 12105, - "volume": 871613 - }, - "id": 2745007, - "non_hive": { - "close": 1986, - "high": 206, - "low": 1600, - "open": 1966, - "volume": 141609 - }, - "open": "2020-10-11T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13175, - "high": 473, - "low": 7920, - "open": 12603, - "volume": 109351 - }, - "id": 2745042, - "non_hive": { - "close": 2141, - "high": 77, - "low": 1287, - "open": 2048, - "volume": 17770 - }, - "open": "2020-10-11T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6926, - "high": 6926, - "low": 37, - "open": 4098, - "volume": 119845 - }, - "id": 2745074, - "non_hive": { - "close": 1128, - "high": 1128, - "low": 6, - "open": 666, - "volume": 19478 - }, - "open": "2020-10-11T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12086, - "high": 169, - "low": 589318, - "open": 18271, - "volume": 1444320 - }, - "id": 2745104, - "non_hive": { - "close": 1990, - "high": 28, - "low": 92228, - "open": 2975, - "volume": 229978 - }, - "open": "2020-10-11T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 972, - "high": 28204, - "low": 32, - "open": 8654, - "volume": 141690 - }, - "id": 2745136, - "non_hive": { - "close": 158, - "high": 4636, - "low": 5, - "open": 1422, - "volume": 23213 - }, - "open": "2020-10-11T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12270, - "high": 936, - "low": 13, - "open": 13, - "volume": 537149 - }, - "id": 2745170, - "non_hive": { - "close": 1999, - "high": 154, - "low": 2, - "open": 2, - "volume": 87501 - }, - "open": "2020-10-11T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5145, - "high": 5145, - "low": 28437, - "open": 12160, - "volume": 1637089 - }, - "id": 2745230, - "non_hive": { - "close": 849, - "high": 849, - "low": 4449, - "open": 1999, - "volume": 263179 - }, - "open": "2020-10-11T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4002, - "high": 4002, - "low": 25, - "open": 7097, - "volume": 227844 - }, - "id": 2745260, - "non_hive": { - "close": 668, - "high": 668, - "low": 4, - "open": 1171, - "volume": 37579 - }, - "open": "2020-10-11T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9683, - "high": 137, - "low": 198, - "open": 28585, - "volume": 213646 - }, - "id": 2745299, - "non_hive": { - "close": 1597, - "high": 23, - "low": 32, - "open": 4768, - "volume": 35253 - }, - "open": "2020-10-11T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8119, - "high": 114, - "low": 27, - "open": 2845, - "volume": 362027 - }, - "id": 2745331, - "non_hive": { - "close": 1345, - "high": 19, - "low": 4, - "open": 472, - "volume": 59783 - }, - "open": "2020-10-11T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22248, - "high": 22248, - "low": 25, - "open": 50825, - "volume": 861144 - }, - "id": 2745367, - "non_hive": { - "close": 3711, - "high": 3711, - "low": 4, - "open": 8386, - "volume": 142079 - }, - "open": "2020-10-11T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4415, - "high": 4415, - "low": 6134, - "open": 12257, - "volume": 235385 - }, - "id": 2745396, - "non_hive": { - "close": 728, - "high": 728, - "low": 994, - "open": 2000, - "volume": 38382 - }, - "open": "2020-10-12T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6384, - "high": 6384, - "low": 13, - "open": 13, - "volume": 76591 - }, - "id": 2745413, - "non_hive": { - "close": 1053, - "high": 1053, - "low": 2, - "open": 2, - "volume": 12628 - }, - "open": "2020-10-12T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55229, - "high": 8705, - "low": 2000, - "open": 11129, - "volume": 159133 - }, - "id": 2745435, - "non_hive": { - "close": 9109, - "high": 1436, - "low": 324, - "open": 1835, - "volume": 26131 - }, - "open": "2020-10-12T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3745, - "high": 5440, - "low": 238816, - "open": 2427, - "volume": 394141 - }, - "id": 2745462, - "non_hive": { - "close": 617, - "high": 897, - "low": 39012, - "open": 400, - "volume": 64536 - }, - "open": "2020-10-12T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18389, - "high": 18389, - "low": 120927, - "open": 12142, - "volume": 1914506 - }, - "id": 2745488, - "non_hive": { - "close": 3029, - "high": 3029, - "low": 19348, - "open": 1999, - "volume": 309028 - }, - "open": "2020-10-12T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1056, - "high": 159351, - "low": 82, - "open": 12272, - "volume": 214031 - }, - "id": 2745500, - "non_hive": { - "close": 172, - "high": 25971, - "low": 13, - "open": 1999, - "volume": 34853 - }, - "open": "2020-10-12T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4695, - "high": 512, - "low": 69, - "open": 11025, - "volume": 105717 - }, - "id": 2745523, - "non_hive": { - "close": 770, - "high": 84, - "low": 11, - "open": 1797, - "volume": 17268 - }, - "open": "2020-10-12T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12262, - "high": 1588, - "low": 12262, - "open": 10743, - "volume": 1093084 - }, - "id": 2745549, - "non_hive": { - "close": 1999, - "high": 262, - "low": 1999, - "open": 1762, - "volume": 178833 - }, - "open": "2020-10-12T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 113196, - "high": 3682, - "low": 1097493, - "open": 10749, - "volume": 2669497 - }, - "id": 2745601, - "non_hive": { - "close": 18223, - "high": 604, - "low": 174378, - "open": 1762, - "volume": 430001 - }, - "open": "2020-10-12T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9028, - "high": 1934, - "low": 12204, - "open": 12204, - "volume": 157033 - }, - "id": 2745654, - "non_hive": { - "close": 1489, - "high": 319, - "low": 1999, - "open": 1999, - "volume": 25857 - }, - "open": "2020-10-12T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3559, - "high": 6190, - "low": 561, - "open": 1468, - "volume": 89047 - }, - "id": 2745674, - "non_hive": { - "close": 587, - "high": 1021, - "low": 89, - "open": 242, - "volume": 14657 - }, - "open": "2020-10-12T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28303, - "high": 9004, - "low": 1901, - "open": 14910, - "volume": 139127 - }, - "id": 2745696, - "non_hive": { - "close": 4641, - "high": 1485, - "low": 308, - "open": 2459, - "volume": 22899 - }, - "open": "2020-10-12T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42623, - "high": 4634, - "low": 42623, - "open": 13630, - "volume": 155076 - }, - "id": 2745719, - "non_hive": { - "close": 6975, - "high": 760, - "low": 6975, - "open": 2235, - "volume": 25398 - }, - "open": "2020-10-12T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2001, - "high": 30, - "low": 2001, - "open": 6343, - "volume": 163828 - }, - "id": 2745750, - "non_hive": { - "close": 322, - "high": 5, - "low": 322, - "open": 1038, - "volume": 26802 - }, - "open": "2020-10-12T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1092352, - "high": 4462, - "low": 1092352, - "open": 1473, - "volume": 3068031 - }, - "id": 2745788, - "non_hive": { - "close": 173561, - "high": 736, - "low": 173561, - "open": 240, - "volume": 495907 - }, - "open": "2020-10-12T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1936122, - "high": 66, - "low": 303, - "open": 12130, - "volume": 3508021 - }, - "id": 2745860, - "non_hive": { - "close": 319460, - "high": 11, - "low": 48, - "open": 1999, - "volume": 578686 - }, - "open": "2020-10-12T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20852, - "high": 9076, - "low": 564, - "open": 11602, - "volume": 3474989 - }, - "id": 2745895, - "non_hive": { - "close": 3413, - "high": 1514, - "low": 90, - "open": 1925, - "volume": 567294 - }, - "open": "2020-10-12T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 97, - "high": 315, - "low": 32, - "open": 427, - "volume": 1685897 - }, - "id": 2745968, - "non_hive": { - "close": 16, - "high": 52, - "low": 5, - "open": 70, - "volume": 271291 - }, - "open": "2020-10-12T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6711, - "high": 188, - "low": 13, - "open": 13034, - "volume": 134115 - }, - "id": 2746017, - "non_hive": { - "close": 1105, - "high": 31, - "low": 2, - "open": 2146, - "volume": 22082 - }, - "open": "2020-10-12T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 80089, - "high": 40032, - "low": 12194, - "open": 28854, - "volume": 623587 - }, - "id": 2746043, - "non_hive": { - "close": 13235, - "high": 6672, - "low": 1999, - "open": 4751, - "volume": 103045 - }, - "open": "2020-10-12T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18, - "high": 11990, - "low": 49322, - "open": 756, - "volume": 2077338 - }, - "id": 2746103, - "non_hive": { - "close": 3, - "high": 1999, - "low": 7847, - "open": 125, - "volume": 336963 - }, - "open": "2020-10-12T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2026, - "high": 2026, - "low": 2762, - "open": 10053, - "volume": 119004 - }, - "id": 2746149, - "non_hive": { - "close": 338, - "high": 338, - "low": 460, - "open": 1675, - "volume": 19841 - }, - "open": "2020-10-12T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22537, - "high": 10468, - "low": 13, - "open": 10468, - "volume": 126372 - }, - "id": 2746180, - "non_hive": { - "close": 3759, - "high": 1746, - "low": 2, - "open": 1746, - "volume": 21052 - }, - "open": "2020-10-12T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12198, - "high": 9688, - "low": 410841, - "open": 28556, - "volume": 3245518 - }, - "id": 2746206, - "non_hive": { - "close": 2000, - "high": 1616, - "low": 63386, - "open": 4763, - "volume": 517046 - }, - "open": "2020-10-12T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49, - "high": 1353, - "low": 174, - "open": 445, - "volume": 143044 - }, - "id": 2746257, - "non_hive": { - "close": 8, - "high": 225, - "low": 28, - "open": 73, - "volume": 23617 - }, - "open": "2020-10-13T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17153, - "high": 6725, - "low": 13, - "open": 13, - "volume": 1303419 - }, - "id": 2746289, - "non_hive": { - "close": 2712, - "high": 1098, - "low": 2, - "open": 2, - "volume": 206715 - }, - "open": "2020-10-13T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1807, - "high": 1807, - "low": 3357, - "open": 9812, - "volume": 94914 - }, - "id": 2746329, - "non_hive": { - "close": 294, - "high": 294, - "low": 527, - "open": 1551, - "volume": 15065 - }, - "open": "2020-10-13T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36394, - "high": 1219, - "low": 12351, - "open": 12351, - "volume": 108284 - }, - "id": 2746339, - "non_hive": { - "close": 5994, - "high": 201, - "low": 1999, - "open": 1999, - "volume": 17699 - }, - "open": "2020-10-13T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 119, - "high": 1154, - "low": 119, - "open": 1209, - "volume": 90612 - }, - "id": 2746357, - "non_hive": { - "close": 19, - "high": 190, - "low": 19, - "open": 199, - "volume": 14907 - }, - "open": "2020-10-13T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8002, - "high": 30, - "low": 80, - "open": 960, - "volume": 152938 - }, - "id": 2746377, - "non_hive": { - "close": 1315, - "high": 5, - "low": 12, - "open": 158, - "volume": 25149 - }, - "open": "2020-10-13T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4396, - "high": 48, - "low": 61, - "open": 3000, - "volume": 396835 - }, - "id": 2746402, - "non_hive": { - "close": 720, - "high": 8, - "low": 9, - "open": 493, - "volume": 64579 - }, - "open": "2020-10-13T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 66665, - "high": 12212, - "low": 11, - "open": 3515, - "volume": 122886 - }, - "id": 2746462, - "non_hive": { - "close": 10900, - "high": 1999, - "low": 1, - "open": 575, - "volume": 20097 - }, - "open": "2020-10-13T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29548, - "high": 128, - "low": 9, - "open": 110, - "volume": 102281 - }, - "id": 2746486, - "non_hive": { - "close": 4819, - "high": 21, - "low": 1, - "open": 18, - "volume": 16690 - }, - "open": "2020-10-13T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23350, - "high": 2974, - "low": 23350, - "open": 13296, - "volume": 529855 - }, - "id": 2746515, - "non_hive": { - "close": 3722, - "high": 486, - "low": 3722, - "open": 2167, - "volume": 85448 - }, - "open": "2020-10-13T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 244739, - "high": 544, - "low": 174, - "open": 174, - "volume": 317932 - }, - "id": 2746536, - "non_hive": { - "close": 40000, - "high": 89, - "low": 28, - "open": 28, - "volume": 51962 - }, - "open": "2020-10-13T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5510, - "high": 1454484, - "low": 23546, - "open": 6425, - "volume": 5678054 - }, - "id": 2746560, - "non_hive": { - "close": 871, - "high": 237721, - "low": 3698, - "open": 1050, - "volume": 911877 - }, - "open": "2020-10-13T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13474, - "high": 930, - "low": 13474, - "open": 6327, - "volume": 85848 - }, - "id": 2746607, - "non_hive": { - "close": 2129, - "high": 147, - "low": 2129, - "open": 1000, - "volume": 13568 - }, - "open": "2020-10-13T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2441, - "high": 17502, - "low": 43280, - "open": 62, - "volume": 1376908 - }, - "id": 2746626, - "non_hive": { - "close": 397, - "high": 2861, - "low": 6708, - "open": 10, - "volume": 217238 - }, - "open": "2020-10-13T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11761, - "high": 18, - "low": 533, - "open": 18, - "volume": 157660 - }, - "id": 2746648, - "non_hive": { - "close": 1841, - "high": 3, - "low": 83, - "open": 3, - "volume": 25254 - }, - "open": "2020-10-13T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3481, - "high": 381, - "low": 12414, - "open": 5674, - "volume": 373308 - }, - "id": 2746674, - "non_hive": { - "close": 566, - "high": 62, - "low": 1999, - "open": 921, - "volume": 60411 - }, - "open": "2020-10-13T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24115, - "high": 3241, - "low": 256803, - "open": 1796, - "volume": 1270967 - }, - "id": 2746709, - "non_hive": { - "close": 3893, - "high": 527, - "low": 39147, - "open": 292, - "volume": 199715 - }, - "open": "2020-10-13T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8244, - "high": 246, - "low": 101, - "open": 104, - "volume": 167259 - }, - "id": 2746731, - "non_hive": { - "close": 1339, - "high": 40, - "low": 15, - "open": 16, - "volume": 27056 - }, - "open": "2020-10-13T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 96859, - "high": 12, - "low": 33, - "open": 68885, - "volume": 2005787 - }, - "id": 2746780, - "non_hive": { - "close": 15045, - "high": 2, - "low": 5, - "open": 11189, - "volume": 319963 - }, - "open": "2020-10-13T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7370, - "high": 860, - "low": 211259, - "open": 5362, - "volume": 1372992 - }, - "id": 2746852, - "non_hive": { - "close": 1156, - "high": 135, - "low": 31688, - "open": 833, - "volume": 209422 - }, - "open": "2020-10-13T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11383, - "high": 9474, - "low": 12661, - "open": 4515, - "volume": 1027081 - }, - "id": 2746886, - "non_hive": { - "close": 1752, - "high": 1486, - "low": 1899, - "open": 708, - "volume": 155854 - }, - "open": "2020-10-13T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47290, - "high": 650006, - "low": 13158, - "open": 3000, - "volume": 5923606 - }, - "id": 2746931, - "non_hive": { - "close": 7566, - "high": 105574, - "low": 1999, - "open": 468, - "volume": 922132 - }, - "open": "2020-10-13T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20082, - "high": 12, - "low": 6740, - "open": 4440, - "volume": 283335 - }, - "id": 2746993, - "non_hive": { - "close": 3211, - "high": 2, - "low": 1022, - "open": 710, - "volume": 43595 - }, - "open": "2020-10-13T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 453, - "high": 12, - "low": 23708, - "open": 645387, - "volume": 5552966 - }, - "id": 2747027, - "non_hive": { - "close": 73, - "high": 2, - "low": 3557, - "open": 103160, - "volume": 870397 - }, - "open": "2020-10-13T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1088, - "high": 201, - "low": 448, - "open": 448, - "volume": 48045 - }, - "id": 2747115, - "non_hive": { - "close": 172, - "high": 32, - "low": 68, - "open": 68, - "volume": 7442 - }, - "open": "2020-10-14T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53, - "high": 1151, - "low": 14, - "open": 14, - "volume": 28170 - }, - "id": 2747133, - "non_hive": { - "close": 8, - "high": 183, - "low": 2, - "open": 2, - "volume": 4453 - }, - "open": "2020-10-14T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 692, - "high": 616, - "low": 12, - "open": 6006, - "volume": 30726 - }, - "id": 2747154, - "non_hive": { - "close": 110, - "high": 98, - "low": 1, - "open": 954, - "volume": 4879 - }, - "open": "2020-10-14T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28362, - "high": 807, - "low": 445, - "open": 165, - "volume": 207291 - }, - "id": 2747171, - "non_hive": { - "close": 4538, - "high": 130, - "low": 70, - "open": 26, - "volume": 32894 - }, - "open": "2020-10-14T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7638, - "high": 8141, - "low": 41207, - "open": 41652, - "volume": 163169 - }, - "id": 2747190, - "non_hive": { - "close": 1228, - "high": 1309, - "low": 6468, - "open": 6538, - "volume": 25765 - }, - "open": "2020-10-14T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 561, - "high": 93, - "low": 561, - "open": 653, - "volume": 41962 - }, - "id": 2747210, - "non_hive": { - "close": 88, - "high": 15, - "low": 88, - "open": 105, - "volume": 6743 - }, - "open": "2020-10-14T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5325, - "high": 1965, - "low": 14, - "open": 1965, - "volume": 71553 - }, - "id": 2747223, - "non_hive": { - "close": 856, - "high": 316, - "low": 2, - "open": 316, - "volume": 11499 - }, - "open": "2020-10-14T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1207, - "high": 161, - "low": 45, - "open": 3124, - "volume": 53729 - }, - "id": 2747242, - "non_hive": { - "close": 194, - "high": 26, - "low": 7, - "open": 502, - "volume": 8632 - }, - "open": "2020-10-14T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13157, - "high": 348, - "low": 3941, - "open": 10924, - "volume": 343367 - }, - "id": 2747269, - "non_hive": { - "close": 2000, - "high": 56, - "low": 594, - "open": 1755, - "volume": 53897 - }, - "open": "2020-10-14T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9358, - "high": 3347, - "low": 9132, - "open": 9132, - "volume": 97143 - }, - "id": 2747295, - "non_hive": { - "close": 1487, - "high": 535, - "low": 1388, - "open": 1388, - "volume": 15431 - }, - "open": "2020-10-14T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5143, - "high": 5143, - "low": 190023, - "open": 12585, - "volume": 348976 - }, - "id": 2747318, - "non_hive": { - "close": 817, - "high": 817, - "low": 28899, - "open": 1999, - "volume": 53926 - }, - "open": "2020-10-14T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 138, - "high": 138, - "low": 219, - "open": 219, - "volume": 145158 - }, - "id": 2747333, - "non_hive": { - "close": 22, - "high": 22, - "low": 34, - "open": 34, - "volume": 23060 - }, - "open": "2020-10-14T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1765, - "high": 200, - "low": 3882, - "open": 7130, - "volume": 159424 - }, - "id": 2747344, - "non_hive": { - "close": 274, - "high": 32, - "low": 602, - "open": 1138, - "volume": 25071 - }, - "open": "2020-10-14T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11067, - "high": 225, - "low": 78, - "open": 9830, - "volume": 232472 - }, - "id": 2747385, - "non_hive": { - "close": 1766, - "high": 36, - "low": 12, - "open": 1526, - "volume": 36986 - }, - "open": "2020-10-14T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12150, - "high": 325, - "low": 2085, - "open": 12901, - "volume": 115252 - }, - "id": 2747429, - "non_hive": { - "close": 1939, - "high": 52, - "low": 332, - "open": 2059, - "volume": 18392 - }, - "open": "2020-10-14T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12650, - "high": 50, - "low": 12, - "open": 13085, - "volume": 185125 - }, - "id": 2747471, - "non_hive": { - "close": 2019, - "high": 8, - "low": 1, - "open": 2088, - "volume": 29543 - }, - "open": "2020-10-14T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5078, - "high": 4335, - "low": 7000, - "open": 3552, - "volume": 69006 - }, - "id": 2747504, - "non_hive": { - "close": 807, - "high": 692, - "low": 1092, - "open": 567, - "volume": 10873 - }, - "open": "2020-10-14T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8, - "high": 68, - "low": 8, - "open": 5196, - "volume": 30097 - }, - "id": 2747531, - "non_hive": { - "close": 1, - "high": 11, - "low": 1, - "open": 829, - "volume": 4800 - }, - "open": "2020-10-14T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7775, - "high": 7775, - "low": 115, - "open": 3569, - "volume": 55501 - }, - "id": 2747548, - "non_hive": { - "close": 1241, - "high": 1241, - "low": 17, - "open": 567, - "volume": 8845 - }, - "open": "2020-10-14T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 495, - "high": 3746, - "low": 240, - "open": 3746, - "volume": 54794 - }, - "id": 2747569, - "non_hive": { - "close": 79, - "high": 598, - "low": 37, - "open": 598, - "volume": 8745 - }, - "open": "2020-10-14T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14352, - "high": 14352, - "low": 14352, - "open": 14352, - "volume": 14352 - }, - "id": 2747588, - "non_hive": { - "close": 2291, - "high": 2291, - "low": 2291, - "open": 2291, - "volume": 2291 - }, - "open": "2020-10-14T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50056, - "high": 50056, - "low": 50056, - "open": 50056, - "volume": 50056 - }, - "id": 2747592, - "non_hive": { - "close": 7990, - "high": 7990, - "low": 7990, - "open": 7990, - "volume": 7990 - }, - "open": "2020-10-14T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16902, - "high": 16902, - "low": 20907, - "open": 2596, - "volume": 798886 - }, - "id": 2747596, - "non_hive": { - "close": 2716, - "high": 2716, - "low": 3261, - "open": 414, - "volume": 127359 - }, - "open": "2020-10-14T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3712, - "high": 28035, - "low": 3712, - "open": 28035, - "volume": 84049 - }, - "id": 2747608, - "non_hive": { - "close": 596, - "high": 4505, - "low": 596, - "open": 4505, - "volume": 13505 - }, - "open": "2020-10-15T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11443, - "high": 908, - "low": 5870, - "open": 14854, - "volume": 141297 - }, - "id": 2747616, - "non_hive": { - "close": 1831, - "high": 146, - "low": 898, - "open": 2387, - "volume": 22407 - }, - "open": "2020-10-15T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2520, - "high": 2520, - "low": 476, - "open": 32193, - "volume": 110844 - }, - "id": 2747626, - "non_hive": { - "close": 405, - "high": 405, - "low": 76, - "open": 5150, - "volume": 17788 - }, - "open": "2020-10-15T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59039, - "high": 155, - "low": 2309, - "open": 155, - "volume": 144120 - }, - "id": 2747641, - "non_hive": { - "close": 9487, - "high": 25, - "low": 371, - "open": 25, - "volume": 23158 - }, - "open": "2020-10-15T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8358, - "high": 479, - "low": 8358, - "open": 479, - "volume": 105316 - }, - "id": 2747653, - "non_hive": { - "close": 1343, - "high": 77, - "low": 1343, - "open": 77, - "volume": 16923 - }, - "open": "2020-10-15T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1587, - "high": 336, - "low": 2512, - "open": 15441, - "volume": 79584 - }, - "id": 2747666, - "non_hive": { - "close": 255, - "high": 54, - "low": 403, - "open": 2481, - "volume": 12787 - }, - "open": "2020-10-15T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24913, - "high": 14288, - "low": 577, - "open": 13418, - "volume": 125265 - }, - "id": 2747680, - "non_hive": { - "close": 4001, - "high": 2296, - "low": 92, - "open": 2156, - "volume": 20125 - }, - "open": "2020-10-15T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 792, - "high": 10237, - "low": 792, - "open": 21838, - "volume": 42781 - }, - "id": 2747696, - "non_hive": { - "close": 127, - "high": 1644, - "low": 127, - "open": 3507, - "volume": 6870 - }, - "open": "2020-10-15T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47475, - "high": 19581, - "low": 42409, - "open": 1244, - "volume": 150365 - }, - "id": 2747713, - "non_hive": { - "close": 7596, - "high": 3143, - "low": 6743, - "open": 199, - "volume": 24046 - }, - "open": "2020-10-15T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 200003, - "high": 200003, - "low": 200003, - "open": 200003, - "volume": 200003 - }, - "id": 2747729, - "non_hive": { - "close": 31993, - "high": 31993, - "low": 31993, - "open": 31993, - "volume": 31993 - }, - "open": "2020-10-15T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 68947, - "high": 6676, - "low": 59674, - "open": 15691, - "volume": 219907 - }, - "id": 2747733, - "non_hive": { - "close": 11024, - "high": 1068, - "low": 9500, - "open": 2510, - "volume": 35126 - }, - "open": "2020-10-15T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18769, - "high": 18769, - "low": 18769, - "open": 18769, - "volume": 18769 - }, - "id": 2747752, - "non_hive": { - "close": 3001, - "high": 3001, - "low": 3001, - "open": 3001, - "volume": 3001 - }, - "open": "2020-10-15T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 224, - "high": 6104, - "low": 224, - "open": 326, - "volume": 138828 - }, - "id": 2747756, - "non_hive": { - "close": 34, - "high": 976, - "low": 34, - "open": 52, - "volume": 22080 - }, - "open": "2020-10-15T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 59023, - "high": 13402, - "low": 37421, - "open": 37421, - "volume": 907566 - }, - "id": 2747779, - "non_hive": { - "close": 9473, - "high": 2151, - "low": 5807, - "open": 5807, - "volume": 145012 - }, - "open": "2020-10-15T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20560, - "high": 20560, - "low": 17665, - "open": 29709, - "volume": 67934 - }, - "id": 2747797, - "non_hive": { - "close": 3300, - "high": 3300, - "low": 2835, - "open": 4768, - "volume": 10903 - }, - "open": "2020-10-15T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18181, - "high": 18181, - "low": 14929, - "open": 2006, - "volume": 67675 - }, - "id": 2747804, - "non_hive": { - "close": 2923, - "high": 2923, - "low": 2396, - "open": 322, - "volume": 10874 - }, - "open": "2020-10-15T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3000, - "high": 1038, - "low": 2490, - "open": 8428, - "volume": 118179 - }, - "id": 2747814, - "non_hive": { - "close": 482, - "high": 167, - "low": 400, - "open": 1355, - "volume": 19001 - }, - "open": "2020-10-15T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 951, - "high": 951, - "low": 15000, - "open": 5653, - "volume": 155426 - }, - "id": 2747838, - "non_hive": { - "close": 153, - "high": 153, - "low": 2369, - "open": 909, - "volume": 24853 - }, - "open": "2020-10-15T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15504, - "high": 6225, - "low": 15504, - "open": 50223, - "volume": 126597 - }, - "id": 2747861, - "non_hive": { - "close": 2493, - "high": 1001, - "low": 2493, - "open": 8076, - "volume": 20357 - }, - "open": "2020-10-15T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3380, - "high": 8972, - "low": 2978, - "open": 2978, - "volume": 129414 - }, - "id": 2747874, - "non_hive": { - "close": 547, - "high": 1452, - "low": 478, - "open": 478, - "volume": 20834 - }, - "open": "2020-10-15T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 58692, - "high": 58692, - "low": 11802, - "open": 11802, - "volume": 193949 - }, - "id": 2747887, - "non_hive": { - "close": 9553, - "high": 9553, - "low": 1911, - "open": 1911, - "volume": 31516 - }, - "open": "2020-10-15T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 556658, - "high": 556658, - "low": 200000, - "open": 200000, - "volume": 899370 - }, - "id": 2747900, - "non_hive": { - "close": 92800, - "high": 92800, - "low": 32556, - "open": 32556, - "volume": 148749 - }, - "open": "2020-10-15T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13394, - "high": 13394, - "low": 16238, - "open": 16238, - "volume": 29632 - }, - "id": 2747904, - "non_hive": { - "close": 2233, - "high": 2233, - "low": 2707, - "open": 2707, - "volume": 4940 - }, - "open": "2020-10-16T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1397, - "high": 53, - "low": 10677, - "open": 53, - "volume": 12127 - }, - "id": 2747912, - "non_hive": { - "close": 233, - "high": 9, - "low": 1780, - "open": 9, - "volume": 2022 - }, - "open": "2020-10-16T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18548, - "high": 5717, - "low": 8953, - "open": 5717, - "volume": 299909 - }, - "id": 2747921, - "non_hive": { - "close": 3004, - "high": 953, - "low": 1450, - "open": 953, - "volume": 48825 - }, - "open": "2020-10-16T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1802, - "high": 21141, - "low": 12019, - "open": 14351, - "volume": 123819 - }, - "id": 2747965, - "non_hive": { - "close": 300, - "high": 3520, - "low": 1999, - "open": 2388, - "volume": 20610 - }, - "open": "2020-10-16T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40098, - "high": 11603, - "low": 6221, - "open": 12020, - "volume": 227286 - }, - "id": 2747976, - "non_hive": { - "close": 6652, - "high": 1932, - "low": 1007, - "open": 1999, - "volume": 37660 - }, - "open": "2020-10-16T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 82626, - "high": 32678, - "low": 41219, - "open": 12019, - "volume": 774900 - }, - "id": 2747997, - "non_hive": { - "close": 13344, - "high": 5421, - "low": 6656, - "open": 1992, - "volume": 125728 - }, - "open": "2020-10-16T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3523, - "high": 13791, - "low": 3523, - "open": 13791, - "volume": 188562 - }, - "id": 2748014, - "non_hive": { - "close": 583, - "high": 2286, - "low": 583, - "open": 2286, - "volume": 31246 - }, - "open": "2020-10-16T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30002, - "high": 1285, - "low": 3121, - "open": 6444, - "volume": 751986 - }, - "id": 2748024, - "non_hive": { - "close": 4972, - "high": 213, - "low": 505, - "open": 1068, - "volume": 122027 - }, - "open": "2020-10-16T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 260, - "high": 163, - "low": 260, - "open": 163, - "volume": 165198 - }, - "id": 2748061, - "non_hive": { - "close": 42, - "high": 27, - "low": 42, - "open": 27, - "volume": 27063 - }, - "open": "2020-10-16T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6172, - "high": 3336, - "low": 26195, - "open": 12243, - "volume": 263329 - }, - "id": 2748081, - "non_hive": { - "close": 997, - "high": 539, - "low": 4215, - "open": 1977, - "volume": 42422 - }, - "open": "2020-10-16T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 30474, - "high": 11762, - "low": 78091, - "open": 11762, - "volume": 773148 - }, - "id": 2748098, - "non_hive": { - "close": 4888, - "high": 1900, - "low": 12120, - "open": 1900, - "volume": 123368 - }, - "open": "2020-10-16T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 93179, - "high": 93179, - "low": 1171, - "open": 12469, - "volume": 419372 - }, - "id": 2748117, - "non_hive": { - "close": 15216, - "high": 15216, - "low": 187, - "open": 1999, - "volume": 67917 - }, - "open": "2020-10-16T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12599, - "high": 18022, - "low": 363, - "open": 8145, - "volume": 361201 - }, - "id": 2748135, - "non_hive": { - "close": 2056, - "high": 2943, - "low": 58, - "open": 1330, - "volume": 58584 - }, - "open": "2020-10-16T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23443, - "high": 18004, - "low": 20855, - "open": 18004, - "volume": 150994 - }, - "id": 2748155, - "non_hive": { - "close": 3821, - "high": 2938, - "low": 3342, - "open": 2938, - "volume": 24371 - }, - "open": "2020-10-16T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11235, - "high": 10351, - "low": 1567, - "open": 1567, - "volume": 171484 - }, - "id": 2748181, - "non_hive": { - "close": 1814, - "high": 1688, - "low": 252, - "open": 252, - "volume": 27665 - }, - "open": "2020-10-16T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11957, - "high": 15701, - "low": 11957, - "open": 15701, - "volume": 215530 - }, - "id": 2748210, - "non_hive": { - "close": 1930, - "high": 2535, - "low": 1930, - "open": 2535, - "volume": 34796 - }, - "open": "2020-10-16T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49883, - "high": 18, - "low": 1000, - "open": 4251, - "volume": 142542 - }, - "id": 2748217, - "non_hive": { - "close": 8131, - "high": 3, - "low": 161, - "open": 686, - "volume": 23189 - }, - "open": "2020-10-16T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24727, - "high": 6, - "low": 382, - "open": 12329, - "volume": 302811 - }, - "id": 2748249, - "non_hive": { - "close": 3981, - "high": 1, - "low": 61, - "open": 1999, - "volume": 48912 - }, - "open": "2020-10-16T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 1149, - "low": 4597, - "open": 1149, - "volume": 120247 - }, - "id": 2748279, - "non_hive": { - "close": 1610, - "high": 185, - "low": 736, - "open": 185, - "volume": 19319 - }, - "open": "2020-10-16T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 106, - "high": 6827, - "low": 8134, - "open": 6827, - "volume": 314074 - }, - "id": 2748297, - "non_hive": { - "close": 17, - "high": 1099, - "low": 1269, - "open": 1099, - "volume": 49996 - }, - "open": "2020-10-16T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5600, - "high": 242, - "low": 6358, - "open": 3400, - "volume": 265988 - }, - "id": 2748317, - "non_hive": { - "close": 879, - "high": 39, - "low": 991, - "open": 546, - "volume": 42421 - }, - "open": "2020-10-16T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11880, - "high": 12403, - "low": 11880, - "open": 6041, - "volume": 155581 - }, - "id": 2748352, - "non_hive": { - "close": 1895, - "high": 1997, - "low": 1895, - "open": 972, - "volume": 25030 - }, - "open": "2020-10-16T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12422, - "high": 24708, - "low": 10652, - "open": 39808, - "volume": 301555 - }, - "id": 2748369, - "non_hive": { - "close": 1999, - "high": 3978, - "low": 1704, - "open": 6409, - "volume": 48475 - }, - "open": "2020-10-16T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11177, - "high": 1509, - "low": 9569, - "open": 37002, - "volume": 814784 - }, - "id": 2748394, - "non_hive": { - "close": 1788, - "high": 243, - "low": 1524, - "open": 5957, - "volume": 131086 - }, - "open": "2020-10-16T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 565, - "high": 565, - "low": 10560, - "open": 9000, - "volume": 191264 - }, - "id": 2748417, - "non_hive": { - "close": 91, - "high": 91, - "low": 1689, - "open": 1449, - "volume": 30774 - }, - "open": "2020-10-17T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31056, - "high": 9968, - "low": 31056, - "open": 9968, - "volume": 50291 - }, - "id": 2748442, - "non_hive": { - "close": 5000, - "high": 1605, - "low": 5000, - "open": 1605, - "volume": 8097 - }, - "open": "2020-10-17T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29000, - "high": 30726, - "low": 817, - "open": 86000, - "volume": 234601 - }, - "id": 2748452, - "non_hive": { - "close": 4669, - "high": 4947, - "low": 131, - "open": 13846, - "volume": 37767 - }, - "open": "2020-10-17T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 150664, - "high": 702, - "low": 150664, - "open": 702, - "volume": 500702 - }, - "id": 2748470, - "non_hive": { - "close": 23505, - "high": 113, - "low": 23505, - "open": 113, - "volume": 79011 - }, - "open": "2020-10-17T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 314, - "high": 8695, - "low": 6385, - "open": 17820, - "volume": 130001 - }, - "id": 2748476, - "non_hive": { - "close": 50, - "high": 1400, - "low": 1015, - "open": 2867, - "volume": 20775 - }, - "open": "2020-10-17T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25848, - "high": 5665, - "low": 166, - "open": 166, - "volume": 31679 - }, - "id": 2748496, - "non_hive": { - "close": 4115, - "high": 902, - "low": 26, - "open": 26, - "volume": 5043 - }, - "open": "2020-10-17T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 307, - "high": 307, - "low": 47968, - "open": 2576, - "volume": 455513 - }, - "id": 2748503, - "non_hive": { - "close": 49, - "high": 49, - "low": 7445, - "open": 410, - "volume": 71155 - }, - "open": "2020-10-17T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 76129, - "high": 1061, - "low": 7736, - "open": 31776, - "volume": 333608 - }, - "id": 2748526, - "non_hive": { - "close": 12120, - "high": 169, - "low": 1230, - "open": 5056, - "volume": 53099 - }, - "open": "2020-10-17T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28027, - "high": 38290, - "low": 28027, - "open": 38290, - "volume": 66317 - }, - "id": 2748550, - "non_hive": { - "close": 4462, - "high": 6096, - "low": 4462, - "open": 6096, - "volume": 10558 - }, - "open": "2020-10-17T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1047, - "high": 12423, - "low": 3300, - "open": 3300, - "volume": 68788 - }, - "id": 2748557, - "non_hive": { - "close": 168, - "high": 1999, - "low": 525, - "open": 525, - "volume": 10997 - }, - "open": "2020-10-17T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12423, - "high": 6, - "low": 460, - "open": 37, - "volume": 369649 - }, - "id": 2748576, - "non_hive": { - "close": 1999, - "high": 1, - "low": 73, - "open": 6, - "volume": 59150 - }, - "open": "2020-10-17T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4130, - "high": 7894, - "low": 458, - "open": 12422, - "volume": 367995 - }, - "id": 2748612, - "non_hive": { - "close": 651, - "high": 1271, - "low": 72, - "open": 1999, - "volume": 58716 - }, - "open": "2020-10-17T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 31817, - "high": 82, - "low": 13629, - "open": 3768, - "volume": 58812 - }, - "id": 2748641, - "non_hive": { - "close": 5015, - "high": 13, - "low": 2148, - "open": 594, - "volume": 9270 - }, - "open": "2020-10-17T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 70132, - "high": 70132, - "low": 613, - "open": 8727, - "volume": 261889 - }, - "id": 2748653, - "non_hive": { - "close": 11281, - "high": 11281, - "low": 96, - "open": 1400, - "volume": 42085 - }, - "open": "2020-10-17T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8622, - "high": 52451, - "low": 7686, - "open": 7686, - "volume": 148074 - }, - "id": 2748660, - "non_hive": { - "close": 1398, - "high": 8509, - "low": 1233, - "open": 1233, - "volume": 23961 - }, - "open": "2020-10-17T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8944, - "high": 844, - "low": 2044, - "open": 3707, - "volume": 65353 - }, - "id": 2748680, - "non_hive": { - "close": 1450, - "high": 137, - "low": 328, - "open": 601, - "volume": 10515 - }, - "open": "2020-10-17T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 177, - "high": 67, - "low": 6345, - "open": 2810, - "volume": 621180 - }, - "id": 2748703, - "non_hive": { - "close": 28, - "high": 11, - "low": 1000, - "open": 455, - "volume": 99147 - }, - "open": "2020-10-17T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43, - "high": 43, - "low": 3390, - "open": 3390, - "volume": 106314 - }, - "id": 2748733, - "non_hive": { - "close": 7, - "high": 7, - "low": 532, - "open": 532, - "volume": 17019 - }, - "open": "2020-10-17T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27274, - "high": 26384, - "low": 12679, - "open": 2444, - "volume": 239305 - }, - "id": 2748756, - "non_hive": { - "close": 4399, - "high": 4257, - "low": 1999, - "open": 390, - "volume": 38421 - }, - "open": "2020-10-17T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1900, - "high": 6, - "low": 7251, - "open": 31368, - "volume": 478962 - }, - "id": 2748772, - "non_hive": { - "close": 300, - "high": 1, - "low": 1137, - "open": 5080, - "volume": 76595 - }, - "open": "2020-10-17T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7518, - "high": 20507, - "low": 7518, - "open": 9082, - "volume": 1181801 - }, - "id": 2748807, - "non_hive": { - "close": 1188, - "high": 3342, - "low": 1188, - "open": 1436, - "volume": 191566 - }, - "open": "2020-10-17T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9578, - "high": 9578, - "low": 6334, - "open": 7524, - "volume": 408663 - }, - "id": 2748836, - "non_hive": { - "close": 1561, - "high": 1561, - "low": 1000, - "open": 1189, - "volume": 64631 - }, - "open": "2020-10-17T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21547, - "high": 6135, - "low": 1302, - "open": 5012, - "volume": 141521 - }, - "id": 2748863, - "non_hive": { - "close": 3445, - "high": 999, - "low": 208, - "open": 816, - "volume": 22659 - }, - "open": "2020-10-17T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20000, - "high": 6, - "low": 11497, - "open": 5992, - "volume": 778080 - }, - "id": 2748888, - "non_hive": { - "close": 3300, - "high": 1, - "low": 1837, - "open": 958, - "volume": 127485 - }, - "open": "2020-10-17T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 96910, - "high": 428533, - "low": 31552, - "open": 31552, - "volume": 605640 - }, - "id": 2748909, - "non_hive": { - "close": 15990, - "high": 70708, - "low": 5195, - "open": 5195, - "volume": 99914 - }, - "open": "2020-10-18T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15184, - "high": 9903, - "low": 2698, - "open": 2698, - "volume": 30155 - }, - "id": 2748924, - "non_hive": { - "close": 2505, - "high": 1634, - "low": 445, - "open": 445, - "volume": 4975 - }, - "open": "2020-10-18T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 121246, - "high": 9166, - "low": 19055, - "open": 31415, - "volume": 267050 - }, - "id": 2748937, - "non_hive": { - "close": 19945, - "high": 1512, - "low": 3049, - "open": 5131, - "volume": 43711 - }, - "open": "2020-10-18T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18975, - "high": 10594, - "low": 12491, - "open": 12491, - "volume": 830700 - }, - "id": 2748958, - "non_hive": { - "close": 3057, - "high": 1748, - "low": 2000, - "open": 2000, - "volume": 136049 - }, - "open": "2020-10-18T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18410, - "high": 30927, - "low": 18410, - "open": 12408, - "volume": 376760 - }, - "id": 2748995, - "non_hive": { - "close": 2947, - "high": 5000, - "low": 2947, - "open": 2000, - "volume": 60706 - }, - "open": "2020-10-18T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 571, - "high": 839, - "low": 31477, - "open": 31477, - "volume": 77209 - }, - "id": 2749026, - "non_hive": { - "close": 93, - "high": 138, - "low": 5039, - "open": 5039, - "volume": 12516 - }, - "open": "2020-10-18T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27804, - "high": 2806, - "low": 51, - "open": 12421, - "volume": 328406 - }, - "id": 2749047, - "non_hive": { - "close": 4396, - "high": 452, - "low": 8, - "open": 1999, - "volume": 52490 - }, - "open": "2020-10-18T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25101, - "high": 25101, - "low": 12553, - "open": 12553, - "volume": 227867 - }, - "id": 2749076, - "non_hive": { - "close": 4085, - "high": 4085, - "low": 1999, - "open": 1999, - "volume": 36553 - }, - "open": "2020-10-18T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11587, - "high": 938, - "low": 391, - "open": 11594, - "volume": 80461 - }, - "id": 2749106, - "non_hive": { - "close": 1902, - "high": 154, - "low": 63, - "open": 1886, - "volume": 13183 - }, - "open": "2020-10-18T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19226, - "high": 678, - "low": 19226, - "open": 12269, - "volume": 202484 - }, - "id": 2749126, - "non_hive": { - "close": 3076, - "high": 111, - "low": 3076, - "open": 2000, - "volume": 32905 - }, - "open": "2020-10-18T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16743, - "high": 13491, - "low": 2184, - "open": 2184, - "volume": 336218 - }, - "id": 2749157, - "non_hive": { - "close": 2729, - "high": 2199, - "low": 349, - "open": 349, - "volume": 54791 - }, - "open": "2020-10-18T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50307, - "high": 2546, - "low": 12271, - "open": 12271, - "volume": 90839 - }, - "id": 2749185, - "non_hive": { - "close": 8200, - "high": 415, - "low": 1999, - "open": 1999, - "volume": 14804 - }, - "open": "2020-10-18T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53383, - "high": 24, - "low": 1426, - "open": 37288, - "volume": 378385 - }, - "id": 2749198, - "non_hive": { - "close": 8691, - "high": 4, - "low": 232, - "open": 6078, - "volume": 61629 - }, - "open": "2020-10-18T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 92406, - "high": 2303, - "low": 6002, - "open": 6002, - "volume": 144622 - }, - "id": 2749225, - "non_hive": { - "close": 15044, - "high": 375, - "low": 977, - "open": 977, - "volume": 23545 - }, - "open": "2020-10-18T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2457, - "high": 6257, - "low": 2457, - "open": 47219, - "volume": 277124 - }, - "id": 2749238, - "non_hive": { - "close": 399, - "high": 1020, - "low": 399, - "open": 7687, - "volume": 45145 - }, - "open": "2020-10-18T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 30, - "low": 1488, - "open": 6981, - "volume": 918145 - }, - "id": 2749267, - "non_hive": { - "close": 162, - "high": 5, - "low": 238, - "open": 1138, - "volume": 148291 - }, - "open": "2020-10-18T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28017, - "high": 4003, - "low": 28017, - "open": 4003, - "volume": 104227 - }, - "id": 2749297, - "non_hive": { - "close": 4554, - "high": 651, - "low": 4554, - "open": 651, - "volume": 16944 - }, - "open": "2020-10-18T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33696, - "high": 33696, - "low": 12403, - "open": 12403, - "volume": 335537 - }, - "id": 2749304, - "non_hive": { - "close": 5480, - "high": 5480, - "low": 1999, - "open": 1999, - "volume": 54505 - }, - "open": "2020-10-18T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 370, - "high": 6, - "low": 1770, - "open": 8332, - "volume": 1335505 - }, - "id": 2749314, - "non_hive": { - "close": 60, - "high": 1, - "low": 283, - "open": 1355, - "volume": 215235 - }, - "open": "2020-10-18T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1921, - "high": 28113, - "low": 2377, - "open": 10396, - "volume": 644765 - }, - "id": 2749385, - "non_hive": { - "close": 311, - "high": 4558, - "low": 380, - "open": 1674, - "volume": 104261 - }, - "open": "2020-10-18T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19022, - "high": 3000, - "low": 19022, - "open": 12357, - "volume": 496542 - }, - "id": 2749419, - "non_hive": { - "close": 3062, - "high": 486, - "low": 3062, - "open": 1999, - "volume": 80304 - }, - "open": "2020-10-18T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23160, - "high": 80, - "low": 1997, - "open": 12348, - "volume": 627556 - }, - "id": 2749462, - "non_hive": { - "close": 3751, - "high": 13, - "low": 319, - "open": 1999, - "volume": 100779 - }, - "open": "2020-10-18T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49887, - "high": 7699, - "low": 5600, - "open": 5600, - "volume": 63186 - }, - "id": 2749488, - "non_hive": { - "close": 8080, - "high": 1247, - "low": 907, - "open": 907, - "volume": 10234 - }, - "open": "2020-10-18T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3704, - "high": 3704, - "low": 10022, - "open": 14287, - "volume": 830347 - }, - "id": 2749498, - "non_hive": { - "close": 600, - "high": 600, - "low": 1603, - "open": 2314, - "volume": 134304 - }, - "open": "2020-10-18T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 37263, - "high": 715, - "low": 232668, - "open": 9423, - "volume": 459573 - }, - "id": 2749515, - "non_hive": { - "close": 6010, - "high": 116, - "low": 37227, - "open": 1526, - "volume": 73892 - }, - "open": "2020-10-19T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4302, - "high": 117, - "low": 6463, - "open": 50565, - "volume": 258935 - }, - "id": 2749557, - "non_hive": { - "close": 697, - "high": 19, - "low": 1034, - "open": 8091, - "volume": 41443 - }, - "open": "2020-10-19T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 45647, - "high": 45647, - "low": 4501, - "open": 1833, - "volume": 53684 - }, - "id": 2749569, - "non_hive": { - "close": 7399, - "high": 7399, - "low": 729, - "open": 297, - "volume": 8701 - }, - "open": "2020-10-19T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6, - "high": 6, - "low": 2506, - "open": 23894, - "volume": 136809 - }, - "id": 2749579, - "non_hive": { - "close": 1, - "high": 1, - "low": 405, - "open": 3873, - "volume": 22180 - }, - "open": "2020-10-19T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12332, - "high": 456, - "low": 113424, - "open": 17695, - "volume": 196877 - }, - "id": 2749605, - "non_hive": { - "close": 1999, - "high": 74, - "low": 17947, - "open": 2870, - "volume": 31430 - }, - "open": "2020-10-19T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13515, - "high": 18499, - "low": 20413, - "open": 1421, - "volume": 265424 - }, - "id": 2749624, - "non_hive": { - "close": 2163, - "high": 3000, - "low": 3266, - "open": 230, - "volume": 42670 - }, - "open": "2020-10-19T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8713, - "high": 8713, - "low": 14938, - "open": 1634, - "volume": 93288 - }, - "id": 2749658, - "non_hive": { - "close": 1411, - "high": 1411, - "low": 2363, - "open": 259, - "volume": 14799 - }, - "open": "2020-10-19T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38223, - "high": 172, - "low": 6294, - "open": 8873, - "volume": 445435 - }, - "id": 2749672, - "non_hive": { - "close": 6061, - "high": 28, - "low": 998, - "open": 1411, - "volume": 71521 - }, - "open": "2020-10-19T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100000, - "high": 14138, - "low": 11291, - "open": 12484, - "volume": 144256 - }, - "id": 2749709, - "non_hive": { - "close": 16020, - "high": 2265, - "low": 1790, - "open": 1999, - "volume": 23080 - }, - "open": "2020-10-19T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15617, - "high": 15617, - "low": 4744, - "open": 14936, - "volume": 93933 - }, - "id": 2749722, - "non_hive": { - "close": 2503, - "high": 2503, - "low": 757, - "open": 2393, - "volume": 15043 - }, - "open": "2020-10-19T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2710, - "high": 755, - "low": 330, - "open": 755, - "volume": 325758 - }, - "id": 2749735, - "non_hive": { - "close": 429, - "high": 121, - "low": 52, - "open": 121, - "volume": 52125 - }, - "open": "2020-10-19T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2905, - "high": 299, - "low": 5229, - "open": 63765, - "volume": 134091 - }, - "id": 2749748, - "non_hive": { - "close": 465, - "high": 48, - "low": 837, - "open": 10214, - "volume": 21475 - }, - "open": "2020-10-19T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24, - "high": 24, - "low": 1581, - "open": 136664, - "volume": 512047 - }, - "id": 2749774, - "non_hive": { - "close": 4, - "high": 4, - "low": 253, - "open": 21890, - "volume": 82326 - }, - "open": "2020-10-19T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3319, - "high": 66201, - "low": 12251, - "open": 4054, - "volume": 127208 - }, - "id": 2749804, - "non_hive": { - "close": 537, - "high": 10722, - "low": 1960, - "open": 656, - "volume": 20571 - }, - "open": "2020-10-19T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5000, - "high": 1050, - "low": 5506, - "open": 9037, - "volume": 423519 - }, - "id": 2749818, - "non_hive": { - "close": 809, - "high": 170, - "low": 881, - "open": 1462, - "volume": 68393 - }, - "open": "2020-10-19T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 53153, - "high": 37079, - "low": 6038, - "open": 12356, - "volume": 411145 - }, - "id": 2749850, - "non_hive": { - "close": 8505, - "high": 6001, - "low": 957, - "open": 1999, - "volume": 66117 - }, - "open": "2020-10-19T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 237, - "high": 162, - "low": 26275, - "open": 10237, - "volume": 245674 - }, - "id": 2749886, - "non_hive": { - "close": 38, - "high": 26, - "low": 4203, - "open": 1638, - "volume": 39302 - }, - "open": "2020-10-19T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 100319, - "high": 93, - "low": 100319, - "open": 6249, - "volume": 522784 - }, - "id": 2749914, - "non_hive": { - "close": 16051, - "high": 15, - "low": 16051, - "open": 1000, - "volume": 83654 - }, - "open": "2020-10-19T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14585, - "high": 13916, - "low": 11912, - "open": 5938, - "volume": 1237059 - }, - "id": 2749930, - "non_hive": { - "close": 2335, - "high": 2228, - "low": 1885, - "open": 950, - "volume": 196426 - }, - "open": "2020-10-19T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 41131, - "high": 1061, - "low": 19326, - "open": 4797, - "volume": 221229 - }, - "id": 2749958, - "non_hive": { - "close": 6584, - "high": 170, - "low": 3072, - "open": 768, - "volume": 35351 - }, - "open": "2020-10-19T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 458, - "high": 61046, - "low": 458, - "open": 106184, - "volume": 638396 - }, - "id": 2749992, - "non_hive": { - "close": 73, - "high": 9878, - "low": 73, - "open": 17000, - "volume": 102373 - }, - "open": "2020-10-19T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2707, - "high": 3936, - "low": 468, - "open": 458, - "volume": 44497 - }, - "id": 2750012, - "non_hive": { - "close": 438, - "high": 637, - "low": 75, - "open": 74, - "volume": 7170 - }, - "open": "2020-10-19T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9524, - "high": 284, - "low": 9524, - "open": 9309, - "volume": 1868115 - }, - "id": 2750029, - "non_hive": { - "close": 1457, - "high": 46, - "low": 1457, - "open": 1506, - "volume": 292183 - }, - "open": "2020-10-19T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 529, - "high": 36287, - "low": 104498, - "open": 12364, - "volume": 252135 - }, - "id": 2750056, - "non_hive": { - "close": 85, - "high": 5870, - "low": 15991, - "open": 1999, - "volume": 39122 - }, - "open": "2020-10-19T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7000, - "high": 37, - "low": 12974, - "open": 12502, - "volume": 175074 - }, - "id": 2750072, - "non_hive": { - "close": 1082, - "high": 6, - "low": 2000, - "open": 1999, - "volume": 27684 - }, - "open": "2020-10-20T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14471, - "high": 7258, - "low": 105326, - "open": 7258, - "volume": 237399 - }, - "id": 2750101, - "non_hive": { - "close": 2287, - "high": 1148, - "low": 16234, - "open": 1148, - "volume": 37062 - }, - "open": "2020-10-20T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 633, - "high": 6, - "low": 44, - "open": 2391, - "volume": 738663 - }, - "id": 2750115, - "non_hive": { - "close": 100, - "high": 1, - "low": 6, - "open": 378, - "volume": 113518 - }, - "open": "2020-10-20T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 726, - "high": 10159, - "low": 15171, - "open": 10159, - "volume": 341188 - }, - "id": 2750145, - "non_hive": { - "close": 114, - "high": 1604, - "low": 2321, - "open": 1604, - "volume": 53343 - }, - "open": "2020-10-20T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12747, - "high": 12747, - "low": 12747, - "open": 12747, - "volume": 12747 - }, - "id": 2750174, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-10-20T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20460, - "high": 10070, - "low": 20460, - "open": 10070, - "volume": 149566 - }, - "id": 2750178, - "non_hive": { - "close": 3208, - "high": 1580, - "low": 3208, - "open": 1580, - "volume": 23462 - }, - "open": "2020-10-20T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 976, - "high": 11263, - "low": 976, - "open": 11263, - "volume": 75384 - }, - "id": 2750191, - "non_hive": { - "close": 153, - "high": 1766, - "low": 153, - "open": 1766, - "volume": 11819 - }, - "open": "2020-10-20T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15479, - "high": 6, - "low": 15479, - "open": 12747, - "volume": 231793 - }, - "id": 2750200, - "non_hive": { - "close": 2372, - "high": 1, - "low": 2372, - "open": 1999, - "volume": 36165 - }, - "open": "2020-10-20T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1034, - "high": 182961, - "low": 177, - "open": 471, - "volume": 525551 - }, - "id": 2750238, - "non_hive": { - "close": 162, - "high": 28703, - "low": 27, - "open": 72, - "volume": 82107 - }, - "open": "2020-10-20T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40979, - "high": 12, - "low": 1814, - "open": 30747, - "volume": 175911 - }, - "id": 2750281, - "non_hive": { - "close": 6421, - "high": 2, - "low": 284, - "open": 4817, - "volume": 27559 - }, - "open": "2020-10-20T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2038, - "high": 134, - "low": 7963, - "open": 88351, - "volume": 485434 - }, - "id": 2750302, - "non_hive": { - "close": 319, - "high": 21, - "low": 1226, - "open": 13837, - "volume": 75683 - }, - "open": "2020-10-20T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3484, - "high": 210, - "low": 201302, - "open": 6864, - "volume": 1633928 - }, - "id": 2750328, - "non_hive": { - "close": 546, - "high": 33, - "low": 31000, - "open": 1074, - "volume": 255521 - }, - "open": "2020-10-20T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2233, - "high": 191, - "low": 2627, - "open": 2627, - "volume": 482060 - }, - "id": 2750355, - "non_hive": { - "close": 347, - "high": 30, - "low": 404, - "open": 404, - "volume": 75186 - }, - "open": "2020-10-20T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1893, - "high": 926, - "low": 1704, - "open": 12780, - "volume": 182313 - }, - "id": 2750392, - "non_hive": { - "close": 295, - "high": 145, - "low": 259, - "open": 1999, - "volume": 28307 - }, - "open": "2020-10-20T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12704, - "high": 43232, - "low": 299749, - "open": 540, - "volume": 1178032 - }, - "id": 2750422, - "non_hive": { - "close": 1967, - "high": 6739, - "low": 45651, - "open": 84, - "volume": 181581 - }, - "open": "2020-10-20T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6716, - "high": 264, - "low": 6716, - "open": 207, - "volume": 320699 - }, - "id": 2750469, - "non_hive": { - "close": 1022, - "high": 41, - "low": 1022, - "open": 32, - "volume": 49636 - }, - "open": "2020-10-20T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1389, - "high": 91767, - "low": 1389, - "open": 16149, - "volume": 1713695 - }, - "id": 2750495, - "non_hive": { - "close": 208, - "high": 14207, - "low": 208, - "open": 2500, - "volume": 258970 - }, - "open": "2020-10-20T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7033, - "high": 6, - "low": 9, - "open": 193814, - "volume": 388325 - }, - "id": 2750527, - "non_hive": { - "close": 1088, - "high": 1, - "low": 1, - "open": 30000, - "volume": 59998 - }, - "open": "2020-10-20T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8559, - "high": 6, - "low": 8559, - "open": 49346, - "volume": 215298 - }, - "id": 2750563, - "non_hive": { - "close": 1283, - "high": 1, - "low": 1283, - "open": 7633, - "volume": 32928 - }, - "open": "2020-10-20T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5405, - "high": 6, - "low": 181, - "open": 12925, - "volume": 91983 - }, - "id": 2750582, - "non_hive": { - "close": 819, - "high": 1, - "low": 27, - "open": 1999, - "volume": 14070 - }, - "open": "2020-10-20T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11429, - "high": 19, - "low": 10381, - "open": 10381, - "volume": 41435 - }, - "id": 2750609, - "non_hive": { - "close": 1749, - "high": 3, - "low": 1572, - "open": 1572, - "volume": 6324 - }, - "open": "2020-10-20T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4261, - "high": 27624, - "low": 100, - "open": 1615, - "volume": 377062 - }, - "id": 2750622, - "non_hive": { - "close": 651, - "high": 4279, - "low": 15, - "open": 247, - "volume": 57643 - }, - "open": "2020-10-20T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 54240, - "high": 18000, - "low": 474, - "open": 13082, - "volume": 212130 - }, - "id": 2750662, - "non_hive": { - "close": 8136, - "high": 2752, - "low": 71, - "open": 1999, - "volume": 31959 - }, - "open": "2020-10-20T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13333, - "high": 53, - "low": 8056, - "open": 446, - "volume": 185205 - }, - "id": 2750698, - "non_hive": { - "close": 2000, - "high": 8, - "low": 1208, - "open": 67, - "volume": 27783 - }, - "open": "2020-10-20T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6652, - "high": 1866, - "low": 19709, - "open": 2006, - "volume": 96722 - }, - "id": 2750718, - "non_hive": { - "close": 998, - "high": 280, - "low": 2956, - "open": 301, - "volume": 14509 - }, - "open": "2020-10-21T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7462, - "high": 11880, - "low": 7462, - "open": 11880, - "volume": 19342 - }, - "id": 2750735, - "non_hive": { - "close": 1119, - "high": 1782, - "low": 1119, - "open": 1782, - "volume": 2901 - }, - "open": "2020-10-21T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9459, - "high": 8005, - "low": 94991, - "open": 25144, - "volume": 137599 - }, - "id": 2750742, - "non_hive": { - "close": 1419, - "high": 1201, - "low": 14250, - "open": 3772, - "volume": 20642 - }, - "open": "2020-10-21T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18064, - "high": 18064, - "low": 3488, - "open": 27537, - "volume": 525947 - }, - "id": 2750753, - "non_hive": { - "close": 2710, - "high": 2710, - "low": 523, - "open": 4131, - "volume": 78899 - }, - "open": "2020-10-21T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 137456, - "high": 16049, - "low": 3065, - "open": 6652, - "volume": 474825 - }, - "id": 2750765, - "non_hive": { - "close": 21000, - "high": 2452, - "low": 459, - "open": 998, - "volume": 71658 - }, - "open": "2020-10-21T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23653, - "high": 98, - "low": 2887, - "open": 11005, - "volume": 101909 - }, - "id": 2750785, - "non_hive": { - "close": 3579, - "high": 15, - "low": 436, - "open": 1682, - "volume": 15533 - }, - "open": "2020-10-21T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2513, - "high": 65364, - "low": 6827, - "open": 6827, - "volume": 190538 - }, - "id": 2750804, - "non_hive": { - "close": 380, - "high": 9890, - "low": 1032, - "open": 1032, - "volume": 28828 - }, - "open": "2020-10-21T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14581, - "high": 14581, - "low": 66458, - "open": 18303, - "volume": 643151 - }, - "id": 2750820, - "non_hive": { - "close": 2231, - "high": 2231, - "low": 10046, - "open": 2769, - "volume": 97546 - }, - "open": "2020-10-21T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20576, - "high": 32661, - "low": 20576, - "open": 32661, - "volume": 53237 - }, - "id": 2750855, - "non_hive": { - "close": 3146, - "high": 4994, - "low": 3146, - "open": 4994, - "volume": 8140 - }, - "open": "2020-10-21T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7142, - "high": 42456, - "low": 13072, - "open": 13072, - "volume": 81010 - }, - "id": 2750862, - "non_hive": { - "close": 1099, - "high": 6536, - "low": 1999, - "open": 1999, - "volume": 12440 - }, - "open": "2020-10-21T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13008, - "high": 13642, - "low": 116, - "open": 106101, - "volume": 404317 - }, - "id": 2750869, - "non_hive": { - "close": 1999, - "high": 2099, - "low": 17, - "open": 16325, - "volume": 61780 - }, - "open": "2020-10-21T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9311, - "high": 3258, - "low": 23034, - "open": 3258, - "volume": 85200 - }, - "id": 2750912, - "non_hive": { - "close": 1429, - "high": 501, - "low": 3455, - "open": 501, - "volume": 12955 - }, - "open": "2020-10-21T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33096, - "high": 735, - "low": 1000, - "open": 9171, - "volume": 552621 - }, - "id": 2750930, - "non_hive": { - "close": 5084, - "high": 113, - "low": 151, - "open": 1408, - "volume": 84090 - }, - "open": "2020-10-21T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6781, - "high": 6, - "low": 1453756, - "open": 42940, - "volume": 2371987 - }, - "id": 2750975, - "non_hive": { - "close": 1042, - "high": 1, - "low": 219517, - "open": 6596, - "volume": 360538 - }, - "open": "2020-10-21T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8550, - "high": 3273, - "low": 8550, - "open": 6206, - "volume": 296408 - }, - "id": 2751010, - "non_hive": { - "close": 1312, - "high": 503, - "low": 1312, - "open": 953, - "volume": 45520 - }, - "open": "2020-10-21T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34337, - "high": 37690, - "low": 34337, - "open": 37690, - "volume": 305058 - }, - "id": 2751042, - "non_hive": { - "close": 5202, - "high": 5783, - "low": 5202, - "open": 5783, - "volume": 46332 - }, - "open": "2020-10-21T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 91624, - "high": 325, - "low": 24954, - "open": 1953, - "volume": 1079093 - }, - "id": 2751063, - "non_hive": { - "close": 14059, - "high": 50, - "low": 3767, - "open": 296, - "volume": 165400 - }, - "open": "2020-10-21T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 93100, - "high": 104, - "low": 93100, - "open": 26074, - "volume": 1551443 - }, - "id": 2751107, - "non_hive": { - "close": 14059, - "high": 16, - "low": 14059, - "open": 4001, - "volume": 237194 - }, - "open": "2020-10-21T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 61412, - "high": 134715, - "low": 2747, - "open": 2323, - "volume": 523709 - }, - "id": 2751140, - "non_hive": { - "close": 9408, - "high": 20651, - "low": 420, - "open": 356, - "volume": 80259 - }, - "open": "2020-10-21T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 379, - "high": 143432, - "low": 379, - "open": 19564, - "volume": 490248 - }, - "id": 2751169, - "non_hive": { - "close": 58, - "high": 22000, - "low": 58, - "open": 2997, - "volume": 75128 - }, - "open": "2020-10-21T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 150000, - "high": 234, - "low": 1540, - "open": 67075, - "volume": 1095449 - }, - "id": 2751195, - "non_hive": { - "close": 22995, - "high": 36, - "low": 232, - "open": 10283, - "volume": 167880 - }, - "open": "2020-10-21T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6736, - "high": 3879, - "low": 6736, - "open": 48479, - "volume": 716477 - }, - "id": 2751226, - "non_hive": { - "close": 1017, - "high": 595, - "low": 1017, - "open": 7433, - "volume": 109255 - }, - "open": "2020-10-21T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17565, - "high": 13, - "low": 594, - "open": 13244, - "volume": 400548 - }, - "id": 2751271, - "non_hive": { - "close": 2634, - "high": 2, - "low": 89, - "open": 2011, - "volume": 60211 - }, - "open": "2020-10-21T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15917, - "high": 223, - "low": 12673, - "open": 494, - "volume": 249303 - }, - "id": 2751291, - "non_hive": { - "close": 2419, - "high": 34, - "low": 1900, - "open": 75, - "volume": 37808 - }, - "open": "2020-10-21T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72807, - "high": 13871, - "low": 72807, - "open": 13871, - "volume": 100000 - }, - "id": 2751308, - "non_hive": { - "close": 10935, - "high": 2108, - "low": 10935, - "open": 2108, - "volume": 15067 - }, - "open": "2020-10-22T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11480, - "high": 6003, - "low": 3037, - "open": 6003, - "volume": 88785 - }, - "id": 2751313, - "non_hive": { - "close": 1744, - "high": 912, - "low": 456, - "open": 912, - "volume": 13393 - }, - "open": "2020-10-22T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25127, - "high": 6, - "low": 148, - "open": 2137, - "volume": 97256 - }, - "id": 2751328, - "non_hive": { - "close": 3819, - "high": 1, - "low": 22, - "open": 320, - "volume": 14597 - }, - "open": "2020-10-22T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6281, - "high": 6281, - "low": 6281, - "open": 6281, - "volume": 6281 - }, - "id": 2751346, - "non_hive": { - "close": 945, - "high": 945, - "low": 945, - "open": 945, - "volume": 945 - }, - "open": "2020-10-22T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1456579, - "high": 1456579, - "low": 93, - "open": 93, - "volume": 3476809 - }, - "id": 2751350, - "non_hive": { - "close": 221400, - "high": 221400, - "low": 14, - "open": 14, - "volume": 528468 - }, - "open": "2020-10-22T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 19300, - "low": 280, - "open": 13158, - "volume": 750764 - }, - "id": 2751360, - "non_hive": { - "close": 153, - "high": 2953, - "low": 42, - "open": 1999, - "volume": 114147 - }, - "open": "2020-10-22T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6542, - "high": 527843, - "low": 9684, - "open": 13072, - "volume": 571182 - }, - "id": 2751373, - "non_hive": { - "close": 1000, - "high": 80760, - "low": 1480, - "open": 1999, - "volume": 87386 - }, - "open": "2020-10-22T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 22738, - "high": 22738, - "low": 7786, - "open": 98188, - "volume": 207672 - }, - "id": 2751389, - "non_hive": { - "close": 3477, - "high": 3477, - "low": 1188, - "open": 15007, - "volume": 31739 - }, - "open": "2020-10-22T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 769337, - "high": 769337, - "low": 268993, - "open": 9273, - "volume": 3780633 - }, - "id": 2751416, - "non_hive": { - "close": 118368, - "high": 118368, - "low": 41075, - "open": 1416, - "volume": 579596 - }, - "open": "2020-10-22T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3959, - "high": 4143, - "low": 113091, - "open": 4143, - "volume": 317131 - }, - "id": 2751426, - "non_hive": { - "close": 606, - "high": 648, - "low": 17302, - "open": 648, - "volume": 49046 - }, - "open": "2020-10-22T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1331, - "high": 16661, - "low": 77917, - "open": 2694, - "volume": 496140 - }, - "id": 2751441, - "non_hive": { - "close": 207, - "high": 2599, - "low": 12000, - "open": 420, - "volume": 77004 - }, - "open": "2020-10-22T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6454, - "high": 4049, - "low": 2439, - "open": 4049, - "volume": 410747 - }, - "id": 2751473, - "non_hive": { - "close": 1000, - "high": 628, - "low": 375, - "open": 628, - "volume": 63640 - }, - "open": "2020-10-22T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10675, - "high": 1464, - "low": 4269, - "open": 1464, - "volume": 1115563 - }, - "id": 2751497, - "non_hive": { - "close": 1653, - "high": 227, - "low": 640, - "open": 227, - "volume": 170517 - }, - "open": "2020-10-22T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11345, - "high": 65516, - "low": 11345, - "open": 6707, - "volume": 205000 - }, - "id": 2751526, - "non_hive": { - "close": 1703, - "high": 10151, - "low": 1703, - "open": 1038, - "volume": 31639 - }, - "open": "2020-10-22T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5101, - "high": 6, - "low": 6150, - "open": 6150, - "volume": 188834 - }, - "id": 2751543, - "non_hive": { - "close": 790, - "high": 1, - "low": 923, - "open": 923, - "volume": 29223 - }, - "open": "2020-10-22T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 181, - "high": 25, - "low": 4250, - "open": 4998, - "volume": 355615 - }, - "id": 2751564, - "non_hive": { - "close": 28, - "high": 4, - "low": 633, - "open": 774, - "volume": 53246 - }, - "open": "2020-10-22T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34098, - "high": 34098, - "low": 560, - "open": 136, - "volume": 142730 - }, - "id": 2751585, - "non_hive": { - "close": 5278, - "high": 5278, - "low": 86, - "open": 21, - "volume": 22019 - }, - "open": "2020-10-22T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11690, - "high": 7272, - "low": 182, - "open": 85605, - "volume": 235622 - }, - "id": 2751612, - "non_hive": { - "close": 1809, - "high": 1126, - "low": 28, - "open": 13254, - "volume": 36460 - }, - "open": "2020-10-22T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15004, - "high": 6445, - "low": 1228, - "open": 1228, - "volume": 135025 - }, - "id": 2751630, - "non_hive": { - "close": 2323, - "high": 998, - "low": 190, - "open": 190, - "volume": 20905 - }, - "open": "2020-10-22T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11087, - "high": 400, - "low": 28917, - "open": 6452, - "volume": 172231 - }, - "id": 2751656, - "non_hive": { - "close": 1717, - "high": 62, - "low": 4477, - "open": 999, - "volume": 26669 - }, - "open": "2020-10-22T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 52, - "high": 200, - "low": 52, - "open": 17705, - "volume": 90355 - }, - "id": 2751679, - "non_hive": { - "close": 8, - "high": 31, - "low": 8, - "open": 2741, - "volume": 13974 - }, - "open": "2020-10-22T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11458, - "high": 74799, - "low": 11458, - "open": 74799, - "volume": 442571 - }, - "id": 2751690, - "non_hive": { - "close": 1742, - "high": 11519, - "low": 1742, - "open": 11519, - "volume": 67595 - }, - "open": "2020-10-22T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3138, - "high": 1036, - "low": 19855, - "open": 11458, - "volume": 157324 - }, - "id": 2751715, - "non_hive": { - "close": 481, - "high": 159, - "low": 3020, - "open": 1758, - "volume": 24092 - }, - "open": "2020-10-22T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1137, - "high": 6, - "low": 47, - "open": 1571, - "volume": 1287082 - }, - "id": 2751735, - "non_hive": { - "close": 174, - "high": 1, - "low": 7, - "open": 241, - "volume": 197211 - }, - "open": "2020-10-22T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19431, - "high": 15498, - "low": 19431, - "open": 216, - "volume": 225543 - }, - "id": 2751817, - "non_hive": { - "close": 2953, - "high": 2385, - "low": 2953, - "open": 33, - "volume": 34533 - }, - "open": "2020-10-23T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14000, - "high": 475, - "low": 14000, - "open": 475, - "volume": 18127 - }, - "id": 2751841, - "non_hive": { - "close": 2150, - "high": 73, - "low": 2150, - "open": 73, - "volume": 2784 - }, - "open": "2020-10-23T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 262451, - "high": 702, - "low": 9362, - "open": 35000, - "volume": 335898 - }, - "id": 2751851, - "non_hive": { - "close": 40313, - "high": 108, - "low": 1438, - "open": 5376, - "volume": 51595 - }, - "open": "2020-10-23T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 928067, - "high": 928067, - "low": 67790, - "open": 134684, - "volume": 2443721 - }, - "id": 2751866, - "non_hive": { - "close": 142922, - "high": 142922, - "low": 10412, - "open": 20688, - "volume": 376218 - }, - "open": "2020-10-23T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 76447, - "high": 2072, - "low": 76447, - "open": 999990, - "volume": 12454514 - }, - "id": 2751881, - "non_hive": { - "close": 11622, - "high": 321, - "low": 11622, - "open": 154863, - "volume": 1926637 - }, - "open": "2020-10-23T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10622, - "high": 1930, - "low": 91, - "open": 12916, - "volume": 181992 - }, - "id": 2751912, - "non_hive": { - "close": 1645, - "high": 299, - "low": 13, - "open": 1999, - "volume": 28173 - }, - "open": "2020-10-23T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 65, - "high": 2085, - "low": 50217, - "open": 15807, - "volume": 203014 - }, - "id": 2751944, - "non_hive": { - "close": 10, - "high": 323, - "low": 7684, - "open": 2448, - "volume": 31322 - }, - "open": "2020-10-23T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12, - "high": 12, - "low": 170, - "open": 20609, - "volume": 397511 - }, - "id": 2751968, - "non_hive": { - "close": 2, - "high": 2, - "low": 26, - "open": 3190, - "volume": 61530 - }, - "open": "2020-10-23T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 81074, - "high": 38783, - "low": 2748, - "open": 13070, - "volume": 815156 - }, - "id": 2751992, - "non_hive": { - "close": 12169, - "high": 6000, - "low": 412, - "open": 2000, - "volume": 124425 - }, - "open": "2020-10-23T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1246, - "high": 765, - "low": 1747, - "open": 18035, - "volume": 211906 - }, - "id": 2752015, - "non_hive": { - "close": 192, - "high": 118, - "low": 269, - "open": 2780, - "volume": 32651 - }, - "open": "2020-10-23T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 901, - "high": 901, - "low": 80, - "open": 11729, - "volume": 27886 - }, - "id": 2752038, - "non_hive": { - "close": 139, - "high": 139, - "low": 12, - "open": 1807, - "volume": 4297 - }, - "open": "2020-10-23T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3996, - "high": 233, - "low": 1265, - "open": 18688, - "volume": 526186 - }, - "id": 2752056, - "non_hive": { - "close": 614, - "high": 36, - "low": 194, - "open": 2880, - "volume": 81000 - }, - "open": "2020-10-23T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 671, - "high": 11700, - "low": 18, - "open": 87152, - "volume": 3426406 - }, - "id": 2752084, - "non_hive": { - "close": 103, - "high": 1812, - "low": 2, - "open": 13407, - "volume": 529892 - }, - "open": "2020-10-23T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 136522, - "high": 12883, - "low": 136522, - "open": 2601, - "volume": 987027 - }, - "id": 2752118, - "non_hive": { - "close": 20943, - "high": 1993, - "low": 20943, - "open": 402, - "volume": 152329 - }, - "open": "2020-10-23T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12996, - "high": 601, - "low": 7, - "open": 16003, - "volume": 63944 - }, - "id": 2752157, - "non_hive": { - "close": 2008, - "high": 93, - "low": 1, - "open": 2474, - "volume": 9880 - }, - "open": "2020-10-23T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13009, - "high": 51, - "low": 83421, - "open": 12299, - "volume": 444271 - }, - "id": 2752174, - "non_hive": { - "close": 1999, - "high": 8, - "low": 12680, - "open": 1901, - "volume": 68096 - }, - "open": "2020-10-23T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34, - "high": 97, - "low": 34, - "open": 12992, - "volume": 405172 - }, - "id": 2752203, - "non_hive": { - "close": 5, - "high": 15, - "low": 5, - "open": 1999, - "volume": 62137 - }, - "open": "2020-10-23T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 832, - "high": 130, - "low": 313, - "open": 7782, - "volume": 263745 - }, - "id": 2752229, - "non_hive": { - "close": 127, - "high": 20, - "low": 47, - "open": 1193, - "volume": 40191 - }, - "open": "2020-10-23T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 500, - "high": 189458, - "low": 12, - "open": 14997, - "volume": 534280 - }, - "id": 2752276, - "non_hive": { - "close": 75, - "high": 29000, - "low": 1, - "open": 2295, - "volume": 81106 - }, - "open": "2020-10-23T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17518, - "high": 17820, - "low": 23, - "open": 4915, - "volume": 452303 - }, - "id": 2752308, - "non_hive": { - "close": 2676, - "high": 2724, - "low": 3, - "open": 751, - "volume": 68687 - }, - "open": "2020-10-23T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 66313, - "high": 726, - "low": 323374, - "open": 32729, - "volume": 917384 - }, - "id": 2752333, - "non_hive": { - "close": 10075, - "high": 111, - "low": 48510, - "open": 5000, - "volume": 138953 - }, - "open": "2020-10-23T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6, - "high": 6, - "low": 14515, - "open": 6551, - "volume": 38266 - }, - "id": 2752362, - "non_hive": { - "close": 1, - "high": 1, - "low": 2212, - "open": 1000, - "volume": 5834 - }, - "open": "2020-10-23T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10237, - "high": 33971, - "low": 10237, - "open": 9707, - "volume": 57436 - }, - "id": 2752380, - "non_hive": { - "close": 1561, - "high": 5186, - "low": 1561, - "open": 1481, - "volume": 8765 - }, - "open": "2020-10-23T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14452, - "high": 712, - "low": 14452, - "open": 10860, - "volume": 2055315 - }, - "id": 2752390, - "non_hive": { - "close": 2183, - "high": 109, - "low": 2183, - "open": 1658, - "volume": 313337 - }, - "open": "2020-10-23T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12500, - "high": 6024, - "low": 6000, - "open": 6024, - "volume": 73528 - }, - "id": 2752407, - "non_hive": { - "close": 1911, - "high": 921, - "low": 917, - "open": 921, - "volume": 11241 - }, - "open": "2020-10-24T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46729, - "high": 9942, - "low": 183, - "open": 9942, - "volume": 490647 - }, - "id": 2752423, - "non_hive": { - "close": 7143, - "high": 1520, - "low": 27, - "open": 1520, - "volume": 74198 - }, - "open": "2020-10-24T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3604, - "high": 3604, - "low": 78, - "open": 37924, - "volume": 61250 - }, - "id": 2752436, - "non_hive": { - "close": 551, - "high": 551, - "low": 11, - "open": 5797, - "volume": 9362 - }, - "open": "2020-10-24T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 115273, - "high": 13, - "low": 12, - "open": 3920, - "volume": 468730 - }, - "id": 2752448, - "non_hive": { - "close": 17613, - "high": 2, - "low": 1, - "open": 599, - "volume": 71490 - }, - "open": "2020-10-24T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 344, - "high": 569, - "low": 344, - "open": 71725, - "volume": 257234 - }, - "id": 2752497, - "non_hive": { - "close": 51, - "high": 87, - "low": 51, - "open": 10959, - "volume": 39228 - }, - "open": "2020-10-24T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13093, - "high": 13276, - "low": 2533, - "open": 6265, - "volume": 413136 - }, - "id": 2752528, - "non_hive": { - "close": 1964, - "high": 2028, - "low": 379, - "open": 957, - "volume": 62780 - }, - "open": "2020-10-24T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 47800, - "high": 14420, - "low": 47800, - "open": 22813, - "volume": 515524 - }, - "id": 2752544, - "non_hive": { - "close": 7128, - "high": 2204, - "low": 7128, - "open": 3422, - "volume": 77827 - }, - "open": "2020-10-24T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2995, - "high": 52, - "low": 31835, - "open": 52, - "volume": 306882 - }, - "id": 2752571, - "non_hive": { - "close": 457, - "high": 8, - "low": 4618, - "open": 8, - "volume": 45503 - }, - "open": "2020-10-24T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 75500, - "high": 11665, - "low": 75500, - "open": 3065, - "volume": 228737 - }, - "id": 2752594, - "non_hive": { - "close": 10965, - "high": 1778, - "low": 10965, - "open": 467, - "volume": 34011 - }, - "open": "2020-10-24T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111, - "high": 14683, - "low": 111, - "open": 13126, - "volume": 43600 - }, - "id": 2752610, - "non_hive": { - "close": 16, - "high": 2237, - "low": 16, - "open": 1999, - "volume": 6639 - }, - "open": "2020-10-24T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9838, - "high": 3279, - "low": 9, - "open": 1188, - "volume": 683690 - }, - "id": 2752617, - "non_hive": { - "close": 1498, - "high": 500, - "low": 1, - "open": 181, - "volume": 104162 - }, - "open": "2020-10-24T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42857, - "high": 13167, - "low": 42857, - "open": 13167, - "volume": 281307 - }, - "id": 2752641, - "non_hive": { - "close": 6501, - "high": 1999, - "low": 6501, - "open": 1999, - "volume": 42677 - }, - "open": "2020-10-24T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1124, - "high": 13183, - "low": 13183, - "open": 13183, - "volume": 263203 - }, - "id": 2752663, - "non_hive": { - "close": 170, - "high": 2000, - "low": 1993, - "open": 2000, - "volume": 39828 - }, - "open": "2020-10-24T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 35017, - "high": 570, - "low": 483985, - "open": 4102, - "volume": 824003 - }, - "id": 2752670, - "non_hive": { - "close": 5268, - "high": 87, - "low": 72811, - "open": 620, - "volume": 124332 - }, - "open": "2020-10-24T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 157, - "high": 157, - "low": 13142, - "open": 13142, - "volume": 53831 - }, - "id": 2752704, - "non_hive": { - "close": 24, - "high": 24, - "low": 1999, - "open": 1999, - "volume": 8191 - }, - "open": "2020-10-24T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2105, - "high": 10000, - "low": 12959, - "open": 12959, - "volume": 99003 - }, - "id": 2752715, - "non_hive": { - "close": 321, - "high": 1525, - "low": 1975, - "open": 1975, - "volume": 15096 - }, - "open": "2020-10-24T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4164, - "high": 7803, - "low": 4164, - "open": 77998, - "volume": 1143466 - }, - "id": 2752725, - "non_hive": { - "close": 632, - "high": 1190, - "low": 632, - "open": 11894, - "volume": 174341 - }, - "open": "2020-10-24T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9607, - "high": 380, - "low": 19701, - "open": 46653, - "volume": 312604 - }, - "id": 2752765, - "non_hive": { - "close": 1465, - "high": 58, - "low": 2985, - "open": 7114, - "volume": 47572 - }, - "open": "2020-10-24T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21550, - "high": 45806, - "low": 3211, - "open": 45806, - "volume": 338354 - }, - "id": 2752796, - "non_hive": { - "close": 3286, - "high": 6985, - "low": 486, - "open": 6985, - "volume": 51429 - }, - "open": "2020-10-24T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 158476, - "high": 786, - "low": 21510, - "open": 9186, - "volume": 559542 - }, - "id": 2752836, - "non_hive": { - "close": 24166, - "high": 120, - "low": 3278, - "open": 1400, - "volume": 85309 - }, - "open": "2020-10-24T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7082, - "high": 33621, - "low": 7082, - "open": 15004, - "volume": 363573 - }, - "id": 2752868, - "non_hive": { - "close": 1077, - "high": 5127, - "low": 1077, - "open": 2288, - "volume": 55393 - }, - "open": "2020-10-24T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8543, - "high": 2387, - "low": 334, - "open": 671, - "volume": 296639 - }, - "id": 2752889, - "non_hive": { - "close": 1302, - "high": 364, - "low": 50, - "open": 102, - "volume": 45080 - }, - "open": "2020-10-24T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 164, - "high": 190, - "low": 1569, - "open": 1569, - "volume": 259614 - }, - "id": 2752933, - "non_hive": { - "close": 25, - "high": 29, - "low": 239, - "open": 239, - "volume": 39583 - }, - "open": "2020-10-24T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1674, - "high": 6497, - "low": 16681, - "open": 28568, - "volume": 1539052 - }, - "id": 2752950, - "non_hive": { - "close": 256, - "high": 998, - "low": 2504, - "open": 4354, - "volume": 235690 - }, - "open": "2020-10-24T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9232, - "high": 5987, - "low": 6099, - "open": 5987, - "volume": 53486 - }, - "id": 2752985, - "non_hive": { - "close": 1421, - "high": 923, - "low": 935, - "open": 923, - "volume": 8212 - }, - "open": "2020-10-25T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13022, - "high": 4752, - "low": 10, - "open": 3756, - "volume": 452227 - }, - "id": 2753004, - "non_hive": { - "close": 2000, - "high": 734, - "low": 1, - "open": 578, - "volume": 69821 - }, - "open": "2020-10-25T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 152695, - "high": 12994, - "low": 16, - "open": 13008, - "volume": 481531 - }, - "id": 2753034, - "non_hive": { - "close": 23115, - "high": 2000, - "low": 2, - "open": 2000, - "volume": 73543 - }, - "open": "2020-10-25T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 899, - "high": 488115, - "low": 98956, - "open": 49999, - "volume": 650898 - }, - "id": 2753066, - "non_hive": { - "close": 136, - "high": 73886, - "low": 14862, - "open": 7568, - "volume": 98401 - }, - "open": "2020-10-25T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2995, - "high": 10217, - "low": 14, - "open": 12316, - "volume": 119019 - }, - "id": 2753076, - "non_hive": { - "close": 459, - "high": 1567, - "low": 2, - "open": 1863, - "volume": 18149 - }, - "open": "2020-10-25T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16201, - "high": 16201, - "low": 7080, - "open": 7080, - "volume": 305417 - }, - "id": 2753096, - "non_hive": { - "close": 2484, - "high": 2484, - "low": 1075, - "open": 1075, - "volume": 46754 - }, - "open": "2020-10-25T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18112, - "high": 31965, - "low": 89, - "open": 31965, - "volume": 314663 - }, - "id": 2753109, - "non_hive": { - "close": 2757, - "high": 4901, - "low": 13, - "open": 4901, - "volume": 47934 - }, - "open": "2020-10-25T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7490, - "high": 6552, - "low": 7490, - "open": 13146, - "volume": 416963 - }, - "id": 2753152, - "non_hive": { - "close": 1126, - "high": 998, - "low": 1126, - "open": 1999, - "volume": 63159 - }, - "open": "2020-10-25T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12395, - "high": 748, - "low": 2211, - "open": 13211, - "volume": 443618 - }, - "id": 2753185, - "non_hive": { - "close": 1875, - "high": 114, - "low": 332, - "open": 2000, - "volume": 66835 - }, - "open": "2020-10-25T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1633, - "high": 13, - "low": 4595, - "open": 186, - "volume": 293006 - }, - "id": 2753217, - "non_hive": { - "close": 248, - "high": 2, - "low": 689, - "open": 28, - "volume": 44240 - }, - "open": "2020-10-25T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6586, - "high": 3253, - "low": 3336, - "open": 13737, - "volume": 809183 - }, - "id": 2753251, - "non_hive": { - "close": 1000, - "high": 494, - "low": 506, - "open": 2086, - "volume": 122876 - }, - "open": "2020-10-25T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3464, - "high": 322, - "low": 754, - "open": 2246, - "volume": 81724 - }, - "id": 2753274, - "non_hive": { - "close": 526, - "high": 49, - "low": 114, - "open": 341, - "volume": 12409 - }, - "open": "2020-10-25T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4781, - "high": 210, - "low": 2209, - "open": 671, - "volume": 31435 - }, - "id": 2753298, - "non_hive": { - "close": 726, - "high": 32, - "low": 335, - "open": 102, - "volume": 4773 - }, - "open": "2020-10-25T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 186653, - "high": 13, - "low": 29905, - "open": 691, - "volume": 420606 - }, - "id": 2753314, - "non_hive": { - "close": 28523, - "high": 2, - "low": 4540, - "open": 105, - "volume": 64168 - }, - "open": "2020-10-25T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1695, - "high": 2925, - "low": 1695, - "open": 2925, - "volume": 69701 - }, - "id": 2753335, - "non_hive": { - "close": 259, - "high": 447, - "low": 259, - "open": 447, - "volume": 10651 - }, - "open": "2020-10-25T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13159, - "high": 45818, - "low": 72, - "open": 13783, - "volume": 660552 - }, - "id": 2753348, - "non_hive": { - "close": 1999, - "high": 7001, - "low": 10, - "open": 2106, - "volume": 100702 - }, - "open": "2020-10-25T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14050, - "high": 46, - "low": 14050, - "open": 5574, - "volume": 448205 - }, - "id": 2753396, - "non_hive": { - "close": 2104, - "high": 7, - "low": 2104, - "open": 846, - "volume": 67485 - }, - "open": "2020-10-25T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10185, - "high": 133587, - "low": 13266, - "open": 44423, - "volume": 3879463 - }, - "id": 2753417, - "non_hive": { - "close": 1556, - "high": 20688, - "low": 1999, - "open": 6704, - "volume": 592447 - }, - "open": "2020-10-25T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13122, - "high": 8487, - "low": 15, - "open": 2900, - "volume": 788981 - }, - "id": 2753466, - "non_hive": { - "close": 1999, - "high": 1310, - "low": 2, - "open": 443, - "volume": 120453 - }, - "open": "2020-10-25T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74996, - "high": 13, - "low": 716, - "open": 74999, - "volume": 648368 - }, - "id": 2753517, - "non_hive": { - "close": 11426, - "high": 2, - "low": 108, - "open": 11425, - "volume": 98788 - }, - "open": "2020-10-25T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2896, - "high": 1405, - "low": 3400, - "open": 13125, - "volume": 737663 - }, - "id": 2753573, - "non_hive": { - "close": 441, - "high": 215, - "low": 513, - "open": 2000, - "volume": 111876 - }, - "open": "2020-10-25T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13194, - "high": 90344, - "low": 1120, - "open": 12644, - "volume": 786572 - }, - "id": 2753607, - "non_hive": { - "close": 1999, - "high": 13822, - "low": 169, - "open": 1926, - "volume": 120028 - }, - "open": "2020-10-25T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5997, - "high": 14801, - "low": 5997, - "open": 14801, - "volume": 78678 - }, - "id": 2753637, - "non_hive": { - "close": 905, - "high": 2264, - "low": 905, - "open": 2264, - "volume": 11966 - }, - "open": "2020-10-25T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5711, - "high": 52, - "low": 1122, - "open": 13127, - "volume": 250400 - }, - "id": 2753654, - "non_hive": { - "close": 868, - "high": 8, - "low": 170, - "open": 1999, - "volume": 38090 - }, - "open": "2020-10-26T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6584, - "high": 657, - "low": 99, - "open": 13208, - "volume": 247380 - }, - "id": 2753686, - "non_hive": { - "close": 1000, - "high": 100, - "low": 14, - "open": 2000, - "volume": 37439 - }, - "open": "2020-10-26T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 271160, - "high": 271160, - "low": 74999, - "open": 60753, - "volume": 406912 - }, - "id": 2753702, - "non_hive": { - "close": 41184, - "high": 41184, - "low": 11390, - "open": 9227, - "volume": 61801 - }, - "open": "2020-10-26T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16923, - "high": 70320, - "low": 9959, - "open": 5540, - "volume": 467778 - }, - "id": 2753708, - "non_hive": { - "close": 2553, - "high": 10685, - "low": 1502, - "open": 841, - "volume": 70855 - }, - "open": "2020-10-26T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9670, - "high": 19, - "low": 7300, - "open": 26, - "volume": 128413 - }, - "id": 2753741, - "non_hive": { - "close": 1467, - "high": 3, - "low": 1105, - "open": 4, - "volume": 19461 - }, - "open": "2020-10-26T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 277, - "high": 13, - "low": 7, - "open": 1700, - "volume": 728438 - }, - "id": 2753769, - "non_hive": { - "close": 42, - "high": 2, - "low": 1, - "open": 256, - "volume": 109026 - }, - "open": "2020-10-26T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9862, - "high": 16372, - "low": 6000, - "open": 13056, - "volume": 277589 - }, - "id": 2753789, - "non_hive": { - "close": 1495, - "high": 2482, - "low": 909, - "open": 1978, - "volume": 42080 - }, - "open": "2020-10-26T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 178, - "high": 593, - "low": 158, - "open": 23842, - "volume": 123831 - }, - "id": 2753806, - "non_hive": { - "close": 27, - "high": 90, - "low": 23, - "open": 3614, - "volume": 18752 - }, - "open": "2020-10-26T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18968, - "high": 19771, - "low": 5711, - "open": 19771, - "volume": 232263 - }, - "id": 2753830, - "non_hive": { - "close": 2874, - "high": 2997, - "low": 855, - "open": 2997, - "volume": 35118 - }, - "open": "2020-10-26T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6503, - "high": 13, - "low": 6601, - "open": 24052, - "volume": 1122152 - }, - "id": 2753852, - "non_hive": { - "close": 968, - "high": 2, - "low": 967, - "open": 3644, - "volume": 167694 - }, - "open": "2020-10-26T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6450, - "high": 6450, - "low": 58, - "open": 7, - "volume": 279832 - }, - "id": 2753891, - "non_hive": { - "close": 973, - "high": 973, - "low": 8, - "open": 1, - "volume": 41711 - }, - "open": "2020-10-26T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12646, - "high": 13662, - "low": 104, - "open": 1552, - "volume": 358019 - }, - "id": 2753925, - "non_hive": { - "close": 1907, - "high": 2071, - "low": 15, - "open": 235, - "volume": 54148 - }, - "open": "2020-10-26T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74994, - "high": 6, - "low": 23140, - "open": 13194, - "volume": 304528 - }, - "id": 2753948, - "non_hive": { - "close": 11360, - "high": 1, - "low": 3490, - "open": 1999, - "volume": 46057 - }, - "open": "2020-10-26T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 422088, - "high": 164, - "low": 65104, - "open": 68064, - "volume": 5854254 - }, - "id": 2753970, - "non_hive": { - "close": 63702, - "high": 25, - "low": 9700, - "open": 10311, - "volume": 888032 - }, - "open": "2020-10-26T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13297, - "high": 377, - "low": 1000, - "open": 7000, - "volume": 367191 - }, - "id": 2754031, - "non_hive": { - "close": 1999, - "high": 57, - "low": 149, - "open": 1056, - "volume": 55022 - }, - "open": "2020-10-26T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24485, - "high": 15443, - "low": 3346, - "open": 9958, - "volume": 518463 - }, - "id": 2754072, - "non_hive": { - "close": 3679, - "high": 2323, - "low": 495, - "open": 1497, - "volume": 77603 - }, - "open": "2020-10-26T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4950, - "high": 638, - "low": 2282, - "open": 20000, - "volume": 305268 - }, - "id": 2754105, - "non_hive": { - "close": 744, - "high": 96, - "low": 337, - "open": 3005, - "volume": 45817 - }, - "open": "2020-10-26T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3436, - "high": 33, - "low": 650, - "open": 9712, - "volume": 1812524 - }, - "id": 2754143, - "non_hive": { - "close": 515, - "high": 5, - "low": 96, - "open": 1461, - "volume": 270877 - }, - "open": "2020-10-26T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8004, - "high": 2028, - "low": 1079, - "open": 7051, - "volume": 2198507 - }, - "id": 2754215, - "non_hive": { - "close": 1188, - "high": 302, - "low": 158, - "open": 1049, - "volume": 326007 - }, - "open": "2020-10-26T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7119, - "high": 1288, - "low": 39944, - "open": 4515, - "volume": 581599 - }, - "id": 2754265, - "non_hive": { - "close": 1042, - "high": 192, - "low": 5654, - "open": 670, - "volume": 84786 - }, - "open": "2020-10-26T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12175, - "high": 5831, - "low": 7102, - "open": 7102, - "volume": 793390 - }, - "id": 2754295, - "non_hive": { - "close": 1837, - "high": 880, - "low": 1043, - "open": 1043, - "volume": 118876 - }, - "open": "2020-10-26T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 75996, - "high": 1716, - "low": 75996, - "open": 12899, - "volume": 1737273 - }, - "id": 2754320, - "non_hive": { - "close": 10913, - "high": 259, - "low": 10913, - "open": 1946, - "volume": 259063 - }, - "open": "2020-10-26T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7117, - "high": 47, - "low": 138997, - "open": 9916, - "volume": 274142 - }, - "id": 2754371, - "non_hive": { - "close": 1038, - "high": 7, - "low": 19960, - "open": 1424, - "volume": 39424 - }, - "open": "2020-10-26T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10074, - "high": 609994, - "low": 10074, - "open": 7147, - "volume": 1364400 - }, - "id": 2754387, - "non_hive": { - "close": 1472, - "high": 91102, - "low": 1472, - "open": 1047, - "volume": 203276 - }, - "open": "2020-10-26T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4095, - "high": 92419, - "low": 7112, - "open": 7112, - "volume": 235155 - }, - "id": 2754407, - "non_hive": { - "close": 611, - "high": 13801, - "low": 1049, - "open": 1049, - "volume": 35070 - }, - "open": "2020-10-27T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 74999, - "high": 5363, - "low": 400, - "open": 75000, - "volume": 822542 - }, - "id": 2754424, - "non_hive": { - "close": 11161, - "high": 801, - "low": 59, - "open": 11199, - "volume": 122753 - }, - "open": "2020-10-27T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5484, - "high": 30821, - "low": 6817, - "open": 13401, - "volume": 91445 - }, - "id": 2754455, - "non_hive": { - "close": 809, - "high": 4600, - "low": 997, - "open": 1999, - "volume": 13552 - }, - "open": "2020-10-27T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13656, - "high": 47, - "low": 2453, - "open": 1628, - "volume": 1195045 - }, - "id": 2754464, - "non_hive": { - "close": 1999, - "high": 7, - "low": 358, - "open": 240, - "volume": 175054 - }, - "open": "2020-10-27T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10432, - "high": 6, - "low": 10432, - "open": 17994, - "volume": 744960 - }, - "id": 2754489, - "non_hive": { - "close": 1500, - "high": 1, - "low": 1500, - "open": 2634, - "volume": 108046 - }, - "open": "2020-10-27T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40000, - "high": 40000, - "low": 4014, - "open": 9295, - "volume": 349295 - }, - "id": 2754513, - "non_hive": { - "close": 5797, - "high": 5797, - "low": 577, - "open": 1347, - "volume": 50605 - }, - "open": "2020-10-27T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 86347, - "high": 282, - "low": 3359, - "open": 282, - "volume": 298225 - }, - "id": 2754525, - "non_hive": { - "close": 12500, - "high": 41, - "low": 486, - "open": 41, - "volume": 43182 - }, - "open": "2020-10-27T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17981, - "high": 6, - "low": 19, - "open": 849, - "volume": 565995 - }, - "id": 2754547, - "non_hive": { - "close": 2603, - "high": 1, - "low": 2, - "open": 123, - "volume": 81584 - }, - "open": "2020-10-27T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 38920, - "high": 38920, - "low": 211, - "open": 19973, - "volume": 419648 - }, - "id": 2754590, - "non_hive": { - "close": 5635, - "high": 5635, - "low": 30, - "open": 2880, - "volume": 60519 - }, - "open": "2020-10-27T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4435, - "high": 64989, - "low": 14, - "open": 58204, - "volume": 417507 - }, - "id": 2754625, - "non_hive": { - "close": 642, - "high": 9410, - "low": 2, - "open": 8427, - "volume": 60447 - }, - "open": "2020-10-27T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55713, - "high": 1623, - "low": 2678, - "open": 5430, - "volume": 1305105 - }, - "id": 2754642, - "non_hive": { - "close": 8000, - "high": 235, - "low": 384, - "open": 786, - "volume": 188763 - }, - "open": "2020-10-27T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17338, - "high": 13, - "low": 9365, - "open": 6498, - "volume": 107901 - }, - "id": 2754672, - "non_hive": { - "close": 2500, - "high": 2, - "low": 1344, - "open": 937, - "volume": 15549 - }, - "open": "2020-10-27T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55606, - "high": 719113, - "low": 4560, - "open": 104, - "volume": 1087505 - }, - "id": 2754692, - "non_hive": { - "close": 8042, - "high": 104117, - "low": 654, - "open": 15, - "volume": 157350 - }, - "open": "2020-10-27T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 436964, - "high": 436964, - "low": 973, - "open": 13823, - "volume": 774528 - }, - "id": 2754726, - "non_hive": { - "close": 63228, - "high": 63228, - "low": 140, - "open": 1999, - "volume": 112065 - }, - "open": "2020-10-27T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6654, - "high": 4146, - "low": 2131, - "open": 13124, - "volume": 157552 - }, - "id": 2754750, - "non_hive": { - "close": 962, - "high": 600, - "low": 308, - "open": 1899, - "volume": 22791 - }, - "open": "2020-10-27T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23128, - "high": 262, - "low": 23128, - "open": 14003, - "volume": 288923 - }, - "id": 2754774, - "non_hive": { - "close": 3318, - "high": 38, - "low": 3318, - "open": 2025, - "volume": 41731 - }, - "open": "2020-10-27T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13870, - "high": 30666, - "low": 36428, - "open": 6619, - "volume": 1104709 - }, - "id": 2754807, - "non_hive": { - "close": 1999, - "high": 4423, - "low": 5212, - "open": 954, - "volume": 158871 - }, - "open": "2020-10-27T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60875, - "high": 34, - "low": 207, - "open": 5378, - "volume": 2404920 - }, - "id": 2754867, - "non_hive": { - "close": 8618, - "high": 5, - "low": 29, - "open": 775, - "volume": 345100 - }, - "open": "2020-10-27T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 90779, - "high": 1263, - "low": 16007, - "open": 1263, - "volume": 598608 - }, - "id": 2754935, - "non_hive": { - "close": 12850, - "high": 181, - "low": 2265, - "open": 181, - "volume": 84936 - }, - "open": "2020-10-27T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 63000, - "high": 49, - "low": 14004, - "open": 14004, - "volume": 679107 - }, - "id": 2754971, - "non_hive": { - "close": 8998, - "high": 7, - "low": 1999, - "open": 1999, - "volume": 96990 - }, - "open": "2020-10-27T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12905, - "high": 2044, - "low": 12905, - "open": 40454, - "volume": 729267 - }, - "id": 2754991, - "non_hive": { - "close": 1826, - "high": 292, - "low": 1826, - "open": 5778, - "volume": 104085 - }, - "open": "2020-10-27T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60873, - "high": 202584, - "low": 28505, - "open": 59000, - "volume": 705182 - }, - "id": 2755023, - "non_hive": { - "close": 8617, - "high": 29211, - "low": 4035, - "open": 8417, - "volume": 100710 - }, - "open": "2020-10-27T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 726164, - "high": 726164, - "low": 21, - "open": 196, - "volume": 2735289 - }, - "id": 2755049, - "non_hive": { - "close": 104710, - "high": 104710, - "low": 2, - "open": 28, - "volume": 391711 - }, - "open": "2020-10-27T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 670667, - "high": 12667, - "low": 670667, - "open": 14027, - "volume": 1709787 - }, - "id": 2755088, - "non_hive": { - "close": 94772, - "high": 1815, - "low": 94772, - "open": 1999, - "volume": 242456 - }, - "open": "2020-10-27T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6113, - "high": 11216, - "low": 7363, - "open": 14048, - "volume": 175782 - }, - "id": 2755123, - "non_hive": { - "close": 870, - "high": 1603, - "low": 1040, - "open": 1999, - "volume": 25020 - }, - "open": "2020-10-28T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 344, - "high": 344, - "low": 7935, - "open": 7935, - "volume": 8279 - }, - "id": 2755142, - "non_hive": { - "close": 49, - "high": 49, - "low": 1129, - "open": 1129, - "volume": 1178 - }, - "open": "2020-10-28T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10869, - "high": 4424, - "low": 598, - "open": 6982, - "volume": 368652 - }, - "id": 2755149, - "non_hive": { - "close": 1545, - "high": 638, - "low": 85, - "open": 994, - "volume": 52699 - }, - "open": "2020-10-28T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1216, - "high": 3084, - "low": 227, - "open": 13958, - "volume": 140525 - }, - "id": 2755183, - "non_hive": { - "close": 175, - "high": 444, - "low": 32, - "open": 1999, - "volume": 20165 - }, - "open": "2020-10-28T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13950, - "high": 6441, - "low": 13950, - "open": 74999, - "volume": 162602 - }, - "id": 2755207, - "non_hive": { - "close": 2000, - "high": 927, - "low": 2000, - "open": 10792, - "volume": 23386 - }, - "open": "2020-10-28T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13962, - "high": 13, - "low": 137, - "open": 70003, - "volume": 504404 - }, - "id": 2755224, - "non_hive": { - "close": 1999, - "high": 2, - "low": 19, - "open": 10065, - "volume": 72387 - }, - "open": "2020-10-28T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 18366, - "high": 14, - "low": 7455, - "open": 14046, - "volume": 1778655 - }, - "id": 2755254, - "non_hive": { - "close": 2571, - "high": 2, - "low": 1039, - "open": 2000, - "volume": 249900 - }, - "open": "2020-10-28T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 58150, - "high": 42, - "low": 14380, - "open": 17651, - "volume": 441201 - }, - "id": 2755277, - "non_hive": { - "close": 8077, - "high": 6, - "low": 1997, - "open": 2471, - "volume": 61455 - }, - "open": "2020-10-28T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21216, - "high": 21216, - "low": 13283, - "open": 13283, - "volume": 34499 - }, - "id": 2755300, - "non_hive": { - "close": 2947, - "high": 2947, - "low": 1845, - "open": 1845, - "volume": 4792 - }, - "open": "2020-10-28T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14, - "high": 14, - "low": 237216, - "open": 6076, - "volume": 860495 - }, - "id": 2755307, - "non_hive": { - "close": 2, - "high": 2, - "low": 32949, - "open": 844, - "volume": 120394 - }, - "open": "2020-10-28T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24510, - "high": 24510, - "low": 96, - "open": 199, - "volume": 101266 - }, - "id": 2755327, - "non_hive": { - "close": 3520, - "high": 3520, - "low": 13, - "open": 28, - "volume": 14393 - }, - "open": "2020-10-28T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 234, - "high": 4349, - "low": 3897, - "open": 4349, - "volume": 485733 - }, - "id": 2755338, - "non_hive": { - "close": 33, - "high": 620, - "low": 547, - "open": 620, - "volume": 68423 - }, - "open": "2020-10-28T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2893, - "high": 14, - "low": 2893, - "open": 81846, - "volume": 394907 - }, - "id": 2755355, - "non_hive": { - "close": 405, - "high": 2, - "low": 405, - "open": 11508, - "volume": 55468 - }, - "open": "2020-10-28T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28589, - "high": 2306, - "low": 456, - "open": 11391, - "volume": 1285658 - }, - "id": 2755382, - "non_hive": { - "close": 4000, - "high": 323, - "low": 63, - "open": 1595, - "volume": 179896 - }, - "open": "2020-10-28T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 341, - "high": 341, - "low": 14305, - "open": 70663, - "volume": 2830873 - }, - "id": 2755434, - "non_hive": { - "close": 48, - "high": 48, - "low": 1999, - "open": 9880, - "volume": 397521 - }, - "open": "2020-10-28T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7402, - "high": 42, - "low": 393754, - "open": 13417, - "volume": 1389095 - }, - "id": 2755473, - "non_hive": { - "close": 1039, - "high": 6, - "low": 54877, - "open": 1885, - "volume": 194617 - }, - "open": "2020-10-28T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 857, - "high": 363, - "low": 857, - "open": 11598, - "volume": 445635 - }, - "id": 2755512, - "non_hive": { - "close": 120, - "high": 51, - "low": 120, - "open": 1628, - "volume": 62549 - }, - "open": "2020-10-28T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7540, - "high": 14, - "low": 7540, - "open": 14325, - "volume": 1507504 - }, - "id": 2755545, - "non_hive": { - "close": 1032, - "high": 2, - "low": 1032, - "open": 2000, - "volume": 209549 - }, - "open": "2020-10-28T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14146, - "high": 23449, - "low": 66536, - "open": 21751, - "volume": 2940112 - }, - "id": 2755578, - "non_hive": { - "close": 2000, - "high": 3343, - "low": 9170, - "open": 3000, - "volume": 410570 - }, - "open": "2020-10-28T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14293, - "high": 274, - "low": 108, - "open": 274, - "volume": 3816054 - }, - "id": 2755667, - "non_hive": { - "close": 1999, - "high": 39, - "low": 15, - "open": 39, - "volume": 534002 - }, - "open": "2020-10-28T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7542, - "high": 3592, - "low": 7542, - "open": 3592, - "volume": 693562 - }, - "id": 2755717, - "non_hive": { - "close": 1037, - "high": 503, - "low": 1037, - "open": 503, - "volume": 96560 - }, - "open": "2020-10-28T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14496, - "high": 21, - "low": 166430, - "open": 72, - "volume": 3192132 - }, - "id": 2755749, - "non_hive": { - "close": 2022, - "high": 3, - "low": 22490, - "open": 10, - "volume": 435010 - }, - "open": "2020-10-28T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25106, - "high": 2932, - "low": 25106, - "open": 14400, - "volume": 1392366 - }, - "id": 2755785, - "non_hive": { - "close": 3391, - "high": 409, - "low": 3391, - "open": 2000, - "volume": 190088 - }, - "open": "2020-10-28T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5244, - "high": 14, - "low": 118615, - "open": 14545, - "volume": 1511723 - }, - "id": 2755814, - "non_hive": { - "close": 710, - "high": 2, - "low": 16013, - "open": 1999, - "volume": 204989 - }, - "open": "2020-10-29T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8099, - "high": 8099, - "low": 1389, - "open": 1389, - "volume": 9488 - }, - "id": 2755843, - "non_hive": { - "close": 1104, - "high": 1104, - "low": 188, - "open": 188, - "volume": 1292 - }, - "open": "2020-10-29T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20058, - "high": 6232, - "low": 43, - "open": 6185, - "volume": 2007912 - }, - "id": 2755850, - "non_hive": { - "close": 2707, - "high": 857, - "low": 5, - "open": 843, - "volume": 271446 - }, - "open": "2020-10-29T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2062, - "high": 2831, - "low": 1355, - "open": 1355, - "volume": 16627 - }, - "id": 2755863, - "non_hive": { - "close": 281, - "high": 386, - "low": 184, - "open": 184, - "volume": 2265 - }, - "open": "2020-10-29T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 9243, - "high": 9243, - "low": 1267, - "open": 1267, - "volume": 11767 - }, - "id": 2755873, - "non_hive": { - "close": 1260, - "high": 1260, - "low": 172, - "open": 172, - "volume": 1603 - }, - "open": "2020-10-29T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3708, - "high": 163754, - "low": 3708, - "open": 5422, - "volume": 217780 - }, - "id": 2755883, - "non_hive": { - "close": 500, - "high": 22332, - "low": 500, - "open": 739, - "volume": 29690 - }, - "open": "2020-10-29T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6725, - "high": 2923, - "low": 3158, - "open": 7560, - "volume": 128099 - }, - "id": 2755895, - "non_hive": { - "close": 916, - "high": 400, - "low": 430, - "open": 1031, - "volume": 17474 - }, - "open": "2020-10-29T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 39444, - "high": 5652, - "low": 444000, - "open": 14663, - "volume": 664337 - }, - "id": 2755919, - "non_hive": { - "close": 5325, - "high": 771, - "low": 59940, - "open": 1999, - "volume": 89747 - }, - "open": "2020-10-29T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14873, - "high": 503, - "low": 1000, - "open": 41488, - "volume": 571037 - }, - "id": 2755942, - "non_hive": { - "close": 1999, - "high": 68, - "low": 134, - "open": 5601, - "volume": 76984 - }, - "open": "2020-10-29T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3944, - "high": 2327, - "low": 2000, - "open": 8809, - "volume": 41802 - }, - "id": 2755990, - "non_hive": { - "close": 532, - "high": 314, - "low": 268, - "open": 1188, - "volume": 5636 - }, - "open": "2020-10-29T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5000, - "high": 107131, - "low": 11300, - "open": 36629, - "volume": 1030228 - }, - "id": 2756011, - "non_hive": { - "close": 674, - "high": 14452, - "low": 1519, - "open": 4940, - "volume": 138968 - }, - "open": "2020-10-29T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43353, - "high": 33988, - "low": 853, - "open": 33988, - "volume": 291349 - }, - "id": 2756029, - "non_hive": { - "close": 5831, - "high": 4585, - "low": 114, - "open": 4585, - "volume": 39107 - }, - "open": "2020-10-29T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 215738, - "high": 215738, - "low": 8854, - "open": 468, - "volume": 310650 - }, - "id": 2756052, - "non_hive": { - "close": 29103, - "high": 29103, - "low": 1190, - "open": 63, - "volume": 41893 - }, - "open": "2020-10-29T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14813, - "high": 5303, - "low": 118342, - "open": 9925, - "volume": 1671948 - }, - "id": 2756065, - "non_hive": { - "close": 2000, - "high": 716, - "low": 15964, - "open": 1339, - "volume": 225699 - }, - "open": "2020-10-29T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7804, - "high": 3193, - "low": 12162, - "open": 14813, - "volume": 200366 - }, - "id": 2756090, - "non_hive": { - "close": 1055, - "high": 434, - "low": 1642, - "open": 2000, - "volume": 27169 - }, - "open": "2020-10-29T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8409, - "high": 2589, - "low": 234, - "open": 1376, - "volume": 1804748 - }, - "id": 2756112, - "non_hive": { - "close": 1134, - "high": 350, - "low": 31, - "open": 186, - "volume": 242362 - }, - "open": "2020-10-29T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1294, - "high": 576, - "low": 6416, - "open": 6416, - "volume": 110848 - }, - "id": 2756153, - "non_hive": { - "close": 175, - "high": 78, - "low": 865, - "open": 865, - "volume": 14982 - }, - "open": "2020-10-29T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111550, - "high": 37593, - "low": 47, - "open": 20890, - "volume": 517882 - }, - "id": 2756176, - "non_hive": { - "close": 15057, - "high": 5083, - "low": 6, - "open": 2823, - "volume": 69893 - }, - "open": "2020-10-29T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 72289, - "high": 2711, - "low": 810, - "open": 810, - "volume": 152312 - }, - "id": 2756204, - "non_hive": { - "close": 9757, - "high": 366, - "low": 108, - "open": 108, - "volume": 20515 - }, - "open": "2020-10-29T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 55907, - "high": 7123, - "low": 6803, - "open": 4650, - "volume": 453883 - }, - "id": 2756226, - "non_hive": { - "close": 7550, - "high": 962, - "low": 911, - "open": 628, - "volume": 61235 - }, - "open": "2020-10-29T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19720, - "high": 3376, - "low": 5454, - "open": 3000, - "volume": 416147 - }, - "id": 2756256, - "non_hive": { - "close": 2663, - "high": 456, - "low": 736, - "open": 405, - "volume": 56198 - }, - "open": "2020-10-29T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 797134, - "high": 525, - "low": 10348, - "open": 8657, - "volume": 1058310 - }, - "id": 2756282, - "non_hive": { - "close": 107645, - "high": 71, - "low": 1397, - "open": 1169, - "volume": 142913 - }, - "open": "2020-10-29T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4377, - "high": 96, - "low": 1684, - "open": 34406, - "volume": 279833 - }, - "id": 2756305, - "non_hive": { - "close": 591, - "high": 13, - "low": 227, - "open": 4646, - "volume": 37782 - }, - "open": "2020-10-29T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 629, - "high": 7, - "low": 8285, - "open": 563, - "volume": 344234 - }, - "id": 2756337, - "non_hive": { - "close": 85, - "high": 1, - "low": 1095, - "open": 76, - "volume": 46250 - }, - "open": "2020-10-30T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33721, - "high": 33721, - "low": 292, - "open": 37040, - "volume": 191124 - }, - "id": 2756364, - "non_hive": { - "close": 4552, - "high": 4552, - "low": 38, - "open": 5000, - "volume": 25777 - }, - "open": "2020-10-30T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13896, - "high": 2140, - "low": 11324, - "open": 11324, - "volume": 52307 - }, - "id": 2756387, - "non_hive": { - "close": 1872, - "high": 289, - "low": 1513, - "open": 1513, - "volume": 7042 - }, - "open": "2020-10-30T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6000, - "high": 15815, - "low": 1792, - "open": 15815, - "volume": 97395 - }, - "id": 2756402, - "non_hive": { - "close": 809, - "high": 2135, - "low": 241, - "open": 2135, - "volume": 13144 - }, - "open": "2020-10-30T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1799, - "high": 185, - "low": 14616, - "open": 2414, - "volume": 1240637 - }, - "id": 2756415, - "non_hive": { - "close": 243, - "high": 25, - "low": 1900, - "open": 326, - "volume": 162821 - }, - "open": "2020-10-30T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7416, - "high": 622, - "low": 386, - "open": 761, - "volume": 164841 - }, - "id": 2756442, - "non_hive": { - "close": 1000, - "high": 84, - "low": 50, - "open": 102, - "volume": 22108 - }, - "open": "2020-10-30T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7788, - "high": 41638, - "low": 160, - "open": 17231, - "volume": 379342 - }, - "id": 2756465, - "non_hive": { - "close": 1049, - "high": 5612, - "low": 20, - "open": 2321, - "volume": 50932 - }, - "open": "2020-10-30T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 701, - "high": 89, - "low": 7867, - "open": 9659, - "volume": 1095879 - }, - "id": 2756504, - "non_hive": { - "close": 94, - "high": 12, - "low": 983, - "open": 1301, - "volume": 142182 - }, - "open": "2020-10-30T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49785, - "high": 9321, - "low": 49785, - "open": 15, - "volume": 510732 - }, - "id": 2756554, - "non_hive": { - "close": 5974, - "high": 1251, - "low": 5974, - "open": 2, - "volume": 64308 - }, - "open": "2020-10-30T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6435, - "high": 106119, - "low": 944, - "open": 15266, - "volume": 167047 - }, - "id": 2756576, - "non_hive": { - "close": 859, - "high": 14196, - "low": 125, - "open": 2038, - "volume": 22332 - }, - "open": "2020-10-30T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 569, - "high": 14654, - "low": 9653, - "open": 14654, - "volume": 156472 - }, - "id": 2756593, - "non_hive": { - "close": 74, - "high": 1958, - "low": 1159, - "open": 1958, - "volume": 19978 - }, - "open": "2020-10-30T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1389, - "high": 17207, - "low": 4644, - "open": 15422, - "volume": 244748 - }, - "id": 2756611, - "non_hive": { - "close": 180, - "high": 2231, - "low": 601, - "open": 1999, - "volume": 31711 - }, - "open": "2020-10-30T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1343, - "high": 86003, - "low": 1343, - "open": 86003, - "volume": 151625 - }, - "id": 2756632, - "non_hive": { - "close": 167, - "high": 11132, - "low": 167, - "open": 11132, - "volume": 19583 - }, - "open": "2020-10-30T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4811, - "high": 24187, - "low": 120, - "open": 24187, - "volume": 130432 - }, - "id": 2756642, - "non_hive": { - "close": 614, - "high": 3094, - "low": 15, - "open": 3094, - "volume": 16665 - }, - "open": "2020-10-30T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17219, - "high": 16084, - "low": 16934, - "open": 5804, - "volume": 617291 - }, - "id": 2756672, - "non_hive": { - "close": 2200, - "high": 2058, - "low": 2162, - "open": 742, - "volume": 78954 - }, - "open": "2020-10-30T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2469, - "high": 62, - "low": 10072, - "open": 5790, - "volume": 103263 - }, - "id": 2756699, - "non_hive": { - "close": 316, - "high": 8, - "low": 1241, - "open": 739, - "volume": 13100 - }, - "open": "2020-10-30T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1250, - "high": 492, - "low": 1250, - "open": 492, - "volume": 2875977 - }, - "id": 2756726, - "non_hive": { - "close": 153, - "high": 63, - "low": 153, - "open": 63, - "volume": 361185 - }, - "open": "2020-10-30T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5222, - "high": 61114, - "low": 101, - "open": 14849, - "volume": 315140 - }, - "id": 2756772, - "non_hive": { - "close": 657, - "high": 7742, - "low": 12, - "open": 1867, - "volume": 39646 - }, - "open": "2020-10-30T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 65, - "high": 2337, - "low": 65, - "open": 15906, - "volume": 558175 - }, - "id": 2756809, - "non_hive": { - "close": 7, - "high": 299, - "low": 7, - "open": 2001, - "volume": 69968 - }, - "open": "2020-10-30T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 149282, - "high": 4175, - "low": 3968, - "open": 3968, - "volume": 506711 - }, - "id": 2756861, - "non_hive": { - "close": 19060, - "high": 534, - "low": 505, - "open": 505, - "volume": 64750 - }, - "open": "2020-10-30T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26984, - "high": 8288, - "low": 115001, - "open": 8288, - "volume": 856816 - }, - "id": 2756902, - "non_hive": { - "close": 3373, - "high": 1060, - "low": 14375, - "open": 1060, - "volume": 109034 - }, - "open": "2020-10-30T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 27078, - "high": 23344, - "low": 9598, - "open": 23344, - "volume": 103163 - }, - "id": 2756918, - "non_hive": { - "close": 3461, - "high": 2984, - "low": 1225, - "open": 2984, - "volume": 13184 - }, - "open": "2020-10-30T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15053, - "high": 15053, - "low": 1573, - "open": 9514, - "volume": 40975 - }, - "id": 2756937, - "non_hive": { - "close": 1924, - "high": 1924, - "low": 201, - "open": 1216, - "volume": 5237 - }, - "open": "2020-10-30T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 26409, - "high": 1682, - "low": 26409, - "open": 23473, - "volume": 675381 - }, - "id": 2756950, - "non_hive": { - "close": 3372, - "high": 215, - "low": 3372, - "open": 3000, - "volume": 86315 - }, - "open": "2020-10-30T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1235, - "high": 20001, - "low": 807970, - "open": 52252, - "volume": 2327454 - }, - "id": 2756968, - "non_hive": { - "close": 157, - "high": 2554, - "low": 99379, - "open": 6672, - "volume": 290022 - }, - "open": "2020-10-31T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3267, - "high": 28800, - "low": 3267, - "open": 8455, - "volume": 110640 - }, - "id": 2756996, - "non_hive": { - "close": 414, - "high": 3677, - "low": 414, - "open": 1079, - "volume": 14111 - }, - "open": "2020-10-31T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 92770, - "high": 7, - "low": 11721, - "open": 8000, - "volume": 372858 - }, - "id": 2757009, - "non_hive": { - "close": 11845, - "high": 1, - "low": 1441, - "open": 1021, - "volume": 47325 - }, - "open": "2020-10-31T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12490, - "high": 15, - "low": 44326, - "open": 117237, - "volume": 728764 - }, - "id": 2757034, - "non_hive": { - "close": 1594, - "high": 2, - "low": 5448, - "open": 14968, - "volume": 92325 - }, - "open": "2020-10-31T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23346, - "high": 28030, - "low": 4372, - "open": 28030, - "volume": 98986 - }, - "id": 2757059, - "non_hive": { - "close": 2979, - "high": 3577, - "low": 557, - "open": 3577, - "volume": 12629 - }, - "open": "2020-10-31T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2335, - "high": 2335, - "low": 4993, - "open": 4993, - "volume": 24107 - }, - "id": 2757072, - "non_hive": { - "close": 298, - "high": 298, - "low": 636, - "open": 636, - "volume": 3075 - }, - "open": "2020-10-31T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3916, - "high": 3916, - "low": 100, - "open": 100, - "volume": 260518 - }, - "id": 2757078, - "non_hive": { - "close": 500, - "high": 500, - "low": 12, - "open": 12, - "volume": 33242 - }, - "open": "2020-10-31T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10729, - "high": 3493, - "low": 10729, - "open": 3493, - "volume": 198021 - }, - "id": 2757091, - "non_hive": { - "close": 1318, - "high": 446, - "low": 1318, - "open": 446, - "volume": 24925 - }, - "open": "2020-10-31T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2062, - "high": 2062, - "low": 6224, - "open": 156879, - "volume": 180198 - }, - "id": 2757112, - "non_hive": { - "close": 263, - "high": 263, - "low": 793, - "open": 20000, - "volume": 22972 - }, - "open": "2020-10-31T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 110233, - "high": 1461, - "low": 38, - "open": 4547, - "volume": 823016 - }, - "id": 2757124, - "non_hive": { - "close": 13448, - "high": 183, - "low": 4, - "open": 569, - "volume": 101153 - }, - "open": "2020-10-31T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 34033, - "high": 175, - "low": 34033, - "open": 15967, - "volume": 557942 - }, - "id": 2757153, - "non_hive": { - "close": 3934, - "high": 22, - "low": 3934, - "open": 1999, - "volume": 67645 - }, - "open": "2020-10-31T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10000, - "high": 5705, - "low": 10000, - "open": 5705, - "volume": 91168 - }, - "id": 2757179, - "non_hive": { - "close": 1181, - "high": 713, - "low": 1181, - "open": 713, - "volume": 11314 - }, - "open": "2020-10-31T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13000, - "high": 30233, - "low": 3656, - "open": 3656, - "volume": 157188 - }, - "id": 2757193, - "non_hive": { - "close": 1623, - "high": 3778, - "low": 456, - "open": 456, - "volume": 19638 - }, - "open": "2020-10-31T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3742, - "high": 216, - "low": 3742, - "open": 4213, - "volume": 59663 - }, - "id": 2757208, - "non_hive": { - "close": 467, - "high": 27, - "low": 467, - "open": 526, - "volume": 7454 - }, - "open": "2020-10-31T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 510446, - "high": 7002, - "low": 6800, - "open": 56126, - "volume": 1729291 - }, - "id": 2757221, - "non_hive": { - "close": 63337, - "high": 875, - "low": 818, - "open": 7000, - "volume": 214656 - }, - "open": "2020-10-31T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 906, - "high": 906, - "low": 9179, - "open": 4561, - "volume": 869198 - }, - "id": 2757257, - "non_hive": { - "close": 116, - "high": 116, - "low": 1147, - "open": 570, - "volume": 109088 - }, - "open": "2020-10-31T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1489, - "high": 70, - "low": 20454, - "open": 13146, - "volume": 270304 - }, - "id": 2757293, - "non_hive": { - "close": 189, - "high": 9, - "low": 2567, - "open": 1680, - "volume": 34428 - }, - "open": "2020-10-31T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13043, - "high": 275, - "low": 16120, - "open": 2653, - "volume": 1315492 - }, - "id": 2757316, - "non_hive": { - "close": 1654, - "high": 35, - "low": 1999, - "open": 333, - "volume": 164011 - }, - "open": "2020-10-31T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 76009, - "high": 686, - "low": 6041, - "open": 1057, - "volume": 2817882 - }, - "id": 2757374, - "non_hive": { - "close": 9501, - "high": 87, - "low": 749, - "open": 134, - "volume": 351285 - }, - "open": "2020-10-31T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 95999, - "high": 5322, - "low": 7599999, - "open": 15999, - "volume": 22396365 - }, - "id": 2757436, - "non_hive": { - "close": 11998, - "high": 675, - "low": 927283, - "open": 2000, - "volume": 2776405 - }, - "open": "2020-10-31T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 16048, - "low": 24101, - "open": 15599, - "volume": 504418 - }, - "id": 2757495, - "non_hive": { - "close": 126, - "high": 2033, - "low": 2988, - "open": 1949, - "volume": 62986 - }, - "open": "2020-10-31T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2165, - "high": 784, - "low": 2165, - "open": 1015, - "volume": 572189 - }, - "id": 2757528, - "non_hive": { - "close": 268, - "high": 98, - "low": 268, - "open": 126, - "volume": 71083 - }, - "open": "2020-10-31T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2872, - "high": 45423, - "low": 1683, - "open": 297, - "volume": 286436 - }, - "id": 2757576, - "non_hive": { - "close": 362, - "high": 5749, - "low": 202, - "open": 37, - "volume": 35729 - }, - "open": "2020-10-31T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16257, - "high": 65195, - "low": 8500, - "open": 15861, - "volume": 2933389 - }, - "id": 2757604, - "non_hive": { - "close": 1999, - "high": 8240, - "low": 1045, - "open": 1999, - "volume": 366188 - }, - "open": "2020-10-31T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11860, - "high": 16593, - "low": 16018, - "open": 15702, - "volume": 777625 - }, - "id": 2757653, - "non_hive": { - "close": 1498, - "high": 2097, - "low": 1999, - "open": 1961, - "volume": 97305 - }, - "open": "2020-11-01T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1920, - "high": 7909, - "low": 14278, - "open": 79877, - "volume": 1490574 - }, - "id": 2757687, - "non_hive": { - "close": 242, - "high": 999, - "low": 1797, - "open": 10088, - "volume": 188249 - }, - "open": "2020-11-01T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 430000, - "high": 179, - "low": 11077, - "open": 29611, - "volume": 3666307 - }, - "id": 2757711, - "non_hive": { - "close": 53752, - "high": 23, - "low": 1384, - "open": 3742, - "volume": 461921 - }, - "open": "2020-11-01T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4943, - "high": 4943, - "low": 10255, - "open": 8935, - "volume": 24133 - }, - "id": 2757758, - "non_hive": { - "close": 632, - "high": 632, - "low": 1261, - "open": 1117, - "volume": 3010 - }, - "open": "2020-11-01T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 86100, - "high": 298444, - "low": 320824, - "open": 15649, - "volume": 1083498 - }, - "id": 2757764, - "non_hive": { - "close": 11011, - "high": 38171, - "low": 39461, - "open": 1999, - "volume": 136196 - }, - "open": "2020-11-01T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 338069, - "high": 117, - "low": 66, - "open": 12205, - "volume": 478068 - }, - "id": 2757790, - "non_hive": { - "close": 43240, - "high": 15, - "low": 8, - "open": 1560, - "volume": 61119 - }, - "open": "2020-11-01T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 79606, - "high": 9103, - "low": 385, - "open": 15872, - "volume": 277982 - }, - "id": 2757812, - "non_hive": { - "close": 10110, - "high": 1163, - "low": 48, - "open": 2000, - "volume": 35240 - }, - "open": "2020-11-01T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 7929, - "high": 10743, - "low": 4181, - "open": 54000, - "volume": 2942213 - }, - "id": 2757837, - "non_hive": { - "close": 990, - "high": 1374, - "low": 503, - "open": 6858, - "volume": 367480 - }, - "open": "2020-11-01T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4238, - "high": 17370, - "low": 22, - "open": 17370, - "volume": 156902 - }, - "id": 2757879, - "non_hive": { - "close": 524, - "high": 2167, - "low": 2, - "open": 2167, - "volume": 19439 - }, - "open": "2020-11-01T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 297888, - "high": 2996, - "low": 13457, - "open": 13457, - "volume": 826625 - }, - "id": 2757904, - "non_hive": { - "close": 36954, - "high": 374, - "low": 1665, - "open": 1665, - "volume": 102845 - }, - "open": "2020-11-01T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8020, - "high": 304, - "low": 1000, - "open": 156875, - "volume": 251185 - }, - "id": 2757940, - "non_hive": { - "close": 1000, - "high": 38, - "low": 124, - "open": 19578, - "volume": 31344 - }, - "open": "2020-11-01T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25424, - "high": 1482, - "low": 487, - "open": 405211, - "volume": 1189193 - }, - "id": 2757961, - "non_hive": { - "close": 3139, - "high": 185, - "low": 60, - "open": 50529, - "volume": 148136 - }, - "open": "2020-11-01T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 42888, - "high": 47422, - "low": 5000, - "open": 16193, - "volume": 466196 - }, - "id": 2757994, - "non_hive": { - "close": 5329, - "high": 5900, - "low": 617, - "open": 2000, - "volume": 57903 - }, - "open": "2020-11-01T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10323, - "high": 24538, - "low": 482, - "open": 4236, - "volume": 677646 - }, - "id": 2758025, - "non_hive": { - "close": 1274, - "high": 3052, - "low": 59, - "open": 526, - "volume": 84252 - }, - "open": "2020-11-01T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 217, - "high": 8, - "low": 232, - "open": 10323, - "volume": 1473899 - }, - "id": 2758056, - "non_hive": { - "close": 27, - "high": 1, - "low": 28, - "open": 1283, - "volume": 182453 - }, - "open": "2020-11-01T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3920, - "high": 40, - "low": 2547, - "open": 24176, - "volume": 900979 - }, - "id": 2758094, - "non_hive": { - "close": 487, - "high": 5, - "low": 316, - "open": 3007, - "volume": 112167 - }, - "open": "2020-11-01T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2405, - "high": 80, - "low": 696, - "open": 10967, - "volume": 213059 - }, - "id": 2758144, - "non_hive": { - "close": 299, - "high": 10, - "low": 86, - "open": 1368, - "volume": 26555 - }, - "open": "2020-11-01T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 12004, - "high": 264, - "low": 9324, - "open": 72400, - "volume": 777705 - }, - "id": 2758170, - "non_hive": { - "close": 1498, - "high": 33, - "low": 1151, - "open": 9001, - "volume": 96759 - }, - "open": "2020-11-01T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 19188, - "high": 168, - "low": 22, - "open": 30726, - "volume": 2126835 - }, - "id": 2758211, - "non_hive": { - "close": 2306, - "high": 21, - "low": 2, - "open": 3834, - "volume": 264297 - }, - "open": "2020-11-01T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16133, - "high": 850, - "low": 1971, - "open": 16076, - "volume": 436033 - }, - "id": 2758270, - "non_hive": { - "close": 1999, - "high": 106, - "low": 242, - "open": 1999, - "volume": 54127 - }, - "open": "2020-11-01T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 21182, - "high": 8302, - "low": 11000, - "open": 16135, - "volume": 708060 - }, - "id": 2758325, - "non_hive": { - "close": 2641, - "high": 1036, - "low": 1352, - "open": 1999, - "volume": 87859 - }, - "open": "2020-11-01T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2170, - "high": 4381, - "low": 2855, - "open": 17403, - "volume": 159989 - }, - "id": 2758384, - "non_hive": { - "close": 267, - "high": 546, - "low": 351, - "open": 2140, - "volume": 19683 - }, - "open": "2020-11-01T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32000, - "high": 3276, - "low": 32000, - "open": 21267, - "volume": 98231 - }, - "id": 2758413, - "non_hive": { - "close": 3936, - "high": 403, - "low": 3936, - "open": 2616, - "volume": 12083 - }, - "open": "2020-11-01T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 28000, - "high": 3952, - "low": 8097, - "open": 8097, - "volume": 752370 - }, - "id": 2758426, - "non_hive": { - "close": 3527, - "high": 498, - "low": 995, - "open": 995, - "volume": 92855 - }, - "open": "2020-11-01T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 95, - "high": 7, - "low": 1167, - "open": 56526, - "volume": 10057690 - }, - "id": 2758455, - "non_hive": { - "close": 12, - "high": 1, - "low": 143, - "open": 7178, - "volume": 1269959 - }, - "open": "2020-11-02T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 200991, - "high": 63362, - "low": 16223, - "open": 103994, - "volume": 1724627 - }, - "id": 2758537, - "non_hive": { - "close": 24923, - "high": 7990, - "low": 1999, - "open": 12998, - "volume": 215821 - }, - "open": "2020-11-02T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 198, - "high": 198, - "low": 13000, - "open": 15862, - "volume": 45648 - }, - "id": 2758572, - "non_hive": { - "close": 25, - "high": 25, - "low": 1638, - "open": 1999, - "volume": 5753 - }, - "open": "2020-11-02T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 107857, - "high": 2595, - "low": 107857, - "open": 2595, - "volume": 417665 - }, - "id": 2758582, - "non_hive": { - "close": 13326, - "high": 327, - "low": 13326, - "open": 327, - "volume": 51672 - }, - "open": "2020-11-02T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 64341, - "high": 64341, - "low": 16000, - "open": 16000, - "volume": 1616424 - }, - "id": 2758608, - "non_hive": { - "close": 8213, - "high": 8213, - "low": 1999, - "open": 1999, - "volume": 202338 - }, - "open": "2020-11-02T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1717248, - "high": 1128, - "low": 1717248, - "open": 1128, - "volume": 2648614 - }, - "id": 2758632, - "non_hive": { - "close": 214656, - "high": 144, - "low": 214656, - "open": 144, - "volume": 331934 - }, - "open": "2020-11-02T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 802, - "high": 4552, - "low": 15079, - "open": 16186, - "volume": 254946 - }, - "id": 2758654, - "non_hive": { - "close": 100, - "high": 569, - "low": 1863, - "open": 2000, - "volume": 31531 - }, - "open": "2020-11-02T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 619682, - "high": 305, - "low": 1727, - "open": 6602, - "volume": 7299615 - }, - "id": 2758666, - "non_hive": { - "close": 79105, - "high": 39, - "low": 213, - "open": 815, - "volume": 923742 - }, - "open": "2020-11-02T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10, - "high": 11210, - "low": 10, - "open": 11210, - "volume": 221314 - }, - "id": 2758699, - "non_hive": { - "close": 1, - "high": 1431, - "low": 1, - "open": 1431, - "volume": 28189 - }, - "open": "2020-11-02T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 15777, - "high": 3506, - "low": 44873, - "open": 16128, - "volume": 1143857 - }, - "id": 2758722, - "non_hive": { - "close": 1999, - "high": 445, - "low": 5564, - "open": 2000, - "volume": 142072 - }, - "open": "2020-11-02T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14485, - "high": 86, - "low": 350, - "open": 350, - "volume": 348863 - }, - "id": 2758741, - "non_hive": { - "close": 1845, - "high": 11, - "low": 44, - "open": 44, - "volume": 44447 - }, - "open": "2020-11-02T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 283, - "high": 557, - "low": 13807, - "open": 7851, - "volume": 235421 - }, - "id": 2758767, - "non_hive": { - "close": 36, - "high": 71, - "low": 1735, - "open": 1000, - "volume": 29843 - }, - "open": "2020-11-02T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11640, - "high": 133, - "low": 78, - "open": 17407, - "volume": 6934531 - }, - "id": 2758802, - "non_hive": { - "close": 1463, - "high": 17, - "low": 9, - "open": 2213, - "volume": 872420 - }, - "open": "2020-11-02T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13670, - "high": 220, - "low": 16000, - "open": 220, - "volume": 451798 - }, - "id": 2758841, - "non_hive": { - "close": 1708, - "high": 28, - "low": 1999, - "open": 28, - "volume": 56507 - }, - "open": "2020-11-02T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13057, - "high": 12608, - "low": 10373, - "open": 2330, - "volume": 2081333 - }, - "id": 2758859, - "non_hive": { - "close": 1630, - "high": 1576, - "low": 1275, - "open": 291, - "volume": 258575 - }, - "open": "2020-11-02T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 25370, - "high": 265665, - "low": 10620, - "open": 2957, - "volume": 713894 - }, - "id": 2758913, - "non_hive": { - "close": 3146, - "high": 33180, - "low": 1306, - "open": 369, - "volume": 88994 - }, - "open": "2020-11-02T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 618, - "high": 368, - "low": 32480, - "open": 16141, - "volume": 929640 - }, - "id": 2758942, - "non_hive": { - "close": 77, - "high": 46, - "low": 3967, - "open": 2000, - "volume": 114913 - }, - "open": "2020-11-02T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16420, - "high": 16, - "low": 3363, - "open": 16054, - "volume": 632366 - }, - "id": 2759000, - "non_hive": { - "close": 2036, - "high": 2, - "low": 404, - "open": 1999, - "volume": 77569 - }, - "open": "2020-11-02T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11063, - "high": 572, - "low": 173, - "open": 572, - "volume": 818010 - }, - "id": 2759038, - "non_hive": { - "close": 1359, - "high": 71, - "low": 20, - "open": 71, - "volume": 100228 - }, - "open": "2020-11-02T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4735, - "high": 6716, - "low": 4735, - "open": 6716, - "volume": 822808 - }, - "id": 2759083, - "non_hive": { - "close": 570, - "high": 825, - "low": 570, - "open": 825, - "volume": 99287 - }, - "open": "2020-11-02T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 63294, - "high": 1135, - "low": 2103, - "open": 1135, - "volume": 91637 - }, - "id": 2759096, - "non_hive": { - "close": 7634, - "high": 137, - "low": 253, - "open": 137, - "volume": 11052 - }, - "open": "2020-11-02T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1095, - "high": 4553, - "low": 50, - "open": 8274, - "volume": 272956 - }, - "id": 2759114, - "non_hive": { - "close": 132, - "high": 557, - "low": 6, - "open": 998, - "volume": 32958 - }, - "open": "2020-11-02T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1144, - "high": 14536, - "low": 22295, - "open": 16488, - "volume": 1410567 - }, - "id": 2759140, - "non_hive": { - "close": 139, - "high": 1778, - "low": 2679, - "open": 1999, - "volume": 171618 - }, - "open": "2020-11-02T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10001, - "high": 5046, - "low": 10001, - "open": 35111, - "volume": 1164680 - }, - "id": 2759173, - "non_hive": { - "close": 1205, - "high": 617, - "low": 1205, - "open": 4293, - "volume": 140860 - }, - "open": "2020-11-02T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 46, - "high": 4952, - "low": 32, - "open": 4952, - "volume": 24285 - }, - "id": 2759200, - "non_hive": { - "close": 5, - "high": 605, - "low": 3, - "open": 605, - "volume": 2963 - }, - "open": "2020-11-03T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5972, - "high": 8175, - "low": 5972, - "open": 8175, - "volume": 14147 - }, - "id": 2759211, - "non_hive": { - "close": 729, - "high": 999, - "low": 729, - "open": 999, - "volume": 1728 - }, - "open": "2020-11-03T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 36908, - "high": 139, - "low": 1478, - "open": 10296, - "volume": 1518191 - }, - "id": 2759218, - "non_hive": { - "close": 4429, - "high": 17, - "low": 177, - "open": 1258, - "volume": 182948 - }, - "open": "2020-11-03T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17650, - "high": 300, - "low": 13, - "open": 32742, - "volume": 186429 - }, - "id": 2759243, - "non_hive": { - "close": 2096, - "high": 36, - "low": 1, - "open": 3929, - "volume": 22227 - }, - "open": "2020-11-03T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11699, - "high": 8, - "low": 277, - "open": 24040, - "volume": 55816 - }, - "id": 2759274, - "non_hive": { - "close": 1402, - "high": 1, - "low": 32, - "open": 2856, - "volume": 6627 - }, - "open": "2020-11-03T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 48, - "high": 893, - "low": 48, - "open": 20000, - "volume": 248972 - }, - "id": 2759289, - "non_hive": { - "close": 5, - "high": 107, - "low": 5, - "open": 2396, - "volume": 29734 - }, - "open": "2020-11-03T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 425, - "high": 8, - "low": 149, - "open": 10507, - "volume": 141039 - }, - "id": 2759319, - "non_hive": { - "close": 51, - "high": 1, - "low": 17, - "open": 1249, - "volume": 16878 - }, - "open": "2020-11-03T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23, - "high": 367, - "low": 23, - "open": 10266, - "volume": 612102 - }, - "id": 2759343, - "non_hive": { - "close": 2, - "high": 44, - "low": 2, - "open": 1229, - "volume": 72405 - }, - "open": "2020-11-03T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 246333, - "high": 1737, - "low": 11967, - "open": 10978, - "volume": 318713 - }, - "id": 2759379, - "non_hive": { - "close": 29056, - "high": 205, - "low": 1410, - "open": 1294, - "volume": 37588 - }, - "open": "2020-11-03T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1004, - "high": 1430, - "low": 7809, - "open": 128959, - "volume": 177804 - }, - "id": 2759396, - "non_hive": { - "close": 120, - "high": 171, - "low": 913, - "open": 15212, - "volume": 20970 - }, - "open": "2020-11-03T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 16, - "high": 1104, - "low": 16, - "open": 6828, - "volume": 1346019 - }, - "id": 2759417, - "non_hive": { - "close": 1, - "high": 132, - "low": 1, - "open": 816, - "volume": 160895 - }, - "open": "2020-11-03T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 516, - "high": 3600, - "low": 516, - "open": 3600, - "volume": 520942 - }, - "id": 2759434, - "non_hive": { - "close": 60, - "high": 430, - "low": 60, - "open": 430, - "volume": 61555 - }, - "open": "2020-11-03T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 277, - "high": 519, - "low": 80, - "open": 37926, - "volume": 290582 - }, - "id": 2759467, - "non_hive": { - "close": 33, - "high": 62, - "low": 9, - "open": 4527, - "volume": 34667 - }, - "open": "2020-11-03T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 428642, - "high": 25088, - "low": 159, - "open": 10141, - "volume": 669807 - }, - "id": 2759507, - "non_hive": { - "close": 50578, - "high": 2995, - "low": 18, - "open": 1210, - "volume": 79142 - }, - "open": "2020-11-03T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 972, - "high": 8, - "low": 42376, - "open": 251, - "volume": 1063353 - }, - "id": 2759527, - "non_hive": { - "close": 116, - "high": 1, - "low": 4957, - "open": 30, - "volume": 126813 - }, - "open": "2020-11-03T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 80547, - "high": 167, - "low": 80547, - "open": 164724, - "volume": 3253885 - }, - "id": 2759558, - "non_hive": { - "close": 9471, - "high": 20, - "low": 9471, - "open": 19653, - "volume": 385224 - }, - "open": "2020-11-03T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 800830, - "high": 9503, - "low": 156, - "open": 16829, - "volume": 1384555 - }, - "id": 2759590, - "non_hive": { - "close": 93697, - "high": 1134, - "low": 18, - "open": 2000, - "volume": 162497 - }, - "open": "2020-11-03T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 129310, - "high": 9783, - "low": 129310, - "open": 17094, - "volume": 968682 - }, - "id": 2759637, - "non_hive": { - "close": 15000, - "high": 1150, - "low": 15000, - "open": 1999, - "volume": 112882 - }, - "open": "2020-11-03T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 49312, - "high": 49312, - "low": 9, - "open": 1973100, - "volume": 2120010 - }, - "id": 2759696, - "non_hive": { - "close": 5799, - "high": 5799, - "low": 1, - "open": 228879, - "volume": 246144 - }, - "open": "2020-11-03T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5136, - "high": 2925, - "low": 866, - "open": 9414, - "volume": 226550 - }, - "id": 2759716, - "non_hive": { - "close": 604, - "high": 344, - "low": 101, - "open": 1106, - "volume": 26610 - }, - "open": "2020-11-03T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 24078, - "high": 21649, - "low": 12, - "open": 88941, - "volume": 386538 - }, - "id": 2759746, - "non_hive": { - "close": 2865, - "high": 2584, - "low": 1, - "open": 10459, - "volume": 45681 - }, - "open": "2020-11-03T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 50445, - "high": 50, - "low": 20, - "open": 57958, - "volume": 736141 - }, - "id": 2759791, - "non_hive": { - "close": 6001, - "high": 6, - "low": 2, - "open": 6897, - "volume": 87640 - }, - "open": "2020-11-03T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4252, - "high": 99008, - "low": 115, - "open": 14379, - "volume": 2051831 - }, - "id": 2759850, - "non_hive": { - "close": 500, - "high": 11782, - "low": 13, - "open": 1711, - "volume": 243619 - }, - "open": "2020-11-03T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 850, - "high": 546, - "low": 851, - "open": 2126, - "volume": 76314 - }, - "id": 2759907, - "non_hive": { - "close": 100, - "high": 65, - "low": 100, - "open": 250, - "volume": 9031 - }, - "open": "2020-11-03T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 542, - "high": 504, - "low": 55, - "open": 16997, - "volume": 8627924 - }, - "id": 2759956, - "non_hive": { - "close": 62, - "high": 60, - "low": 6, - "open": 2000, - "volume": 1003402 - }, - "open": "2020-11-04T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1564, - "high": 1564, - "low": 28138, - "open": 1600, - "volume": 1456189 - }, - "id": 2759995, - "non_hive": { - "close": 186, - "high": 186, - "low": 3267, - "open": 187, - "volume": 172634 - }, - "open": "2020-11-04T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 111, - "high": 111, - "low": 71570, - "open": 13967, - "volume": 435022 - }, - "id": 2760025, - "non_hive": { - "close": 13, - "high": 13, - "low": 8311, - "open": 1622, - "volume": 50517 - }, - "open": "2020-11-04T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 40482, - "high": 8456, - "low": 40482, - "open": 8456, - "volume": 320384 - }, - "id": 2760044, - "non_hive": { - "close": 4700, - "high": 982, - "low": 4700, - "open": 982, - "volume": 37202 - }, - "open": "2020-11-04T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 23288, - "high": 21614, - "low": 12262, - "open": 17395, - "volume": 910398 - }, - "id": 2760067, - "non_hive": { - "close": 2704, - "high": 2510, - "low": 1423, - "open": 2020, - "volume": 105708 - }, - "open": "2020-11-04T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 567585, - "high": 567585, - "low": 3814, - "open": 3814, - "volume": 760783 - }, - "id": 2760089, - "non_hive": { - "close": 67449, - "high": 67449, - "low": 442, - "open": 442, - "volume": 89922 - }, - "open": "2020-11-04T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3845, - "high": 3845, - "low": 67, - "open": 16830, - "volume": 87865 - }, - "id": 2760096, - "non_hive": { - "close": 457, - "high": 457, - "low": 7, - "open": 1999, - "volume": 10435 - }, - "open": "2020-11-04T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 3124, - "high": 791, - "low": 307876, - "open": 791, - "volume": 2026308 - }, - "id": 2760114, - "non_hive": { - "close": 369, - "high": 94, - "low": 35097, - "open": 94, - "volume": 232448 - }, - "open": "2020-11-04T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 194, - "high": 194, - "low": 507, - "open": 15299, - "volume": 167006 - }, - "id": 2760128, - "non_hive": { - "close": 23, - "high": 23, - "low": 57, - "open": 1806, - "volume": 19713 - }, - "open": "2020-11-04T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 60944, - "high": 25, - "low": 11511, - "open": 5085, - "volume": 190513 - }, - "id": 2760157, - "non_hive": { - "close": 7189, - "high": 3, - "low": 1350, - "open": 600, - "volume": 22468 - }, - "open": "2020-11-04T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 85873, - "high": 2698, - "low": 2458, - "open": 17503, - "volume": 178284 - }, - "id": 2760175, - "non_hive": { - "close": 9960, - "high": 313, - "low": 280, - "open": 2000, - "volume": 20541 - }, - "open": "2020-11-04T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 56, - "high": 163, - "low": 105, - "open": 14563, - "volume": 279633 - }, - "id": 2760194, - "non_hive": { - "close": 6, - "high": 19, - "low": 11, - "open": 1689, - "volume": 32245 - }, - "open": "2020-11-04T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2261, - "high": 6771, - "low": 15, - "open": 3121, - "volume": 225715 - }, - "id": 2760228, - "non_hive": { - "close": 266, - "high": 797, - "low": 1, - "open": 362, - "volume": 26162 - }, - "open": "2020-11-04T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 631, - "high": 59, - "low": 631, - "open": 133145, - "volume": 491650 - }, - "id": 2760254, - "non_hive": { - "close": 72, - "high": 7, - "low": 72, - "open": 15655, - "volume": 57796 - }, - "open": "2020-11-04T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8617, - "high": 8571, - "low": 2863, - "open": 62992, - "volume": 932332 - }, - "id": 2760290, - "non_hive": { - "close": 999, - "high": 1007, - "low": 329, - "open": 7400, - "volume": 108141 - }, - "open": "2020-11-04T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 32352, - "high": 129, - "low": 100, - "open": 100, - "volume": 340760 - }, - "id": 2760333, - "non_hive": { - "close": 3720, - "high": 15, - "low": 11, - "open": 11, - "volume": 39304 - }, - "open": "2020-11-04T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 870, - "high": 870, - "low": 103, - "open": 8633, - "volume": 622145 - }, - "id": 2760348, - "non_hive": { - "close": 101, - "high": 101, - "low": 11, - "open": 1001, - "volume": 71425 - }, - "open": "2020-11-04T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 68415, - "high": 51, - "low": 20, - "open": 215, - "volume": 859257 - }, - "id": 2760405, - "non_hive": { - "close": 7724, - "high": 6, - "low": 2, - "open": 25, - "volume": 97488 - }, - "open": "2020-11-04T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20, - "high": 224, - "low": 20, - "open": 2814, - "volume": 200731 - }, - "id": 2760442, - "non_hive": { - "close": 2, - "high": 26, - "low": 2, - "open": 325, - "volume": 23254 - }, - "open": "2020-11-04T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 426400, - "high": 220839, - "low": 100, - "open": 104, - "volume": 693077 - }, - "id": 2760454, - "non_hive": { - "close": 49365, - "high": 25580, - "low": 11, - "open": 12, - "volume": 80237 - }, - "open": "2020-11-04T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 29120, - "high": 9528, - "low": 57, - "open": 4382, - "volume": 297737 - }, - "id": 2760487, - "non_hive": { - "close": 3369, - "high": 1103, - "low": 6, - "open": 507, - "volume": 34460 - }, - "open": "2020-11-04T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 11896, - "high": 3854, - "low": 8862, - "open": 3854, - "volume": 45749 - }, - "id": 2760515, - "non_hive": { - "close": 1356, - "high": 447, - "low": 1010, - "open": 447, - "volume": 5263 - }, - "open": "2020-11-04T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 58446, - "high": 672, - "low": 8738, - "open": 13460, - "volume": 3957544 - }, - "id": 2760539, - "non_hive": { - "close": 6779, - "high": 78, - "low": 996, - "open": 1547, - "volume": 456547 - }, - "open": "2020-11-04T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20912, - "high": 517, - "low": 10942, - "open": 2562, - "volume": 459121 - }, - "id": 2760583, - "non_hive": { - "close": 2424, - "high": 60, - "low": 1250, - "open": 297, - "volume": 53205 - }, - "open": "2020-11-04T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 266349, - "high": 26611, - "low": 310, - "open": 10904, - "volume": 1034672 - }, - "id": 2760620, - "non_hive": { - "close": 30874, - "high": 3085, - "low": 35, - "open": 1264, - "volume": 119332 - }, - "open": "2020-11-05T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 13080, - "high": 3002, - "low": 5610, - "open": 5610, - "volume": 43283 - }, - "id": 2760654, - "non_hive": { - "close": 1516, - "high": 348, - "low": 647, - "open": 647, - "volume": 5013 - }, - "open": "2020-11-05T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 5220, - "high": 1682, - "low": 1231, - "open": 5626, - "volume": 181218 - }, - "id": 2760667, - "non_hive": { - "close": 604, - "high": 195, - "low": 142, - "open": 652, - "volume": 21002 - }, - "open": "2020-11-05T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 114546, - "high": 2769, - "low": 551, - "open": 18722, - "volume": 202574 - }, - "id": 2760686, - "non_hive": { - "close": 13272, - "high": 321, - "low": 63, - "open": 2170, - "volume": 23473 - }, - "open": "2020-11-05T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6315, - "high": 8, - "low": 59, - "open": 1018, - "volume": 3065591 - }, - "id": 2760707, - "non_hive": { - "close": 732, - "high": 1, - "low": 6, - "open": 118, - "volume": 354915 - }, - "open": "2020-11-05T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6445, - "high": 7911, - "low": 5533, - "open": 5533, - "volume": 43477 - }, - "id": 2760755, - "non_hive": { - "close": 746, - "high": 917, - "low": 640, - "open": 640, - "volume": 5037 - }, - "open": "2020-11-05T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6050, - "high": 51, - "low": 615, - "open": 10327, - "volume": 744774 - }, - "id": 2760771, - "non_hive": { - "close": 701, - "high": 6, - "low": 71, - "open": 1197, - "volume": 86322 - }, - "open": "2020-11-05T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17343, - "high": 2286, - "low": 3949, - "open": 17253, - "volume": 1643949 - }, - "id": 2760820, - "non_hive": { - "close": 1999, - "high": 265, - "low": 455, - "open": 1999, - "volume": 189970 - }, - "open": "2020-11-05T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 14656, - "high": 14656, - "low": 88840, - "open": 88840, - "volume": 363698 - }, - "id": 2760875, - "non_hive": { - "close": 1699, - "high": 1699, - "low": 10245, - "open": 10245, - "volume": 42105 - }, - "open": "2020-11-05T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 44935, - "high": 255, - "low": 8663, - "open": 13276, - "volume": 7478329 - }, - "id": 2760888, - "non_hive": { - "close": 5231, - "high": 30, - "low": 1000, - "open": 1539, - "volume": 871837 - }, - "open": "2020-11-05T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 71856, - "high": 8, - "low": 619, - "open": 8908, - "volume": 1490890 - }, - "id": 2760946, - "non_hive": { - "close": 8433, - "high": 1, - "low": 71, - "open": 1046, - "volume": 173265 - }, - "open": "2020-11-05T10:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 17339, - "high": 17, - "low": 4957, - "open": 7769, - "volume": 150242 - }, - "id": 2760970, - "non_hive": { - "close": 1999, - "high": 2, - "low": 567, - "open": 901, - "volume": 17374 - }, - "open": "2020-11-05T11:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 312, - "high": 60, - "low": 4804, - "open": 60, - "volume": 41962 - }, - "id": 2760993, - "non_hive": { - "close": 36, - "high": 7, - "low": 554, - "open": 7, - "volume": 4840 - }, - "open": "2020-11-05T12:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 8151, - "high": 240, - "low": 297, - "open": 17798, - "volume": 1156702 - }, - "id": 2761016, - "non_hive": { - "close": 949, - "high": 28, - "low": 34, - "open": 2052, - "volume": 134603 - }, - "open": "2020-11-05T13:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 43623, - "high": 8328, - "low": 1984, - "open": 8282, - "volume": 2170778 - }, - "id": 2761042, - "non_hive": { - "close": 5138, - "high": 981, - "low": 230, - "open": 964, - "volume": 253194 - }, - "open": "2020-11-05T14:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4295, - "high": 50, - "low": 2599, - "open": 51, - "volume": 1497840 - }, - "id": 2761134, - "non_hive": { - "close": 504, - "high": 6, - "low": 301, - "open": 6, - "volume": 176753 - }, - "open": "2020-11-05T15:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 69657, - "high": 104838, - "low": 20, - "open": 17045, - "volume": 1709632 - }, - "id": 2761192, - "non_hive": { - "close": 8143, - "high": 12359, - "low": 2, - "open": 1999, - "volume": 200394 - }, - "open": "2020-11-05T16:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 712, - "high": 1288, - "low": 57135, - "open": 15920, - "volume": 356611 - }, - "id": 2761230, - "non_hive": { - "close": 84, - "high": 152, - "low": 6672, - "open": 1860, - "volume": 41790 - }, - "open": "2020-11-05T17:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33630, - "high": 1807, - "low": 74, - "open": 1807, - "volume": 142612 - }, - "id": 2761266, - "non_hive": { - "close": 3923, - "high": 213, - "low": 8, - "open": 213, - "volume": 16727 - }, - "open": "2020-11-05T18:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 6463, - "high": 2298, - "low": 8853, - "open": 8130, - "volume": 136107 - }, - "id": 2761295, - "non_hive": { - "close": 762, - "high": 271, - "low": 1042, - "open": 957, - "volume": 16042 - }, - "open": "2020-11-05T19:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 81, - "high": 9007, - "low": 81, - "open": 27260, - "volume": 1554181 - }, - "id": 2761327, - "non_hive": { - "close": 9, - "high": 1062, - "low": 9, - "open": 3214, - "volume": 183129 - }, - "open": "2020-11-05T20:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000, - "high": 838, - "low": 52, - "open": 19085, - "volume": 1209362 - }, - "id": 2761344, - "non_hive": { - "close": 117, - "high": 99, - "low": 6, - "open": 2246, - "volume": 141683 - }, - "open": "2020-11-05T21:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 20867, - "high": 20867, - "low": 16958, - "open": 3476, - "volume": 1448972 - }, - "id": 2761418, - "non_hive": { - "close": 2470, - "high": 2470, - "low": 1999, - "open": 410, - "volume": 171408 - }, - "open": "2020-11-05T22:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 507, - "high": 336, - "low": 72712, - "open": 4004, - "volume": 3089093 - }, - "id": 2761457, - "non_hive": { - "close": 60, - "high": 40, - "low": 8507, - "open": 474, - "volume": 363558 - }, - "open": "2020-11-05T23:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 33000, - "high": 76, - "low": 17022, - "open": 16271, - "volume": 6007344 - }, - "id": 2761505, - "non_hive": { - "close": 3861, - "high": 9, - "low": 1991, - "open": 1904, - "volume": 702981 - }, - "open": "2020-11-06T00:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4698, - "high": 1044, - "low": 1382746, - "open": 1584, - "volume": 1516791 - }, - "id": 2761542, - "non_hive": { - "close": 556, - "high": 124, - "low": 161782, - "open": 186, - "volume": 177630 - }, - "open": "2020-11-06T01:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1055208, - "high": 1055208, - "low": 12193, - "open": 12193, - "volume": 15987932 - }, - "id": 2761570, - "non_hive": { - "close": 125939, - "high": 125939, - "low": 1443, - "open": 1443, - "volume": 1900711 - }, - "open": "2020-11-06T02:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 10755, - "high": 6001, - "low": 265, - "open": 1191, - "volume": 28212 - }, - "id": 2761603, - "non_hive": { - "close": 1283, - "high": 716, - "low": 31, - "open": 142, - "volume": 3352 - }, - "open": "2020-11-06T03:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 425, - "high": 425, - "low": 9994, - "open": 2228, - "volume": 122609 - }, - "id": 2761617, - "non_hive": { - "close": 51, - "high": 51, - "low": 1192, - "open": 266, - "volume": 14690 - }, - "open": "2020-11-06T04:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 4221, - "high": 44894, - "low": 1701, - "open": 8179, - "volume": 290493 - }, - "id": 2761637, - "non_hive": { - "close": 500, - "high": 5380, - "low": 201, - "open": 980, - "volume": 34649 - }, - "open": "2020-11-06T05:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 980, - "high": 10201, - "low": 402, - "open": 1802, - "volume": 1581678 - }, - "id": 2761660, - "non_hive": { - "close": 116, - "high": 1216, - "low": 47, - "open": 213, - "volume": 188493 - }, - "open": "2020-11-06T06:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 463201, - "high": 1459, - "low": 38, - "open": 91996, - "volume": 740369 - }, - "id": 2761686, - "non_hive": { - "close": 55181, - "high": 174, - "low": 4, - "open": 10966, - "volume": 88199 - }, - "open": "2020-11-06T07:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 318, - "high": 318, - "low": 18983, - "open": 1107, - "volume": 1232451 - }, - "id": 2761720, - "non_hive": { - "close": 38, - "high": 38, - "low": 2239, - "open": 132, - "volume": 146806 - }, - "open": "2020-11-06T08:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 2810, - "high": 2365, - "low": 4289, - "open": 4974, - "volume": 160134 - }, - "id": 2761753, - "non_hive": { - "close": 335, - "high": 282, - "low": 511, - "open": 593, - "volume": 19088 - }, - "open": "2020-11-06T09:00:00", - "seconds": 3600 - }, - { - "hive": { - "close": 1000000, - "high": 1000000, - "low": 4250, - "open": 7902, - "volume": 1697065 - }, - "id": 2761771, - "non_hive": { - "close": 119924, - "high": 119924, - "low": 506, - "open": 942, - "volume": 203104 - }, - "open": "2020-11-06T10:00:00", - "seconds": 3600 - } -] diff --git a/hived/tavern/condenser_api_patterns/get_market_history/3600.tavern.yaml b/hived/tavern/condenser_api_patterns/get_market_history/3600.tavern.yaml deleted file mode 100644 index 12fb7d3aef5dbe914ae45c5ff3614413fa08f9bd..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history/3600.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_market_history - - marks: - - patterntest # this works only for new data from less than eight months before now - - includes: - - !include ../../common.yaml - - stages: - - name: get_market_history - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_market_history" - params: [3600,"2019-04-03T00:00:00","2031-09-06T00:00:00"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "3600" - directory: "condenser_api_patterns/get_market_history" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_market_history/60.pat.json b/hived/tavern/condenser_api_patterns/get_market_history/60.pat.json deleted file mode 100644 index 9e3f3ae967f520dfbfcd8f41c7fcfc5c9f9d9933..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history/60.pat.json +++ /dev/null @@ -1,19819 +0,0 @@ -[ - { - "hive": { - "close": 1572, - "high": 7851, - "low": 1572, - "open": 7851, - "volume": 9423 - }, - "id": 2758765, - "non_hive": { - "close": 200, - "high": 1000, - "low": 200, - "open": 1000, - "volume": 1200 - }, - "open": "2020-11-02T11:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 13807, - "high": 557, - "low": 13807, - "open": 557, - "volume": 30272 - }, - "id": 2758769, - "non_hive": { - "close": 1735, - "high": 71, - "low": 1735, - "open": 71, - "volume": 3806 - }, - "open": "2020-11-02T11:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 15909, - "high": 15909, - "low": 15909, - "open": 15909, - "volume": 15909 - }, - "id": 2758771, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-02T11:13:00", - "seconds": 60 - }, - { - "hive": { - "close": 127, - "high": 15783, - "low": 127, - "open": 15783, - "volume": 15910 - }, - "id": 2758774, - "non_hive": { - "close": 16, - "high": 2000, - "low": 16, - "open": 2000, - "volume": 2016 - }, - "open": "2020-11-02T11:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 15783, - "high": 15783, - "low": 15783, - "open": 15783, - "volume": 15783 - }, - "id": 2758776, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-02T11:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 15783, - "high": 15783, - "low": 15783, - "open": 15783, - "volume": 47349 - }, - "id": 2758779, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 6000 - }, - "open": "2020-11-02T11:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 1647, - "high": 2185, - "low": 1647, - "open": 2185, - "volume": 5176 - }, - "id": 2758783, - "non_hive": { - "close": 207, - "high": 277, - "low": 207, - "open": 277, - "volume": 653 - }, - "open": "2020-11-02T11:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 15702, - "high": 15702, - "low": 15702, - "open": 15702, - "volume": 15702 - }, - "id": 2758785, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-02T11:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 4917, - "high": 4917, - "low": 4917, - "open": 4917, - "volume": 4917 - }, - "id": 2758787, - "non_hive": { - "close": 626, - "high": 626, - "low": 626, - "open": 626, - "volume": 626 - }, - "open": "2020-11-02T11:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 7918, - "high": 7918, - "low": 7918, - "open": 7918, - "volume": 7918 - }, - "id": 2758790, - "non_hive": { - "close": 1008, - "high": 1008, - "low": 1008, - "open": 1008, - "volume": 1008 - }, - "open": "2020-11-02T11:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 15711, - "high": 15711, - "low": 15711, - "open": 15711, - "volume": 15711 - }, - "id": 2758793, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-02T11:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 15711, - "high": 15711, - "low": 15711, - "open": 15711, - "volume": 15711 - }, - "id": 2758796, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-02T11:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 283, - "high": 35357, - "low": 283, - "open": 35357, - "volume": 35640 - }, - "id": 2758798, - "non_hive": { - "close": 36, - "high": 4501, - "low": 36, - "open": 4501, - "volume": 4537 - }, - "open": "2020-11-02T11:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 6193, - "high": 17407, - "low": 6193, - "open": 17407, - "volume": 23600 - }, - "id": 2758800, - "non_hive": { - "close": 787, - "high": 2213, - "low": 787, - "open": 2213, - "volume": 3000 - }, - "open": "2020-11-02T12:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 91161, - "high": 91161, - "low": 91161, - "open": 91161, - "volume": 91161 - }, - "id": 2758804, - "non_hive": { - "close": 11590, - "high": 11590, - "low": 11590, - "open": 11590, - "volume": 11590 - }, - "open": "2020-11-02T12:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 303531, - "high": 133, - "low": 3226, - "open": 23597, - "volume": 330487 - }, - "id": 2758807, - "non_hive": { - "close": 38590, - "high": 17, - "low": 410, - "open": 3000, - "volume": 42017 - }, - "open": "2020-11-02T12:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 228, - "high": 228, - "low": 228, - "open": 228, - "volume": 228 - }, - "id": 2758811, - "non_hive": { - "close": 29, - "high": 29, - "low": 29, - "open": 29, - "volume": 29 - }, - "open": "2020-11-02T12:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 78, - "high": 18015, - "low": 78, - "open": 18015, - "volume": 18093 - }, - "id": 2758813, - "non_hive": { - "close": 9, - "high": 2290, - "low": 9, - "open": 2290, - "volume": 2299 - }, - "open": "2020-11-02T12:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 251, - "high": 251, - "low": 251, - "open": 251, - "volume": 251 - }, - "id": 2758815, - "non_hive": { - "close": 32, - "high": 32, - "low": 32, - "open": 32, - "volume": 32 - }, - "open": "2020-11-02T12:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 1054, - "high": 1054, - "low": 1054, - "open": 1054, - "volume": 1054 - }, - "id": 2758818, - "non_hive": { - "close": 134, - "high": 134, - "low": 134, - "open": 134, - "volume": 134 - }, - "open": "2020-11-02T12:21:00", - "seconds": 60 - }, - { - "hive": { - "close": 14169, - "high": 14169, - "low": 14169, - "open": 14169, - "volume": 14169 - }, - "id": 2758820, - "non_hive": { - "close": 1800, - "high": 1800, - "low": 1800, - "open": 1800, - "volume": 1800 - }, - "open": "2020-11-02T12:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 39355, - "high": 39355, - "low": 39355, - "open": 39355, - "volume": 39355 - }, - "id": 2758823, - "non_hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "open": "2020-11-02T12:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 7878, - "high": 7878, - "low": 7878, - "open": 7878, - "volume": 7878 - }, - "id": 2758825, - "non_hive": { - "close": 1001, - "high": 1001, - "low": 1001, - "open": 1001, - "volume": 1001 - }, - "open": "2020-11-02T12:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 7856, - "high": 7856, - "low": 7856, - "open": 7856, - "volume": 7856 - }, - "id": 2758828, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-11-02T12:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 15742, - "high": 15742, - "low": 15742, - "open": 15742, - "volume": 15742 - }, - "id": 2758830, - "non_hive": { - "close": 2001, - "high": 2001, - "low": 2001, - "open": 2001, - "volume": 2001 - }, - "open": "2020-11-02T12:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 11567, - "high": 11567, - "low": 11567, - "open": 11567, - "volume": 11567 - }, - "id": 2758832, - "non_hive": { - "close": 1469, - "high": 1469, - "low": 1469, - "open": 1469, - "volume": 1469 - }, - "open": "2020-11-02T12:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 527, - "high": 527, - "low": 19942, - "open": 19942, - "volume": 20469 - }, - "id": 2758835, - "non_hive": { - "close": 67, - "high": 67, - "low": 2534, - "open": 2534, - "volume": 2601 - }, - "open": "2020-11-02T12:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 11640, - "high": 496, - "low": 11640, - "open": 496, - "volume": 6352621 - }, - "id": 2758837, - "non_hive": { - "close": 1463, - "high": 63, - "low": 1463, - "open": 63, - "volume": 798449 - }, - "open": "2020-11-02T12:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 220, - "high": 220, - "low": 220, - "open": 220, - "volume": 220 - }, - "id": 2758839, - "non_hive": { - "close": 28, - "high": 28, - "low": 28, - "open": 28, - "volume": 28 - }, - "open": "2020-11-02T13:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 10333, - "high": 10333, - "low": 15873, - "open": 15873, - "volume": 26206 - }, - "id": 2758843, - "non_hive": { - "close": 1302, - "high": 1302, - "low": 1999, - "open": 1999, - "volume": 3301 - }, - "open": "2020-11-02T13:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 8767, - "high": 8767, - "low": 8767, - "open": 8767, - "volume": 8767 - }, - "id": 2758846, - "non_hive": { - "close": 1104, - "high": 1104, - "low": 1104, - "open": 1104, - "volume": 1104 - }, - "open": "2020-11-02T13:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 199992, - "high": 15999, - "low": 48, - "open": 15999, - "volume": 242943 - }, - "id": 2758849, - "non_hive": { - "close": 24999, - "high": 2000, - "low": 6, - "open": 2000, - "volume": 30368 - }, - "open": "2020-11-02T13:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 13670, - "high": 143992, - "low": 16000, - "open": 16000, - "volume": 173662 - }, - "id": 2758852, - "non_hive": { - "close": 1708, - "high": 17999, - "low": 1999, - "open": 1999, - "volume": 21706 - }, - "open": "2020-11-02T13:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 12608, - "high": 12608, - "low": 2330, - "open": 2330, - "volume": 14938 - }, - "id": 2758857, - "non_hive": { - "close": 1576, - "high": 1576, - "low": 291, - "open": 291, - "volume": 1867 - }, - "open": "2020-11-02T14:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 5000 - }, - "id": 2758861, - "non_hive": { - "close": 125, - "high": 125, - "low": 125, - "open": 125, - "volume": 625 - }, - "open": "2020-11-02T14:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 2001, - "high": 9408, - "low": 6998, - "open": 2001, - "volume": 24409 - }, - "id": 2758867, - "non_hive": { - "close": 250, - "high": 1176, - "low": 874, - "open": 250, - "volume": 3050 - }, - "open": "2020-11-02T14:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 146848, - "high": 146848, - "low": 5147, - "open": 5427, - "volume": 157422 - }, - "id": 2758871, - "non_hive": { - "close": 18356, - "high": 18356, - "low": 643, - "open": 678, - "volume": 19677 - }, - "open": "2020-11-02T14:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 928, - "high": 928, - "low": 928, - "open": 928, - "volume": 928 - }, - "id": 2758873, - "non_hive": { - "close": 116, - "high": 116, - "low": 116, - "open": 116, - "volume": 116 - }, - "open": "2020-11-02T14:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 832, - "high": 832, - "low": 832, - "open": 832, - "volume": 832 - }, - "id": 2758875, - "non_hive": { - "close": 104, - "high": 104, - "low": 104, - "open": 104, - "volume": 104 - }, - "open": "2020-11-02T14:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 6722, - "high": 6722, - "low": 6722, - "open": 6722, - "volume": 6722 - }, - "id": 2758878, - "non_hive": { - "close": 837, - "high": 837, - "low": 837, - "open": 837, - "volume": 837 - }, - "open": "2020-11-02T14:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 3344, - "high": 3344, - "low": 6722, - "open": 6722, - "volume": 10066 - }, - "id": 2758881, - "non_hive": { - "close": 418, - "high": 418, - "low": 840, - "open": 840, - "volume": 1258 - }, - "open": "2020-11-02T14:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 260768, - "high": 260768, - "low": 260768, - "open": 260768, - "volume": 260768 - }, - "id": 2758884, - "non_hive": { - "close": 32596, - "high": 32596, - "low": 32596, - "open": 32596, - "volume": 32596 - }, - "open": "2020-11-02T14:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 1808, - "high": 1808, - "low": 1808, - "open": 1808, - "volume": 1808 - }, - "id": 2758887, - "non_hive": { - "close": 226, - "high": 226, - "low": 226, - "open": 226, - "volume": 226 - }, - "open": "2020-11-02T14:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 16, - "high": 16, - "low": 16, - "open": 16, - "volume": 16 - }, - "id": 2758889, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-11-02T14:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 312, - "high": 312, - "low": 312, - "open": 312, - "volume": 312 - }, - "id": 2758892, - "non_hive": { - "close": 39, - "high": 39, - "low": 39, - "open": 39, - "volume": 39 - }, - "open": "2020-11-02T14:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 200, - "high": 200, - "low": 200, - "open": 200, - "volume": 200 - }, - "id": 2758894, - "non_hive": { - "close": 25, - "high": 25, - "low": 25, - "open": 25, - "volume": 25 - }, - "open": "2020-11-02T14:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 809544, - "high": 9338, - "low": 809544, - "open": 9338, - "volume": 1177421 - }, - "id": 2758896, - "non_hive": { - "close": 100000, - "high": 1163, - "low": 100000, - "open": 1163, - "volume": 145774 - }, - "open": "2020-11-02T14:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 10373, - "high": 10373, - "low": 10373, - "open": 10373, - "volume": 10373 - }, - "id": 2758898, - "non_hive": { - "close": 1275, - "high": 1275, - "low": 1275, - "open": 1275, - "volume": 1275 - }, - "open": "2020-11-02T14:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 63920, - "high": 63920, - "low": 16000, - "open": 16000, - "volume": 79920 - }, - "id": 2758901, - "non_hive": { - "close": 7990, - "high": 7990, - "low": 1999, - "open": 1999, - "volume": 9989 - }, - "open": "2020-11-02T14:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 17098, - "high": 236056, - "low": 17098, - "open": 3711, - "volume": 306044 - }, - "id": 2758903, - "non_hive": { - "close": 2102, - "high": 29507, - "low": 2102, - "open": 463, - "volume": 38121 - }, - "open": "2020-11-02T14:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 11097, - "high": 11097, - "low": 11097, - "open": 11097, - "volume": 11097 - }, - "id": 2758906, - "non_hive": { - "close": 1364, - "high": 1364, - "low": 1364, - "open": 1364, - "volume": 1364 - }, - "open": "2020-11-02T14:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 13057, - "high": 13057, - "low": 13057, - "open": 13057, - "volume": 13057 - }, - "id": 2758909, - "non_hive": { - "close": 1630, - "high": 1630, - "low": 1630, - "open": 1630, - "volume": 1630 - }, - "open": "2020-11-02T14:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 2957, - "high": 2957, - "low": 2957, - "open": 2957, - "volume": 2957 - }, - "id": 2758911, - "non_hive": { - "close": 369, - "high": 369, - "low": 369, - "open": 369, - "volume": 369 - }, - "open": "2020-11-02T15:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 6486, - "high": 6486, - "low": 6486, - "open": 6486, - "volume": 6486 - }, - "id": 2758915, - "non_hive": { - "close": 810, - "high": 810, - "low": 810, - "open": 810, - "volume": 810 - }, - "open": "2020-11-02T15:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 10620, - "high": 10620, - "low": 10620, - "open": 10620, - "volume": 10620 - }, - "id": 2758917, - "non_hive": { - "close": 1306, - "high": 1306, - "low": 1306, - "open": 1306, - "volume": 1306 - }, - "open": "2020-11-02T15:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 265665, - "high": 265665, - "low": 11596, - "open": 100104, - "volume": 532053 - }, - "id": 2758919, - "non_hive": { - "close": 33180, - "high": 33180, - "low": 1447, - "open": 12500, - "volume": 66446 - }, - "open": "2020-11-02T15:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 16128, - "high": 16128, - "low": 16128, - "open": 16128, - "volume": 16128 - }, - "id": 2758923, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-02T15:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 40354, - "high": 40354, - "low": 40354, - "open": 40354, - "volume": 40354 - }, - "id": 2758926, - "non_hive": { - "close": 5004, - "high": 5004, - "low": 5004, - "open": 5004, - "volume": 5004 - }, - "open": "2020-11-02T15:21:00", - "seconds": 60 - }, - { - "hive": { - "close": 16128, - "high": 16128, - "low": 16128, - "open": 16128, - "volume": 16128 - }, - "id": 2758928, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-02T15:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 21178, - "high": 21178, - "low": 21178, - "open": 21178, - "volume": 21178 - }, - "id": 2758930, - "non_hive": { - "close": 2626, - "high": 2626, - "low": 2626, - "open": 2626, - "volume": 2626 - }, - "open": "2020-11-02T15:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 3028, - "high": 3028, - "low": 3028, - "open": 3028, - "volume": 3028 - }, - "id": 2758933, - "non_hive": { - "close": 378, - "high": 378, - "low": 378, - "open": 378, - "volume": 378 - }, - "open": "2020-11-02T15:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 25370, - "high": 2130, - "low": 16127, - "open": 2130, - "volume": 64962 - }, - "id": 2758936, - "non_hive": { - "close": 3146, - "high": 265, - "low": 1999, - "open": 265, - "volume": 8056 - }, - "open": "2020-11-02T15:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 12817, - "high": 16141, - "low": 12817, - "open": 16141, - "volume": 542425 - }, - "id": 2758940, - "non_hive": { - "close": 1575, - "high": 2000, - "low": 1575, - "open": 2000, - "volume": 66950 - }, - "open": "2020-11-02T16:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 15196, - "high": 1073, - "low": 15196, - "open": 1073, - "volume": 16269 - }, - "id": 2758945, - "non_hive": { - "close": 1868, - "high": 133, - "low": 1868, - "open": 133, - "volume": 2001 - }, - "open": "2020-11-02T16:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 1612, - "high": 5811, - "low": 807, - "open": 16116, - "volume": 39657 - }, - "id": 2758948, - "non_hive": { - "close": 200, - "high": 725, - "low": 100, - "open": 2000, - "volume": 4925 - }, - "open": "2020-11-02T16:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 807, - "high": 14504, - "low": 807, - "open": 14504, - "volume": 50769 - }, - "id": 2758951, - "non_hive": { - "close": 100, - "high": 1800, - "low": 100, - "open": 1800, - "volume": 6300 - }, - "open": "2020-11-02T16:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 13127, - "high": 13127, - "low": 12087, - "open": 3818, - "volume": 78909 - }, - "id": 2758955, - "non_hive": { - "close": 1637, - "high": 1637, - "low": 1500, - "open": 474, - "volume": 9830 - }, - "open": "2020-11-02T16:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 13000, - "high": 13000, - "low": 13000, - "open": 13000, - "volume": 13000 - }, - "id": 2758959, - "non_hive": { - "close": 1614, - "high": 1614, - "low": 1614, - "open": 1614, - "volume": 1614 - }, - "open": "2020-11-02T16:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 1002, - "high": 1002, - "low": 5192, - "open": 16091, - "volume": 25408 - }, - "id": 2758962, - "non_hive": { - "close": 125, - "high": 125, - "low": 638, - "open": 1999, - "volume": 3150 - }, - "open": "2020-11-02T16:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 6203, - "high": 6203, - "low": 1015, - "open": 1015, - "volume": 13348 - }, - "id": 2758964, - "non_hive": { - "close": 764, - "high": 764, - "low": 125, - "open": 125, - "volume": 1644 - }, - "open": "2020-11-02T16:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 7007, - "high": 7007, - "low": 7007, - "open": 7007, - "volume": 7007 - }, - "id": 2758967, - "non_hive": { - "close": 863, - "high": 863, - "low": 863, - "open": 863, - "volume": 863 - }, - "open": "2020-11-02T16:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 7925, - "high": 7925, - "low": 7925, - "open": 7925, - "volume": 7925 - }, - "id": 2758970, - "non_hive": { - "close": 976, - "high": 976, - "low": 976, - "open": 976, - "volume": 976 - }, - "open": "2020-11-02T16:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 1915, - "high": 1915, - "low": 1915, - "open": 1915, - "volume": 1915 - }, - "id": 2758972, - "non_hive": { - "close": 235, - "high": 235, - "low": 235, - "open": 235, - "volume": 235 - }, - "open": "2020-11-02T16:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 32480, - "high": 162, - "low": 32480, - "open": 2940, - "volume": 60193 - }, - "id": 2758975, - "non_hive": { - "close": 3967, - "high": 20, - "low": 3967, - "open": 362, - "volume": 7373 - }, - "open": "2020-11-02T16:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 13006, - "high": 13006, - "low": 13006, - "open": 13006, - "volume": 13006 - }, - "id": 2758979, - "non_hive": { - "close": 1601, - "high": 1601, - "low": 1601, - "open": 1601, - "volume": 1601 - }, - "open": "2020-11-02T16:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 5793, - "high": 5793, - "low": 16049, - "open": 16049, - "volume": 21842 - }, - "id": 2758982, - "non_hive": { - "close": 722, - "high": 722, - "low": 1999, - "open": 1999, - "volume": 2721 - }, - "open": "2020-11-02T16:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 5320, - "high": 5320, - "low": 6239, - "open": 9810, - "volume": 21369 - }, - "id": 2758984, - "non_hive": { - "close": 663, - "high": 663, - "low": 777, - "open": 1222, - "volume": 2662 - }, - "open": "2020-11-02T16:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 11906, - "high": 11906, - "low": 11906, - "open": 11906, - "volume": 11906 - }, - "id": 2758986, - "non_hive": { - "close": 1483, - "high": 1483, - "low": 1483, - "open": 1483, - "volume": 1483 - }, - "open": "2020-11-02T16:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 1819, - "high": 1819, - "low": 1887, - "open": 1887, - "volume": 3706 - }, - "id": 2758989, - "non_hive": { - "close": 227, - "high": 227, - "low": 235, - "open": 235, - "volume": 462 - }, - "open": "2020-11-02T16:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 368, - "high": 368, - "low": 368, - "open": 368, - "volume": 368 - }, - "id": 2758992, - "non_hive": { - "close": 46, - "high": 46, - "low": 46, - "open": 46, - "volume": 46 - }, - "open": "2020-11-02T16:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 618, - "high": 618, - "low": 618, - "open": 618, - "volume": 618 - }, - "id": 2758995, - "non_hive": { - "close": 77, - "high": 77, - "low": 77, - "open": 77, - "volume": 77 - }, - "open": "2020-11-02T16:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 16054, - "high": 16054, - "low": 16054, - "open": 16054, - "volume": 16054 - }, - "id": 2758998, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-02T17:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 6856, - "high": 16, - "low": 6856, - "open": 16, - "volume": 6872 - }, - "id": 2759002, - "non_hive": { - "close": 854, - "high": 2, - "low": 854, - "open": 2, - "volume": 856 - }, - "open": "2020-11-02T17:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 7585, - "high": 16200, - "low": 7585, - "open": 16200, - "volume": 44335 - }, - "id": 2759004, - "non_hive": { - "close": 933, - "high": 2000, - "low": 933, - "open": 2000, - "volume": 5467 - }, - "open": "2020-11-02T17:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 16040, - "high": 16040, - "low": 16040, - "open": 16040, - "volume": 16040 - }, - "id": 2759007, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-02T17:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 30910, - "high": 15748, - "low": 46510, - "open": 15748, - "volume": 215748 - }, - "id": 2759010, - "non_hive": { - "close": 3775, - "high": 1961, - "low": 5680, - "open": 1961, - "volume": 26389 - }, - "open": "2020-11-02T17:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 57002, - "high": 4093, - "low": 57002, - "open": 4093, - "volume": 186957 - }, - "id": 2759014, - "non_hive": { - "close": 6954, - "high": 500, - "low": 6954, - "open": 500, - "volume": 22814 - }, - "open": "2020-11-02T17:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 2228, - "high": 2228, - "low": 2228, - "open": 2228, - "volume": 2228 - }, - "id": 2759018, - "non_hive": { - "close": 277, - "high": 277, - "low": 277, - "open": 277, - "volume": 277 - }, - "open": "2020-11-02T17:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 3363, - "high": 16637, - "low": 3363, - "open": 16637, - "volume": 20000 - }, - "id": 2759020, - "non_hive": { - "close": 404, - "high": 2000, - "low": 404, - "open": 2000, - "volume": 2404 - }, - "open": "2020-11-02T17:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 11343, - "high": 11343, - "low": 13851, - "open": 13851, - "volume": 25194 - }, - "id": 2759023, - "non_hive": { - "close": 1411, - "high": 1411, - "low": 1722, - "open": 1722, - "volume": 3133 - }, - "open": "2020-11-02T17:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 4405, - "high": 4405, - "low": 4405, - "open": 4405, - "volume": 4405 - }, - "id": 2759025, - "non_hive": { - "close": 548, - "high": 548, - "low": 548, - "open": 548, - "volume": 548 - }, - "open": "2020-11-02T17:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 74871, - "high": 74871, - "low": 74871, - "open": 74871, - "volume": 74871 - }, - "id": 2759028, - "non_hive": { - "close": 9245, - "high": 9245, - "low": 9245, - "open": 9245, - "volume": 9245 - }, - "open": "2020-11-02T17:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 3242, - "high": 3242, - "low": 3242, - "open": 3242, - "volume": 3242 - }, - "id": 2759031, - "non_hive": { - "close": 402, - "high": 402, - "low": 402, - "open": 402, - "volume": 402 - }, - "open": "2020-11-02T17:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 16420, - "high": 16420, - "low": 16420, - "open": 16420, - "volume": 16420 - }, - "id": 2759034, - "non_hive": { - "close": 2036, - "high": 2036, - "low": 2036, - "open": 2036, - "volume": 2036 - }, - "open": "2020-11-02T17:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 572, - "high": 572, - "low": 572, - "open": 572, - "volume": 572 - }, - "id": 2759036, - "non_hive": { - "close": 71, - "high": 71, - "low": 71, - "open": 71, - "volume": 71 - }, - "open": "2020-11-02T18:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 115994, - "high": 16581, - "low": 115994, - "open": 16581, - "volume": 132575 - }, - "id": 2759040, - "non_hive": { - "close": 13991, - "high": 2000, - "low": 13991, - "open": 2000, - "volume": 15991 - }, - "open": "2020-11-02T18:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 961, - "high": 961, - "low": 961, - "open": 961, - "volume": 961 - }, - "id": 2759044, - "non_hive": { - "close": 116, - "high": 116, - "low": 116, - "open": 116, - "volume": 116 - }, - "open": "2020-11-02T18:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 48951, - "high": 48951, - "low": 2464, - "open": 2464, - "volume": 67560 - }, - "id": 2759046, - "non_hive": { - "close": 6063, - "high": 6063, - "low": 297, - "open": 297, - "volume": 8359 - }, - "open": "2020-11-02T18:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 333426, - "high": 333426, - "low": 16272, - "open": 16272, - "volume": 349698 - }, - "id": 2759049, - "non_hive": { - "close": 40981, - "high": 40981, - "low": 1999, - "open": 1999, - "volume": 42980 - }, - "open": "2020-11-02T18:13:00", - "seconds": 60 - }, - { - "hive": { - "close": 16281, - "high": 16281, - "low": 16325, - "open": 16325, - "volume": 32606 - }, - "id": 2759051, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-02T18:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 1406, - "high": 16281, - "low": 1406, - "open": 16281, - "volume": 33968 - }, - "id": 2759055, - "non_hive": { - "close": 169, - "high": 2000, - "low": 169, - "open": 2000, - "volume": 4169 - }, - "open": "2020-11-02T18:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 34845, - "high": 34845, - "low": 16276, - "open": 16276, - "volume": 51121 - }, - "id": 2759059, - "non_hive": { - "close": 4282, - "high": 4282, - "low": 1999, - "open": 1999, - "volume": 6281 - }, - "open": "2020-11-02T18:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 699, - "high": 52273, - "low": 699, - "open": 52273, - "volume": 52972 - }, - "id": 2759061, - "non_hive": { - "close": 84, - "high": 6420, - "low": 84, - "open": 6420, - "volume": 6504 - }, - "open": "2020-11-02T18:21:00", - "seconds": 60 - }, - { - "hive": { - "close": 173, - "high": 19695, - "low": 173, - "open": 19695, - "volume": 19868 - }, - "id": 2759064, - "non_hive": { - "close": 20, - "high": 2419, - "low": 20, - "open": 2419, - "volume": 2439 - }, - "open": "2020-11-02T18:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 11577, - "high": 11577, - "low": 11577, - "open": 11577, - "volume": 11577 - }, - "id": 2759067, - "non_hive": { - "close": 1411, - "high": 1411, - "low": 1411, - "open": 1411, - "volume": 1411 - }, - "open": "2020-11-02T18:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 8165, - "high": 8165, - "low": 8165, - "open": 8165, - "volume": 8165 - }, - "id": 2759070, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-11-02T18:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 8158, - "high": 8158, - "low": 8158, - "open": 8158, - "volume": 8158 - }, - "id": 2759073, - "non_hive": { - "close": 999, - "high": 999, - "low": 999, - "open": 999, - "volume": 999 - }, - "open": "2020-11-02T18:48:00", - "seconds": 60 - }, - { - "hive": { - "close": 304, - "high": 36842, - "low": 304, - "open": 36842, - "volume": 37146 - }, - "id": 2759076, - "non_hive": { - "close": 37, - "high": 4512, - "low": 37, - "open": 4512, - "volume": 4549 - }, - "open": "2020-11-02T18:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 11063, - "high": 11063, - "low": 11063, - "open": 11063, - "volume": 11063 - }, - "id": 2759078, - "non_hive": { - "close": 1359, - "high": 1359, - "low": 1359, - "open": 1359, - "volume": 1359 - }, - "open": "2020-11-02T18:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 6716, - "high": 6716, - "low": 6716, - "open": 6716, - "volume": 6716 - }, - "id": 2759081, - "non_hive": { - "close": 825, - "high": 825, - "low": 825, - "open": 825, - "volume": 825 - }, - "open": "2020-11-02T19:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 8851, - "high": 8851, - "low": 8851, - "open": 8851, - "volume": 8851 - }, - "id": 2759085, - "non_hive": { - "close": 1087, - "high": 1087, - "low": 1087, - "open": 1087, - "volume": 1087 - }, - "open": "2020-11-02T19:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 30536, - "high": 1680, - "low": 30536, - "open": 1680, - "volume": 802506 - }, - "id": 2759088, - "non_hive": { - "close": 3683, - "high": 206, - "low": 3683, - "open": 206, - "volume": 96805 - }, - "open": "2020-11-02T19:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 4735, - "high": 4735, - "low": 4735, - "open": 4735, - "volume": 4735 - }, - "id": 2759091, - "non_hive": { - "close": 570, - "high": 570, - "low": 570, - "open": 570, - "volume": 570 - }, - "open": "2020-11-02T19:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 1135, - "high": 1135, - "low": 1135, - "open": 1135, - "volume": 1135 - }, - "id": 2759094, - "non_hive": { - "close": 137, - "high": 137, - "low": 137, - "open": 137, - "volume": 137 - }, - "open": "2020-11-02T20:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 8274, - "high": 8274, - "low": 8274, - "open": 8274, - "volume": 8274 - }, - "id": 2759098, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-11-02T20:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "id": 2759101, - "non_hive": { - "close": 603, - "high": 603, - "low": 603, - "open": 603, - "volume": 603 - }, - "open": "2020-11-02T20:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 11831, - "high": 11831, - "low": 11831, - "open": 11831, - "volume": 11831 - }, - "id": 2759104, - "non_hive": { - "close": 1427, - "high": 1427, - "low": 1427, - "open": 1427, - "volume": 1427 - }, - "open": "2020-11-02T20:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 2103, - "high": 2103, - "low": 2103, - "open": 2103, - "volume": 2103 - }, - "id": 2759107, - "non_hive": { - "close": 253, - "high": 253, - "low": 253, - "open": 253, - "volume": 253 - }, - "open": "2020-11-02T20:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 63294, - "high": 63294, - "low": 63294, - "open": 63294, - "volume": 63294 - }, - "id": 2759109, - "non_hive": { - "close": 7634, - "high": 7634, - "low": 7634, - "open": 7634, - "volume": 7634 - }, - "open": "2020-11-02T20:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 8274, - "high": 8274, - "low": 8274, - "open": 8274, - "volume": 8274 - }, - "id": 2759112, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-11-02T21:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 31345, - "high": 31345, - "low": 31345, - "open": 31345, - "volume": 31345 - }, - "id": 2759116, - "non_hive": { - "close": 3780, - "high": 3780, - "low": 3780, - "open": 3780, - "volume": 3780 - }, - "open": "2020-11-02T21:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 3017, - "high": 3017, - "low": 3017, - "open": 3017, - "volume": 3017 - }, - "id": 2759119, - "non_hive": { - "close": 364, - "high": 364, - "low": 364, - "open": 364, - "volume": 364 - }, - "open": "2020-11-02T21:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 39127, - "high": 39127, - "low": 39127, - "open": 39127, - "volume": 39127 - }, - "id": 2759121, - "non_hive": { - "close": 4719, - "high": 4719, - "low": 4719, - "open": 4719, - "volume": 4719 - }, - "open": "2020-11-02T21:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 9625, - "high": 9625, - "low": 9625, - "open": 9625, - "volume": 9625 - }, - "id": 2759124, - "non_hive": { - "close": 1161, - "high": 1161, - "low": 1161, - "open": 1161, - "volume": 1161 - }, - "open": "2020-11-02T21:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 30148, - "high": 67673, - "low": 30148, - "open": 67673, - "volume": 97821 - }, - "id": 2759127, - "non_hive": { - "close": 3636, - "high": 8162, - "low": 3636, - "open": 8162, - "volume": 11798 - }, - "open": "2020-11-02T21:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 50, - "high": 4553, - "low": 50, - "open": 4553, - "volume": 4603 - }, - "id": 2759129, - "non_hive": { - "close": 6, - "high": 557, - "low": 6, - "open": 557, - "volume": 563 - }, - "open": "2020-11-02T21:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 18025, - "high": 18025, - "low": 18025, - "open": 18025, - "volume": 18025 - }, - "id": 2759132, - "non_hive": { - "close": 2205, - "high": 2205, - "low": 2205, - "open": 2205, - "volume": 2205 - }, - "open": "2020-11-02T21:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 1095, - "high": 16582, - "low": 1095, - "open": 16582, - "volume": 61119 - }, - "id": 2759135, - "non_hive": { - "close": 132, - "high": 2000, - "low": 132, - "open": 2000, - "volume": 7370 - }, - "open": "2020-11-02T21:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 16488, - "high": 16488, - "low": 16488, - "open": 16488, - "volume": 16488 - }, - "id": 2759138, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-02T22:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 16496, - "high": 5096, - "low": 16496, - "open": 5096, - "volume": 45031 - }, - "id": 2759142, - "non_hive": { - "close": 1999, - "high": 618, - "low": 1999, - "open": 618, - "volume": 5459 - }, - "open": "2020-11-02T22:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 12062, - "high": 12062, - "low": 12062, - "open": 12062, - "volume": 12062 - }, - "id": 2759146, - "non_hive": { - "close": 1461, - "high": 1461, - "low": 1461, - "open": 1461, - "volume": 1461 - }, - "open": "2020-11-02T22:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 3786, - "high": 3786, - "low": 12762, - "open": 12762, - "volume": 16548 - }, - "id": 2759148, - "non_hive": { - "close": 459, - "high": 459, - "low": 1541, - "open": 1541, - "volume": 2000 - }, - "open": "2020-11-02T22:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 14536, - "high": 14536, - "low": 14536, - "open": 14536, - "volume": 14536 - }, - "id": 2759151, - "non_hive": { - "close": 1778, - "high": 1778, - "low": 1778, - "open": 1778, - "volume": 1778 - }, - "open": "2020-11-02T22:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 24534, - "high": 30572, - "low": 15061, - "open": 1292, - "volume": 71459 - }, - "id": 2759154, - "non_hive": { - "close": 3000, - "high": 3739, - "low": 1841, - "open": 158, - "volume": 8738 - }, - "open": "2020-11-02T22:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 9156, - "high": 9156, - "low": 9156, - "open": 9156, - "volume": 9156 - }, - "id": 2759158, - "non_hive": { - "close": 1115, - "high": 1115, - "low": 1115, - "open": 1115, - "volume": 1115 - }, - "open": "2020-11-02T22:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 22295, - "high": 191, - "low": 22295, - "open": 191, - "volume": 74907 - }, - "id": 2759161, - "non_hive": { - "close": 2679, - "high": 23, - "low": 2679, - "open": 23, - "volume": 9004 - }, - "open": "2020-11-02T22:48:00", - "seconds": 60 - }, - { - "hive": { - "close": 1035, - "high": 1035, - "low": 1035, - "open": 1035, - "volume": 1035 - }, - "id": 2759163, - "non_hive": { - "close": 126, - "high": 126, - "low": 126, - "open": 126, - "volume": 126 - }, - "open": "2020-11-02T22:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 155169, - "high": 155169, - "low": 49786, - "open": 49786, - "volume": 1148201 - }, - "id": 2759166, - "non_hive": { - "close": 18968, - "high": 18968, - "low": 6055, - "open": 6055, - "volume": 139799 - }, - "open": "2020-11-02T22:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 1144, - "high": 1144, - "low": 1144, - "open": 1144, - "volume": 1144 - }, - "id": 2759169, - "non_hive": { - "close": 139, - "high": 139, - "low": 139, - "open": 139, - "volume": 139 - }, - "open": "2020-11-02T22:57:00", - "seconds": 60 - }, - { - "hive": { - "close": 22901, - "high": 5046, - "low": 22901, - "open": 35111, - "volume": 63058 - }, - "id": 2759171, - "non_hive": { - "close": 2800, - "high": 617, - "low": 2800, - "open": 4293, - "volume": 7710 - }, - "open": "2020-11-02T23:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 9436, - "high": 9436, - "low": 9436, - "open": 9436, - "volume": 9436 - }, - "id": 2759176, - "non_hive": { - "close": 1147, - "high": 1147, - "low": 1147, - "open": 1147, - "volume": 1147 - }, - "open": "2020-11-02T23:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 8740, - "high": 8740, - "low": 8740, - "open": 8740, - "volume": 8740 - }, - "id": 2759179, - "non_hive": { - "close": 1068, - "high": 1068, - "low": 1068, - "open": 1068, - "volume": 1068 - }, - "open": "2020-11-02T23:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 180, - "high": 180, - "low": 180, - "open": 180, - "volume": 180 - }, - "id": 2759181, - "non_hive": { - "close": 22, - "high": 22, - "low": 22, - "open": 22, - "volume": 22 - }, - "open": "2020-11-02T23:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 516, - "high": 516, - "low": 516, - "open": 516, - "volume": 516 - }, - "id": 2759184, - "non_hive": { - "close": 63, - "high": 63, - "low": 63, - "open": 63, - "volume": 63 - }, - "open": "2020-11-02T23:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 14093, - "high": 14093, - "low": 14093, - "open": 14093, - "volume": 14093 - }, - "id": 2759187, - "non_hive": { - "close": 1723, - "high": 1723, - "low": 1723, - "open": 1723, - "volume": 1723 - }, - "open": "2020-11-02T23:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 14863, - "high": 43793, - "low": 14863, - "open": 43793, - "volume": 58656 - }, - "id": 2759190, - "non_hive": { - "close": 1817, - "high": 5354, - "low": 1817, - "open": 5354, - "volume": 7171 - }, - "open": "2020-11-02T23:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 766780, - "high": 2708, - "low": 766780, - "open": 2708, - "volume": 1000000 - }, - "id": 2759193, - "non_hive": { - "close": 92403, - "high": 331, - "low": 92403, - "open": 331, - "volume": 120751 - }, - "open": "2020-11-02T23:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 10001, - "high": 10001, - "low": 10001, - "open": 10001, - "volume": 10001 - }, - "id": 2759195, - "non_hive": { - "close": 1205, - "high": 1205, - "low": 1205, - "open": 1205, - "volume": 1205 - }, - "open": "2020-11-02T23:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 32, - "high": 4952, - "low": 32, - "open": 4952, - "volume": 4984 - }, - "id": 2759198, - "non_hive": { - "close": 3, - "high": 605, - "low": 3, - "open": 605, - "volume": 608 - }, - "open": "2020-11-03T00:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 12000, - "high": 12000, - "low": 1718, - "open": 1718, - "volume": 13718 - }, - "id": 2759203, - "non_hive": { - "close": 1465, - "high": 1465, - "low": 209, - "open": 209, - "volume": 1674 - }, - "open": "2020-11-03T00:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 46, - "high": 5537, - "low": 46, - "open": 5537, - "volume": 5583 - }, - "id": 2759206, - "non_hive": { - "close": 5, - "high": 676, - "low": 5, - "open": 676, - "volume": 681 - }, - "open": "2020-11-03T00:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 8175, - "high": 8175, - "low": 8175, - "open": 8175, - "volume": 8175 - }, - "id": 2759209, - "non_hive": { - "close": 999, - "high": 999, - "low": 999, - "open": 999, - "volume": 999 - }, - "open": "2020-11-03T01:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 5972, - "high": 5972, - "low": 5972, - "open": 5972, - "volume": 5972 - }, - "id": 2759213, - "non_hive": { - "close": 729, - "high": 729, - "low": 729, - "open": 729, - "volume": 729 - }, - "open": "2020-11-03T01:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 8283, - "high": 14666, - "low": 8283, - "open": 10296, - "volume": 33245 - }, - "id": 2759216, - "non_hive": { - "close": 1012, - "high": 1792, - "low": 1012, - "open": 1258, - "volume": 4062 - }, - "open": "2020-11-03T02:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 210223, - "high": 16560, - "low": 210223, - "open": 16560, - "volume": 331953 - }, - "id": 2759220, - "non_hive": { - "close": 25331, - "high": 2000, - "low": 25331, - "open": 2000, - "volume": 40008 - }, - "open": "2020-11-03T02:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 1478, - "high": 3634, - "low": 1478, - "open": 16596, - "volume": 90000 - }, - "id": 2759223, - "non_hive": { - "close": 177, - "high": 438, - "low": 177, - "open": 2000, - "volume": 10824 - }, - "open": "2020-11-03T02:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 971527, - "high": 16596, - "low": 971527, - "open": 16596, - "volume": 988123 - }, - "id": 2759225, - "non_hive": { - "close": 117069, - "high": 2000, - "low": 117069, - "open": 2000, - "volume": 119069 - }, - "open": "2020-11-03T02:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 139, - "high": 139, - "low": 139, - "open": 139, - "volume": 139 - }, - "id": 2759227, - "non_hive": { - "close": 17, - "high": 17, - "low": 17, - "open": 17, - "volume": 17 - }, - "open": "2020-11-03T02:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 50, - "high": 16665, - "low": 18525, - "open": 16665, - "volume": 35240 - }, - "id": 2759230, - "non_hive": { - "close": 6, - "high": 2000, - "low": 2223, - "open": 2000, - "volume": 4229 - }, - "open": "2020-11-03T02:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 2175, - "high": 2175, - "low": 2175, - "open": 2175, - "volume": 2175 - }, - "id": 2759233, - "non_hive": { - "close": 261, - "high": 261, - "low": 261, - "open": 261, - "volume": 261 - }, - "open": "2020-11-03T02:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 408, - "high": 408, - "low": 408, - "open": 408, - "volume": 408 - }, - "id": 2759236, - "non_hive": { - "close": 49, - "high": 49, - "low": 49, - "open": 49, - "volume": 49 - }, - "open": "2020-11-03T02:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 36908, - "high": 36908, - "low": 36908, - "open": 36908, - "volume": 36908 - }, - "id": 2759239, - "non_hive": { - "close": 4429, - "high": 4429, - "low": 4429, - "open": 4429, - "volume": 4429 - }, - "open": "2020-11-03T02:48:00", - "seconds": 60 - }, - { - "hive": { - "close": 32742, - "high": 32742, - "low": 32742, - "open": 32742, - "volume": 32742 - }, - "id": 2759241, - "non_hive": { - "close": 3929, - "high": 3929, - "low": 3929, - "open": 3929, - "volume": 3929 - }, - "open": "2020-11-03T03:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 13, - "high": 659, - "low": 13, - "open": 659, - "volume": 672 - }, - "id": 2759245, - "non_hive": { - "close": 1, - "high": 79, - "low": 1, - "open": 79, - "volume": 80 - }, - "open": "2020-11-03T03:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 300, - "high": 300, - "low": 300, - "open": 300, - "volume": 300 - }, - "id": 2759248, - "non_hive": { - "close": 36, - "high": 36, - "low": 36, - "open": 36, - "volume": 36 - }, - "open": "2020-11-03T03:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 48642, - "high": 48642, - "low": 48642, - "open": 48642, - "volume": 48642 - }, - "id": 2759251, - "non_hive": { - "close": 5803, - "high": 5803, - "low": 5803, - "open": 5803, - "volume": 5803 - }, - "open": "2020-11-03T03:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 16806, - "high": 16806, - "low": 16806, - "open": 16806, - "volume": 33612 - }, - "id": 2759254, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-03T03:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 7746, - "high": 7746, - "low": 7746, - "open": 7746, - "volume": 7746 - }, - "id": 2759258, - "non_hive": { - "close": 921, - "high": 921, - "low": 921, - "open": 921, - "volume": 921 - }, - "open": "2020-11-03T03:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 6627, - "high": 6627, - "low": 6627, - "open": 6627, - "volume": 6627 - }, - "id": 2759260, - "non_hive": { - "close": 788, - "high": 788, - "low": 788, - "open": 788, - "volume": 788 - }, - "open": "2020-11-03T03:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 30103, - "high": 2445, - "low": 30103, - "open": 2445, - "volume": 32548 - }, - "id": 2759262, - "non_hive": { - "close": 3582, - "high": 291, - "low": 3582, - "open": 291, - "volume": 3873 - }, - "open": "2020-11-03T03:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 1858, - "high": 923, - "low": 1858, - "open": 923, - "volume": 5890 - }, - "id": 2759265, - "non_hive": { - "close": 221, - "high": 110, - "low": 221, - "open": 110, - "volume": 701 - }, - "open": "2020-11-03T03:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 17650, - "high": 17650, - "low": 17650, - "open": 17650, - "volume": 17650 - }, - "id": 2759269, - "non_hive": { - "close": 2096, - "high": 2096, - "low": 2096, - "open": 2096, - "volume": 2096 - }, - "open": "2020-11-03T03:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 277, - "high": 24040, - "low": 277, - "open": 24040, - "volume": 24317 - }, - "id": 2759272, - "non_hive": { - "close": 32, - "high": 2856, - "low": 32, - "open": 2856, - "volume": 2888 - }, - "open": "2020-11-03T04:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 1095, - "high": 1095, - "low": 1095, - "open": 1095, - "volume": 1095 - }, - "id": 2759276, - "non_hive": { - "close": 130, - "high": 130, - "low": 130, - "open": 130, - "volume": 130 - }, - "open": "2020-11-03T04:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 11729, - "high": 11729, - "low": 11729, - "open": 11729, - "volume": 11729 - }, - "id": 2759279, - "non_hive": { - "close": 1384, - "high": 1384, - "low": 1384, - "open": 1384, - "volume": 1384 - }, - "open": "2020-11-03T04:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 6968, - "high": 8, - "low": 6968, - "open": 8, - "volume": 6976 - }, - "id": 2759282, - "non_hive": { - "close": 822, - "high": 1, - "low": 822, - "open": 1, - "volume": 823 - }, - "open": "2020-11-03T04:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 11699, - "high": 11699, - "low": 11699, - "open": 11699, - "volume": 11699 - }, - "id": 2759284, - "non_hive": { - "close": 1402, - "high": 1402, - "low": 1402, - "open": 1402, - "volume": 1402 - }, - "open": "2020-11-03T04:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 20000, - "high": 20000, - "low": 20000, - "open": 20000, - "volume": 20000 - }, - "id": 2759287, - "non_hive": { - "close": 2396, - "high": 2396, - "low": 2396, - "open": 2396, - "volume": 2396 - }, - "open": "2020-11-03T05:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 893, - "high": 893, - "low": 893, - "open": 893, - "volume": 893 - }, - "id": 2759291, - "non_hive": { - "close": 107, - "high": 107, - "low": 107, - "open": 107, - "volume": 107 - }, - "open": "2020-11-03T05:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 16695, - "high": 16695, - "low": 16695, - "open": 16695, - "volume": 16695 - }, - "id": 2759294, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T05:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 14350, - "high": 14350, - "low": 14350, - "open": 14350, - "volume": 14350 - }, - "id": 2759297, - "non_hive": { - "close": 1719, - "high": 1719, - "low": 1719, - "open": 1719, - "volume": 1719 - }, - "open": "2020-11-03T05:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 83518, - "high": 83518, - "low": 83518, - "open": 83518, - "volume": 83518 - }, - "id": 2759300, - "non_hive": { - "close": 10000, - "high": 10000, - "low": 10000, - "open": 10000, - "volume": 10000 - }, - "open": "2020-11-03T05:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 11770, - "high": 16802, - "low": 11770, - "open": 16802, - "volume": 28572 - }, - "id": 2759303, - "non_hive": { - "close": 1401, - "high": 2000, - "low": 1401, - "open": 2000, - "volume": 3401 - }, - "open": "2020-11-03T05:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 905, - "high": 905, - "low": 905, - "open": 905, - "volume": 905 - }, - "id": 2759306, - "non_hive": { - "close": 107, - "high": 107, - "low": 107, - "open": 107, - "volume": 107 - }, - "open": "2020-11-03T05:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 14000, - "high": 14000, - "low": 16797, - "open": 16797, - "volume": 30797 - }, - "id": 2759309, - "non_hive": { - "close": 1667, - "high": 1667, - "low": 1999, - "open": 1999, - "volume": 3666 - }, - "open": "2020-11-03T05:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 18000, - "high": 18000, - "low": 15095, - "open": 15095, - "volume": 33095 - }, - "id": 2759311, - "non_hive": { - "close": 2143, - "high": 2143, - "low": 1797, - "open": 1797, - "volume": 3940 - }, - "open": "2020-11-03T05:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 48, - "high": 20099, - "low": 48, - "open": 20099, - "volume": 20147 - }, - "id": 2759314, - "non_hive": { - "close": 5, - "high": 2394, - "low": 5, - "open": 2394, - "volume": 2399 - }, - "open": "2020-11-03T05:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 174, - "high": 10507, - "low": 174, - "open": 10507, - "volume": 10681 - }, - "id": 2759317, - "non_hive": { - "close": 20, - "high": 1249, - "low": 20, - "open": 1249, - "volume": 1269 - }, - "open": "2020-11-03T06:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 11677, - "high": 11677, - "low": 11677, - "open": 11677, - "volume": 11677 - }, - "id": 2759321, - "non_hive": { - "close": 1398, - "high": 1398, - "low": 1398, - "open": 1398, - "volume": 1398 - }, - "open": "2020-11-03T06:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 309, - "high": 309, - "low": 16697, - "open": 16697, - "volume": 17006 - }, - "id": 2759324, - "non_hive": { - "close": 37, - "high": 37, - "low": 1999, - "open": 1999, - "volume": 2036 - }, - "open": "2020-11-03T06:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 16387, - "high": 16387, - "low": 16387, - "open": 16387, - "volume": 16387 - }, - "id": 2759327, - "non_hive": { - "close": 1962, - "high": 1962, - "low": 1962, - "open": 1962, - "volume": 1962 - }, - "open": "2020-11-03T06:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 694, - "high": 16002, - "low": 694, - "open": 16002, - "volume": 16696 - }, - "id": 2759329, - "non_hive": { - "close": 83, - "high": 1916, - "low": 83, - "open": 1916, - "volume": 1999 - }, - "open": "2020-11-03T06:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 16003, - "high": 30002, - "low": 149, - "open": 30002, - "volume": 61460 - }, - "id": 2759332, - "non_hive": { - "close": 1916, - "high": 3594, - "low": 17, - "open": 3594, - "volume": 7360 - }, - "open": "2020-11-03T06:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 8, - "high": 8, - "low": 694, - "open": 694, - "volume": 702 - }, - "id": 2759336, - "non_hive": { - "close": 1, - "high": 1, - "low": 83, - "open": 83, - "volume": 84 - }, - "open": "2020-11-03T06:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 425, - "high": 425, - "low": 6005, - "open": 6005, - "volume": 6430 - }, - "id": 2759338, - "non_hive": { - "close": 51, - "high": 51, - "low": 719, - "open": 719, - "volume": 770 - }, - "open": "2020-11-03T06:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 10266, - "high": 10266, - "low": 10266, - "open": 10266, - "volume": 10266 - }, - "id": 2759341, - "non_hive": { - "close": 1229, - "high": 1229, - "low": 1229, - "open": 1229, - "volume": 1229 - }, - "open": "2020-11-03T07:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 1773, - "high": 1773, - "low": 1773, - "open": 1773, - "volume": 1773 - }, - "id": 2759345, - "non_hive": { - "close": 212, - "high": 212, - "low": 212, - "open": 212, - "volume": 212 - }, - "open": "2020-11-03T07:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 1202, - "high": 18846, - "low": 1202, - "open": 18846, - "volume": 20048 - }, - "id": 2759348, - "non_hive": { - "close": 143, - "high": 2257, - "low": 143, - "open": 2257, - "volume": 2400 - }, - "open": "2020-11-03T07:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 367, - "high": 367, - "low": 367, - "open": 367, - "volume": 367 - }, - "id": 2759351, - "non_hive": { - "close": 44, - "high": 44, - "low": 44, - "open": 44, - "volume": 44 - }, - "open": "2020-11-03T07:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 7853, - "high": 7853, - "low": 7853, - "open": 7853, - "volume": 7853 - }, - "id": 2759353, - "non_hive": { - "close": 940, - "high": 940, - "low": 940, - "open": 940, - "volume": 940 - }, - "open": "2020-11-03T07:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 12000, - "high": 12000, - "low": 12000, - "open": 12000, - "volume": 12000 - }, - "id": 2759355, - "non_hive": { - "close": 1437, - "high": 1437, - "low": 1437, - "open": 1437, - "volume": 1437 - }, - "open": "2020-11-03T07:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 25227, - "high": 25227, - "low": 25227, - "open": 25227, - "volume": 25227 - }, - "id": 2759358, - "non_hive": { - "close": 3022, - "high": 3022, - "low": 3022, - "open": 3022, - "volume": 3022 - }, - "open": "2020-11-03T07:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 282138, - "high": 30379, - "low": 282138, - "open": 30379, - "volume": 482794 - }, - "id": 2759360, - "non_hive": { - "close": 33283, - "high": 3639, - "low": 33283, - "open": 3639, - "volume": 57017 - }, - "open": "2020-11-03T07:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 51, - "high": 51, - "low": 51, - "open": 51, - "volume": 51 - }, - "id": 2759364, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-03T07:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 9609, - "high": 9609, - "low": 9609, - "open": 9609, - "volume": 9609 - }, - "id": 2759366, - "non_hive": { - "close": 1133, - "high": 1133, - "low": 1133, - "open": 1133, - "volume": 1133 - }, - "open": "2020-11-03T07:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 7329, - "high": 7329, - "low": 7329, - "open": 7329, - "volume": 7329 - }, - "id": 2759369, - "non_hive": { - "close": 864, - "high": 864, - "low": 864, - "open": 864, - "volume": 864 - }, - "open": "2020-11-03T07:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 8338, - "high": 24073, - "low": 8338, - "open": 24073, - "volume": 32411 - }, - "id": 2759372, - "non_hive": { - "close": 983, - "high": 2839, - "low": 983, - "open": 2839, - "volume": 3822 - }, - "open": "2020-11-03T07:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 23, - "high": 2351, - "low": 23, - "open": 2351, - "volume": 2374 - }, - "id": 2759375, - "non_hive": { - "close": 2, - "high": 277, - "low": 2, - "open": 277, - "volume": 279 - }, - "open": "2020-11-03T07:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 10978, - "high": 10978, - "low": 10978, - "open": 10978, - "volume": 10978 - }, - "id": 2759377, - "non_hive": { - "close": 1294, - "high": 1294, - "low": 1294, - "open": 1294, - "volume": 1294 - }, - "open": "2020-11-03T08:21:00", - "seconds": 60 - }, - { - "hive": { - "close": 1832, - "high": 1832, - "low": 1832, - "open": 1832, - "volume": 1832 - }, - "id": 2759381, - "non_hive": { - "close": 216, - "high": 216, - "low": 216, - "open": 216, - "volume": 216 - }, - "open": "2020-11-03T08:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 3683, - "high": 5566, - "low": 3683, - "open": 5566, - "volume": 9249 - }, - "id": 2759383, - "non_hive": { - "close": 434, - "high": 656, - "low": 434, - "open": 656, - "volume": 1090 - }, - "open": "2020-11-03T08:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 11967, - "high": 11967, - "low": 11967, - "open": 11967, - "volume": 11967 - }, - "id": 2759386, - "non_hive": { - "close": 1410, - "high": 1410, - "low": 1410, - "open": 1410, - "volume": 1410 - }, - "open": "2020-11-03T08:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 1737, - "high": 1737, - "low": 12771, - "open": 12771, - "volume": 24354 - }, - "id": 2759389, - "non_hive": { - "close": 205, - "high": 205, - "low": 1505, - "open": 1505, - "volume": 2871 - }, - "open": "2020-11-03T08:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 246333, - "high": 246333, - "low": 14000, - "open": 14000, - "volume": 260333 - }, - "id": 2759391, - "non_hive": { - "close": 29056, - "high": 29056, - "low": 1651, - "open": 1651, - "volume": 30707 - }, - "open": "2020-11-03T08:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 128959, - "high": 128959, - "low": 128959, - "open": 128959, - "volume": 128959 - }, - "id": 2759394, - "non_hive": { - "close": 15212, - "high": 15212, - "low": 15212, - "open": 15212, - "volume": 15212 - }, - "open": "2020-11-03T09:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 15107, - "high": 15107, - "low": 15107, - "open": 15107, - "volume": 15107 - }, - "id": 2759398, - "non_hive": { - "close": 1782, - "high": 1782, - "low": 1782, - "open": 1782, - "volume": 1782 - }, - "open": "2020-11-03T09:13:00", - "seconds": 60 - }, - { - "hive": { - "close": 3611, - "high": 3611, - "low": 2391, - "open": 2391, - "volume": 6002 - }, - "id": 2759401, - "non_hive": { - "close": 426, - "high": 426, - "low": 282, - "open": 282, - "volume": 708 - }, - "open": "2020-11-03T09:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 16810, - "high": 16810, - "low": 16810, - "open": 16810, - "volume": 16810 - }, - "id": 2759404, - "non_hive": { - "close": 1983, - "high": 1983, - "low": 1983, - "open": 1983, - "volume": 1983 - }, - "open": "2020-11-03T09:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 1430, - "high": 1430, - "low": 343, - "open": 343, - "volume": 1773 - }, - "id": 2759407, - "non_hive": { - "close": 171, - "high": 171, - "low": 41, - "open": 41, - "volume": 212 - }, - "open": "2020-11-03T09:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 7809, - "high": 340, - "low": 7809, - "open": 340, - "volume": 8149 - }, - "id": 2759410, - "non_hive": { - "close": 913, - "high": 40, - "low": 913, - "open": 40, - "volume": 953 - }, - "open": "2020-11-03T09:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 1004, - "high": 1004, - "low": 1004, - "open": 1004, - "volume": 1004 - }, - "id": 2759412, - "non_hive": { - "close": 120, - "high": 120, - "low": 120, - "open": 120, - "volume": 120 - }, - "open": "2020-11-03T09:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 6828, - "high": 6828, - "low": 6828, - "open": 6828, - "volume": 6828 - }, - "id": 2759415, - "non_hive": { - "close": 816, - "high": 816, - "low": 816, - "open": 816, - "volume": 816 - }, - "open": "2020-11-03T10:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 449151, - "high": 449151, - "low": 449151, - "open": 449151, - "volume": 449151 - }, - "id": 2759419, - "non_hive": { - "close": 53700, - "high": 53700, - "low": 53700, - "open": 53700, - "volume": 53700 - }, - "open": "2020-11-03T10:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 9, - "high": 219128, - "low": 9, - "open": 219128, - "volume": 887921 - }, - "id": 2759421, - "non_hive": { - "close": 1, - "high": 26199, - "low": 1, - "open": 26199, - "volume": 106127 - }, - "open": "2020-11-03T10:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 111, - "high": 888, - "low": 111, - "open": 888, - "volume": 999 - }, - "id": 2759425, - "non_hive": { - "close": 13, - "high": 106, - "low": 13, - "open": 106, - "volume": 119 - }, - "open": "2020-11-03T10:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 16, - "high": 1104, - "low": 16, - "open": 1104, - "volume": 1120 - }, - "id": 2759429, - "non_hive": { - "close": 1, - "high": 132, - "low": 1, - "open": 132, - "volume": 133 - }, - "open": "2020-11-03T10:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 3600, - "high": 3600, - "low": 3600, - "open": 3600, - "volume": 3600 - }, - "id": 2759432, - "non_hive": { - "close": 430, - "high": 430, - "low": 430, - "open": 430, - "volume": 430 - }, - "open": "2020-11-03T11:21:00", - "seconds": 60 - }, - { - "hive": { - "close": 1623, - "high": 1623, - "low": 1623, - "open": 1623, - "volume": 1623 - }, - "id": 2759436, - "non_hive": { - "close": 193, - "high": 193, - "low": 193, - "open": 193, - "volume": 193 - }, - "open": "2020-11-03T11:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 73115, - "high": 9796, - "low": 73115, - "open": 9796, - "volume": 100000 - }, - "id": 2759439, - "non_hive": { - "close": 8556, - "high": 1170, - "low": 8556, - "open": 1170, - "volume": 11726 - }, - "open": "2020-11-03T11:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 24046, - "high": 24046, - "low": 24046, - "open": 24046, - "volume": 24046 - }, - "id": 2759441, - "non_hive": { - "close": 2813, - "high": 2813, - "low": 2813, - "open": 2813, - "volume": 2813 - }, - "open": "2020-11-03T11:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 17091, - "high": 17091, - "low": 17091, - "open": 17091, - "volume": 17091 - }, - "id": 2759444, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T11:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 16845, - "high": 25082, - "low": 16845, - "open": 16845, - "volume": 109211 - }, - "id": 2759447, - "non_hive": { - "close": 2000, - "high": 2995, - "low": 2000, - "open": 2000, - "volume": 12994 - }, - "open": "2020-11-03T11:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 14086, - "high": 2761, - "low": 14086, - "open": 16845, - "volume": 50537 - }, - "id": 2759452, - "non_hive": { - "close": 1672, - "high": 328, - "low": 1672, - "open": 2000, - "volume": 6000 - }, - "open": "2020-11-03T11:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 64968, - "high": 2737, - "low": 14110, - "open": 2737, - "volume": 81815 - }, - "id": 2759456, - "non_hive": { - "close": 7668, - "high": 325, - "low": 1665, - "open": 325, - "volume": 9658 - }, - "open": "2020-11-03T11:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 100000, - "high": 100000, - "low": 100000, - "open": 100000, - "volume": 100000 - }, - "id": 2759459, - "non_hive": { - "close": 11802, - "high": 11802, - "low": 11802, - "open": 11802, - "volume": 11802 - }, - "open": "2020-11-03T11:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 516, - "high": 32503, - "low": 516, - "open": 32503, - "volume": 33019 - }, - "id": 2759462, - "non_hive": { - "close": 60, - "high": 3879, - "low": 60, - "open": 3879, - "volume": 3939 - }, - "open": "2020-11-03T11:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 4650, - "high": 37926, - "low": 4650, - "open": 37926, - "volume": 42576 - }, - "id": 2759465, - "non_hive": { - "close": 555, - "high": 4527, - "low": 555, - "open": 4527, - "volume": 5082 - }, - "open": "2020-11-03T12:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 50276, - "high": 50276, - "low": 50276, - "open": 50276, - "volume": 50276 - }, - "id": 2759469, - "non_hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 6000 - }, - "open": "2020-11-03T12:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 671, - "high": 671, - "low": 671, - "open": 671, - "volume": 671 - }, - "id": 2759472, - "non_hive": { - "close": 80, - "high": 80, - "low": 80, - "open": 80, - "volume": 80 - }, - "open": "2020-11-03T12:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 57424, - "high": 57424, - "low": 57424, - "open": 57424, - "volume": 57424 - }, - "id": 2759474, - "non_hive": { - "close": 6852, - "high": 6852, - "low": 6852, - "open": 6852, - "volume": 6852 - }, - "open": "2020-11-03T12:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 14715, - "high": 14715, - "low": 14715, - "open": 14715, - "volume": 14715 - }, - "id": 2759477, - "non_hive": { - "close": 1756, - "high": 1756, - "low": 1756, - "open": 1756, - "volume": 1756 - }, - "open": "2020-11-03T12:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 16761, - "high": 16761, - "low": 16761, - "open": 16761, - "volume": 33522 - }, - "id": 2759480, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-03T12:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 16761, - "high": 16761, - "low": 16761, - "open": 16761, - "volume": 33522 - }, - "id": 2759483, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-03T12:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 7035, - "high": 7035, - "low": 7035, - "open": 7035, - "volume": 7035 - }, - "id": 2759486, - "non_hive": { - "close": 839, - "high": 839, - "low": 839, - "open": 839, - "volume": 839 - }, - "open": "2020-11-03T12:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 8934, - "high": 8490, - "low": 8934, - "open": 8490, - "volume": 17424 - }, - "id": 2759488, - "non_hive": { - "close": 1064, - "high": 1013, - "low": 1064, - "open": 1013, - "volume": 2077 - }, - "open": "2020-11-03T12:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 2249, - "high": 1082, - "low": 2249, - "open": 1082, - "volume": 3331 - }, - "id": 2759491, - "non_hive": { - "close": 268, - "high": 129, - "low": 268, - "open": 129, - "volume": 397 - }, - "open": "2020-11-03T12:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 12097, - "high": 12097, - "low": 12097, - "open": 12097, - "volume": 12097 - }, - "id": 2759493, - "non_hive": { - "close": 1443, - "high": 1443, - "low": 1443, - "open": 1443, - "volume": 1443 - }, - "open": "2020-11-03T12:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 71, - "high": 71, - "low": 71, - "open": 71, - "volume": 71 - }, - "id": 2759496, - "non_hive": { - "close": 8, - "high": 8, - "low": 8, - "open": 8, - "volume": 8 - }, - "open": "2020-11-03T12:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 519, - "high": 519, - "low": 4662, - "open": 4662, - "volume": 12329 - }, - "id": 2759498, - "non_hive": { - "close": 62, - "high": 62, - "low": 556, - "open": 556, - "volume": 1471 - }, - "open": "2020-11-03T12:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 80, - "high": 5232, - "low": 80, - "open": 5232, - "volume": 5312 - }, - "id": 2759501, - "non_hive": { - "close": 9, - "high": 624, - "low": 9, - "open": 624, - "volume": 633 - }, - "open": "2020-11-03T12:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 277, - "high": 277, - "low": 277, - "open": 277, - "volume": 277 - }, - "id": 2759503, - "non_hive": { - "close": 33, - "high": 33, - "low": 33, - "open": 33, - "volume": 33 - }, - "open": "2020-11-03T12:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 159, - "high": 10141, - "low": 159, - "open": 10141, - "volume": 10300 - }, - "id": 2759505, - "non_hive": { - "close": 18, - "high": 1210, - "low": 18, - "open": 1210, - "volume": 1228 - }, - "open": "2020-11-03T13:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 10002, - "high": 10002, - "low": 10002, - "open": 10002, - "volume": 10002 - }, - "id": 2759509, - "non_hive": { - "close": 1194, - "high": 1194, - "low": 1194, - "open": 1194, - "volume": 1194 - }, - "open": "2020-11-03T13:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 3093, - "high": 3093, - "low": 3093, - "open": 3093, - "volume": 3093 - }, - "id": 2759512, - "non_hive": { - "close": 365, - "high": 365, - "low": 365, - "open": 365, - "volume": 365 - }, - "open": "2020-11-03T13:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 25088, - "high": 25088, - "low": 16751, - "open": 16751, - "volume": 41839 - }, - "id": 2759515, - "non_hive": { - "close": 2995, - "high": 2995, - "low": 1999, - "open": 1999, - "volume": 4994 - }, - "open": "2020-11-03T13:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 14045, - "high": 14045, - "low": 14045, - "open": 14045, - "volume": 14045 - }, - "id": 2759518, - "non_hive": { - "close": 1676, - "high": 1676, - "low": 1676, - "open": 1676, - "volume": 1676 - }, - "open": "2020-11-03T13:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 428642, - "high": 16943, - "low": 428642, - "open": 16943, - "volume": 590528 - }, - "id": 2759521, - "non_hive": { - "close": 50578, - "high": 2000, - "low": 50578, - "open": 2000, - "volume": 69685 - }, - "open": "2020-11-03T13:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 251, - "high": 251, - "low": 251, - "open": 251, - "volume": 251 - }, - "id": 2759525, - "non_hive": { - "close": 30, - "high": 30, - "low": 30, - "open": 30, - "volume": 30 - }, - "open": "2020-11-03T14:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 8, - "high": 8, - "low": 16692, - "open": 16692, - "volume": 16700 - }, - "id": 2759529, - "non_hive": { - "close": 1, - "high": 1, - "low": 1992, - "open": 1992, - "volume": 1993 - }, - "open": "2020-11-03T14:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 49723, - "high": 49723, - "low": 49723, - "open": 49723, - "volume": 49723 - }, - "id": 2759532, - "non_hive": { - "close": 5935, - "high": 5935, - "low": 5935, - "open": 5935, - "volume": 5935 - }, - "open": "2020-11-03T14:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 500550, - "high": 209461, - "low": 500550, - "open": 209461, - "volume": 710011 - }, - "id": 2759535, - "non_hive": { - "close": 59740, - "high": 24999, - "low": 59740, - "open": 24999, - "volume": 84739 - }, - "open": "2020-11-03T14:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 207926, - "high": 207926, - "low": 207926, - "open": 207926, - "volume": 207926 - }, - "id": 2759538, - "non_hive": { - "close": 24818, - "high": 24818, - "low": 24818, - "open": 24818, - "volume": 24818 - }, - "open": "2020-11-03T14:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 25, - "high": 25, - "low": 25, - "open": 25, - "volume": 25 - }, - "id": 2759541, - "non_hive": { - "close": 3, - "high": 3, - "low": 3, - "open": 3, - "volume": 3 - }, - "open": "2020-11-03T14:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 93, - "high": 93, - "low": 93, - "open": 93, - "volume": 93 - }, - "id": 2759543, - "non_hive": { - "close": 11, - "high": 11, - "low": 11, - "open": 11, - "volume": 11 - }, - "open": "2020-11-03T14:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 42376, - "high": 42376, - "low": 42376, - "open": 42376, - "volume": 42376 - }, - "id": 2759546, - "non_hive": { - "close": 4957, - "high": 4957, - "low": 4957, - "open": 4957, - "volume": 4957 - }, - "open": "2020-11-03T14:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 8368, - "high": 8368, - "low": 8368, - "open": 8368, - "volume": 8368 - }, - "id": 2759548, - "non_hive": { - "close": 999, - "high": 999, - "low": 999, - "open": 999, - "volume": 999 - }, - "open": "2020-11-03T14:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 9894, - "high": 17014, - "low": 9894, - "open": 17014, - "volume": 26908 - }, - "id": 2759551, - "non_hive": { - "close": 1181, - "high": 2031, - "low": 1181, - "open": 2031, - "volume": 3212 - }, - "open": "2020-11-03T14:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 972, - "high": 972, - "low": 972, - "open": 972, - "volume": 972 - }, - "id": 2759553, - "non_hive": { - "close": 116, - "high": 116, - "low": 116, - "open": 116, - "volume": 116 - }, - "open": "2020-11-03T14:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 96722, - "high": 96722, - "low": 164724, - "open": 164724, - "volume": 402774 - }, - "id": 2759556, - "non_hive": { - "close": 11549, - "high": 11549, - "low": 19653, - "open": 19653, - "volume": 48068 - }, - "open": "2020-11-03T15:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 62449, - "high": 62449, - "low": 62449, - "open": 62449, - "volume": 62449 - }, - "id": 2759560, - "non_hive": { - "close": 7457, - "high": 7457, - "low": 7457, - "open": 7457, - "volume": 7457 - }, - "open": "2020-11-03T15:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 133016, - "high": 92733, - "low": 133016, - "open": 92733, - "volume": 225749 - }, - "id": 2759562, - "non_hive": { - "close": 15695, - "high": 11073, - "low": 15695, - "open": 11073, - "volume": 26768 - }, - "open": "2020-11-03T15:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 6661, - "high": 6661, - "low": 220606, - "open": 16945, - "volume": 244212 - }, - "id": 2759564, - "non_hive": { - "close": 795, - "high": 795, - "low": 26035, - "open": 2000, - "volume": 28830 - }, - "open": "2020-11-03T15:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 135751, - "high": 16945, - "low": 135751, - "open": 16945, - "volume": 1000000 - }, - "id": 2759568, - "non_hive": { - "close": 16018, - "high": 2000, - "low": 16018, - "open": 2000, - "volume": 118018 - }, - "open": "2020-11-03T15:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 1091, - "high": 234467, - "low": 1091, - "open": 16766, - "volume": 252324 - }, - "id": 2759571, - "non_hive": { - "close": 130, - "high": 27970, - "low": 130, - "open": 1999, - "volume": 30099 - }, - "open": "2020-11-03T15:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 1915, - "high": 1915, - "low": 1915, - "open": 1915, - "volume": 1915 - }, - "id": 2759573, - "non_hive": { - "close": 227, - "high": 227, - "low": 227, - "open": 227, - "volume": 227 - }, - "open": "2020-11-03T15:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 378585, - "high": 16824, - "low": 378585, - "open": 16824, - "volume": 827605 - }, - "id": 2759576, - "non_hive": { - "close": 44673, - "high": 2000, - "low": 44673, - "open": 2000, - "volume": 97730 - }, - "open": "2020-11-03T15:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 167, - "high": 167, - "low": 167, - "open": 167, - "volume": 167 - }, - "id": 2759578, - "non_hive": { - "close": 20, - "high": 20, - "low": 20, - "open": 20, - "volume": 20 - }, - "open": "2020-11-03T15:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 19105, - "high": 6385, - "low": 19105, - "open": 6385, - "volume": 25490 - }, - "id": 2759581, - "non_hive": { - "close": 2280, - "high": 762, - "low": 2280, - "open": 762, - "volume": 3042 - }, - "open": "2020-11-03T15:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 54450, - "high": 54450, - "low": 16750, - "open": 16750, - "volume": 71200 - }, - "id": 2759583, - "non_hive": { - "close": 6501, - "high": 6501, - "low": 1999, - "open": 1999, - "volume": 8500 - }, - "open": "2020-11-03T15:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 80547, - "high": 16997, - "low": 80547, - "open": 16997, - "volume": 140000 - }, - "id": 2759586, - "non_hive": { - "close": 9471, - "high": 2000, - "low": 9471, - "open": 2000, - "volume": 16465 - }, - "open": "2020-11-03T15:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 16829, - "high": 16829, - "low": 16829, - "open": 16829, - "volume": 33658 - }, - "id": 2759588, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-03T16:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 16829, - "high": 16829, - "low": 16829, - "open": 16829, - "volume": 33658 - }, - "id": 2759593, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-03T16:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 11006, - "high": 11006, - "low": 16829, - "open": 16829, - "volume": 27835 - }, - "id": 2759596, - "non_hive": { - "close": 1308, - "high": 1308, - "low": 2000, - "open": 2000, - "volume": 3308 - }, - "open": "2020-11-03T16:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 51754, - "high": 9503, - "low": 51754, - "open": 9503, - "volume": 61257 - }, - "id": 2759599, - "non_hive": { - "close": 6085, - "high": 1134, - "low": 6085, - "open": 1134, - "volume": 7219 - }, - "open": "2020-11-03T16:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 154520, - "high": 9642, - "low": 154520, - "open": 9642, - "volume": 164162 - }, - "id": 2759601, - "non_hive": { - "close": 18170, - "high": 1134, - "low": 18170, - "open": 1134, - "volume": 19304 - }, - "open": "2020-11-03T16:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 16756, - "high": 16756, - "low": 16756, - "open": 16756, - "volume": 16756 - }, - "id": 2759604, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T16:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 156, - "high": 10174, - "low": 156, - "open": 10174, - "volume": 10330 - }, - "id": 2759607, - "non_hive": { - "close": 18, - "high": 1214, - "low": 18, - "open": 1214, - "volume": 1232 - }, - "open": "2020-11-03T16:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 16998, - "high": 16998, - "low": 16998, - "open": 16998, - "volume": 16998 - }, - "id": 2759609, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T16:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 17007, - "high": 17007, - "low": 17007, - "open": 17007, - "volume": 17007 - }, - "id": 2759612, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T16:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 7654, - "high": 12722, - "low": 7654, - "open": 12722, - "volume": 20376 - }, - "id": 2759615, - "non_hive": { - "close": 900, - "high": 1496, - "low": 900, - "open": 1496, - "volume": 2396 - }, - "open": "2020-11-03T16:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 18418, - "high": 18418, - "low": 18418, - "open": 18418, - "volume": 18418 - }, - "id": 2759618, - "non_hive": { - "close": 2164, - "high": 2164, - "low": 2164, - "open": 2164, - "volume": 2164 - }, - "open": "2020-11-03T16:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 10583, - "high": 62299, - "low": 10583, - "open": 62299, - "volume": 72882 - }, - "id": 2759621, - "non_hive": { - "close": 1244, - "high": 7326, - "low": 1244, - "open": 7326, - "volume": 8570 - }, - "open": "2020-11-03T16:48:00", - "seconds": 60 - }, - { - "hive": { - "close": 17015, - "high": 17015, - "low": 17015, - "open": 17015, - "volume": 17015 - }, - "id": 2759624, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T16:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 17015, - "high": 17015, - "low": 17015, - "open": 17015, - "volume": 34030 - }, - "id": 2759627, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-03T16:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 17015, - "high": 17015, - "low": 17015, - "open": 17015, - "volume": 17015 - }, - "id": 2759630, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T16:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 800830, - "high": 341, - "low": 800830, - "open": 341, - "volume": 823158 - }, - "id": 2759633, - "non_hive": { - "close": 93697, - "high": 40, - "low": 93697, - "open": 40, - "volume": 96311 - }, - "open": "2020-11-03T16:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 16718, - "high": 16718, - "low": 17094, - "open": 17094, - "volume": 33812 - }, - "id": 2759635, - "non_hive": { - "close": 1956, - "high": 1956, - "low": 1999, - "open": 1999, - "volume": 3955 - }, - "open": "2020-11-03T17:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 75893, - "high": 676, - "low": 75893, - "open": 676, - "volume": 160124 - }, - "id": 2759639, - "non_hive": { - "close": 8839, - "high": 79, - "low": 8839, - "open": 79, - "volume": 18651 - }, - "open": "2020-11-03T17:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 12907, - "high": 12907, - "low": 12907, - "open": 12907, - "volume": 12907 - }, - "id": 2759641, - "non_hive": { - "close": 1517, - "high": 1517, - "low": 1517, - "open": 1517, - "volume": 1517 - }, - "open": "2020-11-03T17:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 17091, - "high": 17091, - "low": 17091, - "open": 17091, - "volume": 17091 - }, - "id": 2759644, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T17:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 17090, - "high": 17090, - "low": 17090, - "open": 17090, - "volume": 17090 - }, - "id": 2759647, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T17:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 4162, - "high": 12926, - "low": 4162, - "open": 12926, - "volume": 17088 - }, - "id": 2759650, - "non_hive": { - "close": 487, - "high": 1519, - "low": 487, - "open": 1519, - "volume": 2006 - }, - "open": "2020-11-03T17:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 17036, - "high": 12926, - "low": 17036, - "open": 12926, - "volume": 29962 - }, - "id": 2759652, - "non_hive": { - "close": 1994, - "high": 1513, - "low": 1994, - "open": 1513, - "volume": 3507 - }, - "open": "2020-11-03T17:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 17089, - "high": 17089, - "low": 17089, - "open": 17089, - "volume": 17089 - }, - "id": 2759655, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T17:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 17094, - "high": 17094, - "low": 9741, - "open": 17094, - "volume": 43929 - }, - "id": 2759657, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1134, - "open": 1999, - "volume": 5132 - }, - "open": "2020-11-03T17:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 17094, - "high": 17094, - "low": 17094, - "open": 17094, - "volume": 34188 - }, - "id": 2759661, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-03T17:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 17094, - "high": 17094, - "low": 17094, - "open": 17094, - "volume": 17094 - }, - "id": 2759664, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T17:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 17094, - "high": 17094, - "low": 17094, - "open": 17094, - "volume": 17094 - }, - "id": 2759666, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T17:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 17093, - "high": 17093, - "low": 17093, - "open": 17093, - "volume": 17093 - }, - "id": 2759669, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T17:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 9783, - "high": 9783, - "low": 17093, - "open": 17093, - "volume": 26876 - }, - "id": 2759671, - "non_hive": { - "close": 1150, - "high": 1150, - "low": 2000, - "open": 2000, - "volume": 3150 - }, - "open": "2020-11-03T17:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 17089, - "high": 17089, - "low": 17089, - "open": 17089, - "volume": 17089 - }, - "id": 2759674, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T17:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 17090, - "high": 17090, - "low": 17090, - "open": 17090, - "volume": 17090 - }, - "id": 2759677, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T17:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 31130, - "high": 17167, - "low": 31130, - "open": 17167, - "volume": 48297 - }, - "id": 2759680, - "non_hive": { - "close": 3626, - "high": 2000, - "low": 3626, - "open": 2000, - "volume": 5626 - }, - "open": "2020-11-03T17:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 17093, - "high": 17093, - "low": 17093, - "open": 17093, - "volume": 17093 - }, - "id": 2759682, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T17:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 9776, - "high": 9776, - "low": 9776, - "open": 9776, - "volume": 9776 - }, - "id": 2759684, - "non_hive": { - "close": 1144, - "high": 1144, - "low": 1144, - "open": 1144, - "volume": 1144 - }, - "open": "2020-11-03T17:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 111991, - "high": 62416, - "low": 111991, - "open": 62416, - "volume": 198073 - }, - "id": 2759687, - "non_hive": { - "close": 12991, - "high": 7271, - "low": 12991, - "open": 7271, - "volume": 23018 - }, - "open": "2020-11-03T17:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 70517, - "high": 70517, - "low": 70517, - "open": 70517, - "volume": 70517 - }, - "id": 2759689, - "non_hive": { - "close": 8180, - "high": 8180, - "low": 8180, - "open": 8180, - "volume": 8180 - }, - "open": "2020-11-03T17:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 129310, - "high": 129310, - "low": 129310, - "open": 129310, - "volume": 129310 - }, - "id": 2759691, - "non_hive": { - "close": 15000, - "high": 15000, - "low": 15000, - "open": 15000, - "volume": 15000 - }, - "open": "2020-11-03T17:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 1973100, - "high": 1973100, - "low": 1973100, - "open": 1973100, - "volume": 1973100 - }, - "id": 2759694, - "non_hive": { - "close": 228879, - "high": 228879, - "low": 228879, - "open": 228879, - "volume": 228879 - }, - "open": "2020-11-03T18:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 9, - "high": 9, - "low": 9, - "open": 9, - "volume": 9 - }, - "id": 2759698, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 1, - "volume": 1 - }, - "open": "2020-11-03T18:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 10173, - "high": 10747, - "low": 10173, - "open": 10747, - "volume": 20920 - }, - "id": 2759700, - "non_hive": { - "close": 1195, - "high": 1263, - "low": 1195, - "open": 1263, - "volume": 2458 - }, - "open": "2020-11-03T18:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 30432, - "high": 30432, - "low": 6846, - "open": 6846, - "volume": 37278 - }, - "id": 2759704, - "non_hive": { - "close": 3576, - "high": 3576, - "low": 804, - "open": 804, - "volume": 4380 - }, - "open": "2020-11-03T18:13:00", - "seconds": 60 - }, - { - "hive": { - "close": 20800, - "high": 20800, - "low": 20800, - "open": 20800, - "volume": 20800 - }, - "id": 2759706, - "non_hive": { - "close": 2443, - "high": 2443, - "low": 2443, - "open": 2443, - "volume": 2443 - }, - "open": "2020-11-03T18:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 17007, - "high": 17007, - "low": 1584, - "open": 1584, - "volume": 18591 - }, - "id": 2759709, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 185, - "open": 185, - "volume": 2184 - }, - "open": "2020-11-03T18:48:00", - "seconds": 60 - }, - { - "hive": { - "close": 49312, - "high": 49312, - "low": 49312, - "open": 49312, - "volume": 49312 - }, - "id": 2759712, - "non_hive": { - "close": 5799, - "high": 5799, - "low": 5799, - "open": 5799, - "volume": 5799 - }, - "open": "2020-11-03T18:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 9414, - "high": 9414, - "low": 9414, - "open": 9414, - "volume": 9414 - }, - "id": 2759714, - "non_hive": { - "close": 1106, - "high": 1106, - "low": 1106, - "open": 1106, - "volume": 1106 - }, - "open": "2020-11-03T19:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 1796, - "high": 1796, - "low": 1796, - "open": 1796, - "volume": 1796 - }, - "id": 2759718, - "non_hive": { - "close": 211, - "high": 211, - "low": 211, - "open": 211, - "volume": 211 - }, - "open": "2020-11-03T19:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 5806, - "high": 5806, - "low": 5806, - "open": 5806, - "volume": 5806 - }, - "id": 2759720, - "non_hive": { - "close": 682, - "high": 682, - "low": 682, - "open": 682, - "volume": 682 - }, - "open": "2020-11-03T19:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 93753, - "high": 93753, - "low": 93753, - "open": 93753, - "volume": 93753 - }, - "id": 2759723, - "non_hive": { - "close": 11014, - "high": 11014, - "low": 11014, - "open": 11014, - "volume": 11014 - }, - "open": "2020-11-03T19:21:00", - "seconds": 60 - }, - { - "hive": { - "close": 17036, - "high": 17036, - "low": 17036, - "open": 17036, - "volume": 34072 - }, - "id": 2759725, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-03T19:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 3345, - "high": 3345, - "low": 3345, - "open": 3345, - "volume": 3345 - }, - "id": 2759728, - "non_hive": { - "close": 393, - "high": 393, - "low": 393, - "open": 393, - "volume": 393 - }, - "open": "2020-11-03T19:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 22396, - "high": 22396, - "low": 22396, - "open": 22396, - "volume": 22396 - }, - "id": 2759731, - "non_hive": { - "close": 2631, - "high": 2631, - "low": 2631, - "open": 2631, - "volume": 2631 - }, - "open": "2020-11-03T19:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 17025, - "high": 17025, - "low": 17025, - "open": 17025, - "volume": 17025 - }, - "id": 2759734, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T19:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 2925, - "high": 2925, - "low": 17016, - "open": 17016, - "volume": 32941 - }, - "id": 2759736, - "non_hive": { - "close": 344, - "high": 344, - "low": 1999, - "open": 1999, - "volume": 3871 - }, - "open": "2020-11-03T19:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 866, - "high": 866, - "low": 866, - "open": 866, - "volume": 866 - }, - "id": 2759739, - "non_hive": { - "close": 101, - "high": 101, - "low": 101, - "open": 101, - "volume": 101 - }, - "open": "2020-11-03T19:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 5136, - "high": 5136, - "low": 5136, - "open": 5136, - "volume": 5136 - }, - "id": 2759741, - "non_hive": { - "close": 604, - "high": 604, - "low": 604, - "open": 604, - "volume": 604 - }, - "open": "2020-11-03T19:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 88941, - "high": 88941, - "low": 88941, - "open": 88941, - "volume": 88941 - }, - "id": 2759744, - "non_hive": { - "close": 10459, - "high": 10459, - "low": 10459, - "open": 10459, - "volume": 10459 - }, - "open": "2020-11-03T20:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 16998, - "high": 16998, - "low": 16998, - "open": 16998, - "volume": 16998 - }, - "id": 2759748, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T20:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 16998, - "high": 16998, - "low": 16998, - "open": 16998, - "volume": 33996 - }, - "id": 2759751, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-03T20:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 16998, - "high": 16998, - "low": 16998, - "open": 16998, - "volume": 16998 - }, - "id": 2759754, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-03T20:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 42169, - "high": 42169, - "low": 42169, - "open": 42169, - "volume": 42169 - }, - "id": 2759756, - "non_hive": { - "close": 5000, - "high": 5000, - "low": 5000, - "open": 5000, - "volume": 5000 - }, - "open": "2020-11-03T20:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 368, - "high": 42243, - "low": 368, - "open": 42243, - "volume": 42611 - }, - "id": 2759759, - "non_hive": { - "close": 43, - "high": 5000, - "low": 43, - "open": 5000, - "volume": 5043 - }, - "open": "2020-11-03T20:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 997, - "high": 997, - "low": 997, - "open": 997, - "volume": 997 - }, - "id": 2759762, - "non_hive": { - "close": 118, - "high": 118, - "low": 118, - "open": 118, - "volume": 118 - }, - "open": "2020-11-03T20:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 13348, - "high": 13348, - "low": 13348, - "open": 13348, - "volume": 13348 - }, - "id": 2759764, - "non_hive": { - "close": 1579, - "high": 1579, - "low": 1579, - "open": 1579, - "volume": 1579 - }, - "open": "2020-11-03T20:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 16907, - "high": 16907, - "low": 16907, - "open": 16907, - "volume": 16907 - }, - "id": 2759766, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T20:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 16909, - "high": 16909, - "low": 16909, - "open": 16909, - "volume": 16909 - }, - "id": 2759768, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T20:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 16926, - "high": 16926, - "low": 16926, - "open": 16926, - "volume": 16926 - }, - "id": 2759771, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T20:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 12, - "high": 1533, - "low": 12, - "open": 1533, - "volume": 1545 - }, - "id": 2759773, - "non_hive": { - "close": 1, - "high": 181, - "low": 1, - "open": 181, - "volume": 182 - }, - "open": "2020-11-03T20:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 16633, - "high": 16633, - "low": 16633, - "open": 16633, - "volume": 16633 - }, - "id": 2759775, - "non_hive": { - "close": 1967, - "high": 1967, - "low": 1967, - "open": 1967, - "volume": 1967 - }, - "open": "2020-11-03T20:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 244, - "high": 244, - "low": 3305, - "open": 3305, - "volume": 3549 - }, - "id": 2759778, - "non_hive": { - "close": 29, - "high": 29, - "low": 392, - "open": 392, - "volume": 421 - }, - "open": "2020-11-03T20:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 21649, - "high": 21649, - "low": 21649, - "open": 21649, - "volume": 21649 - }, - "id": 2759781, - "non_hive": { - "close": 2584, - "high": 2584, - "low": 2584, - "open": 2584, - "volume": 2584 - }, - "open": "2020-11-03T20:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 12284, - "high": 12284, - "low": 12284, - "open": 12284, - "volume": 12284 - }, - "id": 2759783, - "non_hive": { - "close": 1466, - "high": 1466, - "low": 1466, - "open": 1466, - "volume": 1466 - }, - "open": "2020-11-03T20:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 24078, - "high": 24078, - "low": 24078, - "open": 24078, - "volume": 24078 - }, - "id": 2759786, - "non_hive": { - "close": 2865, - "high": 2865, - "low": 2865, - "open": 2865, - "volume": 2865 - }, - "open": "2020-11-03T20:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 57958, - "high": 57958, - "low": 57958, - "open": 57958, - "volume": 57958 - }, - "id": 2759789, - "non_hive": { - "close": 6897, - "high": 6897, - "low": 6897, - "open": 6897, - "volume": 6897 - }, - "open": "2020-11-03T21:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 10226, - "high": 10226, - "low": 10226, - "open": 10226, - "volume": 10226 - }, - "id": 2759793, - "non_hive": { - "close": 1221, - "high": 1221, - "low": 1221, - "open": 1221, - "volume": 1221 - }, - "open": "2020-11-03T21:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 11164, - "high": 11164, - "low": 11164, - "open": 11164, - "volume": 11164 - }, - "id": 2759796, - "non_hive": { - "close": 1333, - "high": 1333, - "low": 1333, - "open": 1333, - "volume": 1333 - }, - "open": "2020-11-03T21:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 41566, - "high": 41566, - "low": 41566, - "open": 41566, - "volume": 41566 - }, - "id": 2759799, - "non_hive": { - "close": 4963, - "high": 4963, - "low": 4963, - "open": 4963, - "volume": 4963 - }, - "open": "2020-11-03T21:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 140, - "high": 17069, - "low": 140, - "open": 17069, - "volume": 17209 - }, - "id": 2759801, - "non_hive": { - "close": 16, - "high": 2038, - "low": 16, - "open": 2038, - "volume": 2054 - }, - "open": "2020-11-03T21:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 13727, - "high": 20100, - "low": 13727, - "open": 20100, - "volume": 33827 - }, - "id": 2759804, - "non_hive": { - "close": 1639, - "high": 2400, - "low": 1639, - "open": 2400, - "volume": 4039 - }, - "open": "2020-11-03T21:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 16784, - "high": 16784, - "low": 16784, - "open": 16784, - "volume": 16784 - }, - "id": 2759806, - "non_hive": { - "close": 2004, - "high": 2004, - "low": 2004, - "open": 2004, - "volume": 2004 - }, - "open": "2020-11-03T21:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 20000, - "high": 16805, - "low": 20000, - "open": 16805, - "volume": 36805 - }, - "id": 2759808, - "non_hive": { - "close": 2380, - "high": 2000, - "low": 2380, - "open": 2000, - "volume": 4380 - }, - "open": "2020-11-03T21:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 16807, - "high": 16807, - "low": 16807, - "open": 16807, - "volume": 16807 - }, - "id": 2759811, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-03T21:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 20002, - "high": 16805, - "low": 7424, - "open": 16805, - "volume": 64231 - }, - "id": 2759813, - "non_hive": { - "close": 2380, - "high": 2000, - "low": 883, - "open": 2000, - "volume": 7643 - }, - "open": "2020-11-03T21:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 50, - "high": 50, - "low": 50, - "open": 50, - "volume": 50 - }, - "id": 2759818, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-03T21:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 176303, - "high": 176303, - "low": 9469, - "open": 9469, - "volume": 185772 - }, - "id": 2759820, - "non_hive": { - "close": 20980, - "high": 20980, - "low": 1126, - "open": 1126, - "volume": 22106 - }, - "open": "2020-11-03T21:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 15756, - "high": 3292, - "low": 15756, - "open": 3292, - "volume": 19048 - }, - "id": 2759822, - "non_hive": { - "close": 1875, - "high": 393, - "low": 1875, - "open": 393, - "volume": 2268 - }, - "open": "2020-11-03T21:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 30000, - "high": 16805, - "low": 30000, - "open": 16805, - "volume": 46805 - }, - "id": 2759825, - "non_hive": { - "close": 3570, - "high": 2000, - "low": 3570, - "open": 2000, - "volume": 5570 - }, - "open": "2020-11-03T21:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 16808, - "high": 31778, - "low": 246, - "open": 31778, - "volume": 48832 - }, - "id": 2759828, - "non_hive": { - "close": 1999, - "high": 3780, - "low": 28, - "open": 3780, - "volume": 5807 - }, - "open": "2020-11-03T21:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 20209, - "high": 3538, - "low": 20, - "open": 10291, - "volume": 34160 - }, - "id": 2759831, - "non_hive": { - "close": 2404, - "high": 421, - "low": 2, - "open": 1224, - "volume": 4063 - }, - "open": "2020-11-03T21:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 1548, - "high": 1294, - "low": 1305, - "open": 1294, - "volume": 12921 - }, - "id": 2759835, - "non_hive": { - "close": 184, - "high": 154, - "low": 153, - "open": 154, - "volume": 1535 - }, - "open": "2020-11-03T21:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 958, - "high": 958, - "low": 958, - "open": 958, - "volume": 958 - }, - "id": 2759839, - "non_hive": { - "close": 114, - "high": 114, - "low": 114, - "open": 114, - "volume": 114 - }, - "open": "2020-11-03T21:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 4430, - "high": 4430, - "low": 4430, - "open": 4430, - "volume": 4430 - }, - "id": 2759841, - "non_hive": { - "close": 527, - "high": 527, - "low": 527, - "open": 527, - "volume": 527 - }, - "open": "2020-11-03T21:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 26143, - "high": 26143, - "low": 26143, - "open": 26143, - "volume": 26143 - }, - "id": 2759843, - "non_hive": { - "close": 3110, - "high": 3110, - "low": 3110, - "open": 3110, - "volume": 3110 - }, - "open": "2020-11-03T21:48:00", - "seconds": 60 - }, - { - "hive": { - "close": 50445, - "high": 50445, - "low": 50445, - "open": 50445, - "volume": 50445 - }, - "id": 2759845, - "non_hive": { - "close": 6001, - "high": 6001, - "low": 6001, - "open": 6001, - "volume": 6001 - }, - "open": "2020-11-03T21:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 146621, - "high": 14379, - "low": 146621, - "open": 14379, - "volume": 161000 - }, - "id": 2759848, - "non_hive": { - "close": 17301, - "high": 1711, - "low": 17301, - "open": 1711, - "volume": 19012 - }, - "open": "2020-11-03T22:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 61712, - "high": 16996, - "low": 61712, - "open": 16996, - "volume": 78708 - }, - "id": 2759852, - "non_hive": { - "close": 7261, - "high": 2000, - "low": 7261, - "open": 2000, - "volume": 9261 - }, - "open": "2020-11-03T22:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 320, - "high": 16891, - "low": 320, - "open": 16808, - "volume": 76876 - }, - "id": 2759855, - "non_hive": { - "close": 37, - "high": 2010, - "low": 37, - "open": 1999, - "volume": 9143 - }, - "open": "2020-11-03T22:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 621, - "high": 14548, - "low": 115, - "open": 14548, - "volume": 15284 - }, - "id": 2759859, - "non_hive": { - "close": 73, - "high": 1731, - "low": 13, - "open": 1731, - "volume": 1817 - }, - "open": "2020-11-03T22:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 266555, - "high": 266555, - "low": 924, - "open": 924, - "volume": 1095706 - }, - "id": 2759861, - "non_hive": { - "close": 31720, - "high": 31720, - "low": 109, - "open": 109, - "volume": 130382 - }, - "open": "2020-11-03T22:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 8404, - "high": 8404, - "low": 8404, - "open": 8404, - "volume": 8404 - }, - "id": 2759864, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-11-03T22:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 8474, - "high": 8474, - "low": 8474, - "open": 8474, - "volume": 8474 - }, - "id": 2759866, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-11-03T22:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 16808, - "high": 16808, - "low": 8405, - "open": 8405, - "volume": 25213 - }, - "id": 2759869, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 1000, - "open": 1000, - "volume": 3000 - }, - "open": "2020-11-03T22:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 4203, - "high": 4203, - "low": 8474, - "open": 8474, - "volume": 12677 - }, - "id": 2759872, - "non_hive": { - "close": 500, - "high": 500, - "low": 1008, - "open": 1008, - "volume": 1508 - }, - "open": "2020-11-03T22:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 24112, - "high": 24112, - "low": 24112, - "open": 24112, - "volume": 24112 - }, - "id": 2759874, - "non_hive": { - "close": 2869, - "high": 2869, - "low": 2869, - "open": 2869, - "volume": 2869 - }, - "open": "2020-11-03T22:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 11174, - "high": 16999, - "low": 11174, - "open": 16999, - "volume": 75000 - }, - "id": 2759876, - "non_hive": { - "close": 1314, - "high": 2000, - "low": 1314, - "open": 2000, - "volume": 8823 - }, - "open": "2020-11-03T22:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 340, - "high": 16808, - "low": 340, - "open": 16808, - "volume": 17148 - }, - "id": 2759879, - "non_hive": { - "close": 40, - "high": 1999, - "low": 40, - "open": 1999, - "volume": 2039 - }, - "open": "2020-11-03T22:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 12203, - "high": 62797, - "low": 12203, - "open": 62797, - "volume": 75000 - }, - "id": 2759882, - "non_hive": { - "close": 1435, - "high": 7388, - "low": 1435, - "open": 7388, - "volume": 8823 - }, - "open": "2020-11-03T22:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 4252, - "high": 99008, - "low": 4252, - "open": 12394, - "volume": 174265 - }, - "id": 2759884, - "non_hive": { - "close": 500, - "high": 11782, - "low": 500, - "open": 1474, - "volume": 20730 - }, - "open": "2020-11-03T22:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 4252, - "high": 4252, - "low": 4252, - "open": 4252, - "volume": 4252 - }, - "id": 2759887, - "non_hive": { - "close": 505, - "high": 505, - "low": 505, - "open": 505, - "volume": 505 - }, - "open": "2020-11-03T22:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 176419, - "high": 176419, - "low": 4210, - "open": 4210, - "volume": 180629 - }, - "id": 2759890, - "non_hive": { - "close": 20953, - "high": 20953, - "low": 500, - "open": 500, - "volume": 21453 - }, - "open": "2020-11-03T22:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759892, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 250 - }, - "open": "2020-11-03T22:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759894, - "non_hive": { - "close": 252, - "high": 252, - "low": 252, - "open": 252, - "volume": 252 - }, - "open": "2020-11-03T22:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 6327, - "high": 6327, - "low": 6327, - "open": 6327, - "volume": 6327 - }, - "id": 2759896, - "non_hive": { - "close": 750, - "high": 750, - "low": 750, - "open": 750, - "volume": 750 - }, - "open": "2020-11-03T22:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759899, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 250 - }, - "open": "2020-11-03T22:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759901, - "non_hive": { - "close": 252, - "high": 252, - "low": 252, - "open": 252, - "volume": 252 - }, - "open": "2020-11-03T22:57:00", - "seconds": 60 - }, - { - "hive": { - "close": 4252, - "high": 4252, - "low": 4252, - "open": 4252, - "volume": 4252 - }, - "id": 2759903, - "non_hive": { - "close": 500, - "high": 500, - "low": 500, - "open": 500, - "volume": 500 - }, - "open": "2020-11-03T22:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759905, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 250 - }, - "open": "2020-11-03T23:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 6328, - "high": 4252, - "low": 6328, - "open": 4252, - "volume": 10580 - }, - "id": 2759909, - "non_hive": { - "close": 750, - "high": 504, - "low": 750, - "open": 504, - "volume": 1254 - }, - "open": "2020-11-03T23:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 546, - "high": 546, - "low": 546, - "open": 546, - "volume": 546 - }, - "id": 2759912, - "non_hive": { - "close": 65, - "high": 65, - "low": 65, - "open": 65, - "volume": 65 - }, - "open": "2020-11-03T23:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759914, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 250 - }, - "open": "2020-11-03T23:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759917, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 250 - }, - "open": "2020-11-03T23:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759919, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 250 - }, - "open": "2020-11-03T23:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759922, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 250 - }, - "open": "2020-11-03T23:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 851, - "high": 851, - "low": 851, - "open": 851, - "volume": 851 - }, - "id": 2759924, - "non_hive": { - "close": 100, - "high": 100, - "low": 100, - "open": 100, - "volume": 100 - }, - "open": "2020-11-03T23:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 7028, - "high": 7028, - "low": 4215, - "open": 12601, - "volume": 23844 - }, - "id": 2759927, - "non_hive": { - "close": 836, - "high": 836, - "low": 501, - "open": 1498, - "volume": 2835 - }, - "open": "2020-11-03T23:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 447, - "high": 447, - "low": 447, - "open": 447, - "volume": 447 - }, - "id": 2759931, - "non_hive": { - "close": 53, - "high": 53, - "low": 53, - "open": 53, - "volume": 53 - }, - "open": "2020-11-03T23:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 5162, - "high": 5162, - "low": 5162, - "open": 5162, - "volume": 5162 - }, - "id": 2759933, - "non_hive": { - "close": 614, - "high": 614, - "low": 614, - "open": 614, - "volume": 614 - }, - "open": "2020-11-03T23:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 2126, - "high": 2126, - "low": 2126, - "open": 2126, - "volume": 2126 - }, - "id": 2759936, - "non_hive": { - "close": 250, - "high": 250, - "low": 250, - "open": 250, - "volume": 250 - }, - "open": "2020-11-03T23:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 6375, - "high": 6375, - "low": 6375, - "open": 6375, - "volume": 6375 - }, - "id": 2759939, - "non_hive": { - "close": 750, - "high": 750, - "low": 750, - "open": 750, - "volume": 750 - }, - "open": "2020-11-03T23:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 1757, - "high": 1757, - "low": 1757, - "open": 1757, - "volume": 1757 - }, - "id": 2759942, - "non_hive": { - "close": 209, - "high": 209, - "low": 209, - "open": 209, - "volume": 209 - }, - "open": "2020-11-03T23:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 3371, - "high": 3371, - "low": 3371, - "open": 3371, - "volume": 3371 - }, - "id": 2759945, - "non_hive": { - "close": 401, - "high": 401, - "low": 401, - "open": 401, - "volume": 401 - }, - "open": "2020-11-03T23:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 6375, - "high": 6375, - "low": 6375, - "open": 6375, - "volume": 6375 - }, - "id": 2759948, - "non_hive": { - "close": 750, - "high": 750, - "low": 750, - "open": 750, - "volume": 750 - }, - "open": "2020-11-03T23:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 850, - "high": 850, - "low": 850, - "open": 850, - "volume": 4250 - }, - "id": 2759950, - "non_hive": { - "close": 100, - "high": 100, - "low": 100, - "open": 100, - "volume": 500 - }, - "open": "2020-11-03T23:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 57998, - "high": 16997, - "low": 57998, - "open": 16997, - "volume": 74995 - }, - "id": 2759954, - "non_hive": { - "close": 6824, - "high": 2000, - "low": 6824, - "open": 2000, - "volume": 8824 - }, - "open": "2020-11-04T00:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 10178, - "high": 10178, - "low": 21765, - "open": 16998, - "volume": 211028 - }, - "id": 2759959, - "non_hive": { - "close": 1210, - "high": 1210, - "low": 2559, - "open": 2000, - "volume": 24839 - }, - "open": "2020-11-04T00:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 340, - "high": 850, - "low": 850, - "open": 850, - "volume": 3740 - }, - "id": 2759962, - "non_hive": { - "close": 40, - "high": 100, - "low": 100, - "open": 100, - "volume": 440 - }, - "open": "2020-11-04T00:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 3831333, - "high": 504, - "low": 3831333, - "open": 504, - "volume": 5000000 - }, - "id": 2759965, - "non_hive": { - "close": 444817, - "high": 60, - "low": 444817, - "open": 60, - "volume": 580800 - }, - "open": "2020-11-04T00:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 2712730, - "high": 270045, - "low": 2712730, - "open": 270045, - "volume": 3000000 - }, - "id": 2759968, - "non_hive": { - "close": 314947, - "high": 31356, - "low": 314947, - "open": 31356, - "volume": 348303 - }, - "open": "2020-11-04T00:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 455, - "high": 455, - "low": 455, - "open": 455, - "volume": 455 - }, - "id": 2759970, - "non_hive": { - "close": 54, - "high": 54, - "low": 54, - "open": 54, - "volume": 54 - }, - "open": "2020-11-04T00:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 1205, - "high": 11004, - "low": 1205, - "open": 11004, - "volume": 96172 - }, - "id": 2759973, - "non_hive": { - "close": 139, - "high": 1308, - "low": 139, - "open": 1308, - "volume": 11427 - }, - "open": "2020-11-04T00:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 88, - "high": 765, - "low": 88, - "open": 161237, - "volume": 168458 - }, - "id": 2759978, - "non_hive": { - "close": 10, - "high": 91, - "low": 10, - "open": 19174, - "volume": 20032 - }, - "open": "2020-11-04T00:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 55, - "high": 3635, - "low": 55, - "open": 3635, - "volume": 3690 - }, - "id": 2759981, - "non_hive": { - "close": 6, - "high": 432, - "low": 6, - "open": 432, - "volume": 438 - }, - "open": "2020-11-04T00:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 16818, - "high": 16818, - "low": 16818, - "open": 16818, - "volume": 16818 - }, - "id": 2759984, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-04T00:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 16220, - "high": 16220, - "low": 16220, - "open": 16220, - "volume": 16220 - }, - "id": 2759986, - "non_hive": { - "close": 1928, - "high": 1928, - "low": 1928, - "open": 1928, - "volume": 1928 - }, - "open": "2020-11-04T00:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 598, - "high": 598, - "low": 598, - "open": 598, - "volume": 598 - }, - "id": 2759988, - "non_hive": { - "close": 71, - "high": 71, - "low": 71, - "open": 71, - "volume": 71 - }, - "open": "2020-11-04T00:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 542, - "high": 35208, - "low": 542, - "open": 35208, - "volume": 35750 - }, - "id": 2759990, - "non_hive": { - "close": 62, - "high": 4185, - "low": 62, - "open": 4185, - "volume": 4247 - }, - "open": "2020-11-04T00:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 16939, - "high": 16939, - "low": 1600, - "open": 1600, - "volume": 19136 - }, - "id": 2759993, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 187, - "open": 187, - "volume": 2256 - }, - "open": "2020-11-04T01:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 683, - "high": 683, - "low": 683, - "open": 683, - "volume": 683 - }, - "id": 2759997, - "non_hive": { - "close": 81, - "high": 81, - "low": 81, - "open": 81, - "volume": 81 - }, - "open": "2020-11-04T01:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 3155, - "high": 3155, - "low": 3155, - "open": 3155, - "volume": 3155 - }, - "id": 2759999, - "non_hive": { - "close": 374, - "high": 374, - "low": 374, - "open": 374, - "volume": 374 - }, - "open": "2020-11-04T01:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 3787, - "high": 3787, - "low": 4647, - "open": 4647, - "volume": 8434 - }, - "id": 2760002, - "non_hive": { - "close": 449, - "high": 449, - "low": 550, - "open": 550, - "volume": 999 - }, - "open": "2020-11-04T01:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 96324, - "high": 96324, - "low": 6058, - "open": 6058, - "volume": 1240019 - }, - "id": 2760005, - "non_hive": { - "close": 11455, - "high": 11455, - "low": 718, - "open": 718, - "volume": 147461 - }, - "open": "2020-11-04T01:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 44469, - "high": 17221, - "low": 28429, - "open": 17221, - "volume": 90119 - }, - "id": 2760009, - "non_hive": { - "close": 5164, - "high": 2000, - "low": 3301, - "open": 2000, - "volume": 10465 - }, - "open": "2020-11-04T01:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 28138, - "high": 17223, - "low": 28138, - "open": 17223, - "volume": 45361 - }, - "id": 2760013, - "non_hive": { - "close": 3267, - "high": 2000, - "low": 3267, - "open": 2000, - "volume": 5267 - }, - "open": "2020-11-04T01:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 29042, - "high": 17221, - "low": 29042, - "open": 17221, - "volume": 46263 - }, - "id": 2760015, - "non_hive": { - "close": 3372, - "high": 2000, - "low": 3372, - "open": 2000, - "volume": 5372 - }, - "open": "2020-11-04T01:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 1455, - "high": 1455, - "low": 1455, - "open": 1455, - "volume": 1455 - }, - "id": 2760017, - "non_hive": { - "close": 173, - "high": 173, - "low": 173, - "open": 173, - "volume": 173 - }, - "open": "2020-11-04T01:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 1564, - "high": 1564, - "low": 1564, - "open": 1564, - "volume": 1564 - }, - "id": 2760020, - "non_hive": { - "close": 186, - "high": 186, - "low": 186, - "open": 186, - "volume": 186 - }, - "open": "2020-11-04T01:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 13967, - "high": 13967, - "low": 13967, - "open": 13967, - "volume": 13967 - }, - "id": 2760023, - "non_hive": { - "close": 1622, - "high": 1622, - "low": 1622, - "open": 1622, - "volume": 1622 - }, - "open": "2020-11-04T02:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 78665, - "high": 78665, - "low": 253022, - "open": 253022, - "volume": 331687 - }, - "id": 2760027, - "non_hive": { - "close": 9135, - "high": 9135, - "low": 29382, - "open": 29382, - "volume": 38517 - }, - "open": "2020-11-04T02:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 71570, - "high": 71570, - "low": 71570, - "open": 71570, - "volume": 71570 - }, - "id": 2760030, - "non_hive": { - "close": 8311, - "high": 8311, - "low": 8311, - "open": 8311, - "volume": 8311 - }, - "open": "2020-11-04T02:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 12417, - "high": 12417, - "low": 12417, - "open": 12417, - "volume": 12417 - }, - "id": 2760033, - "non_hive": { - "close": 1442, - "high": 1442, - "low": 1442, - "open": 1442, - "volume": 1442 - }, - "open": "2020-11-04T02:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 5270, - "high": 5270, - "low": 5270, - "open": 5270, - "volume": 5270 - }, - "id": 2760036, - "non_hive": { - "close": 612, - "high": 612, - "low": 612, - "open": 612, - "volume": 612 - }, - "open": "2020-11-04T02:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 111, - "high": 111, - "low": 111, - "open": 111, - "volume": 111 - }, - "id": 2760039, - "non_hive": { - "close": 13, - "high": 13, - "low": 13, - "open": 13, - "volume": 13 - }, - "open": "2020-11-04T02:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 8456, - "high": 8456, - "low": 8456, - "open": 8456, - "volume": 8456 - }, - "id": 2760042, - "non_hive": { - "close": 982, - "high": 982, - "low": 982, - "open": 982, - "volume": 982 - }, - "open": "2020-11-04T03:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 77503, - "high": 77503, - "low": 77503, - "open": 77503, - "volume": 77503 - }, - "id": 2760046, - "non_hive": { - "close": 9000, - "high": 9000, - "low": 9000, - "open": 9000, - "volume": 9000 - }, - "open": "2020-11-04T03:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 4366, - "high": 4366, - "low": 4366, - "open": 4366, - "volume": 4366 - }, - "id": 2760049, - "non_hive": { - "close": 507, - "high": 507, - "low": 507, - "open": 507, - "volume": 507 - }, - "open": "2020-11-04T03:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 23922, - "high": 23922, - "low": 23922, - "open": 23922, - "volume": 23922 - }, - "id": 2760052, - "non_hive": { - "close": 2778, - "high": 2778, - "low": 2778, - "open": 2778, - "volume": 2778 - }, - "open": "2020-11-04T03:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 20564, - "high": 20564, - "low": 20564, - "open": 20564, - "volume": 20564 - }, - "id": 2760054, - "non_hive": { - "close": 2388, - "high": 2388, - "low": 2388, - "open": 2388, - "volume": 2388 - }, - "open": "2020-11-04T03:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 70472, - "high": 18851, - "low": 70472, - "open": 18851, - "volume": 89323 - }, - "id": 2760057, - "non_hive": { - "close": 8182, - "high": 2189, - "low": 8182, - "open": 2189, - "volume": 10371 - }, - "open": "2020-11-04T03:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 55768, - "high": 55768, - "low": 55768, - "open": 55768, - "volume": 55768 - }, - "id": 2760060, - "non_hive": { - "close": 6476, - "high": 6476, - "low": 6476, - "open": 6476, - "volume": 6476 - }, - "open": "2020-11-04T03:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 40482, - "high": 40482, - "low": 40482, - "open": 40482, - "volume": 40482 - }, - "id": 2760062, - "non_hive": { - "close": 4700, - "high": 4700, - "low": 4700, - "open": 4700, - "volume": 4700 - }, - "open": "2020-11-04T03:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 17395, - "high": 17395, - "low": 17395, - "open": 17395, - "volume": 17395 - }, - "id": 2760065, - "non_hive": { - "close": 2020, - "high": 2020, - "low": 2020, - "open": 2020, - "volume": 2020 - }, - "open": "2020-11-04T04:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 21614, - "high": 21614, - "low": 21614, - "open": 21614, - "volume": 21614 - }, - "id": 2760069, - "non_hive": { - "close": 2510, - "high": 2510, - "low": 2510, - "open": 2510, - "volume": 2510 - }, - "open": "2020-11-04T04:13:00", - "seconds": 60 - }, - { - "hive": { - "close": 9989, - "high": 9989, - "low": 9989, - "open": 9989, - "volume": 9989 - }, - "id": 2760072, - "non_hive": { - "close": 1160, - "high": 1160, - "low": 1160, - "open": 1160, - "volume": 1160 - }, - "open": "2020-11-04T04:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 49721, - "high": 750279, - "low": 49721, - "open": 750279, - "volume": 800000 - }, - "id": 2760075, - "non_hive": { - "close": 5772, - "high": 87118, - "low": 5772, - "open": 87118, - "volume": 92890 - }, - "open": "2020-11-04T04:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 25850, - "high": 25850, - "low": 25850, - "open": 25850, - "volume": 25850 - }, - "id": 2760078, - "non_hive": { - "close": 3001, - "high": 3001, - "low": 3001, - "open": 3001, - "volume": 3001 - }, - "open": "2020-11-04T04:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 12262, - "high": 12262, - "low": 12262, - "open": 12262, - "volume": 12262 - }, - "id": 2760081, - "non_hive": { - "close": 1423, - "high": 1423, - "low": 1423, - "open": 1423, - "volume": 1423 - }, - "open": "2020-11-04T04:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 23288, - "high": 23288, - "low": 23288, - "open": 23288, - "volume": 23288 - }, - "id": 2760084, - "non_hive": { - "close": 2704, - "high": 2704, - "low": 2704, - "open": 2704, - "volume": 2704 - }, - "open": "2020-11-04T04:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 3814, - "high": 3814, - "low": 3814, - "open": 3814, - "volume": 3814 - }, - "id": 2760087, - "non_hive": { - "close": 442, - "high": 442, - "low": 442, - "open": 442, - "volume": 442 - }, - "open": "2020-11-04T05:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 567585, - "high": 567585, - "low": 4850, - "open": 4850, - "volume": 756969 - }, - "id": 2760091, - "non_hive": { - "close": 67449, - "high": 67449, - "low": 563, - "open": 563, - "volume": 89480 - }, - "open": "2020-11-04T05:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 324, - "high": 16661, - "low": 324, - "open": 16830, - "volume": 33815 - }, - "id": 2760094, - "non_hive": { - "close": 37, - "high": 1979, - "low": 37, - "open": 1999, - "volume": 4015 - }, - "open": "2020-11-04T06:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 14422, - "high": 14422, - "low": 14422, - "open": 14422, - "volume": 14422 - }, - "id": 2760099, - "non_hive": { - "close": 1713, - "high": 1713, - "low": 1713, - "open": 1713, - "volume": 1713 - }, - "open": "2020-11-04T06:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 13960, - "high": 13960, - "low": 2408, - "open": 2408, - "volume": 16368 - }, - "id": 2760101, - "non_hive": { - "close": 1659, - "high": 1659, - "low": 286, - "open": 286, - "volume": 1945 - }, - "open": "2020-11-04T06:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 7282, - "high": 7282, - "low": 7282, - "open": 7282, - "volume": 7282 - }, - "id": 2760104, - "non_hive": { - "close": 865, - "high": 865, - "low": 865, - "open": 865, - "volume": 865 - }, - "open": "2020-11-04T06:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 67, - "high": 4362, - "low": 67, - "open": 4362, - "volume": 4429 - }, - "id": 2760107, - "non_hive": { - "close": 7, - "high": 518, - "low": 7, - "open": 518, - "volume": 525 - }, - "open": "2020-11-04T06:57:00", - "seconds": 60 - }, - { - "hive": { - "close": 3845, - "high": 3845, - "low": 7704, - "open": 7704, - "volume": 11549 - }, - "id": 2760110, - "non_hive": { - "close": 457, - "high": 457, - "low": 915, - "open": 915, - "volume": 1372 - }, - "open": "2020-11-04T06:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 791, - "high": 791, - "low": 791, - "open": 791, - "volume": 791 - }, - "id": 2760112, - "non_hive": { - "close": 94, - "high": 94, - "low": 94, - "open": 94, - "volume": 94 - }, - "open": "2020-11-04T07:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 43979, - "high": 22393, - "low": 43979, - "open": 22393, - "volume": 1022393 - }, - "id": 2760116, - "non_hive": { - "close": 5017, - "high": 2661, - "low": 5017, - "open": 2661, - "volume": 117728 - }, - "open": "2020-11-04T07:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 307876, - "high": 17379, - "low": 307876, - "open": 17379, - "volume": 1000000 - }, - "id": 2760120, - "non_hive": { - "close": 35097, - "high": 2000, - "low": 35097, - "open": 2000, - "volume": 114257 - }, - "open": "2020-11-04T07:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 3124, - "high": 3124, - "low": 3124, - "open": 3124, - "volume": 3124 - }, - "id": 2760123, - "non_hive": { - "close": 369, - "high": 369, - "low": 369, - "open": 369, - "volume": 369 - }, - "open": "2020-11-04T07:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 15299, - "high": 15299, - "low": 15299, - "open": 15299, - "volume": 15299 - }, - "id": 2760126, - "non_hive": { - "close": 1806, - "high": 1806, - "low": 1806, - "open": 1806, - "volume": 1806 - }, - "open": "2020-11-04T08:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 16931, - "high": 16931, - "low": 16931, - "open": 16931, - "volume": 16931 - }, - "id": 2760130, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-04T08:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 362, - "high": 12932, - "low": 362, - "open": 12932, - "volume": 13294 - }, - "id": 2760132, - "non_hive": { - "close": 42, - "high": 1528, - "low": 42, - "open": 1528, - "volume": 1570 - }, - "open": "2020-11-04T08:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 708, - "high": 26010, - "low": 708, - "open": 26010, - "volume": 26718 - }, - "id": 2760135, - "non_hive": { - "close": 80, - "high": 3071, - "low": 80, - "open": 3071, - "volume": 3151 - }, - "open": "2020-11-04T08:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 17000, - "high": 17000, - "low": 17000, - "open": 17000, - "volume": 17000 - }, - "id": 2760137, - "non_hive": { - "close": 2007, - "high": 2007, - "low": 2007, - "open": 2007, - "volume": 2007 - }, - "open": "2020-11-04T08:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 6003, - "high": 6003, - "low": 6003, - "open": 6003, - "volume": 6003 - }, - "id": 2760140, - "non_hive": { - "close": 709, - "high": 709, - "low": 709, - "open": 709, - "volume": 709 - }, - "open": "2020-11-04T08:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 507, - "high": 372, - "low": 507, - "open": 10923, - "volume": 43199 - }, - "id": 2760142, - "non_hive": { - "close": 57, - "high": 44, - "low": 57, - "open": 1290, - "volume": 5101 - }, - "open": "2020-11-04T08:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 6692, - "high": 6692, - "low": 6692, - "open": 6692, - "volume": 6692 - }, - "id": 2760145, - "non_hive": { - "close": 790, - "high": 790, - "low": 790, - "open": 790, - "volume": 790 - }, - "open": "2020-11-04T08:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 4244, - "high": 4244, - "low": 16946, - "open": 16946, - "volume": 21190 - }, - "id": 2760148, - "non_hive": { - "close": 501, - "high": 501, - "low": 1999, - "open": 1999, - "volume": 2500 - }, - "open": "2020-11-04T08:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 486, - "high": 486, - "low": 486, - "open": 486, - "volume": 486 - }, - "id": 2760150, - "non_hive": { - "close": 57, - "high": 57, - "low": 57, - "open": 57, - "volume": 57 - }, - "open": "2020-11-04T08:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 194, - "high": 194, - "low": 194, - "open": 194, - "volume": 194 - }, - "id": 2760153, - "non_hive": { - "close": 23, - "high": 23, - "low": 23, - "open": 23, - "volume": 23 - }, - "open": "2020-11-04T08:57:00", - "seconds": 60 - }, - { - "hive": { - "close": 5085, - "high": 5085, - "low": 5085, - "open": 5085, - "volume": 5085 - }, - "id": 2760155, - "non_hive": { - "close": 600, - "high": 600, - "low": 600, - "open": 600, - "volume": 600 - }, - "open": "2020-11-04T09:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 305, - "high": 305, - "low": 305, - "open": 305, - "volume": 305 - }, - "id": 2760159, - "non_hive": { - "close": 36, - "high": 36, - "low": 36, - "open": 36, - "volume": 36 - }, - "open": "2020-11-04T09:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 14742, - "high": 14742, - "low": 14742, - "open": 14742, - "volume": 14742 - }, - "id": 2760162, - "non_hive": { - "close": 1740, - "high": 1740, - "low": 1740, - "open": 1740, - "volume": 1740 - }, - "open": "2020-11-04T09:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 11511, - "high": 8489, - "low": 11511, - "open": 8489, - "volume": 20000 - }, - "id": 2760164, - "non_hive": { - "close": 1350, - "high": 1000, - "low": 1350, - "open": 1000, - "volume": 2350 - }, - "open": "2020-11-04T09:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 25, - "high": 25, - "low": 89412, - "open": 89412, - "volume": 89437 - }, - "id": 2760167, - "non_hive": { - "close": 3, - "high": 3, - "low": 10550, - "open": 10550, - "volume": 10553 - }, - "open": "2020-11-04T09:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 60944, - "high": 60944, - "low": 60944, - "open": 60944, - "volume": 60944 - }, - "id": 2760170, - "non_hive": { - "close": 7189, - "high": 7189, - "low": 7189, - "open": 7189, - "volume": 7189 - }, - "open": "2020-11-04T09:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 11953, - "high": 17503, - "low": 11953, - "open": 17503, - "volume": 51929 - }, - "id": 2760173, - "non_hive": { - "close": 1362, - "high": 2000, - "low": 1362, - "open": 2000, - "volume": 5926 - }, - "open": "2020-11-04T10:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 2458, - "high": 17542, - "low": 2458, - "open": 17542, - "volume": 20000 - }, - "id": 2760177, - "non_hive": { - "close": 280, - "high": 2000, - "low": 280, - "open": 2000, - "volume": 2280 - }, - "open": "2020-11-04T10:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 2698, - "high": 2698, - "low": 2698, - "open": 2698, - "volume": 2698 - }, - "id": 2760180, - "non_hive": { - "close": 313, - "high": 313, - "low": 313, - "open": 313, - "volume": 313 - }, - "open": "2020-11-04T10:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 8694, - "high": 8694, - "low": 8694, - "open": 8694, - "volume": 8694 - }, - "id": 2760183, - "non_hive": { - "close": 1008, - "high": 1008, - "low": 1008, - "open": 1008, - "volume": 1008 - }, - "open": "2020-11-04T10:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 9090, - "high": 9090, - "low": 9090, - "open": 9090, - "volume": 9090 - }, - "id": 2760186, - "non_hive": { - "close": 1054, - "high": 1054, - "low": 1054, - "open": 1054, - "volume": 1054 - }, - "open": "2020-11-04T10:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 85873, - "high": 85873, - "low": 85873, - "open": 85873, - "volume": 85873 - }, - "id": 2760189, - "non_hive": { - "close": 9960, - "high": 9960, - "low": 9960, - "open": 9960, - "volume": 9960 - }, - "open": "2020-11-04T10:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 2664, - "high": 2664, - "low": 14563, - "open": 14563, - "volume": 17227 - }, - "id": 2760192, - "non_hive": { - "close": 309, - "high": 309, - "low": 1689, - "open": 1689, - "volume": 1998 - }, - "open": "2020-11-04T11:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 62873, - "high": 62873, - "low": 62873, - "open": 62873, - "volume": 62873 - }, - "id": 2760196, - "non_hive": { - "close": 7292, - "high": 7292, - "low": 7292, - "open": 7292, - "volume": 7292 - }, - "open": "2020-11-04T11:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 105, - "high": 22762, - "low": 105, - "open": 22762, - "volume": 31007 - }, - "id": 2760199, - "non_hive": { - "close": 11, - "high": 2640, - "low": 11, - "open": 2640, - "volume": 3595 - }, - "open": "2020-11-04T11:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 5006, - "high": 5006, - "low": 5006, - "open": 5006, - "volume": 5006 - }, - "id": 2760202, - "non_hive": { - "close": 580, - "high": 580, - "low": 580, - "open": 580, - "volume": 580 - }, - "open": "2020-11-04T11:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 510, - "high": 510, - "low": 510, - "open": 510, - "volume": 510 - }, - "id": 2760205, - "non_hive": { - "close": 59, - "high": 59, - "low": 59, - "open": 59, - "volume": 59 - }, - "open": "2020-11-04T11:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 185, - "high": 16738, - "low": 185, - "open": 16738, - "volume": 16923 - }, - "id": 2760208, - "non_hive": { - "close": 21, - "high": 1941, - "low": 21, - "open": 1941, - "volume": 1962 - }, - "open": "2020-11-04T11:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 5331, - "high": 17542, - "low": 5331, - "open": 17542, - "volume": 22873 - }, - "id": 2760210, - "non_hive": { - "close": 607, - "high": 2000, - "low": 607, - "open": 2000, - "volume": 2607 - }, - "open": "2020-11-04T11:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 163, - "high": 163, - "low": 18870, - "open": 18870, - "volume": 19033 - }, - "id": 2760213, - "non_hive": { - "close": 19, - "high": 19, - "low": 2151, - "open": 2151, - "volume": 2170 - }, - "open": "2020-11-04T11:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 17391, - "high": 17389, - "low": 1472, - "open": 1472, - "volume": 83042 - }, - "id": 2760216, - "non_hive": { - "close": 1999, - "high": 2000, - "low": 169, - "open": 169, - "volume": 9549 - }, - "open": "2020-11-04T11:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 149, - "high": 17391, - "low": 149, - "open": 17391, - "volume": 17540 - }, - "id": 2760221, - "non_hive": { - "close": 17, - "high": 1999, - "low": 17, - "open": 1999, - "volume": 2016 - }, - "open": "2020-11-04T11:57:00", - "seconds": 60 - }, - { - "hive": { - "close": 56, - "high": 3543, - "low": 56, - "open": 3543, - "volume": 3599 - }, - "id": 2760224, - "non_hive": { - "close": 6, - "high": 411, - "low": 6, - "open": 411, - "volume": 417 - }, - "open": "2020-11-04T11:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 88, - "high": 83873, - "low": 49, - "open": 3121, - "volume": 92674 - }, - "id": 2760226, - "non_hive": { - "close": 10, - "high": 9730, - "low": 5, - "open": 362, - "volume": 10750 - }, - "open": "2020-11-04T12:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 5864, - "high": 24136, - "low": 5864, - "open": 24136, - "volume": 30000 - }, - "id": 2760231, - "non_hive": { - "close": 680, - "high": 2800, - "low": 680, - "open": 2800, - "volume": 3480 - }, - "open": "2020-11-04T12:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 15, - "high": 948, - "low": 15, - "open": 948, - "volume": 963 - }, - "id": 2760233, - "non_hive": { - "close": 1, - "high": 110, - "low": 1, - "open": 110, - "volume": 111 - }, - "open": "2020-11-04T12:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 1474, - "high": 3026, - "low": 1474, - "open": 3026, - "volume": 4500 - }, - "id": 2760236, - "non_hive": { - "close": 168, - "high": 345, - "low": 168, - "open": 345, - "volume": 513 - }, - "open": "2020-11-04T12:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 1489, - "high": 1489, - "low": 1489, - "open": 1489, - "volume": 1489 - }, - "id": 2760238, - "non_hive": { - "close": 172, - "high": 172, - "low": 172, - "open": 172, - "volume": 172 - }, - "open": "2020-11-04T12:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 15688, - "high": 17312, - "low": 15688, - "open": 17312, - "volume": 33000 - }, - "id": 2760241, - "non_hive": { - "close": 1812, - "high": 2000, - "low": 1812, - "open": 2000, - "volume": 3812 - }, - "open": "2020-11-04T12:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 16985, - "high": 16985, - "low": 16985, - "open": 16985, - "volume": 16985 - }, - "id": 2760244, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-04T12:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 135, - "high": 6771, - "low": 135, - "open": 6771, - "volume": 43843 - }, - "id": 2760247, - "non_hive": { - "close": 15, - "high": 797, - "low": 15, - "open": 797, - "volume": 5059 - }, - "open": "2020-11-04T12:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 2261, - "high": 2261, - "low": 2261, - "open": 2261, - "volume": 2261 - }, - "id": 2760249, - "non_hive": { - "close": 266, - "high": 266, - "low": 266, - "open": 266, - "volume": 266 - }, - "open": "2020-11-04T12:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 133145, - "high": 133145, - "low": 133145, - "open": 133145, - "volume": 133145 - }, - "id": 2760252, - "non_hive": { - "close": 15655, - "high": 15655, - "low": 15655, - "open": 15655, - "volume": 15655 - }, - "open": "2020-11-04T13:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 139760, - "high": 139760, - "low": 12196, - "open": 12196, - "volume": 151956 - }, - "id": 2760256, - "non_hive": { - "close": 16433, - "high": 16433, - "low": 1434, - "open": 1434, - "volume": 17867 - }, - "open": "2020-11-04T13:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 17767, - "high": 17767, - "low": 17767, - "open": 17767, - "volume": 17767 - }, - "id": 2760259, - "non_hive": { - "close": 2089, - "high": 2089, - "low": 2089, - "open": 2089, - "volume": 2089 - }, - "open": "2020-11-04T13:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 59, - "high": 59, - "low": 59, - "open": 59, - "volume": 59 - }, - "id": 2760261, - "non_hive": { - "close": 7, - "high": 7, - "low": 7, - "open": 7, - "volume": 7 - }, - "open": "2020-11-04T13:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 238, - "high": 238, - "low": 238, - "open": 238, - "volume": 238 - }, - "id": 2760264, - "non_hive": { - "close": 28, - "high": 28, - "low": 28, - "open": 28, - "volume": 28 - }, - "open": "2020-11-04T13:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 536, - "high": 536, - "low": 536, - "open": 536, - "volume": 536 - }, - "id": 2760267, - "non_hive": { - "close": 63, - "high": 63, - "low": 63, - "open": 63, - "volume": 63 - }, - "open": "2020-11-04T13:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 5592, - "high": 5592, - "low": 5592, - "open": 5592, - "volume": 5592 - }, - "id": 2760270, - "non_hive": { - "close": 657, - "high": 657, - "low": 657, - "open": 657, - "volume": 657 - }, - "open": "2020-11-04T13:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 78811, - "high": 78811, - "low": 9244, - "open": 9244, - "volume": 88055 - }, - "id": 2760272, - "non_hive": { - "close": 9264, - "high": 9264, - "low": 1086, - "open": 1086, - "volume": 10350 - }, - "open": "2020-11-04T13:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 11799, - "high": 11799, - "low": 11799, - "open": 11799, - "volume": 11799 - }, - "id": 2760275, - "non_hive": { - "close": 1387, - "high": 1387, - "low": 1387, - "open": 1387, - "volume": 1387 - }, - "open": "2020-11-04T13:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 30549, - "high": 30549, - "low": 30549, - "open": 30549, - "volume": 30549 - }, - "id": 2760278, - "non_hive": { - "close": 3591, - "high": 3591, - "low": 3591, - "open": 3591, - "volume": 3591 - }, - "open": "2020-11-04T13:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 8958, - "high": 8958, - "low": 8958, - "open": 8958, - "volume": 8958 - }, - "id": 2760281, - "non_hive": { - "close": 1053, - "high": 1053, - "low": 1053, - "open": 1053, - "volume": 1053 - }, - "open": "2020-11-04T13:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 631, - "high": 9584, - "low": 631, - "open": 9584, - "volume": 42996 - }, - "id": 2760284, - "non_hive": { - "close": 72, - "high": 1126, - "low": 72, - "open": 1126, - "volume": 5049 - }, - "open": "2020-11-04T13:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 62992, - "high": 62992, - "low": 62992, - "open": 62992, - "volume": 62992 - }, - "id": 2760288, - "non_hive": { - "close": 7400, - "high": 7400, - "low": 7400, - "open": 7400, - "volume": 7400 - }, - "open": "2020-11-04T14:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 8571, - "high": 8571, - "low": 631, - "open": 631, - "volume": 9202 - }, - "id": 2760292, - "non_hive": { - "close": 1007, - "high": 1007, - "low": 74, - "open": 74, - "volume": 1081 - }, - "open": "2020-11-04T14:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 2863, - "high": 17391, - "low": 2863, - "open": 17391, - "volume": 20254 - }, - "id": 2760295, - "non_hive": { - "close": 329, - "high": 2000, - "low": 329, - "open": 2000, - "volume": 2329 - }, - "open": "2020-11-04T14:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 17315, - "high": 17315, - "low": 17315, - "open": 17315, - "volume": 17315 - }, - "id": 2760298, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-04T14:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 41883, - "high": 41883, - "low": 17038, - "open": 17038, - "volume": 71382 - }, - "id": 2760300, - "non_hive": { - "close": 4914, - "high": 4914, - "low": 1999, - "open": 1999, - "volume": 8375 - }, - "open": "2020-11-04T14:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 2362, - "high": 2362, - "low": 2362, - "open": 2362, - "volume": 2362 - }, - "id": 2760305, - "non_hive": { - "close": 277, - "high": 277, - "low": 277, - "open": 277, - "volume": 277 - }, - "open": "2020-11-04T14:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 377605, - "high": 17238, - "low": 377605, - "open": 17238, - "volume": 394843 - }, - "id": 2760308, - "non_hive": { - "close": 43808, - "high": 2000, - "low": 43808, - "open": 2000, - "volume": 45808 - }, - "open": "2020-11-04T14:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 92810, - "high": 92810, - "low": 92810, - "open": 92810, - "volume": 92810 - }, - "id": 2760310, - "non_hive": { - "close": 10766, - "high": 10766, - "low": 10766, - "open": 10766, - "volume": 10766 - }, - "open": "2020-11-04T14:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 17315, - "high": 7882, - "low": 17315, - "open": 17315, - "volume": 42512 - }, - "id": 2760312, - "non_hive": { - "close": 2000, - "high": 914, - "low": 2000, - "open": 2000, - "volume": 4914 - }, - "open": "2020-11-04T14:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 155358, - "high": 17389, - "low": 155358, - "open": 17389, - "volume": 172747 - }, - "id": 2760315, - "non_hive": { - "close": 17867, - "high": 2000, - "low": 17867, - "open": 2000, - "volume": 19867 - }, - "open": "2020-11-04T14:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 10582, - "high": 10582, - "low": 10582, - "open": 10582, - "volume": 10582 - }, - "id": 2760318, - "non_hive": { - "close": 1227, - "high": 1227, - "low": 1227, - "open": 1227, - "volume": 1227 - }, - "open": "2020-11-04T14:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 1086, - "high": 1086, - "low": 1086, - "open": 1086, - "volume": 1086 - }, - "id": 2760321, - "non_hive": { - "close": 126, - "high": 126, - "low": 126, - "open": 126, - "volume": 126 - }, - "open": "2020-11-04T14:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 11431, - "high": 11431, - "low": 5573, - "open": 5573, - "volume": 17004 - }, - "id": 2760324, - "non_hive": { - "close": 1326, - "high": 1326, - "low": 646, - "open": 646, - "volume": 1972 - }, - "open": "2020-11-04T14:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 8624, - "high": 8624, - "low": 8624, - "open": 8624, - "volume": 8624 - }, - "id": 2760326, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-11-04T14:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 8617, - "high": 8617, - "low": 8617, - "open": 8617, - "volume": 8617 - }, - "id": 2760329, - "non_hive": { - "close": 999, - "high": 999, - "low": 999, - "open": 999, - "volume": 999 - }, - "open": "2020-11-04T14:57:00", - "seconds": 60 - }, - { - "hive": { - "close": 100, - "high": 100, - "low": 100, - "open": 100, - "volume": 100 - }, - "id": 2760331, - "non_hive": { - "close": 11, - "high": 11, - "low": 11, - "open": 11, - "volume": 11 - }, - "open": "2020-11-04T15:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 67272, - "high": 8526, - "low": 67272, - "open": 8526, - "volume": 85945 - }, - "id": 2760335, - "non_hive": { - "close": 7800, - "high": 989, - "low": 7800, - "open": 989, - "volume": 9966 - }, - "open": "2020-11-04T15:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 656, - "high": 656, - "low": 656, - "open": 656, - "volume": 656 - }, - "id": 2760337, - "non_hive": { - "close": 76, - "high": 76, - "low": 76, - "open": 76, - "volume": 76 - }, - "open": "2020-11-04T15:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2760340, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-04T15:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 32352, - "high": 129, - "low": 32352, - "open": 17242, - "volume": 236818 - }, - "id": 2760343, - "non_hive": { - "close": 3720, - "high": 15, - "low": 3720, - "open": 2000, - "volume": 27252 - }, - "open": "2020-11-04T15:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 8633, - "high": 8633, - "low": 8633, - "open": 8633, - "volume": 8633 - }, - "id": 2760346, - "non_hive": { - "close": 1001, - "high": 1001, - "low": 1001, - "open": 1001, - "volume": 1001 - }, - "open": "2020-11-04T16:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2760350, - "non_hive": { - "close": 116, - "high": 116, - "low": 116, - "open": 116, - "volume": 116 - }, - "open": "2020-11-04T16:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 17390, - "high": 17390, - "low": 17390, - "open": 17390, - "volume": 17390 - }, - "id": 2760353, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-04T16:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 10007, - "high": 10007, - "low": 10007, - "open": 10007, - "volume": 10007 - }, - "id": 2760355, - "non_hive": { - "close": 1151, - "high": 1151, - "low": 1151, - "open": 1151, - "volume": 1151 - }, - "open": "2020-11-04T16:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 1486, - "high": 1486, - "low": 1486, - "open": 1486, - "volume": 1486 - }, - "id": 2760357, - "non_hive": { - "close": 171, - "high": 171, - "low": 171, - "open": 171, - "volume": 171 - }, - "open": "2020-11-04T16:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 11993, - "high": 11993, - "low": 11993, - "open": 11993, - "volume": 11993 - }, - "id": 2760360, - "non_hive": { - "close": 1379, - "high": 1379, - "low": 1379, - "open": 1379, - "volume": 1379 - }, - "open": "2020-11-04T16:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 12209, - "high": 12209, - "low": 12209, - "open": 12209, - "volume": 12209 - }, - "id": 2760362, - "non_hive": { - "close": 1404, - "high": 1404, - "low": 1404, - "open": 1404, - "volume": 1404 - }, - "open": "2020-11-04T16:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 13981, - "high": 13981, - "low": 13981, - "open": 13981, - "volume": 13981 - }, - "id": 2760364, - "non_hive": { - "close": 1608, - "high": 1608, - "low": 1608, - "open": 1608, - "volume": 1608 - }, - "open": "2020-11-04T16:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 5124, - "high": 10869, - "low": 5124, - "open": 10869, - "volume": 15993 - }, - "id": 2760367, - "non_hive": { - "close": 589, - "high": 1250, - "low": 589, - "open": 1250, - "volume": 1839 - }, - "open": "2020-11-04T16:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 17000, - "high": 17000, - "low": 17000, - "open": 17000, - "volume": 17000 - }, - "id": 2760370, - "non_hive": { - "close": 1954, - "high": 1954, - "low": 1954, - "open": 1954, - "volume": 1954 - }, - "open": "2020-11-04T16:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 12221, - "high": 17563, - "low": 12221, - "open": 17563, - "volume": 31384 - }, - "id": 2760373, - "non_hive": { - "close": 1393, - "high": 2020, - "low": 1393, - "open": 2020, - "volume": 3596 - }, - "open": "2020-11-04T16:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 9641, - "high": 17391, - "low": 56090, - "open": 5323, - "volume": 184032 - }, - "id": 2760375, - "non_hive": { - "close": 1108, - "high": 1999, - "low": 6338, - "open": 607, - "volume": 20931 - }, - "open": "2020-11-04T16:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 103, - "high": 11875, - "low": 103, - "open": 11875, - "volume": 11978 - }, - "id": 2760379, - "non_hive": { - "close": 11, - "high": 1365, - "low": 11, - "open": 1365, - "volume": 1376 - }, - "open": "2020-11-04T16:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 661, - "high": 58764, - "low": 661, - "open": 8803, - "volume": 68228 - }, - "id": 2760381, - "non_hive": { - "close": 74, - "high": 6755, - "low": 74, - "open": 1011, - "volume": 7840 - }, - "open": "2020-11-04T16:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 17390, - "high": 17390, - "low": 17390, - "open": 17390, - "volume": 17390 - }, - "id": 2760385, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-04T16:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 3399, - "high": 3399, - "low": 3399, - "open": 3399, - "volume": 3399 - }, - "id": 2760388, - "non_hive": { - "close": 391, - "high": 391, - "low": 391, - "open": 391, - "volume": 391 - }, - "open": "2020-11-04T16:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 73096, - "high": 73096, - "low": 66599, - "open": 66599, - "volume": 139695 - }, - "id": 2760390, - "non_hive": { - "close": 8479, - "high": 8479, - "low": 7659, - "open": 7659, - "volume": 16138 - }, - "open": "2020-11-04T16:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 468, - "high": 35994, - "low": 468, - "open": 35994, - "volume": 36462 - }, - "id": 2760393, - "non_hive": { - "close": 53, - "high": 4173, - "low": 53, - "open": 4173, - "volume": 4226 - }, - "open": "2020-11-04T16:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 17244, - "high": 17244, - "low": 17244, - "open": 17244, - "volume": 17244 - }, - "id": 2760395, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-04T16:48:00", - "seconds": 60 - }, - { - "hive": { - "close": 1771, - "high": 1771, - "low": 1771, - "open": 1771, - "volume": 1771 - }, - "id": 2760397, - "non_hive": { - "close": 205, - "high": 205, - "low": 205, - "open": 205, - "volume": 205 - }, - "open": "2020-11-04T16:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 870, - "high": 870, - "low": 870, - "open": 870, - "volume": 870 - }, - "id": 2760400, - "non_hive": { - "close": 101, - "high": 101, - "low": 101, - "open": 101, - "volume": 101 - }, - "open": "2020-11-04T16:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 215, - "high": 215, - "low": 215, - "open": 215, - "volume": 215 - }, - "id": 2760403, - "non_hive": { - "close": 25, - "high": 25, - "low": 25, - "open": 25, - "volume": 25 - }, - "open": "2020-11-04T17:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 1629, - "high": 1629, - "low": 1629, - "open": 1629, - "volume": 1629 - }, - "id": 2760407, - "non_hive": { - "close": 189, - "high": 189, - "low": 189, - "open": 189, - "volume": 189 - }, - "open": "2020-11-04T17:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 1783, - "high": 1783, - "low": 1783, - "open": 1783, - "volume": 1783 - }, - "id": 2760410, - "non_hive": { - "close": 206, - "high": 206, - "low": 206, - "open": 206, - "volume": 206 - }, - "open": "2020-11-04T17:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 13276, - "high": 13276, - "low": 13276, - "open": 13276, - "volume": 13276 - }, - "id": 2760413, - "non_hive": { - "close": 1540, - "high": 1540, - "low": 1540, - "open": 1540, - "volume": 1540 - }, - "open": "2020-11-04T17:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 37768, - "high": 37768, - "low": 37768, - "open": 37768, - "volume": 37768 - }, - "id": 2760416, - "non_hive": { - "close": 4381, - "high": 4381, - "low": 4381, - "open": 4381, - "volume": 4381 - }, - "open": "2020-11-04T17:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 12776, - "high": 12776, - "low": 12776, - "open": 12776, - "volume": 12776 - }, - "id": 2760418, - "non_hive": { - "close": 1482, - "high": 1482, - "low": 1482, - "open": 1482, - "volume": 1482 - }, - "open": "2020-11-04T17:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 1844, - "high": 1844, - "low": 1844, - "open": 1844, - "volume": 1844 - }, - "id": 2760421, - "non_hive": { - "close": 214, - "high": 214, - "low": 214, - "open": 214, - "volume": 214 - }, - "open": "2020-11-04T17:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 51, - "high": 51, - "low": 51, - "open": 51, - "volume": 51 - }, - "id": 2760423, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-04T17:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 3536, - "high": 3492, - "low": 3536, - "open": 3492, - "volume": 7028 - }, - "id": 2760426, - "non_hive": { - "close": 410, - "high": 405, - "low": 410, - "open": 405, - "volume": 815 - }, - "open": "2020-11-04T17:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 20, - "high": 1596, - "low": 20, - "open": 1596, - "volume": 1616 - }, - "id": 2760429, - "non_hive": { - "close": 2, - "high": 185, - "low": 2, - "open": 185, - "volume": 187 - }, - "open": "2020-11-04T17:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 552, - "high": 58831, - "low": 207, - "open": 207, - "volume": 59590 - }, - "id": 2760432, - "non_hive": { - "close": 64, - "high": 6821, - "low": 24, - "open": 24, - "volume": 6909 - }, - "open": "2020-11-04T17:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 320638, - "high": 17628, - "low": 320638, - "open": 17628, - "volume": 653266 - }, - "id": 2760435, - "non_hive": { - "close": 36200, - "high": 2000, - "low": 36200, - "open": 2000, - "volume": 73810 - }, - "open": "2020-11-04T17:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 68415, - "high": 68415, - "low": 68415, - "open": 68415, - "volume": 68415 - }, - "id": 2760438, - "non_hive": { - "close": 7724, - "high": 7724, - "low": 7724, - "open": 7724, - "volume": 7724 - }, - "open": "2020-11-04T17:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 2814, - "high": 2814, - "low": 2814, - "open": 2814, - "volume": 2814 - }, - "id": 2760440, - "non_hive": { - "close": 325, - "high": 325, - "low": 325, - "open": 325, - "volume": 325 - }, - "open": "2020-11-04T18:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 159986, - "high": 159986, - "low": 17257, - "open": 17257, - "volume": 177243 - }, - "id": 2760444, - "non_hive": { - "close": 18542, - "high": 18542, - "low": 1999, - "open": 1999, - "volume": 20541 - }, - "open": "2020-11-04T18:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 224, - "high": 224, - "low": 3286, - "open": 3286, - "volume": 20654 - }, - "id": 2760446, - "non_hive": { - "close": 26, - "high": 26, - "low": 379, - "open": 379, - "volume": 2386 - }, - "open": "2020-11-04T18:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 20, - "high": 20, - "low": 20, - "open": 20, - "volume": 20 - }, - "id": 2760449, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-11-04T18:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 104, - "high": 104, - "low": 104, - "open": 104, - "volume": 104 - }, - "id": 2760452, - "non_hive": { - "close": 12, - "high": 12, - "low": 12, - "open": 12, - "volume": 12 - }, - "open": "2020-11-04T19:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 3764, - "high": 3764, - "low": 3764, - "open": 3764, - "volume": 3764 - }, - "id": 2760456, - "non_hive": { - "close": 435, - "high": 435, - "low": 435, - "open": 435, - "volume": 435 - }, - "open": "2020-11-04T19:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 649, - "high": 649, - "low": 649, - "open": 649, - "volume": 649 - }, - "id": 2760459, - "non_hive": { - "close": 75, - "high": 75, - "low": 75, - "open": 75, - "volume": 75 - }, - "open": "2020-11-04T19:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 3034, - "high": 2288, - "low": 3034, - "open": 2288, - "volume": 5322 - }, - "id": 2760462, - "non_hive": { - "close": 350, - "high": 264, - "low": 350, - "open": 264, - "volume": 614 - }, - "open": "2020-11-04T19:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 6346, - "high": 6346, - "low": 6346, - "open": 6346, - "volume": 6346 - }, - "id": 2760464, - "non_hive": { - "close": 732, - "high": 732, - "low": 732, - "open": 732, - "volume": 732 - }, - "open": "2020-11-04T19:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 2948, - "high": 2948, - "low": 2948, - "open": 2948, - "volume": 2948 - }, - "id": 2760467, - "non_hive": { - "close": 339, - "high": 339, - "low": 339, - "open": 339, - "volume": 339 - }, - "open": "2020-11-04T19:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 220839, - "high": 220839, - "low": 14000, - "open": 14000, - "volume": 234839 - }, - "id": 2760470, - "non_hive": { - "close": 25580, - "high": 25580, - "low": 1617, - "open": 1617, - "volume": 27197 - }, - "open": "2020-11-04T19:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 100, - "high": 2003, - "low": 100, - "open": 2003, - "volume": 2103 - }, - "id": 2760473, - "non_hive": { - "close": 11, - "high": 232, - "low": 11, - "open": 232, - "volume": 243 - }, - "open": "2020-11-04T19:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 5882, - "high": 5882, - "low": 5882, - "open": 5882, - "volume": 5882 - }, - "id": 2760477, - "non_hive": { - "close": 681, - "high": 681, - "low": 681, - "open": 681, - "volume": 681 - }, - "open": "2020-11-04T19:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 100, - "high": 100, - "low": 100, - "open": 100, - "volume": 100 - }, - "id": 2760480, - "non_hive": { - "close": 11, - "high": 11, - "low": 11, - "open": 11, - "volume": 11 - }, - "open": "2020-11-04T19:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 426400, - "high": 426400, - "low": 4620, - "open": 4620, - "volume": 431020 - }, - "id": 2760482, - "non_hive": { - "close": 49365, - "high": 49365, - "low": 533, - "open": 533, - "volume": 49898 - }, - "open": "2020-11-04T19:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 57, - "high": 70621, - "low": 57, - "open": 4382, - "volume": 81301 - }, - "id": 2760485, - "non_hive": { - "close": 6, - "high": 8175, - "low": 6, - "open": 507, - "volume": 9410 - }, - "open": "2020-11-04T20:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 4466, - "high": 4466, - "low": 4466, - "open": 4466, - "volume": 4466 - }, - "id": 2760490, - "non_hive": { - "close": 517, - "high": 517, - "low": 517, - "open": 517, - "volume": 517 - }, - "open": "2020-11-04T20:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 8768, - "high": 8768, - "low": 8768, - "open": 8768, - "volume": 8768 - }, - "id": 2760492, - "non_hive": { - "close": 1015, - "high": 1015, - "low": 1015, - "open": 1015, - "volume": 1015 - }, - "open": "2020-11-04T20:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 9528, - "high": 9528, - "low": 9528, - "open": 9528, - "volume": 9528 - }, - "id": 2760495, - "non_hive": { - "close": 1103, - "high": 1103, - "low": 1103, - "open": 1103, - "volume": 1103 - }, - "open": "2020-11-04T20:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 15593, - "high": 15593, - "low": 15593, - "open": 15593, - "volume": 15593 - }, - "id": 2760497, - "non_hive": { - "close": 1805, - "high": 1805, - "low": 1805, - "open": 1805, - "volume": 1805 - }, - "open": "2020-11-04T20:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 137992, - "high": 137992, - "low": 137992, - "open": 137992, - "volume": 137992 - }, - "id": 2760499, - "non_hive": { - "close": 15972, - "high": 15972, - "low": 15972, - "open": 15972, - "volume": 15972 - }, - "open": "2020-11-04T20:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 1037, - "high": 1037, - "low": 1037, - "open": 1037, - "volume": 1037 - }, - "id": 2760502, - "non_hive": { - "close": 120, - "high": 120, - "low": 120, - "open": 120, - "volume": 120 - }, - "open": "2020-11-04T20:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 4183, - "high": 4183, - "low": 4183, - "open": 4183, - "volume": 4183 - }, - "id": 2760504, - "non_hive": { - "close": 484, - "high": 484, - "low": 484, - "open": 484, - "volume": 484 - }, - "open": "2020-11-04T20:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 53, - "high": 5696, - "low": 53, - "open": 5696, - "volume": 5749 - }, - "id": 2760507, - "non_hive": { - "close": 6, - "high": 659, - "low": 6, - "open": 659, - "volume": 665 - }, - "open": "2020-11-04T20:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 29120, - "high": 29120, - "low": 29120, - "open": 29120, - "volume": 29120 - }, - "id": 2760510, - "non_hive": { - "close": 3369, - "high": 3369, - "low": 3369, - "open": 3369, - "volume": 3369 - }, - "open": "2020-11-04T20:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 3854, - "high": 3854, - "low": 3854, - "open": 3854, - "volume": 3854 - }, - "id": 2760513, - "non_hive": { - "close": 447, - "high": 447, - "low": 447, - "open": 447, - "volume": 447 - }, - "open": "2020-11-04T21:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 4251, - "high": 4251, - "low": 5262, - "open": 5262, - "volume": 9513 - }, - "id": 2760517, - "non_hive": { - "close": 493, - "high": 493, - "low": 610, - "open": 610, - "volume": 1103 - }, - "open": "2020-11-04T21:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 8862, - "high": 8862, - "low": 8862, - "open": 8862, - "volume": 8862 - }, - "id": 2760520, - "non_hive": { - "close": 1010, - "high": 1010, - "low": 1010, - "open": 1010, - "volume": 1010 - }, - "open": "2020-11-04T21:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 621, - "high": 621, - "low": 621, - "open": 621, - "volume": 621 - }, - "id": 2760523, - "non_hive": { - "close": 72, - "high": 72, - "low": 72, - "open": 72, - "volume": 72 - }, - "open": "2020-11-04T21:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 1501, - "high": 1501, - "low": 1501, - "open": 1501, - "volume": 1501 - }, - "id": 2760526, - "non_hive": { - "close": 174, - "high": 174, - "low": 174, - "open": 174, - "volume": 174 - }, - "open": "2020-11-04T21:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 5229, - "high": 5229, - "low": 5229, - "open": 5229, - "volume": 5229 - }, - "id": 2760529, - "non_hive": { - "close": 606, - "high": 606, - "low": 606, - "open": 606, - "volume": 606 - }, - "open": "2020-11-04T21:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 587, - "high": 3582, - "low": 587, - "open": 3582, - "volume": 4169 - }, - "id": 2760532, - "non_hive": { - "close": 68, - "high": 415, - "low": 68, - "open": 415, - "volume": 483 - }, - "open": "2020-11-04T21:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 11896, - "high": 104, - "low": 11896, - "open": 104, - "volume": 12000 - }, - "id": 2760534, - "non_hive": { - "close": 1356, - "high": 12, - "low": 1356, - "open": 12, - "volume": 1368 - }, - "open": "2020-11-04T21:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 13460, - "high": 13460, - "low": 13460, - "open": 13460, - "volume": 13460 - }, - "id": 2760537, - "non_hive": { - "close": 1547, - "high": 1547, - "low": 1547, - "open": 1547, - "volume": 1547 - }, - "open": "2020-11-04T22:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 2200, - "high": 2200, - "low": 2200, - "open": 2200, - "volume": 2200 - }, - "id": 2760541, - "non_hive": { - "close": 253, - "high": 253, - "low": 253, - "open": 253, - "volume": 253 - }, - "open": "2020-11-04T22:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 12290, - "high": 12290, - "low": 12290, - "open": 12290, - "volume": 12290 - }, - "id": 2760543, - "non_hive": { - "close": 1425, - "high": 1425, - "low": 1425, - "open": 1425, - "volume": 1425 - }, - "open": "2020-11-04T22:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 36284, - "high": 36284, - "low": 5215, - "open": 5215, - "volume": 102994 - }, - "id": 2760546, - "non_hive": { - "close": 4208, - "high": 4208, - "low": 604, - "open": 604, - "volume": 11942 - }, - "open": "2020-11-04T22:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 8738, - "high": 25894, - "low": 8738, - "open": 25894, - "volume": 52022 - }, - "id": 2760549, - "non_hive": { - "close": 996, - "high": 3000, - "low": 996, - "open": 3000, - "volume": 5996 - }, - "open": "2020-11-04T22:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 17390, - "high": 17390, - "low": 17390, - "open": 17390, - "volume": 17390 - }, - "id": 2760552, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-04T22:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 1179, - "high": 1179, - "low": 1179, - "open": 1179, - "volume": 1179 - }, - "id": 2760554, - "non_hive": { - "close": 136, - "high": 136, - "low": 136, - "open": 136, - "volume": 136 - }, - "open": "2020-11-04T22:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 9046, - "high": 9046, - "low": 9046, - "open": 9046, - "volume": 9046 - }, - "id": 2760557, - "non_hive": { - "close": 1043, - "high": 1043, - "low": 1043, - "open": 1043, - "volume": 1043 - }, - "open": "2020-11-04T22:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 432543, - "high": 432543, - "low": 432543, - "open": 432543, - "volume": 432543 - }, - "id": 2760560, - "non_hive": { - "close": 49950, - "high": 49950, - "low": 49950, - "open": 49950, - "volume": 49950 - }, - "open": "2020-11-04T22:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 17390, - "high": 17390, - "low": 17390, - "open": 17390, - "volume": 17390 - }, - "id": 2760562, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-04T22:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 948941, - "high": 948941, - "low": 455011, - "open": 14567, - "volume": 1425001 - }, - "id": 2760565, - "non_hive": { - "close": 109593, - "high": 109593, - "low": 52475, - "open": 1680, - "volume": 164496 - }, - "open": "2020-11-04T22:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 129266, - "high": 129266, - "low": 129266, - "open": 129266, - "volume": 129266 - }, - "id": 2760567, - "non_hive": { - "close": 14929, - "high": 14929, - "low": 14929, - "open": 14929, - "volume": 14929 - }, - "open": "2020-11-04T22:48:00", - "seconds": 60 - }, - { - "hive": { - "close": 3905, - "high": 3905, - "low": 637356, - "open": 637356, - "volume": 641261 - }, - "id": 2760570, - "non_hive": { - "close": 453, - "high": 453, - "low": 73608, - "open": 73608, - "volume": 74061 - }, - "open": "2020-11-04T22:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 14092, - "high": 14092, - "low": 14092, - "open": 14092, - "volume": 14092 - }, - "id": 2760572, - "non_hive": { - "close": 1634, - "high": 1634, - "low": 1634, - "open": 1634, - "volume": 1634 - }, - "open": "2020-11-04T22:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 507782, - "high": 672, - "low": 507782, - "open": 672, - "volume": 929053 - }, - "id": 2760575, - "non_hive": { - "close": 58395, - "high": 78, - "low": 58395, - "open": 78, - "volume": 106859 - }, - "open": "2020-11-04T22:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 58446, - "high": 58446, - "low": 17391, - "open": 17391, - "volume": 158357 - }, - "id": 2760578, - "non_hive": { - "close": 6779, - "high": 6779, - "low": 1999, - "open": 1999, - "volume": 18277 - }, - "open": "2020-11-04T22:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 6547, - "high": 6547, - "low": 2562, - "open": 2562, - "volume": 9109 - }, - "id": 2760581, - "non_hive": { - "close": 759, - "high": 759, - "low": 297, - "open": 297, - "volume": 1056 - }, - "open": "2020-11-04T23:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 53481, - "high": 53481, - "low": 53481, - "open": 53481, - "volume": 53481 - }, - "id": 2760586, - "non_hive": { - "close": 6200, - "high": 6200, - "low": 6200, - "open": 6200, - "volume": 6200 - }, - "open": "2020-11-04T23:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 40464, - "high": 40464, - "low": 40464, - "open": 40464, - "volume": 40464 - }, - "id": 2760589, - "non_hive": { - "close": 4691, - "high": 4691, - "low": 4691, - "open": 4691, - "volume": 4691 - }, - "open": "2020-11-04T23:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 34141, - "high": 34141, - "low": 34141, - "open": 34141, - "volume": 34141 - }, - "id": 2760591, - "non_hive": { - "close": 3958, - "high": 3958, - "low": 3958, - "open": 3958, - "volume": 3958 - }, - "open": "2020-11-04T23:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 25998, - "high": 25998, - "low": 25998, - "open": 25998, - "volume": 25998 - }, - "id": 2760593, - "non_hive": { - "close": 3014, - "high": 3014, - "low": 3014, - "open": 3014, - "volume": 3014 - }, - "open": "2020-11-04T23:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 32571, - "high": 32571, - "low": 32571, - "open": 32571, - "volume": 32571 - }, - "id": 2760596, - "non_hive": { - "close": 3776, - "high": 3776, - "low": 3776, - "open": 3776, - "volume": 3776 - }, - "open": "2020-11-04T23:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 5232, - "high": 5232, - "low": 5232, - "open": 5232, - "volume": 5232 - }, - "id": 2760599, - "non_hive": { - "close": 606, - "high": 606, - "low": 606, - "open": 606, - "volume": 606 - }, - "open": "2020-11-04T23:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 14552, - "high": 14552, - "low": 14552, - "open": 14552, - "volume": 14552 - }, - "id": 2760601, - "non_hive": { - "close": 1687, - "high": 1687, - "low": 1687, - "open": 1687, - "volume": 1687 - }, - "open": "2020-11-04T23:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 517, - "high": 517, - "low": 20000, - "open": 20000, - "volume": 173042 - }, - "id": 2760603, - "non_hive": { - "close": 60, - "high": 60, - "low": 2318, - "open": 2318, - "volume": 20060 - }, - "open": "2020-11-04T23:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 10942, - "high": 10942, - "low": 10942, - "open": 10942, - "volume": 10942 - }, - "id": 2760607, - "non_hive": { - "close": 1250, - "high": 1250, - "low": 1250, - "open": 1250, - "volume": 1250 - }, - "open": "2020-11-04T23:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 3080, - "high": 3080, - "low": 3080, - "open": 3080, - "volume": 3080 - }, - "id": 2760610, - "non_hive": { - "close": 357, - "high": 357, - "low": 357, - "open": 357, - "volume": 357 - }, - "open": "2020-11-04T23:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 27735, - "high": 27735, - "low": 7862, - "open": 7862, - "volume": 35597 - }, - "id": 2760612, - "non_hive": { - "close": 3215, - "high": 3215, - "low": 911, - "open": 911, - "volume": 4126 - }, - "open": "2020-11-04T23:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 20912, - "high": 20912, - "low": 20912, - "open": 20912, - "volume": 20912 - }, - "id": 2760615, - "non_hive": { - "close": 2424, - "high": 2424, - "low": 2424, - "open": 2424, - "volume": 2424 - }, - "open": "2020-11-04T23:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 10904, - "high": 10904, - "low": 10904, - "open": 10904, - "volume": 10904 - }, - "id": 2760618, - "non_hive": { - "close": 1264, - "high": 1264, - "low": 1264, - "open": 1264, - "volume": 1264 - }, - "open": "2020-11-05T00:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 141408, - "high": 141408, - "low": 141408, - "open": 141408, - "volume": 141408 - }, - "id": 2760623, - "non_hive": { - "close": 16391, - "high": 16391, - "low": 16391, - "open": 16391, - "volume": 16391 - }, - "open": "2020-11-05T00:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 433, - "high": 59509, - "low": 433, - "open": 59509, - "volume": 59942 - }, - "id": 2760626, - "non_hive": { - "close": 49, - "high": 6898, - "low": 49, - "open": 6898, - "volume": 6947 - }, - "open": "2020-11-05T00:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 145566, - "high": 6136, - "low": 145566, - "open": 6136, - "volume": 300000 - }, - "id": 2760628, - "non_hive": { - "close": 16627, - "high": 701, - "low": 16627, - "open": 701, - "volume": 34269 - }, - "open": "2020-11-05T00:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 2074, - "high": 2074, - "low": 6569, - "open": 6569, - "volume": 8643 - }, - "id": 2760630, - "non_hive": { - "close": 240, - "high": 240, - "low": 759, - "open": 759, - "volume": 999 - }, - "open": "2020-11-05T00:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 26611, - "high": 26611, - "low": 26611, - "open": 26611, - "volume": 26611 - }, - "id": 2760633, - "non_hive": { - "close": 3085, - "high": 3085, - "low": 3085, - "open": 3085, - "volume": 3085 - }, - "open": "2020-11-05T00:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 36229, - "high": 36229, - "low": 36229, - "open": 36229, - "volume": 36229 - }, - "id": 2760636, - "non_hive": { - "close": 4200, - "high": 4200, - "low": 4200, - "open": 4200, - "volume": 4200 - }, - "open": "2020-11-05T00:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 73874, - "high": 73874, - "low": 73874, - "open": 73874, - "volume": 73874 - }, - "id": 2760638, - "non_hive": { - "close": 8564, - "high": 8564, - "low": 8564, - "open": 8564, - "volume": 8564 - }, - "open": "2020-11-05T00:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 7514, - "high": 7514, - "low": 19092, - "open": 78, - "volume": 58347 - }, - "id": 2760641, - "non_hive": { - "close": 870, - "high": 870, - "low": 2180, - "open": 9, - "volume": 6676 - }, - "open": "2020-11-05T00:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 310, - "high": 33451, - "low": 310, - "open": 9752, - "volume": 43513 - }, - "id": 2760645, - "non_hive": { - "close": 35, - "high": 3873, - "low": 35, - "open": 1129, - "volume": 5037 - }, - "open": "2020-11-05T00:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 266349, - "high": 266349, - "low": 8852, - "open": 8852, - "volume": 275201 - }, - "id": 2760649, - "non_hive": { - "close": 30874, - "high": 30874, - "low": 1026, - "open": 1026, - "volume": 31900 - }, - "open": "2020-11-05T00:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 19714, - "high": 19714, - "low": 5610, - "open": 5610, - "volume": 25324 - }, - "id": 2760652, - "non_hive": { - "close": 2285, - "high": 2285, - "low": 647, - "open": 647, - "volume": 2932 - }, - "open": "2020-11-05T01:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 1877, - "high": 1877, - "low": 1877, - "open": 1877, - "volume": 1877 - }, - "id": 2760656, - "non_hive": { - "close": 217, - "high": 217, - "low": 217, - "open": 217, - "volume": 217 - }, - "open": "2020-11-05T01:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 3002, - "high": 3002, - "low": 3002, - "open": 3002, - "volume": 3002 - }, - "id": 2760659, - "non_hive": { - "close": 348, - "high": 348, - "low": 348, - "open": 348, - "volume": 348 - }, - "open": "2020-11-05T01:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 13080, - "high": 13080, - "low": 13080, - "open": 13080, - "volume": 13080 - }, - "id": 2760662, - "non_hive": { - "close": 1516, - "high": 1516, - "low": 1516, - "open": 1516, - "volume": 1516 - }, - "open": "2020-11-05T01:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 5626, - "high": 5626, - "low": 5626, - "open": 5626, - "volume": 5626 - }, - "id": 2760665, - "non_hive": { - "close": 652, - "high": 652, - "low": 652, - "open": 652, - "volume": 652 - }, - "open": "2020-11-05T02:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 164164, - "high": 164164, - "low": 164164, - "open": 164164, - "volume": 164164 - }, - "id": 2760669, - "non_hive": { - "close": 19027, - "high": 19027, - "low": 19027, - "open": 19027, - "volume": 19027 - }, - "open": "2020-11-05T02:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 3295, - "high": 3295, - "low": 3295, - "open": 3295, - "volume": 3295 - }, - "id": 2760672, - "non_hive": { - "close": 382, - "high": 382, - "low": 382, - "open": 382, - "volume": 382 - }, - "open": "2020-11-05T02:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 1231, - "high": 1231, - "low": 1231, - "open": 1231, - "volume": 1231 - }, - "id": 2760675, - "non_hive": { - "close": 142, - "high": 142, - "low": 142, - "open": 142, - "volume": 142 - }, - "open": "2020-11-05T02:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 1682, - "high": 1682, - "low": 1682, - "open": 1682, - "volume": 1682 - }, - "id": 2760678, - "non_hive": { - "close": 195, - "high": 195, - "low": 195, - "open": 195, - "volume": 195 - }, - "open": "2020-11-05T02:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 5220, - "high": 5220, - "low": 5220, - "open": 5220, - "volume": 5220 - }, - "id": 2760681, - "non_hive": { - "close": 604, - "high": 604, - "low": 604, - "open": 604, - "volume": 604 - }, - "open": "2020-11-05T02:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 18722, - "high": 18722, - "low": 18722, - "open": 18722, - "volume": 18722 - }, - "id": 2760684, - "non_hive": { - "close": 2170, - "high": 2170, - "low": 2170, - "open": 2170, - "volume": 2170 - }, - "open": "2020-11-05T03:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 2769, - "high": 2769, - "low": 2769, - "open": 2769, - "volume": 2769 - }, - "id": 2760688, - "non_hive": { - "close": 321, - "high": 321, - "low": 321, - "open": 321, - "volume": 321 - }, - "open": "2020-11-05T03:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2760691, - "non_hive": { - "close": 115, - "high": 115, - "low": 115, - "open": 115, - "volume": 115 - }, - "open": "2020-11-05T03:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 56970, - "high": 56970, - "low": 56970, - "open": 56970, - "volume": 56970 - }, - "id": 2760694, - "non_hive": { - "close": 6603, - "high": 6603, - "low": 6603, - "open": 6603, - "volume": 6603 - }, - "open": "2020-11-05T03:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 551, - "high": 4616, - "low": 551, - "open": 673, - "volume": 5840 - }, - "id": 2760697, - "non_hive": { - "close": 63, - "high": 535, - "low": 63, - "open": 78, - "volume": 676 - }, - "open": "2020-11-05T03:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 2727, - "high": 2727, - "low": 2727, - "open": 2727, - "volume": 2727 - }, - "id": 2760700, - "non_hive": { - "close": 316, - "high": 316, - "low": 316, - "open": 316, - "volume": 316 - }, - "open": "2020-11-05T03:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 114546, - "high": 114546, - "low": 114546, - "open": 114546, - "volume": 114546 - }, - "id": 2760703, - "non_hive": { - "close": 13272, - "high": 13272, - "low": 13272, - "open": 13272, - "volume": 13272 - }, - "open": "2020-11-05T03:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 1018, - "high": 1018, - "low": 1018, - "open": 1018, - "volume": 1018 - }, - "id": 2760705, - "non_hive": { - "close": 118, - "high": 118, - "low": 118, - "open": 118, - "volume": 118 - }, - "open": "2020-11-05T04:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 52, - "high": 54112, - "low": 52, - "open": 54112, - "volume": 54164 - }, - "id": 2760709, - "non_hive": { - "close": 6, - "high": 6244, - "low": 6, - "open": 6244, - "volume": 6250 - }, - "open": "2020-11-05T04:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 253525, - "high": 253525, - "low": 65386, - "open": 65386, - "volume": 1192149 - }, - "id": 2760712, - "non_hive": { - "close": 29375, - "high": 29375, - "low": 7545, - "open": 7545, - "volume": 138087 - }, - "open": "2020-11-05T04:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 260, - "high": 27170, - "low": 260, - "open": 27170, - "volume": 27586 - }, - "id": 2760715, - "non_hive": { - "close": 29, - "high": 3148, - "low": 29, - "open": 3148, - "volume": 3195 - }, - "open": "2020-11-05T04:21:00", - "seconds": 60 - }, - { - "hive": { - "close": 14000, - "high": 14000, - "low": 14000, - "open": 14000, - "volume": 14000 - }, - "id": 2760718, - "non_hive": { - "close": 1597, - "high": 1597, - "low": 1597, - "open": 1597, - "volume": 1597 - }, - "open": "2020-11-05T04:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 7267, - "high": 3532, - "low": 7267, - "open": 3532, - "volume": 17452 - }, - "id": 2760721, - "non_hive": { - "close": 829, - "high": 403, - "low": 829, - "open": 403, - "volume": 1991 - }, - "open": "2020-11-05T04:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 191272, - "high": 191272, - "low": 17263, - "open": 17263, - "volume": 1581663 - }, - "id": 2760724, - "non_hive": { - "close": 22164, - "high": 22164, - "low": 1999, - "open": 1999, - "volume": 183261 - }, - "open": "2020-11-05T04:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 8, - "high": 8, - "low": 8728, - "open": 8728, - "volume": 8736 - }, - "id": 2760727, - "non_hive": { - "close": 1, - "high": 1, - "low": 1011, - "open": 1011, - "volume": 1012 - }, - "open": "2020-11-05T04:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 17580, - "high": 17580, - "low": 17580, - "open": 17580, - "volume": 17580 - }, - "id": 2760729, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T04:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 17544, - "high": 17544, - "low": 17544, - "open": 17544, - "volume": 17544 - }, - "id": 2760732, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T04:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 17394, - "high": 17394, - "low": 17451, - "open": 17451, - "volume": 34845 - }, - "id": 2760734, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-05T04:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 17394, - "high": 17394, - "low": 17394, - "open": 17394, - "volume": 34788 - }, - "id": 2760737, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-05T04:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 12261, - "high": 12261, - "low": 17356, - "open": 17356, - "volume": 29617 - }, - "id": 2760740, - "non_hive": { - "close": 1413, - "high": 1413, - "low": 2000, - "open": 2000, - "volume": 3413 - }, - "open": "2020-11-05T04:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 59, - "high": 59, - "low": 59, - "open": 59, - "volume": 59 - }, - "id": 2760743, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-05T04:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 17256, - "high": 17256, - "low": 17256, - "open": 17256, - "volume": 17256 - }, - "id": 2760746, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T04:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 10819, - "high": 10819, - "low": 10819, - "open": 10819, - "volume": 10819 - }, - "id": 2760749, - "non_hive": { - "close": 1254, - "high": 1254, - "low": 1254, - "open": 1254, - "volume": 1254 - }, - "open": "2020-11-05T04:57:00", - "seconds": 60 - }, - { - "hive": { - "close": 6315, - "high": 6315, - "low": 6315, - "open": 6315, - "volume": 6315 - }, - "id": 2760751, - "non_hive": { - "close": 732, - "high": 732, - "low": 732, - "open": 732, - "volume": 732 - }, - "open": "2020-11-05T04:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 5533, - "high": 5533, - "low": 5533, - "open": 5533, - "volume": 5533 - }, - "id": 2760753, - "non_hive": { - "close": 640, - "high": 640, - "low": 640, - "open": 640, - "volume": 640 - }, - "open": "2020-11-05T05:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 12407, - "high": 12407, - "low": 12407, - "open": 12407, - "volume": 12407 - }, - "id": 2760757, - "non_hive": { - "close": 1438, - "high": 1438, - "low": 1438, - "open": 1438, - "volume": 1438 - }, - "open": "2020-11-05T05:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 7911, - "high": 7911, - "low": 7911, - "open": 7911, - "volume": 7911 - }, - "id": 2760760, - "non_hive": { - "close": 917, - "high": 917, - "low": 917, - "open": 917, - "volume": 917 - }, - "open": "2020-11-05T05:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 11181, - "high": 11181, - "low": 11181, - "open": 11181, - "volume": 11181 - }, - "id": 2760763, - "non_hive": { - "close": 1296, - "high": 1296, - "low": 1296, - "open": 1296, - "volume": 1296 - }, - "open": "2020-11-05T05:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 6445, - "high": 6445, - "low": 6445, - "open": 6445, - "volume": 6445 - }, - "id": 2760766, - "non_hive": { - "close": 746, - "high": 746, - "low": 746, - "open": 746, - "volume": 746 - }, - "open": "2020-11-05T05:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 10327, - "high": 10327, - "low": 10327, - "open": 10327, - "volume": 10327 - }, - "id": 2760769, - "non_hive": { - "close": 1197, - "high": 1197, - "low": 1197, - "open": 1197, - "volume": 1197 - }, - "open": "2020-11-05T06:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 5851, - "high": 5851, - "low": 5851, - "open": 5851, - "volume": 5851 - }, - "id": 2760773, - "non_hive": { - "close": 678, - "high": 678, - "low": 678, - "open": 678, - "volume": 678 - }, - "open": "2020-11-05T06:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 853, - "high": 853, - "low": 344514, - "open": 344514, - "volume": 345367 - }, - "id": 2760776, - "non_hive": { - "close": 99, - "high": 99, - "low": 39929, - "open": 39929, - "volume": 40028 - }, - "open": "2020-11-05T06:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 51, - "high": 51, - "low": 51, - "open": 51, - "volume": 51 - }, - "id": 2760778, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-05T06:13:00", - "seconds": 60 - }, - { - "hive": { - "close": 154993, - "high": 154993, - "low": 154993, - "open": 154993, - "volume": 154993 - }, - "id": 2760780, - "non_hive": { - "close": 17968, - "high": 17968, - "low": 17968, - "open": 17968, - "volume": 17968 - }, - "open": "2020-11-05T06:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 9903, - "high": 9903, - "low": 9903, - "open": 9903, - "volume": 9903 - }, - "id": 2760783, - "non_hive": { - "close": 1148, - "high": 1148, - "low": 1148, - "open": 1148, - "volume": 1148 - }, - "open": "2020-11-05T06:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 13544, - "high": 13544, - "low": 13544, - "open": 13544, - "volume": 13544 - }, - "id": 2760785, - "non_hive": { - "close": 1570, - "high": 1570, - "low": 1570, - "open": 1570, - "volume": 1570 - }, - "open": "2020-11-05T06:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 28341, - "high": 6635, - "low": 28341, - "open": 6635, - "volume": 58214 - }, - "id": 2760788, - "non_hive": { - "close": 3283, - "high": 769, - "low": 3283, - "open": 769, - "volume": 6744 - }, - "open": "2020-11-05T06:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 3332, - "high": 3332, - "low": 3332, - "open": 3332, - "volume": 3332 - }, - "id": 2760791, - "non_hive": { - "close": 386, - "high": 386, - "low": 386, - "open": 386, - "volume": 386 - }, - "open": "2020-11-05T06:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 1467, - "high": 1467, - "low": 1467, - "open": 1467, - "volume": 1467 - }, - "id": 2760793, - "non_hive": { - "close": 170, - "high": 170, - "low": 170, - "open": 170, - "volume": 170 - }, - "open": "2020-11-05T06:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 6076, - "high": 6076, - "low": 615, - "open": 6068, - "volume": 12759 - }, - "id": 2760796, - "non_hive": { - "close": 704, - "high": 704, - "low": 71, - "open": 703, - "volume": 1478 - }, - "open": "2020-11-05T06:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 4330, - "high": 4330, - "low": 1753, - "open": 6076, - "volume": 14040 - }, - "id": 2760799, - "non_hive": { - "close": 502, - "high": 502, - "low": 203, - "open": 704, - "volume": 1627 - }, - "open": "2020-11-05T06:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 6064, - "high": 6064, - "low": 6064, - "open": 6064, - "volume": 12128 - }, - "id": 2760804, - "non_hive": { - "close": 703, - "high": 703, - "low": 703, - "open": 703, - "volume": 1406 - }, - "open": "2020-11-05T06:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 6064, - "high": 6064, - "low": 6064, - "open": 6064, - "volume": 6064 - }, - "id": 2760807, - "non_hive": { - "close": 703, - "high": 703, - "low": 703, - "open": 703, - "volume": 703 - }, - "open": "2020-11-05T06:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 21714, - "high": 65519, - "low": 21714, - "open": 65519, - "volume": 87233 - }, - "id": 2760809, - "non_hive": { - "close": 2517, - "high": 7595, - "low": 2517, - "open": 7595, - "volume": 10112 - }, - "open": "2020-11-05T06:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 3451, - "high": 3451, - "low": 3451, - "open": 3451, - "volume": 3451 - }, - "id": 2760813, - "non_hive": { - "close": 400, - "high": 400, - "low": 400, - "open": 400, - "volume": 400 - }, - "open": "2020-11-05T06:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 6050, - "high": 6050, - "low": 6050, - "open": 6050, - "volume": 6050 - }, - "id": 2760815, - "non_hive": { - "close": 701, - "high": 701, - "low": 701, - "open": 701, - "volume": 701 - }, - "open": "2020-11-05T06:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 17253, - "high": 17253, - "low": 17253, - "open": 17253, - "volume": 17253 - }, - "id": 2760818, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T07:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 6040, - "high": 6040, - "low": 6040, - "open": 6040, - "volume": 6040 - }, - "id": 2760822, - "non_hive": { - "close": 699, - "high": 699, - "low": 699, - "open": 699, - "volume": 699 - }, - "open": "2020-11-05T07:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 13106, - "high": 13106, - "low": 13106, - "open": 13106, - "volume": 13106 - }, - "id": 2760824, - "non_hive": { - "close": 1518, - "high": 1518, - "low": 1518, - "open": 1518, - "volume": 1518 - }, - "open": "2020-11-05T07:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 2286, - "high": 2286, - "low": 2286, - "open": 2286, - "volume": 2286 - }, - "id": 2760826, - "non_hive": { - "close": 265, - "high": 265, - "low": 265, - "open": 265, - "volume": 265 - }, - "open": "2020-11-05T07:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 79325, - "high": 79325, - "low": 79325, - "open": 79325, - "volume": 79325 - }, - "id": 2760829, - "non_hive": { - "close": 9191, - "high": 9191, - "low": 9191, - "open": 9191, - "volume": 9191 - }, - "open": "2020-11-05T07:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 341690, - "high": 341690, - "low": 341690, - "open": 341690, - "volume": 341690 - }, - "id": 2760831, - "non_hive": { - "close": 39450, - "high": 39450, - "low": 39450, - "open": 39450, - "volume": 39450 - }, - "open": "2020-11-05T07:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 17321, - "high": 17321, - "low": 17321, - "open": 17321, - "volume": 17321 - }, - "id": 2760834, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T07:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 17290, - "high": 4979, - "low": 17321, - "open": 17321, - "volume": 39590 - }, - "id": 2760836, - "non_hive": { - "close": 1997, - "high": 577, - "low": 2000, - "open": 2000, - "volume": 4574 - }, - "open": "2020-11-05T07:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 202049, - "high": 7793, - "low": 202049, - "open": 7793, - "volume": 739762 - }, - "id": 2760841, - "non_hive": { - "close": 23326, - "high": 900, - "low": 23326, - "open": 900, - "volume": 85409 - }, - "open": "2020-11-05T07:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 3949, - "high": 6106, - "low": 3949, - "open": 6106, - "volume": 10055 - }, - "id": 2760843, - "non_hive": { - "close": 455, - "high": 705, - "low": 455, - "open": 705, - "volume": 1160 - }, - "open": "2020-11-05T07:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 51889, - "high": 51889, - "low": 17256, - "open": 17256, - "volume": 79145 - }, - "id": 2760846, - "non_hive": { - "close": 6015, - "high": 6015, - "low": 1999, - "open": 1999, - "volume": 9173 - }, - "open": "2020-11-05T07:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 146750, - "high": 146750, - "low": 146750, - "open": 146750, - "volume": 146750 - }, - "id": 2760849, - "non_hive": { - "close": 17000, - "high": 17000, - "low": 17000, - "open": 17000, - "volume": 17000 - }, - "open": "2020-11-05T07:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 17271, - "high": 17271, - "low": 17271, - "open": 17271, - "volume": 34542 - }, - "id": 2760851, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-05T07:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 17271, - "high": 17271, - "low": 17271, - "open": 17271, - "volume": 34542 - }, - "id": 2760855, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-05T07:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 17271, - "high": 17271, - "low": 17271, - "open": 17271, - "volume": 34542 - }, - "id": 2760858, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-05T07:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 9453, - "high": 9453, - "low": 9453, - "open": 9453, - "volume": 9453 - }, - "id": 2760861, - "non_hive": { - "close": 1094, - "high": 1094, - "low": 1094, - "open": 1094, - "volume": 1094 - }, - "open": "2020-11-05T07:48:00", - "seconds": 60 - }, - { - "hive": { - "close": 6185, - "high": 6185, - "low": 6185, - "open": 6185, - "volume": 6185 - }, - "id": 2760863, - "non_hive": { - "close": 713, - "high": 713, - "low": 713, - "open": 713, - "volume": 713 - }, - "open": "2020-11-05T07:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 11160, - "high": 11160, - "low": 11160, - "open": 11160, - "volume": 11160 - }, - "id": 2760866, - "non_hive": { - "close": 1287, - "high": 1287, - "low": 1287, - "open": 1287, - "volume": 1287 - }, - "open": "2020-11-05T07:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 3859, - "high": 3859, - "low": 3859, - "open": 3859, - "volume": 3859 - }, - "id": 2760869, - "non_hive": { - "close": 445, - "high": 445, - "low": 445, - "open": 445, - "volume": 445 - }, - "open": "2020-11-05T07:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 17343, - "high": 17343, - "low": 17343, - "open": 17343, - "volume": 17343 - }, - "id": 2760871, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T07:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 239320, - "high": 239320, - "low": 88840, - "open": 88840, - "volume": 328160 - }, - "id": 2760873, - "non_hive": { - "close": 27742, - "high": 27742, - "low": 10245, - "open": 10245, - "volume": 37987 - }, - "open": "2020-11-05T08:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 17003, - "high": 17003, - "low": 17003, - "open": 17003, - "volume": 17003 - }, - "id": 2760877, - "non_hive": { - "close": 1971, - "high": 1971, - "low": 1971, - "open": 1971, - "volume": 1971 - }, - "open": "2020-11-05T08:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 3879, - "high": 3879, - "low": 3879, - "open": 3879, - "volume": 3879 - }, - "id": 2760880, - "non_hive": { - "close": 448, - "high": 448, - "low": 448, - "open": 448, - "volume": 448 - }, - "open": "2020-11-05T08:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 14656, - "high": 14656, - "low": 14656, - "open": 14656, - "volume": 14656 - }, - "id": 2760883, - "non_hive": { - "close": 1699, - "high": 1699, - "low": 1699, - "open": 1699, - "volume": 1699 - }, - "open": "2020-11-05T08:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 13276, - "high": 13276, - "low": 13276, - "open": 13276, - "volume": 13276 - }, - "id": 2760886, - "non_hive": { - "close": 1539, - "high": 1539, - "low": 1539, - "open": 1539, - "volume": 1539 - }, - "open": "2020-11-05T09:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 17313, - "high": 17313, - "low": 17313, - "open": 17313, - "volume": 17313 - }, - "id": 2760890, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T09:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 17313, - "high": 17313, - "low": 8663, - "open": 17313, - "volume": 43289 - }, - "id": 2760893, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 1000, - "open": 2000, - "volume": 5000 - }, - "open": "2020-11-05T09:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 8658, - "high": 8658, - "low": 8658, - "open": 8658, - "volume": 8658 - }, - "id": 2760896, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-11-05T09:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 821430, - "high": 821430, - "low": 17253, - "open": 17253, - "volume": 4017999 - }, - "id": 2760898, - "non_hive": { - "close": 96518, - "high": 96518, - "low": 1999, - "open": 1999, - "volume": 470040 - }, - "open": "2020-11-05T09:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 17024, - "high": 17024, - "low": 17024, - "open": 17024, - "volume": 17024 - }, - "id": 2760901, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T09:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 72362, - "high": 72362, - "low": 72362, - "open": 72362, - "volume": 72362 - }, - "id": 2760904, - "non_hive": { - "close": 8497, - "high": 8497, - "low": 8497, - "open": 8497, - "volume": 8497 - }, - "open": "2020-11-05T09:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 255, - "high": 255, - "low": 255, - "open": 255, - "volume": 255 - }, - "id": 2760906, - "non_hive": { - "close": 30, - "high": 30, - "low": 30, - "open": 30, - "volume": 30 - }, - "open": "2020-11-05T09:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 17180, - "high": 17180, - "low": 17180, - "open": 17180, - "volume": 17180 - }, - "id": 2760909, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T09:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 6048, - "high": 6048, - "low": 6048, - "open": 6048, - "volume": 6048 - }, - "id": 2760911, - "non_hive": { - "close": 704, - "high": 704, - "low": 704, - "open": 704, - "volume": 704 - }, - "open": "2020-11-05T09:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 77, - "high": 77, - "low": 77, - "open": 77, - "volume": 77 - }, - "id": 2760914, - "non_hive": { - "close": 9, - "high": 9, - "low": 9, - "open": 9, - "volume": 9 - }, - "open": "2020-11-05T09:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 7808, - "high": 7808, - "low": 7808, - "open": 7808, - "volume": 7808 - }, - "id": 2760916, - "non_hive": { - "close": 904, - "high": 904, - "low": 904, - "open": 904, - "volume": 904 - }, - "open": "2020-11-05T09:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 17245, - "high": 17245, - "low": 17245, - "open": 17245, - "volume": 17245 - }, - "id": 2760919, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T09:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 17238, - "high": 17238, - "low": 2219136, - "open": 17240, - "volume": 2270854 - }, - "id": 2760921, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 257419, - "open": 2000, - "volume": 263419 - }, - "open": "2020-11-05T09:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 50000, - "high": 17238, - "low": 50000, - "open": 17238, - "volume": 67238 - }, - "id": 2760926, - "non_hive": { - "close": 5800, - "high": 2000, - "low": 5800, - "open": 2000, - "volume": 7800 - }, - "open": "2020-11-05T09:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 17240, - "high": 17240, - "low": 17240, - "open": 17240, - "volume": 17240 - }, - "id": 2760928, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T09:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 134139, - "high": 5861, - "low": 134139, - "open": 1000, - "volume": 141000 - }, - "id": 2760930, - "non_hive": { - "close": 15560, - "high": 680, - "low": 15560, - "open": 116, - "volume": 16356 - }, - "open": "2020-11-05T09:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 17182, - "high": 17182, - "low": 17182, - "open": 17182, - "volume": 17182 - }, - "id": 2760933, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T09:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 567060, - "high": 567060, - "low": 567060, - "open": 567060, - "volume": 567060 - }, - "id": 2760935, - "non_hive": { - "close": 66007, - "high": 66007, - "low": 66007, - "open": 66007, - "volume": 66007 - }, - "open": "2020-11-05T09:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 1486, - "high": 1486, - "low": 1486, - "open": 1486, - "volume": 1486 - }, - "id": 2760938, - "non_hive": { - "close": 173, - "high": 173, - "low": 173, - "open": 173, - "volume": 173 - }, - "open": "2020-11-05T09:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 44935, - "high": 44935, - "low": 17527, - "open": 95273, - "volume": 157735 - }, - "id": 2760940, - "non_hive": { - "close": 5231, - "high": 5231, - "low": 2040, - "open": 11090, - "volume": 18361 - }, - "open": "2020-11-05T09:57:00", - "seconds": 60 - }, - { - "hive": { - "close": 8908, - "high": 8908, - "low": 8908, - "open": 8908, - "volume": 8908 - }, - "id": 2760944, - "non_hive": { - "close": 1046, - "high": 1046, - "low": 1046, - "open": 1046, - "volume": 1046 - }, - "open": "2020-11-05T10:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 360000, - "high": 17189, - "low": 10604, - "open": 17189, - "volume": 394179 - }, - "id": 2760948, - "non_hive": { - "close": 41811, - "high": 2000, - "low": 1231, - "open": 2000, - "volume": 45785 - }, - "open": "2020-11-05T10:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 250286, - "high": 8996, - "low": 250286, - "open": 8996, - "volume": 999381 - }, - "id": 2760951, - "non_hive": { - "close": 29069, - "high": 1045, - "low": 29069, - "open": 1045, - "volume": 116072 - }, - "open": "2020-11-05T10:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 8, - "high": 8, - "low": 619, - "open": 619, - "volume": 627 - }, - "id": 2760954, - "non_hive": { - "close": 1, - "high": 1, - "low": 71, - "open": 71, - "volume": 72 - }, - "open": "2020-11-05T10:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 3698, - "high": 3698, - "low": 3698, - "open": 3698, - "volume": 3698 - }, - "id": 2760957, - "non_hive": { - "close": 434, - "high": 434, - "low": 434, - "open": 434, - "volume": 434 - }, - "open": "2020-11-05T10:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 374, - "high": 374, - "low": 374, - "open": 374, - "volume": 374 - }, - "id": 2760960, - "non_hive": { - "close": 44, - "high": 44, - "low": 44, - "open": 44, - "volume": 44 - }, - "open": "2020-11-05T10:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 7746, - "high": 4121, - "low": 7746, - "open": 4121, - "volume": 11867 - }, - "id": 2760962, - "non_hive": { - "close": 900, - "high": 479, - "low": 900, - "open": 479, - "volume": 1379 - }, - "open": "2020-11-05T10:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 71856, - "high": 71856, - "low": 71856, - "open": 71856, - "volume": 71856 - }, - "id": 2760965, - "non_hive": { - "close": 8433, - "high": 8433, - "low": 8433, - "open": 8433, - "volume": 8433 - }, - "open": "2020-11-05T10:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 7769, - "high": 7769, - "low": 7769, - "open": 7769, - "volume": 7769 - }, - "id": 2760968, - "non_hive": { - "close": 901, - "high": 901, - "low": 901, - "open": 901, - "volume": 901 - }, - "open": "2020-11-05T11:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 22259, - "high": 2525, - "low": 22259, - "open": 2525, - "volume": 46548 - }, - "id": 2760972, - "non_hive": { - "close": 2570, - "high": 296, - "low": 2570, - "open": 296, - "volume": 5383 - }, - "open": "2020-11-05T11:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 4878, - "high": 190, - "low": 4878, - "open": 23500, - "volume": 40500 - }, - "id": 2760976, - "non_hive": { - "close": 563, - "high": 22, - "low": 563, - "open": 2714, - "volume": 4677 - }, - "open": "2020-11-05T11:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 8589, - "high": 8589, - "low": 8589, - "open": 8589, - "volume": 8589 - }, - "id": 2760979, - "non_hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "open": "2020-11-05T11:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 4957, - "high": 1229, - "low": 4957, - "open": 1229, - "volume": 12312 - }, - "id": 2760982, - "non_hive": { - "close": 567, - "high": 142, - "low": 567, - "open": 142, - "volume": 1413 - }, - "open": "2020-11-05T11:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 17168, - "high": 17168, - "low": 17168, - "open": 17168, - "volume": 17168 - }, - "id": 2760984, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T11:13:00", - "seconds": 60 - }, - { - "hive": { - "close": 17, - "high": 17, - "low": 17, - "open": 17, - "volume": 17 - }, - "id": 2760986, - "non_hive": { - "close": 2, - "high": 2, - "low": 2, - "open": 2, - "volume": 2 - }, - "open": "2020-11-05T11:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 17339, - "high": 17339, - "low": 17339, - "open": 17339, - "volume": 17339 - }, - "id": 2760988, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T11:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 60, - "high": 60, - "low": 60, - "open": 60, - "volume": 60 - }, - "id": 2760991, - "non_hive": { - "close": 7, - "high": 7, - "low": 7, - "open": 7, - "volume": 7 - }, - "open": "2020-11-05T12:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 23625, - "high": 23625, - "low": 23625, - "open": 23625, - "volume": 23625 - }, - "id": 2760995, - "non_hive": { - "close": 2725, - "high": 2725, - "low": 2725, - "open": 2725, - "volume": 2725 - }, - "open": "2020-11-05T12:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 667, - "high": 667, - "low": 667, - "open": 667, - "volume": 667 - }, - "id": 2760998, - "non_hive": { - "close": 77, - "high": 77, - "low": 77, - "open": 77, - "volume": 77 - }, - "open": "2020-11-05T12:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 69, - "high": 69, - "low": 69, - "open": 69, - "volume": 69 - }, - "id": 2761000, - "non_hive": { - "close": 8, - "high": 8, - "low": 8, - "open": 8, - "volume": 8 - }, - "open": "2020-11-05T12:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 52, - "high": 52, - "low": 52, - "open": 52, - "volume": 52 - }, - "id": 2761003, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-05T12:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 9087, - "high": 3286, - "low": 9087, - "open": 3286, - "volume": 12373 - }, - "id": 2761005, - "non_hive": { - "close": 1048, - "high": 379, - "low": 1048, - "open": 379, - "volume": 1427 - }, - "open": "2020-11-05T12:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 4804, - "high": 4804, - "low": 4804, - "open": 4804, - "volume": 4804 - }, - "id": 2761008, - "non_hive": { - "close": 554, - "high": 554, - "low": 554, - "open": 554, - "volume": 554 - }, - "open": "2020-11-05T12:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 312, - "high": 312, - "low": 312, - "open": 312, - "volume": 312 - }, - "id": 2761011, - "non_hive": { - "close": 36, - "high": 36, - "low": 36, - "open": 36, - "volume": 36 - }, - "open": "2020-11-05T12:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 75290, - "high": 75290, - "low": 6023, - "open": 17798, - "volume": 99111 - }, - "id": 2761014, - "non_hive": { - "close": 8684, - "high": 8684, - "low": 694, - "open": 2052, - "volume": 11430 - }, - "open": "2020-11-05T13:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 6085, - "high": 6085, - "low": 297, - "open": 297, - "volume": 12460 - }, - "id": 2761018, - "non_hive": { - "close": 705, - "high": 705, - "low": 34, - "open": 34, - "volume": 1440 - }, - "open": "2020-11-05T13:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 16801, - "high": 16801, - "low": 16801, - "open": 16801, - "volume": 16801 - }, - "id": 2761021, - "non_hive": { - "close": 1956, - "high": 1956, - "low": 1956, - "open": 1956, - "volume": 1956 - }, - "open": "2020-11-05T13:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 240, - "high": 240, - "low": 370, - "open": 370, - "volume": 610 - }, - "id": 2761024, - "non_hive": { - "close": 28, - "high": 28, - "low": 43, - "open": 43, - "volume": 71 - }, - "open": "2020-11-05T13:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 50671, - "high": 50671, - "low": 50671, - "open": 50671, - "volume": 50671 - }, - "id": 2761027, - "non_hive": { - "close": 5902, - "high": 5902, - "low": 5902, - "open": 5902, - "volume": 5902 - }, - "open": "2020-11-05T13:48:00", - "seconds": 60 - }, - { - "hive": { - "close": 487948, - "high": 13263, - "low": 487948, - "open": 13263, - "volume": 501211 - }, - "id": 2761029, - "non_hive": { - "close": 56835, - "high": 1545, - "low": 56835, - "open": 1545, - "volume": 58380 - }, - "open": "2020-11-05T13:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 11000, - "high": 50989, - "low": 11000, - "open": 50989, - "volume": 466949 - }, - "id": 2761032, - "non_hive": { - "close": 1280, - "high": 5940, - "low": 1280, - "open": 5940, - "volume": 54389 - }, - "open": "2020-11-05T13:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 738, - "high": 738, - "low": 738, - "open": 738, - "volume": 738 - }, - "id": 2761036, - "non_hive": { - "close": 86, - "high": 86, - "low": 86, - "open": 86, - "volume": 86 - }, - "open": "2020-11-05T13:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 8151, - "high": 8151, - "low": 8151, - "open": 8151, - "volume": 8151 - }, - "id": 2761038, - "non_hive": { - "close": 949, - "high": 949, - "low": 949, - "open": 949, - "volume": 949 - }, - "open": "2020-11-05T13:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 231, - "high": 231, - "low": 8282, - "open": 8282, - "volume": 8513 - }, - "id": 2761040, - "non_hive": { - "close": 27, - "high": 27, - "low": 964, - "open": 964, - "volume": 991 - }, - "open": "2020-11-05T14:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 17191, - "high": 17191, - "low": 17191, - "open": 17191, - "volume": 17191 - }, - "id": 2761045, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T14:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 17191, - "high": 17191, - "low": 17191, - "open": 17191, - "volume": 34382 - }, - "id": 2761047, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-05T14:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 86, - "high": 1855, - "low": 86, - "open": 1855, - "volume": 1941 - }, - "id": 2761050, - "non_hive": { - "close": 10, - "high": 216, - "low": 10, - "open": 216, - "volume": 226 - }, - "open": "2020-11-05T14:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 249, - "high": 249, - "low": 249, - "open": 249, - "volume": 249 - }, - "id": 2761052, - "non_hive": { - "close": 29, - "high": 29, - "low": 29, - "open": 29, - "volume": 29 - }, - "open": "2020-11-05T14:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 15120, - "high": 15120, - "low": 15120, - "open": 15120, - "volume": 15120 - }, - "id": 2761055, - "non_hive": { - "close": 1760, - "high": 1760, - "low": 1760, - "open": 1760, - "volume": 1760 - }, - "open": "2020-11-05T14:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 6010, - "high": 6010, - "low": 6010, - "open": 6010, - "volume": 6010 - }, - "id": 2761057, - "non_hive": { - "close": 700, - "high": 700, - "low": 700, - "open": 700, - "volume": 700 - }, - "open": "2020-11-05T14:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 309759, - "high": 17241, - "low": 309759, - "open": 17241, - "volume": 327000 - }, - "id": 2761060, - "non_hive": { - "close": 35932, - "high": 2000, - "low": 35932, - "open": 2000, - "volume": 37932 - }, - "open": "2020-11-05T14:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 4295, - "high": 4295, - "low": 4295, - "open": 4295, - "volume": 4295 - }, - "id": 2761062, - "non_hive": { - "close": 500, - "high": 500, - "low": 500, - "open": 500, - "volume": 500 - }, - "open": "2020-11-05T14:21:00", - "seconds": 60 - }, - { - "hive": { - "close": 17239, - "high": 17239, - "low": 17239, - "open": 17239, - "volume": 17239 - }, - "id": 2761065, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 17241, - "high": 11828, - "low": 17241, - "open": 11828, - "volume": 29069 - }, - "id": 2761067, - "non_hive": { - "close": 2000, - "high": 1377, - "low": 2000, - "open": 1377, - "volume": 3377 - }, - "open": "2020-11-05T14:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 34482 - }, - "id": 2761071, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-05T14:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 112070, - "high": 17239, - "low": 17242, - "open": 17242, - "volume": 146551 - }, - "id": 2761074, - "non_hive": { - "close": 13000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 17000 - }, - "open": "2020-11-05T14:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 34482 - }, - "id": 2761077, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-05T14:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 9741, - "high": 9741, - "low": 9741, - "open": 9741, - "volume": 9741 - }, - "id": 2761080, - "non_hive": { - "close": 1130, - "high": 1130, - "low": 1130, - "open": 1130, - "volume": 1130 - }, - "open": "2020-11-05T14:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 196815, - "high": 17180, - "low": 17181, - "open": 17180, - "volume": 248356 - }, - "id": 2761082, - "non_hive": { - "close": 22912, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 28912 - }, - "open": "2020-11-05T14:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 233801, - "high": 10012, - "low": 233801, - "open": 10012, - "volume": 253855 - }, - "id": 2761086, - "non_hive": { - "close": 27121, - "high": 1165, - "low": 27121, - "open": 1165, - "volume": 29451 - }, - "open": "2020-11-05T14:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 85811, - "high": 85811, - "low": 17181, - "open": 17181, - "volume": 102992 - }, - "id": 2761089, - "non_hive": { - "close": 9989, - "high": 9989, - "low": 1999, - "open": 1999, - "volume": 11988 - }, - "open": "2020-11-05T14:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 17232, - "high": 17232, - "low": 17232, - "open": 17232, - "volume": 17232 - }, - "id": 2761091, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T14:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 84994, - "high": 3643, - "low": 17171, - "open": 17171, - "volume": 162009 - }, - "id": 2761093, - "non_hive": { - "close": 9985, - "high": 428, - "low": 1999, - "open": 1999, - "volume": 18960 - }, - "open": "2020-11-05T14:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 1984, - "high": 1984, - "low": 1984, - "open": 1984, - "volume": 1984 - }, - "id": 2761097, - "non_hive": { - "close": 230, - "high": 230, - "low": 230, - "open": 230, - "volume": 230 - }, - "open": "2020-11-05T14:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 7250, - "high": 7250, - "low": 2392, - "open": 2392, - "volume": 9642 - }, - "id": 2761099, - "non_hive": { - "close": 854, - "high": 854, - "low": 281, - "open": 281, - "volume": 1135 - }, - "open": "2020-11-05T14:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 17241 - }, - "id": 2761101, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T14:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 44999, - "high": 8328, - "low": 9722, - "open": 9722, - "volume": 63049 - }, - "id": 2761104, - "non_hive": { - "close": 5300, - "high": 981, - "low": 1145, - "open": 1145, - "volume": 7426 - }, - "open": "2020-11-05T14:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 229759, - "high": 229759, - "low": 14949, - "open": 6000, - "volume": 267740 - }, - "id": 2761107, - "non_hive": { - "close": 27061, - "high": 27061, - "low": 1734, - "open": 698, - "volume": 31498 - }, - "open": "2020-11-05T14:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 127010, - "high": 127010, - "low": 16981, - "open": 16981, - "volume": 143991 - }, - "id": 2761112, - "non_hive": { - "close": 14959, - "high": 14959, - "low": 1999, - "open": 1999, - "volume": 16958 - }, - "open": "2020-11-05T14:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 17241, - "high": 17241, - "low": 17241, - "open": 17241, - "volume": 34482 - }, - "id": 2761114, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-05T14:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 13276, - "high": 13276, - "low": 13276, - "open": 13276, - "volume": 13276 - }, - "id": 2761117, - "non_hive": { - "close": 1558, - "high": 1558, - "low": 1558, - "open": 1558, - "volume": 1558 - }, - "open": "2020-11-05T14:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 13000, - "high": 13000, - "low": 13000, - "open": 13000, - "volume": 13000 - }, - "id": 2761120, - "non_hive": { - "close": 1508, - "high": 1508, - "low": 1508, - "open": 1508, - "volume": 1508 - }, - "open": "2020-11-05T14:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 1681, - "high": 1681, - "low": 3758, - "open": 3758, - "volume": 11469 - }, - "id": 2761122, - "non_hive": { - "close": 198, - "high": 198, - "low": 441, - "open": 441, - "volume": 1347 - }, - "open": "2020-11-05T14:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 6000 - }, - "id": 2761124, - "non_hive": { - "close": 698, - "high": 698, - "low": 698, - "open": 698, - "volume": 698 - }, - "open": "2020-11-05T14:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 4480, - "high": 4480, - "low": 4480, - "open": 4480, - "volume": 4480 - }, - "id": 2761127, - "non_hive": { - "close": 521, - "high": 521, - "low": 521, - "open": 521, - "volume": 521 - }, - "open": "2020-11-05T14:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 43623, - "high": 43623, - "low": 18311, - "open": 28717, - "volume": 113715 - }, - "id": 2761129, - "non_hive": { - "close": 5138, - "high": 5138, - "low": 2148, - "open": 3370, - "volume": 13363 - }, - "open": "2020-11-05T14:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 50, - "high": 50, - "low": 17191, - "open": 51, - "volume": 24797 - }, - "id": 2761132, - "non_hive": { - "close": 6, - "high": 6, - "low": 1994, - "open": 6, - "volume": 2890 - }, - "open": "2020-11-05T15:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 6000, - "high": 6000, - "low": 6000, - "open": 6000, - "volume": 6000 - }, - "id": 2761138, - "non_hive": { - "close": 698, - "high": 698, - "low": 698, - "open": 698, - "volume": 698 - }, - "open": "2020-11-05T15:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 178017, - "high": 28926, - "low": 16981, - "open": 7500, - "volume": 237455 - }, - "id": 2761140, - "non_hive": { - "close": 20964, - "high": 3407, - "low": 1999, - "open": 883, - "volume": 27963 - }, - "open": "2020-11-05T15:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 2588, - "high": 51, - "low": 2588, - "open": 51, - "volume": 17242 - }, - "id": 2761144, - "non_hive": { - "close": 300, - "high": 6, - "low": 300, - "open": 6, - "volume": 2006 - }, - "open": "2020-11-05T15:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 17172, - "high": 17172, - "low": 17172, - "open": 17172, - "volume": 17172 - }, - "id": 2761147, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T15:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 5884, - "high": 5116, - "low": 5884, - "open": 5116, - "volume": 11000 - }, - "id": 2761149, - "non_hive": { - "close": 685, - "high": 596, - "low": 685, - "open": 596, - "volume": 1281 - }, - "open": "2020-11-05T15:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 10481, - "high": 10481, - "low": 163, - "open": 163, - "volume": 106768 - }, - "id": 2761151, - "non_hive": { - "close": 1230, - "high": 1230, - "low": 19, - "open": 19, - "volume": 12529 - }, - "open": "2020-11-05T15:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 10000, - "high": 10000, - "low": 10000, - "open": 10000, - "volume": 10000 - }, - "id": 2761156, - "non_hive": { - "close": 1160, - "high": 1160, - "low": 1160, - "open": 1160, - "volume": 1160 - }, - "open": "2020-11-05T15:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 42, - "high": 42, - "low": 2000, - "open": 2000, - "volume": 2042 - }, - "id": 2761159, - "non_hive": { - "close": 5, - "high": 5, - "low": 234, - "open": 234, - "volume": 239 - }, - "open": "2020-11-05T15:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 758127, - "high": 758127, - "low": 6511, - "open": 6511, - "volume": 1000157 - }, - "id": 2761161, - "non_hive": { - "close": 89735, - "high": 89735, - "low": 764, - "open": 764, - "volume": 118364 - }, - "open": "2020-11-05T15:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 5740, - "high": 4075, - "low": 2925, - "open": 4075, - "volume": 17000 - }, - "id": 2761164, - "non_hive": { - "close": 670, - "high": 482, - "low": 341, - "open": 482, - "volume": 1991 - }, - "open": "2020-11-05T15:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 5718, - "high": 282, - "low": 5718, - "open": 282, - "volume": 6000 - }, - "id": 2761168, - "non_hive": { - "close": 665, - "high": 33, - "low": 665, - "open": 33, - "volume": 698 - }, - "open": "2020-11-05T15:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 562, - "high": 562, - "low": 562, - "open": 562, - "volume": 562 - }, - "id": 2761171, - "non_hive": { - "close": 66, - "high": 66, - "low": 66, - "open": 66, - "volume": 66 - }, - "open": "2020-11-05T15:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 2599, - "high": 566, - "low": 2599, - "open": 566, - "volume": 3500 - }, - "id": 2761174, - "non_hive": { - "close": 301, - "high": 66, - "low": 301, - "open": 66, - "volume": 406 - }, - "open": "2020-11-05T15:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 9190, - "high": 810, - "low": 9190, - "open": 810, - "volume": 10000 - }, - "id": 2761176, - "non_hive": { - "close": 1065, - "high": 94, - "low": 1065, - "open": 94, - "volume": 1159 - }, - "open": "2020-11-05T15:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 16472, - "high": 16472, - "low": 16472, - "open": 16472, - "volume": 16472 - }, - "id": 2761179, - "non_hive": { - "close": 1933, - "high": 1933, - "low": 1933, - "open": 1933, - "volume": 1933 - }, - "open": "2020-11-05T15:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 5674, - "high": 5674, - "low": 5674, - "open": 5674, - "volume": 5674 - }, - "id": 2761182, - "non_hive": { - "close": 666, - "high": 666, - "low": 666, - "open": 666, - "volume": 666 - }, - "open": "2020-11-05T15:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 1704, - "high": 1704, - "low": 1704, - "open": 1704, - "volume": 1704 - }, - "id": 2761185, - "non_hive": { - "close": 200, - "high": 200, - "low": 200, - "open": 200, - "volume": 200 - }, - "open": "2020-11-05T15:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 4295, - "high": 4295, - "low": 4295, - "open": 4295, - "volume": 4295 - }, - "id": 2761188, - "non_hive": { - "close": 504, - "high": 504, - "low": 504, - "open": 504, - "volume": 504 - }, - "open": "2020-11-05T15:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 8505, - "high": 8505, - "low": 17045, - "open": 17045, - "volume": 25550 - }, - "id": 2761190, - "non_hive": { - "close": 998, - "high": 998, - "low": 1999, - "open": 1999, - "volume": 2997 - }, - "open": "2020-11-05T16:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 14631, - "high": 14631, - "low": 14631, - "open": 14631, - "volume": 14631 - }, - "id": 2761194, - "non_hive": { - "close": 1716, - "high": 1716, - "low": 1716, - "open": 1716, - "volume": 1716 - }, - "open": "2020-11-05T16:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 31072, - "high": 31072, - "low": 2414, - "open": 2414, - "volume": 33486 - }, - "id": 2761196, - "non_hive": { - "close": 3646, - "high": 3646, - "low": 283, - "open": 283, - "volume": 3929 - }, - "open": "2020-11-05T16:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 503950, - "high": 366, - "low": 250, - "open": 250, - "volume": 504566 - }, - "id": 2761200, - "non_hive": { - "close": 59102, - "high": 43, - "low": 29, - "open": 29, - "volume": 59174 - }, - "open": "2020-11-05T16:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 320032, - "high": 24356, - "low": 320032, - "open": 24356, - "volume": 344388 - }, - "id": 2761203, - "non_hive": { - "close": 37532, - "high": 2858, - "low": 37532, - "open": 2858, - "volume": 40390 - }, - "open": "2020-11-05T16:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 18203, - "high": 707, - "low": 18203, - "open": 707, - "volume": 36003 - }, - "id": 2761206, - "non_hive": { - "close": 2129, - "high": 83, - "low": 2129, - "open": 83, - "volume": 4212 - }, - "open": "2020-11-05T16:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 20, - "high": 50000, - "low": 20, - "open": 50000, - "volume": 50020 - }, - "id": 2761208, - "non_hive": { - "close": 2, - "high": 5850, - "low": 2, - "open": 5850, - "volume": 5852 - }, - "open": "2020-11-05T16:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 1116, - "high": 1116, - "low": 1116, - "open": 1116, - "volume": 1116 - }, - "id": 2761212, - "non_hive": { - "close": 131, - "high": 131, - "low": 131, - "open": 131, - "volume": 131 - }, - "open": "2020-11-05T16:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 9996, - "high": 9996, - "low": 9996, - "open": 9996, - "volume": 9996 - }, - "id": 2761214, - "non_hive": { - "close": 1173, - "high": 1173, - "low": 1173, - "open": 1173, - "volume": 1173 - }, - "open": "2020-11-05T16:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 22047, - "high": 22047, - "low": 22047, - "open": 22047, - "volume": 22047 - }, - "id": 2761217, - "non_hive": { - "close": 2587, - "high": 2587, - "low": 2587, - "open": 2587, - "volume": 2587 - }, - "open": "2020-11-05T16:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 5664, - "high": 5664, - "low": 16841, - "open": 16841, - "volume": 22505 - }, - "id": 2761219, - "non_hive": { - "close": 665, - "high": 665, - "low": 1976, - "open": 1976, - "volume": 2641 - }, - "open": "2020-11-05T16:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 2242, - "high": 104838, - "low": 2242, - "open": 104838, - "volume": 560800 - }, - "id": 2761222, - "non_hive": { - "close": 262, - "high": 12359, - "low": 262, - "open": 12359, - "volume": 65712 - }, - "open": "2020-11-05T16:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 69657, - "high": 69657, - "low": 14867, - "open": 14867, - "volume": 84524 - }, - "id": 2761225, - "non_hive": { - "close": 8143, - "high": 8143, - "low": 1737, - "open": 1737, - "volume": 9880 - }, - "open": "2020-11-05T16:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 15920, - "high": 15920, - "low": 15920, - "open": 15920, - "volume": 15920 - }, - "id": 2761228, - "non_hive": { - "close": 1860, - "high": 1860, - "low": 1860, - "open": 1860, - "volume": 1860 - }, - "open": "2020-11-05T17:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 141440, - "high": 141440, - "low": 17118, - "open": 17118, - "volume": 158558 - }, - "id": 2761232, - "non_hive": { - "close": 16525, - "high": 16525, - "low": 1999, - "open": 1999, - "volume": 18524 - }, - "open": "2020-11-05T17:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 57135, - "high": 57135, - "low": 57135, - "open": 57135, - "volume": 57135 - }, - "id": 2761234, - "non_hive": { - "close": 6672, - "high": 6672, - "low": 6672, - "open": 6672, - "volume": 6672 - }, - "open": "2020-11-05T17:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 14922, - "high": 14922, - "low": 14922, - "open": 14922, - "volume": 14922 - }, - "id": 2761237, - "non_hive": { - "close": 1760, - "high": 1760, - "low": 1760, - "open": 1760, - "volume": 1760 - }, - "open": "2020-11-05T17:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 3510, - "high": 3510, - "low": 3510, - "open": 3510, - "volume": 3510 - }, - "id": 2761240, - "non_hive": { - "close": 414, - "high": 414, - "low": 414, - "open": 414, - "volume": 414 - }, - "open": "2020-11-05T17:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 13440, - "high": 13440, - "low": 13440, - "open": 13440, - "volume": 13440 - }, - "id": 2761242, - "non_hive": { - "close": 1585, - "high": 1585, - "low": 1585, - "open": 1585, - "volume": 1585 - }, - "open": "2020-11-05T17:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 1288, - "high": 1288, - "low": 1288, - "open": 1288, - "volume": 1288 - }, - "id": 2761245, - "non_hive": { - "close": 152, - "high": 152, - "low": 152, - "open": 152, - "volume": 152 - }, - "open": "2020-11-05T17:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 19603, - "high": 19603, - "low": 19603, - "open": 19603, - "volume": 19603 - }, - "id": 2761247, - "non_hive": { - "close": 2312, - "high": 2312, - "low": 2312, - "open": 2312, - "volume": 2312 - }, - "open": "2020-11-05T17:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 40463, - "high": 40463, - "low": 40463, - "open": 40463, - "volume": 40463 - }, - "id": 2761250, - "non_hive": { - "close": 4768, - "high": 4768, - "low": 4768, - "open": 4768, - "volume": 4768 - }, - "open": "2020-11-05T17:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 12897, - "high": 12897, - "low": 12897, - "open": 12897, - "volume": 12897 - }, - "id": 2761253, - "non_hive": { - "close": 1519, - "high": 1519, - "low": 1519, - "open": 1519, - "volume": 1519 - }, - "open": "2020-11-05T17:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 4076, - "high": 4076, - "low": 4076, - "open": 4076, - "volume": 4076 - }, - "id": 2761256, - "non_hive": { - "close": 480, - "high": 480, - "low": 480, - "open": 480, - "volume": 480 - }, - "open": "2020-11-05T17:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 14087, - "high": 14087, - "low": 14087, - "open": 14087, - "volume": 14087 - }, - "id": 2761259, - "non_hive": { - "close": 1660, - "high": 1660, - "low": 1660, - "open": 1660, - "volume": 1660 - }, - "open": "2020-11-05T17:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 712, - "high": 712, - "low": 712, - "open": 712, - "volume": 712 - }, - "id": 2761261, - "non_hive": { - "close": 84, - "high": 84, - "low": 84, - "open": 84, - "volume": 84 - }, - "open": "2020-11-05T17:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 1807, - "high": 1807, - "low": 1807, - "open": 1807, - "volume": 1807 - }, - "id": 2761264, - "non_hive": { - "close": 213, - "high": 213, - "low": 213, - "open": 213, - "volume": 213 - }, - "open": "2020-11-05T18:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 37628, - "high": 37628, - "low": 37628, - "open": 37628, - "volume": 37628 - }, - "id": 2761268, - "non_hive": { - "close": 4434, - "high": 4434, - "low": 4434, - "open": 4434, - "volume": 4434 - }, - "open": "2020-11-05T18:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 10234, - "high": 10234, - "low": 10234, - "open": 10234, - "volume": 10234 - }, - "id": 2761270, - "non_hive": { - "close": 1206, - "high": 1206, - "low": 1206, - "open": 1206, - "volume": 1206 - }, - "open": "2020-11-05T18:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 3988, - "high": 3988, - "low": 3988, - "open": 3988, - "volume": 3988 - }, - "id": 2761273, - "non_hive": { - "close": 470, - "high": 470, - "low": 470, - "open": 470, - "volume": 470 - }, - "open": "2020-11-05T18:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 2172, - "high": 2172, - "low": 2172, - "open": 2172, - "volume": 2172 - }, - "id": 2761276, - "non_hive": { - "close": 256, - "high": 256, - "low": 256, - "open": 256, - "volume": 256 - }, - "open": "2020-11-05T18:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 128, - "high": 1349, - "low": 128, - "open": 5058, - "volume": 22320 - }, - "id": 2761279, - "non_hive": { - "close": 15, - "high": 159, - "low": 15, - "open": 596, - "volume": 2629 - }, - "open": "2020-11-05T18:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 16066, - "high": 16066, - "low": 16066, - "open": 16066, - "volume": 16066 - }, - "id": 2761282, - "non_hive": { - "close": 1874, - "high": 1874, - "low": 1874, - "open": 1874, - "volume": 1874 - }, - "open": "2020-11-05T18:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 4758, - "high": 4758, - "low": 4758, - "open": 4758, - "volume": 4758 - }, - "id": 2761285, - "non_hive": { - "close": 555, - "high": 555, - "low": 555, - "open": 555, - "volume": 555 - }, - "open": "2020-11-05T18:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 9935, - "high": 9935, - "low": 74, - "open": 74, - "volume": 10009 - }, - "id": 2761288, - "non_hive": { - "close": 1159, - "high": 1159, - "low": 8, - "open": 8, - "volume": 1167 - }, - "open": "2020-11-05T18:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 33630, - "high": 33630, - "low": 33630, - "open": 33630, - "volume": 33630 - }, - "id": 2761291, - "non_hive": { - "close": 3923, - "high": 3923, - "low": 3923, - "open": 3923, - "volume": 3923 - }, - "open": "2020-11-05T18:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 8130, - "high": 8130, - "low": 8130, - "open": 8130, - "volume": 8130 - }, - "id": 2761293, - "non_hive": { - "close": 957, - "high": 957, - "low": 957, - "open": 957, - "volume": 957 - }, - "open": "2020-11-05T19:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 8853, - "high": 8853, - "low": 8853, - "open": 8853, - "volume": 8853 - }, - "id": 2761297, - "non_hive": { - "close": 1042, - "high": 1042, - "low": 1042, - "open": 1042, - "volume": 1042 - }, - "open": "2020-11-05T19:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 34546, - "high": 34546, - "low": 4043, - "open": 4043, - "volume": 38589 - }, - "id": 2761299, - "non_hive": { - "close": 4073, - "high": 4073, - "low": 476, - "open": 476, - "volume": 4549 - }, - "open": "2020-11-05T19:30:00", - "seconds": 60 - }, - { - "hive": { - "close": 2985, - "high": 2985, - "low": 2985, - "open": 2985, - "volume": 2985 - }, - "id": 2761302, - "non_hive": { - "close": 352, - "high": 352, - "low": 352, - "open": 352, - "volume": 352 - }, - "open": "2020-11-05T19:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 2298, - "high": 2298, - "low": 10419, - "open": 10419, - "volume": 12717 - }, - "id": 2761304, - "non_hive": { - "close": 271, - "high": 271, - "low": 1227, - "open": 1227, - "volume": 1498 - }, - "open": "2020-11-05T19:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 15369, - "high": 15369, - "low": 15369, - "open": 15369, - "volume": 15369 - }, - "id": 2761307, - "non_hive": { - "close": 1812, - "high": 1812, - "low": 1812, - "open": 1812, - "volume": 1812 - }, - "open": "2020-11-05T19:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 5827, - "high": 5827, - "low": 5827, - "open": 5827, - "volume": 5827 - }, - "id": 2761309, - "non_hive": { - "close": 687, - "high": 687, - "low": 687, - "open": 687, - "volume": 687 - }, - "open": "2020-11-05T19:39:00", - "seconds": 60 - }, - { - "hive": { - "close": 1696, - "high": 1696, - "low": 1696, - "open": 1696, - "volume": 1696 - }, - "id": 2761311, - "non_hive": { - "close": 200, - "high": 200, - "low": 200, - "open": 200, - "volume": 200 - }, - "open": "2020-11-05T19:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 30678, - "high": 30678, - "low": 30678, - "open": 30678, - "volume": 30678 - }, - "id": 2761314, - "non_hive": { - "close": 3617, - "high": 3617, - "low": 3617, - "open": 3617, - "volume": 3617 - }, - "open": "2020-11-05T19:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 1696, - "high": 1696, - "low": 1696, - "open": 1696, - "volume": 1696 - }, - "id": 2761317, - "non_hive": { - "close": 200, - "high": 200, - "low": 200, - "open": 200, - "volume": 200 - }, - "open": "2020-11-05T19:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 3104, - "high": 3104, - "low": 3104, - "open": 3104, - "volume": 3104 - }, - "id": 2761320, - "non_hive": { - "close": 366, - "high": 366, - "low": 366, - "open": 366, - "volume": 366 - }, - "open": "2020-11-05T19:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 6463, - "high": 6463, - "low": 6463, - "open": 6463, - "volume": 6463 - }, - "id": 2761322, - "non_hive": { - "close": 762, - "high": 762, - "low": 762, - "open": 762, - "volume": 762 - }, - "open": "2020-11-05T19:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 27260, - "high": 27260, - "low": 27260, - "open": 27260, - "volume": 27260 - }, - "id": 2761325, - "non_hive": { - "close": 3214, - "high": 3214, - "low": 3214, - "open": 3214, - "volume": 3214 - }, - "open": "2020-11-05T20:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 9007, - "high": 9007, - "low": 9007, - "open": 9007, - "volume": 9007 - }, - "id": 2761329, - "non_hive": { - "close": 1062, - "high": 1062, - "low": 1062, - "open": 1062, - "volume": 1062 - }, - "open": "2020-11-05T20:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 1413828, - "high": 1413828, - "low": 1413828, - "open": 1413828, - "volume": 1413828 - }, - "id": 2761332, - "non_hive": { - "close": 166689, - "high": 166689, - "low": 166689, - "open": 166689, - "volume": 166689 - }, - "open": "2020-11-05T20:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 4005, - "high": 4005, - "low": 4005, - "open": 4005, - "volume": 4005 - }, - "id": 2761334, - "non_hive": { - "close": 472, - "high": 472, - "low": 472, - "open": 472, - "volume": 472 - }, - "open": "2020-11-05T20:13:00", - "seconds": 60 - }, - { - "hive": { - "close": 89932, - "high": 6755, - "low": 89932, - "open": 6755, - "volume": 100000 - }, - "id": 2761336, - "non_hive": { - "close": 10497, - "high": 796, - "low": 10497, - "open": 796, - "volume": 11683 - }, - "open": "2020-11-05T20:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 81, - "high": 81, - "low": 81, - "open": 81, - "volume": 81 - }, - "id": 2761339, - "non_hive": { - "close": 9, - "high": 9, - "low": 9, - "open": 9, - "volume": 9 - }, - "open": "2020-11-05T20:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 19085, - "high": 19085, - "low": 19085, - "open": 19085, - "volume": 19085 - }, - "id": 2761342, - "non_hive": { - "close": 2246, - "high": 2246, - "low": 2246, - "open": 2246, - "volume": 2246 - }, - "open": "2020-11-05T21:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 117431, - "high": 17003, - "low": 117431, - "open": 3782, - "volume": 497003 - }, - "id": 2761346, - "non_hive": { - "close": 13707, - "high": 2001, - "low": 13707, - "open": 445, - "volume": 58035 - }, - "open": "2020-11-05T21:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 17103, - "high": 17103, - "low": 17103, - "open": 17103, - "volume": 34206 - }, - "id": 2761349, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-05T21:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 17046, - "high": 17046, - "low": 17103, - "open": 17103, - "volume": 51195 - }, - "id": 2761352, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 6000 - }, - "open": "2020-11-05T21:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 17046, - "high": 17046, - "low": 17046, - "open": 17046, - "volume": 34092 - }, - "id": 2761356, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-05T21:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 16995, - "high": 16995, - "low": 16995, - "open": 16995, - "volume": 16995 - }, - "id": 2761360, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-05T21:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 4444, - "high": 4011, - "low": 4444, - "open": 4011, - "volume": 8455 - }, - "id": 2761362, - "non_hive": { - "close": 522, - "high": 472, - "low": 522, - "open": 472, - "volume": 994 - }, - "open": "2020-11-05T21:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 8459, - "high": 8459, - "low": 8459, - "open": 8459, - "volume": 8459 - }, - "id": 2761365, - "non_hive": { - "close": 995, - "high": 995, - "low": 995, - "open": 995, - "volume": 995 - }, - "open": "2020-11-05T21:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 8484, - "high": 8484, - "low": 8484, - "open": 8484, - "volume": 8484 - }, - "id": 2761368, - "non_hive": { - "close": 998, - "high": 998, - "low": 998, - "open": 998, - "volume": 998 - }, - "open": "2020-11-05T21:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 17046, - "high": 17046, - "low": 17046, - "open": 17046, - "volume": 34092 - }, - "id": 2761371, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-05T21:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 1234, - "high": 1234, - "low": 17046, - "open": 17046, - "volume": 18280 - }, - "id": 2761374, - "non_hive": { - "close": 145, - "high": 145, - "low": 2000, - "open": 2000, - "volume": 2145 - }, - "open": "2020-11-05T21:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 52, - "high": 52, - "low": 52, - "open": 52, - "volume": 52 - }, - "id": 2761378, - "non_hive": { - "close": 6, - "high": 6, - "low": 6, - "open": 6, - "volume": 6 - }, - "open": "2020-11-05T21:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 12699, - "high": 12301, - "low": 12699, - "open": 12301, - "volume": 25000 - }, - "id": 2761380, - "non_hive": { - "close": 1493, - "high": 1447, - "low": 1493, - "open": 1447, - "volume": 2940 - }, - "open": "2020-11-05T21:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 5987, - "high": 161, - "low": 5987, - "open": 161, - "volume": 13845 - }, - "id": 2761382, - "non_hive": { - "close": 700, - "high": 19, - "low": 700, - "open": 19, - "volume": 1622 - }, - "open": "2020-11-05T21:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 4152, - "high": 838, - "low": 16949, - "open": 16949, - "volume": 21939 - }, - "id": 2761385, - "non_hive": { - "close": 490, - "high": 99, - "low": 1999, - "open": 1999, - "volume": 2588 - }, - "open": "2020-11-05T21:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 17101, - "high": 17100, - "low": 1901, - "open": 17100, - "volume": 36102 - }, - "id": 2761388, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 222, - "open": 2000, - "volume": 4222 - }, - "open": "2020-11-05T21:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 755, - "high": 755, - "low": 755, - "open": 755, - "volume": 755 - }, - "id": 2761392, - "non_hive": { - "close": 89, - "high": 89, - "low": 89, - "open": 89, - "volume": 89 - }, - "open": "2020-11-05T21:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 2020, - "high": 2020, - "low": 2020, - "open": 2020, - "volume": 2020 - }, - "id": 2761394, - "non_hive": { - "close": 238, - "high": 238, - "low": 238, - "open": 238, - "volume": 238 - }, - "open": "2020-11-05T21:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 9364, - "high": 8501, - "low": 9364, - "open": 8501, - "volume": 17865 - }, - "id": 2761397, - "non_hive": { - "close": 1095, - "high": 1001, - "low": 1095, - "open": 1001, - "volume": 2096 - }, - "open": "2020-11-05T21:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 6021, - "high": 6021, - "low": 6021, - "open": 6021, - "volume": 6021 - }, - "id": 2761401, - "non_hive": { - "close": 704, - "high": 704, - "low": 704, - "open": 704, - "volume": 704 - }, - "open": "2020-11-05T21:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 34415, - "high": 77, - "low": 34415, - "open": 77, - "volume": 123000 - }, - "id": 2761403, - "non_hive": { - "close": 4014, - "high": 9, - "low": 4014, - "open": 9, - "volume": 14348 - }, - "open": "2020-11-05T21:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 601, - "high": 601, - "low": 8477, - "open": 8477, - "volume": 111990 - }, - "id": 2761405, - "non_hive": { - "close": 71, - "high": 71, - "low": 998, - "open": 998, - "volume": 13209 - }, - "open": "2020-11-05T21:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 409, - "high": 409, - "low": 409, - "open": 409, - "volume": 409 - }, - "id": 2761408, - "non_hive": { - "close": 48, - "high": 48, - "low": 48, - "open": 48, - "volume": 48 - }, - "open": "2020-11-05T21:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 113016, - "high": 113016, - "low": 6002, - "open": 6002, - "volume": 119018 - }, - "id": 2761410, - "non_hive": { - "close": 13336, - "high": 13336, - "low": 708, - "open": 708, - "volume": 14044 - }, - "open": "2020-11-05T21:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 1000, - "high": 1000, - "low": 1000, - "open": 1000, - "volume": 1000 - }, - "id": 2761414, - "non_hive": { - "close": 117, - "high": 117, - "low": 117, - "open": 117, - "volume": 117 - }, - "open": "2020-11-05T21:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 3476, - "high": 3476, - "low": 3476, - "open": 3476, - "volume": 3476 - }, - "id": 2761416, - "non_hive": { - "close": 410, - "high": 410, - "low": 410, - "open": 410, - "volume": 410 - }, - "open": "2020-11-05T22:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 13474, - "high": 13474, - "low": 13474, - "open": 13474, - "volume": 13474 - }, - "id": 2761420, - "non_hive": { - "close": 1589, - "high": 1589, - "low": 1589, - "open": 1589, - "volume": 1589 - }, - "open": "2020-11-05T22:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 15143, - "high": 992, - "low": 15143, - "open": 992, - "volume": 16135 - }, - "id": 2761422, - "non_hive": { - "close": 1786, - "high": 117, - "low": 1786, - "open": 117, - "volume": 1903 - }, - "open": "2020-11-05T22:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 16958, - "high": 16958, - "low": 16958, - "open": 16958, - "volume": 33916 - }, - "id": 2761424, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-05T22:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 16958, - "high": 16958, - "low": 16958, - "open": 16958, - "volume": 50874 - }, - "id": 2761427, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 5997 - }, - "open": "2020-11-05T22:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 31966, - "high": 31966, - "low": 16958, - "open": 16958, - "volume": 59964 - }, - "id": 2761432, - "non_hive": { - "close": 3770, - "high": 3770, - "low": 1999, - "open": 1999, - "volume": 7071 - }, - "open": "2020-11-05T22:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 84268, - "high": 84268, - "low": 84268, - "open": 84268, - "volume": 84268 - }, - "id": 2761435, - "non_hive": { - "close": 9973, - "high": 9973, - "low": 9973, - "open": 9973, - "volume": 9973 - }, - "open": "2020-11-05T22:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 30432, - "high": 30432, - "low": 30432, - "open": 30432, - "volume": 30432 - }, - "id": 2761438, - "non_hive": { - "close": 3602, - "high": 3602, - "low": 3602, - "open": 3602, - "volume": 3602 - }, - "open": "2020-11-05T22:22:00", - "seconds": 60 - }, - { - "hive": { - "close": 32652, - "high": 32652, - "low": 32652, - "open": 32652, - "volume": 32652 - }, - "id": 2761441, - "non_hive": { - "close": 3864, - "high": 3864, - "low": 3864, - "open": 3864, - "volume": 3864 - }, - "open": "2020-11-05T22:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 253202, - "high": 253202, - "low": 253202, - "open": 253202, - "volume": 253202 - }, - "id": 2761443, - "non_hive": { - "close": 29969, - "high": 29969, - "low": 29969, - "open": 29969, - "volume": 29969 - }, - "open": "2020-11-05T22:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 382734, - "high": 25347, - "low": 382734, - "open": 25347, - "volume": 408081 - }, - "id": 2761446, - "non_hive": { - "close": 45296, - "high": 3000, - "low": 45296, - "open": 3000, - "volume": 48296 - }, - "open": "2020-11-05T22:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 441631, - "high": 441631, - "low": 441631, - "open": 441631, - "volume": 441631 - }, - "id": 2761449, - "non_hive": { - "close": 52266, - "high": 52266, - "low": 52266, - "open": 52266, - "volume": 52266 - }, - "open": "2020-11-05T22:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 20867, - "high": 20867, - "low": 20867, - "open": 20867, - "volume": 20867 - }, - "id": 2761452, - "non_hive": { - "close": 2470, - "high": 2470, - "low": 2470, - "open": 2470, - "volume": 2470 - }, - "open": "2020-11-05T22:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 4004, - "high": 4004, - "low": 4004, - "open": 4004, - "volume": 4004 - }, - "id": 2761455, - "non_hive": { - "close": 474, - "high": 474, - "low": 474, - "open": 474, - "volume": 474 - }, - "open": "2020-11-05T23:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 149865, - "high": 149865, - "low": 217002, - "open": 217002, - "volume": 1043809 - }, - "id": 2761459, - "non_hive": { - "close": 17804, - "high": 17804, - "low": 25685, - "open": 25685, - "volume": 123639 - }, - "open": "2020-11-05T23:03:00", - "seconds": 60 - }, - { - "hive": { - "close": 336, - "high": 336, - "low": 336, - "open": 336, - "volume": 336 - }, - "id": 2761461, - "non_hive": { - "close": 40, - "high": 40, - "low": 40, - "open": 40, - "volume": 40 - }, - "open": "2020-11-05T23:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 64225, - "high": 1979, - "low": 64225, - "open": 1979, - "volume": 83102 - }, - "id": 2761464, - "non_hive": { - "close": 7601, - "high": 235, - "low": 7601, - "open": 235, - "volume": 9836 - }, - "open": "2020-11-05T23:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 499819, - "high": 175181, - "low": 499819, - "open": 175181, - "volume": 675000 - }, - "id": 2761467, - "non_hive": { - "close": 58728, - "high": 20584, - "low": 58728, - "open": 20584, - "volume": 79312 - }, - "open": "2020-11-05T23:33:00", - "seconds": 60 - }, - { - "hive": { - "close": 17020, - "high": 17020, - "low": 17020, - "open": 17020, - "volume": 51060 - }, - "id": 2761469, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 6000 - }, - "open": "2020-11-05T23:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 17020, - "high": 17020, - "low": 17020, - "open": 17020, - "volume": 17020 - }, - "id": 2761473, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T23:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 16992, - "high": 16992, - "low": 17020, - "open": 17020, - "volume": 34012 - }, - "id": 2761476, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 4000 - }, - "open": "2020-11-05T23:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 16992, - "high": 16992, - "low": 16992, - "open": 16992, - "volume": 16992 - }, - "id": 2761479, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-05T23:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 15102, - "high": 2492, - "low": 15102, - "open": 2492, - "volume": 27288 - }, - "id": 2761481, - "non_hive": { - "close": 1767, - "high": 293, - "low": 1767, - "open": 293, - "volume": 3199 - }, - "open": "2020-11-05T23:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 245, - "high": 245, - "low": 72712, - "open": 72712, - "volume": 72957 - }, - "id": 2761484, - "non_hive": { - "close": 29, - "high": 29, - "low": 8507, - "open": 8507, - "volume": 8536 - }, - "open": "2020-11-05T23:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 7686, - "high": 14007, - "low": 7686, - "open": 14007, - "volume": 35834 - }, - "id": 2761486, - "non_hive": { - "close": 900, - "high": 1656, - "low": 900, - "open": 1656, - "volume": 4212 - }, - "open": "2020-11-05T23:45:00", - "seconds": 60 - }, - { - "hive": { - "close": 27076, - "high": 27076, - "low": 2902, - "open": 2902, - "volume": 37769 - }, - "id": 2761490, - "non_hive": { - "close": 3201, - "high": 3201, - "low": 343, - "open": 343, - "volume": 4465 - }, - "open": "2020-11-05T23:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 6829, - "high": 2580, - "low": 6829, - "open": 2580, - "volume": 12580 - }, - "id": 2761493, - "non_hive": { - "close": 800, - "high": 305, - "low": 800, - "open": 305, - "volume": 1477 - }, - "open": "2020-11-05T23:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 960262, - "high": 964, - "low": 960262, - "open": 964, - "volume": 961226 - }, - "id": 2761496, - "non_hive": { - "close": 112351, - "high": 113, - "low": 112351, - "open": 113, - "volume": 112464 - }, - "open": "2020-11-05T23:53:00", - "seconds": 60 - }, - { - "hive": { - "close": 15597, - "high": 15597, - "low": 15597, - "open": 15597, - "volume": 15597 - }, - "id": 2761498, - "non_hive": { - "close": 1844, - "high": 1844, - "low": 1844, - "open": 1844, - "volume": 1844 - }, - "open": "2020-11-05T23:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 507, - "high": 507, - "low": 507, - "open": 507, - "volume": 507 - }, - "id": 2761501, - "non_hive": { - "close": 60, - "high": 60, - "low": 60, - "open": 60, - "volume": 60 - }, - "open": "2020-11-05T23:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 1129101, - "high": 16271, - "low": 1129101, - "open": 16271, - "volume": 2000000 - }, - "id": 2761503, - "non_hive": { - "close": 132105, - "high": 1904, - "low": 132105, - "open": 1904, - "volume": 234009 - }, - "open": "2020-11-06T00:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 10953, - "high": 10953, - "low": 10953, - "open": 10953, - "volume": 10953 - }, - "id": 2761508, - "non_hive": { - "close": 1295, - "high": 1295, - "low": 1295, - "open": 1295, - "volume": 1295 - }, - "open": "2020-11-06T00:04:00", - "seconds": 60 - }, - { - "hive": { - "close": 1500000, - "high": 11067, - "low": 1500000, - "open": 11067, - "volume": 2905623 - }, - "id": 2761510, - "non_hive": { - "close": 175501, - "high": 1295, - "low": 175501, - "open": 1295, - "volume": 339960 - }, - "open": "2020-11-06T00:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 110000, - "high": 110000, - "low": 110000, - "open": 110000, - "volume": 110000 - }, - "id": 2761514, - "non_hive": { - "close": 12870, - "high": 12870, - "low": 12870, - "open": 12870, - "volume": 12870 - }, - "open": "2020-11-06T00:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 11481, - "high": 11481, - "low": 11481, - "open": 11481, - "volume": 11481 - }, - "id": 2761516, - "non_hive": { - "close": 1353, - "high": 1353, - "low": 1353, - "open": 1353, - "volume": 1353 - }, - "open": "2020-11-06T00:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 2994, - "high": 2994, - "low": 5483, - "open": 5483, - "volume": 8477 - }, - "id": 2761519, - "non_hive": { - "close": 353, - "high": 353, - "low": 646, - "open": 646, - "volume": 999 - }, - "open": "2020-11-06T00:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 267642, - "high": 76, - "low": 267642, - "open": 17084, - "volume": 284802 - }, - "id": 2761521, - "non_hive": { - "close": 31314, - "high": 9, - "low": 31314, - "open": 1999, - "volume": 33322 - }, - "open": "2020-11-06T00:17:00", - "seconds": 60 - }, - { - "hive": { - "close": 3767, - "high": 3767, - "low": 3767, - "open": 3767, - "volume": 3767 - }, - "id": 2761523, - "non_hive": { - "close": 444, - "high": 444, - "low": 444, - "open": 444, - "volume": 444 - }, - "open": "2020-11-06T00:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 132527, - "high": 132527, - "low": 17021, - "open": 17021, - "volume": 149548 - }, - "id": 2761526, - "non_hive": { - "close": 15572, - "high": 15572, - "low": 1999, - "open": 1999, - "volume": 17571 - }, - "open": "2020-11-06T00:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 323953, - "high": 3015, - "low": 323953, - "open": 3015, - "volume": 344060 - }, - "id": 2761529, - "non_hive": { - "close": 37906, - "high": 353, - "low": 37906, - "open": 353, - "volume": 40259 - }, - "open": "2020-11-06T00:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 124826, - "high": 3785, - "low": 124826, - "open": 3785, - "volume": 128611 - }, - "id": 2761532, - "non_hive": { - "close": 14604, - "high": 443, - "low": 14604, - "open": 443, - "volume": 15047 - }, - "open": "2020-11-06T00:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 17022, - "high": 17022, - "low": 17022, - "open": 17022, - "volume": 17022 - }, - "id": 2761534, - "non_hive": { - "close": 1991, - "high": 1991, - "low": 1991, - "open": 1991, - "volume": 1991 - }, - "open": "2020-11-06T00:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 33000, - "high": 33000, - "low": 33000, - "open": 33000, - "volume": 33000 - }, - "id": 2761537, - "non_hive": { - "close": 3861, - "high": 3861, - "low": 3861, - "open": 3861, - "volume": 3861 - }, - "open": "2020-11-06T00:55:00", - "seconds": 60 - }, - { - "hive": { - "close": 1584, - "high": 1584, - "low": 1584, - "open": 1584, - "volume": 1584 - }, - "id": 2761540, - "non_hive": { - "close": 186, - "high": 186, - "low": 186, - "open": 186, - "volume": 186 - }, - "open": "2020-11-06T01:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 16891, - "high": 16891, - "low": 16891, - "open": 16891, - "volume": 16891 - }, - "id": 2761544, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-06T01:13:00", - "seconds": 60 - }, - { - "hive": { - "close": 8227, - "high": 8227, - "low": 5971, - "open": 5971, - "volume": 14198 - }, - "id": 2761547, - "non_hive": { - "close": 977, - "high": 977, - "low": 707, - "open": 707, - "volume": 1684 - }, - "open": "2020-11-06T01:14:00", - "seconds": 60 - }, - { - "hive": { - "close": 1044, - "high": 1044, - "low": 1044, - "open": 1044, - "volume": 1044 - }, - "id": 2761549, - "non_hive": { - "close": 124, - "high": 124, - "low": 124, - "open": 124, - "volume": 124 - }, - "open": "2020-11-06T01:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 918, - "high": 918, - "low": 16837, - "open": 16837, - "volume": 17755 - }, - "id": 2761552, - "non_hive": { - "close": 109, - "high": 109, - "low": 1999, - "open": 1999, - "volume": 2108 - }, - "open": "2020-11-06T01:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 7657, - "high": 7657, - "low": 7657, - "open": 7657, - "volume": 7657 - }, - "id": 2761555, - "non_hive": { - "close": 909, - "high": 909, - "low": 909, - "open": 909, - "volume": 909 - }, - "open": "2020-11-06T01:37:00", - "seconds": 60 - }, - { - "hive": { - "close": 8415, - "high": 8415, - "low": 8415, - "open": 8415, - "volume": 8415 - }, - "id": 2761558, - "non_hive": { - "close": 999, - "high": 999, - "low": 999, - "open": 999, - "volume": 999 - }, - "open": "2020-11-06T01:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 16806, - "high": 16806, - "low": 16806, - "open": 16806, - "volume": 16806 - }, - "id": 2761560, - "non_hive": { - "close": 1995, - "high": 1995, - "low": 1995, - "open": 1995, - "volume": 1995 - }, - "open": "2020-11-06T01:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 1382746, - "high": 17010, - "low": 1382746, - "open": 17010, - "volume": 1427743 - }, - "id": 2761563, - "non_hive": { - "close": 161782, - "high": 2000, - "low": 161782, - "open": 2000, - "volume": 167070 - }, - "open": "2020-11-06T01:44:00", - "seconds": 60 - }, - { - "hive": { - "close": 4698, - "high": 4698, - "low": 4698, - "open": 4698, - "volume": 4698 - }, - "id": 2761565, - "non_hive": { - "close": 556, - "high": 556, - "low": 556, - "open": 556, - "volume": 556 - }, - "open": "2020-11-06T01:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 150994, - "high": 665313, - "low": 12193, - "open": 12193, - "volume": 1009757 - }, - "id": 2761568, - "non_hive": { - "close": 17936, - "high": 79030, - "low": 1443, - "open": 1443, - "volume": 119937 - }, - "open": "2020-11-06T02:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 2188343, - "high": 2188343, - "low": 435231, - "open": 435231, - "volume": 2623574 - }, - "id": 2761573, - "non_hive": { - "close": 259975, - "high": 259975, - "low": 51700, - "open": 51700, - "volume": 311675 - }, - "open": "2020-11-06T02:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 2421718, - "high": 2421718, - "low": 2421718, - "open": 2421718, - "volume": 2421718 - }, - "id": 2761576, - "non_hive": { - "close": 287700, - "high": 287700, - "low": 287700, - "open": 287700, - "volume": 287700 - }, - "open": "2020-11-06T02:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 819546, - "high": 819546, - "low": 1992688, - "open": 1992688, - "volume": 2812234 - }, - "id": 2761578, - "non_hive": { - "close": 97444, - "high": 97444, - "low": 236731, - "open": 236731, - "volume": 334175 - }, - "open": "2020-11-06T02:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 1054483, - "high": 1054483, - "low": 1054483, - "open": 1054483, - "volume": 1054483 - }, - "id": 2761581, - "non_hive": { - "close": 125378, - "high": 125378, - "low": 125378, - "open": 125378, - "volume": 125378 - }, - "open": "2020-11-06T02:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 1614096, - "high": 1614096, - "low": 1614096, - "open": 1614096, - "volume": 1614096 - }, - "id": 2761583, - "non_hive": { - "close": 191916, - "high": 191916, - "low": 191916, - "open": 191916, - "volume": 191916 - }, - "open": "2020-11-06T02:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 1202431, - "high": 1202431, - "low": 1202431, - "open": 1202431, - "volume": 1202431 - }, - "id": 2761586, - "non_hive": { - "close": 142969, - "high": 142969, - "low": 142969, - "open": 142969, - "volume": 142969 - }, - "open": "2020-11-06T02:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 11278, - "high": 11278, - "low": 11278, - "open": 11278, - "volume": 11278 - }, - "id": 2761589, - "non_hive": { - "close": 1341, - "high": 1341, - "low": 1341, - "open": 1341, - "volume": 1341 - }, - "open": "2020-11-06T02:32:00", - "seconds": 60 - }, - { - "hive": { - "close": 20227, - "high": 20227, - "low": 20227, - "open": 20227, - "volume": 20227 - }, - "id": 2761592, - "non_hive": { - "close": 2405, - "high": 2405, - "low": 2405, - "open": 2405, - "volume": 2405 - }, - "open": "2020-11-06T02:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 3411, - "high": 40875, - "low": 3411, - "open": 40875, - "volume": 44286 - }, - "id": 2761595, - "non_hive": { - "close": 405, - "high": 4860, - "low": 405, - "open": 4860, - "volume": 5265 - }, - "open": "2020-11-06T02:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 1055208, - "high": 1055208, - "low": 16821, - "open": 16821, - "volume": 3173848 - }, - "id": 2761598, - "non_hive": { - "close": 125939, - "high": 125939, - "low": 1999, - "open": 1999, - "volume": 377950 - }, - "open": "2020-11-06T02:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 1191, - "high": 1191, - "low": 1191, - "open": 1191, - "volume": 1191 - }, - "id": 2761601, - "non_hive": { - "close": 142, - "high": 142, - "low": 142, - "open": 142, - "volume": 142 - }, - "open": "2020-11-06T03:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 10000, - "high": 10000, - "low": 10000, - "open": 10000, - "volume": 10000 - }, - "id": 2761605, - "non_hive": { - "close": 1180, - "high": 1180, - "low": 1180, - "open": 1180, - "volume": 1180 - }, - "open": "2020-11-06T03:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 6001, - "high": 6001, - "low": 6001, - "open": 6001, - "volume": 6001 - }, - "id": 2761607, - "non_hive": { - "close": 716, - "high": 716, - "low": 716, - "open": 716, - "volume": 716 - }, - "open": "2020-11-06T03:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 265, - "high": 265, - "low": 265, - "open": 265, - "volume": 265 - }, - "id": 2761610, - "non_hive": { - "close": 31, - "high": 31, - "low": 31, - "open": 31, - "volume": 31 - }, - "open": "2020-11-06T03:38:00", - "seconds": 60 - }, - { - "hive": { - "close": 10755, - "high": 10755, - "low": 10755, - "open": 10755, - "volume": 10755 - }, - "id": 2761612, - "non_hive": { - "close": 1283, - "high": 1283, - "low": 1283, - "open": 1283, - "volume": 1283 - }, - "open": "2020-11-06T03:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 2228, - "high": 2228, - "low": 2228, - "open": 2228, - "volume": 2228 - }, - "id": 2761615, - "non_hive": { - "close": 266, - "high": 266, - "low": 266, - "open": 266, - "volume": 266 - }, - "open": "2020-11-06T04:29:00", - "seconds": 60 - }, - { - "hive": { - "close": 9994, - "high": 9994, - "low": 9994, - "open": 9994, - "volume": 9994 - }, - "id": 2761619, - "non_hive": { - "close": 1192, - "high": 1192, - "low": 1192, - "open": 1192, - "volume": 1192 - }, - "open": "2020-11-06T04:43:00", - "seconds": 60 - }, - { - "hive": { - "close": 88024, - "high": 88024, - "low": 3645, - "open": 3645, - "volume": 91669 - }, - "id": 2761622, - "non_hive": { - "close": 10554, - "high": 10554, - "low": 435, - "open": 435, - "volume": 10989 - }, - "open": "2020-11-06T04:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 2645, - "high": 2645, - "low": 2645, - "open": 2645, - "volume": 2645 - }, - "id": 2761625, - "non_hive": { - "close": 317, - "high": 317, - "low": 317, - "open": 317, - "volume": 317 - }, - "open": "2020-11-06T04:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 14036, - "high": 14036, - "low": 14036, - "open": 14036, - "volume": 14036 - }, - "id": 2761627, - "non_hive": { - "close": 1682, - "high": 1682, - "low": 1682, - "open": 1682, - "volume": 1682 - }, - "open": "2020-11-06T04:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 1612, - "high": 1612, - "low": 1612, - "open": 1612, - "volume": 1612 - }, - "id": 2761630, - "non_hive": { - "close": 193, - "high": 193, - "low": 193, - "open": 193, - "volume": 193 - }, - "open": "2020-11-06T04:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 425, - "high": 425, - "low": 425, - "open": 425, - "volume": 425 - }, - "id": 2761632, - "non_hive": { - "close": 51, - "high": 51, - "low": 51, - "open": 51, - "volume": 51 - }, - "open": "2020-11-06T04:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 8179, - "high": 8179, - "low": 8179, - "open": 8179, - "volume": 8179 - }, - "id": 2761635, - "non_hive": { - "close": 980, - "high": 980, - "low": 980, - "open": 980, - "volume": 980 - }, - "open": "2020-11-06T05:02:00", - "seconds": 60 - }, - { - "hive": { - "close": 44894, - "high": 44894, - "low": 44894, - "open": 44894, - "volume": 44894 - }, - "id": 2761639, - "non_hive": { - "close": 5380, - "high": 5380, - "low": 5380, - "open": 5380, - "volume": 5380 - }, - "open": "2020-11-06T05:07:00", - "seconds": 60 - }, - { - "hive": { - "close": 83237, - "high": 16763, - "low": 83237, - "open": 16763, - "volume": 100000 - }, - "id": 2761642, - "non_hive": { - "close": 9930, - "high": 2000, - "low": 9930, - "open": 2000, - "volume": 11930 - }, - "open": "2020-11-06T05:10:00", - "seconds": 60 - }, - { - "hive": { - "close": 16763, - "high": 16763, - "low": 16763, - "open": 16763, - "volume": 16763 - }, - "id": 2761645, - "non_hive": { - "close": 2000, - "high": 2000, - "low": 2000, - "open": 2000, - "volume": 2000 - }, - "open": "2020-11-06T05:15:00", - "seconds": 60 - }, - { - "hive": { - "close": 64651, - "high": 64651, - "low": 64651, - "open": 64651, - "volume": 64651 - }, - "id": 2761648, - "non_hive": { - "close": 7713, - "high": 7713, - "low": 7713, - "open": 7713, - "volume": 7713 - }, - "open": "2020-11-06T05:16:00", - "seconds": 60 - }, - { - "hive": { - "close": 1701, - "high": 16886, - "low": 1701, - "open": 16886, - "volume": 18587 - }, - "id": 2761650, - "non_hive": { - "close": 201, - "high": 2000, - "low": 201, - "open": 2000, - "volume": 2201 - }, - "open": "2020-11-06T05:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 16312, - "high": 16312, - "low": 16312, - "open": 16312, - "volume": 16312 - }, - "id": 2761652, - "non_hive": { - "close": 1945, - "high": 1945, - "low": 1945, - "open": 1945, - "volume": 1945 - }, - "open": "2020-11-06T05:46:00", - "seconds": 60 - }, - { - "hive": { - "close": 4221, - "high": 4221, - "low": 16886, - "open": 16886, - "volume": 21107 - }, - "id": 2761655, - "non_hive": { - "close": 500, - "high": 500, - "low": 2000, - "open": 2000, - "volume": 2500 - }, - "open": "2020-11-06T05:50:00", - "seconds": 60 - }, - { - "hive": { - "close": 158993, - "high": 158993, - "low": 3765, - "open": 1802, - "volume": 958483 - }, - "id": 2761658, - "non_hive": { - "close": 18952, - "high": 18952, - "low": 445, - "open": 213, - "volume": 114211 - }, - "open": "2020-11-06T06:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 11255, - "high": 11255, - "low": 11255, - "open": 11255, - "volume": 11255 - }, - "id": 2761663, - "non_hive": { - "close": 1341, - "high": 1341, - "low": 1341, - "open": 1341, - "volume": 1341 - }, - "open": "2020-11-06T06:21:00", - "seconds": 60 - }, - { - "hive": { - "close": 10201, - "high": 10201, - "low": 5524, - "open": 5524, - "volume": 15725 - }, - "id": 2761666, - "non_hive": { - "close": 1216, - "high": 1216, - "low": 658, - "open": 658, - "volume": 1874 - }, - "open": "2020-11-06T06:24:00", - "seconds": 60 - }, - { - "hive": { - "close": 208994, - "high": 5151, - "low": 208994, - "open": 5151, - "volume": 214145 - }, - "id": 2761669, - "non_hive": { - "close": 24912, - "high": 614, - "low": 24912, - "open": 614, - "volume": 25526 - }, - "open": "2020-11-06T06:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 402, - "high": 208994, - "low": 402, - "open": 208994, - "volume": 211443 - }, - "id": 2761673, - "non_hive": { - "close": 47, - "high": 24912, - "low": 47, - "open": 24912, - "volume": 25203 - }, - "open": "2020-11-06T06:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 4874, - "high": 4874, - "low": 150998, - "open": 150998, - "volume": 155872 - }, - "id": 2761677, - "non_hive": { - "close": 581, - "high": 581, - "low": 17999, - "open": 17999, - "volume": 18580 - }, - "open": "2020-11-06T06:56:00", - "seconds": 60 - }, - { - "hive": { - "close": 980, - "high": 13775, - "low": 980, - "open": 13775, - "volume": 14755 - }, - "id": 2761681, - "non_hive": { - "close": 116, - "high": 1642, - "low": 116, - "open": 1642, - "volume": 1758 - }, - "open": "2020-11-06T06:59:00", - "seconds": 60 - }, - { - "hive": { - "close": 91996, - "high": 91996, - "low": 91996, - "open": 91996, - "volume": 91996 - }, - "id": 2761684, - "non_hive": { - "close": 10966, - "high": 10966, - "low": 10966, - "open": 10966, - "volume": 10966 - }, - "open": "2020-11-06T07:00:00", - "seconds": 60 - }, - { - "hive": { - "close": 1459, - "high": 1459, - "low": 1459, - "open": 1459, - "volume": 1459 - }, - "id": 2761688, - "non_hive": { - "close": 174, - "high": 174, - "low": 174, - "open": 174, - "volume": 174 - }, - "open": "2020-11-06T07:01:00", - "seconds": 60 - }, - { - "hive": { - "close": 2626, - "high": 2357, - "low": 2249, - "open": 2357, - "volume": 7232 - }, - "id": 2761690, - "non_hive": { - "close": 313, - "high": 281, - "low": 268, - "open": 281, - "volume": 862 - }, - "open": "2020-11-06T07:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 16779, - "high": 16779, - "low": 16779, - "open": 16779, - "volume": 16779 - }, - "id": 2761693, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 1999 - }, - "open": "2020-11-06T07:08:00", - "seconds": 60 - }, - { - "hive": { - "close": 38, - "high": 84297, - "low": 38, - "open": 84297, - "volume": 84335 - }, - "id": 2761695, - "non_hive": { - "close": 4, - "high": 10043, - "low": 4, - "open": 10043, - "volume": 10047 - }, - "open": "2020-11-06T07:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 16807, - "high": 16807, - "low": 16807, - "open": 16807, - "volume": 33614 - }, - "id": 2761698, - "non_hive": { - "close": 1999, - "high": 1999, - "low": 1999, - "open": 1999, - "volume": 3998 - }, - "open": "2020-11-06T07:11:00", - "seconds": 60 - }, - { - "hive": { - "close": 14493, - "high": 14493, - "low": 14493, - "open": 14493, - "volume": 14493 - }, - "id": 2761702, - "non_hive": { - "close": 1724, - "high": 1724, - "low": 1724, - "open": 1724, - "volume": 1724 - }, - "open": "2020-11-06T07:12:00", - "seconds": 60 - }, - { - "hive": { - "close": 5926, - "high": 5926, - "low": 5926, - "open": 5926, - "volume": 5926 - }, - "id": 2761704, - "non_hive": { - "close": 705, - "high": 705, - "low": 705, - "open": 705, - "volume": 705 - }, - "open": "2020-11-06T07:18:00", - "seconds": 60 - }, - { - "hive": { - "close": 18464, - "high": 18464, - "low": 18464, - "open": 18464, - "volume": 18464 - }, - "id": 2761707, - "non_hive": { - "close": 2201, - "high": 2201, - "low": 2201, - "open": 2201, - "volume": 2201 - }, - "open": "2020-11-06T07:41:00", - "seconds": 60 - }, - { - "hive": { - "close": 1704, - "high": 1704, - "low": 1704, - "open": 1704, - "volume": 1704 - }, - "id": 2761710, - "non_hive": { - "close": 203, - "high": 203, - "low": 203, - "open": 203, - "volume": 203 - }, - "open": "2020-11-06T07:42:00", - "seconds": 60 - }, - { - "hive": { - "close": 1166, - "high": 1166, - "low": 1166, - "open": 1166, - "volume": 1166 - }, - "id": 2761712, - "non_hive": { - "close": 139, - "high": 139, - "low": 139, - "open": 139, - "volume": 139 - }, - "open": "2020-11-06T07:54:00", - "seconds": 60 - }, - { - "hive": { - "close": 463201, - "high": 463201, - "low": 463201, - "open": 463201, - "volume": 463201 - }, - "id": 2761715, - "non_hive": { - "close": 55181, - "high": 55181, - "low": 55181, - "open": 55181, - "volume": 55181 - }, - "open": "2020-11-06T07:58:00", - "seconds": 60 - }, - { - "hive": { - "close": 1107, - "high": 1107, - "low": 1107, - "open": 1107, - "volume": 1107 - }, - "id": 2761718, - "non_hive": { - "close": 132, - "high": 132, - "low": 132, - "open": 132, - "volume": 132 - }, - "open": "2020-11-06T08:06:00", - "seconds": 60 - }, - { - "hive": { - "close": 1000000, - "high": 1000000, - "low": 1000000, - "open": 1000000, - "volume": 1000000 - }, - "id": 2761722, - "non_hive": { - "close": 119130, - "high": 119130, - "low": 119130, - "open": 119130, - "volume": 119130 - }, - "open": "2020-11-06T08:09:00", - "seconds": 60 - }, - { - "hive": { - "close": 4289, - "high": 4289, - "low": 4289, - "open": 4289, - "volume": 4289 - }, - "id": 2761724, - "non_hive": { - "close": 511, - "high": 511, - "low": 511, - "open": 511, - "volume": 511 - }, - "open": "2020-11-06T08:19:00", - "seconds": 60 - }, - { - "hive": { - "close": 8271, - "high": 8271, - "low": 8271, - "open": 8271, - "volume": 8271 - }, - "id": 2761727, - "non_hive": { - "close": 986, - "high": 986, - "low": 986, - "open": 986, - "volume": 986 - }, - "open": "2020-11-06T08:20:00", - "seconds": 60 - }, - { - "hive": { - "close": 33559, - "high": 11485, - "low": 33559, - "open": 11485, - "volume": 47872 - }, - "id": 2761730, - "non_hive": { - "close": 3997, - "high": 1369, - "low": 3997, - "open": 1369, - "volume": 5703 - }, - "open": "2020-11-06T08:21:00", - "seconds": 60 - }, - { - "hive": { - "close": 31061, - "high": 31061, - "low": 31061, - "open": 31061, - "volume": 31061 - }, - "id": 2761732, - "non_hive": { - "close": 3700, - "high": 3700, - "low": 3700, - "open": 3700, - "volume": 3700 - }, - "open": "2020-11-06T08:23:00", - "seconds": 60 - }, - { - "hive": { - "close": 8624, - "high": 8624, - "low": 8624, - "open": 8624, - "volume": 8624 - }, - "id": 2761734, - "non_hive": { - "close": 1028, - "high": 1028, - "low": 1028, - "open": 1028, - "volume": 1028 - }, - "open": "2020-11-06T08:28:00", - "seconds": 60 - }, - { - "hive": { - "close": 4437, - "high": 4437, - "low": 4437, - "open": 4437, - "volume": 4437 - }, - "id": 2761737, - "non_hive": { - "close": 529, - "high": 529, - "low": 529, - "open": 529, - "volume": 529 - }, - "open": "2020-11-06T08:35:00", - "seconds": 60 - }, - { - "hive": { - "close": 18983, - "high": 11024, - "low": 18983, - "open": 11024, - "volume": 44570 - }, - "id": 2761740, - "non_hive": { - "close": 2239, - "high": 1314, - "low": 2239, - "open": 1314, - "volume": 5288 - }, - "open": "2020-11-06T08:36:00", - "seconds": 60 - }, - { - "hive": { - "close": 62919, - "high": 62919, - "low": 62919, - "open": 62919, - "volume": 62919 - }, - "id": 2761742, - "non_hive": { - "close": 7500, - "high": 7500, - "low": 7500, - "open": 7500, - "volume": 7500 - }, - "open": "2020-11-06T08:40:00", - "seconds": 60 - }, - { - "hive": { - "close": 18983, - "high": 18983, - "low": 18983, - "open": 18983, - "volume": 18983 - }, - "id": 2761745, - "non_hive": { - "close": 2261, - "high": 2261, - "low": 2261, - "open": 2261, - "volume": 2261 - }, - "open": "2020-11-06T08:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 318, - "high": 318, - "low": 318, - "open": 318, - "volume": 318 - }, - "id": 2761748, - "non_hive": { - "close": 38, - "high": 38, - "low": 38, - "open": 38, - "volume": 38 - }, - "open": "2020-11-06T08:51:00", - "seconds": 60 - }, - { - "hive": { - "close": 4974, - "high": 4974, - "low": 4974, - "open": 4974, - "volume": 4974 - }, - "id": 2761751, - "non_hive": { - "close": 593, - "high": 593, - "low": 593, - "open": 593, - "volume": 593 - }, - "open": "2020-11-06T09:05:00", - "seconds": 60 - }, - { - "hive": { - "close": 125839, - "high": 125839, - "low": 125839, - "open": 125839, - "volume": 125839 - }, - "id": 2761755, - "non_hive": { - "close": 15000, - "high": 15000, - "low": 15000, - "open": 15000, - "volume": 15000 - }, - "open": "2020-11-06T09:13:00", - "seconds": 60 - }, - { - "hive": { - "close": 19857, - "high": 19857, - "low": 19857, - "open": 19857, - "volume": 19857 - }, - "id": 2761758, - "non_hive": { - "close": 2367, - "high": 2367, - "low": 2367, - "open": 2367, - "volume": 2367 - }, - "open": "2020-11-06T09:26:00", - "seconds": 60 - }, - { - "hive": { - "close": 4289, - "high": 4289, - "low": 4289, - "open": 4289, - "volume": 4289 - }, - "id": 2761761, - "non_hive": { - "close": 511, - "high": 511, - "low": 511, - "open": 511, - "volume": 511 - }, - "open": "2020-11-06T09:27:00", - "seconds": 60 - }, - { - "hive": { - "close": 2365, - "high": 2365, - "low": 2365, - "open": 2365, - "volume": 2365 - }, - "id": 2761763, - "non_hive": { - "close": 282, - "high": 282, - "low": 282, - "open": 282, - "volume": 282 - }, - "open": "2020-11-06T09:31:00", - "seconds": 60 - }, - { - "hive": { - "close": 2810, - "high": 2810, - "low": 2810, - "open": 2810, - "volume": 2810 - }, - "id": 2761766, - "non_hive": { - "close": 335, - "high": 335, - "low": 335, - "open": 335, - "volume": 335 - }, - "open": "2020-11-06T09:52:00", - "seconds": 60 - }, - { - "hive": { - "close": 7902, - "high": 7902, - "low": 7902, - "open": 7902, - "volume": 7902 - }, - "id": 2761769, - "non_hive": { - "close": 942, - "high": 942, - "low": 942, - "open": 942, - "volume": 942 - }, - "open": "2020-11-06T10:25:00", - "seconds": 60 - }, - { - "hive": { - "close": 4250, - "high": 4250, - "low": 4250, - "open": 4250, - "volume": 4250 - }, - "id": 2761773, - "non_hive": { - "close": 506, - "high": 506, - "low": 506, - "open": 506, - "volume": 506 - }, - "open": "2020-11-06T10:34:00", - "seconds": 60 - }, - { - "hive": { - "close": 64001, - "high": 64001, - "low": 64001, - "open": 64001, - "volume": 64001 - }, - "id": 2761776, - "non_hive": { - "close": 7629, - "high": 7629, - "low": 7629, - "open": 7629, - "volume": 7629 - }, - "open": "2020-11-06T10:47:00", - "seconds": 60 - }, - { - "hive": { - "close": 218, - "high": 218, - "low": 218, - "open": 218, - "volume": 218 - }, - "id": 2761779, - "non_hive": { - "close": 26, - "high": 26, - "low": 26, - "open": 26, - "volume": 26 - }, - "open": "2020-11-06T10:49:00", - "seconds": 60 - }, - { - "hive": { - "close": 485620, - "high": 485620, - "low": 485620, - "open": 485620, - "volume": 485620 - }, - "id": 2761781, - "non_hive": { - "close": 57885, - "high": 57885, - "low": 57885, - "open": 57885, - "volume": 57885 - }, - "open": "2020-11-06T10:57:00", - "seconds": 60 - }, - { - "hive": { - "close": 1000000, - "high": 1000000, - "low": 5881, - "open": 5881, - "volume": 1135074 - }, - "id": 2761784, - "non_hive": { - "close": 119924, - "high": 119924, - "low": 702, - "open": 702, - "volume": 136116 - }, - "open": "2020-11-06T10:58:00", - "seconds": 60 - } -] diff --git a/hived/tavern/condenser_api_patterns/get_market_history/60.tavern.yaml b/hived/tavern/condenser_api_patterns/get_market_history/60.tavern.yaml deleted file mode 100644 index 9b51c017c0014dcaa2c310eea43406c6aef2b232..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history/60.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_market_history - - marks: - - patterntest #this works only for new data from four days before now - - includes: - - !include ../../common.yaml - - stages: - - name: get_market_history - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_market_history" - params: [60,"2016-04-03T00:00:00","2031-11-01T00:00:00"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "60" - directory: "condenser_api_patterns/get_market_history" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_market_history/86400.pat.json b/hived/tavern/condenser_api_patterns/get_market_history/86400.pat.json deleted file mode 100644 index 495d0059dfade572331d003b731947c0490ca5db..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history/86400.pat.json +++ /dev/null @@ -1,4581 +0,0 @@ -[ - { - "hive": { - "close": 638967, - "high": 1, - "low": 751, - "open": 33000, - "volume": 633560581 - }, - "id": 4, - "non_hive": { - "close": 200000, - "high": 1, - "low": 99, - "open": 6105, - "volume": 190179911 - }, - "open": "2016-07-04T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1000000, - "high": 6, - "low": 30957, - "open": 638967, - "volume": 472846288 - }, - "id": 2187, - "non_hive": { - "close": 296999, - "high": 2, - "low": 8513, - "open": 200000, - "volume": 139311448 - }, - "open": "2016-07-05T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1490, - "high": 3, - "low": 1489516, - "open": 10000, - "volume": 557689494 - }, - "id": 3232, - "non_hive": { - "close": 401, - "high": 1, - "low": 388764, - "open": 2970, - "volume": 152389270 - }, - "open": "2016-07-06T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1248498, - "high": 2, - "low": 4, - "open": 1271000, - "volume": 578758827 - }, - "id": 4120, - "non_hive": { - "close": 412003, - "high": 1, - "low": 1, - "open": 341898, - "volume": 175109781 - }, - "open": "2016-07-07T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 2997, - "high": 2, - "low": 537068, - "open": 1000000, - "volume": 2251234264 - }, - "id": 4835, - "non_hive": { - "close": 1004, - "high": 1, - "low": 155749, - "open": 301000, - "volume": 718887099 - }, - "open": "2016-07-08T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 10000000, - "high": 13235, - "low": 1640149, - "open": 37794, - "volume": 2189640825 - }, - "id": 5883, - "non_hive": { - "close": 6108000, - "high": 9000, - "low": 543377, - "open": 12661, - "volume": 839764094 - }, - "open": "2016-07-09T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 9681699, - "high": 1, - "low": 236504, - "open": 200000, - "volume": 1805284141 - }, - "id": 6878, - "non_hive": { - "close": 6766171, - "high": 1, - "low": 82776, - "open": 122180, - "volume": 1183573803 - }, - "open": "2016-07-10T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 26401, - "high": 1, - "low": 13333160, - "open": 100000, - "volume": 1325679741 - }, - "id": 7644, - "non_hive": { - "close": 25081, - "high": 1, - "low": 5999924, - "open": 56000, - "volume": 959491012 - }, - "open": "2016-07-11T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4970826, - "high": 4970826, - "low": 17997, - "open": 100000, - "volume": 1349632620 - }, - "id": 8370, - "non_hive": { - "close": 11830566, - "high": 11830566, - "low": 16557, - "open": 95500, - "volume": 1952715444 - }, - "open": "2016-07-12T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 8924644, - "high": 3, - "low": 153145, - "open": 2119, - "volume": 2080098858 - }, - "id": 9321, - "non_hive": { - "close": 26327701, - "high": 11, - "low": 76572, - "open": 5000, - "volume": "5954589735" - }, - "open": "2016-07-13T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 74981, - "high": 23, - "low": 1, - "open": 12015784, - "volume": "5373349596" - }, - "id": 10917, - "non_hive": { - "close": 277056, - "high": 100, - "low": 1, - "open": 35446563, - "volume": "17121076896" - }, - "open": "2016-07-14T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 500000, - "high": 2, - "low": 1, - "open": 184, - "volume": 500190948 - }, - "id": 13328, - "non_hive": { - "close": 1577500, - "high": 9, - "low": 2, - "open": 580, - "volume": 1728074095 - }, - "open": "2016-07-15T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1000, - "high": 8, - "low": 251232, - "open": 10000, - "volume": 232810303 - }, - "id": 14214, - "non_hive": { - "close": 3008, - "high": 30, - "low": 678326, - "open": 32168, - "volume": 754555214 - }, - "open": "2016-07-16T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 224605, - "high": 2, - "low": 1, - "open": 10000, - "volume": 138790218 - }, - "id": 14673, - "non_hive": { - "close": 696275, - "high": 8, - "low": 2, - "open": 28600, - "volume": 404890309 - }, - "open": "2016-07-17T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 225395, - "high": 8, - "low": 1, - "open": 10000, - "volume": 257620866 - }, - "id": 15421, - "non_hive": { - "close": 635569, - "high": 29, - "low": 2, - "open": 32000, - "volume": 799340145 - }, - "open": "2016-07-18T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 250964, - "high": 21, - "low": 1, - "open": 3546, - "volume": 295678919 - }, - "id": 16722, - "non_hive": { - "close": 780500, - "high": 76, - "low": 2, - "open": 10000, - "volume": 913055011 - }, - "open": "2016-07-19T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 39624, - "high": 2, - "low": 1, - "open": 95109, - "volume": 319437282 - }, - "id": 18375, - "non_hive": { - "close": 113943, - "high": 8, - "low": 2, - "open": 283236, - "volume": 957290486 - }, - "open": "2016-07-20T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1000, - "high": 3, - "low": 1, - "open": 9, - "volume": 675189108 - }, - "id": 20101, - "non_hive": { - "close": 2694, - "high": 10, - "low": 2, - "open": 28, - "volume": 1882621722 - }, - "open": "2016-07-21T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 6970, - "high": 3, - "low": 1, - "open": 13641, - "volume": 739116548 - }, - "id": 21765, - "non_hive": { - "close": 19698, - "high": 11, - "low": 2, - "open": 36745, - "volume": 2075810928 - }, - "open": "2016-07-22T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 40520, - "high": 1, - "low": 1, - "open": 2834, - "volume": 936388404 - }, - "id": 23208, - "non_hive": { - "close": 137101, - "high": 4, - "low": 2, - "open": 8009, - "volume": 2839224482 - }, - "open": "2016-07-23T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 2013, - "high": 2, - "low": 1, - "open": 2704, - "volume": 725390050 - }, - "id": 24639, - "non_hive": { - "close": 7313, - "high": 10, - "low": 3, - "open": 9150, - "volume": 2489143277 - }, - "open": "2016-07-24T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1512, - "high": 4, - "low": 1, - "open": 19058, - "volume": 1578916695 - }, - "id": 26075, - "non_hive": { - "close": 5244, - "high": 18, - "low": 3, - "open": 69100, - "volume": "5670629301" - }, - "open": "2016-07-25T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 86, - "high": 2, - "low": 10000, - "open": 34910, - "volume": 639846146 - }, - "id": 27279, - "non_hive": { - "close": 281, - "high": 10, - "low": 30000, - "open": 121071, - "volume": 2215485605 - }, - "open": "2016-07-26T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 100, - "high": 1, - "low": 100000, - "open": 1827, - "volume": 25957986 - }, - "id": 28394, - "non_hive": { - "close": 326, - "high": 6, - "low": 250000, - "open": 5964, - "volume": 82360531 - }, - "open": "2016-07-27T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 555, - "high": 3, - "low": 1, - "open": 2103, - "volume": 16666443 - }, - "id": 29927, - "non_hive": { - "close": 1666, - "high": 12, - "low": 2, - "open": 6842, - "volume": 49433794 - }, - "open": "2016-07-28T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 57471, - "high": 2, - "low": 1, - "open": 39620, - "volume": 37595761 - }, - "id": 30822, - "non_hive": { - "close": 133332, - "high": 8, - "low": 2, - "open": 118861, - "volume": 90670715 - }, - "open": "2016-07-29T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 348, - "high": 5, - "low": 1, - "open": 10827, - "volume": 8442124 - }, - "id": 31908, - "non_hive": { - "close": 1013, - "high": 17, - "low": 2, - "open": 25120, - "volume": 21888811 - }, - "open": "2016-07-30T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 24642, - "high": 3, - "low": 1, - "open": 53, - "volume": 5647139 - }, - "id": 32598, - "non_hive": { - "close": 71675, - "high": 11, - "low": 2, - "open": 156, - "volume": 16617843 - }, - "open": "2016-07-31T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 56502, - "high": 3, - "low": 1, - "open": 46221, - "volume": 10291597 - }, - "id": 33214, - "non_hive": { - "close": 159788, - "high": 10, - "low": 2, - "open": 134481, - "volume": 29371173 - }, - "open": "2016-08-01T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4800, - "high": 6, - "low": 1, - "open": 83, - "volume": 24795240 - }, - "id": 33970, - "non_hive": { - "close": 11378, - "high": 19, - "low": 2, - "open": 235, - "volume": 65236050 - }, - "open": "2016-08-02T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1765, - "high": 1, - "low": 1, - "open": 149805, - "volume": 11732862 - }, - "id": 34845, - "non_hive": { - "close": 4414, - "high": 3, - "low": 2, - "open": 371516, - "volume": 28660731 - }, - "open": "2016-08-03T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 22045, - "high": 3, - "low": 1, - "open": 1631, - "volume": 23662783 - }, - "id": 35596, - "non_hive": { - "close": 49601, - "high": 9, - "low": 2, - "open": 3935, - "volume": 54783225 - }, - "open": "2016-08-04T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 81894, - "high": 4, - "low": 1, - "open": 46, - "volume": 8258457 - }, - "id": 36467, - "non_hive": { - "close": 187553, - "high": 11, - "low": 2, - "open": 103, - "volume": 19016076 - }, - "open": "2016-08-05T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 577, - "high": 1, - "low": 1, - "open": 2261, - "volume": 12488457 - }, - "id": 37101, - "non_hive": { - "close": 1320, - "high": 3, - "low": 2, - "open": 5200, - "volume": 28282259 - }, - "open": "2016-08-06T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 98, - "high": 1, - "low": 1, - "open": 2541, - "volume": 15585071 - }, - "id": 37666, - "non_hive": { - "close": 230, - "high": 3, - "low": 2, - "open": 5807, - "volume": 35505922 - }, - "open": "2016-08-07T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 45471, - "high": 1, - "low": 1, - "open": 1474, - "volume": 22428227 - }, - "id": 38251, - "non_hive": { - "close": 98674, - "high": 3, - "low": 2, - "open": 3597, - "volume": 49419291 - }, - "open": "2016-08-08T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 415, - "high": 1, - "low": 1, - "open": 467, - "volume": 13863754 - }, - "id": 39228, - "non_hive": { - "close": 792, - "high": 3, - "low": 1, - "open": 1008, - "volume": 28915901 - }, - "open": "2016-08-09T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 84496, - "high": 3, - "low": 2, - "open": 176, - "volume": 4875427 - }, - "id": 39909, - "non_hive": { - "close": 158008, - "high": 8, - "low": 3, - "open": 370, - "volume": 9609697 - }, - "open": "2016-08-10T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 9838, - "high": 18, - "low": 1, - "open": 112, - "volume": 46934318 - }, - "id": 40280, - "non_hive": { - "close": 15250, - "high": 37, - "low": 1, - "open": 211, - "volume": 76602065 - }, - "open": "2016-08-11T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 2287, - "high": 1, - "low": 1, - "open": 162091, - "volume": 26595888 - }, - "id": 41249, - "non_hive": { - "close": 3545, - "high": 2, - "low": 1, - "open": 251242, - "volume": 41356980 - }, - "open": "2016-08-12T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 55865, - "high": 1, - "low": 1, - "open": 56372, - "volume": 14835506 - }, - "id": 42062, - "non_hive": { - "close": 100000, - "high": 2, - "low": 1, - "open": 87376, - "volume": 23275275 - }, - "open": "2016-08-13T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 6005, - "high": 5, - "low": 1, - "open": 15313, - "volume": 3012819 - }, - "id": 42653, - "non_hive": { - "close": 10080, - "high": 10, - "low": 1, - "open": 27412, - "volume": 5209523 - }, - "open": "2016-08-14T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 6181, - "high": 1, - "low": 1, - "open": 43598, - "volume": 5739487 - }, - "id": 43263, - "non_hive": { - "close": 10193, - "high": 2, - "low": 1, - "open": 73000, - "volume": 9169057 - }, - "open": "2016-08-15T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 59656, - "high": 1, - "low": 1, - "open": 428, - "volume": 33004275 - }, - "id": 43746, - "non_hive": { - "close": 84712, - "high": 2, - "low": 1, - "open": 707, - "volume": 48400176 - }, - "open": "2016-08-16T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 58290, - "high": 1, - "low": 1, - "open": 585003, - "volume": 40646155 - }, - "id": 44479, - "non_hive": { - "close": 89768, - "high": 2, - "low": 1, - "open": 830704, - "volume": 61130769 - }, - "open": "2016-08-17T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 54587, - "high": 1, - "low": 1, - "open": 30000, - "volume": 13919200 - }, - "id": 45266, - "non_hive": { - "close": 80788, - "high": 3, - "low": 1, - "open": 46200, - "volume": 21329935 - }, - "open": "2016-08-18T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 37681, - "high": 1, - "low": 1, - "open": 1562, - "volume": 16709235 - }, - "id": 46145, - "non_hive": { - "close": 62062, - "high": 2, - "low": 1, - "open": 2312, - "volume": 25167234 - }, - "open": "2016-08-19T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 2150, - "high": 1, - "low": 1, - "open": 10118, - "volume": 5183006 - }, - "id": 46971, - "non_hive": { - "close": 3440, - "high": 2, - "low": 1, - "open": 16213, - "volume": 8274299 - }, - "open": "2016-08-20T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 130, - "high": 2, - "low": 1, - "open": 1060, - "volume": 4537167 - }, - "id": 47573, - "non_hive": { - "close": 208, - "high": 4, - "low": 1, - "open": 1685, - "volume": 7351723 - }, - "open": "2016-08-21T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 9872, - "high": 1, - "low": 1, - "open": 53, - "volume": 7289980 - }, - "id": 48073, - "non_hive": { - "close": 14813, - "high": 2, - "low": 1, - "open": 87, - "volume": 11275567 - }, - "open": "2016-08-22T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1542, - "high": 78, - "low": 1, - "open": 1, - "volume": 21112703 - }, - "id": 48774, - "non_hive": { - "close": 2100, - "high": 121, - "low": 1, - "open": 1, - "volume": 26904265 - }, - "open": "2016-08-23T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 10399, - "high": 1, - "low": 1, - "open": 1000, - "volume": 27724041 - }, - "id": 49439, - "non_hive": { - "close": 12998, - "high": 2, - "low": 1, - "open": 1288, - "volume": 32887083 - }, - "open": "2016-08-24T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 40636, - "high": 1, - "low": 2, - "open": 850, - "volume": 17063059 - }, - "id": 50451, - "non_hive": { - "close": 43074, - "high": 2, - "low": 2, - "open": 1071, - "volume": 18742667 - }, - "open": "2016-08-25T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 20000, - "high": 1, - "low": 10000, - "open": 7781, - "volume": 42645983 - }, - "id": 51094, - "non_hive": { - "close": 19100, - "high": 2, - "low": 8900, - "open": 8287, - "volume": 41181383 - }, - "open": "2016-08-26T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 190775, - "high": 1, - "low": 1572, - "open": 500052, - "volume": 51321832 - }, - "id": 51903, - "non_hive": { - "close": 182191, - "high": 1, - "low": 1400, - "open": 477550, - "volume": 47974216 - }, - "open": "2016-08-27T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 2228, - "high": 1, - "low": 129527, - "open": 30000, - "volume": 4627575 - }, - "id": 52844, - "non_hive": { - "close": 2000, - "high": 1, - "low": 111522, - "open": 28650, - "volume": 4367963 - }, - "open": "2016-08-28T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 302, - "high": 1, - "low": 3, - "open": 5000, - "volume": 36005030 - }, - "id": 53210, - "non_hive": { - "close": 376, - "high": 2, - "low": 2, - "open": 4396, - "volume": 37546287 - }, - "open": "2016-08-29T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 2241, - "high": 1, - "low": 3100, - "open": 4501, - "volume": 15321535 - }, - "id": 54171, - "non_hive": { - "close": 2677, - "high": 2, - "low": 2929, - "open": 5062, - "volume": 16179597 - }, - "open": "2016-08-30T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 37, - "high": 1, - "low": 10, - "open": 6232, - "volume": 3545447 - }, - "id": 54727, - "non_hive": { - "close": 41, - "high": 2, - "low": 10, - "open": 7230, - "volume": 3836196 - }, - "open": "2016-08-31T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1281, - "high": 1, - "low": 177, - "open": 8000, - "volume": 9363989 - }, - "id": 55288, - "non_hive": { - "close": 1184, - "high": 2, - "low": 141, - "open": 8639, - "volume": 8800923 - }, - "open": "2016-09-01T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4920, - "high": 149963, - "low": 1885, - "open": 11511, - "volume": 20105800 - }, - "id": 55833, - "non_hive": { - "close": 4832, - "high": 162409, - "low": 1696, - "open": 10814, - "volume": 19141955 - }, - "open": "2016-09-02T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 18405, - "high": 11, - "low": 11, - "open": 4637, - "volume": 3805695 - }, - "id": 56581, - "non_hive": { - "close": 17833, - "high": 12, - "low": 10, - "open": 4329, - "volume": 3711306 - }, - "open": "2016-09-03T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 329, - "high": 10, - "low": 6, - "open": 1300, - "volume": 2086876 - }, - "id": 56962, - "non_hive": { - "close": 328, - "high": 11, - "low": 5, - "open": 1299, - "volume": 2034882 - }, - "open": "2016-09-04T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 5009, - "high": 1, - "low": 446306, - "open": 7905, - "volume": 14358640 - }, - "id": 57384, - "non_hive": { - "close": 4734, - "high": 1, - "low": 397212, - "open": 7892, - "volume": 13730864 - }, - "open": "2016-09-05T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 19, - "high": 3451, - "low": 405, - "open": 10467, - "volume": 4815859 - }, - "id": 57958, - "non_hive": { - "close": 19, - "high": 3961, - "low": 372, - "open": 9839, - "volume": 4798741 - }, - "open": "2016-09-06T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1000, - "high": 19, - "low": 1205, - "open": 3044, - "volume": 5022646 - }, - "id": 58369, - "non_hive": { - "close": 950, - "high": 19, - "low": 1073, - "open": 3001, - "volume": 4658058 - }, - "open": "2016-09-07T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3097, - "high": 1, - "low": 4000, - "open": 500, - "volume": 26835532 - }, - "id": 58807, - "non_hive": { - "close": 2508, - "high": 1, - "low": 2759, - "open": 475, - "volume": 22106387 - }, - "open": "2016-09-08T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 31647, - "high": 1, - "low": 15, - "open": 1358, - "volume": 8228907 - }, - "id": 59508, - "non_hive": { - "close": 25981, - "high": 1, - "low": 9, - "open": 1212, - "volume": 6085088 - }, - "open": "2016-09-09T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 7767, - "high": 1, - "low": 5320, - "open": 9459, - "volume": 3313138 - }, - "id": 60114, - "non_hive": { - "close": 6835, - "high": 1, - "low": 4315, - "open": 7842, - "volume": 2844868 - }, - "open": "2016-09-10T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 41, - "high": 1, - "low": 41, - "open": 1946, - "volume": 3099687 - }, - "id": 60681, - "non_hive": { - "close": 33, - "high": 1, - "low": 33, - "open": 1712, - "volume": 2734079 - }, - "open": "2016-09-11T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 56430, - "high": 1, - "low": 288645, - "open": 9808, - "volume": 36811007 - }, - "id": 61262, - "non_hive": { - "close": 42323, - "high": 1, - "low": 144322, - "open": 8016, - "volume": 25460353 - }, - "open": "2016-09-12T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 693, - "high": 1, - "low": 11912, - "open": 1400, - "volume": 34497248 - }, - "id": 62088, - "non_hive": { - "close": 451, - "high": 1, - "low": 7580, - "open": 1050, - "volume": 22969179 - }, - "open": "2016-09-13T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 7004, - "high": 1, - "low": 1962, - "open": 14895, - "volume": 22214479 - }, - "id": 62828, - "non_hive": { - "close": 4202, - "high": 1, - "low": 1177, - "open": 9681, - "volume": 14023235 - }, - "open": "2016-09-14T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1438, - "high": 1, - "low": 115104, - "open": 12649, - "volume": 10206322 - }, - "id": 63427, - "non_hive": { - "close": 877, - "high": 1, - "low": 58128, - "open": 8563, - "volume": 6391099 - }, - "open": "2016-09-15T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4123, - "high": 1, - "low": 17161, - "open": 1605, - "volume": 36534332 - }, - "id": 63909, - "non_hive": { - "close": 2268, - "high": 1, - "low": 9086, - "open": 979, - "volume": 19922927 - }, - "open": "2016-09-16T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 200, - "high": 1, - "low": 900, - "open": 238445, - "volume": 9250103 - }, - "id": 64639, - "non_hive": { - "close": 123, - "high": 1, - "low": 495, - "open": 131145, - "volume": 5654465 - }, - "open": "2016-09-17T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 28, - "high": 1, - "low": 9, - "open": 9972, - "volume": 1001614 - }, - "id": 64951, - "non_hive": { - "close": 18, - "high": 1, - "low": 5, - "open": 6132, - "volume": 628747 - }, - "open": "2016-09-18T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1600, - "high": 1, - "low": 1000000, - "open": 713, - "volume": 69617010 - }, - "id": 65232, - "non_hive": { - "close": 848, - "high": 1, - "low": 50000, - "open": 429, - "volume": 28959058 - }, - "open": "2016-09-19T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 25754, - "high": 1, - "low": 1880, - "open": 1605, - "volume": 22020263 - }, - "id": 66132, - "non_hive": { - "close": 13391, - "high": 1, - "low": 754, - "open": 848, - "volume": 10985780 - }, - "open": "2016-09-20T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 11877, - "high": 1, - "low": 2425589, - "open": 1, - "volume": 10024742 - }, - "id": 66529, - "non_hive": { - "close": 5592, - "high": 1, - "low": 975072, - "open": 1, - "volume": 4351494 - }, - "open": "2016-09-21T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1000, - "high": 1, - "low": 8, - "open": 12268, - "volume": 12440573 - }, - "id": 66977, - "non_hive": { - "close": 569, - "high": 1, - "low": 3, - "open": 5949, - "volume": 6498573 - }, - "open": "2016-09-22T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 69, - "high": 1, - "low": 9, - "open": 3584, - "volume": 4531366 - }, - "id": 67597, - "non_hive": { - "close": 36, - "high": 1, - "low": 4, - "open": 2036, - "volume": 2547129 - }, - "open": "2016-09-23T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4563, - "high": 1, - "low": 3312, - "open": 1019, - "volume": 7156660 - }, - "id": 68032, - "non_hive": { - "close": 2993, - "high": 1, - "low": 1656, - "open": 541, - "volume": 4757462 - }, - "open": "2016-09-24T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 6480, - "high": 1, - "low": 11, - "open": 7802, - "volume": 7454992 - }, - "id": 68478, - "non_hive": { - "close": 4299, - "high": 1, - "low": 6, - "open": 5072, - "volume": 5021274 - }, - "open": "2016-09-25T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1, - "high": 1, - "low": 3, - "open": 1, - "volume": 10202732 - }, - "id": 68995, - "non_hive": { - "close": 1, - "high": 1, - "low": 1, - "open": 1, - "volume": 6062452 - }, - "open": "2016-09-26T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1001, - "high": 1, - "low": 26, - "open": 980, - "volume": 8387302 - }, - "id": 69434, - "non_hive": { - "close": 573, - "high": 1, - "low": 14, - "open": 566, - "volume": 5107646 - }, - "open": "2016-09-27T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 8396, - "high": 1, - "low": 14, - "open": 40, - "volume": 9370437 - }, - "id": 69776, - "non_hive": { - "close": 4315, - "high": 1, - "low": 7, - "open": 23, - "volume": 5281452 - }, - "open": "2016-09-28T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3770, - "high": 1, - "low": 23, - "open": 11600, - "volume": 11100192 - }, - "id": 70059, - "non_hive": { - "close": 2141, - "high": 1, - "low": 11, - "open": 6564, - "volume": 6150931 - }, - "open": "2016-09-29T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 57, - "high": 1, - "low": 5, - "open": 605, - "volume": 924659 - }, - "id": 70525, - "non_hive": { - "close": 32, - "high": 1, - "low": 2, - "open": 344, - "volume": 504117 - }, - "open": "2016-09-30T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 25181, - "high": 1, - "low": 7, - "open": 850935, - "volume": 12429747 - }, - "id": 70763, - "non_hive": { - "close": 12087, - "high": 1, - "low": 3, - "open": 468014, - "volume": 6588852 - }, - "open": "2016-10-01T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 28161, - "high": 1, - "low": 5, - "open": 2249, - "volume": 7289464 - }, - "id": 71170, - "non_hive": { - "close": 13236, - "high": 1, - "low": 2, - "open": 1149, - "volume": 3635854 - }, - "open": "2016-10-02T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 14854, - "high": 1, - "low": 3, - "open": 9371, - "volume": 7986237 - }, - "id": 71609, - "non_hive": { - "close": 7011, - "high": 1, - "low": 1, - "open": 4520, - "volume": 3929587 - }, - "open": "2016-10-03T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 9798, - "high": 2, - "low": 833, - "open": 50, - "volume": 13181214 - }, - "id": 71994, - "non_hive": { - "close": 4497, - "high": 1, - "low": 346, - "open": 23, - "volume": 5983409 - }, - "open": "2016-10-04T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 17081, - "high": 2, - "low": 3, - "open": 199, - "volume": 5030060 - }, - "id": 72411, - "non_hive": { - "close": 7583, - "high": 1, - "low": 1, - "open": 93, - "volume": 2201222 - }, - "open": "2016-10-05T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4762, - "high": 2, - "low": 37, - "open": 1628, - "volume": 15238834 - }, - "id": 72762, - "non_hive": { - "close": 2044, - "high": 1, - "low": 15, - "open": 722, - "volume": 6574708 - }, - "open": "2016-10-06T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 16361, - "high": 2, - "low": 12, - "open": 9617, - "volume": 32343344 - }, - "id": 73137, - "non_hive": { - "close": 6293, - "high": 1, - "low": 4, - "open": 4129, - "volume": 12695981 - }, - "open": "2016-10-07T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 631, - "high": 2, - "low": 23, - "open": 429, - "volume": 4694111 - }, - "id": 73651, - "non_hive": { - "close": 278, - "high": 1, - "low": 8, - "open": 163, - "volume": 1913852 - }, - "open": "2016-10-08T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1000, - "high": 2, - "low": 12, - "open": 25411, - "volume": 2824933 - }, - "id": 74055, - "non_hive": { - "close": 361, - "high": 1, - "low": 4, - "open": 11154, - "volume": 1071573 - }, - "open": "2016-10-09T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 9872, - "high": 2, - "low": 11, - "open": 1000, - "volume": 22875756 - }, - "id": 74499, - "non_hive": { - "close": 3164, - "high": 1, - "low": 3, - "open": 361, - "volume": 7756609 - }, - "open": "2016-10-10T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 6227, - "high": 2, - "low": 10, - "open": 208, - "volume": 10467702 - }, - "id": 75382, - "non_hive": { - "close": 1725, - "high": 1, - "low": 2, - "open": 70, - "volume": 3027029 - }, - "open": "2016-10-11T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 15509, - "high": 10003, - "low": 14, - "open": 21708, - "volume": 5426490 - }, - "id": 75909, - "non_hive": { - "close": 4615, - "high": 3341, - "low": 3, - "open": 6078, - "volume": 1629470 - }, - "open": "2016-10-12T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3800, - "high": 2, - "low": 17, - "open": 9568, - "volume": 9830018 - }, - "id": 76351, - "non_hive": { - "close": 1141, - "high": 1, - "low": 4, - "open": 2847, - "volume": 2986293 - }, - "open": "2016-10-13T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3, - "high": 2, - "low": 6649, - "open": 253, - "volume": 3659049 - }, - "id": 76891, - "non_hive": { - "close": 1, - "high": 1, - "low": 1805, - "open": 76, - "volume": 1090730 - }, - "open": "2016-10-14T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 265, - "high": 3, - "low": 11, - "open": 664, - "volume": 2517708 - }, - "id": 77272, - "non_hive": { - "close": 73, - "high": 1, - "low": 2, - "open": 186, - "volume": 695103 - }, - "open": "2016-10-15T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 34, - "high": 2, - "low": 27, - "open": 280, - "volume": 10080631 - }, - "id": 77702, - "non_hive": { - "close": 8, - "high": 1, - "low": 6, - "open": 73, - "volume": 2866020 - }, - "open": "2016-10-16T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3606, - "high": 3, - "low": 10, - "open": 1800, - "volume": 9720445 - }, - "id": 78198, - "non_hive": { - "close": 976, - "high": 1, - "low": 2, - "open": 477, - "volume": 2638303 - }, - "open": "2016-10-17T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 97777, - "high": 3, - "low": 47, - "open": 3, - "volume": 22777810 - }, - "id": 79045, - "non_hive": { - "close": 24756, - "high": 1, - "low": 11, - "open": 1, - "volume": 5823062 - }, - "open": "2016-10-18T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 858105, - "high": 3, - "low": 5589, - "open": 3199, - "volume": 22154153 - }, - "id": 80028, - "non_hive": { - "close": 211522, - "high": 1, - "low": 1176, - "open": 813, - "volume": 5547112 - }, - "open": "2016-10-19T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 11079, - "high": 3, - "low": 10, - "open": 4, - "volume": 13520644 - }, - "id": 80788, - "non_hive": { - "close": 2836, - "high": 1, - "low": 2, - "open": 1, - "volume": 3329695 - }, - "open": "2016-10-20T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1703, - "high": 3, - "low": 20, - "open": 11783, - "volume": 7135933 - }, - "id": 81516, - "non_hive": { - "close": 433, - "high": 1, - "low": 4, - "open": 3000, - "volume": 1777295 - }, - "open": "2016-10-21T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4302, - "high": 3, - "low": 10, - "open": 1901, - "volume": 7476400 - }, - "id": 82301, - "non_hive": { - "close": 1014, - "high": 1, - "low": 2, - "open": 480, - "volume": 1811624 - }, - "open": "2016-10-22T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 84339, - "high": 4, - "low": 7, - "open": 2000, - "volume": 17422444 - }, - "id": 83598, - "non_hive": { - "close": 17887, - "high": 1, - "low": 1, - "open": 471, - "volume": 3843881 - }, - "open": "2016-10-23T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 5000, - "high": 4, - "low": 19, - "open": 9530, - "volume": 10996057 - }, - "id": 85042, - "non_hive": { - "close": 1017, - "high": 1, - "low": 3, - "open": 2018, - "volume": 2334979 - }, - "open": "2016-10-24T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 45000, - "high": 4, - "low": 7726, - "open": 17567, - "volume": 14122447 - }, - "id": 86226, - "non_hive": { - "close": 8136, - "high": 1, - "low": 1313, - "open": 3571, - "volume": 2733418 - }, - "open": "2016-10-25T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 15660, - "high": 1629, - "low": 8691, - "open": 9779, - "volume": 29105453 - }, - "id": 87112, - "non_hive": { - "close": 2293, - "high": 328, - "low": 956, - "open": 1770, - "volume": 4710557 - }, - "open": "2016-10-26T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 104, - "high": 5, - "low": 10, - "open": 77846, - "volume": 8049942 - }, - "id": 88324, - "non_hive": { - "close": 15, - "high": 1, - "low": 1, - "open": 11886, - "volume": 1224195 - }, - "open": "2016-10-27T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 2000, - "high": 5, - "low": 17031, - "open": 33099, - "volume": 13322845 - }, - "id": 88975, - "non_hive": { - "close": 357, - "high": 1, - "low": 2299, - "open": 4985, - "volume": 2051773 - }, - "open": "2016-10-28T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 133355, - "high": 2292, - "low": 21, - "open": 2962, - "volume": 10224009 - }, - "id": 90080, - "non_hive": { - "close": 20000, - "high": 481, - "low": 3, - "open": 529, - "volume": 1693680 - }, - "open": "2016-10-29T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 47053, - "high": 392318, - "low": 63, - "open": 26679, - "volume": 10964053 - }, - "id": 91589, - "non_hive": { - "close": 7740, - "high": 70617, - "low": 9, - "open": 4020, - "volume": 1754817 - }, - "open": "2016-10-30T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4965, - "high": 6, - "low": 63847, - "open": 122128, - "volume": 10273980 - }, - "id": 92478, - "non_hive": { - "close": 810, - "high": 1, - "low": 9767, - "open": 20000, - "volume": 1683802 - }, - "open": "2016-10-31T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 132506, - "high": 6, - "low": 36, - "open": 2482, - "volume": 17078153 - }, - "id": 93614, - "non_hive": { - "close": 20000, - "high": 1, - "low": 5, - "open": 404, - "volume": 2599313 - }, - "open": "2016-11-01T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 9823, - "high": 6, - "low": 15990, - "open": 6625, - "volume": 29227900 - }, - "id": 94550, - "non_hive": { - "close": 1313, - "high": 1, - "low": 2023, - "open": 1000, - "volume": 4039474 - }, - "open": "2016-11-02T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 46005, - "high": 7, - "low": 28, - "open": 4915, - "volume": 36154644 - }, - "id": 95174, - "non_hive": { - "close": 5469, - "high": 1, - "low": 3, - "open": 657, - "volume": 4461963 - }, - "open": "2016-11-03T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 82968, - "high": 5, - "low": 34, - "open": 40083, - "volume": 40631413 - }, - "id": 95854, - "non_hive": { - "close": 12132, - "high": 1, - "low": 4, - "open": 4765, - "volume": 5931508 - }, - "open": "2016-11-04T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4830, - "high": 3, - "low": 64639, - "open": 6, - "volume": 69419514 - }, - "id": 97385, - "non_hive": { - "close": 1101, - "high": 1, - "low": 9049, - "open": 1, - "volume": 15581178 - }, - "open": "2016-11-05T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1437, - "high": 4, - "low": 7, - "open": 59760, - "volume": 24389191 - }, - "id": 100450, - "non_hive": { - "close": 309, - "high": 1, - "low": 1, - "open": 13705, - "volume": 5356563 - }, - "open": "2016-11-06T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4521, - "high": 4, - "low": 68414, - "open": 61825, - "volume": 17426017 - }, - "id": 101673, - "non_hive": { - "close": 734, - "high": 1, - "low": 10839, - "open": 13355, - "volume": 3254281 - }, - "open": "2016-11-07T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 638, - "high": 5, - "low": 109, - "open": 82605, - "volume": 7561692 - }, - "id": 102256, - "non_hive": { - "close": 86, - "high": 1, - "low": 14, - "open": 14445, - "volume": 1160730 - }, - "open": "2016-11-08T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 50000, - "high": 135, - "low": 538, - "open": 79520, - "volume": 9944187 - }, - "id": 102555, - "non_hive": { - "close": 7550, - "high": 23, - "low": 64, - "open": 10732, - "volume": 1449799 - }, - "open": "2016-11-09T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 100000, - "high": 11, - "low": 37, - "open": 6488, - "volume": 14513110 - }, - "id": 102908, - "non_hive": { - "close": 15000, - "high": 2, - "low": 5, - "open": 969, - "volume": 2188707 - }, - "open": "2016-11-10T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 13915, - "high": 6, - "low": 66586, - "open": 46576, - "volume": 15934690 - }, - "id": 103282, - "non_hive": { - "close": 1809, - "high": 1, - "low": 6991, - "open": 6986, - "volume": 2295799 - }, - "open": "2016-11-11T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 678, - "high": 7, - "low": 35552, - "open": 1000, - "volume": 4830221 - }, - "id": 103737, - "non_hive": { - "close": 84, - "high": 1, - "low": 3910, - "open": 124, - "volume": 627235 - }, - "open": "2016-11-12T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 977, - "high": 7, - "low": 2356, - "open": 540, - "volume": 4656303 - }, - "id": 104155, - "non_hive": { - "close": 117, - "high": 1, - "low": 282, - "open": 69, - "volume": 588968 - }, - "open": "2016-11-13T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 45323, - "high": 3044, - "low": 1390, - "open": 993, - "volume": 5153362 - }, - "id": 104451, - "non_hive": { - "close": 5219, - "high": 392, - "low": 153, - "open": 123, - "volume": 616057 - }, - "open": "2016-11-14T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 12142, - "high": 8, - "low": 14, - "open": 1000, - "volume": 9206309 - }, - "id": 104826, - "non_hive": { - "close": 1367, - "high": 1, - "low": 1, - "open": 120, - "volume": 1073118 - }, - "open": "2016-11-15T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 47147, - "high": 85713, - "low": 83, - "open": 88374, - "volume": 31682387 - }, - "id": 105203, - "non_hive": { - "close": 5006, - "high": 9956, - "low": 8, - "open": 10000, - "volume": 3446908 - }, - "open": "2016-11-16T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 24972, - "high": 9, - "low": 332, - "open": 7550, - "volume": 15067947 - }, - "id": 105854, - "non_hive": { - "close": 2608, - "high": 1, - "low": 34, - "open": 800, - "volume": 1582322 - }, - "open": "2016-11-17T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 209446, - "high": 8, - "low": 1108969, - "open": 200000, - "volume": 70063237 - }, - "id": 106281, - "non_hive": { - "close": 24322, - "high": 1, - "low": 111007, - "open": 20889, - "volume": 7628044 - }, - "open": "2016-11-18T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 27033, - "high": 1003, - "low": 39441, - "open": 10678, - "volume": 26968535 - }, - "id": 107259, - "non_hive": { - "close": 3394, - "high": 127, - "low": 4420, - "open": 1236, - "volume": 3189137 - }, - "open": "2016-11-19T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 46855, - "high": 7, - "low": 13, - "open": 277, - "volume": 76652422 - }, - "id": 107866, - "non_hive": { - "close": 5359, - "high": 1, - "low": 1, - "open": 35, - "volume": 9473140 - }, - "open": "2016-11-20T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 9187, - "high": 8, - "low": 48, - "open": 345788, - "volume": 31925134 - }, - "id": 109109, - "non_hive": { - "close": 1050, - "high": 1, - "low": 5, - "open": 39765, - "volume": 3649891 - }, - "open": "2016-11-21T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 69149, - "high": 8, - "low": 55, - "open": 401, - "volume": 14043341 - }, - "id": 109704, - "non_hive": { - "close": 7789, - "high": 1, - "low": 6, - "open": 45, - "volume": 1595631 - }, - "open": "2016-11-22T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 218, - "high": 8, - "low": 164, - "open": 322652, - "volume": 26908852 - }, - "id": 110207, - "non_hive": { - "close": 24, - "high": 1, - "low": 18, - "open": 36545, - "volume": 3022806 - }, - "open": "2016-11-23T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 8000, - "high": 8, - "low": 141, - "open": 18198, - "volume": 43567271 - }, - "id": 110816, - "non_hive": { - "close": 978, - "high": 1, - "low": 15, - "open": 2043, - "volume": 5151556 - }, - "open": "2016-11-24T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 79690, - "high": 6, - "low": 9, - "open": 50494, - "volume": 83935642 - }, - "id": 111609, - "non_hive": { - "close": 11028, - "high": 1, - "low": 1, - "open": 6175, - "volume": 11828448 - }, - "open": "2016-11-25T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 15630, - "high": 15095, - "low": 1000, - "open": 39313, - "volume": 27209772 - }, - "id": 112966, - "non_hive": { - "close": 2280, - "high": 2253, - "low": 136, - "open": 5450, - "volume": 3804888 - }, - "open": "2016-11-26T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4576, - "high": 5, - "low": 10, - "open": 213401, - "volume": 64111667 - }, - "id": 113483, - "non_hive": { - "close": 815, - "high": 1, - "low": 1, - "open": 31279, - "volume": 11062442 - }, - "open": "2016-11-27T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 155165, - "high": 5, - "low": 180, - "open": 60000, - "volume": 56954996 - }, - "id": 114600, - "non_hive": { - "close": 25855, - "high": 1, - "low": 29, - "open": 10650, - "volume": 10004104 - }, - "open": "2016-11-28T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 18700, - "high": 5, - "low": 800, - "open": 900, - "volume": 55828680 - }, - "id": 115745, - "non_hive": { - "close": 3037, - "high": 1, - "low": 119, - "open": 150, - "volume": 8843283 - }, - "open": "2016-11-29T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 16658, - "high": 2032, - "low": 16658, - "open": 73903, - "volume": 42889199 - }, - "id": 116733, - "non_hive": { - "close": 2571, - "high": 346, - "low": 2571, - "open": 12002, - "volume": 7016841 - }, - "open": "2016-11-30T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 35000, - "high": 6, - "low": 15, - "open": 28900, - "volume": 47148239 - }, - "id": 117613, - "non_hive": { - "close": 5338, - "high": 1, - "low": 2, - "open": 4461, - "volume": 7175471 - }, - "open": "2016-12-01T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 237639, - "high": 5, - "low": 74, - "open": 9000, - "volume": 43827835 - }, - "id": 118520, - "non_hive": { - "close": 40000, - "high": 1, - "low": 11, - "open": 1366, - "volume": 6955661 - }, - "open": "2016-12-02T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 10277, - "high": 5, - "low": 13, - "open": 13811, - "volume": 63495362 - }, - "id": 119407, - "non_hive": { - "close": 2024, - "high": 1, - "low": 2, - "open": 2319, - "volume": 11681313 - }, - "open": "2016-12-03T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 8200, - "high": 5328, - "low": 44792, - "open": 172212, - "volume": 28076459 - }, - "id": 120567, - "non_hive": { - "close": 1685, - "high": 1108, - "low": 8062, - "open": 33926, - "volume": 5484944 - }, - "open": "2016-12-04T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 126268, - "high": 10781, - "low": 32, - "open": 7722, - "volume": 76676817 - }, - "id": 121460, - "non_hive": { - "close": 30465, - "high": 2899, - "low": 6, - "open": 1594, - "volume": 16952688 - }, - "open": "2016-12-05T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 9500, - "high": 3, - "low": 11, - "open": 140001, - "volume": 102442363 - }, - "id": 123013, - "non_hive": { - "close": 2327, - "high": 1, - "low": 2, - "open": 33454, - "volume": 28110329 - }, - "open": "2016-12-06T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3360, - "high": 4, - "low": 29, - "open": 30938, - "volume": 25169978 - }, - "id": 125160, - "non_hive": { - "close": 733, - "high": 1, - "low": 5, - "open": 7580, - "volume": 5745507 - }, - "open": "2016-12-07T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 206073, - "high": 159, - "low": 203, - "open": 208314, - "volume": 51566035 - }, - "id": 125957, - "non_hive": { - "close": 50000, - "high": 40, - "low": 42, - "open": 45682, - "volume": 11847765 - }, - "open": "2016-12-08T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 200001, - "high": 38966, - "low": 10, - "open": 206547, - "volume": 48900021 - }, - "id": 126809, - "non_hive": { - "close": 47259, - "high": 9974, - "low": 2, - "open": 50000, - "volume": 12024787 - }, - "open": "2016-12-09T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 70877, - "high": 4, - "low": 70877, - "open": 100000, - "volume": 71734833 - }, - "id": 127700, - "non_hive": { - "close": 15305, - "high": 1, - "low": 15305, - "open": 23561, - "volume": 16538106 - }, - "open": "2016-12-10T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 13061, - "high": 4, - "low": 17, - "open": 206604, - "volume": 39761019 - }, - "id": 128741, - "non_hive": { - "close": 2424, - "high": 1, - "low": 3, - "open": 44695, - "volume": 8009827 - }, - "open": "2016-12-11T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 349000, - "high": 5, - "low": 10, - "open": 321736, - "volume": 30596032 - }, - "id": 129459, - "non_hive": { - "close": 67416, - "high": 1, - "low": 1, - "open": 60000, - "volume": 5846823 - }, - "open": "2016-12-12T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 12365, - "high": 9753558, - "low": 27, - "open": 200, - "volume": 70372343 - }, - "id": 130057, - "non_hive": { - "close": 1956, - "high": 1939000, - "low": 4, - "open": 38, - "volume": 12367829 - }, - "open": "2016-12-13T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 289487, - "high": 5, - "low": 49, - "open": 68574, - "volume": 96292114 - }, - "id": 130957, - "non_hive": { - "close": 49321, - "high": 1, - "low": 7, - "open": 10852, - "volume": 15935047 - }, - "open": "2016-12-14T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 333574, - "high": 5, - "low": 13749, - "open": 353150, - "volume": 82395962 - }, - "id": 131953, - "non_hive": { - "close": 60000, - "high": 1, - "low": 2245, - "open": 60000, - "volume": 14605040 - }, - "open": "2016-12-15T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 283160, - "high": 5, - "low": 78, - "open": 334478, - "volume": 38384712 - }, - "id": 132883, - "non_hive": { - "close": 47724, - "high": 1, - "low": 13, - "open": 60000, - "volume": 6732192 - }, - "open": "2016-12-16T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 106273, - "high": 108, - "low": 395668, - "open": 295940, - "volume": 34750779 - }, - "id": 133569, - "non_hive": { - "close": 17105, - "high": 19, - "low": 59468, - "open": 50000, - "volume": 5718374 - }, - "open": "2016-12-17T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 2037, - "high": 5, - "low": 9726, - "open": 28544, - "volume": 23022307 - }, - "id": 134206, - "non_hive": { - "close": 339, - "high": 1, - "low": 1461, - "open": 4572, - "volume": 3818682 - }, - "open": "2016-12-18T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3531, - "high": 201163, - "low": 335578, - "open": 5813, - "volume": 56229347 - }, - "id": 134829, - "non_hive": { - "close": 530, - "high": 33657, - "low": 50000, - "open": 968, - "volume": 8678131 - }, - "open": "2016-12-19T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 839595, - "high": 6, - "low": 97, - "open": 32826, - "volume": 57169421 - }, - "id": 135418, - "non_hive": { - "close": 114397, - "high": 1, - "low": 13, - "open": 4926, - "volume": 8373996 - }, - "open": "2016-12-20T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 342101, - "high": 2402, - "low": 33, - "open": 30910, - "volume": 97486574 - }, - "id": 136342, - "non_hive": { - "close": 45770, - "high": 338, - "low": 4, - "open": 4275, - "volume": 13107200 - }, - "open": "2016-12-21T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 61528, - "high": 7, - "low": 41, - "open": 340614, - "volume": 36710221 - }, - "id": 137249, - "non_hive": { - "close": 8194, - "high": 1, - "low": 5, - "open": 45682, - "volume": 4773989 - }, - "open": "2016-12-22T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3536, - "high": 6, - "low": 108, - "open": 16184, - "volume": 56273399 - }, - "id": 137853, - "non_hive": { - "close": 498, - "high": 1, - "low": 14, - "open": 2145, - "volume": 7770888 - }, - "open": "2016-12-23T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 102, - "high": 7, - "low": 50, - "open": 9200, - "volume": 55177229 - }, - "id": 138786, - "non_hive": { - "close": 14, - "high": 1, - "low": 6, - "open": 1289, - "volume": 7627132 - }, - "open": "2016-12-24T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 23448, - "high": 7, - "low": 18, - "open": 368001, - "volume": 33375874 - }, - "id": 139374, - "non_hive": { - "close": 3152, - "high": 1, - "low": 2, - "open": 50000, - "volume": 4418888 - }, - "open": "2016-12-25T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 67000, - "high": 6, - "low": 10, - "open": 3544, - "volume": 44270637 - }, - "id": 139832, - "non_hive": { - "close": 10144, - "high": 1, - "low": 1, - "open": 474, - "volume": 6469868 - }, - "open": "2016-12-26T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 47005, - "high": 12, - "low": 14611, - "open": 244672, - "volume": 30997979 - }, - "id": 140727, - "non_hive": { - "close": 7051, - "high": 2, - "low": 2157, - "open": 37046, - "volume": 4676232 - }, - "open": "2016-12-27T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 329203, - "high": 6, - "low": 37, - "open": 242, - "volume": 35253142 - }, - "id": 141354, - "non_hive": { - "close": 50000, - "high": 1, - "low": 5, - "open": 36, - "volume": 5335742 - }, - "open": "2016-12-28T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 32293, - "high": 3175, - "low": 2414, - "open": 1379, - "volume": 41351177 - }, - "id": 141933, - "non_hive": { - "close": 5218, - "high": 516, - "low": 367, - "open": 210, - "volume": 6509830 - }, - "open": "2016-12-29T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 293359, - "high": 70708, - "low": 39, - "open": 24721, - "volume": 37581279 - }, - "id": 142612, - "non_hive": { - "close": 47436, - "high": 12360, - "low": 6, - "open": 4014, - "volume": 6288376 - }, - "open": "2016-12-30T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4529, - "high": 5, - "low": 28, - "open": 97044, - "volume": 40272547 - }, - "id": 143300, - "non_hive": { - "close": 768, - "high": 1, - "low": 4, - "open": 15705, - "volume": 6629957 - }, - "open": "2016-12-31T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 39163, - "high": 5, - "low": 21, - "open": 7073, - "volume": 47719107 - }, - "id": 143910, - "non_hive": { - "close": 6307, - "high": 1, - "low": 3, - "open": 1206, - "volume": 7931540 - }, - "open": "2017-01-01T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 849, - "high": 45280, - "low": 100, - "open": 7848, - "volume": 33978197 - }, - "id": 144633, - "non_hive": { - "close": 131, - "high": 7590, - "low": 15, - "open": 1264, - "volume": 5508307 - }, - "open": "2017-01-02T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 22821, - "high": 1003, - "low": 7, - "open": 100, - "volume": 24135173 - }, - "id": 145336, - "non_hive": { - "close": 3611, - "high": 160, - "low": 1, - "open": 15, - "volume": 3800790 - }, - "open": "2017-01-03T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 254768, - "high": 5, - "low": 27, - "open": 314416, - "volume": 62264766 - }, - "id": 145965, - "non_hive": { - "close": 44209, - "high": 1, - "low": 4, - "open": 49512, - "volume": 10421483 - }, - "open": "2017-01-04T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 30472, - "high": 33, - "low": 25, - "open": 49669, - "volume": 62067339 - }, - "id": 146790, - "non_hive": { - "close": 4705, - "high": 6, - "low": 3, - "open": 8599, - "volume": 10288134 - }, - "open": "2017-01-05T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 2338, - "high": 6, - "low": 26, - "open": 8201, - "volume": 48502350 - }, - "id": 147525, - "non_hive": { - "close": 362, - "high": 1, - "low": 3, - "open": 1267, - "volume": 7472059 - }, - "open": "2017-01-06T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 5216, - "high": 6, - "low": 46, - "open": 9320, - "volume": 56794933 - }, - "id": 148223, - "non_hive": { - "close": 781, - "high": 1, - "low": 6, - "open": 1449, - "volume": 8489574 - }, - "open": "2017-01-07T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 41082, - "high": 6, - "low": 35, - "open": 396457, - "volume": 28713456 - }, - "id": 149145, - "non_hive": { - "close": 6243, - "high": 1, - "low": 5, - "open": 59059, - "volume": 4332560 - }, - "open": "2017-01-08T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 11287, - "high": 6, - "low": 40, - "open": 50000, - "volume": 31090591 - }, - "id": 149754, - "non_hive": { - "close": 1677, - "high": 1, - "low": 5, - "open": 7617, - "volume": 4724000 - }, - "open": "2017-01-09T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 8544, - "high": 6, - "low": 13, - "open": 13, - "volume": 31787185 - }, - "id": 150267, - "non_hive": { - "close": 1290, - "high": 1, - "low": 1, - "open": 2, - "volume": 4798179 - }, - "open": "2017-01-10T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4246, - "high": 6, - "low": 13, - "open": 58581, - "volume": 80251490 - }, - "id": 150906, - "non_hive": { - "close": 562, - "high": 1, - "low": 1, - "open": 8890, - "volume": 11162694 - }, - "open": "2017-01-11T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3040, - "high": 6, - "low": 9219, - "open": 410341, - "volume": 32629477 - }, - "id": 151743, - "non_hive": { - "close": 424, - "high": 1, - "low": 1198, - "open": 54305, - "volume": 4406572 - }, - "open": "2017-01-12T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1440, - "high": 7, - "low": 176825, - "open": 360265, - "volume": 51404788 - }, - "id": 152289, - "non_hive": { - "close": 195, - "high": 1, - "low": 22686, - "open": 50000, - "volume": 6824356 - }, - "open": "2017-01-13T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 14926, - "high": 7, - "low": 63, - "open": 14283, - "volume": 43130680 - }, - "id": 152949, - "non_hive": { - "close": 2031, - "high": 1, - "low": 8, - "open": 1928, - "volume": 5920056 - }, - "open": "2017-01-14T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1603, - "high": 7, - "low": 49, - "open": 7312, - "volume": 41975741 - }, - "id": 153592, - "non_hive": { - "close": 220, - "high": 1, - "low": 6, - "open": 1000, - "volume": 5762629 - }, - "open": "2017-01-15T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3704, - "high": 5, - "low": 31, - "open": 50000, - "volume": 131498136 - }, - "id": 154116, - "non_hive": { - "close": 668, - "high": 1, - "low": 4, - "open": 6828, - "volume": 21450429 - }, - "open": "2017-01-16T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3660, - "high": 5, - "low": 10, - "open": 4020, - "volume": 98586515 - }, - "id": 155544, - "non_hive": { - "close": 605, - "high": 1, - "low": 1, - "open": 725, - "volume": 16814608 - }, - "open": "2017-01-17T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 214865, - "high": 6, - "low": 10, - "open": 11605, - "volume": 68509366 - }, - "id": 156882, - "non_hive": { - "close": 34933, - "high": 1, - "low": 1, - "open": 1918, - "volume": 10779932 - }, - "open": "2017-01-18T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 51729, - "high": 301327, - "low": 90, - "open": 30000, - "volume": 37188264 - }, - "id": 157861, - "non_hive": { - "close": 8305, - "high": 51527, - "low": 14, - "open": 4885, - "volume": 6141693 - }, - "open": "2017-01-19T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1049, - "high": 6883, - "low": 72, - "open": 200002, - "volume": 52547802 - }, - "id": 158376, - "non_hive": { - "close": 169, - "high": 1150, - "low": 11, - "open": 32316, - "volume": 8649068 - }, - "open": "2017-01-20T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 4425, - "high": 71, - "low": 142352, - "open": 50381, - "volume": 38700544 - }, - "id": 159051, - "non_hive": { - "close": 738, - "high": 12, - "low": 21781, - "open": 8115, - "volume": 6168841 - }, - "open": "2017-01-21T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 18267, - "high": 5, - "low": 128, - "open": 1061, - "volume": 48588993 - }, - "id": 159731, - "non_hive": { - "close": 2929, - "high": 1, - "low": 20, - "open": 178, - "volume": 7946515 - }, - "open": "2017-01-22T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 711, - "high": 6, - "low": 24, - "open": 9922, - "volume": 31430822 - }, - "id": 160380, - "non_hive": { - "close": 112, - "high": 1, - "low": 3, - "open": 1591, - "volume": 4964642 - }, - "open": "2017-01-23T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 344758, - "high": 6, - "low": 344758, - "open": 1639, - "volume": 76247491 - }, - "id": 160976, - "non_hive": { - "close": 50000, - "high": 1, - "low": 50000, - "open": 258, - "volume": 11773005 - }, - "open": "2017-01-24T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 301575, - "high": 5619, - "low": 439, - "open": 51609, - "volume": 56171805 - }, - "id": 161726, - "non_hive": { - "close": 43571, - "high": 839, - "low": 63, - "open": 7503, - "volume": 8222768 - }, - "open": "2017-01-25T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 23666, - "high": 6, - "low": 43, - "open": 27415, - "volume": 28478686 - }, - "id": 162563, - "non_hive": { - "close": 3459, - "high": 1, - "low": 6, - "open": 3952, - "volume": 4147316 - }, - "open": "2017-01-26T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 9072, - "high": 6, - "low": 38, - "open": 254488, - "volume": 62263546 - }, - "id": 163136, - "non_hive": { - "close": 1353, - "high": 1, - "low": 5, - "open": 37196, - "volume": 9133191 - }, - "open": "2017-01-27T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 39425, - "high": 6, - "low": 69, - "open": 108600, - "volume": 21190875 - }, - "id": 163823, - "non_hive": { - "close": 6387, - "high": 1, - "low": 10, - "open": 16283, - "volume": 3284967 - }, - "open": "2017-01-28T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 167865, - "high": 3756, - "low": 43, - "open": 7524, - "volume": 21182033 - }, - "id": 164442, - "non_hive": { - "close": 27188, - "high": 631, - "low": 6, - "open": 1219, - "volume": 3436434 - }, - "open": "2017-01-29T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 19466, - "high": 215005, - "low": 8, - "open": 125674, - "volume": 13706157 - }, - "id": 165078, - "non_hive": { - "close": 3134, - "high": 35074, - "low": 1, - "open": 20325, - "volume": 2210340 - }, - "open": "2017-01-30T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 12913, - "high": 6, - "low": 100, - "open": 259685, - "volume": 38021871 - }, - "id": 165553, - "non_hive": { - "close": 2040, - "high": 1, - "low": 15, - "open": 41758, - "volume": 6018084 - }, - "open": "2017-01-31T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 110563, - "high": 16695, - "low": 73, - "open": 5532, - "volume": 23012452 - }, - "id": 166277, - "non_hive": { - "close": 17782, - "high": 2705, - "low": 11, - "open": 875, - "volume": 3672299 - }, - "open": "2017-02-01T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3690, - "high": 476207, - "low": 28, - "open": 9550, - "volume": 33626293 - }, - "id": 166852, - "non_hive": { - "close": 606, - "high": 80955, - "low": 4, - "open": 1536, - "volume": 5419970 - }, - "open": "2017-02-02T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 304915, - "high": 29045, - "low": 25, - "open": 727, - "volume": 24273065 - }, - "id": 167513, - "non_hive": { - "close": 48402, - "high": 4769, - "low": 3, - "open": 118, - "volume": 3886815 - }, - "open": "2017-02-03T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 3303, - "high": 54, - "low": 15, - "open": 143028, - "volume": 37162567 - }, - "id": 168100, - "non_hive": { - "close": 541, - "high": 9, - "low": 2, - "open": 22755, - "volume": 6060623 - }, - "open": "2017-02-04T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 2000, - "high": 6, - "low": 18, - "open": 157, - "volume": 15762496 - }, - "id": 168788, - "non_hive": { - "close": 315, - "high": 1, - "low": 2, - "open": 25, - "volume": 2525016 - }, - "open": "2017-02-05T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 1974, - "high": 62, - "low": 321706, - "open": 118725, - "volume": 13105072 - }, - "id": 169218, - "non_hive": { - "close": 313, - "high": 10, - "low": 50000, - "open": 18727, - "volume": 2056874 - }, - "open": "2017-02-06T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 271215, - "high": 6, - "low": 9463, - "open": 4323, - "volume": 51085045 - }, - "id": 169707, - "non_hive": { - "close": 41687, - "high": 1, - "low": 1423, - "open": 687, - "volume": 8072209 - }, - "open": "2017-02-07T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 131649, - "high": 6, - "low": 50, - "open": 296474, - "volume": 37283381 - }, - "id": 170553, - "non_hive": { - "close": 19846, - "high": 1, - "low": 7, - "open": 45682, - "volume": 5685240 - }, - "open": "2017-02-08T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 11656, - "high": 6, - "low": 50, - "open": 5321, - "volume": 44080348 - }, - "id": 171212, - "non_hive": { - "close": 1661, - "high": 1, - "low": 7, - "open": 798, - "volume": 6566800 - }, - "open": "2017-02-09T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 8817, - "high": 8817, - "low": 20, - "open": 8887, - "volume": 65493730 - }, - "id": 171830, - "non_hive": { - "close": 1279, - "high": 1279, - "low": 2, - "open": 1273, - "volume": 9197166 - }, - "open": "2017-02-10T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 6830, - "high": 249, - "low": 59, - "open": 75331, - "volume": 29520927 - }, - "id": 172468, - "non_hive": { - "close": 1000, - "high": 37, - "low": 8, - "open": 10927, - "volume": 4312957 - }, - "open": "2017-02-11T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 11649, - "high": 6, - "low": 86, - "open": 7000000, - "volume": 23107705 - }, - "id": 173007, - "non_hive": { - "close": 1648, - "high": 1, - "low": 12, - "open": 1022000, - "volume": 3346964 - }, - "open": "2017-02-12T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 364968, - "high": 21523, - "low": 94, - "open": 209050, - "volume": 41111747 - }, - "id": 173399, - "non_hive": { - "close": 50000, - "high": 3074, - "low": 12, - "open": 29715, - "volume": 5654087 - }, - "open": "2017-02-13T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 337499, - "high": 7, - "low": 15, - "open": 13000, - "volume": 42344041 - }, - "id": 173994, - "non_hive": { - "close": 45454, - "high": 1, - "low": 1, - "open": 1776, - "volume": 5675774 - }, - "open": "2017-02-14T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 89891, - "high": 7, - "low": 58, - "open": 15003, - "volume": 37555256 - }, - "id": 174746, - "non_hive": { - "close": 12000, - "high": 1, - "low": 7, - "open": 2026, - "volume": 5013483 - }, - "open": "2017-02-15T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 10167, - "high": 233956, - "low": 20, - "open": 74422, - "volume": 30416160 - }, - "id": 175379, - "non_hive": { - "close": 1412, - "high": 32861, - "low": 2, - "open": 9935, - "volume": 4090440 - }, - "open": "2017-02-16T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 134153, - "high": 305025, - "low": 33, - "open": 56381, - "volume": 58876105 - }, - "id": 175952, - "non_hive": { - "close": 18000, - "high": 44281, - "low": 4, - "open": 7830, - "volume": 8228802 - }, - "open": "2017-02-17T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 27914, - "high": 7, - "low": 120, - "open": 336338, - "volume": 72070545 - }, - "id": 176576, - "non_hive": { - "close": 3379, - "high": 1, - "low": 14, - "open": 45348, - "volume": 8935664 - }, - "open": "2017-02-18T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 32500, - "high": 7, - "low": 31, - "open": 336714, - "volume": 58294059 - }, - "id": 177460, - "non_hive": { - "close": 3901, - "high": 1, - "low": 3, - "open": 40972, - "volume": 7150676 - }, - "open": "2017-02-19T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 278846, - "high": 8, - "low": 47, - "open": 341533, - "volume": 47897437 - }, - "id": 178142, - "non_hive": { - "close": 32740, - "high": 1, - "low": 5, - "open": 40996, - "volume": 5632768 - }, - "open": "2017-02-20T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 489823, - "high": 332384, - "low": 36085, - "open": 34582, - "volume": 137289589 - }, - "id": 178635, - "non_hive": { - "close": 50000, - "high": 38925, - "low": 3654, - "open": 4049, - "volume": 14867149 - }, - "open": "2017-02-21T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 381954, - "high": 8, - "low": 64, - "open": 491145, - "volume": 151995667 - }, - "id": 179757, - "non_hive": { - "close": 43003, - "high": 1, - "low": 6, - "open": 50000, - "volume": 16044078 - }, - "open": "2017-02-22T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 6787, - "high": 7, - "low": 1991, - "open": 6682, - "volume": 143333781 - }, - "id": 180959, - "non_hive": { - "close": 793, - "high": 1, - "low": 217, - "open": 751, - "volume": 16780727 - }, - "open": "2017-02-23T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 390005, - "high": 8, - "low": 2236, - "open": 76, - "volume": 70236542 - }, - "id": 182160, - "non_hive": { - "close": 42701, - "high": 1, - "low": 241, - "open": 9, - "volume": 7934878 - }, - "open": "2017-02-24T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 427361, - "high": 8, - "low": 443634, - "open": 145690, - "volume": 66100626 - }, - "id": 182944, - "non_hive": { - "close": 50000, - "high": 1, - "low": 48395, - "open": 15979, - "volume": 7797410 - }, - "open": "2017-02-25T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 143636, - "high": 2088, - "low": 56, - "open": 115242, - "volume": 34958810 - }, - "id": 183657, - "non_hive": { - "close": 15983, - "high": 247, - "low": 6, - "open": 13518, - "volume": 4004270 - }, - "open": "2017-02-26T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 273375, - "high": 35, - "low": 23, - "open": 3960, - "volume": 70549139 - }, - "id": 184354, - "non_hive": { - "close": 31056, - "high": 4, - "low": 2, - "open": 439, - "volume": 7805362 - }, - "open": "2017-02-27T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 450171, - "high": 8, - "low": 107, - "open": 441296, - "volume": 75304460 - }, - "id": 184937, - "non_hive": { - "close": 48758, - "high": 1, - "low": 11, - "open": 50000, - "volume": 8407048 - }, - "open": "2017-02-28T00:00:00", - "seconds": 86400 - }, - { - "hive": { - "close": 464490, - "high": 9, - "low": 464490, - "open": 462878, - "volume": 74803622 - }, - "id": 185709, - "non_hive": { - "close": 46310, - "high": 1, - "low": 46310, - "open": 50000, - "volume": 7833519 - }, - "open": "2017-03-01T00:00:00", - "seconds": 86400 - } -] diff --git a/hived/tavern/condenser_api_patterns/get_market_history/86400.tavern.yaml b/hived/tavern/condenser_api_patterns/get_market_history/86400.tavern.yaml deleted file mode 100644 index 0b60d3f1e4d5f8f6435df276716f36a4d112619c..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history/86400.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_market_history - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_market_history - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_market_history" - params: [86400,"2016-03-01T00:00:00","2017-03-02T00:00:00"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "86400" - directory: "condenser_api_patterns/get_market_history" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_market_history_buckets/get.pat.json b/hived/tavern/condenser_api_patterns/get_market_history_buckets/get.pat.json deleted file mode 100644 index 301e0d2233539fc4cdf95afdb3bafe1b99cb5836..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history_buckets/get.pat.json +++ /dev/null @@ -1,7 +0,0 @@ -[ - 15, - 60, - 300, - 3600, - 86400 -] diff --git a/hived/tavern/condenser_api_patterns/get_market_history_buckets/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_market_history_buckets/get.tavern.yaml deleted file mode 100644 index 507af02a2b35ffc794448f236da43b7380f3fdb1..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_market_history_buckets/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_market_history_buckets - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_market_history_buckets - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_market_history_buckets" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_market_history_buckets" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_next_scheduled_hardfork/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_next_scheduled_hardfork/get.tavern.yaml deleted file mode 100644 index 33b252bda775c6d71833a1168ced90e168218cbb..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_next_scheduled_hardfork/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_next_scheduled_hardfork - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_next_scheduled_hardfork - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_next_scheduled_hardfork" - params: [] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_next_scheduled_hardfork" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_open_orders/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_open_orders/get.tavern.yaml deleted file mode 100644 index 89b6de9d7d482df0154c0973981040f34fbf8e2e..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_open_orders/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_open_orders - - marks: - - patterntest #todo find open orders to get ;) - - includes: - - !include ../../common.yaml - - stages: - - name: get_open_orders - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_open_orders" - params: ["steemit"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_open_orders" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_false.pat.json b/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_false.pat.json deleted file mode 100644 index 107c12922042004d31b5801786644d57eca00cf2..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_false.pat.json +++ /dev/null @@ -1,34 +0,0 @@ -[ - { - "block": 2797050, - "op": [ - "vote", - { - "author": "pfunk", - "permlink": "guide-maximize-your-mining-hashrate-in-windows-by-mining-steem-in-a-vm", - "voter": "gtg", - "weight": 10000 - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-30T19:12:21", - "trx_id": "e0cd24f9aba3b668b1443786bee3891f644ff23f", - "trx_in_block": 0, - "virtual_op": 0 - }, - { - "block": 2797050, - "op": [ - "producer_reward", - { - "producer": "roadscape", - "vesting_shares": "5400.959205 VESTS" - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-30T19:12:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } -] diff --git a/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_false.tavern.yaml b/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_false.tavern.yaml deleted file mode 100644 index c8a6dcdb5fb1c3bf22568049b078a13585b307bb..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_false.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_ops_in_block only virtual false - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_ops_in_block only virtual false - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_ops_in_block" - params: [2797050, false] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "only_virtual_false" - directory: "condenser_api_patterns/get_ops_in_block" diff --git a/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_true.pat.json b/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_true.pat.json deleted file mode 100644 index ee2c95ac40874ad7de2893db15da341d280586cd..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_true.pat.json +++ /dev/null @@ -1,17 +0,0 @@ -[ - { - "block": 2797050, - "op": [ - "producer_reward", - { - "producer": "roadscape", - "vesting_shares": "5400.959205 VESTS" - } - ], - "op_in_trx": 0, - "timestamp": "2016-06-30T19:12:24", - "trx_id": "0000000000000000000000000000000000000000", - "trx_in_block": 4294967295, - "virtual_op": 1 - } -] diff --git a/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_true.tavern.yaml b/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_true.tavern.yaml deleted file mode 100644 index 288e711539db776df89c0d0f54834c7b26c3981f..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_ops_in_block/only_virtual_true.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived account_history_api get_ops_in_block only virtual true - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: account_history_api get_ops_in_block only virtual true - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_ops_in_block" - params: [2797050, true] - response: - status_code: 200 - verify_response_with: - function: validate_response:compare_response_with_pattern - extra_kwargs: - method: "only_virtual_true" - directory: "condenser_api_patterns/get_ops_in_block" diff --git a/hived/tavern/condenser_api_patterns/get_order_book/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_order_book/get.tavern.yaml deleted file mode 100644 index 6aa39d1e49cd87ec1f6eedb5ae3811a5b3211590..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_order_book/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_order_book - - marks: - - patterntest - - includes: - - !include ../../common.yaml - - stages: - - name: get_order_book - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_order_book" - params: [500] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_order_book" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_owner_history/get.tavern.yaml b/hived/tavern/condenser_api_patterns/get_owner_history/get.tavern.yaml deleted file mode 100644 index 49ed950e0b179c288bf89b588c993e12da255f04..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_owner_history/get.tavern.yaml +++ /dev/null @@ -1,28 +0,0 @@ ---- - test_name: Hived get_owner_history - - marks: - - patterntest #todo find is there any owner history? - - includes: - - !include ../../common.yaml - - stages: - - name: get_owner_history - request: - url: "{service.url:s}" - method: POST - headers: - content-type: application/json - json: - jsonrpc: "2.0" - id: 1 - method: "condenser_api.get_owner_history" - params: ["gtg"] - response: - status_code: 200 - verify_response_with: - function: validate_response:has_valid_response - extra_kwargs: - method: "get" - directory: "condenser_api_patterns/get_owner_history" \ No newline at end of file diff --git a/hived/tavern/condenser_api_patterns/get_post_discussions_by_payout/smolalit.pat.json b/hived/tavern/condenser_api_patterns/get_post_discussions_by_payout/smolalit.pat.json deleted file mode 100644 index a712bf60f61aabb2d028d776b05d57816ff679a3..0000000000000000000000000000000000000000 --- a/hived/tavern/condenser_api_patterns/get_post_discussions_by_payout/smolalit.pat.json +++ /dev/null @@ -1,44014 +0,0 @@ -[ - { - "active_votes": [ - { - "percent": "10000", - "reputation": 520861791056580, - "rshares": 164139801366903, - "voter": "blocktrades" - }, - { - "percent": "4000", - "reputation": 341233778618, - "rshares": 626943807301, - "voter": "boatymcboatface" - }, - { - "percent": "4000", - "reputation": 1957220358850383, - "rshares": 1548611533291, - "voter": "kingscrown" - }, - { - "percent": "4000", - "reputation": 4509451541223, - "rshares": 168325128890, - "voter": "theshell" - }, - { - "percent": "1000", - "reputation": 109424005488868, - "rshares": 54240958922, - "voter": "mammasitta" - }, - { - "percent": "10000", - "reputation": 159372103844946, - "rshares": 21825427759754, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 591298160171898, - "rshares": 1461457299543, - "voter": "good-karma" - }, - { - "percent": "10000", - "reputation": 315496990256842, - "rshares": 5619989839379, - "voter": "arcange" - }, - { - "percent": "5000", - "reputation": 315247082036226, - "rshares": 1036053933654, - "voter": "lichtblick" - }, - { - "percent": "300", - "reputation": 501315151365, - "rshares": 3249055439, - "voter": "raphaelle" - }, - { - "percent": "800", - "reputation": 560943007638090, - "rshares": 291420955651, - "voter": "ace108" - }, - { - "percent": "10000", - "reputation": 266498838207861, - "rshares": 1189083640441, - "voter": "timcliff" - }, - { - "percent": "10000", - "reputation": 8868395123281, - "rshares": 6857123935175, - "voter": "jphamer1" - }, - { - "percent": "9900", - "reputation": 3434871602070, - "rshares": 37207990222, - "voter": "fooblic" - }, - { - "percent": "10000", - "reputation": 30591567767954, - "rshares": 73631823285, - "voter": "the-bitcoin-dood" - }, - { - "percent": "8400", - "reputation": 209327783268279, - "rshares": 179095965900, - "voter": "foxkoit" - }, - { - "percent": "2000", - "reputation": 1087858767216, - "rshares": 184997806, - "voter": "micefy" - }, - { - "percent": "10000", - "reputation": 25231292536535, - "rshares": 274205878456, - "voter": "chrisaiki" - }, - { - "percent": "1900", - "reputation": 49426223217225, - "rshares": 25835491503, - "voter": "phusionphil" - }, - { - "percent": "750", - "reputation": 144108882515293, - "rshares": 60654313702, - "voter": "petrvl" - }, - { - "percent": "3610", - "reputation": 85981642650865, - "rshares": 41014273037, - "voter": "seckorama" - }, - { - "percent": "2000", - "reputation": 280674585514224, - "rshares": 188790835574, - "voter": "justyy" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 40775125861, - "voter": "ssekulji" - }, - { - "percent": "5000", - "reputation": 111778832002739, - "rshares": 283118506033, - "voter": "techslut" - }, - { - "percent": "10000", - "reputation": 420443679514793, - "rshares": 1727422057043, - "voter": "esteemapp" - }, - { - "percent": "5000", - "reputation": 22050619475702, - "rshares": 28430983451, - "voter": "wagnertamanaha" - }, - { - "percent": "10000", - "reputation": 306546327230906, - "rshares": 17044093581, - "voter": "teamhumble" - }, - { - "percent": "3500", - "reputation": 0, - "rshares": 3436139883224, - "voter": "newhope" - }, - { - "percent": "10000", - "reputation": 96057608537550, - "rshares": 74050746878, - "voter": "kotturinn" - }, - { - "percent": "10000", - "reputation": 30904254166753, - "rshares": 733694717208, - "voter": "distantsignal" - }, - { - "percent": "300", - "reputation": 38975615169260, - "rshares": 7642834007, - "voter": "steemitboard" - }, - { - "percent": "10000", - "reputation": 36214470543162, - "rshares": 18404635328, - "voter": "assasin" - }, - { - "percent": "10000", - "reputation": 43129757389335, - "rshares": 8764346415, - "voter": "sudutpandang" - }, - { - "percent": "10000", - "reputation": 10514448764402, - "rshares": 1645122623, - "voter": "syarrf" - }, - { - "percent": "10000", - "reputation": 48268764621908, - "rshares": 80462681008, - "voter": "fingersik" - }, - { - "percent": "3000", - "reputation": 288303313542379, - "rshares": 175294413888, - "voter": "melinda010100" - }, - { - "percent": "10000", - "reputation": 16365587743468, - "rshares": 121605486661, - "voter": "tamaralovelace" - }, - { - "percent": "10000", - "reputation": 29513750536324, - "rshares": 6106421501, - "voter": "kennyroy" - }, - { - "percent": "10000", - "reputation": 11555767777330, - "rshares": 34973669996, - "voter": "thethreehugs" - }, - { - "percent": "10000", - "reputation": 103190531489536, - "rshares": 171612994642, - "voter": "ayijufridar" - }, - { - "percent": "7500", - "reputation": 26275815650202, - "rshares": 52814098408, - "voter": "trumpikas" - }, - { - "percent": "10000", - "reputation": 112994806497327, - "rshares": 2862282091, - "voter": "varunpinto" - }, - { - "percent": "5000", - "reputation": 80123076461352, - "rshares": 396699780148, - "voter": "xels" - }, - { - "percent": "10000", - "reputation": 206759412552, - "rshares": 112522039371, - "voter": "tomaszs77" - }, - { - "percent": "6300", - "reputation": 11503516163103, - "rshares": 30078542082, - "voter": "mdosev" - }, - { - "percent": "750", - "reputation": 3553102205525, - "rshares": 10485097361, - "voter": "belahejna" - }, - { - "percent": "10000", - "reputation": 7901101123497, - "rshares": 1110824554565, - "voter": "giuatt07" - }, - { - "percent": "2900", - "reputation": 19643697626632, - "rshares": 119543567578, - "voter": "sam99" - }, - { - "percent": "10000", - "reputation": 20355801093011, - "rshares": 490784838060, - "voter": "jingdol" - }, - { - "percent": "5000", - "reputation": 494228895348, - "rshares": 773006596, - "voter": "saintopic" - }, - { - "percent": "10000", - "reputation": 219366237221002, - "rshares": 579935195725, - "voter": "offgridlife" - }, - { - "percent": "9600", - "reputation": 59746720810341, - "rshares": 43536615066, - "voter": "simaroy" - }, - { - "percent": "10000", - "reputation": 29540199236425, - "rshares": 3763727982154, - "voter": "eturnerx" - }, - { - "percent": "10000", - "reputation": 29666985623497, - "rshares": 87307148792, - "voter": "vallesleoruther" - }, - { - "percent": "10000", - "reputation": 223486867868, - "rshares": 18762679709, - "voter": "captainquack22" - }, - { - "percent": "3000", - "reputation": 115269542036380, - "rshares": 397298299519, - "voter": "vikisecrets" - }, - { - "percent": "5000", - "reputation": 1844447662772, - "rshares": 13433650020, - "voter": "khalil319" - }, - { - "percent": "4930", - "reputation": 31580212794880, - "rshares": 3745247664, - "voter": "ahlawat" - }, - { - "percent": "4000", - "reputation": 453941972104547, - "rshares": 341767665503, - "voter": "nainaztengra" - }, - { - "percent": "10000", - "reputation": 81836455418258, - "rshares": 89247513590, - "voter": "noboxes" - }, - { - "percent": "10000", - "reputation": 24639928209538, - "rshares": 57182695174, - "voter": "santigs" - }, - { - "percent": "500", - "reputation": 149085530853696, - "rshares": 106516376405, - "voter": "karja" - }, - { - "percent": "10000", - "reputation": 196571377648, - "rshares": 18495791759, - "voter": "nadhora" - }, - { - "percent": "1000", - "reputation": 51994511985595, - "rshares": 4011556510857, - "voter": "tipu" - }, - { - "percent": "10000", - "reputation": 537290602112, - "rshares": 271999751945, - "voter": "chinchilla" - }, - { - "percent": "10000", - "reputation": 110750156547770, - "rshares": 535519919057, - "voter": "drax" - }, - { - "percent": "1475", - "reputation": 0, - "rshares": 34140254931, - "voter": "tomiscurious" - }, - { - "percent": "10000", - "reputation": 1356757205252, - "rshares": 1590452290, - "voter": "imawreader" - }, - { - "percent": "10000", - "reputation": 47587090795963, - "rshares": 30141445179, - "voter": "szabolcs" - }, - { - "percent": "845", - "reputation": 0, - "rshares": 340211275776, - "voter": "investegg" - }, - { - "percent": "10000", - "reputation": 57597252449855, - "rshares": 460190544871, - "voter": "florian-glechner" - }, - { - "percent": "10000", - "reputation": 128158005695390, - "rshares": 63536401922, - "voter": "arabisouri" - }, - { - "percent": "10000", - "reputation": 1436962620884, - "rshares": 1004372737, - "voter": "mrsyria" - }, - { - "percent": "10000", - "reputation": 770453535542, - "rshares": 2413646233, - "voter": "bangjuh" - }, - { - "percent": "10000", - "reputation": 1807139565916, - "rshares": 113762333, - "voter": "irynochka" - }, - { - "percent": "10000", - "reputation": 61297887349807, - "rshares": 22248309781, - "voter": "nathen007" - }, - { - "percent": "5000", - "reputation": 1143658933344, - "rshares": 710889591, - "voter": "machroezar" - }, - { - "percent": "10000", - "reputation": 43698921303676, - "rshares": 47308382843, - "voter": "afril" - }, - { - "percent": "10000", - "reputation": 2902161253893, - "rshares": 3662317342, - "voter": "mamaloves" - }, - { - "percent": "5000", - "reputation": 14337364044156, - "rshares": 6682767507, - "voter": "tomatom" - }, - { - "percent": "2500", - "reputation": 2675225025765, - "rshares": 7295732274, - "voter": "fourfourfun" - }, - { - "percent": "10000", - "reputation": 112593705937795, - "rshares": 177700627854, - "voter": "apnigrich" - }, - { - "percent": "3000", - "reputation": 417897915586235, - "rshares": 219740165641, - "voter": "phortun" - }, - { - "percent": "10000", - "reputation": 6721086978707, - "rshares": 7226173336, - "voter": "ronpurteetv" - }, - { - "percent": "2000", - "reputation": 87246032649758, - "rshares": 30590741583, - "voter": "gabrielatravels" - }, - { - "percent": "1000", - "reputation": 169520027942230, - "rshares": 9190823597, - "voter": "r351574nc3" - }, - { - "percent": "10000", - "reputation": 114122304547286, - "rshares": 223797236303, - "voter": "iamjadeline" - }, - { - "percent": "500", - "reputation": 21720371357335, - "rshares": 2366418146, - "voter": "bartheek" - }, - { - "percent": "8500", - "reputation": 14084205914169, - "rshares": 31093183201, - "voter": "kiwibloke" - }, - { - "percent": "10000", - "reputation": 7833564344722, - "rshares": 2179632784, - "voter": "ahmedsy" - }, - { - "percent": "10000", - "reputation": 170235537022, - "rshares": 1410422723, - "voter": "auracraft" - }, - { - "percent": "1900", - "reputation": 8003119161188, - "rshares": 3967900550, - "voter": "brewery" - }, - { - "percent": "5000", - "reputation": 330396781271113, - "rshares": 2331710454539, - "voter": "holger80" - }, - { - "percent": "2000", - "reputation": 50124640567504, - "rshares": 7510110487, - "voter": "dailychina" - }, - { - "percent": "5000", - "reputation": 442944056818, - "rshares": 1894655279, - "voter": "minerspost" - }, - { - "percent": "10000", - "reputation": 344804463734258, - "rshares": 21316593878, - "voter": "dirapa" - }, - { - "percent": "500", - "reputation": 411588240625, - "rshares": 330267804, - "voter": "happy-soul" - }, - { - "percent": "9500", - "reputation": 4819938972371, - "rshares": 7100523922, - "voter": "green77" - }, - { - "percent": "10000", - "reputation": 12435393608248, - "rshares": 15428822393, - "voter": "jhoxiris" - }, - { - "percent": "10000", - "reputation": 98135484010, - "rshares": 9403076199, - "voter": "dasc" - }, - { - "percent": "1500", - "reputation": 17846302258319, - "rshares": 6383147020, - "voter": "onlavu" - }, - { - "percent": "6500", - "reputation": 6264286399143, - "rshares": 1949178207646, - "voter": "asgarth" - }, - { - "percent": "10000", - "reputation": 4418427243653, - "rshares": 6056636429, - "voter": "memesdaily" - }, - { - "percent": "9900", - "reputation": 3065535471122, - "rshares": 4629977913, - "voter": "mutiahanum" - }, - { - "percent": "10000", - "reputation": 62406194635091, - "rshares": 38279635540, - "voter": "russellstockley" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 29772086599, - "voter": "futurecurrency" - }, - { - "percent": "4000", - "reputation": 93067317204510, - "rshares": 70091827906, - "voter": "almi" - }, - { - "percent": "10000", - "reputation": 21188653353975, - "rshares": 145459795324, - "voter": "erikklok" - }, - { - "percent": "10000", - "reputation": 1172852327717, - "rshares": 226645045886, - "voter": "watchlist" - }, - { - "percent": "5000", - "reputation": 2256065348315, - "rshares": 8397420830, - "voter": "frassman" - }, - { - "percent": "10000", - "reputation": 9839458481068, - "rshares": 23658641802, - "voter": "kryptik.tigrrr3d" - }, - { - "percent": "9000", - "reputation": 541369096879, - "rshares": 8144822661, - "voter": "yusrizakaria" - }, - { - "percent": "10000", - "reputation": 15649272505736, - "rshares": 29638355866, - "voter": "lionsuit" - }, - { - "percent": "10000", - "reputation": 274229697842, - "rshares": 20138127862, - "voter": "abwasserrohr" - }, - { - "percent": "10000", - "reputation": 315987272518746, - "rshares": 214361230704, - "voter": "jeronimorubio" - }, - { - "percent": "1000", - "reputation": 10250642778719, - "rshares": 3160648924, - "voter": "salty-mcgriddles" - }, - { - "percent": "10000", - "reputation": 108726560523456, - "rshares": 382059986921, - "voter": "immanuel94" - }, - { - "percent": "10000", - "reputation": 8107441442652, - "rshares": 10478353262, - "voter": "deltasteem" - }, - { - "percent": "10000", - "reputation": 2059690236788, - "rshares": 18211370706, - "voter": "flyerchen" - }, - { - "percent": "10000", - "reputation": 85422666406, - "rshares": 10958499915, - "voter": "skyroad" - }, - { - "percent": "10000", - "reputation": 11722167805732, - "rshares": 30341297043, - "voter": "phasewalker" - }, - { - "percent": "5000", - "reputation": 112109667795, - "rshares": 1508359174, - "voter": "memepress" - }, - { - "percent": "10000", - "reputation": 37835744295387, - "rshares": 8737699955, - "voter": "gogreenbuddy" - }, - { - "percent": "10000", - "reputation": 2481629111221, - "rshares": 2285171223, - "voter": "manojbhatt" - }, - { - "percent": "5000", - "reputation": 1705998268893, - "rshares": 975259617, - "voter": "dodolzk" - }, - { - "percent": "10000", - "reputation": 23720177528186, - "rshares": 31092988731, - "voter": "wirago" - }, - { - "percent": "9900", - "reputation": 1127084830607, - "rshares": 3246896521, - "voter": "irwandarasyid" - }, - { - "percent": "10000", - "reputation": 9020628089607, - "rshares": 87837595401, - "voter": "promobot" - }, - { - "percent": "3000", - "reputation": 25665583431709, - "rshares": 51349533741, - "voter": "rozku" - }, - { - "percent": "1700", - "reputation": 13183527861245, - "rshares": 4526992893, - "voter": "celinavisaez" - }, - { - "percent": "2200", - "reputation": 51236835710173, - "rshares": 10125360314, - "voter": "davidesimoncini" - }, - { - "percent": "10000", - "reputation": 16608311881044, - "rshares": 47978835354, - "voter": "curatorcat" - }, - { - "percent": "5000", - "reputation": 108486108034648, - "rshares": 43124190353, - "voter": "muelli" - }, - { - "percent": "10000", - "reputation": 1849208884723, - "rshares": 1969785589, - "voter": "mulawarman" - }, - { - "percent": "10000", - "reputation": 2634900617603, - "rshares": 3895592213, - "voter": "vampire-steem" - }, - { - "percent": "10000", - "reputation": 63239211262012, - "rshares": 89423480679, - "voter": "marblely" - }, - { - "percent": "250", - "reputation": 20023805487967, - "rshares": 834610338, - "voter": "moneybaby" - }, - { - "percent": "1000", - "reputation": 599567348573, - "rshares": 484543730, - "voter": "exifr" - }, - { - "percent": "250", - "reputation": 27522752785541, - "rshares": 1235048920, - "voter": "longer" - }, - { - "percent": "10000", - "reputation": 495132113427, - "rshares": 566004132, - "voter": "cowboyup" - }, - { - "percent": "2300", - "reputation": 33860216986858, - "rshares": 138549040602, - "voter": "donald.porter" - }, - { - "percent": "10000", - "reputation": 2207289544318, - "rshares": 34239083822, - "voter": "amigareaction" - }, - { - "percent": "5000", - "reputation": 3211710745138, - "rshares": 2504064464, - "voter": "yayan" - }, - { - "percent": "10000", - "reputation": 12686175479867, - "rshares": 23938258784, - "voter": "steem.craft" - }, - { - "percent": "1000", - "reputation": 116804559, - "rshares": 578750584, - "voter": "exifr0" - }, - { - "percent": "5000", - "reputation": 1204818316663, - "rshares": 934129211, - "voter": "ekafao" - }, - { - "percent": "10000", - "reputation": 62302307783, - "rshares": 28776625851, - "voter": "pvinny69" - }, - { - "percent": "5000", - "reputation": 347950223775, - "rshares": 4239610443, - "voter": "minnowspeed" - }, - { - "percent": "5000", - "reputation": 36498710441788, - "rshares": 17184114348, - "voter": "fullnodeupdate" - }, - { - "percent": "10000", - "reputation": 3358406403537, - "rshares": 432105926288, - "voter": "fijimermaid" - }, - { - "percent": "10000", - "reputation": 133998446537867, - "rshares": 5578565595, - "voter": "bluengel" - }, - { - "percent": "10000", - "reputation": 10278138043955, - "rshares": 84599022, - "voter": "laissez-faire" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 12742536278, - "voter": "quediceharry" - }, - { - "percent": "10000", - "reputation": 13238201387755, - "rshares": 80543371623, - "voter": "stefano.massari" - }, - { - "percent": "5000", - "reputation": 3816319637111, - "rshares": 1550097916, - "voter": "jackofcrows" - }, - { - "percent": "10000", - "reputation": 7593689306652, - "rshares": 98657846433387, - "voter": "encrypt3dbr0k3r" - }, - { - "percent": "10000", - "reputation": 1648086760085, - "rshares": 30963297658, - "voter": "diegor" - }, - { - "percent": "10000", - "reputation": 13222548277758, - "rshares": 288796167910, - "voter": "wil.metcalfe" - }, - { - "percent": "6000", - "reputation": 11213150975739, - "rshares": 135912371708, - "voter": "kristall97" - }, - { - "percent": "3000", - "reputation": 94595445913731, - "rshares": 373920356762, - "voter": "glastar" - }, - { - "percent": "10000", - "reputation": 5493311683937, - "rshares": 106025830971, - "voter": "kekos" - }, - { - "percent": "10000", - "reputation": 1125763140050, - "rshares": 3787032200, - "voter": "maajaanaa" - }, - { - "percent": "10000", - "reputation": 309500689901, - "rshares": 733120711, - "voter": "mariyem" - }, - { - "percent": "500", - "reputation": 86443203025994, - "rshares": 2244822543, - "voter": "jacuzzi" - }, - { - "percent": "5000", - "reputation": 4352592902254, - "rshares": 1013022165, - "voter": "teamawesome" - }, - { - "percent": "10000", - "reputation": 13586627169473, - "rshares": 18026411503, - "voter": "marianomariano" - }, - { - "percent": "10000", - "reputation": 27966845679308, - "rshares": 81496022272, - "voter": "e-r-k-a-n" - }, - { - "percent": "10000", - "reputation": 216020035070, - "rshares": 23781460500, - "voter": "agolasol" - }, - { - "percent": "5000", - "reputation": 215729406529, - "rshares": 251025815, - "voter": "yingtaodaren" - }, - { - "percent": "4000", - "reputation": 16012295789643, - "rshares": 3803926079, - "voter": "cooperfelix" - }, - { - "percent": "1000", - "reputation": 9483360545909, - "rshares": 1241094160, - "voter": "mia-cc" - }, - { - "percent": "5000", - "reputation": 5616865862, - "rshares": 11035703975, - "voter": "abbenay" - }, - { - "percent": "10000", - "reputation": 2910555091868, - "rshares": 964442825, - "voter": "denizcakmak" - }, - { - "percent": "10000", - "reputation": 925135168509, - "rshares": 486204220, - "voter": "marymi" - }, - { - "percent": "1000", - "reputation": 69910542366759, - "rshares": 48954725964, - "voter": "kryptogames" - }, - { - "percent": "10000", - "reputation": 469728104061, - "rshares": 1143383282, - "voter": "cindis" - }, - { - "percent": "10000", - "reputation": 1481460968819, - "rshares": 1173731108, - "voter": "linur" - }, - { - "percent": "10000", - "reputation": 8977413850563, - "rshares": 23799728890, - "voter": "maryincryptoland" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 0, - "voter": "tenyears" - }, - { - "percent": "10000", - "reputation": 4034609326936, - "rshares": 24925477209, - "voter": "saxx1304" - }, - { - "percent": "10000", - "reputation": 61476958391321, - "rshares": 5116279074, - "voter": "ricardomello" - }, - { - "percent": "10000", - "reputation": 29888557634290, - "rshares": 22341483558, - "voter": "helgalubevi" - }, - { - "percent": "10000", - "reputation": 2179800525681, - "rshares": 815918545, - "voter": "ladeen" - }, - { - "percent": "2500", - "reputation": 38385505883708, - "rshares": 98024177093, - "voter": "cezary-io" - }, - { - "percent": "10000", - "reputation": 36087507440149, - "rshares": 862671585401, - "voter": "likwid" - }, - { - "percent": "5000", - "reputation": 735428674412, - "rshares": 7235280421, - "voter": "sor31" - }, - { - "percent": "10000", - "reputation": 499450701404, - "rshares": 532360501, - "voter": "iktisat" - }, - { - "percent": "750", - "reputation": 0, - "rshares": 1444869138, - "voter": "cryptogambit" - }, - { - "percent": "2200", - "reputation": 50073588499121, - "rshares": 38363067820, - "voter": "golden.future" - }, - { - "percent": "10000", - "reputation": 10026344348529, - "rshares": 588420435, - "voter": "alexvalera" - }, - { - "percent": "10000", - "reputation": 165757662725, - "rshares": 3149752009, - "voter": "lrekt01" - }, - { - "percent": "2000", - "reputation": 154281807627727, - "rshares": 38317188386, - "voter": "iamraincrystal" - }, - { - "percent": "5000", - "reputation": 1704173681552, - "rshares": 1111101757, - "voter": "bilpcoinbot" - }, - { - "percent": "750", - "reputation": 17687551130249, - "rshares": 914106419, - "voter": "bcm" - }, - { - "percent": "1750", - "reputation": 3257555962879, - "rshares": 910218813, - "voter": "bilpcoinrecords" - }, - { - "percent": "1500", - "reputation": 2754871650749, - "rshares": 3631592430, - "voter": "dappstats" - }, - { - "percent": "1180", - "reputation": 20606238744, - "rshares": 0, - "voter": "leviet7490" - }, - { - "percent": "9200", - "reputation": 108134629920, - "rshares": 2184275967, - "voter": "dexy50" - }, - { - "percent": "10000", - "reputation": 9783009514092, - "rshares": 290955148239, - "voter": "sharkthelion" - }, - { - "percent": "10000", - "reputation": 1316380362597, - "rshares": 2800355956, - "voter": "neoado" - }, - { - "percent": "10000", - "reputation": 786619143592, - "rshares": 22821342, - "voter": "cmdd" - }, - { - "percent": "10000", - "reputation": 336154498730, - "rshares": 6478635306, - "voter": "marblesz" - }, - { - "percent": "5000", - "reputation": 32542453022, - "rshares": 5087282477, - "voter": "bilpcoinbpc" - }, - { - "percent": "10000", - "reputation": 76222060731, - "rshares": 1211872847, - "voter": "wallytiteuf" - }, - { - "percent": "5000", - "reputation": 30837223420968, - "rshares": 7645183705, - "voter": "drew0" - }, - { - "percent": "9000", - "reputation": 15614014721682, - "rshares": 5785449434, - "voter": "cornavirus" - }, - { - "percent": "10000", - "reputation": 362096342263, - "rshares": 503075702, - "voter": "sujaytechnicals" - }, - { - "percent": "2800", - "reputation": 7921327410319, - "rshares": 6282246094, - "voter": "euc" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 535751563080, - "voter": "fengchao" - }, - { - "percent": "3300", - "reputation": 3242356575612, - "rshares": 6715278124, - "voter": "walarhein" - }, - { - "percent": "990", - "reputation": 10364363981695, - "rshares": 522371489672, - "voter": "visionaer3003" - }, - { - "percent": "10000", - "reputation": 1209731647511, - "rshares": 1713189201243, - "voter": "softworld" - }, - { - "percent": "10000", - "reputation": 7288245429962, - "rshares": 3191904433, - "voter": "ghastlygames" - }, - { - "percent": "10000", - "reputation": 17500919200979, - "rshares": 58481842132, - "voter": "arlettemsalase" - }, - { - "percent": "10000", - "reputation": 679455507132, - "rshares": 501734030, - "voter": "hjchilb" - }, - { - "percent": "10000", - "reputation": 4274979461460, - "rshares": 45816792310, - "voter": "neon3000" - }, - { - "percent": "10000", - "reputation": 5277345693920, - "rshares": 1602903370, - "voter": "andrewmusic" - }, - { - "percent": "1000", - "reputation": 604442758770, - "rshares": 44917129454, - "voter": "ninnu" - }, - { - "percent": "10000", - "reputation": 56328328383994, - "rshares": 6150021183130, - "voter": "ecency" - }, - { - "percent": "10000", - "reputation": 37908486627936, - "rshares": 35663871793, - "voter": "ykroys" - }, - { - "percent": "10000", - "reputation": 4389470907154, - "rshares": 4378385985, - "voter": "musicua" - }, - { - "percent": "5000", - "reputation": 13758538330601, - "rshares": 6363164405, - "voter": "reza-shamim" - }, - { - "percent": "970", - "reputation": 4358352640716, - "rshares": 253771658015, - "voter": "hatschi0773" - }, - { - "percent": "1000", - "reputation": 0, - "rshares": 57987914623, - "voter": "hivecur2" - }, - { - "percent": "5000", - "reputation": 10230005925375, - "rshares": 14697227352, - "voter": "issymarie2" - }, - { - "percent": "10000", - "reputation": 2830639099232, - "rshares": 268212102, - "voter": "koxmicart" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 36008411783, - "voter": "gohive" - }, - { - "percent": "10000", - "reputation": 22143037222260, - "rshares": 21438646069, - "voter": "marytp20" - }, - { - "percent": "10000", - "reputation": 4399967431494, - "rshares": 3111640598, - "voter": "epilatero" - }, - { - "percent": "10000", - "reputation": 3005998414144, - "rshares": 3134560924, - "voter": "kraken99" - }, - { - "percent": "4000", - "reputation": 2662893985125, - "rshares": 8292357082, - "voter": "chaodietas" - }, - { - "percent": "2000", - "reputation": 0, - "rshares": 2964434060963, - "voter": "usainvote" - }, - { - "percent": "980", - "reputation": 3174507364780, - "rshares": 369733311283, - "voter": "dagobert007" - }, - { - "percent": "10000", - "reputation": 6260561866202, - "rshares": 195464877727, - "voter": "idea-make-rich" - }, - { - "percent": "3321", - "reputation": 16307549034965, - "rshares": 7109940804, - "voter": "trangbaby" - }, - { - "percent": "2500", - "reputation": 3679705780578, - "rshares": 3817736063, - "voter": "senseiphil" - }, - { - "percent": "200", - "reputation": 0, - "rshares": 23960036407, - "voter": "intacto" - }, - { - "percent": "10000", - "reputation": 5167918, - "rshares": 0, - "voter": "vionline" - }, - { - "percent": "10000", - "reputation": 86405531104, - "rshares": 1954083117, - "voter": "text2speech" - }, - { - "percent": "10000", - "reputation": 112363590278, - "rshares": 49453797, - "voter": "dango1411" - }, - { - "percent": "10000", - "reputation": 974130034782, - "rshares": 4560308528, - "voter": "aiovo" - }, - { - "percent": "1000", - "reputation": 434627240, - "rshares": 15721660916, - "voter": "boneym" - }, - { - "percent": "10000", - "reputation": 1523084393, - "rshares": 9982017, - "voter": "osiri" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "larotayogaming" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 182791827, - "voter": "braveonlines" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "sameer78" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 60260772760, - "voter": "betterdev" - }, - { - "percent": "10000", - "reputation": 3594869451, - "rshares": 0, - "voter": "mythcrusher" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "arlier1" - } - ], - "author": "ecency", - "author_reputation": 56328328383994, - "beneficiaries": [], - "body": "Over last 2 weeks, we have pushed 2 more new updates to Ecency mobile app making it faster and better with recent Hardfork of the network. Many pages got performance boosts and are free from different bugs.\nIn this post we will talk about some of these changes.\n\n<center>\n\n</center>\n\n## What's new\n\n- Feed refresh improved - some Android devices struggled on startup to load feed and required Pull down to refresh action unnecessarily, so we fixed that.\n- Timeout increase on node connections - nodes have had issues after recent hardfork and we noticed a lot of timeouts, improved connection to help minimize such instances.\n- Fix editor options menu title - small cosmetic fixes on title.\n- Fix for loading more posts issue - infinite post loading is crucial for a lot of people to be able to check posts in their community and feed, having small bug there was causing issues, we addressed that in this release.\n- Fix for api failover and threshold increase - node connection failover threshold is increased to help unstable mobile connection to retry.\n- Posts cards improvements - some design improvements\n- Turkish and Indonesian languages order fix - thanks to our awesome detail oriented users, language order was mixed up, we have corrected it.\n- Error screen improvements - error screen has bit more appealing view.\n- Fix vote registering issue - vote registering wasn't working as expected after hardfork, fixed that as well.\n- Fix communities page crash - in some instances communities page was crashing, we have improved that pages.\n- Fix commenting and posting issue - new editor format handling and fixes\n- Profile page improvements - profile page was also improved with better performance tweaks.\n- Fix pointing and wallet pages transaction history - transaction history now using new api endpoint.\n- Replies fix - profile page replies tab was not loading always due to some bug, now fixed.\n- Reputation fix - when user reputation comes in converted format, previously we had to convert to readable format.\n- Update to all Languages\n- AppStore and PlayStore listing screens updated\n\nIf you are React Native developer, feel free to join and help developing features we all love using, [our mobile application is opensource](https://github.com/ecency/ecency-mobile). \n \n**Looking for Ecency related footers? Check here examples awesome footers:** https://ecency.com/ocd/@dunsky/footers-for-ecency-lovers \n \n<center>\n\n</center>\n \nStay tuned, stay excited, stay united! Don't forget to share news with your friends. \n \n### https://ecency.com \n##### iOS https://ios.ecency.com \n##### Android https://android.ecency.com \n##### Desktop https://desktop.ecency.com\n\n<center>`hello@ecency.com` \n\ud83c\udf10[`Ecency.com`](https://ecency.com) | \u270d\ud83c\udffb [`Telegram`](https://t.me/ecency) | \ud83d\udcac[`Discord`](https://discord.me/ecency) \n[`Approve Hivesigner`](https://hivesigner.com/sign/update-proposal-votes?proposal_ids=%5B88%5D&approve=true) | [`Approve Hivesearcher`](https://hivesigner.com/sign/update-proposal-votes?proposal_ids=%5B114%5D&approve=true) \n[](https://hivesigner.com/sign/account-witness-vote?witness=good-karma&approve=1) \n</center>", - "body_length": 3565, - "cashout_time": "2020-11-06T03:43:36", - "category": "hive-125125", - "children": 26, - "created": "2020-10-30T03:43:36", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"app\":\"ecency/3.0.7-vision\",\"format\":\"markdown+html\",\"image\":[\"https://images.ecency.com/DQmUU6ZPhQ9PJNtGzPsxMFFTSArXLx2yVcbuxJ4ZT4aYYoT/mobile_release309.png\",\"https://images.ecency.com/DQmcWV77JEu58vUcarxwFgKzyufKbTiw88FM922exvHsN84/featured_image.jpg\"],\"links\":[\"https://github.com/ecency/ecency-mobile\",\"https://ecency.com/ocd/@dunsky/footers-for-ecency-lovers\",\"https://ecency.com\",\"https://ios.ecency.com\",\"https://android.ecency.com\",\"https://desktop.ecency.com\",\"https://ecency.com\",\"https://t.me/ecency\",\"https://discord.me/ecency\",\"https://hivesigner.com/sign/update-proposal-votes?proposal_ids=%5B88%5D&approve=true\",\"https://hivesigner.com/sign/update-proposal-votes?proposal_ids=%5B114%5D&approve=true\",\"https://images.ecency.com/p/o1AJ9qDyyJNSpZWhUgGYc3MngFqoAN2qn9AiTn8UpLP6Qb5TL?format=match&mode=fit\",\"https://hivesigner.com/sign/account-witness-vote?witness=good-karma&approve=1\"],\"tags\":[\"hive-125125\",\"bitcoin\",\"ecency\",\"mobile\",\"hive\",\"dapp\",\"wallet\"],\"users\":[\"good-karma\"]}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-10-30T11:56:03", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 348304346209276, - "parent_author": "", - "parent_permlink": "hive-125125", - "pending_payout_value": "82.454 HBD", - "percent_hbd": 10000, - "permlink": "fast-surfing-with-ecency-mobile-app", - "post_id": 100313067, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Fast surfing with Ecency mobile app", - "title": "Fast surfing with Ecency mobile app", - "total_payout_value": "0.000 HBD", - "url": "/hive-125125/@ecency/fast-surfing-with-ecency-mobile-app" - }, - { - "active_votes": [ - { - "percent": "10000", - "reputation": 99636215095482, - "rshares": 1176397150175, - "voter": "dan" - }, - { - "percent": "1190", - "reputation": 1049281228830, - "rshares": 2281745691176, - "voter": "enki" - }, - { - "percent": "918", - "reputation": 19245698180508, - "rshares": 199307697060, - "voter": "tombstone" - }, - { - "percent": "1000", - "reputation": 211696269298912, - "rshares": 800145770892, - "voter": "pfunk" - }, - { - "percent": "10000", - "reputation": 192454047339929, - "rshares": 455968387058, - "voter": "ervin-lemark" - }, - { - "percent": "10000", - "reputation": 30111821406182, - "rshares": 663095542379, - "voter": "bleepcoin" - }, - { - "percent": "10000", - "reputation": 351157717865710, - "rshares": 1977464746345, - "voter": "onealfa" - }, - { - "percent": "3000", - "reputation": 1594304819447449, - "rshares": 3045528796072, - "voter": "acidyo" - }, - { - "percent": "5100", - "reputation": 506372806974428, - "rshares": 4496657215491, - "voter": "kevinwong" - }, - { - "percent": "5000", - "reputation": 42689564397220, - "rshares": 350334496900, - "voter": "kenny-crane" - }, - { - "percent": "64", - "reputation": 109424005488868, - "rshares": 3475890839, - "voter": "mammasitta" - }, - { - "percent": "300", - "reputation": 20148016993603, - "rshares": 52177380336, - "voter": "gerber" - }, - { - "percent": "10000", - "reputation": 60346510542088, - "rshares": 35313465223, - "voter": "artem-sokoloff" - }, - { - "percent": "649", - "reputation": 591298160171898, - "rshares": 97426934252, - "voter": "good-karma" - }, - { - "percent": "800", - "reputation": 68491352821982, - "rshares": 52486480227, - "voter": "daan" - }, - { - "percent": "300", - "reputation": 639040633115794, - "rshares": 201332020035, - "voter": "ezzy" - }, - { - "percent": "10000", - "reputation": 9763982600833, - "rshares": 24706570144, - "voter": "rxhector" - }, - { - "percent": "10000", - "reputation": 229982756480919, - "rshares": 2988155372506, - "voter": "ausbitbank" - }, - { - "percent": "2550", - "reputation": 86171006185191, - "rshares": 10060173393, - "voter": "mrwang" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 276855291307, - "voter": "livingfree" - }, - { - "percent": "10000", - "reputation": 67858732461644, - "rshares": 977130038735, - "voter": "fulltimegeek" - }, - { - "percent": "10000", - "reputation": 273738883635043, - "rshares": 1051487783372, - "voter": "inertia" - }, - { - "percent": "1000", - "reputation": 315496990256842, - "rshares": 552546799709, - "voter": "arcange" - }, - { - "percent": "10000", - "reputation": 4957775761011, - "rshares": 4848903462256, - "voter": "ubg" - }, - { - "percent": "300", - "reputation": 1231399052746489, - "rshares": 114797116744, - "voter": "exyle" - }, - { - "percent": "2550", - "reputation": 2558692465689, - "rshares": 18317364904, - "voter": "arconite" - }, - { - "percent": "300", - "reputation": 501315151365, - "rshares": 3176899031, - "voter": "raphaelle" - }, - { - "percent": "10000", - "reputation": 997789975190862, - "rshares": 952473475891, - "voter": "joythewanderer" - }, - { - "percent": "10000", - "reputation": 145783930955299, - "rshares": 600342241141, - "voter": "moon32walker" - }, - { - "percent": "700", - "reputation": 560943007638090, - "rshares": 244256206483, - "voter": "ace108" - }, - { - "percent": "10000", - "reputation": 72178763490828, - "rshares": 313613261360, - "voter": "felixxx" - }, - { - "percent": "10000", - "reputation": 8868395123281, - "rshares": 6424238336878, - "voter": "jphamer1" - }, - { - "percent": "5000", - "reputation": 50032785591993, - "rshares": 530858254412, - "voter": "bryan-imhoff" - }, - { - "percent": "4000", - "reputation": 363924035887507, - "rshares": 568287132809, - "voter": "steevc" - }, - { - "percent": "10000", - "reputation": 92147852982136, - "rshares": 419651063228, - "voter": "lasseehlers" - }, - { - "percent": "300", - "reputation": 96871175157544, - "rshares": 52707010013, - "voter": "someguy123" - }, - { - "percent": "10000", - "reputation": 209327783268279, - "rshares": 202474018536, - "voter": "foxkoit" - }, - { - "percent": "10000", - "reputation": 461742024181352, - "rshares": 41636580232, - "voter": "jlufer" - }, - { - "percent": "10000", - "reputation": 25231292536535, - "rshares": 261788132911, - "voter": "chrisaiki" - }, - { - "percent": "10000", - "reputation": 96059856770036, - "rshares": 15354385725277, - "voter": "canadian-coconut" - }, - { - "percent": "7000", - "reputation": 54361243039563, - "rshares": 61635495692, - "voter": "joanstewart" - }, - { - "percent": "10000", - "reputation": 262691650197556, - "rshares": 64058605201, - "voter": "titusfrost" - }, - { - "percent": "10000", - "reputation": 561323412822933, - "rshares": 5026752487, - "voter": "discernente" - }, - { - "percent": "10000", - "reputation": 339523341647146, - "rshares": 501974674487, - "voter": "whatsup" - }, - { - "percent": "4000", - "reputation": 120512824315021, - "rshares": 1491971227176, - "voter": "funnyman" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 48881445482, - "voter": "ssekulji" - }, - { - "percent": "10000", - "reputation": 559165550719, - "rshares": 2531825516, - "voter": "syahhiran" - }, - { - "percent": "500", - "reputation": 121575052614664, - "rshares": 5693996036, - "voter": "vannour" - }, - { - "percent": "10000", - "reputation": 371604430408116, - "rshares": 2352049499853, - "voter": "nonameslefttouse" - }, - { - "percent": "2000", - "reputation": 7547083616142, - "rshares": 25003769920, - "voter": "edb" - }, - { - "percent": "660", - "reputation": 420443679514793, - "rshares": 109500878045, - "voter": "esteemapp" - }, - { - "percent": "5000", - "reputation": 22050619475702, - "rshares": 30799155004, - "voter": "wagnertamanaha" - }, - { - "percent": "1600", - "reputation": 13145453641526, - "rshares": 10759015680, - "voter": "bigtakosensei" - }, - { - "percent": "10000", - "reputation": 2670247068687, - "rshares": 480321903884, - "voter": "walterjay" - }, - { - "percent": "10000", - "reputation": 2233359256240, - "rshares": 5578645481, - "voter": "sinistry" - }, - { - "percent": "1700", - "reputation": 215061230497985, - "rshares": 2808738575367, - "voter": "v4vapid" - }, - { - "percent": "1300", - "reputation": 5554224447155, - "rshares": 13115399808, - "voter": "lovemetouchme2" - }, - { - "percent": "1500", - "reputation": 27214240242904, - "rshares": 12729464771, - "voter": "rahul.stan" - }, - { - "percent": "10000", - "reputation": 5163257691, - "rshares": 4783303138, - "voter": "koncreteninja" - }, - { - "percent": "300", - "reputation": 38975615169260, - "rshares": 7651374337, - "voter": "steemitboard" - }, - { - "percent": "10000", - "reputation": 231532310014301, - "rshares": 179060891422, - "voter": "denmarkguy" - }, - { - "percent": "600", - "reputation": 24653813359294, - "rshares": 5689790804, - "voter": "privex" - }, - { - "percent": "10000", - "reputation": 43129757389335, - "rshares": 8588120698, - "voter": "sudutpandang" - }, - { - "percent": "10000", - "reputation": 1199149180987, - "rshares": 44713898646809, - "voter": "ranchorelaxo" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 2764259147, - "voter": "dyrt88" - }, - { - "percent": "10000", - "reputation": 19406409261381, - "rshares": 3474946843434, - "voter": "diggndeeper.com" - }, - { - "percent": "10000", - "reputation": 7088385398925, - "rshares": 79081454318, - "voter": "cosmictriage" - }, - { - "percent": "10000", - "reputation": 68502244465536, - "rshares": 14804712300, - "voter": "eirik" - }, - { - "percent": "10000", - "reputation": 450338781685383, - "rshares": 144088138108, - "voter": "louisthomas" - }, - { - "percent": "10000", - "reputation": 203599542560635, - "rshares": 6606328720, - "voter": "lordneroo" - }, - { - "percent": "10000", - "reputation": 286164345508, - "rshares": 808597671, - "voter": "dapu" - }, - { - "percent": "10000", - "reputation": 39205354424754, - "rshares": 6016251797746, - "voter": "dhimmel" - }, - { - "percent": "10000", - "reputation": 153925847897756, - "rshares": 926331562274, - "voter": "preparedwombat" - }, - { - "percent": "500", - "reputation": 1614643030899, - "rshares": 912435103, - "voter": "freebornsociety" - }, - { - "percent": "10000", - "reputation": 10514448764402, - "rshares": 1784880197, - "voter": "syarrf" - }, - { - "percent": "5000", - "reputation": 71901302671995, - "rshares": 14682152188, - "voter": "hiroyamagishi" - }, - { - "percent": "300", - "reputation": 21701614328748, - "rshares": 22558504241, - "voter": "dune69" - }, - { - "percent": "10000", - "reputation": 74329552872641, - "rshares": 264429866494, - "voter": "mes" - }, - { - "percent": "10000", - "reputation": 66024390617355, - "rshares": 736012913460, - "voter": "elizahfhaye" - }, - { - "percent": "186", - "reputation": 39969242565947, - "rshares": 3406701512, - "voter": "iansart" - }, - { - "percent": "6100", - "reputation": 139091215313620, - "rshares": 830199984837, - "voter": "whatamidoing" - }, - { - "percent": "10000", - "reputation": 13370190184046, - "rshares": 1821848367, - "voter": "martie7" - }, - { - "percent": "10000", - "reputation": 32595690215621, - "rshares": 668851107744, - "voter": "forykw" - }, - { - "percent": "10000", - "reputation": 1748651637089, - "rshares": 4734131735, - "voter": "khussan" - }, - { - "percent": "2500", - "reputation": 76741990090791, - "rshares": 22420389851, - "voter": "valued-customer" - }, - { - "percent": "10000", - "reputation": 48378473521256, - "rshares": 36147195813, - "voter": "drag33" - }, - { - "percent": "10000", - "reputation": 23819307616416, - "rshares": 23618521525, - "voter": "farizal" - }, - { - "percent": "10000", - "reputation": 14638546901678, - "rshares": 4917165554847, - "voter": "fatimajunio" - }, - { - "percent": "10000", - "reputation": 413408024106, - "rshares": 1279234556663, - "voter": "nrg" - }, - { - "percent": "10000", - "reputation": 3773610796661, - "rshares": 2027659504, - "voter": "alainite" - }, - { - "percent": "10000", - "reputation": 26275815650202, - "rshares": 71497931790, - "voter": "trumpikas" - }, - { - "percent": "10000", - "reputation": 19675504562112, - "rshares": 198122927840, - "voter": "amirl" - }, - { - "percent": "10000", - "reputation": 112994806497327, - "rshares": 1643010329, - "voter": "varunpinto" - }, - { - "percent": "5000", - "reputation": 80123076461352, - "rshares": 392737885877, - "voter": "xels" - }, - { - "percent": "1000", - "reputation": 19001680286018, - "rshares": 1206516316, - "voter": "rycharde" - }, - { - "percent": "10000", - "reputation": 9701652715721, - "rshares": 131658274720, - "voter": "masterthematrix" - }, - { - "percent": "10000", - "reputation": 2271312461941, - "rshares": 3095773101, - "voter": "taitux" - }, - { - "percent": "10000", - "reputation": 329877254452254, - "rshares": 8556817259, - "voter": "steeminator3000" - }, - { - "percent": "270", - "reputation": 23577497062186, - "rshares": 52957874654, - "voter": "alphacore" - }, - { - "percent": "10000", - "reputation": 46150080976545, - "rshares": 3460260806495, - "voter": "cryptographic" - }, - { - "percent": "10000", - "reputation": 94117611307146, - "rshares": 215412904661, - "voter": "ammonite" - }, - { - "percent": "10000", - "reputation": 110211133478274, - "rshares": 1609518940767, - "voter": "drakos" - }, - { - "percent": "10000", - "reputation": 20355801093011, - "rshares": 480974958449, - "voter": "jingdol" - }, - { - "percent": "5000", - "reputation": 383718200382, - "rshares": 810520587, - "voter": "kramarenko" - }, - { - "percent": "10000", - "reputation": 219366237221002, - "rshares": 588913603308, - "voter": "offgridlife" - }, - { - "percent": "5000", - "reputation": 9899728290123, - "rshares": 61318019708, - "voter": "rarej" - }, - { - "percent": "10000", - "reputation": 205181088227172, - "rshares": 1381459226231, - "voter": "geekgirl" - }, - { - "percent": "3100", - "reputation": 171498070594985, - "rshares": 124842047071, - "voter": "toofasteddie" - }, - { - "percent": "10000", - "reputation": 184191136030059, - "rshares": 946195611084, - "voter": "runicar" - }, - { - "percent": "-10000", - "reputation": 223486867868, - "rshares": -95842718166, - "voter": "captainquack22" - }, - { - "percent": "10000", - "reputation": 59695875646638, - "rshares": 902282209, - "voter": "theguruasia" - }, - { - "percent": "10000", - "reputation": 5313719494876540, - "rshares": 12720015246109, - "voter": "haejin" - }, - { - "percent": "10000", - "reputation": 466532027405, - "rshares": 1656862785, - "voter": "dado13btc" - }, - { - "percent": "10000", - "reputation": 6887925214950, - "rshares": 211311449875, - "voter": "droida" - }, - { - "percent": "300", - "reputation": 18018060826148, - "rshares": 1240819202, - "voter": "shitsignals" - }, - { - "percent": "10000", - "reputation": 412383998781, - "rshares": 5358208235, - "voter": "last.german" - }, - { - "percent": "10000", - "reputation": 190691885658151, - "rshares": 7208743437441, - "voter": "nathanmars" - }, - { - "percent": "3000", - "reputation": 115269542036380, - "rshares": 390008817582, - "voter": "vikisecrets" - }, - { - "percent": "1000", - "reputation": 68448132279986, - "rshares": 794405922, - "voter": "fatherfaith" - }, - { - "percent": "10000", - "reputation": 98412847766611, - "rshares": 820600320631, - "voter": "resiliencia" - }, - { - "percent": "500", - "reputation": 1844447662772, - "rshares": 1315088429, - "voter": "khalil319" - }, - { - "percent": "10000", - "reputation": 3449425709461, - "rshares": 197184046628, - "voter": "pardeepkumar" - }, - { - "percent": "10000", - "reputation": 1528965628556, - "rshares": 2220061514, - "voter": "chey" - }, - { - "percent": "10000", - "reputation": 72946432090481, - "rshares": 127321705399, - "voter": "my451r" - }, - { - "percent": "300", - "reputation": 173069624727027, - "rshares": 14141938471, - "voter": "felander" - }, - { - "percent": "4840", - "reputation": 24639928209538, - "rshares": 26835577137, - "voter": "santigs" - }, - { - "percent": "10000", - "reputation": 196571377648, - "rshares": 18127709309, - "voter": "nadhora" - }, - { - "percent": "10000", - "reputation": 11131064226204, - "rshares": 17636757813, - "voter": "angelusnoctum" - }, - { - "percent": "2500", - "reputation": 54379045488206, - "rshares": 67543767987, - "voter": "bashadow" - }, - { - "percent": "100", - "reputation": 51994511985595, - "rshares": 399407165137, - "voter": "tipu" - }, - { - "percent": "10000", - "reputation": 2736265537663, - "rshares": 60449688827, - "voter": "massivevibration" - }, - { - "percent": "5100", - "reputation": 5754775236968, - "rshares": 2306872016, - "voter": "nurhayati" - }, - { - "percent": "10000", - "reputation": 140483466255317, - "rshares": 579980511366, - "voter": "fbslo" - }, - { - "percent": "500", - "reputation": 47942503950741, - "rshares": 49927105281, - "voter": "accelerator" - }, - { - "percent": "10000", - "reputation": 4629448536475, - "rshares": 58104134515, - "voter": "wholeself-in" - }, - { - "percent": "10000", - "reputation": 669346799793, - "rshares": 15205223254, - "voter": "voxmonkey" - }, - { - "percent": "300", - "reputation": 731791169403, - "rshares": 2698078508, - "voter": "yogacoach" - }, - { - "percent": "10000", - "reputation": 167991810520774, - "rshares": 665569711617, - "voter": "celestal" - }, - { - "percent": "10000", - "reputation": 110750156547770, - "rshares": 520765550224, - "voter": "drax" - }, - { - "percent": "448", - "reputation": 0, - "rshares": 10225293391, - "voter": "tomiscurious" - }, - { - "percent": "10000", - "reputation": 61350839312689, - "rshares": 46752310018, - "voter": "termitemusic" - }, - { - "percent": "10000", - "reputation": 79764359720874, - "rshares": 101322883314, - "voter": "sayee" - }, - { - "percent": "658", - "reputation": 0, - "rshares": 1973329564, - "voter": "esteem.app" - }, - { - "percent": "841", - "reputation": 0, - "rshares": 340110652249, - "voter": "investegg" - }, - { - "percent": "2500", - "reputation": 141970828773777, - "rshares": 398358525454, - "voter": "tobetada" - }, - { - "percent": "10000", - "reputation": 30679468047783, - "rshares": 10662762995959, - "voter": "fedesox" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 5726510347, - "voter": "fulcrumleader" - }, - { - "percent": "3700", - "reputation": 393133538290267, - "rshares": 31783011464, - "voter": "madushanka" - }, - { - "percent": "10000", - "reputation": 635150854067, - "rshares": 9619769554, - "voter": "teknow" - }, - { - "percent": "10000", - "reputation": 128158005695390, - "rshares": 63286914563, - "voter": "arabisouri" - }, - { - "percent": "10000", - "reputation": 103915899650487, - "rshares": 725271779242, - "voter": "traciyork" - }, - { - "percent": "2500", - "reputation": 663634027075, - "rshares": 660536230, - "voter": "nexusvortex777" - }, - { - "percent": "285", - "reputation": 7232716062458, - "rshares": 5588627748, - "voter": "caladan" - }, - { - "percent": "10000", - "reputation": 1436962620884, - "rshares": 793454462, - "voter": "mrsyria" - }, - { - "percent": "2000", - "reputation": 72060804308794, - "rshares": 524696608109, - "voter": "eonwarped" - }, - { - "percent": "300", - "reputation": 377317663062316, - "rshares": 77507226038, - "voter": "emrebeyler" - }, - { - "percent": "10000", - "reputation": 579461579559, - "rshares": 175445075783, - "voter": "sankysanket18" - }, - { - "percent": "100", - "reputation": 127902933991218, - "rshares": 436423391, - "voter": "felipejoys" - }, - { - "percent": "5000", - "reputation": 398820969940927, - "rshares": 861682963, - "voter": "etherpunk" - }, - { - "percent": "3100", - "reputation": 172804239020062, - "rshares": 101619104886, - "voter": "chekohler" - }, - { - "percent": "10000", - "reputation": 39868149562725, - "rshares": 990923768485, - "voter": "armentor" - }, - { - "percent": "10000", - "reputation": 52628537782133, - "rshares": 144459808558, - "voter": "azwarrangkuti" - }, - { - "percent": "10000", - "reputation": 76831784793845, - "rshares": 326451544183, - "voter": "ocupation" - }, - { - "percent": "10000", - "reputation": 17711690265347, - "rshares": 110727893807, - "voter": "sgt-dan" - }, - { - "percent": "10000", - "reputation": 224345212509723, - "rshares": 40168111158, - "voter": "olawalium" - }, - { - "percent": "10000", - "reputation": 9896237488, - "rshares": 18595491811, - "voter": "howiemac" - }, - { - "percent": "10000", - "reputation": 12025175588719, - "rshares": 13421376808, - "voter": "jicrochet" - }, - { - "percent": "10000", - "reputation": 199114477141557, - "rshares": 11020196452, - "voter": "enjoyinglife" - }, - { - "percent": "10000", - "reputation": 52315833113791, - "rshares": 73495177351, - "voter": "inalittlewhile" - }, - { - "percent": "10000", - "reputation": 112593705937795, - "rshares": 174149357579, - "voter": "apnigrich" - }, - { - "percent": "10000", - "reputation": 23470732984911, - "rshares": 147375560214, - "voter": "aussieninja" - }, - { - "percent": "10000", - "reputation": 73184833081444, - "rshares": 2843999511374, - "voter": "oliverschmid" - }, - { - "percent": "7400", - "reputation": 305437093461437, - "rshares": 144544654642, - "voter": "abitcoinskeptic" - }, - { - "percent": "10000", - "reputation": 469346970536721, - "rshares": 745468101997, - "voter": "daltono" - }, - { - "percent": "5000", - "reputation": 368970410036642, - "rshares": 719223874682, - "voter": "jongolson" - }, - { - "percent": "10000", - "reputation": 21639191847021, - "rshares": 8056423288542, - "voter": "mmmmkkkk311" - }, - { - "percent": "300", - "reputation": 138441860072892, - "rshares": 17782155704, - "voter": "nealmcspadden" - }, - { - "percent": "10000", - "reputation": 279507826168043, - "rshares": 2887706042544, - "voter": "edicted" - }, - { - "percent": "10000", - "reputation": 7833564344722, - "rshares": 2179555995, - "voter": "ahmedsy" - }, - { - "percent": "300", - "reputation": 7978719984268, - "rshares": 3672347744, - "voter": "purefood" - }, - { - "percent": "5000", - "reputation": 184489319084, - "rshares": 549389194, - "voter": "steemboat-steve" - }, - { - "percent": "10000", - "reputation": 3344383644535, - "rshares": 1283078421, - "voter": "justasking" - }, - { - "percent": "3500", - "reputation": 37970568723804, - "rshares": 24855914143, - "voter": "psos" - }, - { - "percent": "1900", - "reputation": 8003119161188, - "rshares": 3952774676, - "voter": "brewery" - }, - { - "percent": "10000", - "reputation": 19975856950324, - "rshares": 954468651, - "voter": "eosgo" - }, - { - "percent": "10000", - "reputation": 11165935981106, - "rshares": 1053886897, - "voter": "icuz" - }, - { - "percent": "5000", - "reputation": 4156063258051, - "rshares": 1941963922, - "voter": "investprosper" - }, - { - "percent": "5000", - "reputation": 54137549885572, - "rshares": 29297665153, - "voter": "angryman" - }, - { - "percent": "2500", - "reputation": 8496919418242, - "rshares": 3228237917, - "voter": "ericburgoyne" - }, - { - "percent": "240", - "reputation": 10898214322749, - "rshares": 1179840919, - "voter": "pkocjan" - }, - { - "percent": "5000", - "reputation": 1209226474661, - "rshares": 995483925, - "voter": "josh26" - }, - { - "percent": "5000", - "reputation": 483223942335, - "rshares": 2118982347, - "voter": "elektr1ker" - }, - { - "percent": "10000", - "reputation": 8405881148978, - "rshares": 12935668781, - "voter": "thebluewin" - }, - { - "percent": "10000", - "reputation": 4895191598205, - "rshares": 4153538585, - "voter": "auminda" - }, - { - "percent": "10000", - "reputation": 64242146543076, - "rshares": 208127827769, - "voter": "citimillz" - }, - { - "percent": "10000", - "reputation": 274611114102331, - "rshares": 3198367493936, - "voter": "mindtrap" - }, - { - "percent": "3000", - "reputation": 62406194635091, - "rshares": 11194802009, - "voter": "russellstockley" - }, - { - "percent": "10000", - "reputation": 10610474160807, - "rshares": 234206185225, - "voter": "harpagon" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 28978784438, - "voter": "futurecurrency" - }, - { - "percent": "10000", - "reputation": 73042470414733, - "rshares": 402186880842, - "voter": "rubencress" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 6216625480, - "voter": "wildarms65" - }, - { - "percent": "500", - "reputation": 2256065348315, - "rshares": 796380456, - "voter": "frassman" - }, - { - "percent": "300", - "reputation": 61129901433914, - "rshares": 12670209579, - "voter": "reazuliqbal" - }, - { - "percent": "-10000", - "reputation": 46955154178798, - "rshares": -2097360156622, - "voter": "amico" - }, - { - "percent": "10000", - "reputation": 983103554489, - "rshares": 3273494286, - "voter": "jo5h" - }, - { - "percent": "300", - "reputation": 1170108458021, - "rshares": 1370993467, - "voter": "bestboom" - }, - { - "percent": "10000", - "reputation": 25978211905358, - "rshares": 277808640545, - "voter": "manniman" - }, - { - "percent": "10000", - "reputation": 367201484924059, - "rshares": 1234339527759, - "voter": "louis88" - }, - { - "percent": "10000", - "reputation": 4110594694460, - "rshares": 1036186035297, - "voter": "jkramer" - }, - { - "percent": "10000", - "reputation": 373679836722908, - "rshares": 3455956679576, - "voter": "derangedvisions" - }, - { - "percent": "10000", - "reputation": 4038024331096, - "rshares": 490771253707, - "voter": "foxon" - }, - { - "percent": "10000", - "reputation": 6746077696008, - "rshares": 21617447602, - "voter": "radard" - }, - { - "percent": "10000", - "reputation": 2184610478491, - "rshares": 10199143912, - "voter": "ex-exploitation" - }, - { - "percent": "1500", - "reputation": 7324548372470, - "rshares": 21946856598, - "voter": "freddio" - }, - { - "percent": "1500", - "reputation": 570270524259, - "rshares": 16187125315, - "voter": "blainjones" - }, - { - "percent": "10000", - "reputation": 11722167805732, - "rshares": 29138439050, - "voter": "phasewalker" - }, - { - "percent": "5000", - "reputation": 32061253351, - "rshares": 8581541571, - "voter": "informator" - }, - { - "percent": "300", - "reputation": 18055325674078, - "rshares": 27626089330, - "voter": "themightyvolcano" - }, - { - "percent": "10000", - "reputation": 2481629111221, - "rshares": 2154224858, - "voter": "manojbhatt" - }, - { - "percent": "1000", - "reputation": 454088646937365, - "rshares": 31449538509, - "voter": "kgakakillerg" - }, - { - "percent": "10000", - "reputation": 19409877558565, - "rshares": 99311404953, - "voter": "mraggaj" - }, - { - "percent": "10000", - "reputation": 7977152581078, - "rshares": 22904712146, - "voter": "khrom" - }, - { - "percent": "10000", - "reputation": 4521400761737, - "rshares": 7445024641, - "voter": "towilmasz" - }, - { - "percent": "340", - "reputation": 9020628089607, - "rshares": 2912156787, - "voter": "promobot" - }, - { - "percent": "100", - "reputation": 3042286903871, - "rshares": 404122924, - "voter": "jancharlest" - }, - { - "percent": "75", - "reputation": 1963153455547, - "rshares": 16916222316, - "voter": "steem.services" - }, - { - "percent": "10000", - "reputation": 4637465899679, - "rshares": 11994856594, - "voter": "pannapysia" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 19000454877, - "voter": "diabonua" - }, - { - "percent": "8000", - "reputation": 35592667940354, - "rshares": 15487467354, - "voter": "zaxan" - }, - { - "percent": "5000", - "reputation": 29227572228613, - "rshares": 25990914346, - "voter": "techcoderx" - }, - { - "percent": "1000", - "reputation": 1993672004294, - "rshares": 50926618606, - "voter": "pladozero" - }, - { - "percent": "10000", - "reputation": 813698891718, - "rshares": 3432962199, - "voter": "minerthreat" - }, - { - "percent": "10000", - "reputation": 13550154929336, - "rshares": 5158396408, - "voter": "ntowl" - }, - { - "percent": "800", - "reputation": 70214892950356, - "rshares": 301479406085, - "voter": "nateaguila" - }, - { - "percent": "2200", - "reputation": 51236835710173, - "rshares": 9991988056, - "voter": "davidesimoncini" - }, - { - "percent": "1500", - "reputation": 153947835280090, - "rshares": 127756185184, - "voter": "enforcer48" - }, - { - "percent": "4000", - "reputation": 56448250239493, - "rshares": 17689543324, - "voter": "sketch17" - }, - { - "percent": "10000", - "reputation": 13630732276127, - "rshares": 13976419427, - "voter": "elmundodexao" - }, - { - "percent": "300", - "reputation": 655223881789, - "rshares": 1740684993, - "voter": "swisswitness" - }, - { - "percent": "10000", - "reputation": 52047051239253, - "rshares": 321856485870, - "voter": "apshamilton" - }, - { - "percent": "10000", - "reputation": 31579572689, - "rshares": 744933088, - "voter": "agathusia" - }, - { - "percent": "10000", - "reputation": 49365177906156, - "rshares": 855737795, - "voter": "milaan" - }, - { - "percent": "10000", - "reputation": 6836653370416, - "rshares": 9893062096, - "voter": "reyvaj" - }, - { - "percent": "200", - "reputation": 33860216986858, - "rshares": 12273479986, - "voter": "donald.porter" - }, - { - "percent": "10000", - "reputation": 2075722785, - "rshares": 0, - "voter": "mmmplus" - }, - { - "percent": "10000", - "reputation": 558380924105, - "rshares": 424608243954, - "voter": "hamismsf" - }, - { - "percent": "150", - "reputation": 156376467869107, - "rshares": 4807205234, - "voter": "dalz" - }, - { - "percent": "9000", - "reputation": 425226010828, - "rshares": 46363020035, - "voter": "yaelg" - }, - { - "percent": "150", - "reputation": 18790919715448, - "rshares": 22193817567, - "voter": "luppers" - }, - { - "percent": "300", - "reputation": 103580743464850, - "rshares": 35013860565, - "voter": "dlike" - }, - { - "percent": "50", - "reputation": 144910360949054, - "rshares": 949613651, - "voter": "voxmortis" - }, - { - "percent": "240", - "reputation": 58772053733860, - "rshares": 48011072215, - "voter": "engrave" - }, - { - "percent": "10000", - "reputation": 133998446537867, - "rshares": 5590085969, - "voter": "bluengel" - }, - { - "percent": "5000", - "reputation": 77764751586248, - "rshares": 1568545947919, - "voter": "frot" - }, - { - "percent": "10000", - "reputation": 1494229786604, - "rshares": 142807583852, - "voter": "pboulet" - }, - { - "percent": "10000", - "reputation": 10278138043955, - "rshares": 17210040, - "voter": "laissez-faire" - }, - { - "percent": "964", - "reputation": 0, - "rshares": 10252262023, - "voter": "voter003" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 12487342046, - "voter": "quediceharry" - }, - { - "percent": "1500", - "reputation": 6687049412835, - "rshares": 12190399420, - "voter": "mister-meeseeks" - }, - { - "percent": "10000", - "reputation": 7593689306652, - "rshares": 100306637610993, - "voter": "encrypt3dbr0k3r" - }, - { - "percent": "300", - "reputation": 0, - "rshares": 9217481706, - "voter": "merlin7" - }, - { - "percent": "10000", - "reputation": 1648086760085, - "rshares": 29141410587, - "voter": "diegor" - }, - { - "percent": "1500", - "reputation": 570615134196274, - "rshares": 60498038537, - "voter": "priyanarc" - }, - { - "percent": "10000", - "reputation": 13222548277758, - "rshares": 283561453890, - "voter": "wil.metcalfe" - }, - { - "percent": "6000", - "reputation": 11213150975739, - "rshares": 100563172951, - "voter": "kristall97" - }, - { - "percent": "3000", - "reputation": 2878433743657, - "rshares": 10500277669, - "voter": "faeryboots" - }, - { - "percent": "10000", - "reputation": 14391246828388, - "rshares": 16445163689, - "voter": "geeklania" - }, - { - "percent": "9000", - "reputation": 49288860027665, - "rshares": 753094556886, - "voter": "cwow2" - }, - { - "percent": "300", - "reputation": 2716815451543, - "rshares": 22160877911, - "voter": "followjohngalt" - }, - { - "percent": "10000", - "reputation": 45419481582603, - "rshares": 217075039975, - "voter": "michealb" - }, - { - "percent": "5500", - "reputation": 539147053023232, - "rshares": 25272276583356, - "voter": "theycallmedan" - }, - { - "percent": "150", - "reputation": 3420036317592, - "rshares": 1889866143, - "voter": "cakemonster" - }, - { - "percent": "10000", - "reputation": 30281770348, - "rshares": 3584979531, - "voter": "themightysquid" - }, - { - "percent": "10000", - "reputation": 3633716322140, - "rshares": 3878543875, - "voter": "marki99" - }, - { - "percent": "10000", - "reputation": 38750190104631, - "rshares": 105245447783, - "voter": "owasco" - }, - { - "percent": "10000", - "reputation": 3051298620925, - "rshares": 373741785723, - "voter": "jpbliberty" - }, - { - "percent": "10000", - "reputation": 4104615584, - "rshares": 94285862968, - "voter": "steem-on-2020" - }, - { - "percent": "2000", - "reputation": 3606329902229, - "rshares": 2985071069, - "voter": "shainemata" - }, - { - "percent": "10000", - "reputation": 13815761380, - "rshares": 44445766370, - "voter": "tigerrkg" - }, - { - "percent": "1700", - "reputation": 971181267, - "rshares": 19523957699, - "voter": "primeradue" - }, - { - "percent": "300", - "reputation": 1213063566738, - "rshares": 1130529524, - "voter": "permaculturedude" - }, - { - "percent": "10000", - "reputation": 3188730840665, - "rshares": 37140273028, - "voter": "loliver" - }, - { - "percent": "5500", - "reputation": 0, - "rshares": 293984560986, - "voter": "johnmadden" - }, - { - "percent": "1750", - "reputation": 6085708906847, - "rshares": 2610974919, - "voter": "creary" - }, - { - "percent": "10000", - "reputation": 12361832402331, - "rshares": 58384020878, - "voter": "idiosyncratic1" - }, - { - "percent": "10000", - "reputation": 97219598927367, - "rshares": 60731430128, - "voter": "josehany" - }, - { - "percent": "10000", - "reputation": 75911580, - "rshares": 164705903827, - "voter": "jamesbattler" - }, - { - "percent": "10000", - "reputation": -15243422544198, - "rshares": 2417323952, - "voter": "overall-servant" - }, - { - "percent": "10000", - "reputation": 82203812282991, - "rshares": 180059610753, - "voter": "monster-one" - }, - { - "percent": "1500", - "reputation": 6301639433510, - "rshares": 806783930, - "voter": "goodcontentbot" - }, - { - "percent": "10000", - "reputation": 25016264134313, - "rshares": 796719662454, - "voter": "dcommerce" - }, - { - "percent": "5000", - "reputation": 5616865862, - "rshares": 11093438707, - "voter": "abbenay" - }, - { - "percent": "10000", - "reputation": 193706512612, - "rshares": 1335565639, - "voter": "stevenmalik" - }, - { - "percent": "3500", - "reputation": 96673801127912, - "rshares": 83461331689, - "voter": "doze" - }, - { - "percent": "10000", - "reputation": 4774689093914, - "rshares": 8655822760, - "voter": "osavi" - }, - { - "percent": "9000", - "reputation": 44106429466, - "rshares": 1281949203, - "voter": "goodcontentbot1" - }, - { - "percent": "1000", - "reputation": 22403588555771, - "rshares": 21555741638, - "voter": "thelogicaldude" - }, - { - "percent": "10000", - "reputation": 2910555091868, - "rshares": 1069757538, - "voter": "denizcakmak" - }, - { - "percent": "2000", - "reputation": 27838835653920, - "rshares": 5666415243, - "voter": "kggymlife" - }, - { - "percent": "-10000", - "reputation": 193060942276, - "rshares": 0, - "voter": "holydog" - }, - { - "percent": "100", - "reputation": 69910542366759, - "rshares": 4840214743, - "voter": "kryptogames" - }, - { - "percent": "285", - "reputation": 17287220932883, - "rshares": 3099762771, - "voter": "mfblack" - }, - { - "percent": "10000", - "reputation": 305410265087460, - "rshares": 330290734057, - "voter": "claudio83" - }, - { - "percent": "5000", - "reputation": 459526835755, - "rshares": 900459494, - "voter": "bigmoneyman" - }, - { - "percent": "10000", - "reputation": 29888557634290, - "rshares": 24452453814, - "voter": "helgalubevi" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 52522691035, - "voter": "banvie" - }, - { - "percent": "150", - "reputation": 33348221474331, - "rshares": 791362546, - "voter": "threejay" - }, - { - "percent": "10000", - "reputation": 91489915760, - "rshares": 785046248, - "voter": "canercanbolat" - }, - { - "percent": "10000", - "reputation": 4912769043403, - "rshares": 1627107038, - "voter": "theshaki" - }, - { - "percent": "1500", - "reputation": 9382926732500, - "rshares": 25464268803, - "voter": "morwen" - }, - { - "percent": "340", - "reputation": 36087507440149, - "rshares": 29033357159, - "voter": "likwid" - }, - { - "percent": "10000", - "reputation": 36857316180998, - "rshares": 65488385307, - "voter": "blumela" - }, - { - "percent": "3900", - "reputation": 201674995003388, - "rshares": 258052628624, - "voter": "borjan" - }, - { - "percent": "10000", - "reputation": 499450701404, - "rshares": 585637341, - "voter": "iktisat" - }, - { - "percent": "5000", - "reputation": 927259760311, - "rshares": 553402668, - "voter": "oxoskva" - }, - { - "percent": "750", - "reputation": 0, - "rshares": 1444869138, - "voter": "cryptogambit" - }, - { - "percent": "10000", - "reputation": 64787679421704, - "rshares": 22794338359, - "voter": "katiefreespirit" - }, - { - "percent": "10000", - "reputation": 537015876037, - "rshares": 3126773665, - "voter": "vxc" - }, - { - "percent": "300", - "reputation": 52974480102727, - "rshares": 1737963819, - "voter": "shimozurdo" - }, - { - "percent": "2000", - "reputation": 773985194624, - "rshares": 540997539, - "voter": "kgswallet" - }, - { - "percent": "300", - "reputation": 40619501571, - "rshares": 1261320466, - "voter": "milu-the-dog" - }, - { - "percent": "10000", - "reputation": 116210100336, - "rshares": 2081987301, - "voter": "mustakkio" - }, - { - "percent": "300", - "reputation": 9905442858, - "rshares": 1030746382, - "voter": "triplea.bot" - }, - { - "percent": "300", - "reputation": 115912639173774, - "rshares": 29872480486, - "voter": "steem.leo" - }, - { - "percent": "10000", - "reputation": 2891275992098, - "rshares": 67665673514, - "voter": "teo93" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 20514206183, - "voter": "good.game" - }, - { - "percent": "10000", - "reputation": 622788295137, - "rshares": 2574532979, - "voter": "ali-h" - }, - { - "percent": "10000", - "reputation": 6411393851, - "rshares": 881619638, - "voter": "driedfruit" - }, - { - "percent": "270", - "reputation": 14604990824032, - "rshares": 3314713748, - "voter": "asteroids" - }, - { - "percent": "75", - "reputation": 1410161022, - "rshares": 1762516484, - "voter": "botante" - }, - { - "percent": "10000", - "reputation": 118320666727, - "rshares": 5456507811, - "voter": "dechuck" - }, - { - "percent": "694", - "reputation": 18505058691598, - "rshares": 37231330779, - "voter": "logiczombie" - }, - { - "percent": "10000", - "reputation": 26814777059782, - "rshares": 553647686037, - "voter": "lynds" - }, - { - "percent": "600", - "reputation": 426404968, - "rshares": 23717174450, - "voter": "maxuvd" - }, - { - "percent": "600", - "reputation": 6113738572, - "rshares": 30398415409, - "voter": "maxuve" - }, - { - "percent": "5000", - "reputation": 1704173681552, - "rshares": 1128221857, - "voter": "bilpcoinbot" - }, - { - "percent": "10000", - "reputation": 5976543610782, - "rshares": 114803907, - "voter": "alicargofer" - }, - { - "percent": "1500", - "reputation": 2754871650749, - "rshares": 3627144006, - "voter": "dappstats" - }, - { - "percent": "2500", - "reputation": 447569668922, - "rshares": 1048518741, - "voter": "bilpcoin.pay" - }, - { - "percent": "10000", - "reputation": 4298572479419, - "rshares": 6667748362, - "voter": "bakingjazzpower" - }, - { - "percent": "10000", - "reputation": 17325573495839, - "rshares": 48476527727, - "voter": "kaeserotor" - }, - { - "percent": "10000", - "reputation": 51941880676536, - "rshares": 29280054281, - "voter": "sacrosanct" - }, - { - "percent": "10000", - "reputation": 15076530384277, - "rshares": 99920979221, - "voter": "oblivioncubed" - }, - { - "percent": "10000", - "reputation": 131203903096144, - "rshares": 38974215117, - "voter": "firayumni" - }, - { - "percent": "150", - "reputation": 0, - "rshares": 961463347, - "voter": "ribary" - }, - { - "percent": "1000", - "reputation": 32542453022, - "rshares": 973770987, - "voter": "bilpcoinbpc" - }, - { - "percent": "9900", - "reputation": 3843288884834, - "rshares": 2935873540, - "voter": "der-fahrlehrer" - }, - { - "percent": "300", - "reputation": 549265305265, - "rshares": 15229104385, - "voter": "mice-k" - }, - { - "percent": "5000", - "reputation": 679076789336, - "rshares": 9518658113, - "voter": "davidlionfish" - }, - { - "percent": "-10000", - "reputation": 178783384909, - "rshares": -166436595, - "voter": "ainsuphaur" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1606230178046, - "voter": "gleam-of-light" - }, - { - "percent": "10000", - "reputation": 362096342263, - "rshares": 384414251, - "voter": "sujaytechnicals" - }, - { - "percent": "2500", - "reputation": 30620520771, - "rshares": 3306825035, - "voter": "hive-168088" - }, - { - "percent": "10000", - "reputation": 2829358208974, - "rshares": 9644236974, - "voter": "adamdabeast" - }, - { - "percent": "4000", - "reputation": 38576347919541, - "rshares": 3940408761, - "voter": "dapplr" - }, - { - "percent": "2000", - "reputation": 16625796586365, - "rshares": 2919698888, - "voter": "femcy-willy" - }, - { - "percent": "300", - "reputation": 0, - "rshares": 15759855851, - "voter": "fengchao" - }, - { - "percent": "10000", - "reputation": 86868289888522, - "rshares": 1811582636690, - "voter": "peakd" - }, - { - "percent": "10000", - "reputation": 358839904767, - "rshares": 56902075150, - "voter": "akunull" - }, - { - "percent": "6580", - "reputation": 1209731647511, - "rshares": 1104730823632, - "voter": "softworld" - }, - { - "percent": "300", - "reputation": 5571802524553, - "rshares": 7927727848, - "voter": "polish.hive" - }, - { - "percent": "10000", - "reputation": 2193438115036, - "rshares": 3195445606, - "voter": "mpath" - }, - { - "percent": "100", - "reputation": 0, - "rshares": 28080365, - "voter": "tips.tracker" - }, - { - "percent": "300", - "reputation": 0, - "rshares": 92777821343, - "voter": "dcityrewards" - }, - { - "percent": "6600", - "reputation": 1292923913128, - "rshares": 13079488440, - "voter": "hextech" - }, - { - "percent": "10000", - "reputation": 5277345693920, - "rshares": 1715291941, - "voter": "andrewmusic" - }, - { - "percent": "300", - "reputation": 3177222978373, - "rshares": 2708867402, - "voter": "hivelist" - }, - { - "percent": "1500", - "reputation": 604442758770, - "rshares": 67163304024, - "voter": "ninnu" - }, - { - "percent": "10000", - "reputation": 32381994985806, - "rshares": 36838660825, - "voter": "yolimarag" - }, - { - "percent": "658", - "reputation": 56328328383994, - "rshares": 394879682087, - "voter": "ecency" - }, - { - "percent": "10000", - "reputation": 474817393502, - "rshares": 372280638, - "voter": "parung76" - }, - { - "percent": "3000", - "reputation": 429763208250, - "rshares": 1266920657, - "voter": "ghaazi" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "zarevaneli" - }, - { - "percent": "300", - "reputation": 0, - "rshares": 12590071812, - "voter": "hivecur" - }, - { - "percent": "10000", - "reputation": 37908486627936, - "rshares": 34251323681, - "voter": "ykroys" - }, - { - "percent": "10000", - "reputation": 1289890269498, - "rshares": 824783481, - "voter": "greengalletti" - }, - { - "percent": "10000", - "reputation": 11320226922294, - "rshares": 3338300088715, - "voter": "hiro-hive" - }, - { - "percent": "10000", - "reputation": 18263907419485, - "rshares": 5612610948, - "voter": "zhoten" - }, - { - "percent": "658", - "reputation": 12378826857256, - "rshares": 1184795548, - "voter": "ecency.stats" - }, - { - "percent": "10000", - "reputation": 15596004215170, - "rshares": 1060275196, - "voter": "rojiso2411" - }, - { - "percent": "10000", - "reputation": 2830639099232, - "rshares": 261866749, - "voter": "koxmicart" - }, - { - "percent": "5000", - "reputation": 35280907862981, - "rshares": 10259973992, - "voter": "hive-data" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 21765102972, - "voter": "gohive" - }, - { - "percent": "800", - "reputation": 15234109271535, - "rshares": 953834024, - "voter": "vicnzia" - }, - { - "percent": "10000", - "reputation": 3528170359266, - "rshares": 7117713404, - "voter": "cryptosimplify" - }, - { - "percent": "10000", - "reputation": 33401976736, - "rshares": 49938222105, - "voter": "aceh-media" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 15629950438, - "voter": "vtol79" - }, - { - "percent": "5000", - "reputation": 1447273576100, - "rshares": 2715073640, - "voter": "bitcome" - }, - { - "percent": "3400", - "reputation": 16307549034965, - "rshares": 5418531736, - "voter": "trangbaby" - }, - { - "percent": "10000", - "reputation": 656202726984, - "rshares": 9561387504, - "voter": "monster-retos" - }, - { - "percent": "10000", - "reputation": 4104673569963, - "rshares": 4372576927, - "voter": "gilliatt" - }, - { - "percent": "5000", - "reputation": 102092235719, - "rshares": 884892339, - "voter": "clifth" - }, - { - "percent": "10000", - "reputation": 86405531104, - "rshares": 1954083117, - "voter": "text2speech" - }, - { - "percent": "10000", - "reputation": 284208655587, - "rshares": 1121474774, - "voter": "freemarihuana" - }, - { - "percent": "10000", - "reputation": 112363590278, - "rshares": 45554385, - "voter": "dango1411" - }, - { - "percent": "10000", - "reputation": 1303993724855, - "rshares": 80531371813, - "voter": "damus-nostra" - }, - { - "percent": "10000", - "reputation": 2350243356279, - "rshares": 3601066911, - "voter": "omonteleone" - }, - { - "percent": "10000", - "reputation": 270295677528, - "rshares": 237953329, - "voter": "hauptfleisch" - }, - { - "percent": "10000", - "reputation": 191920431453, - "rshares": 1014862882, - "voter": "ventucollado12" - }, - { - "percent": "10000", - "reputation": 1523084393, - "rshares": 7727034, - "voter": "osiri" - }, - { - "percent": "10000", - "reputation": 12807018861, - "rshares": 2913985358, - "voter": "q42" - }, - { - "percent": "10000", - "reputation": 48820807481, - "rshares": 384310591, - "voter": "officialhisha" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "kabitadesign" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "sameer78" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 1788790210, - "voter": "brofund-weed" - } - ], - "author": "dan", - "author_reputation": 99636215095482, - "beneficiaries": [], - "body": "<html>\n<p><img src=\"https://images.hive.blog/DQmNN6qGEQcdS4yQNp3vS2H8tT6AiQSEwFXSNmz59rfme4C/image.png\" alt=\"image.png\"/></p>\n<p>I just posted a sneak peek of a chapter from my upcomming book on how to decentralize power, build consensus, and secure life, liberty, property, and justice for all. You can check it out on my new blog site, <a href=\"https://moreequalanimals.com/posts/Tyranny-of-the-Status-Quo\">More Equal Animals</a>.</p>\n<p>I am encouraging everyone to <a href=\"https://moreequalanimals.com/posts/why-subscribe\">subscribe</a> to my mailing list to be the first to know when my new book becomes available.</p>\n<p>You may recognize some of the content from my prior voice post: <a href=\"https://app.voice.com/post/@dan/can-we-end-riots-with-a-new-kind-of-government-1594063789-1\">"Can we end riots with a new kind of Government?"</a>, I want to thank the Voice and Steem community for helping me build out the ideas in the book. Please leave your thoughts! </p>\n</html>", - "body_length": 995, - "cashout_time": "2020-11-05T18:59:30", - "category": "moreequalanimals", - "children": 145, - "created": "2020-10-29T18:59:30", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"image\":[\"https://images.hive.blog/DQmNN6qGEQcdS4yQNp3vS2H8tT6AiQSEwFXSNmz59rfme4C/image.png\"],\"links\":[\"https://moreequalanimals.com/posts/Tyranny-of-the-Status-Quo\",\"https://moreequalanimals.com/posts/why-subscribe\",\"https://app.voice.com/post/@dan/can-we-end-riots-with-a-new-kind-of-government-1594063789-1\"],\"app\":\"hiveblog/0.1\",\"format\":\"html\"}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-10-29T19:01:21", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 342128675442069, - "parent_author": "", - "parent_permlink": "moreequalanimals", - "pending_payout_value": "80.986 HBD", - "percent_hbd": 10000, - "permlink": "tyranny-of-the-status-quo", - "post_id": 100308140, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Tyranny of the Status Quo", - "title": "Tyranny of the Status Quo", - "total_payout_value": "0.000 HBD", - "url": "/moreequalanimals/@dan/tyranny-of-the-status-quo" - }, - { - "active_votes": [ - { - "percent": "75", - "reputation": 19245698180508, - "rshares": 16086200428, - "voter": "tombstone" - }, - { - "percent": "4500", - "reputation": 341233778618, - "rshares": 704251311751, - "voter": "boatymcboatface" - }, - { - "percent": "10000", - "reputation": 5983374206813, - "rshares": 23261303081, - "voter": "fractalnode" - }, - { - "percent": "2000", - "reputation": 351157717865710, - "rshares": 379320562588, - "voter": "onealfa" - }, - { - "percent": "4500", - "reputation": 1957220358850383, - "rshares": 1608856174104, - "voter": "kingscrown" - }, - { - "percent": "10000", - "reputation": 1594304819447449, - "rshares": 10275674240434, - "voter": "acidyo" - }, - { - "percent": "2500", - "reputation": 506372806974428, - "rshares": 2087159495066, - "voter": "kevinwong" - }, - { - "percent": "4500", - "reputation": 4509451541223, - "rshares": 189085638482, - "voter": "theshell" - }, - { - "percent": "10000", - "reputation": 4233322581773, - "rshares": 85466696868, - "voter": "hedge-x" - }, - { - "percent": "10000", - "reputation": 159372103844946, - "rshares": 21685939783111, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 618931574338675, - "rshares": 730508277511, - "voter": "ericvancewalton" - }, - { - "percent": "1750", - "reputation": 34103703963623, - "rshares": 7841079251, - "voter": "matt-a" - }, - { - "percent": "10000", - "reputation": 229982756480919, - "rshares": 2509669363039, - "voter": "ausbitbank" - }, - { - "percent": "1250", - "reputation": 86171006185191, - "rshares": 4902731372, - "voter": "mrwang" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 292408161360, - "voter": "livingfree" - }, - { - "percent": "10000", - "reputation": 67858732461644, - "rshares": 1124948188970, - "voter": "fulltimegeek" - }, - { - "percent": "5000", - "reputation": 342312471468, - "rshares": 2255863948173, - "voter": "bobbylee" - }, - { - "percent": "10000", - "reputation": 8349683635857, - "rshares": 6068505126, - "voter": "nascimentoab" - }, - { - "percent": "10000", - "reputation": 372226967801214, - "rshares": 46213749241, - "voter": "allmonitors" - }, - { - "percent": "2500", - "reputation": 315496990256842, - "rshares": 1337351083071, - "voter": "arcange" - }, - { - "percent": "10000", - "reputation": 978188457801574, - "rshares": 3326041508714, - "voter": "deanliu" - }, - { - "percent": "1250", - "reputation": 2558692465689, - "rshares": 8943671105, - "voter": "arconite" - }, - { - "percent": "300", - "reputation": 501315151365, - "rshares": 3087897717, - "voter": "raphaelle" - }, - { - "percent": "10000", - "reputation": 98765147786888, - "rshares": 244256857319, - "voter": "logic" - }, - { - "percent": "10000", - "reputation": 90235201589691, - "rshares": 458726296149, - "voter": "always1success" - }, - { - "percent": "10000", - "reputation": 266498838207861, - "rshares": 1473413787814, - "voter": "timcliff" - }, - { - "percent": "10000", - "reputation": 3774393595703, - "rshares": 47266832578, - "voter": "laoyao" - }, - { - "percent": "10000", - "reputation": 6042026377398, - "rshares": 84664245, - "voter": "somebody" - }, - { - "percent": "10000", - "reputation": 97845484474, - "rshares": 165914128626, - "voter": "midnightoil" - }, - { - "percent": "10000", - "reputation": 47820660266221, - "rshares": 938879624746, - "voter": "xiaohui" - }, - { - "percent": "10000", - "reputation": 8868395123281, - "rshares": 5874433204442, - "voter": "jphamer1" - }, - { - "percent": "10000", - "reputation": 1493318183193201, - "rshares": 5455134639791, - "voter": "oflyhigh" - }, - { - "percent": "10000", - "reputation": 143768203235023, - "rshares": 17781335546, - "voter": "shanghaipreneur" - }, - { - "percent": "5000", - "reputation": 50032785591993, - "rshares": 541875322154, - "voter": "bryan-imhoff" - }, - { - "percent": "5500", - "reputation": 133665343804692, - "rshares": 453263084747, - "voter": "borran" - }, - { - "percent": "10000", - "reputation": 32866384013620, - "rshares": 124838087392, - "voter": "frankbacon" - }, - { - "percent": "10000", - "reputation": 97229489927307, - "rshares": 347990798652, - "voter": "rubenalexander" - }, - { - "percent": "10000", - "reputation": 181963199440755, - "rshares": 1235057559289, - "voter": "helene" - }, - { - "percent": "10000", - "reputation": 30591567767954, - "rshares": 78609219497, - "voter": "the-bitcoin-dood" - }, - { - "percent": "3000", - "reputation": 113291389678283, - "rshares": 651295775, - "voter": "jsantana" - }, - { - "percent": "1300", - "reputation": 363924035887507, - "rshares": 184658274359, - "voter": "steevc" - }, - { - "percent": "5000", - "reputation": 134570234493033, - "rshares": 83203692789, - "voter": "shadowspub" - }, - { - "percent": "10000", - "reputation": 461742024181352, - "rshares": 48181862416, - "voter": "jlufer" - }, - { - "percent": "10000", - "reputation": 7886415406854, - "rshares": 54455644588, - "voter": "notconvinced" - }, - { - "percent": "7500", - "reputation": 59537594546955, - "rshares": 31248979158, - "voter": "por500bolos" - }, - { - "percent": "500", - "reputation": 505596674036569, - "rshares": 343219339230, - "voter": "penguinpablo" - }, - { - "percent": "2000", - "reputation": 390433925983792, - "rshares": 207004569941, - "voter": "uwelang" - }, - { - "percent": "4900", - "reputation": 96059856770036, - "rshares": 7810231982183, - "voter": "canadian-coconut" - }, - { - "percent": "10000", - "reputation": 262691650197556, - "rshares": 190255477809, - "voter": "titusfrost" - }, - { - "percent": "2500", - "reputation": 706874908331117, - "rshares": 593206136954, - "voter": "abh12345" - }, - { - "percent": "200", - "reputation": 120512824315021, - "rshares": 78521544070, - "voter": "funnyman" - }, - { - "percent": "2250", - "reputation": 280674585514224, - "rshares": 223209890855, - "voter": "justyy" - }, - { - "percent": "1250", - "reputation": 149827038166480, - "rshares": 51269127782, - "voter": "clayboyn" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 56155469204, - "voter": "ssekulji" - }, - { - "percent": "5000", - "reputation": 111778832002739, - "rshares": 281125916902, - "voter": "techslut" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 546070228, - "voter": "triviummethod" - }, - { - "percent": "10000", - "reputation": 234795708570321, - "rshares": 250162412792, - "voter": "jaybird" - }, - { - "percent": "10000", - "reputation": 371604430408116, - "rshares": 2352049499853, - "voter": "nonameslefttouse" - }, - { - "percent": "10000", - "reputation": 118794412395373, - "rshares": 38429796381, - "voter": "alcibiades" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 696967038875, - "voter": "created" - }, - { - "percent": "10000", - "reputation": 4110132379317, - "rshares": 16914614122, - "voter": "sabsel" - }, - { - "percent": "650", - "reputation": 7547083616142, - "rshares": 8145480109, - "voter": "edb" - }, - { - "percent": "10000", - "reputation": 142803808522779, - "rshares": 497662285504, - "voter": "anthonyadavisii" - }, - { - "percent": "2500", - "reputation": 59094884211730, - "rshares": 3536404231, - "voter": "saleg25" - }, - { - "percent": "3590", - "reputation": 101539530127063, - "rshares": 139515252434, - "voter": "edje" - }, - { - "percent": "10000", - "reputation": 19610705040132, - "rshares": 45061925775, - "voter": "askari" - }, - { - "percent": "4000", - "reputation": 7177514553823, - "rshares": 85479566283, - "voter": "gabriele-gio" - }, - { - "percent": "10000", - "reputation": 221144436321945, - "rshares": 74186421185, - "voter": "blackbunny" - }, - { - "percent": "5000", - "reputation": 27214240242904, - "rshares": 42427497108, - "voter": "rahul.stan" - }, - { - "percent": "10000", - "reputation": 1049642294074, - "rshares": 46470622283, - "voter": "cpol" - }, - { - "percent": "10000", - "reputation": 36248450113797, - "rshares": 79380113451, - "voter": "lingfei" - }, - { - "percent": "300", - "reputation": 38975615169260, - "rshares": 7746446308, - "voter": "steemitboard" - }, - { - "percent": "7200", - "reputation": 96863592771723, - "rshares": 87543029500, - "voter": "noemilunastorta" - }, - { - "percent": "10000", - "reputation": 36214470543162, - "rshares": 18152929601, - "voter": "assasin" - }, - { - "percent": "3300", - "reputation": 12325904700970, - "rshares": 7715619853, - "voter": "justinashby" - }, - { - "percent": "10000", - "reputation": 215229803246462, - "rshares": 163624737073, - "voter": "ackza" - }, - { - "percent": "2500", - "reputation": 15925403061479, - "rshares": 1398345398, - "voter": "titianus" - }, - { - "percent": "10000", - "reputation": 450338781685383, - "rshares": 204139815824, - "voter": "louisthomas" - }, - { - "percent": "1000", - "reputation": 1614643030899, - "rshares": 1916983070, - "voter": "freebornsociety" - }, - { - "percent": "7523", - "reputation": 307916617000098, - "rshares": 784037069415, - "voter": "detlev" - }, - { - "percent": "4000", - "reputation": 42214550905806, - "rshares": 1607099325744, - "voter": "smasssh" - }, - { - "percent": "10000", - "reputation": 16365587743468, - "rshares": 114025257088, - "voter": "tamaralovelace" - }, - { - "percent": "10000", - "reputation": 74329552872641, - "rshares": 287833641207, - "voter": "mes" - }, - { - "percent": "8000", - "reputation": 79234586811944, - "rshares": 237694492076, - "voter": "thenightflier" - }, - { - "percent": "2500", - "reputation": 91224250124, - "rshares": 5659348373, - "voter": "kingkinslow" - }, - { - "percent": "10000", - "reputation": 813135864401476, - "rshares": 14042097917673, - "voter": "broncnutz" - }, - { - "percent": "3000", - "reputation": 216790353857759, - "rshares": 267285100372, - "voter": "barbara-orenya" - }, - { - "percent": "5500", - "reputation": 29845436743688, - "rshares": 380054915869, - "voter": "cryptocurator" - }, - { - "percent": "3500", - "reputation": 39969242565947, - "rshares": 63742832197, - "voter": "iansart" - }, - { - "percent": "2000", - "reputation": 23391602452678, - "rshares": 74200911951, - "voter": "schlees" - }, - { - "percent": "2000", - "reputation": 5899232344203, - "rshares": 567311585, - "voter": "yeaho" - }, - { - "percent": "10000", - "reputation": 1748651637089, - "rshares": 3820772003, - "voter": "khussan" - }, - { - "percent": "10000", - "reputation": 832896994040741, - "rshares": 7646134544, - "voter": "stackin" - }, - { - "percent": "2500", - "reputation": 76741990090791, - "rshares": 25183415631, - "voter": "valued-customer" - }, - { - "percent": "187", - "reputation": 11448902903972, - "rshares": 94505453650, - "voter": "roomservice" - }, - { - "percent": "10000", - "reputation": 294281694879864, - "rshares": 1549506020662, - "voter": "bigram13" - }, - { - "percent": "10000", - "reputation": 130573901548108, - "rshares": 972551680423, - "voter": "fredrikaa" - }, - { - "percent": "10000", - "reputation": 19675504562112, - "rshares": 278576232246, - "voter": "amirl" - }, - { - "percent": "10000", - "reputation": 112994806497327, - "rshares": 2971986767, - "voter": "varunpinto" - }, - { - "percent": "2500", - "reputation": 441960688612864, - "rshares": 547566255789, - "voter": "isaria" - }, - { - "percent": "10000", - "reputation": 23634061827279, - "rshares": 143430412337, - "voter": "stevelivingston" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 251410733083, - "voter": "exec" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 853252545, - "voter": "eval" - }, - { - "percent": "10000", - "reputation": 7901101123497, - "rshares": 1089772483896, - "voter": "giuatt07" - }, - { - "percent": "3000", - "reputation": 69653104045391, - "rshares": 34173931906, - "voter": "anacristinasilva" - }, - { - "percent": "10000", - "reputation": 655474560066583, - "rshares": 2245055825521, - "voter": "galenkp" - }, - { - "percent": "10000", - "reputation": 22475002262691, - "rshares": 40875446142, - "voter": "reddragonfly" - }, - { - "percent": "2900", - "reputation": 19643697626632, - "rshares": 121936674207, - "voter": "sam99" - }, - { - "percent": "2500", - "reputation": 383145046100830, - "rshares": 438752350468, - "voter": "jaynie" - }, - { - "percent": "3000", - "reputation": 104644958945964, - "rshares": 1543424153624, - "voter": "spectrumecons" - }, - { - "percent": "1000", - "reputation": 120327041068001, - "rshares": 34353926980, - "voter": "jayna" - }, - { - "percent": "2500", - "reputation": 30158976168359, - "rshares": 5521466428, - "voter": "guchtere" - }, - { - "percent": "5000", - "reputation": 698336179865, - "rshares": 536782942, - "voter": "sirjaxxy" - }, - { - "percent": "5000", - "reputation": 284852594097573, - "rshares": 3114198335, - "voter": "reseller" - }, - { - "percent": "10000", - "reputation": 13037201970659, - "rshares": 47537694086, - "voter": "corsica" - }, - { - "percent": "10000", - "reputation": 582437706834948, - "rshares": 468926689581, - "voter": "theouterlight" - }, - { - "percent": "10000", - "reputation": 76998450222036, - "rshares": 670117682485, - "voter": "forexbrokr" - }, - { - "percent": "6500", - "reputation": 14619210831916, - "rshares": 8459408422, - "voter": "kiokizz" - }, - { - "percent": "3000", - "reputation": 8535083509600, - "rshares": 15196777557, - "voter": "otom" - }, - { - "percent": "2000", - "reputation": 14175507042005, - "rshares": 1536441085, - "voter": "adambarratt" - }, - { - "percent": "10000", - "reputation": 3430589330891, - "rshares": 23842459618, - "voter": "whitelightxpress" - }, - { - "percent": "6500", - "reputation": 0, - "rshares": 1968824549, - "voter": "approximate" - }, - { - "percent": "10000", - "reputation": 91410383693752, - "rshares": 305633680954, - "voter": "vincentnijman" - }, - { - "percent": "10000", - "reputation": 23747652895956, - "rshares": 974520063, - "voter": "richi0927" - }, - { - "percent": "1000", - "reputation": 1060930411809617, - "rshares": 2451886570890, - "voter": "themarkymark" - }, - { - "percent": "10000", - "reputation": 190691885658151, - "rshares": 6379658348572, - "voter": "nathanmars" - }, - { - "percent": "10000", - "reputation": 21870820797138, - "rshares": 44428536036, - "voter": "marian0" - }, - { - "percent": "3500", - "reputation": 36780217425224, - "rshares": 29943894679, - "voter": "dine77" - }, - { - "percent": "10000", - "reputation": 1192307877995, - "rshares": 714927673, - "voter": "teukumuhas" - }, - { - "percent": "3000", - "reputation": 115269542036380, - "rshares": 359194417362, - "voter": "vikisecrets" - }, - { - "percent": "1000", - "reputation": 68448132279986, - "rshares": 784553797, - "voter": "fatherfaith" - }, - { - "percent": "10000", - "reputation": 1465267842688, - "rshares": 1515623027, - "voter": "jorge248" - }, - { - "percent": "10000", - "reputation": 1095186992075950, - "rshares": 176106568320, - "voter": "cryptopassion" - }, - { - "percent": "5000", - "reputation": 10111973688443, - "rshares": 13900613671, - "voter": "gamesjoyce" - }, - { - "percent": "3125", - "reputation": 16251675397221, - "rshares": 68940198678, - "voter": "jasonbu" - }, - { - "percent": "7000", - "reputation": 616021827459, - "rshares": 9248536004, - "voter": "yacobh" - }, - { - "percent": "2750", - "reputation": 61249536206183, - "rshares": 31469249449, - "voter": "redrica" - }, - { - "percent": "1000", - "reputation": 73283380450035, - "rshares": 104012852871, - "voter": "shanibeer" - }, - { - "percent": "10000", - "reputation": 57682371069310, - "rshares": 27001150804, - "voter": "gardeningchannel" - }, - { - "percent": "5000", - "reputation": 26317620061322, - "rshares": 2083980792, - "voter": "foreveraverage" - }, - { - "percent": "10000", - "reputation": 659946748896, - "rshares": 1518819180, - "voter": "nascimentocb" - }, - { - "percent": "10000", - "reputation": 72946432090481, - "rshares": 135023906368, - "voter": "my451r" - }, - { - "percent": "10000", - "reputation": 16348092082289, - "rshares": 742323761, - "voter": "pojan" - }, - { - "percent": "6000", - "reputation": 24639928209538, - "rshares": 36356938622, - "voter": "santigs" - }, - { - "percent": "10000", - "reputation": 35972230673675, - "rshares": 238750767032, - "voter": "reinhard-schmid" - }, - { - "percent": "10000", - "reputation": 196571377648, - "rshares": 18495791759, - "voter": "nadhora" - }, - { - "percent": "2500", - "reputation": 54379045488206, - "rshares": 69952029739, - "voter": "bashadow" - }, - { - "percent": "3000", - "reputation": 67191720608970, - "rshares": 103490871513, - "voter": "pele23" - }, - { - "percent": "400", - "reputation": 101280142849344, - "rshares": 10879688246, - "voter": "kimzwarch" - }, - { - "percent": "10000", - "reputation": 72347595650439, - "rshares": 1212352667, - "voter": "ponpase" - }, - { - "percent": "2500", - "reputation": 5754775236968, - "rshares": 1092976659, - "voter": "nurhayati" - }, - { - "percent": "10000", - "reputation": 140483466255317, - "rshares": 495305723811, - "voter": "fbslo" - }, - { - "percent": "1000", - "reputation": 355541395288151, - "rshares": 7647003657799, - "voter": "buildawhale" - }, - { - "percent": "10000", - "reputation": 275615258032, - "rshares": 11321298283, - "voter": "eurodale" - }, - { - "percent": "10000", - "reputation": 669346799793, - "rshares": 20125476238, - "voter": "voxmonkey" - }, - { - "percent": "10000", - "reputation": 51585017229874, - "rshares": 92068044228, - "voter": "eastmael" - }, - { - "percent": "1000", - "reputation": 139904553806579, - "rshares": 580496912803, - "voter": "joshman" - }, - { - "percent": "10000", - "reputation": 110750156547770, - "rshares": 407917800538, - "voter": "drax" - }, - { - "percent": "5000", - "reputation": 399247061484993, - "rshares": 339072772009, - "voter": "rosatravels" - }, - { - "percent": "250", - "reputation": 445234100950820, - "rshares": 797033163346, - "voter": "therealwolf" - }, - { - "percent": "10000", - "reputation": 1386913003857667, - "rshares": 3064762519960, - "voter": "taskmaster4450" - }, - { - "percent": "5000", - "reputation": 72384838675160, - "rshares": 297935797891, - "voter": "roleerob" - }, - { - "percent": "3775", - "reputation": 0, - "rshares": 66615754127, - "voter": "fatman" - }, - { - "percent": "10000", - "reputation": 48712593060561, - "rshares": 22126410630, - "voter": "albanna" - }, - { - "percent": "5000", - "reputation": 336063014350538, - "rshares": 708913924739, - "voter": "revisesociology" - }, - { - "percent": "2500", - "reputation": 21798737047108, - "rshares": 50142319408, - "voter": "yangyanje" - }, - { - "percent": "10000", - "reputation": 206055018792025, - "rshares": 51531066937, - "voter": "silversaver888" - }, - { - "percent": "9000", - "reputation": 28057351253216, - "rshares": 578762657, - "voter": "superbing" - }, - { - "percent": "500", - "reputation": 1179789091392, - "rshares": 1073577058, - "voter": "superdavey" - }, - { - "percent": "10000", - "reputation": 3054701407913, - "rshares": 2060296850, - "voter": "thatterrioguy" - }, - { - "percent": "10000", - "reputation": 58282771573923, - "rshares": 35555344332, - "voter": "dilimunanzar" - }, - { - "percent": "2250", - "reputation": 64078918102750, - "rshares": 14950418590, - "voter": "dailystats" - }, - { - "percent": "5000", - "reputation": 104418116636989, - "rshares": 24717704281, - "voter": "mcfarhat" - }, - { - "percent": "3500", - "reputation": 57287800105812, - "rshares": 1190222358, - "voter": "calimeatwagon" - }, - { - "percent": "4000", - "reputation": 38394184486553, - "rshares": 160587385126, - "voter": "sharpshot" - }, - { - "percent": "5000", - "reputation": 6727973666699, - "rshares": 83681121277, - "voter": "omra-sky" - }, - { - "percent": "1800", - "reputation": 137567392768, - "rshares": 2292147442, - "voter": "rodent" - }, - { - "percent": "1000", - "reputation": 47982158911387, - "rshares": 30907193623, - "voter": "makerhacks" - }, - { - "percent": "10000", - "reputation": 1876754372524, - "rshares": 9536344261, - "voter": "shadflyfilms" - }, - { - "percent": "8000", - "reputation": 12066290877974, - "rshares": 61047803396, - "voter": "heidi71" - }, - { - "percent": "240", - "reputation": 0, - "rshares": 84880205158, - "voter": "investegg" - }, - { - "percent": "10000", - "reputation": 24343533253934, - "rshares": 30695655004, - "voter": "doifeellucky" - }, - { - "percent": "3000", - "reputation": 38950506479154, - "rshares": 19966663618, - "voter": "vegoutt-travel" - }, - { - "percent": "3000", - "reputation": 513799575547713, - "rshares": 175780804531, - "voter": "josediccus" - }, - { - "percent": "10000", - "reputation": 3291168947960, - "rshares": 3502523089, - "voter": "enolife" - }, - { - "percent": "10000", - "reputation": 30679468047783, - "rshares": 10043853944548, - "voter": "fedesox" - }, - { - "percent": "2000", - "reputation": 10939046475227, - "rshares": 747282667, - "voter": "liverpool-fan" - }, - { - "percent": "10000", - "reputation": 57597252449855, - "rshares": 442688817672, - "voter": "florian-glechner" - }, - { - "percent": "5000", - "reputation": 12918217107297, - "rshares": 5401542105, - "voter": "afterglow" - }, - { - "percent": "10000", - "reputation": 103915899650487, - "rshares": 770740822027, - "voter": "traciyork" - }, - { - "percent": "10000", - "reputation": 26544594519126, - "rshares": 20710455121, - "voter": "dkid14" - }, - { - "percent": "10000", - "reputation": 1737660226555, - "rshares": 1495444662, - "voter": "meincluyo" - }, - { - "percent": "10000", - "reputation": 24529315355817, - "rshares": 26767284235, - "voter": "mrhill" - }, - { - "percent": "10000", - "reputation": 473928985768900, - "rshares": 3108869836, - "voter": "tradingideas" - }, - { - "percent": "5000", - "reputation": 12722616650811, - "rshares": 2180264376751, - "voter": "postpromoter" - }, - { - "percent": "10000", - "reputation": 14210818977999, - "rshares": 20075552734, - "voter": "faizarfatria" - }, - { - "percent": "5000", - "reputation": 31360323509866, - "rshares": 65363267772, - "voter": "steveconnor" - }, - { - "percent": "4000", - "reputation": 1989736847412, - "rshares": 1336069741, - "voter": "kph" - }, - { - "percent": "250", - "reputation": 157805541487641, - "rshares": 157524565552, - "voter": "smartsteem" - }, - { - "percent": "5000", - "reputation": 61257367144299, - "rshares": 609875970, - "voter": "publicumaurora" - }, - { - "percent": "10000", - "reputation": 15317934745865, - "rshares": 177839785447, - "voter": "yogajill" - }, - { - "percent": "10000", - "reputation": 2045506354809, - "rshares": 1841944151, - "voter": "steeminer4up" - }, - { - "percent": "10000", - "reputation": 42134765111603, - "rshares": 24099834312, - "voter": "mariana4ve" - }, - { - "percent": "10000", - "reputation": 5341088715064, - "rshares": 1824435485, - "voter": "aquinotyron3" - }, - { - "percent": "5000", - "reputation": 66785151966461, - "rshares": 239610317626, - "voter": "itchyfeetdonica" - }, - { - "percent": "10000", - "reputation": 61297887349807, - "rshares": 21692651597, - "voter": "nathen007" - }, - { - "percent": "5000", - "reputation": 12709262361968, - "rshares": 3125218183, - "voter": "syedubair" - }, - { - "percent": "10000", - "reputation": 9904578498117, - "rshares": 20960218510, - "voter": "vaneaventuras" - }, - { - "percent": "10000", - "reputation": 9896237488, - "rshares": 18232051421, - "voter": "howiemac" - }, - { - "percent": "2500", - "reputation": 110889227376345, - "rshares": 2254030035, - "voter": "rpcaceres" - }, - { - "percent": "5000", - "reputation": 5994128131575, - "rshares": 1928798343, - "voter": "jrungi" - }, - { - "percent": "5000", - "reputation": 618706115961, - "rshares": 602896103, - "voter": "lethsrock" - }, - { - "percent": "2500", - "reputation": 2675225025765, - "rshares": 7621234115, - "voter": "fourfourfun" - }, - { - "percent": "1000", - "reputation": 2680015924516, - "rshares": 7380054457, - "voter": "upmyvote" - }, - { - "percent": "10000", - "reputation": 65152755152316, - "rshares": 35071844739, - "voter": "theonlyway" - }, - { - "percent": "10000", - "reputation": 73184833081444, - "rshares": 4033818019247, - "voter": "oliverschmid" - }, - { - "percent": "1500", - "reputation": 305437093461437, - "rshares": 28446874347, - "voter": "abitcoinskeptic" - }, - { - "percent": "3000", - "reputation": 6074854639098, - "rshares": 47736061218, - "voter": "deepresearch" - }, - { - "percent": "10000", - "reputation": 36559175792044, - "rshares": 1661009865, - "voter": "jewel-lover" - }, - { - "percent": "10000", - "reputation": 106197032747732, - "rshares": 36328305572, - "voter": "kevinli" - }, - { - "percent": "5000", - "reputation": 87246032649758, - "rshares": 97540871011, - "voter": "gabrielatravels" - }, - { - "percent": "10000", - "reputation": 368970410036642, - "rshares": 1550718736800, - "voter": "jongolson" - }, - { - "percent": "1000", - "reputation": 6794111379268, - "rshares": 1095459511, - "voter": "dudeontheweb" - }, - { - "percent": "7523", - "reputation": 86529641974877, - "rshares": 444117712036, - "voter": "wehmoen" - }, - { - "percent": "7500", - "reputation": 7648880714525, - "rshares": 885776151, - "voter": "mirkon86" - }, - { - "percent": "500", - "reputation": 25048759621308, - "rshares": 4680121827, - "voter": "tryskele" - }, - { - "percent": "10000", - "reputation": 279507826168043, - "rshares": 2505291368865, - "voter": "edicted" - }, - { - "percent": "10000", - "reputation": 3895318402889, - "rshares": 1505156474, - "voter": "criptonotas" - }, - { - "percent": "5100", - "reputation": 127592029555558, - "rshares": 573745370292, - "voter": "chorock" - }, - { - "percent": "10000", - "reputation": 170235537022, - "rshares": 1407016368, - "voter": "auracraft" - }, - { - "percent": "3000", - "reputation": 62275371513698, - "rshares": 1147802445, - "voter": "vaansteam" - }, - { - "percent": "4000", - "reputation": 164071035369220, - "rshares": 164100452627, - "voter": "ikrahch" - }, - { - "percent": "1250", - "reputation": 4755551366717, - "rshares": 859269575, - "voter": "philnewton" - }, - { - "percent": "10000", - "reputation": 63812007256914, - "rshares": 75814761618, - "voter": "k-banti" - }, - { - "percent": "750", - "reputation": 79537112262409, - "rshares": 13537150130, - "voter": "tobias-g" - }, - { - "percent": "1000", - "reputation": 4436444427883, - "rshares": 884864541, - "voter": "tricki" - }, - { - "percent": "10000", - "reputation": 216783552688214, - "rshares": 50021604542, - "voter": "chronocrypto" - }, - { - "percent": "10000", - "reputation": 11165935981106, - "rshares": 703884534, - "voter": "icuz" - }, - { - "percent": "10000", - "reputation": 91651981795187, - "rshares": 23861837880, - "voter": "lunaticpandora" - }, - { - "percent": "3300", - "reputation": 330396781271113, - "rshares": 1495430312785, - "voter": "holger80" - }, - { - "percent": "2500", - "reputation": 403417137596680, - "rshares": 29948301028, - "voter": "adetorrent" - }, - { - "percent": "2250", - "reputation": 50124640567504, - "rshares": 7626194035, - "voter": "dailychina" - }, - { - "percent": "10000", - "reputation": 22367616882821, - "rshares": 5724629132, - "voter": "mr-phoenix" - }, - { - "percent": "10000", - "reputation": 86916035515124, - "rshares": 126653132091, - "voter": "cryptosharon" - }, - { - "percent": "5000", - "reputation": 8496919418242, - "rshares": 2910635420, - "voter": "ericburgoyne" - }, - { - "percent": "7000", - "reputation": 39443399637767, - "rshares": 23760853157, - "voter": "joelai" - }, - { - "percent": "10000", - "reputation": 110218754575910, - "rshares": 366848860185, - "voter": "eddiespino" - }, - { - "percent": "9500", - "reputation": 4819938972371, - "rshares": 6798746627, - "voter": "green77" - }, - { - "percent": "7523", - "reputation": 26891833471686, - "rshares": 155135315700, - "voter": "sisygoboom" - }, - { - "percent": "10000", - "reputation": 1275793729622, - "rshares": 4458881104, - "voter": "macslin" - }, - { - "percent": "5000", - "reputation": 1912983934049, - "rshares": 1014671584, - "voter": "overmedia" - }, - { - "percent": "10000", - "reputation": 20304784933273, - "rshares": 185107629518, - "voter": "barge" - }, - { - "percent": "5000", - "reputation": 2823573898487, - "rshares": 2977144452, - "voter": "thisbejake" - }, - { - "percent": "10000", - "reputation": 17846302258319, - "rshares": 42832708473, - "voter": "onlavu" - }, - { - "percent": "10000", - "reputation": 12186132605, - "rshares": 1631178120, - "voter": "magicbirds" - }, - { - "percent": "10000", - "reputation": 8653571432, - "rshares": 1632849801, - "voter": "blueproject" - }, - { - "percent": "10000", - "reputation": 41394952718, - "rshares": 1633758208, - "voter": "django137" - }, - { - "percent": "10000", - "reputation": 5904893661018, - "rshares": 1499325491, - "voter": "fiveelements5" - }, - { - "percent": "10000", - "reputation": 8493141387, - "rshares": 1632963152, - "voter": "goldeneye64" - }, - { - "percent": "10000", - "reputation": 65151962039, - "rshares": 1629786533, - "voter": "ludezma" - }, - { - "percent": "10000", - "reputation": 11867738310700, - "rshares": 1496070234, - "voter": "oleg5430" - }, - { - "percent": "500", - "reputation": 192724898646069, - "rshares": 34945001741, - "voter": "bozz" - }, - { - "percent": "10000", - "reputation": 2506608157147, - "rshares": 78990262760, - "voter": "cst90" - }, - { - "percent": "10000", - "reputation": 64242146543076, - "rshares": 208149212739, - "voter": "citimillz" - }, - { - "percent": "250", - "reputation": 26898775193091, - "rshares": 2359914423, - "voter": "movement19" - }, - { - "percent": "3800", - "reputation": 120749150606820, - "rshares": 123495760917, - "voter": "hosgug" - }, - { - "percent": "10000", - "reputation": 20074444806548, - "rshares": 7598815188, - "voter": "arac" - }, - { - "percent": "4200", - "reputation": 192465234875420, - "rshares": 240516819527, - "voter": "whack.science" - }, - { - "percent": "500", - "reputation": 6268791987206, - "rshares": 4035121613, - "voter": "ajaxalot" - }, - { - "percent": "10000", - "reputation": 83447433709898, - "rshares": 549874532953, - "voter": "ashtv" - }, - { - "percent": "3000", - "reputation": 62406194635091, - "rshares": 13240230600, - "voter": "russellstockley" - }, - { - "percent": "10000", - "reputation": 5067166508268, - "rshares": 102719018593, - "voter": "kitalee" - }, - { - "percent": "1750", - "reputation": 17385822919900, - "rshares": 8618265674, - "voter": "forester-joe" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 29366912369, - "voter": "futurecurrency" - }, - { - "percent": "2500", - "reputation": 18673367244, - "rshares": 10606324839, - "voter": "g4fun" - }, - { - "percent": "10000", - "reputation": 73042470414733, - "rshares": 410632113004, - "voter": "rubencress" - }, - { - "percent": "5000", - "reputation": 15537102540153, - "rshares": 46166503453, - "voter": "siphon" - }, - { - "percent": "5500", - "reputation": 93067317204510, - "rshares": 101721191372, - "voter": "almi" - }, - { - "percent": "10000", - "reputation": 6368389637818, - "rshares": 323090352, - "voter": "dunkman" - }, - { - "percent": "10000", - "reputation": 168836422039217, - "rshares": 51906598313, - "voter": "fego" - }, - { - "percent": "10000", - "reputation": 3414691338324, - "rshares": 2324226052, - "voter": "homeginkit" - }, - { - "percent": "5000", - "reputation": 743605910590, - "rshares": 1001710713, - "voter": "carlosbp" - }, - { - "percent": "10000", - "reputation": 24659735546029, - "rshares": 35023961681, - "voter": "ange.nkuru" - }, - { - "percent": "5000", - "reputation": 2256065348315, - "rshares": 8412368988, - "voter": "frassman" - }, - { - "percent": "10000", - "reputation": 3441314325667, - "rshares": 126869932105, - "voter": "norwegianbikeman" - }, - { - "percent": "2500", - "reputation": 158256810302501, - "rshares": 40166825319, - "voter": "miroslavrc" - }, - { - "percent": "700", - "reputation": 62798275108724, - "rshares": 31894598501, - "voter": "j85063" - }, - { - "percent": "5000", - "reputation": 45433987678513, - "rshares": 37736794245, - "voter": "khaimi" - }, - { - "percent": "10000", - "reputation": 15649272505736, - "rshares": 29566925897, - "voter": "lionsuit" - }, - { - "percent": "2000", - "reputation": 115008738303016, - "rshares": 3155674099984, - "voter": "azircon" - }, - { - "percent": "10000", - "reputation": 397421034392, - "rshares": 170435476381, - "voter": "steemitcolombia" - }, - { - "percent": "5000", - "reputation": 1137313020354, - "rshares": 1789481616, - "voter": "nebuladream" - }, - { - "percent": "2000", - "reputation": 492319527534844, - "rshares": 150371207554, - "voter": "ronaldoavelino" - }, - { - "percent": "7523", - "reputation": 367201484924059, - "rshares": 512716568063, - "voter": "louis88" - }, - { - "percent": "9000", - "reputation": 1108677568897, - "rshares": 5762250392, - "voter": "jan23com" - }, - { - "percent": "10000", - "reputation": 28643888754007, - "rshares": 33272878263, - "voter": "paragism" - }, - { - "percent": "10000", - "reputation": 4110594694460, - "rshares": 1102323464966, - "voter": "jkramer" - }, - { - "percent": "10000", - "reputation": 174856977309980, - "rshares": 347151209049, - "voter": "ivansnz" - }, - { - "percent": "10000", - "reputation": 4038024331096, - "rshares": 497302434843, - "voter": "foxon" - }, - { - "percent": "4000", - "reputation": 17410482134249, - "rshares": 16215547104, - "voter": "wolfhart" - }, - { - "percent": "10000", - "reputation": 489416883814, - "rshares": 539113079, - "voter": "presidentslabber" - }, - { - "percent": "2000", - "reputation": 50091053272936, - "rshares": 66019534419, - "voter": "yameen" - }, - { - "percent": "5000", - "reputation": 52997770938854, - "rshares": 74246874449, - "voter": "prydefoltz" - }, - { - "percent": "1000", - "reputation": 23942138274308, - "rshares": 22184197928, - "voter": "braaiboy" - }, - { - "percent": "1500", - "reputation": 183095375076710, - "rshares": 24132727400, - "voter": "prettynicevideo" - }, - { - "percent": "10000", - "reputation": 26253168400063, - "rshares": 2455293419879, - "voter": "rawutah" - }, - { - "percent": "10000", - "reputation": 1451811665047, - "rshares": 6726424792, - "voter": "orkin420" - }, - { - "percent": "3000", - "reputation": 570270524259, - "rshares": 33207559331, - "voter": "blainjones" - }, - { - "percent": "10000", - "reputation": 14193144291158, - "rshares": 6226435596, - "voter": "abdulmath" - }, - { - "percent": "384", - "reputation": 40776009332161, - "rshares": 5888491337, - "voter": "gadrian" - }, - { - "percent": "10000", - "reputation": 151166868816119, - "rshares": 23158949372, - "voter": "jordangerder" - }, - { - "percent": "3761", - "reputation": 112109667795, - "rshares": 1126113308, - "voter": "memepress" - }, - { - "percent": "10000", - "reputation": 13910589758110, - "rshares": 11890782503, - "voter": "garybilbao" - }, - { - "percent": "10000", - "reputation": 18055325674078, - "rshares": 139092185510, - "voter": "themightyvolcano" - }, - { - "percent": "9000", - "reputation": 158718167236, - "rshares": 3526241122, - "voter": "choco11oreo11" - }, - { - "percent": "10000", - "reputation": 16499809682833, - "rshares": 21692347234, - "voter": "geadriana" - }, - { - "percent": "10000", - "reputation": 36875713446685, - "rshares": 83164347925, - "voter": "artmom" - }, - { - "percent": "5000", - "reputation": 8020932518298, - "rshares": 6201718616, - "voter": "naruitchi" - }, - { - "percent": "10000", - "reputation": 2481629111221, - "rshares": 2259776061, - "voter": "manojbhatt" - }, - { - "percent": "10000", - "reputation": 28114370178955, - "rshares": 524896136694, - "voter": "adeyemidrey" - }, - { - "percent": "10000", - "reputation": 17764793535203, - "rshares": 10379829649, - "voter": "josebenavente" - }, - { - "percent": "5000", - "reputation": 162917506903765, - "rshares": 182982538965, - "voter": "artemislives" - }, - { - "percent": "10000", - "reputation": 42246987760, - "rshares": 8670118750, - "voter": "feedmytwi" - }, - { - "percent": "2000", - "reputation": 1072061813246, - "rshares": 3909813929, - "voter": "yaraha" - }, - { - "percent": "10000", - "reputation": 9020628089607, - "rshares": 92951727435, - "voter": "promobot" - }, - { - "percent": "1000", - "reputation": 36024596907536, - "rshares": 13628464480, - "voter": "incubot" - }, - { - "percent": "5000", - "reputation": 48846679188, - "rshares": 634140016, - "voter": "youraverageguy" - }, - { - "percent": "10000", - "reputation": 25780595648429, - "rshares": 24653500609, - "voter": "superlao" - }, - { - "percent": "3000", - "reputation": 10484590752220, - "rshares": 51897507886, - "voter": "veteranforcrypto" - }, - { - "percent": "10000", - "reputation": 13196413386545, - "rshares": 18472541383, - "voter": "dunite" - }, - { - "percent": "500", - "reputation": 120756196920388, - "rshares": 1883139055, - "voter": "playdice" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 18657571412, - "voter": "diabonua" - }, - { - "percent": "5000", - "reputation": 28237853787625, - "rshares": 556937306, - "voter": "crystalhuman" - }, - { - "percent": "5000", - "reputation": 8665552760808, - "rshares": 5025246232, - "voter": "aceaeterna" - }, - { - "percent": "10000", - "reputation": 1131137096970, - "rshares": 3939405711, - "voter": "justasperm" - }, - { - "percent": "1000", - "reputation": 1993672004294, - "rshares": 51069871834, - "voter": "pladozero" - }, - { - "percent": "1000", - "reputation": 1917096247997, - "rshares": 564948543, - "voter": "crimo" - }, - { - "percent": "10000", - "reputation": 70214892950356, - "rshares": 3777011720819, - "voter": "nateaguila" - }, - { - "percent": "10000", - "reputation": 9095206, - "rshares": 868790358565, - "voter": "steem-tube" - }, - { - "percent": "10000", - "reputation": 6746593076733, - "rshares": 52604298634, - "voter": "aliento" - }, - { - "percent": "5000", - "reputation": 1915818854367, - "rshares": 1273661284, - "voter": "parauri" - }, - { - "percent": "10000", - "reputation": 16608311881044, - "rshares": 50254983406, - "voter": "curatorcat" - }, - { - "percent": "5900", - "reputation": 42499254461187, - "rshares": 77982799392, - "voter": "oldmans" - }, - { - "percent": "6000", - "reputation": 1123068542384, - "rshares": 1485065472, - "voter": "juliocesar7" - }, - { - "percent": "4000", - "reputation": 71049104003913, - "rshares": 38577356885, - "voter": "irvinc" - }, - { - "percent": "10000", - "reputation": 2634900617603, - "rshares": 3472237400, - "voter": "vampire-steem" - }, - { - "percent": "5000", - "reputation": 3261043121278, - "rshares": 2266006009, - "voter": "somegaming" - }, - { - "percent": "10000", - "reputation": 18918733577, - "rshares": 376261937, - "voter": "youngelder" - }, - { - "percent": "250", - "reputation": 20023805487967, - "rshares": 866928990, - "voter": "moneybaby" - }, - { - "percent": "5500", - "reputation": 17562491646897, - "rshares": 4925114454, - "voter": "newsnownorthwest" - }, - { - "percent": "10000", - "reputation": 6184938444683, - "rshares": 7042136768, - "voter": "sthephany" - }, - { - "percent": "1000", - "reputation": 193071506988065, - "rshares": 251003604992, - "voter": "blewitt" - }, - { - "percent": "10000", - "reputation": 9718632602, - "rshares": 1631992415, - "voter": "prudencia" - }, - { - "percent": "10000", - "reputation": 12580096938, - "rshares": 1629203573, - "voter": "gorka" - }, - { - "percent": "10000", - "reputation": 13059317148, - "rshares": 1630286186, - "voter": "suley" - }, - { - "percent": "10000", - "reputation": 6990715593, - "rshares": 1629153113, - "voter": "zulema" - }, - { - "percent": "10000", - "reputation": 10089127541, - "rshares": 1631037458, - "voter": "herencia" - }, - { - "percent": "10000", - "reputation": 9724555279, - "rshares": 1628646790, - "voter": "zoraida" - }, - { - "percent": "10000", - "reputation": 4252099247, - "rshares": 1629683972, - "voter": "embera" - }, - { - "percent": "10000", - "reputation": 6224691662, - "rshares": 1630340713, - "voter": "medula" - }, - { - "percent": "10000", - "reputation": 3158689060, - "rshares": 1710358959, - "voter": "chavela" - }, - { - "percent": "10000", - "reputation": 7291463397, - "rshares": 1629183854, - "voter": "tierra" - }, - { - "percent": "10000", - "reputation": 17043601892482, - "rshares": 29174606223, - "voter": "k0wsk1" - }, - { - "percent": "10000", - "reputation": 1943885599331, - "rshares": 992889763, - "voter": "noobster" - }, - { - "percent": "4000", - "reputation": 37902408766598, - "rshares": 132521182078, - "voter": "cmplxty" - }, - { - "percent": "9500", - "reputation": 33860216986858, - "rshares": 560437745488, - "voter": "donald.porter" - }, - { - "percent": "10000", - "reputation": 18701622566955, - "rshares": 37645021432, - "voter": "ambiguity" - }, - { - "percent": "5000", - "reputation": 3516028097696, - "rshares": 37695915663464, - "voter": "ocdb" - }, - { - "percent": "10000", - "reputation": 570535977, - "rshares": 646584112, - "voter": "therealnigerianp" - }, - { - "percent": "2000", - "reputation": 31223477279974, - "rshares": 3035519566, - "voter": "tiffany4ever" - }, - { - "percent": "10000", - "reputation": 21771305129128, - "rshares": 3287026710, - "voter": "mightypanda" - }, - { - "percent": "2000", - "reputation": 6592285898816, - "rshares": 11783146719, - "voter": "schlunior" - }, - { - "percent": "10000", - "reputation": 1487820702005, - "rshares": 859850454, - "voter": "rufruf" - }, - { - "percent": "10000", - "reputation": 20224059636954, - "rshares": 24325962192, - "voter": "adonisr" - }, - { - "percent": "3100", - "reputation": 3017320382626, - "rshares": 12209203935, - "voter": "yestermorrow" - }, - { - "percent": "7000", - "reputation": 44525854399402, - "rshares": 118173256526, - "voter": "thehive" - }, - { - "percent": "10000", - "reputation": 1615900628665, - "rshares": 1089130523, - "voter": "elmauza" - }, - { - "percent": "1000", - "reputation": 52837110172217, - "rshares": 645695407, - "voter": "jk6276" - }, - { - "percent": "10000", - "reputation": 2376783964081, - "rshares": 4549624475, - "voter": "arrixion" - }, - { - "percent": "5000", - "reputation": 2880166082909, - "rshares": 1431222909, - "voter": "kepslok" - }, - { - "percent": "10000", - "reputation": 88520220786775, - "rshares": 64823337164, - "voter": "wendyth16" - }, - { - "percent": "500", - "reputation": 17939211305935, - "rshares": 25710107088, - "voter": "raiseup" - }, - { - "percent": "9000", - "reputation": 22555392895, - "rshares": 5332205801, - "voter": "littleshadow" - }, - { - "percent": "5000", - "reputation": 3425708064391, - "rshares": 32293159090, - "voter": "goingbonkers" - }, - { - "percent": "4000", - "reputation": 1649687301692, - "rshares": 479622147, - "voter": "mrultracheese" - }, - { - "percent": "50", - "reputation": 18790919715448, - "rshares": 7055163109, - "voter": "luppers" - }, - { - "percent": "2500", - "reputation": 7532194699640, - "rshares": 2006271030, - "voter": "cubapl" - }, - { - "percent": "10000", - "reputation": 7854554032620, - "rshares": 6841443796, - "voter": "judethedude" - }, - { - "percent": "10000", - "reputation": 71506876418188, - "rshares": 148673884691, - "voter": "cryptoyzzy" - }, - { - "percent": "2000", - "reputation": 144910360949054, - "rshares": 38655409822, - "voter": "voxmortis" - }, - { - "percent": "10000", - "reputation": 30014809530606, - "rshares": 15655752857, - "voter": "ysmael20" - }, - { - "percent": "9000", - "reputation": 17567685388, - "rshares": 1813205263, - "voter": "joseph6232" - }, - { - "percent": "7500", - "reputation": 19783849759, - "rshares": 3289080821, - "voter": "emaillisahere" - }, - { - "percent": "10000", - "reputation": 836324990221, - "rshares": 4397168788, - "voter": "jokinmenipieleen" - }, - { - "percent": "500", - "reputation": 57031563221, - "rshares": 43928665357, - "voter": "steemaction" - }, - { - "percent": "5000", - "reputation": 19563050817, - "rshares": 627894653, - "voter": "buzzbee" - }, - { - "percent": "3300", - "reputation": 36498710441788, - "rshares": 10585295634, - "voter": "fullnodeupdate" - }, - { - "percent": "10000", - "reputation": 1799537482104, - "rshares": 3058720594, - "voter": "shepherd-stories" - }, - { - "percent": "5000", - "reputation": 770558880558, - "rshares": 16594541320, - "voter": "developspanish" - }, - { - "percent": "10000", - "reputation": 133998446537867, - "rshares": 5693231586, - "voter": "bluengel" - }, - { - "percent": "9900", - "reputation": 77764751586248, - "rshares": 3354099392316, - "voter": "frot" - }, - { - "percent": "10000", - "reputation": 37453744817, - "rshares": 4153053283, - "voter": "caoimhin" - }, - { - "percent": "7500", - "reputation": 27727654562, - "rshares": 1480819889, - "voter": "djtrucker" - }, - { - "percent": "2500", - "reputation": 6660418695187, - "rshares": 654456242, - "voter": "steemituplife" - }, - { - "percent": "10000", - "reputation": 71329447478265, - "rshares": 580374559481, - "voter": "vimm" - }, - { - "percent": "120", - "reputation": 381305299520882, - "rshares": 904125978, - "voter": "alokkumar121" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 18770080533, - "voter": "quediceharry" - }, - { - "percent": "8500", - "reputation": 260876943473, - "rshares": 76750945531, - "voter": "marshalmugi" - }, - { - "percent": "9000", - "reputation": 1786834467, - "rshares": 1722794275, - "voter": "podg3" - }, - { - "percent": "2500", - "reputation": 41288629235348, - "rshares": 2808793432, - "voter": "mariluna" - }, - { - "percent": "5000", - "reputation": 6687049412835, - "rshares": 40470543597, - "voter": "mister-meeseeks" - }, - { - "percent": "9000", - "reputation": 2040204650, - "rshares": 6505303370, - "voter": "misstaken" - }, - { - "percent": "10000", - "reputation": 34340166233452, - "rshares": 4628216109, - "voter": "perfspots" - }, - { - "percent": "10000", - "reputation": 13238201387755, - "rshares": 54464055731, - "voter": "stefano.massari" - }, - { - "percent": "10000", - "reputation": 29250095880922, - "rshares": 7174012775, - "voter": "anroja" - }, - { - "percent": "5000", - "reputation": 552103378980, - "rshares": 34072006883, - "voter": "jimbi" - }, - { - "percent": "2500", - "reputation": 16966885974746, - "rshares": 927647376, - "voter": "steemitcuration" - }, - { - "percent": "10000", - "reputation": 8904532917885, - "rshares": 684245523, - "voter": "jamzmie" - }, - { - "percent": "10000", - "reputation": 321885398962151, - "rshares": 48945719448, - "voter": "the4thmusketeer" - }, - { - "percent": "10000", - "reputation": 1648086760085, - "rshares": 30493870008, - "voter": "diegor" - }, - { - "percent": "6000", - "reputation": 788852406777, - "rshares": 2048003722, - "voter": "thrasher666" - }, - { - "percent": "2000", - "reputation": 570615134196274, - "rshares": 81520573577, - "voter": "priyanarc" - }, - { - "percent": "7000", - "reputation": 89966310084, - "rshares": 454705047, - "voter": "steempope" - }, - { - "percent": "5000", - "reputation": 153344652102711, - "rshares": 37537420651, - "voter": "nonsowrites" - }, - { - "percent": "10000", - "reputation": 51899127306885, - "rshares": 8176171748, - "voter": "mystry360" - }, - { - "percent": "10000", - "reputation": 13222548277758, - "rshares": 292014212962, - "voter": "wil.metcalfe" - }, - { - "percent": "10000", - "reputation": 14391246828388, - "rshares": 19686411758, - "voter": "geeklania" - }, - { - "percent": "10000", - "reputation": 45419481582603, - "rshares": 361173962186, - "voter": "michealb" - }, - { - "percent": "9000", - "reputation": 4187177339, - "rshares": 2017539705, - "voter": "jussbren" - }, - { - "percent": "10000", - "reputation": 30281770348, - "rshares": 3514126029, - "voter": "themightysquid" - }, - { - "percent": "10000", - "reputation": 247504096816, - "rshares": 915390038, - "voter": "apofis" - }, - { - "percent": "10000", - "reputation": 3633716322140, - "rshares": 5749095935, - "voter": "marki99" - }, - { - "percent": "10000", - "reputation": 2934210455665, - "rshares": 6849319769, - "voter": "noeliazul" - }, - { - "percent": "10000", - "reputation": 122960274717009, - "rshares": 187882165000, - "voter": "pablo1601" - }, - { - "percent": "10000", - "reputation": 5493311683937, - "rshares": 104353886903, - "voter": "kekos" - }, - { - "percent": "7000", - "reputation": 5092515559222, - "rshares": 145333258802, - "voter": "kiel91" - }, - { - "percent": "5000", - "reputation": 1867101038475, - "rshares": 1048492524, - "voter": "starrouge" - }, - { - "percent": "3000", - "reputation": 854097216443, - "rshares": 576290107, - "voter": "redheaddemon" - }, - { - "percent": "9900", - "reputation": 4104615584, - "rshares": 70519991561, - "voter": "steem-on-2020" - }, - { - "percent": "10000", - "reputation": 57787971691858, - "rshares": 320730356030, - "voter": "wherein" - }, - { - "percent": "10000", - "reputation": 11499521185204, - "rshares": 20855101623, - "voter": "yuriy4" - }, - { - "percent": "2000", - "reputation": 30517153592067, - "rshares": 6551047513, - "voter": "variedades" - }, - { - "percent": "10000", - "reputation": 157363211781421, - "rshares": 232673583962, - "voter": "equipodelta" - }, - { - "percent": "5000", - "reputation": 1330122595989, - "rshares": 923559888, - "voter": "zerofive" - }, - { - "percent": "10000", - "reputation": 111080703420070, - "rshares": 80146100115, - "voter": "ferrate" - }, - { - "percent": "10000", - "reputation": 13815761380, - "rshares": 43578449011, - "voter": "tigerrkg" - }, - { - "percent": "10000", - "reputation": 671364595259, - "rshares": 1984037965, - "voter": "smon-fan" - }, - { - "percent": "10000", - "reputation": 27846394621362, - "rshares": 19497520066, - "voter": "angelik-a" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 602957476203, - "voter": "bluesniper" - }, - { - "percent": "10000", - "reputation": 393881380327, - "rshares": 971904329, - "voter": "bilderkiste" - }, - { - "percent": "10000", - "reputation": 8193736963639, - "rshares": 1891649238, - "voter": "breakforbook" - }, - { - "percent": "10000", - "reputation": 2488971014315, - "rshares": 1667308323, - "voter": "tr777" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1286581665, - "voter": "sm-jewel" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 43344030607, - "voter": "coriolis" - }, - { - "percent": "5000", - "reputation": 11919855606, - "rshares": 647522070, - "voter": "escuadron201" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1616508543, - "voter": "tr77" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1449048575, - "voter": "smoner" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1685253957, - "voter": "smonian" - }, - { - "percent": "10000", - "reputation": 61527381175492, - "rshares": 257842804360, - "voter": "cnstm" - }, - { - "percent": "10000", - "reputation": 64935903718, - "rshares": 665860519, - "voter": "likuang007" - }, - { - "percent": "6500", - "reputation": 0, - "rshares": 942324384, - "voter": "sm-lvl1" - }, - { - "percent": "10000", - "reputation": 551470681550, - "rshares": 35095518011, - "voter": "whiterosecoffee" - }, - { - "percent": "6500", - "reputation": 126439858549, - "rshares": 688648515, - "voter": "sm-starter-beta" - }, - { - "percent": "10000", - "reputation": 21122210064846, - "rshares": 22380266511, - "voter": "vickyguevara" - }, - { - "percent": "500", - "reputation": -4467669911343, - "rshares": 781832602456, - "voter": "ctime" - }, - { - "percent": "10000", - "reputation": 90366789538083, - "rshares": 25794211756, - "voter": "miguelmederico" - }, - { - "percent": "10000", - "reputation": 774128044503, - "rshares": 1725138152, - "voter": "caribehub" - }, - { - "percent": "10000", - "reputation": 75911580, - "rshares": 189422233111, - "voter": "jamesbattler" - }, - { - "percent": "1000", - "reputation": 670907511465, - "rshares": 1359754189, - "voter": "baasdebeer" - }, - { - "percent": "10000", - "reputation": 5230675637, - "rshares": 1443345146, - "voter": "jjangjjanggirl" - }, - { - "percent": "10000", - "reputation": 644453947703, - "rshares": 1002184346, - "voter": "lianjingmedia" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 5256837230, - "voter": "spiderwhale" - }, - { - "percent": "6500", - "reputation": 19916963405, - "rshares": 973455273, - "voter": "darhainer" - }, - { - "percent": "10000", - "reputation": 665695019321, - "rshares": 965698516, - "voter": "realgoodcontent" - }, - { - "percent": "3000", - "reputation": 16012295789643, - "rshares": 2880675964, - "voter": "cooperfelix" - }, - { - "percent": "10000", - "reputation": 6833021323980, - "rshares": 9516214762, - "voter": "cabalen" - }, - { - "percent": "10000", - "reputation": 362719994675379, - "rshares": 109688140361, - "voter": "dfacademy" - }, - { - "percent": "1000", - "reputation": 127150826252, - "rshares": 1394003991, - "voter": "afternoondrinks" - }, - { - "percent": "10000", - "reputation": 5833218185, - "rshares": 1663547827, - "voter": "smonbear" - }, - { - "percent": "10000", - "reputation": 2910555091868, - "rshares": 1081210894, - "voter": "denizcakmak" - }, - { - "percent": "10000", - "reputation": 42787624679630, - "rshares": 30279713219, - "voter": "hungryharish" - }, - { - "percent": "4000", - "reputation": 38434598893424, - "rshares": 18797718896, - "voter": "historiasamorlez" - }, - { - "percent": "10000", - "reputation": 3148507896676, - "rshares": 11347746078, - "voter": "wolffeys" - }, - { - "percent": "10000", - "reputation": 8974489893990, - "rshares": 10349683964, - "voter": "lionsaturbix" - }, - { - "percent": "10000", - "reputation": 7716047369242, - "rshares": 27788981032, - "voter": "alchemystones" - }, - { - "percent": "1000", - "reputation": 914395073422, - "rshares": 894641563, - "voter": "ilanisnapshots" - }, - { - "percent": "10000", - "reputation": 137410610469, - "rshares": 607834973, - "voter": "theinspiration" - }, - { - "percent": "5000", - "reputation": 4189876953003, - "rshares": 2812897490, - "voter": "hungryanu" - }, - { - "percent": "10000", - "reputation": 173132216601, - "rshares": 616866143, - "voter": "epic4chris" - }, - { - "percent": "600", - "reputation": 161840569289533, - "rshares": 5900083579, - "voter": "engrsayful" - }, - { - "percent": "10000", - "reputation": 305410265087460, - "rshares": 310478958795, - "voter": "claudio83" - }, - { - "percent": "10000", - "reputation": 68146878689125, - "rshares": 502314907538, - "voter": "rollie1212" - }, - { - "percent": "5000", - "reputation": 2180219855181, - "rshares": 1050506583, - "voter": "hungerstream" - }, - { - "percent": "10000", - "reputation": 1269845209863, - "rshares": 1179093178, - "voter": "krunkypuram" - }, - { - "percent": "2000", - "reputation": 1243018036066, - "rshares": 1311862813, - "voter": "wallvater" - }, - { - "percent": "10000", - "reputation": 10223084450765, - "rshares": 3813636218, - "voter": "draconel" - }, - { - "percent": "75", - "reputation": 120936131908085, - "rshares": 5638359773, - "voter": "epicdice" - }, - { - "percent": "9500", - "reputation": 14559983850035, - "rshares": 52636466008, - "voter": "poliwalt10" - }, - { - "percent": "7523", - "reputation": 153229969445473, - "rshares": 40850250699308, - "voter": "threespeak" - }, - { - "percent": "10000", - "reputation": 2127457046090, - "rshares": 139952843020, - "voter": "star.lord" - }, - { - "percent": "10000", - "reputation": 152169516576120, - "rshares": 65680991849, - "voter": "deeanndmathews" - }, - { - "percent": "2500", - "reputation": 2597264781531, - "rshares": 3907162639, - "voter": "akumagai" - }, - { - "percent": "5000", - "reputation": 10702198150786, - "rshares": 141881516638, - "voter": "scholaris" - }, - { - "percent": "10000", - "reputation": 46361104011592, - "rshares": 3074921685, - "voter": "kayda-ventures" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 51497928785, - "voter": "banvie" - }, - { - "percent": "10000", - "reputation": 19219822868653, - "rshares": 103854310035, - "voter": "beerlover" - }, - { - "percent": "10000", - "reputation": 9877498112396, - "rshares": 1295116188, - "voter": "ssc-token" - }, - { - "percent": "1500", - "reputation": 9382926732500, - "rshares": 25472075836, - "voter": "morwen" - }, - { - "percent": "10000", - "reputation": 36087507440149, - "rshares": 786227279014, - "voter": "likwid" - }, - { - "percent": "5000", - "reputation": 2686371810001, - "rshares": 12924184421, - "voter": "x6oc5" - }, - { - "percent": "10000", - "reputation": 36857316180998, - "rshares": 65305418664, - "voter": "blumela" - }, - { - "percent": "10000", - "reputation": 185044670844, - "rshares": 0, - "voter": "tradingideas2" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 12694056640, - "voter": "xyz001" - }, - { - "percent": "250", - "reputation": 20916803594387, - "rshares": 1144062236, - "voter": "leighscotford" - }, - { - "percent": "10000", - "reputation": 499450701404, - "rshares": 728577632, - "voter": "iktisat" - }, - { - "percent": "1200", - "reputation": 2407117821761, - "rshares": 2707329519, - "voter": "travelwritemoney" - }, - { - "percent": "10000", - "reputation": 11624344336093, - "rshares": 380222082137, - "voter": "recording-box" - }, - { - "percent": "10000", - "reputation": 1181351512634, - "rshares": 4059254993, - "voter": "kendra19" - }, - { - "percent": "2500", - "reputation": 301977647, - "rshares": 45132335406, - "voter": "xyz004" - }, - { - "percent": "10000", - "reputation": 442801860940, - "rshares": 18091342609, - "voter": "steemindian" - }, - { - "percent": "750", - "reputation": 0, - "rshares": 1444869138, - "voter": "cryptogambit" - }, - { - "percent": "10000", - "reputation": 64787679421704, - "rshares": 24088546113, - "voter": "katiefreespirit" - }, - { - "percent": "10000", - "reputation": 17647336098195, - "rshares": 36469120802, - "voter": "c21c" - }, - { - "percent": "10000", - "reputation": 6321736492006, - "rshares": 1812784224, - "voter": "waraira777" - }, - { - "percent": "10000", - "reputation": 16098690446016, - "rshares": 28265594987, - "voter": "denisdenis" - }, - { - "percent": "5000", - "reputation": 4535234888457, - "rshares": 2443092824, - "voter": "uncafeyalgomas" - }, - { - "percent": "10000", - "reputation": 9163578879, - "rshares": 536628123, - "voter": "suigener1s" - }, - { - "percent": "10000", - "reputation": 52974480102727, - "rshares": 52929685383, - "voter": "shimozurdo" - }, - { - "percent": "2500", - "reputation": 517055194981, - "rshares": 16222705803, - "voter": "mind.force" - }, - { - "percent": "10000", - "reputation": 513457484733, - "rshares": 1320187500, - "voter": "strongwoman" - }, - { - "percent": "9000", - "reputation": 12552713403211, - "rshares": 17409575694, - "voter": "yeswecan" - }, - { - "percent": "10000", - "reputation": 6342420743238, - "rshares": 53156350243, - "voter": "asmr.tist" - }, - { - "percent": "10000", - "reputation": 116210100336, - "rshares": 1897342470, - "voter": "mustakkio" - }, - { - "percent": "4000", - "reputation": 9742808963304, - "rshares": 8767018527, - "voter": "ssiena" - }, - { - "percent": "5000", - "reputation": 7343650800496, - "rshares": 766018298, - "voter": "angelesdesteemit" - }, - { - "percent": "10000", - "reputation": 2891275992098, - "rshares": 63311765126, - "voter": "teo93" - }, - { - "percent": "4250", - "reputation": 14712355832971, - "rshares": 5941433452, - "voter": "reggaesteem" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 3114628597, - "voter": "nichemarket" - }, - { - "percent": "1200", - "reputation": 1681134665977, - "rshares": 3022254685479, - "voter": "leo.voter" - }, - { - "percent": "10000", - "reputation": 612780456165, - "rshares": 8535963401, - "voter": "freedomring" - }, - { - "percent": "10000", - "reputation": 874275545416, - "rshares": 1921017225, - "voter": "tys-project" - }, - { - "percent": "5000", - "reputation": 15808214765405, - "rshares": 409032575835, - "voter": "votebetting" - }, - { - "percent": "3000", - "reputation": 91115882126, - "rshares": 2422184213, - "voter": "hyborian-strain" - }, - { - "percent": "10000", - "reputation": 2391221597007, - "rshares": 2707897821, - "voter": "pablo1601.sports" - }, - { - "percent": "10000", - "reputation": 376803157115, - "rshares": 362492251, - "voter": "tina-tina" - }, - { - "percent": "2000", - "reputation": 144361697175024, - "rshares": 38789273686, - "voter": "taskmaster4450le" - }, - { - "percent": "1500", - "reputation": 91995455090943, - "rshares": 4782205157, - "voter": "thranax" - }, - { - "percent": "4500", - "reputation": 6156906005, - "rshares": 602274168, - "voter": "brainhacks" - }, - { - "percent": "10000", - "reputation": 241740100, - "rshares": 80571346197, - "voter": "beta500" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "happiness19" - }, - { - "percent": "10000", - "reputation": 97507720606, - "rshares": 31819490, - "voter": "gdhaetae" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 5562438919, - "voter": "fucanglong" - }, - { - "percent": "10000", - "reputation": 770042020971, - "rshares": 28629203783, - "voter": "acta" - }, - { - "percent": "10000", - "reputation": 5889854881, - "rshares": 21643837908, - "voter": "the-table" - }, - { - "percent": "10000", - "reputation": 266042222060, - "rshares": 2307260811, - "voter": "cardtrader" - }, - { - "percent": "5000", - "reputation": 1704173681552, - "rshares": 1111168772, - "voter": "bilpcoinbot" - }, - { - "percent": "6500", - "reputation": 0, - "rshares": 941965639, - "voter": "espni" - }, - { - "percent": "10000", - "reputation": 43374515375527, - "rshares": 233247320939, - "voter": "build-it" - }, - { - "percent": "9000", - "reputation": 19199339523, - "rshares": 1878142440, - "voter": "thehouse" - }, - { - "percent": "10000", - "reputation": 384232801439956, - "rshares": 117071207885, - "voter": "mattsanthonyit" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1636686891, - "voter": "dnflsms" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 887737375, - "voter": "jessy22" - }, - { - "percent": "500", - "reputation": 7809695368217, - "rshares": 366622432, - "voter": "spinvest-leo" - }, - { - "percent": "690", - "reputation": 3195367578140, - "rshares": 7340971804, - "voter": "joshmania" - }, - { - "percent": "2500", - "reputation": 3257555962879, - "rshares": 1322389125, - "voter": "bilpcoinrecords" - }, - { - "percent": "1500", - "reputation": 2754871650749, - "rshares": 3627424157, - "voter": "dappstats" - }, - { - "percent": "9000", - "reputation": 967638294423, - "rshares": 142772199533, - "voter": "silverquest" - }, - { - "percent": "8500", - "reputation": 268654389989, - "rshares": 10075609388, - "voter": "honeychip" - }, - { - "percent": "1000", - "reputation": 0, - "rshares": 6433658281, - "voter": "snoochieboochies" - }, - { - "percent": "500", - "reputation": 2957803689, - "rshares": 24212151868, - "voter": "policewala" - }, - { - "percent": "10000", - "reputation": 18319034606, - "rshares": 7993636269, - "voter": "marlians.token" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1199022058, - "voter": "galenkp.aus" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1160258786, - "voter": "ang.pal" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 778895515, - "voter": "ang.spc" - }, - { - "percent": "10000", - "reputation": 51941880676536, - "rshares": 29820650821, - "voter": "sacrosanct" - }, - { - "percent": "100", - "reputation": 0, - "rshares": 0, - "voter": "toni.curation" - }, - { - "percent": "10000", - "reputation": 96421687900488, - "rshares": 46757401744, - "voter": "lachg89" - }, - { - "percent": "10000", - "reputation": 80375925270366, - "rshares": 10146124908, - "voter": "lorentm" - }, - { - "percent": "5000", - "reputation": 1201079161955, - "rshares": 623696886, - "voter": "gmlrecordz" - }, - { - "percent": "6500", - "reputation": 0, - "rshares": 2422724863, - "voter": "dec-market" - }, - { - "percent": "2000", - "reputation": 133245362827, - "rshares": 1436473606, - "voter": "mvanhauten" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1308144705, - "voter": "keepit2" - }, - { - "percent": "10000", - "reputation": 40716090911, - "rshares": 642588051, - "voter": "angel33" - }, - { - "percent": "10000", - "reputation": 48837444735821, - "rshares": 24037358824, - "voter": "build-it.daily" - }, - { - "percent": "10000", - "reputation": 5096756512026, - "rshares": 9793539712, - "voter": "dec.entralized" - }, - { - "percent": "10000", - "reputation": 20853422627715, - "rshares": 1381703536, - "voter": "im-ridd" - }, - { - "percent": "1650", - "reputation": 32542453022, - "rshares": 1553609394, - "voter": "bilpcoinbpc" - }, - { - "percent": "10000", - "reputation": 76222060731, - "rshares": 2507705389, - "voter": "wallytiteuf" - }, - { - "percent": "6500", - "reputation": 0, - "rshares": 938403632, - "voter": "ragequitter" - }, - { - "percent": "10000", - "reputation": 12710819754219, - "rshares": 1330670263, - "voter": "disagio.gang" - }, - { - "percent": "1600", - "reputation": 16354753869293, - "rshares": 4237585335, - "voter": "busybody" - }, - { - "percent": "5000", - "reputation": 14577043701834, - "rshares": 7578267554, - "voter": "julesquirin" - }, - { - "percent": "5000", - "reputation": 164450582774349, - "rshares": 68815282103, - "voter": "alexa.art" - }, - { - "percent": "9000", - "reputation": 30255784377, - "rshares": 1722249670, - "voter": "tommys.shop" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 1917280169, - "voter": "hive-127039" - }, - { - "percent": "300", - "reputation": 0, - "rshares": 15806087005, - "voter": "fengchao" - }, - { - "percent": "5000", - "reputation": 86868289888522, - "rshares": 1013492021046, - "voter": "peakd" - }, - { - "percent": "7500", - "reputation": 355888101482, - "rshares": 574396568, - "voter": "hivewaves" - }, - { - "percent": "200", - "reputation": 35668660233941, - "rshares": 5290079848, - "voter": "hivebuzz" - }, - { - "percent": "1200", - "reputation": 48866708333183, - "rshares": 22775928393, - "voter": "leofinance" - }, - { - "percent": "10000", - "reputation": 3184292050332, - "rshares": 118946362482, - "voter": "st8z" - }, - { - "percent": "1000", - "reputation": 25946748345182, - "rshares": 15193833747, - "voter": "iliyan90" - }, - { - "percent": "4200", - "reputation": 3242356575612, - "rshares": 8197628208, - "voter": "walarhein" - }, - { - "percent": "10000", - "reputation": 3234260836080, - "rshares": 2621947578, - "voter": "solcycler" - }, - { - "percent": "400", - "reputation": 91987050007999, - "rshares": 1328480581, - "voter": "hiveyoda" - }, - { - "percent": "10000", - "reputation": 12467280124701, - "rshares": 9028987366, - "voter": "filoriologo" - }, - { - "percent": "10000", - "reputation": 342960762579, - "rshares": 2463295708, - "voter": "alphahippie" - }, - { - "percent": "5000", - "reputation": 5219413655836, - "rshares": 216772014495, - "voter": "reggaejahm" - }, - { - "percent": "10000", - "reputation": 2236858422320, - "rshares": 17822846707, - "voter": "benthomaswwd" - }, - { - "percent": "10000", - "reputation": 535017327867, - "rshares": 613406139, - "voter": "krypto-hilfe" - }, - { - "percent": "5400", - "reputation": 1209731647511, - "rshares": 925581252451, - "voter": "softworld" - }, - { - "percent": "3000", - "reputation": 441327975768, - "rshares": 605445497518, - "voter": "captainhive" - }, - { - "percent": "10000", - "reputation": 3065772781664, - "rshares": 3040790353, - "voter": "smp.cardio" - }, - { - "percent": "10000", - "reputation": 3063240678224, - "rshares": 3194147386, - "voter": "netdent" - }, - { - "percent": "5000", - "reputation": 17500919200979, - "rshares": 28739305574, - "voter": "arlettemsalase" - }, - { - "percent": "10000", - "reputation": 19849622918233, - "rshares": 112182370081, - "voter": "behiver" - }, - { - "percent": "10000", - "reputation": 6105958100194, - "rshares": 1686554105, - "voter": "dagamers" - }, - { - "percent": "10000", - "reputation": 679455507132, - "rshares": 439104127, - "voter": "hjchilb" - }, - { - "percent": "750", - "reputation": 22060257076152, - "rshares": 3014310920, - "voter": "quello" - }, - { - "percent": "1000", - "reputation": 56831893473913, - "rshares": 8029993994, - "voter": "globalcurrencies" - }, - { - "percent": "10000", - "reputation": 90463833228384, - "rshares": 45145196496, - "voter": "freakeao" - }, - { - "percent": "1000", - "reputation": 661148949025, - "rshares": 590611800, - "voter": "gradeon" - }, - { - "percent": "10000", - "reputation": 5540338918826, - "rshares": 5602010618, - "voter": "ppinillos" - }, - { - "percent": "6600", - "reputation": 1292923913128, - "rshares": 11650674920, - "voter": "hextech" - }, - { - "percent": "7500", - "reputation": 5014132859, - "rshares": 1505350725, - "voter": "paulman" - }, - { - "percent": "187", - "reputation": 47896334821902, - "rshares": 2588544048, - "voter": "hiveonboard" - }, - { - "percent": "10000", - "reputation": 10637170241006, - "rshares": 12197894953, - "voter": "miriannalis" - }, - { - "percent": "1000", - "reputation": 3177222978373, - "rshares": 9123483012, - "voter": "hivelist" - }, - { - "percent": "10000", - "reputation": 5226428326302, - "rshares": 4652481591, - "voter": "argenvista" - }, - { - "percent": "2500", - "reputation": 604442758770, - "rshares": 112394395606, - "voter": "ninnu" - }, - { - "percent": "3125", - "reputation": 0, - "rshares": 564330226, - "voter": "tattytoque" - }, - { - "percent": "10000", - "reputation": 7072458061355, - "rshares": 6649143762, - "voter": "threespeak-es" - }, - { - "percent": "10000", - "reputation": 2612900116, - "rshares": 534969099, - "voter": "mynewlifeai" - }, - { - "percent": "500", - "reputation": 9650263289467, - "rshares": 2703794436, - "voter": "localgrower" - }, - { - "percent": "2000", - "reputation": 19058471657, - "rshares": 1259196367, - "voter": "vibrasphere" - }, - { - "percent": "10000", - "reputation": 62691685333073, - "rshares": 108743985411, - "voter": "xxxthorxxx" - }, - { - "percent": "10000", - "reputation": 21651678370275, - "rshares": 22219266320, - "voter": "pcojines" - }, - { - "percent": "10000", - "reputation": 7436529679810, - "rshares": 7240252026, - "voter": "belen0949" - }, - { - "percent": "10000", - "reputation": 429763208250, - "rshares": 4368091122, - "voter": "ghaazi" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 7674822776, - "voter": "hive-101493" - }, - { - "percent": "600", - "reputation": 8412246560343, - "rshares": 571336994, - "voter": "beehivetrader" - }, - { - "percent": "10000", - "reputation": 849928020180, - "rshares": 728758415, - "voter": "jaguarosky" - }, - { - "percent": "1500", - "reputation": 74732394114947, - "rshares": 268756758469, - "voter": "ronavel" - }, - { - "percent": "7000", - "reputation": 29579801503382, - "rshares": 13295744382, - "voter": "maleklopez" - }, - { - "percent": "10000", - "reputation": 2100762851576, - "rshares": 66528981278, - "voter": "daniky" - }, - { - "percent": "2500", - "reputation": 5470715229938, - "rshares": 1068161972, - "voter": "jsalvage" - }, - { - "percent": "10000", - "reputation": 69575389840495, - "rshares": 79629572063, - "voter": "lucianav" - }, - { - "percent": "10000", - "reputation": 17583644867, - "rshares": 340483366, - "voter": "heinkhantmaung" - }, - { - "percent": "5000", - "reputation": 8994726862497, - "rshares": 3402653786, - "voter": "filosbonus1" - }, - { - "percent": "10000", - "reputation": 16545252407954, - "rshares": 17395941724, - "voter": "zullyarte" - }, - { - "percent": "10000", - "reputation": 43332467245, - "rshares": 44010456809, - "voter": "beezdak" - }, - { - "percent": "10000", - "reputation": 4503226485042, - "rshares": 8581964678, - "voter": "roberto58" - }, - { - "percent": "10000", - "reputation": 11320226922294, - "rshares": 3784286154948, - "voter": "hiro-hive" - }, - { - "percent": "5000", - "reputation": 833805368390, - "rshares": 217507180414, - "voter": "hivebuilder" - }, - { - "percent": "10000", - "reputation": 65519590598, - "rshares": 2002149901, - "voter": "plusvault" - }, - { - "percent": "6500", - "reputation": 2957353135624, - "rshares": 1854561277, - "voter": "splinterstats" - }, - { - "percent": "2500", - "reputation": 23814154211011, - "rshares": 1009240269, - "voter": "syberia" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "cocos168" - }, - { - "percent": "10000", - "reputation": 792202344312, - "rshares": 757520888, - "voter": "ollasysartenes" - }, - { - "percent": "500", - "reputation": 25284728169845, - "rshares": 137988541198, - "voter": "recoveryinc" - }, - { - "percent": "1250", - "reputation": 76284107957, - "rshares": 3321021123, - "voter": "hive-108278" - }, - { - "percent": "10000", - "reputation": 9517022344384, - "rshares": 38743135987, - "voter": "rafaelgreen" - }, - { - "percent": "500", - "reputation": 906267166974, - "rshares": 528446260, - "voter": "sevenoh-fiveoh" - }, - { - "percent": "10000", - "reputation": 57171664726, - "rshares": 3849646469, - "voter": "btsarps" - }, - { - "percent": "10000", - "reputation": 1329302309, - "rshares": 24061686818, - "voter": "starlord2826" - }, - { - "percent": "10000", - "reputation": 33401976736, - "rshares": 50957141047, - "voter": "aceh-media" - }, - { - "percent": "10000", - "reputation": 4399967431494, - "rshares": 3577219004, - "voter": "epilatero" - }, - { - "percent": "10000", - "reputation": 3005998414144, - "rshares": 4112309727, - "voter": "kraken99" - }, - { - "percent": "10000", - "reputation": 9058618771553, - "rshares": 8916768661, - "voter": "aurauramagic" - }, - { - "percent": "500", - "reputation": 0, - "rshares": 4936419863, - "voter": "dying" - }, - { - "percent": "10000", - "reputation": 6748176467764, - "rshares": 7665065698, - "voter": "rafabvr" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 19503505850, - "voter": "vtol79" - }, - { - "percent": "10000", - "reputation": 7254965979645, - "rshares": 6796896180, - "voter": "sshila" - }, - { - "percent": "6400", - "reputation": 6260561866202, - "rshares": 123993586011, - "voter": "idea-make-rich" - }, - { - "percent": "10000", - "reputation": 3452328685273, - "rshares": 5217678720, - "voter": "aaalviarez" - }, - { - "percent": "2500", - "reputation": 1447273576100, - "rshares": 1312262214, - "voter": "bitcome" - }, - { - "percent": "5100", - "reputation": 16307549034965, - "rshares": 18642849141, - "voter": "trangbaby" - }, - { - "percent": "1000", - "reputation": 9727854376347, - "rshares": 1507573780, - "voter": "borbolet" - }, - { - "percent": "5000", - "reputation": 1668767539307, - "rshares": 684621443, - "voter": "gonklavez9" - }, - { - "percent": "10000", - "reputation": 102092235719, - "rshares": 1865782497, - "voter": "clifth" - }, - { - "percent": "10000", - "reputation": 907489862666, - "rshares": 1228257355, - "voter": "danielhive2021" - }, - { - "percent": "10000", - "reputation": 92721511576, - "rshares": 517021045, - "voter": "rhinoceros" - }, - { - "percent": "10000", - "reputation": 86405531104, - "rshares": 1929431210, - "voter": "text2speech" - }, - { - "percent": "1350", - "reputation": 2004903, - "rshares": 0, - "voter": "panprezes" - }, - { - "percent": "10000", - "reputation": 49318924596, - "rshares": 749973155, - "voter": "pelulacro" - }, - { - "percent": "200", - "reputation": 0, - "rshares": 0, - "voter": "jac-inthebox" - }, - { - "percent": "10000", - "reputation": 654061210157, - "rshares": 696709568, - "voter": "vaishnavi24" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "josepa10" - } - ], - "author": "theycallmedan", - "author_reputation": 539147053023232, - "beneficiaries": [ - { - "account": "threespeakwallet", - "weight": 1100 - } - ], - "body": "<center>\r\n\r\n[](https://3speak.co/watch?v=theycallmedan/raaxtlrg)\r\n\r\n\u25b6\ufe0f [Watch on 3Speak](https://3speak.co/watch?v=theycallmedan/raaxtlrg)\r\n\r\n</center>\r\n\r\n---\r\n\r\nThis Is My Journey - My Content Is Not Financial Advice.\n\nWhy do you invest in ethereum? Ethereum the token, it's actual use is for gas on the platform. It can also be used as collateral for defi but that is something brand new and wasn't the reason it shot to 1400 the first time. \n\nDefi craze what was the use case of most of the tokens? Governance.\nHive has that too.\n\nIf you're trying to compare why coins pump with their use case, you'll find that \"gas\" and governance is literally the only use cases of these tokens. Do people want to be on your blockchain bad enough to pay a small fee or hoard tokens? Bitcoin is the same, you're buying space on the chain.\n\nWhat is Ethereums roadmap?\nWell, they don't have one. Well, not in the \"vision\" department at least. Their roadmaps are purely technical. They don't care what you do with the technology they just tell you what tech they built.\n\nHaving a vision roadmap only happens in centralized chains. If I recall, Bitcoin had the vision to be peer 2 peer cash, but now its digital gold. You can't force a vision onto anyone, not even Satoshi. \n\nSo I believe we should focus on promoting what we are building and let the market decide what they want to use it for. Have a Hive technical roadmap if anything.\r\n\r\n---\r\n\r\n\u25b6\ufe0f [3Speak](https://3speak.co/watch?v=theycallmedan/raaxtlrg)\r\n", - "body_length": 1546, - "cashout_time": "2020-11-08T19:20:03", - "category": "hive-167922", - "children": 51, - "created": "2020-11-01T19:20:03", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"tags\":[\"hive\",\"ethereum\",\"crypto\",\"bitcoin\"],\"app\":\"3speak/0.3.0\",\"type\":\"3speak/video\",\"image\":[\"https://img.3speakcontent.co/raaxtlrg/post.png\"],\"video\":{\"info\":{\"platform\":\"3speak\",\"title\":\"Hive's \\\"Vision\\\" & Usecase - Let's Talk About It\",\"author\":\"theycallmedan\",\"permlink\":\"raaxtlrg\",\"duration\":1282.8,\"filesize\":336259400,\"file\":\"bvOphAFgTFyJeeOPcuGYUcueMcNUmewxZSTXSJYwrimhdwcIYIVFbwbnbmqnqlNf.mp4\",\"ipfs\":null},\"content\":{\"description\":\"This Is My Journey - My Content Is Not Financial Advice.\\n\\nWhy do you invest in ethereum? Ethereum the token, it's actual use is for gas on the platform. It can also be used as collateral for defi but that is something brand new and wasn't the reason it shot to 1400 the first time. \\n\\nDefi craze what was the use case of most of the tokens? Governance.\\nHive has that too.\\n\\nIf you're trying to compare why coins pump with their use case, you'll find that \\\"gas\\\" and governance is literally the only use cases of these tokens. Do people want to be on your blockchain bad enough to pay a small fee or hoard tokens? Bitcoin is the same, you're buying space on the chain.\\n\\nWhat is Ethereums roadmap?\\nWell, they don't have one. Well, not in the \\\"vision\\\" department at least. Their roadmaps are purely technical. They don't care what you do with the technology they just tell you what tech they built.\\n\\nHaving a vision roadmap only happens in centralized chains. If I recall, Bitcoin had the vision to be peer 2 peer cash, but now its digital gold. You can't force a vision onto anyone, not even Satoshi. \\n\\nSo I believe we should focus on promoting what we are building and let the market decide what they want to use it for. Have a Hive technical roadmap if anything.\",\"tags\":[\"hive\",\"ethereum\",\"crypto\",\"bitcoin\"]}}}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-11-01T19:20:03", - "max_accepted_payout": "100000.000 HBD", - "net_rshares": 284197560973379, - "parent_author": "", - "parent_permlink": "hive-167922", - "pending_payout_value": "67.166 HBD", - "percent_hbd": 0, - "permlink": "raaxtlrg", - "post_id": 100348035, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hive's \"Vision\" & Usecase - Let's Talk About It", - "title": "Hive's \"Vision\" & Usecase - Let's Talk About It", - "total_payout_value": "0.000 HBD", - "url": "/hive-167922/@theycallmedan/raaxtlrg" - }, - { - "active_votes": [ - { - "percent": "1800", - "reputation": 19245698180508, - "rshares": 386472263540, - "voter": "tombstone" - }, - { - "percent": "3000", - "reputation": 341233778618, - "rshares": 470579344521, - "voter": "boatymcboatface" - }, - { - "percent": "10000", - "reputation": 30111821406182, - "rshares": 633537353809, - "voter": "bleepcoin" - }, - { - "percent": "3000", - "reputation": 1957220358850383, - "rshares": 1170474032507, - "voter": "kingscrown" - }, - { - "percent": "8000", - "reputation": 137382059999971, - "rshares": 187021236364, - "voter": "flemingfarm" - }, - { - "percent": "10000", - "reputation": 1594304819447449, - "rshares": 9575696252465, - "voter": "acidyo" - }, - { - "percent": "3000", - "reputation": 4509451541223, - "rshares": 126334032670, - "voter": "theshell" - }, - { - "percent": "10000", - "reputation": 1574265047259, - "rshares": 102759824733, - "voter": "judyhopps" - }, - { - "percent": "10000", - "reputation": 159372103844946, - "rshares": 21856583998394, - "voter": "gtg" - }, - { - "percent": "10000", - "reputation": 618931574338675, - "rshares": 1063203234633, - "voter": "ericvancewalton" - }, - { - "percent": "5000", - "reputation": 352970428645376, - "rshares": 3421853089179, - "voter": "roelandp" - }, - { - "percent": "10000", - "reputation": 229982756480919, - "rshares": 2228634939169, - "voter": "ausbitbank" - }, - { - "percent": "10000", - "reputation": 67858732461644, - "rshares": 1051275384384, - "voter": "fulltimegeek" - }, - { - "percent": "10000", - "reputation": 372226967801214, - "rshares": 54267406805, - "voter": "allmonitors" - }, - { - "percent": "10000", - "reputation": 315496990256842, - "rshares": 5350505430219, - "voter": "arcange" - }, - { - "percent": "10000", - "reputation": 4957775761011, - "rshares": 6293492927122, - "voter": "ubg" - }, - { - "percent": "10000", - "reputation": 978188457801574, - "rshares": 3275303134796, - "voter": "deanliu" - }, - { - "percent": "300", - "reputation": 501315151365, - "rshares": 3039991307, - "voter": "raphaelle" - }, - { - "percent": "1700", - "reputation": 560943007638090, - "rshares": 638232085045, - "voter": "ace108" - }, - { - "percent": "10000", - "reputation": 72178763490828, - "rshares": 299376776497, - "voter": "felixxx" - }, - { - "percent": "10000", - "reputation": 266498838207861, - "rshares": 1506012064423, - "voter": "timcliff" - }, - { - "percent": "10000", - "reputation": 3774393595703, - "rshares": 45873688848, - "voter": "laoyao" - }, - { - "percent": "10000", - "reputation": 6042026377398, - "rshares": 80458859, - "voter": "somebody" - }, - { - "percent": "10000", - "reputation": 97845484474, - "rshares": 161030697911, - "voter": "midnightoil" - }, - { - "percent": "10000", - "reputation": 47820660266221, - "rshares": 911234588506, - "voter": "xiaohui" - }, - { - "percent": "10000", - "reputation": 8868395123281, - "rshares": 5037831179418, - "voter": "jphamer1" - }, - { - "percent": "10000", - "reputation": 2705431887559, - "rshares": 3463953088758, - "voter": "joele" - }, - { - "percent": "10000", - "reputation": 1493318183193201, - "rshares": 5387217751870, - "voter": "oflyhigh" - }, - { - "percent": "10000", - "reputation": 143768203235023, - "rshares": 16936975734, - "voter": "shanghaipreneur" - }, - { - "percent": "9900", - "reputation": 3434871602070, - "rshares": 39882254722, - "voter": "fooblic" - }, - { - "percent": "10000", - "reputation": 14314005204488, - "rshares": 153783362844, - "voter": "fingolfin" - }, - { - "percent": "5000", - "reputation": 74151779329146, - "rshares": 10545410315, - "voter": "rmach" - }, - { - "percent": "10000", - "reputation": 97229489927307, - "rshares": 425849430405, - "voter": "rubenalexander" - }, - { - "percent": "10000", - "reputation": 181963199440755, - "rshares": 1198700600913, - "voter": "helene" - }, - { - "percent": "1900", - "reputation": 363924035887507, - "rshares": 281800072693, - "voter": "steevc" - }, - { - "percent": "10000", - "reputation": 74863127712333, - "rshares": 1580774827658, - "voter": "mattclarke" - }, - { - "percent": "10000", - "reputation": 3619593982366, - "rshares": 3839927844, - "voter": "esecholo" - }, - { - "percent": "800", - "reputation": 505596674036569, - "rshares": 519638351868, - "voter": "penguinpablo" - }, - { - "percent": "2000", - "reputation": 390433925983792, - "rshares": 215451816414, - "voter": "uwelang" - }, - { - "percent": "5000", - "reputation": 662250918016, - "rshares": 3190024131042, - "voter": "cornerstone" - }, - { - "percent": "10000", - "reputation": 262691650197556, - "rshares": 82696616415, - "voter": "titusfrost" - }, - { - "percent": "1900", - "reputation": 49426223217225, - "rshares": 17986427100, - "voter": "phusionphil" - }, - { - "percent": "2300", - "reputation": 258744306894076, - "rshares": 37313469504, - "voter": "edgarsart" - }, - { - "percent": "10000", - "reputation": 4667597876284, - "rshares": 358151251883, - "voter": "mjhomb" - }, - { - "percent": "5000", - "reputation": 120512824315021, - "rshares": 1940559311903, - "voter": "funnyman" - }, - { - "percent": "1500", - "reputation": 280674585514224, - "rshares": 144453953989, - "voter": "justyy" - }, - { - "percent": "10000", - "reputation": 361633122480444, - "rshares": 107405537930, - "voter": "michelle.gent" - }, - { - "percent": "10000", - "reputation": 183465603606151, - "rshares": 247362196706, - "voter": "jang" - }, - { - "percent": "10000", - "reputation": 1973032531145, - "rshares": 9931355795, - "voter": "whoib" - }, - { - "percent": "10000", - "reputation": 169658123107479, - "rshares": 113607271281, - "voter": "lloyddavis" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 45373560518, - "voter": "ssekulji" - }, - { - "percent": "10000", - "reputation": 137790425095985, - "rshares": 203423566985, - "voter": "lighteye" - }, - { - "percent": "10000", - "reputation": 559165550719, - "rshares": 2531825516, - "voter": "syahhiran" - }, - { - "percent": "5000", - "reputation": 111778832002739, - "rshares": 283118506033, - "voter": "techslut" - }, - { - "percent": "10000", - "reputation": 221491576015, - "rshares": 1059068215, - "voter": "consciousness" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 553845981, - "voter": "triviummethod" - }, - { - "percent": "10000", - "reputation": 234795708570321, - "rshares": 240822242191, - "voter": "jaybird" - }, - { - "percent": "10000", - "reputation": 330858064593420, - "rshares": 7442568911540, - "voter": "jaki01" - }, - { - "percent": "4500", - "reputation": 0, - "rshares": 2753474126796, - "voter": "redes" - }, - { - "percent": "10000", - "reputation": 19868139032, - "rshares": 722731504, - "voter": "whistleblower" - }, - { - "percent": "5000", - "reputation": 5043649436983, - "rshares": 5440374908, - "voter": "amadeus" - }, - { - "percent": "10000", - "reputation": 4110132379317, - "rshares": 14691383603, - "voter": "sabsel" - }, - { - "percent": "950", - "reputation": 7547083616142, - "rshares": 12382381954, - "voter": "edb" - }, - { - "percent": "10000", - "reputation": 430990815671, - "rshares": 3219734322, - "voter": "veganism" - }, - { - "percent": "5000", - "reputation": 37769232724831, - "rshares": 99987992384, - "voter": "ixindamix" - }, - { - "percent": "1500", - "reputation": 5554224447155, - "rshares": 15928288003, - "voter": "lovemetouchme2" - }, - { - "percent": "10000", - "reputation": 14875692021756, - "rshares": 4396098826, - "voter": "chiren" - }, - { - "percent": "10000", - "reputation": 283274585990, - "rshares": 1354326995, - "voter": "steemitcommunity" - }, - { - "percent": "2000", - "reputation": 101539530127063, - "rshares": 66605402111, - "voter": "edje" - }, - { - "percent": "10000", - "reputation": 19610705040132, - "rshares": 48895637732, - "voter": "askari" - }, - { - "percent": "4000", - "reputation": 7177514553823, - "rshares": 85518190814, - "voter": "gabriele-gio" - }, - { - "percent": "10000", - "reputation": 221144436321945, - "rshares": 72001035159, - "voter": "blackbunny" - }, - { - "percent": "10000", - "reputation": 138663179735601, - "rshares": 319380835394, - "voter": "kobold-djawa" - }, - { - "percent": "5000", - "reputation": 27214240242904, - "rshares": 43153109167, - "voter": "rahul.stan" - }, - { - "percent": "10000", - "reputation": 30904254166753, - "rshares": 752993466135, - "voter": "distantsignal" - }, - { - "percent": "10000", - "reputation": 32556441828843, - "rshares": 10862556870, - "voter": "ades" - }, - { - "percent": "10000", - "reputation": 36248450113797, - "rshares": 77041848751, - "voter": "lingfei" - }, - { - "percent": "5000", - "reputation": 1696316811168628, - "rshares": 2726207170468, - "voter": "tarazkp" - }, - { - "percent": "3300", - "reputation": 12325904700970, - "rshares": 7783486908, - "voter": "justinashby" - }, - { - "percent": "10000", - "reputation": 169327551453023, - "rshares": 11416559248, - "voter": "evildeathcore" - }, - { - "percent": "10000", - "reputation": 19406409261381, - "rshares": 4219698921056, - "voter": "diggndeeper.com" - }, - { - "percent": "10000", - "reputation": 450338781685383, - "rshares": 128616062182, - "voter": "louisthomas" - }, - { - "percent": "10000", - "reputation": 286164345508, - "rshares": 808597671, - "voter": "dapu" - }, - { - "percent": "10000", - "reputation": 22376987942580, - "rshares": 1596762984, - "voter": "freedomexists" - }, - { - "percent": "10000", - "reputation": 12241947357981, - "rshares": 43423758650, - "voter": "dickturpin" - }, - { - "percent": "10000", - "reputation": 4061300561388, - "rshares": 10225271626, - "voter": "ocisly" - }, - { - "percent": "10000", - "reputation": 96744768495, - "rshares": 5123239448699, - "voter": "davidorcamuriel" - }, - { - "percent": "10000", - "reputation": 14794792589844, - "rshares": 2473545933, - "voter": "imperfect-one" - }, - { - "percent": "10000", - "reputation": 15465640814476, - "rshares": 3826527866708, - "voter": "sepracore" - }, - { - "percent": "8000", - "reputation": 62578995648516, - "rshares": 124964030259, - "voter": "zaragast" - }, - { - "percent": "3000", - "reputation": 42214550905806, - "rshares": 1206121856764, - "voter": "smasssh" - }, - { - "percent": "10000", - "reputation": 74329552872641, - "rshares": 279611097583, - "voter": "mes" - }, - { - "percent": "8000", - "reputation": 79234586811944, - "rshares": 236954934949, - "voter": "thenightflier" - }, - { - "percent": "10000", - "reputation": 20837541242644, - "rshares": 2293741092, - "voter": "grider123" - }, - { - "percent": "10000", - "reputation": 39969242565947, - "rshares": 174180838253, - "voter": "iansart" - }, - { - "percent": "2000", - "reputation": 23391602452678, - "rshares": 75521233877, - "voter": "schlees" - }, - { - "percent": "10000", - "reputation": 13370190184046, - "rshares": 2059842026, - "voter": "martie7" - }, - { - "percent": "2000", - "reputation": 5899232344203, - "rshares": 575555602, - "voter": "yeaho" - }, - { - "percent": "10000", - "reputation": 19666794654684, - "rshares": 20968789773, - "voter": "aaliyahholt" - }, - { - "percent": "4500", - "reputation": 11448902903972, - "rshares": 2228460527049, - "voter": "roomservice" - }, - { - "percent": "10000", - "reputation": 294281694879864, - "rshares": 1439821930314, - "voter": "bigram13" - }, - { - "percent": "10000", - "reputation": 48378473521256, - "rshares": 4498690646, - "voter": "drag33" - }, - { - "percent": "10000", - "reputation": 413408024106, - "rshares": 1686961916643, - "voter": "nrg" - }, - { - "percent": "10000", - "reputation": 19675504562112, - "rshares": 281763511777, - "voter": "amirl" - }, - { - "percent": "10000", - "reputation": 112994806497327, - "rshares": 3261853978, - "voter": "varunpinto" - }, - { - "percent": "10000", - "reputation": 9701652715721, - "rshares": 115353304916, - "voter": "masterthematrix" - }, - { - "percent": "10000", - "reputation": 3381778816658, - "rshares": 5136930380, - "voter": "aditor" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 244008203468, - "voter": "exec" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 825050232, - "voter": "eval" - }, - { - "percent": "2500", - "reputation": 7901101123497, - "rshares": 279580420942, - "voter": "giuatt07" - }, - { - "percent": "10000", - "reputation": 329877254452254, - "rshares": 8556817259, - "voter": "steeminator3000" - }, - { - "percent": "10000", - "reputation": 655474560066583, - "rshares": 2172818105567, - "voter": "galenkp" - }, - { - "percent": "10000", - "reputation": 175710482549410, - "rshares": 726825177173, - "voter": "enjar" - }, - { - "percent": "10000", - "reputation": 46150080976545, - "rshares": 3512595359791, - "voter": "cryptographic" - }, - { - "percent": "10000", - "reputation": 62238574494569, - "rshares": 688459029756, - "voter": "mahdiyari" - }, - { - "percent": "10000", - "reputation": 15824477347297, - "rshares": 14170957835, - "voter": "tracer-paulo" - }, - { - "percent": "10000", - "reputation": 507780353842783, - "rshares": 1642183000222, - "voter": "brian.rrr" - }, - { - "percent": "10000", - "reputation": 110211133478274, - "rshares": 1717651689282, - "voter": "drakos" - }, - { - "percent": "900", - "reputation": 122017350265257, - "rshares": 188531348121, - "voter": "ew-and-patterns" - }, - { - "percent": "5000", - "reputation": 698336179865, - "rshares": 548629044, - "voter": "sirjaxxy" - }, - { - "percent": "10000", - "reputation": 1734633072808, - "rshares": 9446389400, - "voter": "bruzzy" - }, - { - "percent": "10000", - "reputation": 219366237221002, - "rshares": 595566399605, - "voter": "offgridlife" - }, - { - "percent": "10000", - "reputation": 582437706834948, - "rshares": 508323146963, - "voter": "theouterlight" - }, - { - "percent": "5000", - "reputation": 110067930671619, - "rshares": 109345113517, - "voter": "benedict08" - }, - { - "percent": "10000", - "reputation": 29540199236425, - "rshares": 3331691852926, - "voter": "eturnerx" - }, - { - "percent": "10000", - "reputation": 29666985623497, - "rshares": 88146966735, - "voter": "vallesleoruther" - }, - { - "percent": "4040", - "reputation": 77457609763631, - "rshares": 763979575386, - "voter": "face2face" - }, - { - "percent": "10000", - "reputation": 4511212385057, - "rshares": 3870143224, - "voter": "nicniezgrublem" - }, - { - "percent": "10000", - "reputation": 23747652895956, - "rshares": 1058441024, - "voter": "richi0927" - }, - { - "percent": "3000", - "reputation": 135808252885028, - "rshares": 1093451424619, - "voter": "stayoutoftherz" - }, - { - "percent": "2800", - "reputation": 190691885658151, - "rshares": 1971244027266, - "voter": "nathanmars" - }, - { - "percent": "2500", - "reputation": 31463721820884, - "rshares": 257587813339, - "voter": "sanjeevm" - }, - { - "percent": "10000", - "reputation": 21870820797138, - "rshares": 40526156026, - "voter": "marian0" - }, - { - "percent": "8000", - "reputation": 64603876629814, - "rshares": 345824501013, - "voter": "steempostitalia" - }, - { - "percent": "3000", - "reputation": 115269542036380, - "rshares": 389274633353, - "voter": "vikisecrets" - }, - { - "percent": "10000", - "reputation": 58065010354603, - "rshares": 118624007745, - "voter": "shasta" - }, - { - "percent": "10000", - "reputation": 26703458140145, - "rshares": 14315534906, - "voter": "kharrazi" - }, - { - "percent": "5000", - "reputation": 1844447662772, - "rshares": 4408299427, - "voter": "khalil319" - }, - { - "percent": "10000", - "reputation": 1465267842688, - "rshares": 1558049304, - "voter": "jorge248" - }, - { - "percent": "5000", - "reputation": 10111973688443, - "rshares": 14182067634, - "voter": "gamesjoyce" - }, - { - "percent": "7000", - "reputation": 616021827459, - "rshares": 9369075312, - "voter": "yacobh" - }, - { - "percent": "6000", - "reputation": 3550952434511, - "rshares": 62047301282, - "voter": "oldman28" - }, - { - "percent": "10000", - "reputation": 225885956343558, - "rshares": 79268694155, - "voter": "horpey" - }, - { - "percent": "10000", - "reputation": 14702291738356, - "rshares": 4851252546, - "voter": "moromaro" - }, - { - "percent": "700", - "reputation": 73283380450035, - "rshares": 78404913328, - "voter": "shanibeer" - }, - { - "percent": "10000", - "reputation": 72586430101690, - "rshares": 84610840464, - "voter": "aurodivys" - }, - { - "percent": "10000", - "reputation": 63776063283749, - "rshares": 256207330358, - "voter": "shogo" - }, - { - "percent": "2500", - "reputation": 52406600539994, - "rshares": 30510144655, - "voter": "fionasfavourites" - }, - { - "percent": "5000", - "reputation": 26317620061322, - "rshares": 2127064444, - "voter": "foreveraverage" - }, - { - "percent": "10000", - "reputation": 72946432090481, - "rshares": 135114573932, - "voter": "my451r" - }, - { - "percent": "10000", - "reputation": 328923251098727, - "rshares": 37239830740, - "voter": "elenasteem" - }, - { - "percent": "6400", - "reputation": 24639928209538, - "rshares": 38277801491, - "voter": "santigs" - }, - { - "percent": "10000", - "reputation": 196571377648, - "rshares": 15403917487, - "voter": "nadhora" - }, - { - "percent": "2500", - "reputation": 54379045488206, - "rshares": 64707710414, - "voter": "bashadow" - }, - { - "percent": "10000", - "reputation": 477437764741, - "rshares": 877658368, - "voter": "ekkah" - }, - { - "percent": "400", - "reputation": 101280142849344, - "rshares": 11165947310, - "voter": "kimzwarch" - }, - { - "percent": "10000", - "reputation": 31474391219, - "rshares": 21323378181, - "voter": "onartbali" - }, - { - "percent": "500", - "reputation": 47942503950741, - "rshares": 47107795691, - "voter": "accelerator" - }, - { - "percent": "8000", - "reputation": 35075558795655, - "rshares": 116121718949, - "voter": "alinakot" - }, - { - "percent": "10000", - "reputation": 669346799793, - "rshares": 19474163828, - "voter": "voxmonkey" - }, - { - "percent": "10000", - "reputation": 51585017229874, - "rshares": 92081984402, - "voter": "eastmael" - }, - { - "percent": "10000", - "reputation": 537290602112, - "rshares": 235080432704, - "voter": "chinchilla" - }, - { - "percent": "10000", - "reputation": 11593916038941, - "rshares": 114242092, - "voter": "itikna09" - }, - { - "percent": "1000", - "reputation": 139904553806579, - "rshares": 555901482187, - "voter": "joshman" - }, - { - "percent": "10000", - "reputation": 110750156547770, - "rshares": 406677216593, - "voter": "drax" - }, - { - "percent": "10000", - "reputation": 14688526974259, - "rshares": 3370332177, - "voter": "helix" - }, - { - "percent": "6000", - "reputation": 445234100950820, - "rshares": 19040202765766, - "voter": "therealwolf" - }, - { - "percent": "10000", - "reputation": 1386913003857667, - "rshares": 3030092743552, - "voter": "taskmaster4450" - }, - { - "percent": "8200", - "reputation": 16864868829890, - "rshares": 40707050167, - "voter": "karlin" - }, - { - "percent": "10000", - "reputation": 72384838675160, - "rshares": 598458680498, - "voter": "roleerob" - }, - { - "percent": "10000", - "reputation": 73578445665754, - "rshares": 242634240061, - "voter": "deathwing" - }, - { - "percent": "10000", - "reputation": 5079875936675, - "rshares": 5345719185, - "voter": "scorer" - }, - { - "percent": "6000", - "reputation": 336063014350538, - "rshares": 817363995914, - "voter": "revisesociology" - }, - { - "percent": "10000", - "reputation": 206055018792025, - "rshares": 49114780796, - "voter": "silversaver888" - }, - { - "percent": "9000", - "reputation": 28057351253216, - "rshares": 579612902, - "voter": "superbing" - }, - { - "percent": "10000", - "reputation": 3054701407913, - "rshares": 2146851130, - "voter": "thatterrioguy" - }, - { - "percent": "10000", - "reputation": 58282771573923, - "rshares": 35660092435, - "voter": "dilimunanzar" - }, - { - "percent": "10000", - "reputation": 822218064662, - "rshares": 1779748815, - "voter": "acehnature" - }, - { - "percent": "1500", - "reputation": 64078918102750, - "rshares": 9953320654, - "voter": "dailystats" - }, - { - "percent": "3500", - "reputation": 57287800105812, - "rshares": 1207619736, - "voter": "calimeatwagon" - }, - { - "percent": "5000", - "reputation": 6727973666699, - "rshares": 83108136805, - "voter": "omra-sky" - }, - { - "percent": "10000", - "reputation": 4237603797309, - "rshares": 472455549, - "voter": "imamalkimas" - }, - { - "percent": "10000", - "reputation": 2799624882441, - "rshares": 10670094710, - "voter": "borgheseglass" - }, - { - "percent": "10000", - "reputation": 1876754372524, - "rshares": 9379397246, - "voter": "shadflyfilms" - }, - { - "percent": "4300", - "reputation": 78606817928738, - "rshares": 63243558868, - "voter": "happydolphin" - }, - { - "percent": "8000", - "reputation": 12066290877974, - "rshares": 61787127708, - "voter": "heidi71" - }, - { - "percent": "10000", - "reputation": 19533997928933, - "rshares": 38490686391, - "voter": "daisyphotography" - }, - { - "percent": "157", - "reputation": 0, - "rshares": 67355382372, - "voter": "investegg" - }, - { - "percent": "10000", - "reputation": 14538002619952, - "rshares": 458717589638, - "voter": "gank" - }, - { - "percent": "2500", - "reputation": 141970828773777, - "rshares": 413864431629, - "voter": "tobetada" - }, - { - "percent": "10000", - "reputation": 3291168947960, - "rshares": 2944390552, - "voter": "enolife" - }, - { - "percent": "10000", - "reputation": 400141436611, - "rshares": 4230316121, - "voter": "spacesheep" - }, - { - "percent": "2000", - "reputation": 10939046475227, - "rshares": 753600782, - "voter": "liverpool-fan" - }, - { - "percent": "5000", - "reputation": 12918217107297, - "rshares": 4855034583, - "voter": "afterglow" - }, - { - "percent": "5000", - "reputation": 1920136042828, - "rshares": 5904178405, - "voter": "petrolinivideo" - }, - { - "percent": "1000", - "reputation": 2106804845688, - "rshares": 5121165333, - "voter": "cryptotradingfr" - }, - { - "percent": "10000", - "reputation": 1737660226555, - "rshares": 1537489685, - "voter": "meincluyo" - }, - { - "percent": "10000", - "reputation": 473928985768900, - "rshares": 3045995506, - "voter": "tradingideas" - }, - { - "percent": "10000", - "reputation": 2302152857587, - "rshares": 1476309580, - "voter": "jexus77" - }, - { - "percent": "10000", - "reputation": 616201530, - "rshares": 108658389215, - "voter": "new-world-steem" - }, - { - "percent": "10000", - "reputation": 735157464719, - "rshares": 5003897486, - "voter": "mtl1979" - }, - { - "percent": "5000", - "reputation": 31360323509866, - "rshares": 64621608710, - "voter": "steveconnor" - }, - { - "percent": "6000", - "reputation": 4848090755452, - "rshares": 34813016494, - "voter": "gazbaz4000" - }, - { - "percent": "6000", - "reputation": 157805541487641, - "rshares": 3763899414195, - "voter": "smartsteem" - }, - { - "percent": "10000", - "reputation": 691048366382, - "rshares": 2425924664, - "voter": "jes2850" - }, - { - "percent": "10000", - "reputation": 14709760292085, - "rshares": 19321220012, - "voter": "yazp" - }, - { - "percent": "10000", - "reputation": 6823769560292, - "rshares": 6228550749, - "voter": "thesobuz" - }, - { - "percent": "10000", - "reputation": 2045506354809, - "rshares": 1704154825, - "voter": "steeminer4up" - }, - { - "percent": "10000", - "reputation": 10582665763690, - "rshares": 553802663508, - "voter": "tazi" - }, - { - "percent": "10000", - "reputation": 686525892561, - "rshares": 162811953758, - "voter": "lebin" - }, - { - "percent": "10000", - "reputation": 66785151966461, - "rshares": 482726538369, - "voter": "itchyfeetdonica" - }, - { - "percent": "10000", - "reputation": 61297887349807, - "rshares": 17624752195, - "voter": "nathen007" - }, - { - "percent": "7000", - "reputation": 22087086438734, - "rshares": 37701863537, - "voter": "fjcalduch" - }, - { - "percent": "4000", - "reputation": 9242922601755, - "rshares": 17615434619, - "voter": "marcolino76" - }, - { - "percent": "10000", - "reputation": 9896237488, - "rshares": 18817844596, - "voter": "howiemac" - }, - { - "percent": "10000", - "reputation": 9705859783834, - "rshares": 24116284082, - "voter": "spiritabsolute" - }, - { - "percent": "5000", - "reputation": 5994128131575, - "rshares": 1968720360, - "voter": "jrungi" - }, - { - "percent": "5000", - "reputation": 618706115961, - "rshares": 616068298, - "voter": "lethsrock" - }, - { - "percent": "2500", - "reputation": 2675225025765, - "rshares": 7143399851, - "voter": "fourfourfun" - }, - { - "percent": "10000", - "reputation": 9071994818465, - "rshares": 626927056, - "voter": "candyboy" - }, - { - "percent": "10000", - "reputation": 112593705937795, - "rshares": 173591580441, - "voter": "apnigrich" - }, - { - "percent": "10000", - "reputation": 6926909241271, - "rshares": 20543871630, - "voter": "dechastre" - }, - { - "percent": "10000", - "reputation": 73184833081444, - "rshares": 4270867201477, - "voter": "oliverschmid" - }, - { - "percent": "5000", - "reputation": 87246032649758, - "rshares": 87307583155, - "voter": "gabrielatravels" - }, - { - "percent": "10000", - "reputation": 226477842496, - "rshares": 826600484, - "voter": "thedrewshow" - }, - { - "percent": "1000", - "reputation": 6794111379268, - "rshares": 1099927057, - "voter": "dudeontheweb" - }, - { - "percent": "10000", - "reputation": 109380383179709, - "rshares": 79885653459, - "voter": "edwardstobia" - }, - { - "percent": "10000", - "reputation": 105965337989608, - "rshares": 1510222515, - "voter": "koreaminer" - }, - { - "percent": "2250", - "reputation": 1451555574844, - "rshares": 845768980, - "voter": "korinkrafting" - }, - { - "percent": "5000", - "reputation": 34389579567199, - "rshares": 49637101794, - "voter": "hijosdelhombre" - }, - { - "percent": "10000", - "reputation": 3933285221882, - "rshares": 1000326096, - "voter": "santarius" - }, - { - "percent": "10000", - "reputation": 167323940570569, - "rshares": 519930458140, - "voter": "bala41288" - }, - { - "percent": "10000", - "reputation": 316164161900, - "rshares": 1334569230, - "voter": "acronyms" - }, - { - "percent": "10000", - "reputation": 51453243377505, - "rshares": 1768200399, - "voter": "socialmediaseo" - }, - { - "percent": "10000", - "reputation": 3895318402889, - "rshares": 1547281751, - "voter": "criptonotas" - }, - { - "percent": "4500", - "reputation": 191209873138075, - "rshares": 50588464904, - "voter": "mermaidvampire" - }, - { - "percent": "5000", - "reputation": 22213386192816, - "rshares": 1853670548, - "voter": "jimcustodio" - }, - { - "percent": "3000", - "reputation": 62275371513698, - "rshares": 1147837008, - "voter": "vaansteam" - }, - { - "percent": "10000", - "reputation": 959567888850, - "rshares": 128909772986, - "voter": "nnaraoh" - }, - { - "percent": "1000", - "reputation": 4436444427883, - "rshares": 888510694, - "voter": "tricki" - }, - { - "percent": "10000", - "reputation": 11165935981106, - "rshares": 713754509, - "voter": "icuz" - }, - { - "percent": "10000", - "reputation": 2848881778824, - "rshares": 934856878, - "voter": "srikandi" - }, - { - "percent": "5100", - "reputation": 330396781271113, - "rshares": 2339024152373, - "voter": "holger80" - }, - { - "percent": "1500", - "reputation": 50124640567504, - "rshares": 4711101092, - "voter": "dailychina" - }, - { - "percent": "5000", - "reputation": 51254284112658, - "rshares": 59296817393, - "voter": "shmoogleosukami" - }, - { - "percent": "3000", - "reputation": 94029543548042, - "rshares": 244934782230, - "voter": "glenalbrethsen" - }, - { - "percent": "2500", - "reputation": 8909326634629, - "rshares": 16646668272, - "voter": "upfundme" - }, - { - "percent": "10000", - "reputation": 86916035515124, - "rshares": 123170830778, - "voter": "cryptosharon" - }, - { - "percent": "2000", - "reputation": 442944056818, - "rshares": 759823650, - "voter": "minerspost" - }, - { - "percent": "10000", - "reputation": 2383466345866, - "rshares": 2241151841, - "voter": "sweetkathy" - }, - { - "percent": "10000", - "reputation": 64116667167136, - "rshares": 39388181327, - "voter": "emiliocabrera" - }, - { - "percent": "9500", - "reputation": 4819938972371, - "rshares": 6808836855, - "voter": "green77" - }, - { - "percent": "10000", - "reputation": 1275793729622, - "rshares": 4644173239, - "voter": "macslin" - }, - { - "percent": "5000", - "reputation": 1912983934049, - "rshares": 1036174289, - "voter": "overmedia" - }, - { - "percent": "2000", - "reputation": 25585298727868, - "rshares": 65058676, - "voter": "maxpatternman" - }, - { - "percent": "10000", - "reputation": 20304784933273, - "rshares": 179642596914, - "voter": "barge" - }, - { - "percent": "5000", - "reputation": 2823573898487, - "rshares": 3038216726, - "voter": "thisbejake" - }, - { - "percent": "10000", - "reputation": 17846302258319, - "rshares": 38948720050, - "voter": "onlavu" - }, - { - "percent": "5000", - "reputation": 6264286399143, - "rshares": 1123109129309, - "voter": "asgarth" - }, - { - "percent": "10000", - "reputation": 12186132605, - "rshares": 1673485239, - "voter": "magicbirds" - }, - { - "percent": "10000", - "reputation": 8653571432, - "rshares": 1675248109, - "voter": "blueproject" - }, - { - "percent": "10000", - "reputation": 41394952718, - "rshares": 1676147728, - "voter": "django137" - }, - { - "percent": "10000", - "reputation": 5904893661018, - "rshares": 1541533272, - "voter": "fiveelements5" - }, - { - "percent": "10000", - "reputation": 8493141387, - "rshares": 1674626228, - "voter": "goldeneye64" - }, - { - "percent": "10000", - "reputation": 65151962039, - "rshares": 1672109905, - "voter": "ludezma" - }, - { - "percent": "10000", - "reputation": 11867738310700, - "rshares": 1538193863, - "voter": "oleg5430" - }, - { - "percent": "10000", - "reputation": 22399611269460, - "rshares": 20112956280, - "voter": "cyprianj" - }, - { - "percent": "10000", - "reputation": 2506608157147, - "rshares": 72896302977, - "voter": "cst90" - }, - { - "percent": "500", - "reputation": 6268791987206, - "rshares": 4042791874, - "voter": "ajaxalot" - }, - { - "percent": "10000", - "reputation": 62406194635091, - "rshares": 44376600973, - "voter": "russellstockley" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 29607760115, - "voter": "futurecurrency" - }, - { - "percent": "2500", - "reputation": 18673367244, - "rshares": 10604279796, - "voter": "g4fun" - }, - { - "percent": "1000", - "reputation": 85785149964848, - "rshares": 2640185969, - "voter": "putu300" - }, - { - "percent": "10000", - "reputation": 29800653327707, - "rshares": 255710216578, - "voter": "cryptictruth" - }, - { - "percent": "10000", - "reputation": 5056541317353, - "rshares": 5525361618, - "voter": "carlosp18" - }, - { - "percent": "2500", - "reputation": 15537102540153, - "rshares": 26456870724, - "voter": "siphon" - }, - { - "percent": "10000", - "reputation": 3414691338324, - "rshares": 2314358667, - "voter": "homeginkit" - }, - { - "percent": "10000", - "reputation": 19394821686291, - "rshares": 3370184156, - "voter": "idkpdx" - }, - { - "percent": "50", - "reputation": 243323637506719, - "rshares": 681815064, - "voter": "richatvns" - }, - { - "percent": "5000", - "reputation": 36232951266359, - "rshares": 9193000632, - "voter": "adenijiadeshina" - }, - { - "percent": "10000", - "reputation": 3441314325667, - "rshares": 132101275860, - "voter": "norwegianbikeman" - }, - { - "percent": "10000", - "reputation": 297110152696, - "rshares": 47344242950, - "voter": "properfraction" - }, - { - "percent": "3000", - "reputation": 15600949256447, - "rshares": 649349269, - "voter": "lordjames" - }, - { - "percent": "10000", - "reputation": 12743657794449, - "rshares": 21744835707, - "voter": "wedacoalition" - }, - { - "percent": "2307", - "reputation": 46955154178798, - "rshares": 164307548983, - "voter": "amico" - }, - { - "percent": "5000", - "reputation": 2590633023991, - "rshares": 1770879758, - "voter": "sunshinebear" - }, - { - "percent": "10000", - "reputation": 397421034392, - "rshares": 174715566403, - "voter": "steemitcolombia" - }, - { - "percent": "800", - "reputation": 420243407095468, - "rshares": 9799448754, - "voter": "onepercentbetter" - }, - { - "percent": "5000", - "reputation": 1137313020354, - "rshares": 1826606230, - "voter": "nebuladream" - }, - { - "percent": "10000", - "reputation": 25978211905358, - "rshares": 270610451810, - "voter": "manniman" - }, - { - "percent": "10000", - "reputation": 13852827798556, - "rshares": 627546953838, - "voter": "dera123" - }, - { - "percent": "9000", - "reputation": 1108677568897, - "rshares": 5885124412, - "voter": "jan23com" - }, - { - "percent": "10000", - "reputation": 28643888754007, - "rshares": 35617158228, - "voter": "paragism" - }, - { - "percent": "10000", - "reputation": 4110594694460, - "rshares": 1156833406576, - "voter": "jkramer" - }, - { - "percent": "10000", - "reputation": 373679836722908, - "rshares": 3267540130725, - "voter": "derangedvisions" - }, - { - "percent": "4000", - "reputation": 17410482134249, - "rshares": 16258470092, - "voter": "wolfhart" - }, - { - "percent": "10000", - "reputation": 489416883814, - "rshares": 563329159, - "voter": "presidentslabber" - }, - { - "percent": "2000", - "reputation": 12031073074545, - "rshares": 6623188610, - "voter": "jemmanuel" - }, - { - "percent": "10000", - "reputation": 10532279362821, - "rshares": 3847150150, - "voter": "sallyfun" - }, - { - "percent": "10000", - "reputation": 3447262923438, - "rshares": 22048347049, - "voter": "xchng" - }, - { - "percent": "10000", - "reputation": 176940331439, - "rshares": 828995181, - "voter": "gifty-e" - }, - { - "percent": "9000", - "reputation": 158718167236, - "rshares": 3601833973, - "voter": "choco11oreo11" - }, - { - "percent": "5000", - "reputation": 3773982767261, - "rshares": 2748677518, - "voter": "michellpiala" - }, - { - "percent": "5000", - "reputation": 8020932518298, - "rshares": 6327891469, - "voter": "naruitchi" - }, - { - "percent": "10000", - "reputation": 3019627724863, - "rshares": 1374366453, - "voter": "bukfast" - }, - { - "percent": "10000", - "reputation": 109742320788, - "rshares": 6747535287, - "voter": "thegoldencobra" - }, - { - "percent": "10000", - "reputation": 2481629111221, - "rshares": 2102369711, - "voter": "manojbhatt" - }, - { - "percent": "10000", - "reputation": 947135854087, - "rshares": 8062909577, - "voter": "flyinghigher" - }, - { - "percent": "5000", - "reputation": 2418623268693, - "rshares": 1520944803, - "voter": "joeytechtalks" - }, - { - "percent": "10000", - "reputation": 9088730363734, - "rshares": 6367783345, - "voter": "razeiv" - }, - { - "percent": "10000", - "reputation": 17764793535203, - "rshares": 8076899141, - "voter": "josebenavente" - }, - { - "percent": "10000", - "reputation": 19409877558565, - "rshares": 99670219380, - "voter": "mraggaj" - }, - { - "percent": "10000", - "reputation": 5764675494828, - "rshares": 78885299526, - "voter": "calisay" - }, - { - "percent": "3500", - "reputation": 515972450479, - "rshares": 1328529467, - "voter": "raorac" - }, - { - "percent": "10000", - "reputation": 3964698404, - "rshares": 20515753902, - "voter": "savagebits" - }, - { - "percent": "2000", - "reputation": 1072061813246, - "rshares": 3980228199, - "voter": "yaraha" - }, - { - "percent": "10000", - "reputation": 9020628089607, - "rshares": 86416686962, - "voter": "promobot" - }, - { - "percent": "5000", - "reputation": 48846679188, - "rshares": 647942458, - "voter": "youraverageguy" - }, - { - "percent": "10000", - "reputation": 7093325367923, - "rshares": 2319420185, - "voter": "glodniwiedzy" - }, - { - "percent": "3000", - "reputation": 10484590752220, - "rshares": 54228837864, - "voter": "veteranforcrypto" - }, - { - "percent": "10000", - "reputation": 13196413386545, - "rshares": 19234061637, - "voter": "dunite" - }, - { - "percent": "3000", - "reputation": 25665583431709, - "rshares": 47916222435, - "voter": "rozku" - }, - { - "percent": "10000", - "reputation": 461201929261615, - "rshares": 5056566786889, - "voter": "slobberchops" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 19255656600, - "voter": "diabonua" - }, - { - "percent": "10000", - "reputation": 2483077279116, - "rshares": 987301900, - "voter": "ederaleng" - }, - { - "percent": "10000", - "reputation": 42432428703, - "rshares": 1068576363, - "voter": "jasuly" - }, - { - "percent": "5000", - "reputation": 12736574015031, - "rshares": 4188777017, - "voter": "sayago" - }, - { - "percent": "5000", - "reputation": 8665552760808, - "rshares": 5127748025, - "voter": "aceaeterna" - }, - { - "percent": "10000", - "reputation": 1131137096970, - "rshares": 4103338391, - "voter": "justasperm" - }, - { - "percent": "1000", - "reputation": 1917096247997, - "rshares": 531044949, - "voter": "crimo" - }, - { - "percent": "10000", - "reputation": 30354224324543, - "rshares": 35826772393, - "voter": "aliriera" - }, - { - "percent": "10000", - "reputation": 27745376951772, - "rshares": 93392007390, - "voter": "stevenwood" - }, - { - "percent": "1500", - "reputation": 153947835280090, - "rshares": 128618442497, - "voter": "enforcer48" - }, - { - "percent": "10000", - "reputation": 17999475515816, - "rshares": 9427262249, - "voter": "sk1920" - }, - { - "percent": "10000", - "reputation": 17822269400, - "rshares": 1112153655, - "voter": "competeapp" - }, - { - "percent": "5000", - "reputation": 5250606461850, - "rshares": 3304792411, - "voter": "angatt" - }, - { - "percent": "10000", - "reputation": 4762022043623, - "rshares": 3224154903, - "voter": "fernandosoder" - }, - { - "percent": "1000", - "reputation": 406732179716535, - "rshares": 133228589168, - "voter": "cryptoandcoffee" - }, - { - "percent": "10000", - "reputation": 254937223188, - "rshares": 889157457, - "voter": "svm038" - }, - { - "percent": "10000", - "reputation": 15332816096733, - "rshares": 3158611746, - "voter": "luminaryhmo" - }, - { - "percent": "800", - "reputation": 56006422908236, - "rshares": 2605801617, - "voter": "merlion" - }, - { - "percent": "5000", - "reputation": 119602971288, - "rshares": 3314986072, - "voter": "bububoomt" - }, - { - "percent": "10000", - "reputation": 2634900617603, - "rshares": 3265506667, - "voter": "vampire-steem" - }, - { - "percent": "5000", - "reputation": 3261043121278, - "rshares": 2312763580, - "voter": "somegaming" - }, - { - "percent": "250", - "reputation": 20023805487967, - "rshares": 858829318, - "voter": "moneybaby" - }, - { - "percent": "4900", - "reputation": 52047051239253, - "rshares": 141945276338, - "voter": "apshamilton" - }, - { - "percent": "1300", - "reputation": 68462748929740, - "rshares": 127400601592, - "voter": "roger5120" - }, - { - "percent": "10000", - "reputation": 9718632602, - "rshares": 1674370891, - "voter": "prudencia" - }, - { - "percent": "10000", - "reputation": 12580096938, - "rshares": 1671512525, - "voter": "gorka" - }, - { - "percent": "10000", - "reputation": 13059317148, - "rshares": 1672626913, - "voter": "suley" - }, - { - "percent": "10000", - "reputation": 77339641259264, - "rshares": 120052094431, - "voter": "nathyortiz" - }, - { - "percent": "10000", - "reputation": 199806038280, - "rshares": 801936301, - "voter": "bingbabe" - }, - { - "percent": "10000", - "reputation": 6990715593, - "rshares": 1671466022, - "voter": "zulema" - }, - { - "percent": "10000", - "reputation": 10089127541, - "rshares": 1673430423, - "voter": "herencia" - }, - { - "percent": "10000", - "reputation": 9724555279, - "rshares": 1670946959, - "voter": "zoraida" - }, - { - "percent": "10000", - "reputation": 4252099247, - "rshares": 1672042857, - "voter": "embera" - }, - { - "percent": "10000", - "reputation": 6224691662, - "rshares": 1672715760, - "voter": "medula" - }, - { - "percent": "10000", - "reputation": 3158689060, - "rshares": 1752534777, - "voter": "chavela" - }, - { - "percent": "10000", - "reputation": 7291463397, - "rshares": 1671535076, - "voter": "tierra" - }, - { - "percent": "10000", - "reputation": 38511327979971, - "rshares": 2530016957, - "voter": "scrawly" - }, - { - "percent": "10000", - "reputation": 14885099448, - "rshares": 3438026813, - "voter": "hemo" - }, - { - "percent": "9500", - "reputation": 33860216986858, - "rshares": 582334336311, - "voter": "donald.porter" - }, - { - "percent": "10000", - "reputation": 662038866365, - "rshares": 2280074139, - "voter": "antoniojoseha" - }, - { - "percent": "2000", - "reputation": 6592285898816, - "rshares": 11997723208, - "voter": "schlunior" - }, - { - "percent": "10000", - "reputation": 671978982346, - "rshares": 439790726, - "voter": "sonder-an" - }, - { - "percent": "2000", - "reputation": 118140326729872, - "rshares": 7279228277, - "voter": "sadbear" - }, - { - "percent": "3100", - "reputation": 3017320382626, - "rshares": 12248801498, - "voter": "yestermorrow" - }, - { - "percent": "4900", - "reputation": 558380924105, - "rshares": 162646577036, - "voter": "hamismsf" - }, - { - "percent": "7000", - "reputation": 44525854399402, - "rshares": 111533526822, - "voter": "thehive" - }, - { - "percent": "10000", - "reputation": 2676237097489, - "rshares": 4077236016, - "voter": "fandelkefir" - }, - { - "percent": "10000", - "reputation": 218631318815, - "rshares": 869426618, - "voter": "light-hearted" - }, - { - "percent": "4410", - "reputation": 425226010828, - "rshares": 20228275927, - "voter": "yaelg" - }, - { - "percent": "5000", - "reputation": 2880166082909, - "rshares": 1461117108, - "voter": "kepslok" - }, - { - "percent": "10000", - "reputation": 224248801997887, - "rshares": 4902651369, - "voter": "flaws" - }, - { - "percent": "800", - "reputation": 17939211305935, - "rshares": 39091601256, - "voter": "raiseup" - }, - { - "percent": "9000", - "reputation": 22555392895, - "rshares": 5443302487, - "voter": "littleshadow" - }, - { - "percent": "10000", - "reputation": 3425708064391, - "rshares": 64636318180, - "voter": "goingbonkers" - }, - { - "percent": "10000", - "reputation": 71506876418188, - "rshares": 151706765166, - "voter": "cryptoyzzy" - }, - { - "percent": "2000", - "reputation": 144910360949054, - "rshares": 38319201392, - "voter": "voxmortis" - }, - { - "percent": "9000", - "reputation": 17567685388, - "rshares": 1852560373, - "voter": "joseph6232" - }, - { - "percent": "7500", - "reputation": 19783849759, - "rshares": 3295439662, - "voter": "emaillisahere" - }, - { - "percent": "800", - "reputation": 57031563221, - "rshares": 65460226568, - "voter": "steemaction" - }, - { - "percent": "5000", - "reputation": 19563050817, - "rshares": 632125607, - "voter": "buzzbee" - }, - { - "percent": "5100", - "reputation": 36498710441788, - "rshares": 17224105238, - "voter": "fullnodeupdate" - }, - { - "percent": "10000", - "reputation": 58772053733860, - "rshares": 1750241898018, - "voter": "engrave" - }, - { - "percent": "2500", - "reputation": 7344887427854, - "rshares": 36011797101, - "voter": "altonos" - }, - { - "percent": "10000", - "reputation": 1503728134655, - "rshares": 505997077844, - "voter": "tipsybosphorus" - }, - { - "percent": "10000", - "reputation": 133998446537867, - "rshares": 3588854658, - "voter": "bluengel" - }, - { - "percent": "10000", - "reputation": 1494229786604, - "rshares": 147683294469, - "voter": "pboulet" - }, - { - "percent": "10000", - "reputation": 22644614192, - "rshares": 971775653, - "voter": "chike4545" - }, - { - "percent": "10000", - "reputation": 37453744817, - "rshares": 4286364306, - "voter": "caoimhin" - }, - { - "percent": "7500", - "reputation": 27727654562, - "rshares": 1483593024, - "voter": "djtrucker" - }, - { - "percent": "2500", - "reputation": 6660418695187, - "rshares": 654456242, - "voter": "steemituplife" - }, - { - "percent": "10000", - "reputation": 71329447478265, - "rshares": 604231910835, - "voter": "vimm" - }, - { - "percent": "10000", - "reputation": 45236011216729, - "rshares": 64323922590, - "voter": "cultus-forex" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 7713280408, - "voter": "quediceharry" - }, - { - "percent": "8500", - "reputation": 260876943473, - "rshares": 76235904642, - "voter": "marshalmugi" - }, - { - "percent": "9000", - "reputation": 1786834467, - "rshares": 1760214698, - "voter": "podg3" - }, - { - "percent": "10000", - "reputation": 517609266090, - "rshares": 966516944, - "voter": "steemwhalepower" - }, - { - "percent": "10000", - "reputation": 41288629235348, - "rshares": 10454589540, - "voter": "mariluna" - }, - { - "percent": "5000", - "reputation": 6687049412835, - "rshares": 38408450058, - "voter": "mister-meeseeks" - }, - { - "percent": "9000", - "reputation": 2040204650, - "rshares": 6643992142, - "voter": "misstaken" - }, - { - "percent": "10000", - "reputation": 1648086760085, - "rshares": 29220284847, - "voter": "diegor" - }, - { - "percent": "10000", - "reputation": 4900638213894, - "rshares": 131780218684, - "voter": "dallas27" - }, - { - "percent": "10000", - "reputation": 9265885311129, - "rshares": 2692007541, - "voter": "gameeit" - }, - { - "percent": "1500", - "reputation": 3325840223967, - "rshares": 821825505, - "voter": "florino" - }, - { - "percent": "10000", - "reputation": 14391246828388, - "rshares": 17621859293, - "voter": "geeklania" - }, - { - "percent": "10000", - "reputation": 44905371389767, - "rshares": 1869005942, - "voter": "cremisi" - }, - { - "percent": "10000", - "reputation": 2693573458412, - "rshares": 659749384, - "voter": "carbodexkim" - }, - { - "percent": "10000", - "reputation": 45419481582603, - "rshares": 369752348459, - "voter": "michealb" - }, - { - "percent": "9000", - "reputation": 4187177339, - "rshares": 2061148360, - "voter": "jussbren" - }, - { - "percent": "10000", - "reputation": 57025241141, - "rshares": 3753448309, - "voter": "iovoccae" - }, - { - "percent": "10000", - "reputation": 94595445913731, - "rshares": 1230564291769, - "voter": "glastar" - }, - { - "percent": "10000", - "reputation": 30281770348, - "rshares": 3628327693, - "voter": "themightysquid" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1079102362, - "voter": "bgornicki" - }, - { - "percent": "10000", - "reputation": 3633716322140, - "rshares": 1324693455, - "voter": "marki99" - }, - { - "percent": "5000", - "reputation": 49418347777, - "rshares": 671207880, - "voter": "keket" - }, - { - "percent": "10000", - "reputation": 53589831946, - "rshares": 3726601488, - "voter": "steemlandia" - }, - { - "percent": "10000", - "reputation": 5493311683937, - "rshares": 105557644427, - "voter": "kekos" - }, - { - "percent": "10000", - "reputation": 107566925670081, - "rshares": 1682032818, - "voter": "tamito0201" - }, - { - "percent": "5000", - "reputation": 1867101038475, - "rshares": 1048492524, - "voter": "starrouge" - }, - { - "percent": "3000", - "reputation": 854097216443, - "rshares": 602258631, - "voter": "redheaddemon" - }, - { - "percent": "4900", - "reputation": 3051298620925, - "rshares": 143184254513, - "voter": "jpbliberty" - }, - { - "percent": "10000", - "reputation": 57787971691858, - "rshares": 297841794027, - "voter": "wherein" - }, - { - "percent": "-10000", - "reputation": 3822265637039, - "rshares": -477690050, - "voter": "atempt" - }, - { - "percent": "10000", - "reputation": 21654779562526, - "rshares": 123592013196, - "voter": "bluerobo" - }, - { - "percent": "5000", - "reputation": 1330122595989, - "rshares": 828499889, - "voter": "zerofive" - }, - { - "percent": "10000", - "reputation": 13815761380, - "rshares": 44976391019, - "voter": "tigerrkg" - }, - { - "percent": "10000", - "reputation": 3320862551756, - "rshares": 3020056690, - "voter": "giampaolo19" - }, - { - "percent": "10000", - "reputation": 671364595259, - "rshares": 1986895268, - "voter": "smon-fan" - }, - { - "percent": "10000", - "reputation": 393881380327, - "rshares": 1013916165, - "voter": "bilderkiste" - }, - { - "percent": "10000", - "reputation": 2488971014315, - "rshares": 1669886999, - "voter": "tr777" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 605774787, - "voter": "sm-jewel" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 42791446404, - "voter": "coriolis" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1419182804, - "voter": "smoner" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1652330297, - "voter": "smonian" - }, - { - "percent": "10000", - "reputation": -599589680091, - "rshares": 981777794, - "voter": "carrycarrie" - }, - { - "percent": "10000", - "reputation": 143953645708, - "rshares": 697289012, - "voter": "honeygirl" - }, - { - "percent": "10000", - "reputation": 61527381175492, - "rshares": 238638108172, - "voter": "cnstm" - }, - { - "percent": "10000", - "reputation": 64935903718, - "rshares": 662320986, - "voter": "likuang007" - }, - { - "percent": "3500", - "reputation": 72504131225, - "rshares": 418826948, - "voter": "agent14" - }, - { - "percent": "10000", - "reputation": 21122210064846, - "rshares": 20073524130, - "voter": "vickyguevara" - }, - { - "percent": "10000", - "reputation": 97219598927367, - "rshares": 59727138768, - "voter": "josehany" - }, - { - "percent": "10000", - "reputation": 774128044503, - "rshares": 1769353238, - "voter": "caribehub" - }, - { - "percent": "10000", - "reputation": 54892997399545, - "rshares": 965737276, - "voter": "smon-joa" - }, - { - "percent": "10000", - "reputation": 5230675637, - "rshares": 1443023662, - "voter": "jjangjjanggirl" - }, - { - "percent": "10000", - "reputation": 644453947703, - "rshares": 998794037, - "voter": "lianjingmedia" - }, - { - "percent": "5000", - "reputation": 7461416844046, - "rshares": 8160517958, - "voter": "broxi" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 7241539236, - "voter": "hanke" - }, - { - "percent": "10000", - "reputation": 32642102387768, - "rshares": 27797171420, - "voter": "creacioneslelys" - }, - { - "percent": "800", - "reputation": 18701458698214, - "rshares": 222525617, - "voter": "hungrybear" - }, - { - "percent": "2500", - "reputation": 5616865862, - "rshares": 5492695151, - "voter": "abbenay" - }, - { - "percent": "5000", - "reputation": 5833218185, - "rshares": 809256591, - "voter": "smonbear" - }, - { - "percent": "10000", - "reputation": 42787624679630, - "rshares": 29136894485, - "voter": "hungryharish" - }, - { - "percent": "10000", - "reputation": 2687072106243, - "rshares": 1849043263, - "voter": "steem-queen" - }, - { - "percent": "500", - "reputation": 2829489656369, - "rshares": 3299157391, - "voter": "src3" - }, - { - "percent": "10000", - "reputation": 489555860442, - "rshares": 3223205970, - "voter": "bitcoinator" - }, - { - "percent": "5000", - "reputation": 4189876953003, - "rshares": 2732501876, - "voter": "hungryanu" - }, - { - "percent": "5000", - "reputation": 221868627502, - "rshares": 689393493, - "voter": "szf" - }, - { - "percent": "10000", - "reputation": 1363042643708, - "rshares": 182960973258, - "voter": "maini" - }, - { - "percent": "2000", - "reputation": 1243018036066, - "rshares": 1336089560, - "voter": "wallvater" - }, - { - "percent": "10000", - "reputation": 57809481255873, - "rshares": 4334735569428, - "voter": "memehub" - }, - { - "percent": "10000", - "reputation": 19813410675811, - "rshares": 455048660327, - "voter": "battlegames" - }, - { - "percent": "9500", - "reputation": 14559983850035, - "rshares": 45366356950, - "voter": "poliwalt10" - }, - { - "percent": "10000", - "reputation": 11781430795832, - "rshares": 284280679855, - "voter": "kytka" - }, - { - "percent": "10000", - "reputation": 10702198150786, - "rshares": 280101419686, - "voter": "scholaris" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 53149628839, - "voter": "banvie" - }, - { - "percent": "10000", - "reputation": 9877498112396, - "rshares": 1296518479, - "voter": "ssc-token" - }, - { - "percent": "10000", - "reputation": 36087507440149, - "rshares": 746245461823, - "voter": "likwid" - }, - { - "percent": "3000", - "reputation": 35255278557021, - "rshares": 4863680568, - "voter": "plankton.token" - }, - { - "percent": "300", - "reputation": 16313879716356, - "rshares": 1197343632, - "voter": "tggr" - }, - { - "percent": "2500", - "reputation": 1138890453375, - "rshares": 1526743621, - "voter": "iamjohn" - }, - { - "percent": "10000", - "reputation": 11624344336093, - "rshares": 372683651806, - "voter": "recording-box" - }, - { - "percent": "4900", - "reputation": 268359697210194, - "rshares": 171461891112, - "voter": "maxwellmarcusart" - }, - { - "percent": "10000", - "reputation": 63432644423328, - "rshares": 72601273620, - "voter": "helcim" - }, - { - "percent": "2500", - "reputation": 301977647, - "rshares": 45124004248, - "voter": "xyz004" - }, - { - "percent": "10000", - "reputation": 442801860940, - "rshares": 16810415009, - "voter": "steemindian" - }, - { - "percent": "10000", - "reputation": 2895538498602, - "rshares": 141576157650, - "voter": "oldoneeye" - }, - { - "percent": "10000", - "reputation": 10026344348529, - "rshares": 589044498, - "voter": "alexvalera" - }, - { - "percent": "10000", - "reputation": 9163578879, - "rshares": 546927561, - "voter": "suigener1s" - }, - { - "percent": "3750", - "reputation": 10434685317438, - "rshares": 545016267, - "voter": "the.circle" - }, - { - "percent": "10000", - "reputation": 13293436383248, - "rshares": 961971869, - "voter": "lookplz" - }, - { - "percent": "10000", - "reputation": 773985194624, - "rshares": 2910509709, - "voter": "kgswallet" - }, - { - "percent": "9000", - "reputation": 12552713403211, - "rshares": 17077732547, - "voter": "yeswecan" - }, - { - "percent": "10000", - "reputation": 165757662725, - "rshares": 2946495191, - "voter": "lrekt01" - }, - { - "percent": "2975", - "reputation": 14712355832971, - "rshares": 4043846388, - "voter": "reggaesteem" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 2922662629, - "voter": "nichemarket" - }, - { - "percent": "3000", - "reputation": 91115882126, - "rshares": 2431007347, - "voter": "hyborian-strain" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 2818351886, - "voter": "babytarazkp" - }, - { - "percent": "2900", - "reputation": 9809854088378, - "rshares": 2801744145, - "voter": "mapxv" - }, - { - "percent": "10000", - "reputation": 376803157115, - "rshares": 354264725, - "voter": "tina-tina" - }, - { - "percent": "10000", - "reputation": 24427078477153, - "rshares": 6290832653, - "voter": "prolinuxua" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "happiness19" - }, - { - "percent": "2777", - "reputation": 18505058691598, - "rshares": 146089944172, - "voter": "logiczombie" - }, - { - "percent": "10000", - "reputation": 97507720606, - "rshares": 31902342, - "voter": "gdhaetae" - }, - { - "percent": "10000", - "reputation": 770042020971, - "rshares": 27223636499, - "voter": "acta" - }, - { - "percent": "10000", - "reputation": 5889854881, - "rshares": 20382539503, - "voter": "the-table" - }, - { - "percent": "10000", - "reputation": 266042222060, - "rshares": 2381746461, - "voter": "cardtrader" - }, - { - "percent": "700", - "reputation": 426404968, - "rshares": 30035531007, - "voter": "maxuvd" - }, - { - "percent": "600", - "reputation": 6113738572, - "rshares": 31714110695, - "voter": "maxuve" - }, - { - "percent": "10000", - "reputation": 1704173681552, - "rshares": 2268268450, - "voter": "bilpcoinbot" - }, - { - "percent": "2500", - "reputation": 31428862615, - "rshares": 4396596379, - "voter": "tmps" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 412168923393, - "voter": "btscn" - }, - { - "percent": "9000", - "reputation": 19199339523, - "rshares": 1918787160, - "voter": "thehouse" - }, - { - "percent": "690", - "reputation": 3195367578140, - "rshares": 7528413514, - "voter": "joshmania" - }, - { - "percent": "7000", - "reputation": 207492608614, - "rshares": 7560131581, - "voter": "ibelin" - }, - { - "percent": "10000", - "reputation": 5553103337423, - "rshares": 5074392362, - "voter": "youngmusician" - }, - { - "percent": "9000", - "reputation": 967638294423, - "rshares": 134465363714, - "voter": "silverquest" - }, - { - "percent": "10000", - "reputation": 31259099187671, - "rshares": 27445033110, - "voter": "lesmann" - }, - { - "percent": "10000", - "reputation": 17325573495839, - "rshares": 47514744073, - "voter": "kaeserotor" - }, - { - "percent": "8500", - "reputation": 268654389989, - "rshares": 10236793566, - "voter": "honeychip" - }, - { - "percent": "1000", - "reputation": 0, - "rshares": 6620249068, - "voter": "snoochieboochies" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 4338161411, - "voter": "rockface" - }, - { - "percent": "800", - "reputation": 2957803689, - "rshares": 35742720308, - "voter": "policewala" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1134824898, - "voter": "galenkp.aus" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 807571529, - "voter": "hatta.jahm" - }, - { - "percent": "10000", - "reputation": 96421687900488, - "rshares": 48308204566, - "voter": "lachg89" - }, - { - "percent": "5000", - "reputation": 1201079161955, - "rshares": 554011490, - "voter": "gmlrecordz" - }, - { - "percent": "2000", - "reputation": 133245362827, - "rshares": 1463328514, - "voter": "mvanhauten" - }, - { - "percent": "4000", - "reputation": 4834237142168, - "rshares": 4809669920, - "voter": "journeyofanomad" - }, - { - "percent": "10000", - "reputation": 40716090911, - "rshares": 642588051, - "voter": "angel33" - }, - { - "percent": "10000", - "reputation": 23010538544428, - "rshares": 27635753831, - "voter": "garlet" - }, - { - "percent": "10000", - "reputation": 9115515000, - "rshares": 571987748, - "voter": "onestop" - }, - { - "percent": "5000", - "reputation": 32542453022, - "rshares": 4805427019, - "voter": "bilpcoinbpc" - }, - { - "percent": "5000", - "reputation": 14577043701834, - "rshares": 7435934040, - "voter": "julesquirin" - }, - { - "percent": "4000", - "reputation": 9624495306704, - "rshares": 48355554547298, - "voter": "darthknight" - }, - { - "percent": "1000", - "reputation": 29462647124297, - "rshares": 7474106508, - "voter": "mehmetfix" - }, - { - "percent": "10000", - "reputation": 1588836327, - "rshares": 0, - "voter": "wasifbhutto" - }, - { - "percent": "10000", - "reputation": 164450582774349, - "rshares": 130759037757, - "voter": "alexa.art" - }, - { - "percent": "9000", - "reputation": 30255784377, - "rshares": 1758808181, - "voter": "tommys.shop" - }, - { - "percent": "5000", - "reputation": 38576347919541, - "rshares": 5048806407, - "voter": "dapplr" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 524095245538, - "voter": "fengchao" - }, - { - "percent": "10000", - "reputation": 86868289888522, - "rshares": 1856613668861, - "voter": "peakd" - }, - { - "percent": "7500", - "reputation": 355888101482, - "rshares": 575329119, - "voter": "hivewaves" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 2190956128, - "voter": "blue-witness" - }, - { - "percent": "4500", - "reputation": 3242356575612, - "rshares": 9112536497, - "voter": "walarhein" - }, - { - "percent": "10000", - "reputation": 37484144443, - "rshares": 398858588254, - "voter": "mountaingod" - }, - { - "percent": "400", - "reputation": 91987050007999, - "rshares": 157407114, - "voter": "hiveyoda" - }, - { - "percent": "3500", - "reputation": 5219413655836, - "rshares": 137215706382, - "voter": "reggaejahm" - }, - { - "percent": "10000", - "reputation": 23527547722482, - "rshares": 2593266366, - "voter": "diosaerys" - }, - { - "percent": "6000", - "reputation": 1209731647511, - "rshares": 1028633246321, - "voter": "softworld" - }, - { - "percent": "8000", - "reputation": 27809550004754, - "rshares": 5431293378, - "voter": "uzzca" - }, - { - "percent": "10000", - "reputation": 17500919200979, - "rshares": 47078229492, - "voter": "arlettemsalase" - }, - { - "percent": "10000", - "reputation": 6105958100194, - "rshares": 1757949335, - "voter": "dagamers" - }, - { - "percent": "10000", - "reputation": 90463833228384, - "rshares": 40753926220, - "voter": "freakeao" - }, - { - "percent": "1000", - "reputation": 661148949025, - "rshares": 614571574, - "voter": "gradeon" - }, - { - "percent": "7500", - "reputation": 5014132859, - "rshares": 1507650831, - "voter": "paulman" - }, - { - "percent": "2500", - "reputation": 5158152832671, - "rshares": 885111604, - "voter": "thelemonjay" - }, - { - "percent": "1000", - "reputation": 3177222978373, - "rshares": 9203970347, - "voter": "hivelist" - }, - { - "percent": "10000", - "reputation": 5226428326302, - "rshares": 4512465701, - "voter": "argenvista" - }, - { - "percent": "3000", - "reputation": 604442758770, - "rshares": 134321983370, - "voter": "ninnu" - }, - { - "percent": "10000", - "reputation": 21138042019452, - "rshares": 43466630372, - "voter": "quantumg" - }, - { - "percent": "2000", - "reputation": 19058471657, - "rshares": 1282843150, - "voter": "vibrasphere" - }, - { - "percent": "10000", - "reputation": 474817393502, - "rshares": 432454769, - "voter": "parung76" - }, - { - "percent": "10000", - "reputation": 7436529679810, - "rshares": 8169837250, - "voter": "belen0949" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1965543520, - "voter": "traduzindojogos" - }, - { - "percent": "10000", - "reputation": 12958683306, - "rshares": 42133567148, - "voter": "tbl" - }, - { - "percent": "10000", - "reputation": 429763208250, - "rshares": 4016240982, - "voter": "ghaazi" - }, - { - "percent": "10000", - "reputation": 849928020180, - "rshares": 719028103, - "voter": "jaguarosky" - }, - { - "percent": "10000", - "reputation": 69575389840495, - "rshares": 73914580629, - "voter": "lucianav" - }, - { - "percent": "1900", - "reputation": 15777185838, - "rshares": 526833916, - "voter": "patronpass" - }, - { - "percent": "10000", - "reputation": 28740663053599, - "rshares": 3947303657, - "voter": "heracleia" - }, - { - "percent": "10000", - "reputation": 13758538330601, - "rshares": 13171010156, - "voter": "reza-shamim" - }, - { - "percent": "2800", - "reputation": 65519590598, - "rshares": 532363790, - "voter": "plusvault" - }, - { - "percent": "4000", - "reputation": 3731217144, - "rshares": 811325936, - "voter": "sn0n.dev" - }, - { - "percent": "10000", - "reputation": 15596004215170, - "rshares": 1050285592, - "voter": "rojiso2411" - }, - { - "percent": "10000", - "reputation": 792202344312, - "rshares": 751150677, - "voter": "ollasysartenes" - }, - { - "percent": "7500", - "reputation": 218724307238, - "rshares": 12421815827, - "voter": "facilitymanager" - }, - { - "percent": "10000", - "reputation": 9517022344384, - "rshares": 35109262163, - "voter": "rafaelgreen" - }, - { - "percent": "10000", - "reputation": 7461354093641, - "rshares": 4965087820, - "voter": "kasna" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 50734005866, - "voter": "gohive" - }, - { - "percent": "10000", - "reputation": 243931894056, - "rshares": 3099501840, - "voter": "mborg" - }, - { - "percent": "10000", - "reputation": 33401976736, - "rshares": 50964011106, - "voter": "aceh-media" - }, - { - "percent": "10000", - "reputation": 285629218280, - "rshares": 687122876, - "voter": "camplife" - }, - { - "percent": "10000", - "reputation": 3005998414144, - "rshares": 4138022894, - "voter": "kraken99" - }, - { - "percent": "10000", - "reputation": 387294506337, - "rshares": 3512389789, - "voter": "borghesegardens" - }, - { - "percent": "10000", - "reputation": 9058618771553, - "rshares": 8745030134, - "voter": "aurauramagic" - }, - { - "percent": "10000", - "reputation": 1263414154742, - "rshares": 4018800022, - "voter": "nalesnik" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 19212547370, - "voter": "vtol79" - }, - { - "percent": "2500", - "reputation": 222094236041, - "rshares": 5304276484, - "voter": "discohedge" - }, - { - "percent": "10000", - "reputation": 3452328685273, - "rshares": 5324877250, - "voter": "aaalviarez" - }, - { - "percent": "10000", - "reputation": 4982409525, - "rshares": 0, - "voter": "benny.blockchain" - }, - { - "percent": "3000", - "reputation": 1447273576100, - "rshares": 1584986803, - "voter": "bitcome" - }, - { - "percent": "10000", - "reputation": 16307549034965, - "rshares": 35670342811, - "voter": "trangbaby" - }, - { - "percent": "1000", - "reputation": 9727854376347, - "rshares": 1735576026, - "voter": "borbolet" - }, - { - "percent": "5000", - "reputation": 3679705780578, - "rshares": 7529809214, - "voter": "senseiphil" - }, - { - "percent": "10000", - "reputation": 26404156, - "rshares": 1005553188, - "voter": "zangano" - }, - { - "percent": "1500", - "reputation": 6746082021780, - "rshares": 1700318275, - "voter": "omarcitorojas" - }, - { - "percent": "5000", - "reputation": 2082696644794, - "rshares": 766210597, - "voter": "crazy-science" - }, - { - "percent": "10000", - "reputation": 92721511576, - "rshares": 484133200, - "voter": "rhinoceros" - }, - { - "percent": "10000", - "reputation": 86405531104, - "rshares": 1969756203, - "voter": "text2speech" - }, - { - "percent": "10000", - "reputation": 974130034782, - "rshares": 6006057377, - "voter": "aiovo" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 3818846759, - "voter": "mismo" - }, - { - "percent": "10000", - "reputation": 48820807481, - "rshares": 34448807082, - "voter": "officialhisha" - }, - { - "percent": "10000", - "reputation": 69621106111, - "rshares": 1414291796, - "voter": "violinistmarian" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 53341940726, - "voter": "betterdev" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "arlier1" - } - ], - "author": "blocktrades", - "author_reputation": 520861791056580, - "beneficiaries": [], - "body": "Last week we continued work on post-HF24 optimizations. Below is a summary of the work done last week and our plans for the upcoming week.\n\n# Hived work (blockchain node software)\n\nWe added reporting of some virtual ops related to hive fund for better accounting by a block explorer (done in conjunction with @howo):\nhttps://gitlab.syncad.com/hive/hive/-/merge_requests/144\nhttps://gitlab.syncad.com/hive/hive/-/merge_requests/135\n\nWe made fixes to filtering of get_account_history functionality and a fix to the legacy get_account_history plugin (it used a 1-based indexing of operation history instead of a 0-based indexing like the get_account_history_rocksdb plugin, now they both use 0-based indexing):\nhttps://gitlab.syncad.com/hive/hive/-/merge_requests/145\nhttps://gitlab.syncad.com/hive/hive/-/merge_requests/146\nhttps://gitlab.syncad.com/hive/hive/-/merge_requests/148\n\nFixes to hived API tests:\nhttps://gitlab.syncad.com/hive/hive/-/merge_requests/141\n\nMiscellaneous:\nhttps://gitlab.syncad.com/hive/hive/-/merge_requests/147 (set reported version to 1.24.6)\n\nWe\u2019re currently working on a major optimization to the get_block_api plugin that should likely provide a big boost in performance for the get_block API call (this will likely enable us to speed up the hivemind \u201cfull sync\u201d process as well). The old implementation used an overly pessimistic mutex locking scheme that severely degraded potential performance under loading.\n\n## Hived status\n\nWe completed all tests on changes made in the previous week and this week and there are no known outstanding issues with hived operation (other than the known longstanding issue with servicing of API requests during startup of a hived node).\n\nWe plan to tag v1.24.7 as soon as we complete the optimizations to the get_block_api plugin. V1.24.7 will be a recommended upgrade for API node operators, but it doesn\u2019t contain changes needed by witness nodes or exchanges.\n\n# Hivemind\n\nWe made numerous optimizations and bug fixes in hivemind this past week:\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/332\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/333\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/330\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/334\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/228\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/335\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/211\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/338\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/341\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/342\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/343\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/344\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/345\nOne of the more visible fixes is the comment counts are correctly reported now for posts.\n\nDue to the rapid pace with which changes are being made to hivemind, we also started upgrading our automated build-and-test (CI) system to support building on multiple gitlab runners so that our devs could get faster feedback on changes they make. \n\nThe primary challenge was to setup more than one system configured for performing a hivemind sync and to allow troubleshooting in the case of test fails). For speed reasons, hivemind\u2019s CI system is configured to only sync to the 5 millionth block, but we\u2019re adding an option to do testing with a full sync as well (via a manual trigger, as this test is much more time-consuming).\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/327\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/340\n\nWe also introduced mock data providers to allow testing of operations that didn\u2019t occur by the 5 millionth block:\nhttps://gitlab.syncad.com/hive/hivemind/-/merge_requests/336\n\n\n## Hivemind status (2nd layer social media microservice)\n\nWe deployed all the above changes to hived and hivemind to our production node, api.hive.blog, and they\u2019re working well. We still have a few optimizations to make (mostly importantly, further speedups to unread_notifications which averages around 1.5 seconds to complete right now).\n\nWe created a data dump of our hivemind database and several other API node operators have used that data dump to update their node and begin serving data with the latest code.\n\n# Condenser + Condenser wallet (open-source code for **hive.blog**)\n\nThe most visible change that BlockTrades made to condenser last week will likely be deployed tomorrow. This change will update the vote information for a post 9 seconds after the user does an upvote or a downovte on the post:\nhttps://gitlab.syncad.com/hive/condenser/-/merge_requests/136\n\n\n# What\u2019s the plan for next week?\n\nWe\u2019ll be finishing up a few more optimizations to hived and hivemind. In addition to speeding up API calls for both, we\u2019re also going to look at speeding up the hivemind full sync time (currently it takes 4 days). And we\u2019ll continue filling out the test cases in the automated testing suites for both projects.\n\nI\u2019d hope to begin analysis of future features for Hive (both for hardfork 25 and for 2nd layer apps support), but most of our time last week was consumed with optimization of the current system. We did make a little headway on this issue in the Hive developers meeting we had earlier today, though. \n\nI\u2019ll make a post later this week in the Hive improvements community on some of the features we\u2019re considering both for HF25 and for 2nd layer features (all the hardfork features are ones that have been previously discussed many times by the Hive community and have met general approval). \n\nOne of the nice things about the architecture we\u2019re moving Hive towards is that we can now add more capabilities to Hive without requiring a hardfork to do so. We will still need to do a hardfork when we make governance improvements, of course, but for many of our future features, these features can be released as they become ready, without having to coordinate their release with other features and with exchanges.", - "body_length": 6058, - "cashout_time": "2020-11-10T00:20:21", - "category": "hive-139531", - "children": 30, - "created": "2020-11-03T00:20:21", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"tags\":[\"hive\",\"blockchain\",\"software\",\"blocktrades\"],\"users\":[\"howo\"],\"links\":[\"https://gitlab.syncad.com/hive/hive/-/merge_requests/144\",\"https://gitlab.syncad.com/hive/hive/-/merge_requests/135\",\"https://gitlab.syncad.com/hive/hive/-/merge_requests/145\",\"https://gitlab.syncad.com/hive/hive/-/merge_requests/146\",\"https://gitlab.syncad.com/hive/hive/-/merge_requests/148\",\"https://gitlab.syncad.com/hive/hive/-/merge_requests/141\",\"https://gitlab.syncad.com/hive/hive/-/merge_requests/147\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/332\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/333\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/330\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/334\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/228\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/335\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/211\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/338\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/341\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/342\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/343\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/344\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/345\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/327\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/340\",\"https://gitlab.syncad.com/hive/hivemind/-/merge_requests/336\",\"https://gitlab.syncad.com/hive/condenser/-/merge_requests/136\"],\"app\":\"hiveblog/0.1\",\"format\":\"markdown\"}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-11-03T00:20:21", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 267299825359819, - "parent_author": "", - "parent_permlink": "hive-139531", - "pending_payout_value": "63.108 HBD", - "percent_hbd": 10000, - "permlink": "blocktrades-update-on-hive-development-work", - "post_id": 100367399, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "BlockTrades update on Hive development work", - "title": "BlockTrades update on Hive development work", - "total_payout_value": "0.000 HBD", - "url": "/hive-139531/@blocktrades/blocktrades-update-on-hive-development-work" - }, - { - "active_votes": [ - { - "percent": "1080", - "reputation": 19245698180508, - "rshares": 234488467129, - "voter": "tombstone" - }, - { - "percent": "10000", - "reputation": 1594304819447449, - "rshares": 10306683681088, - "voter": "acidyo" - }, - { - "percent": "6000", - "reputation": 506372806974428, - "rshares": 5411312761764, - "voter": "kevinwong" - }, - { - "percent": "5000", - "reputation": 42689564397220, - "rshares": 348167882902, - "voter": "kenny-crane" - }, - { - "percent": "600", - "reputation": 109424005488868, - "rshares": 32813155122, - "voter": "mammasitta" - }, - { - "percent": "2100", - "reputation": 34103703963623, - "rshares": 9663910902, - "voter": "matt-a" - }, - { - "percent": "10000", - "reputation": 97717041, - "rshares": 16368175008, - "voter": "field" - }, - { - "percent": "3000", - "reputation": 86171006185191, - "rshares": 11843607092, - "voter": "mrwang" - }, - { - "percent": "10000", - "reputation": 877894048504689, - "rshares": 1461798491875, - "voter": "meesterboom" - }, - { - "percent": "6000", - "reputation": 52176741632512, - "rshares": 242344196175, - "voter": "fiveboringgames" - }, - { - "percent": "3000", - "reputation": 2558692465689, - "rshares": 21558664592, - "voter": "arconite" - }, - { - "percent": "5000", - "reputation": 25362757889840, - "rshares": 5660240113, - "voter": "sazbird" - }, - { - "percent": "5100", - "reputation": 112214838160650, - "rshares": 364065231416, - "voter": "originate" - }, - { - "percent": "6000", - "reputation": 70285620531768, - "rshares": 757554040202, - "voter": "steempress" - }, - { - "percent": "10000", - "reputation": 266498838207861, - "rshares": 1394506891153, - "voter": "timcliff" - }, - { - "percent": "5000", - "reputation": 50032785591993, - "rshares": 551608546845, - "voter": "bryan-imhoff" - }, - { - "percent": "10000", - "reputation": 1911942896577, - "rshares": 8138402548, - "voter": "wisbeech" - }, - { - "percent": "10000", - "reputation": 262691650197556, - "rshares": 348794764726, - "voter": "titusfrost" - }, - { - "percent": "4800", - "reputation": -1181563121002, - "rshares": 944719239, - "voter": "steemyoda" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 45091609891, - "voter": "ssekulji" - }, - { - "percent": "10000", - "reputation": 16169915416749, - "rshares": 10879353417, - "voter": "planosdeunacasa" - }, - { - "percent": "5000", - "reputation": 57123303734647, - "rshares": 1062826729, - "voter": "safar01" - }, - { - "percent": "1500", - "reputation": 35641258249201, - "rshares": 3287007846, - "voter": "oleg326756" - }, - { - "percent": "10000", - "reputation": 4110132379317, - "rshares": 18263824469, - "voter": "sabsel" - }, - { - "percent": "10000", - "reputation": 942473876919, - "rshares": 728145478, - "voter": "mow" - }, - { - "percent": "10000", - "reputation": 30766232797635, - "rshares": 20134889852, - "voter": "cardboard" - }, - { - "percent": "3000", - "reputation": 59094884211730, - "rshares": 4261765922, - "voter": "saleg25" - }, - { - "percent": "5000", - "reputation": 27214240242904, - "rshares": 41745745084, - "voter": "rahul.stan" - }, - { - "percent": "10000", - "reputation": 43129757389335, - "rshares": 8415480705, - "voter": "sudutpandang" - }, - { - "percent": "10000", - "reputation": 1199149180987, - "rshares": 44659758403283, - "voter": "ranchorelaxo" - }, - { - "percent": "3000", - "reputation": 15925403061479, - "rshares": 1770053712, - "voter": "titianus" - }, - { - "percent": "10000", - "reputation": 450338781685383, - "rshares": 117784411939, - "voter": "louisthomas" - }, - { - "percent": "3000", - "reputation": 14059622019382, - "rshares": 14575886623, - "voter": "damla" - }, - { - "percent": "3000", - "reputation": 307916617000098, - "rshares": 372403463854, - "voter": "detlev" - }, - { - "percent": "3000", - "reputation": 15135006110391, - "rshares": 6491192391, - "voter": "thatsweeneyguy" - }, - { - "percent": "1500", - "reputation": 42486683511346, - "rshares": 4837598284, - "voter": "ma1neevent" - }, - { - "percent": "3000", - "reputation": 91224250124, - "rshares": 3903352973, - "voter": "kingkinslow" - }, - { - "percent": "10000", - "reputation": 39412919556388, - "rshares": 95586730071, - "voter": "ribalinux" - }, - { - "percent": "1800", - "reputation": 11448902903972, - "rshares": 905322529989, - "voter": "roomservice" - }, - { - "percent": "10000", - "reputation": 130573901548108, - "rshares": 981935443738, - "voter": "fredrikaa" - }, - { - "percent": "10000", - "reputation": 112994806497327, - "rshares": 3214810820, - "voter": "varunpinto" - }, - { - "percent": "5000", - "reputation": 80123076461352, - "rshares": 373531476828, - "voter": "xels" - }, - { - "percent": "10000", - "reputation": 64794945990086, - "rshares": 14757640473, - "voter": "shikika" - }, - { - "percent": "10000", - "reputation": 9701652715721, - "rshares": 134481372560, - "voter": "masterthematrix" - }, - { - "percent": "5000", - "reputation": 62289248493193, - "rshares": 1658178787, - "voter": "mxzn" - }, - { - "percent": "10000", - "reputation": 7901101123497, - "rshares": 1165342669175, - "voter": "giuatt07" - }, - { - "percent": "10000", - "reputation": 10194751636845, - "rshares": 49902171478, - "voter": "swaraj" - }, - { - "percent": "10000", - "reputation": 20355801093011, - "rshares": 426111160334, - "voter": "jingdol" - }, - { - "percent": "3000", - "reputation": 30158976168359, - "rshares": 6704892102, - "voter": "guchtere" - }, - { - "percent": "10000", - "reputation": 219366237221002, - "rshares": 581676420711, - "voter": "offgridlife" - }, - { - "percent": "3000", - "reputation": 44467529253991, - "rshares": 9101753540, - "voter": "dante31" - }, - { - "percent": "3000", - "reputation": 20285621918859, - "rshares": 9793210738, - "voter": "wandergirl" - }, - { - "percent": "10000", - "reputation": 5313719494876540, - "rshares": 12663101000031, - "voter": "haejin" - }, - { - "percent": "6000", - "reputation": 181080687674137, - "rshares": 2268177614177, - "voter": "howo" - }, - { - "percent": "10000", - "reputation": 37009106416903, - "rshares": 804883370, - "voter": "yann0975" - }, - { - "percent": "10000", - "reputation": 190691885658151, - "rshares": 7020538416695, - "voter": "nathanmars" - }, - { - "percent": "6000", - "reputation": 895691331080490, - "rshares": 6194259140626, - "voter": "ocd" - }, - { - "percent": "4250", - "reputation": 1458333931454, - "rshares": 1205517327, - "voter": "showtime" - }, - { - "percent": "3300", - "reputation": 61249536206183, - "rshares": 38570631638, - "voter": "redrica" - }, - { - "percent": "6000", - "reputation": 44309577530003, - "rshares": 1577875376, - "voter": "hdmed" - }, - { - "percent": "3000", - "reputation": 4261067407032, - "rshares": 2097255897, - "voter": "genesisojeda" - }, - { - "percent": "5360", - "reputation": 24639928209538, - "rshares": 30958489612, - "voter": "santigs" - }, - { - "percent": "10000", - "reputation": 11131064226204, - "rshares": 18365288753, - "voter": "angelusnoctum" - }, - { - "percent": "10000", - "reputation": 51994511985595, - "rshares": 40931757582371, - "voter": "tipu" - }, - { - "percent": "400", - "reputation": 101280142849344, - "rshares": 11236693090, - "voter": "kimzwarch" - }, - { - "percent": "6000", - "reputation": 5754775236968, - "rshares": 2788064509, - "voter": "nurhayati" - }, - { - "percent": "5000", - "reputation": 41069012860239, - "rshares": 10261614594, - "voter": "markjason" - }, - { - "percent": "6000", - "reputation": 50202305045423, - "rshares": 48088645663, - "voter": "mikepm74" - }, - { - "percent": "10000", - "reputation": 43425068714198, - "rshares": 56070061756, - "voter": "chrisroberts" - }, - { - "percent": "10000", - "reputation": 669346799793, - "rshares": 15639380960, - "voter": "voxmonkey" - }, - { - "percent": "8968", - "reputation": 0, - "rshares": 206181194230, - "voter": "tomiscurious" - }, - { - "percent": "10000", - "reputation": 3699571350702, - "rshares": 7794323217, - "voter": "cesinfenianos" - }, - { - "percent": "10000", - "reputation": 45930585883630, - "rshares": 94924952057, - "voter": "carrieallen" - }, - { - "percent": "2400", - "reputation": 445234100950820, - "rshares": 7431502451632, - "voter": "therealwolf" - }, - { - "percent": "3000", - "reputation": 16626980732032, - "rshares": 2732680247, - "voter": "diosarich" - }, - { - "percent": "3000", - "reputation": 20299637563719, - "rshares": 1519106195, - "voter": "critic-on" - }, - { - "percent": "100", - "reputation": 86519807944048, - "rshares": 9402021207, - "voter": "jlsplatts" - }, - { - "percent": "10000", - "reputation": 2589882190131, - "rshares": 823459870, - "voter": "kevinbacon" - }, - { - "percent": "3000", - "reputation": 54554402347359, - "rshares": 10888471258, - "voter": "hanggggbeeee" - }, - { - "percent": "83", - "reputation": 0, - "rshares": 33780523000, - "voter": "investegg" - }, - { - "percent": "10000", - "reputation": 767197612874, - "rshares": 2454240325, - "voter": "morwhale" - }, - { - "percent": "10000", - "reputation": 1436962620884, - "rshares": 168023189, - "voter": "mrsyria" - }, - { - "percent": "6800", - "reputation": 6785724346858, - "rshares": 101287331127, - "voter": "drillith" - }, - { - "percent": "1800", - "reputation": 72060804308794, - "rshares": 476355503788, - "voter": "eonwarped" - }, - { - "percent": "6000", - "reputation": 12722616650811, - "rshares": 2877000394715, - "voter": "postpromoter" - }, - { - "percent": "3000", - "reputation": 5643908417284, - "rshares": 19600092733, - "voter": "mejustandrew" - }, - { - "percent": "1500", - "reputation": 9111881686322, - "rshares": 680547849, - "voter": "steinhammer" - }, - { - "percent": "3000", - "reputation": 579461579559, - "rshares": 52198064660, - "voter": "sankysanket18" - }, - { - "percent": "1400", - "reputation": 31514254451254, - "rshares": 1053738850, - "voter": "lordnigel" - }, - { - "percent": "10000", - "reputation": 1807139565916, - "rshares": 113762333, - "voter": "irynochka" - }, - { - "percent": "10000", - "reputation": 1794885660938, - "rshares": 2604462770, - "voter": "soydiegorojas" - }, - { - "percent": "2400", - "reputation": 157805541487641, - "rshares": 1457168338302, - "voter": "smartsteem" - }, - { - "percent": "10000", - "reputation": 14709760292085, - "rshares": 18481456618, - "voter": "yazp" - }, - { - "percent": "10000", - "reputation": 52628537782133, - "rshares": 139333368519, - "voter": "azwarrangkuti" - }, - { - "percent": "10000", - "reputation": 35969154710476, - "rshares": 42970682432, - "voter": "b00m" - }, - { - "percent": "1200", - "reputation": 66785151966461, - "rshares": 57366150533, - "voter": "itchyfeetdonica" - }, - { - "percent": "5000", - "reputation": 5385705125126, - "rshares": 1171082551, - "voter": "tpkidkai" - }, - { - "percent": "10000", - "reputation": 1788144848047, - "rshares": 3282987454, - "voter": "najat" - }, - { - "percent": "3000", - "reputation": 110889227376345, - "rshares": 2864668340, - "voter": "rpcaceres" - }, - { - "percent": "3000", - "reputation": 75425198894223, - "rshares": 19993507440, - "voter": "straykat" - }, - { - "percent": "3000", - "reputation": 14337364044156, - "rshares": 3821427654, - "voter": "tomatom" - }, - { - "percent": "2500", - "reputation": 2675225025765, - "rshares": 7482231087, - "voter": "fourfourfun" - }, - { - "percent": "10000", - "reputation": 112593705937795, - "rshares": 169079887629, - "voter": "apnigrich" - }, - { - "percent": "10000", - "reputation": 73184833081444, - "rshares": 2881665810955, - "voter": "oliverschmid" - }, - { - "percent": "1200", - "reputation": 87246032649758, - "rshares": 17745794069, - "voter": "gabrielatravels" - }, - { - "percent": "5000", - "reputation": 21720371357335, - "rshares": 23613778014, - "voter": "bartheek" - }, - { - "percent": "2500", - "reputation": 292980730708468, - "rshares": 29292821654, - "voter": "bengy" - }, - { - "percent": "10000", - "reputation": 7833564344722, - "rshares": 1659606648, - "voter": "ahmedsy" - }, - { - "percent": "10000", - "reputation": 467563251193, - "rshares": 635859047, - "voter": "durbisrodriguez" - }, - { - "percent": "5000", - "reputation": 184489319084, - "rshares": 539831850, - "voter": "steemboat-steve" - }, - { - "percent": "8500", - "reputation": 415587305875676, - "rshares": 20655926932697, - "voter": "steemmonsters" - }, - { - "percent": "1500", - "reputation": 37350775715979, - "rshares": 741822554, - "voter": "kanabeatz" - }, - { - "percent": "6000", - "reputation": 170235537022, - "rshares": 826253634, - "voter": "auracraft" - }, - { - "percent": "1900", - "reputation": 8003119161188, - "rshares": 3937677851, - "voter": "brewery" - }, - { - "percent": "10000", - "reputation": 951646894776, - "rshares": 565601638, - "voter": "cfminer" - }, - { - "percent": "10000", - "reputation": 1117386651740, - "rshares": 547917345, - "voter": "betbet" - }, - { - "percent": "5000", - "reputation": 4156063258051, - "rshares": 1910316727, - "voter": "investprosper" - }, - { - "percent": "3000", - "reputation": 2705558121541, - "rshares": 1125733690, - "voter": "debilog" - }, - { - "percent": "2500", - "reputation": 746831400427, - "rshares": 6283230655, - "voter": "jazzhero" - }, - { - "percent": "3000", - "reputation": 47747940709375, - "rshares": 6268008476, - "voter": "gatolector" - }, - { - "percent": "6000", - "reputation": 4705304861944, - "rshares": 830935284732, - "voter": "ocd-witness" - }, - { - "percent": "10000", - "reputation": 2384160913530, - "rshares": 20994830222, - "voter": "blog-beginner" - }, - { - "percent": "5000", - "reputation": 411588240625, - "rshares": 3821196093, - "voter": "happy-soul" - }, - { - "percent": "3000", - "reputation": 2317835263767, - "rshares": 86615701, - "voter": "sizandyola" - }, - { - "percent": "10000", - "reputation": 8405881148978, - "rshares": 12484511835, - "voter": "thebluewin" - }, - { - "percent": "10000", - "reputation": 4895191598205, - "rshares": 4007461431, - "voter": "auminda" - }, - { - "percent": "6000", - "reputation": 16573145540401, - "rshares": 862327058778, - "voter": "takowi" - }, - { - "percent": "5000", - "reputation": 6264286399143, - "rshares": 1384605470540, - "voter": "asgarth" - }, - { - "percent": "8500", - "reputation": 51146712815953, - "rshares": 1653718779, - "voter": "dreamryder007" - }, - { - "percent": "2100", - "reputation": 17385822919900, - "rshares": 10705652950, - "voter": "forester-joe" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 29504242115, - "voter": "futurecurrency" - }, - { - "percent": "9000", - "reputation": 81234063423, - "rshares": 680172454, - "voter": "jasonwaterfalls" - }, - { - "percent": "7000", - "reputation": 168836422039217, - "rshares": 39634473965, - "voter": "fego" - }, - { - "percent": "2125", - "reputation": 2256065348315, - "rshares": 3541198840, - "voter": "frassman" - }, - { - "percent": "3000", - "reputation": 158256810302501, - "rshares": 47952767285, - "voter": "miroslavrc" - }, - { - "percent": "4250", - "reputation": 3213218714574, - "rshares": 159072656726, - "voter": "bscrypto" - }, - { - "percent": "6000", - "reputation": 1069508711711, - "rshares": 2880566451, - "voter": "greendo" - }, - { - "percent": "10000", - "reputation": 1140372911417, - "rshares": 829941807, - "voter": "zainnyferdhoy" - }, - { - "percent": "10000", - "reputation": 25978211905358, - "rshares": 289256442862, - "voter": "manniman" - }, - { - "percent": "10000", - "reputation": 13852827798556, - "rshares": 1065624288490, - "voter": "dera123" - }, - { - "percent": "10000", - "reputation": 4110594694460, - "rshares": 1116978080730, - "voter": "jkramer" - }, - { - "percent": "3000", - "reputation": 24305829848893, - "rshares": 5063835687, - "voter": "racibo" - }, - { - "percent": "10000", - "reputation": 453247934272, - "rshares": 607463637, - "voter": "albus.draco" - }, - { - "percent": "10000", - "reputation": 5238378681910, - "rshares": 30949128899, - "voter": "m2nnari" - }, - { - "percent": "5000", - "reputation": 112109667795, - "rshares": 1570301311, - "voter": "memepress" - }, - { - "percent": "6000", - "reputation": 8477903566406, - "rshares": 1025470081, - "voter": "berien" - }, - { - "percent": "10000", - "reputation": 27390685365705, - "rshares": 23149928794, - "voter": "tsnaks" - }, - { - "percent": "10000", - "reputation": 2481629111221, - "rshares": 2199175851, - "voter": "manojbhatt" - }, - { - "percent": "3000", - "reputation": 77476461100689, - "rshares": 119085220516, - "voter": "indigoocean" - }, - { - "percent": "1200", - "reputation": 42246987760, - "rshares": 998762374, - "voter": "feedmytwi" - }, - { - "percent": "10000", - "reputation": 58352135446544, - "rshares": 96599202577, - "voter": "romeskie" - }, - { - "percent": "6000", - "reputation": 3042286903871, - "rshares": 28954778839, - "voter": "jancharlest" - }, - { - "percent": "10000", - "reputation": 38572708862364, - "rshares": 72403734626, - "voter": "cryptomaniacsgr" - }, - { - "percent": "6000", - "reputation": 28237853787625, - "rshares": 678324767, - "voter": "crystalhuman" - }, - { - "percent": "2200", - "reputation": 51236835710173, - "rshares": 10080659391, - "voter": "davidesimoncini" - }, - { - "percent": "10000", - "reputation": 4253324762944, - "rshares": 821239339, - "voter": "viniciotricolor" - }, - { - "percent": "10000", - "reputation": 8108191904890, - "rshares": 3880380316, - "voter": "nante" - }, - { - "percent": "10000", - "reputation": 3240290091340, - "rshares": 7785150897, - "voter": "stevecronin" - }, - { - "percent": "2500", - "reputation": 27522752785541, - "rshares": 12777270009, - "voter": "longer" - }, - { - "percent": "10000", - "reputation": 11651978003921, - "rshares": 12610267892, - "voter": "jmauring" - }, - { - "percent": "10000", - "reputation": 3499284562289, - "rshares": 3590032807, - "voter": "carita-feliz" - }, - { - "percent": "3000", - "reputation": 3936396886211, - "rshares": 857481782, - "voter": "diegopadilla" - }, - { - "percent": "1600", - "reputation": 37902408766598, - "rshares": 52764194281, - "voter": "cmplxty" - }, - { - "percent": "6000", - "reputation": 3516028097696, - "rshares": 49548498807405, - "voter": "ocdb" - }, - { - "percent": "7000", - "reputation": 21771305129128, - "rshares": 2285918697, - "voter": "mightypanda" - }, - { - "percent": "10000", - "reputation": 148875031036, - "rshares": 411583108, - "voter": "steinz" - }, - { - "percent": "10000", - "reputation": 156376467869107, - "rshares": 336769003023, - "voter": "dalz" - }, - { - "percent": "1500", - "reputation": 24307599069679, - "rshares": 5286157222, - "voter": "dronegraphica" - }, - { - "percent": "2000", - "reputation": 2376783964081, - "rshares": 831008499, - "voter": "arrixion" - }, - { - "percent": "3000", - "reputation": 45111147422257, - "rshares": 6356004033, - "voter": "mobi72" - }, - { - "percent": "10000", - "reputation": 11871368204448, - "rshares": 15104718287, - "voter": "ynwa.andree" - }, - { - "percent": "10000", - "reputation": 10278138043955, - "rshares": 8760730, - "voter": "laissez-faire" - }, - { - "percent": "7795", - "reputation": 0, - "rshares": 83034707209, - "voter": "voter003" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 17807178284, - "voter": "quediceharry" - }, - { - "percent": "5000", - "reputation": 6687049412835, - "rshares": 40114877658, - "voter": "mister-meeseeks" - }, - { - "percent": "3000", - "reputation": 6098497276677, - "rshares": 37253319823, - "voter": "xmauron3" - }, - { - "percent": "10000", - "reputation": 1648086760085, - "rshares": 24799788121, - "voter": "diegor" - }, - { - "percent": "4000", - "reputation": 18273031359521, - "rshares": 1364059211, - "voter": "adyorka" - }, - { - "percent": "3000", - "reputation": 57675290515578, - "rshares": 2458444576, - "voter": "pushpedal" - }, - { - "percent": "10000", - "reputation": 1956837123131, - "rshares": 5073574337, - "voter": "atnep111" - }, - { - "percent": "10000", - "reputation": 45419481582603, - "rshares": 229525569884, - "voter": "michealb" - }, - { - "percent": "2400", - "reputation": 30517153592067, - "rshares": 8301912599, - "voter": "variedades" - }, - { - "percent": "3000", - "reputation": 21657939011190, - "rshares": 2043803703, - "voter": "kork75" - }, - { - "percent": "10000", - "reputation": 311056789115, - "rshares": 3431163097, - "voter": "toycard" - }, - { - "percent": "5100", - "reputation": 72504131225, - "rshares": 3430813200, - "voter": "agent14" - }, - { - "percent": "3000", - "reputation": 706943279500, - "rshares": 666862945, - "voter": "junnel123" - }, - { - "percent": "3000", - "reputation": 15820967488528, - "rshares": 1967868292, - "voter": "mazima" - }, - { - "percent": "10000", - "reputation": 75911580, - "rshares": 167222362228, - "voter": "jamesbattler" - }, - { - "percent": "5000", - "reputation": 22639587796, - "rshares": 42752545402, - "voter": "eternalsuccess" - }, - { - "percent": "10000", - "reputation": 9483360545909, - "rshares": 12853958175, - "voter": "mia-cc" - }, - { - "percent": "10000", - "reputation": 25016264134313, - "rshares": 796719662454, - "voter": "dcommerce" - }, - { - "percent": "10000", - "reputation": 193706512612, - "rshares": 1314467790, - "voter": "stevenmalik" - }, - { - "percent": "2000", - "reputation": 22403588555771, - "rshares": 43447140518, - "voter": "thelogicaldude" - }, - { - "percent": "8500", - "reputation": 1443660475563, - "rshares": 15942903312, - "voter": "monster-mountain" - }, - { - "percent": "2000", - "reputation": 27838835653920, - "rshares": 5665102200, - "voter": "kggymlife" - }, - { - "percent": "10000", - "reputation": 925135168509, - "rshares": 486204220, - "voter": "marymi" - }, - { - "percent": "10000", - "reputation": 69910542366759, - "rshares": 501897567941, - "voter": "kryptogames" - }, - { - "percent": "3000", - "reputation": 6605568359560, - "rshares": 719808554, - "voter": "smalltall" - }, - { - "percent": "3000", - "reputation": 12351124481881, - "rshares": 6311523139, - "voter": "squareonefarms" - }, - { - "percent": "720", - "reputation": 120936131908085, - "rshares": 54555354364, - "voter": "epicdice" - }, - { - "percent": "5000", - "reputation": 459526835755, - "rshares": 901994133, - "voter": "bigmoneyman" - }, - { - "percent": "3000", - "reputation": 116854383655456, - "rshares": 7537152363, - "voter": "yiobri" - }, - { - "percent": "6000", - "reputation": 61476958391321, - "rshares": 2963432310, - "voter": "ricardomello" - }, - { - "percent": "10000", - "reputation": 29888557634290, - "rshares": 21927379241, - "voter": "helgalubevi" - }, - { - "percent": "5000", - "reputation": 405927252791, - "rshares": 528008059, - "voter": "iamangierose" - }, - { - "percent": "8500", - "reputation": 7323299875964, - "rshares": 737255392, - "voter": "borodas" - }, - { - "percent": "3000", - "reputation": 2597264781531, - "rshares": 4203942321, - "voter": "akumagai" - }, - { - "percent": "3000", - "reputation": 19219822868653, - "rshares": 32876130385, - "voter": "beerlover" - }, - { - "percent": "170", - "reputation": 36857316180998, - "rshares": 1033189966, - "voter": "blumela" - }, - { - "percent": "300", - "reputation": 20916803594387, - "rshares": 1367496028, - "voter": "leighscotford" - }, - { - "percent": "10000", - "reputation": 11624344336093, - "rshares": 334875869773, - "voter": "recording-box" - }, - { - "percent": "10000", - "reputation": 62906992432, - "rshares": 38512166, - "voter": "nathaliag0" - }, - { - "percent": "10000", - "reputation": 37703239366, - "rshares": 0, - "voter": "curthodler" - }, - { - "percent": "10000", - "reputation": 17647336098195, - "rshares": 38522803168, - "voter": "c21c" - }, - { - "percent": "10000", - "reputation": 969105063971, - "rshares": 1674075756, - "voter": "elclements" - }, - { - "percent": "1600", - "reputation": 9742808963304, - "rshares": 3535248116, - "voter": "ssiena" - }, - { - "percent": "4250", - "reputation": 356055297693, - "rshares": 1002657397, - "voter": "everythingsmgirl" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 19799579531, - "voter": "good.game" - }, - { - "percent": "7650", - "reputation": 13727208041496, - "rshares": 2702465501, - "voter": "ejmh" - }, - { - "percent": "3000", - "reputation": 1385472095541, - "rshares": 618521338, - "voter": "athomewithcraig" - }, - { - "percent": "5000", - "reputation": 5514422300, - "rshares": 680160980, - "voter": "rusbe" - }, - { - "percent": "10000", - "reputation": 1146189014563, - "rshares": 1160888826, - "voter": "urun" - }, - { - "percent": "8500", - "reputation": 5118740660861, - "rshares": 14172022983, - "voter": "joshmansters" - }, - { - "percent": "25", - "reputation": 0, - "rshares": 1173505985, - "voter": "urtrailer" - }, - { - "percent": "3000", - "reputation": 62988003579804, - "rshares": 10623929523, - "voter": "zeesh" - }, - { - "percent": "4250", - "reputation": 0, - "rshares": 637977195, - "voter": "pietpompies" - }, - { - "percent": "1200", - "reputation": 3257555962879, - "rshares": 609876487, - "voter": "bilpcoinrecords" - }, - { - "percent": "6000", - "reputation": 257075039692, - "rshares": 5434899242, - "voter": "efathenub" - }, - { - "percent": "10000", - "reputation": 59827171041931, - "rshares": 43982059, - "voter": "fearlessgu" - }, - { - "percent": "5000", - "reputation": 21757713000, - "rshares": 0, - "voter": "vinicio.spt" - }, - { - "percent": "4250", - "reputation": 356840875823, - "rshares": 1485628665, - "voter": "kgsupport" - }, - { - "percent": "2000", - "reputation": 14577043701834, - "rshares": 2470246887, - "voter": "julesquirin" - }, - { - "percent": "3000", - "reputation": 13104433773695, - "rshares": 2918115166, - "voter": "mengene" - }, - { - "percent": "3000", - "reputation": 4006970074087, - "rshares": 1042409111, - "voter": "goldstreet" - }, - { - "percent": "1200", - "reputation": 9568816243033, - "rshares": 11581319133, - "voter": "dpend.active" - }, - { - "percent": "10000", - "reputation": 17892806983898, - "rshares": 22877261350, - "voter": "splinterlore" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 213752405876, - "voter": "hivephilippines" - }, - { - "percent": "4770", - "reputation": 1209731647511, - "rshares": 790298213270, - "voter": "softworld" - }, - { - "percent": "10000", - "reputation": 388630945885, - "rshares": 10552185628, - "voter": "jwynqc" - }, - { - "percent": "10000", - "reputation": 631765969428, - "rshares": 30756503339, - "voter": "hiveshout" - }, - { - "percent": "6000", - "reputation": 17298355816851, - "rshares": 73433939433, - "voter": "the100" - }, - { - "percent": "1800", - "reputation": 47896334821902, - "rshares": 25233008966, - "voter": "hiveonboard" - }, - { - "percent": "1000", - "reputation": 3177222978373, - "rshares": 9169282668, - "voter": "hivelist" - }, - { - "percent": "5000", - "reputation": 604442758770, - "rshares": 223879691857, - "voter": "ninnu" - }, - { - "percent": "3000", - "reputation": 7034683328715, - "rshares": 2494741879, - "voter": "iameden" - }, - { - "percent": "10000", - "reputation": 71740148, - "rshares": 2286633832, - "voter": "tschew" - }, - { - "percent": "10000", - "reputation": 429763208250, - "rshares": 4369567738, - "voter": "ghaazi" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "zarevaneli" - }, - { - "percent": "5000", - "reputation": -588508122267, - "rshares": 45111378395, - "voter": "poshbot" - }, - { - "percent": "3000", - "reputation": 5470715229938, - "rshares": 1121616908, - "voter": "jsalvage" - }, - { - "percent": "10000", - "reputation": 142457281, - "rshares": 736419980, - "voter": "p3ntar0u" - }, - { - "percent": "10000", - "reputation": 65519590598, - "rshares": 1926712585, - "voter": "plusvault" - }, - { - "percent": "3000", - "reputation": 23814154211011, - "rshares": 1269725373, - "voter": "syberia" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 318105117, - "voter": "splinterlandspro" - }, - { - "percent": "3000", - "reputation": 10486305203609, - "rshares": 2415403782, - "voter": "tasri" - }, - { - "percent": "10000", - "reputation": 6289759921042, - "rshares": 13120716824, - "voter": "mercurial9" - }, - { - "percent": "10000", - "reputation": 2830639099232, - "rshares": 430487716, - "voter": "koxmicart" - }, - { - "percent": "5000", - "reputation": 10164951682919, - "rshares": 3563667826, - "voter": "bdkabbo" - }, - { - "percent": "3000", - "reputation": 11758355842388, - "rshares": 3432255956, - "voter": "libbi" - }, - { - "percent": "3000", - "reputation": 3274158340408, - "rshares": 6294940731, - "voter": "spirall" - }, - { - "percent": "10000", - "reputation": 3156702940170, - "rshares": 1913793534, - "voter": "mmdacanay" - }, - { - "percent": "3000", - "reputation": 5921144534369, - "rshares": 1727755310, - "voter": "zoeanavid" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 16143958915, - "voter": "vtol79" - }, - { - "percent": "3000", - "reputation": 7330275018374, - "rshares": 1769631763, - "voter": "tinta-tertuang" - }, - { - "percent": "10000", - "reputation": 199217586040, - "rshares": 6940785085, - "voter": "sirdemian" - }, - { - "percent": "1000", - "reputation": 0, - "rshares": 1491122882796, - "voter": "usainvote" - }, - { - "percent": "5000", - "reputation": 9930293019864, - "rshares": 3929251026, - "voter": "jonalyn2020" - }, - { - "percent": "3000", - "reputation": 1447273576100, - "rshares": 1717697327, - "voter": "bitcome" - }, - { - "percent": "1450", - "reputation": 16307549034965, - "rshares": 2769260421, - "voter": "trangbaby" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "victorjopaca" - }, - { - "percent": "6000", - "reputation": 1668767539307, - "rshares": 860291004, - "voter": "gonklavez9" - }, - { - "percent": "3000", - "reputation": 2192133308841, - "rshares": 601513283, - "voter": "mau189gg" - }, - { - "percent": "6000", - "reputation": 3038383065569, - "rshares": 37035324372, - "voter": "crypto-gamer" - }, - { - "percent": "3000", - "reputation": 86405531104, - "rshares": 529157141, - "voter": "text2speech" - }, - { - "percent": "10000", - "reputation": 17081211030, - "rshares": 507679447, - "voter": "stormigirl" - }, - { - "percent": "10000", - "reputation": 112363590278, - "rshares": 41785721, - "voter": "dango1411" - }, - { - "percent": "10000", - "reputation": 681362515038, - "rshares": 1827804091, - "voter": "m2fermin" - }, - { - "percent": "3000", - "reputation": 2629706127841, - "rshares": 729458338, - "voter": "kattycrochet" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 19603350308, - "voter": "shroomfund" - }, - { - "percent": "10000", - "reputation": 876243100, - "rshares": 0, - "voter": "dheagle" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 3793462735, - "voter": "rafelluz" - }, - { - "percent": "10000", - "reputation": 531969022, - "rshares": 674365740, - "voter": "psicologiaexpres" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "mlehntorh" - } - ], - "author": "synergyofserra", - "author_reputation": 2179492699548, - "beneficiaries": [ - { - "account": "hiveonboard", - "weight": 100 - }, - { - "account": "theycallmedan", - "weight": 100 - }, - { - "account": "urun", - "weight": 300 - } - ], - "body": "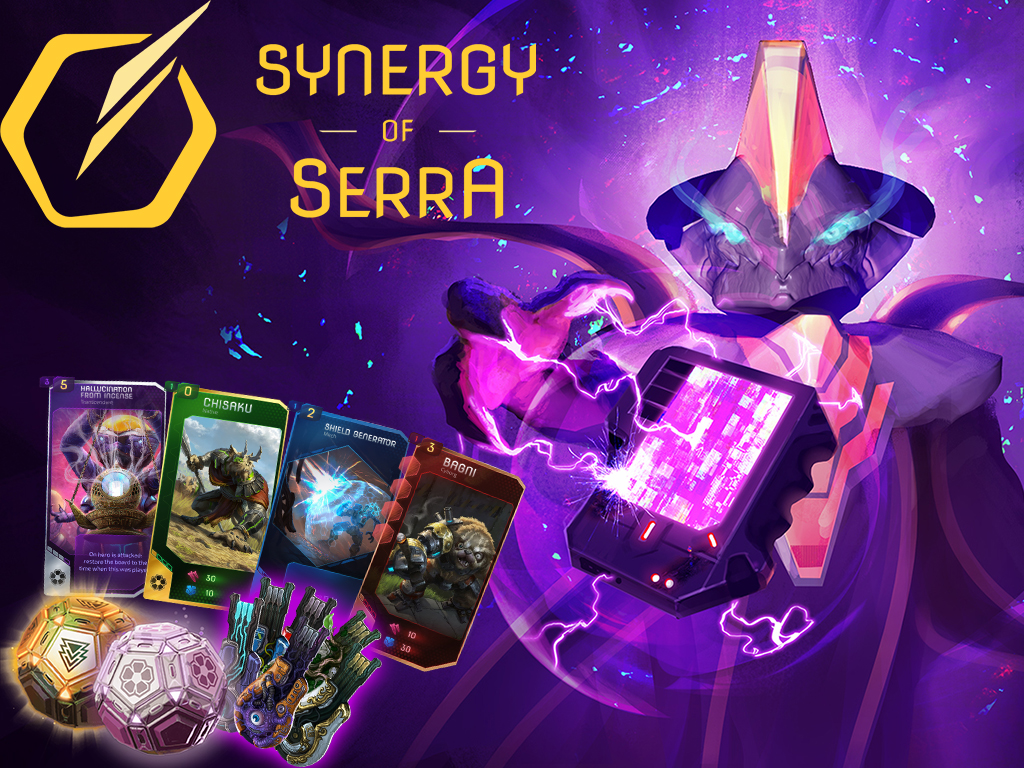\n\n[Synergy of Serra](https://synergyofserra.com/?utm_source=peakd&utm_medium=article) shapes a new game genre, merging classic Strategy Card Games with the infinite game experience possibilities of Deck-building Card Games. Join the six factions of biological and mechanical creatures in the defense of the planet Serra from an alien threat. Become the leading commander of Serra! It's a free-to-play game without annoying pay walls.\n\n## The World of\u00a0Serra\nIn a distant solar system caught between two stars, there is a planet overflowing with life and a prospering society. The society overcame their differences and created the perfect economy overseen by a just and rational council. This planet is called Serra.\n\nThe planet is inhabited by an advanced and diverse society that found ways to sustain the planet's ecosystem. This society is divided into six factions, that live together in harmony. At least most of the time.\nThis peace was one day disturbed by findings during one of the deep space explorations to chart the unknown galaxy around Serra. The findings indicated a distant threat, devouring one planet system after another. Simulations demonstrated that sooner or later Serra would be one of the planets falling prey to this ancient threat.\n\nRead more about the [World of Serra](https://medium.com/calystral/the-world-of-serra-afb06034619).\n\n## Deck-building\nInspired by Deck-building games like [Dominion](https://en.wikipedia.org/wiki/Dominion_(card_game)), Synergy of Serra uses construction of a deck as the main element of gameplay. It is similar to collectible card games (CCGs) in that each player has their own deck. However, unlike CCGs, the majority of the deck is built during the game, instead of before the game. Typically, the cards provide a type of game currency that allows players to buy more cards to add to their decks. We wrote a [full article about Constructed, Roguelike and Deckbuilder Card Games](https://medium.com/calystral/constructed-roguelike-and-deckbuilder-card-games-they-are-not-the-same-47ea8ecd62bb).\n\n## Gameplay\nDisclaimer: The game is in a pre-alpha stage. At this stage, it is hard to find the sweet spot between publishing information about the gameplay and still be open-minded / flexible about strategy drawbacks that may occur while onboarding more players into the game. Meaning, all game mechanics are still subject for change.\n\n#### Game Flow\nBefore a game starts, players are able to create a **Suitcase** full of 100 cards. When an opponent is matched, both suitcases are poured onto the table creating the **Card Pool**. Each player starts the game with only 10 cards (Starter Deck), taking 5 cards to their hand each turn. Whenever a turn is finished, the remaining hand cards are discarded and 5 new cards are drawn. So after 2 turns, the deck is empty. Thus, the **Discard Pile** is shuffled and becomes the **Draw Pile** again. The deck is played over and over again.\n\nTo create a stronger and deeply strategic deck, each turn a player is able to buy new cards from the **River** putting them into their **Discard Pile**. The **River** shows 5~6 semi-random cards (an algorithm decides only for cards that you are able to buy) from the **Card Pool** and offers them to the player.\n\nThere are two resource systems in Synergy of Serra, mana points to play cards from your hand into the battlefield. And there are gold coins to buy cards from the river. Each turn the mana points and gold coins are increased/replenished.\n\nThe goal of the game is to reduce your opponent's hero to 0 life points.\n\n#### Card Types\nThere are three types of cards: Power, Attachment, and unit cards:\n\n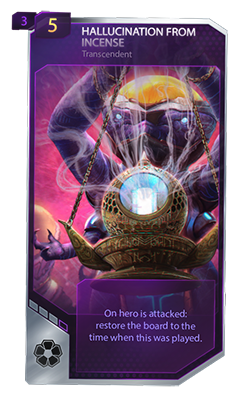\n\nPower cards like Hallucination from Incense can be thought of as a spell card. However, we are living in a sci-fi world without magic.\u00a0\ud83d\ude09\nThis card's power for example is time traveling, enabling complex strategies for sure. Further, power cards are used to heal, damage or buff units.\n\n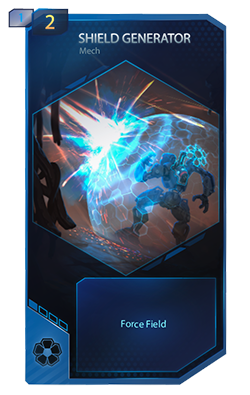\n\nAttachment cards can be attached to slots on unit cards. The key word will become the unit's effect. E.g. Shield generator provides a unit permanently with \"Force Field\", which absorbs full damage once. The color of the attachment and the color of a used slot decides how many faction points are awarded. (More on faction points and boni in another article.)\n\n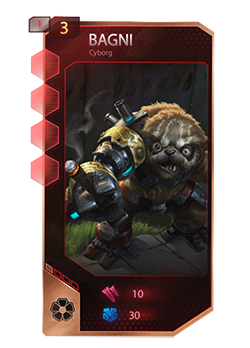\n\nUnit cards are.. well.. they are units to fight your opponent! They have attack/defense similar to other CCG games and when their defense drops to 0 the unit goes into the Discard Pile. Each unit has a specific number of slots that can be filled with attachments to enhance the unit. An attachment can be replaced, moving the previous attachment to the discard pile.\n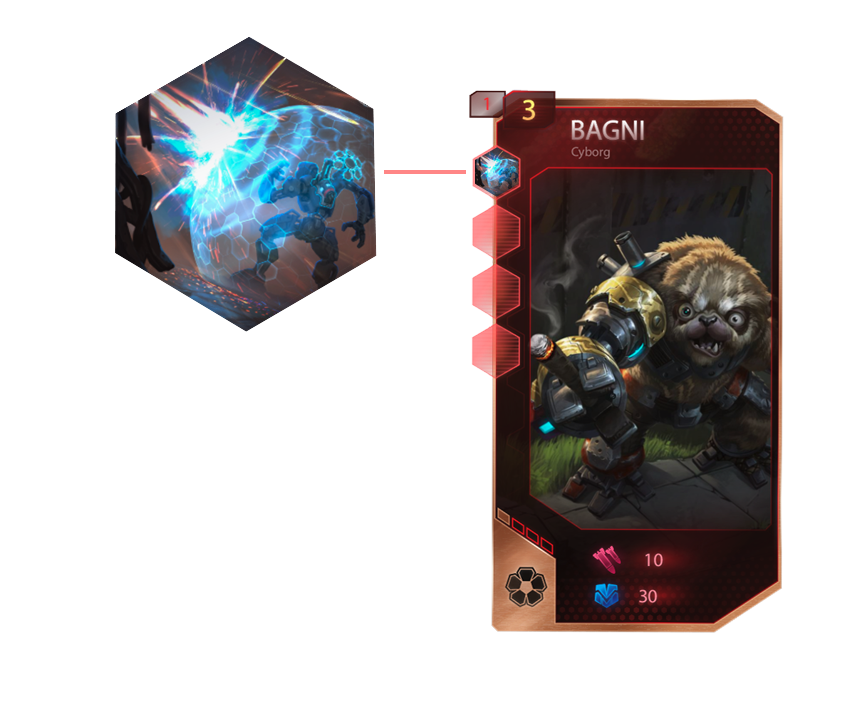\n\n\nWhen a unit with an attachment goes to the discard pile, the attachment goes with the unit and is still there when played to the battlefield the next time.\n\n#### Ressource System\n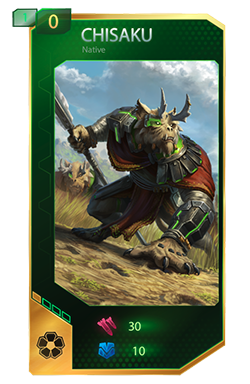\n\n\nEach card shows multiple numbers. The \"mana costs\" (green) used to play a card into the battle field. The \"gold costs\" (gold)to actually buy the card from the river into your deck. The attack (red rockets) and the defense (blue shield).\n\n\n---\n\n## Collectible Cards\n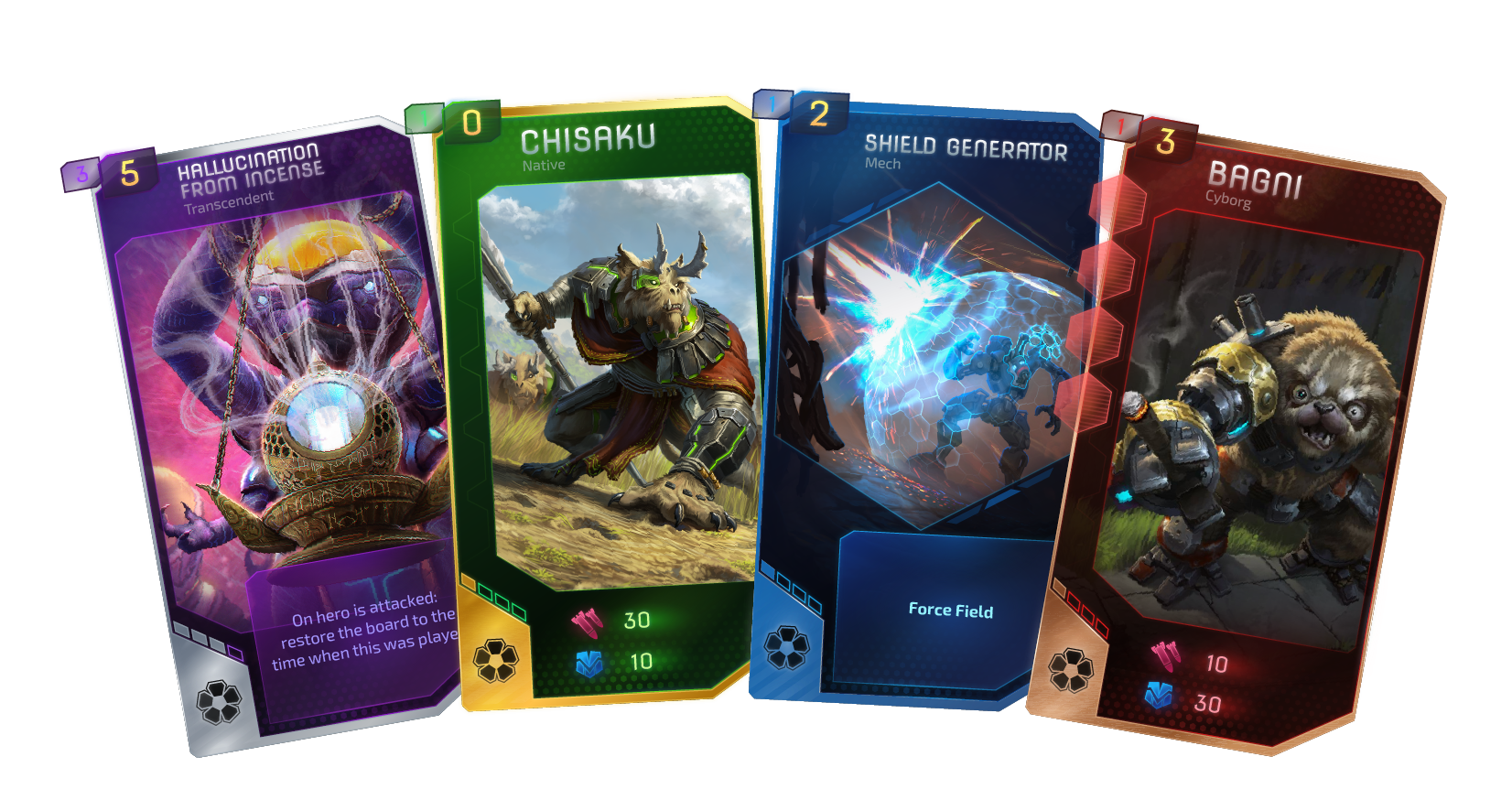\n\n#### Card Sets\nAt the moment, there are 159 different cards available in Synergy of Serra, divided into 4 different Sets: Promo Set, Starter Set, Base Set, and Transcendent Set. Cards **without** Edition Symbol are free cards that can't be traded and thus are **no NFTs**!\n\n**Starter Set [Free cards]**\nThese are 6 different cards, which are your start deck. A seed from which you will build your deck during the game (deck-building).\n\n**Base Set [Free cards]**\nThe Base Set contains 90 different cards, which every player gets for free. With these you are ready to play. All Base Set cards are of Common Rarity.\n\n**Promo Set [Early Adopters; Promotions]**\n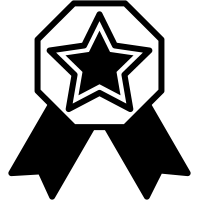\nOne of three limited Tech-Pioneer cards is rewarded to each early adopter of Synergy of Serra by signing up before the alpha starts. [NFT]\n\n**Base Set [Cosmetics]**\n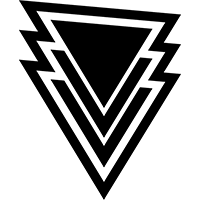\nAll free Base Set cards can be received through crates or trading as a higher Quality (see below) cosmetic version. [NFT]\n\n**Transcendent Set [First expansion]**\n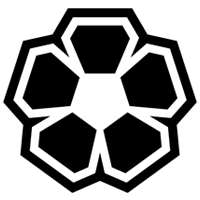\nTranscendent SymbolThe Transcendent Set contains 60 distinct Expansion Cards, including the new Transcendent faction, in different Qualities and Rarities. [NFT]\n\n#### Card Rarity\n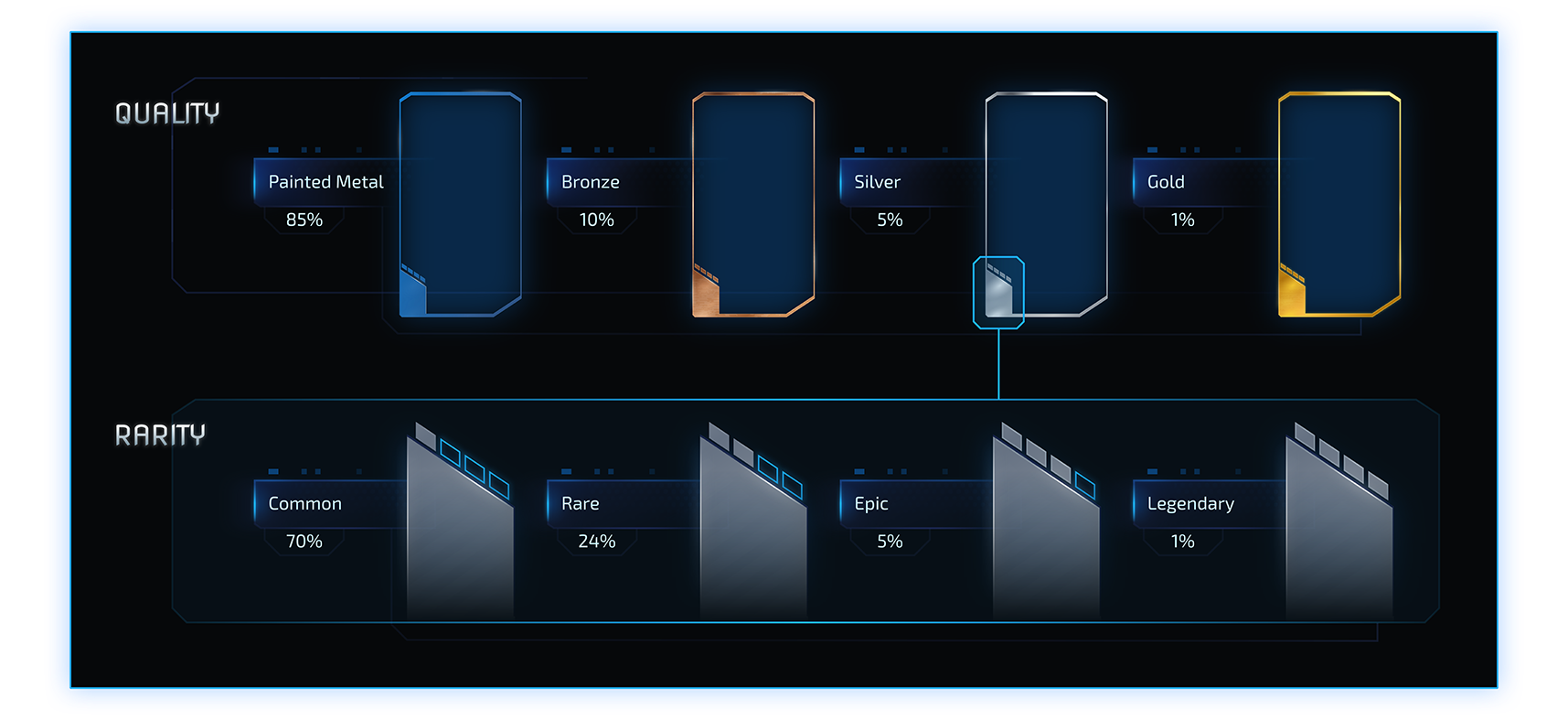\n*Scarcity = Quality X\u00a0Rarity*\n\nScarcity has two dimensions on Serra. There is the Rarity dimension (Common 70%, Rare 24%, Epic 5%, Legendary 1%) and the Quality dimension (Painted Metal 84%, Bronze 10%, Silver 5%, Gold 1%). Multiplying both values gives you the overall scarcity of a card (chance to receive from a crate / $$$ value).\n\n\n---\n\n## Crates\n#### Transcendent Set\u00a0Crates\n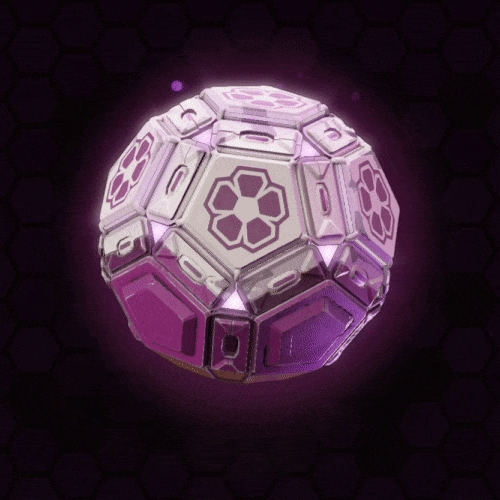\n\nThe Transcendent Set contains 60 distinct Expansion Cards, including the new Transcendent faction, in different Qualities and Rarities. Each Transcendent Set Crate starts from $2 and contains 6 cards with different drop chances, scarcity in two dimensions. There is the Rarity dimension (Common 70%, Rare 24%, Epic 5%, Legendary 1%) and the Quality dimension (Painted Metal 84%, Bronze 10%, Silver 5%, Gold 1%). The most scarce card drawn from this crate is a golden Legendary (0.01% Chance)!\n\n#### Base Set\u00a0Crates\n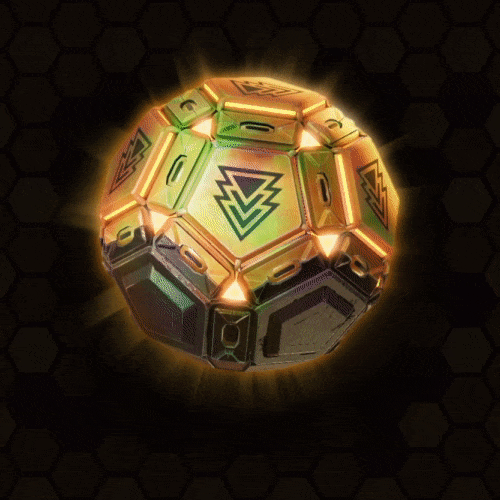\n\nThe Base Set contains the 90 free Base Set Cards in higher Qualities. Each Base Set Crate starts from $1 and contains 6 cards with different drop chances regarding Quality (Bronze 75%, Silver 24%, Gold 1%). Since each player gets all 90 cards from the Base Set for free, there is no Rarity\u200a-\u200aall Base Set cards are valued as Common. Therefore, cards from Base Set Crates are higher quality, cosmetic versions of Base Set cards. These cosmetic cards are also tradable!\n\n#### Crate Opening\u00a0Event\n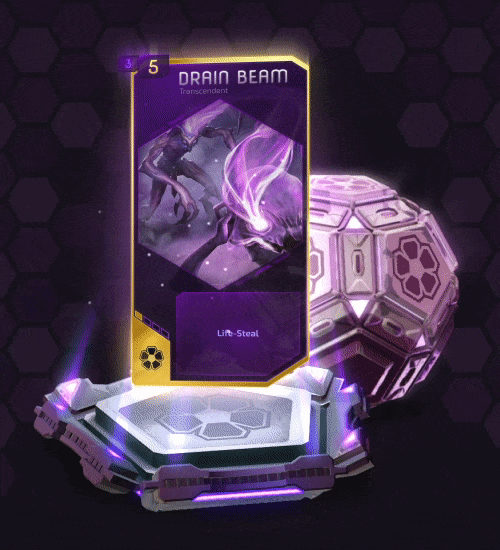\n\nSingle card from a Crate opening\u00a0exampleCrates can be traded from day one but remain locked until the Crate Opening Event because of our great demand for perfection. We wish to finish all card illustrations before unlocking the crates.\n\n\n---\n\n## Blockchain\nThe goal for Synergy of Serra is to utilize blockchain technology in a way which enables specific features like true ownership and play-to-earn. It should be a bonus to gamers and not a barrier for playing! In Synergy of Serra players don't even need to know that we are actually using blockchain in the background. There is no need for wallets, plugins, paying transaction fees or owning cryptocurrencies in the first place. All this is possible since we are hiding the technology in the background and use [Matic Network](https://medium.com/matic-network/what-is-matic-network-466a2c493ae1) as our underlying blockchain infrastructure.\n\n#### Fungible Tokens (FTs) & Non-Fungible Tokens\u00a0(NFTs)\nAn NFT is essentially a unique representation of an asset or good in the form of a virtual token. NFTs are unique and cannot be replaced by another item. Users currently \"hold\" objects that have real value in the game but remain virtual on the company's servers. If the company closes its doors, the objects \"disappear\". With NFTs, objects will continue to exist on the Ethereum blockchain long after the issuing company is gone.\nAll cards received from crates are Non-Fungible Tokens (ERC1155 NFTs) while crates are Fungible Tokens itself. Meaning all creates can be traded and sold from day one on. The [smart contract](https://explorer.matic.network/address/0xddEaD819Fa3e2bEA0C2E33Ab71204240ebc0739e/contracts) for all Synergy of Serra Assets is public and verified for anybody to check.\n\n#### Matic Network\n[Matic](https://matic.network/) enables scalable and instant blockchain transactions. It brings massive scale to Ethereum using an adapted version of Plasma with PoS based side chains.\n\nWe chose Matic Network since it is very accessible for developers and users at the same time. It's very similar to Ethereum, building on the EVM developed through Solidity. Also Ethereum addresses can be used as Matic addresses, no need to recreate existing accounts / wallets.\nMost gamers playing blockchain games are thrilled by the [new possibilities](https://medium.com/calystral/calystral-blockchain-gaming-1df6cd8baada) while at the same time not getting used to the slow and expensive experience on Ethereum. Matic is a solution to fix these issues. Because of the fast block time (1\u20132secs) and the low transactions fees. We as developers are able to hide the blockchain technology in the background and make use of meta transactions. So users don't have to pay fees but still have full control over their assets.\n\n## Economy\n#### Pricing\n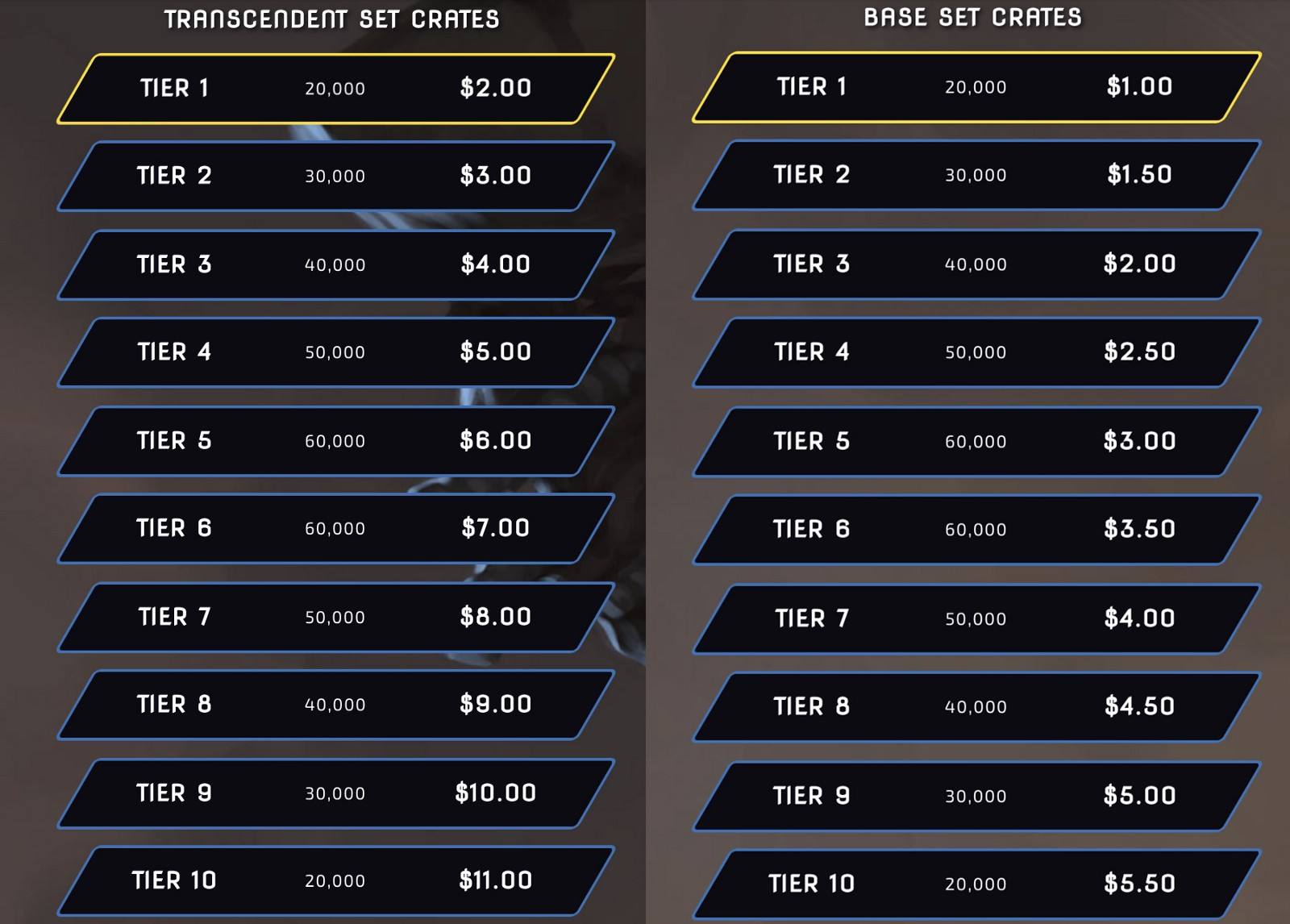\n\nCrate prices are escalating depending on how many crates of each type are sold already, supporting our early adopters! There won't be bundles or any other kind of price reductions. The pricing structure is transparent from the beginning and anybody is able to enter when ever they feel comfortable. The project is still in development, thus the pricing starts very low for [early believers](https://www.youtube.com/watch?v=0mYBSayCsH0).\n\n#### Scrapping\n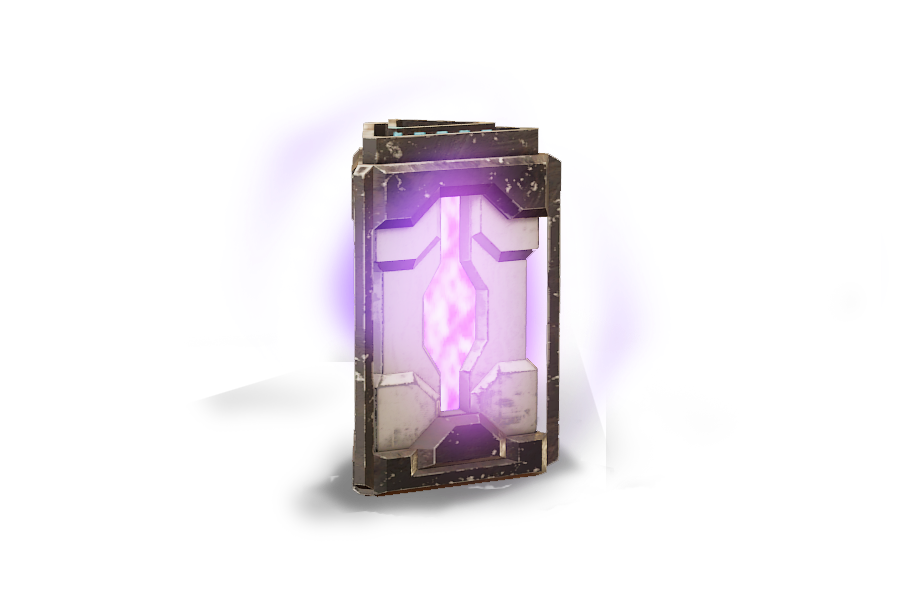\n*In-Game currency SCRAP*\n\nTo ensure no \"useless\" cards are lying around.. players can turn these cards into SCRAP and use it to buy new crates. Depending on the buy price, crates have a payback value of up to ~80% in SCRAP!\n\nAs part of the economy, scrapping will lead to less cards in circulating making the remaining cards more valuable over time. At the same time it creates a theoretical bottom limit for each card's value since people would buy them to scrape and buy crates (conversion rate).\n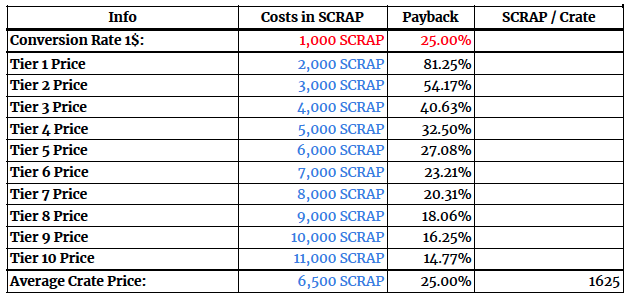\n*Average 25% Payback on\u00a0crates.*\n\nDisclaimer: Scrapping is a future feature and subject to change!\n\n#### Seasonal Ladders (Play-To-Earn)\nA specific amount (10~25%) of all revenue is put into a prize pool. That includes buys from crates but also a fee on trades. All players participating in ranked ladder games are able to fight for their share of the prize pool each season (1~4months). In contrast to other game tournaments, not only 1\u20138 people are earning rewards but hundreds or thousands of players. The payout is weighted depending on the rank reached on the ladder.\n\n#### Minting Device Keys & Artifacts\n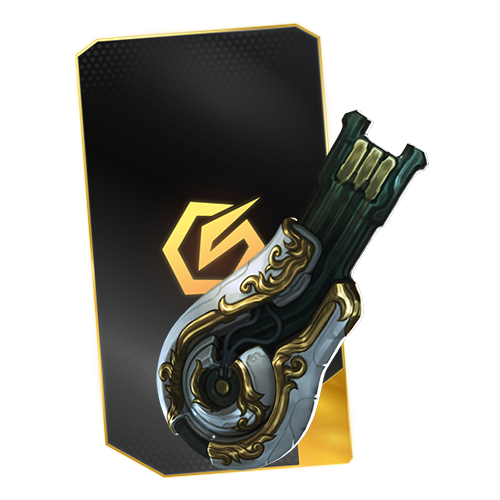\n\nCrates also contain super rare NFTs. There are 6 unique Minting Device Keys and 12 Artifacts cards hidden in crates. Each artifact is a tradable, rare card (NFT) found in Transcendent Set Crates. The card's forgotten power is revealed on discovery.\n\nEach Minting Device Key is a tradable, rare object (NFT) found in Base Set Crates. Each unique key grants the power to refine any copy of a specific card into its highest Quality. Only the owner decides the price for this service. Each key is linked to a specific card from the starter set and can modify the Quality of all existing copies of that card.\n\nThere is no other way to get a Starter Set card in golden Quality except through these keys. Cards from the Starter Set are no NFTs, so you are not able to sell them (or the golden version of it).\n\n\n---\n\n## Who are\u00a0we?\nWe are [Calystral](https://calystral.com/), a team of game developers that set out to unleash the potential of gamers. We are focusing on building games where the time and effort put in, is rewarded with real value. Enhancing the players' experience and empowering gamers to achieve more. In the process, we strive to overcome the technical limitations of Blockchain Technology and use its benefits to lift games to the next level. We would love to share these solutions with the community and other developers. Towards a better future of Gaming!\n________________________________________\n**Join our journey**:\n[Website](https://synergyofserra.com/?utm_source=peakd&utm_medium=article) [Discord](https://discord.gg/SYZK9gN) [Twitter](https://twitter.com/synergyofserra)", - "body_length": 15216, - "cashout_time": "2020-11-05T14:24:36", - "category": "tcg", - "children": 18, - "created": "2020-10-29T14:24:36", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"app\":\"peakd/2020.10.9\",\"format\":\"markdown\",\"description\":\"A new game genre, merging Strategy Card Games with infinite game experience possibilities of Deck-building Card Games\",\"tags\":[\"tcg\",\"blockchain\",\"game\",\"strategy\",\"playtoearn\"],\"links\":[\"https://synergyofserra.com/?utm_source=peakd&utm_medium=article\",\"https://medium.com/calystral/the-world-of-serra-afb06034619\",\"https://en.wikipedia.org/wiki/Dominion_(card_game)\",\"https://medium.com/calystral/constructed-roguelike-and-deckbuilder-card-games-they-are-not-the-same-47ea8ecd62bb\",\"https://medium.com/matic-network/what-is-matic-network-466a2c493ae1\",\"https://explorer.matic.network/address/0xddEaD819Fa3e2bEA0C2E33Ab71204240ebc0739e/contracts\",\"https://matic.network/\",\"https://medium.com/calystral/calystral-blockchain-gaming-1df6cd8baada\",\"https://www.youtube.com/watch?v=0mYBSayCsH0\",\"https://calystral.com/\"],\"image\":[\"https://files.peakd.com/file/peakd-hive/synergyofserra/Rw1JiYK8-SoS_Banner.jpg\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/LBjpD1R2-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/n3M3HNTT-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/uttnWn7p-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/nYqne0r6-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/CQgRrViL-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/G99SXo4a-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/zOUgrsPD-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/gZcR8Br5-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/CpOj86uD-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/x97ykunN-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/Im3LNIKj-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/fEQRNEG1-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/QaRC4tr3-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/z1zfNjb6-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/WQ7N8zmM-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/EF5wXqHe-image.png\",\"https://files.peakd.com/file/peakd-hive/synergyofserra/UqJY7YsS-image.png\"]}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-10-29T14:24:36", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 239844405483718, - "parent_author": "", - "parent_permlink": "tcg", - "pending_payout_value": "56.526 HBD", - "percent_hbd": 10000, - "permlink": "synergy-of-serra-the-free-to-play-deck-building-trading-card-game", - "post_id": 100304117, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Synergy of Serra: The Free-to-Play Deck-building Trading Card Game", - "title": "Synergy of Serra: The Free-to-Play Deck-building Trading Card Game", - "total_payout_value": "0.000 HBD", - "url": "/tcg/@synergyofserra/synergy-of-serra-the-free-to-play-deck-building-trading-card-game" - }, - { - "active_votes": [ - { - "percent": "3000", - "reputation": 520861791056580, - "rshares": 49769141565955, - "voter": "blocktrades" - }, - { - "percent": "75", - "reputation": 19245698180508, - "rshares": 16189196673, - "voter": "tombstone" - }, - { - "percent": "10000", - "reputation": 1594304819447449, - "rshares": 10862888986821, - "voter": "acidyo" - }, - { - "percent": "10000", - "reputation": 506372806974428, - "rshares": 8306211778993, - "voter": "kevinwong" - }, - { - "percent": "73", - "reputation": 109424005488868, - "rshares": 4075700724, - "voter": "mammasitta" - }, - { - "percent": "737", - "reputation": 591298160171898, - "rshares": 112073202735, - "voter": "good-karma" - }, - { - "percent": "750", - "reputation": 352970428645376, - "rshares": 501715566803, - "voter": "roelandp" - }, - { - "percent": "5000", - "reputation": 86171006185191, - "rshares": 19728069749, - "voter": "mrwang" - }, - { - "percent": "10000", - "reputation": 1139583710641, - "rshares": 53410707088, - "voter": "ardina" - }, - { - "percent": "300", - "reputation": 315496990256842, - "rshares": 169322507805, - "voter": "arcange" - }, - { - "percent": "10000", - "reputation": 978188457801574, - "rshares": 3259723577804, - "voter": "deanliu" - }, - { - "percent": "5000", - "reputation": 2558692465689, - "rshares": 35842937067, - "voter": "arconite" - }, - { - "percent": "300", - "reputation": 501315151365, - "rshares": 3188183795, - "voter": "raphaelle" - }, - { - "percent": "600", - "reputation": 560943007638090, - "rshares": 218389412144, - "voter": "ace108" - }, - { - "percent": "10000", - "reputation": 266498838207861, - "rshares": 1430482023741, - "voter": "timcliff" - }, - { - "percent": "10000", - "reputation": 65439199468202, - "rshares": 142074110482, - "voter": "crosheille" - }, - { - "percent": "10000", - "reputation": 143768203235023, - "rshares": 17535850217, - "voter": "shanghaipreneur" - }, - { - "percent": "750", - "reputation": 736102194542370, - "rshares": 311980880665, - "voter": "daveks" - }, - { - "percent": "10000", - "reputation": 262691650197556, - "rshares": 42101103097, - "voter": "titusfrost" - }, - { - "percent": "1500", - "reputation": 144108882515293, - "rshares": 121756045584, - "voter": "petrvl" - }, - { - "percent": "2000", - "reputation": 706874908331117, - "rshares": 528407222712, - "voter": "abh12345" - }, - { - "percent": "10000", - "reputation": 26672580951186, - "rshares": 32025742429, - "voter": "fukako" - }, - { - "percent": "1250", - "reputation": 149827038166480, - "rshares": 50006771441, - "voter": "clayboyn" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 44070722299, - "voter": "ssekulji" - }, - { - "percent": "10000", - "reputation": 34131657938027, - "rshares": 278525510845, - "voter": "dylanhobalart" - }, - { - "percent": "10000", - "reputation": 9057975527982, - "rshares": 69988542616, - "voter": "barcisz" - }, - { - "percent": "10000", - "reputation": 4110132379317, - "rshares": 17333167526, - "voter": "sabsel" - }, - { - "percent": "764", - "reputation": 420443679514793, - "rshares": 131749453146, - "voter": "esteemapp" - }, - { - "percent": "1000", - "reputation": 137759377200970, - "rshares": 6625515589, - "voter": "yadamaniart" - }, - { - "percent": "6000", - "reputation": 37769232724831, - "rshares": 113516791302, - "voter": "ixindamix" - }, - { - "percent": "2500", - "reputation": 59094884211730, - "rshares": 3268031215, - "voter": "saleg25" - }, - { - "percent": "2400", - "reputation": 7177514553823, - "rshares": 51098253319, - "voter": "gabriele-gio" - }, - { - "percent": "5000", - "reputation": 27214240242904, - "rshares": 46656397208, - "voter": "rahul.stan" - }, - { - "percent": "3000", - "reputation": 15850781860580, - "rshares": 87850808875, - "voter": "neuerko" - }, - { - "percent": "2800", - "reputation": 45761827786994, - "rshares": 39884051429, - "voter": "ryivhnn" - }, - { - "percent": "5000", - "reputation": 1696316811168628, - "rshares": 2679082462958, - "voter": "tarazkp" - }, - { - "percent": "300", - "reputation": 38975615169260, - "rshares": 7715633212, - "voter": "steemitboard" - }, - { - "percent": "10000", - "reputation": 36214470543162, - "rshares": 18794969909, - "voter": "assasin" - }, - { - "percent": "10000", - "reputation": 169327551453023, - "rshares": 7925674824, - "voter": "evildeathcore" - }, - { - "percent": "2500", - "reputation": 15925403061479, - "rshares": 1463125543, - "voter": "titianus" - }, - { - "percent": "10000", - "reputation": 85095413504513, - "rshares": 7948117840, - "voter": "singa" - }, - { - "percent": "3500", - "reputation": 288303313542379, - "rshares": 201668433968, - "voter": "melinda010100" - }, - { - "percent": "3500", - "reputation": 42486683511346, - "rshares": 11240342998, - "voter": "ma1neevent" - }, - { - "percent": "4800", - "reputation": 62578995648516, - "rshares": 75572055651, - "voter": "zaragast" - }, - { - "percent": "10000", - "reputation": 74329552872641, - "rshares": 286658626425, - "voter": "mes" - }, - { - "percent": "10000", - "reputation": 46266249863448, - "rshares": 19281860731, - "voter": "arnel" - }, - { - "percent": "4800", - "reputation": 79234586811944, - "rshares": 143904574033, - "voter": "thenightflier" - }, - { - "percent": "2500", - "reputation": 91224250124, - "rshares": 6430046726, - "voter": "kingkinslow" - }, - { - "percent": "5000", - "reputation": 8778598823202, - "rshares": 2693109647, - "voter": "jraysteem" - }, - { - "percent": "10000", - "reputation": 150168471794812, - "rshares": 75027823164, - "voter": "jznsamuel" - }, - { - "percent": "187", - "reputation": 11448902903972, - "rshares": 92410360312, - "voter": "roomservice" - }, - { - "percent": "3000", - "reputation": 29513750536324, - "rshares": 1811562755, - "voter": "kennyroy" - }, - { - "percent": "5000", - "reputation": 49292892182110, - "rshares": 12664481554, - "voter": "gmuxx" - }, - { - "percent": "10000", - "reputation": 48378473521256, - "rshares": 27000280897, - "voter": "drag33" - }, - { - "percent": "10000", - "reputation": 72689952666000, - "rshares": 280126614344, - "voter": "ninahaskin" - }, - { - "percent": "8000", - "reputation": 108813324772579, - "rshares": 310621072034, - "voter": "nanosesame" - }, - { - "percent": "2500", - "reputation": 441960688612864, - "rshares": 519918948215, - "voter": "isaria" - }, - { - "percent": "10000", - "reputation": 71915783295563, - "rshares": 128290019387, - "voter": "elteamgordo" - }, - { - "percent": "10000", - "reputation": 21298951755480, - "rshares": 297807151993, - "voter": "tonyz" - }, - { - "percent": "6600", - "reputation": 110350001199994, - "rshares": 73647972810, - "voter": "stortebeker" - }, - { - "percent": "5000", - "reputation": 22688897469714, - "rshares": 67656186458, - "voter": "jeanpi1908" - }, - { - "percent": "2500", - "reputation": 45692041727733, - "rshares": 19995487335, - "voter": "carolkean" - }, - { - "percent": "1500", - "reputation": 3553102205525, - "rshares": 21049697237, - "voter": "belahejna" - }, - { - "percent": "10000", - "reputation": 7901101123497, - "rshares": 1116388212326, - "voter": "giuatt07" - }, - { - "percent": "6000", - "reputation": 655474560066583, - "rshares": 1325117764556, - "voter": "galenkp" - }, - { - "percent": "8000", - "reputation": 80786753664668, - "rshares": 25316081220, - "voter": "freddbrito" - }, - { - "percent": "1750", - "reputation": 120327041068001, - "rshares": 60362319621, - "voter": "jayna" - }, - { - "percent": "900", - "reputation": 122017350265257, - "rshares": 186273766979, - "voter": "ew-and-patterns" - }, - { - "percent": "2500", - "reputation": 30158976168359, - "rshares": 5544754361, - "voter": "guchtere" - }, - { - "percent": "10000", - "reputation": 53671677237969, - "rshares": 2020676660, - "voter": "rival" - }, - { - "percent": "10000", - "reputation": 32908808816942, - "rshares": 58380200836, - "voter": "guyfawkes4-20" - }, - { - "percent": "10000", - "reputation": 46399597511616, - "rshares": 114227321241, - "voter": "ladybug146" - }, - { - "percent": "5000", - "reputation": 72804718490057, - "rshares": 43483573080, - "voter": "binkyprod" - }, - { - "percent": "10000", - "reputation": 1961206307670, - "rshares": 23659105262, - "voter": "alfonzo" - }, - { - "percent": "10000", - "reputation": 148419365168, - "rshares": 933465370, - "voter": "abdulshakun" - }, - { - "percent": "1250", - "reputation": 202651998358237, - "rshares": 59150128461, - "voter": "felt.buzz" - }, - { - "percent": "2600", - "reputation": 145609724101, - "rshares": 228219769580, - "voter": "hope-on-fire" - }, - { - "percent": "10000", - "reputation": 62623152420388, - "rshares": 122890528235, - "voter": "nelinoeva" - }, - { - "percent": "1000", - "reputation": 1060930411809617, - "rshares": 2470060996800, - "voter": "themarkymark" - }, - { - "percent": "5500", - "reputation": 190691885658151, - "rshares": 4282110499436, - "voter": "nathanmars" - }, - { - "percent": "10000", - "reputation": 134718275167014, - "rshares": 379759031299, - "voter": "catwomanteresa" - }, - { - "percent": "10000", - "reputation": 895691331080490, - "rshares": 10671328569136, - "voter": "ocd" - }, - { - "percent": "4800", - "reputation": 64603876629814, - "rshares": 206697096983, - "voter": "steempostitalia" - }, - { - "percent": "10000", - "reputation": 1095186992075950, - "rshares": 138474842209, - "voter": "cryptopassion" - }, - { - "percent": "7000", - "reputation": 616021827459, - "rshares": 8729088229, - "voter": "yacobh" - }, - { - "percent": "2750", - "reputation": 61249536206183, - "rshares": 31781150446, - "voter": "redrica" - }, - { - "percent": "10000", - "reputation": 9446256817327, - "rshares": 8335972596, - "voter": "june21neneng" - }, - { - "percent": "5000", - "reputation": 3732045519725, - "rshares": 2029436371, - "voter": "mangoanddaddy" - }, - { - "percent": "2500", - "reputation": 52406600539994, - "rshares": 30143155455, - "voter": "fionasfavourites" - }, - { - "percent": "2500", - "reputation": 15106373780430, - "rshares": 42803917658, - "voter": "spiceboyz" - }, - { - "percent": "1000", - "reputation": 53958201682724, - "rshares": 10202256996, - "voter": "aaronleang" - }, - { - "percent": "2500", - "reputation": 16928832707753, - "rshares": 22753499070, - "voter": "sunravelme" - }, - { - "percent": "4200", - "reputation": 24639928209538, - "rshares": 24303277863, - "voter": "santigs" - }, - { - "percent": "10000", - "reputation": 35972230673675, - "rshares": 246979632890, - "voter": "reinhard-schmid" - }, - { - "percent": "2500", - "reputation": 54379045488206, - "rshares": 65124856953, - "voter": "bashadow" - }, - { - "percent": "750", - "reputation": 101280142849344, - "rshares": 21027318056, - "voter": "kimzwarch" - }, - { - "percent": "10000", - "reputation": 5754775236968, - "rshares": 4410613276, - "voter": "nurhayati" - }, - { - "percent": "1000", - "reputation": 355541395288151, - "rshares": 7707965193721, - "voter": "buildawhale" - }, - { - "percent": "375", - "reputation": 33280218537901, - "rshares": 4193958513, - "voter": "artonmysleeve" - }, - { - "percent": "10000", - "reputation": 169540068420922, - "rshares": 41158470873, - "voter": "raj808" - }, - { - "percent": "4800", - "reputation": 35075558795655, - "rshares": 69441591320, - "voter": "alinakot" - }, - { - "percent": "10000", - "reputation": 669346799793, - "rshares": 21897757237, - "voter": "voxmonkey" - }, - { - "percent": "3000", - "reputation": 128229050079, - "rshares": 1184909369, - "voter": "cconn" - }, - { - "percent": "3000", - "reputation": 82083033108, - "rshares": 819433173, - "voter": "tomwafula" - }, - { - "percent": "250", - "reputation": 445234100950820, - "rshares": 766087672478, - "voter": "therealwolf" - }, - { - "percent": "5000", - "reputation": 65316782795996, - "rshares": 33150969254, - "voter": "mballesteros" - }, - { - "percent": "5637", - "reputation": 0, - "rshares": 91327022732, - "voter": "fatman" - }, - { - "percent": "1000", - "reputation": 336063014350538, - "rshares": 152895540351, - "voter": "revisesociology" - }, - { - "percent": "4000", - "reputation": 6727973666699, - "rshares": 67093846729, - "voter": "omra-sky" - }, - { - "percent": "1000", - "reputation": 86519807944048, - "rshares": 90269083076, - "voter": "jlsplatts" - }, - { - "percent": "1000", - "reputation": 47982158911387, - "rshares": 31156696038, - "voter": "makerhacks" - }, - { - "percent": "4800", - "reputation": 12066290877974, - "rshares": 36888905674, - "voter": "heidi71" - }, - { - "percent": "772", - "reputation": 0, - "rshares": 2448332277, - "voter": "esteem.app" - }, - { - "percent": "186", - "reputation": 0, - "rshares": 67605276598, - "voter": "investegg" - }, - { - "percent": "10000", - "reputation": 3291168947960, - "rshares": 3502523089, - "voter": "enolife" - }, - { - "percent": "10000", - "reputation": 66305366564261, - "rshares": 132669377904, - "voter": "planetauto" - }, - { - "percent": "10000", - "reputation": 29313772061277, - "rshares": 120119577771, - "voter": "castleberry" - }, - { - "percent": "10000", - "reputation": 5205862159626, - "rshares": 216018711119, - "voter": "brisby" - }, - { - "percent": "5000", - "reputation": 1920136042828, - "rshares": 5904178405, - "voter": "petrolinivideo" - }, - { - "percent": "5000", - "reputation": 13524573429346, - "rshares": 1327794333, - "voter": "bangmimi" - }, - { - "percent": "10000", - "reputation": 6785724346858, - "rshares": 149139947192, - "voter": "drillith" - }, - { - "percent": "10000", - "reputation": 57968856130168, - "rshares": 4295582062, - "voter": "otemzi" - }, - { - "percent": "2500", - "reputation": 11326960455322, - "rshares": 14717529232, - "voter": "amritadeva" - }, - { - "percent": "10000", - "reputation": 4223670366661, - "rshares": 27920370108, - "voter": "cizzo" - }, - { - "percent": "7500", - "reputation": 136276266931207, - "rshares": 94829086398, - "voter": "ybanezkim26" - }, - { - "percent": "10000", - "reputation": 473928985768900, - "rshares": 3168408857, - "voter": "tradingideas" - }, - { - "percent": "5000", - "reputation": 12722616650811, - "rshares": 2202907876969, - "voter": "postpromoter" - }, - { - "percent": "4800", - "reputation": 236384820778321, - "rshares": 184861816308, - "voter": "steemflow" - }, - { - "percent": "10000", - "reputation": 1338862822184, - "rshares": 4252536827, - "voter": "potplucker" - }, - { - "percent": "250", - "reputation": 157805541487641, - "rshares": 151789512035, - "voter": "smartsteem" - }, - { - "percent": "1500", - "reputation": 9517778819103, - "rshares": 43178287293, - "voter": "mytechtrail" - }, - { - "percent": "1500", - "reputation": 13569938137899, - "rshares": 3604537541, - "voter": "kneelyrac" - }, - { - "percent": "10000", - "reputation": 5341088715064, - "rshares": 1451342594, - "voter": "aquinotyron3" - }, - { - "percent": "3000", - "reputation": 66785151966461, - "rshares": 143516303848, - "voter": "itchyfeetdonica" - }, - { - "percent": "10000", - "reputation": 3639315776643, - "rshares": 545292010, - "voter": "wild.nature" - }, - { - "percent": "2400", - "reputation": 9242922601755, - "rshares": 10707171563, - "voter": "marcolino76" - }, - { - "percent": "10000", - "reputation": 2868117332382, - "rshares": 46396793085, - "voter": "as31" - }, - { - "percent": "2500", - "reputation": 110889227376345, - "rshares": 2400464022, - "voter": "rpcaceres" - }, - { - "percent": "1500", - "reputation": 14337364044156, - "rshares": 2272702563, - "voter": "tomatom" - }, - { - "percent": "2500", - "reputation": 2675225025765, - "rshares": 7322524209, - "voter": "fourfourfun" - }, - { - "percent": "10000", - "reputation": 9071994818465, - "rshares": 871238509, - "voter": "candyboy" - }, - { - "percent": "1000", - "reputation": 2680015924516, - "rshares": 7442951775, - "voter": "upmyvote" - }, - { - "percent": "650", - "reputation": 94326416009060, - "rshares": 8536023213, - "voter": "paradigmprospect" - }, - { - "percent": "2500", - "reputation": 13345171215929, - "rshares": 13248753237, - "voter": "r00sj3" - }, - { - "percent": "2800", - "reputation": 87198152925, - "rshares": 1241263905, - "voter": "gorc" - }, - { - "percent": "2800", - "reputation": 61649444966, - "rshares": 710438935, - "voter": "pixietrix" - }, - { - "percent": "2800", - "reputation": 55737122541, - "rshares": 828537686, - "voter": "shadowlioncub" - }, - { - "percent": "2200", - "reputation": 7107778763, - "rshares": 340902777381, - "voter": "jim888" - }, - { - "percent": "5000", - "reputation": 39432928472357, - "rshares": 2769928359, - "voter": "stormlight24" - }, - { - "percent": "10000", - "reputation": 36559175792044, - "rshares": 1678632871, - "voter": "jewel-lover" - }, - { - "percent": "5000", - "reputation": 87246032649758, - "rshares": 86115255565, - "voter": "gabrielatravels" - }, - { - "percent": "10000", - "reputation": 59361041133414, - "rshares": 74280355129, - "voter": "bossel" - }, - { - "percent": "1000", - "reputation": 169520027942230, - "rshares": 9917908800, - "voter": "r351574nc3" - }, - { - "percent": "10000", - "reputation": 34815203173240, - "rshares": 11782089998, - "voter": "yakubenko" - }, - { - "percent": "400", - "reputation": 25048759621308, - "rshares": 3869168493, - "voter": "tryskele" - }, - { - "percent": "3750", - "reputation": 10165169816277, - "rshares": 2465710673, - "voter": "leslierevales" - }, - { - "percent": "10000", - "reputation": 22312621205482, - "rshares": 117925144737, - "voter": "enmaart" - }, - { - "percent": "2500", - "reputation": 5600484925419, - "rshares": 1133898059, - "voter": "ricardo993" - }, - { - "percent": "5000", - "reputation": 170235537022, - "rshares": 680211362, - "voter": "auracraft" - }, - { - "percent": "1000", - "reputation": 4755551366717, - "rshares": 670968979, - "voter": "philnewton" - }, - { - "percent": "5000", - "reputation": 37970568723804, - "rshares": 35297644320, - "voter": "psos" - }, - { - "percent": "2100", - "reputation": 8003119161188, - "rshares": 4461781838, - "voter": "brewery" - }, - { - "percent": "10000", - "reputation": 19604135658239, - "rshares": 35631887581, - "voter": "zoricatech" - }, - { - "percent": "10000", - "reputation": 5290234783266, - "rshares": 7354537635, - "voter": "pandasquad" - }, - { - "percent": "1500", - "reputation": 79537112262409, - "rshares": 29345178840, - "voter": "tobias-g" - }, - { - "percent": "2500", - "reputation": 6684590265952, - "rshares": 12653848734, - "voter": "nobyeni" - }, - { - "percent": "10000", - "reputation": 3127964874194, - "rshares": 619030198, - "voter": "randomwanderings" - }, - { - "percent": "10000", - "reputation": 91651981795187, - "rshares": 23369258178, - "voter": "lunaticpandora" - }, - { - "percent": "10000", - "reputation": 12864088653653, - "rshares": 950809975712, - "voter": "lemony-cricket" - }, - { - "percent": "2500", - "reputation": 48991085568043, - "rshares": 11296587215, - "voter": "warpedpoetic" - }, - { - "percent": "10000", - "reputation": 148975588734578, - "rshares": 7828300579, - "voter": "svemirac" - }, - { - "percent": "10000", - "reputation": 8228071109188, - "rshares": 935557570, - "voter": "bet1x2" - }, - { - "percent": "10000", - "reputation": 22367616882821, - "rshares": 5817089570, - "voter": "mr-phoenix" - }, - { - "percent": "10000", - "reputation": 4705304861944, - "rshares": 1487934113232, - "voter": "ocd-witness" - }, - { - "percent": "10000", - "reputation": 86916035515124, - "rshares": 121800009821, - "voter": "cryptosharon" - }, - { - "percent": "2500", - "reputation": 442944056818, - "rshares": 905162431, - "voter": "minerspost" - }, - { - "percent": "3000", - "reputation": 44591308905549, - "rshares": 130077690663, - "voter": "bigtom13" - }, - { - "percent": "1100", - "reputation": 344804463734258, - "rshares": 2469871669, - "voter": "dirapa" - }, - { - "percent": "100", - "reputation": 107854173717168, - "rshares": 3577204213, - "voter": "moeenali" - }, - { - "percent": "10000", - "reputation": 8534044826795, - "rshares": 71724746366, - "voter": "d0zer" - }, - { - "percent": "3000", - "reputation": 182685861369, - "rshares": 851524998, - "voter": "darkpylon" - }, - { - "percent": "10000", - "reputation": 483223942335, - "rshares": 4008676549, - "voter": "elektr1ker" - }, - { - "percent": "5000", - "reputation": 724923766221, - "rshares": 1701925953, - "voter": "liberviarum" - }, - { - "percent": "10000", - "reputation": 22399611269460, - "rshares": 19720842705, - "voter": "cyprianj" - }, - { - "percent": "250", - "reputation": 26898775193091, - "rshares": 2392007368, - "voter": "movement19" - }, - { - "percent": "4200", - "reputation": 192465234875420, - "rshares": 232774973035, - "voter": "whack.science" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 27007409851, - "voter": "futurecurrency" - }, - { - "percent": "1000", - "reputation": 85785149964848, - "rshares": 2640188235, - "voter": "putu300" - }, - { - "percent": "3000", - "reputation": 15537102540153, - "rshares": 28126914412, - "voter": "siphon" - }, - { - "percent": "10000", - "reputation": 6368389637818, - "rshares": 287297061, - "voter": "dunkman" - }, - { - "percent": "10000", - "reputation": 3414691338324, - "rshares": 2302876941, - "voter": "homeginkit" - }, - { - "percent": "1500", - "reputation": 2256065348315, - "rshares": 2488223556, - "voter": "frassman" - }, - { - "percent": "8000", - "reputation": 158256810302501, - "rshares": 137134992416, - "voter": "miroslavrc" - }, - { - "percent": "5000", - "reputation": 45433987678513, - "rshares": 38473605280, - "voter": "khaimi" - }, - { - "percent": "1125", - "reputation": 3636565312717, - "rshares": 1670243119, - "voter": "kymio" - }, - { - "percent": "8888", - "reputation": 46955154178798, - "rshares": 1008003095299, - "voter": "amico" - }, - { - "percent": "1000", - "reputation": 10250642778719, - "rshares": 3401814626, - "voter": "salty-mcgriddles" - }, - { - "percent": "10000", - "reputation": 8107441442652, - "rshares": 10493115058, - "voter": "deltasteem" - }, - { - "percent": "10000", - "reputation": 4528527620889, - "rshares": 863957328, - "voter": "elider11" - }, - { - "percent": "9000", - "reputation": 1108677568897, - "rshares": 5957890520, - "voter": "jan23com" - }, - { - "percent": "10000", - "reputation": 373679836722908, - "rshares": 3415174572023, - "voter": "derangedvisions" - }, - { - "percent": "7500", - "reputation": 35499944396318, - "rshares": 25713188211, - "voter": "tesmoforia" - }, - { - "percent": "10000", - "reputation": 14193144291158, - "rshares": 2864770933, - "voter": "abdulmath" - }, - { - "percent": "10000", - "reputation": 10653602334497, - "rshares": 60036064434, - "voter": "melbourneswest" - }, - { - "percent": "10000", - "reputation": 10532279362821, - "rshares": 3847426305, - "voter": "sallyfun" - }, - { - "percent": "10000", - "reputation": 37847496032881, - "rshares": 13487278148, - "voter": "z3ll" - }, - { - "percent": "10000", - "reputation": 11722167805732, - "rshares": 30957198588, - "voter": "phasewalker" - }, - { - "percent": "9000", - "reputation": 158718167236, - "rshares": 3646594887, - "voter": "choco11oreo11" - }, - { - "percent": "1243", - "reputation": 329293736617948, - "rshares": 208781581880, - "voter": "quochuy" - }, - { - "percent": "10000", - "reputation": 2481629111221, - "rshares": 1955390031, - "voter": "manojbhatt" - }, - { - "percent": "5000", - "reputation": 817110539670, - "rshares": 668880167, - "voter": "vanuzza" - }, - { - "percent": "10000", - "reputation": 42246987760, - "rshares": 8673203037, - "voter": "feedmytwi" - }, - { - "percent": "10000", - "reputation": 49896781217774, - "rshares": 43312878670, - "voter": "cesaramos" - }, - { - "percent": "4500", - "reputation": 4158142244411, - "rshares": 1248776555, - "voter": "oluwashinaayomi" - }, - { - "percent": "3000", - "reputation": 54368794178735, - "rshares": 89910440559, - "voter": "birdsinparadise" - }, - { - "percent": "10000", - "reputation": 476429169806, - "rshares": 1305725356, - "voter": "lemcriq" - }, - { - "percent": "3000", - "reputation": 10484590752220, - "rshares": 48018844394, - "voter": "veteranforcrypto" - }, - { - "percent": "10000", - "reputation": 1180987164511, - "rshares": 3636094662, - "voter": "coarebabes" - }, - { - "percent": "5000", - "reputation": 715633044078, - "rshares": 3747888772, - "voter": "smacommunity" - }, - { - "percent": "3000", - "reputation": 73448836500533, - "rshares": 7715412668, - "voter": "eii" - }, - { - "percent": "5000", - "reputation": 28237853787625, - "rshares": 556937306, - "voter": "crystalhuman" - }, - { - "percent": "5000", - "reputation": 12736574015031, - "rshares": 4635841365, - "voter": "sayago" - }, - { - "percent": "2200", - "reputation": 51236835710173, - "rshares": 9913045603, - "voter": "davidesimoncini" - }, - { - "percent": "10000", - "reputation": 400173500953, - "rshares": 561137629, - "voter": "gmlgang" - }, - { - "percent": "2250", - "reputation": 31963786148569, - "rshares": 3793303454, - "voter": "archisteem" - }, - { - "percent": "10000", - "reputation": 5298034156084, - "rshares": 27801899715, - "voter": "motherofalegend" - }, - { - "percent": "10000", - "reputation": 401559670619, - "rshares": 3175554877, - "voter": "onethousandpics" - }, - { - "percent": "1000", - "reputation": 599567348573, - "rshares": 524643994, - "voter": "exifr" - }, - { - "percent": "1200", - "reputation": 68462748929740, - "rshares": 120977801527, - "voter": "roger5120" - }, - { - "percent": "3000", - "reputation": 1401585186039, - "rshares": 4945121548, - "voter": "sivehead" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1337984045, - "voter": "abacam" - }, - { - "percent": "10000", - "reputation": 254709457166, - "rshares": 7254274853, - "voter": "abcor" - }, - { - "percent": "2780", - "reputation": 65690218229412, - "rshares": 56778867093, - "voter": "annephilbrick" - }, - { - "percent": "10000", - "reputation": 84809016946227, - "rshares": 12484416788, - "voter": "kapitanrosomak" - }, - { - "percent": "3400", - "reputation": 20764791822405, - "rshares": 6912953465, - "voter": "desireeart" - }, - { - "percent": "1500", - "reputation": 97973570792503, - "rshares": 7005728493, - "voter": "mrnightmare89" - }, - { - "percent": "750", - "reputation": 18701622566955, - "rshares": 2757703920, - "voter": "ambiguity" - }, - { - "percent": "5000", - "reputation": 3516028097696, - "rshares": 41920687980328, - "voter": "ocdb" - }, - { - "percent": "10000", - "reputation": 104172726518074, - "rshares": 604972541940, - "voter": "pdc" - }, - { - "percent": "10000", - "reputation": 20224059636954, - "rshares": 24325962192, - "voter": "adonisr" - }, - { - "percent": "10000", - "reputation": 16007619374672, - "rshares": 4857840102, - "voter": "cyberspacegod" - }, - { - "percent": "10000", - "reputation": 114256216898620, - "rshares": 55702035993, - "voter": "digi-me" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 853158864, - "voter": "zeeon" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 741566769, - "voter": "davidox" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 741529790, - "voter": "daleron" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 741648774, - "voter": "fireon" - }, - { - "percent": "10000", - "reputation": 7038024937952, - "rshares": 43788748988, - "voter": "dog-marley" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1178903309, - "voter": "nh3" - }, - { - "percent": "7000", - "reputation": 44525854399402, - "rshares": 126602572107, - "voter": "thehive" - }, - { - "percent": "1000", - "reputation": 116804559, - "rshares": 626380820, - "voter": "exifr0" - }, - { - "percent": "10000", - "reputation": 58717222761366, - "rshares": 11305371250, - "voter": "gohalber" - }, - { - "percent": "9000", - "reputation": 22555392895, - "rshares": 5514356912, - "voter": "littleshadow" - }, - { - "percent": "30", - "reputation": 18790919715448, - "rshares": 4185151288, - "voter": "luppers" - }, - { - "percent": "2500", - "reputation": 7532194699640, - "rshares": 2030009444, - "voter": "cubapl" - }, - { - "percent": "10000", - "reputation": 12546717165553, - "rshares": 27754647544, - "voter": "tommasobusiello" - }, - { - "percent": "2000", - "reputation": 144910360949054, - "rshares": 39594355265, - "voter": "voxmortis" - }, - { - "percent": "9000", - "reputation": 17567685388, - "rshares": 1876050107, - "voter": "joseph6232" - }, - { - "percent": "7500", - "reputation": 19783849759, - "rshares": 3313067951, - "voter": "emaillisahere" - }, - { - "percent": "5000", - "reputation": 19563050817, - "rshares": 632615863, - "voter": "buzzbee" - }, - { - "percent": "2500", - "reputation": 7344887427854, - "rshares": 37795393981, - "voter": "altonos" - }, - { - "percent": "10000", - "reputation": 1503728134655, - "rshares": 505618169006, - "voter": "tipsybosphorus" - }, - { - "percent": "2000", - "reputation": 22970128827598, - "rshares": 17837974990, - "voter": "foodfightfriday" - }, - { - "percent": "10000", - "reputation": 37453744817, - "rshares": 4378553908, - "voter": "caoimhin" - }, - { - "percent": "7500", - "reputation": 27727654562, - "rshares": 1491883674, - "voter": "djtrucker" - }, - { - "percent": "4000", - "reputation": 66724559701872, - "rshares": 81526501371, - "voter": "muscara" - }, - { - "percent": "10000", - "reputation": 76163497686104, - "rshares": 23877257875, - "voter": "franz54" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 14408264337, - "voter": "quediceharry" - }, - { - "percent": "8500", - "reputation": 260876943473, - "rshares": 78578979857, - "voter": "marshalmugi" - }, - { - "percent": "9000", - "reputation": 1786834467, - "rshares": 1782542600, - "voter": "podg3" - }, - { - "percent": "5000", - "reputation": 6687049412835, - "rshares": 40663348996, - "voter": "mister-meeseeks" - }, - { - "percent": "9000", - "reputation": 2040204650, - "rshares": 6725797664, - "voter": "misstaken" - }, - { - "percent": "5000", - "reputation": 28081859964582, - "rshares": 12812011279, - "voter": "naty16" - }, - { - "percent": "4000", - "reputation": 1460324570172, - "rshares": 7184702138, - "voter": "kuku-splatts" - }, - { - "percent": "10000", - "reputation": 1591954997388, - "rshares": 762115798, - "voter": "mannaman" - }, - { - "percent": "2500", - "reputation": 55273774845202, - "rshares": 2427689766, - "voter": "elsaenroute" - }, - { - "percent": "10000", - "reputation": 18273031359521, - "rshares": 4099902038, - "voter": "adyorka" - }, - { - "percent": "10000", - "reputation": 37901964014543, - "rshares": 5688220102, - "voter": "estefania3" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 341791049533, - "voter": "milky-concrete" - }, - { - "percent": "3000", - "reputation": 0, - "rshares": 538535159, - "voter": "conectionbot" - }, - { - "percent": "5000", - "reputation": 5548258794861, - "rshares": 747201869, - "voter": "onze" - }, - { - "percent": "9000", - "reputation": 4187177339, - "rshares": 2087265711, - "voter": "jussbren" - }, - { - "percent": "2500", - "reputation": 6212919718205, - "rshares": 2258291791, - "voter": "letalis-laetitia" - }, - { - "percent": "10000", - "reputation": 1813737497231, - "rshares": 4048797647, - "voter": "knjigesuin" - }, - { - "percent": "10000", - "reputation": 8468960967138, - "rshares": 1951029935, - "voter": "smonia" - }, - { - "percent": "10000", - "reputation": 430824325047, - "rshares": 1377674162, - "voter": "pandapuzzles" - }, - { - "percent": "3000", - "reputation": 854097216443, - "rshares": 526922991, - "voter": "redheaddemon" - }, - { - "percent": "2900", - "reputation": 4104615584, - "rshares": 30668763590, - "voter": "steem-on-2020" - }, - { - "percent": "2000", - "reputation": 30517153592067, - "rshares": 6888348240, - "voter": "variedades" - }, - { - "percent": "5000", - "reputation": 185852714902, - "rshares": 1024092418, - "voter": "j-p-bs" - }, - { - "percent": "5000", - "reputation": 4043053357511, - "rshares": 215653904752, - "voter": "anmitsu" - }, - { - "percent": "10000", - "reputation": 671364595259, - "rshares": 2028497110, - "voter": "smon-fan" - }, - { - "percent": "1000", - "reputation": 86443203025994, - "rshares": 4532144771, - "voter": "jacuzzi" - }, - { - "percent": "10000", - "reputation": 6944150333466, - "rshares": 4663788865, - "voter": "milyy" - }, - { - "percent": "10000", - "reputation": 8193736963639, - "rshares": 1891457205, - "voter": "breakforbook" - }, - { - "percent": "10000", - "reputation": 2488971014315, - "rshares": 1737296461, - "voter": "tr777" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 618475906, - "voter": "sm-jewel" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1619035418, - "voter": "tr77" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1450066538, - "voter": "smoner" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1688449372, - "voter": "smonian" - }, - { - "percent": "2500", - "reputation": 6085708906847, - "rshares": 3704597571, - "voter": "creary" - }, - { - "percent": "10000", - "reputation": 71911881788546, - "rshares": 562816413864, - "voter": "dlstudios" - }, - { - "percent": "500", - "reputation": -4467669911343, - "rshares": 778210973404, - "voter": "ctime" - }, - { - "percent": "10000", - "reputation": 75911580, - "rshares": 175298269711, - "voter": "jamesbattler" - }, - { - "percent": "10000", - "reputation": 54892997399545, - "rshares": 1422090500, - "voter": "smon-joa" - }, - { - "percent": "10000", - "reputation": 5230675637, - "rshares": 1476422811, - "voter": "jjangjjanggirl" - }, - { - "percent": "10000", - "reputation": 275674068830, - "rshares": 589306972, - "voter": "qei" - }, - { - "percent": "368", - "reputation": 5616865862, - "rshares": 765603489, - "voter": "abbenay" - }, - { - "percent": "5000", - "reputation": 96673801127912, - "rshares": 117299309310, - "voter": "doze" - }, - { - "percent": "10000", - "reputation": 5833218185, - "rshares": 1701542042, - "voter": "smonbear" - }, - { - "percent": "75", - "reputation": 120936131908085, - "rshares": 5560494134, - "voter": "epicdice" - }, - { - "percent": "10000", - "reputation": 13650758281946, - "rshares": 500169151595, - "voter": "rodrook" - }, - { - "percent": "5000", - "reputation": 61476958391321, - "rshares": 2454341546, - "voter": "ricardomello" - }, - { - "percent": "10000", - "reputation": 98578472333076, - "rshares": 491355235594, - "voter": "minigame" - }, - { - "percent": "2500", - "reputation": 2597264781531, - "rshares": 3894717635, - "voter": "akumagai" - }, - { - "percent": "5000", - "reputation": 10702198150786, - "rshares": 142443264830, - "voter": "scholaris" - }, - { - "percent": "7000", - "reputation": 5706500792678, - "rshares": 7788777840, - "voter": "spurisna" - }, - { - "percent": "10000", - "reputation": 14615302348169, - "rshares": 8782846432, - "voter": "dutybound" - }, - { - "percent": "10000", - "reputation": 9877498112396, - "rshares": 1349989372, - "voter": "ssc-token" - }, - { - "percent": "5000", - "reputation": 270631290373, - "rshares": 581138265, - "voter": "treze" - }, - { - "percent": "10000", - "reputation": 185044670844, - "rshares": 0, - "voter": "tradingideas2" - }, - { - "percent": "10000", - "reputation": 13622047625602, - "rshares": 1400075533, - "voter": "ocd-accountant" - }, - { - "percent": "250", - "reputation": 20916803594387, - "rshares": 1145702151, - "voter": "leighscotford" - }, - { - "percent": "10000", - "reputation": 16313879716356, - "rshares": 40858846507, - "voter": "tggr" - }, - { - "percent": "2500", - "reputation": 1138890453375, - "rshares": 1546018008, - "voter": "iamjohn" - }, - { - "percent": "10000", - "reputation": 17647336098195, - "rshares": 35034145753, - "voter": "c21c" - }, - { - "percent": "10000", - "reputation": 9163578879, - "rshares": 556829290, - "voter": "suigener1s" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 935392217, - "voter": "joker777" - }, - { - "percent": "9000", - "reputation": 12552713403211, - "rshares": 18338991778, - "voter": "yeswecan" - }, - { - "percent": "2500", - "reputation": 14030750678, - "rshares": 939000219, - "voter": "pal-isaria" - }, - { - "percent": "10000", - "reputation": 113172734, - "rshares": 1157361703, - "voter": "penpals" - }, - { - "percent": "1500", - "reputation": 612780456165, - "rshares": 1236901781, - "voter": "freedomring" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 3025785413, - "voter": "babytarazkp" - }, - { - "percent": "10000", - "reputation": 17170954649, - "rshares": 937868109, - "voter": "cards4u" - }, - { - "percent": "10000", - "reputation": 376803157115, - "rshares": 370442566, - "voter": "tina-tina" - }, - { - "percent": "10000", - "reputation": 45364952422214, - "rshares": 62867666541, - "voter": "soyunasantacruz" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "happiness19" - }, - { - "percent": "10000", - "reputation": 97507720606, - "rshares": 35151834, - "voter": "gdhaetae" - }, - { - "percent": "5000", - "reputation": 1281549773, - "rshares": 962105358, - "voter": "tggr.trb" - }, - { - "percent": "10000", - "reputation": 110971278120219, - "rshares": 133526746454, - "voter": "janaveda" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 548374746, - "voter": "loperdt" - }, - { - "percent": "1000", - "reputation": 4144830007563, - "rshares": 4051934066, - "voter": "idig" - }, - { - "percent": "10000", - "reputation": 770042020971, - "rshares": 30055105653, - "voter": "acta" - }, - { - "percent": "9000", - "reputation": 5889854881, - "rshares": 20321616697, - "voter": "the-table" - }, - { - "percent": "10000", - "reputation": 266042222060, - "rshares": 2433797960, - "voter": "cardtrader" - }, - { - "percent": "9000", - "reputation": 19199339523, - "rshares": 1943146990, - "voter": "thehouse" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1670274417, - "voter": "dnflsms" - }, - { - "percent": "10000", - "reputation": 848203521475, - "rshares": 117553078814, - "voter": "downvoteme" - }, - { - "percent": "4000", - "reputation": 0, - "rshares": 191229282822, - "voter": "urtrailer" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 899090935, - "voter": "jessy22" - }, - { - "percent": "10000", - "reputation": 34345409186789, - "rshares": 375313306527, - "voter": "homeedders" - }, - { - "percent": "1500", - "reputation": 3257555962879, - "rshares": 774866598, - "voter": "bilpcoinrecords" - }, - { - "percent": "2500", - "reputation": 0, - "rshares": 939041436, - "voter": "isaria-ccc" - }, - { - "percent": "9000", - "reputation": 967638294423, - "rshares": 149140694115, - "voter": "silverquest" - }, - { - "percent": "4250", - "reputation": 257075039692, - "rshares": 3893700470, - "voter": "efathenub" - }, - { - "percent": "8500", - "reputation": 268654389989, - "rshares": 10349880517, - "voter": "honeychip" - }, - { - "percent": "10000", - "reputation": 6232300918, - "rshares": 625391006, - "voter": "artmusiclife" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 1529784193, - "voter": "khalccc" - }, - { - "percent": "4000", - "reputation": 13338109908260, - "rshares": 35480685238, - "voter": "splatts" - }, - { - "percent": "750", - "reputation": 0, - "rshares": 16628711, - "voter": "kryptoformator" - }, - { - "percent": "6000", - "reputation": 0, - "rshares": 706547347, - "voter": "galenkp.aus" - }, - { - "percent": "10000", - "reputation": 6574541842654, - "rshares": 14456413868, - "voter": "hjmarseille" - }, - { - "percent": "5000", - "reputation": 1201079161955, - "rshares": 710641640, - "voter": "gmlrecordz" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1321568471, - "voter": "keepit2" - }, - { - "percent": "6000", - "reputation": 4834237142168, - "rshares": 7721534323, - "voter": "journeyofanomad" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 935355741, - "voter": "gogoo" - }, - { - "percent": "2500", - "reputation": 23010538544428, - "rshares": 6215778030, - "voter": "garlet" - }, - { - "percent": "7500", - "reputation": 0, - "rshares": 621449034, - "voter": "zappyzap" - }, - { - "percent": "10000", - "reputation": 76222060731, - "rshares": 2262071460, - "voter": "wallytiteuf" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 764313462, - "voter": "garlet-68" - }, - { - "percent": "5000", - "reputation": 9624495306704, - "rshares": 54572735539363, - "voter": "darthknight" - }, - { - "percent": "10000", - "reputation": 12277716584335, - "rshares": 13809175581, - "voter": "orestistrips" - }, - { - "percent": "10000", - "reputation": 3260566328403, - "rshares": 12710957128, - "voter": "gorllara" - }, - { - "percent": "10000", - "reputation": 1588836327, - "rshares": 0, - "voter": "wasifbhutto" - }, - { - "percent": "5000", - "reputation": 164450582774349, - "rshares": 67784844628, - "voter": "alexa.art" - }, - { - "percent": "9000", - "reputation": 30255784377, - "rshares": 1782228802, - "voter": "tommys.shop" - }, - { - "percent": "5000", - "reputation": 18071750627046, - "rshares": 119879656744, - "voter": "theinkwell" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 935399266, - "voter": "edaz" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 935405716, - "voter": "qtu" - }, - { - "percent": "300", - "reputation": 0, - "rshares": 15763678532, - "voter": "fengchao" - }, - { - "percent": "7500", - "reputation": 355888101482, - "rshares": 578980729, - "voter": "hivewaves" - }, - { - "percent": "10000", - "reputation": 35894562143595, - "rshares": 261125851713, - "voter": "radiohive" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 935422421, - "voter": "neo2" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 623097497, - "voter": "kravata" - }, - { - "percent": "3900", - "reputation": 1209731647511, - "rshares": 668315405549, - "voter": "softworld" - }, - { - "percent": "10000", - "reputation": 17500919200979, - "rshares": 56134129158, - "voter": "arlettemsalase" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 954619389, - "voter": "morpheus2" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 954618559, - "voter": "trinity2" - }, - { - "percent": "1500", - "reputation": 22060257076152, - "rshares": 6576938151, - "voter": "quello" - }, - { - "percent": "10000", - "reputation": 7005445491415, - "rshares": 5179554898, - "voter": "patagonian-nomad" - }, - { - "percent": "2500", - "reputation": 19801520667766, - "rshares": 2538800663, - "voter": "maitt87" - }, - { - "percent": "1000", - "reputation": 661148949025, - "rshares": 599525163, - "voter": "gradeon" - }, - { - "percent": "7500", - "reputation": 5014132859, - "rshares": 1516314299, - "voter": "paulman" - }, - { - "percent": "187", - "reputation": 47896334821902, - "rshares": 2531238776, - "voter": "hiveonboard" - }, - { - "percent": "1000", - "reputation": 3177222978373, - "rshares": 9168234171, - "voter": "hivelist" - }, - { - "percent": "500", - "reputation": 604442758770, - "rshares": 22341776091, - "voter": "ninnu" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 122230195155, - "voter": "kiemurainen" - }, - { - "percent": "757", - "reputation": 56328328383994, - "rshares": 465318233707, - "voter": "ecency" - }, - { - "percent": "300", - "reputation": 9650263289467, - "rshares": 1600500246, - "voter": "localgrower" - }, - { - "percent": "10000", - "reputation": 7436529679810, - "rshares": 7113577334, - "voter": "belen0949" - }, - { - "percent": "10000", - "reputation": 429763208250, - "rshares": 4561981052, - "voter": "ghaazi" - }, - { - "percent": "10000", - "reputation": 54824847159245, - "rshares": 104777245624, - "voter": "discoveringarni" - }, - { - "percent": "5000", - "reputation": -588508122267, - "rshares": 43005179104, - "voter": "poshbot" - }, - { - "percent": "10000", - "reputation": 840880043644, - "rshares": 5977172202, - "voter": "smh01" - }, - { - "percent": "2500", - "reputation": 5470715229938, - "rshares": 1064639937, - "voter": "jsalvage" - }, - { - "percent": "10000", - "reputation": 630799830005, - "rshares": 5777049476, - "voter": "memesforhive" - }, - { - "percent": "3000", - "reputation": 15777185838, - "rshares": 860940581, - "voter": "patronpass" - }, - { - "percent": "10000", - "reputation": 2014353692383, - "rshares": 776120486, - "voter": "ralphsam2" - }, - { - "percent": "10000", - "reputation": 869572128132, - "rshares": 6124901012, - "voter": "myword" - }, - { - "percent": "5500", - "reputation": 65519590598, - "rshares": 1139946996, - "voter": "plusvault" - }, - { - "percent": "7500", - "reputation": 17939114076906, - "rshares": 16823152410, - "voter": "viviana28" - }, - { - "percent": "10000", - "reputation": 23814154211011, - "rshares": 4314151129, - "voter": "syberia" - }, - { - "percent": "4000", - "reputation": 0, - "rshares": 6713349092, - "voter": "w-splatts" - }, - { - "percent": "772", - "reputation": 12378826857256, - "rshares": 1474660155, - "voter": "ecency.stats" - }, - { - "percent": "10000", - "reputation": 2742340079942, - "rshares": 2620085527, - "voter": "dikshabihani" - }, - { - "percent": "10000", - "reputation": 792202344312, - "rshares": 798553070, - "voter": "ollasysartenes" - }, - { - "percent": "5000", - "reputation": 7273089567, - "rshares": 625730932, - "voter": "hivecoffee" - }, - { - "percent": "10000", - "reputation": 2830639099232, - "rshares": 205995741, - "voter": "koxmicart" - }, - { - "percent": "500", - "reputation": 25284728169845, - "rshares": 135820917516, - "voter": "recoveryinc" - }, - { - "percent": "1000", - "reputation": 76284107957, - "rshares": 2646938618, - "voter": "hive-108278" - }, - { - "percent": "10000", - "reputation": 9517022344384, - "rshares": 38990646309, - "voter": "rafaelgreen" - }, - { - "percent": "10000", - "reputation": 7461354093641, - "rshares": 4767426268, - "voter": "kasna" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 64439058552, - "voter": "gohive" - }, - { - "percent": "1800", - "reputation": 15234109271535, - "rshares": 2498924758, - "voter": "vicnzia" - }, - { - "percent": "2500", - "reputation": 3274158340408, - "rshares": 5179406333, - "voter": "spirall" - }, - { - "percent": "500", - "reputation": 0, - "rshares": 4799681325, - "voter": "dying" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 21228046452, - "voter": "vtol79" - }, - { - "percent": "6500", - "reputation": 837854855679, - "rshares": 16631870280, - "voter": "rituraz17" - }, - { - "percent": "1000", - "reputation": 0, - "rshares": 1518585848177, - "voter": "usainvote" - }, - { - "percent": "2500", - "reputation": 1447273576100, - "rshares": 1310963915, - "voter": "bitcome" - }, - { - "percent": "2500", - "reputation": 3679705780578, - "rshares": 4195214559, - "voter": "senseiphil" - }, - { - "percent": "5000", - "reputation": 1668767539307, - "rshares": 681157365, - "voter": "gonklavez9" - }, - { - "percent": "6000", - "reputation": 3038383065569, - "rshares": 37239651444, - "voter": "crypto-gamer" - }, - { - "percent": "10000", - "reputation": 210835970747, - "rshares": 3923956317, - "voter": "william-gregory" - }, - { - "percent": "10000", - "reputation": 86405531104, - "rshares": 1964725036, - "voter": "text2speech" - }, - { - "percent": "10000", - "reputation": 369404455919, - "rshares": 17314348311, - "voter": "enrico2gameplays" - }, - { - "percent": "10000", - "reputation": 654061210157, - "rshares": 2062266688, - "voter": "vaishnavi24" - } - ], - "author": "ocd", - "author_reputation": 895691331080490, - "beneficiaries": [ - { - "account": "jznsamuel", - "weight": 5000 - } - ], - "body": "https://images.hive.blog/768x0/https://files.peakd.com/file/peakd-hive/ocd/f0T8DgNw-image.png\n\nHey everyone! Time for another update on our ongoing Community Incubation Program from Team OCD. In [our last post](https://peakd.com/hive-174578/@ocd/ocd-communities-incubation-program-update-8), we saw some activity-related stats about the communities we're currently supporting and it was interesting to see the graphs about number of active users and count of posts. The communities are doing their best to keep engagement levels up and are ready to welcome and guide new users. Community leaders/moderators continue to highlight posts in our discord for some added curation. We also hope to conduct some contests in future to further boost engagement in smaller communities and give them added visibility.\n\nOur aim is to support communities, help them grow and allow users posting on genre specific topics find the right community spaces to interact with like-minded individuals, and gain through the focused curation done by community leaders.\n\nIf you've started new and unique genre-specific community that has the potential to raise a lot of activity and doesn't exist yet, feel free to apply for our incubation program - either by writing in the comment section or contacting us on [our Discord in the community-incubation channel](https://discord.gg/bnGc4BU). You can get more info about the program [here](https://peakd.com/hive-174578/@ocd/ocd-community-incubation-program-finalized).\n\n##### Without further wait, below we give you details on the subscriber growth as well as the new communities who've joined us...\n\n</n>\n* [Alien Art Hive](https://peakd.com/c/hive-158694/trending) - @juliakponsford - 1124 subs -> 1142 subs\n* [Amazing Nature](https://peakd.com/c/hive-127788/trending) - @hive-127788 - 1632 subs -> 1665 subs\n* [Build-it](https://peakd.com/c/hive-129017/trending) - @build-it - @build-it.daily (for reports) - 639 subs -> 672 subs\n* [ecoTrain](https://peakd.com/c/hive-123046/trending) - @ecotrain - 1108 -> 1122 subs\n* [Feathered Friends](https://peakd.com/c/hive-106444/trending) - @hive-106444 - 693 subs -> 705 subs\n* [Foodies Bee Hive](https://peakd.com/c/hive-120586/trending) - 1928 subs -> 1974 subs\n* [Fungi Lovers](https://peakd.com/c/hive-166168/trending) - @hive-166168 - 330 subs -> 355 subs\n* [Fascinating Insects](https://peakd.com/c/hive-101587/trending) - 871subs -> 891 subs\n* [Haveyoubeenhere](https://peakd.com/c/hive-163772/trending) - @pinmapple - 866 subs -> 931 subs\n* [Hive Gaming](https://peakd.com/c/hive-140217/trending) - @hivegc - 3449 subs -> 3508 subs\n* [Hive Open Mic](https://peakd.com/c/hive-105786/trending) - 248 subs -> 254 subs\n* [Home Edders](https://peakd.com/c/hive-199420/trending) - @homeedders - 160 subs -> 167 subs\n* [Lightpainters United](https://peakd.com/c/hive-108913/trending) - @lightpaintershub - 362 subs -> 365 subs\n* [Manipulation Station](https://peakd.com/c/hive-122516/trending) - @hive-122516 - 91 subs -> 91 subs\n* [Natural Medicine](https://peakd.com/c/hive-120078/trending) - @naturalmedicine - 2315 subs -> 2347 subs\n* [Needle Work Monday](https://peakd.com/c/hive-127911/trending) - @needleworkmonday - 396 subs -> 408 subs\n* [On Chain Art](https://peakd.com/c/hive-156509/trending) - @onchainart - 2860 subs -> 2917 subs\n* [Photography Lovers](https://peakd.com/c/hive-194913/trending) - @hive-194913 - 2590 subs -> 2654 subs\n* [Pixel Art](https://peakd.com/c/hive-102502/trending) - @kristyglas - 273 subs -> 282 subs\n* [Programming](https://peakd.com/c/hive-169321/trending) - 123 subs -> 129 subs\n* [ReggaeJAHM](https://peakd.com/c/hive-183952/trending) - 139 subs -> 148 subs\n* [Skate Hive](https://peakd.com/c/hive-173115/trending) - @skatehive - 199 subs -> 209 subs\n* [Sports Talk Social](https://peakd.com/c/hive-101690/trending) - 817 subs -> 830 subs\n* [StemSocial](https://peakd.com/c/hive-196387/trending) - 1117 subs -> 1125 subs\n* [Stock images](https://peakd.com/c/hive-118554/trending) - @hive-118554 - 312 subs -> 328 subs\n* [The Anime Realm](https://peakd.com/c/hive-158489/trending) - @dlstudios - 149 subs -> 165 subs\n* [The Ink Well](https://peakd.com/c/hive-170798/trending) - @theinkwell - 956 subs -> 982 subs\n* [Vimm](https://peakd.com/c/hive-169926/trending) - 366 subs -> 372 subs\n\nMany communities had good subscriber growth, and were quite active too. People are certainly checking them out as they explore diverse topics and contribute to interesting conversations across hive. In terms of engagement, communities like Haveyoubeenhere, Sports Talk Social, Amazing Nature and Foodies Bee Hive had over 500 interactions and 140+ active users. Hive Gaming, OnChainArt, Natural Medicine and Photography Lovers are some of the bigger communities that are popular and generate a lot of interaction and engagement on hive. *What is the one new community you subscribed to recently? - we'd love to know in comments.*\n\n##### New additions:\nWe'd like to welcome 3 new communities into the program!\n\n* [Movies & TV Shows](https://peakd.com/c/hive-166847/trending) - 928 subs\n* [Music](https://peakd.com/c/hive-193816/trending) - 1273 subs \n* [Shadow Hunters](https://peakd.com/c/hive-179017/trending) - 374 subs\n\nHead over to Movies & TV Shows for interesting entertainmentrelated content and participate in discussions on related topics. For all things about Music, check out the Music community. And Shadow Hunters is the place for you to showcase your cool shadow pictures.\n\n ---\n\nWe're looking forward to adding more communities focusing on different subjects to our incubation program, and helping them grow with curation/delegations.\n\nThese posts aim to highlight the communities as well as their curation accounts that post the curation reports, and if you find a community interesting, do feel free to have a look around/subscribe. They'd be happy to welcome/guide you.\n\nThe community moderators/curators are doing a great job trying to onboard and help new users as well as highlighting great content for curation. It's important that users find the right communities and subscribe to those that interest them, which keeps them active and engaged. Thanks for reading!\n\n___\n\n###### We would appreciate it if you want to delegate to @ocdb and earn some daily returns with a low fee which can be tracked on [our website](https://thegoodwhales.io/delegators.html) and vote for our [witness if you appreciate what we're doing on Hive](https://hivesigner.com/sign/account-witness-vote?witness=ocd-witness&approve=1). \n\n___\n\nHave you stumbled upon some new communities that are unique to the ones above and would like to see them get supported? Feel free to mention them here in the comments or join us on [Discord](https://discord.gg/bnGc4BU) and talk about it there in the #community-incubation channel! Please note that this is only for unique niches/genres of communities, nothing \"general original content\" or country/language based.", - "body_length": 6953, - "cashout_time": "2020-11-08T00:10:36", - "category": "hive-174578", - "children": 8, - "created": "2020-11-01T00:10:36", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"app\":\"peakd/2020.10.9\",\"format\":\"markdown\",\"tags\":[\"communities\",\"curation\",\"delegation\",\"community-incubation\",\"ocd\"],\"users\":[\"ocd\",\"juliakponsford\",\"hive-127788\",\"build-it\",\"build-it.daily\",\"ecotrain\",\"hive-106444\",\"hive-166168\",\"pinmapple\",\"hivegc\",\"homeedders\",\"lightpaintershub\",\"hive-122516\",\"naturalmedicine\",\"needleworkmonday\",\"onchainart\",\"hive-194913\",\"kristyglas\",\"skatehive\",\"hive-118554\",\"dlstudios\",\"theinkwell\",\"ocdb\"],\"links\":[\"/hive-174578/@ocd/ocd-communities-incubation-program-update-8\",\"https://discord.gg/bnGc4BU\",\"/hive-174578/@ocd/ocd-community-incubation-program-finalized\",\"/c/hive-158694/trending\",\"/@juliakponsford\",\"/c/hive-127788/trending\",\"/@hive-127788\",\"/c/hive-129017/trending\",\"/@build-it\",\"/@build-it.daily\"],\"image\":[\"https://images.hive.blog/768x0/https://files.peakd.com/file/peakd-hive/ocd/f0T8DgNw-image.png\"]}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-11-01T00:10:36", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 233499172173430, - "parent_author": "", - "parent_permlink": "hive-174578", - "pending_payout_value": "54.581 HBD", - "percent_hbd": 0, - "permlink": "ocd-communities-incubation-program-update-9", - "post_id": 100337289, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "OCD Communities Incubation Program - Update #9", - "title": "OCD Communities Incubation Program - Update #9", - "total_payout_value": "0.000 HBD", - "url": "/hive-174578/@ocd/ocd-communities-incubation-program-update-9" - }, - { - "active_votes": [ - { - "percent": "10000", - "reputation": 1594304819447449, - "rshares": 11231798509970, - "voter": "acidyo" - }, - { - "percent": "3300", - "reputation": 87563369794060, - "rshares": 61157016327, - "voter": "churdtzu" - }, - { - "percent": "1100", - "reputation": 239589086810753, - "rshares": 27493062513, - "voter": "kennyskitchen" - }, - { - "percent": "750", - "reputation": 394956111554767, - "rshares": 11518487255, - "voter": "kaylinart" - }, - { - "percent": "10000", - "reputation": 97717041, - "rshares": 20842325770, - "voter": "field" - }, - { - "percent": "10000", - "reputation": 1139583710641, - "rshares": 37662419603, - "voter": "ardina" - }, - { - "percent": "-10000", - "reputation": 877894048504689, - "rshares": -1554696067429, - "voter": "meesterboom" - }, - { - "percent": "750", - "reputation": 116064392747995, - "rshares": 2782825297, - "voter": "sokoloffa" - }, - { - "percent": "750", - "reputation": 9758823762159, - "rshares": 833946242, - "voter": "joshglen" - }, - { - "percent": "-10000", - "reputation": 25362757889840, - "rshares": -11381580926, - "voter": "sazbird" - }, - { - "percent": "2000", - "reputation": 83817649948971, - "rshares": 9058241057, - "voter": "sterlinluxan" - }, - { - "percent": "10000", - "reputation": 266498838207861, - "rshares": 1342193309769, - "voter": "timcliff" - }, - { - "percent": "10000", - "reputation": 8868395123281, - "rshares": 6025414964381, - "voter": "jphamer1" - }, - { - "percent": "1500", - "reputation": 29981921402, - "rshares": 930756655, - "voter": "randomblock1" - }, - { - "percent": "1800", - "reputation": 42492835245572, - "rshares": 5384651893, - "voter": "alchemage" - }, - { - "percent": "1100", - "reputation": 4901694902621, - "rshares": 1356359411, - "voter": "burntmd" - }, - { - "percent": "220", - "reputation": 281480979701034, - "rshares": 4601691025, - "voter": "johnvibes" - }, - { - "percent": "1700", - "reputation": 82486907404938, - "rshares": 27402669738, - "voter": "elamental" - }, - { - "percent": "550", - "reputation": 17992748433372, - "rshares": 1139959110, - "voter": "catherinebleish" - }, - { - "percent": "1700", - "reputation": 215380666736, - "rshares": 319643622816, - "voter": "netaterra" - }, - { - "percent": "550", - "reputation": 173585217066813, - "rshares": 16471164729, - "voter": "terrybrock" - }, - { - "percent": "385", - "reputation": 51948618902979, - "rshares": 2518533568, - "voter": "dannyshine" - }, - { - "percent": "165", - "reputation": 22707497662074, - "rshares": 552969911, - "voter": "brightstar" - }, - { - "percent": "1100", - "reputation": 471080239200252, - "rshares": 12523029104, - "voter": "dbroze" - }, - { - "percent": "10000", - "reputation": 262691650197556, - "rshares": 82152017956, - "voter": "titusfrost" - }, - { - "percent": "10000", - "reputation": 339523341647146, - "rshares": 510671612770, - "voter": "whatsup" - }, - { - "percent": "2300", - "reputation": 171005551503977, - "rshares": 2357266336, - "voter": "freebornangel" - }, - { - "percent": "10000", - "reputation": 244475173484259, - "rshares": 190001564304, - "voter": "nelyp" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 51932550768, - "voter": "ssekulji" - }, - { - "percent": "1100", - "reputation": 13729625239772, - "rshares": 34855911740, - "voter": "wearechange-co" - }, - { - "percent": "75", - "reputation": 104771506408830, - "rshares": 5046865287, - "voter": "gamer00" - }, - { - "percent": "550", - "reputation": 70201360432015, - "rshares": 6272641544, - "voter": "makinstuff" - }, - { - "percent": "10000", - "reputation": 942473876919, - "rshares": 675053836, - "voter": "mow" - }, - { - "percent": "10000", - "reputation": 52209749433588, - "rshares": 18751585536, - "voter": "loreennaa" - }, - { - "percent": "10000", - "reputation": 37741005622401, - "rshares": 1569234596, - "voter": "amryksr" - }, - { - "percent": "11", - "reputation": 1098231391901, - "rshares": 567936010, - "voter": "ambyr00" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 236180416079, - "voter": "teammo" - }, - { - "percent": "10000", - "reputation": 450338781685383, - "rshares": 102493961871, - "voter": "louisthomas" - }, - { - "percent": "600", - "reputation": 1614643030899, - "rshares": 1148856944, - "voter": "freebornsociety" - }, - { - "percent": "2000", - "reputation": 40716544458929, - "rshares": 626769370, - "voter": "haileyscomet" - }, - { - "percent": "500", - "reputation": 15135006110391, - "rshares": 1057652952, - "voter": "thatsweeneyguy" - }, - { - "percent": "10000", - "reputation": 74329552872641, - "rshares": 261502888510, - "voter": "mes" - }, - { - "percent": "400", - "reputation": 139091215313620, - "rshares": 53338854584, - "voter": "whatamidoing" - }, - { - "percent": "10000", - "reputation": 112994806497327, - "rshares": 1427983707, - "voter": "varunpinto" - }, - { - "percent": "5000", - "reputation": 80123076461352, - "rshares": 388815610548, - "voter": "xels" - }, - { - "percent": "10000", - "reputation": 91665477300645, - "rshares": 178086164639, - "voter": "galberto" - }, - { - "percent": "10000", - "reputation": 9701652715721, - "rshares": 129027913719, - "voter": "masterthematrix" - }, - { - "percent": "750", - "reputation": 21992421049352, - "rshares": 835156243, - "voter": "wishmaiden" - }, - { - "percent": "1100", - "reputation": 8429517470052, - "rshares": 1760986868, - "voter": "antimedia" - }, - { - "percent": "10000", - "reputation": 7901101123497, - "rshares": 1156556078088, - "voter": "giuatt07" - }, - { - "percent": "750", - "reputation": 2615028020818, - "rshares": 3070875885, - "voter": "zerotoone" - }, - { - "percent": "10000", - "reputation": 329877254452254, - "rshares": 8556817259, - "voter": "steeminator3000" - }, - { - "percent": "10000", - "reputation": 20355801093011, - "rshares": 471361276430, - "voter": "jingdol" - }, - { - "percent": "1300", - "reputation": 14221709557275, - "rshares": 2877953643, - "voter": "colinhoward" - }, - { - "percent": "500", - "reputation": 13450585898518, - "rshares": 2447883408, - "voter": "duckmast3r" - }, - { - "percent": "10000", - "reputation": 183137663743603, - "rshares": 257885059196, - "voter": "dexpartacus" - }, - { - "percent": "4500", - "reputation": 219366237221002, - "rshares": 263634890249, - "voter": "offgridlife" - }, - { - "percent": "1000", - "reputation": 68902318315273, - "rshares": 765085884, - "voter": "bien" - }, - { - "percent": "9400", - "reputation": 816902238265938, - "rshares": 335706372716, - "voter": "cryptopie" - }, - { - "percent": "1100", - "reputation": 67559076759827, - "rshares": 4757359112, - "voter": "bryandivisions" - }, - { - "percent": "220", - "reputation": 96424119267202, - "rshares": 1359735398, - "voter": "heart-to-heart" - }, - { - "percent": "1500", - "reputation": 91410383693752, - "rshares": 44399072853, - "voter": "vincentnijman" - }, - { - "percent": "2500", - "reputation": 55801543512306, - "rshares": 77586921251119, - "voter": "appreciator" - }, - { - "percent": "10000", - "reputation": 135586951873479, - "rshares": 114452290866, - "voter": "shrazi" - }, - { - "percent": "1000", - "reputation": 22755665714428, - "rshares": 2432033504, - "voter": "jonyoudyer" - }, - { - "percent": "750", - "reputation": 108130082943915, - "rshares": 3077426722, - "voter": "a-alice" - }, - { - "percent": "1100", - "reputation": 62879471706711, - "rshares": 3466050389482, - "voter": "tribesteemup" - }, - { - "percent": "10000", - "reputation": 72946432090481, - "rshares": 123355223723, - "voter": "my451r" - }, - { - "percent": "4840", - "reputation": 24639928209538, - "rshares": 26847315620, - "voter": "santigs" - }, - { - "percent": "10000", - "reputation": 11131064226204, - "rshares": 13176444194, - "voter": "angelusnoctum" - }, - { - "percent": "750", - "reputation": 11931129346110, - "rshares": 673948339, - "voter": "salim001" - }, - { - "percent": "-10000", - "reputation": 140483466255317, - "rshares": -645316267458, - "voter": "fbslo" - }, - { - "percent": "1100", - "reputation": 12362125628290, - "rshares": 2173733748, - "voter": "solarsupermama" - }, - { - "percent": "10000", - "reputation": 110750156547770, - "rshares": 418911152665, - "voter": "drax" - }, - { - "percent": "-10000", - "reputation": 445234100950820, - "rshares": -34936796609959, - "voter": "therealwolf" - }, - { - "percent": "10000", - "reputation": 1386913003857667, - "rshares": 3228135625142, - "voter": "taskmaster4450" - }, - { - "percent": "220", - "reputation": 336063014350538, - "rshares": 31861151613, - "voter": "revisesociology" - }, - { - "percent": "750", - "reputation": 22607491274241, - "rshares": 596560542, - "voter": "suheri" - }, - { - "percent": "10000", - "reputation": 20299637563719, - "rshares": 6003896245, - "voter": "critic-on" - }, - { - "percent": "500", - "reputation": 44234924510749, - "rshares": 6081952645, - "voter": "canadianrenegade" - }, - { - "percent": "830", - "reputation": 0, - "rshares": 340065393122, - "voter": "investegg" - }, - { - "percent": "2500", - "reputation": 141970828773777, - "rshares": 400268267260, - "voter": "tobetada" - }, - { - "percent": "3300", - "reputation": 948045752156, - "rshares": 1464145171, - "voter": "kieranpearson" - }, - { - "percent": "10000", - "reputation": 128158005695390, - "rshares": 63633997403, - "voter": "arabisouri" - }, - { - "percent": "1100", - "reputation": 59318242581030, - "rshares": 1599171435, - "voter": "nomad-magus" - }, - { - "percent": "550", - "reputation": 267507614382074, - "rshares": 24580672114, - "voter": "trucklife-family" - }, - { - "percent": "1100", - "reputation": 7796332445413, - "rshares": 2154997005, - "voter": "lishu" - }, - { - "percent": "-5000", - "reputation": 172804239020062, - "rshares": -181059864577, - "voter": "chekohler" - }, - { - "percent": "10000", - "reputation": 23846303818550, - "rshares": 4734859115, - "voter": "arindammroy" - }, - { - "percent": "1425", - "reputation": 6807030719811, - "rshares": 18146455306, - "voter": "danile666" - }, - { - "percent": "10000", - "reputation": 14709760292085, - "rshares": 17404139065, - "voter": "yazp" - }, - { - "percent": "275", - "reputation": 1010224399840, - "rshares": 562616150, - "voter": "belleamie" - }, - { - "percent": "-10000", - "reputation": 18150082730, - "rshares": -386572617388, - "voter": "citizensmith" - }, - { - "percent": "1100", - "reputation": 34321980459304, - "rshares": 7020500018, - "voter": "careywedler" - }, - { - "percent": "-10000", - "reputation": 35969154710476, - "rshares": -50757931807, - "voter": "b00m" - }, - { - "percent": "1000", - "reputation": 33651612530191, - "rshares": 667321201, - "voter": "bebeomega" - }, - { - "percent": "1000", - "reputation": 15033197516023, - "rshares": 655949080, - "voter": "naymur" - }, - { - "percent": "550", - "reputation": 218187411229, - "rshares": 802663713, - "voter": "firststeps" - }, - { - "percent": "288", - "reputation": 2675225025765, - "rshares": 832377906, - "voter": "fourfourfun" - }, - { - "percent": "517", - "reputation": 94326416009060, - "rshares": 6713692389, - "voter": "paradigmprospect" - }, - { - "percent": "3500", - "reputation": 100680995127079, - "rshares": 23209300153, - "voter": "krishool" - }, - { - "percent": "500", - "reputation": 37850353024191, - "rshares": 10267772680, - "voter": "eaglespirit" - }, - { - "percent": "550", - "reputation": 78729229063850, - "rshares": 15895856382, - "voter": "mountainjewel" - }, - { - "percent": "10000", - "reputation": 28704887249463, - "rshares": 114441391879982, - "voter": "upmewhale" - }, - { - "percent": "1500", - "reputation": 30267561775104, - "rshares": 1231907335, - "voter": "dynamicgreentk" - }, - { - "percent": "2000", - "reputation": 5232800097978, - "rshares": 1349304453, - "voter": "moxieme" - }, - { - "percent": "3000", - "reputation": 188713944759376, - "rshares": 26413574642, - "voter": "roadstories" - }, - { - "percent": "500", - "reputation": 292980730708468, - "rshares": 6800102126, - "voter": "bengy" - }, - { - "percent": "10000", - "reputation": 61733393150334, - "rshares": 125243796782, - "voter": "sidekickmatt" - }, - { - "percent": "5000", - "reputation": 184489319084, - "rshares": 544485199, - "voter": "steemboat-steve" - }, - { - "percent": "375", - "reputation": 8614577464152, - "rshares": 1018995292, - "voter": "thehoneys" - }, - { - "percent": "10000", - "reputation": 170235537022, - "rshares": 1410422723, - "voter": "auracraft" - }, - { - "percent": "8000", - "reputation": 53758992804448, - "rshares": 8311446672, - "voter": "gardenofcarmen" - }, - { - "percent": "1500", - "reputation": 12685053702515, - "rshares": 2068846097, - "voter": "kpopjera" - }, - { - "percent": "10000", - "reputation": 959567888850, - "rshares": 157597067813, - "voter": "nnaraoh" - }, - { - "percent": "10000", - "reputation": 11165935981106, - "rshares": 1076292042, - "voter": "icuz" - }, - { - "percent": "5000", - "reputation": 4156063258051, - "rshares": 1936031459, - "voter": "investprosper" - }, - { - "percent": "750", - "reputation": 5981462266033, - "rshares": 1004374840, - "voter": "yadah04" - }, - { - "percent": "3300", - "reputation": 330396781271113, - "rshares": 1466009449748, - "voter": "holger80" - }, - { - "percent": "10000", - "reputation": 154728059906539, - "rshares": 52529294991, - "voter": "trudeehunter" - }, - { - "percent": "10000", - "reputation": 2384160913530, - "rshares": 22081930797, - "voter": "blog-beginner" - }, - { - "percent": "550", - "reputation": 4348421965460, - "rshares": 857774786, - "voter": "borrowedearth" - }, - { - "percent": "1100", - "reputation": 1868454212319, - "rshares": 937283340, - "voter": "hempress" - }, - { - "percent": "10000", - "reputation": 12435393608248, - "rshares": 15120132136, - "voter": "jhoxiris" - }, - { - "percent": "5000", - "reputation": 15049076647839, - "rshares": 4194529894, - "voter": "kirito-freud" - }, - { - "percent": "10000", - "reputation": 17362520024307, - "rshares": 4663635727, - "voter": "bhattg" - }, - { - "percent": "10000", - "reputation": 8405881148978, - "rshares": 12144026154, - "voter": "thebluewin" - }, - { - "percent": "10000", - "reputation": 4895191598205, - "rshares": 4052644325, - "voter": "auminda" - }, - { - "percent": "2200", - "reputation": 10164569088184, - "rshares": 3395629839, - "voter": "vegan.niinja" - }, - { - "percent": "550", - "reputation": 65173331301128, - "rshares": 3281540495, - "voter": "homestead-guru" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 28297978775, - "voter": "futurecurrency" - }, - { - "percent": "300", - "reputation": 981068471716, - "rshares": 609642985, - "voter": "tamala" - }, - { - "percent": "750", - "reputation": 195976717034, - "rshares": 617451363, - "voter": "joshh71390" - }, - { - "percent": "363", - "reputation": 21691252850789, - "rshares": 1473739456, - "voter": "steemsmarter" - }, - { - "percent": "1250", - "reputation": 9524880395280, - "rshares": 1189760448, - "voter": "djoi" - }, - { - "percent": "1150", - "reputation": 126900854621, - "rshares": 731768962, - "voter": "antisocialists" - }, - { - "percent": "500", - "reputation": 12028991969777, - "rshares": 1267946403, - "voter": "evlachsblog" - }, - { - "percent": "700", - "reputation": 27788030222507, - "rshares": 29980695984, - "voter": "antisocialist" - }, - { - "percent": "1200", - "reputation": 102994813920170, - "rshares": 1215302469, - "voter": "movingman" - }, - { - "percent": "1000", - "reputation": 9979740504084, - "rshares": 1774137266, - "voter": "shaheerbari" - }, - { - "percent": "10000", - "reputation": 8147228251105, - "rshares": 75352321121, - "voter": "jocieprosza" - }, - { - "percent": "750", - "reputation": 54208883787930, - "rshares": 11113098873, - "voter": "outlinez" - }, - { - "percent": "2500", - "reputation": 1069508711711, - "rshares": 1165130561, - "voter": "greendo" - }, - { - "percent": "500", - "reputation": 55261387418883, - "rshares": 3410400907, - "voter": "scottshots" - }, - { - "percent": "242", - "reputation": 33393562665149, - "rshares": 1461600491, - "voter": "celestialcow" - }, - { - "percent": "55", - "reputation": 40558753792526, - "rshares": 917182706, - "voter": "sanderjansenart" - }, - { - "percent": "10000", - "reputation": 13852827798556, - "rshares": 1033413984239, - "voter": "dera123" - }, - { - "percent": "10000", - "reputation": 5238378681910, - "rshares": 28834111463, - "voter": "m2nnari" - }, - { - "percent": "1000", - "reputation": 86599313584641, - "rshares": 3586583580, - "voter": "bobaphet" - }, - { - "percent": "10000", - "reputation": 11722167805732, - "rshares": 29733901757, - "voter": "phasewalker" - }, - { - "percent": "10000", - "reputation": 37835744295387, - "rshares": 8738429792, - "voter": "gogreenbuddy" - }, - { - "percent": "10000", - "reputation": 27390685365705, - "rshares": 21576770046, - "voter": "tsnaks" - }, - { - "percent": "10000", - "reputation": 99020284852233, - "rshares": 6124575301, - "voter": "lorenzopistolesi" - }, - { - "percent": "250", - "reputation": 454088646937365, - "rshares": 7840852481, - "voter": "kgakakillerg" - }, - { - "percent": "10000", - "reputation": 80339022404959, - "rshares": 69052737441, - "voter": "yonilkar" - }, - { - "percent": "1500", - "reputation": 19547161660934, - "rshares": 1215760718, - "voter": "msearles" - }, - { - "percent": "550", - "reputation": 162917506903765, - "rshares": 19759437966, - "voter": "artemislives" - }, - { - "percent": "550", - "reputation": 77476461100689, - "rshares": 21753534515, - "voter": "indigoocean" - }, - { - "percent": "750", - "reputation": 18193153151295, - "rshares": 5398677445, - "voter": "a1-shroom-spores" - }, - { - "percent": "1155", - "reputation": 9020628089607, - "rshares": 10514917744, - "voter": "promobot" - }, - { - "percent": "800", - "reputation": 11571764790123, - "rshares": 1593977580, - "voter": "ikarus56" - }, - { - "percent": "5400", - "reputation": 434858705218015, - "rshares": 92563181292, - "voter": "hafizullah" - }, - { - "percent": "2200", - "reputation": 51236835710173, - "rshares": 10125360314, - "voter": "davidesimoncini" - }, - { - "percent": "750", - "reputation": 27284305279794, - "rshares": 894515376, - "voter": "rachelleignacio" - }, - { - "percent": "750", - "reputation": 5394497796590, - "rshares": 885339348, - "voter": "adam.tran" - }, - { - "percent": "550", - "reputation": 1357573929274, - "rshares": 644171405, - "voter": "cambridgeport90" - }, - { - "percent": "550", - "reputation": 158239032685507, - "rshares": 588241480, - "voter": "metama" - }, - { - "percent": "5000", - "reputation": 18169458188110, - "rshares": 20932997171, - "voter": "simplegame" - }, - { - "percent": "-5000", - "reputation": 244842259743, - "rshares": -2046646308, - "voter": "ihal0001" - }, - { - "percent": "2500", - "reputation": 63239211262012, - "rshares": 22142392389, - "voter": "marblely" - }, - { - "percent": "165", - "reputation": 22556964504537, - "rshares": 30043624426, - "voter": "inspirewithwords" - }, - { - "percent": "1500", - "reputation": 10563258681111, - "rshares": 1840999194, - "voter": "dynamicsteemians" - }, - { - "percent": "750", - "reputation": 2013148972137, - "rshares": 750924896, - "voter": "steemian258" - }, - { - "percent": "10000", - "reputation": 65268642593339, - "rshares": 44447355585, - "voter": "sabari18" - }, - { - "percent": "10000", - "reputation": 84809016946227, - "rshares": 14199931763, - "voter": "kapitanrosomak" - }, - { - "percent": "3500", - "reputation": 37902408766598, - "rshares": 118779735858, - "voter": "cmplxty" - }, - { - "percent": "225", - "reputation": 32187725403162, - "rshares": 1758432255, - "voter": "angelica7" - }, - { - "percent": "10000", - "reputation": 1342046775688, - "rshares": 4096723170, - "voter": "ridor5301" - }, - { - "percent": "3000", - "reputation": 3794066899821, - "rshares": 859548505, - "voter": "sr-nikon" - }, - { - "percent": "750", - "reputation": 12515201868146, - "rshares": 1631565399, - "voter": "honeycup-waters" - }, - { - "percent": "10000", - "reputation": 62302307783, - "rshares": 28201143498, - "voter": "pvinny69" - }, - { - "percent": "-10000", - "reputation": 0, - "rshares": -1318646727, - "voter": "gorbisan" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 187442795, - "voter": "iconode" - }, - { - "percent": "3300", - "reputation": 36498710441788, - "rshares": 10710685311, - "voter": "fullnodeupdate" - }, - { - "percent": "2000", - "reputation": 144361338080419, - "rshares": 14300084648, - "voter": "porters" - }, - { - "percent": "250", - "reputation": 48728351683805, - "rshares": 7174812867, - "voter": "starstrings01" - }, - { - "percent": "450", - "reputation": 9613565810161, - "rshares": 562957891, - "voter": "praditya" - }, - { - "percent": "10000", - "reputation": 10278138043955, - "rshares": 9589612, - "voter": "laissez-faire" - }, - { - "percent": "5000", - "reputation": 6660418695187, - "rshares": 1358912484, - "voter": "steemituplife" - }, - { - "percent": "10000", - "reputation": 45236011216729, - "rshares": 81202280023, - "voter": "cultus-forex" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 13467440649, - "voter": "quediceharry" - }, - { - "percent": "5000", - "reputation": 6687049412835, - "rshares": 41488529186, - "voter": "mister-meeseeks" - }, - { - "percent": "750", - "reputation": 60790537367735, - "rshares": 7332116519, - "voter": "the.success.club" - }, - { - "percent": "10000", - "reputation": 1648086760085, - "rshares": 29736477900, - "voter": "diegor" - }, - { - "percent": "-5000", - "reputation": 185520053513587, - "rshares": -717003206715, - "voter": "brianoflondon" - }, - { - "percent": "1250", - "reputation": 570615134196274, - "rshares": 52371949929, - "voter": "priyanarc" - }, - { - "percent": "750", - "reputation": 57675290515578, - "rshares": 677473470, - "voter": "pushpedal" - }, - { - "percent": "1250", - "reputation": 56881377417174, - "rshares": 2329013647, - "voter": "miguelbaez" - }, - { - "percent": "10000", - "reputation": 539147053023232, - "rshares": 49346571253122, - "voter": "theycallmedan" - }, - { - "percent": "1500", - "reputation": 25418920757486, - "rshares": 3536716643, - "voter": "vibesforlife" - }, - { - "percent": "550", - "reputation": 13343612534181, - "rshares": 840932890, - "voter": "bia.birch" - }, - { - "percent": "1000", - "reputation": 2152680611464, - "rshares": 655432322, - "voter": "hedidylan" - }, - { - "percent": "10000", - "reputation": 5927670145042, - "rshares": 25790701980, - "voter": "northmountain" - }, - { - "percent": "10000", - "reputation": 7476997614820, - "rshares": 161283228788, - "voter": "johan.norberg" - }, - { - "percent": "1100", - "reputation": 1575698742, - "rshares": 21872601292, - "voter": "tribevibes" - }, - { - "percent": "10000", - "reputation": 21122210064846, - "rshares": 20941836860, - "voter": "vickyguevara" - }, - { - "percent": "10000", - "reputation": 75911580, - "rshares": 171493122042, - "voter": "jamesbattler" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 190929901, - "voter": "amityconcord" - }, - { - "percent": "4000", - "reputation": 16012295789643, - "rshares": 3659212304, - "voter": "cooperfelix" - }, - { - "percent": "10000", - "reputation": 25016264134313, - "rshares": 659362197063, - "voter": "dcommerce" - }, - { - "percent": "550", - "reputation": 21703325577576, - "rshares": 1455747037, - "voter": "bewithbreath" - }, - { - "percent": "10000", - "reputation": 2910555091868, - "rshares": 997187990, - "voter": "denizcakmak" - }, - { - "percent": "750", - "reputation": 14812725521919, - "rshares": 4387200562, - "voter": "filosof103" - }, - { - "percent": "10000", - "reputation": 161840569289533, - "rshares": 112766553856, - "voter": "engrsayful" - }, - { - "percent": "10000", - "reputation": 64248408550752, - "rshares": 35041922457, - "voter": "qbas116" - }, - { - "percent": "2000", - "reputation": 2308553479395, - "rshares": 2742202798, - "voter": "steelborne" - }, - { - "percent": "2500", - "reputation": 61476958391321, - "rshares": 1205423521, - "voter": "ricardomello" - }, - { - "percent": "550", - "reputation": 152169516576120, - "rshares": 3784685496, - "voter": "deeanndmathews" - }, - { - "percent": "1155", - "reputation": 36087507440149, - "rshares": 94538877814, - "voter": "likwid" - }, - { - "percent": "125", - "reputation": 20916803594387, - "rshares": 547938275, - "voter": "leighscotford" - }, - { - "percent": "10000", - "reputation": 499450701404, - "rshares": 552677665, - "voter": "iktisat" - }, - { - "percent": "880", - "reputation": 0, - "rshares": 11702352977, - "voter": "haleakala" - }, - { - "percent": "5000", - "reputation": 1138890453375, - "rshares": 3253203526, - "voter": "iamjohn" - }, - { - "percent": "750", - "reputation": 3178044276987, - "rshares": 728478943, - "voter": "psyo" - }, - { - "percent": "3500", - "reputation": 9742808963304, - "rshares": 8043315303, - "voter": "ssiena" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 1862857552, - "voter": "tiffin" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 20020619572, - "voter": "good.game" - }, - { - "percent": "4000", - "reputation": 9779686833688, - "rshares": 5544179599, - "voter": "valerianis" - }, - { - "percent": "858", - "reputation": 236888057, - "rshares": 5025871607, - "voter": "psycultureradio" - }, - { - "percent": "-5000", - "reputation": 975591177021, - "rshares": -4210787933, - "voter": "drlobes" - }, - { - "percent": "10000", - "reputation": 1146189014563, - "rshares": 896869316, - "voter": "urun" - }, - { - "percent": "10000", - "reputation": 11547503414, - "rshares": 571598003, - "voter": "the.unicorn" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 530768665, - "voter": "roadstories.trib" - }, - { - "percent": "88", - "reputation": 43374515375527, - "rshares": 1983727538, - "voter": "build-it" - }, - { - "percent": "1250", - "reputation": 62988003579804, - "rshares": 4062714263, - "voter": "zeesh" - }, - { - "percent": "10000", - "reputation": 860847639115, - "rshares": 970807653, - "voter": "pogarda" - }, - { - "percent": "1700", - "reputation": 0, - "rshares": 1348349099, - "voter": "therealyme" - }, - { - "percent": "-5000", - "reputation": 49705529506199, - "rshares": -37378460892, - "voter": "fenngen" - }, - { - "percent": "10000", - "reputation": 59827171041931, - "rshares": 43795567, - "voter": "fearlessgu" - }, - { - "percent": "-10000", - "reputation": 0, - "rshares": -465867807152, - "voter": "azabu" - }, - { - "percent": "1500", - "reputation": 15076530384277, - "rshares": 16533756991, - "voter": "oblivioncubed" - }, - { - "percent": "-10000", - "reputation": 0, - "rshares": -461383770903, - "voter": "acram64" - }, - { - "percent": "-10000", - "reputation": 0, - "rshares": -397457878804, - "voter": "willowranger" - }, - { - "percent": "2500", - "reputation": 336154498730, - "rshares": 1580352755, - "voter": "marblesz" - }, - { - "percent": "5000", - "reputation": 32542453022, - "rshares": 5069570657, - "voter": "bilpcoinbpc" - }, - { - "percent": "10000", - "reputation": 76222060731, - "rshares": 1137775553, - "voter": "wallytiteuf" - }, - { - "percent": "-5000", - "reputation": 2934995178664, - "rshares": -31308469857, - "voter": "vxn666" - }, - { - "percent": "1000", - "reputation": 4040555687270, - "rshares": 1070706077, - "voter": "theisacoin" - }, - { - "percent": "10000", - "reputation": 362096342263, - "rshares": 402287485, - "voter": "sujaytechnicals" - }, - { - "percent": "750", - "reputation": 667490902327, - "rshares": 545676972, - "voter": "groove-logic" - }, - { - "percent": "4800", - "reputation": 86868289888522, - "rshares": 919451952704, - "voter": "peakd" - }, - { - "percent": "5000", - "reputation": 4912339327248, - "rshares": 38500985255, - "voter": "hiveqa" - }, - { - "percent": "10000", - "reputation": 47815067431587, - "rshares": 27653657749, - "voter": "luckyali" - }, - { - "percent": "4000", - "reputation": 3242356575612, - "rshares": 7910660980, - "voter": "walarhein" - }, - { - "percent": "-10000", - "reputation": 2837344243508, - "rshares": -211721357000, - "voter": "hivetrending" - }, - { - "percent": "1100", - "reputation": 85827117950379, - "rshares": 42747632547, - "voter": "abundance.tribe" - }, - { - "percent": "5630", - "reputation": 1209731647511, - "rshares": 953813061268, - "voter": "softworld" - }, - { - "percent": "10000", - "reputation": 6644296348943, - "rshares": 2417787565, - "voter": "tahsination" - }, - { - "percent": "10000", - "reputation": 631765969428, - "rshares": 31025769508, - "voter": "hiveshout" - }, - { - "percent": "1000", - "reputation": 661148949025, - "rshares": 552379314, - "voter": "gradeon" - }, - { - "percent": "1000", - "reputation": 3177222978373, - "rshares": 9184280924, - "voter": "hivelist" - }, - { - "percent": "5000", - "reputation": 604442758770, - "rshares": 224391167226, - "voter": "ninnu" - }, - { - "percent": "37", - "reputation": 0, - "rshares": 1436849755, - "voter": "kiemis" - }, - { - "percent": "10000", - "reputation": 32381994985806, - "rshares": 37588956668, - "voter": "yolimarag" - }, - { - "percent": "10000", - "reputation": 14767283059507, - "rshares": 14769143684, - "voter": "cieliss" - }, - { - "percent": "10000", - "reputation": 429763208250, - "rshares": 4712535002, - "voter": "ghaazi" - }, - { - "percent": "10000", - "reputation": 19240829356115, - "rshares": 7922252658, - "voter": "diego1306" - }, - { - "percent": "10000", - "reputation": 30660771321168, - "rshares": 18786575096, - "voter": "sultan-indo" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "zarevaneli" - }, - { - "percent": "7000", - "reputation": 29579801503382, - "rshares": 13405030905, - "voter": "maleklopez" - }, - { - "percent": "10000", - "reputation": 1289890269498, - "rshares": 842621649, - "voter": "greengalletti" - }, - { - "percent": "10000", - "reputation": 65519590598, - "rshares": 1938256210, - "voter": "plusvault" - }, - { - "percent": "605", - "reputation": 5991812078235, - "rshares": 1402616810, - "voter": "anafae" - }, - { - "percent": "10000", - "reputation": 17804701, - "rshares": 5770983881, - "voter": "notaboutme" - }, - { - "percent": "10000", - "reputation": 7273089567, - "rshares": 1347591656, - "voter": "hivecoffee" - }, - { - "percent": "10000", - "reputation": 2830639099232, - "rshares": 96312733, - "voter": "koxmicart" - }, - { - "percent": "10000", - "reputation": 3196812936473, - "rshares": 3052620925, - "voter": "sants1311" - }, - { - "percent": "10000", - "reputation": 7813514868027, - "rshares": 6903012674, - "voter": "francielis" - }, - { - "percent": "10000", - "reputation": 6535791110640, - "rshares": 4504206328, - "voter": "cryptoanalist" - }, - { - "percent": "10000", - "reputation": 5513884073608, - "rshares": 3748331234, - "voter": "blackheart1" - }, - { - "percent": "150", - "reputation": 12098748930881, - "rshares": 1797040061, - "voter": "questcrypto" - }, - { - "percent": "10000", - "reputation": 1607006115905, - "rshares": 2508773907, - "voter": "sony101" - }, - { - "percent": "4000", - "reputation": 2662893985125, - "rshares": 8033789630, - "voter": "chaodietas" - }, - { - "percent": "5000", - "reputation": 7330275018374, - "rshares": 2865647190, - "voter": "tinta-tertuang" - }, - { - "percent": "10000", - "reputation": 2047210744537, - "rshares": 2350630625, - "voter": "moesa" - }, - { - "percent": "-10000", - "reputation": 0, - "rshares": -17479608280433, - "voter": "usainvote" - }, - { - "percent": "10000", - "reputation": 3702763181424, - "rshares": 2142847183, - "voter": "youngwild" - }, - { - "percent": "5000", - "reputation": 102092235719, - "rshares": 907648040, - "voter": "clifth" - }, - { - "percent": "10000", - "reputation": 86405531104, - "rshares": 1925047663, - "voter": "text2speech" - }, - { - "percent": "10000", - "reputation": 112363590278, - "rshares": 47497737, - "voter": "dango1411" - }, - { - "percent": "10000", - "reputation": 270295677528, - "rshares": 243825679, - "voter": "hauptfleisch" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "sameer78" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "artx" - }, - { - "percent": "10000", - "reputation": 3594869451, - "rshares": 0, - "voter": "mythcrusher" - } - ], - "author": "goatgang", - "author_reputation": 2937670098475, - "beneficiaries": [], - "body": "(GGP) GoatGang Growth Proposal for the HIVE ecosystem - Enabling Hive Help Desk Administration and Contest Management. \n\nOnly a tiny fraction of the total population of the world is currently using Blockchain technology. So, instead of focusing on getting crypto users from other platforms to visit Hive - we plan to tap into the non-crypto world to have those users join us and experience the crypto side.\n\nHow do we do that? By Giving incentives so they can use the primary feature sets that give Hive its unique value proposition. Making users feel comfortable within the HIVE ecosystem and help them engage in the field they are confident in. While, expanding their experience deeper into the Hive ecosystem with learning material and supportive community engagement.\n\n---\n\nThis proposal is to establish a long-term funding mechanism to increase and reward the work of Hive community members dedicating time and effort to promote awareness, understanding, and adoption. Community participants will construct the full spectrum of content that will capture the attention of users of traditional centralized social networks. GGP funding will provide the resources to design and implement a marketing campaign powered by community generated content. Additionally, the proposal seeks to fund users administering and contributing content to the Hive help desk. This initiative aims to enhance the adoption and onboarding of new users. This would be achieved by creating and making readily available a series of educational materials and best practice guidelines, explaining how to access, navigate and interact with the Hive ecosystem and feature set.\n\nHow will these proposals benefit the Hive Community?\n\n**Step 1:** Creation of events targeting non-crypto users - The bigger the prize pool, the bigger the attention it will gain.\n\n**Step 2:** Have them join the GOAT Gang and introduce them to the crypto world - The well versed and experienced members will help them understand all there is to crypto, both gaming wise and as a social tool.\n\n**Step 3:** Help them on every step as they explore - We will help them create accounts and provide them the best tools and knowledge about Hive and how to use it correctly.\n\n**Step 4:** Paid in crypto - As we proceed with many contests, giveaways and events we slowly help users engage with the crypto world by paying the prizes in HIVE. The idea of earning will make trying the Hive platform more appealing and having some HIVE will make it accessible, reducing barriers to entry.\n\nGrowth Potential: it's equal to the population of non-crypto users, so it's just huge!\n\n**Our Target Audience:**\nArtists, video streamers, bloggers, designers, content writers, gamers. Basically everyone active\n\n**Artists:** They can get rewarded for their graphic design contributions.\n**Video streamers:** They attract a lot of gamers. Goat Gang Contests will make them stream on Hive. They would additionally enjoy the fact that each of their streams can earn them crypto, something most people have not experienced.\nGamers: The traditional gaming industry is worth billions. Gamers put in a lot of money without any guarantee of proper returns. We will have them informed about the advantages of crypto-gaming and how their investments in games can actually pay off.\n**Bloggers:** Every experience can be shared and the best part is that it can generate income. This prospect will have more active bloggers and Hive will grow.\n**Redditors:** Reddit medium and other traditional markets will be our strong focus. They have a very active community and having them experience Hive would be very beneficial.\n\n**Everyone:** The crypto world is so diverse that everyone could find a niche and earn while doing so. As we, the GOAT Gang, increase our reach, more and more people will be able to experience the Hive blockchain.\n\n**About the marketing campaign:**\n\nThe target group of this campaign is traditional users of centralized social networks, with an emphasis on gamers and bloggers. The objective is to increase this group\u2019s interest in adopting blockchain-based social networks, ultimately, to raise awareness among traditional game players operating on legacy platforms; we want those users to join the Hive ecosystem.\n\nWe will achieve that by showing the benefits provided by crypto gaming and blogging platforms. During the first phase of the campaign, we will target the following marketing channels: \n- Reddit (targeted to specific subreddits)\n- Steam\n- Twitch\n- Discord\n\nWhen the first phase of the contests are complete, there will be a second phase where we will target the following traditional social networks and blogging platforms:\n- Medium\n- Youtube\n- Twitter\n\nWhen a reasonable level of participation during the second phase can be determined in parallel, we will start running the third phase expansion. During this phase, we will target the leading game console communities through a new series of contests specifically designed for engagement with such platforms.\n- Playstation Network\n- Xbox Live\n\nOur support systems and personnel are currently being expanded, so that with funding assistance will be available continuously and 24/7, based on an inclusive policy, and with diverse languages and chatbot translators. This enhanced system will help new users with the on-boarding process, while also providing new promotional materials for users actively promoting Hive adoption.\n\nLearn more about our efforts already underway [here](https://bit.ly/GOATGang)\nRead about the contest structure [here](https://docs.google.com/spreadsheets/d/1dIY_w1lA-DI_DMsKWUDT67M_sPhOplMLkgdO4VioieA/edit?usp=sharing).\n\nIn order for us to be able to provide the necessary resources to design and implement these persistent marketing campaigns, support team management, for the adoption and on-boarding of new users, for prizes to enable the proliferation and disbursement of educational materials.\n\nShow your support for growing Hive and VOTE GOAT!\n\nPrize system for user retention: https://peakd.com/proposals/136\n\nGrowth proposal for the Hive ecosystem: https://peakd.com/proposals/135 \n", - "body_length": 6125, - "cashout_time": "2020-11-06T16:01:39", - "category": "hive-116702", - "children": 29, - "created": "2020-10-30T16:01:39", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"app\":\"peakd/2020.10.9\",\"format\":\"markdown\",\"tags\":[\"hive\",\"growth\",\"proposal\",\"goat\",\"gang\",\"ecosystem\",\"contest\",\"administration\",\"management\"],\"links\":[\"https://bit.ly/GOATGang\",\"https://docs.google.com/spreadsheets/d/1dIY_w1lA-DI_DMsKWUDT67M_sPhOplMLkgdO4VioieA/edit?usp=sharing\",\"https://peakd.com/proposals/136\",\"https://peakd.com/proposals/135\"],\"image\":[]}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-10-30T19:08:36", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 224003887683307, - "parent_author": "", - "parent_permlink": "hive-116702", - "pending_payout_value": "52.707 HBD", - "percent_hbd": 10000, - "permlink": "goatgang-growth-proposal-for-the-hive-ecosystem", - "post_id": 100319940, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "GoatGang Growth Proposal for the HIVE ecosystem", - "title": "GoatGang Growth Proposal for the HIVE ecosystem", - "total_payout_value": "0.000 HBD", - "url": "/hive-116702/@goatgang/goatgang-growth-proposal-for-the-hive-ecosystem" - }, - { - "active_votes": [ - { - "percent": "1500", - "reputation": 520861791056580, - "rshares": 22944567471656, - "voter": "blocktrades" - }, - { - "percent": "75", - "reputation": 19245698180508, - "rshares": 16223536179, - "voter": "tombstone" - }, - { - "percent": "10000", - "reputation": 211696269298912, - "rshares": 7918267794391, - "voter": "pfunk" - }, - { - "percent": "4000", - "reputation": 1179169121785, - "rshares": 4353076214, - "voter": "rarepepe" - }, - { - "percent": "10000", - "reputation": 1594304819447449, - "rshares": 10855500867080, - "voter": "acidyo" - }, - { - "percent": "45", - "reputation": 109424005488868, - "rshares": 2454806996, - "voter": "mammasitta" - }, - { - "percent": "150", - "reputation": 20148016993603, - "rshares": 25922488229, - "voter": "gerber" - }, - { - "percent": "5000", - "reputation": 54984589934, - "rshares": 526430365, - "voter": "kosimoos" - }, - { - "percent": "375", - "reputation": 352970428645376, - "rshares": 287043329201, - "voter": "roelandp" - }, - { - "percent": "800", - "reputation": 68491352821982, - "rshares": 52555135657, - "voter": "daan" - }, - { - "percent": "150", - "reputation": 639040633115794, - "rshares": 99135282284, - "voter": "ezzy" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 300835418714, - "voter": "livingfree" - }, - { - "percent": "400", - "reputation": 169354445790807, - "rshares": 3291022771, - "voter": "donatello" - }, - { - "percent": "300", - "reputation": 315496990256842, - "rshares": 165380659074, - "voter": "arcange" - }, - { - "percent": "5000", - "reputation": 398433936014047, - "rshares": 32233671974, - "voter": "xpilar" - }, - { - "percent": "150", - "reputation": 1231399052746489, - "rshares": 59243400643, - "voter": "exyle" - }, - { - "percent": "1237", - "reputation": 76598598706463, - "rshares": 9127728830, - "voter": "sharker" - }, - { - "percent": "10000", - "reputation": 116064392747995, - "rshares": 38384584206, - "voter": "sokoloffa" - }, - { - "percent": "300", - "reputation": 501315151365, - "rshares": 3120559562, - "voter": "raphaelle" - }, - { - "percent": "5000", - "reputation": 9758823762159, - "rshares": 5932115917, - "voter": "joshglen" - }, - { - "percent": "1200", - "reputation": 560943007638090, - "rshares": 445635764767, - "voter": "ace108" - }, - { - "percent": "1375", - "reputation": 5762427931179, - "rshares": 4507314375, - "voter": "kibela" - }, - { - "percent": "10000", - "reputation": 266498838207861, - "rshares": 1443965404589, - "voter": "timcliff" - }, - { - "percent": "1000", - "reputation": 13042944822941, - "rshares": 1161101407, - "voter": "alinalazareva" - }, - { - "percent": "1000", - "reputation": 3832784276078, - "rshares": 1301417497, - "voter": "djennyfloro" - }, - { - "percent": "40", - "reputation": 316730231019855, - "rshares": 2095332448, - "voter": "bert0" - }, - { - "percent": "2500", - "reputation": 8401560618909, - "rshares": 8267052056, - "voter": "anech512" - }, - { - "percent": "10000", - "reputation": 13943622727458, - "rshares": 17921444899, - "voter": "thedesertlynx" - }, - { - "percent": "2500", - "reputation": 139814057263648, - "rshares": 31079177237, - "voter": "themanualbot" - }, - { - "percent": "150", - "reputation": 96871175157544, - "rshares": 30683918894, - "voter": "someguy123" - }, - { - "percent": "1500", - "reputation": 736102194542370, - "rshares": 620652318039, - "voter": "daveks" - }, - { - "percent": "10000", - "reputation": 262691650197556, - "rshares": 172001148317, - "voter": "titusfrost" - }, - { - "percent": "1500", - "reputation": 144108882515293, - "rshares": 121616154432, - "voter": "petrvl" - }, - { - "percent": "2000", - "reputation": 706874908331117, - "rshares": 513956886577, - "voter": "abh12345" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 52852980508, - "voter": "ssekulji" - }, - { - "percent": "5000", - "reputation": 121575052614664, - "rshares": 53982527828, - "voter": "vannour" - }, - { - "percent": "5000", - "reputation": 121977551389360, - "rshares": 7126099914, - "voter": "elias15g" - }, - { - "percent": "5000", - "reputation": 8998674935359, - "rshares": 654717527, - "voter": "steemcultures" - }, - { - "percent": "10000", - "reputation": 371604430408116, - "rshares": 2305203518147, - "voter": "nonameslefttouse" - }, - { - "percent": "5000", - "reputation": 1140777865558, - "rshares": 2145326656, - "voter": "steemworld" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 718989623694, - "voter": "created" - }, - { - "percent": "5000", - "reputation": 2100858010527, - "rshares": 680833132, - "voter": "creat" - }, - { - "percent": "2500", - "reputation": 16372175688451, - "rshares": 965509022, - "voter": "mapesa" - }, - { - "percent": "2000", - "reputation": 36357535837745, - "rshares": 1357174055, - "voter": "doodleman" - }, - { - "percent": "5000", - "reputation": 8910166842489, - "rshares": 671774141, - "voter": "cisah" - }, - { - "percent": "5000", - "reputation": 137759377200970, - "rshares": 35798297304, - "voter": "yadamaniart" - }, - { - "percent": "5000", - "reputation": 37769232724831, - "rshares": 93742206733, - "voter": "ixindamix" - }, - { - "percent": "5000", - "reputation": 708133861814, - "rshares": 1353909986, - "voter": "pedir-museum" - }, - { - "percent": "3000", - "reputation": 59094884211730, - "rshares": 4457609448, - "voter": "saleg25" - }, - { - "percent": "95", - "reputation": 11219538466960, - "rshares": 592382483, - "voter": "lastminuteman" - }, - { - "percent": "10000", - "reputation": 88477817076925, - "rshares": 94218583625, - "voter": "driptorchpress" - }, - { - "percent": "1160", - "reputation": 7177514553823, - "rshares": 24764775336, - "voter": "gabriele-gio" - }, - { - "percent": "5000", - "reputation": 27214240242904, - "rshares": 42152966155, - "voter": "rahul.stan" - }, - { - "percent": "2000", - "reputation": 26597553649648, - "rshares": 3124505845, - "voter": "mauriciozoch" - }, - { - "percent": "5000", - "reputation": 60770071429, - "rshares": 743441789, - "voter": "aceh" - }, - { - "percent": "200", - "reputation": 38975615169260, - "rshares": 5146083022, - "voter": "steemitboard" - }, - { - "percent": "7200", - "reputation": 96863592771723, - "rshares": 83820421582, - "voter": "noemilunastorta" - }, - { - "percent": "300", - "reputation": 24653813359294, - "rshares": 2821279348, - "voter": "privex" - }, - { - "percent": "500", - "reputation": 1098231391901, - "rshares": 27876067889, - "voter": "ambyr00" - }, - { - "percent": "5000", - "reputation": 5311084723081, - "rshares": 1338824795, - "voter": "yuslindwi" - }, - { - "percent": "10000", - "reputation": 200440810412328, - "rshares": 98562446880, - "voter": "gric" - }, - { - "percent": "10000", - "reputation": 1199149180987, - "rshares": 43502782283380, - "voter": "ranchorelaxo" - }, - { - "percent": "1000", - "reputation": 17465905454847, - "rshares": 1102643980, - "voter": "bloghound" - }, - { - "percent": "10000", - "reputation": 450338781685383, - "rshares": 132868588165, - "voter": "louisthomas" - }, - { - "percent": "10000", - "reputation": 153925847897756, - "rshares": 939283453229, - "voter": "preparedwombat" - }, - { - "percent": "10000", - "reputation": 307916617000098, - "rshares": 1033460823348, - "voter": "detlev" - }, - { - "percent": "300", - "reputation": 163127928998530, - "rshares": 6843071849, - "voter": "lizanomadsoul" - }, - { - "percent": "5000", - "reputation": 295913411385, - "rshares": 768590313, - "voter": "munzir" - }, - { - "percent": "2320", - "reputation": 62578995648516, - "rshares": 36322726565, - "voter": "zaragast" - }, - { - "percent": "150", - "reputation": 21701614328748, - "rshares": 11163153533, - "voter": "dune69" - }, - { - "percent": "5000", - "reputation": 35475928556261, - "rshares": 181630488883, - "voter": "federacion45" - }, - { - "percent": "10000", - "reputation": 1800183893618, - "rshares": 2652762748, - "voter": "ninyea" - }, - { - "percent": "10000", - "reputation": 89582114994256, - "rshares": 31251381362, - "voter": "melooo182" - }, - { - "percent": "2320", - "reputation": 79234586811944, - "rshares": 69250091744, - "voter": "thenightflier" - }, - { - "percent": "10000", - "reputation": 813135864401476, - "rshares": 13486415019200, - "voter": "broncnutz" - }, - { - "percent": "93", - "reputation": 39969242565947, - "rshares": 1662313510, - "voter": "iansart" - }, - { - "percent": "10000", - "reputation": 1200997544863, - "rshares": 71976546000, - "voter": "bosman" - }, - { - "percent": "75", - "reputation": 32595690215621, - "rshares": 5946766549, - "voter": "forykw" - }, - { - "percent": "50", - "reputation": 358172961752718, - "rshares": 7162676839, - "voter": "jerrybanfield" - }, - { - "percent": "187", - "reputation": 11448902903972, - "rshares": 93672334353, - "voter": "roomservice" - }, - { - "percent": "1500", - "reputation": 29513750536324, - "rshares": 871898142, - "voter": "kennyroy" - }, - { - "percent": "1000", - "reputation": 174399448898803, - "rshares": 19723113331, - "voter": "aleister" - }, - { - "percent": "10000", - "reputation": 694242244776583, - "rshares": 242696978503, - "voter": "eveuncovered" - }, - { - "percent": "10000", - "reputation": 112994806497327, - "rshares": 3359031682, - "voter": "varunpinto" - }, - { - "percent": "1000", - "reputation": 19001680286018, - "rshares": 1207756497, - "voter": "rycharde" - }, - { - "percent": "1000", - "reputation": 77602585289853, - "rshares": 7405215359, - "voter": "arrliinn" - }, - { - "percent": "1500", - "reputation": 3553102205525, - "rshares": 21069769346, - "voter": "belahejna" - }, - { - "percent": "5000", - "reputation": 783744181388, - "rshares": 1033188042, - "voter": "slefesteem" - }, - { - "percent": "1600", - "reputation": 104292943123675, - "rshares": 46651431534, - "voter": "bearone" - }, - { - "percent": "135", - "reputation": 23577497062186, - "rshares": 30522044827, - "voter": "alphacore" - }, - { - "percent": "2900", - "reputation": 655474560066583, - "rshares": 646170482974, - "voter": "galenkp" - }, - { - "percent": "2500", - "reputation": 36400689869483, - "rshares": 122720419610, - "voter": "maxer27" - }, - { - "percent": "6500", - "reputation": 94117611307146, - "rshares": 145083467652, - "voter": "ammonite" - }, - { - "percent": "2000", - "reputation": 6025146240290, - "rshares": 1529637802, - "voter": "bellatravelph" - }, - { - "percent": "10000", - "reputation": 15824477347297, - "rshares": 15107712936, - "voter": "tracer-paulo" - }, - { - "percent": "10000", - "reputation": 5614961144204, - "rshares": 43417024782, - "voter": "raizel" - }, - { - "percent": "400", - "reputation": 120327041068001, - "rshares": 13762371794, - "voter": "jayna" - }, - { - "percent": "900", - "reputation": 122017350265257, - "rshares": 190683722856, - "voter": "ew-and-patterns" - }, - { - "percent": "1000", - "reputation": 7905346917299, - "rshares": 3649128659, - "voter": "monkeypattycake" - }, - { - "percent": "10000", - "reputation": 25623234518181, - "rshares": 32998723701, - "voter": "theia7" - }, - { - "percent": "10000", - "reputation": 71417124465576, - "rshares": 79307642557, - "voter": "erangvee" - }, - { - "percent": "10000", - "reputation": 1734633072808, - "rshares": 9255245097, - "voter": "bruzzy" - }, - { - "percent": "5000", - "reputation": 1494763095243, - "rshares": 1751467091, - "voter": "jamiz" - }, - { - "percent": "800", - "reputation": 0, - "rshares": 370099756983, - "voter": "gunthertopp" - }, - { - "percent": "1000", - "reputation": 20285621918859, - "rshares": 3800699762, - "voter": "wandergirl" - }, - { - "percent": "10000", - "reputation": 5313719494876540, - "rshares": 12478859864700, - "voter": "haejin" - }, - { - "percent": "2600", - "reputation": 145609724101, - "rshares": 240846596958, - "voter": "hope-on-fire" - }, - { - "percent": "10000", - "reputation": 91410383693752, - "rshares": 298898416303, - "voter": "vincentnijman" - }, - { - "percent": "150", - "reputation": 18018060826148, - "rshares": 602876522, - "voter": "shitsignals" - }, - { - "percent": "1000", - "reputation": 1060930411809617, - "rshares": 2490614227380, - "voter": "themarkymark" - }, - { - "percent": "10000", - "reputation": 171787286919500, - "rshares": 84913517836, - "voter": "yanes94" - }, - { - "percent": "5000", - "reputation": 29124480726940, - "rshares": 1905342002, - "voter": "greatness96" - }, - { - "percent": "4500", - "reputation": 36780217425224, - "rshares": 39938651800, - "voter": "dine77" - }, - { - "percent": "2320", - "reputation": 64603876629814, - "rshares": 98978693993, - "voter": "steempostitalia" - }, - { - "percent": "3000", - "reputation": 115269542036380, - "rshares": 401789741894, - "voter": "vikisecrets" - }, - { - "percent": "10000", - "reputation": 30408715367838, - "rshares": 158788136809, - "voter": "steemitri" - }, - { - "percent": "10000", - "reputation": 1095186992075950, - "rshares": 169215220532, - "voter": "cryptopassion" - }, - { - "percent": "10000", - "reputation": 1861063781145, - "rshares": 590377982, - "voter": "leryam12" - }, - { - "percent": "5000", - "reputation": 4022219848165, - "rshares": 3023476491, - "voter": "jenesa" - }, - { - "percent": "10000", - "reputation": 205993978812776, - "rshares": 121729107072, - "voter": "masummim50" - }, - { - "percent": "1000", - "reputation": 3574566966768, - "rshares": 3185013567, - "voter": "appleskie" - }, - { - "percent": "5000", - "reputation": 1189111686, - "rshares": 550603352, - "voter": "chunnorris" - }, - { - "percent": "5000", - "reputation": 135564305882897, - "rshares": 27868559333, - "voter": "nuthman" - }, - { - "percent": "4000", - "reputation": 15106373780430, - "rshares": 71880792461, - "voter": "spiceboyz" - }, - { - "percent": "150", - "reputation": 173069624727027, - "rshares": 7213295762, - "voter": "felander" - }, - { - "percent": "5000", - "reputation": 1087907674628, - "rshares": 1081613186, - "voter": "pjmisa" - }, - { - "percent": "3700", - "reputation": 24639928209538, - "rshares": 21386789609, - "voter": "santigs" - }, - { - "percent": "10000", - "reputation": 196571377648, - "rshares": 17058039993, - "voter": "nadhora" - }, - { - "percent": "10000", - "reputation": 5757657643273, - "rshares": 31412812898, - "voter": "noloafing" - }, - { - "percent": "75", - "reputation": 51994511985595, - "rshares": 328221671911, - "voter": "tipu" - }, - { - "percent": "400", - "reputation": 101280142849344, - "rshares": 10872033606, - "voter": "kimzwarch" - }, - { - "percent": "5000", - "reputation": 2656035667454, - "rshares": 1754547543, - "voter": "agentzero" - }, - { - "percent": "10000", - "reputation": 11653416526162, - "rshares": 30687762502, - "voter": "randyw" - }, - { - "percent": "500", - "reputation": 47942503950741, - "rshares": 48835534130, - "voter": "accelerator" - }, - { - "percent": "1000", - "reputation": 355541395288151, - "rshares": 7768398740974, - "voter": "buildawhale" - }, - { - "percent": "750", - "reputation": 33280218537901, - "rshares": 8430853873, - "voter": "artonmysleeve" - }, - { - "percent": "1000", - "reputation": 41069012860239, - "rshares": 1752125104, - "voter": "markjason" - }, - { - "percent": "10000", - "reputation": 2206804389678, - "rshares": 5014020204, - "voter": "marysent" - }, - { - "percent": "2320", - "reputation": 35075558795655, - "rshares": 33226751200, - "voter": "alinakot" - }, - { - "percent": "10000", - "reputation": 669346799793, - "rshares": 21044804847, - "voter": "voxmonkey" - }, - { - "percent": "10000", - "reputation": 537290602112, - "rshares": 221085055651, - "voter": "chinchilla" - }, - { - "percent": "10000", - "reputation": 167991810520774, - "rshares": 655070224234, - "voter": "celestal" - }, - { - "percent": "600", - "reputation": 2448367928201, - "rshares": 1337798610, - "voter": "pingcess" - }, - { - "percent": "250", - "reputation": 445234100950820, - "rshares": 788774861713, - "voter": "therealwolf" - }, - { - "percent": "10000", - "reputation": 481457224468, - "rshares": 1809208394, - "voter": "gnarlyanimations" - }, - { - "percent": "10000", - "reputation": 804792498605, - "rshares": 1595064042, - "voter": "hillaryaa" - }, - { - "percent": "5000", - "reputation": 336063014350538, - "rshares": 721918102810, - "voter": "revisesociology" - }, - { - "percent": "5000", - "reputation": 21798737047108, - "rshares": 100337730468, - "voter": "yangyanje" - }, - { - "percent": "5000", - "reputation": 959835803794, - "rshares": 600639000, - "voter": "mercy11" - }, - { - "percent": "1000", - "reputation": 927618060395434, - "rshares": 113438365889, - "voter": "majes.tytyty" - }, - { - "percent": "1000", - "reputation": 16626980732032, - "rshares": 937140623, - "voter": "diosarich" - }, - { - "percent": "800", - "reputation": 22607491274241, - "rshares": 672101884, - "voter": "suheri" - }, - { - "percent": "1000", - "reputation": 47982158911387, - "rshares": 31398832232, - "voter": "makerhacks" - }, - { - "percent": "10000", - "reputation": 769442706037, - "rshares": 1094873309, - "voter": "tedzwhistle" - }, - { - "percent": "2000", - "reputation": 6445110251502, - "rshares": 2622383993, - "voter": "divinekids" - }, - { - "percent": "2320", - "reputation": 12066290877974, - "rshares": 17623838781, - "voter": "heidi71" - }, - { - "percent": "10000", - "reputation": 5244834322487, - "rshares": 11083582626, - "voter": "andywong31" - }, - { - "percent": "10000", - "reputation": 33507158333033, - "rshares": 39396249891, - "voter": "mllg" - }, - { - "percent": "76", - "reputation": 0, - "rshares": 27129368624, - "voter": "investegg" - }, - { - "percent": "2000", - "reputation": 141970828773777, - "rshares": 355695491136, - "voter": "tobetada" - }, - { - "percent": "5000", - "reputation": 935189040639, - "rshares": 571183778, - "voter": "maaz23" - }, - { - "percent": "5000", - "reputation": 513799575547713, - "rshares": 301532658387, - "voter": "josediccus" - }, - { - "percent": "10000", - "reputation": 29313772061277, - "rshares": 130089207629, - "voter": "castleberry" - }, - { - "percent": "2000", - "reputation": 10939046475227, - "rshares": 762071984, - "voter": "liverpool-fan" - }, - { - "percent": "1000", - "reputation": 12918217107297, - "rshares": 1110140714, - "voter": "afterglow" - }, - { - "percent": "5000", - "reputation": 2501064007913, - "rshares": 2296329862, - "voter": "reyarobo" - }, - { - "percent": "10000", - "reputation": 28740832773198, - "rshares": 38707251641, - "voter": "steemph.cebu" - }, - { - "percent": "142", - "reputation": 7232716062458, - "rshares": 2767780603, - "voter": "caladan" - }, - { - "percent": "10000", - "reputation": 51827894908559, - "rshares": 52994273339, - "voter": "lays" - }, - { - "percent": "7500", - "reputation": 136276266931207, - "rshares": 117030122083, - "voter": "ybanezkim26" - }, - { - "percent": "5000", - "reputation": 2561399399469, - "rshares": 2200350990, - "voter": "legendarryll" - }, - { - "percent": "2800", - "reputation": 21694534890224, - "rshares": 51599562864, - "voter": "gianluccio" - }, - { - "percent": "3000", - "reputation": 2341876684406, - "rshares": 1910888554, - "voter": "steemph.manila" - }, - { - "percent": "10000", - "reputation": 106411145191010, - "rshares": 76769559873, - "voter": "ultravioletmag" - }, - { - "percent": "10000", - "reputation": 2913812595405, - "rshares": 3123035459, - "voter": "sorenkierkegaard" - }, - { - "percent": "2000", - "reputation": 14699853056335, - "rshares": 17367308395, - "voter": "ciuoto" - }, - { - "percent": "150", - "reputation": 377317663062316, - "rshares": 38436739630, - "voter": "emrebeyler" - }, - { - "percent": "5000", - "reputation": 1571846435496, - "rshares": 3980368527, - "voter": "dizzyapple" - }, - { - "percent": "1000", - "reputation": 28722297623429, - "rshares": 8275030569, - "voter": "maverickinvictus" - }, - { - "percent": "5000", - "reputation": 6231776804278, - "rshares": 950349136, - "voter": "brandt" - }, - { - "percent": "4000", - "reputation": 40643925986727, - "rshares": 38343746661, - "voter": "carolineschell" - }, - { - "percent": "250", - "reputation": 157805541487641, - "rshares": 155942422637, - "voter": "smartsteem" - }, - { - "percent": "800", - "reputation": 10160344260214, - "rshares": 1577390930, - "voter": "xsasj" - }, - { - "percent": "5000", - "reputation": 1752578402102, - "rshares": 674015919, - "voter": "iamqueenlevita" - }, - { - "percent": "1000", - "reputation": 10092142213140, - "rshares": 1111961983, - "voter": "thegaillery" - }, - { - "percent": "10000", - "reputation": 14709760292085, - "rshares": 18066679965, - "voter": "yazp" - }, - { - "percent": "500", - "reputation": 30362686718221, - "rshares": 2992624078, - "voter": "junebride" - }, - { - "percent": "5000", - "reputation": 2678661471750, - "rshares": 1079480309, - "voter": "janicemars" - }, - { - "percent": "10000", - "reputation": 1451995142982, - "rshares": 922192602, - "voter": "vishire" - }, - { - "percent": "10000", - "reputation": 18150082730, - "rshares": 386836552173, - "voter": "citizensmith" - }, - { - "percent": "1000", - "reputation": 13569938137899, - "rshares": 1611831834, - "voter": "kneelyrac" - }, - { - "percent": "5000", - "reputation": 2045506354809, - "rshares": 875231291, - "voter": "steeminer4up" - }, - { - "percent": "5000", - "reputation": 66785151966461, - "rshares": 242423818651, - "voter": "itchyfeetdonica" - }, - { - "percent": "1160", - "reputation": 9242922601755, - "rshares": 5224093548, - "voter": "marcolino76" - }, - { - "percent": "10000", - "reputation": 570069464324, - "rshares": 1776990359, - "voter": "namranna" - }, - { - "percent": "1800", - "reputation": 7653694649262, - "rshares": 548043119, - "voter": "godlovermel25" - }, - { - "percent": "1000", - "reputation": 75425198894223, - "rshares": 6460976412, - "voter": "straykat" - }, - { - "percent": "750", - "reputation": 14337364044156, - "rshares": 955128337, - "voter": "tomatom" - }, - { - "percent": "2500", - "reputation": 2675225025765, - "rshares": 7582443995, - "voter": "fourfourfun" - }, - { - "percent": "1000", - "reputation": 2680015924516, - "rshares": 7498589238, - "voter": "upmyvote" - }, - { - "percent": "5000", - "reputation": 1222519641209, - "rshares": 1115103977, - "voter": "pizzanniza" - }, - { - "percent": "10000", - "reputation": 73184833081444, - "rshares": 3363668766931, - "voter": "oliverschmid" - }, - { - "percent": "10000", - "reputation": 142520915467066, - "rshares": 85669905573, - "voter": "for91days" - }, - { - "percent": "10000", - "reputation": 13267517492775, - "rshares": 9850192102, - "voter": "juecoree" - }, - { - "percent": "10000", - "reputation": 11279365843876, - "rshares": 9757788099, - "voter": "nikkabomb" - }, - { - "percent": "300", - "reputation": 87246032649758, - "rshares": 5707335643, - "voter": "gabrielatravels" - }, - { - "percent": "5000", - "reputation": 1483157104148, - "rshares": 876926749, - "voter": "cyyy1998" - }, - { - "percent": "10000", - "reputation": 5995949670664, - "rshares": 1202279494, - "voter": "jude.villarta" - }, - { - "percent": "1000", - "reputation": 169520027942230, - "rshares": 9554442993, - "voter": "r351574nc3" - }, - { - "percent": "10000", - "reputation": 478617433585, - "rshares": 1706596546, - "voter": "glennamayjumaoas" - }, - { - "percent": "10000", - "reputation": 801609916213, - "rshares": 905746612, - "voter": "jonnahmatias1016" - }, - { - "percent": "10000", - "reputation": 718140601874, - "rshares": 1097550817, - "voter": "francesgardose" - }, - { - "percent": "5000", - "reputation": 20747172002123, - "rshares": 1300215151, - "voter": "shoganaii" - }, - { - "percent": "10000", - "reputation": 19169896425273, - "rshares": 4222266879, - "voter": "saintchristopher" - }, - { - "percent": "5000", - "reputation": 1451555574844, - "rshares": 1950578675, - "voter": "korinkrafting" - }, - { - "percent": "10000", - "reputation": 4255833468352, - "rshares": 866262118, - "voter": "thinkingmind" - }, - { - "percent": "10000", - "reputation": 9772009360245, - "rshares": 111783222536, - "voter": "fineartnow" - }, - { - "percent": "10000", - "reputation": 11200470488081, - "rshares": 20527802488, - "voter": "josejirafa" - }, - { - "percent": "2000", - "reputation": 84499975573513, - "rshares": 6067318829, - "voter": "alequandro" - }, - { - "percent": "10000", - "reputation": 52022541631815, - "rshares": 29325632137, - "voter": "soufianechakrouf" - }, - { - "percent": "4000", - "reputation": 7648880714525, - "rshares": 449080614, - "voter": "mirkon86" - }, - { - "percent": "150", - "reputation": 138441860072892, - "rshares": 8667078727, - "voter": "nealmcspadden" - }, - { - "percent": "1000", - "reputation": 4882081332011, - "rshares": 1920301528, - "voter": "christianyocte" - }, - { - "percent": "400", - "reputation": 25048759621308, - "rshares": 3797289990, - "voter": "tryskele" - }, - { - "percent": "4000", - "reputation": 347022507711444, - "rshares": 167170145245, - "voter": "piotrgrafik" - }, - { - "percent": "300", - "reputation": 38330902987406, - "rshares": 1895717228, - "voter": "manncpt" - }, - { - "percent": "10000", - "reputation": 5902918865373, - "rshares": 4477193475, - "voter": "m1alsan" - }, - { - "percent": "5000", - "reputation": 219242636184, - "rshares": 612132323, - "voter": "brusd" - }, - { - "percent": "150", - "reputation": 7978719984268, - "rshares": 1836390518, - "voter": "purefood" - }, - { - "percent": "5000", - "reputation": 822709975413, - "rshares": 718014022, - "voter": "honeyletsgo" - }, - { - "percent": "5000", - "reputation": 1627145024010, - "rshares": 796930050, - "voter": "georgie84" - }, - { - "percent": "7706", - "reputation": 5977990737151, - "rshares": 4071894256, - "voter": "knfitaly" - }, - { - "percent": "5000", - "reputation": 1298566083487, - "rshares": 1329852123, - "voter": "davids-tales" - }, - { - "percent": "300", - "reputation": 7036325034531, - "rshares": 550564635, - "voter": "jnmarteau" - }, - { - "percent": "1000", - "reputation": 4755551366717, - "rshares": 706497613, - "voter": "philnewton" - }, - { - "percent": "5000", - "reputation": 2374130318210, - "rshares": 1256665149, - "voter": "cheesom" - }, - { - "percent": "10000", - "reputation": 21875725656972, - "rshares": 10931018057, - "voter": "ligarayk" - }, - { - "percent": "750", - "reputation": 79537112262409, - "rshares": 14638325908, - "voter": "tobias-g" - }, - { - "percent": "5000", - "reputation": 47747940709375, - "rshares": 11171306961, - "voter": "gatolector" - }, - { - "percent": "10000", - "reputation": 148975588734578, - "rshares": 7828300579, - "voter": "svemirac" - }, - { - "percent": "2000", - "reputation": 442944056818, - "rshares": 732813846, - "voter": "minerspost" - }, - { - "percent": "10000", - "reputation": 354023179708, - "rshares": 744189111, - "voter": "raquelita" - }, - { - "percent": "5000", - "reputation": 874108865684, - "rshares": 602064293, - "voter": "geeyang15" - }, - { - "percent": "4000", - "reputation": 12153845416413, - "rshares": 12219616398, - "voter": "piumadoro" - }, - { - "percent": "100", - "reputation": 107854173717168, - "rshares": 3571971556, - "voter": "moeenali" - }, - { - "percent": "10000", - "reputation": 100885493809, - "rshares": 6074941331, - "voter": "lerma" - }, - { - "percent": "10000", - "reputation": 18641585439625, - "rshares": 22451104198, - "voter": "sudefteri" - }, - { - "percent": "5000", - "reputation": 12334368355423, - "rshares": 1547892183, - "voter": "loydjayme25" - }, - { - "percent": "10000", - "reputation": 78606992818, - "rshares": 1111950762, - "voter": "photohunter3" - }, - { - "percent": "5000", - "reputation": 71504792482, - "rshares": 559710186, - "voter": "photohunter5" - }, - { - "percent": "120", - "reputation": 10898214322749, - "rshares": 590543310, - "voter": "pkocjan" - }, - { - "percent": "5000", - "reputation": 71185892857862, - "rshares": 49107270234, - "voter": "chungsu1" - }, - { - "percent": "10000", - "reputation": 86376131766962, - "rshares": 169700473270, - "voter": "gohenry" - }, - { - "percent": "5000", - "reputation": 2866827942623, - "rshares": 3170224595, - "voter": "starzy" - }, - { - "percent": "250", - "reputation": 26898775193091, - "rshares": 2392007368, - "voter": "movement19" - }, - { - "percent": "10000", - "reputation": 1173641910090, - "rshares": 3752383955, - "voter": "akifane" - }, - { - "percent": "10000", - "reputation": 8323395238723, - "rshares": 1819634754, - "voter": "technologix" - }, - { - "percent": "10000", - "reputation": 11193010266714, - "rshares": 13681360811, - "voter": "elfranz" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 28840617171, - "voter": "futurecurrency" - }, - { - "percent": "10000", - "reputation": 73042470414733, - "rshares": 420265801646, - "voter": "rubencress" - }, - { - "percent": "10000", - "reputation": 5195582997313, - "rshares": 1072721305, - "voter": "leebaong" - }, - { - "percent": "10000", - "reputation": 121063235291, - "rshares": 702079311, - "voter": "thisisruby" - }, - { - "percent": "10000", - "reputation": 87629137367, - "rshares": 1979400442, - "voter": "rasty.demecillo" - }, - { - "percent": "10000", - "reputation": 154592881404, - "rshares": 636298659, - "voter": "memeitbaby" - }, - { - "percent": "10000", - "reputation": 1835670573034, - "rshares": 906189829, - "voter": "missdonna" - }, - { - "percent": "5000", - "reputation": 2654510329914, - "rshares": 1835524479, - "voter": "sawi" - }, - { - "percent": "2800", - "reputation": 130666880687240, - "rshares": 55318504399, - "voter": "mad-runner" - }, - { - "percent": "2500", - "reputation": 2256065348315, - "rshares": 4162553868, - "voter": "frassman" - }, - { - "percent": "10000", - "reputation": 3441314325667, - "rshares": 103662959321, - "voter": "norwegianbikeman" - }, - { - "percent": "10000", - "reputation": 9839458481068, - "rshares": 23231096029, - "voter": "kryptik.tigrrr3d" - }, - { - "percent": "4000", - "reputation": 10685467371502, - "rshares": 9378565242, - "voter": "sbarandelli" - }, - { - "percent": "1200", - "reputation": 54208883787930, - "rshares": 17140053331, - "voter": "outlinez" - }, - { - "percent": "1000", - "reputation": 10250642778719, - "rshares": 3278130865, - "voter": "salty-mcgriddles" - }, - { - "percent": "5000", - "reputation": 742795053593, - "rshares": 1101999681, - "voter": "themarshann" - }, - { - "percent": "10000", - "reputation": 24953974425628, - "rshares": 1811051907476, - "voter": "a0i" - }, - { - "percent": "150", - "reputation": 1170108458021, - "rshares": 628395081, - "voter": "bestboom" - }, - { - "percent": "2000", - "reputation": 21335847636859, - "rshares": 12675519061, - "voter": "vittoriozuccala" - }, - { - "percent": "10000", - "reputation": 19069547910280, - "rshares": 319689586587, - "voter": "adamada" - }, - { - "percent": "5000", - "reputation": 63828014180083, - "rshares": 29041113861, - "voter": "marcybetancourt" - }, - { - "percent": "5000", - "reputation": 1553888219838, - "rshares": 938664558, - "voter": "jackobeat" - }, - { - "percent": "10000", - "reputation": 1238565986273, - "rshares": 1762690117, - "voter": "carlitojoshua" - }, - { - "percent": "1258", - "reputation": 9124351654981, - "rshares": 125306064395, - "voter": "sbi2" - }, - { - "percent": "2000", - "reputation": 3002913313511, - "rshares": 1586138771, - "voter": "lycos" - }, - { - "percent": "10000", - "reputation": 24955952314452, - "rshares": 23702084377, - "voter": "koto-art" - }, - { - "percent": "10000", - "reputation": 4110594694460, - "rshares": 1147742343668, - "voter": "jkramer" - }, - { - "percent": "10000", - "reputation": 256919577419282, - "rshares": 326532714238, - "voter": "cyberdemon531" - }, - { - "percent": "7500", - "reputation": 35499944396318, - "rshares": 27129757317, - "voter": "tesmoforia" - }, - { - "percent": "5000", - "reputation": 5228129093643, - "rshares": 1648901189, - "voter": "elvys" - }, - { - "percent": "10000", - "reputation": 1451811665047, - "rshares": 6590945164, - "voter": "orkin420" - }, - { - "percent": "1500", - "reputation": 7324548372470, - "rshares": 22505848740, - "voter": "freddio" - }, - { - "percent": "10000", - "reputation": 14193144291158, - "rshares": 5623859595, - "voter": "abdulmath" - }, - { - "percent": "10000", - "reputation": 231872774161550, - "rshares": 2177293579069, - "voter": "midlet" - }, - { - "percent": "10000", - "reputation": 17018469559317, - "rshares": 21199808006, - "voter": "allover" - }, - { - "percent": "10000", - "reputation": 131658428787788, - "rshares": 53136191371, - "voter": "richjr" - }, - { - "percent": "3000", - "reputation": 750879699293, - "rshares": 1776454988, - "voter": "steemitachievers" - }, - { - "percent": "4000", - "reputation": 15997954268310, - "rshares": 56810233183, - "voter": "spaghettiscience" - }, - { - "percent": "5000", - "reputation": 9877041015840, - "rshares": 14418855659, - "voter": "misia1979" - }, - { - "percent": "5000", - "reputation": 564946014672, - "rshares": 654464113, - "voter": "aljunecastro" - }, - { - "percent": "10000", - "reputation": 2481629111221, - "rshares": 2168373327, - "voter": "manojbhatt" - }, - { - "percent": "3200", - "reputation": 65521507383931, - "rshares": 15740313956, - "voter": "oscurity" - }, - { - "percent": "10000", - "reputation": 301808772904, - "rshares": 2186236485, - "voter": "kendallron" - }, - { - "percent": "10000", - "reputation": 37055878863116, - "rshares": 18925698098, - "voter": "thilah" - }, - { - "percent": "10000", - "reputation": 5764675494828, - "rshares": 80867082313, - "voter": "calisay" - }, - { - "percent": "150", - "reputation": 15436790913241, - "rshares": 1296038933, - "voter": "globalschool" - }, - { - "percent": "4000", - "reputation": 58972096571144, - "rshares": 3306767013, - "voter": "bafi" - }, - { - "percent": "2000", - "reputation": 1471256869354, - "rshares": 2562372424, - "voter": "steemph.uae" - }, - { - "percent": "5400", - "reputation": 43948808161603, - "rshares": 26236872482, - "voter": "phage93" - }, - { - "percent": "10000", - "reputation": 1180987164511, - "rshares": 3889313045, - "voter": "coarebabes" - }, - { - "percent": "5000", - "reputation": 2068514185656, - "rshares": 1861256988, - "voter": "anneporter" - }, - { - "percent": "5000", - "reputation": 152242646200543, - "rshares": 19569737749, - "voter": "serialfiller" - }, - { - "percent": "5000", - "reputation": 3115428694948, - "rshares": 1960462858, - "voter": "indayclara" - }, - { - "percent": "37", - "reputation": 1963153455547, - "rshares": 8335189069, - "voter": "steem.services" - }, - { - "percent": "1000", - "reputation": 1993672004294, - "rshares": 51038090039, - "voter": "pladozero" - }, - { - "percent": "800", - "reputation": 70214892950356, - "rshares": 301551776260, - "voter": "nateaguila" - }, - { - "percent": "2200", - "reputation": 51236835710173, - "rshares": 9869278918, - "voter": "davidesimoncini" - }, - { - "percent": "3000", - "reputation": 39672928171915, - "rshares": 5319622024, - "voter": "darthgexe" - }, - { - "percent": "1000", - "reputation": 27284305279794, - "rshares": 1212367718, - "voter": "rachelleignacio" - }, - { - "percent": "10000", - "reputation": 347301947461, - "rshares": 530524351, - "voter": "blackelephant" - }, - { - "percent": "5000", - "reputation": 24852530816752, - "rshares": 11614109569, - "voter": "jason04" - }, - { - "percent": "10000", - "reputation": 6464145962606, - "rshares": 16106278766, - "voter": "jemzem" - }, - { - "percent": "5000", - "reputation": 1722687991193, - "rshares": 662849856, - "voter": "pinas" - }, - { - "percent": "2658", - "reputation": 0, - "rshares": 4824449506, - "voter": "thefoundation" - }, - { - "percent": "5000", - "reputation": 57721280407516, - "rshares": 60480016275, - "voter": "insaneworks" - }, - { - "percent": "5000", - "reputation": 772102466926, - "rshares": 615739560, - "voter": "kyanzieuno" - }, - { - "percent": "4000", - "reputation": 11198941837827, - "rshares": 6585808896, - "voter": "giuseppemasala" - }, - { - "percent": "10000", - "reputation": 15332816096733, - "rshares": 2625553819, - "voter": "luminaryhmo" - }, - { - "percent": "4000", - "reputation": 4314543468754, - "rshares": 2513680063, - "voter": "acquarius30" - }, - { - "percent": "1000", - "reputation": 95233949488692, - "rshares": 18095298599, - "voter": "sgbonus" - }, - { - "percent": "5000", - "reputation": 244842259743, - "rshares": 1189082585, - "voter": "ihal0001" - }, - { - "percent": "10000", - "reputation": 3274691895642, - "rshares": 1754465927, - "voter": "soma909" - }, - { - "percent": "150", - "reputation": 655223881789, - "rshares": 833970168, - "voter": "swisswitness" - }, - { - "percent": "10000", - "reputation": 6370085312, - "rshares": 1305835631, - "voter": "kahvesizlik" - }, - { - "percent": "5000", - "reputation": 778260061223, - "rshares": 606535579, - "voter": "mojacko" - }, - { - "percent": "10000", - "reputation": 13210715947156, - "rshares": 9054525167, - "voter": "astrolabio" - }, - { - "percent": "1000", - "reputation": 599567348573, - "rshares": 503595922, - "voter": "exifr" - }, - { - "percent": "5000", - "reputation": 1137655654537, - "rshares": 1562502119, - "voter": "czera" - }, - { - "percent": "10000", - "reputation": 65268642593339, - "rshares": 47220808603, - "voter": "sabari18" - }, - { - "percent": "400", - "reputation": 46213136832830, - "rshares": 14955293802, - "voter": "nattybongo" - }, - { - "percent": "1000", - "reputation": 30631004038630, - "rshares": 3052335106, - "voter": "sarimanok" - }, - { - "percent": "4000", - "reputation": 6326146746853, - "rshares": 2319600771, - "voter": "doodle.danga" - }, - { - "percent": "50", - "reputation": 292465506149952, - "rshares": 1032013294, - "voter": "shortsegments" - }, - { - "percent": "10000", - "reputation": 6931413629, - "rshares": 898223994, - "voter": "tiffcisme" - }, - { - "percent": "1000", - "reputation": 97973570792503, - "rshares": 4722523016, - "voter": "mrnightmare89" - }, - { - "percent": "150", - "reputation": 33860216986858, - "rshares": 9200546181, - "voter": "donald.porter" - }, - { - "percent": "5000", - "reputation": 776039665811, - "rshares": 589795996, - "voter": "kayegrasya" - }, - { - "percent": "5000", - "reputation": 655454638322, - "rshares": 7052393314, - "voter": "bigpower" - }, - { - "percent": "2800", - "reputation": 312194048136745, - "rshares": 133686720853, - "voter": "armandosodano" - }, - { - "percent": "2000", - "reputation": 118140326729872, - "rshares": 7482811298, - "voter": "sadbear" - }, - { - "percent": "10000", - "reputation": 711352468979, - "rshares": 24866342709, - "voter": "bestofph" - }, - { - "percent": "1000", - "reputation": 116804559, - "rshares": 601738373, - "voter": "exifr0" - }, - { - "percent": "75", - "reputation": 156376467869107, - "rshares": 2596515931, - "voter": "dalz" - }, - { - "percent": "2000", - "reputation": 32718897828056, - "rshares": 38848379907, - "voter": "ilnegro" - }, - { - "percent": "2500", - "reputation": 24307599069679, - "rshares": 8831705105, - "voter": "dronegraphica" - }, - { - "percent": "75", - "reputation": 18790919715448, - "rshares": 10703981581, - "voter": "luppers" - }, - { - "percent": "150", - "reputation": 103580743464850, - "rshares": 17360961564, - "voter": "dlike" - }, - { - "percent": "10000", - "reputation": 7532194699640, - "rshares": 7449383794, - "voter": "cubapl" - }, - { - "percent": "3200", - "reputation": 12546717165553, - "rshares": 8530371222, - "voter": "tommasobusiello" - }, - { - "percent": "4000", - "reputation": 5384315527317, - "rshares": 5806300193, - "voter": "coccodema" - }, - { - "percent": "50", - "reputation": 144910360949054, - "rshares": 920757335, - "voter": "voxmortis" - }, - { - "percent": "10000", - "reputation": 836324990221, - "rshares": 4474675467, - "voter": "jokinmenipieleen" - }, - { - "percent": "120", - "reputation": 58772053733860, - "rshares": 24043800602, - "voter": "engrave" - }, - { - "percent": "5000", - "reputation": 77764751586248, - "rshares": 1734072259576, - "voter": "frot" - }, - { - "percent": "6100", - "reputation": 25516225567436, - "rshares": 42917011948, - "voter": "nowargraffitis" - }, - { - "percent": "5264", - "reputation": 0, - "rshares": 4857692161, - "voter": "voter002" - }, - { - "percent": "267", - "reputation": 0, - "rshares": 2475462010, - "voter": "voter003" - }, - { - "percent": "10000", - "reputation": 5426260749375, - "rshares": 14214046644, - "voter": "agromeror" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 16964338633, - "voter": "quediceharry" - }, - { - "percent": "10000", - "reputation": 686691140588, - "rshares": 671390861, - "voter": "juliettana" - }, - { - "percent": "5000", - "reputation": 2029932349983, - "rshares": 1544843672, - "voter": "angoujkalis" - }, - { - "percent": "10000", - "reputation": 39556037473, - "rshares": 2061717990, - "voter": "worksinsane" - }, - { - "percent": "2000", - "reputation": 41288629235348, - "rshares": 2248535961, - "voter": "mariluna" - }, - { - "percent": "5000", - "reputation": 6687049412835, - "rshares": 40857653081, - "voter": "mister-meeseeks" - }, - { - "percent": "10000", - "reputation": 158552584457210, - "rshares": 177949277080, - "voter": "macoolette" - }, - { - "percent": "2000", - "reputation": 21160020180639, - "rshares": 1475535465, - "voter": "javier.dejuan" - }, - { - "percent": "5000", - "reputation": 28282881619671, - "rshares": 14491846702, - "voter": "fulani" - }, - { - "percent": "1600", - "reputation": 36278740673923, - "rshares": 538436468598, - "voter": "cryptoxicate" - }, - { - "percent": "2000", - "reputation": 16571339882650, - "rshares": 1278453386, - "voter": "elyon" - }, - { - "percent": "4000", - "reputation": 9413067039884, - "rshares": 1202503343, - "voter": "itegoarcanadei" - }, - { - "percent": "150", - "reputation": 0, - "rshares": 4552498185, - "voter": "merlin7" - }, - { - "percent": "10000", - "reputation": 1648086760085, - "rshares": 27561794087, - "voter": "diegor" - }, - { - "percent": "1000", - "reputation": 32423491687236, - "rshares": 1591394495, - "voter": "maonx" - }, - { - "percent": "150", - "reputation": 2716815451543, - "rshares": 10795688836, - "voter": "followjohngalt" - }, - { - "percent": "4000", - "reputation": 1886525460663, - "rshares": 1637594120, - "voter": "middleearth" - }, - { - "percent": "100", - "reputation": 4915527864940, - "rshares": 65148478, - "voter": "dashand" - }, - { - "percent": "2000", - "reputation": 25104308682855, - "rshares": 5393224821, - "voter": "adinapoli" - }, - { - "percent": "10000", - "reputation": 2191807, - "rshares": 741699209, - "voter": "props" - }, - { - "percent": "75", - "reputation": 3420036317592, - "rshares": 924671558, - "voter": "cakemonster" - }, - { - "percent": "4000", - "reputation": 38453773394334, - "rshares": 2543671219, - "voter": "akireuna" - }, - { - "percent": "4000", - "reputation": 10397283210375, - "rshares": 493186242302, - "voter": "discovery-it" - }, - { - "percent": "10000", - "reputation": 2898780281687, - "rshares": 90426980273, - "voter": "crypto-pixie" - }, - { - "percent": "10000", - "reputation": 6865606194, - "rshares": 15042238870, - "voter": "star-x" - }, - { - "percent": "10000", - "reputation": 180858284734, - "rshares": 3353461497, - "voter": "sergeyberkova" - }, - { - "percent": "2000", - "reputation": 21657939011190, - "rshares": 1325122242, - "voter": "kork75" - }, - { - "percent": "10000", - "reputation": 1158706837675, - "rshares": 648803548463, - "voter": "lestrange" - }, - { - "percent": "5000", - "reputation": 5873083, - "rshares": 34657698160, - "voter": "reflektor" - }, - { - "percent": "5000", - "reputation": 5884437, - "rshares": 8487104385, - "voter": "bippe" - }, - { - "percent": "150", - "reputation": 1213063566738, - "rshares": 540636668, - "voter": "permaculturedude" - }, - { - "percent": "5000", - "reputation": 6085708906847, - "rshares": 7456525291, - "voter": "creary" - }, - { - "percent": "4000", - "reputation": 10262180239331, - "rshares": 7505965585, - "voter": "lallo" - }, - { - "percent": "5000", - "reputation": 5878610, - "rshares": 31845184002, - "voter": "hingsten" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 26417776438, - "voter": "tankometry" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 26255517979, - "voter": "jetometry" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 23636935395, - "voter": "rockstarbm" - }, - { - "percent": "1500", - "reputation": 6301639433510, - "rshares": 806991193, - "voter": "goodcontentbot" - }, - { - "percent": "2800", - "reputation": 16012295789643, - "rshares": 2695071590, - "voter": "cooperfelix" - }, - { - "percent": "3500", - "reputation": 410620631652, - "rshares": 4420503749, - "voter": "ava77" - }, - { - "percent": "5000", - "reputation": 611845544559, - "rshares": 1948740302, - "voter": "parth7878" - }, - { - "percent": "10000", - "reputation": 25016264134313, - "rshares": 743182265835, - "voter": "dcommerce" - }, - { - "percent": "500", - "reputation": 5616865862, - "rshares": 1058218978, - "voter": "abbenay" - }, - { - "percent": "5000", - "reputation": 2910555091868, - "rshares": 532842460, - "voter": "denizcakmak" - }, - { - "percent": "10000", - "reputation": 3550575399642, - "rshares": 4841310903, - "voter": "kaptnkalle" - }, - { - "percent": "2500", - "reputation": 27838835653920, - "rshares": 7099034089, - "voter": "kggymlife" - }, - { - "percent": "3600", - "reputation": 2761382305579, - "rshares": 1703608788, - "voter": "david.steem" - }, - { - "percent": "75", - "reputation": 69910542366759, - "rshares": 3942483477, - "voter": "kryptogames" - }, - { - "percent": "2000", - "reputation": 277256769997, - "rshares": 16366288987, - "voter": "ambifokus" - }, - { - "percent": "142", - "reputation": 17287220932883, - "rshares": 1397666846, - "voter": "mfblack" - }, - { - "percent": "10000", - "reputation": 4663518569101, - "rshares": 872870615331, - "voter": "blue.rabbit" - }, - { - "percent": "4000", - "reputation": 26656982596638, - "rshares": 16463449762, - "voter": "titti" - }, - { - "percent": "10000", - "reputation": 51900218528169, - "rshares": 1968095708, - "voter": "alexvanaken" - }, - { - "percent": "4000", - "reputation": 8977413850563, - "rshares": 9605140923, - "voter": "maryincryptoland" - }, - { - "percent": "75", - "reputation": 120936131908085, - "rshares": 5616137340, - "voter": "epicdice" - }, - { - "percent": "7500", - "reputation": 3909341978875, - "rshares": 1385994751, - "voter": "bitcoingodmode" - }, - { - "percent": "5000", - "reputation": 58574039848, - "rshares": 1336708426, - "voter": "zackarie" - }, - { - "percent": "4000", - "reputation": 3026614496178, - "rshares": 2174753249, - "voter": "stregamorgana" - }, - { - "percent": "2400", - "reputation": 4511660112150, - "rshares": 3195523263, - "voter": "meeplecomposer" - }, - { - "percent": "4000", - "reputation": 130839158526947, - "rshares": 116253847317, - "voter": "libertycrypto27" - }, - { - "percent": "10000", - "reputation": 98578472333076, - "rshares": 458200528586, - "voter": "minigame" - }, - { - "percent": "5000", - "reputation": 2597264781531, - "rshares": 8384152646, - "voter": "akumagai" - }, - { - "percent": "5000", - "reputation": 64603915090283, - "rshares": 24622277845, - "voter": "caro-art" - }, - { - "percent": "4000", - "reputation": 1694014220511, - "rshares": 667379992, - "voter": "karamazov00" - }, - { - "percent": "5000", - "reputation": 1099279919915, - "rshares": 655663264, - "voter": "abdulmatin69" - }, - { - "percent": "5000", - "reputation": 468629183386, - "rshares": 1083183432, - "voter": "oemplus" - }, - { - "percent": "500", - "reputation": 584484995619, - "rshares": 527686171, - "voter": "tinyhousecryptos" - }, - { - "percent": "10000", - "reputation": 28083763213144, - "rshares": 3809108072, - "voter": "victartex" - }, - { - "percent": "2000", - "reputation": 8180177327690, - "rshares": 12067791552, - "voter": "maruskina" - }, - { - "percent": "2000", - "reputation": 9123079111767, - "rshares": 3832845306, - "voter": "claudietto" - }, - { - "percent": "10000", - "reputation": 11624344336093, - "rshares": 380236137709, - "voter": "recording-box" - }, - { - "percent": "4000", - "reputation": 2161742682386, - "rshares": 4672547566, - "voter": "omodei" - }, - { - "percent": "750", - "reputation": 0, - "rshares": 1444869138, - "voter": "cryptogambit" - }, - { - "percent": "150", - "reputation": 52974480102727, - "rshares": 745489678, - "voter": "shimozurdo" - }, - { - "percent": "150", - "reputation": 40619501571, - "rshares": 585637949, - "voter": "milu-the-dog" - }, - { - "percent": "150", - "reputation": 115912639173774, - "rshares": 14706987209, - "voter": "steem.leo" - }, - { - "percent": "10000", - "reputation": 16446725825074, - "rshares": 668347143, - "voter": "onecent" - }, - { - "percent": "10000", - "reputation": 6411393851, - "rshares": 838957036, - "voter": "driedfruit" - }, - { - "percent": "135", - "reputation": 14604990824032, - "rshares": 1619675712, - "voter": "asteroids" - }, - { - "percent": "4000", - "reputation": 23874904197926, - "rshares": 5056267028, - "voter": "capitanonema" - }, - { - "percent": "2000", - "reputation": 4589502219665, - "rshares": 846534186, - "voter": "damaskinus" - }, - { - "percent": "10000", - "reputation": 5610257148, - "rshares": 946433876, - "voter": "dfacademy-ccc" - }, - { - "percent": "1500", - "reputation": 91995455090943, - "rshares": 4979420027, - "voter": "thranax" - }, - { - "percent": "100", - "reputation": 6481559677290, - "rshares": 153496718234, - "voter": "project.hope" - }, - { - "percent": "10000", - "reputation": 45364952422214, - "rshares": 62331236190, - "voter": "soyunasantacruz" - }, - { - "percent": "37", - "reputation": 1410161022, - "rshares": 940976525, - "voter": "botante" - }, - { - "percent": "150", - "reputation": 241740100, - "rshares": 1161371816, - "voter": "beta500" - }, - { - "percent": "2000", - "reputation": 635849426463, - "rshares": 31800576, - "voter": "fifay" - }, - { - "percent": "5000", - "reputation": 1161034162384, - "rshares": 979624450, - "voter": "joshuafootball" - }, - { - "percent": "75", - "reputation": 426404968, - "rshares": 3014319937, - "voter": "maxuvd" - }, - { - "percent": "10000", - "reputation": 36363665396749, - "rshares": 146723100328, - "voter": "rootdraws" - }, - { - "percent": "10000", - "reputation": 15980504190694, - "rshares": 30227927023, - "voter": "lackofcolor" - }, - { - "percent": "2000", - "reputation": 825635650718, - "rshares": 402244693, - "voter": "freedomteam2019" - }, - { - "percent": "2000", - "reputation": 17403163667146, - "rshares": 2393595400, - "voter": "leoneil" - }, - { - "percent": "2500", - "reputation": 0, - "rshares": 937292928, - "voter": "isaria-ccc" - }, - { - "percent": "1500", - "reputation": 2754871650749, - "rshares": 3627038741, - "voter": "dappstats" - }, - { - "percent": "10000", - "reputation": 5553103337423, - "rshares": 5391443121, - "voter": "youngmusician" - }, - { - "percent": "4000", - "reputation": 11655092610, - "rshares": 25162545964, - "voter": "axel-blaze" - }, - { - "percent": "4000", - "reputation": 50737043318048, - "rshares": 5461173423, - "voter": "discovery-blog" - }, - { - "percent": "4000", - "reputation": 92463206107216, - "rshares": 43492942646, - "voter": "zacknorman97" - }, - { - "percent": "5000", - "reputation": 447569668922, - "rshares": 2145742587, - "voter": "bilpcoin.pay" - }, - { - "percent": "2000", - "reputation": 26678047282163, - "rshares": 8165657908, - "voter": "riccc96" - }, - { - "percent": "10000", - "reputation": -3148190173591, - "rshares": 292314410503, - "voter": "huaren.news" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 1529784193, - "voter": "khalccc" - }, - { - "percent": "1000", - "reputation": 0, - "rshares": 4307648196, - "voter": "bella.bear" - }, - { - "percent": "750", - "reputation": 0, - "rshares": 16467254, - "voter": "kryptoformator" - }, - { - "percent": "4000", - "reputation": 24720459476673, - "rshares": 15028983841, - "voter": "delilhavores" - }, - { - "percent": "3600", - "reputation": 6574541842654, - "rshares": 5365956936, - "voter": "hjmarseille" - }, - { - "percent": "3400", - "reputation": 590689352460, - "rshares": 782754652, - "voter": "boomalex" - }, - { - "percent": "8500", - "reputation": 32518344799, - "rshares": 326447780282, - "voter": "artcentral" - }, - { - "percent": "10000", - "reputation": 1017434237384, - "rshares": 8764405758, - "voter": "jeffmackinnon" - }, - { - "percent": "10000", - "reputation": 19310647761618, - "rshares": 167859476638, - "voter": "onchainart" - }, - { - "percent": "5000", - "reputation": 356840875823, - "rshares": 1757048244, - "voter": "kgsupport" - }, - { - "percent": "5000", - "reputation": 20853422627715, - "rshares": 654624297, - "voter": "im-ridd" - }, - { - "percent": "5000", - "reputation": 32542453022, - "rshares": 4812002016, - "voter": "bilpcoinbpc" - }, - { - "percent": "10000", - "reputation": 76222060731, - "rshares": 2170550777, - "voter": "wallytiteuf" - }, - { - "percent": "5000", - "reputation": 12710819754219, - "rshares": 629520110, - "voter": "disagio.gang" - }, - { - "percent": "150", - "reputation": 549265305265, - "rshares": 8918381923, - "voter": "mice-k" - }, - { - "percent": "10000", - "reputation": 14577043701834, - "rshares": 15308821398, - "voter": "julesquirin" - }, - { - "percent": "2000", - "reputation": 13104433773695, - "rshares": 1990159519, - "voter": "mengene" - }, - { - "percent": "10000", - "reputation": 5922809472275, - "rshares": 5332037448, - "voter": "jessescenica" - }, - { - "percent": "2000", - "reputation": 8718865668366, - "rshares": 1277488656, - "voter": "romytokic" - }, - { - "percent": "4000", - "reputation": 9624495306704, - "rshares": 47869480095626, - "voter": "darthknight" - }, - { - "percent": "500", - "reputation": 30837223420968, - "rshares": 725755547, - "voter": "drew0" - }, - { - "percent": "10000", - "reputation": 3260566328403, - "rshares": 13236746921, - "voter": "gorllara" - }, - { - "percent": "300", - "reputation": 0, - "rshares": 16006199638, - "voter": "fengchao" - }, - { - "percent": "300", - "reputation": 35668660233941, - "rshares": 8348671589, - "voter": "hivebuzz" - }, - { - "percent": "400", - "reputation": 91987050007999, - "rshares": 1371899910, - "voter": "hiveyoda" - }, - { - "percent": "1700", - "reputation": 7945277929340, - "rshares": 15182570983, - "voter": "wolven-znz" - }, - { - "percent": "2000", - "reputation": 5222879596789, - "rshares": 51627635287, - "voter": "hiveph" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 400543009712, - "voter": "hivephilippines" - }, - { - "percent": "5200", - "reputation": 131000724653734, - "rshares": 71230843687, - "voter": "creativemary" - }, - { - "percent": "10000", - "reputation": 88669665911129, - "rshares": 4195547961760, - "voter": "hivegc" - }, - { - "percent": "2800", - "reputation": 1209731647511, - "rshares": 468181924031, - "voter": "softworld" - }, - { - "percent": "150", - "reputation": 5571802524553, - "rshares": 3975900668, - "voter": "polish.hive" - }, - { - "percent": "10000", - "reputation": 388630945885, - "rshares": 10595539594, - "voter": "jwynqc" - }, - { - "percent": "4000", - "reputation": 7288245429962, - "rshares": 1373012771, - "voter": "ghastlygames" - }, - { - "percent": "4000", - "reputation": 16687677218, - "rshares": 44341702828, - "voter": "peterpanpan" - }, - { - "percent": "750", - "reputation": 22060257076152, - "rshares": 3262497793, - "voter": "quello" - }, - { - "percent": "10000", - "reputation": 4274979461460, - "rshares": 46836203090, - "voter": "neon3000" - }, - { - "percent": "150", - "reputation": 0, - "rshares": 42593036802, - "voter": "dcityrewards" - }, - { - "percent": "100", - "reputation": 2054630599034, - "rshares": 0, - "voter": "lhen18" - }, - { - "percent": "100", - "reputation": 1444444874297, - "rshares": 0, - "voter": "photodashph" - }, - { - "percent": "1000", - "reputation": 7317895795260, - "rshares": 536908146, - "voter": "mami.sheh7" - }, - { - "percent": "1000", - "reputation": 661148949025, - "rshares": 587827727, - "voter": "gradeon" - }, - { - "percent": "10000", - "reputation": 4070409415, - "rshares": 565531800, - "voter": "marvschurchill1" - }, - { - "percent": "4000", - "reputation": 65585429713, - "rshares": 202814207424, - "voter": "meppij" - }, - { - "percent": "4000", - "reputation": 3049760416566, - "rshares": 1569114858, - "voter": "matteus57" - }, - { - "percent": "187", - "reputation": 47896334821902, - "rshares": 2568017932, - "voter": "hiveonboard" - }, - { - "percent": "1000", - "reputation": 3177222978373, - "rshares": 9141561051, - "voter": "hivelist" - }, - { - "percent": "2500", - "reputation": 604442758770, - "rshares": 111971429220, - "voter": "ninnu" - }, - { - "percent": "1000", - "reputation": 0, - "rshares": 39863569747, - "voter": "kiemis" - }, - { - "percent": "10000", - "reputation": 978489051717, - "rshares": 776679205, - "voter": "jesuscasalesson" - }, - { - "percent": "1000", - "reputation": 7034683328715, - "rshares": 849451573, - "voter": "iameden" - }, - { - "percent": "2000", - "reputation": 9650263289467, - "rshares": 11087773591, - "voter": "localgrower" - }, - { - "percent": "10000", - "reputation": 23897781953466, - "rshares": 23741864159, - "voter": "ssygmr" - }, - { - "percent": "2000", - "reputation": 1497557935185, - "rshares": 660519633, - "voter": "tokichope" - }, - { - "percent": "10000", - "reputation": 429763208250, - "rshares": 4648229546, - "voter": "ghaazi" - }, - { - "percent": "5000", - "reputation": 34825547728550, - "rshares": 5448814828, - "voter": "mandate" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 527396610, - "voter": "kran1" - }, - { - "percent": "150", - "reputation": 0, - "rshares": 6263014593, - "voter": "hivecur" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 526213511, - "voter": "kran2" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 527338054, - "voter": "kran3" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 526384798, - "voter": "kran5" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 578181961, - "voter": "kran6" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 527334496, - "voter": "kran7" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 527462061, - "voter": "kran8" - }, - { - "percent": "4000", - "reputation": 9164403901874, - "rshares": 7868519661, - "voter": "flewsplash" - }, - { - "percent": "7500", - "reputation": 17939114076906, - "rshares": 16234923931, - "voter": "viviana28" - }, - { - "percent": "500", - "reputation": 0, - "rshares": 30186514604, - "voter": "hivecur2" - }, - { - "percent": "10000", - "reputation": 23713278910073, - "rshares": 47157853918, - "voter": "dom.pino" - }, - { - "percent": "5000", - "reputation": 7775140788254, - "rshares": 1696866275, - "voter": "jearo101" - }, - { - "percent": "500", - "reputation": 25284728169845, - "rshares": 140444458069, - "voter": "recoveryinc" - }, - { - "percent": "2500", - "reputation": 76284107957, - "rshares": 6692093171, - "voter": "hive-108278" - }, - { - "percent": "10000", - "reputation": 14818091107386, - "rshares": 16306940122, - "voter": "josemoises" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 7230674965, - "voter": "gohive" - }, - { - "percent": "2900", - "reputation": 15234109271535, - "rshares": 4096516465, - "voter": "vicnzia" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1500605956, - "voter": "enilemor" - }, - { - "percent": "10000", - "reputation": 33401976736, - "rshares": 56372268147, - "voter": "aceh-media" - }, - { - "percent": "2000", - "reputation": 3274158340408, - "rshares": 4237016460, - "voter": "spirall" - }, - { - "percent": "10000", - "reputation": 3005998414144, - "rshares": 4029161201, - "voter": "kraken99" - }, - { - "percent": "500", - "reputation": 0, - "rshares": 5019779700, - "voter": "dying" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 20398678383, - "voter": "vtol79" - }, - { - "percent": "1000", - "reputation": 9930293019864, - "rshares": 730622891, - "voter": "jonalyn2020" - }, - { - "percent": "2000", - "reputation": 2673691564772, - "rshares": 3117939676, - "voter": "yellowdoodle" - }, - { - "percent": "5000", - "reputation": 3679705780578, - "rshares": 7913326698, - "voter": "senseiphil" - }, - { - "percent": "5000", - "reputation": 102092235719, - "rshares": 893287285, - "voter": "clifth" - }, - { - "percent": "10000", - "reputation": 92721511576, - "rshares": 505743307, - "voter": "rhinoceros" - }, - { - "percent": "10000", - "reputation": 305133977360, - "rshares": 1503841372, - "voter": "artxmike" - }, - { - "percent": "10000", - "reputation": 2965518795104, - "rshares": 1420160974, - "voter": "doudoer" - }, - { - "percent": "10000", - "reputation": 654061210157, - "rshares": 910199761, - "voter": "vaishnavi24" - }, - { - "percent": "10000", - "reputation": 121297795184, - "rshares": 110077658, - "voter": "desro" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "baidyabulu" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "brujulamusical" - } - ], - "author": "hiddenblade", - "author_reputation": 353099598336644, - "beneficiaries": [], - "body": "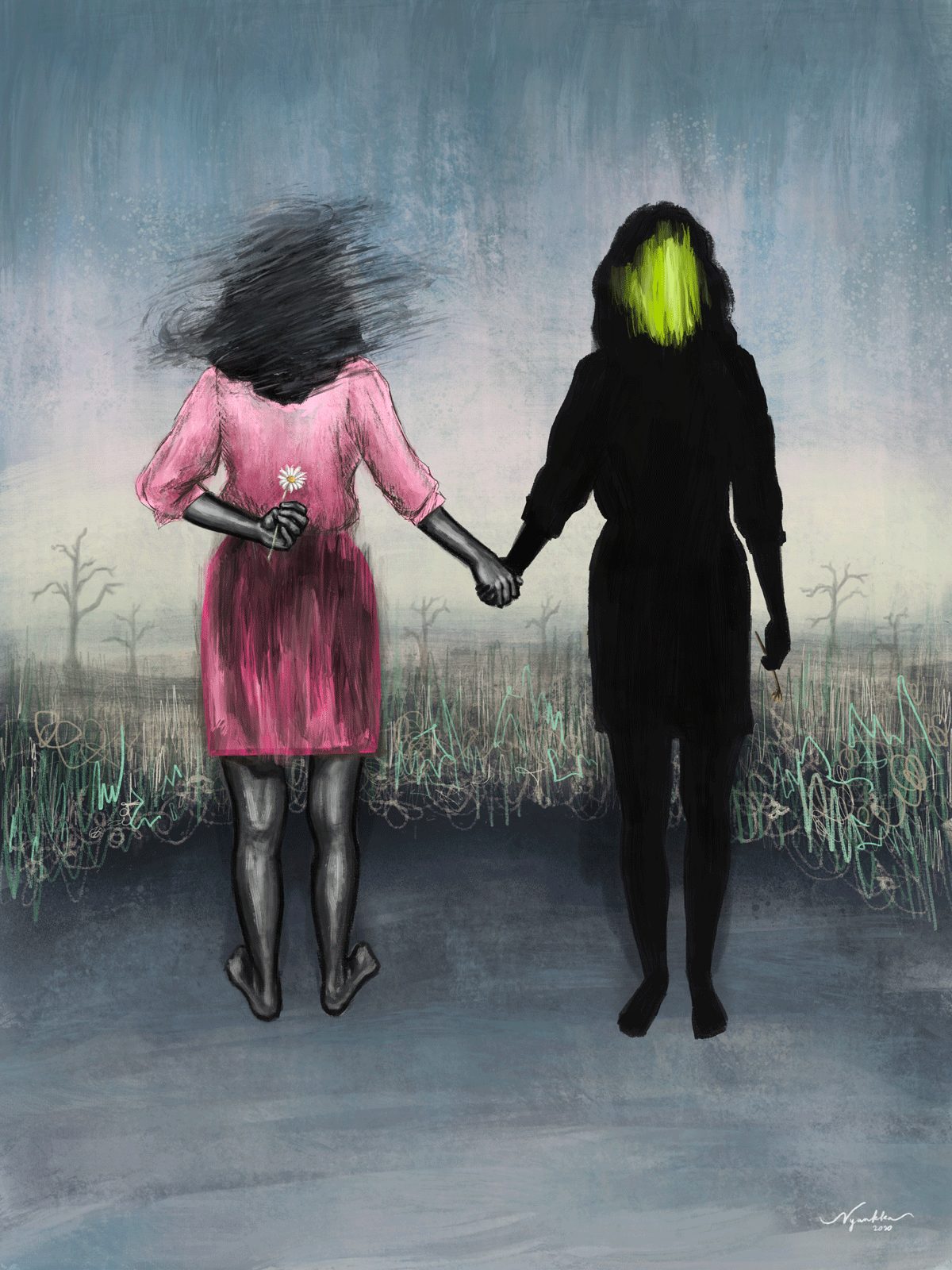\n\n<center> <sub>\nThe Twins\nDigital\n2020\n </sub>\n</center> \n\n<br>\n\n---\n\n<br>\n<div class=text-justify>\n\nA digital painting that I finished a few weeks ago. I was stressing about this too much and I'm just glad that it's already finished and I love the result! I'm pretty burnout at the moment so I can't think of a poem that go with this so interpret it how you like. \n\nI love this personally as it's quite different from my usual works. I mean, most of my subjects are always \"indoors\" and this gives me a creepy kinda surreal vibes. I also love the obvious connection that is seen between the two figures. Like, literally how their hands meet. I'm proud of this work and I personally think it's one of my best works!\n\n<br>\n## Timelapse\nHere's a timelapse of this painting. It is also clear that I was changing the backgrounds and everything too much until I get what really fits it lol cos I didn't have a concrete plan when I started this. I hope you enjoy the process as much as I enjoyed watching it!\n\n\nhttps://youtu.be/IUh4fmFyA-4\n\n<br> <br>\n\n</div>", - "body_length": 1148, - "cashout_time": "2020-11-08T16:46:24", - "category": "hive-156509", - "children": 15, - "created": "2020-11-01T16:46:24", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"app\":\"peakd/2020.10.9\",\"format\":\"markdown\",\"tags\":[\"art\",\"digitalpainting\",\"painting\"],\"image\":[\"https://files.peakd.com/file/peakd-hive/hiddenblade/aYMFK964-The-Twins--s.gif\"]}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-11-01T16:46:24", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 215852490094029, - "parent_author": "", - "parent_permlink": "hive-156509", - "pending_payout_value": "50.798 HBD", - "percent_hbd": 10000, - "permlink": "the-twins", - "post_id": 100346266, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "The Twins", - "title": "The Twins", - "total_payout_value": "0.000 HBD", - "url": "/hive-156509/@hiddenblade/the-twins" - }, - { - "active_votes": [ - { - "percent": "2310", - "reputation": 1049281228830, - "rshares": 5164501450550, - "voter": "enki" - }, - { - "percent": "450", - "reputation": 19245698180508, - "rshares": 97247019478, - "voter": "tombstone" - }, - { - "percent": "10000", - "reputation": 75642328330679, - "rshares": 2017885060319, - "voter": "gregory-f" - }, - { - "percent": "2200", - "reputation": 351157717865710, - "rshares": 418540364545, - "voter": "onealfa" - }, - { - "percent": "2500", - "reputation": 302877660779033, - "rshares": 184767711294, - "voter": "nanzo-scoop" - }, - { - "percent": "2500", - "reputation": 36575940676930, - "rshares": 26132058236, - "voter": "mummyimperfect" - }, - { - "percent": "2500", - "reputation": 8099535991460, - "rshares": 10403615095, - "voter": "ak2020" - }, - { - "percent": "2500", - "reputation": 7060322821389, - "rshares": 766994951, - "voter": "emily-cook" - }, - { - "percent": "10000", - "reputation": 25238894193683, - "rshares": 5729791569, - "voter": "stranger27" - }, - { - "percent": "1500", - "reputation": 124679140412839, - "rshares": 2091889814713, - "voter": "neoxian" - }, - { - "percent": "10000", - "reputation": 229982756480919, - "rshares": 2488281444331, - "voter": "ausbitbank" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 277077616348, - "voter": "livingfree" - }, - { - "percent": "3000", - "reputation": 35502557942867, - "rshares": 91662544468, - "voter": "edouard" - }, - { - "percent": "1000", - "reputation": 560943007638090, - "rshares": 363496824354, - "voter": "ace108" - }, - { - "percent": "10000", - "reputation": 13042944822941, - "rshares": 11701766231, - "voter": "alinalazareva" - }, - { - "percent": "10000", - "reputation": 37558088074509, - "rshares": 18012504763, - "voter": "dolov" - }, - { - "percent": "10000", - "reputation": 8868395123281, - "rshares": 7862976392492, - "voter": "jphamer1" - }, - { - "percent": "10000", - "reputation": 3434871602070, - "rshares": 38086771068, - "voter": "fooblic" - }, - { - "percent": "4100", - "reputation": 133665343804692, - "rshares": 352270837223, - "voter": "borran" - }, - { - "percent": "10000", - "reputation": 97229489927307, - "rshares": 434535096429, - "voter": "rubenalexander" - }, - { - "percent": "10000", - "reputation": 83171240832240, - "rshares": 12470979980, - "voter": "stevescoins" - }, - { - "percent": "10000", - "reputation": 92147852982136, - "rshares": 420340339251, - "voter": "lasseehlers" - }, - { - "percent": "2000", - "reputation": 390433925983792, - "rshares": 222841939378, - "voter": "uwelang" - }, - { - "percent": "10000", - "reputation": 55728416577458, - "rshares": 698764495013, - "voter": "richardcrill" - }, - { - "percent": "5000", - "reputation": 61189446271425, - "rshares": 101763328000, - "voter": "wakeupnd" - }, - { - "percent": "10000", - "reputation": 956789271077715, - "rshares": 7298779445080, - "voter": "aggroed" - }, - { - "percent": "10000", - "reputation": 262691650197556, - "rshares": 109689299200, - "voter": "titusfrost" - }, - { - "percent": "10000", - "reputation": 32517873899432, - "rshares": 166321792770, - "voter": "tfeldman" - }, - { - "percent": "5270", - "reputation": 85981642650865, - "rshares": 58166128298, - "voter": "seckorama" - }, - { - "percent": "5000", - "reputation": 149827038166480, - "rshares": 208661986295, - "voter": "clayboyn" - }, - { - "percent": "10000", - "reputation": 183465603606151, - "rshares": 244272935733, - "voter": "jang" - }, - { - "percent": "10000", - "reputation": 57195255001945, - "rshares": 119262823109, - "voter": "koskl" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 57816934061, - "voter": "ssekulji" - }, - { - "percent": "10000", - "reputation": 23903564446260, - "rshares": 134813739446, - "voter": "r0nd0n" - }, - { - "percent": "5000", - "reputation": 57123303734647, - "rshares": 1062826729, - "voter": "safar01" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 671524915166, - "voter": "created" - }, - { - "percent": "5000", - "reputation": 22050619475702, - "rshares": 30249854859, - "voter": "wagnertamanaha" - }, - { - "percent": "2500", - "reputation": 0, - "rshares": 31632815897, - "voter": "mafeeva" - }, - { - "percent": "10000", - "reputation": 942473876919, - "rshares": 661269532, - "voter": "mow" - }, - { - "percent": "10000", - "reputation": 30766232797635, - "rshares": 18725674823, - "voter": "cardboard" - }, - { - "percent": "3300", - "reputation": 215061230497985, - "rshares": 5163694706495, - "voter": "v4vapid" - }, - { - "percent": "10000", - "reputation": 12645528366358, - "rshares": 20648750384, - "voter": "baah" - }, - { - "percent": "10000", - "reputation": 637780952773, - "rshares": 339810976531, - "voter": "da-dawn" - }, - { - "percent": "10000", - "reputation": 30904254166753, - "rshares": 720873653159, - "voter": "distantsignal" - }, - { - "percent": "10000", - "reputation": 43129757389335, - "rshares": 7165806525, - "voter": "sudutpandang" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 279767388125, - "voter": "teammo" - }, - { - "percent": "10000", - "reputation": 19406409261381, - "rshares": 4147917822117, - "voter": "diggndeeper.com" - }, - { - "percent": "4500", - "reputation": 0, - "rshares": 3846351346839, - "voter": "alexis555" - }, - { - "percent": "10000", - "reputation": 2569106003548, - "rshares": 143993352740, - "voter": "borislavzlatanov" - }, - { - "percent": "10000", - "reputation": 450338781685383, - "rshares": 100681534628, - "voter": "louisthomas" - }, - { - "percent": "5000", - "reputation": 29280462665961, - "rshares": 50849080726, - "voter": "kunschj" - }, - { - "percent": "10000", - "reputation": 39205354424754, - "rshares": 5487127073442, - "voter": "dhimmel" - }, - { - "percent": "500", - "reputation": 1614643030899, - "rshares": 909886005, - "voter": "freebornsociety" - }, - { - "percent": "10000", - "reputation": 10514448764402, - "rshares": 1784957004, - "voter": "syarrf" - }, - { - "percent": "10000", - "reputation": 15465640814476, - "rshares": 3860698816875, - "voter": "sepracore" - }, - { - "percent": "5000", - "reputation": 3199539583247, - "rshares": 5674335453, - "voter": "frankydoodle" - }, - { - "percent": "10000", - "reputation": 74329552872641, - "rshares": 280438250548, - "voter": "mes" - }, - { - "percent": "10000", - "reputation": 46266249863448, - "rshares": 16653136241, - "voter": "arnel" - }, - { - "percent": "10000", - "reputation": 20837541242644, - "rshares": 2026354478, - "voter": "grider123" - }, - { - "percent": "2000", - "reputation": 23391602452678, - "rshares": 76376962507, - "voter": "schlees" - }, - { - "percent": "2000", - "reputation": 5899232344203, - "rshares": 582693360, - "voter": "yeaho" - }, - { - "percent": "10000", - "reputation": 1748651637089, - "rshares": 4006398848, - "voter": "khussan" - }, - { - "percent": "1125", - "reputation": 11448902903972, - "rshares": 561684761209, - "voter": "roomservice" - }, - { - "percent": "10000", - "reputation": 48378473521256, - "rshares": 3702876495, - "voter": "drag33" - }, - { - "percent": "3500", - "reputation": 93577510809146, - "rshares": 26969029073, - "voter": "ironshield" - }, - { - "percent": "10000", - "reputation": 112994806497327, - "rshares": 2674813780, - "voter": "varunpinto" - }, - { - "percent": "10000", - "reputation": 13194990234307, - "rshares": 1339787977, - "voter": "theywillkillyou" - }, - { - "percent": "10000", - "reputation": 9701652715721, - "rshares": 123814329764, - "voter": "masterthematrix" - }, - { - "percent": "5000", - "reputation": 62289248493193, - "rshares": 1197396660, - "voter": "mxzn" - }, - { - "percent": "10000", - "reputation": 7901101123497, - "rshares": 1151166354915, - "voter": "giuatt07" - }, - { - "percent": "10000", - "reputation": 46150080976545, - "rshares": 3539025829822, - "voter": "cryptographic" - }, - { - "percent": "10000", - "reputation": 15824477347297, - "rshares": 14108118985, - "voter": "tracer-paulo" - }, - { - "percent": "2782", - "reputation": 19643697626632, - "rshares": 116379474753, - "voter": "sam99" - }, - { - "percent": "10000", - "reputation": 6831945184021, - "rshares": 507542952904, - "voter": "offoodandart" - }, - { - "percent": "5000", - "reputation": 284852594097573, - "rshares": 3131265212, - "voter": "reseller" - }, - { - "percent": "10000", - "reputation": 29671054086427, - "rshares": 45276642773, - "voter": "deniskj" - }, - { - "percent": "10000", - "reputation": 219366237221002, - "rshares": 595064802922, - "voter": "offgridlife" - }, - { - "percent": "2700", - "reputation": 171498070594985, - "rshares": 111816946001, - "voter": "toofasteddie" - }, - { - "percent": "10000", - "reputation": 39468497815242, - "rshares": 58591626892, - "voter": "jacekw" - }, - { - "percent": "5000", - "reputation": 17642870895991, - "rshares": 34601764417, - "voter": "amblog" - }, - { - "percent": "4600", - "reputation": 190691885658151, - "rshares": 3242966497425, - "voter": "nathanmars" - }, - { - "percent": "6000", - "reputation": 104387564071706, - "rshares": 30846903490, - "voter": "jjprac" - }, - { - "percent": "10000", - "reputation": 69301263729937, - "rshares": 32509897841, - "voter": "bronkong" - }, - { - "percent": "10000", - "reputation": 8523774317190, - "rshares": 272939405447, - "voter": "investingpennies" - }, - { - "percent": "7500", - "reputation": 16251675397221, - "rshares": 165229987437, - "voter": "jasonbu" - }, - { - "percent": "10000", - "reputation": 68883700819155, - "rshares": 1206818164626, - "voter": "msp-waves" - }, - { - "percent": "10000", - "reputation": 204525683313029, - "rshares": 178398987042, - "voter": "vimukthi" - }, - { - "percent": "10000", - "reputation": 38267969484707, - "rshares": 43305579949, - "voter": "tesaganewton" - }, - { - "percent": "3550", - "reputation": 31580212794880, - "rshares": 2480994416, - "voter": "ahlawat" - }, - { - "percent": "10000", - "reputation": 17950931998953, - "rshares": 89108543317, - "voter": "chrisparis" - }, - { - "percent": "10000", - "reputation": 14311794737668, - "rshares": 960437663389, - "voter": "onetin84" - }, - { - "percent": "10000", - "reputation": 6811403363234, - "rshares": 21136495814, - "voter": "ragnarokdel" - }, - { - "percent": "3300", - "reputation": 24639928209538, - "rshares": 19931452964, - "voter": "santigs" - }, - { - "percent": "10000", - "reputation": 196571377648, - "rshares": 17388271165, - "voter": "nadhora" - }, - { - "percent": "2500", - "reputation": 54379045488206, - "rshares": 69823763082, - "voter": "bashadow" - }, - { - "percent": "10000", - "reputation": 51994511985595, - "rshares": 38442200433486, - "voter": "tipu" - }, - { - "percent": "10000", - "reputation": 803746331959, - "rshares": 164232906561, - "voter": "thelordsharvest" - }, - { - "percent": "10000", - "reputation": 170496334031050, - "rshares": 1913687702192, - "voter": "jedigeiss" - }, - { - "percent": "10000", - "reputation": 140483466255317, - "rshares": 474880419117, - "voter": "fbslo" - }, - { - "percent": "750", - "reputation": 112726048778758, - "rshares": 921255028, - "voter": "aamirijaz" - }, - { - "percent": "10000", - "reputation": 669346799793, - "rshares": 19111396810, - "voter": "voxmonkey" - }, - { - "percent": "10000", - "reputation": 110750156547770, - "rshares": 428926264570, - "voter": "drax" - }, - { - "percent": "1500", - "reputation": 445234100950820, - "rshares": 4577098758671, - "voter": "therealwolf" - }, - { - "percent": "10000", - "reputation": 2282968347927, - "rshares": 85597991962, - "voter": "psychkrhoz" - }, - { - "percent": "1000", - "reputation": 336063014350538, - "rshares": 146168558362, - "voter": "revisesociology" - }, - { - "percent": "10000", - "reputation": 121466355474501, - "rshares": 3336027881331, - "voter": "yabapmatt" - }, - { - "percent": "4000", - "reputation": 6727973666699, - "rshares": 66447559562, - "voter": "omra-sky" - }, - { - "percent": "10000", - "reputation": 4237603797309, - "rshares": 483042362, - "voter": "imamalkimas" - }, - { - "percent": "750", - "reputation": 79764359720874, - "rshares": 7713597577, - "voter": "sayee" - }, - { - "percent": "823", - "reputation": 0, - "rshares": 340004644756, - "voter": "investegg" - }, - { - "percent": "10000", - "reputation": 3291168947960, - "rshares": 2944390552, - "voter": "enolife" - }, - { - "percent": "10000", - "reputation": 20376371047950, - "rshares": 30185588611, - "voter": "globetrottergcc" - }, - { - "percent": "10000", - "reputation": 172558630853, - "rshares": 1509909920191, - "voter": "msp-mods" - }, - { - "percent": "10000", - "reputation": 3978902059799, - "rshares": 1194105755, - "voter": "dedarknes" - }, - { - "percent": "7000", - "reputation": 24039483683062, - "rshares": 757508556397, - "voter": "familyprotection" - }, - { - "percent": "10000", - "reputation": 2302152857587, - "rshares": 1714546004, - "voter": "jexus77" - }, - { - "percent": "10000", - "reputation": 91507008146138, - "rshares": 134213347153, - "voter": "zekepickleman" - }, - { - "percent": "1500", - "reputation": 157805541487641, - "rshares": 903206495909, - "voter": "smartsteem" - }, - { - "percent": "2500", - "reputation": 13140240090015, - "rshares": 1231045767, - "voter": "joedukeg" - }, - { - "percent": "10000", - "reputation": 14709760292085, - "rshares": 19718423545, - "voter": "yazp" - }, - { - "percent": "5000", - "reputation": 61257367144299, - "rshares": 586494402, - "voter": "publicumaurora" - }, - { - "percent": "2800", - "reputation": 22087086438734, - "rshares": 15605322638, - "voter": "fjcalduch" - }, - { - "percent": "3000", - "reputation": 14979716889766, - "rshares": 70347234302, - "voter": "cervisia" - }, - { - "percent": "10000", - "reputation": 43698921303676, - "rshares": 47713608736, - "voter": "afril" - }, - { - "percent": "10000", - "reputation": 1585652568356, - "rshares": 545084435, - "voter": "tiazakaria" - }, - { - "percent": "10000", - "reputation": 9071994818465, - "rshares": 667217898, - "voter": "candyboy" - }, - { - "percent": "10000", - "reputation": 23470732984911, - "rshares": 138146950187, - "voter": "aussieninja" - }, - { - "percent": "5000", - "reputation": 21720371357335, - "rshares": 19616019244, - "voter": "bartheek" - }, - { - "percent": "10000", - "reputation": 29574388471678, - "rshares": 1928683669, - "voter": "ydavgonzalez" - }, - { - "percent": "10000", - "reputation": 40170232921, - "rshares": 34316950736, - "voter": "steemvault" - }, - { - "percent": "10000", - "reputation": 8848700369, - "rshares": 936815005, - "voter": "samueladams314" - }, - { - "percent": "10000", - "reputation": 467563251193, - "rshares": 635859047, - "voter": "durbisrodriguez" - }, - { - "percent": "5000", - "reputation": 11568276180723, - "rshares": 38366887422, - "voter": "aagabriel" - }, - { - "percent": "10000", - "reputation": 161107296598465, - "rshares": 738117399171, - "voter": "jarvie" - }, - { - "percent": "10000", - "reputation": 3813598706209, - "rshares": 64247615209, - "voter": "sportscontest" - }, - { - "percent": "10000", - "reputation": 11165935981106, - "rshares": 1285122917, - "voter": "icuz" - }, - { - "percent": "10000", - "reputation": 1352038307664, - "rshares": 805388403, - "voter": "isisfemale" - }, - { - "percent": "10000", - "reputation": 51254284112658, - "rshares": 129440348036, - "voter": "shmoogleosukami" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 938184393, - "voter": "gavinatorial" - }, - { - "percent": "5000", - "reputation": 411588240625, - "rshares": 3831170804, - "voter": "happy-soul" - }, - { - "percent": "10000", - "reputation": 463006291120, - "rshares": 122530498280, - "voter": "photohunt" - }, - { - "percent": "10000", - "reputation": 89781199195, - "rshares": 762248319, - "voter": "photohunter1" - }, - { - "percent": "10000", - "reputation": 75267801675, - "rshares": 1881722812, - "voter": "photohunter2" - }, - { - "percent": "10000", - "reputation": 78606992818, - "rshares": 1104006965, - "voter": "photohunter3" - }, - { - "percent": "10000", - "reputation": 62254250833, - "rshares": 1166293681, - "voter": "photohunter4" - }, - { - "percent": "10000", - "reputation": 71504792482, - "rshares": 1124793554, - "voter": "photohunter5" - }, - { - "percent": "2000", - "reputation": 25585298727868, - "rshares": 70940466, - "voter": "maxpatternman" - }, - { - "percent": "9900", - "reputation": 6264286399143, - "rshares": 2663427088640, - "voter": "asgarth" - }, - { - "percent": "10000", - "reputation": 2506608157147, - "rshares": 73828355184, - "voter": "cst90" - }, - { - "percent": "9900", - "reputation": 3065535471122, - "rshares": 4657582225, - "voter": "mutiahanum" - }, - { - "percent": "10000", - "reputation": 20074444806548, - "rshares": 7544661560, - "voter": "arac" - }, - { - "percent": "10000", - "reputation": 76312761389929, - "rshares": 117019421623, - "voter": "victoriabsb" - }, - { - "percent": "10000", - "reputation": 10610474160807, - "rshares": 228288066859, - "voter": "harpagon" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 29584557005, - "voter": "futurecurrency" - }, - { - "percent": "2500", - "reputation": 18673367244, - "rshares": 10628483246, - "voter": "g4fun" - }, - { - "percent": "5000", - "reputation": 93067317204510, - "rshares": 84553752413, - "voter": "almi" - }, - { - "percent": "7000", - "reputation": 168836422039217, - "rshares": 36326796529, - "voter": "fego" - }, - { - "percent": "10000", - "reputation": 297110152696, - "rshares": 47619656076, - "voter": "properfraction" - }, - { - "percent": "9000", - "reputation": 541369096879, - "rshares": 8163254498, - "voter": "yusrizakaria" - }, - { - "percent": "10000", - "reputation": 5887559768197, - "rshares": 4925460293, - "voter": "nervi" - }, - { - "percent": "10000", - "reputation": 8147228251105, - "rshares": 91741597985, - "voter": "jocieprosza" - }, - { - "percent": "10000", - "reputation": 1140372911417, - "rshares": 829941807, - "voter": "zainnyferdhoy" - }, - { - "percent": "3300", - "reputation": 25978211905358, - "rshares": 85257933085, - "voter": "manniman" - }, - { - "percent": "10000", - "reputation": 7701655982065, - "rshares": 337429914465, - "voter": "schlafhacking" - }, - { - "percent": "10000", - "reputation": 4110594694460, - "rshares": 1052017001515, - "voter": "jkramer" - }, - { - "percent": "10000", - "reputation": 5238378681910, - "rshares": 28336727285, - "voter": "m2nnari" - }, - { - "percent": "2000", - "reputation": 50091053272936, - "rshares": 67211308189, - "voter": "yameen" - }, - { - "percent": "10000", - "reputation": 26253168400063, - "rshares": 2603386538227, - "voter": "rawutah" - }, - { - "percent": "10000", - "reputation": 10532279362821, - "rshares": 3926627468, - "voter": "sallyfun" - }, - { - "percent": "10000", - "reputation": 112109667795, - "rshares": 3107523623, - "voter": "memepress" - }, - { - "percent": "200", - "reputation": 374608224532644, - "rshares": 5032859979, - "voter": "rollandthomas" - }, - { - "percent": "10000", - "reputation": 27390685365705, - "rshares": 21195707498, - "voter": "tsnaks" - }, - { - "percent": "10000", - "reputation": 11437207523627, - "rshares": 33746731479, - "voter": "legendchew" - }, - { - "percent": "10000", - "reputation": 59869537846861, - "rshares": 66677170474, - "voter": "nancybriti" - }, - { - "percent": "10000", - "reputation": 2481629111221, - "rshares": 1825587140, - "voter": "manojbhatt" - }, - { - "percent": "10000", - "reputation": 3640929701953, - "rshares": 235167724019, - "voter": "steemexperience" - }, - { - "percent": "10000", - "reputation": 47468205924858, - "rshares": 14441666564, - "voter": "flores39" - }, - { - "percent": "9900", - "reputation": 1127084830607, - "rshares": 3247961121, - "voter": "irwandarasyid" - }, - { - "percent": "3500", - "reputation": 515972450479, - "rshares": 1316792726, - "voter": "raorac" - }, - { - "percent": "2000", - "reputation": 1072061813246, - "rshares": 4025864181, - "voter": "yaraha" - }, - { - "percent": "660", - "reputation": 9020628089607, - "rshares": 6014585580, - "voter": "promobot" - }, - { - "percent": "10000", - "reputation": 25780595648429, - "rshares": 21868715462, - "voter": "superlao" - }, - { - "percent": "3000", - "reputation": 10484590752220, - "rshares": 56399143431, - "voter": "veteranforcrypto" - }, - { - "percent": "10000", - "reputation": 16913733527980, - "rshares": 28153674542, - "voter": "coloneljethro" - }, - { - "percent": "10000", - "reputation": 3042286903871, - "rshares": 45389698428, - "voter": "jancharlest" - }, - { - "percent": "3000", - "reputation": 0, - "rshares": 861569758, - "voter": "tronsformer" - }, - { - "percent": "10000", - "reputation": 2048957598096, - "rshares": 1530828379244, - "voter": "fw206" - }, - { - "percent": "8000", - "reputation": 35592667940354, - "rshares": 14527110804, - "voter": "zaxan" - }, - { - "percent": "10000", - "reputation": 270502900099691, - "rshares": 126301201377, - "voter": "honoru" - }, - { - "percent": "10000", - "reputation": 1896917288665, - "rshares": 1744478818, - "voter": "steemgridcoin" - }, - { - "percent": "1000", - "reputation": 1917096247997, - "rshares": 537537428, - "voter": "crimo" - }, - { - "percent": "10000", - "reputation": 17999475515816, - "rshares": 10859221580, - "voter": "sk1920" - }, - { - "percent": "10000", - "reputation": 9095206, - "rshares": 836792623627, - "voter": "steem-tube" - }, - { - "percent": "3500", - "reputation": 3267008243675, - "rshares": 4568261558, - "voter": "commonlaw" - }, - { - "percent": "10000", - "reputation": 254937223188, - "rshares": 870380830, - "voter": "svm038" - }, - { - "percent": "10000", - "reputation": 13630732276127, - "rshares": 15512919528, - "voter": "elmundodexao" - }, - { - "percent": "2500", - "reputation": 36276346103051, - "rshares": 4791357378, - "voter": "fitat40" - }, - { - "percent": "5500", - "reputation": 17562491646897, - "rshares": 8836662256, - "voter": "newsnownorthwest" - }, - { - "percent": "2500", - "reputation": 27522752785541, - "rshares": 12758788863, - "voter": "longer" - }, - { - "percent": "2000", - "reputation": 32187725403162, - "rshares": 15291830647, - "voter": "angelica7" - }, - { - "percent": "10000", - "reputation": 33860216986858, - "rshares": 617409057902, - "voter": "donald.porter" - }, - { - "percent": "750", - "reputation": 18701622566955, - "rshares": 2769745769, - "voter": "ambiguity" - }, - { - "percent": "10000", - "reputation": 34329245416723, - "rshares": 104324502334, - "voter": "bflanagin" - }, - { - "percent": "7000", - "reputation": 21771305129128, - "rshares": 2285918697, - "voter": "mightypanda" - }, - { - "percent": "2000", - "reputation": 6592285898816, - "rshares": 12135921957, - "voter": "schlunior" - }, - { - "percent": "5000", - "reputation": 953600633912, - "rshares": 844678603, - "voter": "izzynoel" - }, - { - "percent": "10000", - "reputation": 671978982346, - "rshares": 442547625, - "voter": "sonder-an" - }, - { - "percent": "10000", - "reputation": 17939211305935, - "rshares": 495678813905, - "voter": "raiseup" - }, - { - "percent": "1250", - "reputation": 18790919715448, - "rshares": 163086923187, - "voter": "luppers" - }, - { - "percent": "2500", - "reputation": 7344887427854, - "rshares": 37186187714, - "voter": "altonos" - }, - { - "percent": "1000", - "reputation": 39219460301392, - "rshares": 9820753270, - "voter": "pedrocanella" - }, - { - "percent": "10000", - "reputation": 133998446537867, - "rshares": 4274688573, - "voter": "bluengel" - }, - { - "percent": "10000", - "reputation": 1494229786604, - "rshares": 147590241606, - "voter": "pboulet" - }, - { - "percent": "10000", - "reputation": 604548689345, - "rshares": 1212087309, - "voter": "kr-coffeesteem" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 7871023669, - "voter": "quediceharry" - }, - { - "percent": "10000", - "reputation": 12753472048067, - "rshares": 2277142480, - "voter": "numanbutt" - }, - { - "percent": "2500", - "reputation": 22687035003240, - "rshares": 26411119755, - "voter": "dosh" - }, - { - "percent": "1000", - "reputation": 6441794454523, - "rshares": 685582299, - "voter": "mk992039" - }, - { - "percent": "10000", - "reputation": 2796520970488, - "rshares": 43927523444, - "voter": "truthbot" - }, - { - "percent": "2500", - "reputation": 16966885974746, - "rshares": 878534093, - "voter": "steemitcuration" - }, - { - "percent": "10000", - "reputation": 14619735224503, - "rshares": 4689019480, - "voter": "thisnewgirl" - }, - { - "percent": "10000", - "reputation": 1648086760085, - "rshares": 29817186810, - "voter": "diegor" - }, - { - "percent": "6000", - "reputation": 788852406777, - "rshares": 2111789554, - "voter": "thrasher666" - }, - { - "percent": "4000", - "reputation": 18273031359521, - "rshares": 1467808372, - "voter": "adyorka" - }, - { - "percent": "2500", - "reputation": 65369212708003, - "rshares": 36451877505, - "voter": "marianaemilia" - }, - { - "percent": "3000", - "reputation": 0, - "rshares": 473770240, - "voter": "conectionbot" - }, - { - "percent": "10000", - "reputation": 96044719844697, - "rshares": 1310932967899, - "voter": "actnearn" - }, - { - "percent": "10000", - "reputation": 180239386448888, - "rshares": 8904731835, - "voter": "forecasteem" - }, - { - "percent": "10000", - "reputation": 378578455129, - "rshares": 3335087689, - "voter": "papowich" - }, - { - "percent": "10000", - "reputation": 14501049475964, - "rshares": 56027973003, - "voter": "teenagecrypto" - }, - { - "percent": "10000", - "reputation": 45419481582603, - "rshares": 370940694963, - "voter": "michealb" - }, - { - "percent": "10000", - "reputation": 539147053023232, - "rshares": 48806690100202, - "voter": "theycallmedan" - }, - { - "percent": "3000", - "reputation": 94595445913731, - "rshares": 354817973420, - "voter": "glastar" - }, - { - "percent": "10000", - "reputation": 3633716322140, - "rshares": 1441800881, - "voter": "marki99" - }, - { - "percent": "10000", - "reputation": 1792115358, - "rshares": 1088931797, - "voter": "criptoanarquista" - }, - { - "percent": "10000", - "reputation": 8468960967138, - "rshares": 1912562866, - "voter": "smonia" - }, - { - "percent": "3000", - "reputation": 854097216443, - "rshares": 627860343, - "voter": "redheaddemon" - }, - { - "percent": "10000", - "reputation": 309500689901, - "rshares": 736805168, - "voter": "mariyem" - }, - { - "percent": "10000", - "reputation": 4449571796125, - "rshares": 5435778325, - "voter": "khan.dayyanz" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 961321492, - "voter": "leviackerman" - }, - { - "percent": "10000", - "reputation": 21654779562526, - "rshares": 119026920511, - "voter": "bluerobo" - }, - { - "percent": "5000", - "reputation": 1330122595989, - "rshares": 874881183, - "voter": "zerofive" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1090348344665, - "voter": "gokuisreal" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 2802193400888, - "voter": "realself" - }, - { - "percent": "10000", - "reputation": 671364595259, - "rshares": 2024791864, - "voter": "smon-fan" - }, - { - "percent": "5000", - "reputation": 86443203025994, - "rshares": 22640107488, - "voter": "jacuzzi" - }, - { - "percent": "10000", - "reputation": 117611578301, - "rshares": 74575887919, - "voter": "steemstorage" - }, - { - "percent": "10000", - "reputation": 92013311245, - "rshares": 1473157176, - "voter": "fire451" - }, - { - "percent": "10000", - "reputation": 100094377829, - "rshares": 771643438, - "voter": "seekingalpha" - }, - { - "percent": "3300", - "reputation": 971181267, - "rshares": 36008816721, - "voter": "primeradue" - }, - { - "percent": "10000", - "reputation": 1158706837675, - "rshares": 648472622544, - "voter": "lestrange" - }, - { - "percent": "10000", - "reputation": 2488971014315, - "rshares": 1701848007, - "voter": "tr777" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 611484907, - "voter": "sm-jewel" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1615904460, - "voter": "tr77" - }, - { - "percent": "10000", - "reputation": 429255483, - "rshares": 5554357997467, - "voter": "navyactifit" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1447522244, - "voter": "smoner" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 577521927389, - "voter": "johnmadden" - }, - { - "percent": "250", - "reputation": 30571782588622, - "rshares": 2515377834, - "voter": "brancarosamel" - }, - { - "percent": "10000", - "reputation": 54892997399545, - "rshares": 1036380727, - "voter": "smon-joa" - }, - { - "percent": "2500", - "reputation": 20568275034818, - "rshares": 6686475623, - "voter": "scoopstakes" - }, - { - "percent": "2500", - "reputation": 3785105096670, - "rshares": 4421425794, - "voter": "nanzo-snaps" - }, - { - "percent": "5000", - "reputation": 3561101525046, - "rshares": 22142877414, - "voter": "gribbles" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 7321528959, - "voter": "hanke" - }, - { - "percent": "10000", - "reputation": 3551528709513, - "rshares": 80256182368, - "voter": "holdonla" - }, - { - "percent": "10000", - "reputation": 939385930638, - "rshares": 1502746128, - "voter": "blockbeard" - }, - { - "percent": "10000", - "reputation": 410620631652, - "rshares": 10168845464, - "voter": "ava77" - }, - { - "percent": "5000", - "reputation": 5616865862, - "rshares": 11066842958, - "voter": "abbenay" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 2311022895, - "voter": "sm-skynet" - }, - { - "percent": "5000", - "reputation": 5833218185, - "rshares": 809003246, - "voter": "smonbear" - }, - { - "percent": "3000", - "reputation": 4801514372822, - "rshares": 1182936001, - "voter": "kharma.scribbles" - }, - { - "percent": "10000", - "reputation": 69910542366759, - "rshares": 471211283390, - "voter": "kryptogames" - }, - { - "percent": "10000", - "reputation": 23136498467479, - "rshares": 10631605416, - "voter": "vcdragon" - }, - { - "percent": "9722", - "reputation": 199808905522, - "rshares": 3438804804, - "voter": "bynarikode" - }, - { - "percent": "10000", - "reputation": 469728104061, - "rshares": 1149054445, - "voter": "cindis" - }, - { - "percent": "10000", - "reputation": 1481460968819, - "rshares": 1179543685, - "voter": "linur" - }, - { - "percent": "625", - "reputation": 17277358768462, - "rshares": 802003188, - "voter": "caaio" - }, - { - "percent": "10000", - "reputation": 68146878689125, - "rshares": 532837482776, - "voter": "rollie1212" - }, - { - "percent": "10000", - "reputation": 4663518569101, - "rshares": 891840834458, - "voter": "blue.rabbit" - }, - { - "percent": "2000", - "reputation": 1243018036066, - "rshares": 1351774922, - "voter": "wallvater" - }, - { - "percent": "450", - "reputation": 120936131908085, - "rshares": 33964926610, - "voter": "epicdice" - }, - { - "percent": "2500", - "reputation": 24483130736672, - "rshares": 720459863, - "voter": "dtrade" - }, - { - "percent": "10000", - "reputation": 9877498112396, - "rshares": 1324759691, - "voter": "ssc-token" - }, - { - "percent": "10000", - "reputation": 2179800525681, - "rshares": 819988168, - "voter": "ladeen" - }, - { - "percent": "660", - "reputation": 36087507440149, - "rshares": 52001929003, - "voter": "likwid" - }, - { - "percent": "875", - "reputation": 6484190050495, - "rshares": 7719891334, - "voter": "bastter" - }, - { - "percent": "500", - "reputation": 584484995619, - "rshares": 526508086, - "voter": "tinyhousecryptos" - }, - { - "percent": "10000", - "reputation": 185044670844, - "rshares": 0, - "voter": "tradingideas2" - }, - { - "percent": "500", - "reputation": 20916803594387, - "rshares": 2342555723, - "voter": "leighscotford" - }, - { - "percent": "5200", - "reputation": 268359697210194, - "rshares": 188663765575, - "voter": "maxwellmarcusart" - }, - { - "percent": "2500", - "reputation": 301977647, - "rshares": 45226738090, - "voter": "xyz004" - }, - { - "percent": "10000", - "reputation": 69388355197, - "rshares": 861527614, - "voter": "anosys" - }, - { - "percent": "10000", - "reputation": 17647336098195, - "rshares": 34426584209, - "voter": "c21c" - }, - { - "percent": "10000", - "reputation": 6321736492006, - "rshares": 1796816538, - "voter": "waraira777" - }, - { - "percent": "10000", - "reputation": 35156976882333, - "rshares": 334502349471, - "voter": "cryptobrewmaster" - }, - { - "percent": "1500", - "reputation": 612780456165, - "rshares": 1282167011, - "voter": "freedomring" - }, - { - "percent": "5000", - "reputation": 15808214765405, - "rshares": 330133829649, - "voter": "votebetting" - }, - { - "percent": "10000", - "reputation": 622788295137, - "rshares": 2568638489, - "voter": "ali-h" - }, - { - "percent": "9700", - "reputation": 9929331074, - "rshares": 685320118, - "voter": "gingerbyna" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 908793794, - "voter": "arctis" - }, - { - "percent": "10000", - "reputation": 41533066327739, - "rshares": 10508920115, - "voter": "lordwinty" - }, - { - "percent": "10000", - "reputation": 376803157115, - "rshares": 362706430, - "voter": "tina-tina" - }, - { - "percent": "10000", - "reputation": 211230649528, - "rshares": 1137048602, - "voter": "knightsunited" - }, - { - "percent": "10000", - "reputation": 97507720606, - "rshares": 33583245, - "voter": "gdhaetae" - }, - { - "percent": "5000", - "reputation": 30737900381526, - "rshares": 11122368089, - "voter": "boga4" - }, - { - "percent": "5000", - "reputation": 316994224748, - "rshares": 3875474835, - "voter": "dark-queen" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1637996578, - "voter": "dnflsms" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1264734435, - "voter": "jessy22" - }, - { - "percent": "7500", - "reputation": 17687551130249, - "rshares": 9386904516, - "voter": "bcm" - }, - { - "percent": "10000", - "reputation": 860847639115, - "rshares": 990899859, - "voter": "pogarda" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 764159412, - "voter": "khalneox" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 3713424365, - "voter": "ilidan" - }, - { - "percent": "5000", - "reputation": 1136926765, - "rshares": 661063746, - "voter": "chicoduro" - }, - { - "percent": "10000", - "reputation": 1278938832896, - "rshares": 6855042111, - "voter": "spinvest-neo" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 716987891, - "voter": "rehan-pal" - }, - { - "percent": "1250", - "reputation": 68095377181977, - "rshares": 5092929448, - "voter": "tariqul.bibm" - }, - { - "percent": "10000", - "reputation": 8018069095949, - "rshares": 10896888676, - "voter": "madgol" - }, - { - "percent": "2000", - "reputation": 133245362827, - "rshares": 1480638514, - "voter": "mvanhauten" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1308430757, - "voter": "keepit2" - }, - { - "percent": "10000", - "reputation": 1626985046, - "rshares": 1550000000, - "voter": "vasitocoin" - }, - { - "percent": "2000", - "reputation": 14577043701834, - "rshares": 3072479127, - "voter": "julesquirin" - }, - { - "percent": "5000", - "reputation": 679076789336, - "rshares": 14027280480, - "voter": "davidlionfish" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1046864688, - "voter": "dalz4" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 936890146, - "voter": "libertypal27" - }, - { - "percent": "10000", - "reputation": 8008056893145, - "rshares": 186981297254, - "voter": "khazrakh" - }, - { - "percent": "10000", - "reputation": 1588836327, - "rshares": 0, - "voter": "wasifbhutto" - }, - { - "percent": "10000", - "reputation": 26055895370219, - "rshares": 26721303213, - "voter": "shinoxl" - }, - { - "percent": "1500", - "reputation": 15639069180, - "rshares": 0, - "voter": "dagadu" - }, - { - "percent": "10000", - "reputation": 86868289888522, - "rshares": 2134433936987, - "voter": "peakd" - }, - { - "percent": "5400", - "reputation": 120929866340, - "rshares": 1953887412, - "voter": "terezka1" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 2180710972, - "voter": "blue-witness" - }, - { - "percent": "10000", - "reputation": 37484144443, - "rshares": 415291878093, - "voter": "mountaingod" - }, - { - "percent": "400", - "reputation": 91987050007999, - "rshares": 150107546, - "voter": "hiveyoda" - }, - { - "percent": "10000", - "reputation": 8390312, - "rshares": 1200214538, - "voter": "hivevenezuela" - }, - { - "percent": "4300", - "reputation": 1209731647511, - "rshares": 693654148537, - "voter": "softworld" - }, - { - "percent": "10000", - "reputation": 15744785453353, - "rshares": 16477603691, - "voter": "beerguide" - }, - { - "percent": "10000", - "reputation": 8499617108715, - "rshares": 54115322471, - "voter": "martasugiriy" - }, - { - "percent": "10000", - "reputation": 959238840371, - "rshares": 30020527445, - "voter": "mark3004" - }, - { - "percent": "10000", - "reputation": 2000419940779, - "rshares": 3558088998, - "voter": "polyannie" - }, - { - "percent": "1125", - "reputation": 47896334821902, - "rshares": 15883700899, - "voter": "hiveonboard" - }, - { - "percent": "500", - "reputation": 604442758770, - "rshares": 22355928401, - "voter": "ninnu" - }, - { - "percent": "500", - "reputation": 9650263289467, - "rshares": 2727600812, - "voter": "localgrower" - }, - { - "percent": "2000", - "reputation": 19058471657, - "rshares": 1298090224, - "voter": "vibrasphere" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 947971475, - "voter": "yugimuto" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 947226951, - "voter": "magodelcaosnegro" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 939721273, - "voter": "hivebtc" - }, - { - "percent": "1000", - "reputation": 0, - "rshares": 794823979282, - "voter": "nautilus-up" - }, - { - "percent": "10000", - "reputation": 50677675399, - "rshares": 0, - "voter": "pyramidone" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 949128815, - "voter": "sagadegeminis" - }, - { - "percent": "10000", - "reputation": 37908486627936, - "rshares": 28793819034, - "voter": "ykroys" - }, - { - "percent": "10000", - "reputation": 17583644867, - "rshares": 317558532, - "voter": "heinkhantmaung" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 944836698, - "voter": "constantinopla" - }, - { - "percent": "10000", - "reputation": 8142738000461, - "rshares": 12590637384, - "voter": "splintern" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 940423479, - "voter": "bellaciao" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 943619026, - "voter": "jesucristo" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 943465244, - "voter": "yodinzaku" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 943325627, - "voter": "lordarianthus" - }, - { - "percent": "4600", - "reputation": 65519590598, - "rshares": 884619697, - "voter": "plusvault" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 942978447, - "voter": "spiritminer" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 942449107, - "voter": "drfate" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 942438039, - "voter": "confucio" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 942184362, - "voter": "splintergod" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 942259241, - "voter": "magnor" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 941178230, - "voter": "meele" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 941246194, - "voter": "kretchtallevor" - }, - { - "percent": "10000", - "reputation": 17804701, - "rshares": 1680253269, - "voter": "notaboutme" - }, - { - "percent": "500", - "reputation": 0, - "rshares": 31288366705, - "voter": "hivecur2" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 939694453, - "voter": "onyxsentinel" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 939548272, - "voter": "khymeria" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 939579369, - "voter": "sicmundus" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1193390329, - "voter": "lainiwakura" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 938595419, - "voter": "roymustang" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 938597760, - "voter": "darkarmy" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 938181397, - "voter": "reiayanami" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 938348378, - "voter": "kaiosama" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 938153790, - "voter": "pitagoras" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 938301502, - "voter": "steinitz" - }, - { - "percent": "5000", - "reputation": 906267166974, - "rshares": 5209989894, - "voter": "sevenoh-fiveoh" - }, - { - "percent": "10000", - "reputation": 3005998414144, - "rshares": 2960301200, - "voter": "kraken99" - }, - { - "percent": "10000", - "reputation": 10742256157644, - "rshares": 9095654049, - "voter": "motherhood" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 18986000318, - "voter": "vtol79" - }, - { - "percent": "6500", - "reputation": 837854855679, - "rshares": 20776441195, - "voter": "rituraz17" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1428698400, - "voter": "anillounico" - }, - { - "percent": "5000", - "reputation": 449110004, - "rshares": 1436395629, - "voter": "magnolia-maggie" - }, - { - "percent": "10000", - "reputation": 3452328685273, - "rshares": 6116009077, - "voter": "aaalviarez" - }, - { - "percent": "1000", - "reputation": 9727854376347, - "rshares": 1764667299, - "voter": "borbolet" - }, - { - "percent": "5000", - "reputation": 3679705780578, - "rshares": 7645213173, - "voter": "senseiphil" - }, - { - "percent": "10000", - "reputation": 26404156, - "rshares": 986084096, - "voter": "zangano" - }, - { - "percent": "5000", - "reputation": 31934947340, - "rshares": 1010597462, - "voter": "sovereign-apis" - }, - { - "percent": "10000", - "reputation": 136169900291, - "rshares": 1479643153, - "voter": "revise.spt" - }, - { - "percent": "10000", - "reputation": 4048629470155, - "rshares": 5110381049, - "voter": "dubishin" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 38622489517, - "voter": "didcraftbeer" - }, - { - "percent": "10000", - "reputation": 681362515038, - "rshares": 1827804091, - "voter": "m2fermin" - }, - { - "percent": "10000", - "reputation": 654061210157, - "rshares": 654994158, - "voter": "vaishnavi24" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "sameer78" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "vene-a-jaja" - } - ], - "author": "aggroed", - "author_reputation": 956789271077715, - "beneficiaries": [], - "body": "\n\nHive-Engine is an opensource, layer-2, smart contract platform built on top of Hive, which powers communities and Dapps. Hive-Engine is seeking development funding to provide a more decentralized service, a greater range of off-the-shelf, modular, smart contracts that developers and dapp designers can use to create novel services, communities, and dapps, a number of back end performance enhancements, and lastly better interoperability with ETH and BTC.\n\n# What's the purpose of Hive-Engine?\n\nHive-Engine is a witness project managed by @aggroed which seeks to develop the Hive ecosystem by enabling community formation and dapp creation. The backend is an opensource community project hosted on github. The front-end, hive-engine.com as well as tribaldex.com are part of a private business.\n\n# Who are the founders of Hive-Engine?\n\nThe original founders of Hive-Engine are yabapamatt, aggroed, and harpagon.\n\n# Who works on the project now?\n\naggroed - Director\ncryptomancer - smart contract and dev ops lead\nlion200 - dswap & hive-engine.com front-end development lead\nbait002 - web designer, mobile app designer\nreaziliqbal - front end developer, and dapp developer\neonwarped - Nitrous creator and developer, smart contract developer\ndonchate - smart contract developer\ninertia - documentation and block explorer guru\ngerber - advisor and support\nclayboyn - customer support lead\nsomeguy123 - Privex support for the crypto token converter\n\n# What can Hive-Engine do now?\n\n- Hive Engine supports community and dapp creation. Hive-Engine users can create:\n\n- Fungible Tokens - which can be allowed to stake or be delegated, these power communities and businesses.\n\n- Nitrous - A fork of condenser, which uses Fungible Tokens created on Hive-Engine as an additional reward pool. Essentially users can create their own blog with rewards.\n\n- PoS mining - This is supported both as an off the chain and on-chain smart contract. Users can create inflation pools and doll out rewards to stakeholders.\n\n- Non-Fungible Tokens- NFTs from Hive-Engine power multiple Dapps already. Dcity, Rising Star, and nftshowroom are 3 examples. \n\n- Nested FTs in NFTs - Users can create NFTs with FTs inside of them.\n\n- Beechat - A chat tool (and mobile app soon) that can be used to communicate between members of the Hive Community. It's able to be integrated for free into any dapp. The client side is open source.\n\n# What is Hive-Engine developing?\n\nHive-Engine is looking to be the home of no-code, modular, smart contracts, which a project manager can integrate into services and dapps without the need to code anything (just configure). By doing this we're hoping to make Hive the easiest place in crypto to create a community and form a business.\n\nIn order to have the best experience possible we're looking to implement several things:\n\n1. A P2P system \n2. More modular smart contracts\n3. General performance enhancements\n4. Bridge to Ethereum and ERC20s \n5. BTC markets\n\n# What's are the benefits?\n\n1. A P2P system helps ensure that servers operate a specific way and continue to operate that way. Hive-Engine can make unilateral decisions on hard-forks now, which is a concern to many users. By implementing a voting system and ensuring that the network consents to upgrade the system is protected from unpopular and potentially unethical changes.\n\n2. Modular Smart Contracts - The range of options to Dapp designers and features of various websites are completely dependent on what Smart Contracts exist. While not every contract should live on the Hive-Engine platform itself there's many examples of smart contracts which benefit multiple apps, which we intend to create. Examples include:\n\nPack Manager - sell packs containing randomized NFTs\nBid Markets - Bid market where users can put up funds to purchase certain NFTs at a specific price\nAuction Contracts - ebay inspired auction of NFTs\nLiquidity Pools - Uniswap inspired liquidity pools\nWitness-style Voting Systems - community leadership by consensus\nAutomated Payouts - Automated payouts for convenience and dapp development\nAir Drops - trustless air drops\nClaim Drops - trustless claim drops\n\nThese projects typically take at least a month by seasoned developers. Coding can typically be done much faster, but unit testing and maximizing resource efficiency on the blockchain end up being significant contributors.\n\nEach one also needs front-end website development to allow a simple interface for community and dapp developers to easily utilize them.\n\n3. General Performance Enhancements - full block log replays take 2.5 days to complete. That's pretty long. We're looking to cut that down both for the core node and account history.\n\n4. Bridge to Ethereum and ERC20s - Hive is a fantastic resource for developing community and dapps, but one place that it's severely weak is in the price of the $HIVE token. This one gap creates endless headaches in that it's difficult to get the funding required to build here because HIVE is essentially looked at as a backwater chain by serious blockchain investors. To overcome this gap we have to tie the financial markets of Ethereum to the ease of use of Hive, and that can be accomplished by created SWAP.ETH, SWAP.ERC20s, SWAP.ERC721s as well as the reverse action of creation Wrapped Hive, Hive-Engine Fungible Tokens, and lastly Hive-Engine NFTs.\n\n5. BTC Markets - every exchange out there bases trading pairs on BTC. Hive-Engine needs to add the same capability so that users can choose their market of trading tokens against BTC or Hive.\n\n# Budget\n\nI'm requesting 1000 HBD for 100 days for a total of 100,000 HBD. \n\nP2P development and testing - 20,000 HBD\nSmart Contract Development - 40,000 HBD\nPerformance Enhancements - 20,000 HBD\nEthereum Bridge - 10,000 HBD\nBTC market - 10,000 HBD\n\nPayment request will start Nov 24 (after the refund proposal is finished).\n\n# What's the result?\n\nBy funding this project over the next 3 months Hive-Engine can continue development of the smart contract platform as a base for community and business growth, in a provably fair and community driven ecosystem, which is connected to the financial powerhouses of BTC and ETH. By combining ease of use of Hive/Hive-Engine with new modular smart contract capabilities powered by Hive-Engine and powered by the capital markets of the larger marketcap coins Hive will finally be setup for community and business growth.\n\nIf you want people, you need communities and businesses, if you want those they need an easy to use and robust smart contract platform to use. Hive-Engine is built for that goal specifically and needs your help to get all the pieces in place to do it.\n\nNote these funds are ~98% for the development of the public, open source, community driven backend aspects of Hive-Engine and only a minor part are devoted to creating a handful of front-end forms for the smart contracts on hive-engine.com.\n\n\n", - "body_length": 7011, - "cashout_time": "2020-11-10T14:29:09", - "category": "dhf", - "children": 66, - "created": "2020-11-03T14:29:09", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"app\":\"peakd/2020.10.9\",\"format\":\"markdown\",\"description\":\"A proposal to the DHF for Hive-Engine opensource development of the Hive Engine Smart Contract Platform.\",\"tags\":[\"dhf\",\"proposal\",\"hive\",\"hive-engine\",\"dapp\",\"smartcontract\"],\"users\":[\"aggroed\"],\"links\":[\"/@aggroed\"],\"image\":[\"https://files.peakd.com/file/peakd-hive/aggroed/j14ctB8e-image.png\"]}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-11-03T14:29:09", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 207338343395682, - "parent_author": "", - "parent_permlink": "dhf", - "pending_payout_value": "48.702 HBD", - "percent_hbd": 10000, - "permlink": "hive-engine-dao-funding-proposal", - "post_id": 100374668, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Hive-Engine DAO Funding Proposal", - "title": "Hive-Engine DAO Funding Proposal", - "total_payout_value": "0.000 HBD", - "url": "/dhf/@aggroed/hive-engine-dao-funding-proposal" - }, - { - "active_votes": [ - { - "percent": "3000", - "reputation": 19245698180508, - "rshares": 645268425523, - "voter": "tombstone" - }, - { - "percent": "1000", - "reputation": 341233778618, - "rshares": 156642390452, - "voter": "boatymcboatface" - }, - { - "percent": "10000", - "reputation": 211696269298912, - "rshares": 8097882207143, - "voter": "pfunk" - }, - { - "percent": "10000", - "reputation": 237896238421327, - "rshares": 693937764440, - "voter": "ash" - }, - { - "percent": "1000", - "reputation": 1957220358850383, - "rshares": 375514953460, - "voter": "kingscrown" - }, - { - "percent": "6000", - "reputation": 1594304819447449, - "rshares": 6353671804172, - "voter": "acidyo" - }, - { - "percent": "1000", - "reputation": 4509451541223, - "rshares": 42029432451, - "voter": "theshell" - }, - { - "percent": "10000", - "reputation": 2119699556937, - "rshares": 227146739394, - "voter": "sqlinsix" - }, - { - "percent": "5000", - "reputation": 42689564397220, - "rshares": 338201630747, - "voter": "kenny-crane" - }, - { - "percent": "960", - "reputation": 20148016993603, - "rshares": 167163490148, - "voter": "gerber" - }, - { - "percent": "960", - "reputation": 639040633115794, - "rshares": 641067049080, - "voter": "ezzy" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 275936077840, - "voter": "livingfree" - }, - { - "percent": "10000", - "reputation": 11367396598462, - "rshares": 100531155058, - "voter": "drakernoise" - }, - { - "percent": "10000", - "reputation": 273738883635043, - "rshares": 1228211699120, - "voter": "inertia" - }, - { - "percent": "10000", - "reputation": 315496990256842, - "rshares": 5469189887883, - "voter": "arcange" - }, - { - "percent": "960", - "reputation": 1231399052746489, - "rshares": 397740452452, - "voter": "exyle" - }, - { - "percent": "1237", - "reputation": 76598598706463, - "rshares": 8689601747, - "voter": "sharker" - }, - { - "percent": "10000", - "reputation": 501315151365, - "rshares": 109819881341, - "voter": "raphaelle" - }, - { - "percent": "700", - "reputation": 560943007638090, - "rshares": 260245385666, - "voter": "ace108" - }, - { - "percent": "1375", - "reputation": 5762427931179, - "rshares": 4105746383, - "voter": "kibela" - }, - { - "percent": "1000", - "reputation": 13042944822941, - "rshares": 1123001872, - "voter": "alinalazareva" - }, - { - "percent": "10000", - "reputation": 8868395123281, - "rshares": 6720113513844, - "voter": "jphamer1" - }, - { - "percent": "10000", - "reputation": 143768203235023, - "rshares": 16344176469, - "voter": "shanghaipreneur" - }, - { - "percent": "9900", - "reputation": 3434871602070, - "rshares": 36795994580, - "voter": "fooblic" - }, - { - "percent": "5000", - "reputation": 50032785591993, - "rshares": 522910802929, - "voter": "bryan-imhoff" - }, - { - "percent": "10000", - "reputation": 14314005204488, - "rshares": 156868333696, - "voter": "fingolfin" - }, - { - "percent": "4500", - "reputation": 133665343804692, - "rshares": 368104481173, - "voter": "borran" - }, - { - "percent": "40", - "reputation": 316730231019855, - "rshares": 2102917340, - "voter": "bert0" - }, - { - "percent": "2500", - "reputation": 8401560618909, - "rshares": 8002817332, - "voter": "anech512" - }, - { - "percent": "10000", - "reputation": 32866384013620, - "rshares": 100935911088, - "voter": "frankbacon" - }, - { - "percent": "2000", - "reputation": 363924035887507, - "rshares": 284108738386, - "voter": "steevc" - }, - { - "percent": "864", - "reputation": 215380666736, - "rshares": 162009753862, - "voter": "netaterra" - }, - { - "percent": "960", - "reputation": 96871175157544, - "rshares": 166962752707, - "voter": "someguy123" - }, - { - "percent": "10000", - "reputation": 6166374189863, - "rshares": 37115742184, - "voter": "sammie" - }, - { - "percent": "5000", - "reputation": 96059856770036, - "rshares": 8202985558918, - "voter": "canadian-coconut" - }, - { - "percent": "10000", - "reputation": 262691650197556, - "rshares": 115751950992, - "voter": "titusfrost" - }, - { - "percent": "900", - "reputation": 120512824315021, - "rshares": 343949070688, - "voter": "funnyman" - }, - { - "percent": "500", - "reputation": 280674585514224, - "rshares": 48626254983, - "voter": "justyy" - }, - { - "percent": "10000", - "reputation": 244475173484259, - "rshares": 161000648672, - "voter": "nelyp" - }, - { - "percent": "5000", - "reputation": 149827038166480, - "rshares": 198544877421, - "voter": "clayboyn" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 39959622318, - "voter": "ssekulji" - }, - { - "percent": "5000", - "reputation": 57123303734647, - "rshares": 1062826729, - "voter": "safar01" - }, - { - "percent": "5000", - "reputation": 121575052614664, - "rshares": 48064529396, - "voter": "vannour" - }, - { - "percent": "5000", - "reputation": 8998674935359, - "rshares": 534796911, - "voter": "steemcultures" - }, - { - "percent": "5000", - "reputation": 1140777865558, - "rshares": 1785513584, - "voter": "steemworld" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 670809071053, - "voter": "created" - }, - { - "percent": "5000", - "reputation": 2100858010527, - "rshares": 579442804, - "voter": "creat" - }, - { - "percent": "2500", - "reputation": 16372175688451, - "rshares": 880054063, - "voter": "mapesa" - }, - { - "percent": "10000", - "reputation": 4110132379317, - "rshares": 18505569296, - "voter": "sabsel" - }, - { - "percent": "1000", - "reputation": 7547083616142, - "rshares": 12248679459, - "voter": "edb" - }, - { - "percent": "5000", - "reputation": 8910166842489, - "rshares": 571653442, - "voter": "cisah" - }, - { - "percent": "5000", - "reputation": 22050619475702, - "rshares": 28301698270, - "voter": "wagnertamanaha" - }, - { - "percent": "10000", - "reputation": 306546327230906, - "rshares": 17343282192, - "voter": "teamhumble" - }, - { - "percent": "10000", - "reputation": 942473876919, - "rshares": 649479151, - "voter": "mow" - }, - { - "percent": "10000", - "reputation": 30766232797635, - "rshares": 18303038744, - "voter": "cardboard" - }, - { - "percent": "5000", - "reputation": 708133861814, - "rshares": 1137247470, - "voter": "pedir-museum" - }, - { - "percent": "95", - "reputation": 11219538466960, - "rshares": 598179542, - "voter": "lastminuteman" - }, - { - "percent": "3000", - "reputation": 27214240242904, - "rshares": 25147970206, - "voter": "rahul.stan" - }, - { - "percent": "10000", - "reputation": 96057608537550, - "rshares": 72569825313, - "voter": "kotturinn" - }, - { - "percent": "5000", - "reputation": 60770071429, - "rshares": 633370021, - "voter": "aceh" - }, - { - "percent": "300", - "reputation": 38975615169260, - "rshares": 7699044536, - "voter": "steemitboard" - }, - { - "percent": "3100", - "reputation": 0, - "rshares": 2628043948360, - "voter": "alexis555" - }, - { - "percent": "10000", - "reputation": 450338781685383, - "rshares": 114716880350, - "voter": "louisthomas" - }, - { - "percent": "2910", - "reputation": 203599542560635, - "rshares": 1876206676, - "voter": "lordneroo" - }, - { - "percent": "10000", - "reputation": 39205354424754, - "rshares": 6692383659487, - "voter": "dhimmel" - }, - { - "percent": "10000", - "reputation": 130869649256432, - "rshares": 243631005664, - "voter": "elevator09" - }, - { - "percent": "5000", - "reputation": 295913411385, - "rshares": 655055111, - "voter": "munzir" - }, - { - "percent": "1000", - "reputation": 2545935026777, - "rshares": 11183123501, - "voter": "frankk" - }, - { - "percent": "3000", - "reputation": 87650171273192, - "rshares": 42606460147, - "voter": "sidwrites" - }, - { - "percent": "960", - "reputation": 21701614328748, - "rshares": 72267309971, - "voter": "dune69" - }, - { - "percent": "3000", - "reputation": 42214550905806, - "rshares": 1188176200474, - "voter": "smasssh" - }, - { - "percent": "10000", - "reputation": 74329552872641, - "rshares": 255892385708, - "voter": "mes" - }, - { - "percent": "595", - "reputation": 39969242565947, - "rshares": 10799196678, - "voter": "iansart" - }, - { - "percent": "3000", - "reputation": 30070248546777, - "rshares": 58836840000, - "voter": "eliel" - }, - { - "percent": "50", - "reputation": 358172961752718, - "rshares": 7356180457, - "voter": "jerrybanfield" - }, - { - "percent": "5000", - "reputation": 93577510809146, - "rshares": 39618454448, - "voter": "ironshield" - }, - { - "percent": "10000", - "reputation": 26275815650202, - "rshares": 75321418800, - "voter": "trumpikas" - }, - { - "percent": "10000", - "reputation": 108813324772579, - "rshares": 396846476687, - "voter": "nanosesame" - }, - { - "percent": "450", - "reputation": 58768713310116, - "rshares": 932027233, - "voter": "improv" - }, - { - "percent": "1500", - "reputation": 62289248493193, - "rshares": 462465161, - "voter": "mxzn" - }, - { - "percent": "2976", - "reputation": 23577497062186, - "rshares": 654473256105, - "voter": "alphacore" - }, - { - "percent": "2500", - "reputation": 36400689869483, - "rshares": 135124759297, - "voter": "maxer27" - }, - { - "percent": "2900", - "reputation": 19643697626632, - "rshares": 118551376181, - "voter": "sam99" - }, - { - "percent": "10000", - "reputation": 110211133478274, - "rshares": 1596331540084, - "voter": "drakos" - }, - { - "percent": "900", - "reputation": 122017350265257, - "rshares": 182528720424, - "voter": "ew-and-patterns" - }, - { - "percent": "160", - "reputation": 2826592035, - "rshares": 963577177, - "voter": "techken" - }, - { - "percent": "2500", - "reputation": 14311160567730, - "rshares": 10860272538, - "voter": "wesphilbin" - }, - { - "percent": "2000", - "reputation": 113462562962552, - "rshares": 548480841, - "voter": "furious-one" - }, - { - "percent": "3000", - "reputation": 62019238048390, - "rshares": 53293983201, - "voter": "gniksivart" - }, - { - "percent": "2910", - "reputation": 184191136030059, - "rshares": 277964467439, - "voter": "runicar" - }, - { - "percent": "10000", - "reputation": 29666985623497, - "rshares": 95762802646, - "voter": "vallesleoruther" - }, - { - "percent": "2400", - "reputation": 85086374950236, - "rshares": 77116217648, - "voter": "codingdefined" - }, - { - "percent": "10000", - "reputation": 145609724101, - "rshares": 974584809842, - "voter": "hope-on-fire" - }, - { - "percent": "2910", - "reputation": 466532027405, - "rshares": 446697071, - "voter": "dado13btc" - }, - { - "percent": "10000", - "reputation": 23747652895956, - "rshares": 918691916, - "voter": "richi0927" - }, - { - "percent": "960", - "reputation": 18018060826148, - "rshares": 4307901595, - "voter": "shitsignals" - }, - { - "percent": "7000", - "reputation": 135808252885028, - "rshares": 2461277958091, - "voter": "stayoutoftherz" - }, - { - "percent": "5000", - "reputation": 190691885658151, - "rshares": 3598513300975, - "voter": "nathanmars" - }, - { - "percent": "3000", - "reputation": 115269542036380, - "rshares": 394920458333, - "voter": "vikisecrets" - }, - { - "percent": "5000", - "reputation": 1844447662772, - "rshares": 13874064631, - "voter": "khalil319" - }, - { - "percent": "10000", - "reputation": 1465267842688, - "rshares": 1510963065, - "voter": "jorge248" - }, - { - "percent": "7000", - "reputation": 616021827459, - "rshares": 8309189919, - "voter": "yacobh" - }, - { - "percent": "3000", - "reputation": 8780411474631, - "rshares": 1960041905, - "voter": "stinawog" - }, - { - "percent": "3000", - "reputation": 45409555092812, - "rshares": 3282920414, - "voter": "ernick" - }, - { - "percent": "2500", - "reputation": 52406600539994, - "rshares": 30554615032, - "voter": "fionasfavourites" - }, - { - "percent": "2910", - "reputation": 3449425709461, - "rshares": 57898456222, - "voter": "pardeepkumar" - }, - { - "percent": "960", - "reputation": 173069624727027, - "rshares": 45933214291, - "voter": "felander" - }, - { - "percent": "4950", - "reputation": 24639928209538, - "rshares": 28384851485, - "voter": "santigs" - }, - { - "percent": "10000", - "reputation": 196571377648, - "rshares": 17762398424, - "voter": "nadhora" - }, - { - "percent": "10000", - "reputation": 11131064226204, - "rshares": 13446076454, - "voter": "angelusnoctum" - }, - { - "percent": "2500", - "reputation": 54379045488206, - "rshares": 67088597396, - "voter": "bashadow" - }, - { - "percent": "10000", - "reputation": 51994511985595, - "rshares": 42412101134664, - "voter": "tipu" - }, - { - "percent": "400", - "reputation": 101280142849344, - "rshares": 11200356877, - "voter": "kimzwarch" - }, - { - "percent": "2100", - "reputation": 80455071898751, - "rshares": 11820317299, - "voter": "crokkon" - }, - { - "percent": "500", - "reputation": 47942503950741, - "rshares": 50980131657, - "voter": "accelerator" - }, - { - "percent": "5000", - "reputation": 128229050079, - "rshares": 2002759013, - "voter": "cconn" - }, - { - "percent": "960", - "reputation": 731791169403, - "rshares": 8729095257, - "voter": "yogacoach" - }, - { - "percent": "10000", - "reputation": 537290602112, - "rshares": 286489322235, - "voter": "chinchilla" - }, - { - "percent": "5000", - "reputation": 167991810520774, - "rshares": 347262135206, - "voter": "celestal" - }, - { - "percent": "3000", - "reputation": 23783050750962, - "rshares": 1333888149, - "voter": "estream.studios" - }, - { - "percent": "10000", - "reputation": 82083033108, - "rshares": 2833718222, - "voter": "tomwafula" - }, - { - "percent": "10000", - "reputation": 445234100950820, - "rshares": 31739506316105, - "voter": "therealwolf" - }, - { - "percent": "5000", - "reputation": 72384838675160, - "rshares": 323896372886, - "voter": "roleerob" - }, - { - "percent": "6000", - "reputation": 336063014350538, - "rshares": 875767575841, - "voter": "revisesociology" - }, - { - "percent": "10000", - "reputation": 206055018792025, - "rshares": 58851841199, - "voter": "silversaver888" - }, - { - "percent": "2400", - "reputation": 46994381748371, - "rshares": 1504947502, - "voter": "rakkasan84" - }, - { - "percent": "10000", - "reputation": 20299637563719, - "rshares": 5118747752, - "voter": "critic-on" - }, - { - "percent": "10000", - "reputation": 19533997928933, - "rshares": 37906033949, - "voter": "daisyphotography" - }, - { - "percent": "845", - "reputation": 0, - "rshares": 339638996176, - "voter": "investegg" - }, - { - "percent": "2500", - "reputation": 141970828773777, - "rshares": 412208377426, - "voter": "tobetada" - }, - { - "percent": "912", - "reputation": 7232716062458, - "rshares": 18082891823, - "voter": "caladan" - }, - { - "percent": "10000", - "reputation": 29578711833199, - "rshares": 68846044788, - "voter": "captainklaus" - }, - { - "percent": "10000", - "reputation": 1737660226555, - "rshares": 1490397434, - "voter": "meincluyo" - }, - { - "percent": "10000", - "reputation": 24039483683062, - "rshares": 1082994959073, - "voter": "familyprotection" - }, - { - "percent": "10000", - "reputation": 473928985768900, - "rshares": 2979294009, - "voter": "tradingideas" - }, - { - "percent": "960", - "reputation": 377317663062316, - "rshares": 250676472763, - "voter": "emrebeyler" - }, - { - "percent": "10000", - "reputation": 1338862822184, - "rshares": 4252536827, - "voter": "potplucker" - }, - { - "percent": "10000", - "reputation": 157805541487641, - "rshares": 6317266240942, - "voter": "smartsteem" - }, - { - "percent": "1500", - "reputation": 9517778819103, - "rshares": 42976651587, - "voter": "mytechtrail" - }, - { - "percent": "240", - "reputation": 149410557707829, - "rshares": 1495699256, - "voter": "mhm-philippines" - }, - { - "percent": "2910", - "reputation": 76831784793845, - "rshares": 95314543718, - "voter": "ocupation" - }, - { - "percent": "10000", - "reputation": 66785151966461, - "rshares": 479850991631, - "voter": "itchyfeetdonica" - }, - { - "percent": "7000", - "reputation": 22087086438734, - "rshares": 40114010593, - "voter": "fjcalduch" - }, - { - "percent": "2910", - "reputation": 199114477141557, - "rshares": 3202482736, - "voter": "enjoyinglife" - }, - { - "percent": "2500", - "reputation": 2675225025765, - "rshares": 7337050727, - "voter": "fourfourfun" - }, - { - "percent": "10000", - "reputation": 9071994818465, - "rshares": 854126977, - "voter": "candyboy" - }, - { - "percent": "10000", - "reputation": 7107778763, - "rshares": 1901219647249, - "voter": "jim888" - }, - { - "percent": "10000", - "reputation": 36559175792044, - "rshares": 1661119984, - "voter": "jewel-lover" - }, - { - "percent": "3000", - "reputation": 46800704673839, - "rshares": 9288530596, - "voter": "adityajainxds" - }, - { - "percent": "160", - "reputation": 21720371357335, - "rshares": 5714940163, - "voter": "bartheek" - }, - { - "percent": "10000", - "reputation": 24009382437663, - "rshares": 169008219899, - "voter": "ddrfr33k" - }, - { - "percent": "3000", - "reputation": 188713944759376, - "rshares": 25813629871, - "voter": "roadstories" - }, - { - "percent": "960", - "reputation": 138441860072892, - "rshares": 55145900138, - "voter": "nealmcspadden" - }, - { - "percent": "3000", - "reputation": 0, - "rshares": 112704600920, - "voter": "curx" - }, - { - "percent": "10000", - "reputation": 3895318402889, - "rshares": 1500550639, - "voter": "criptonotas" - }, - { - "percent": "5000", - "reputation": 10165169816277, - "rshares": 3299332108, - "voter": "leslierevales" - }, - { - "percent": "960", - "reputation": 7978719984268, - "rshares": 11961898994, - "voter": "purefood" - }, - { - "percent": "10000", - "reputation": 467563251193, - "rshares": 635859047, - "voter": "durbisrodriguez" - }, - { - "percent": "10000", - "reputation": 170235537022, - "rshares": 1379902931, - "voter": "auracraft" - }, - { - "percent": "10000", - "reputation": 161107296598465, - "rshares": 783738831981, - "voter": "jarvie" - }, - { - "percent": "1700", - "reputation": 8003119161188, - "rshares": 3551523098, - "voter": "brewery" - }, - { - "percent": "10000", - "reputation": 951646894776, - "rshares": 555890492, - "voter": "cfminer" - }, - { - "percent": "1500", - "reputation": 12685053702515, - "rshares": 2081921303, - "voter": "kpopjera" - }, - { - "percent": "10000", - "reputation": 91651981795187, - "rshares": 23482440062, - "voter": "lunaticpandora" - }, - { - "percent": "7500", - "reputation": 330396781271113, - "rshares": 3339440021902, - "voter": "holger80" - }, - { - "percent": "10000", - "reputation": 9644507431392, - "rshares": 38296536041, - "voter": "mproxima" - }, - { - "percent": "500", - "reputation": 50124640567504, - "rshares": 1795647599, - "voter": "dailychina" - }, - { - "percent": "10000", - "reputation": 51254284112658, - "rshares": 137687161865, - "voter": "shmoogleosukami" - }, - { - "percent": "1500", - "reputation": 240105584587243, - "rshares": 696465159, - "voter": "mahtabansari370" - }, - { - "percent": "480", - "reputation": 88510175502, - "rshares": 851402909, - "voter": "unconditionalove" - }, - { - "percent": "10000", - "reputation": 4053153102731, - "rshares": 39782135049, - "voter": "natha93" - }, - { - "percent": "10000", - "reputation": 86916035515124, - "rshares": 121199349573, - "voter": "cryptosharon" - }, - { - "percent": "5000", - "reputation": 442944056818, - "rshares": 1861843104, - "voter": "minerspost" - }, - { - "percent": "5000", - "reputation": 411588240625, - "rshares": 3823291304, - "voter": "happy-soul" - }, - { - "percent": "768", - "reputation": 10898214322749, - "rshares": 3945270556, - "voter": "pkocjan" - }, - { - "percent": "3000", - "reputation": 1010495313616, - "rshares": 2755326200, - "voter": "ofildutemps" - }, - { - "percent": "10000", - "reputation": 12435393608248, - "rshares": 14817837232, - "voter": "jhoxiris" - }, - { - "percent": "5000", - "reputation": 15049076647839, - "rshares": 3915270701, - "voter": "kirito-freud" - }, - { - "percent": "10000", - "reputation": 182685861369, - "rshares": 2928254301, - "voter": "darkpylon" - }, - { - "percent": "10000", - "reputation": 41449838641209, - "rshares": 199568172442, - "voter": "pundito" - }, - { - "percent": "1455", - "reputation": 483223942335, - "rshares": 620940906, - "voter": "elektr1ker" - }, - { - "percent": "10000", - "reputation": 103087682988141, - "rshares": 165230955474, - "voter": "mysearchisover" - }, - { - "percent": "10000", - "reputation": 6264286399143, - "rshares": 2911092458827, - "voter": "asgarth" - }, - { - "percent": "10000", - "reputation": 2192876855791, - "rshares": 152384511568, - "voter": "hasmez" - }, - { - "percent": "10000", - "reputation": 12186132605, - "rshares": 1615218349, - "voter": "magicbirds" - }, - { - "percent": "10000", - "reputation": 8653571432, - "rshares": 1616849718, - "voter": "blueproject" - }, - { - "percent": "10000", - "reputation": 41394952718, - "rshares": 1615900298, - "voter": "django137" - }, - { - "percent": "10000", - "reputation": 5904893661018, - "rshares": 1494268471, - "voter": "fiveelements5" - }, - { - "percent": "10000", - "reputation": 8493141387, - "rshares": 1618612014, - "voter": "goldeneye64" - }, - { - "percent": "10000", - "reputation": 65151962039, - "rshares": 1613726450, - "voter": "ludezma" - }, - { - "percent": "10000", - "reputation": 11867738310700, - "rshares": 1490976242, - "voter": "oleg5430" - }, - { - "percent": "10000", - "reputation": 4418427243653, - "rshares": 5815023118, - "voter": "memesdaily" - }, - { - "percent": "960", - "reputation": 832069339446, - "rshares": 1815294658, - "voter": "ladysalsa" - }, - { - "percent": "10000", - "reputation": 76312761389929, - "rshares": 123469976450, - "voter": "victoriabsb" - }, - { - "percent": "2910", - "reputation": 274611114102331, - "rshares": 917082743530, - "voter": "mindtrap" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 28554868295, - "voter": "futurecurrency" - }, - { - "percent": "7000", - "reputation": 168836422039217, - "rshares": 39635199984, - "voter": "fego" - }, - { - "percent": "10000", - "reputation": 87712999336423, - "rshares": 16356111529, - "voter": "hadley4" - }, - { - "percent": "10000", - "reputation": 89325558244, - "rshares": 28467191343, - "voter": "steemporras" - }, - { - "percent": "1250", - "reputation": 2256065348315, - "rshares": 2055622188, - "voter": "frassman" - }, - { - "percent": "960", - "reputation": 61129901433914, - "rshares": 40702217874, - "voter": "reazuliqbal" - }, - { - "percent": "10000", - "reputation": 89461766913, - "rshares": 12713214206, - "voter": "artemisa7" - }, - { - "percent": "800", - "reputation": 62798275108724, - "rshares": 36501070586, - "voter": "j85063" - }, - { - "percent": "5000", - "reputation": 15600949256447, - "rshares": 1115582115, - "voter": "lordjames" - }, - { - "percent": "10000", - "reputation": 672719487100937, - "rshares": 2680332526, - "voter": "donekim" - }, - { - "percent": "10000", - "reputation": 46955154178798, - "rshares": 1271954304592, - "voter": "amico" - }, - { - "percent": "10000", - "reputation": 397421034392, - "rshares": 168565533420, - "voter": "steemitcolombia" - }, - { - "percent": "10000", - "reputation": 108726560523456, - "rshares": 374420494859, - "voter": "immanuel94" - }, - { - "percent": "4000", - "reputation": 93591631110831, - "rshares": 140212457479, - "voter": "bil.prag" - }, - { - "percent": "960", - "reputation": 1170108458021, - "rshares": 4480876963, - "voter": "bestboom" - }, - { - "percent": "3300", - "reputation": 70120060790855, - "rshares": 136817866587, - "voter": "dotwin1981" - }, - { - "percent": "10000", - "reputation": 1140372911417, - "rshares": 829941807, - "voter": "zainnyferdhoy" - }, - { - "percent": "3300", - "reputation": 25978211905358, - "rshares": 86877365422, - "voter": "manniman" - }, - { - "percent": "10000", - "reputation": 367201484924059, - "rshares": 1150424783457, - "voter": "louis88" - }, - { - "percent": "10000", - "reputation": 4038024331096, - "rshares": 481710348025, - "voter": "foxon" - }, - { - "percent": "10000", - "reputation": 7254888236822, - "rshares": 7279172172, - "voter": "herbacianymag" - }, - { - "percent": "10000", - "reputation": 33316181985289, - "rshares": 263138883340, - "voter": "barmbo" - }, - { - "percent": "10000", - "reputation": 5238378681910, - "rshares": 27815287315, - "voter": "m2nnari" - }, - { - "percent": "10000", - "reputation": 2184610478491, - "rshares": 10407982320, - "voter": "ex-exploitation" - }, - { - "percent": "1500", - "reputation": 7324548372470, - "rshares": 22465093768, - "voter": "freddio" - }, - { - "percent": "10000", - "reputation": 14193144291158, - "rshares": 582825807, - "voter": "abdulmath" - }, - { - "percent": "10000", - "reputation": 10532279362821, - "rshares": 3769158821, - "voter": "sallyfun" - }, - { - "percent": "10000", - "reputation": 40776009332161, - "rshares": 160513994812, - "voter": "gadrian" - }, - { - "percent": "5000", - "reputation": 112109667795, - "rshares": 1515865570, - "voter": "memepress" - }, - { - "percent": "1500", - "reputation": 1553052470569, - "rshares": 1563357921, - "voter": "sustainablelivin" - }, - { - "percent": "10000", - "reputation": 103039633795574, - "rshares": 459465975137, - "voter": "sjarvie5" - }, - { - "percent": "10000", - "reputation": 27390685365705, - "rshares": 20816574115, - "voter": "tsnaks" - }, - { - "percent": "3000", - "reputation": 51336896, - "rshares": 920913354, - "voter": "megalithic" - }, - { - "percent": "7000", - "reputation": 11266459355852, - "rshares": 4042774150, - "voter": "jadnven" - }, - { - "percent": "10000", - "reputation": 9020628089607, - "rshares": 91989386250, - "voter": "promobot" - }, - { - "percent": "10000", - "reputation": 3042286903871, - "rshares": 45341618873, - "voter": "jancharlest" - }, - { - "percent": "3000", - "reputation": 1963153455547, - "rshares": 680710907097, - "voter": "steem.services" - }, - { - "percent": "10000", - "reputation": 2483077279116, - "rshares": 987301900, - "voter": "ederaleng" - }, - { - "percent": "1500", - "reputation": 153947835280090, - "rshares": 130694171929, - "voter": "enforcer48" - }, - { - "percent": "10000", - "reputation": 30049907218185, - "rshares": 300497912746, - "voter": "kirstin" - }, - { - "percent": "10000", - "reputation": 4762022043623, - "rshares": 5784549658, - "voter": "fernandosoder" - }, - { - "percent": "10000", - "reputation": 16608311881044, - "rshares": 55084312413, - "voter": "curatorcat" - }, - { - "percent": "10000", - "reputation": 172465515392487, - "rshares": 1085855473097, - "voter": "steempeak" - }, - { - "percent": "7500", - "reputation": 9108091227516, - "rshares": 24147346486, - "voter": "bodie7" - }, - { - "percent": "960", - "reputation": 655223881789, - "rshares": 5639125967, - "voter": "swisswitness" - }, - { - "percent": "250", - "reputation": 20023805487967, - "rshares": 843534833, - "voter": "moneybaby" - }, - { - "percent": "2500", - "reputation": 27522752785541, - "rshares": 12827261197, - "voter": "longer" - }, - { - "percent": "1100", - "reputation": 68462748929740, - "rshares": 107597104658, - "voter": "roger5120" - }, - { - "percent": "10000", - "reputation": 9718632602, - "rshares": 1615915755, - "voter": "prudencia" - }, - { - "percent": "10000", - "reputation": 12580096938, - "rshares": 1613149382, - "voter": "gorka" - }, - { - "percent": "10000", - "reputation": 13059317148, - "rshares": 1614211690, - "voter": "suley" - }, - { - "percent": "10000", - "reputation": 6990715593, - "rshares": 1613089148, - "voter": "zulema" - }, - { - "percent": "10000", - "reputation": 10089127541, - "rshares": 1614966924, - "voter": "herencia" - }, - { - "percent": "10000", - "reputation": 9724555279, - "rshares": 1612586731, - "voter": "zoraida" - }, - { - "percent": "10000", - "reputation": 4252099247, - "rshares": 1613625553, - "voter": "embera" - }, - { - "percent": "10000", - "reputation": 6224691662, - "rshares": 1614278287, - "voter": "medula" - }, - { - "percent": "10000", - "reputation": 3158689060, - "rshares": 1618068470, - "voter": "chavela" - }, - { - "percent": "10000", - "reputation": 7291463397, - "rshares": 1613118731, - "voter": "tierra" - }, - { - "percent": "10000", - "reputation": 38511327979971, - "rshares": 2529786524, - "voter": "scrawly" - }, - { - "percent": "50", - "reputation": 292465506149952, - "rshares": 1001200772, - "voter": "shortsegments" - }, - { - "percent": "10000", - "reputation": 33860216986858, - "rshares": 616046552511, - "voter": "donald.porter" - }, - { - "percent": "960", - "reputation": 783817057887927, - "rshares": 567448938, - "voter": "gallerani" - }, - { - "percent": "10000", - "reputation": 34329245416723, - "rshares": 92229100050, - "voter": "bflanagin" - }, - { - "percent": "7000", - "reputation": 21771305129128, - "rshares": 2285918697, - "voter": "mightypanda" - }, - { - "percent": "1650", - "reputation": 1628398959081, - "rshares": 831787578, - "voter": "mastersa" - }, - { - "percent": "6500", - "reputation": 38547632600631, - "rshares": 3065020352, - "voter": "baiboua" - }, - { - "percent": "6500", - "reputation": 1825588222206, - "rshares": 14679014, - "voter": "akioexzgamer" - }, - { - "percent": "480", - "reputation": 156376467869107, - "rshares": 15432937270, - "voter": "dalz" - }, - { - "percent": "3000", - "reputation": 3794066899821, - "rshares": 861445267, - "voter": "sr-nikon" - }, - { - "percent": "1000", - "reputation": 21042238218731, - "rshares": 417194419989, - "voter": "zuerich" - }, - { - "percent": "6500", - "reputation": 8552552060679, - "rshares": 69373944108, - "voter": "french-tech" - }, - { - "percent": "6500", - "reputation": 6422573087015, - "rshares": 1463803915, - "voter": "yuza" - }, - { - "percent": "10000", - "reputation": 13163286762168, - "rshares": 16005382969, - "voter": "pavanjr" - }, - { - "percent": "480", - "reputation": 18790919715448, - "rshares": 69163970062, - "voter": "luppers" - }, - { - "percent": "960", - "reputation": 103580743464850, - "rshares": 112024619845, - "voter": "dlike" - }, - { - "percent": "6500", - "reputation": 7612430700896, - "rshares": 1952432334, - "voter": "paopaoza" - }, - { - "percent": "50", - "reputation": 144910360949054, - "rshares": 925705647, - "voter": "voxmortis" - }, - { - "percent": "7500", - "reputation": 36498710441788, - "rshares": 24460657694, - "voter": "fullnodeupdate" - }, - { - "percent": "6500", - "reputation": 7147565952243, - "rshares": 255588017, - "voter": "ten-years-before" - }, - { - "percent": "768", - "reputation": 58772053733860, - "rshares": 155730159109, - "voter": "engrave" - }, - { - "percent": "10000", - "reputation": 1503728134655, - "rshares": 505769106364, - "voter": "tipsybosphorus" - }, - { - "percent": "10000", - "reputation": 133998446537867, - "rshares": 5466154174, - "voter": "bluengel" - }, - { - "percent": "5000", - "reputation": 73595707264782, - "rshares": 557469138327, - "voter": "definethedollar" - }, - { - "percent": "10000", - "reputation": 144361338080419, - "rshares": 76815853272, - "voter": "porters" - }, - { - "percent": "240", - "reputation": 32853338946186, - "rshares": 892936461, - "voter": "bobby.madagascar" - }, - { - "percent": "10000", - "reputation": 10278138043955, - "rshares": 59792685, - "voter": "laissez-faire" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 15247458994, - "voter": "quediceharry" - }, - { - "percent": "10000", - "reputation": 5005137856099, - "rshares": 17222865721, - "voter": "palasatenea" - }, - { - "percent": "6500", - "reputation": 5464454876938, - "rshares": 1267445821, - "voter": "puza" - }, - { - "percent": "3000", - "reputation": 6687049412835, - "rshares": 23856140312, - "voter": "mister-meeseeks" - }, - { - "percent": "6500", - "reputation": 21145061340, - "rshares": 56325175, - "voter": "crypto.story" - }, - { - "percent": "3300", - "reputation": 1178472146943, - "rshares": 1718333390, - "voter": "gamer0815" - }, - { - "percent": "6500", - "reputation": 104143098516, - "rshares": 180929285, - "voter": "univers.crypto" - }, - { - "percent": "6500", - "reputation": 1276101904921, - "rshares": 5135032509, - "voter": "mintrawa" - }, - { - "percent": "960", - "reputation": 35303974424, - "rshares": 1243159699, - "voter": "ldp" - }, - { - "percent": "5000", - "reputation": 16966885974746, - "rshares": 1711910984, - "voter": "steemitcuration" - }, - { - "percent": "10000", - "reputation": 69053951644888, - "rshares": 19515101755, - "voter": "jacobpeacock" - }, - { - "percent": "5100", - "reputation": 93708060335638, - "rshares": 241702624441, - "voter": "actifit-peter" - }, - { - "percent": "10000", - "reputation": 1648086760085, - "rshares": 30343914698, - "voter": "diegor" - }, - { - "percent": "6000", - "reputation": 788852406777, - "rshares": 2029645048, - "voter": "thrasher666" - }, - { - "percent": "4000", - "reputation": 18273031359521, - "rshares": 1440166833, - "voter": "adyorka" - }, - { - "percent": "1500", - "reputation": 9135704094808, - "rshares": 832440508, - "voter": "eliasseth" - }, - { - "percent": "10000", - "reputation": 14391246828388, - "rshares": 16046555780, - "voter": "geeklania" - }, - { - "percent": "2619", - "reputation": 49288860027665, - "rshares": 211232327716, - "voter": "cwow2" - }, - { - "percent": "960", - "reputation": 2716815451543, - "rshares": 71086623122, - "voter": "followjohngalt" - }, - { - "percent": "2800", - "reputation": 539147053023232, - "rshares": 13422251731310, - "voter": "theycallmedan" - }, - { - "percent": "10000", - "reputation": 94595445913731, - "rshares": 1283259621332, - "voter": "glastar" - }, - { - "percent": "3000", - "reputation": 109213591273, - "rshares": 1334347657, - "voter": "vcs" - }, - { - "percent": "10000", - "reputation": 5493311683937, - "rshares": 106046612953, - "voter": "kekos" - }, - { - "percent": "10000", - "reputation": 8468960967138, - "rshares": 1911739402, - "voter": "smonia" - }, - { - "percent": "3000", - "reputation": 0, - "rshares": 1922081114, - "voter": "infinite-love" - }, - { - "percent": "10000", - "reputation": 5927670145042, - "rshares": 21851972782, - "voter": "northmountain" - }, - { - "percent": "10000", - "reputation": 671364595259, - "rshares": 1986384581, - "voter": "smon-fan" - }, - { - "percent": "160", - "reputation": 86443203025994, - "rshares": 685396263, - "voter": "jacuzzi" - }, - { - "percent": "3300", - "reputation": 13976967005540, - "rshares": 9237457196, - "voter": "kaldewei" - }, - { - "percent": "10000", - "reputation": 2488971014315, - "rshares": 1704423231, - "voter": "tr777" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1285912061, - "voter": "sm-jewel" - }, - { - "percent": "10000", - "reputation": 7476997614820, - "rshares": 136615598878, - "voter": "johan.norberg" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1447714289, - "voter": "smoner" - }, - { - "percent": "960", - "reputation": 2455748131750, - "rshares": 689383067, - "voter": "flyingbolt" - }, - { - "percent": "960", - "reputation": 711680161769462, - "rshares": 552898358, - "voter": "determine" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1687185058, - "voter": "smonian" - }, - { - "percent": "960", - "reputation": 1213063566738, - "rshares": 3737317492, - "voter": "permaculturedude" - }, - { - "percent": "10000", - "reputation": 3188730840665, - "rshares": 43396314000, - "voter": "loliver" - }, - { - "percent": "3000", - "reputation": 80366561377585, - "rshares": 325160739864, - "voter": "ajks" - }, - { - "percent": "10000", - "reputation": 774128044503, - "rshares": 1709410525, - "voter": "caribehub" - }, - { - "percent": "10000", - "reputation": 75911580, - "rshares": 189704618954, - "voter": "jamesbattler" - }, - { - "percent": "10000", - "reputation": 54892997399545, - "rshares": 1393012904, - "voter": "smon-joa" - }, - { - "percent": "10000", - "reputation": 1868507198312, - "rshares": 6859849710, - "voter": "greendeliver-sm" - }, - { - "percent": "10000", - "reputation": 9483360545909, - "rshares": 12889534290, - "voter": "mia-cc" - }, - { - "percent": "10000", - "reputation": 5888509729519, - "rshares": 38400575095, - "voter": "hans001" - }, - { - "percent": "10000", - "reputation": 91502264313, - "rshares": 136037009, - "voter": "maitre" - }, - { - "percent": "1000", - "reputation": 22403588555771, - "rshares": 21564008008, - "voter": "thelogicaldude" - }, - { - "percent": "10000", - "reputation": 5833218185, - "rshares": 1699432194, - "voter": "smonbear" - }, - { - "percent": "3000", - "reputation": 581240927, - "rshares": 0, - "voter": "smileyboy" - }, - { - "percent": "10000", - "reputation": 42787624679630, - "rshares": 32465561267, - "voter": "hungryharish" - }, - { - "percent": "1250", - "reputation": 27838835653920, - "rshares": 3522997208, - "voter": "kggymlife" - }, - { - "percent": "10000", - "reputation": 34359845798847, - "rshares": 77610615141, - "voter": "pedrobrito2004" - }, - { - "percent": "320", - "reputation": 69910542366759, - "rshares": 16339715157, - "voter": "kryptogames" - }, - { - "percent": "5000", - "reputation": 4189876953003, - "rshares": 2830217384, - "voter": "hungryanu" - }, - { - "percent": "10000", - "reputation": 72643755866464, - "rshares": 11646837675, - "voter": "jeremiahcustis" - }, - { - "percent": "912", - "reputation": 17287220932883, - "rshares": 10074872416, - "voter": "mfblack" - }, - { - "percent": "10000", - "reputation": 8977413850563, - "rshares": 23323579310, - "voter": "maryincryptoland" - }, - { - "percent": "3000", - "reputation": 29873843000, - "rshares": 1735658373, - "voter": "bergelmirsenpai" - }, - { - "percent": "3000", - "reputation": 120936131908085, - "rshares": 226999741750, - "voter": "epicdice" - }, - { - "percent": "10000", - "reputation": 98578472333076, - "rshares": 463648690334, - "voter": "minigame" - }, - { - "percent": "480", - "reputation": 33348221474331, - "rshares": 2650047049, - "voter": "threejay" - }, - { - "percent": "480", - "reputation": 1067767102949, - "rshares": 1080455399, - "voter": "sm-silva" - }, - { - "percent": "10000", - "reputation": 9877498112396, - "rshares": 1323778727, - "voter": "ssc-token" - }, - { - "percent": "6500", - "reputation": 7788522548, - "rshares": 230325491, - "voter": "steementertainer" - }, - { - "percent": "10000", - "reputation": 36087507440149, - "rshares": 823753443012, - "voter": "likwid" - }, - { - "percent": "10000", - "reputation": 185044670844, - "rshares": 0, - "voter": "tradingideas2" - }, - { - "percent": "500", - "reputation": 20916803594387, - "rshares": 2341503431, - "voter": "leighscotford" - }, - { - "percent": "10000", - "reputation": 11624344336093, - "rshares": 341782493500, - "voter": "recording-box" - }, - { - "percent": "480", - "reputation": 0, - "rshares": 543151253, - "voter": "firefuture" - }, - { - "percent": "10000", - "reputation": 458684528775, - "rshares": 581623404, - "voter": "zimnaherbata" - }, - { - "percent": "10000", - "reputation": 442801860940, - "rshares": 19835030085, - "voter": "steemindian" - }, - { - "percent": "6500", - "reputation": 413384764384, - "rshares": 295475267, - "voter": "nalexadre" - }, - { - "percent": "2700", - "reputation": 50073588499121, - "rshares": 45895424957, - "voter": "golden.future" - }, - { - "percent": "5000", - "reputation": 3804804773, - "rshares": 1577310931, - "voter": "imbartley" - }, - { - "percent": "8000", - "reputation": 9593095604859, - "rshares": 667511413, - "voter": "jimhawkins" - }, - { - "percent": "960", - "reputation": 52974480102727, - "rshares": 5447446247, - "voter": "shimozurdo" - }, - { - "percent": "960", - "reputation": 40619501571, - "rshares": 4248549110, - "voter": "milu-the-dog" - }, - { - "percent": "10000", - "reputation": 51374620316173, - "rshares": 37830789438, - "voter": "badfinger" - }, - { - "percent": "960", - "reputation": 9905442858, - "rshares": 3403971058, - "voter": "triplea.bot" - }, - { - "percent": "10000", - "reputation": 266864338439, - "rshares": 609364084, - "voter": "mytunes" - }, - { - "percent": "960", - "reputation": 115912639173774, - "rshares": 95141143816, - "voter": "steem.leo" - }, - { - "percent": "10000", - "reputation": 15808214765405, - "rshares": 686873232388, - "voter": "votebetting" - }, - { - "percent": "3000", - "reputation": 26642963135, - "rshares": 403137954, - "voter": "voltair" - }, - { - "percent": "3000", - "reputation": 556441464941, - "rshares": 59178992, - "voter": "sportsinfo" - }, - { - "percent": "10000", - "reputation": 6411393851, - "rshares": 927888118, - "voter": "driedfruit" - }, - { - "percent": "864", - "reputation": 14604990824032, - "rshares": 10709502089, - "voter": "asteroids" - }, - { - "percent": "10000", - "reputation": 376803157115, - "rshares": 362564155, - "voter": "tina-tina" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 13004690660, - "voter": "ticketyboo" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 13002733074, - "voter": "ticketywoof" - }, - { - "percent": "1500", - "reputation": 91995455090943, - "rshares": 5878549443, - "voter": "thranax" - }, - { - "percent": "100", - "reputation": 6481559677290, - "rshares": 152279382918, - "voter": "project.hope" - }, - { - "percent": "10000", - "reputation": 45364952422214, - "rshares": 60609615930, - "voter": "soyunasantacruz" - }, - { - "percent": "3000", - "reputation": 1410161022, - "rshares": 71225072531, - "voter": "botante" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "happiness19" - }, - { - "percent": "3000", - "reputation": 64791465095312, - "rshares": 62312257186, - "voter": "justclickindiva" - }, - { - "percent": "10000", - "reputation": 97507720606, - "rshares": 33564826, - "voter": "gdhaetae" - }, - { - "percent": "600", - "reputation": 426404968, - "rshares": 24226160235, - "voter": "maxuvd" - }, - { - "percent": "600", - "reputation": 6113738572, - "rshares": 30457859034, - "voter": "maxuve" - }, - { - "percent": "5000", - "reputation": 1704173681552, - "rshares": 1106898504, - "voter": "bilpcoinbot" - }, - { - "percent": "5000", - "reputation": 0, - "rshares": 526333601, - "voter": "roadstories.trib" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 13095121873, - "voter": "borbina" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1637395125, - "voter": "dnflsms" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 887414255, - "voter": "jessy22" - }, - { - "percent": "7500", - "reputation": 17687551130249, - "rshares": 9591208239, - "voter": "bcm" - }, - { - "percent": "5000", - "reputation": 3257555962879, - "rshares": 2694867858, - "voter": "bilpcoinrecords" - }, - { - "percent": "768", - "reputation": 0, - "rshares": 581924861, - "voter": "therealyme" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 10023177095, - "voter": "upyournose" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 10012468202, - "voter": "rubberhose" - }, - { - "percent": "10000", - "reputation": 2375835852, - "rshares": 3956018657, - "voter": "oopsie-poopsie" - }, - { - "percent": "5000", - "reputation": 1136926765, - "rshares": 661063746, - "voter": "chicoduro" - }, - { - "percent": "10000", - "reputation": 11637763209204, - "rshares": 210693750297, - "voter": "levi-miron" - }, - { - "percent": "2910", - "reputation": 51941880676536, - "rshares": 8636803771, - "voter": "sacrosanct" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 526812977, - "voter": "rus-lifestyle" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1308031233, - "voter": "keepit2" - }, - { - "percent": "480", - "reputation": 0, - "rshares": 3183445395, - "voter": "ribary" - }, - { - "percent": "10000", - "reputation": 786619143592, - "rshares": 0, - "voter": "cmdd" - }, - { - "percent": "2500", - "reputation": 356840875823, - "rshares": 853815532, - "voter": "kgsupport" - }, - { - "percent": "1500", - "reputation": 32542453022, - "rshares": 1405296292, - "voter": "bilpcoinbpc" - }, - { - "percent": "960", - "reputation": 549265305265, - "rshares": 48660403895, - "voter": "mice-k" - }, - { - "percent": "2000", - "reputation": 14577043701834, - "rshares": 3273674394, - "voter": "julesquirin" - }, - { - "percent": "10000", - "reputation": 362096342263, - "rshares": 481278937, - "voter": "sujaytechnicals" - }, - { - "percent": "192", - "reputation": 9568816243033, - "rshares": 1780065675, - "voter": "dpend.active" - }, - { - "percent": "2800", - "reputation": 7921327410319, - "rshares": 6247287771, - "voter": "euc" - }, - { - "percent": "900", - "reputation": 38576347919541, - "rshares": 896062208, - "voter": "dapplr" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 526245397843, - "voter": "fengchao" - }, - { - "percent": "10000", - "reputation": 37484144443, - "rshares": 375413615602, - "voter": "mountaingod" - }, - { - "percent": "10000", - "reputation": 2837344243508, - "rshares": 210569086625, - "voter": "hivetrending" - }, - { - "percent": "400", - "reputation": 91987050007999, - "rshares": 1247057073, - "voter": "hiveyoda" - }, - { - "percent": "480", - "reputation": 717095735374, - "rshares": 758483127, - "voter": "folklure" - }, - { - "percent": "10000", - "reputation": 11943864627652, - "rshares": 8230514212, - "voter": "hiveghost" - }, - { - "percent": "990", - "reputation": 10364363981695, - "rshares": 498934245665, - "voter": "visionaer3003" - }, - { - "percent": "5630", - "reputation": 1209731647511, - "rshares": 964662323246, - "voter": "softworld" - }, - { - "percent": "960", - "reputation": 5571802524553, - "rshares": 25626435710, - "voter": "polish.hive" - }, - { - "percent": "3000", - "reputation": 10188264707492, - "rshares": 1930556841, - "voter": "mynima" - }, - { - "percent": "960", - "reputation": 0, - "rshares": 279554043342, - "voter": "dcityrewards" - }, - { - "percent": "10000", - "reputation": 90463833228384, - "rshares": 44672237993, - "voter": "freakeao" - }, - { - "percent": "10000", - "reputation": 10637170241006, - "rshares": 10666074383, - "voter": "miriannalis" - }, - { - "percent": "96", - "reputation": 3177222978373, - "rshares": 836523052, - "voter": "hivelist" - }, - { - "percent": "3000", - "reputation": 604442758770, - "rshares": 134370736271, - "voter": "ninnu" - }, - { - "percent": "10000", - "reputation": 32381994985806, - "rshares": 38238846745, - "voter": "yolimarag" - }, - { - "percent": "10000", - "reputation": 14767283059507, - "rshares": 12222377588, - "voter": "cieliss" - }, - { - "percent": "300", - "reputation": 9650263289467, - "rshares": 1605925570, - "voter": "localgrower" - }, - { - "percent": "6000", - "reputation": 429763208250, - "rshares": 2711395604, - "voter": "ghaazi" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "zarevaneli" - }, - { - "percent": "3000", - "reputation": 0, - "rshares": 10435183855, - "voter": "comunidadactiva" - }, - { - "percent": "960", - "reputation": 0, - "rshares": 39967474731, - "voter": "hivecur" - }, - { - "percent": "10000", - "reputation": 37908486627936, - "rshares": 34950338396, - "voter": "ykroys" - }, - { - "percent": "10000", - "reputation": 15777185838, - "rshares": 2983988042, - "voter": "patronpass" - }, - { - "percent": "10000", - "reputation": 1289890269498, - "rshares": 798291889, - "voter": "greengalletti" - }, - { - "percent": "10000", - "reputation": 65519590598, - "rshares": 1951206002, - "voter": "plusvault" - }, - { - "percent": "970", - "reputation": 4358352640716, - "rshares": 232558746762, - "voter": "hatschi0773" - }, - { - "percent": "10000", - "reputation": 15596004215170, - "rshares": 1055701854, - "voter": "rojiso2411" - }, - { - "percent": "500", - "reputation": 0, - "rshares": 28710306350, - "voter": "hivecur2" - }, - { - "percent": "10000", - "reputation": 792202344312, - "rshares": 787492214, - "voter": "ollasysartenes" - }, - { - "percent": "10000", - "reputation": 2830639099232, - "rshares": 596797562, - "voter": "koxmicart" - }, - { - "percent": "3000", - "reputation": 28929095584285, - "rshares": 7018412778, - "voter": "notacinephile" - }, - { - "percent": "5000", - "reputation": 10164951682919, - "rshares": 3598361978, - "voter": "bdkabbo" - }, - { - "percent": "5000", - "reputation": 17832043721, - "rshares": 2733813335, - "voter": "laughingdesert" - }, - { - "percent": "10000", - "reputation": 7813514868027, - "rshares": 5705116431, - "voter": "francielis" - }, - { - "percent": "10000", - "reputation": 6535791110640, - "rshares": 3720326138, - "voter": "cryptoanalist" - }, - { - "percent": "10000", - "reputation": 4399967431494, - "rshares": 3177063994, - "voter": "epilatero" - }, - { - "percent": "10000", - "reputation": 3005998414144, - "rshares": 3307366067, - "voter": "kraken99" - }, - { - "percent": "10000", - "reputation": 10742256157644, - "rshares": 9800204122, - "voter": "motherhood" - }, - { - "percent": "480", - "reputation": 222094236041, - "rshares": 950069198, - "voter": "discohedge" - }, - { - "percent": "2000", - "reputation": 0, - "rshares": 3048839695336, - "voter": "usainvote" - }, - { - "percent": "980", - "reputation": 3174507364780, - "rshares": 355259306106, - "voter": "dagobert007" - }, - { - "percent": "10000", - "reputation": 3452328685273, - "rshares": 6186848694, - "voter": "aaalviarez" - }, - { - "percent": "5000", - "reputation": 1447273576100, - "rshares": 2682203736, - "voter": "bitcome" - }, - { - "percent": "5000", - "reputation": 978629567643, - "rshares": 904623603, - "voter": "arrrds" - }, - { - "percent": "2500", - "reputation": 3679705780578, - "rshares": 4108625642, - "voter": "senseiphil" - }, - { - "percent": "300", - "reputation": 0, - "rshares": 34524927334, - "voter": "intacto" - }, - { - "percent": "10000", - "reputation": 92721511576, - "rshares": 494820669, - "voter": "rhinoceros" - }, - { - "percent": "10000", - "reputation": 5167918, - "rshares": 0, - "voter": "vionline" - }, - { - "percent": "10000", - "reputation": 86405531104, - "rshares": 1914029290, - "voter": "text2speech" - }, - { - "percent": "10000", - "reputation": 1303993724855, - "rshares": 82625273821, - "voter": "damus-nostra" - }, - { - "percent": "10000", - "reputation": 270295677528, - "rshares": 249815748, - "voter": "hauptfleisch" - }, - { - "percent": "1000", - "reputation": 434627240, - "rshares": 15275505862, - "voter": "boneym" - }, - { - "percent": "10000", - "reputation": 1523084393, - "rshares": 8786125, - "voter": "osiri" - }, - { - "percent": "10000", - "reputation": 48820807481, - "rshares": 34358762489, - "voter": "officialhisha" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 3109652100, - "voter": "brofund-stem" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "sameer78" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 62696536993, - "voter": "betterdev" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "artx" - }, - { - "percent": "10000", - "reputation": 3594869451, - "rshares": 0, - "voter": "mythcrusher" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 65218185, - "voter": "cryptor3ader" - } - ], - "author": "peakd", - "author_reputation": 86868289888522, - "beneficiaries": [ - { - "account": "asgarth", - "weight": 3000 - }, - { - "account": "hive.fund", - "weight": 5500 - }, - { - "account": "jarvie", - "weight": 1500 - } - ], - "body": "Today we are happy to announce a new tool created to improve the experience of PeakD (and Hive) users.\n\n<center><h5>Check it out on https://beacon.peakd.com</h5></center>\n\n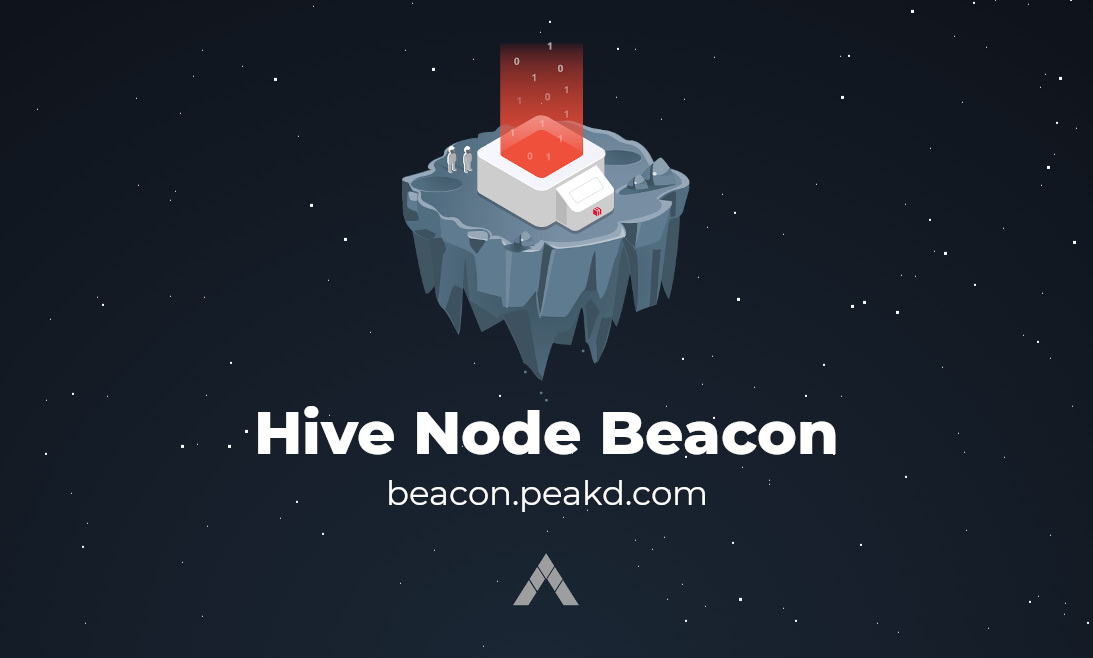\n\nThis new tool is already integrated into PeakD itself and allow users to automatically switch the API node to the best one available.\n\nAlso this is the first project released as [open source](https://github.com/peak-projects/peakd-node-beacon) so others can benefit from the work we do on PeakD.\nWe are planning to release more tools and libraries as open source in the near future, so hopefully this is only the first post of the list :)\n\n\n#### Current status for the API nodes\n\nYou can check this on the website itself, but sharing here for a quick look\n\n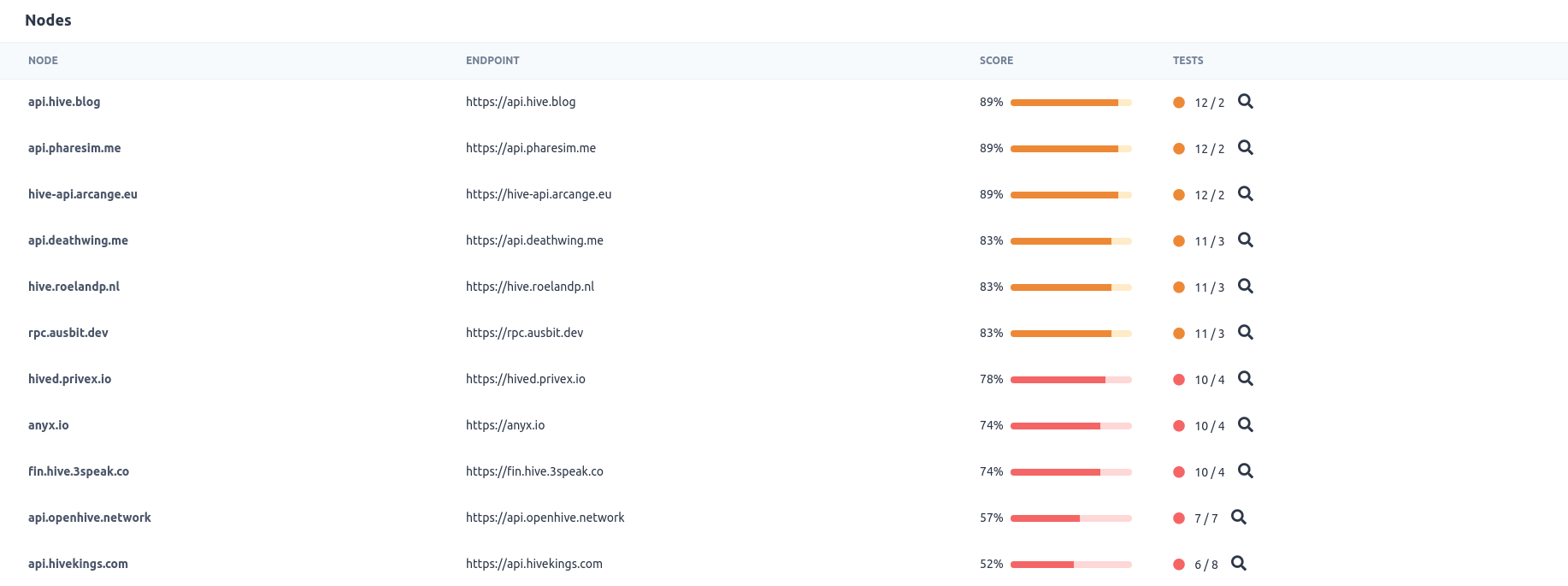\n\nAs you can see there are no nodes at the moment fully working. Please keep in mind that nodes are working perfectly fine on the chain level, just the social part powered by Hivemind still have some things to be fixed after the last HF. \n\n\n#### API checks\n\nTo understand how the above scores are computed you can check the list of the tests performed for each node (clicking on the magnifying glass).\n\n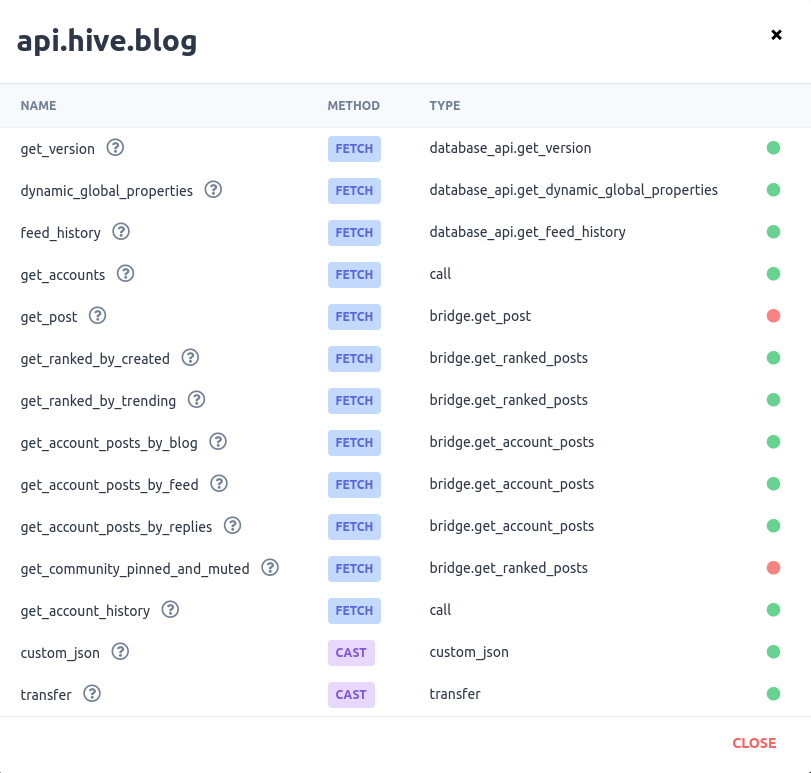\n\nTo get a better understanding of each check you can hover with the mouse on the (?) icon.\n\n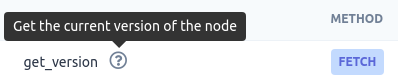\n\n\n#### Future plans and improvements\n\nThe tool is up and running and free to use right now, but we already have some ideas to make it better and to integrate more with [peakd.com](https://peakd.com) itself:\n- Integrate in the [Node Benchmark](https://peakd.com/me/tools/benchmark) page\n- Allow users to perform a speed test from Beacon itself\n- Integrate in the [Status](https://status.peakd.com) page\n- Allow users to select multiple nodes in their Settings with a specified order preference.\n\n---\n\n\n## Support the @peakd project\n\nNow is probably a nice time to remind you of the ways you can support the development of @peakd.\n\n\n\nWe have a proposal on the Decentralized Hive Fund. You can review the proposal [here](/proposals/52).\nIf you agree to the proposal and you think the points mentioned add value to the Hive Blockchain consider supporting it using the above links or directly with **[this link](https://hivesigner.com/sign/update-proposal-votes?proposal_ids=[52]&approve=true)**.\n\n--- \n\nWe also run a witness server to help produce the blocks for the Hive Blockchain. You can vote for our witness so we are in a position to process more of them.\n\nVote on the witness page: https://peakd.com/witnesses\n\n- Using Keychain: You just have to click and approve the transaction\n- Using HiveSigner: You'll need access to confirm the transaction with your Active Key at least\n\n---\n\n###### *The PeakD Team*\n_About us: https://peakd.com/about_\n_Join us on Discord: https://discord.gg/Fy3bca5_", - "body_length": 3147, - "cashout_time": "2020-11-06T21:48:03", - "category": "hive-139531", - "children": 25, - "created": "2020-10-30T21:48:03", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"app\":\"peakd/2020.10.9\",\"format\":\"markdown\",\"author\":\"asgarth\",\"description\":\"Releasing Hive Node Beacon, main features and future plans.\",\"tags\":[\"peakd\",\"hive\",\"hiveblockchain\",\"dapps\",\"hf24\",\"tools\",\"hive-dev\"],\"users\":[\"peakd\",\"peakd.\"],\"links\":[\"https://beacon.peakd.com\",\"https://github.com/peak-projects/peakd-node-beacon\",\"https://peakd.com\",\"/me/tools/benchmark\",\"https://status.peakd.com\",\"/@peakd\",\"/@peakd\",\"/proposals/52\",\"https://hivesigner.com/sign/update-proposal-votes?proposal_ids=[52]&approve=true\",\"https://peakd.com/witnesses\"],\"image\":[\"https://files.peakd.com/file/peakd-hive/peakd/9RKDLN5s-beacon_cover_v1.jpg\",\"https://files.peakd.com/file/peakd-hive/peakd/Xi1xPui8-image.png\",\"https://files.peakd.com/file/peakd-hive/peakd/1RoII1j0-image.png\",\"https://files.peakd.com/file/peakd-hive/peakd/R5NKk1hz-image.png\",\"https://files.peakd.com/file/peakd-hive/peakd/w36DQpO7-Selection_298.png\"]}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-10-30T22:21:09", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 201193936783958, - "parent_author": "", - "parent_permlink": "hive-139531", - "pending_payout_value": "47.246 HBD", - "percent_hbd": 10000, - "permlink": "announcing-the-hive-node-beacon-project", - "post_id": 100323666, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Announcing the Hive Node Beacon project", - "title": "Announcing the Hive Node Beacon project", - "total_payout_value": "0.000 HBD", - "url": "/hive-139531/@peakd/announcing-the-hive-node-beacon-project" - }, - { - "active_votes": [ - { - "percent": "1500", - "reputation": 520861791056580, - "rshares": 24676655342193, - "voter": "blocktrades" - }, - { - "percent": "10000", - "reputation": 1594304819447449, - "rshares": 10638817820734, - "voter": "acidyo" - }, - { - "percent": "1000", - "reputation": 109424005488868, - "rshares": 55324582434, - "voter": "mammasitta" - }, - { - "percent": "375", - "reputation": 352970428645376, - "rshares": 263212894435, - "voter": "roelandp" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 300631282732, - "voter": "livingfree" - }, - { - "percent": "10000", - "reputation": 372226967801214, - "rshares": 50733142677, - "voter": "allmonitors" - }, - { - "percent": "300", - "reputation": 315496990256842, - "rshares": 164357235559, - "voter": "arcange" - }, - { - "percent": "300", - "reputation": 501315151365, - "rshares": 3102062663, - "voter": "raphaelle" - }, - { - "percent": "5000", - "reputation": 9758823762159, - "rshares": 5845302744, - "voter": "joshglen" - }, - { - "percent": "2000", - "reputation": 12227576577296, - "rshares": 7275940266, - "voter": "anarcist69" - }, - { - "percent": "2500", - "reputation": 139814057263648, - "rshares": 30948681854, - "voter": "themanualbot" - }, - { - "percent": "10000", - "reputation": 262691650197556, - "rshares": 60446437130, - "voter": "titusfrost" - }, - { - "percent": "1500", - "reputation": 144108882515293, - "rshares": 121293223895, - "voter": "petrvl" - }, - { - "percent": "5000", - "reputation": 34211152246428, - "rshares": 119812786340, - "voter": "mcsvi" - }, - { - "percent": "1200", - "reputation": 25539300441985, - "rshares": 15759771187, - "voter": "natubat" - }, - { - "percent": "2000", - "reputation": 149827038166480, - "rshares": 83668341003, - "voter": "clayboyn" - }, - { - "percent": "10000", - "reputation": 414273820226452, - "rshares": 51796370975, - "voter": "ssekulji" - }, - { - "percent": "5000", - "reputation": 7729510836090, - "rshares": 276669148205, - "voter": "discovereurovelo" - }, - { - "percent": "2800", - "reputation": 0, - "rshares": 1707014410299, - "voter": "redes" - }, - { - "percent": "400", - "reputation": 0, - "rshares": 718437655288, - "voter": "created" - }, - { - "percent": "2000", - "reputation": 36357535837745, - "rshares": 1352375983, - "voter": "doodleman" - }, - { - "percent": "7200", - "reputation": 96863592771723, - "rshares": 82614677523, - "voter": "noemilunastorta" - }, - { - "percent": "10000", - "reputation": 1199149180987, - "rshares": 42633037270577, - "voter": "ranchorelaxo" - }, - { - "percent": "1000", - "reputation": 17465905454847, - "rshares": 1101035082, - "voter": "bloghound" - }, - { - "percent": "5000", - "reputation": 78330783694578, - "rshares": 97099790495, - "voter": "miniature-tiger" - }, - { - "percent": "5000", - "reputation": 27950490153931, - "rshares": 17164210594, - "voter": "choogirl" - }, - { - "percent": "5000", - "reputation": 71901302671995, - "rshares": 14746699722, - "voter": "hiroyamagishi" - }, - { - "percent": "10000", - "reputation": 66024390617355, - "rshares": 789244436839, - "voter": "elizahfhaye" - }, - { - "percent": "10000", - "reputation": 813135864401476, - "rshares": 12693876450070, - "voter": "broncnutz" - }, - { - "percent": "10000", - "reputation": 20837541242644, - "rshares": 1944370723, - "voter": "grider123" - }, - { - "percent": "5000", - "reputation": 1748651637089, - "rshares": 2030381395, - "voter": "khussan" - }, - { - "percent": "4000", - "reputation": 50342140379200, - "rshares": 1097641068612, - "voter": "followbtcnews" - }, - { - "percent": "500", - "reputation": 26139147505179, - "rshares": 923091089, - "voter": "rt395" - }, - { - "percent": "1500", - "reputation": 29513750536324, - "rshares": 884981833, - "voter": "kennyroy" - }, - { - "percent": "5000", - "reputation": 174399448898803, - "rshares": 98675157973, - "voter": "aleister" - }, - { - "percent": "10000", - "reputation": 14638546901678, - "rshares": 5269092563486, - "voter": "fatimajunio" - }, - { - "percent": "1000", - "reputation": 77602585289853, - "rshares": 7395278882, - "voter": "arrliinn" - }, - { - "percent": "1500", - "reputation": 3553102205525, - "rshares": 21013563438, - "voter": "belahejna" - }, - { - "percent": "10000", - "reputation": 7901101123497, - "rshares": 1046659200643, - "voter": "giuatt07" - }, - { - "percent": "5000", - "reputation": 783744181388, - "rshares": 1023122886, - "voter": "slefesteem" - }, - { - "percent": "3000", - "reputation": 69653104045391, - "rshares": 34173931906, - "voter": "anacristinasilva" - }, - { - "percent": "1600", - "reputation": 104292943123675, - "rshares": 46534573559, - "voter": "bearone" - }, - { - "percent": "5030", - "reputation": 23577497062186, - "rshares": 1133767589414, - "voter": "alphacore" - }, - { - "percent": "10000", - "reputation": 22475002262691, - "rshares": 38472286422, - "voter": "reddragonfly" - }, - { - "percent": "2000", - "reputation": 6025146240290, - "rshares": 1524251676, - "voter": "bellatravelph" - }, - { - "percent": "4000", - "reputation": 52265957847349, - "rshares": 378520469283, - "voter": "crimsonclad" - }, - { - "percent": "5000", - "reputation": 1494763095243, - "rshares": 1734565826, - "voter": "jamiz" - }, - { - "percent": "8000", - "reputation": 24347064093050, - "rshares": 30393888875, - "voter": "mynewlife" - }, - { - "percent": "10000", - "reputation": 219366237221002, - "rshares": 616217454668, - "voter": "offgridlife" - }, - { - "percent": "800", - "reputation": 0, - "rshares": 368703936737, - "voter": "gunthertopp" - }, - { - "percent": "10000", - "reputation": 383614057025318, - "rshares": 1605740188810, - "voter": "livinguktaiwan" - }, - { - "percent": "5000", - "reputation": 24021698289849, - "rshares": 5621494475, - "voter": "damm-steemit" - }, - { - "percent": "10000", - "reputation": 61905096596174, - "rshares": 101172584842, - "voter": "mers" - }, - { - "percent": "1000", - "reputation": 14764501234506, - "rshares": 2233920625, - "voter": "nolasco" - }, - { - "percent": "10000", - "reputation": 219861479290565, - "rshares": 1806614877, - "voter": "hangin" - }, - { - "percent": "10000", - "reputation": 20285621918859, - "rshares": 39187040005, - "voter": "wandergirl" - }, - { - "percent": "10000", - "reputation": 5313719494876540, - "rshares": 12229551564685, - "voter": "haejin" - }, - { - "percent": "10000", - "reputation": 23747652895956, - "rshares": 989959127, - "voter": "richi0927" - }, - { - "percent": "5000", - "reputation": 29124480726940, - "rshares": 1879266974, - "voter": "greatness96" - }, - { - "percent": "8900", - "reputation": 66529579059852, - "rshares": 85853117650, - "voter": "leaky20" - }, - { - "percent": "4000", - "reputation": 1790763390354, - "rshares": 1115371474, - "voter": "koh" - }, - { - "percent": "10000", - "reputation": 1465267842688, - "rshares": 1538446792, - "voter": "jorge248" - }, - { - "percent": "10000", - "reputation": 1095186992075950, - "rshares": 156189330599, - "voter": "cryptopassion" - }, - { - "percent": "5000", - "reputation": 1912914147521, - "rshares": 1876113143, - "voter": "onethousandwords" - }, - { - "percent": "2000", - "reputation": 25406027451406, - "rshares": 6824026874, - "voter": "martibis" - }, - { - "percent": "10000", - "reputation": 1861063781145, - "rshares": 577963478, - "voter": "leryam12" - }, - { - "percent": "5000", - "reputation": 4022219848165, - "rshares": 2994619962, - "voter": "jenesa" - }, - { - "percent": "1000", - "reputation": 3574566966768, - "rshares": 3180809894, - "voter": "appleskie" - }, - { - "percent": "10000", - "reputation": 713624891901, - "rshares": 75028966735, - "voter": "plainoldme" - }, - { - "percent": "5000", - "reputation": 1087907674628, - "rshares": 1071004312, - "voter": "pjmisa" - }, - { - "percent": "3300", - "reputation": 24639928209538, - "rshares": 19196038815, - "voter": "santigs" - }, - { - "percent": "1000", - "reputation": 149085530853696, - "rshares": 218596887922, - "voter": "karja" - }, - { - "percent": "10000", - "reputation": 196571377648, - "rshares": 18125648666, - "voter": "nadhora" - }, - { - "percent": "5000", - "reputation": 2656035667454, - "rshares": 1737629909, - "voter": "agentzero" - }, - { - "percent": "5000", - "reputation": 140483466255317, - "rshares": 266629402187, - "voter": "fbslo" - }, - { - "percent": "1000", - "reputation": 41069012860239, - "rshares": 1746985393, - "voter": "markjason" - }, - { - "percent": "10000", - "reputation": 2206804389678, - "rshares": 4915742239, - "voter": "marysent" - }, - { - "percent": "10000", - "reputation": 669346799793, - "rshares": 21901960392, - "voter": "voxmonkey" - }, - { - "percent": "5000", - "reputation": 139904553806579, - "rshares": 2847862438272, - "voter": "joshman" - }, - { - "percent": "600", - "reputation": 2448367928201, - "rshares": 1336971714, - "voter": "pingcess" - }, - { - "percent": "10000", - "reputation": 804792498605, - "rshares": 1563183969, - "voter": "hillaryaa" - }, - { - "percent": "5000", - "reputation": 959835803794, - "rshares": 594507631, - "voter": "mercy11" - }, - { - "percent": "10000", - "reputation": 366168985985447, - "rshares": 127028645607, - "voter": "steemitworldmap" - }, - { - "percent": "1000", - "reputation": 16626980732032, - "rshares": 935947930, - "voter": "diosarich" - }, - { - "percent": "10000", - "reputation": 769442706037, - "rshares": 1072678636, - "voter": "tedzwhistle" - }, - { - "percent": "10000", - "reputation": 13971360461719, - "rshares": 17664852694, - "voter": "bigdizzle91" - }, - { - "percent": "2000", - "reputation": 6445110251502, - "rshares": 2613507049, - "voter": "divinekids" - }, - { - "percent": "1000", - "reputation": 78606817928738, - "rshares": 15399863665, - "voter": "happydolphin" - }, - { - "percent": "5000", - "reputation": 935189040639, - "rshares": 565308414, - "voter": "maaz23" - }, - { - "percent": "2000", - "reputation": 10939046475227, - "rshares": 759389370, - "voter": "liverpool-fan" - }, - { - "percent": "1000", - "reputation": 12918217107297, - "rshares": 1108818807, - "voter": "afterglow" - }, - { - "percent": "5000", - "reputation": 2501064007913, - "rshares": 2274284138, - "voter": "reyarobo" - }, - { - "percent": "10000", - "reputation": 28740832773198, - "rshares": 37955355822, - "voter": "steemph.cebu" - }, - { - "percent": "2500", - "reputation": 663634027075, - "rshares": 660555436, - "voter": "nexusvortex777" - }, - { - "percent": "200", - "reputation": 50675272500651, - "rshares": 754371029, - "voter": "dejan.vuckovic" - }, - { - "percent": "10000", - "reputation": 1737660226555, - "rshares": 1517953979, - "voter": "meincluyo" - }, - { - "percent": "10000", - "reputation": 2561399399469, - "rshares": 4473493475, - "voter": "legendarryll" - }, - { - "percent": "5000", - "reputation": 15453581060510, - "rshares": 13618876893, - "voter": "smaeunabs" - }, - { - "percent": "2000", - "reputation": 2341876684406, - "rshares": 1250227008, - "voter": "steemph.manila" - }, - { - "percent": "10000", - "reputation": 2913812595405, - "rshares": 3061611017, - "voter": "sorenkierkegaard" - }, - { - "percent": "5000", - "reputation": 4844110760338, - "rshares": 14505060484, - "voter": "steef-05" - }, - { - "percent": "5000", - "reputation": 1571846435496, - "rshares": 3942303936, - "voter": "dizzyapple" - }, - { - "percent": "1000", - "reputation": 28722297623429, - "rshares": 8263352402, - "voter": "maverickinvictus" - }, - { - "percent": "10000", - "reputation": 77492130259, - "rshares": 1869344152, - "voter": "awesome-gadgets" - }, - { - "percent": "10000", - "reputation": 103556422730563, - "rshares": 68644459451, - "voter": "katerinaramm" - }, - { - "percent": "800", - "reputation": 10160344260214, - "rshares": 1575860731, - "voter": "xsasj" - }, - { - "percent": "10000", - "reputation": 10092142213140, - "rshares": 11569619828, - "voter": "thegaillery" - }, - { - "percent": "500", - "reputation": 30362686718221, - "rshares": 2991377448, - "voter": "junebride" - }, - { - "percent": "5000", - "reputation": 2678661471750, - "rshares": 1068914962, - "voter": "janicemars" - }, - { - "percent": "10000", - "reputation": 26561211539981, - "rshares": 164722009932, - "voter": "meetmysuperego" - }, - { - "percent": "10000", - "reputation": 6823769560292, - "rshares": 6112430749, - "voter": "thesobuz" - }, - { - "percent": "1000", - "reputation": 13569938137899, - "rshares": 1609513010, - "voter": "kneelyrac" - }, - { - "percent": "5000", - "reputation": 2045506354809, - "rshares": 857505269, - "voter": "steeminer4up" - }, - { - "percent": "10000", - "reputation": 61297887349807, - "rshares": 21499881341, - "voter": "nathen007" - }, - { - "percent": "400", - "reputation": 1521170786625, - "rshares": 783052045, - "voter": "romanaround" - }, - { - "percent": "4500", - "reputation": 1494643252435, - "rshares": 15686616867, - "voter": "lpv" - }, - { - "percent": "10000", - "reputation": 570069464324, - "rshares": 1741597446, - "voter": "namranna" - }, - { - "percent": "1800", - "reputation": 7653694649262, - "rshares": 546539727, - "voter": "godlovermel25" - }, - { - "percent": "1000", - "reputation": 75425198894223, - "rshares": 6442867544, - "voter": "straykat" - }, - { - "percent": "750", - "reputation": 14337364044156, - "rshares": 1103700842, - "voter": "tomatom" - }, - { - "percent": "2500", - "reputation": 2675225025765, - "rshares": 7553071302, - "voter": "fourfourfun" - }, - { - "percent": "5000", - "reputation": 1222519641209, - "rshares": 1104173036, - "voter": "pizzanniza" - }, - { - "percent": "1000", - "reputation": 13345171215929, - "rshares": 5232493175, - "voter": "r00sj3" - }, - { - "percent": "10000", - "reputation": 417897915586235, - "rshares": 847330718162, - "voter": "phortun" - }, - { - "percent": "10000", - "reputation": 13267517492775, - "rshares": 9658058007, - "voter": "juecoree" - }, - { - "percent": "10000", - "reputation": 11279365843876, - "rshares": 9567105127, - "voter": "nikkabomb" - }, - { - "percent": "2000", - "reputation": 87246032649758, - "rshares": 39463577220, - "voter": "gabrielatravels" - }, - { - "percent": "5000", - "reputation": 1483157104148, - "rshares": 868185315, - "voter": "cyyy1998" - }, - { - "percent": "10000", - "reputation": 478617433585, - "rshares": 1672395808, - "voter": "glennamayjumaoas" - }, - { - "percent": "10000", - "reputation": 801609916213, - "rshares": 887211667, - "voter": "jonnahmatias1016" - }, - { - "percent": "10000", - "reputation": 718140601874, - "rshares": 1075216143, - "voter": "francesgardose" - }, - { - "percent": "5000", - "reputation": 20747172002123, - "rshares": 1287594390, - "voter": "shoganaii" - }, - { - "percent": "10000", - "reputation": 41547223265560, - "rshares": 96404811024, - "voter": "girolamomarotta" - }, - { - "percent": "5000", - "reputation": -83817811053, - "rshares": 5579942726, - "voter": "payger" - }, - { - "percent": "10000", - "reputation": 4255833468352, - "rshares": 848480124, - "voter": "thinkingmind" - }, - { - "percent": "10000", - "reputation": 11200470488081, - "rshares": 20128458910, - "voter": "josejirafa" - }, - { - "percent": "300", - "reputation": 127375153532443, - "rshares": 1333946296, - "voter": "fun2learn" - }, - { - "percent": "1000", - "reputation": 4882081332011, - "rshares": 1917523950, - "voter": "christianyocte" - }, - { - "percent": "1000", - "reputation": 12722627918140, - "rshares": 39233592554, - "voter": "heidimarie" - }, - { - "percent": "4000", - "reputation": 49210493751560, - "rshares": 16292346043, - "voter": "sinochip" - }, - { - "percent": "10000", - "reputation": 3895318402889, - "rshares": 1527800929, - "voter": "criptonotas" - }, - { - "percent": "5000", - "reputation": 219242636184, - "rshares": 605938627, - "voter": "brusd" - }, - { - "percent": "5000", - "reputation": 822709975413, - "rshares": 710755223, - "voter": "honeyletsgo" - }, - { - "percent": "5000", - "reputation": 1627145024010, - "rshares": 788937147, - "voter": "georgie84" - }, - { - "percent": "10000", - "reputation": 1507449214051, - "rshares": 761959758, - "voter": "abandi" - }, - { - "percent": "5000", - "reputation": 1298566083487, - "rshares": 1316743528, - "voter": "davids-tales" - }, - { - "percent": "10000", - "reputation": 63812007256914, - "rshares": 91868666795, - "voter": "k-banti" - }, - { - "percent": "5000", - "reputation": 2374130318210, - "rshares": 1231430097, - "voter": "cheesom" - }, - { - "percent": "10000", - "reputation": 21875725656972, - "rshares": 10716912409, - "voter": "ligarayk" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 10621588077, - "voter": "best-strategy" - }, - { - "percent": "5000", - "reputation": 48456913838551, - "rshares": 9592477618, - "voter": "haydae" - }, - { - "percent": "5000", - "reputation": 2826422159971, - "rshares": 2777451314, - "voter": "piotr42" - }, - { - "percent": "2500", - "reputation": 442944056818, - "rshares": 936805027, - "voter": "minerspost" - }, - { - "percent": "10000", - "reputation": 354023179708, - "rshares": 728776200, - "voter": "raquelita" - }, - { - "percent": "5000", - "reputation": 874108865684, - "rshares": 595991945, - "voter": "geeyang15" - }, - { - "percent": "5000", - "reputation": 12334368355423, - "rshares": 1517116500, - "voter": "loydjayme25" - }, - { - "percent": "2500", - "reputation": 1119318751394, - "rshares": 629139939, - "voter": "noechie1827" - }, - { - "percent": "5000", - "reputation": 71504792482, - "rshares": 554018260, - "voter": "photohunter5" - }, - { - "percent": "5000", - "reputation": 71185892857862, - "rshares": 47852833932, - "voter": "chungsu1" - }, - { - "percent": "10000", - "reputation": 86376131766962, - "rshares": 166404334421, - "voter": "gohenry" - }, - { - "percent": "5000", - "reputation": 2866827942623, - "rshares": 3139856088, - "voter": "starzy" - }, - { - "percent": "5000", - "reputation": 17846302258319, - "rshares": 21830203535, - "voter": "onlavu" - }, - { - "percent": "10000", - "reputation": 12186132605, - "rshares": 1659237359, - "voter": "magicbirds" - }, - { - "percent": "10000", - "reputation": 8653571432, - "rshares": 1660963136, - "voter": "blueproject" - }, - { - "percent": "10000", - "reputation": 41394952718, - "rshares": 1661886167, - "voter": "django137" - }, - { - "percent": "10000", - "reputation": 5904893661018, - "rshares": 1521864476, - "voter": "fiveelements5" - }, - { - "percent": "10000", - "reputation": 8493141387, - "rshares": 1661126340, - "voter": "goldeneye64" - }, - { - "percent": "10000", - "reputation": 65151962039, - "rshares": 1657817349, - "voter": "ludezma" - }, - { - "percent": "10000", - "reputation": 11867738310700, - "rshares": 1518562066, - "voter": "oleg5430" - }, - { - "percent": "200", - "reputation": 26898775193091, - "rshares": 1903510927, - "voter": "movement19" - }, - { - "percent": "4500", - "reputation": 48038501416805, - "rshares": 28068943177, - "voter": "futurecurrency" - }, - { - "percent": "10000", - "reputation": 5195582997313, - "rshares": 1050940354, - "voter": "leebaong" - }, - { - "percent": "10000", - "reputation": 121063235291, - "rshares": 687520372, - "voter": "thisisruby" - }, - { - "percent": "10000", - "reputation": 87629137367, - "rshares": 1940114774, - "voter": "rasty.demecillo" - }, - { - "percent": "5000", - "reputation": 6347278940631, - "rshares": 9020842057, - "voter": "steempampanga" - }, - { - "percent": "10000", - "reputation": 1835670573034, - "rshares": 887657011, - "voter": "missdonna" - }, - { - "percent": "5000", - "reputation": 2654510329914, - "rshares": 1817847687, - "voter": "sawi" - }, - { - "percent": "750", - "reputation": 2256065348315, - "rshares": 1213605281, - "voter": "frassman" - }, - { - "percent": "10000", - "reputation": 3441314325667, - "rshares": 88192110508, - "voter": "norwegianbikeman" - }, - { - "percent": "5000", - "reputation": 4188238259870, - "rshares": 3192888981, - "voter": "reewritesthings" - }, - { - "percent": "10000", - "reputation": 397421034392, - "rshares": 173282841453, - "voter": "steemitcolombia" - }, - { - "percent": "5000", - "reputation": 742795053593, - "rshares": 1091159684, - "voter": "themarshann" - }, - { - "percent": "4000", - "reputation": 58256862375288, - "rshares": 23465430699, - "voter": "vcclothing" - }, - { - "percent": "5000", - "reputation": 1553888219838, - "rshares": 929382096, - "voter": "jackobeat" - }, - { - "percent": "10000", - "reputation": 1238565986273, - "rshares": 1788790821, - "voter": "carlitojoshua" - }, - { - "percent": "800", - "reputation": 174856977309980, - "rshares": 27573326785, - "voter": "ivansnz" - }, - { - "percent": "10000", - "reputation": 373679836722908, - "rshares": 3311604837202, - "voter": "derangedvisions" - }, - { - "percent": "10000", - "reputation": 14193144291158, - "rshares": 4777652422, - "voter": "abdulmath" - }, - { - "percent": "10000", - "reputation": 353099598336644, - "rshares": 521905119, - "voter": "hiddenblade" - }, - { - "percent": "5000", - "reputation": 564946014672, - "rshares": 647835302, - "voter": "aljunecastro" - }, - { - "percent": "10000", - "reputation": 2481629111221, - "rshares": 2038010863, - "voter": "manojbhatt" - }, - { - "percent": "1000", - "reputation": 454088646937365, - "rshares": 31496438875, - "voter": "kgakakillerg" - }, - { - "percent": "1500", - "reputation": 65521507383931, - "rshares": 7330121347, - "voter": "oscurity" - }, - { - "percent": "9900", - "reputation": 51336896, - "rshares": 3634788240, - "voter": "megalithic" - }, - { - "percent": "2000", - "reputation": 1471256869354, - "rshares": 2553501243, - "voter": "steemph.uae" - }, - { - "percent": "800", - "reputation": 287995498402522, - "rshares": 262365451151, - "voter": "solominer" - }, - { - "percent": "8000", - "reputation": 804111529580, - "rshares": 1024437266, - "voter": "kahawenz" - }, - { - "percent": "1000", - "reputation": 27284305279794, - "rshares": 1211018244, - "voter": "rachelleignacio" - }, - { - "percent": "10000", - "reputation": 1730152493668, - "rshares": 3187702685, - "voter": "steemsummit" - }, - { - "percent": "5000", - "reputation": 24852530816752, - "rshares": 11505001544, - "voter": "jason04" - }, - { - "percent": "5000", - "reputation": 401559670619, - "rshares": 1546772863, - "voter": "onethousandpics" - }, - { - "percent": "10000", - "reputation": 254937223188, - "rshares": 870685867, - "voter": "svm038" - }, - { - "percent": "5000", - "reputation": 772102466926, - "rshares": 609428904, - "voter": "kyanzieuno" - }, - { - "percent": "10000", - "reputation": 5018145865179, - "rshares": 5984197778, - "voter": "bimaladevi" - }, - { - "percent": "10000", - "reputation": 95233949488692, - "rshares": 181148289985, - "voter": "sgbonus" - }, - { - "percent": "5000", - "reputation": 244842259743, - "rshares": 1177929465, - "voter": "ihal0001" - }, - { - "percent": "10000", - "reputation": 3274691895642, - "rshares": 1719603582, - "voter": "soma909" - }, - { - "percent": "5000", - "reputation": 778260061223, - "rshares": 600334965, - "voter": "mojacko" - }, - { - "percent": "10000", - "reputation": 9718632602, - "rshares": 1660087526, - "voter": "prudencia" - }, - { - "percent": "10000", - "reputation": 12580096938, - "rshares": 1657224656, - "voter": "gorka" - }, - { - "percent": "10000", - "reputation": 13059317148, - "rshares": 1658324891, - "voter": "suley" - }, - { - "percent": "5000", - "reputation": 1137655654537, - "rshares": 1547372304, - "voter": "czera" - }, - { - "percent": "10000", - "reputation": 6990715593, - "rshares": 1657200124, - "voter": "zulema" - }, - { - "percent": "10000", - "reputation": 10089127541, - "rshares": 1659116520, - "voter": "herencia" - }, - { - "percent": "10000", - "reputation": 9724555279, - "rshares": 1656658067, - "voter": "zoraida" - }, - { - "percent": "10000", - "reputation": 4252099247, - "rshares": 1657740356, - "voter": "embera" - }, - { - "percent": "10000", - "reputation": 6224691662, - "rshares": 1658408163, - "voter": "medula" - }, - { - "percent": "10000", - "reputation": 3158689060, - "rshares": 1739989927, - "voter": "chavela" - }, - { - "percent": "10000", - "reputation": 7291463397, - "rshares": 1657204118, - "voter": "tierra" - }, - { - "percent": "1000", - "reputation": 97973570792503, - "rshares": 4715836233, - "voter": "mrnightmare89" - }, - { - "percent": "5000", - "reputation": 776039665811, - "rshares": 583775694, - "voter": "kayegrasya" - }, - { - "percent": "2500", - "reputation": 118140326729872, - "rshares": 9444814566, - "voter": "sadbear" - }, - { - "percent": "10000", - "reputation": 114256216898620, - "rshares": 48858484684, - "voter": "digi-me" - }, - { - "percent": "5000", - "reputation": 62302307783, - "rshares": 14244459557, - "voter": "pvinny69" - }, - { - "percent": "10000", - "reputation": 58717222761366, - "rshares": 11078352241, - "voter": "gohalber" - }, - { - "percent": "2500", - "reputation": 1519166384164, - "rshares": 5523905453, - "voter": "randomkindness" - }, - { - "percent": "5000", - "reputation": 32787366325026, - "rshares": 20673582376, - "voter": "rayshiuimages" - }, - { - "percent": "10000", - "reputation": 29569797322510, - "rshares": 15987067237, - "voter": "quediceharry" - }, - { - "percent": "5000", - "reputation": 2029932349983, - "rshares": 1514171155, - "voter": "angoujkalis" - }, - { - "percent": "5000", - "reputation": 6687049412835, - "rshares": 40449441937, - "voter": "mister-meeseeks" - }, - { - "percent": "10000", - "reputation": 158552584457210, - "rshares": 174339805116, - "voter": "macoolette" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 68537840861, - "voter": "artistsrising" - }, - { - "percent": "10000", - "reputation": 4880788820007, - "rshares": 700459340, - "voter": "malricinferno" - }, - { - "percent": "10000", - "reputation": 12156366634704, - "rshares": 810948973, - "voter": "zintarmortalis" - }, - { - "percent": "10000", - "reputation": 2161236841409, - "rshares": 857683222, - "voter": "natur-pur" - }, - { - "percent": "5000", - "reputation": 7385938119874, - "rshares": 178102825326, - "voter": "thevil" - }, - { - "percent": "10000", - "reputation": 16966885974746, - "rshares": 4006286563, - "voter": "steemitcuration" - }, - { - "percent": "4000", - "reputation": -68594610567, - "rshares": 65584488781, - "voter": "blarchive" - }, - { - "percent": "10000", - "reputation": 1648086760085, - "rshares": 24413076006, - "voter": "diegor" - }, - { - "percent": "4000", - "reputation": 916241881, - "rshares": 2259158626, - "voter": "anarcist" - }, - { - "percent": "10000", - "reputation": 4096336787572, - "rshares": 8471102621, - "voter": "rakk3187" - }, - { - "percent": "100", - "reputation": 4915527864940, - "rshares": 65128338, - "voter": "dashand" - }, - { - "percent": "2500", - "reputation": 765680106224, - "rshares": 576452928, - "voter": "hubyr" - }, - { - "percent": "10000", - "reputation": 5493311683937, - "rshares": 104242881644, - "voter": "kekos" - }, - { - "percent": "10000", - "reputation": 2485823261653, - "rshares": 5390533648, - "voter": "unit101" - }, - { - "percent": "10000", - "reputation": 4235286777563, - "rshares": 8580308667, - "voter": "littlegurl747" - }, - { - "percent": "10000", - "reputation": 11499521185204, - "rshares": 18851513501, - "voter": "yuriy4" - }, - { - "percent": "5000", - "reputation": 88423473475, - "rshares": 1730172872, - "voter": "thehealthylife" - }, - { - "percent": "5000", - "reputation": 124735349102, - "rshares": 6959649569, - "voter": "enthef" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 603220734684, - "voter": "bluesniper" - }, - { - "percent": "2000", - "reputation": 0, - "rshares": 9151701960, - "voter": "coriolis" - }, - { - "percent": "500", - "reputation": -4467669911343, - "rshares": 775487745865, - "voter": "ctime" - }, - { - "percent": "10000", - "reputation": 97219598927367, - "rshares": 53514272355, - "voter": "josehany" - }, - { - "percent": "10000", - "reputation": 774128044503, - "rshares": 1754866654, - "voter": "caribehub" - }, - { - "percent": "3500", - "reputation": 410620631652, - "rshares": 4365747716, - "voter": "ava77" - }, - { - "percent": "5000", - "reputation": 611845544559, - "rshares": 1910289791, - "voter": "parth7878" - }, - { - "percent": "10000", - "reputation": 25016264134313, - "rshares": 699501379473, - "voter": "dcommerce" - }, - { - "percent": "2500", - "reputation": 4594852669149, - "rshares": 1032791841, - "voter": "rappler" - }, - { - "percent": "10000", - "reputation": 404952233227379, - "rshares": 155863625146, - "voter": "apix" - }, - { - "percent": "1900", - "reputation": 13328375619094, - "rshares": 223334334650, - "voter": "russia-btc" - }, - { - "percent": "10000", - "reputation": 51900218528169, - "rshares": 2051240452, - "voter": "alexvanaken" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 4563250155, - "voter": "steem-fund" - }, - { - "percent": "7500", - "reputation": 3909341978875, - "rshares": 1366422165, - "voter": "bitcoingodmode" - }, - { - "percent": "5000", - "reputation": 58574039848, - "rshares": 1310081699, - "voter": "zackarie" - }, - { - "percent": "5000", - "reputation": 4219925012330, - "rshares": 18104505791, - "voter": "scarletreaper" - }, - { - "percent": "10000", - "reputation": 3995516605448, - "rshares": 692053518, - "voter": "izhmash" - }, - { - "percent": "10000", - "reputation": 3669327624935, - "rshares": 599220582, - "voter": "sevensixtwo" - }, - { - "percent": "10000", - "reputation": 3352742040192, - "rshares": 623822527, - "voter": "bastogne" - }, - { - "percent": "10000", - "reputation": 3322393839118, - "rshares": 627713787, - "voter": "thirdarmy" - }, - { - "percent": "10000", - "reputation": 29888557634290, - "rshares": 19071426438, - "voter": "helgalubevi" - }, - { - "percent": "5000", - "reputation": 2597264781531, - "rshares": 8305305272, - "voter": "akumagai" - }, - { - "percent": "5000", - "reputation": 1099279919915, - "rshares": 649096640, - "voter": "abdulmatin69" - }, - { - "percent": "10000", - "reputation": 63432644423328, - "rshares": 107771720865, - "voter": "helcim" - }, - { - "percent": "10000", - "reputation": 64787679421704, - "rshares": 25896262804, - "voter": "katiefreespirit" - }, - { - "percent": "2000", - "reputation": 773985194624, - "rshares": 541518098, - "voter": "kgswallet" - }, - { - "percent": "10000", - "reputation": 376755974186, - "rshares": 1047916771, - "voter": "voodooranger" - }, - { - "percent": "10000", - "reputation": 8242232748979, - "rshares": 1346104308, - "voter": "online-24-7" - }, - { - "percent": "2000", - "reputation": 635849426463, - "rshares": 31771835, - "voter": "fifay" - }, - { - "percent": "5000", - "reputation": 1161034162384, - "rshares": 969971721, - "voter": "joshuafootball" - }, - { - "percent": "2500", - "reputation": 1704173681552, - "rshares": 527890260, - "voter": "bilpcoinbot" - }, - { - "percent": "10000", - "reputation": 11383054385506, - "rshares": 6158303227, - "voter": "korver" - }, - { - "percent": "1250", - "reputation": 5118740660861, - "rshares": 1925578405, - "voter": "joshmansters" - }, - { - "percent": "2000", - "reputation": 17403163667146, - "rshares": 2384685491, - "voter": "leoneil" - }, - { - "percent": "3449", - "reputation": 3195367578140, - "rshares": 36461436772, - "voter": "joshmania" - }, - { - "percent": "1000", - "reputation": 0, - "rshares": 4302589758, - "voter": "bella.bear" - }, - { - "percent": "750", - "reputation": 0, - "rshares": 16389767, - "voter": "kryptoformator" - }, - { - "percent": "1500", - "reputation": 24720459476673, - "rshares": 5588189487, - "voter": "delilhavores" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1610366295, - "voter": "coin-doubler" - }, - { - "percent": "5000", - "reputation": 20853422627715, - "rshares": 641172335, - "voter": "im-ridd" - }, - { - "percent": "10000", - "reputation": 76222060731, - "rshares": 2406476465, - "voter": "wallytiteuf" - }, - { - "percent": "5000", - "reputation": 12710819754219, - "rshares": 616555742, - "voter": "disagio.gang" - }, - { - "percent": "2000", - "reputation": 2934995178664, - "rshares": 12174294032, - "voter": "vxn666" - }, - { - "percent": "5000", - "reputation": 9624495306704, - "rshares": 60894153981336, - "voter": "darthknight" - }, - { - "percent": "2500", - "reputation": 30837223420968, - "rshares": 3827635043, - "voter": "drew0" - }, - { - "percent": "10000", - "reputation": 13236845942735, - "rshares": 5463215185, - "voter": "lucky-guy" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 1722408495451, - "voter": "gleam-of-light" - }, - { - "percent": "10000", - "reputation": 1588836327, - "rshares": 0, - "voter": "wasifbhutto" - }, - { - "percent": "300", - "reputation": 0, - "rshares": 15878089482, - "voter": "fengchao" - }, - { - "percent": "200", - "reputation": 35668660233941, - "rshares": 5295388502, - "voter": "hivebuzz" - }, - { - "percent": "10000", - "reputation": 172540054299306, - "rshares": 1896397451935, - "voter": "pinmapple" - }, - { - "percent": "400", - "reputation": 91987050007999, - "rshares": 1370769335, - "voter": "hiveyoda" - }, - { - "percent": "2000", - "reputation": 5222879596789, - "rshares": 51452146651, - "voter": "hiveph" - }, - { - "percent": "297", - "reputation": 10364363981695, - "rshares": 173521130033, - "voter": "visionaer3003" - }, - { - "percent": "3900", - "reputation": 1209731647511, - "rshares": 638947537700, - "voter": "softworld" - }, - { - "percent": "1000", - "reputation": 16687677218, - "rshares": 11036290743, - "voter": "peterpanpan" - }, - { - "percent": "100", - "reputation": 2054630599034, - "rshares": 0, - "voter": "lhen18" - }, - { - "percent": "100", - "reputation": 1444444874297, - "rshares": 0, - "voter": "photodashph" - }, - { - "percent": "1000", - "reputation": 7317895795260, - "rshares": 536203596, - "voter": "mami.sheh7" - }, - { - "percent": "1000", - "reputation": 661148949025, - "rshares": 586580394, - "voter": "gradeon" - }, - { - "percent": "1000", - "reputation": 3177222978373, - "rshares": 9123498616, - "voter": "hivelist" - }, - { - "percent": "10000", - "reputation": 5226428326302, - "rshares": 4511451155, - "voter": "argenvista" - }, - { - "percent": "10000", - "reputation": 978489051717, - "rshares": 760826637, - "voter": "jesuscasalesson" - }, - { - "percent": "1000", - "reputation": 7034683328715, - "rshares": 848268136, - "voter": "iameden" - }, - { - "percent": "10000", - "reputation": 429763208250, - "rshares": 4554446545, - "voter": "ghaazi" - }, - { - "percent": "10000", - "reputation": 54824847159245, - "rshares": 99875619925, - "voter": "discoveringarni" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 75396260748, - "voter": "executive-board" - }, - { - "percent": "10000", - "reputation": 19772353248382, - "rshares": 36079696657, - "voter": "iamyohann" - }, - { - "percent": "7500", - "reputation": 11943708395924, - "rshares": 9437910925, - "voter": "millycf1976" - }, - { - "percent": "10000", - "reputation": 18792089037144, - "rshares": 21483327796, - "voter": "paulajogalix" - }, - { - "percent": "5000", - "reputation": 11320226922294, - "rshares": 1870504877826, - "voter": "hiro-hive" - }, - { - "percent": "7500", - "reputation": 17939114076906, - "rshares": 15993540832, - "voter": "viviana28" - }, - { - "percent": "291", - "reputation": 4358352640716, - "rshares": 70482830461, - "voter": "hatschi0773" - }, - { - "percent": "1000", - "reputation": 0, - "rshares": 60401209657, - "voter": "hivecur2" - }, - { - "percent": "5000", - "reputation": 7273089567, - "rshares": 648795828, - "voter": "hivecoffee" - }, - { - "percent": "5000", - "reputation": 7775140788254, - "rshares": 1680574321, - "voter": "jearo101" - }, - { - "percent": "400", - "reputation": 25284728169845, - "rshares": 111423610360, - "voter": "recoveryinc" - }, - { - "percent": "5000", - "reputation": 35280907862981, - "rshares": 11041760369, - "voter": "hive-data" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 7419191173, - "voter": "gohive" - }, - { - "percent": "10000", - "reputation": 10541935857015, - "rshares": 21308841915, - "voter": "storiesoferne" - }, - { - "percent": "800", - "reputation": 0, - "rshares": 8008005042, - "voter": "dying" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 21228948616, - "voter": "vtol79" - }, - { - "percent": "10000", - "reputation": 14231734699696, - "rshares": 44828553039, - "voter": "whoaretheyph" - }, - { - "percent": "294", - "reputation": 3174507364780, - "rshares": 101785117790, - "voter": "dagobert007" - }, - { - "percent": "1000", - "reputation": 9930293019864, - "rshares": 729604570, - "voter": "jonalyn2020" - }, - { - "percent": "7520", - "reputation": 16307549034965, - "rshares": 26672929017, - "voter": "trangbaby" - }, - { - "percent": "300", - "reputation": 0, - "rshares": 35474744485, - "voter": "intacto" - }, - { - "percent": "300", - "reputation": 434627240, - "rshares": 4581115938, - "voter": "boneym" - }, - { - "percent": "10000", - "reputation": 654061210157, - "rshares": 480796917, - "voter": "vaishnavi24" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "lalakhes" - }, - { - "percent": "10000", - "reputation": 0, - "rshares": 0, - "voter": "baidyabulu" - } - ], - "author": "ybanezkim26", - "author_reputation": 136276266931207, - "beneficiaries": [], - "body": "<center>https://files.peakd.com/file/peakd-hive/ybanezkim26/fQpqfkra-IMG_20201101_121245.jpg</center>\n\n<div class=\"text-justify\">\nHello Hive!\n</div>\n<br>\n<div class=\"text-justify\">\nIt's time for another entry under my series <b>Philippine Islands</b>. Last entry under this series was all about Cebu and Visayas Islands as a celebratory post when it has been awarded as the <b><a href=\"https://www.cntraveler.com/galleries/2014-10-20/top-30-islands-in-the-world-readers-choice-awards-2014\">Best in Asia by Conde Nast Traveler's The Best Islands in the World: 2020 Readers' Choice Awards</a></b>. To find more about why the recognition is well deserved, you can check out my compilation post in the link below:\n</div>\n\n-----\n\n<div class=\"pull-left\">\n\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/vskTNnnI-IMG_20201008_112306.jpg\n</div>\n<div class=\"pull-right\">\n<center><sub><b><a href=\"https://peakd.com/hive-163772/@ybanezkim26/philippine-islands-5-cebu-and-visayas-islands-conde-nast-traveler-s-best-in-asia-the-best-islands-in-the-world-2020-readers\">Philippine Islands #5: Cebu and Visayas Islands - Conde Nast Traveler's Best in Asia [The Best Islands in the World: 2020 Readers' Choice Awards]</a></b></sub></center>\n</div>\n\n-----\n\n<div class=\"text-justify\">\nThis time, I have two islands to feature. These islands are part of my Camiguin Island tour which is still dragging along even if it was already more than a month since I started this. Anyway, you can find the first two parts of this series in the links below:\n</div>\n\n-----\n\n<div class=\"pull-left\">\n\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/lOubS8XJ-GOPR3565_1544243864148_high.jpg\n</div>\n<div class=\"pull-right\">\n<center><sub><b><a href=\"https://peakd.com/hive-163772/@ybanezkim26/kim-s-quest-45-climbing-mt-hibok-hibok-in-camiguin-island\">Kim's Quest #45: Climbing Mt. Hibok-Hibok in Camiguin Island</a></b></sub></center>\n</div>\n\n-----\n\n<div class=\"pull-left\">\n\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/GmSEdGKG-IMG_20201024_003342.jpg\n</div>\n<div class=\"pull-right\">\n<center><sub><b><a href=\"https://peakd.com/hive-163772/@ybanezkim26/kim-s-quest-46-the-sunken-cemetery-of-camiguin-island\">Kim's Quest #46: The Sunken Cemetery of Camiguin Island</a></b></sub></center>\n</div>\n\n-----\n\n<div class=\"text-justify\">\nAlright! Let's get it on.\n</div>\n\n-----\n\n<center><b>MANTIGUE ISLAND & WHITE ISLAND</b></center><center><sub><b>Camiguin's Pride</b></sub></center>\n\n-----\n\n<div class=\"text-justify\">\nBoth islands are off the coast of the volcanic island province of Camiguin. The first island is larger than the second one, but both have gleaming white sand beaches. I'll start with Mantigue Island.\n</div>\n<br>\n\n<h4>Mantigue Island</h4>\n\n<center>https://files.peakd.com/file/peakd-hive/ybanezkim26/nUWlJnGI-IMG_20201101_121324.jpg</center>\n\n<div class=\"text-justify\">\nMantigue is just 3.5 kilometers off the coast of the municipality of Mahinog in Camiguin. It's one of Camiguin's top tourist spots because of its white sand beach and crystal clear waters that's surrounding the island while a deep drop-off that's good for scuba diving is located on one side of the island.\n</div>\n\n<center>https://files.peakd.com/file/peakd-hive/ybanezkim26/MfMZWbmE-IMG_20201101_121905.jpg</center>\n\n<div class=\"pull-left\">\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/4Afxvnl0-IMG20181208140033.jpg\n</div>\n\n<div class=\"text-justify\">\nAfter that gruelling climb to Mt. Hibok-Hibok, we immediately went to San Roque Port in Mahinog and then rode a boat to Mantigue Island. It would take about 20 minutes boat ride and then you'll be welcomed by that gleaming white sand beach, a lush nature park, and the crystal clear waters surrounding the whole island.\n</div>\n\n<center>https://files.peakd.com/file/peakd-hive/ybanezkim26/VNTVoqiP-IMG_20201101_121527.jpg</center>\n\n<div class=\"pull-left\">\n\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/BUb3tzu4-IMG_20201101_121712.jpg\n</div>\n<div class=\"pull-right\">\n\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/DTsclLGU-IMG_20201101_121209.jpg\n</div>\n\n-----\n\n<div class=\"pull-right\">\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/CwO2vL13-IMG20181208141809.jpg\n</div>\n\n<div class=\"text-justify\">\nI got really tired with the climb that I didn't do much on the island which was one of my many regrets after visiting Camiguin. We just rented one cottage and I just stayed there most of the time while my friends were snorkelling and swimming to their heart's content.\n</div>\n\n<center>https://files.peakd.com/file/peakd-hive/ybanezkim26/eVXDjOpi-IMG_20201101_121954.jpg</center>\n\n<div class=\"pull-left\">\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/ik8iU3G3-IMG20181208163545.jpg\n</div>\n\n<div class=\"text-justify\">\nIf given an opportunity to visit Camiguin again, I will definitely try all the activities in Mantigue. From what I learned, you can stroll around the island in just 30 minutes. Coral reefs are also abundant in there so scuba diving will be a great activity. I missed the nature walk that would take you from one end of the island to the other end.\n</div>\n<br>\n<div class=\"text-justify\">\n<b>Map Coordinates:</b> [//]:# (!pinmapple 9.172214 lat 124.824224 long d3scr)\n</div>\n<br>\n\n<h4>White Island</h4>\n\n<center>https://files.peakd.com/file/peakd-hive/ybanezkim26/ZpbGSGJZ-IMG_20201101_205659.jpg</center><center><sub>I took this photo while on the plane to Camiguin Island and that white sandbar is one of Camiguin's top tourist destination: the instagrammable <b>White Island</b>.</sub></center>\n\n<div class=\"text-justify\">\nWhite Island is a horseshoe-shaped sandbar that's 1.4 kilometers off the shore of Mambajao. From our rented apartment, we just walk a few meters to where the boats were that will bring us to the sandbar. There's no plant nor permanent structure. It's just pure white sand that's constantly reshaping and resizing due to the tides. However, the water surrounding the island is so pristine.\n</div>\n\n<center>https://files.peakd.com/file/peakd-hive/ybanezkim26/pmEaSwDC-IMG_20201101_121733.jpg</center>\n\n<div class=\"text-justify\">\nThe sandbar provides a panoramic view of the whole Camiguin Island. It has also a very good view of Mt. Hibok-Hibok that's usually shrouded by clouds especially early in the morning.\n</div>\n\n<center>https://files.peakd.com/file/peakd-hive/ybanezkim26/U3CikHVy-IMG_20201101_122012.jpg</center>\n\n<div class=\"pull-left\">\n\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/HusomgyR-IMG_20201101_121928.jpg\n</div>\n<div class=\"pull-right\">\n\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/jV8Qnr5x-IMG_20201101_122031.jpg\n</div>\n\n-----\n\n<div class=\"text-justify\">\nWe went there on our last day in Camiguin and it was around 6:00 AM, but there are already a lot of tourists in the sandbar. Maybe because there's no structure that could provide shade, staying in the sandbar until noon is not really a good idea unless you wanted to have a good tan. Snorkelling is also a great activity there.\n</div>\n<center>https://files.peakd.com/file/peakd-hive/ybanezkim26/lvJ52q0G-IMG_20201101_121614.jpg</center>\n\n<div class=\"pull-left\">\n\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/2w2NrWKE-IMG_20201101_121547.jpg\n</div>\n<div class=\"pull-right\">\n\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/hgqGoWGL-IMG_20201101_121444.jpg\n</div>\n\n-----\n\n<div class=\"text-justify\">\nIt's just sad that my photos are not that great. I will definitely go back there someday and take as many photos as I want. I just find it really strange to have a white sandbar on a volcanic island. The sandbar is a very stark contrast to the black sand across Mambajao.\n</div>\n<br>\n<div class=\"text-justify\">\n<b>Map Coordinates:</b> [//]:# (!pinmapple 9.259443 lat 124.655139 long d3scr)\n</div>\n\n<center>https://files.peakd.com/file/peakd-hive/ybanezkim26/zOH6P2s7-IMG_20201101_122131.jpg</center>\n\n<div class=\"text-justify\">\nI guess that's all for this showcase of <b>Philippine Islands</b>. I hope you'll be able to visit one of these islands and also share your experiences. As for the last post under my Camiguin series, I will share more of the places we visited especially the springs. I don't have photos of those springs though. It's because we visited them at night. Our schedule was too packed at that time.\n</div>\n<br>\n<div class=\"text-justify\">\nSee you in my next post!\n</div>\n\n-----\n\n<div class=\"pull-left\">\nhttps://files.peakd.com/file/peakd-hive/ybanezkim26/6Qa4aTfH-KIM20bubble.png\n</div>\n\n<div class=\"text-justify\">\n<b><a href=\"https://peakd.com/@ybanezkim26/posts\">Kim Yba\u00f1ez</a></b>\n\n<sub>Welcome to Kim's small corner in Hive. He is a chemical engineer by profession, but a blogger by passion. He is a wanderlust and an adventure seeker. Join his quests as he visits secluded destinations, climbs mountains, tries new and exotic dishes, and explores his country (The Philippines) and the rest of the world even if he's still a poor corporate slave with tons of bills to pay and two siblings to support in college.</sub>\n\n<sub>If you like his content, don't forget to upvote and leave a comment to show some love. You can also reblog if you want to. Also, don't forget to follow him to be updated with his latest posts.\n</sub>\n</div>", - "body_length": 9236, - "cashout_time": "2020-11-08T16:50:39", - "category": "hive-163772", - "children": 27, - "created": "2020-11-01T16:50:39", - "curator_payout_value": "0.000 HBD", - "depth": 0, - "json_metadata": "{\"app\":\"peakd/2020.10.9\",\"format\":\"markdown\",\"tags\":[\"travel\",\"islands\",\"philippines\",\"tropical\",\"paradise\",\"white\",\"sand\",\"beaches\"],\"users\":[\"ybanezkim26\"],\"links\":[\"https://www.cntraveler.com/galleries/2014-10-20/top-30-islands-in-the-world-readers-choice-awards-2014\",\"/hive-163772/@ybanezkim26/philippine-islands-5-cebu-and-visayas-islands-conde-nast-traveler-s-best-in-asia-the-best-islands-in-the-world-2020-readers\",\"/hive-163772/@ybanezkim26/kim-s-quest-45-climbing-mt-hibok-hibok-in-camiguin-island\",\"/hive-163772/@ybanezkim26/kim-s-quest-46-the-sunken-cemetery-of-camiguin-island\",\"/@ybanezkim26/posts\"],\"image\":[\"https://files.peakd.com/file/peakd-hive/ybanezkim26/fQpqfkra-IMG_20201101_121245.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/vskTNnnI-IMG_20201008_112306.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/lOubS8XJ-GOPR3565_1544243864148_high.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/GmSEdGKG-IMG_20201024_003342.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/nUWlJnGI-IMG_20201101_121324.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/MfMZWbmE-IMG_20201101_121905.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/4Afxvnl0-IMG20181208140033.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/VNTVoqiP-IMG_20201101_121527.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/BUb3tzu4-IMG_20201101_121712.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/DTsclLGU-IMG_20201101_121209.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/CwO2vL13-IMG20181208141809.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/eVXDjOpi-IMG_20201101_121954.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/ik8iU3G3-IMG20181208163545.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/ZpbGSGJZ-IMG_20201101_205659.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/pmEaSwDC-IMG_20201101_121733.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/U3CikHVy-IMG_20201101_122012.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/HusomgyR-IMG_20201101_121928.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/jV8Qnr5x-IMG_20201101_122031.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/lvJ52q0G-IMG_20201101_121614.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/2w2NrWKE-IMG_20201101_121547.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/hgqGoWGL-IMG_20201101_121444.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/zOH6P2s7-IMG_20201101_122131.jpg\",\"https://files.peakd.com/file/peakd-hive/ybanezkim26/6Qa4aTfH-KIM20bubble.png\"]}", - "last_payout": "1969-12-31T23:59:59", - "last_update": "2020-11-01T16:50:39", - "max_accepted_payout": "1000000.000 HBD", - "net_rshares": 201638335241889, - "parent_author": "", - "parent_permlink": "hive-163772", - "pending_payout_value": "47.004 HBD", - "percent_hbd": 10000, - "permlink": "philippine-islands-6-mantigue-island-and-white-island-of-camiguin", - "post_id": 100346331, - "promoted": "0.000 HBD", - "replies": [], - "root_title": "Philippine Islands #6: Mantigue Island and White Island of Camiguin", - "title": "Philippine Islands #6: Mantigue Island and White Island of Camiguin", - "total_payout_value": "0.000 HBD", - "url": "/hive-163772/@ybanezkim26/philippine-islands-6-mantigue-island-and-white-island-of-camiguin" - }, - { - "active_votes": [ - { - "percent": "1500", - "reputation": 19245698180508, - "rshares": 322972779022, - "voter": "tombstone" - }, - { - "percent": "10000", - "reputation": 68993171140258, - "rshares": 63519848948, - "voter": "simba" - }, - { - "percent": "4000", - "reputation": 341233778618, - "rshares": 626880618061, - "voter": "boatymcboatface" - }, - { - "percent": "5000", - "reputation": 351157717865710, - "rshares": 944070366893, - "voter": "onealfa" - }, - { - "percent": "4000", - "reputation": 1957220358850383, - "rshares": 1546238578768, - "voter": "kingscrown" - }, - { - "percent": "2500", - "reputation": 506372806974428, - "rshares": 2068436233987, - "voter": "kevinwong" - }, - { - "percent": "4000", - "reputation": 4509451541223, - "rshares": 168307407532, - "voter": "theshell" - }, - { - "percent": "10000", - "reputation": 97717041, - "rshares": 20842325770, - "voter": "field" - }, - { - "perce